Introduction to GLSL Patrick Cozzi University of Pennsylvania
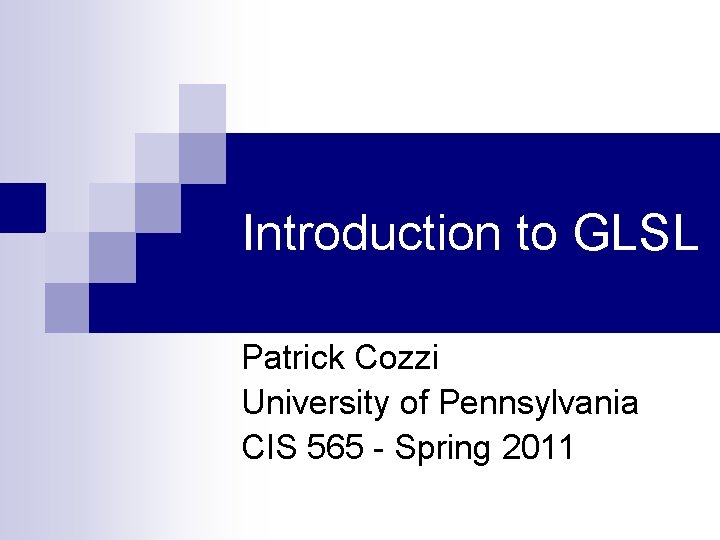
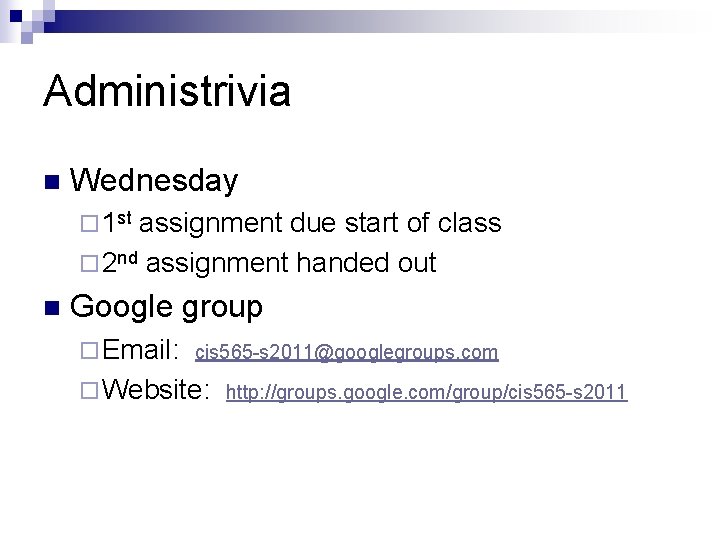
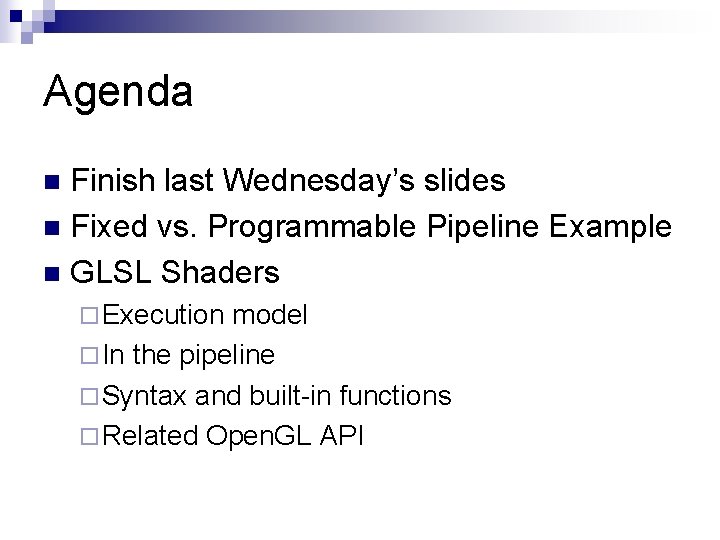
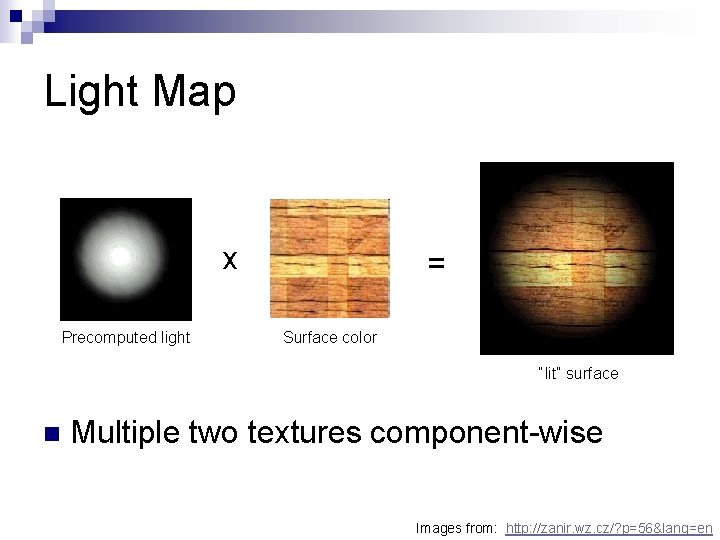
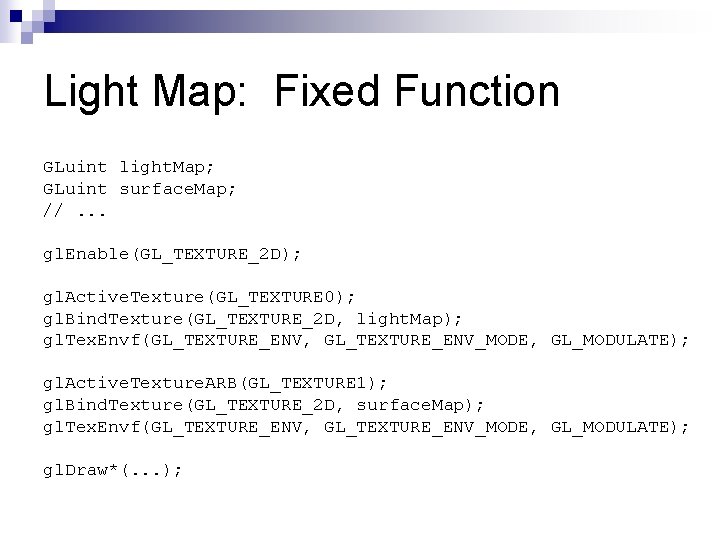
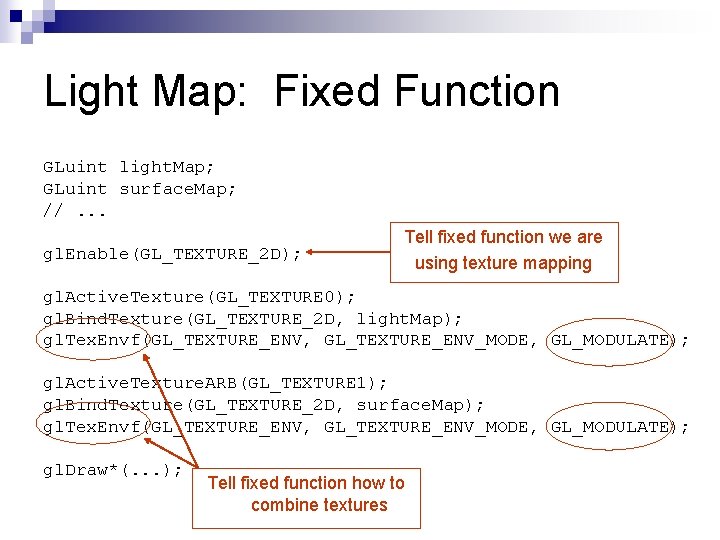
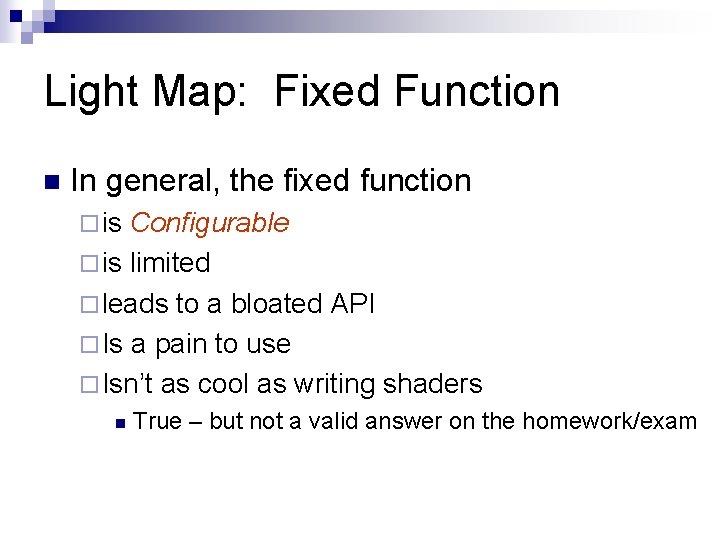
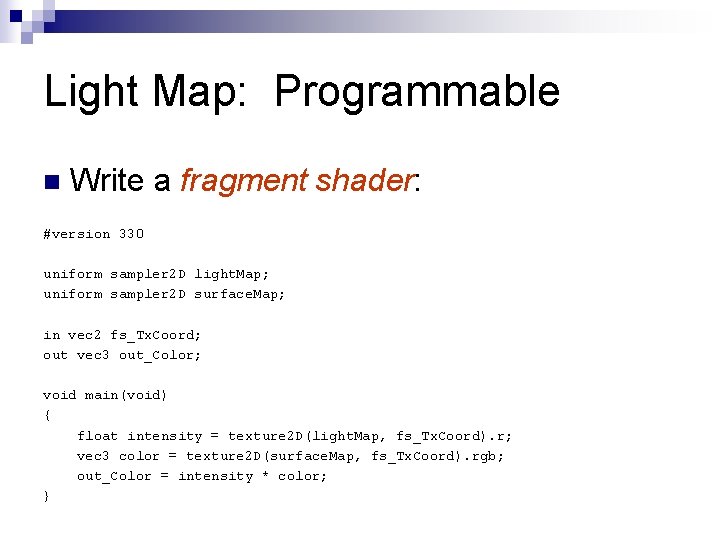
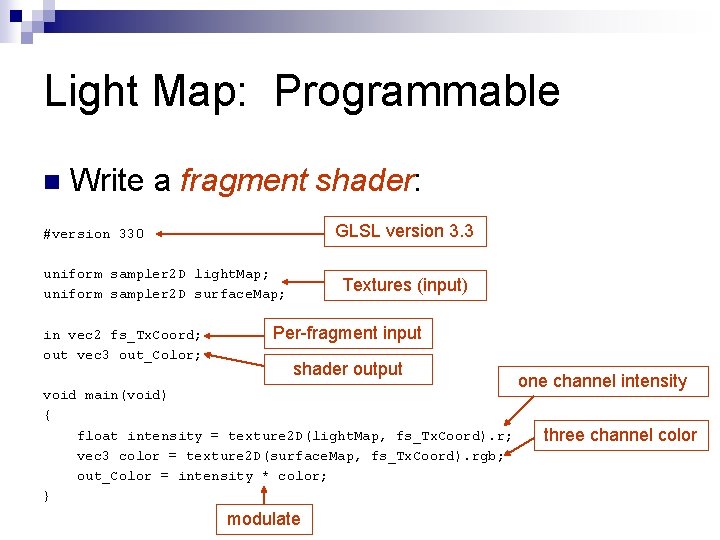
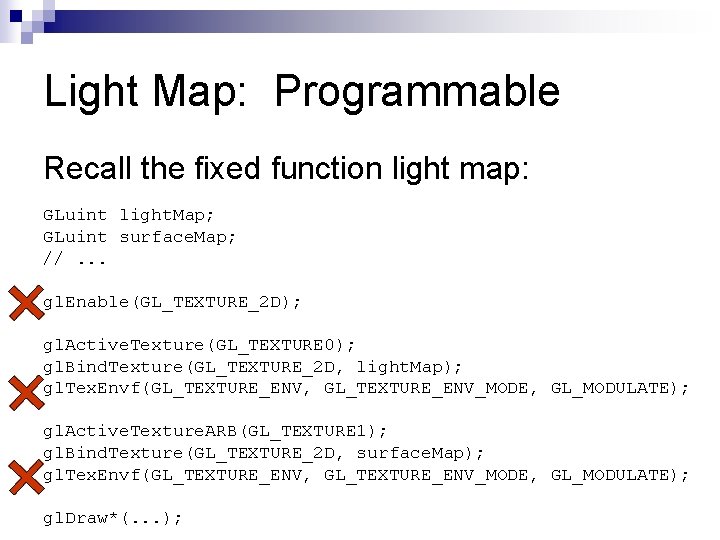
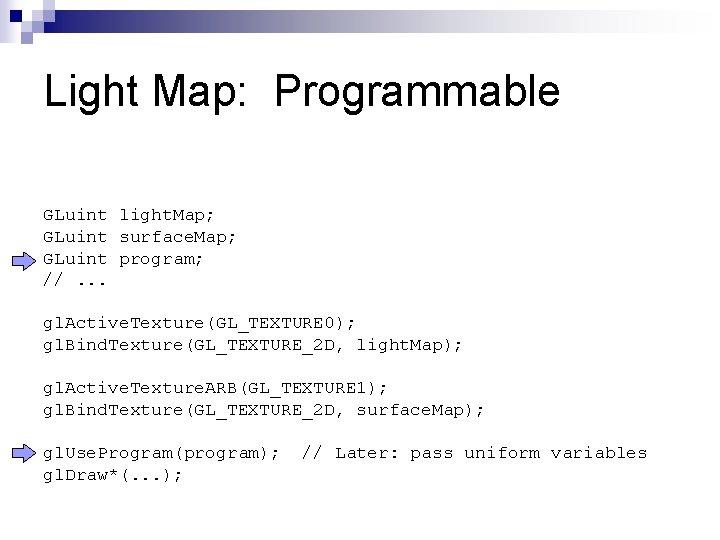
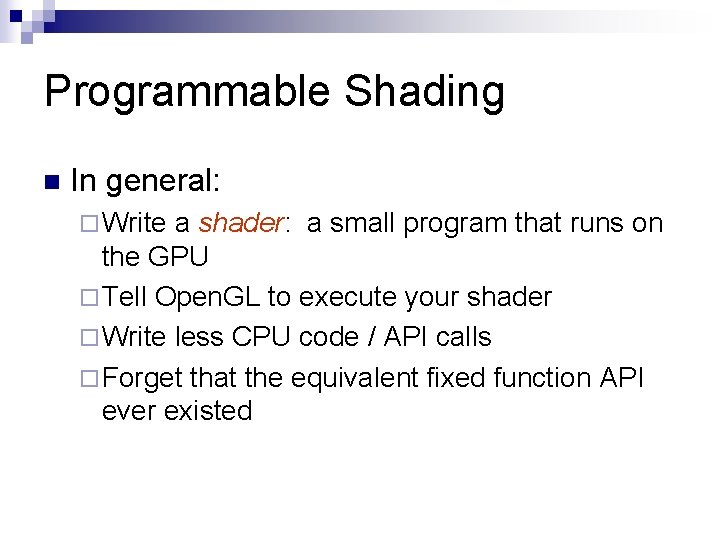
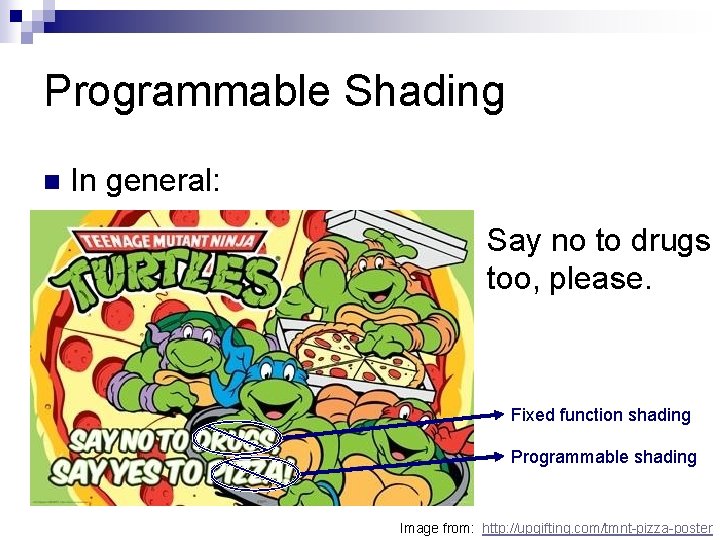
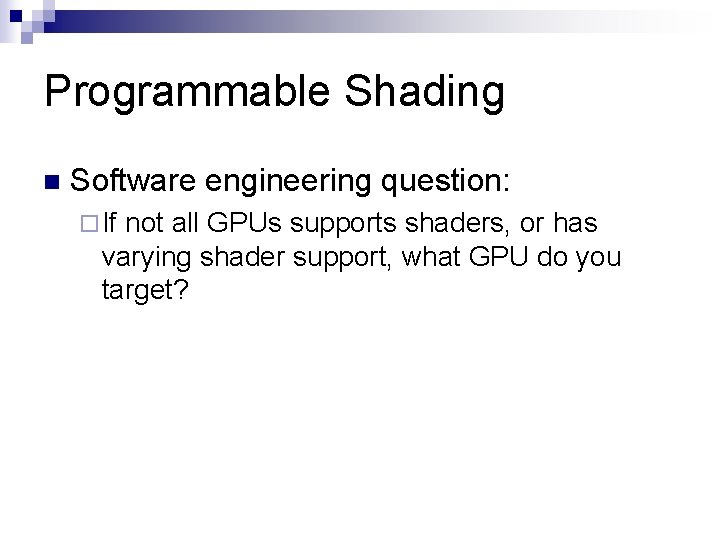
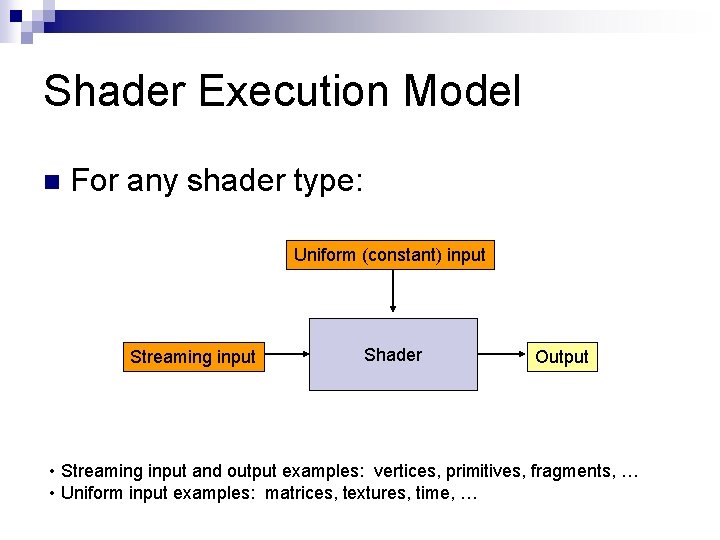
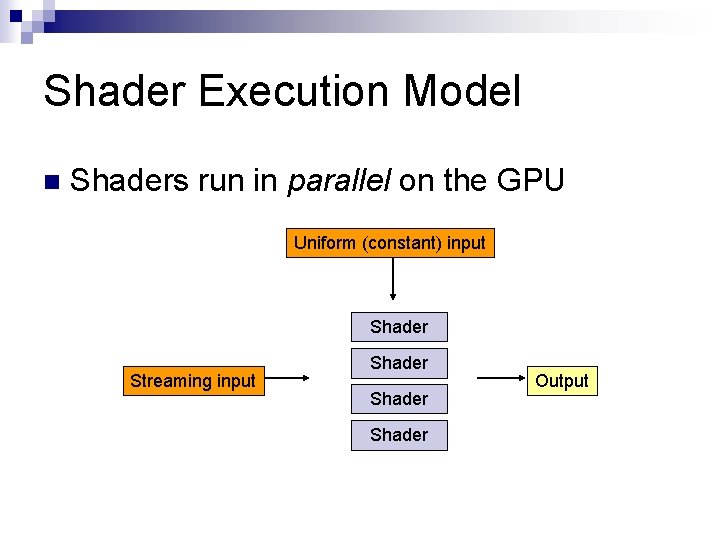
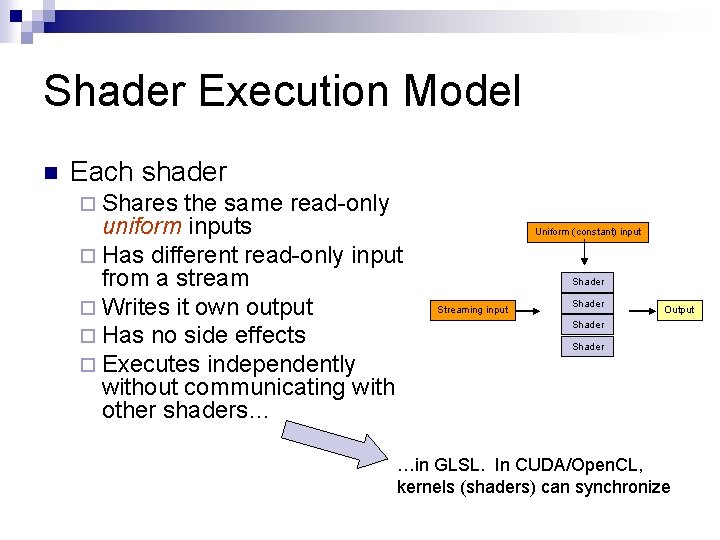
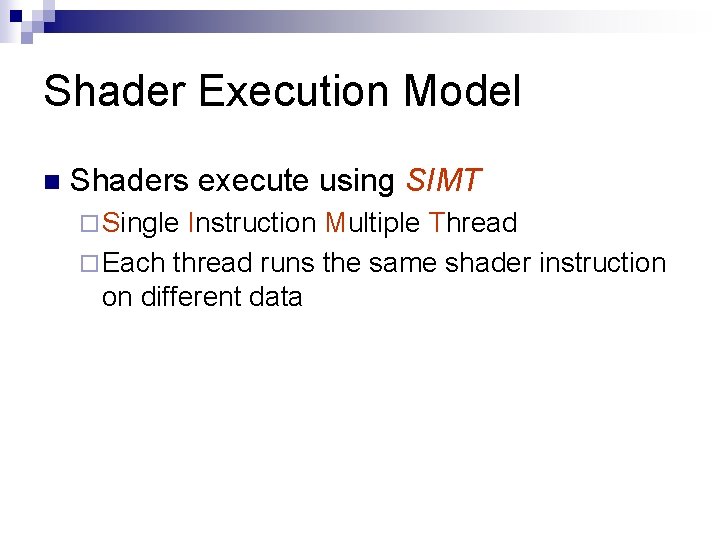
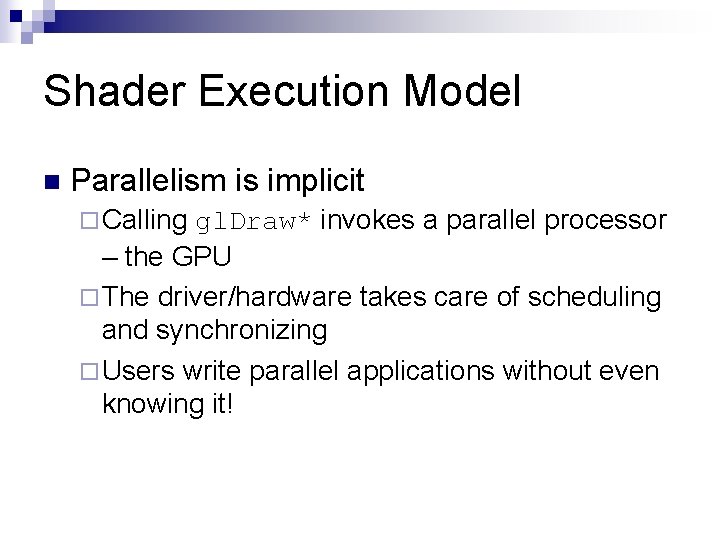
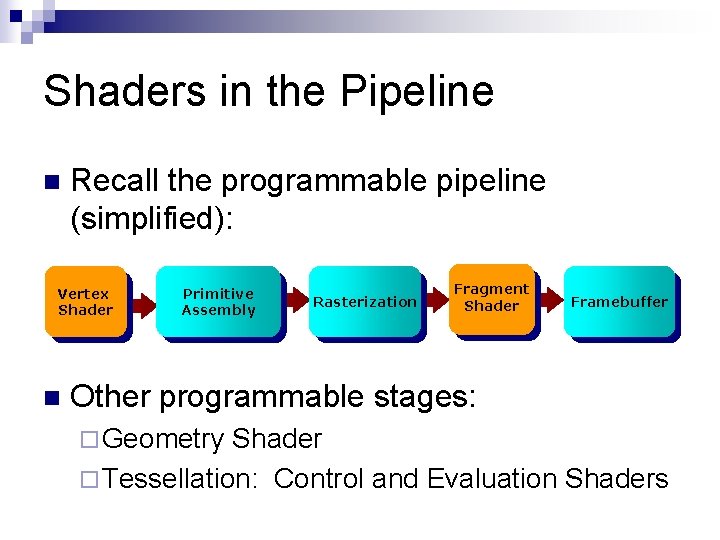
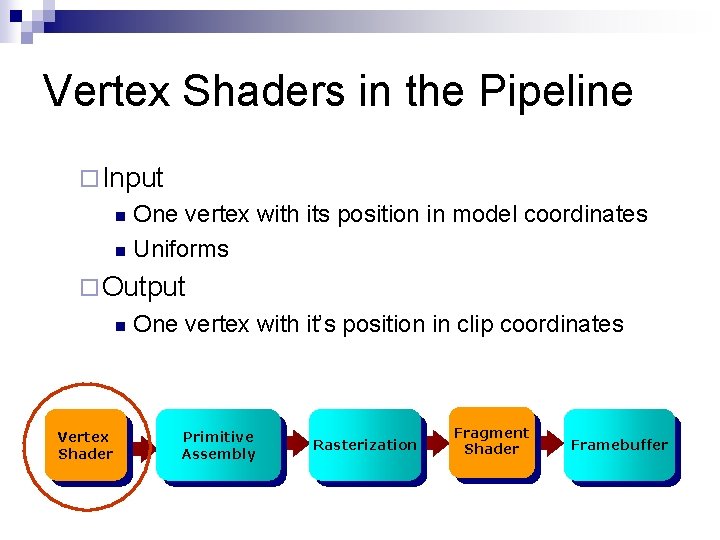
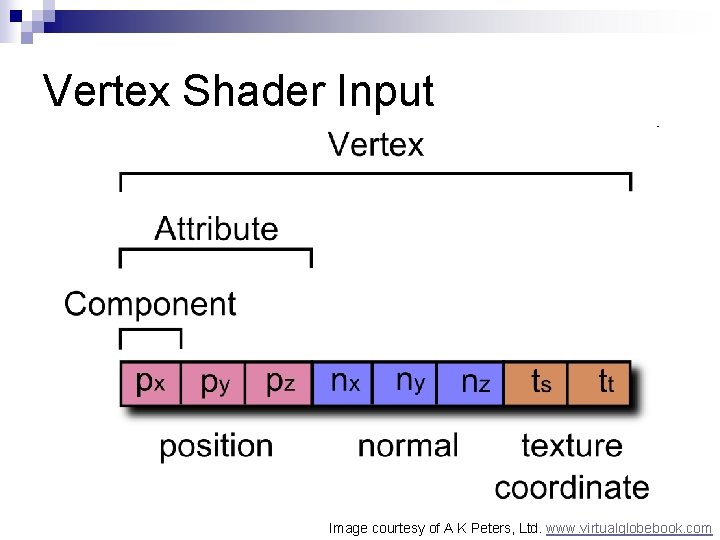
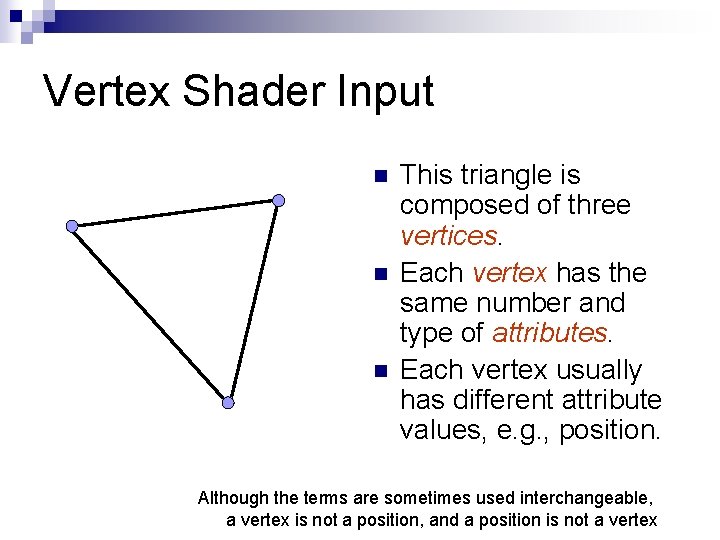
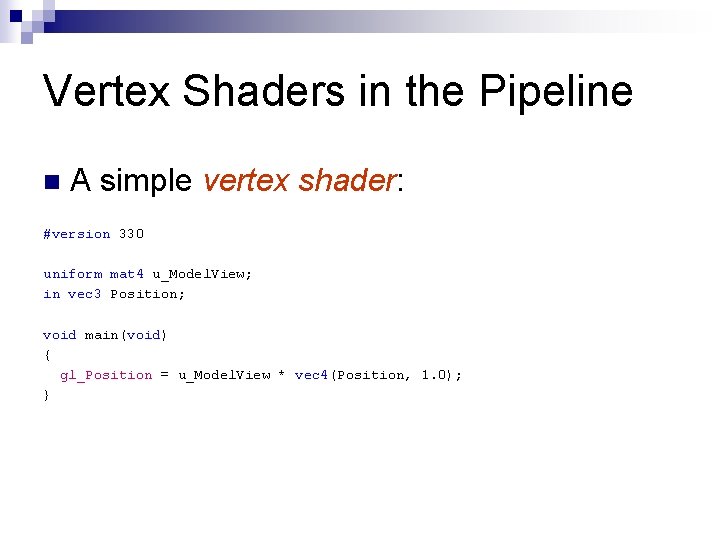
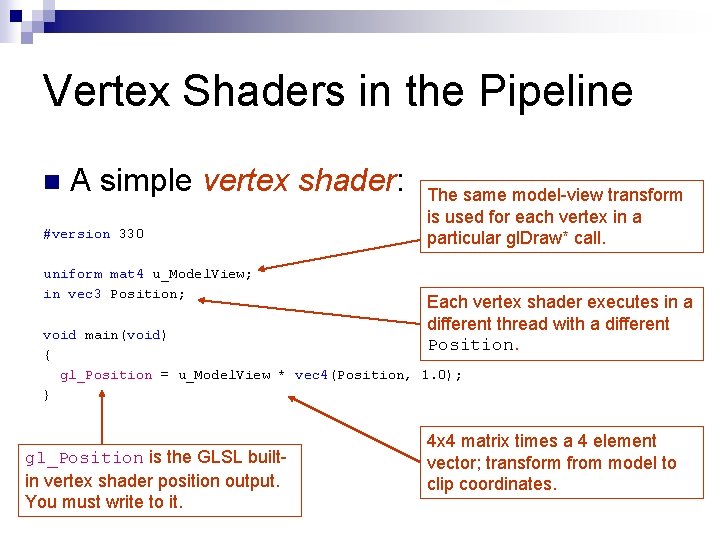
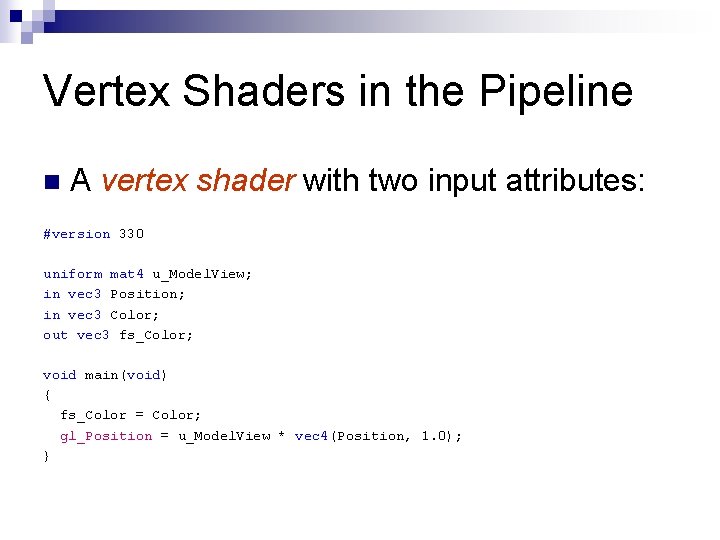
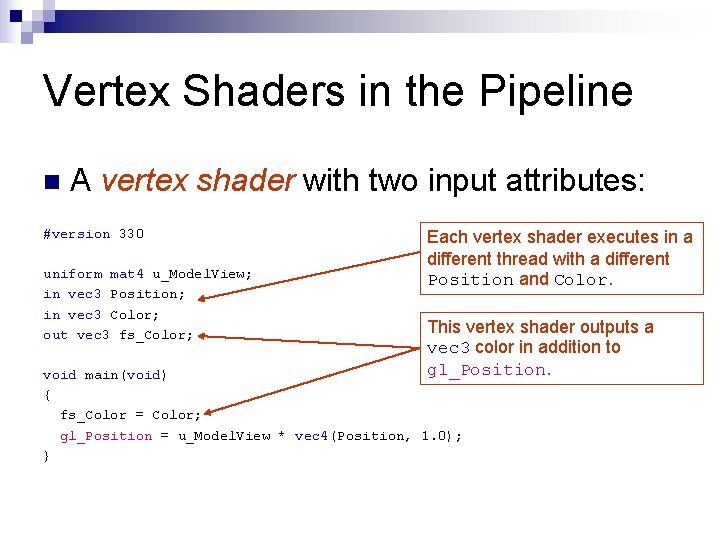
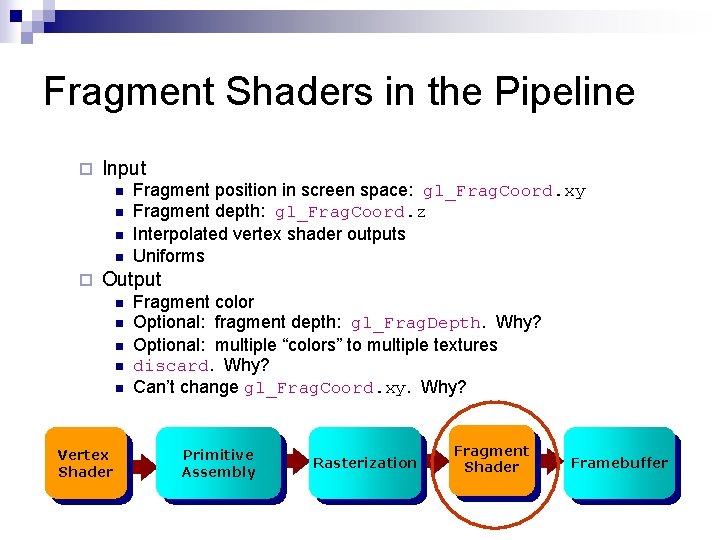
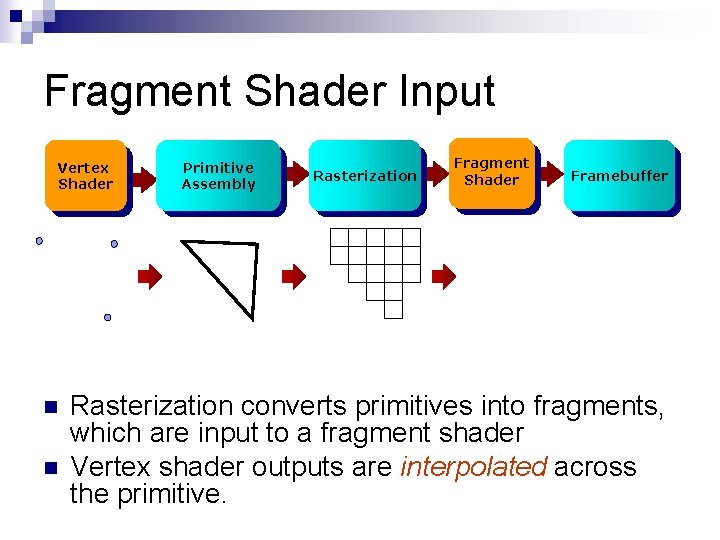
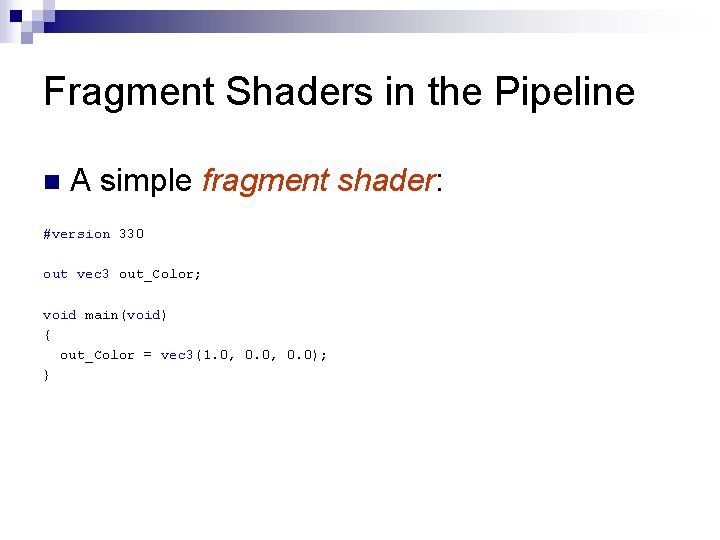
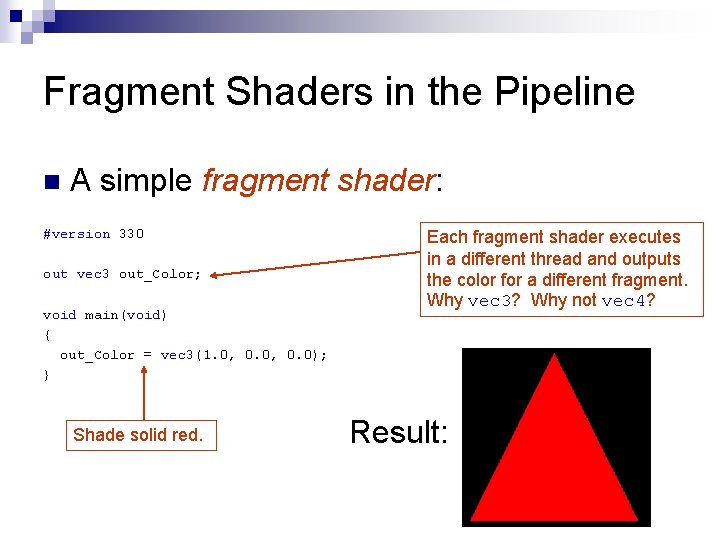
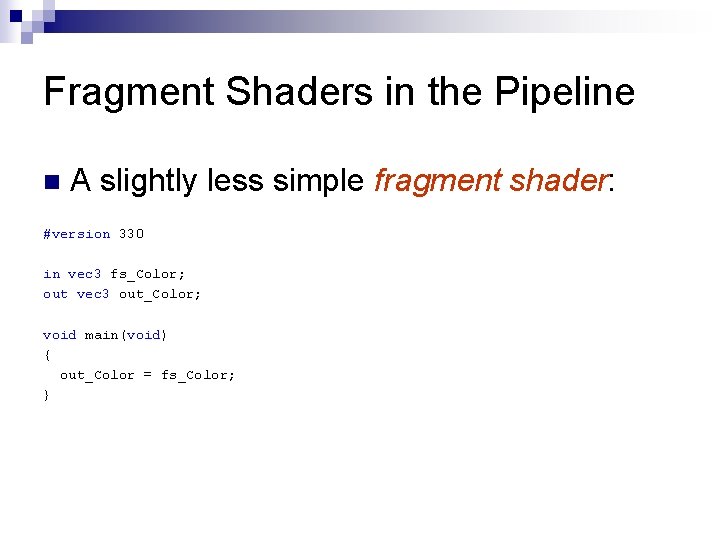
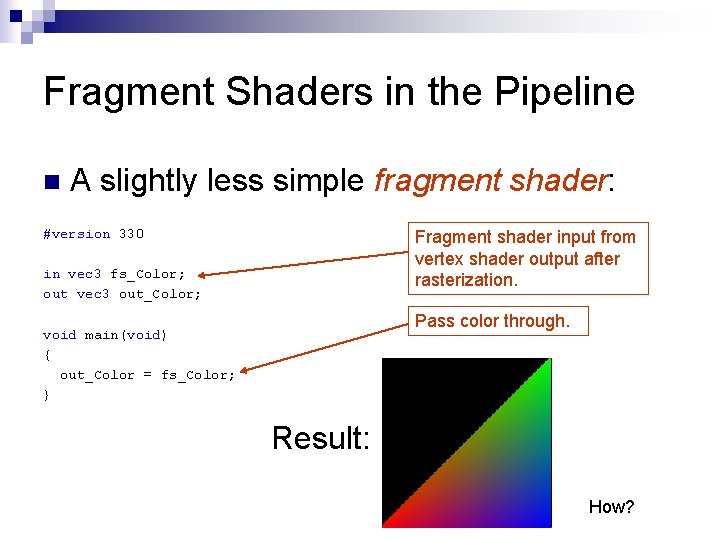
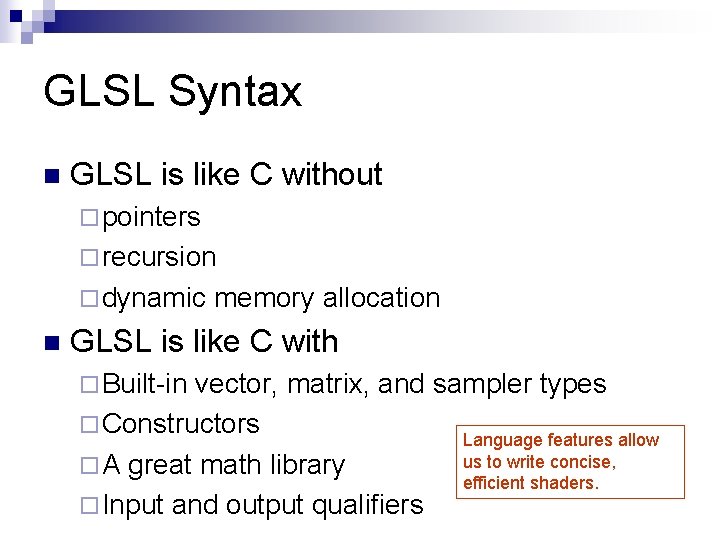
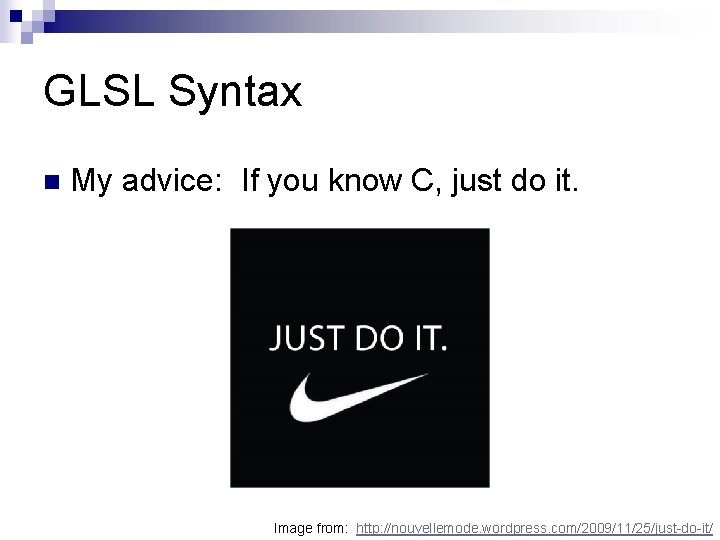
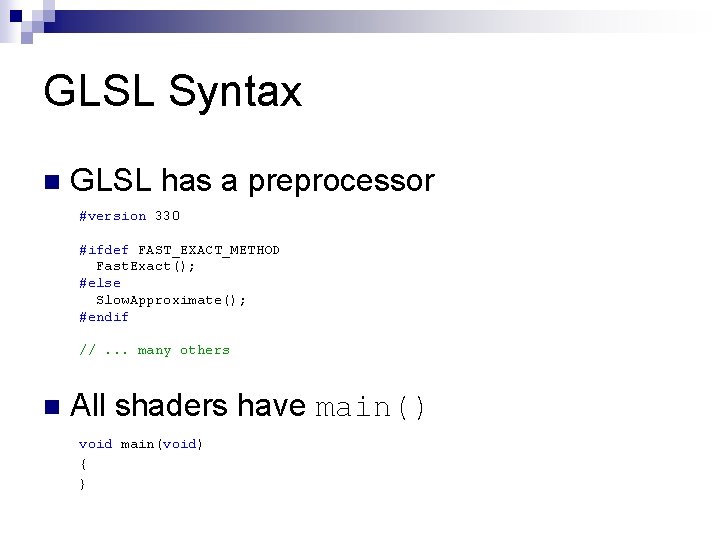
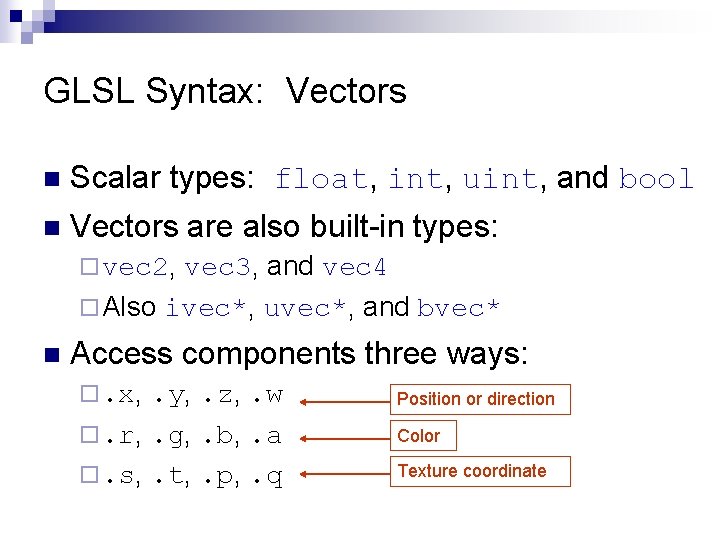
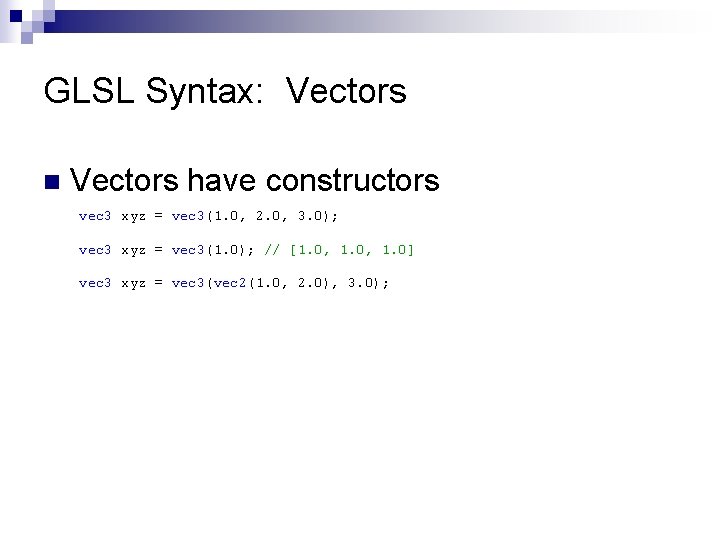
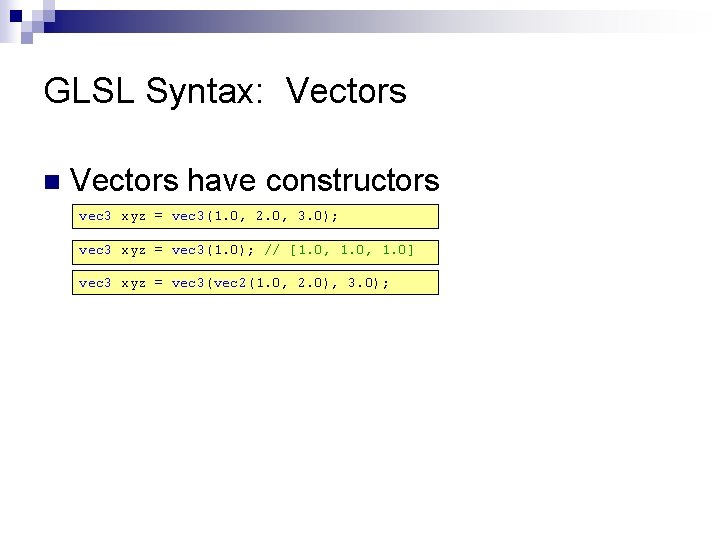
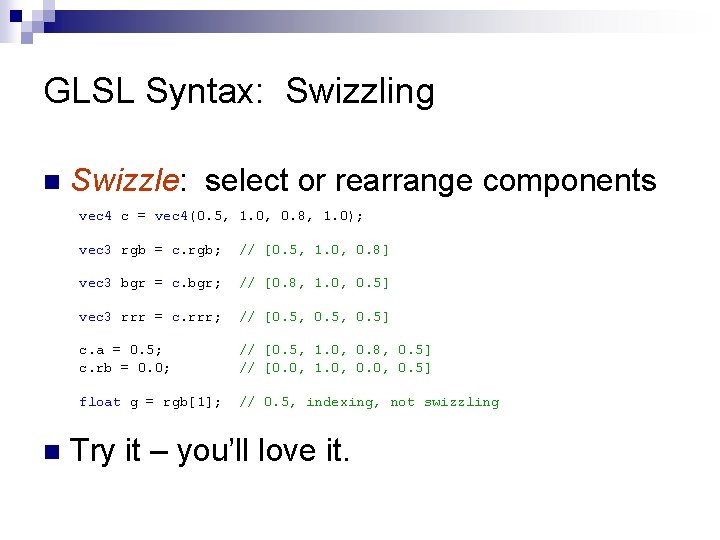
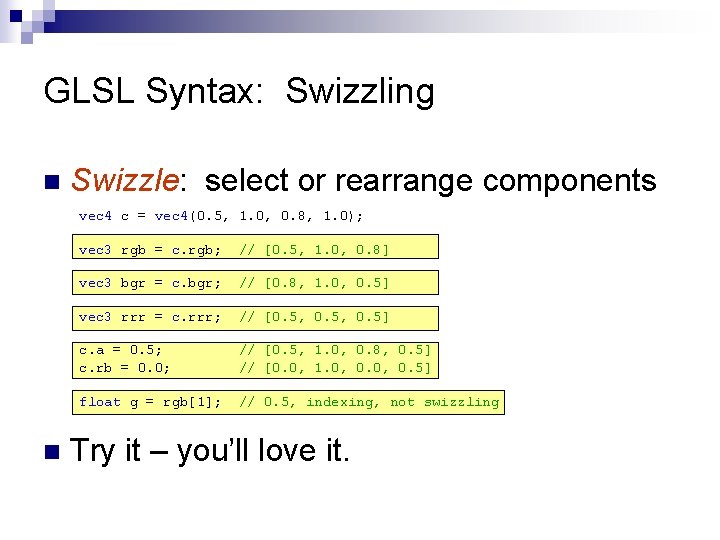
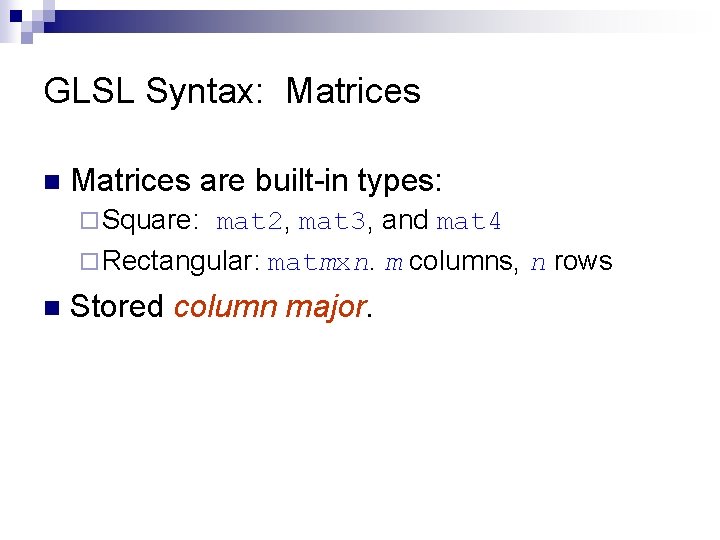
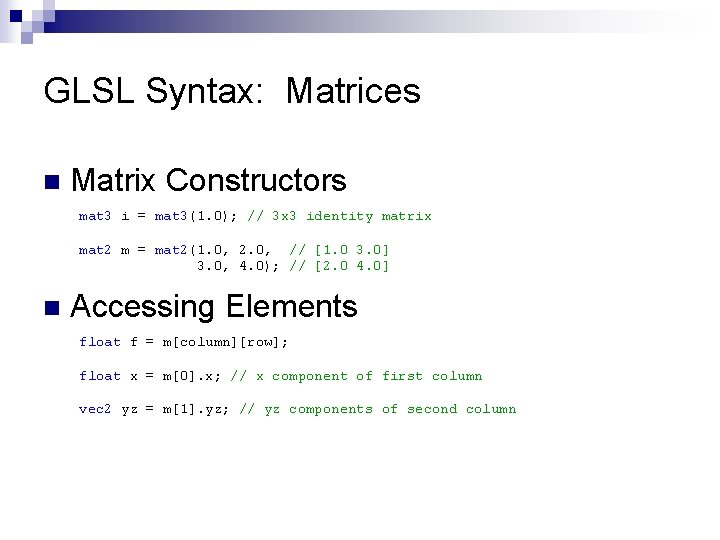
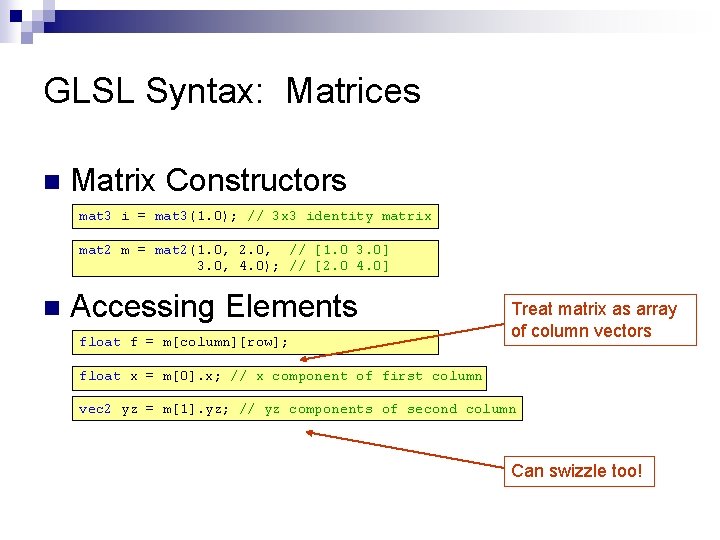
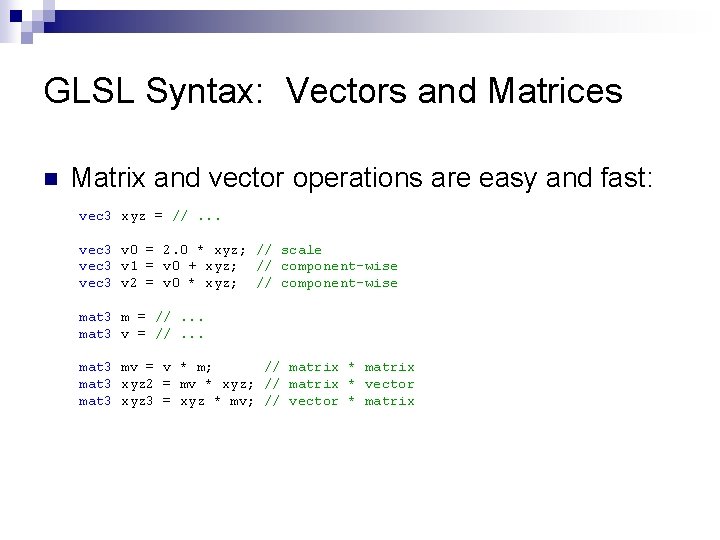
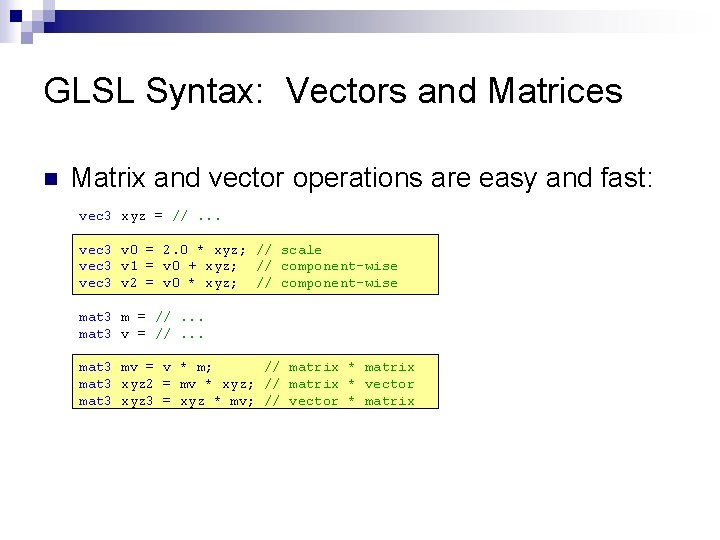
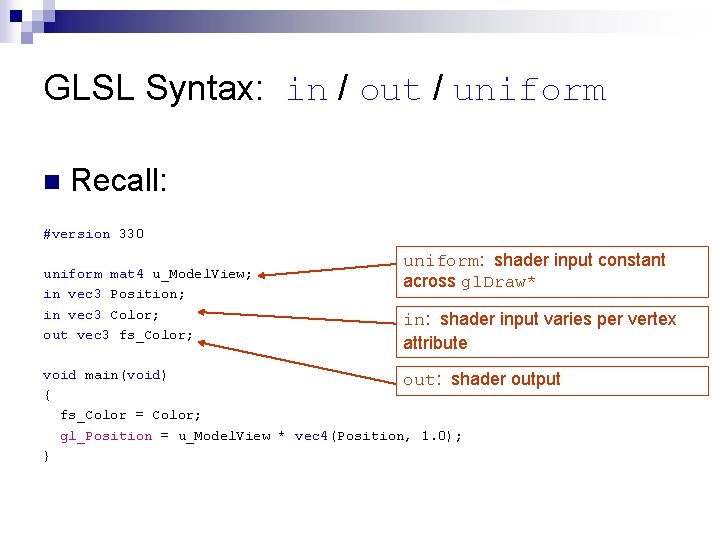
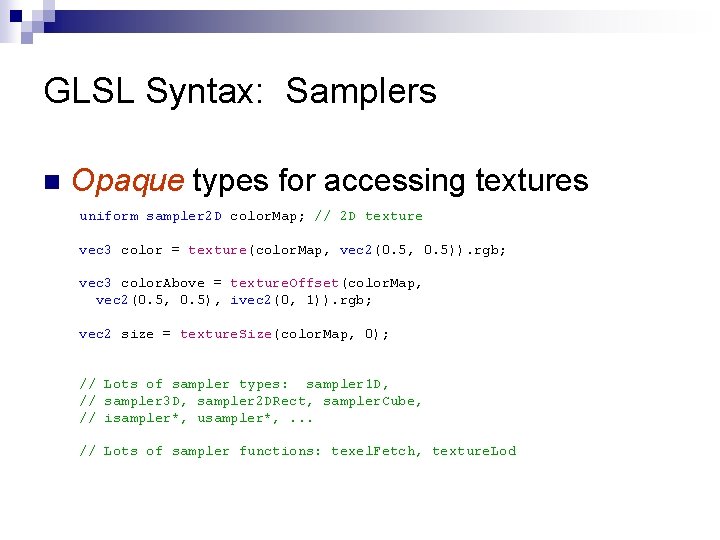
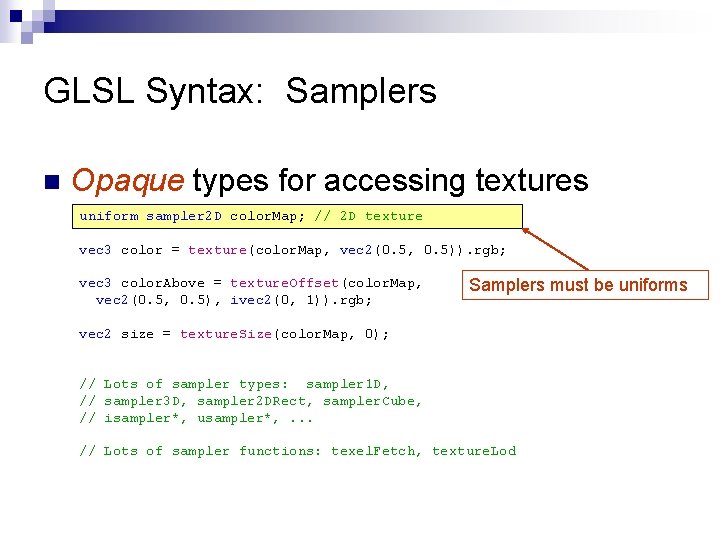
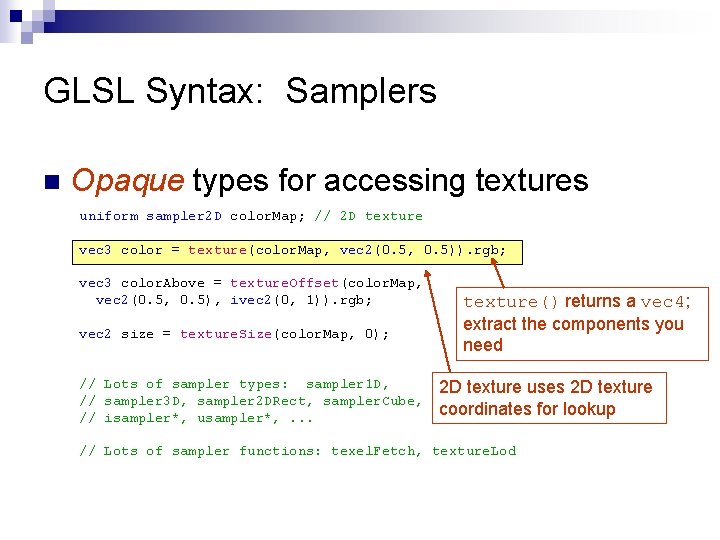
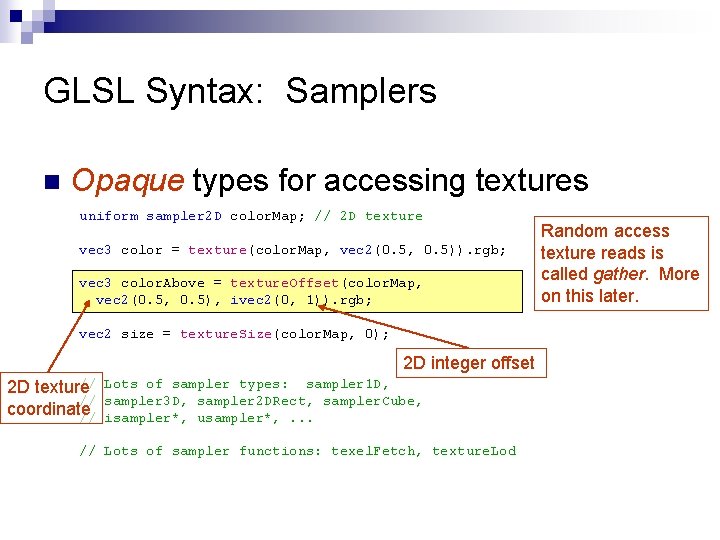
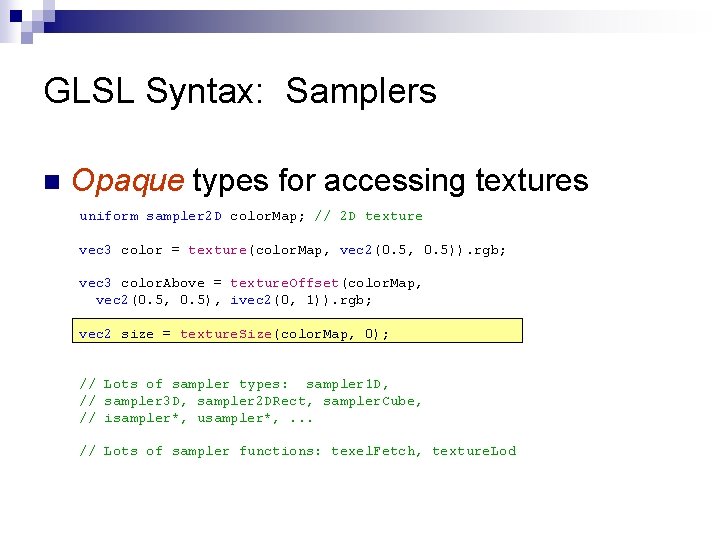
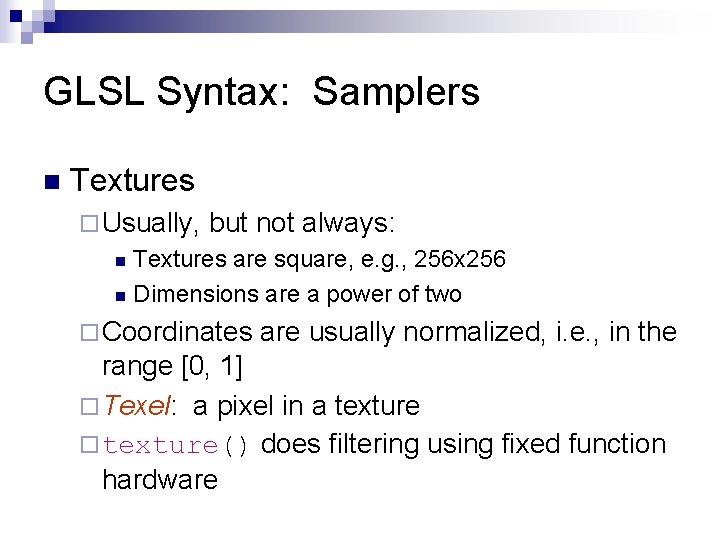
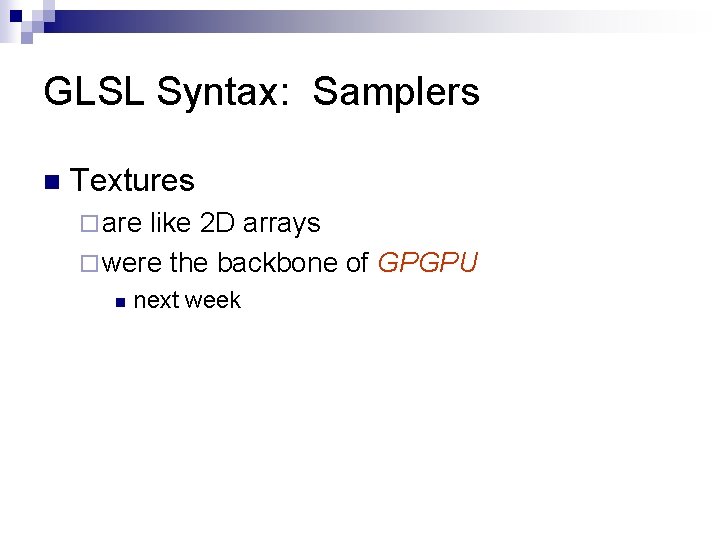
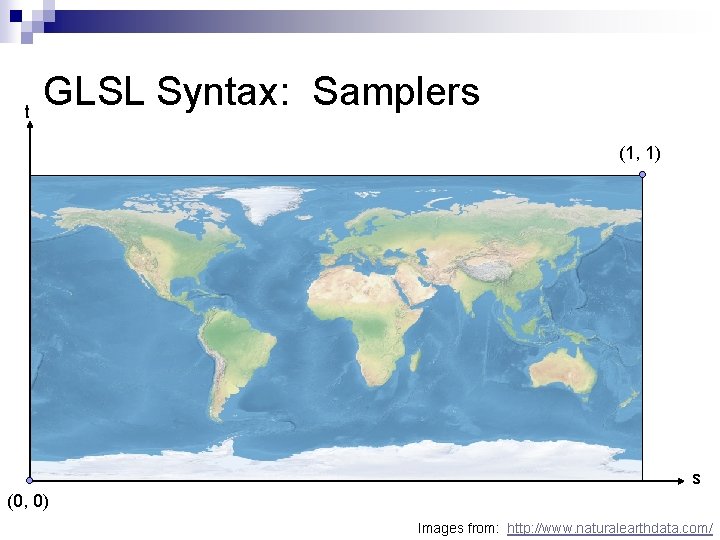
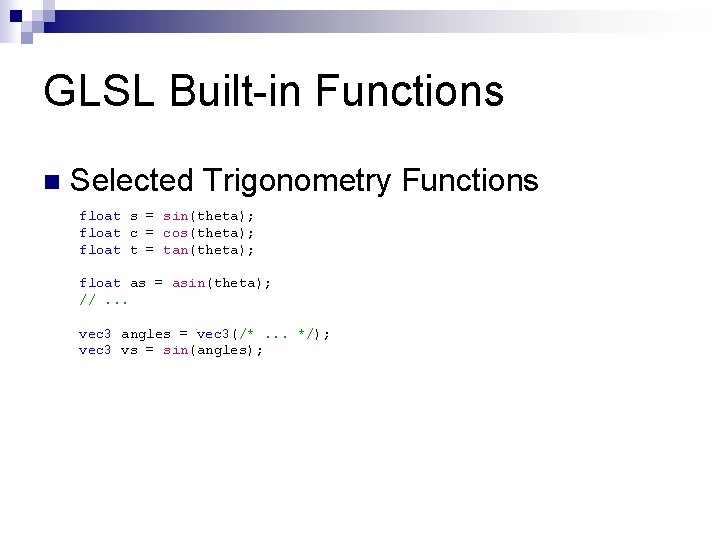
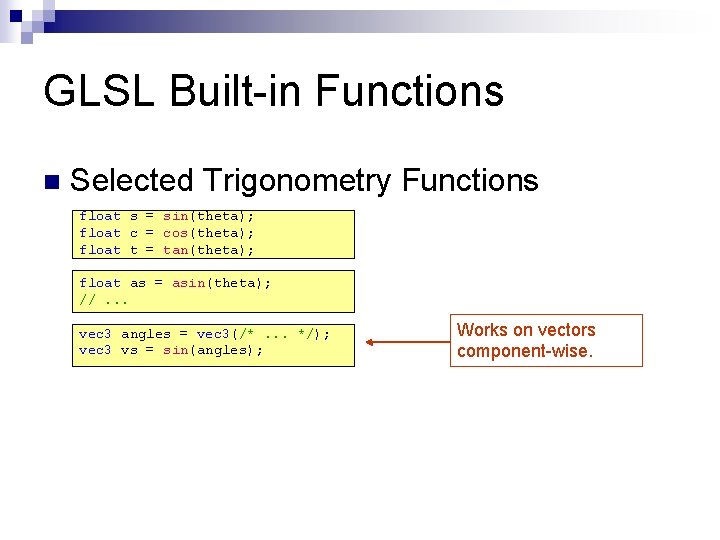
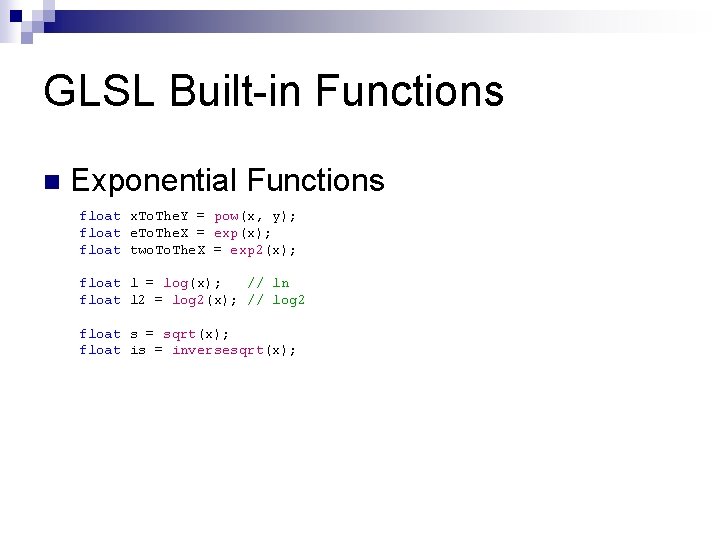
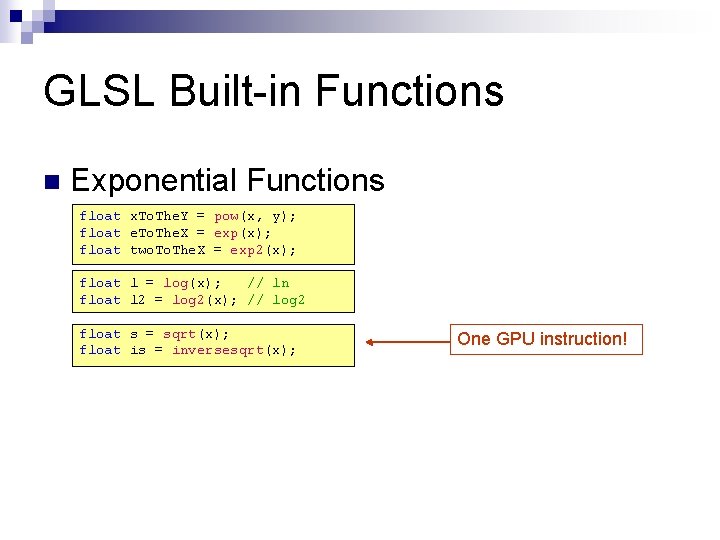
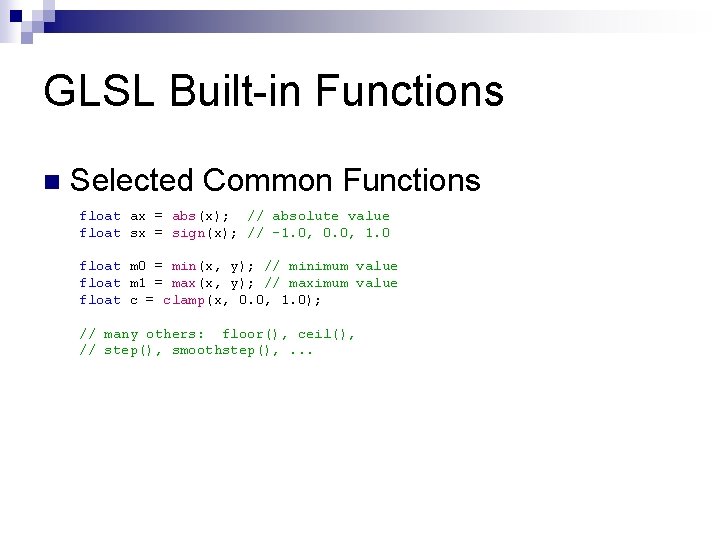
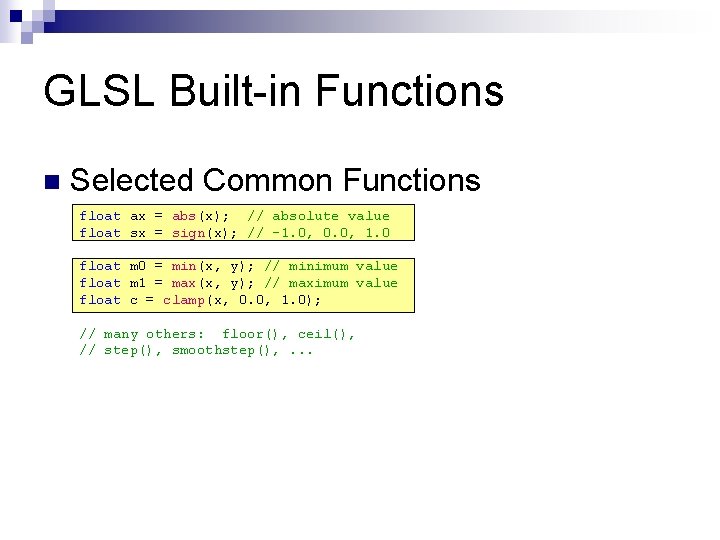
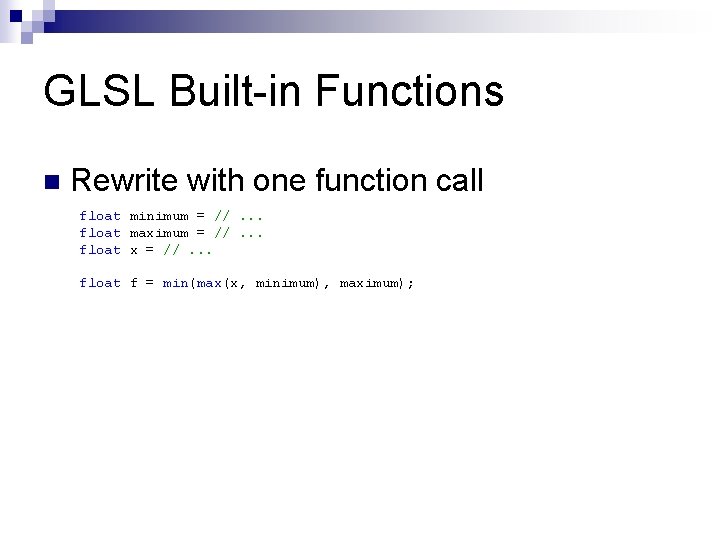
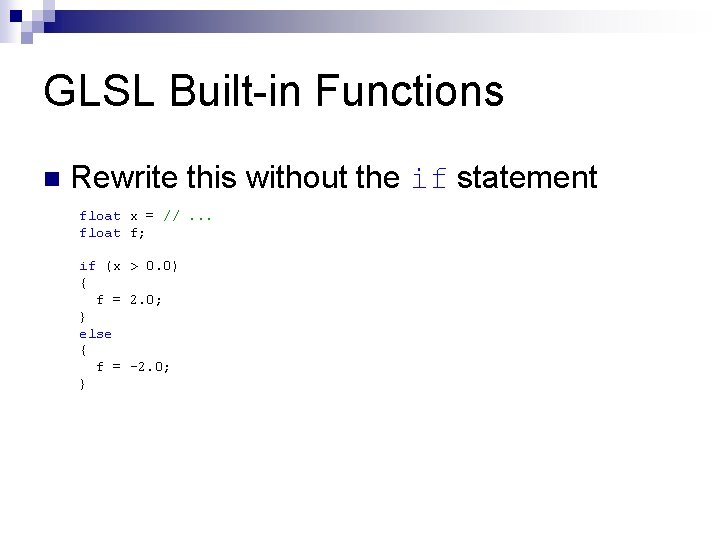
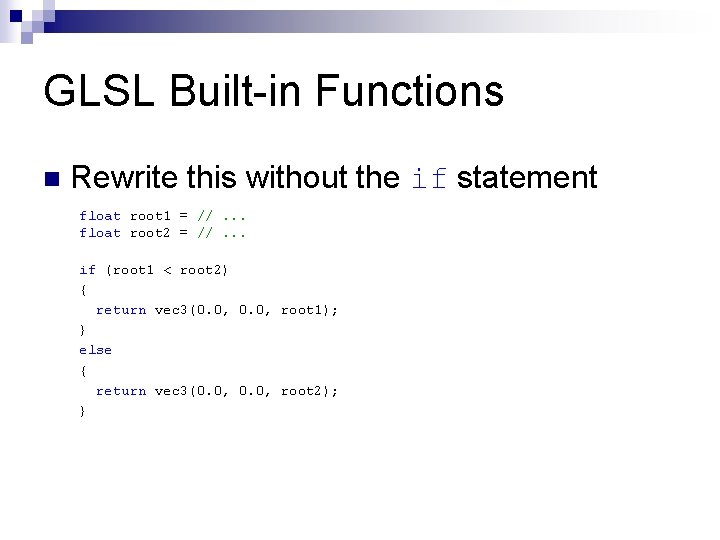
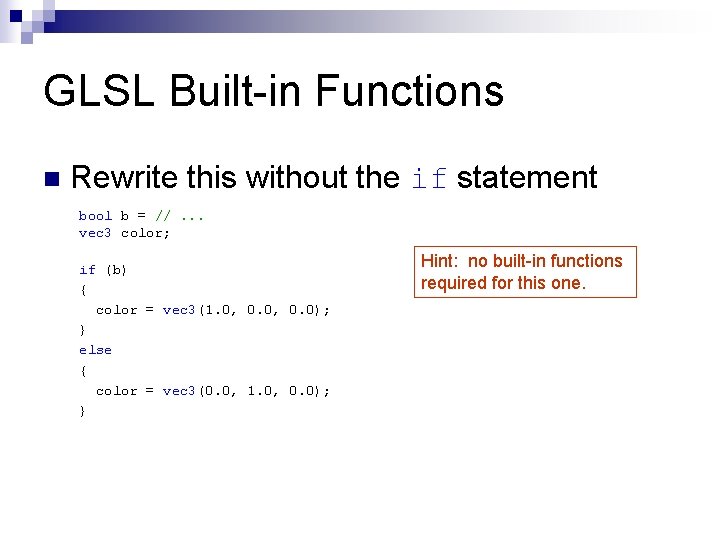
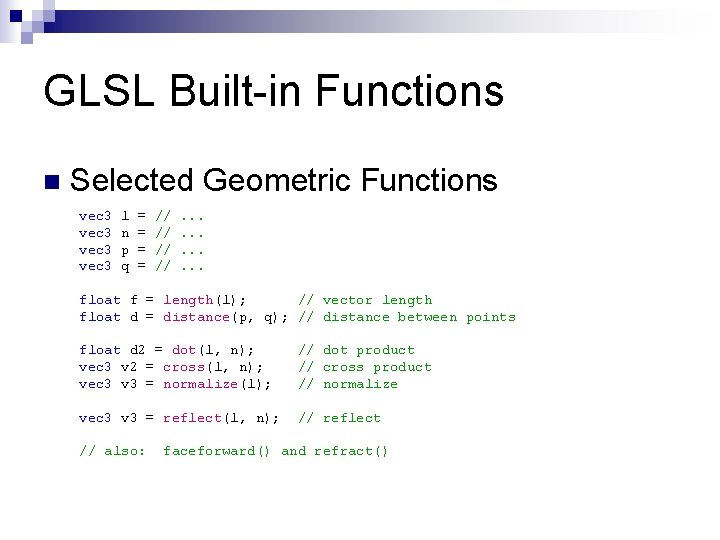
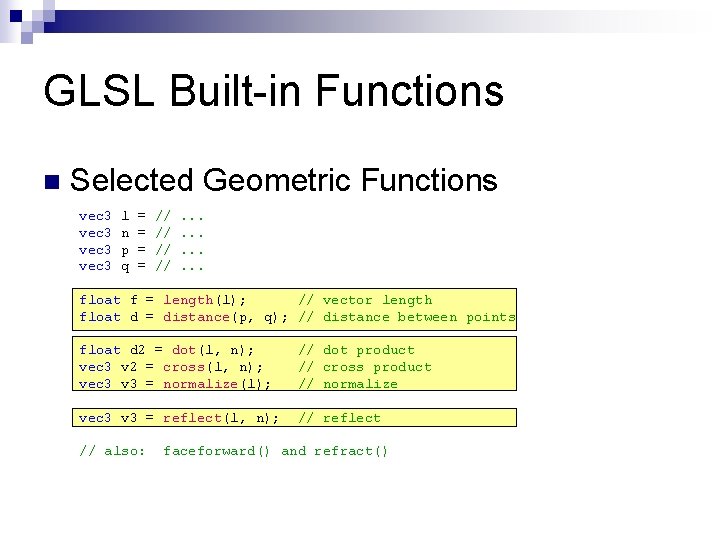
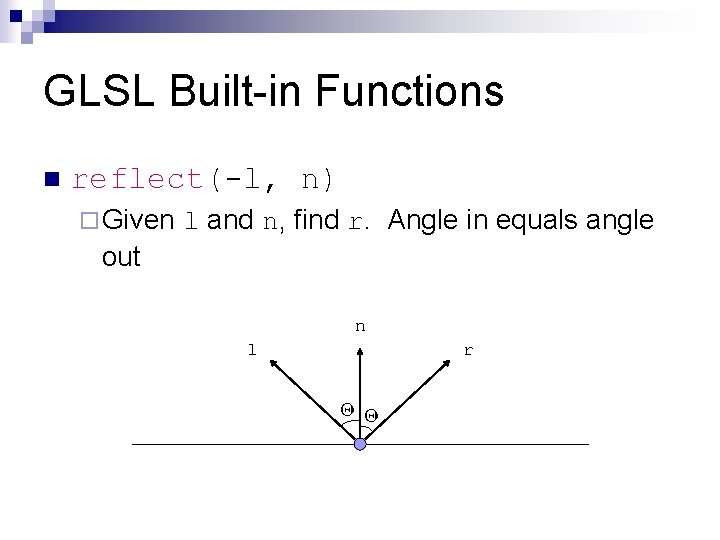
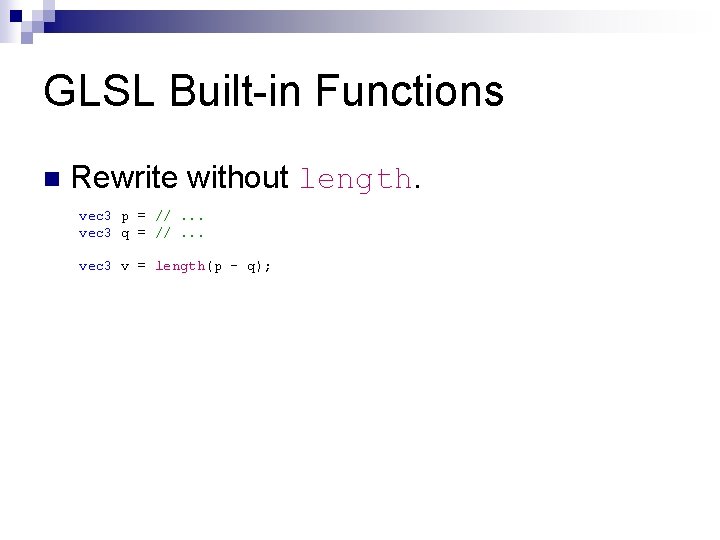
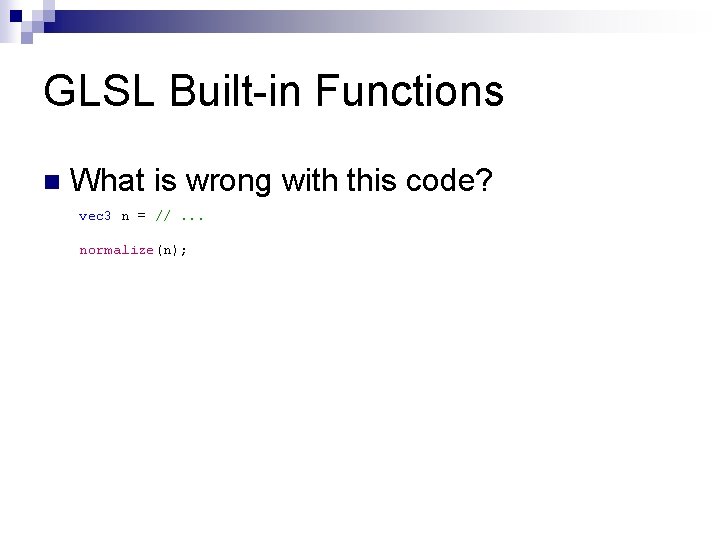
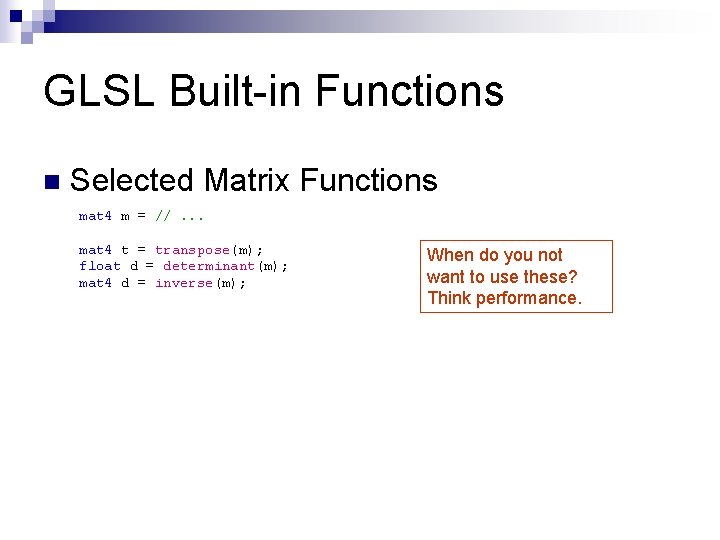
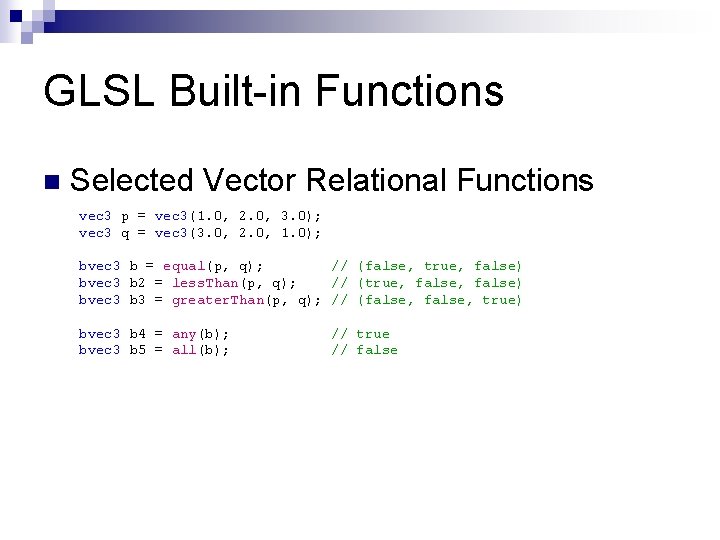
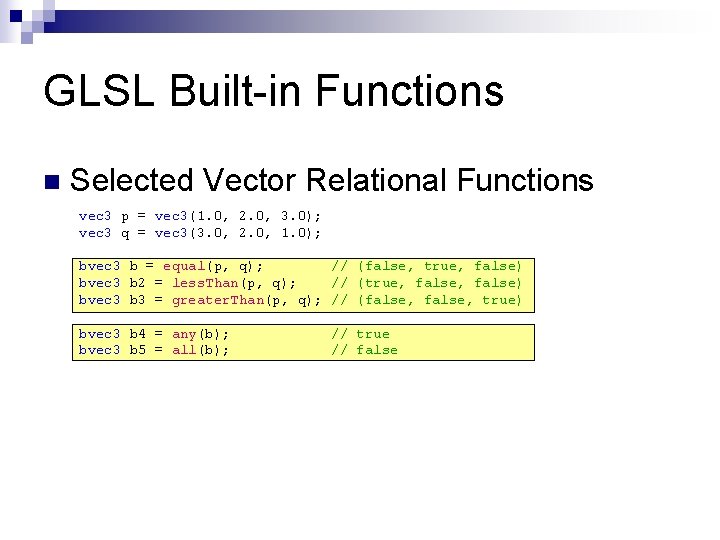
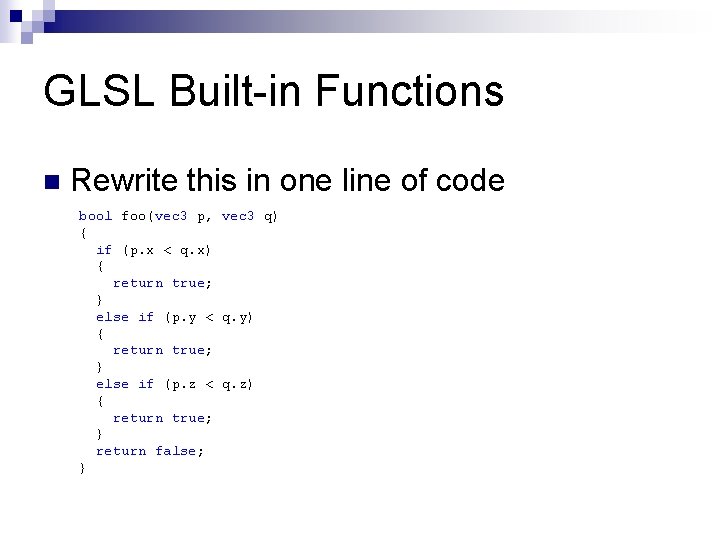
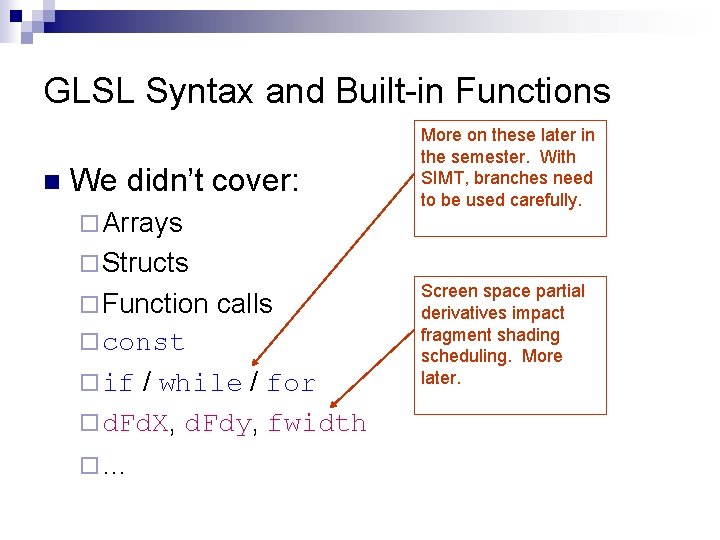
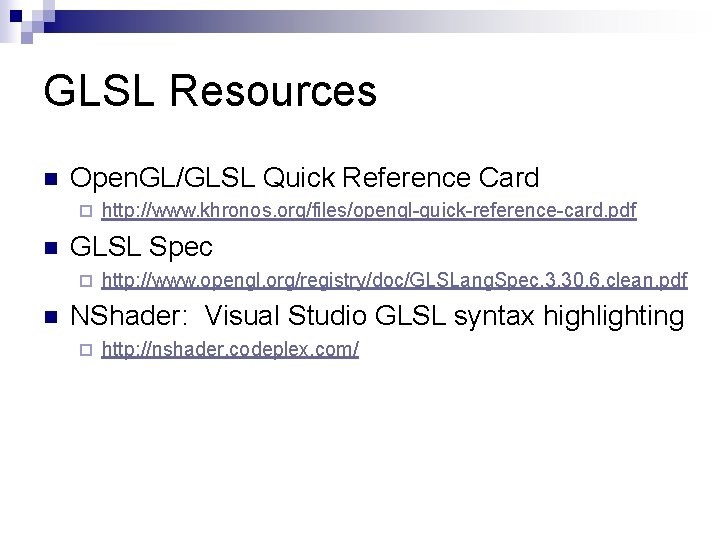
- Slides: 76
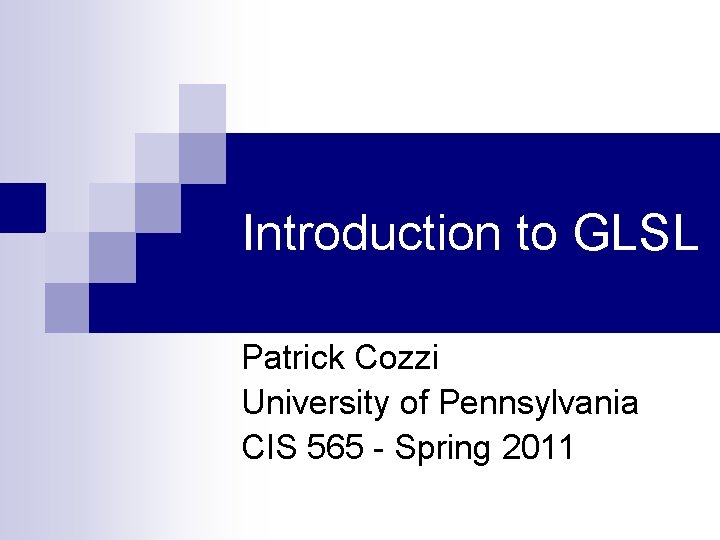
Introduction to GLSL Patrick Cozzi University of Pennsylvania CIS 565 - Spring 2011
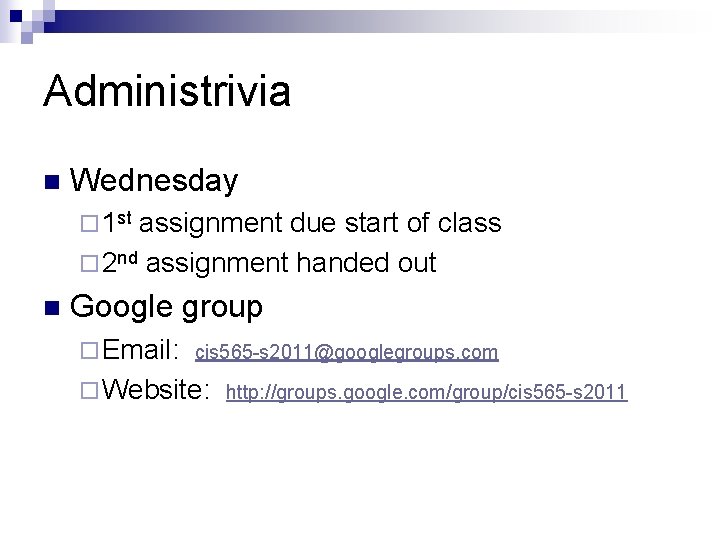
Administrivia n Wednesday ¨ 1 st assignment due start of class ¨ 2 nd assignment handed out n Google group ¨ Email: cis 565 -s 2011@googlegroups. com ¨ Website: http: //groups. google. com/group/cis 565 -s 2011
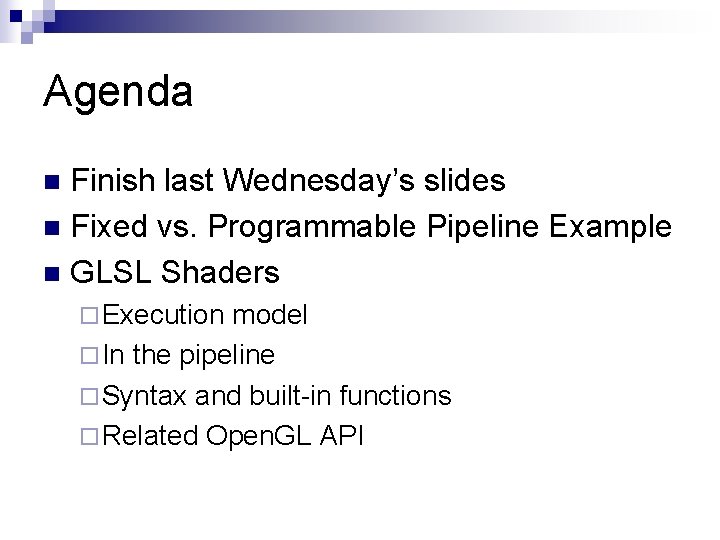
Agenda Finish last Wednesday’s slides n Fixed vs. Programmable Pipeline Example n GLSL Shaders n ¨ Execution model ¨ In the pipeline ¨ Syntax and built-in functions ¨ Related Open. GL API
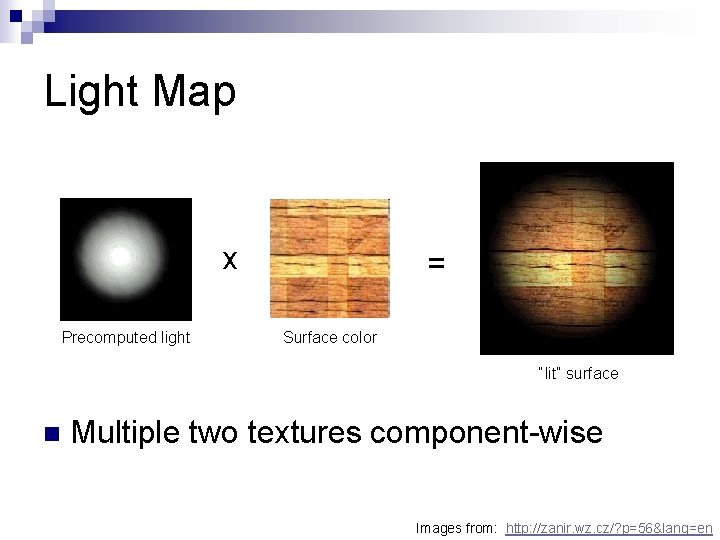
Light Map x Precomputed light = Surface color “lit” surface n Multiple two textures component-wise Images from: http: //zanir. wz. cz/? p=56&lang=en
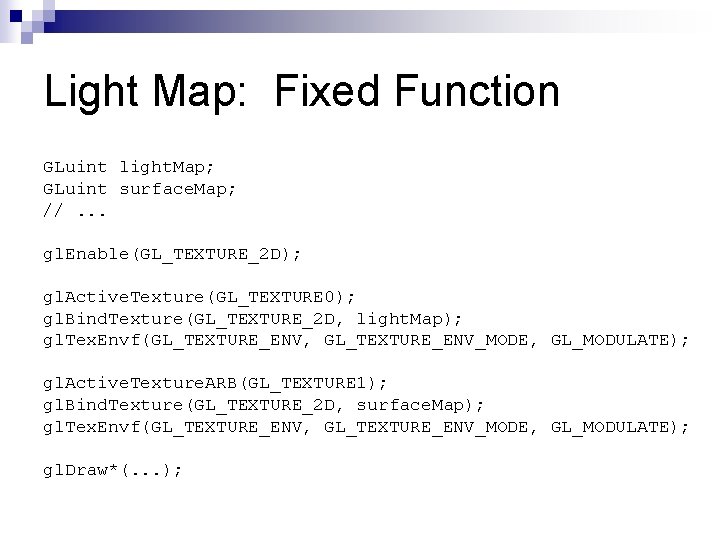
Light Map: Fixed Function GLuint light. Map; GLuint surface. Map; //. . . gl. Enable(GL_TEXTURE_2 D); gl. Active. Texture(GL_TEXTURE 0); gl. Bind. Texture(GL_TEXTURE_2 D, light. Map); gl. Tex. Envf(GL_TEXTURE_ENV, GL_TEXTURE_ENV_MODE, GL_MODULATE); gl. Active. Texture. ARB(GL_TEXTURE 1); gl. Bind. Texture(GL_TEXTURE_2 D, surface. Map); gl. Tex. Envf(GL_TEXTURE_ENV, GL_TEXTURE_ENV_MODE, GL_MODULATE); gl. Draw*(. . . );
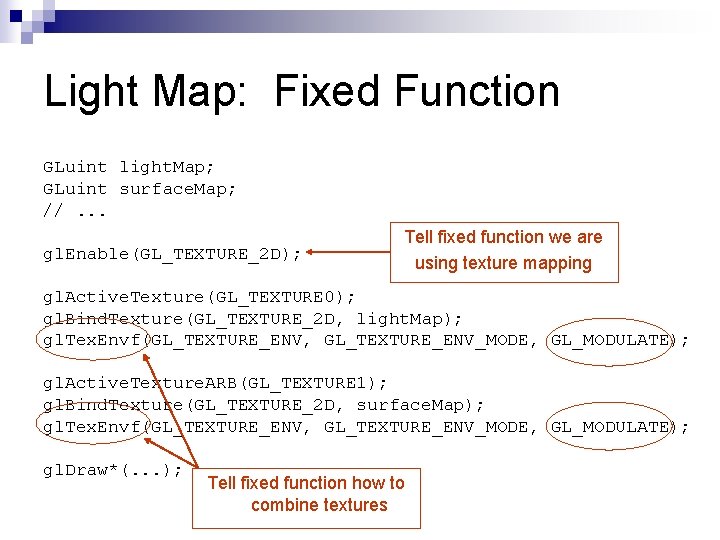
Light Map: Fixed Function GLuint light. Map; GLuint surface. Map; //. . . gl. Enable(GL_TEXTURE_2 D); Tell fixed function we are using texture mapping gl. Active. Texture(GL_TEXTURE 0); gl. Bind. Texture(GL_TEXTURE_2 D, light. Map); gl. Tex. Envf(GL_TEXTURE_ENV, GL_TEXTURE_ENV_MODE, GL_MODULATE); gl. Active. Texture. ARB(GL_TEXTURE 1); gl. Bind. Texture(GL_TEXTURE_2 D, surface. Map); gl. Tex. Envf(GL_TEXTURE_ENV, GL_TEXTURE_ENV_MODE, GL_MODULATE); gl. Draw*(. . . ); Tell fixed function how to combine textures
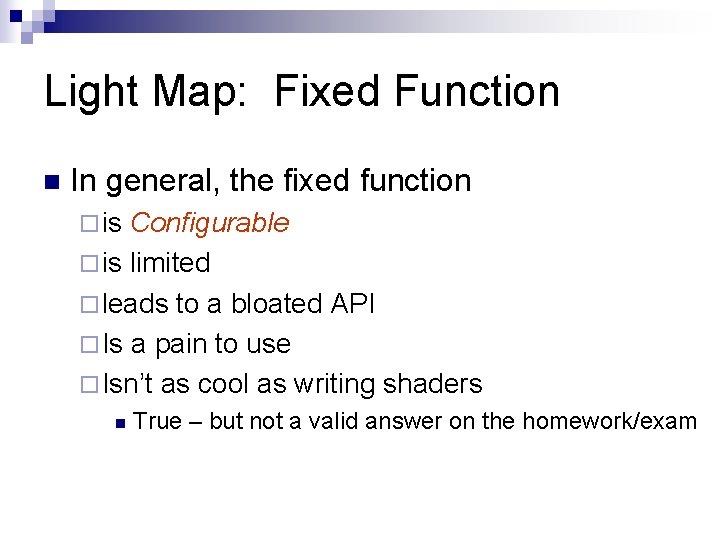
Light Map: Fixed Function n In general, the fixed function ¨ is Configurable ¨ is limited ¨ leads to a bloated API ¨ Is a pain to use ¨ Isn’t as cool as writing shaders n True – but not a valid answer on the homework/exam
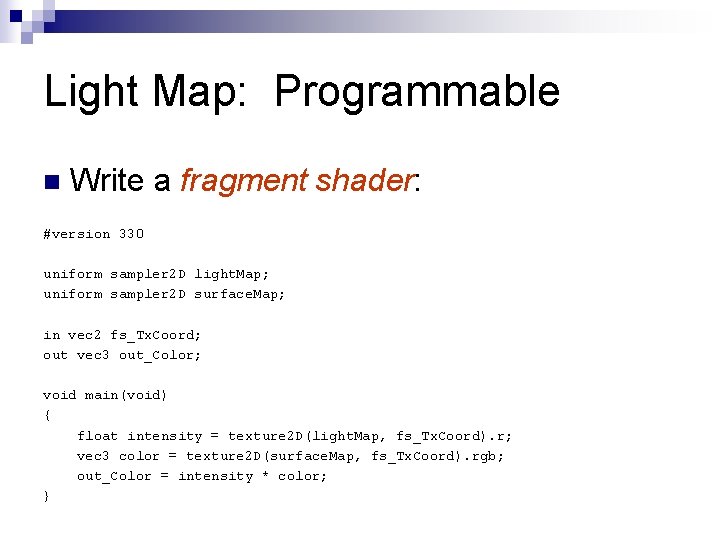
Light Map: Programmable n Write a fragment shader: #version 330 uniform sampler 2 D light. Map; uniform sampler 2 D surface. Map; in vec 2 fs_Tx. Coord; out vec 3 out_Color; void main(void) { float intensity = texture 2 D(light. Map, fs_Tx. Coord). r; vec 3 color = texture 2 D(surface. Map, fs_Tx. Coord). rgb; out_Color = intensity * color; }
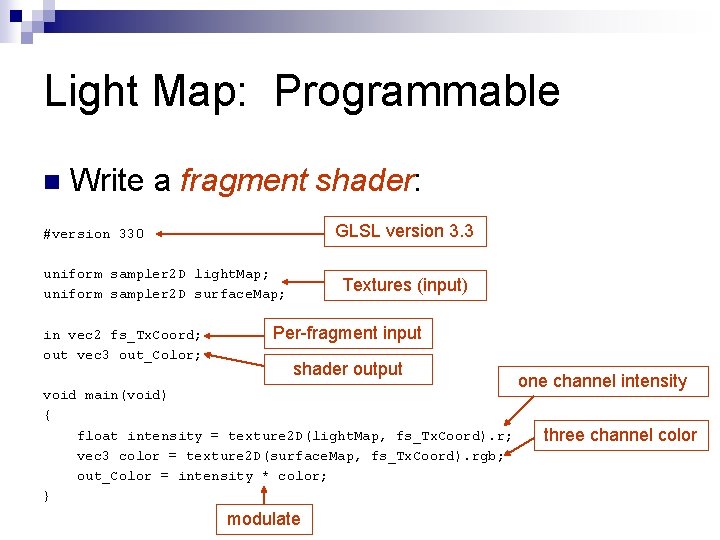
Light Map: Programmable n Write a fragment shader: GLSL version 3. 3 #version 330 uniform sampler 2 D light. Map; uniform sampler 2 D surface. Map; in vec 2 fs_Tx. Coord; out vec 3 out_Color; Textures (input) Per-fragment input shader output void main(void) { float intensity = texture 2 D(light. Map, fs_Tx. Coord). r; vec 3 color = texture 2 D(surface. Map, fs_Tx. Coord). rgb; out_Color = intensity * color; } modulate one channel intensity three channel color
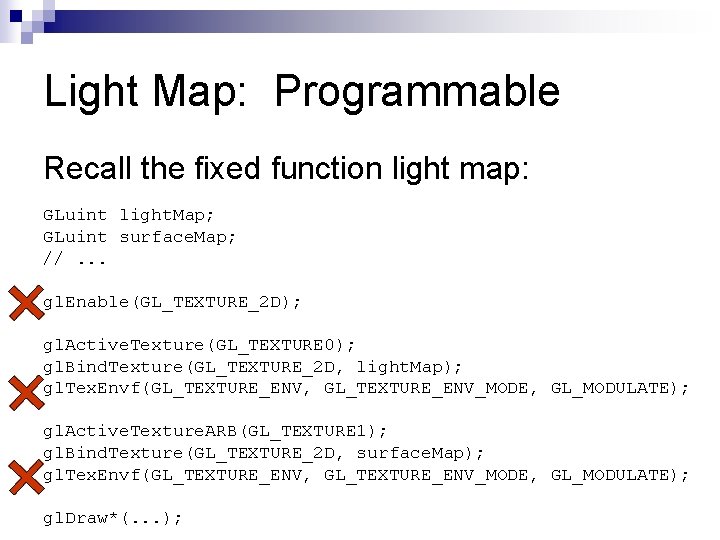
Light Map: Programmable Recall the fixed function light map: GLuint light. Map; GLuint surface. Map; //. . . gl. Enable(GL_TEXTURE_2 D); gl. Active. Texture(GL_TEXTURE 0); gl. Bind. Texture(GL_TEXTURE_2 D, light. Map); gl. Tex. Envf(GL_TEXTURE_ENV, GL_TEXTURE_ENV_MODE, GL_MODULATE); gl. Active. Texture. ARB(GL_TEXTURE 1); gl. Bind. Texture(GL_TEXTURE_2 D, surface. Map); gl. Tex. Envf(GL_TEXTURE_ENV, GL_TEXTURE_ENV_MODE, GL_MODULATE); gl. Draw*(. . . );
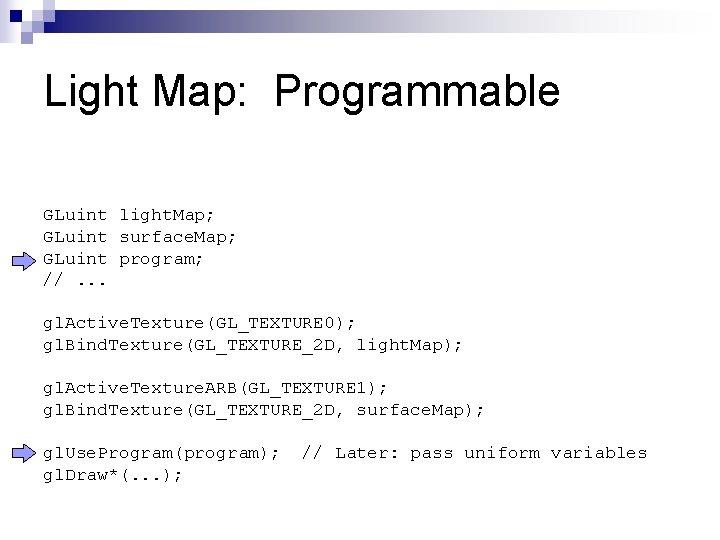
Light Map: Programmable GLuint light. Map; GLuint surface. Map; GLuint program; //. . . gl. Active. Texture(GL_TEXTURE 0); gl. Bind. Texture(GL_TEXTURE_2 D, light. Map); gl. Active. Texture. ARB(GL_TEXTURE 1); gl. Bind. Texture(GL_TEXTURE_2 D, surface. Map); gl. Use. Program(program); gl. Draw*(. . . ); // Later: pass uniform variables
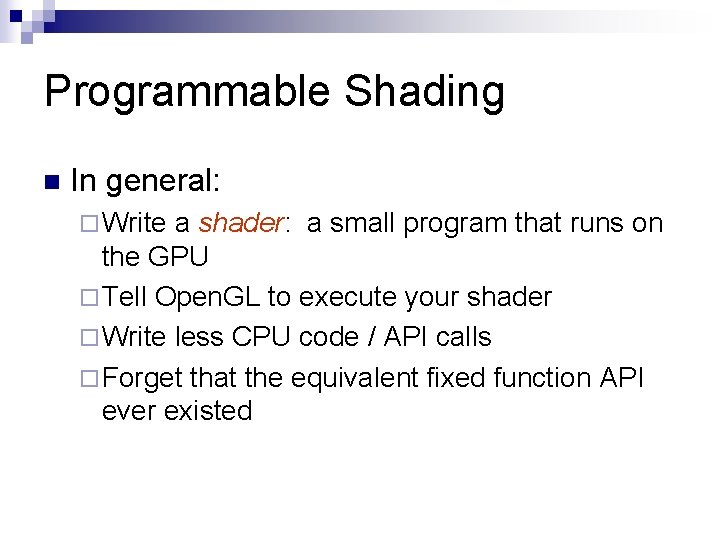
Programmable Shading n In general: ¨ Write a shader: a small program that runs on the GPU ¨ Tell Open. GL to execute your shader ¨ Write less CPU code / API calls ¨ Forget that the equivalent fixed function API ever existed
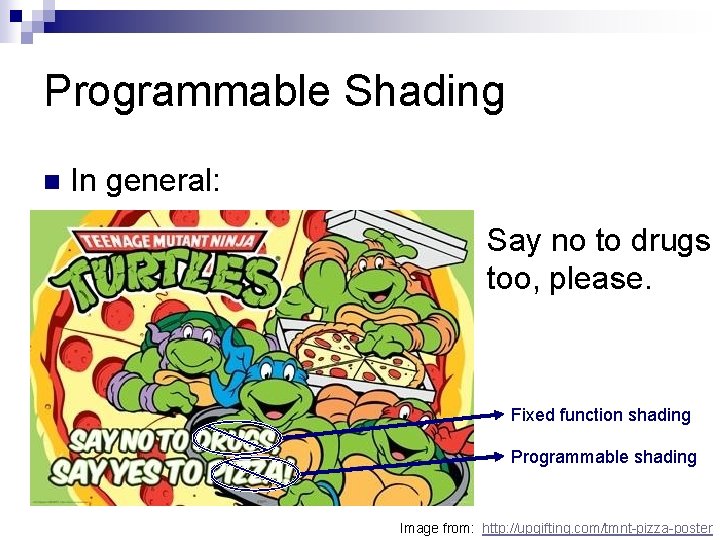
Programmable Shading n In general: Say no to drugs too, please. Fixed function shading Programmable shading Image from: http: //upgifting. com/tmnt-pizza-poster
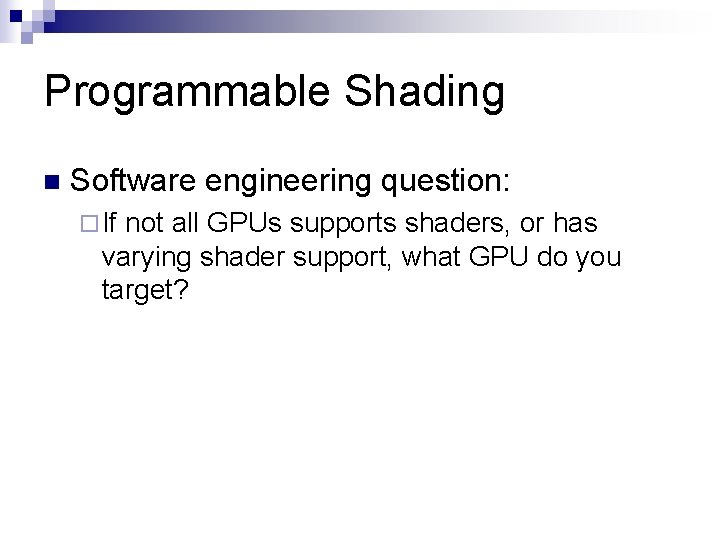
Programmable Shading n Software engineering question: ¨ If not all GPUs supports shaders, or has varying shader support, what GPU do you target?
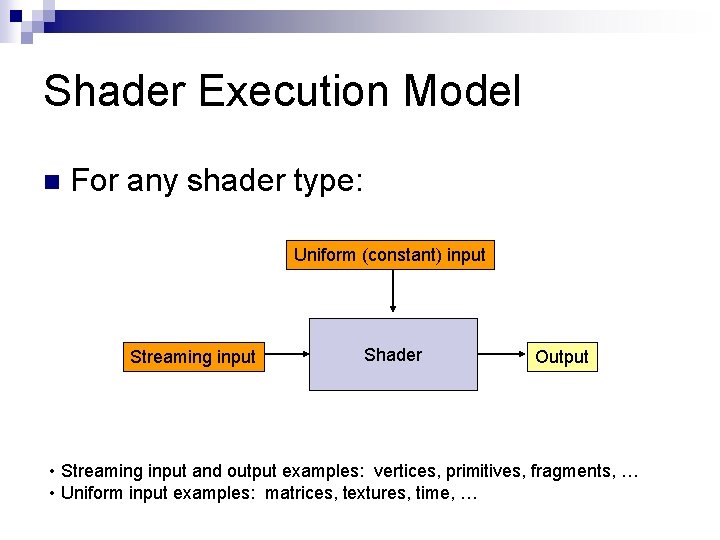
Shader Execution Model n For any shader type: Uniform (constant) input Streaming input Shader Output • Streaming input and output examples: vertices, primitives, fragments, … • Uniform input examples: matrices, textures, time, …
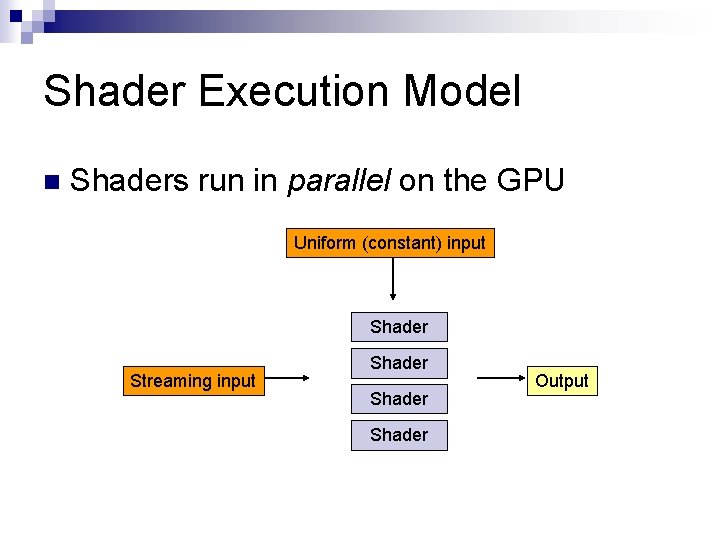
Shader Execution Model n Shaders run in parallel on the GPU Uniform (constant) input Shader Streaming input Shader Output
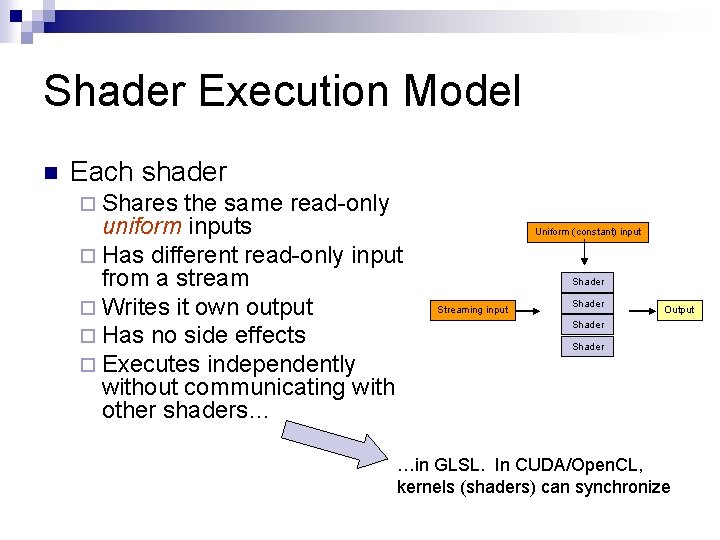
Shader Execution Model n Each shader ¨ Shares the same read-only uniform inputs ¨ Has different read-only input from a stream ¨ Writes it own output ¨ Has no side effects ¨ Executes independently without communicating with other shaders… Uniform (constant) input Shader Streaming input Shader Output Shader …in GLSL. In CUDA/Open. CL, kernels (shaders) can synchronize
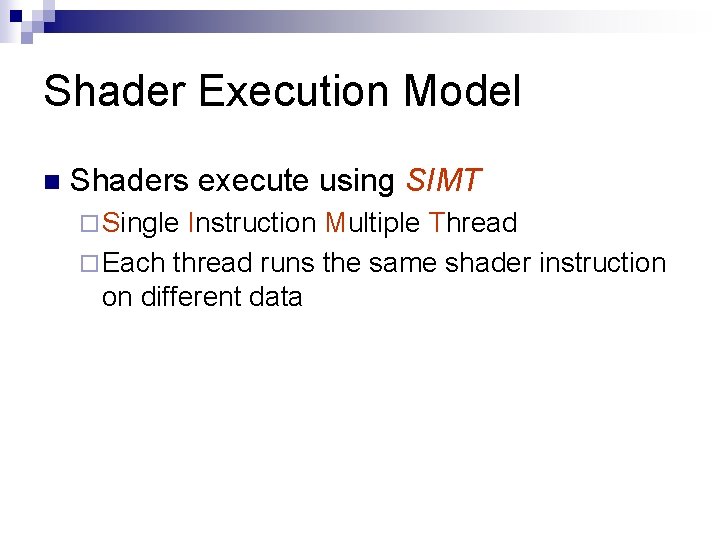
Shader Execution Model n Shaders execute using SIMT ¨ Single Instruction Multiple Thread ¨ Each thread runs the same shader instruction on different data
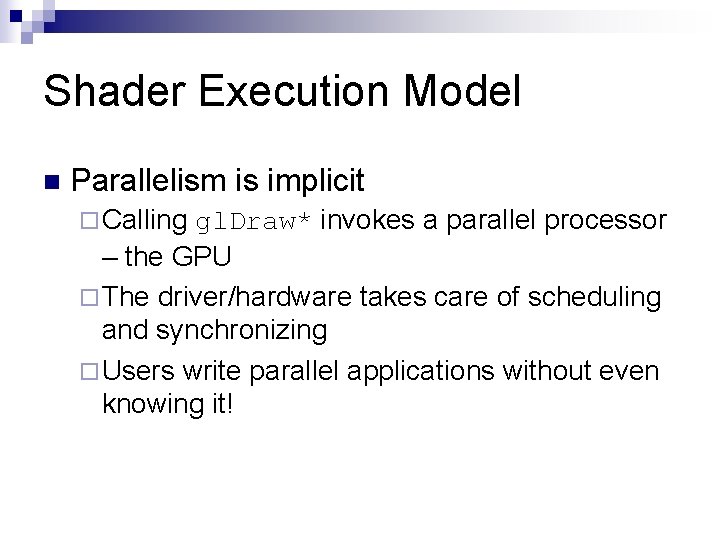
Shader Execution Model n Parallelism is implicit ¨ Calling gl. Draw* invokes a parallel processor – the GPU ¨ The driver/hardware takes care of scheduling and synchronizing ¨ Users write parallel applications without even knowing it!
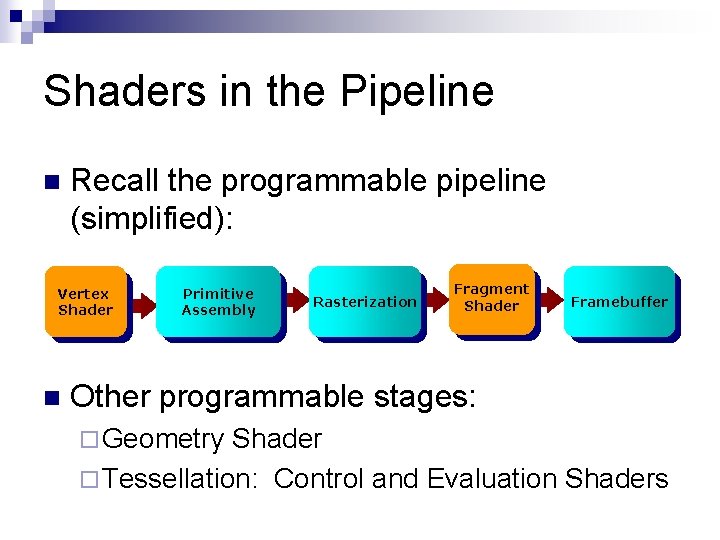
Shaders in the Pipeline n Recall the programmable pipeline (simplified): Vertex Shader n Primitive Assembly Rasterization Fragment Shader Framebuffer Other programmable stages: ¨ Geometry Shader ¨ Tessellation: Control and Evaluation Shaders
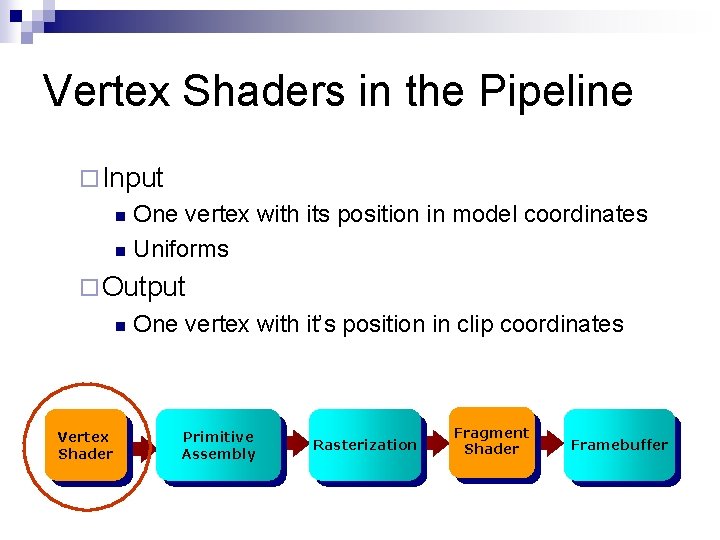
Vertex Shaders in the Pipeline ¨ Input One vertex with its position in model coordinates n Uniforms n ¨ Output n Vertex Shader One vertex with it’s position in clip coordinates Primitive Assembly Rasterization Fragment Shader Framebuffer
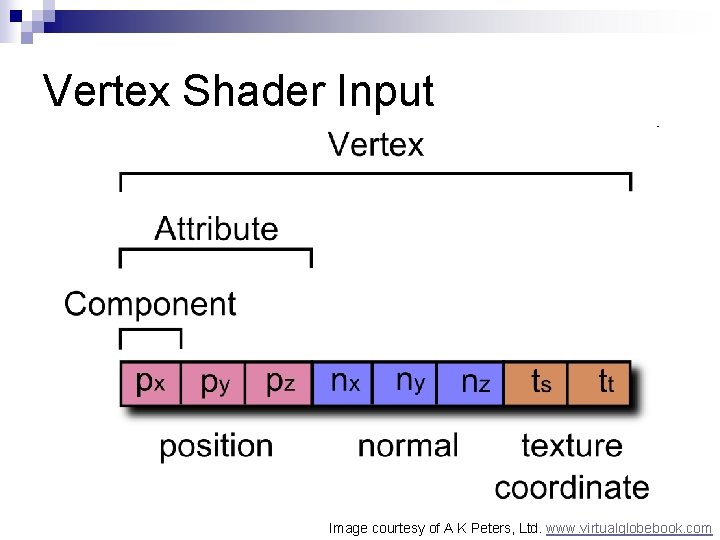
Vertex Shader Input Image courtesy of A K Peters, Ltd. www. virtualglobebook. com
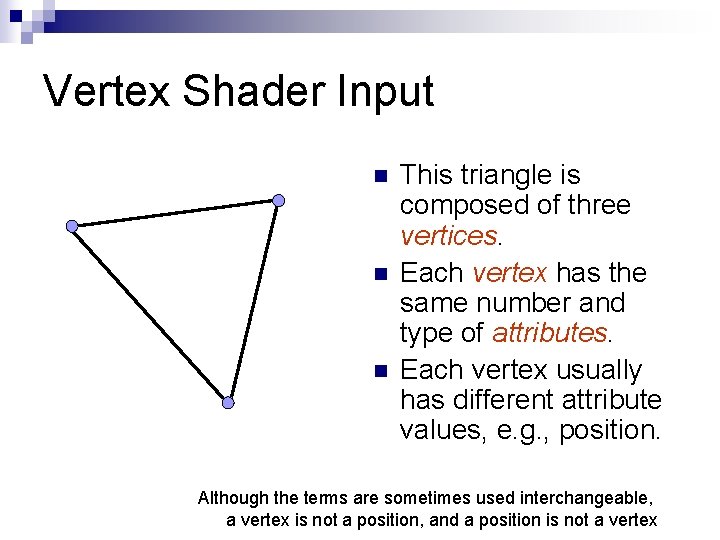
Vertex Shader Input n n n This triangle is composed of three vertices. Each vertex has the same number and type of attributes. Each vertex usually has different attribute values, e. g. , position. Although the terms are sometimes used interchangeable, a vertex is not a position, and a position is not a vertex
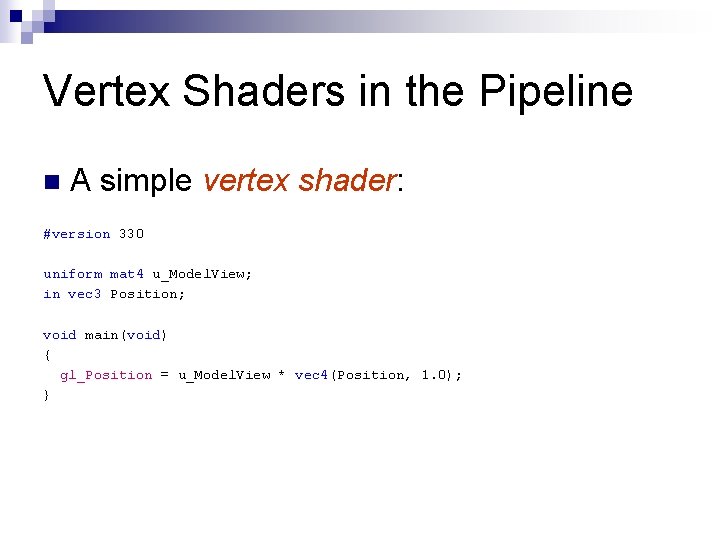
Vertex Shaders in the Pipeline n A simple vertex shader: #version 330 uniform mat 4 u_Model. View; in vec 3 Position; void main(void) { gl_Position = u_Model. View * vec 4(Position, 1. 0); }
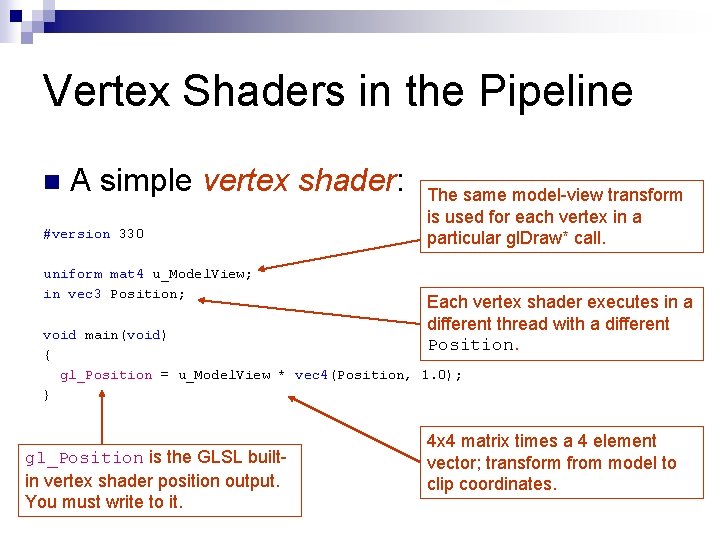
Vertex Shaders in the Pipeline n A simple vertex shader: #version 330 uniform mat 4 u_Model. View; in vec 3 Position; The same model-view transform is used for each vertex in a particular gl. Draw* call. Each vertex shader executes in a different thread with a different Position. void main(void) { gl_Position = u_Model. View * vec 4(Position, 1. 0); } gl_Position is the GLSL builtin vertex shader position output. You must write to it. 4 x 4 matrix times a 4 element vector; transform from model to clip coordinates.
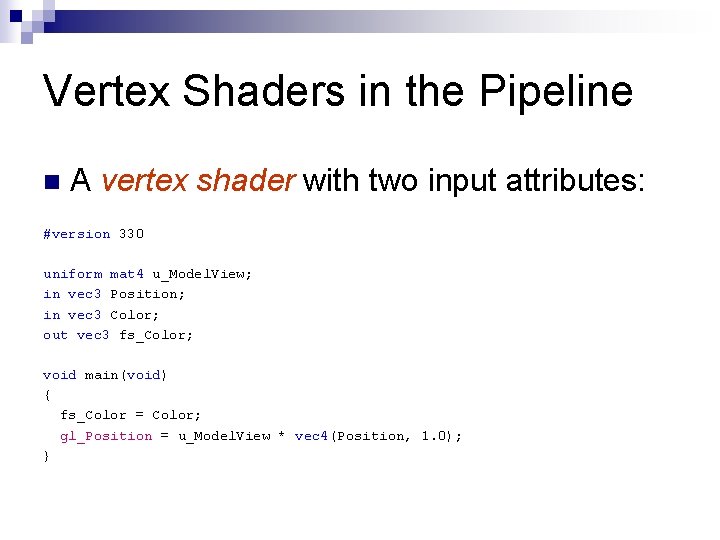
Vertex Shaders in the Pipeline n A vertex shader with two input attributes: #version 330 uniform mat 4 u_Model. View; in vec 3 Position; in vec 3 Color; out vec 3 fs_Color; void main(void) { fs_Color = Color; gl_Position = u_Model. View * vec 4(Position, 1. 0); }
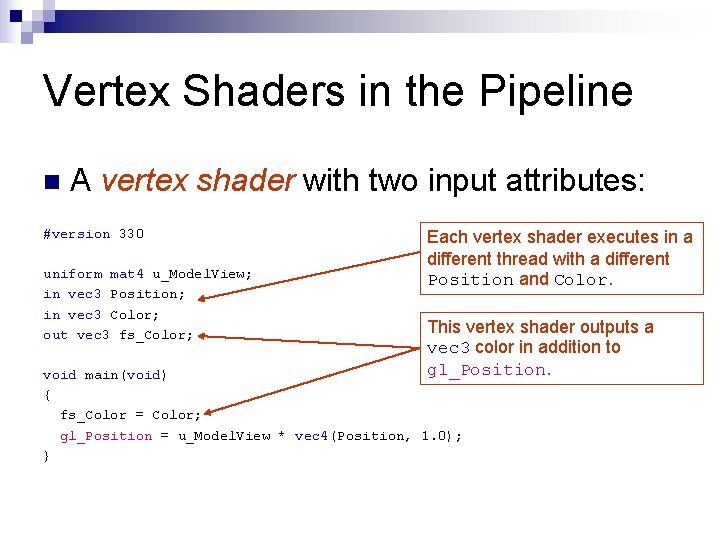
Vertex Shaders in the Pipeline n A vertex shader with two input attributes: #version 330 uniform mat 4 u_Model. View; in vec 3 Position; in vec 3 Color; out vec 3 fs_Color; Each vertex shader executes in a different thread with a different Position and Color. This vertex shader outputs a vec 3 color in addition to gl_Position. void main(void) { fs_Color = Color; gl_Position = u_Model. View * vec 4(Position, 1. 0); }
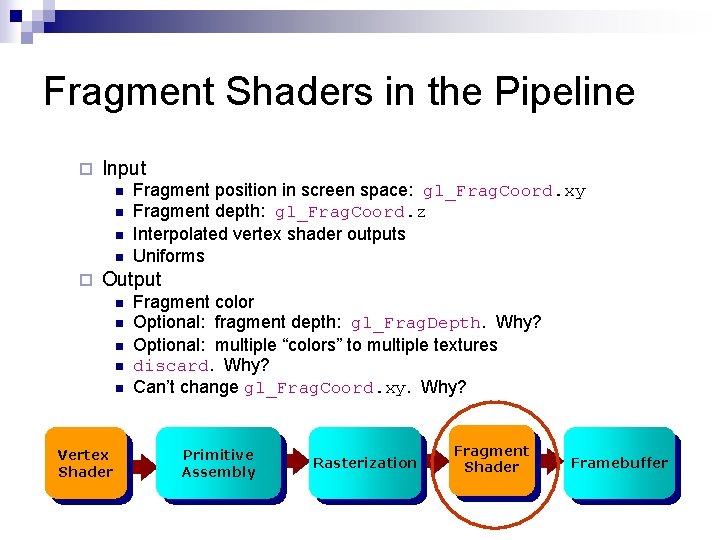
Fragment Shaders in the Pipeline ¨ Input n n ¨ Fragment position in screen space: gl_Frag. Coord. xy Fragment depth: gl_Frag. Coord. z Interpolated vertex shader outputs Uniforms Output n n n Vertex Shader Fragment color Optional: fragment depth: gl_Frag. Depth. Why? Optional: multiple “colors” to multiple textures discard. Why? Can’t change gl_Frag. Coord. xy. Why? Primitive Assembly Rasterization Fragment Shader Framebuffer
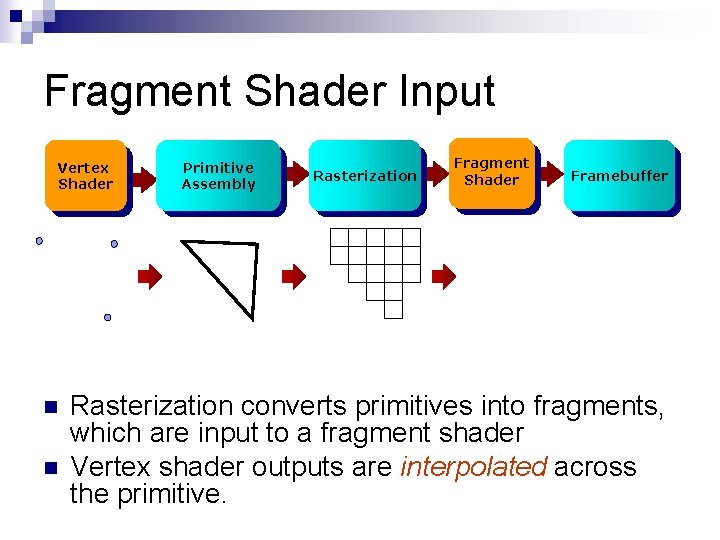
Fragment Shader Input Vertex Shader n n Primitive Assembly Rasterization Fragment Shader Framebuffer Rasterization converts primitives into fragments, which are input to a fragment shader Vertex shader outputs are interpolated across the primitive.
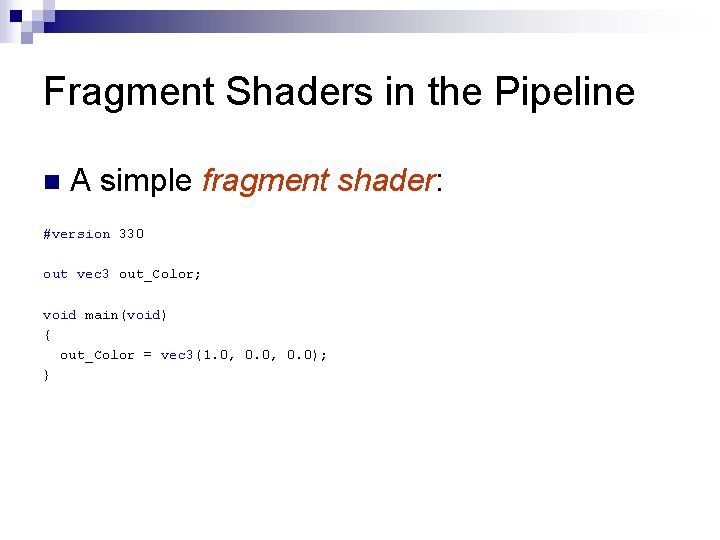
Fragment Shaders in the Pipeline n A simple fragment shader: #version 330 out vec 3 out_Color; void main(void) { out_Color = vec 3(1. 0, 0. 0); }
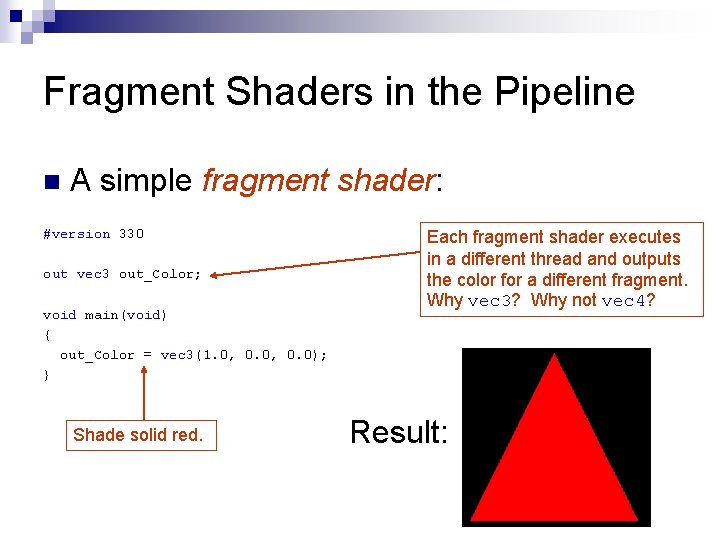
Fragment Shaders in the Pipeline n A simple fragment shader: #version 330 out vec 3 out_Color; void main(void) { out_Color = vec 3(1. 0, 0. 0); } Shade solid red. Each fragment shader executes in a different thread and outputs the color for a different fragment. Why vec 3? Why not vec 4? Result:
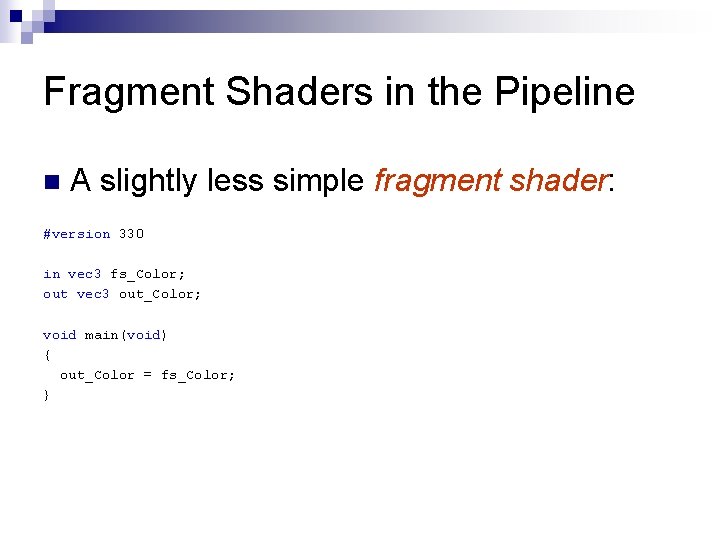
Fragment Shaders in the Pipeline n A slightly less simple fragment shader: #version 330 in vec 3 fs_Color; out vec 3 out_Color; void main(void) { out_Color = fs_Color; }
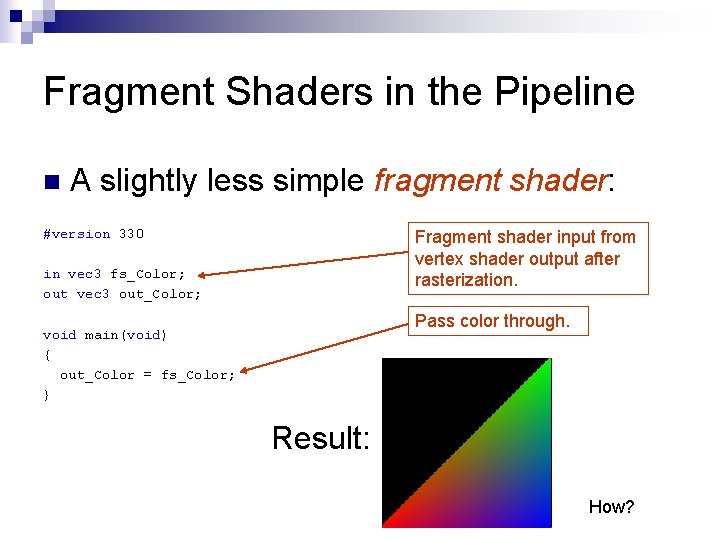
Fragment Shaders in the Pipeline n A slightly less simple fragment shader: #version 330 Fragment shader input from vertex shader output after rasterization. in vec 3 fs_Color; out vec 3 out_Color; Pass color through. void main(void) { out_Color = fs_Color; } Result: How?
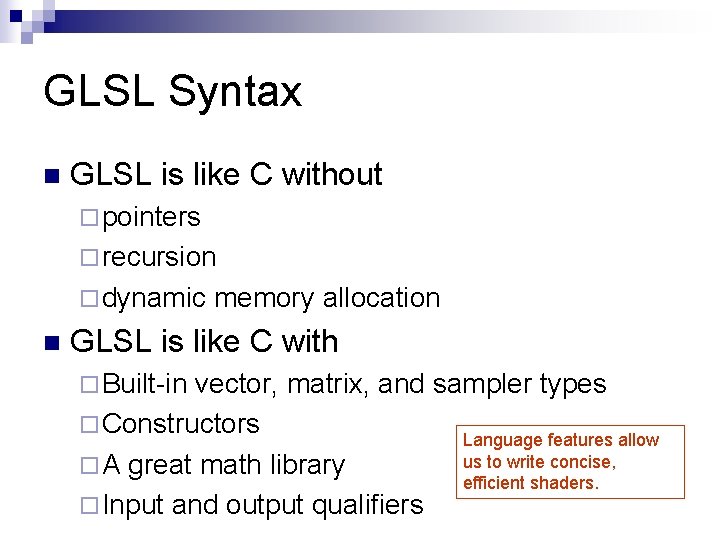
GLSL Syntax n GLSL is like C without ¨ pointers ¨ recursion ¨ dynamic n memory allocation GLSL is like C with ¨ Built-in vector, matrix, and sampler types ¨ Constructors Language features allow us to write concise, ¨ A great math library efficient shaders. ¨ Input and output qualifiers
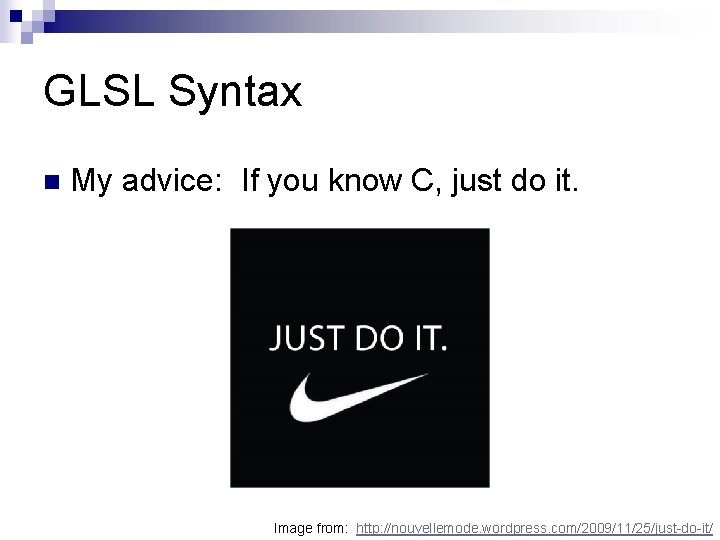
GLSL Syntax n My advice: If you know C, just do it. Image from: http: //nouvellemode. wordpress. com/2009/11/25/just-do-it/
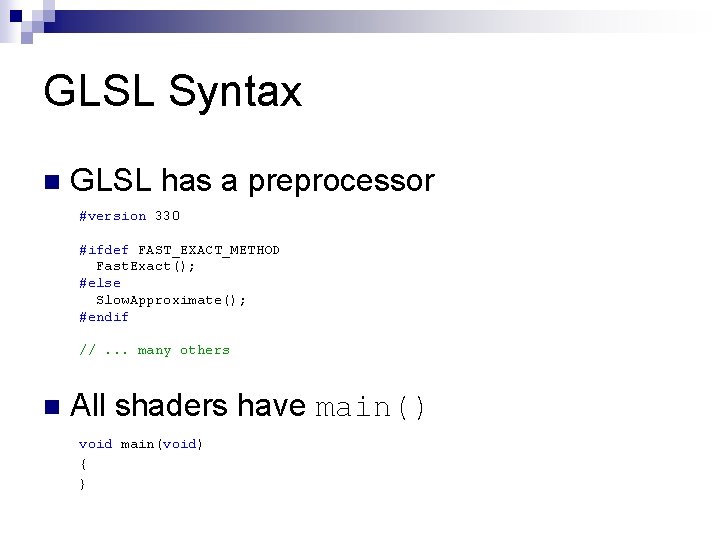
GLSL Syntax n GLSL has a preprocessor #version 330 #ifdef FAST_EXACT_METHOD Fast. Exact(); #else Slow. Approximate(); #endif //. . . many others n All shaders have main() void main(void) { }
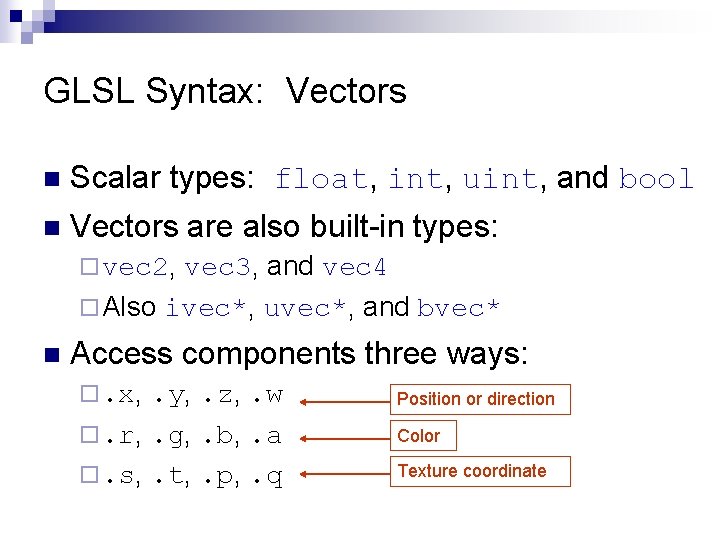
GLSL Syntax: Vectors n Scalar types: float, int, uint, and bool n Vectors are also built-in types: vec 3, and vec 4 ¨ Also ivec*, uvec*, and bvec* ¨ vec 2, n Access components three ways: ¨. x, . y, . z, . w ¨. r, . g, . b, . a ¨. s, . t, . p, . q Position or direction Color Texture coordinate
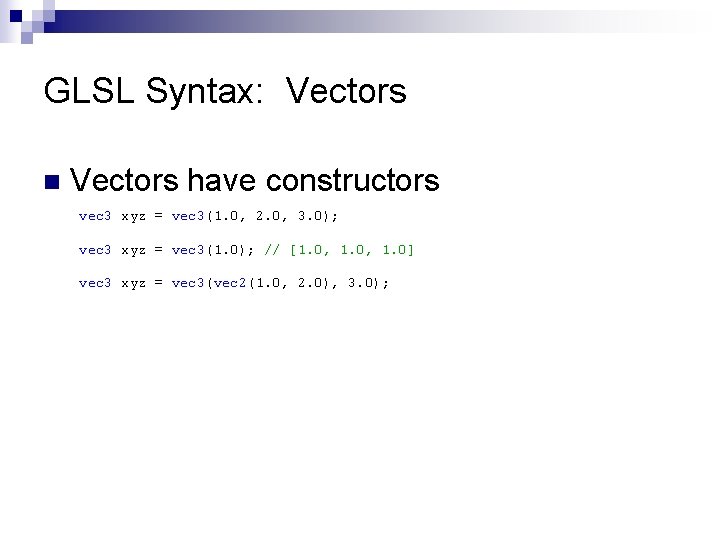
GLSL Syntax: Vectors n Vectors have constructors vec 3 xyz = vec 3(1. 0, 2. 0, 3. 0); vec 3 xyz = vec 3(1. 0); // [1. 0, 1. 0] vec 3 xyz = vec 3(vec 2(1. 0, 2. 0), 3. 0);
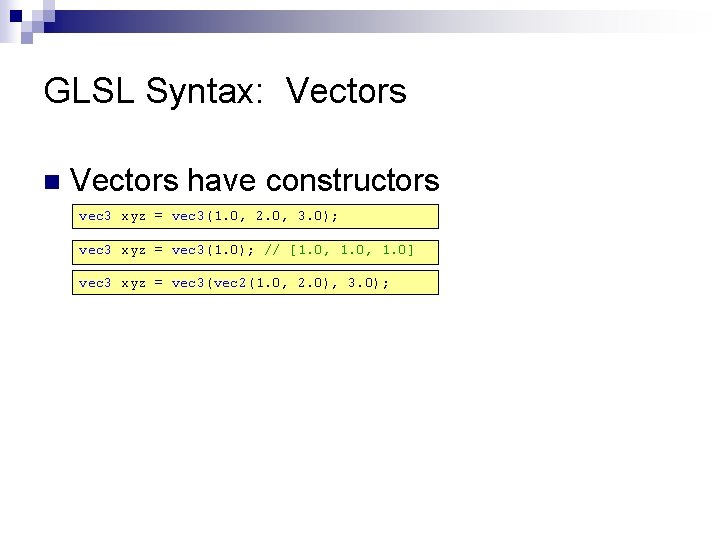
GLSL Syntax: Vectors n Vectors have constructors vec 3 xyz = vec 3(1. 0, 2. 0, 3. 0); vec 3 xyz = vec 3(1. 0); // [1. 0, 1. 0] vec 3 xyz = vec 3(vec 2(1. 0, 2. 0), 3. 0);
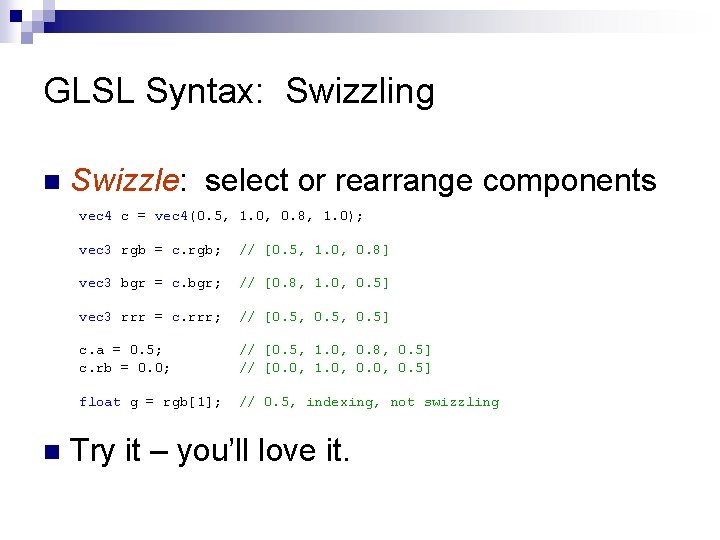
GLSL Syntax: Swizzling n Swizzle: select or rearrange components vec 4 c = vec 4(0. 5, 1. 0, 0. 8, 1. 0); n vec 3 rgb = c. rgb; // [0. 5, 1. 0, 0. 8] vec 3 bgr = c. bgr; // [0. 8, 1. 0, 0. 5] vec 3 rrr = c. rrr; // [0. 5, 0. 5] c. a = 0. 5; c. rb = 0. 0; // [0. 5, 1. 0, 0. 8, 0. 5] // [0. 0, 1. 0, 0. 5] float g = rgb[1]; // 0. 5, indexing, not swizzling Try it – you’ll love it.
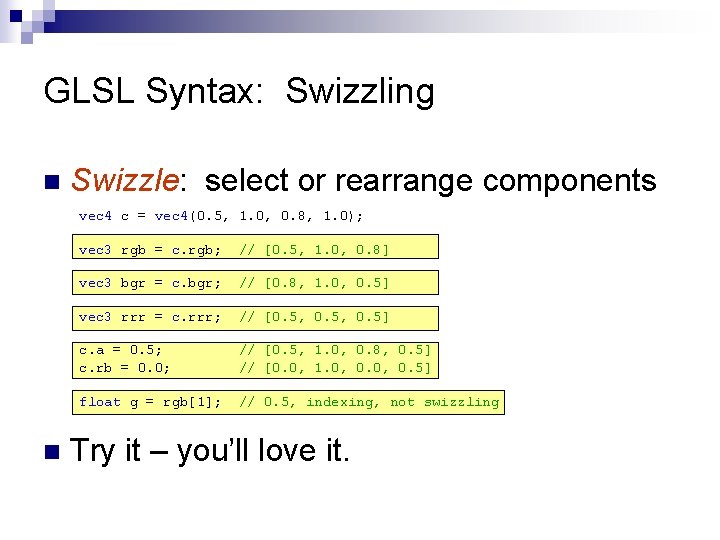
GLSL Syntax: Swizzling n Swizzle: select or rearrange components vec 4 c = vec 4(0. 5, 1. 0, 0. 8, 1. 0); n vec 3 rgb = c. rgb; // [0. 5, 1. 0, 0. 8] vec 3 bgr = c. bgr; // [0. 8, 1. 0, 0. 5] vec 3 rrr = c. rrr; // [0. 5, 0. 5] c. a = 0. 5; c. rb = 0. 0; // [0. 5, 1. 0, 0. 8, 0. 5] // [0. 0, 1. 0, 0. 5] float g = rgb[1]; // 0. 5, indexing, not swizzling Try it – you’ll love it.
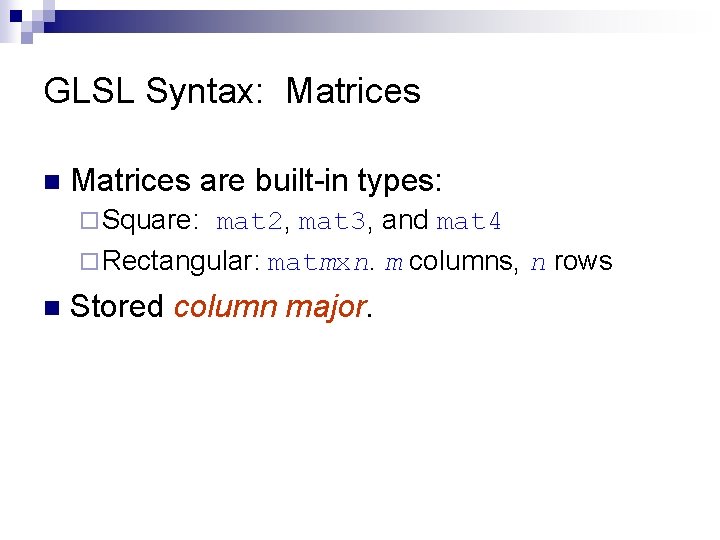
GLSL Syntax: Matrices n Matrices are built-in types: ¨ Square: mat 2, mat 3, and mat 4 ¨ Rectangular: matmxn. m columns, n rows n Stored column major.
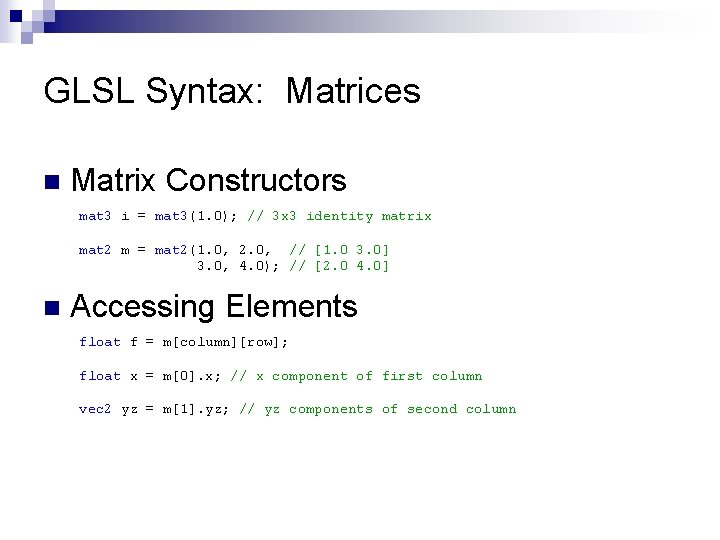
GLSL Syntax: Matrices n Matrix Constructors mat 3 i = mat 3(1. 0); // 3 x 3 identity matrix mat 2 m = mat 2(1. 0, 2. 0, // [1. 0 3. 0] 3. 0, 4. 0); // [2. 0 4. 0] n Accessing Elements float f = m[column][row]; float x = m[0]. x; // x component of first column vec 2 yz = m[1]. yz; // yz components of second column
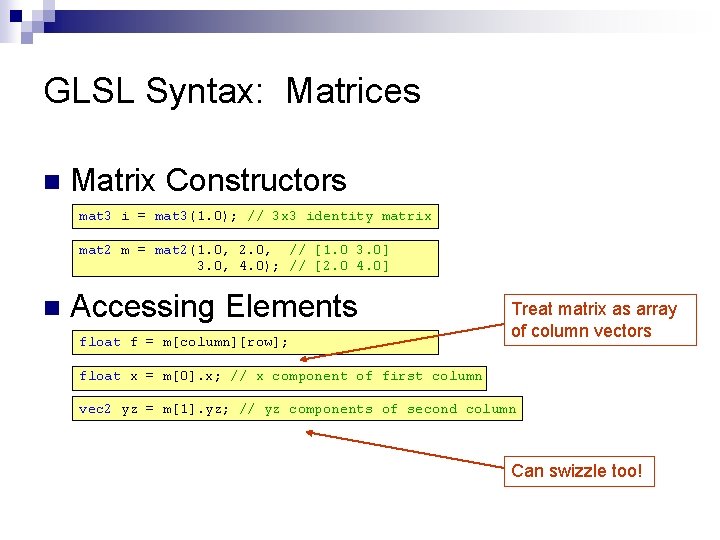
GLSL Syntax: Matrices n Matrix Constructors mat 3 i = mat 3(1. 0); // 3 x 3 identity matrix mat 2 m = mat 2(1. 0, 2. 0, // [1. 0 3. 0] 3. 0, 4. 0); // [2. 0 4. 0] n Accessing Elements float f = m[column][row]; Treat matrix as array of column vectors float x = m[0]. x; // x component of first column vec 2 yz = m[1]. yz; // yz components of second column Can swizzle too!
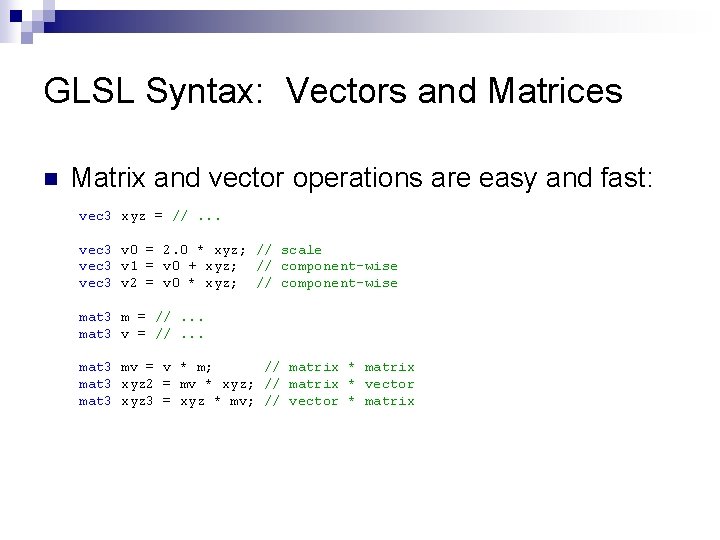
GLSL Syntax: Vectors and Matrices n Matrix and vector operations are easy and fast: vec 3 xyz = //. . . vec 3 v 0 = 2. 0 * xyz; // scale vec 3 v 1 = v 0 + xyz; // component-wise vec 3 v 2 = v 0 * xyz; // component-wise mat 3 m = //. . . mat 3 v = //. . . mat 3 mv = v * m; // matrix * matrix mat 3 xyz 2 = mv * xyz; // matrix * vector mat 3 xyz 3 = xyz * mv; // vector * matrix
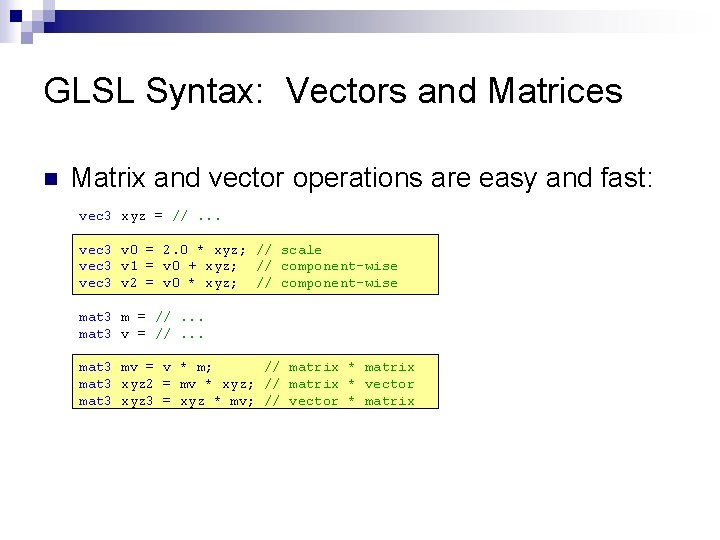
GLSL Syntax: Vectors and Matrices n Matrix and vector operations are easy and fast: vec 3 xyz = //. . . vec 3 v 0 = 2. 0 * xyz; // scale vec 3 v 1 = v 0 + xyz; // component-wise vec 3 v 2 = v 0 * xyz; // component-wise mat 3 m = //. . . mat 3 v = //. . . mat 3 mv = v * m; // matrix * matrix mat 3 xyz 2 = mv * xyz; // matrix * vector mat 3 xyz 3 = xyz * mv; // vector * matrix
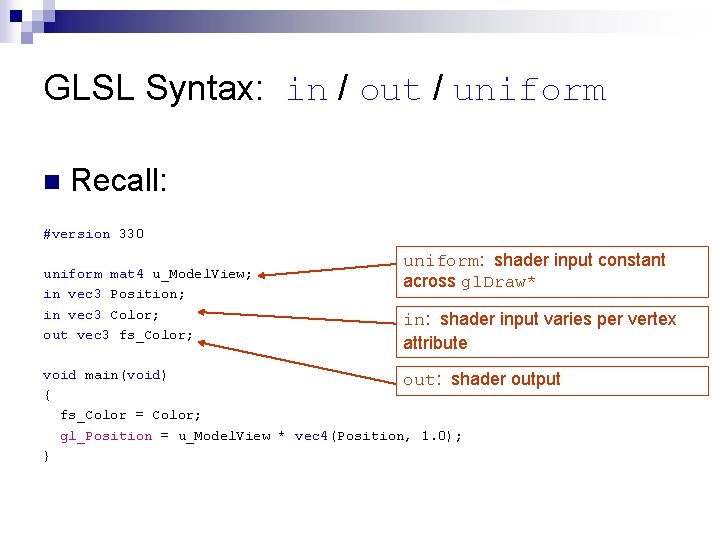
GLSL Syntax: in / out / uniform n Recall: #version 330 uniform mat 4 u_Model. View; in vec 3 Position; in vec 3 Color; out vec 3 fs_Color; uniform: shader input constant across gl. Draw* in: shader input varies per vertex attribute void main(void) out: shader { fs_Color = Color; gl_Position = u_Model. View * vec 4(Position, 1. 0); } output
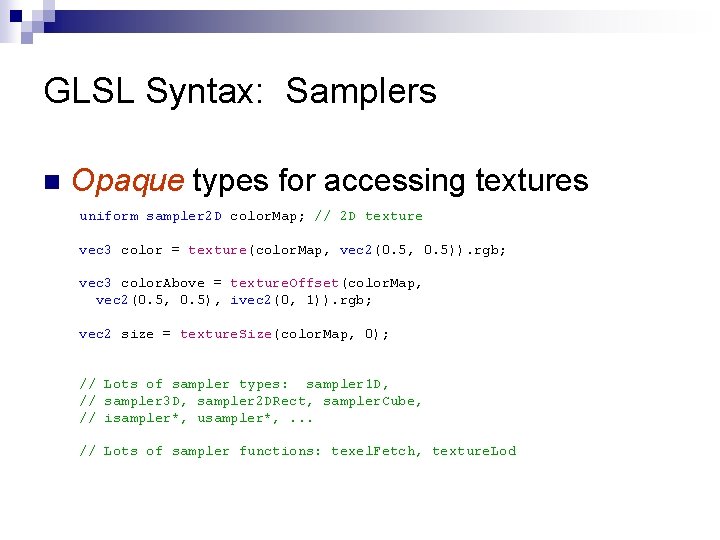
GLSL Syntax: Samplers n Opaque types for accessing textures uniform sampler 2 D color. Map; // 2 D texture vec 3 color = texture(color. Map, vec 2(0. 5, 0. 5)). rgb; vec 3 color. Above = texture. Offset(color. Map, vec 2(0. 5, 0. 5), ivec 2(0, 1)). rgb; vec 2 size = texture. Size(color. Map, 0); // Lots of sampler types: sampler 1 D, // sampler 3 D, sampler 2 DRect, sampler. Cube, // isampler*, usampler*, . . . // Lots of sampler functions: texel. Fetch, texture. Lod
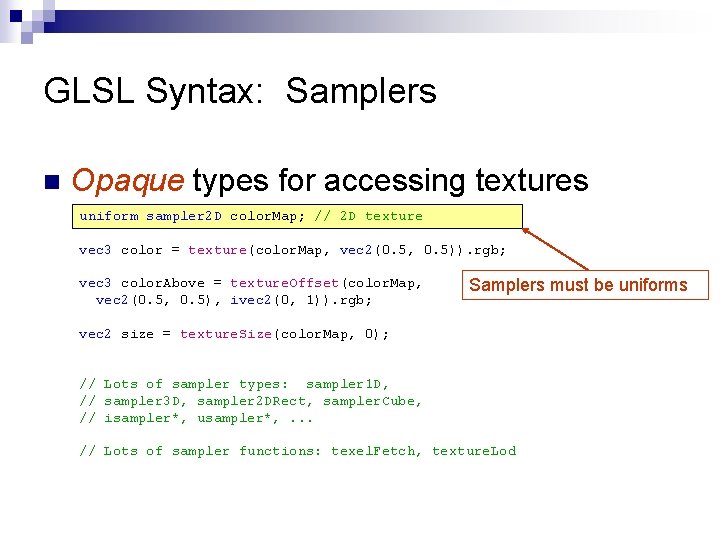
GLSL Syntax: Samplers n Opaque types for accessing textures uniform sampler 2 D color. Map; // 2 D texture vec 3 color = texture(color. Map, vec 2(0. 5, 0. 5)). rgb; vec 3 color. Above = texture. Offset(color. Map, vec 2(0. 5, 0. 5), ivec 2(0, 1)). rgb; Samplers must be uniforms vec 2 size = texture. Size(color. Map, 0); // Lots of sampler types: sampler 1 D, // sampler 3 D, sampler 2 DRect, sampler. Cube, // isampler*, usampler*, . . . // Lots of sampler functions: texel. Fetch, texture. Lod
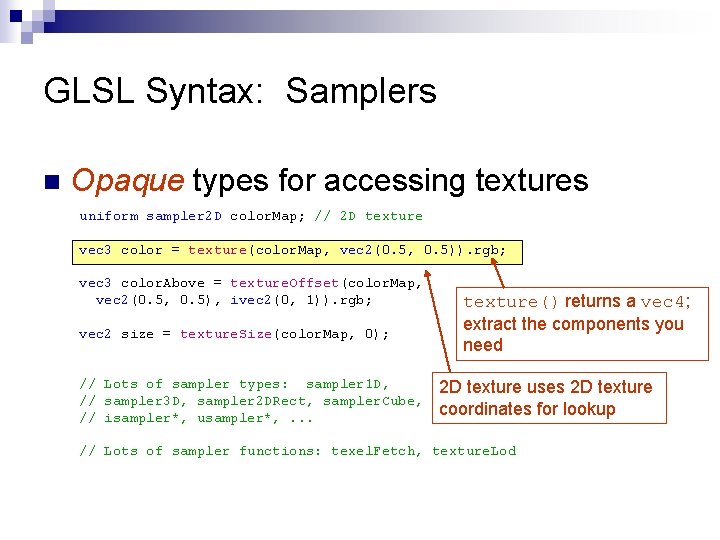
GLSL Syntax: Samplers n Opaque types for accessing textures uniform sampler 2 D color. Map; // 2 D texture vec 3 color = texture(color. Map, vec 2(0. 5, 0. 5)). rgb; vec 3 color. Above = texture. Offset(color. Map, vec 2(0. 5, 0. 5), ivec 2(0, 1)). rgb; vec 2 size = texture. Size(color. Map, 0); // Lots of sampler types: sampler 1 D, // sampler 3 D, sampler 2 DRect, sampler. Cube, // isampler*, usampler*, . . . texture() returns a vec 4; extract the components you need 2 D texture uses 2 D texture coordinates for lookup // Lots of sampler functions: texel. Fetch, texture. Lod
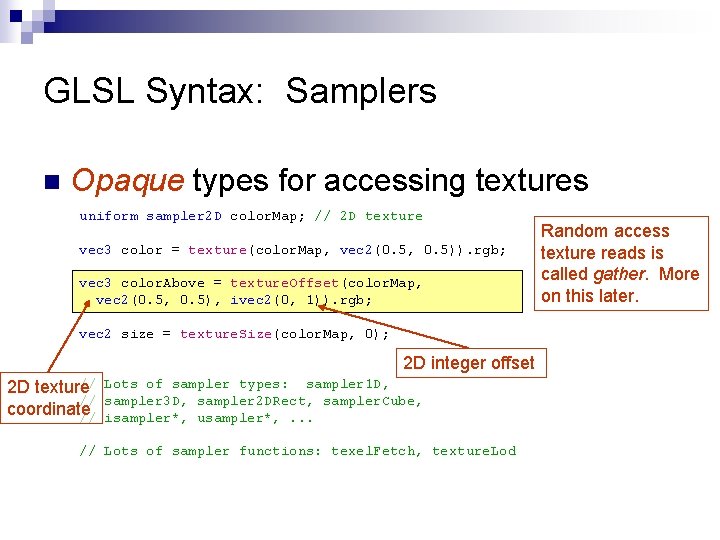
GLSL Syntax: Samplers n Opaque types for accessing textures uniform sampler 2 D color. Map; // 2 D texture vec 3 color = texture(color. Map, vec 2(0. 5, 0. 5)). rgb; vec 3 color. Above = texture. Offset(color. Map, vec 2(0. 5, 0. 5), ivec 2(0, 1)). rgb; vec 2 size = texture. Size(color. Map, 0); 2 D integer offset // 2 D texture // coordinate // Lots of sampler types: sampler 1 D, sampler 3 D, sampler 2 DRect, sampler. Cube, isampler*, usampler*, . . . // Lots of sampler functions: texel. Fetch, texture. Lod Random access texture reads is called gather. More on this later.
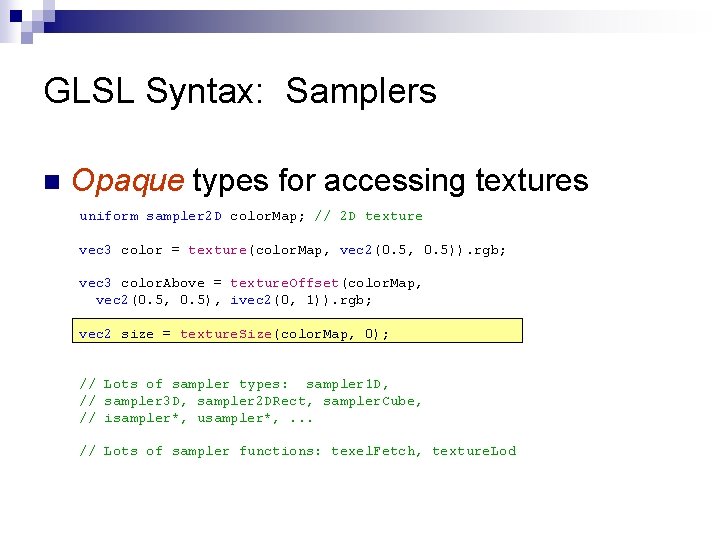
GLSL Syntax: Samplers n Opaque types for accessing textures uniform sampler 2 D color. Map; // 2 D texture vec 3 color = texture(color. Map, vec 2(0. 5, 0. 5)). rgb; vec 3 color. Above = texture. Offset(color. Map, vec 2(0. 5, 0. 5), ivec 2(0, 1)). rgb; vec 2 size = texture. Size(color. Map, 0); // Lots of sampler types: sampler 1 D, // sampler 3 D, sampler 2 DRect, sampler. Cube, // isampler*, usampler*, . . . // Lots of sampler functions: texel. Fetch, texture. Lod
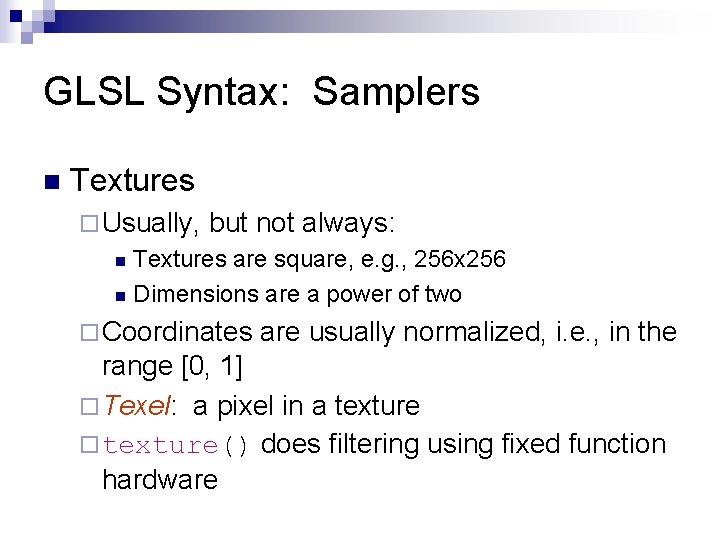
GLSL Syntax: Samplers n Textures ¨ Usually, but not always: Textures are square, e. g. , 256 x 256 n Dimensions are a power of two n ¨ Coordinates are usually normalized, i. e. , in the range [0, 1] ¨ Texel: a pixel in a texture ¨ texture() does filtering using fixed function hardware
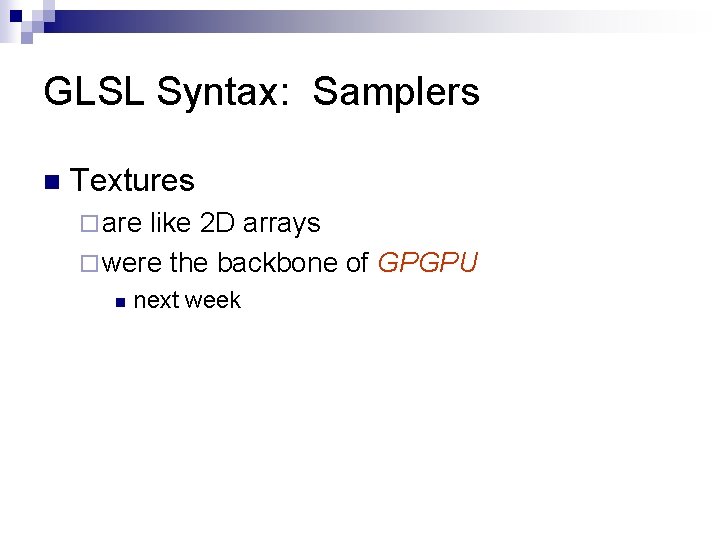
GLSL Syntax: Samplers n Textures ¨ are like 2 D arrays ¨ were the backbone of GPGPU n next week
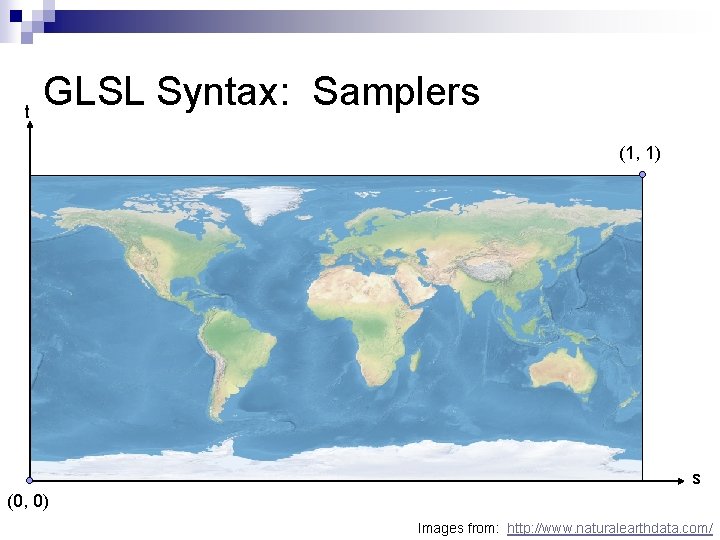
t GLSL Syntax: Samplers (1, 1) s (0, 0) Images from: http: //www. naturalearthdata. com/
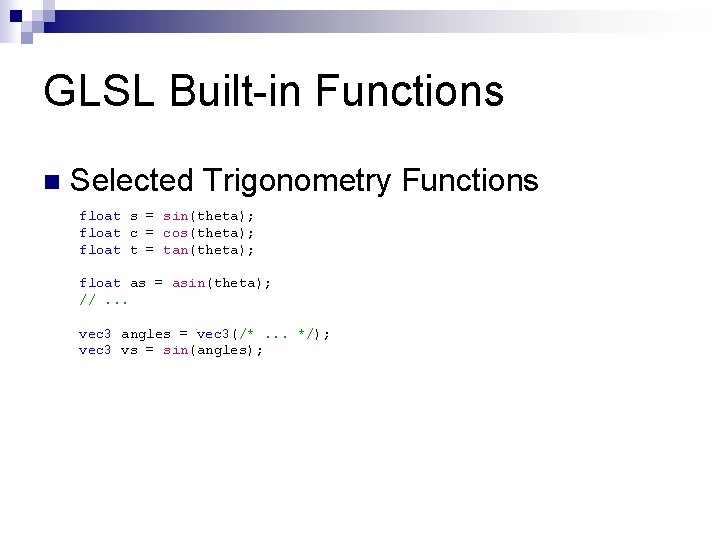
GLSL Built-in Functions n Selected Trigonometry Functions float s = sin(theta); float c = cos(theta); float t = tan(theta); float as = asin(theta); //. . . vec 3 angles = vec 3(/*. . . */); vec 3 vs = sin(angles);
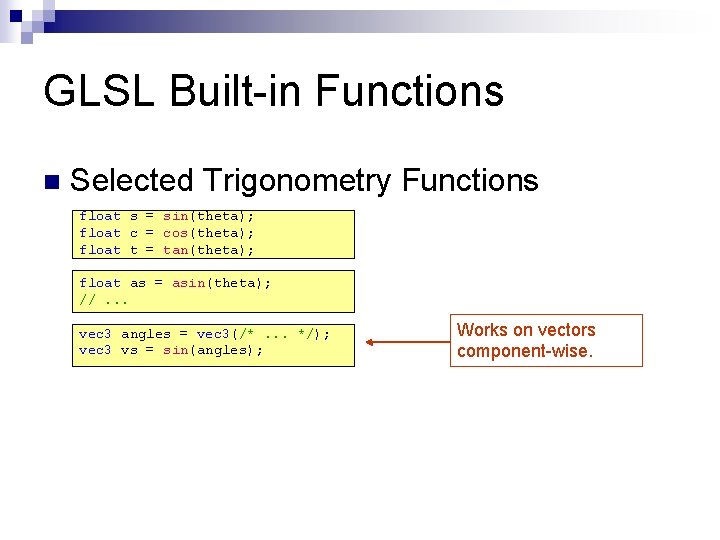
GLSL Built-in Functions n Selected Trigonometry Functions float s = sin(theta); float c = cos(theta); float t = tan(theta); float as = asin(theta); //. . . vec 3 angles = vec 3(/*. . . */); vec 3 vs = sin(angles); Works on vectors component-wise.
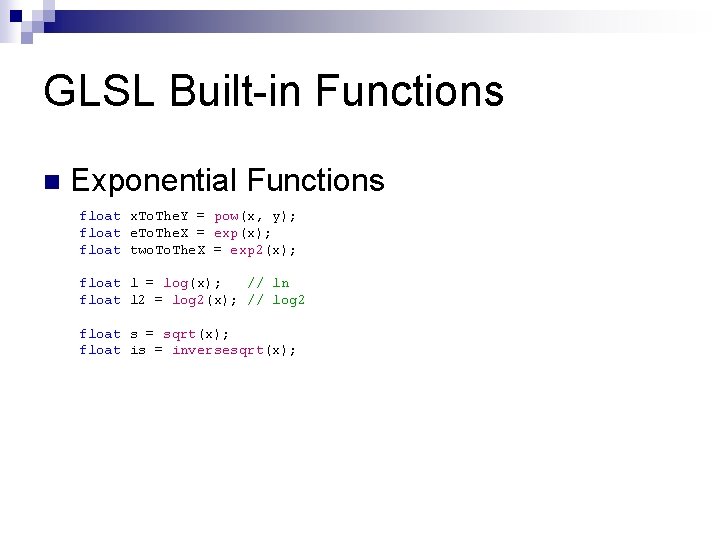
GLSL Built-in Functions n Exponential Functions float x. To. The. Y = pow(x, y); float e. To. The. X = exp(x); float two. The. X = exp 2(x); float l = log(x); // ln float l 2 = log 2(x); // log 2 float s = sqrt(x); float is = inversesqrt(x);
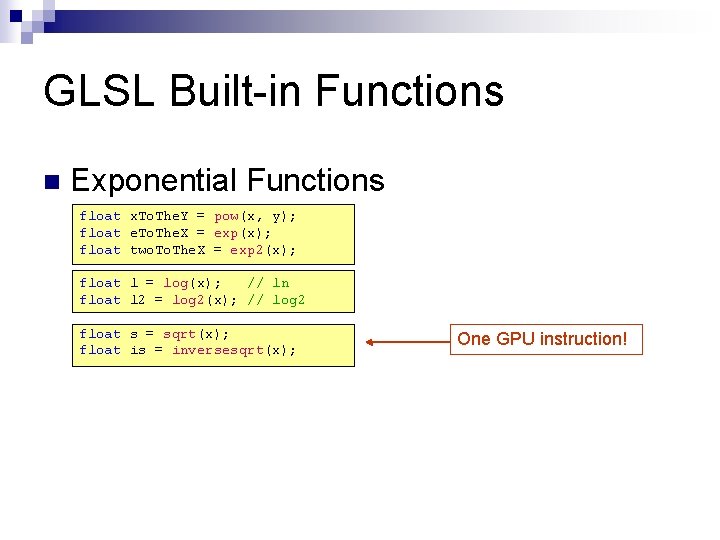
GLSL Built-in Functions n Exponential Functions float x. To. The. Y = pow(x, y); float e. To. The. X = exp(x); float two. The. X = exp 2(x); float l = log(x); // ln float l 2 = log 2(x); // log 2 float s = sqrt(x); float is = inversesqrt(x); One GPU instruction!
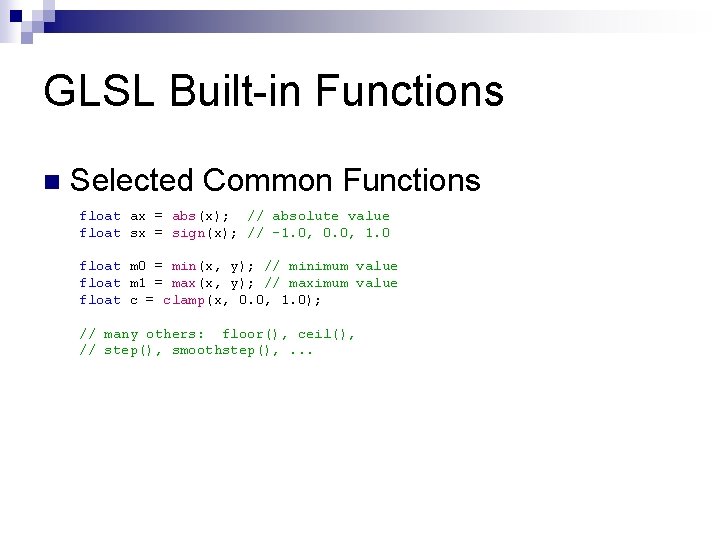
GLSL Built-in Functions n Selected Common Functions float ax = abs(x); // absolute value float sx = sign(x); // -1. 0, 0. 0, 1. 0 float m 0 = min(x, y); // minimum value float m 1 = max(x, y); // maximum value float c = clamp(x, 0. 0, 1. 0); // many others: floor(), ceil(), // step(), smoothstep(), . . .
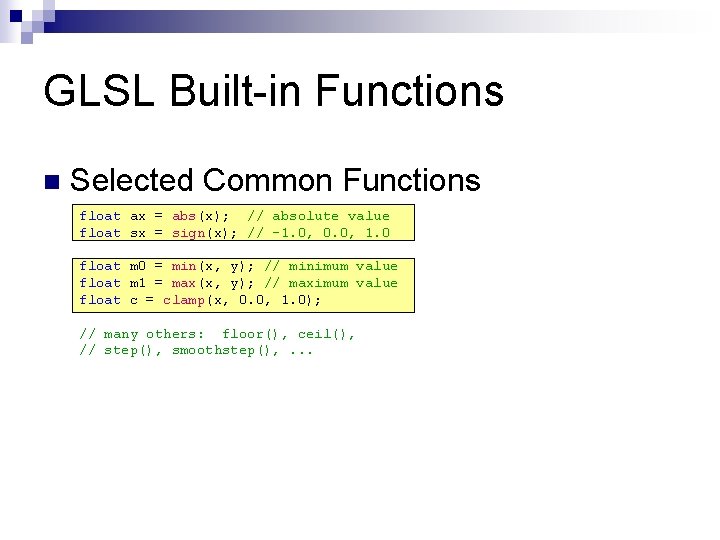
GLSL Built-in Functions n Selected Common Functions float ax = abs(x); // absolute value float sx = sign(x); // -1. 0, 0. 0, 1. 0 float m 0 = min(x, y); // minimum value float m 1 = max(x, y); // maximum value float c = clamp(x, 0. 0, 1. 0); // many others: floor(), ceil(), // step(), smoothstep(), . . .
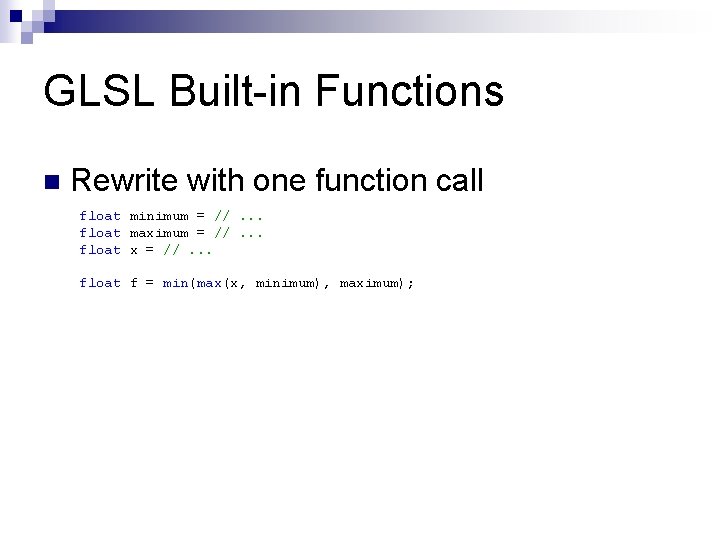
GLSL Built-in Functions n Rewrite with one function call float minimum = //. . . float maximum = //. . . float x = //. . . float f = min(max(x, minimum), maximum);
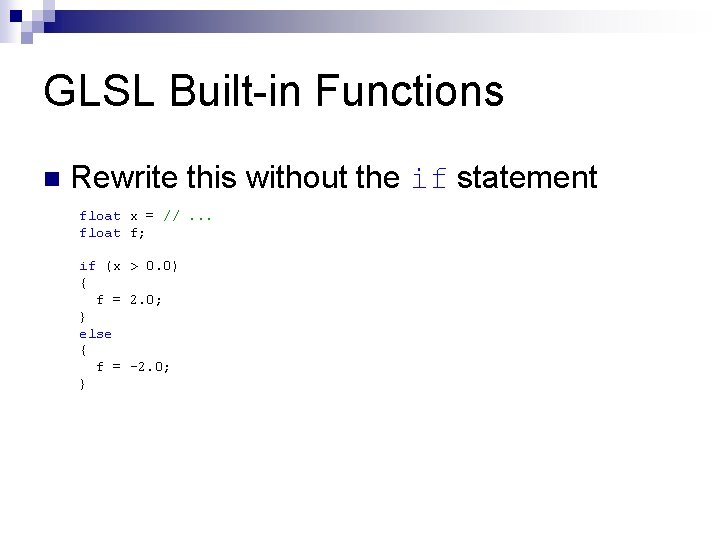
GLSL Built-in Functions n Rewrite this without the if statement float x = //. . . float f; if (x > 0. 0) { f = 2. 0; } else { f = -2. 0; }
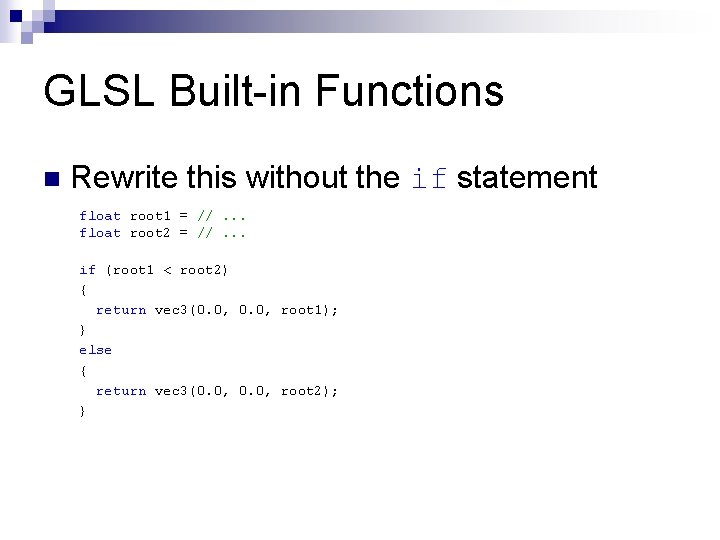
GLSL Built-in Functions n Rewrite this without the if statement float root 1 = //. . . float root 2 = //. . . if (root 1 < root 2) { return vec 3(0. 0, root 1); } else { return vec 3(0. 0, root 2); }
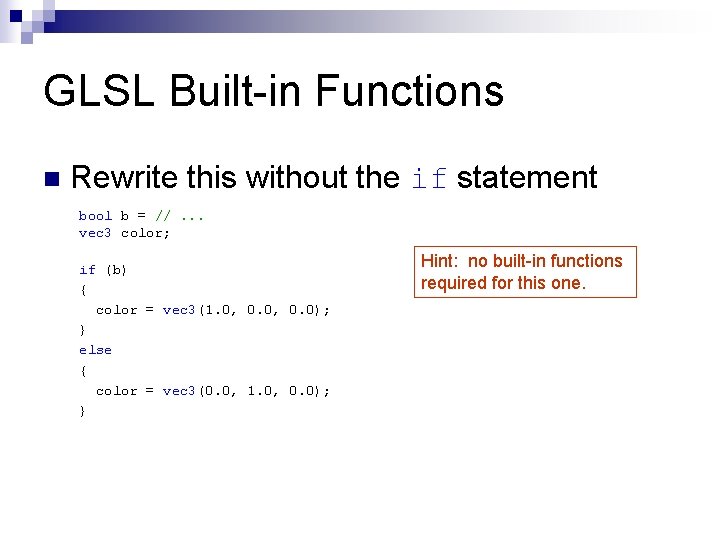
GLSL Built-in Functions n Rewrite this without the if statement bool b = //. . . vec 3 color; if (b) { color = vec 3(1. 0, 0. 0); } else { color = vec 3(0. 0, 1. 0, 0. 0); } Hint: no built-in functions required for this one.
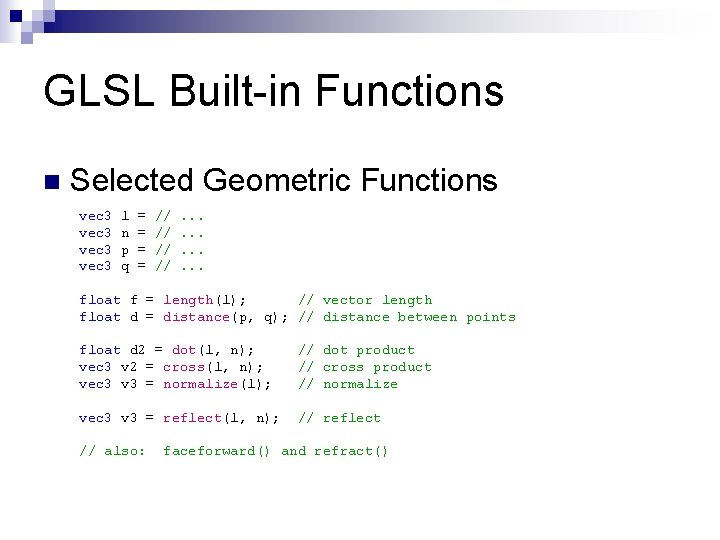
GLSL Built-in Functions n Selected Geometric Functions vec 3 l n p q = = // // . . . float f = length(l); // vector length float d = distance(p, q); // distance between points float d 2 = dot(l, n); vec 3 v 2 = cross(l, n); vec 3 v 3 = normalize(l); // dot product // cross product // normalize vec 3 v 3 = reflect(l, n); // reflect // also: faceforward() and refract()
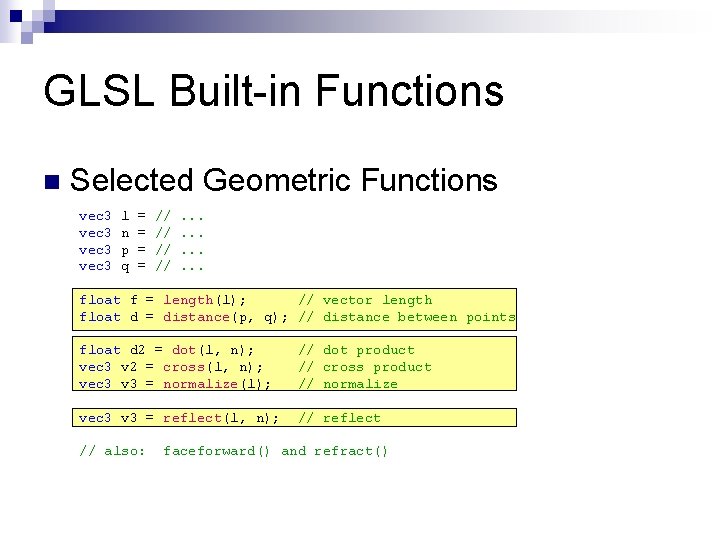
GLSL Built-in Functions n Selected Geometric Functions vec 3 l n p q = = // // . . . float f = length(l); // vector length float d = distance(p, q); // distance between points float d 2 = dot(l, n); vec 3 v 2 = cross(l, n); vec 3 v 3 = normalize(l); // dot product // cross product // normalize vec 3 v 3 = reflect(l, n); // reflect // also: faceforward() and refract()
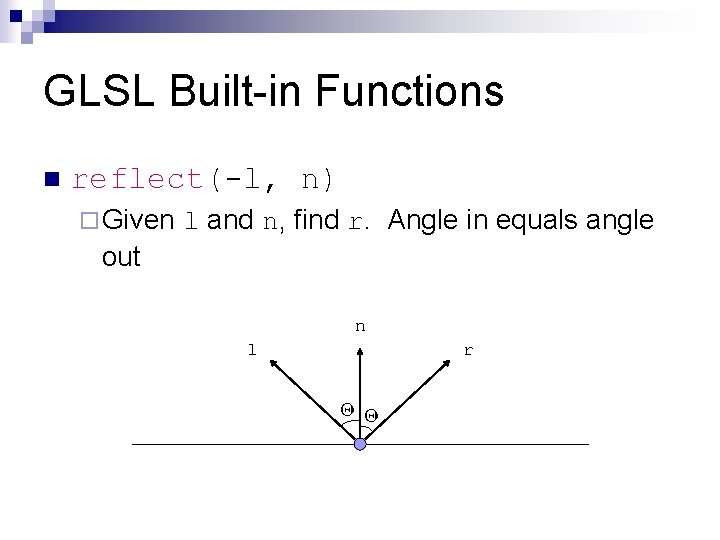
GLSL Built-in Functions n reflect(-l, n) ¨ Given l and n, find r. Angle in equals angle out n l r
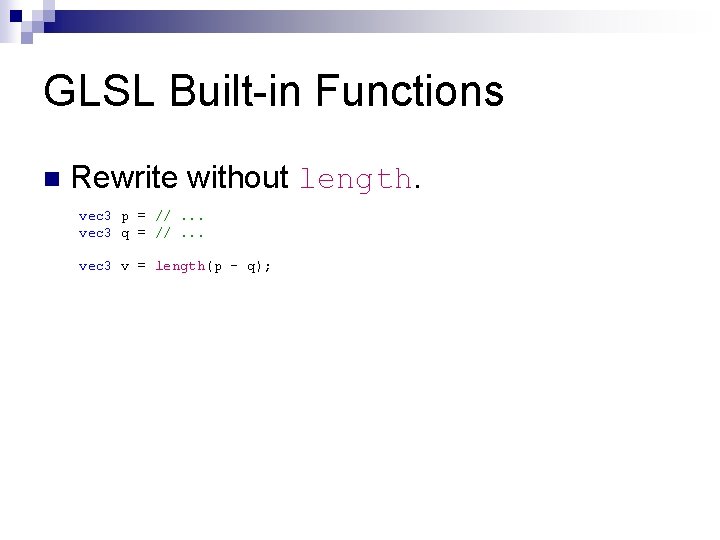
GLSL Built-in Functions n Rewrite without length. vec 3 p = //. . . vec 3 q = //. . . vec 3 v = length(p – q);
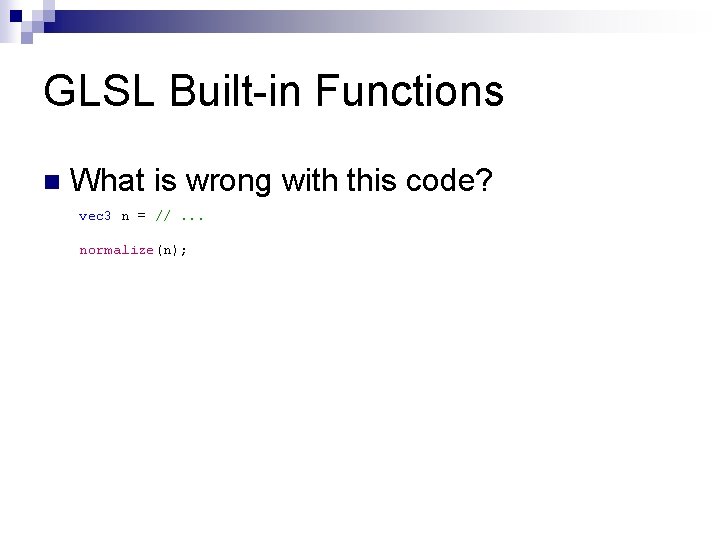
GLSL Built-in Functions n What is wrong with this code? vec 3 n = //. . . normalize(n);
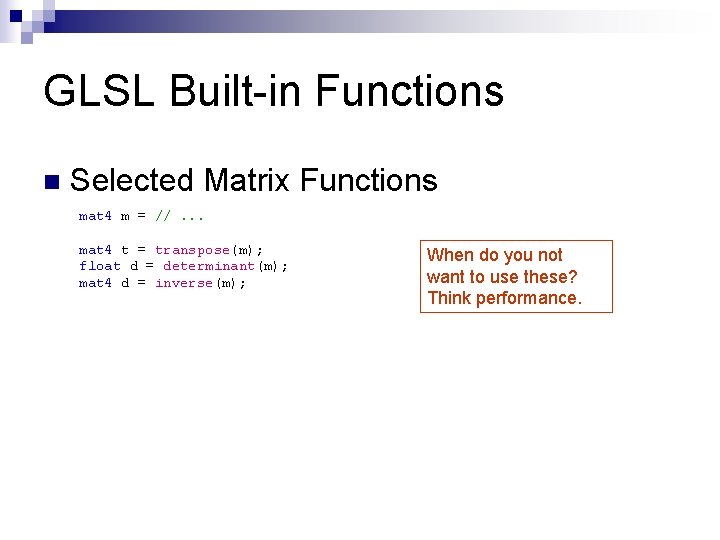
GLSL Built-in Functions n Selected Matrix Functions mat 4 m = //. . . mat 4 t = transpose(m); float d = determinant(m); mat 4 d = inverse(m); When do you not want to use these? Think performance.
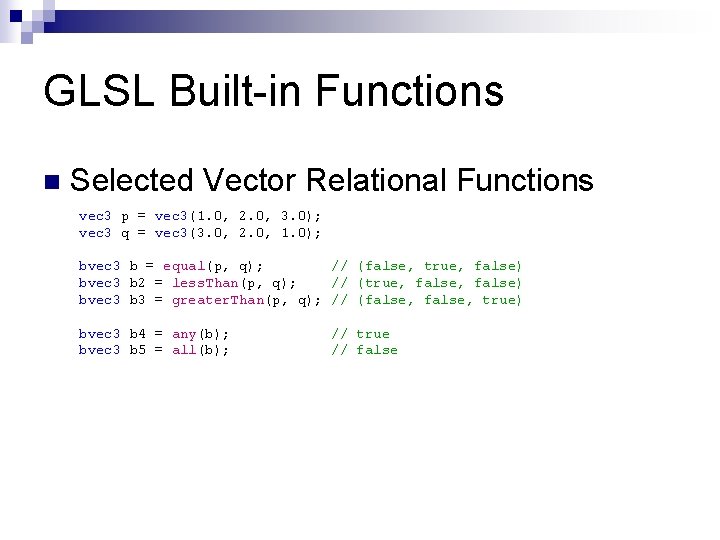
GLSL Built-in Functions n Selected Vector Relational Functions vec 3 p = vec 3(1. 0, 2. 0, 3. 0); vec 3 q = vec 3(3. 0, 2. 0, 1. 0); bvec 3 b = equal(p, q); // (false, true, false) bvec 3 b 2 = less. Than(p, q); // (true, false) bvec 3 b 3 = greater. Than(p, q); // (false, true) bvec 3 b 4 = any(b); bvec 3 b 5 = all(b); // true // false
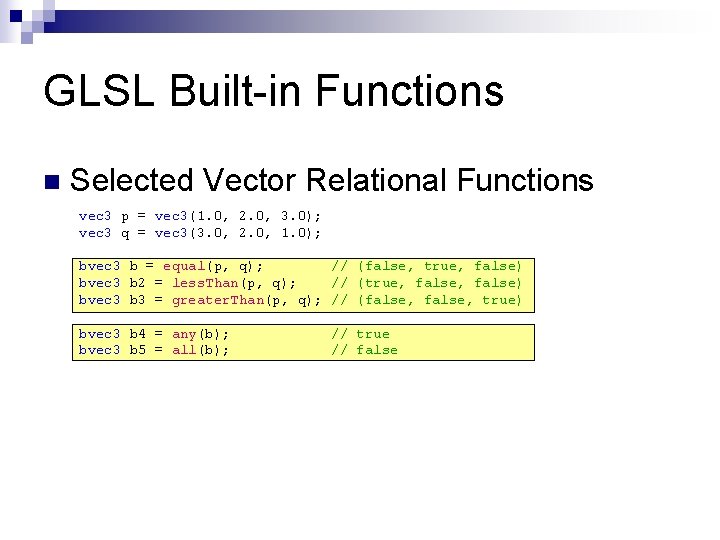
GLSL Built-in Functions n Selected Vector Relational Functions vec 3 p = vec 3(1. 0, 2. 0, 3. 0); vec 3 q = vec 3(3. 0, 2. 0, 1. 0); bvec 3 b = equal(p, q); // (false, true, false) bvec 3 b 2 = less. Than(p, q); // (true, false) bvec 3 b 3 = greater. Than(p, q); // (false, true) bvec 3 b 4 = any(b); bvec 3 b 5 = all(b); // true // false
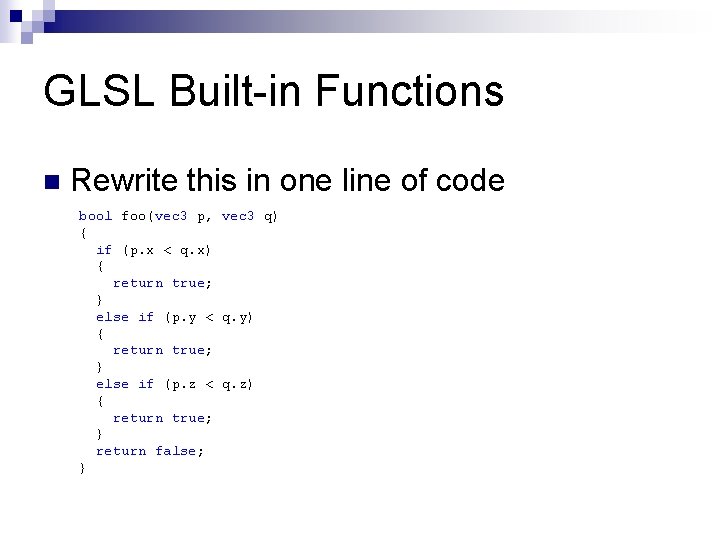
GLSL Built-in Functions n Rewrite this in one line of code bool foo(vec 3 p, vec 3 q) { if (p. x < q. x) { return true; } else if (p. y < q. y) { return true; } else if (p. z < q. z) { return true; } return false; }
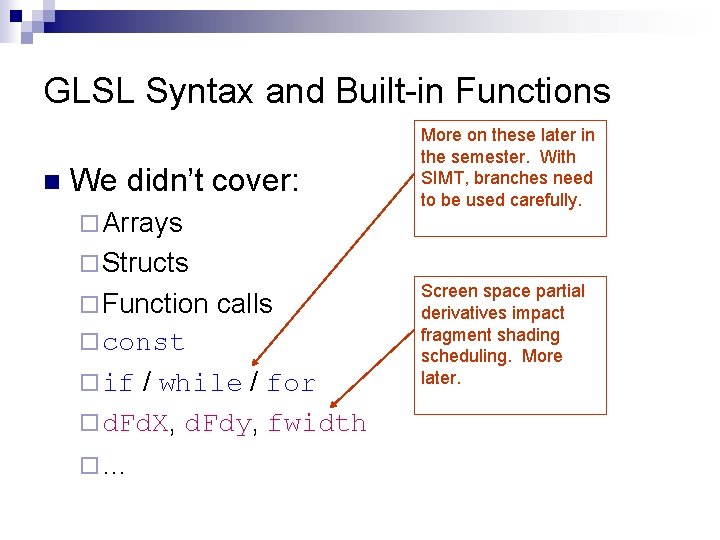
GLSL Syntax and Built-in Functions n We didn’t cover: ¨ Arrays More on these later in the semester. With SIMT, branches need to be used carefully. ¨ Structs ¨ Function calls ¨ const / while / for ¨ d. Fd. X, d. Fdy, fwidth ¨ if ¨… Screen space partial derivatives impact fragment shading scheduling. More later.
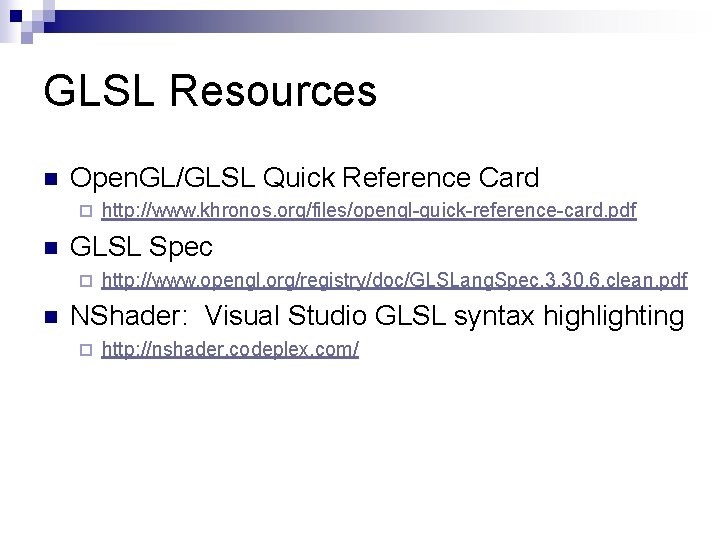
GLSL Resources n Open. GL/GLSL Quick Reference Card ¨ n GLSL Spec ¨ n http: //www. khronos. org/files/opengl-quick-reference-card. pdf http: //www. opengl. org/registry/doc/GLSLang. Spec. 3. 30. 6. clean. pdf NShader: Visual Studio GLSL syntax highlighting ¨ http: //nshader. codeplex. com/
Dr laura cozzi
Glsl asin
Dshaders
Glsl pointers
Glsl sampler
Glsl outline
Differential diagnosis for atopic dermatitis
California university of pennsylvania global online
Patrick: an introduction to medicinal chemistry 3e
Pennsylvania state plane zones
Atlantic coastal plain region pennsylvania
Rachel carson was born in 1907
Pennsylvania
Xoom energy pennsylvania
New hire reporting program
New york pennsylvania new jersey delaware
Sandata electronic visit verification
My uncle from pittsburgh pennsylvania
Bharp
Community health
Pennsylvania ecosystems
Pennsylvania dutch council
Modern real estate practice in pennsylvania 14th edition
Prescriptive easement pennsylvania
Pa forest products association
Pennsylvania association of school business officials
Pictures of quakers in pennsylvania
The southernmost part of pennsylvania was once called
Summer key for pennsylvania trees
Nfce pennsylvania
Pennsylvania
Liquor liability insurance pennsylvania
Modern real estate practice in pennsylvania
What causes stuttering
Commonwealth of pennsylvania department of public welfare
Who is in this image
Homestead pennsylvania tourism
Pennsylvania state animal response team
Pa space grant
Free radon test kit pennsylvania
John yelenic crime scene
Act 72 pennsylvania
"university of maryland university college"
Patrick biddix
Dr zeller wheaton
Patrick robbe
Mllw meaning
Patrick nolan amway
Patrick barrie
Npro
Saint patrick day maestra lidia
Patrick wendell net worth
Patrick fuhrmann
Saint patrick day symbols
Intolerable acts simple definition
Patrick wendell
Patrick wendell
Databricks patrick wendell
Patrick durner
Patrick rang
Patrick bosshart
Patrick is designing a large
Skrzynecki pronunciation
Patrick nunes leite
Rouwcyclus
Patrick sze
In memory of my mother poetic techniques
The direct audience of patrick henry's speech was ___.
Parallel structure in speech to the virginia convention
Obra literaria el perfume
Patrick henry airport
Oyston v st patrick’s college
Slax builder
Patrick brochet
Patrick loquet
Faber ve fadir testi
Why is patrick henry important