Inheritance and Class Hierarchies Chapter 3 Inheritance and
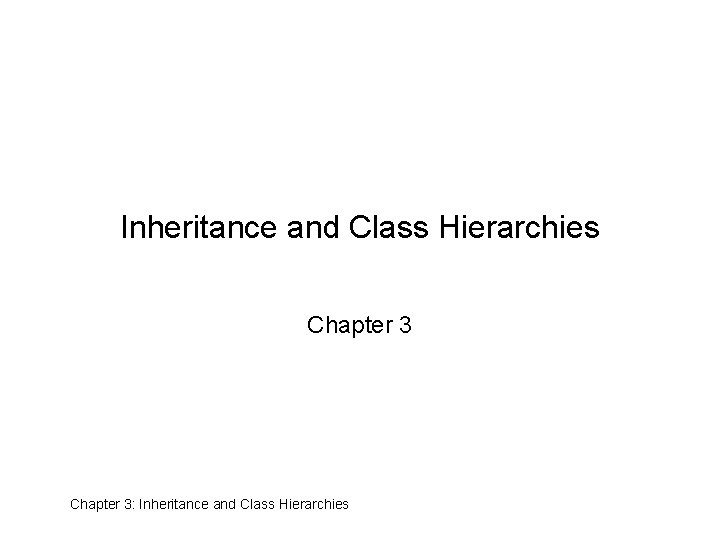
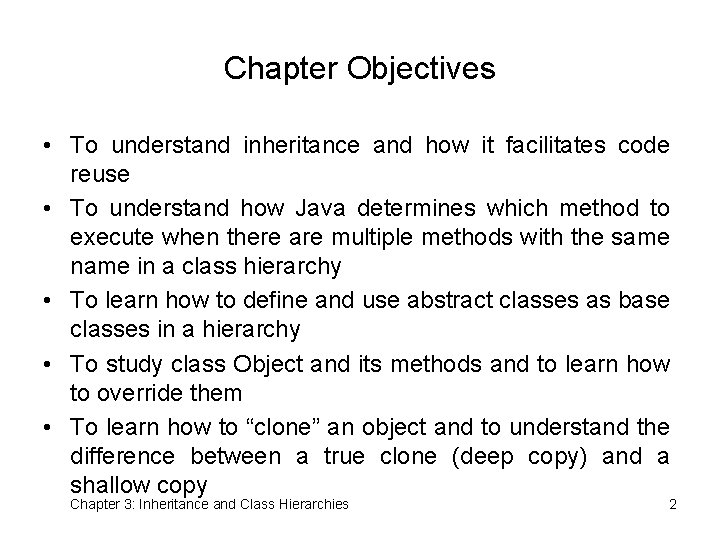
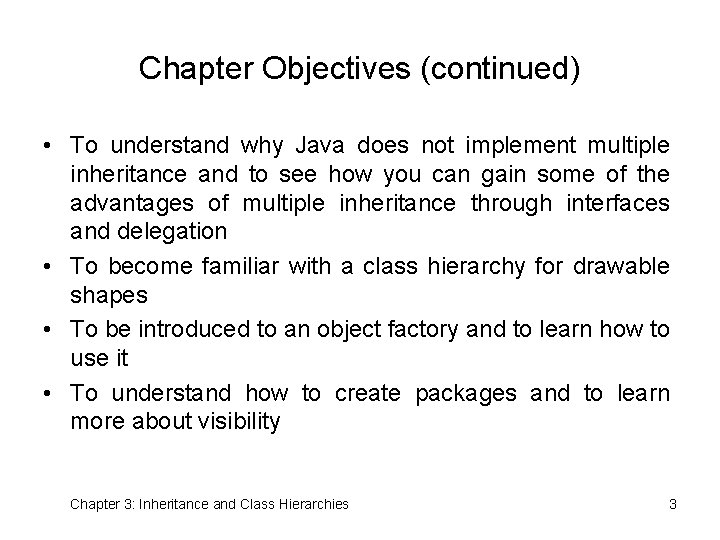
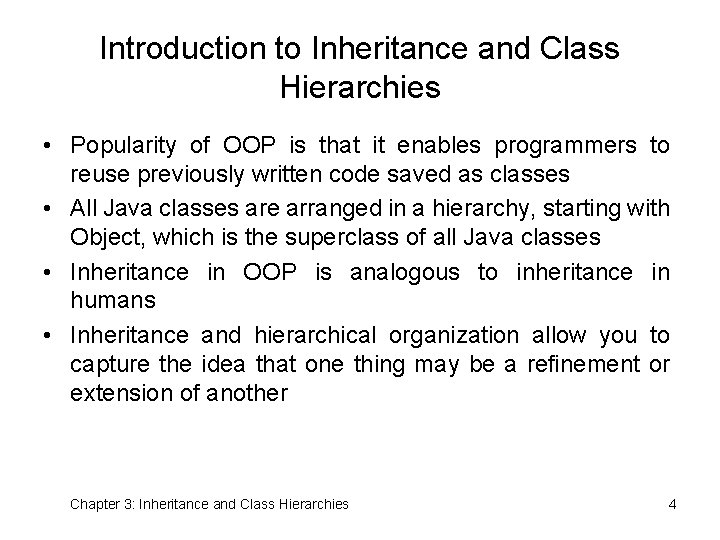
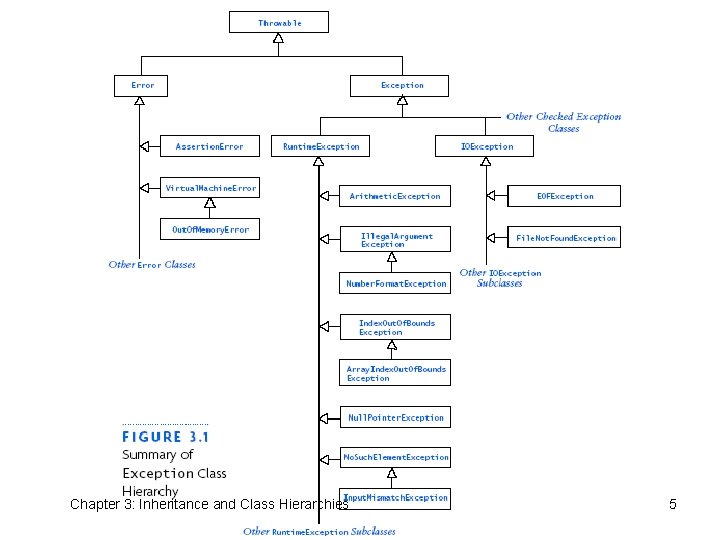
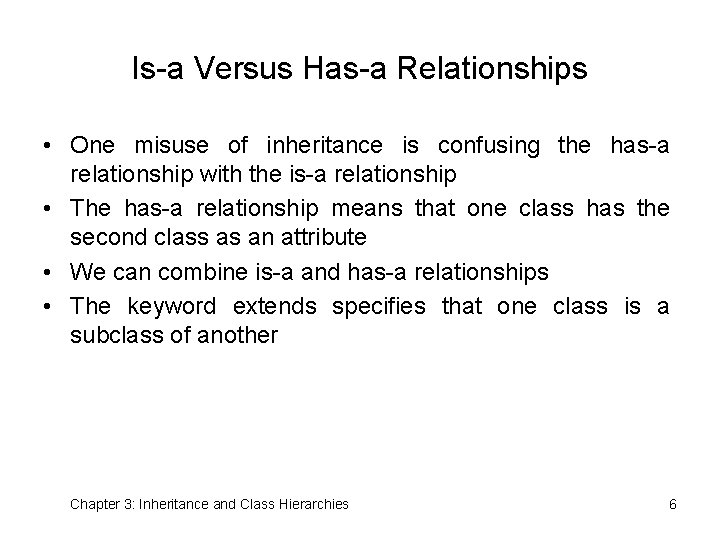
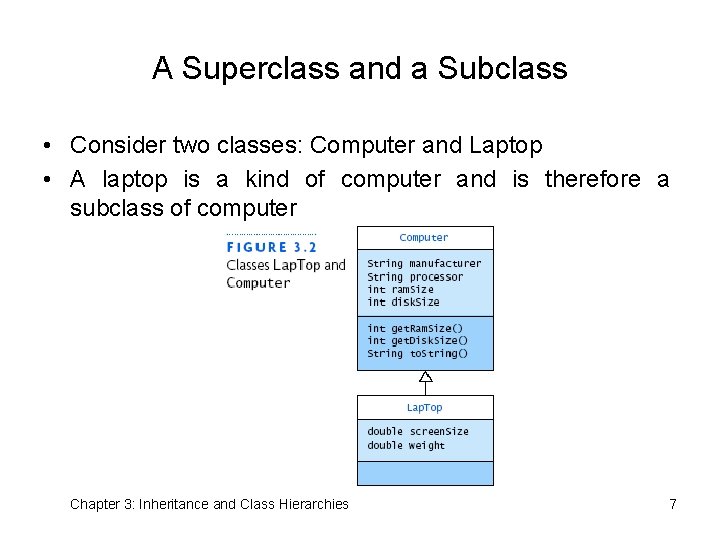
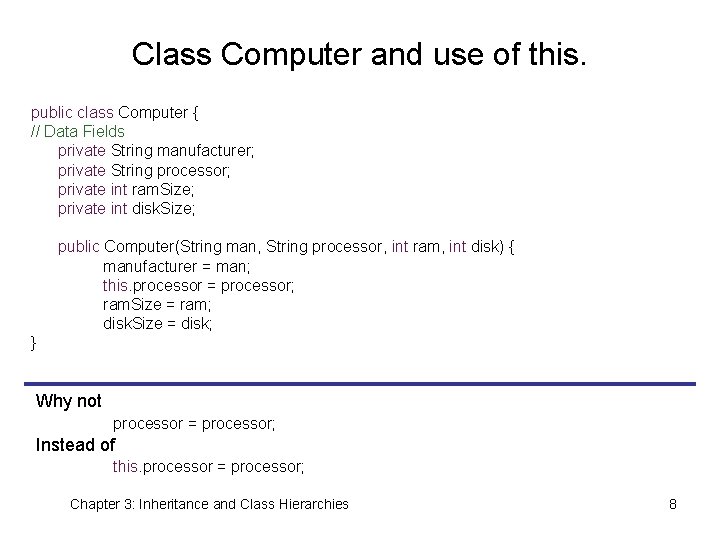
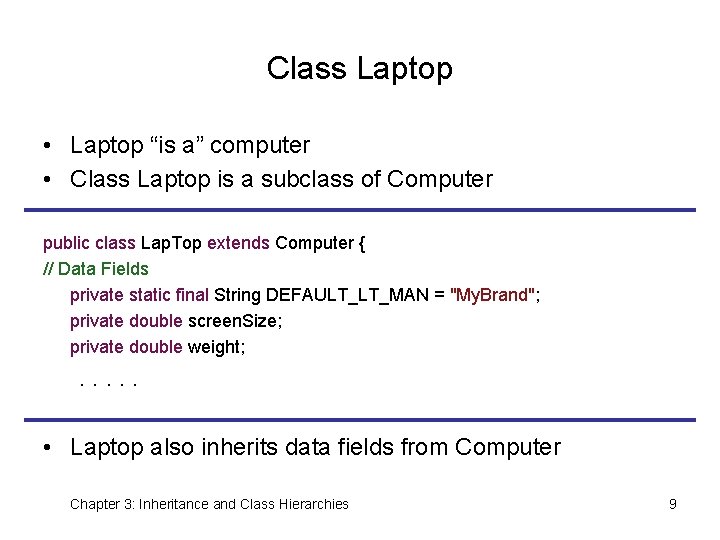
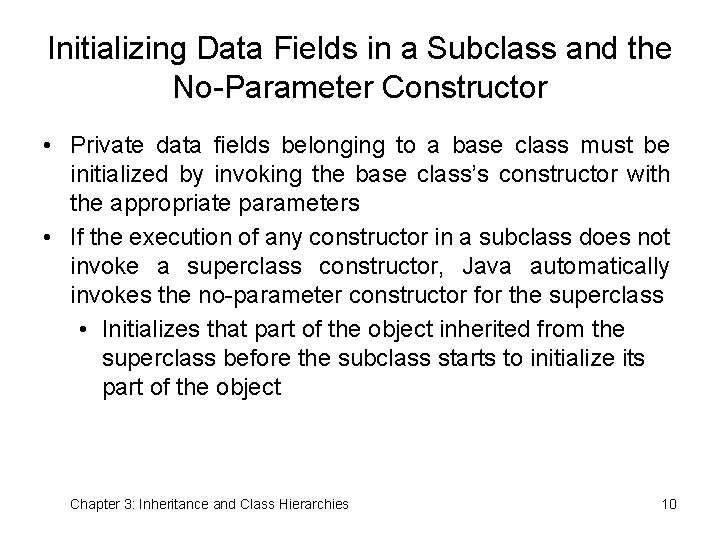
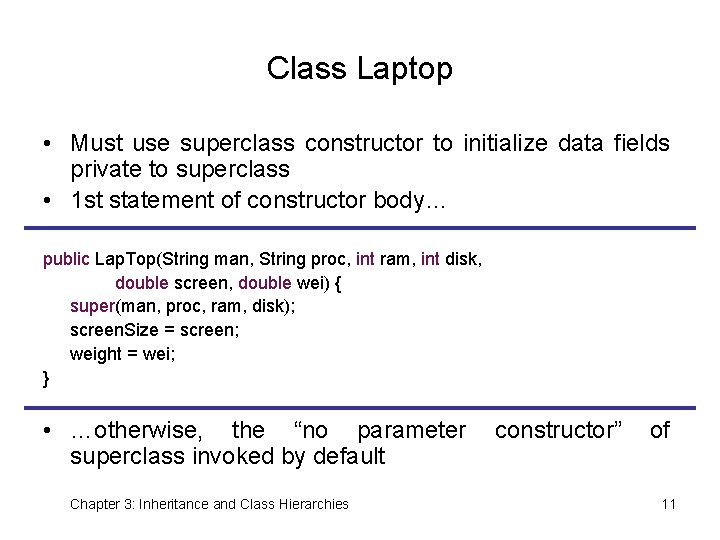
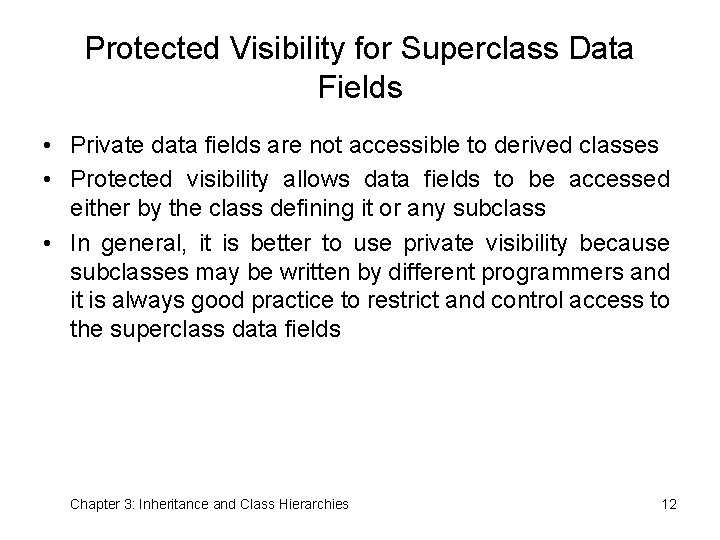
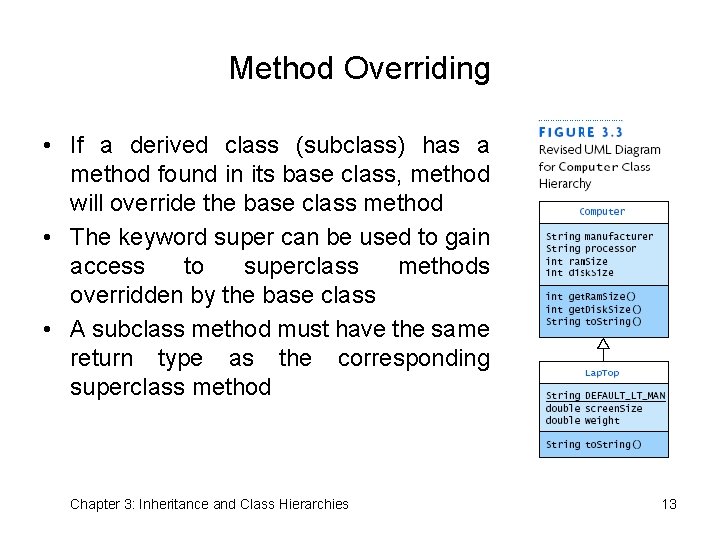
![Method Overriding • Suppose main is… public static void main(String[] args) { Computer my. Method Overriding • Suppose main is… public static void main(String[] args) { Computer my.](https://slidetodoc.com/presentation_image_h2/a7a9ccab65cb1a09d50620d7fc5a5c12/image-14.jpg)
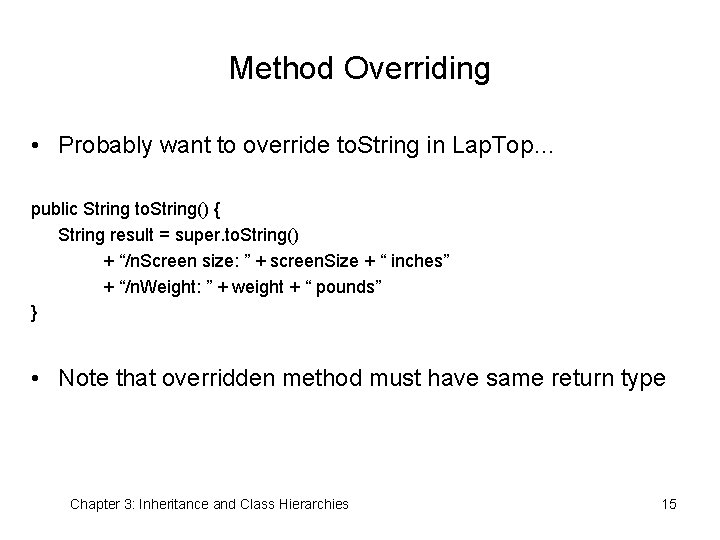
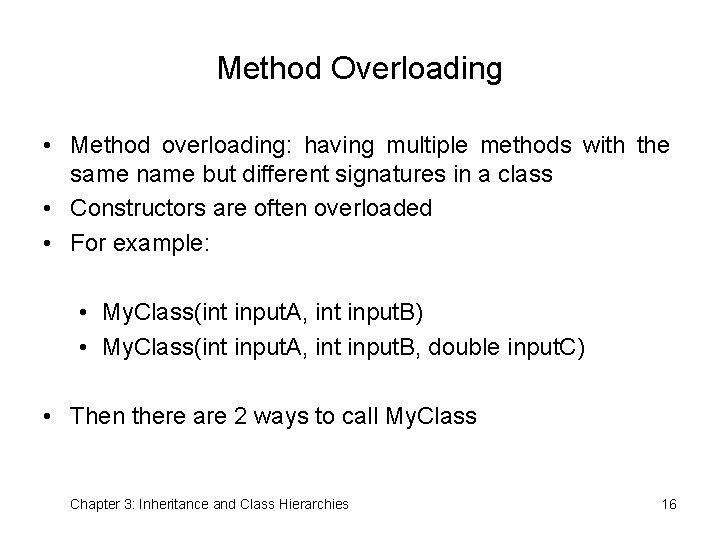
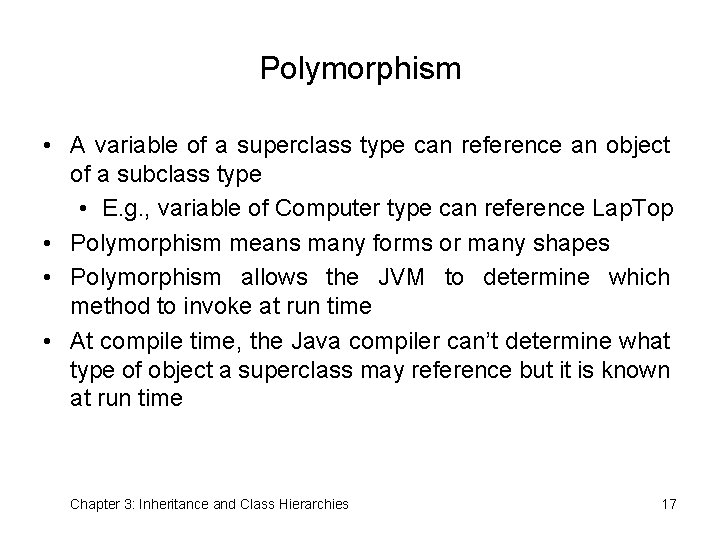
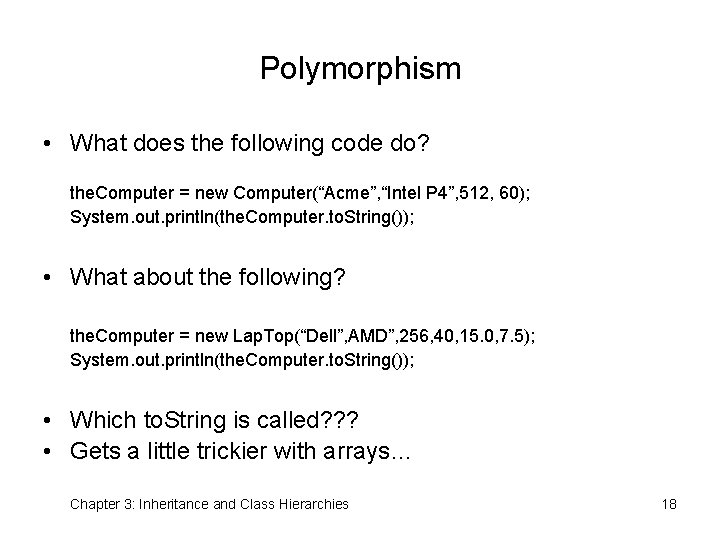
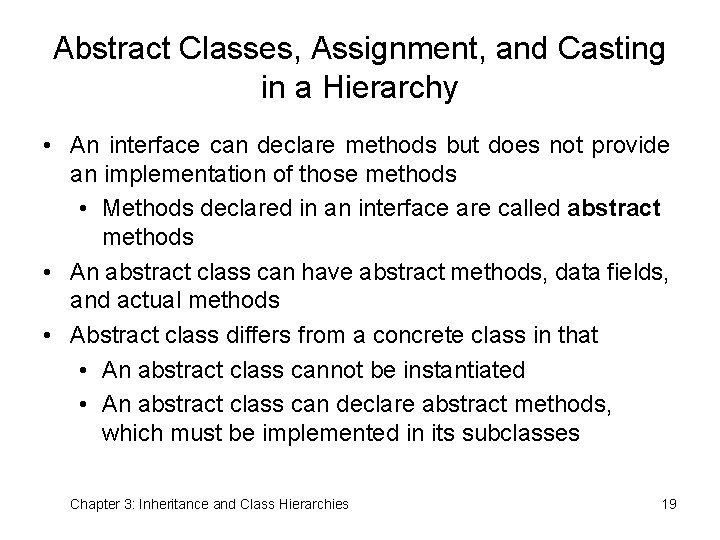
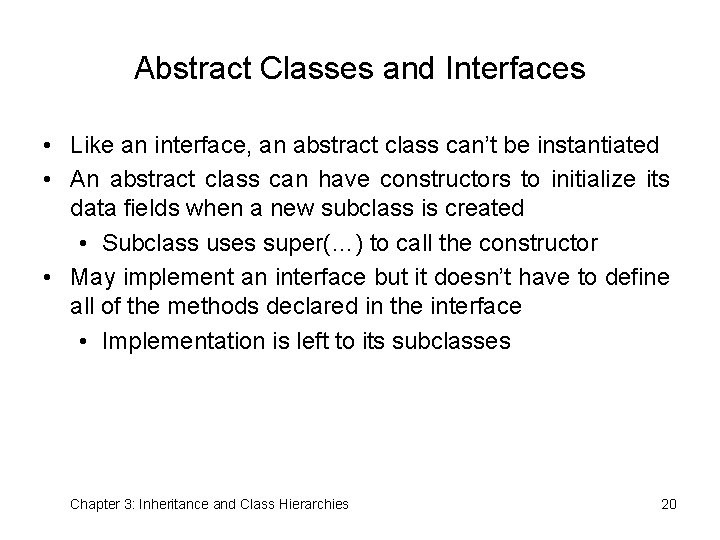
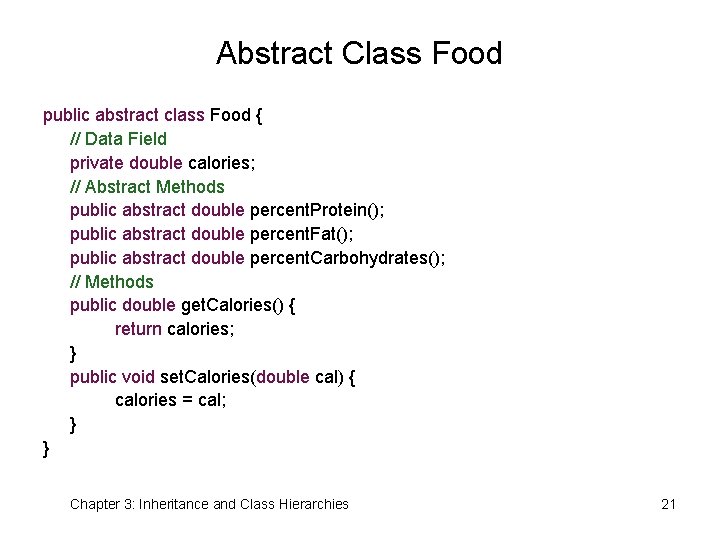
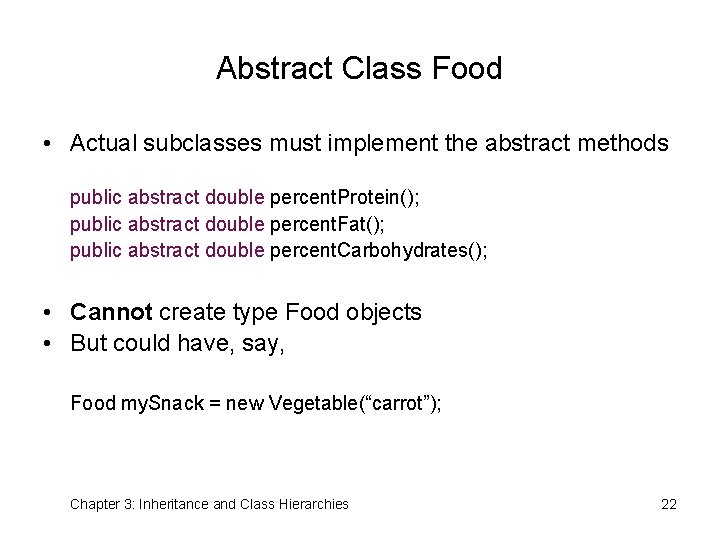
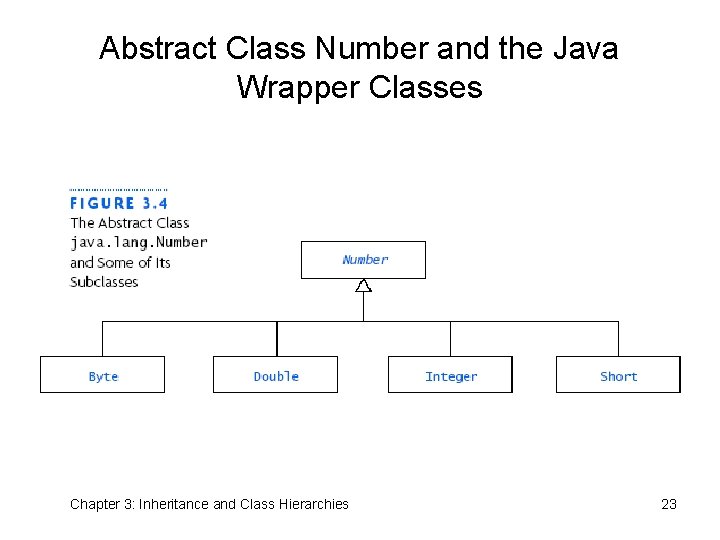
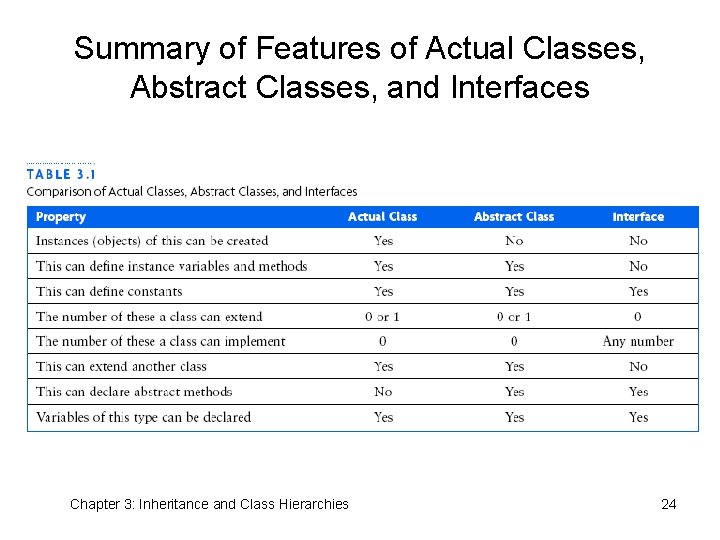
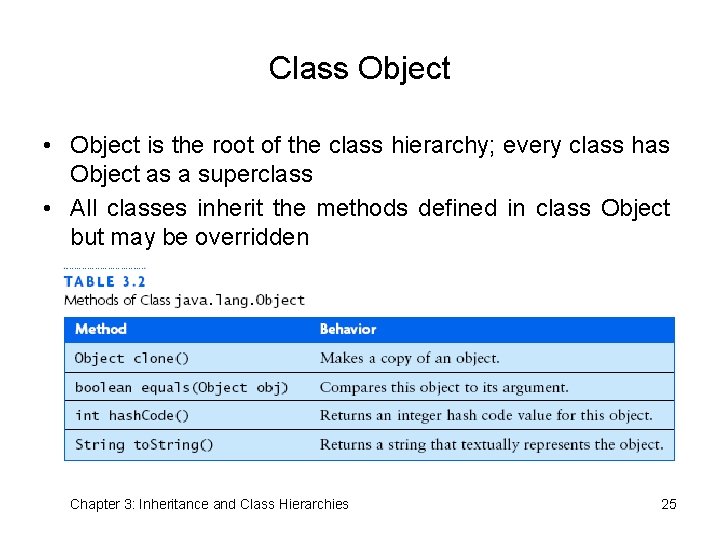
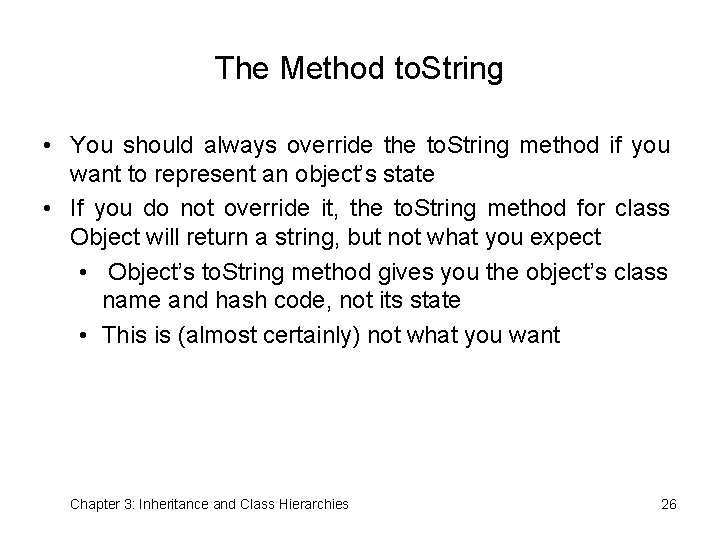
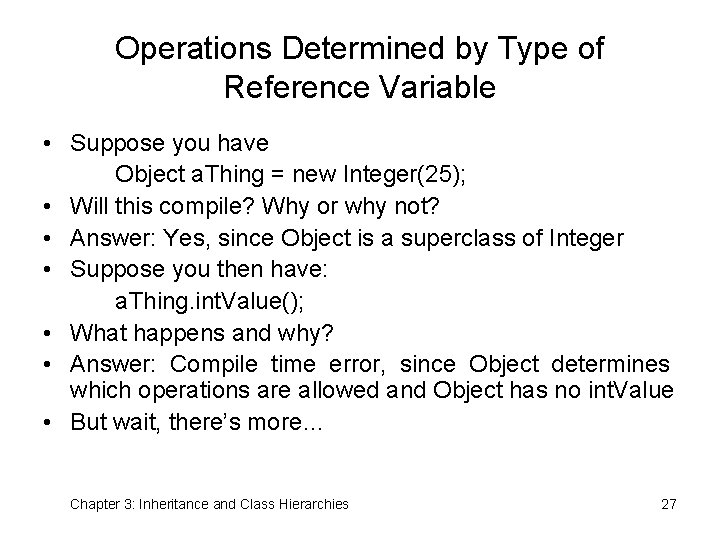
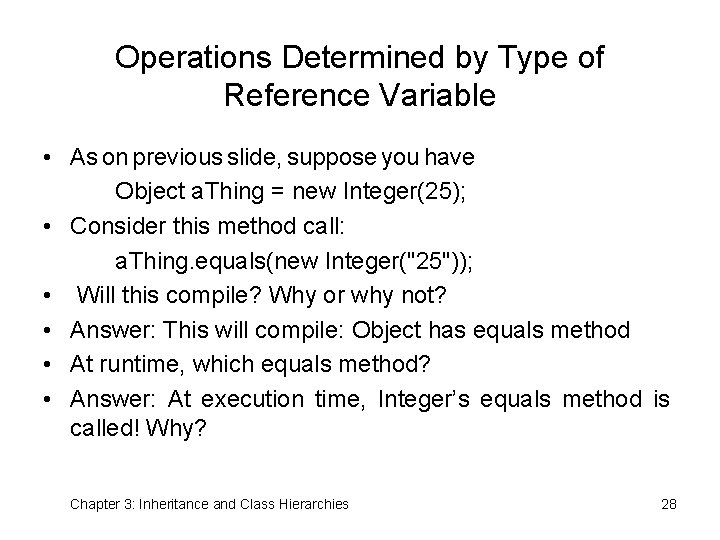
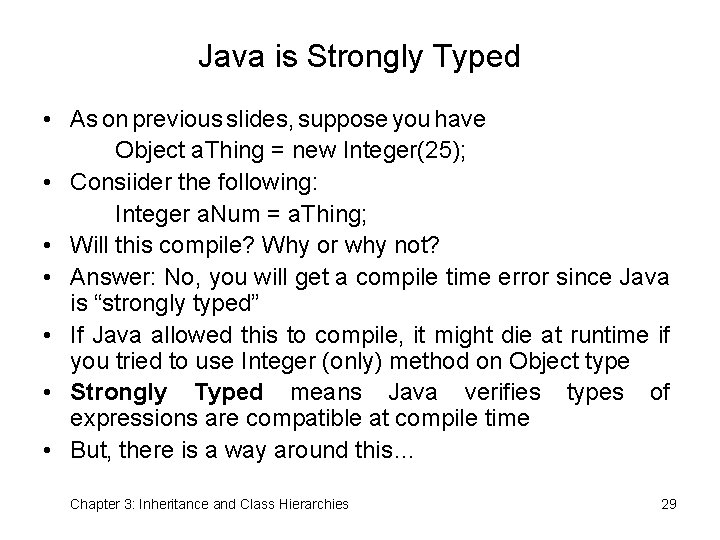
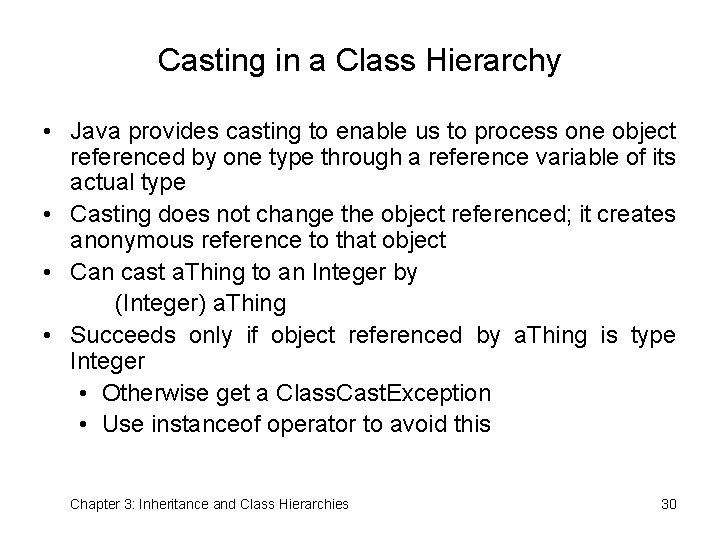
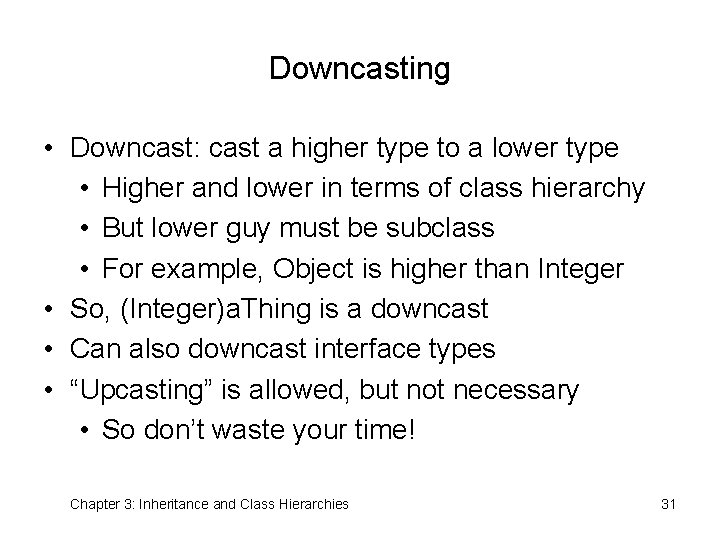
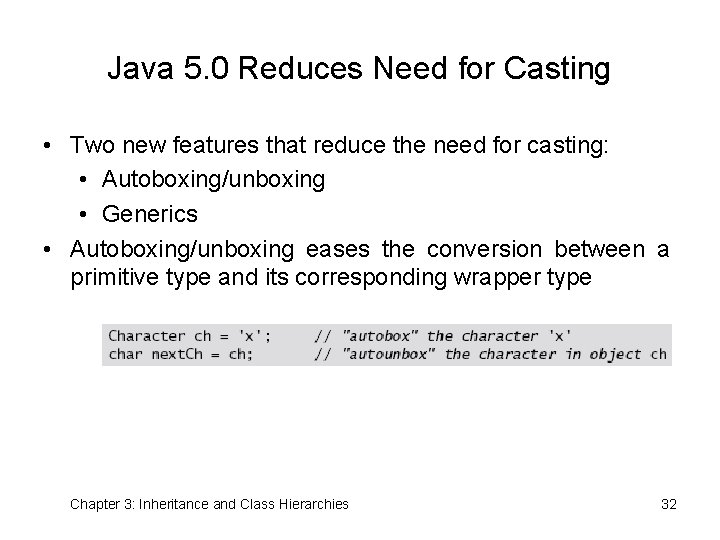
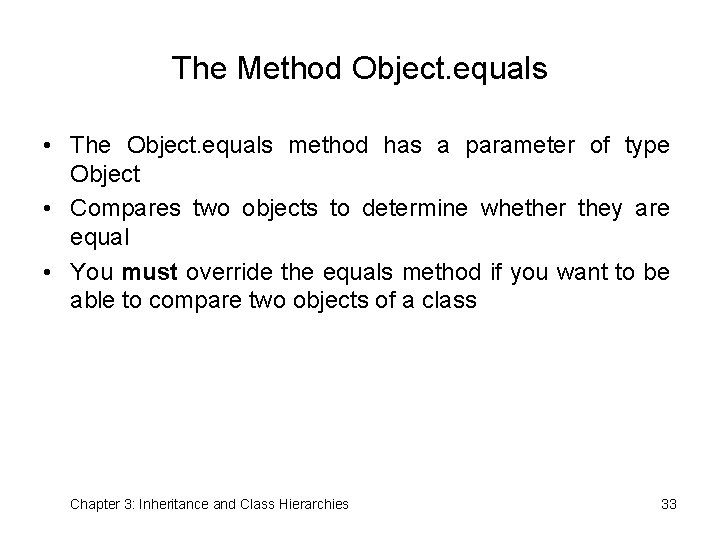
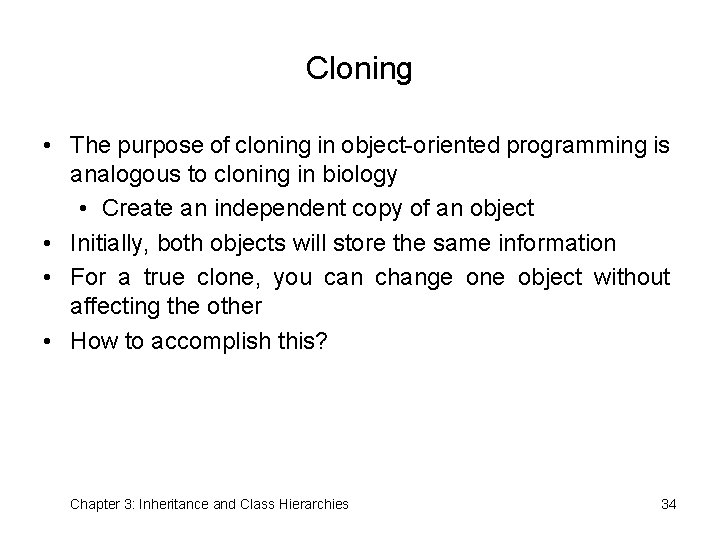
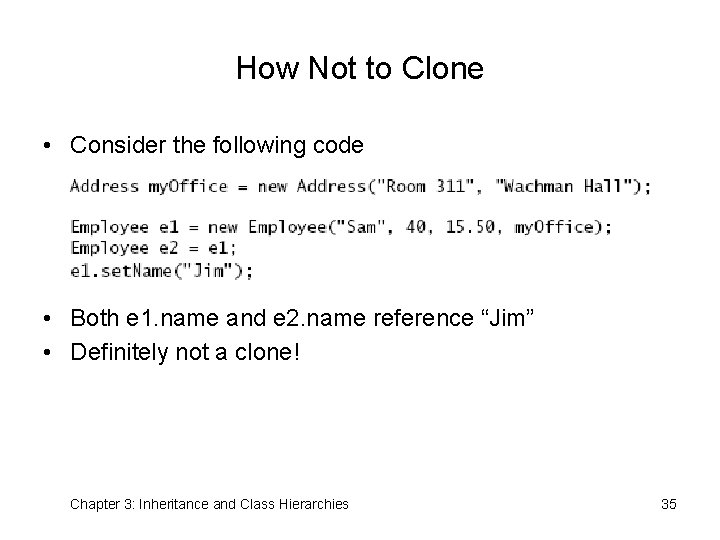
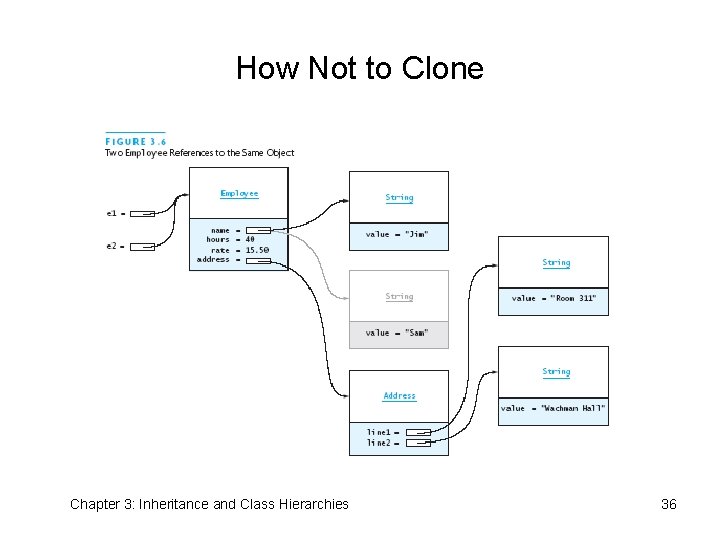
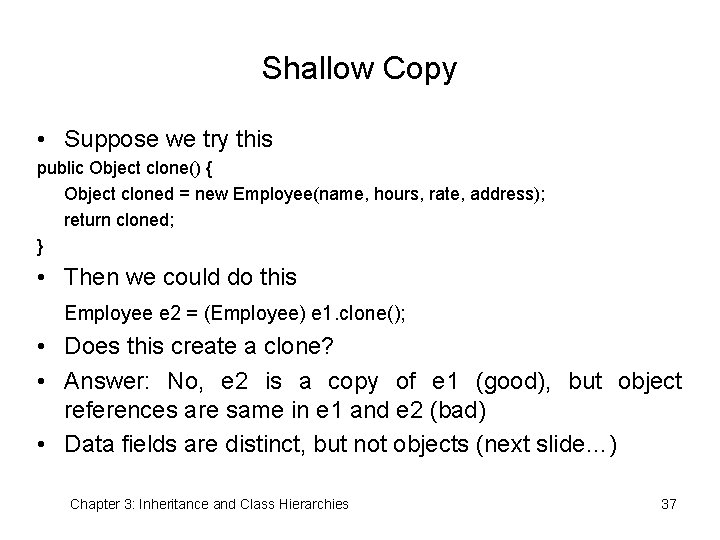
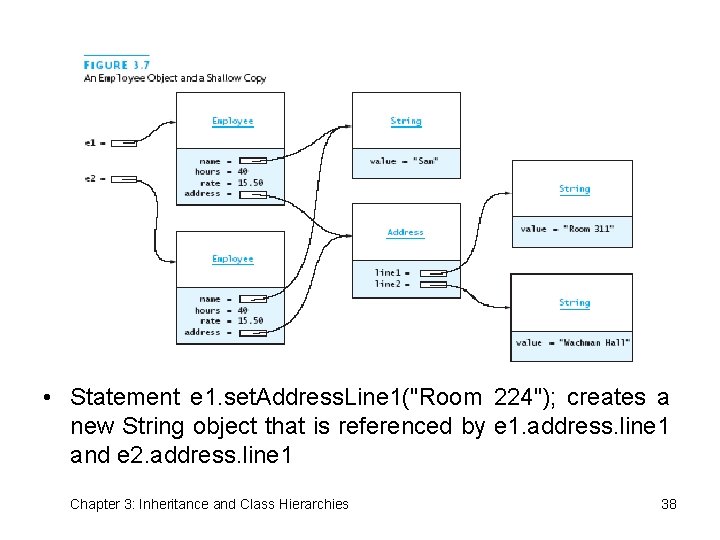
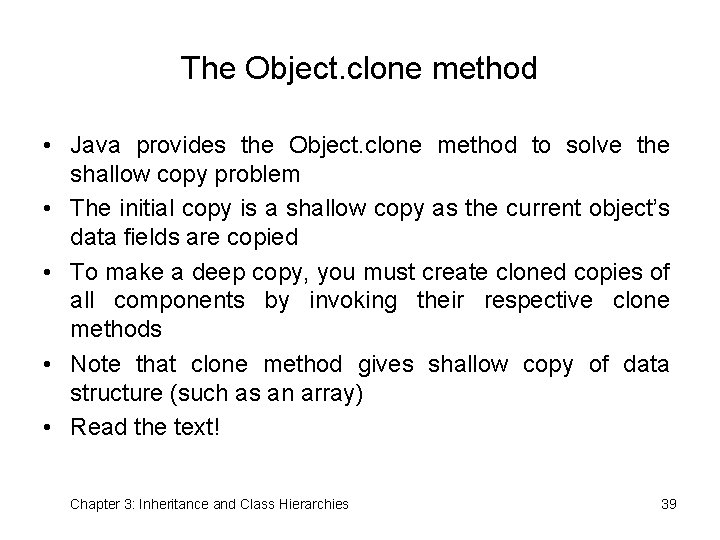
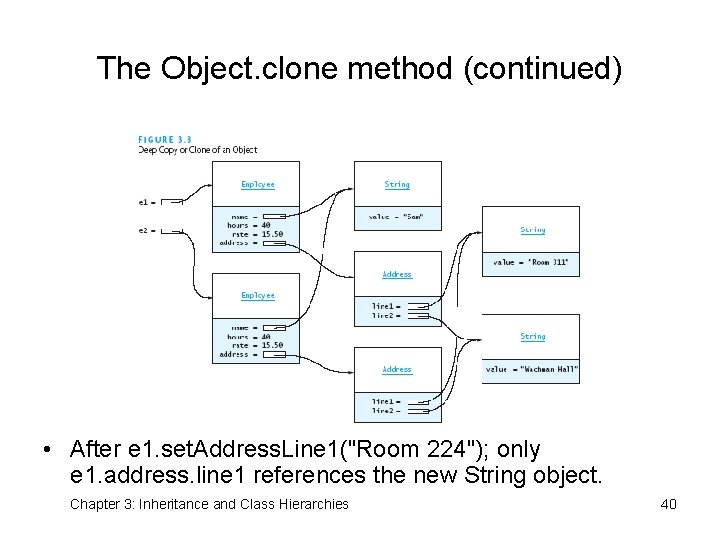
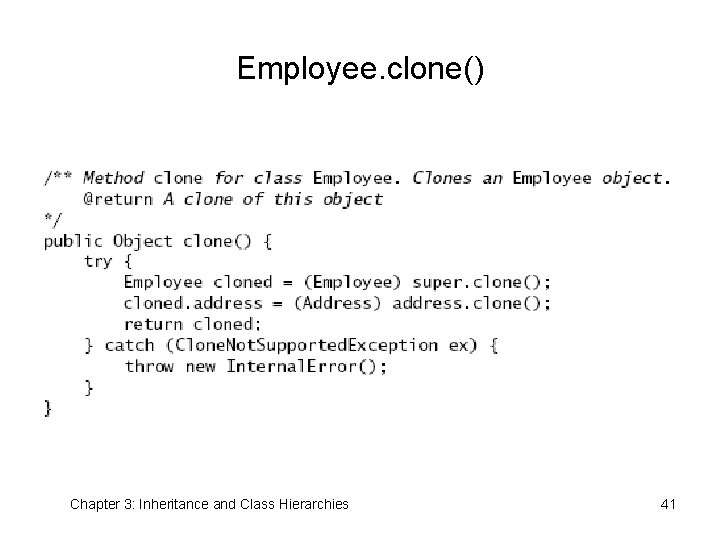
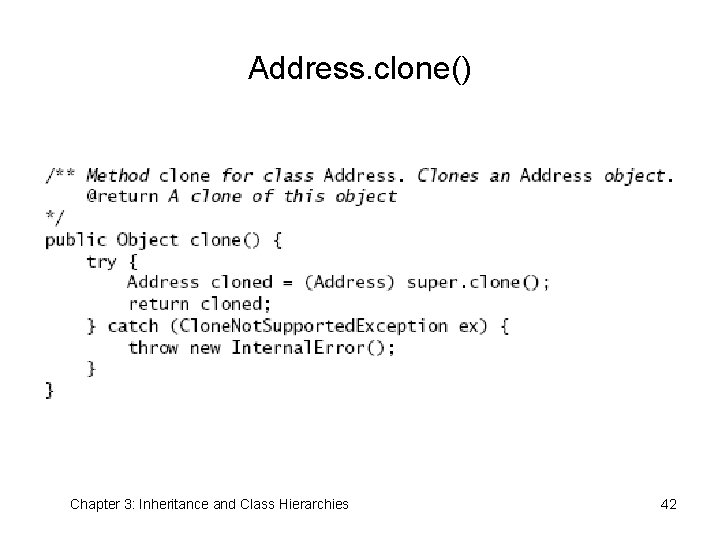
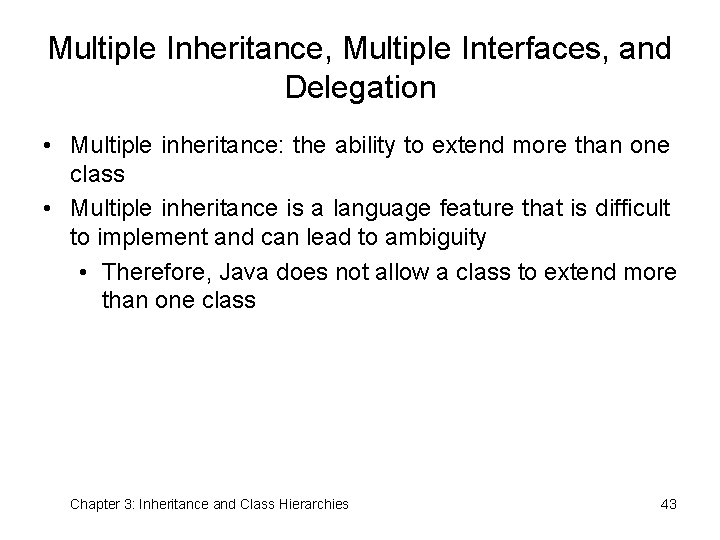
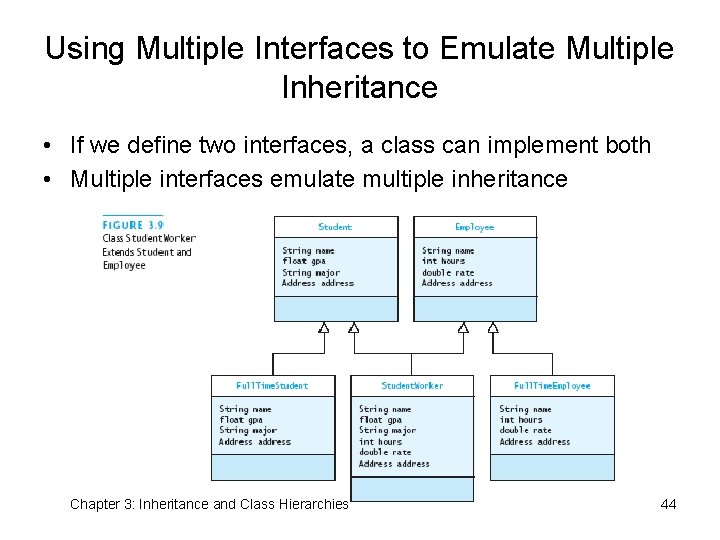
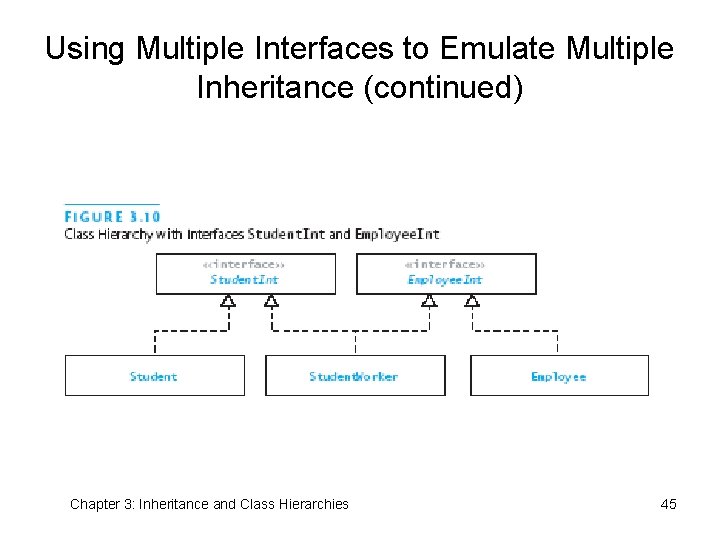
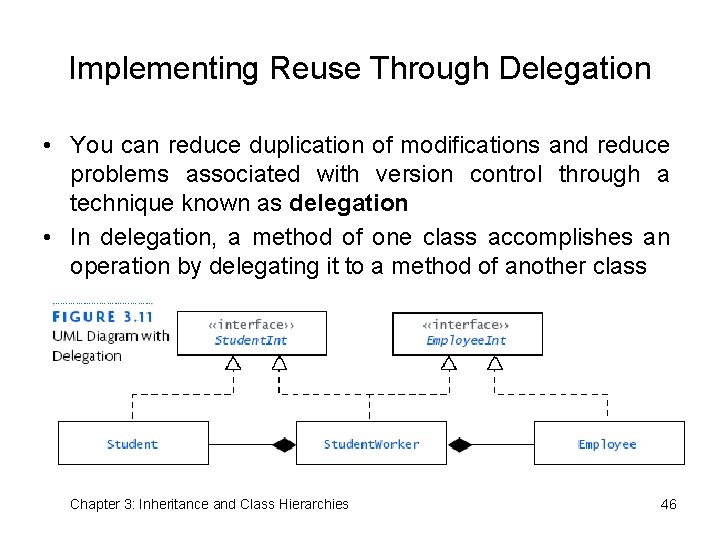
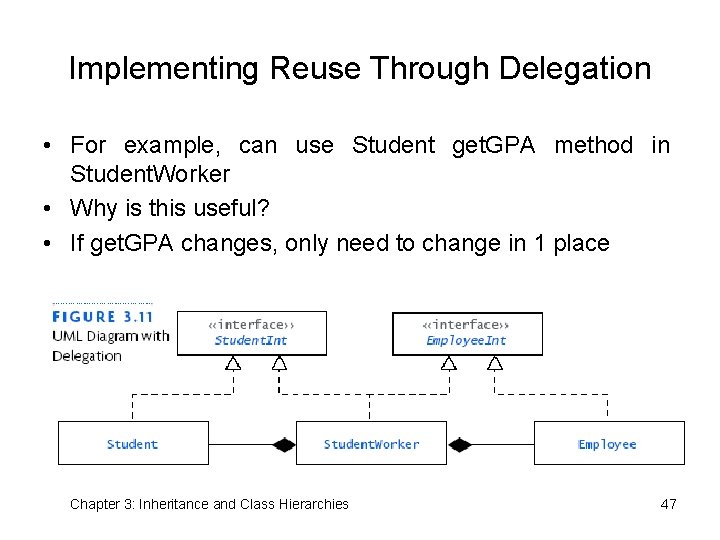
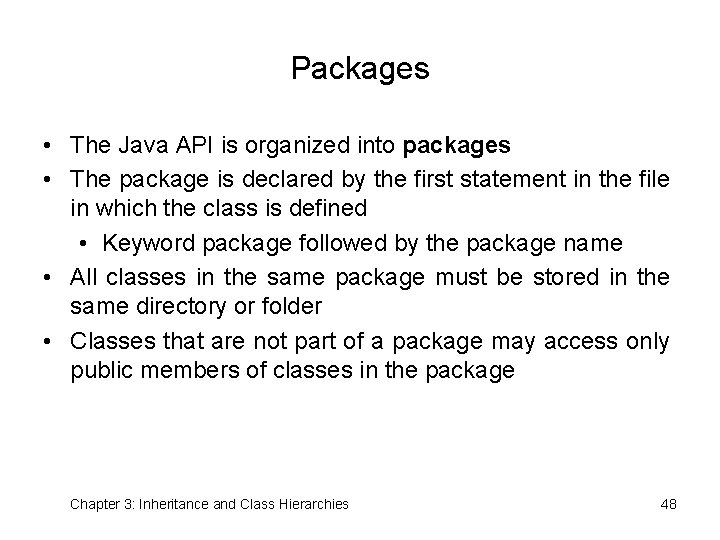
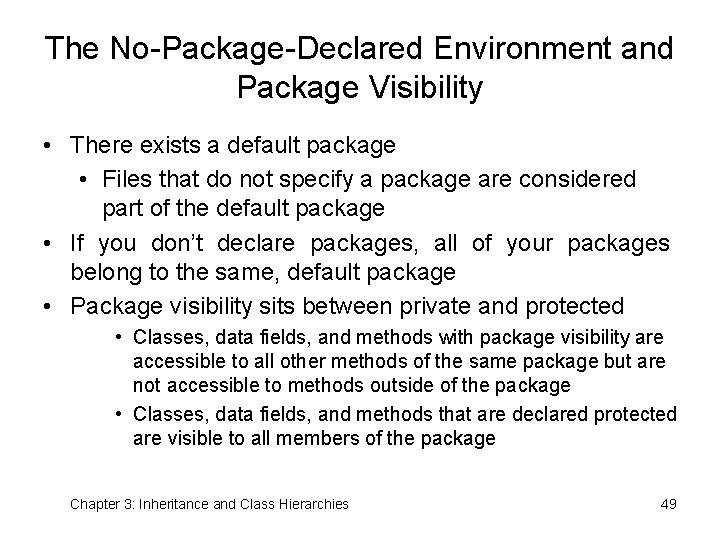
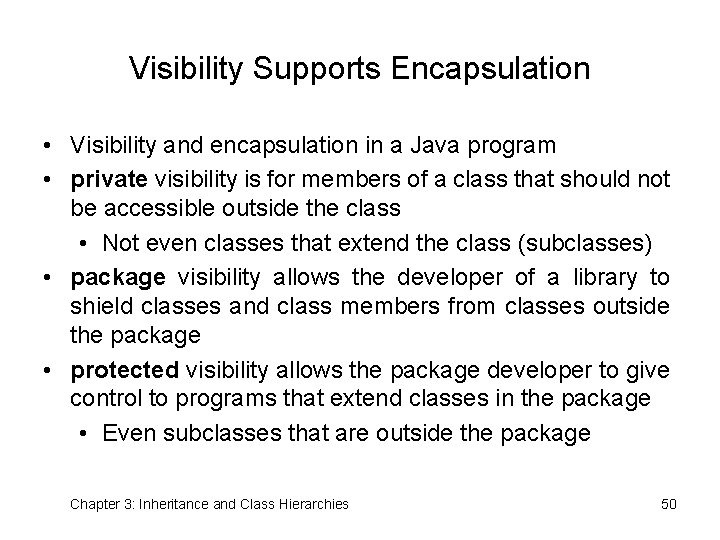
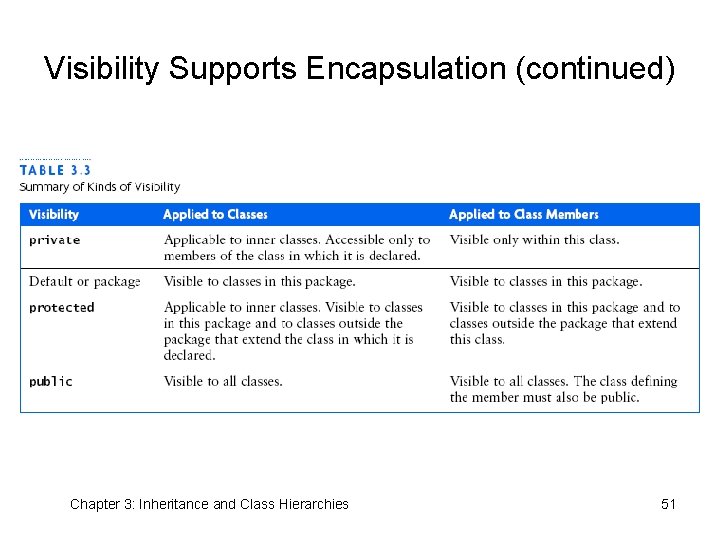
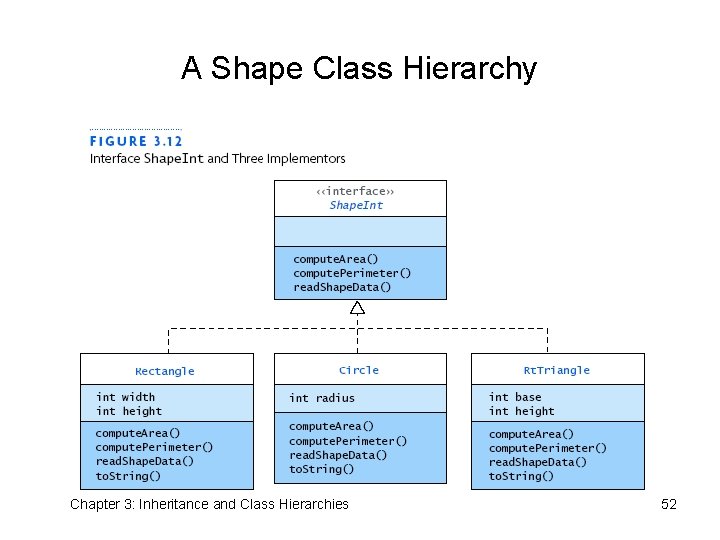
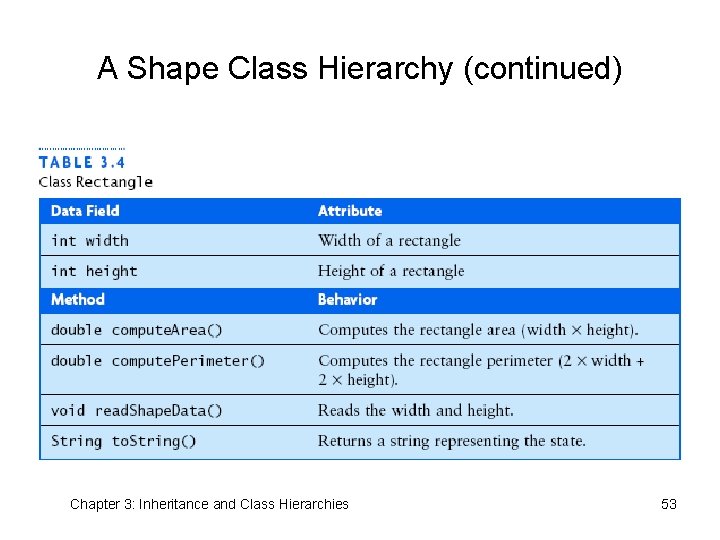
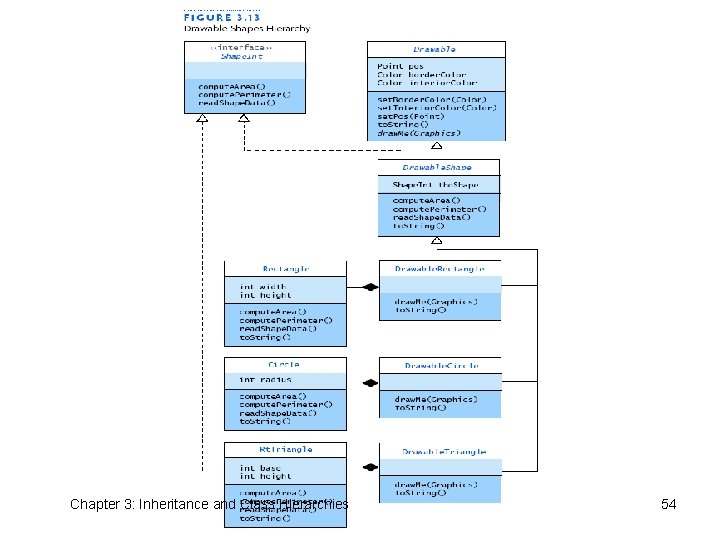
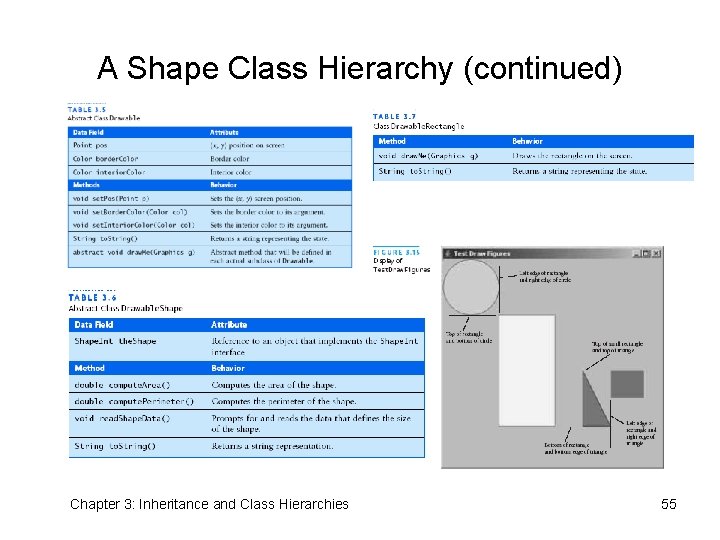
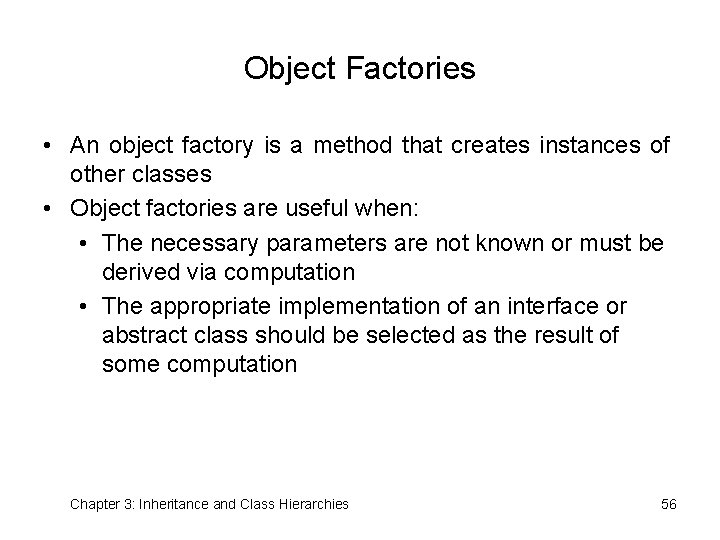
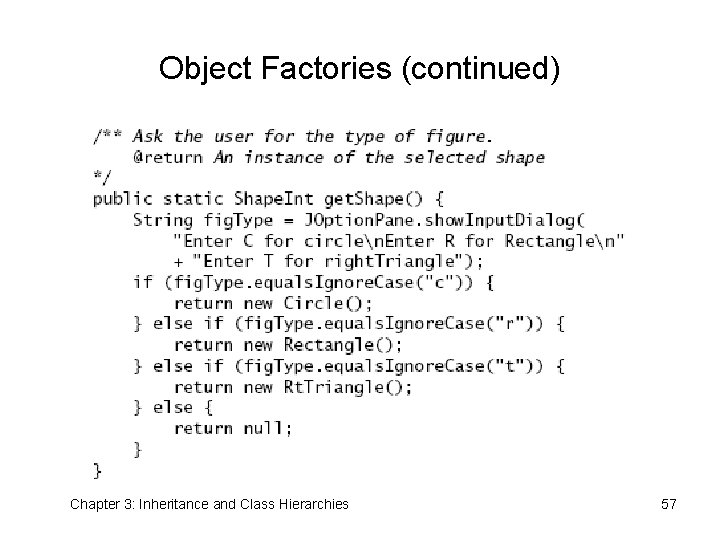
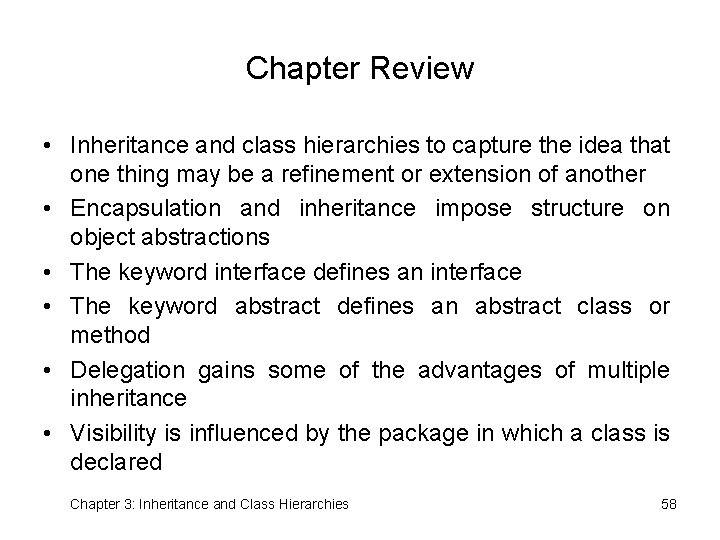
- Slides: 58
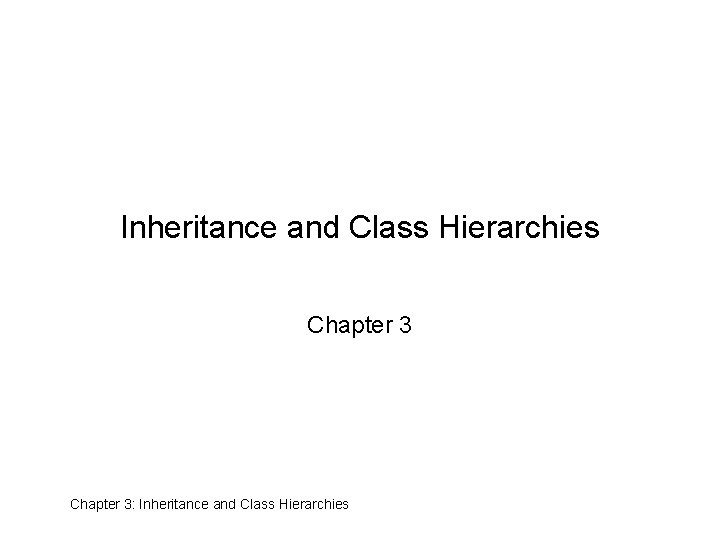
Inheritance and Class Hierarchies Chapter 3: Inheritance and Class Hierarchies
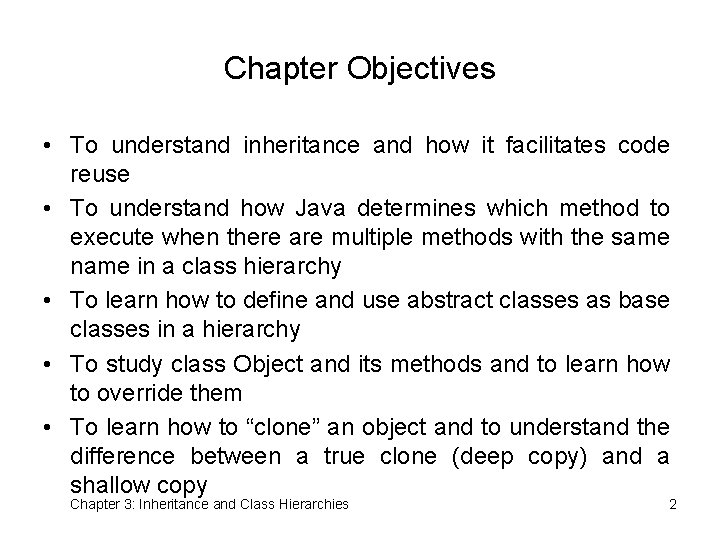
Chapter Objectives • To understand inheritance and how it facilitates code reuse • To understand how Java determines which method to execute when there are multiple methods with the same name in a class hierarchy • To learn how to define and use abstract classes as base classes in a hierarchy • To study class Object and its methods and to learn how to override them • To learn how to “clone” an object and to understand the difference between a true clone (deep copy) and a shallow copy Chapter 3: Inheritance and Class Hierarchies 2
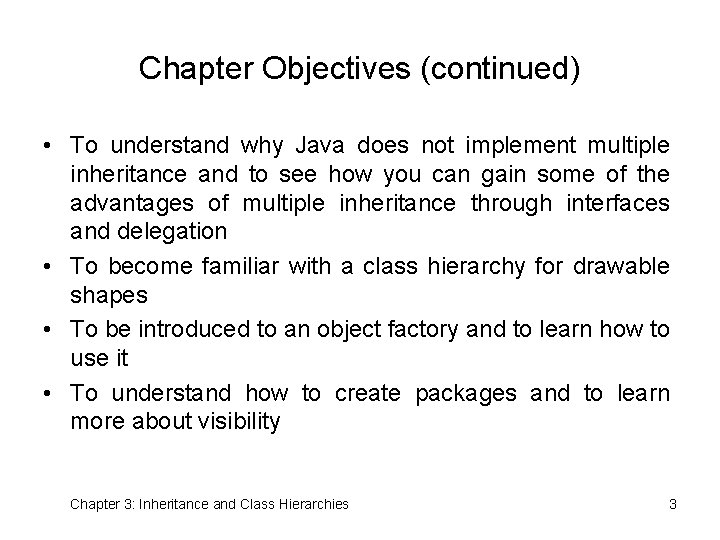
Chapter Objectives (continued) • To understand why Java does not implement multiple inheritance and to see how you can gain some of the advantages of multiple inheritance through interfaces and delegation • To become familiar with a class hierarchy for drawable shapes • To be introduced to an object factory and to learn how to use it • To understand how to create packages and to learn more about visibility Chapter 3: Inheritance and Class Hierarchies 3
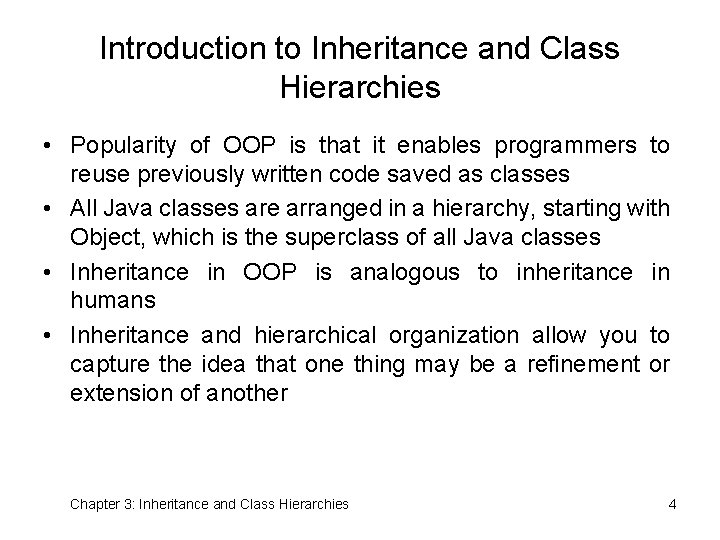
Introduction to Inheritance and Class Hierarchies • Popularity of OOP is that it enables programmers to reuse previously written code saved as classes • All Java classes are arranged in a hierarchy, starting with Object, which is the superclass of all Java classes • Inheritance in OOP is analogous to inheritance in humans • Inheritance and hierarchical organization allow you to capture the idea that one thing may be a refinement or extension of another Chapter 3: Inheritance and Class Hierarchies 4
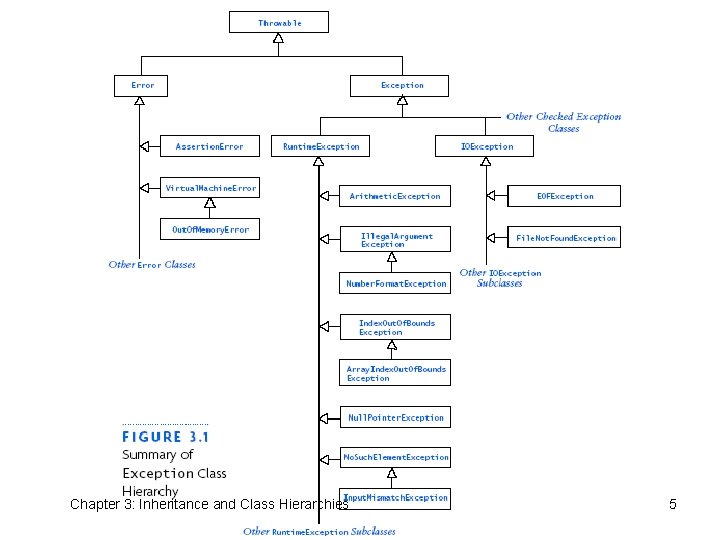
Chapter 3: Inheritance and Class Hierarchies 5
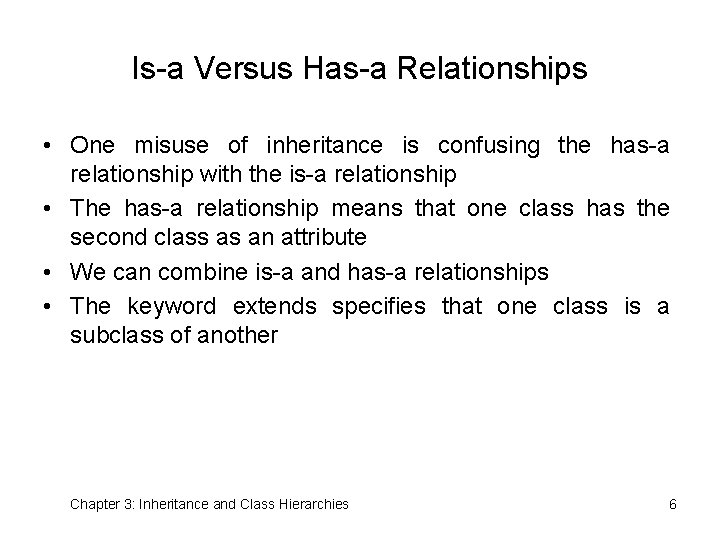
Is-a Versus Has-a Relationships • One misuse of inheritance is confusing the has-a relationship with the is-a relationship • The has-a relationship means that one class has the second class as an attribute • We can combine is-a and has-a relationships • The keyword extends specifies that one class is a subclass of another Chapter 3: Inheritance and Class Hierarchies 6
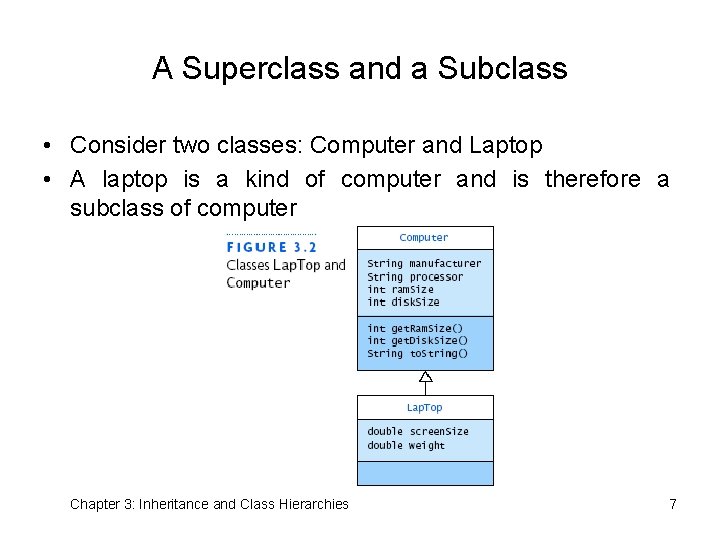
A Superclass and a Subclass • Consider two classes: Computer and Laptop • A laptop is a kind of computer and is therefore a subclass of computer Chapter 3: Inheritance and Class Hierarchies 7
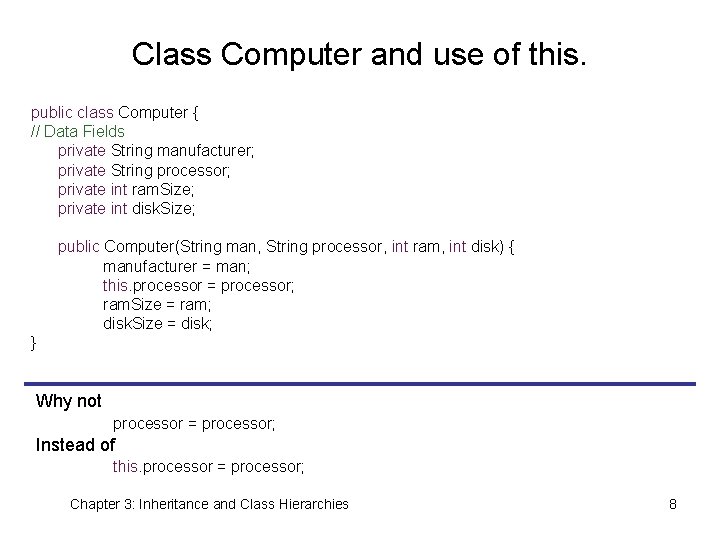
Class Computer and use of this. public class Computer { // Data Fields private String manufacturer; private String processor; private int ram. Size; private int disk. Size; public Computer(String man, String processor, int ram, int disk) { manufacturer = man; this. processor = processor; ram. Size = ram; disk. Size = disk; } Why not processor = processor; Instead of this. processor = processor; Chapter 3: Inheritance and Class Hierarchies 8
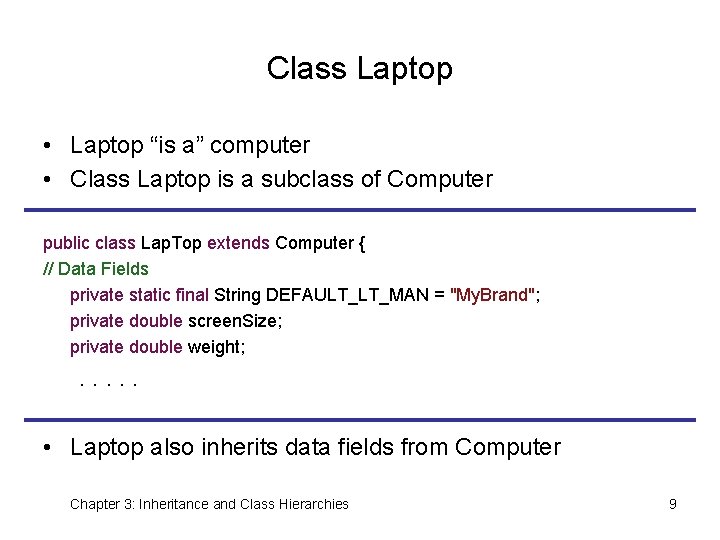
Class Laptop • Laptop “is a” computer • Class Laptop is a subclass of Computer public class Lap. Top extends Computer { // Data Fields private static final String DEFAULT_LT_MAN = "My. Brand"; private double screen. Size; private double weight; . . . • Laptop also inherits data fields from Computer Chapter 3: Inheritance and Class Hierarchies 9
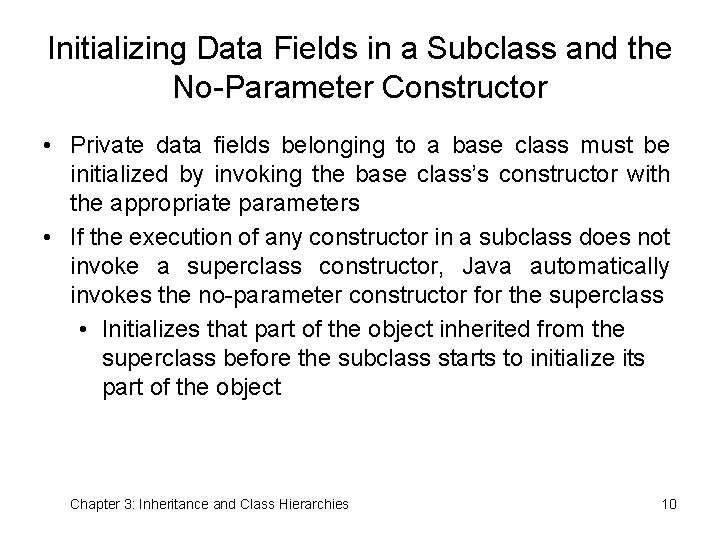
Initializing Data Fields in a Subclass and the No-Parameter Constructor • Private data fields belonging to a base class must be initialized by invoking the base class’s constructor with the appropriate parameters • If the execution of any constructor in a subclass does not invoke a superclass constructor, Java automatically invokes the no-parameter constructor for the superclass • Initializes that part of the object inherited from the superclass before the subclass starts to initialize its part of the object Chapter 3: Inheritance and Class Hierarchies 10
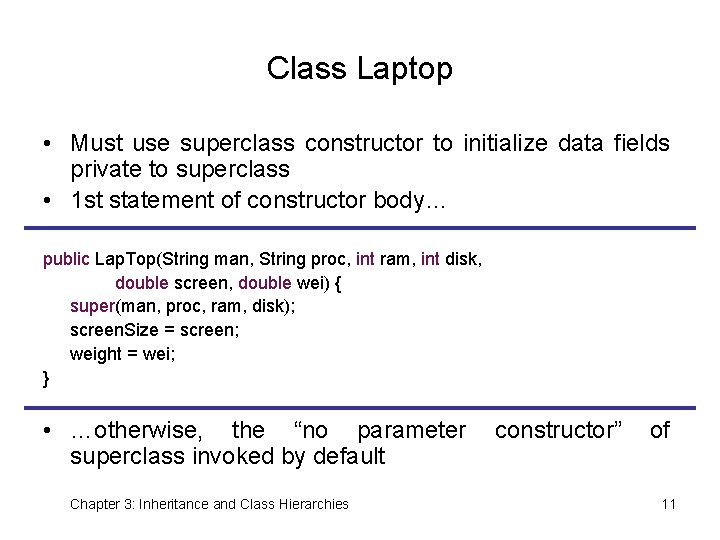
Class Laptop • Must use superclass constructor to initialize data fields private to superclass • 1 st statement of constructor body… public Lap. Top(String man, String proc, int ram, int disk, double screen, double wei) { super(man, proc, ram, disk); screen. Size = screen; weight = wei; } • …otherwise, the “no parameter superclass invoked by default Chapter 3: Inheritance and Class Hierarchies constructor” of 11
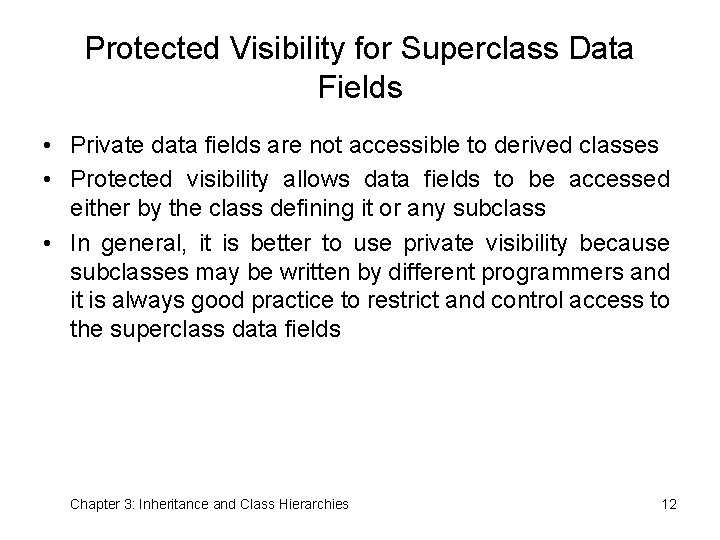
Protected Visibility for Superclass Data Fields • Private data fields are not accessible to derived classes • Protected visibility allows data fields to be accessed either by the class defining it or any subclass • In general, it is better to use private visibility because subclasses may be written by different programmers and it is always good practice to restrict and control access to the superclass data fields Chapter 3: Inheritance and Class Hierarchies 12
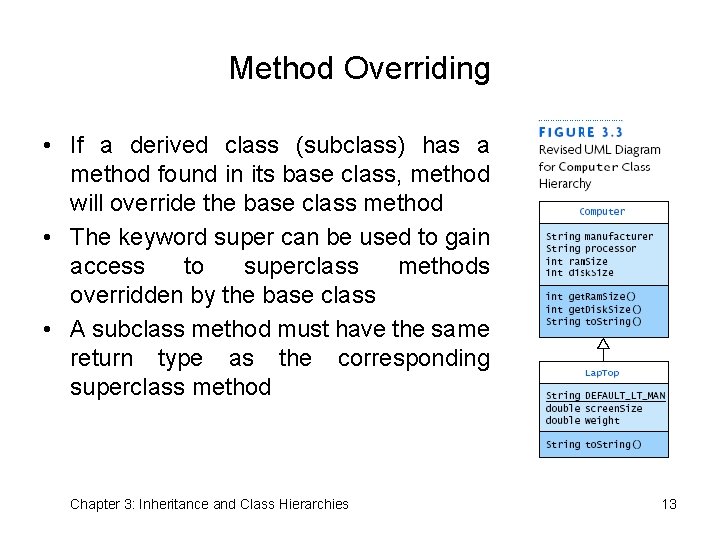
Method Overriding • If a derived class (subclass) has a method found in its base class, method will override the base class method • The keyword super can be used to gain access to superclass methods overridden by the base class • A subclass method must have the same return type as the corresponding superclass method Chapter 3: Inheritance and Class Hierarchies 13
![Method Overriding Suppose main is public static void mainString args Computer my Method Overriding • Suppose main is… public static void main(String[] args) { Computer my.](https://slidetodoc.com/presentation_image_h2/a7a9ccab65cb1a09d50620d7fc5a5c12/image-14.jpg)
Method Overriding • Suppose main is… public static void main(String[] args) { Computer my. Computer = new Computer(“Acme”, “Intel P 4”, 512, 60); Lap. Top your. Computer = new Lap. Top(“Dell”, AMD”, 256, 40, 15. 0, 7. 5); System. out. println(“My computer is: n” + my. Computer. to. String()); System. out. println(“Your computer is: n” + your. Computer. to. String()); } • Suppose Computer defines to. String, but Lap. Top does not • So, what is printed by 2 nd println? Chapter 3: Inheritance and Class Hierarchies 14
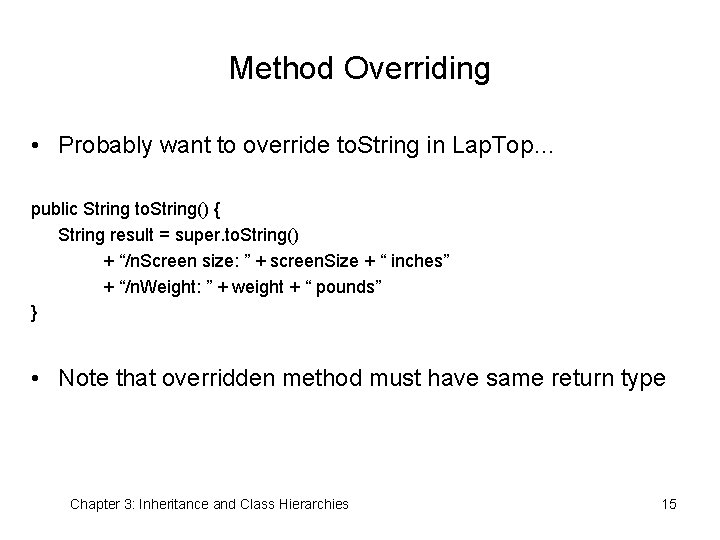
Method Overriding • Probably want to override to. String in Lap. Top… public String to. String() { String result = super. to. String() + “/n. Screen size: ” + screen. Size + “ inches” + “/n. Weight: ” + weight + “ pounds” } • Note that overridden method must have same return type Chapter 3: Inheritance and Class Hierarchies 15
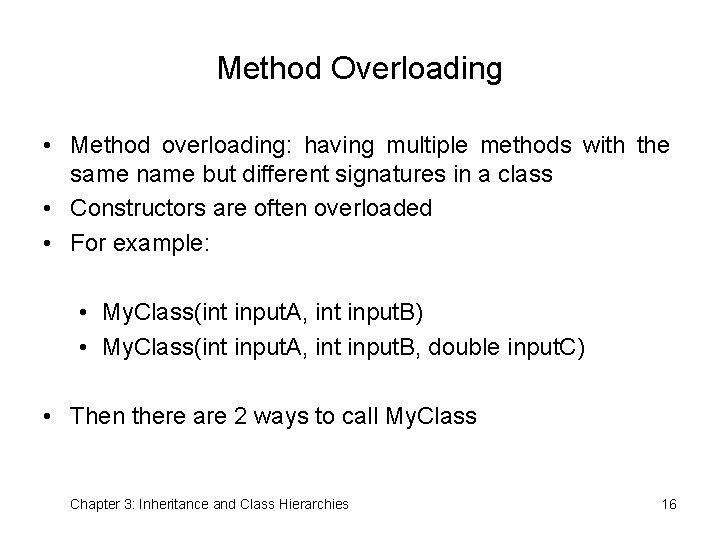
Method Overloading • Method overloading: having multiple methods with the same name but different signatures in a class • Constructors are often overloaded • For example: • My. Class(int input. A, int input. B) • My. Class(int input. A, int input. B, double input. C) • Then there are 2 ways to call My. Class Chapter 3: Inheritance and Class Hierarchies 16
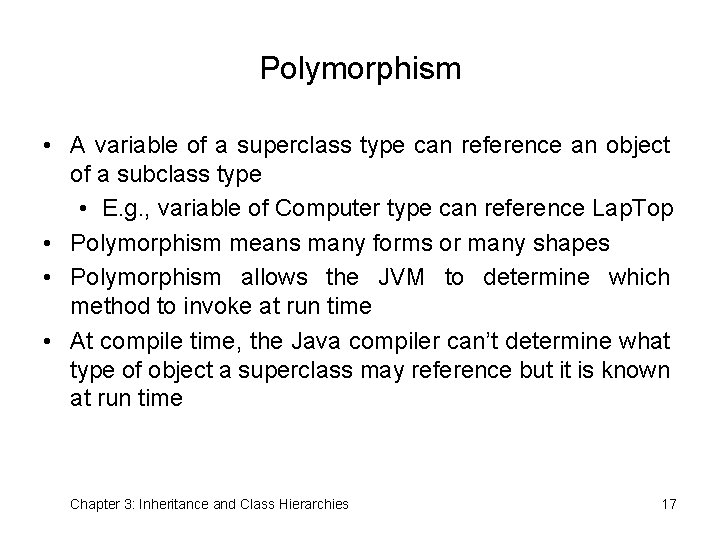
Polymorphism • A variable of a superclass type can reference an object of a subclass type • E. g. , variable of Computer type can reference Lap. Top • Polymorphism means many forms or many shapes • Polymorphism allows the JVM to determine which method to invoke at run time • At compile time, the Java compiler can’t determine what type of object a superclass may reference but it is known at run time Chapter 3: Inheritance and Class Hierarchies 17
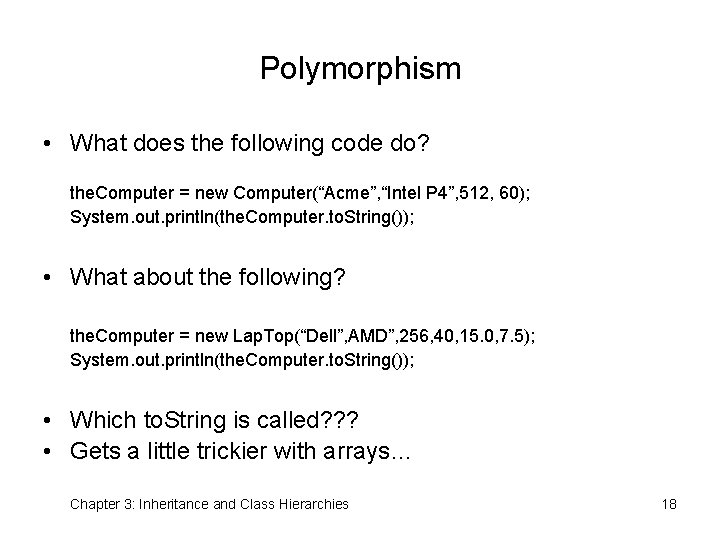
Polymorphism • What does the following code do? the. Computer = new Computer(“Acme”, “Intel P 4”, 512, 60); System. out. println(the. Computer. to. String()); • What about the following? the. Computer = new Lap. Top(“Dell”, AMD”, 256, 40, 15. 0, 7. 5); System. out. println(the. Computer. to. String()); • Which to. String is called? ? ? • Gets a little trickier with arrays… Chapter 3: Inheritance and Class Hierarchies 18
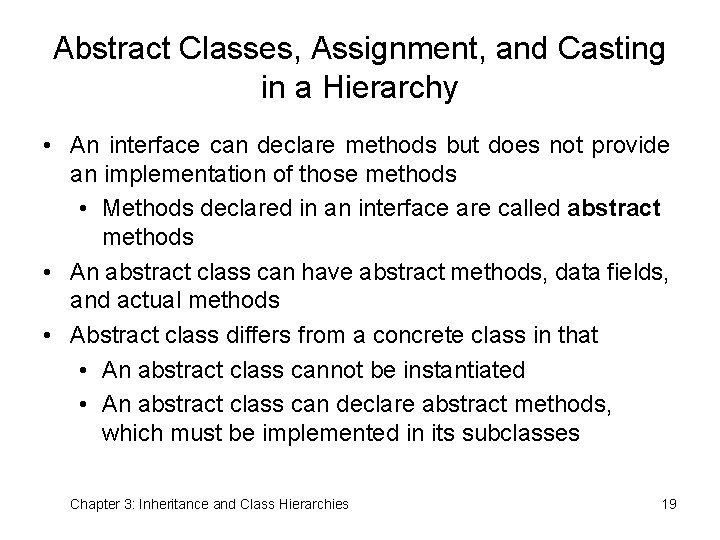
Abstract Classes, Assignment, and Casting in a Hierarchy • An interface can declare methods but does not provide an implementation of those methods • Methods declared in an interface are called abstract methods • An abstract class can have abstract methods, data fields, and actual methods • Abstract class differs from a concrete class in that • An abstract class cannot be instantiated • An abstract class can declare abstract methods, which must be implemented in its subclasses Chapter 3: Inheritance and Class Hierarchies 19
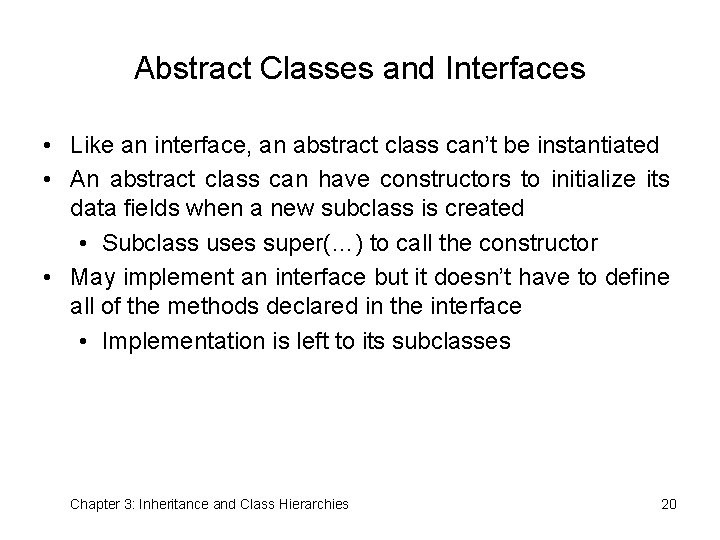
Abstract Classes and Interfaces • Like an interface, an abstract class can’t be instantiated • An abstract class can have constructors to initialize its data fields when a new subclass is created • Subclass uses super(…) to call the constructor • May implement an interface but it doesn’t have to define all of the methods declared in the interface • Implementation is left to its subclasses Chapter 3: Inheritance and Class Hierarchies 20
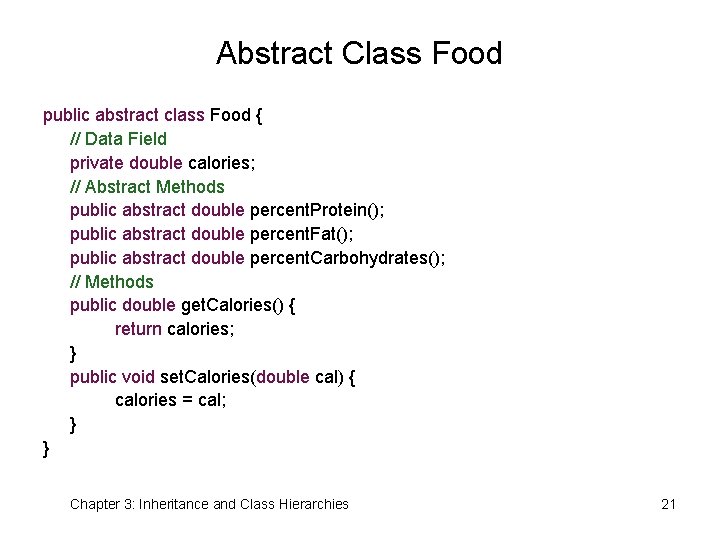
Abstract Class Food public abstract class Food { // Data Field private double calories; // Abstract Methods public abstract double percent. Protein(); public abstract double percent. Fat(); public abstract double percent. Carbohydrates(); // Methods public double get. Calories() { return calories; } public void set. Calories(double cal) { calories = cal; } } Chapter 3: Inheritance and Class Hierarchies 21
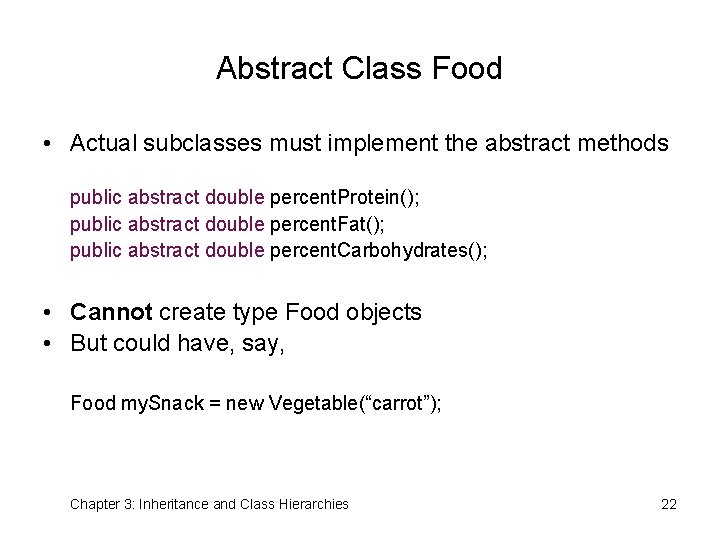
Abstract Class Food • Actual subclasses must implement the abstract methods public abstract double percent. Protein(); public abstract double percent. Fat(); public abstract double percent. Carbohydrates(); • Cannot create type Food objects • But could have, say, Food my. Snack = new Vegetable(“carrot”); Chapter 3: Inheritance and Class Hierarchies 22
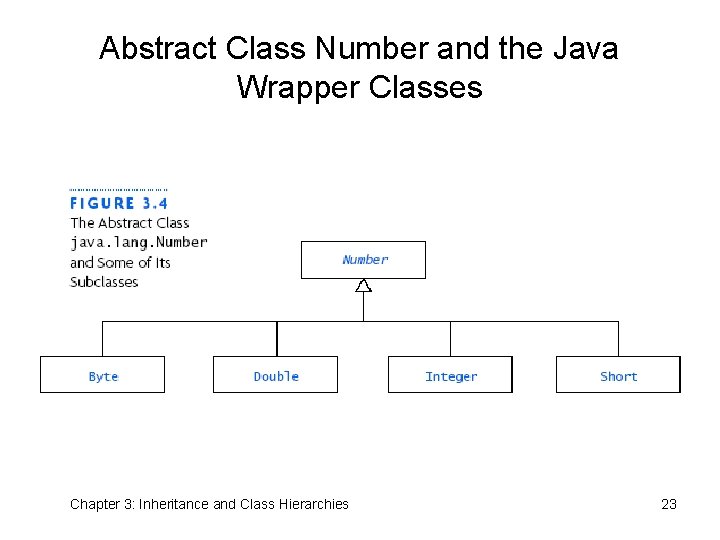
Abstract Class Number and the Java Wrapper Classes Chapter 3: Inheritance and Class Hierarchies 23
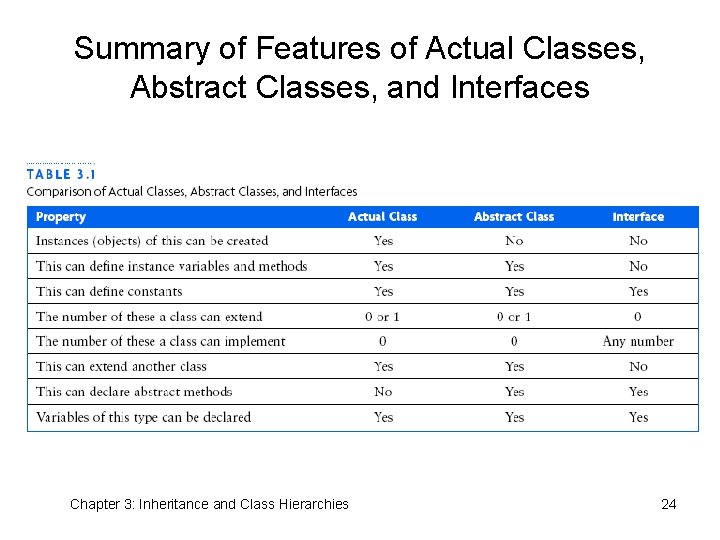
Summary of Features of Actual Classes, Abstract Classes, and Interfaces Chapter 3: Inheritance and Class Hierarchies 24
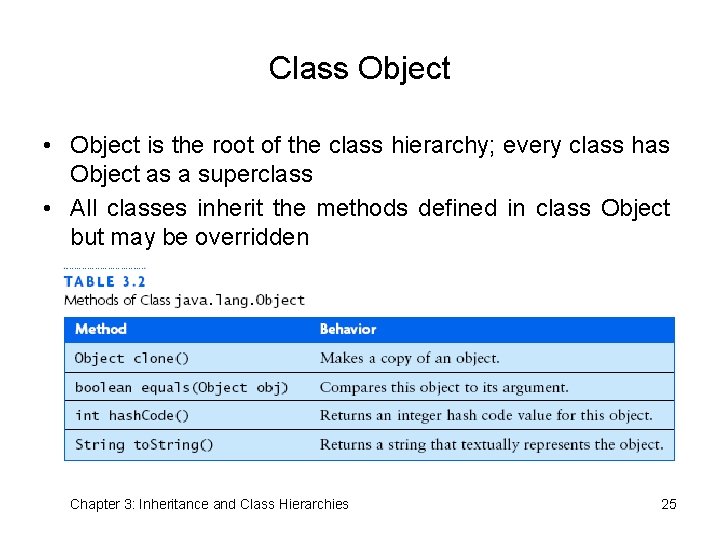
Class Object • Object is the root of the class hierarchy; every class has Object as a superclass • All classes inherit the methods defined in class Object but may be overridden Chapter 3: Inheritance and Class Hierarchies 25
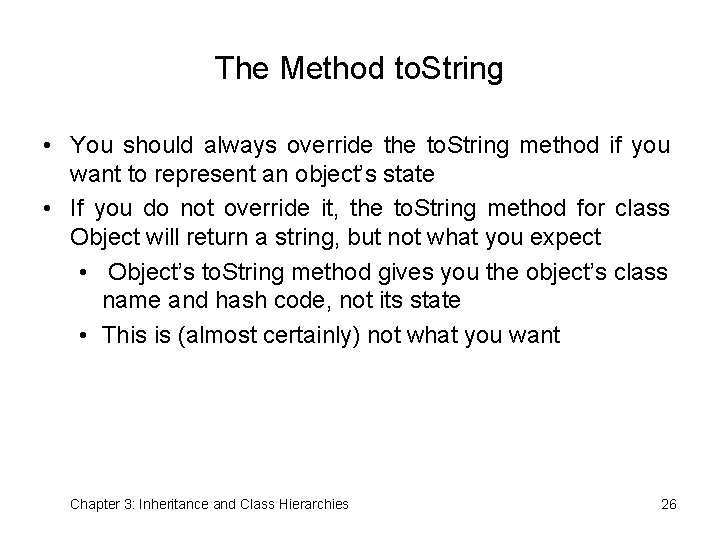
The Method to. String • You should always override the to. String method if you want to represent an object’s state • If you do not override it, the to. String method for class Object will return a string, but not what you expect • Object’s to. String method gives you the object’s class name and hash code, not its state • This is (almost certainly) not what you want Chapter 3: Inheritance and Class Hierarchies 26
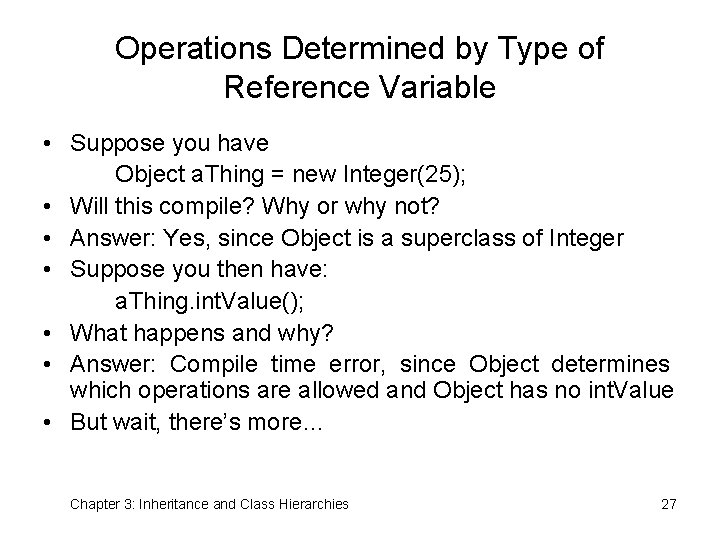
Operations Determined by Type of Reference Variable • Suppose you have Object a. Thing = new Integer(25); • Will this compile? Why or why not? • Answer: Yes, since Object is a superclass of Integer • Suppose you then have: a. Thing. int. Value(); • What happens and why? • Answer: Compile time error, since Object determines which operations are allowed and Object has no int. Value • But wait, there’s more… Chapter 3: Inheritance and Class Hierarchies 27
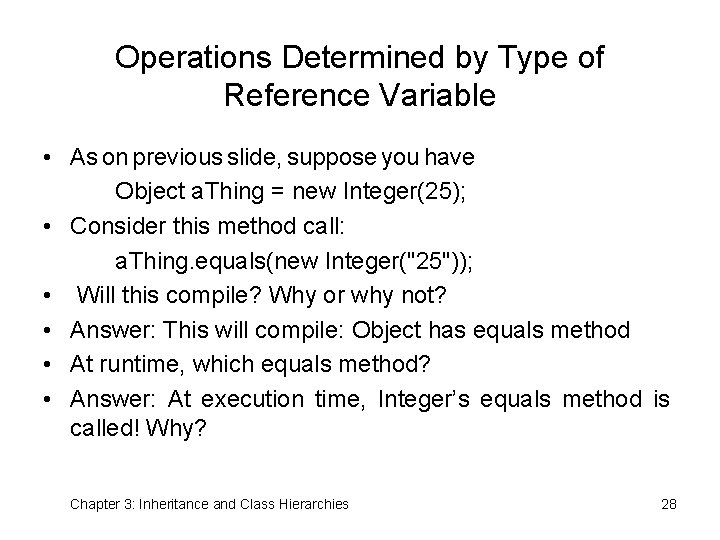
Operations Determined by Type of Reference Variable • As on previous slide, suppose you have Object a. Thing = new Integer(25); • Consider this method call: a. Thing. equals(new Integer("25")); • Will this compile? Why or why not? • Answer: This will compile: Object has equals method • At runtime, which equals method? • Answer: At execution time, Integer’s equals method is called! Why? Chapter 3: Inheritance and Class Hierarchies 28
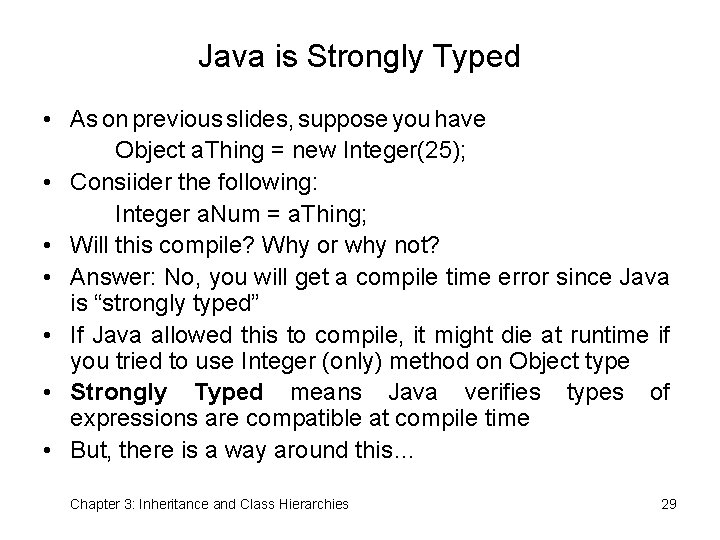
Java is Strongly Typed • As on previous slides, suppose you have Object a. Thing = new Integer(25); • Consiider the following: Integer a. Num = a. Thing; • Will this compile? Why or why not? • Answer: No, you will get a compile time error since Java is “strongly typed” • If Java allowed this to compile, it might die at runtime if you tried to use Integer (only) method on Object type • Strongly Typed means Java verifies types of expressions are compatible at compile time • But, there is a way around this… Chapter 3: Inheritance and Class Hierarchies 29
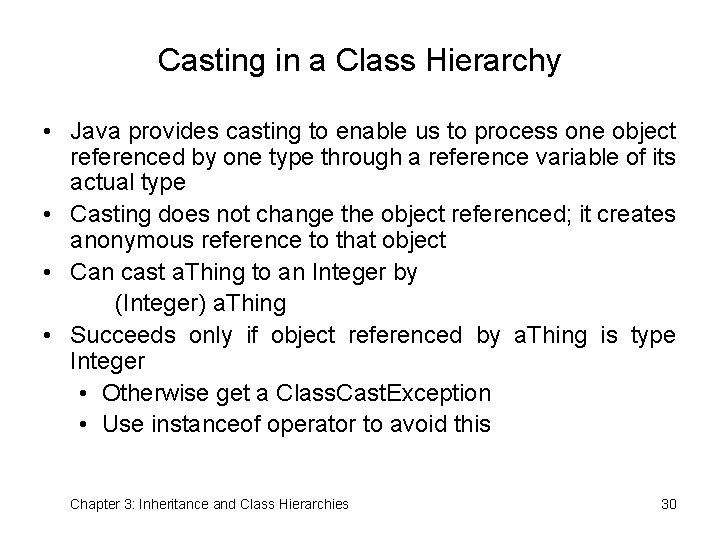
Casting in a Class Hierarchy • Java provides casting to enable us to process one object referenced by one type through a reference variable of its actual type • Casting does not change the object referenced; it creates anonymous reference to that object • Can cast a. Thing to an Integer by (Integer) a. Thing • Succeeds only if object referenced by a. Thing is type Integer • Otherwise get a Class. Cast. Exception • Use instanceof operator to avoid this Chapter 3: Inheritance and Class Hierarchies 30
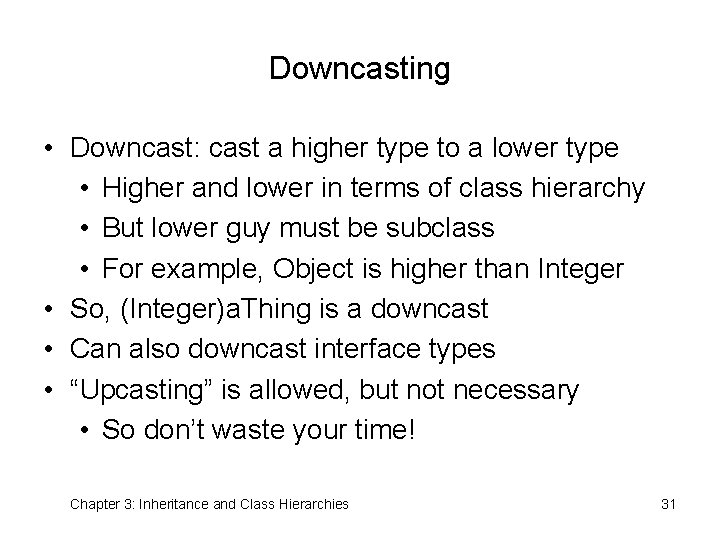
Downcasting • Downcast: cast a higher type to a lower type • Higher and lower in terms of class hierarchy • But lower guy must be subclass • For example, Object is higher than Integer • So, (Integer)a. Thing is a downcast • Can also downcast interface types • “Upcasting” is allowed, but not necessary • So don’t waste your time! Chapter 3: Inheritance and Class Hierarchies 31
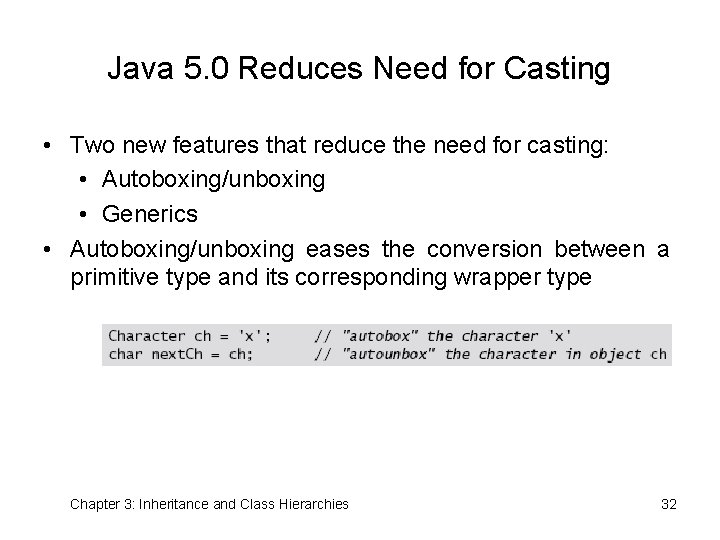
Java 5. 0 Reduces Need for Casting • Two new features that reduce the need for casting: • Autoboxing/unboxing • Generics • Autoboxing/unboxing eases the conversion between a primitive type and its corresponding wrapper type Chapter 3: Inheritance and Class Hierarchies 32
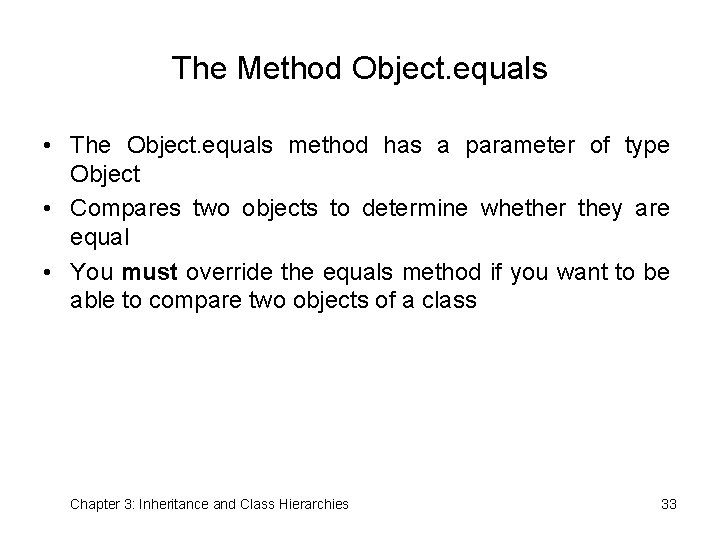
The Method Object. equals • The Object. equals method has a parameter of type Object • Compares two objects to determine whether they are equal • You must override the equals method if you want to be able to compare two objects of a class Chapter 3: Inheritance and Class Hierarchies 33
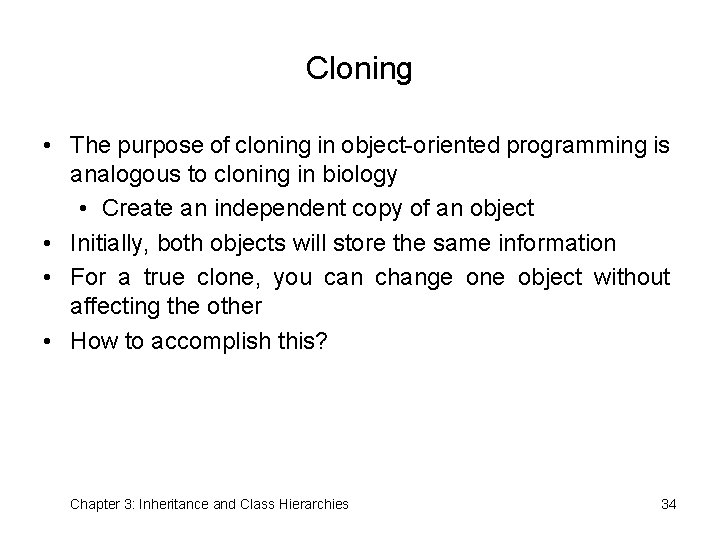
Cloning • The purpose of cloning in object-oriented programming is analogous to cloning in biology • Create an independent copy of an object • Initially, both objects will store the same information • For a true clone, you can change one object without affecting the other • How to accomplish this? Chapter 3: Inheritance and Class Hierarchies 34
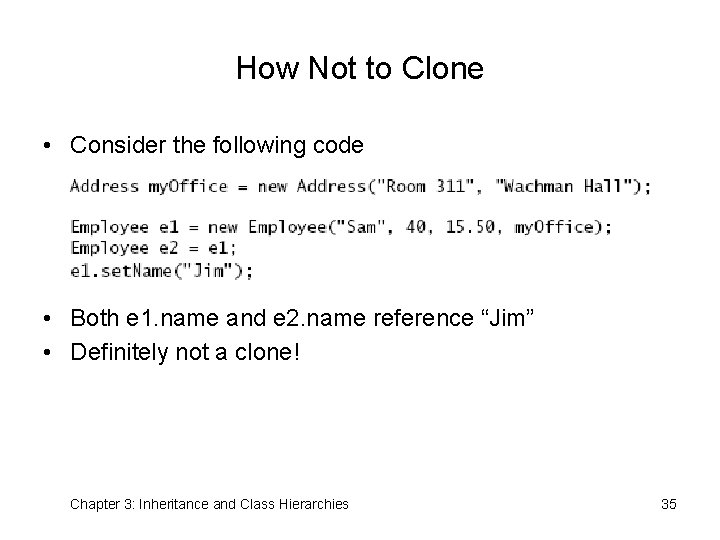
How Not to Clone • Consider the following code • Both e 1. name and e 2. name reference “Jim” • Definitely not a clone! Chapter 3: Inheritance and Class Hierarchies 35
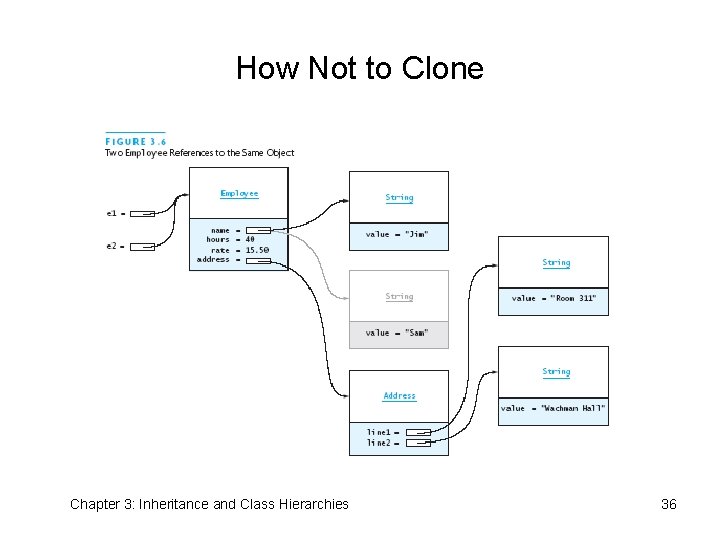
How Not to Clone Chapter 3: Inheritance and Class Hierarchies 36
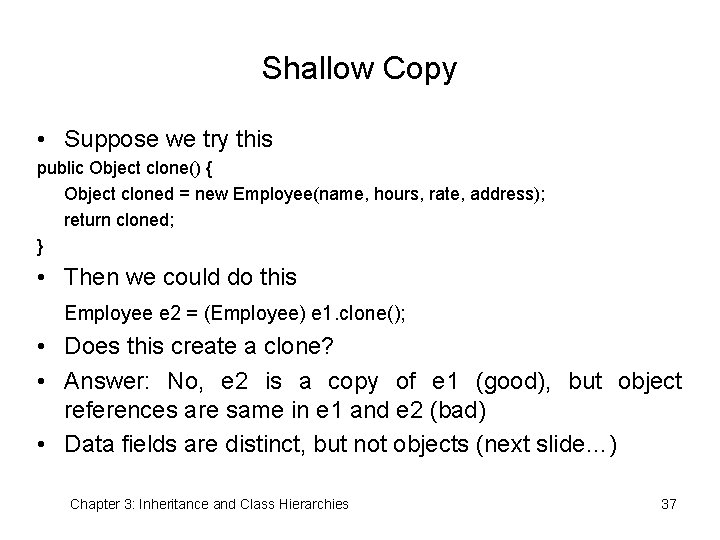
Shallow Copy • Suppose we try this public Object clone() { Object cloned = new Employee(name, hours, rate, address); return cloned; } • Then we could do this Employee e 2 = (Employee) e 1. clone(); • Does this create a clone? • Answer: No, e 2 is a copy of e 1 (good), but object references are same in e 1 and e 2 (bad) • Data fields are distinct, but not objects (next slide…) Chapter 3: Inheritance and Class Hierarchies 37
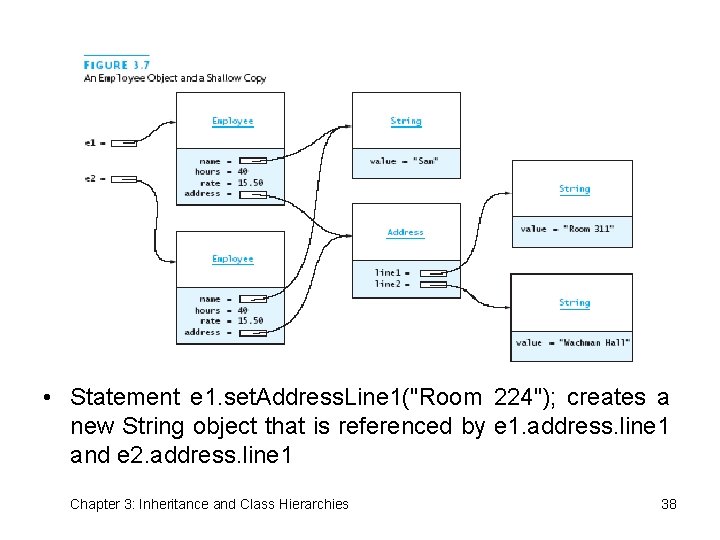
• Statement e 1. set. Address. Line 1("Room 224"); creates a new String object that is referenced by e 1. address. line 1 and e 2. address. line 1 Chapter 3: Inheritance and Class Hierarchies 38
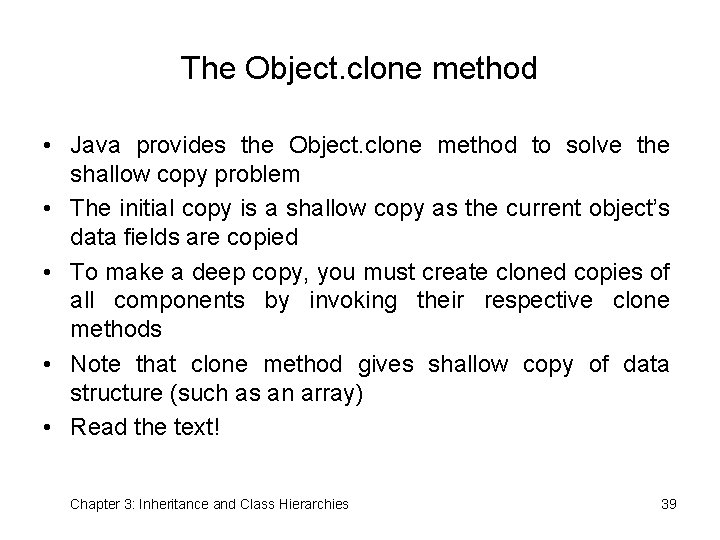
The Object. clone method • Java provides the Object. clone method to solve the shallow copy problem • The initial copy is a shallow copy as the current object’s data fields are copied • To make a deep copy, you must create cloned copies of all components by invoking their respective clone methods • Note that clone method gives shallow copy of data structure (such as an array) • Read the text! Chapter 3: Inheritance and Class Hierarchies 39
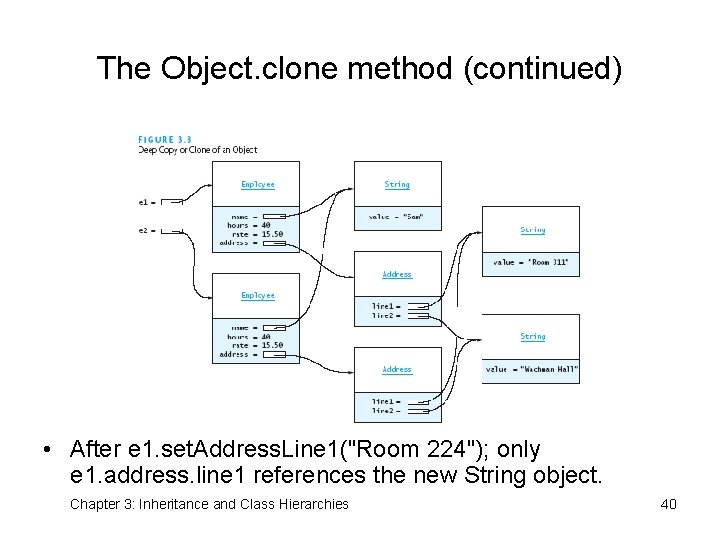
The Object. clone method (continued) • After e 1. set. Address. Line 1("Room 224"); only e 1. address. line 1 references the new String object. Chapter 3: Inheritance and Class Hierarchies 40
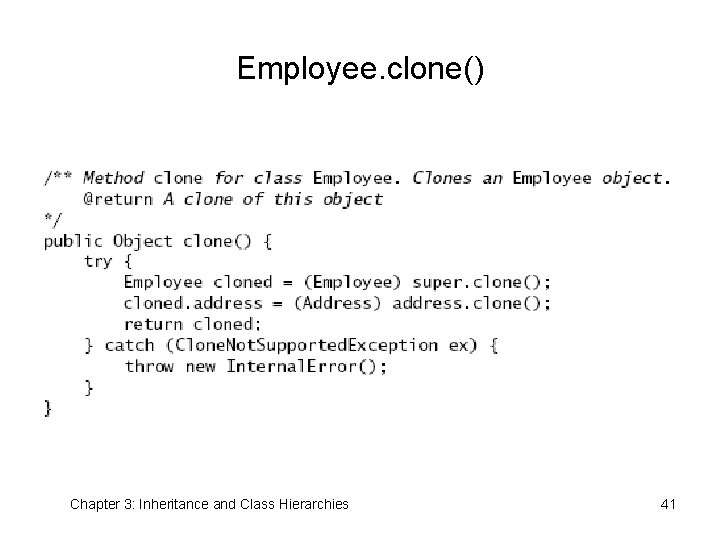
Employee. clone() Chapter 3: Inheritance and Class Hierarchies 41
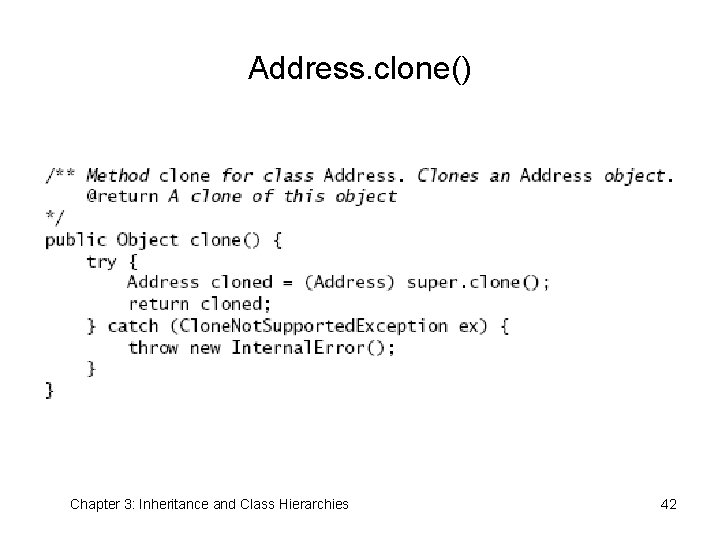
Address. clone() Chapter 3: Inheritance and Class Hierarchies 42
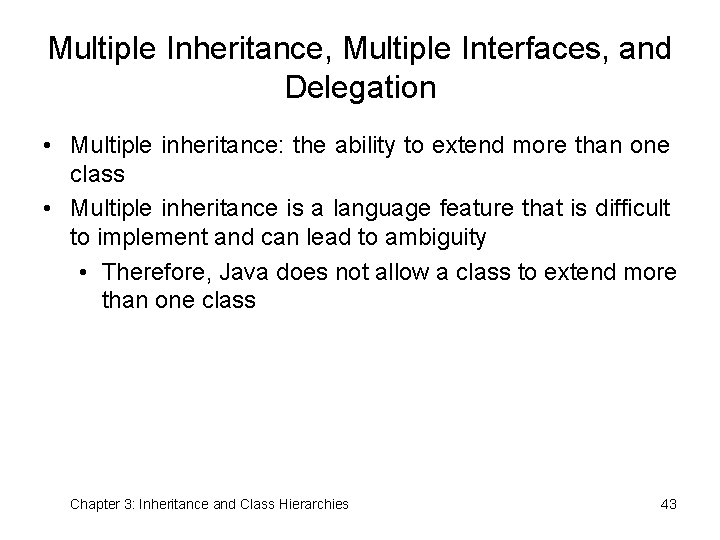
Multiple Inheritance, Multiple Interfaces, and Delegation • Multiple inheritance: the ability to extend more than one class • Multiple inheritance is a language feature that is difficult to implement and can lead to ambiguity • Therefore, Java does not allow a class to extend more than one class Chapter 3: Inheritance and Class Hierarchies 43
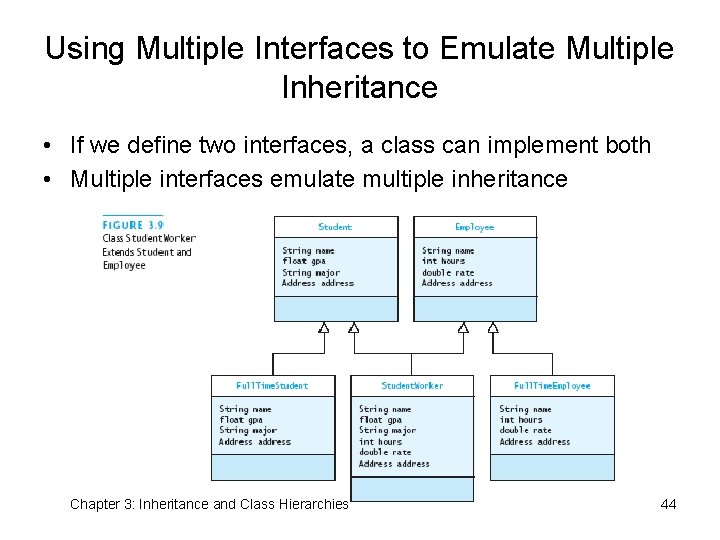
Using Multiple Interfaces to Emulate Multiple Inheritance • If we define two interfaces, a class can implement both • Multiple interfaces emulate multiple inheritance Chapter 3: Inheritance and Class Hierarchies 44
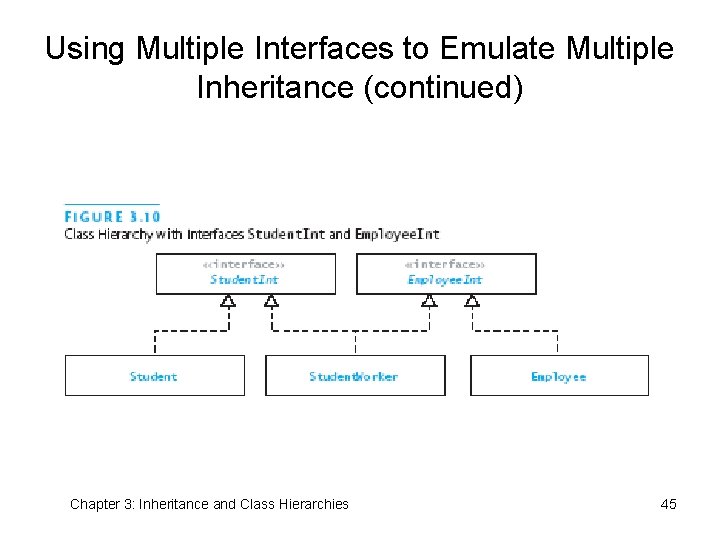
Using Multiple Interfaces to Emulate Multiple Inheritance (continued) Chapter 3: Inheritance and Class Hierarchies 45
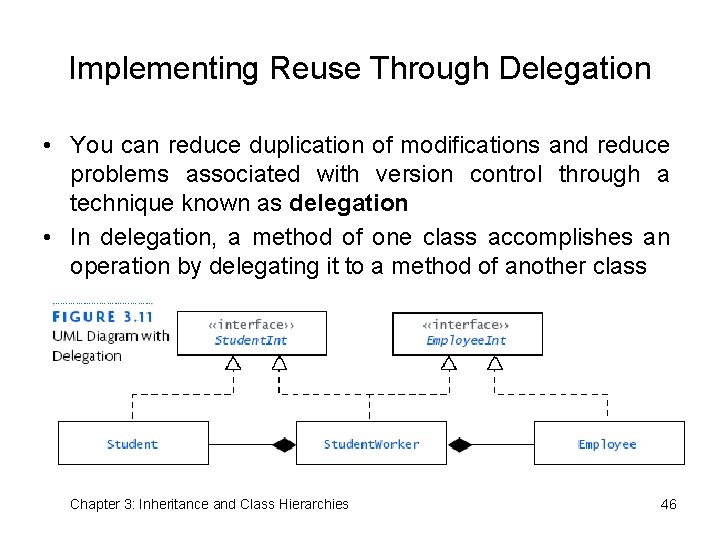
Implementing Reuse Through Delegation • You can reduce duplication of modifications and reduce problems associated with version control through a technique known as delegation • In delegation, a method of one class accomplishes an operation by delegating it to a method of another class Chapter 3: Inheritance and Class Hierarchies 46
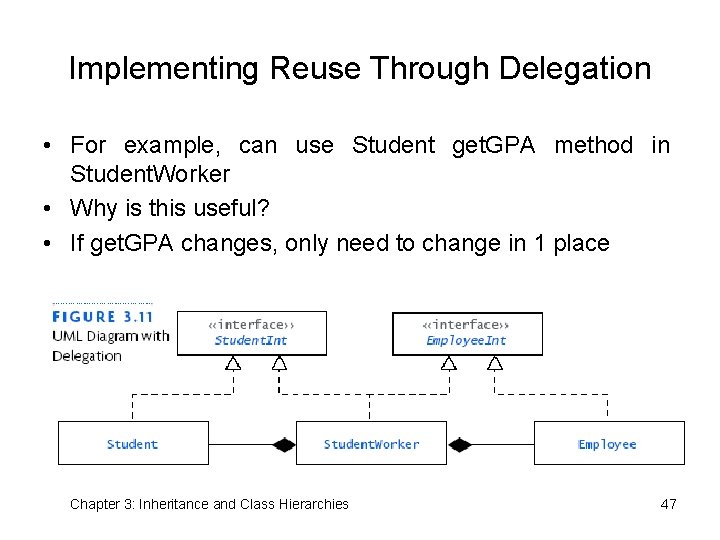
Implementing Reuse Through Delegation • For example, can use Student get. GPA method in Student. Worker • Why is this useful? • If get. GPA changes, only need to change in 1 place Chapter 3: Inheritance and Class Hierarchies 47
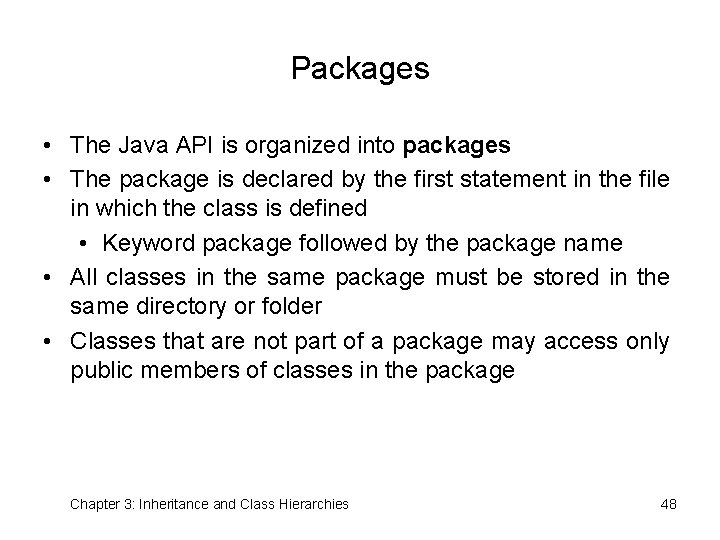
Packages • The Java API is organized into packages • The package is declared by the first statement in the file in which the class is defined • Keyword package followed by the package name • All classes in the same package must be stored in the same directory or folder • Classes that are not part of a package may access only public members of classes in the package Chapter 3: Inheritance and Class Hierarchies 48
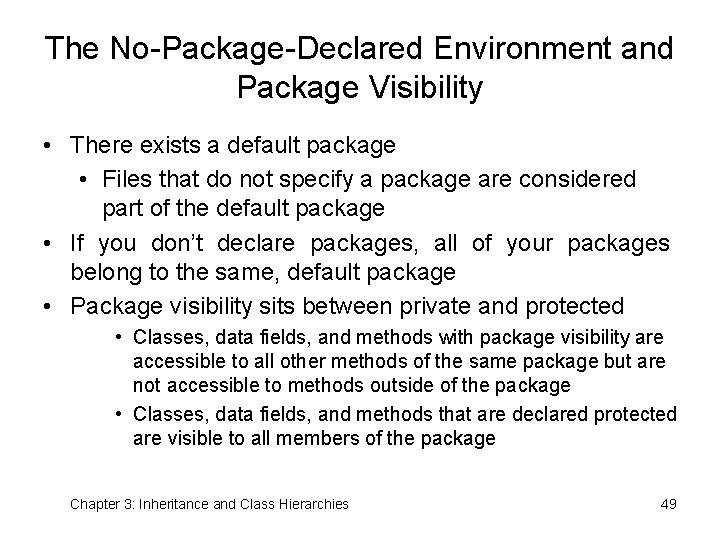
The No-Package-Declared Environment and Package Visibility • There exists a default package • Files that do not specify a package are considered part of the default package • If you don’t declare packages, all of your packages belong to the same, default package • Package visibility sits between private and protected • Classes, data fields, and methods with package visibility are accessible to all other methods of the same package but are not accessible to methods outside of the package • Classes, data fields, and methods that are declared protected are visible to all members of the package Chapter 3: Inheritance and Class Hierarchies 49
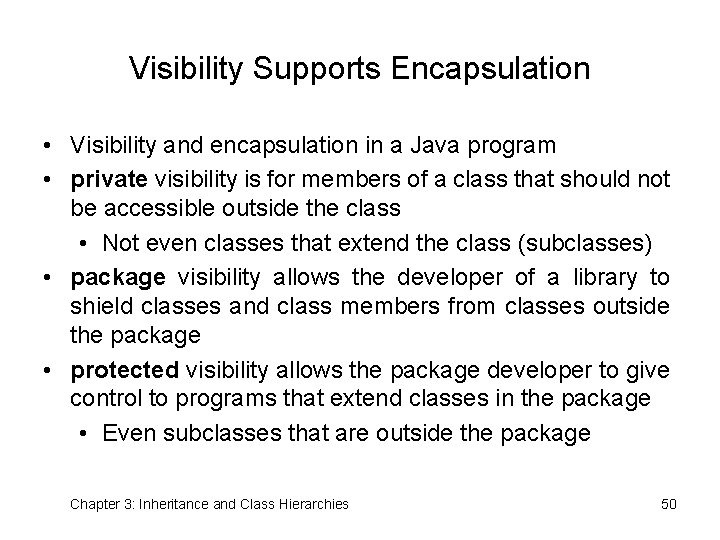
Visibility Supports Encapsulation • Visibility and encapsulation in a Java program • private visibility is for members of a class that should not be accessible outside the class • Not even classes that extend the class (subclasses) • package visibility allows the developer of a library to shield classes and class members from classes outside the package • protected visibility allows the package developer to give control to programs that extend classes in the package • Even subclasses that are outside the package Chapter 3: Inheritance and Class Hierarchies 50
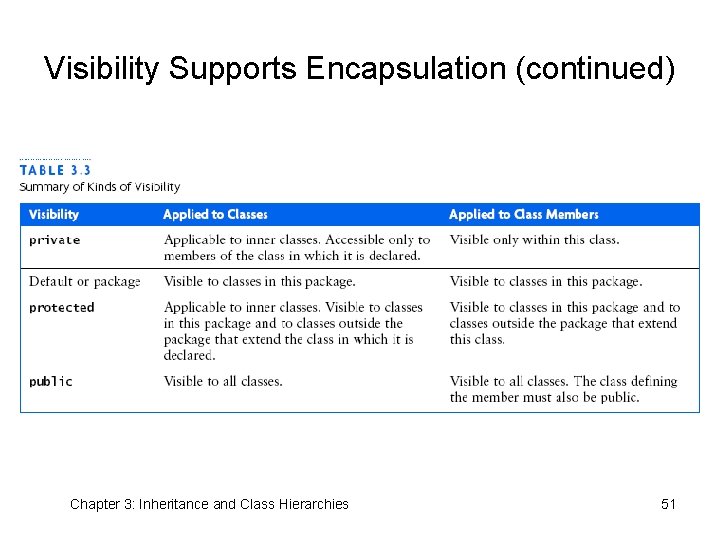
Visibility Supports Encapsulation (continued) Chapter 3: Inheritance and Class Hierarchies 51
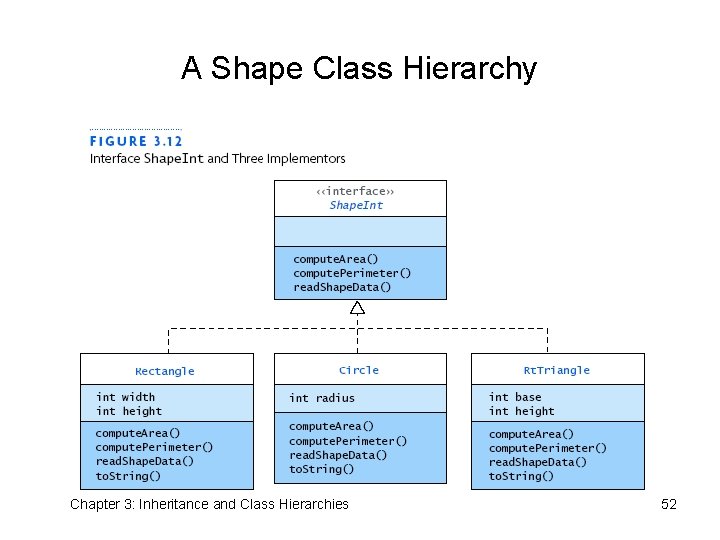
A Shape Class Hierarchy Chapter 3: Inheritance and Class Hierarchies 52
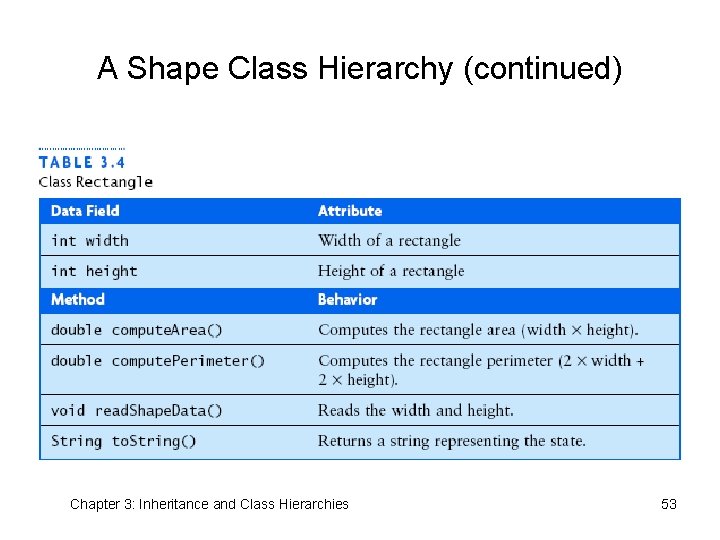
A Shape Class Hierarchy (continued) Chapter 3: Inheritance and Class Hierarchies 53
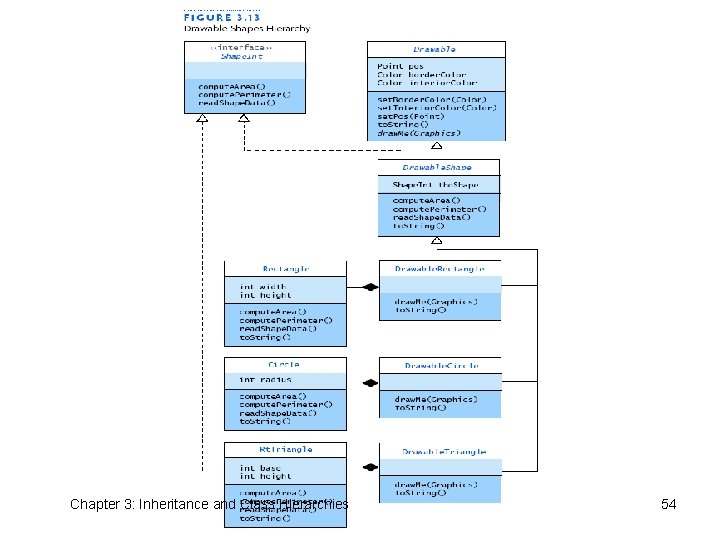
Chapter 3: Inheritance and Class Hierarchies 54
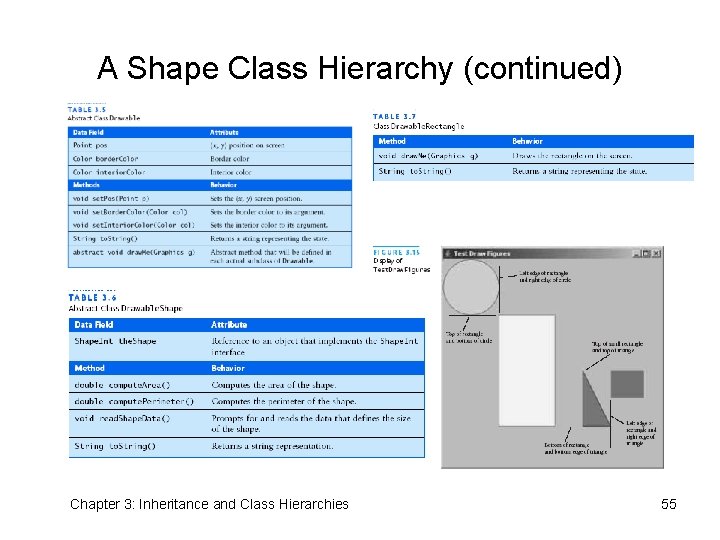
A Shape Class Hierarchy (continued) Chapter 3: Inheritance and Class Hierarchies 55
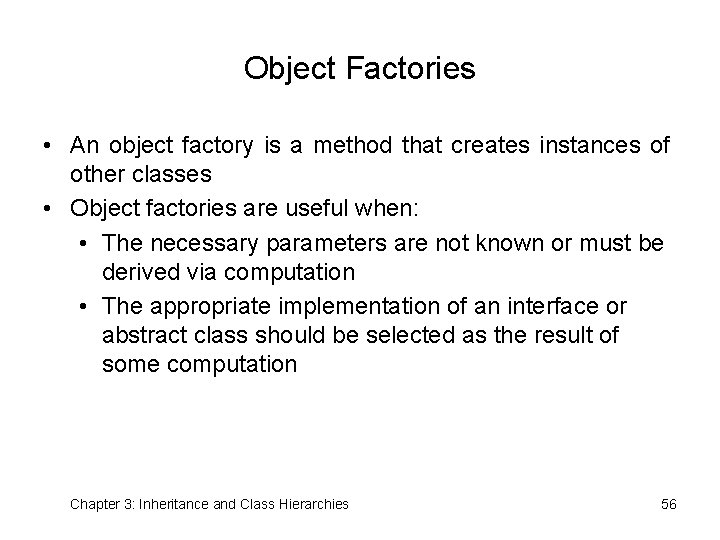
Object Factories • An object factory is a method that creates instances of other classes • Object factories are useful when: • The necessary parameters are not known or must be derived via computation • The appropriate implementation of an interface or abstract class should be selected as the result of some computation Chapter 3: Inheritance and Class Hierarchies 56
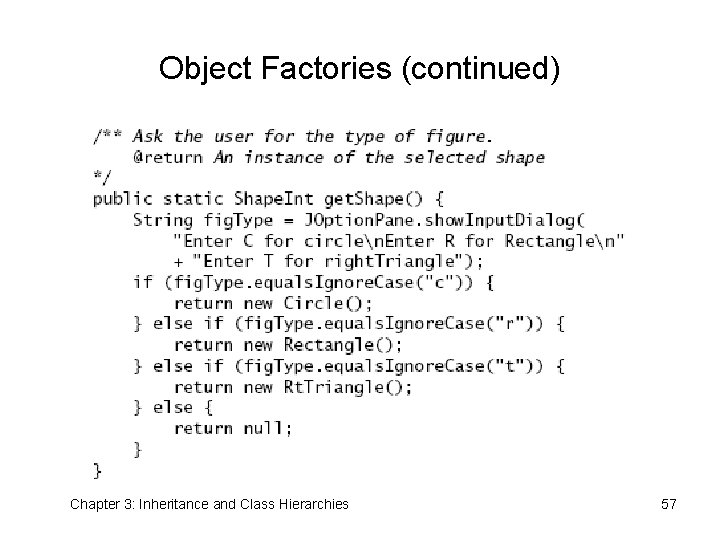
Object Factories (continued) Chapter 3: Inheritance and Class Hierarchies 57
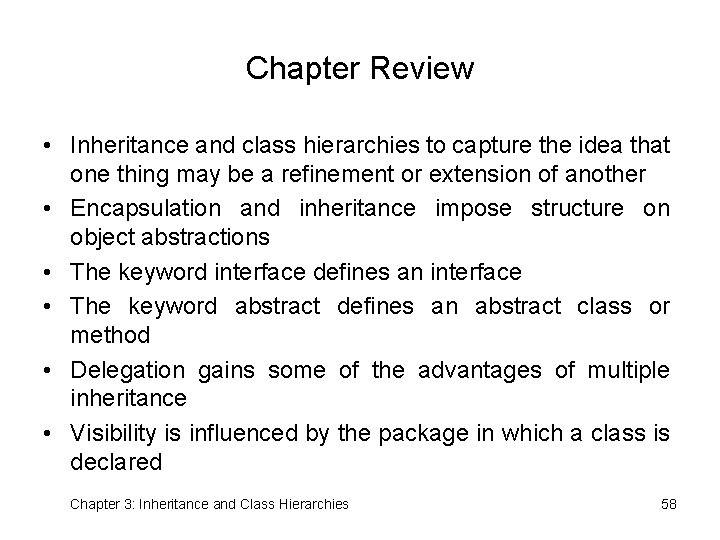
Chapter Review • Inheritance and class hierarchies to capture the idea that one thing may be a refinement or extension of another • Encapsulation and inheritance impose structure on object abstractions • The keyword interface defines an interface • The keyword abstract defines an abstract class or method • Delegation gains some of the advantages of multiple inheritance • Visibility is influenced by the package in which a class is declared Chapter 3: Inheritance and Class Hierarchies 58
Issues for goal hierarchies
Issues for goal hierarchies
Rich feature hierarchies for accurate object detection
Memory storage is never automatic; it always takes effort.
Network software protocol hierarchies
Misguided little unforgivable hierarchies
Protocol hierarchies
Chapter 11 complex inheritance and human heredity test
Section 2 complex patterns of inheritance
Chapter 16 the molecular basis of inheritance
Chapter 15: the chromosomal basis of inheritance
A gene locus is
The molecular basis of inheritance chapter 16
Chapter 15 the chromosomal basis of inheritance
The chromosomal basis of inheritance chapter 15
Chapter 15 the chromosomal basis of inheritance
Chapter 11 section 1 basic patterns of human inheritance
Chapter 11 section 1 basic patterns of human inheritance
Bioflix dna replication
Chapter 9 patterns of inheritance
Chapter 15 the chromosomal basis of inheritance
Abstract class vs concrete class
Abstract concrete class relationship
7 rights of medication administration in order
Java dynamic class loading
Today's class
Package mypackage; class first { /* class body */ }
Mode of grouped data
Class i vs class ii mhc
Relative frequency table meaning
Stimuli vs stimulus
Discrimination training
Class maths student student1 class student string name
What is the class width for the given class (28-33)
In greenfoot, you can cast an actor class to a world class?
Esd class levels
Static class diagram
Class 2 class 3
Public class test subject extends test class
Package mypackage class first class body
Class third class
Uml class diagram inner class
Component class has composite class as collaborator
Mitochondria non examples
Advantages and disadvantages of inheritance in java
Maternal effect and maternal inheritance
Section 12-1 chromosomes and inheritance
Ic code
Inheritance encapsulation
Reproduction
Difference between mendelian and non mendelian inheritance
Mendel's first and second law of inheritance
Sex cat
The cellular basis of reproduction and inheritance
Prisma model inheritance
Uml inheritance
Priority inheritance
Inheritance scala
Inheritance of loss