Graphics Graphics Graphical Spreadsheets Java Pixels and Coordinate
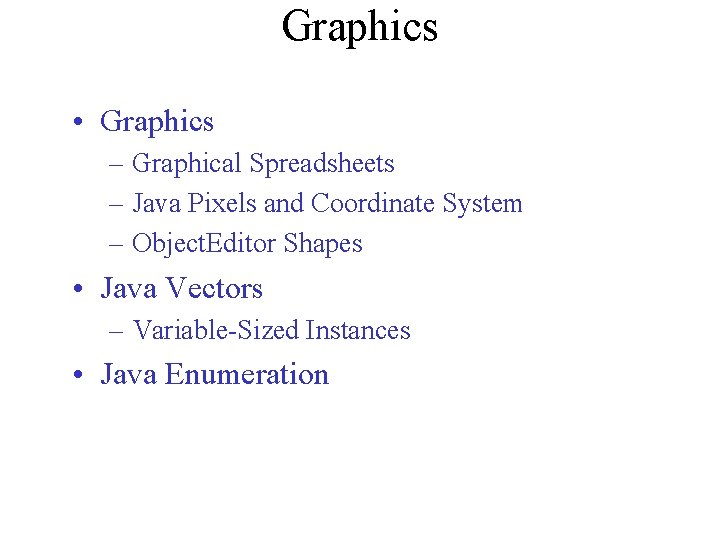
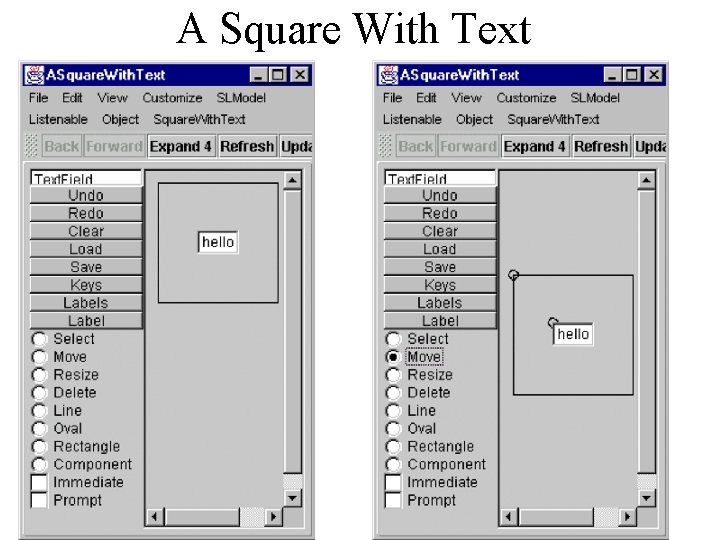
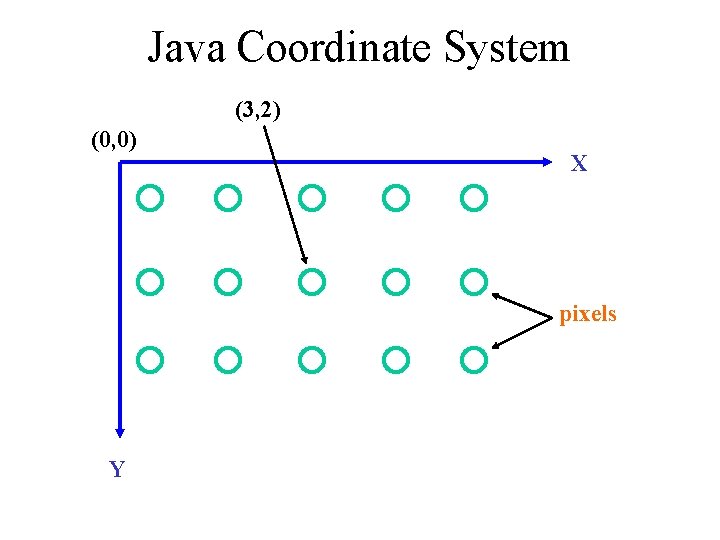
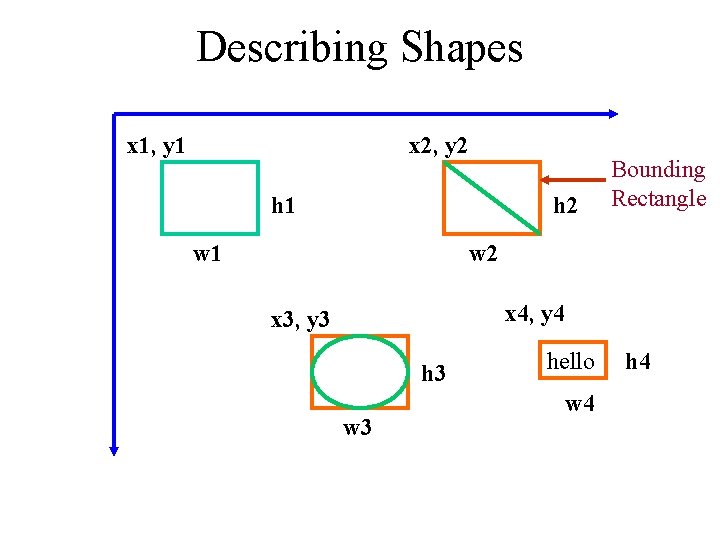
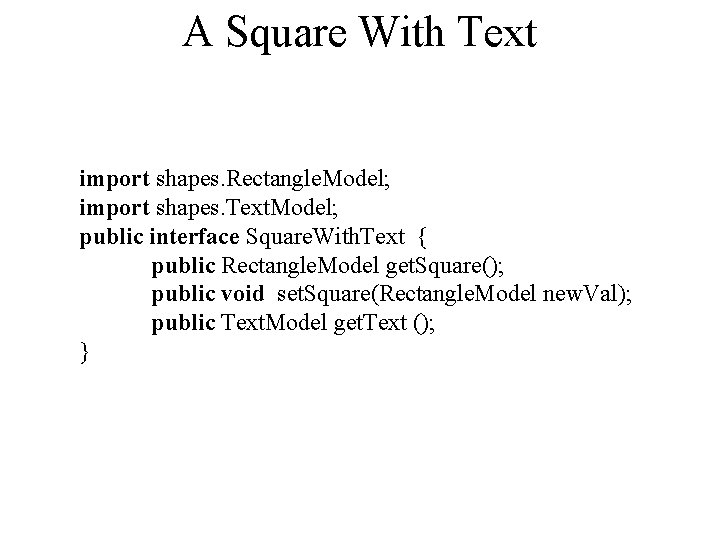
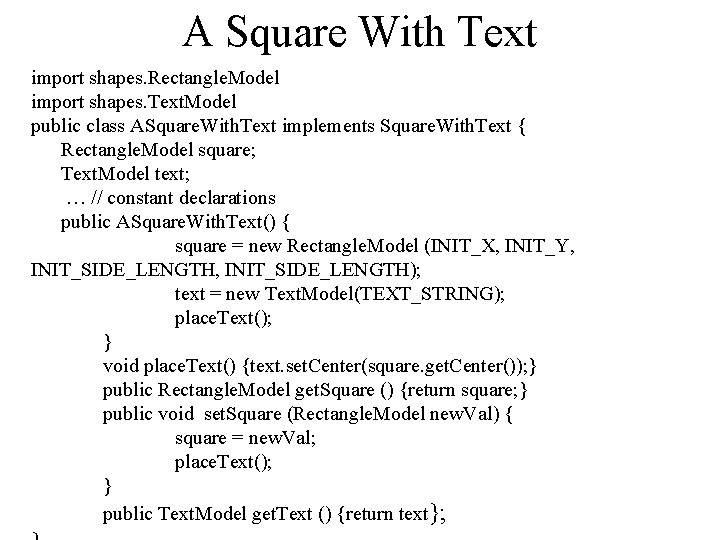
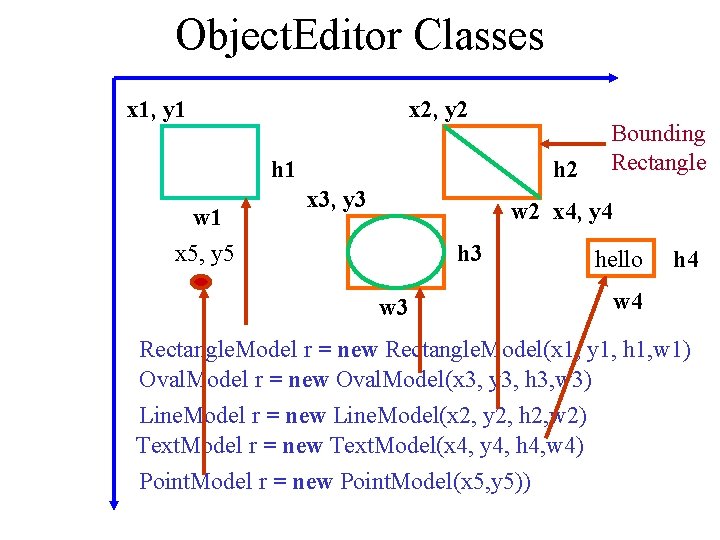
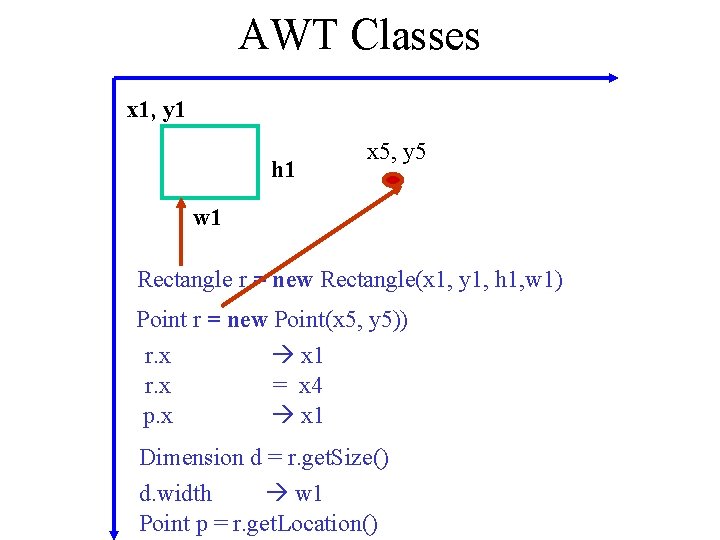
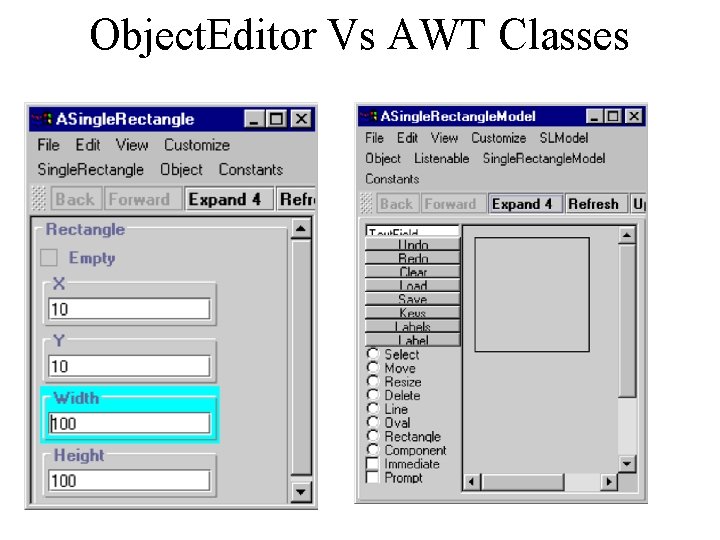
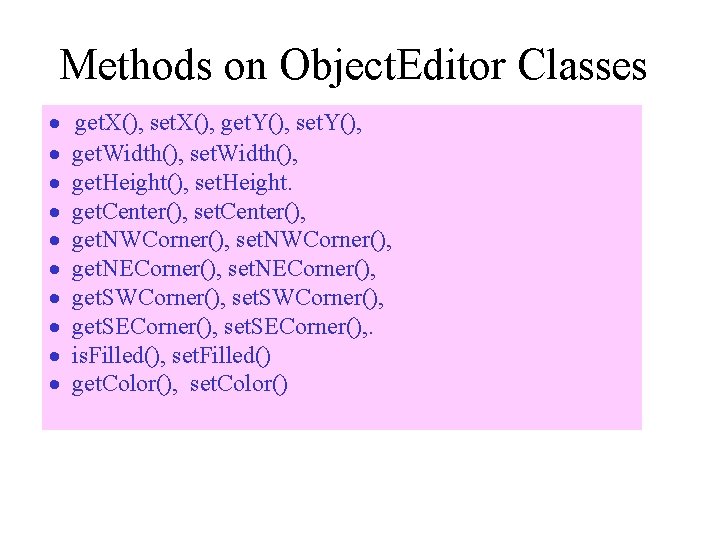
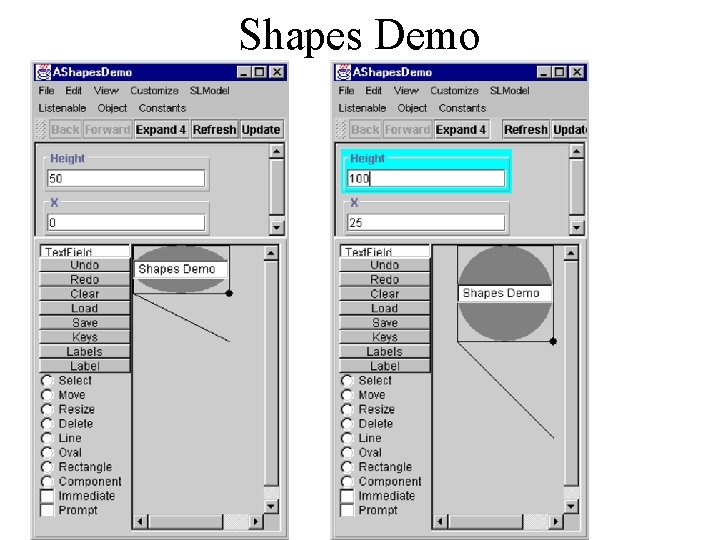
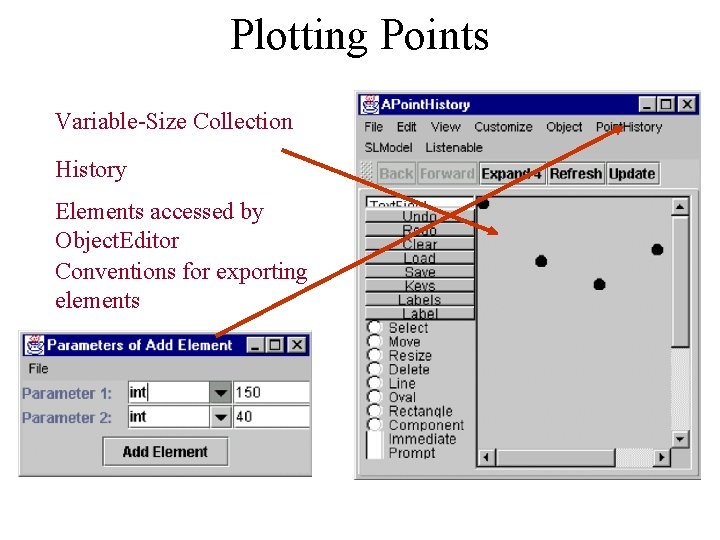
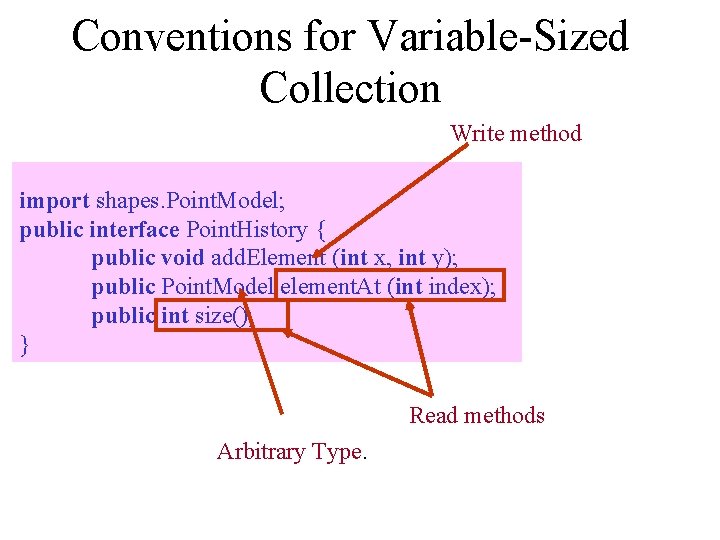
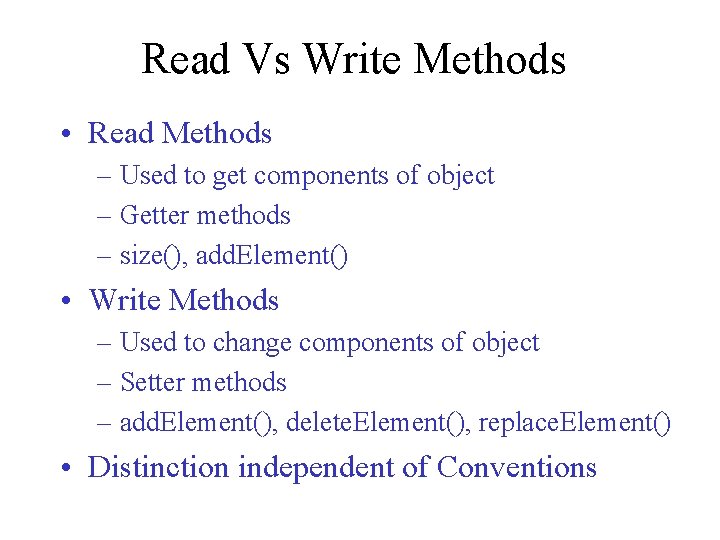
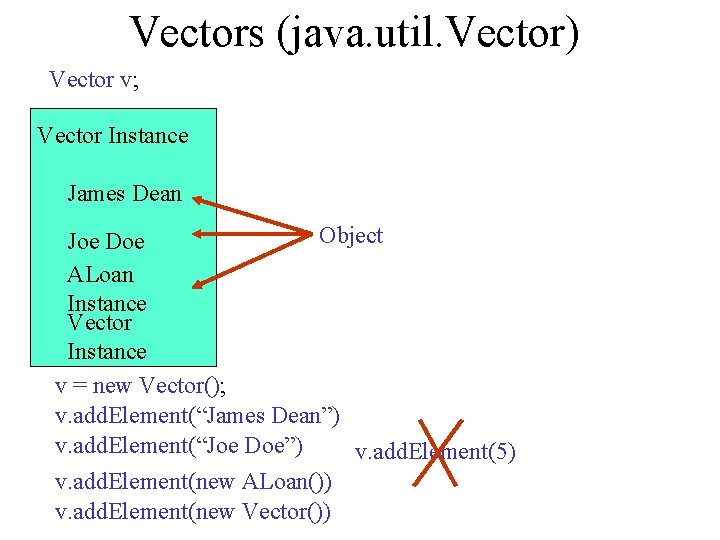
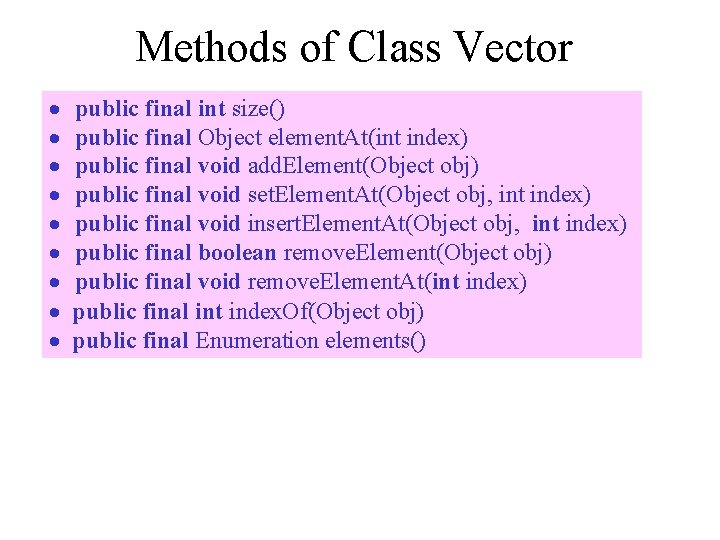
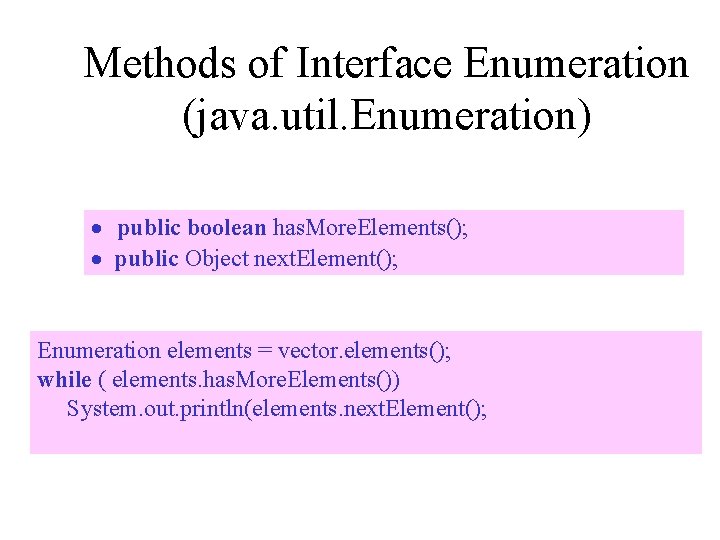
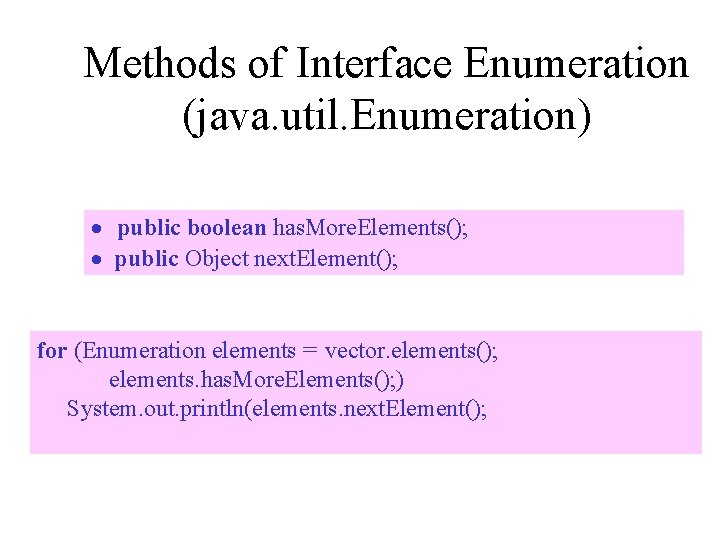
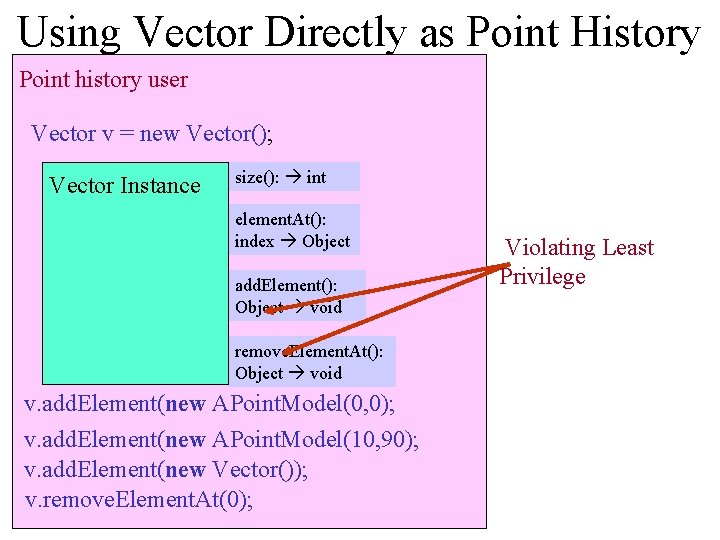
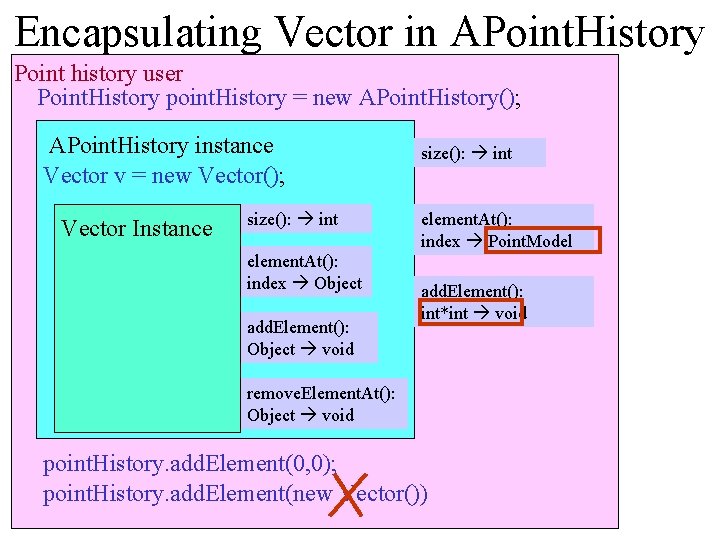
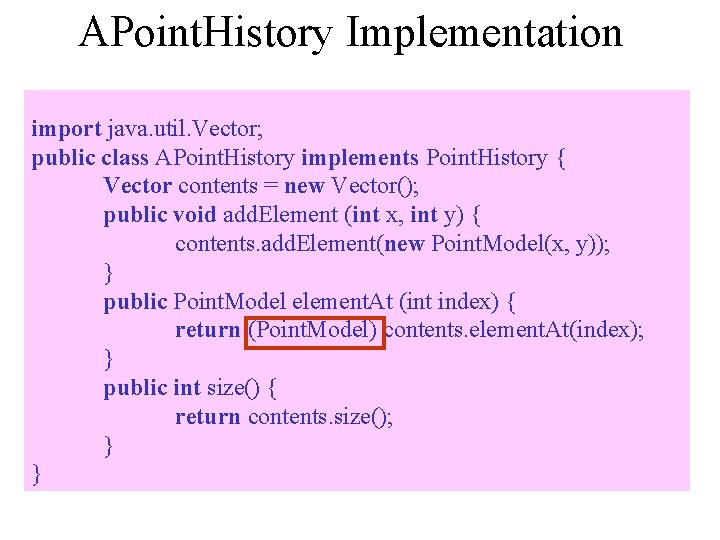
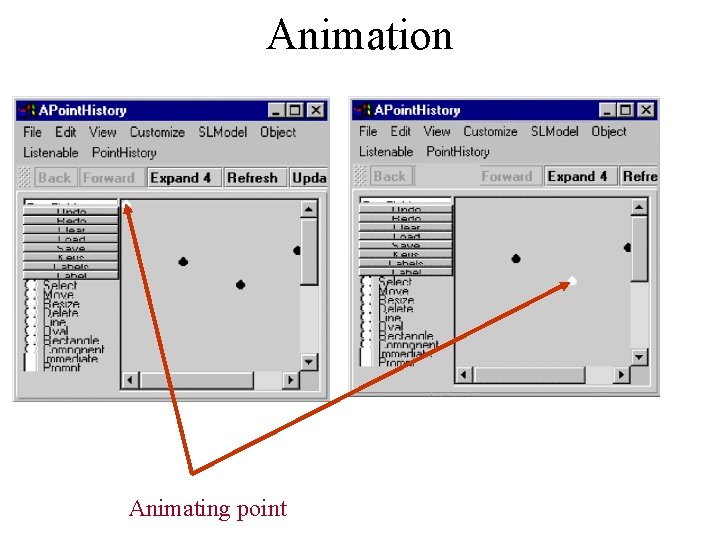
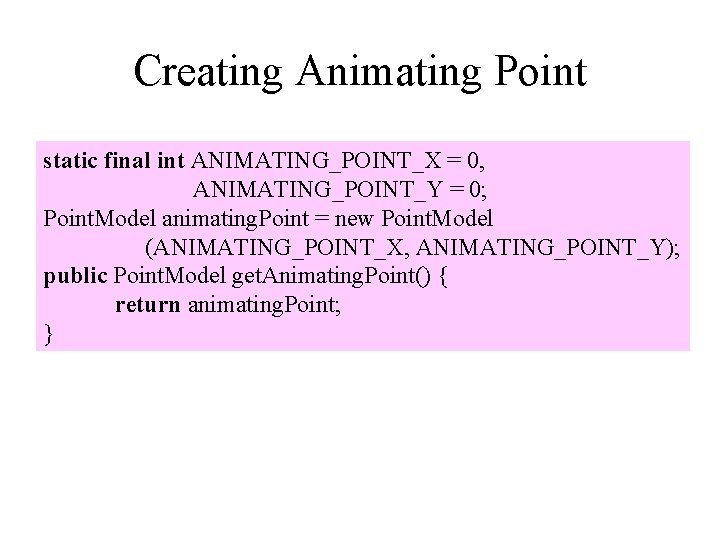
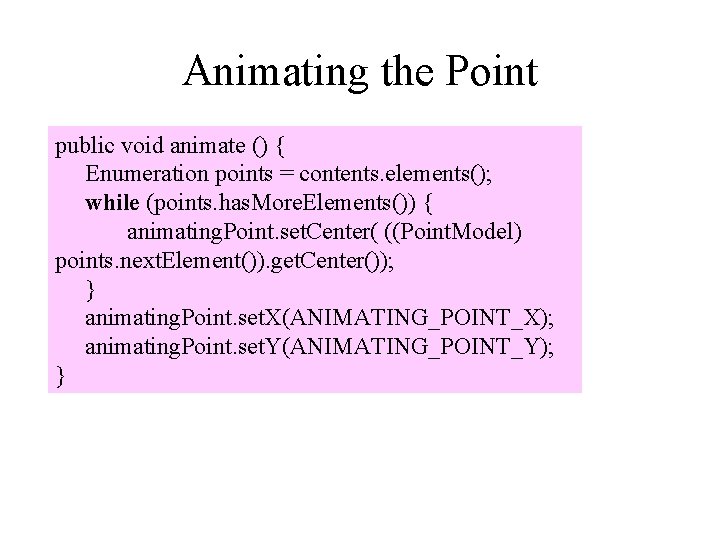
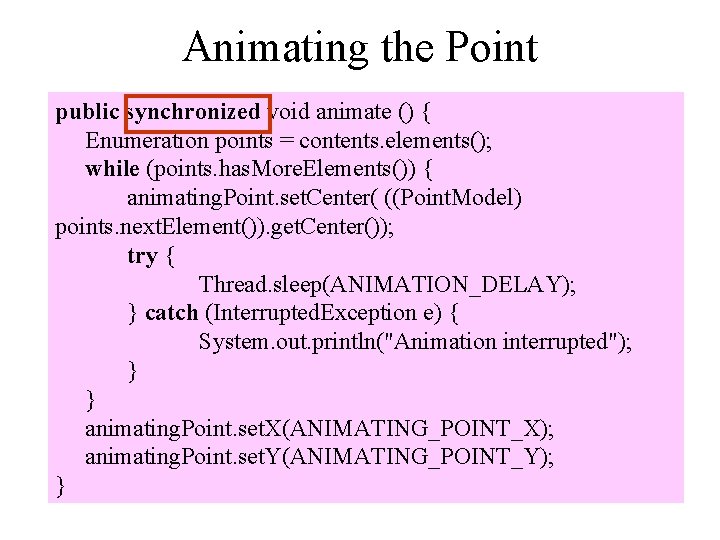
- Slides: 25
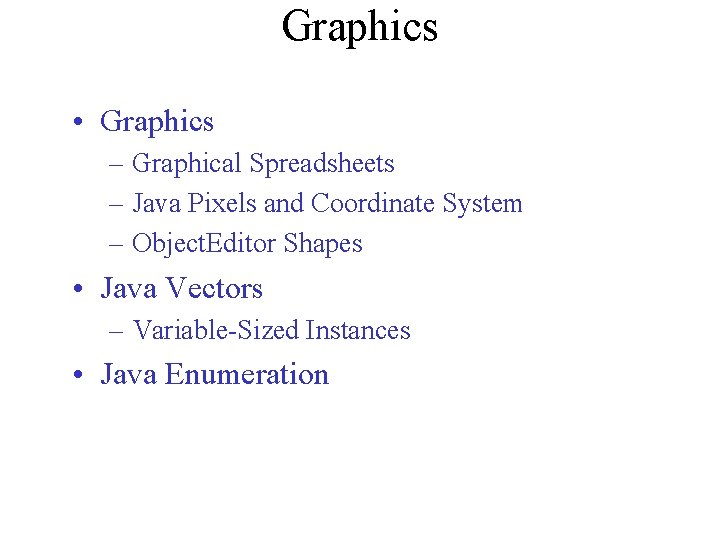
Graphics • Graphics – Graphical Spreadsheets – Java Pixels and Coordinate System – Object. Editor Shapes • Java Vectors – Variable-Sized Instances • Java Enumeration
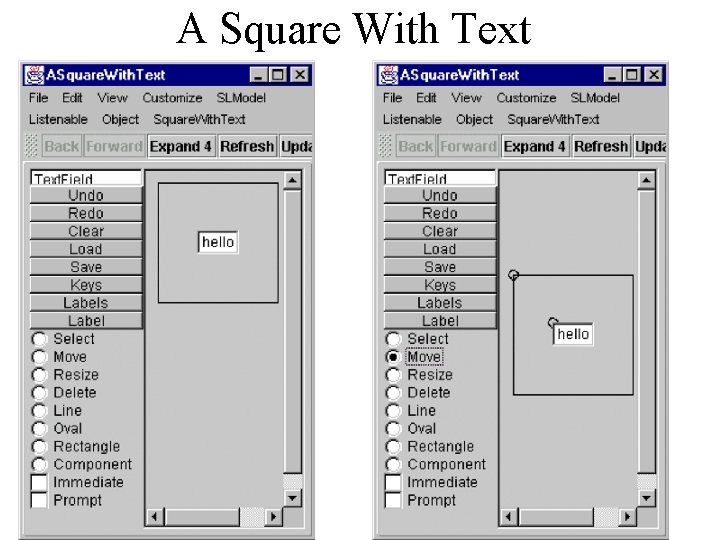
A Square With Text
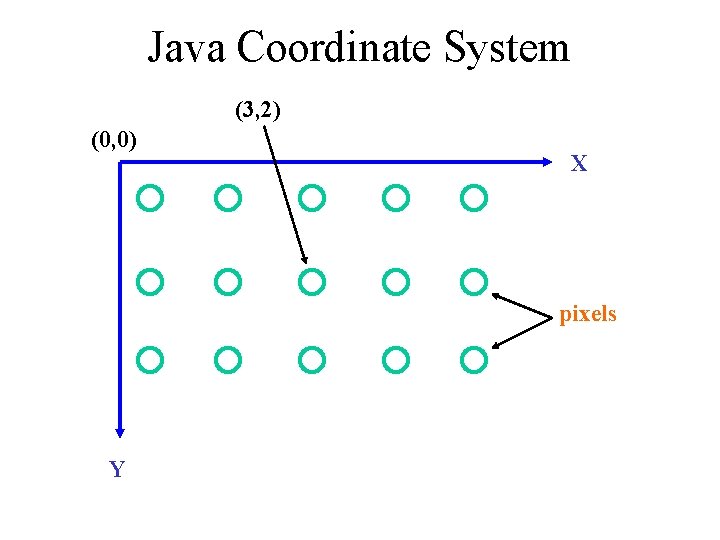
Java Coordinate System (3, 2) (0, 0) X pixels Y
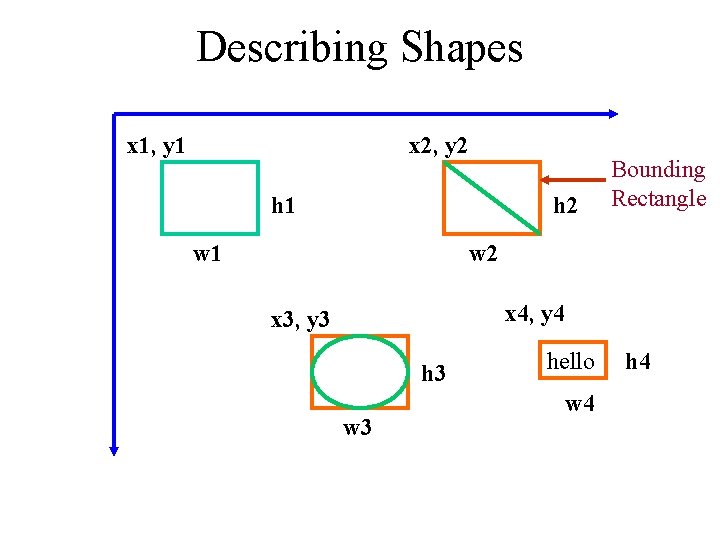
Describing Shapes x 1, y 1 x 2, y 2 h 1 h 2 w 1 Bounding Rectangle w 2 x 4, y 4 x 3, y 3 h 3 w 3 hello w 4 h 4
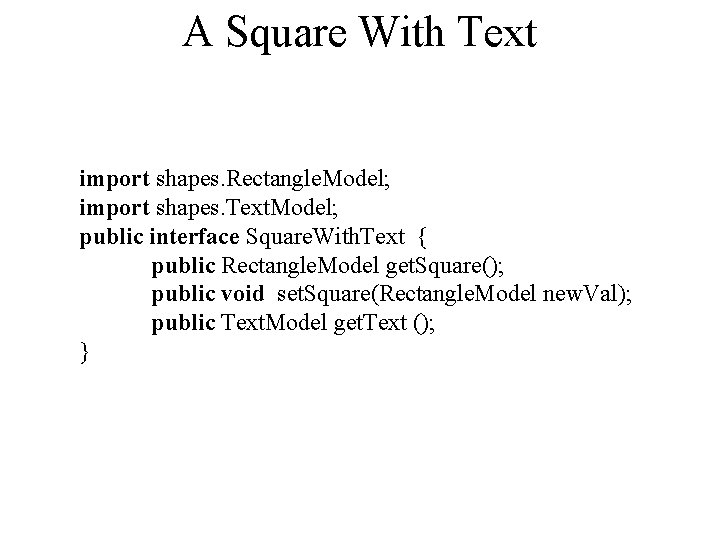
A Square With Text import shapes. Rectangle. Model; import shapes. Text. Model; public interface Square. With. Text { public Rectangle. Model get. Square(); public void set. Square(Rectangle. Model new. Val); public Text. Model get. Text (); }
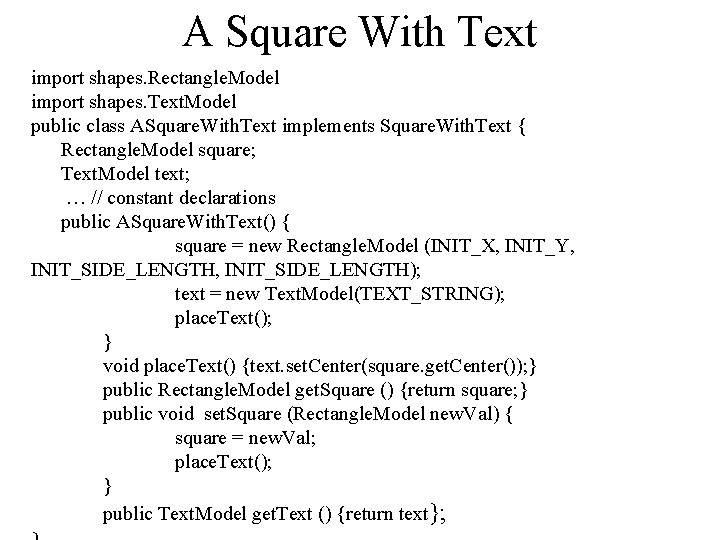
A Square With Text import shapes. Rectangle. Model import shapes. Text. Model public class ASquare. With. Text implements Square. With. Text { Rectangle. Model square; Text. Model text; … // constant declarations public ASquare. With. Text() { square = new Rectangle. Model (INIT_X, INIT_Y, INIT_SIDE_LENGTH); text = new Text. Model(TEXT_STRING); place. Text(); } void place. Text() {text. set. Center(square. get. Center()); } public Rectangle. Model get. Square () {return square; } public void set. Square (Rectangle. Model new. Val) { square = new. Val; place. Text(); } public Text. Model get. Text () {return text};
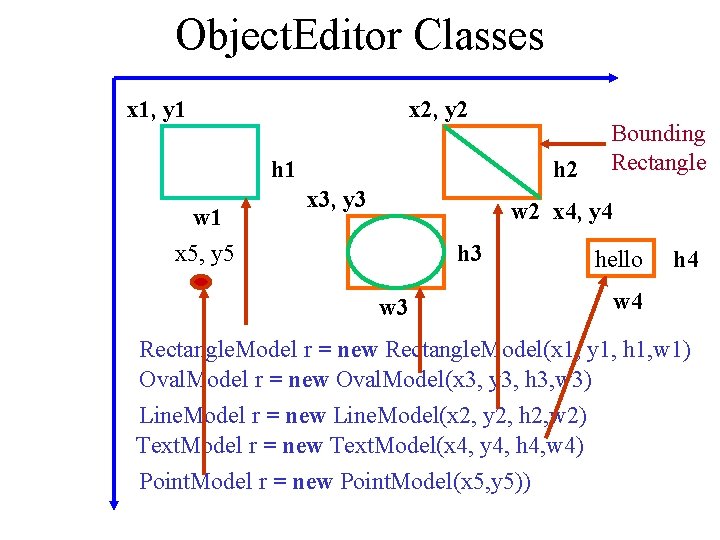
Object. Editor Classes x 1, y 1 x 2, y 2 h 1 w 1 x 5, y 5 h 2 x 3, y 3 Bounding Rectangle w 2 x 4, y 4 h 3 w 3 hello h 4 w 4 Rectangle. Model r = new Rectangle. Model(x 1, y 1, h 1, w 1) Oval. Model r = new Oval. Model(x 3, y 3, h 3, w 3) Line. Model r = new Line. Model(x 2, y 2, h 2, w 2) Text. Model r = new Text. Model(x 4, y 4, h 4, w 4) Point. Model r = new Point. Model(x 5, y 5))
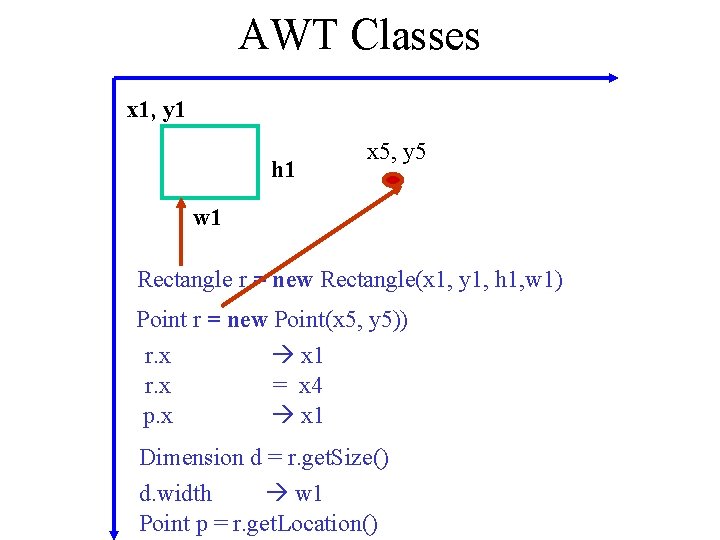
AWT Classes x 1, y 1 h 1 x 5, y 5 w 1 Rectangle r = new Rectangle(x 1, y 1, h 1, w 1) Point r = new Point(x 5, y 5)) r. x x 1 r. x = x 4 p. x x 1 Dimension d = r. get. Size() d. width w 1 Point p = r. get. Location()
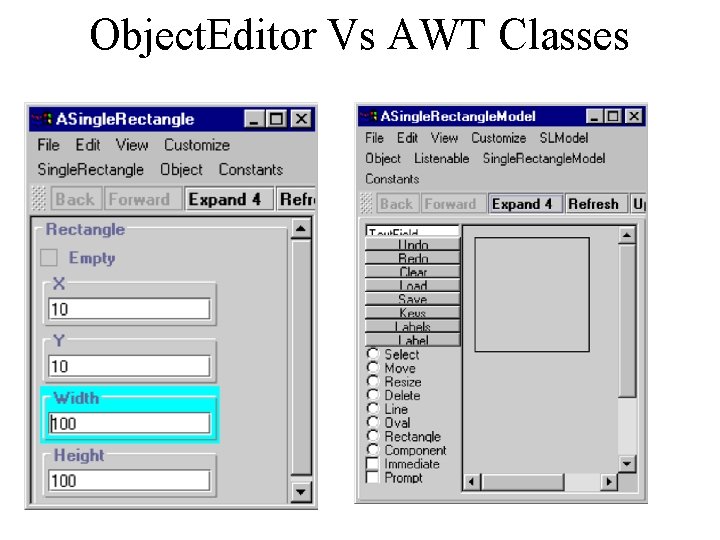
Object. Editor Vs AWT Classes
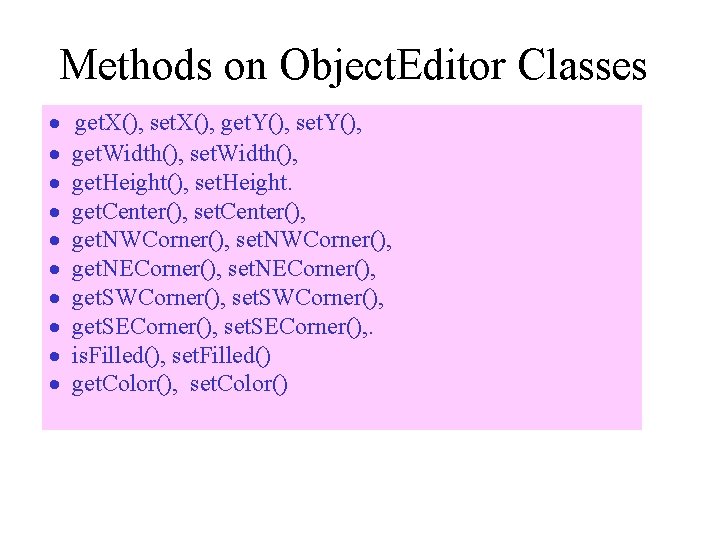
Methods on Object. Editor Classes · · · · · get. X(), set. X(), get. Y(), set. Y(), get. Width(), set. Width(), get. Height(), set. Height. get. Center(), set. Center(), get. NWCorner(), set. NWCorner(), get. NECorner(), set. NECorner(), get. SWCorner(), set. SWCorner(), get. SECorner(), set. SECorner(), . is. Filled(), set. Filled() get. Color(), set. Color()
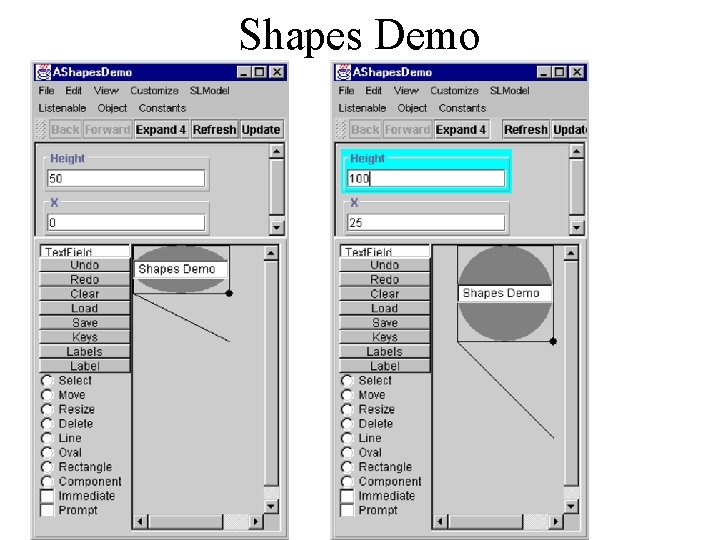
Shapes Demo
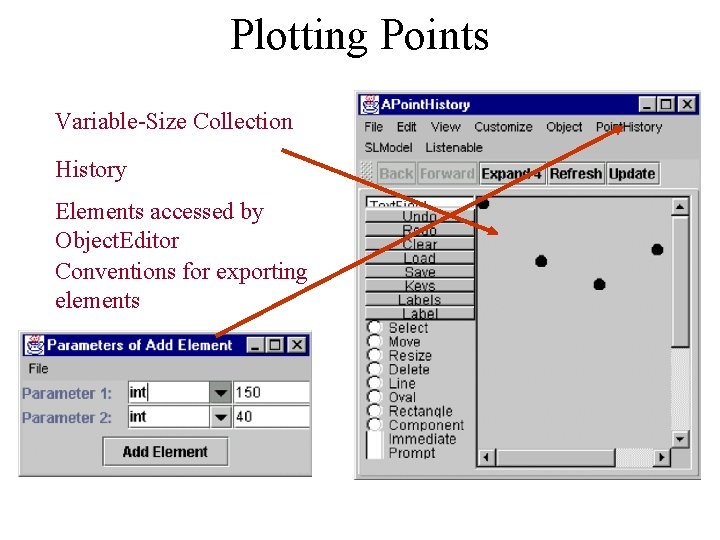
Plotting Points Variable-Size Collection History Elements accessed by Object. Editor Conventions for exporting elements
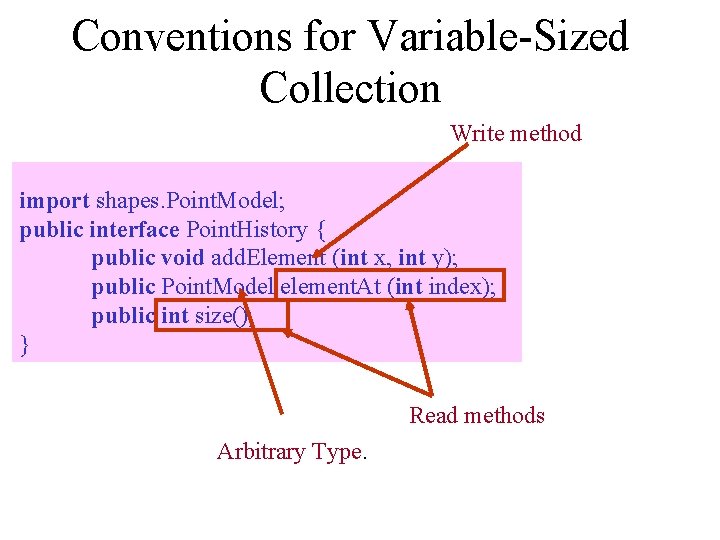
Conventions for Variable-Sized Collection Write method import shapes. Point. Model; public interface Point. History { public void add. Element (int x, int y); public Point. Model element. At (int index); public int size(); } Read methods Arbitrary Type.
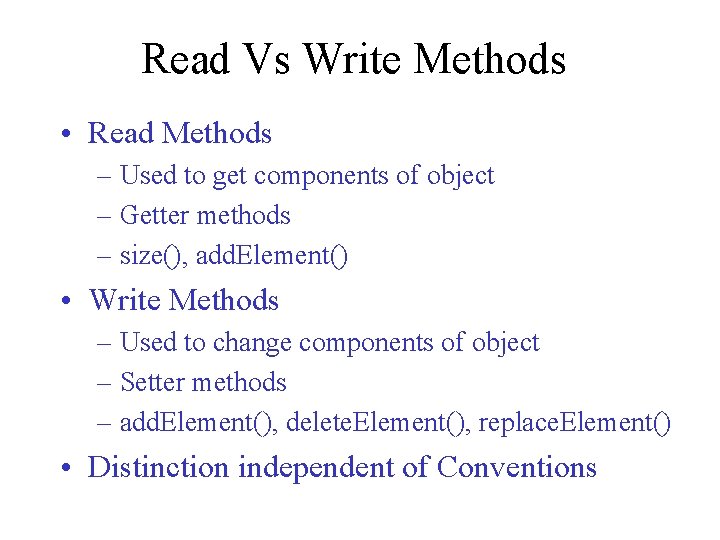
Read Vs Write Methods • Read Methods – Used to get components of object – Getter methods – size(), add. Element() • Write Methods – Used to change components of object – Setter methods – add. Element(), delete. Element(), replace. Element() • Distinction independent of Conventions
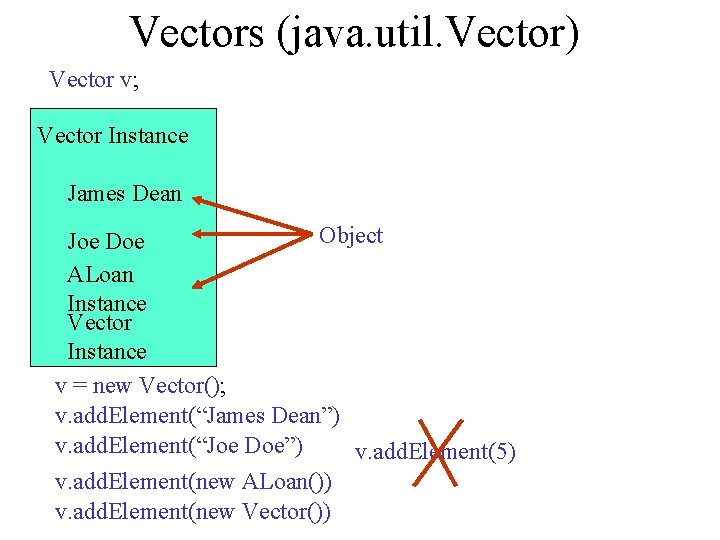
Vectors (java. util. Vector) Vector v; null Instance Vector James Dean Object Joe Doe ALoan Instance Vector Instance v = new Vector(); v. add. Element(“James Dean”) v. add. Element(“Joe Doe”) v. add. Element(5) v. add. Element(new ALoan()) v. add. Element(new Vector())
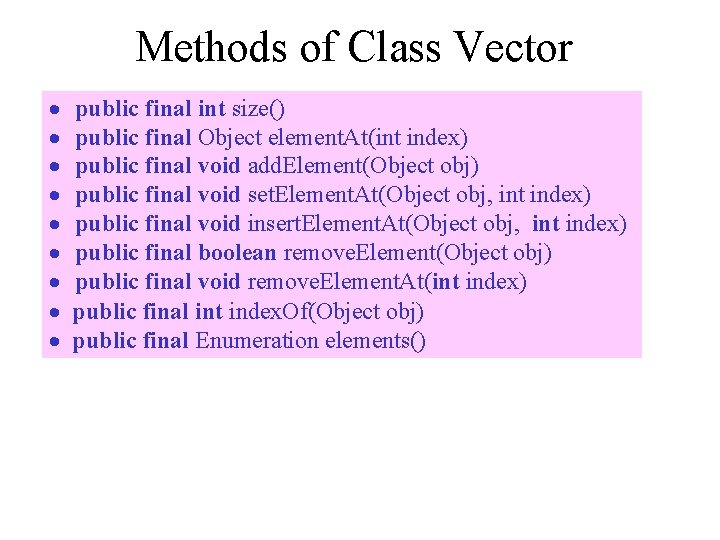
Methods of Class Vector · · · · · public final int size() public final Object element. At(int index) public final void add. Element(Object obj) public final void set. Element. At(Object obj, int index) public final void insert. Element. At(Object obj, int index) public final boolean remove. Element(Object obj) public final void remove. Element. At(int index) public final int index. Of(Object obj) public final Enumeration elements()
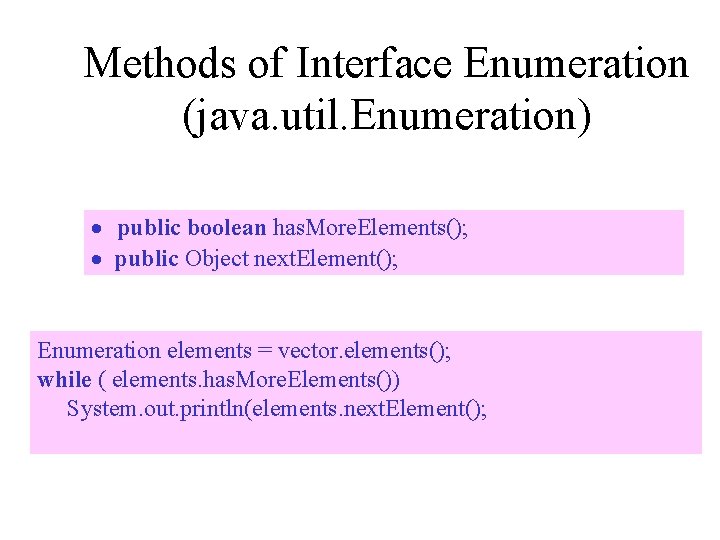
Methods of Interface Enumeration (java. util. Enumeration) · public boolean has. More. Elements(); · public Object next. Element(); Enumeration elements = vector. elements(); while ( elements. has. More. Elements()) System. out. println(elements. next. Element();
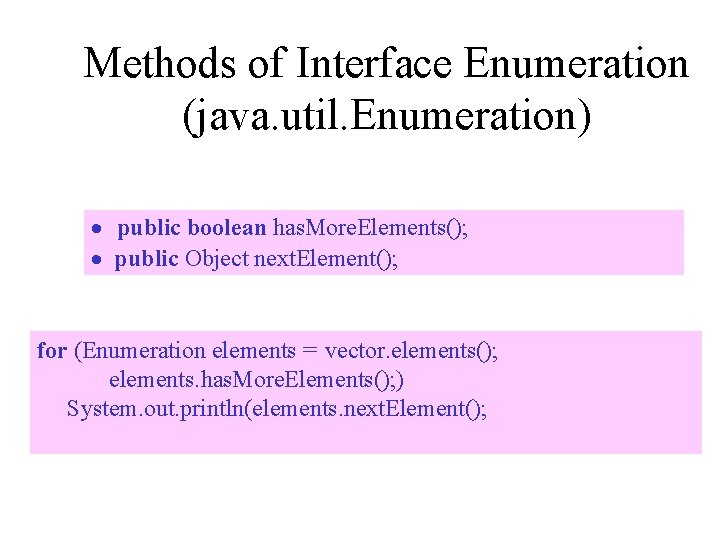
Methods of Interface Enumeration (java. util. Enumeration) · public boolean has. More. Elements(); · public Object next. Element(); for (Enumeration elements = vector. elements(); elements. has. More. Elements(); ) System. out. println(elements. next. Element();
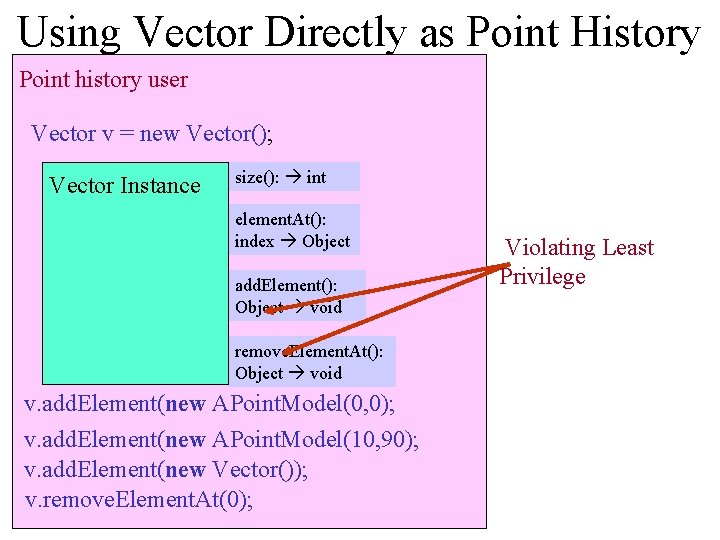
Using Vector Directly as Point History Point history user Vector v = new Vector(); null Instance Vector Point. Model Instance Vector Instance size(): int element. At(): index Object add. Element(): Object void remove. Element. At(): Object void v. add. Element(new APoint. Model(0, 0); v. add. Element(new APoint. Model(10, 90); v. add. Element(new Vector()); v. remove. Element. At(0); Violating Least Privilege
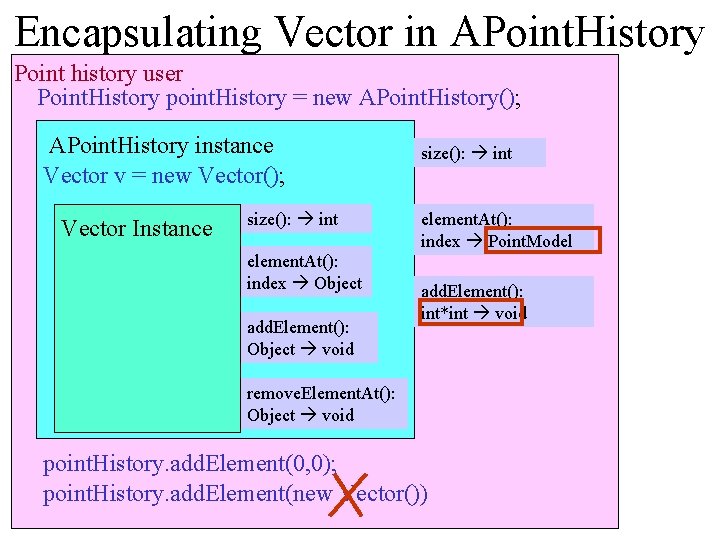
Encapsulating Vector in APoint. History Point history user Point. History point. History = new APoint. History(); APoint. History instance Vector v = new Vector(); null Instance Vector Point. Model Instance size(): int element. At(): index Object add. Element(): Object void size(): int element. At(): index Point. Model add. Element(): int*int void remove. Element. At(): Object void point. History. add. Element(0, 0); point. History. add. Element(new Vector())
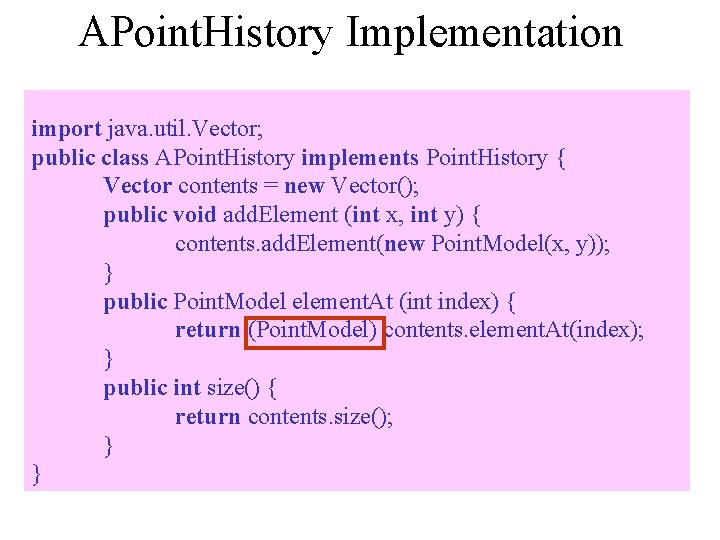
APoint. History Implementation import java. util. Vector; public class APoint. History implements Point. History { Vector contents = new Vector(); public void add. Element (int x, int y) { contents. add. Element(new Point. Model(x, y)); } public Point. Model element. At (int index) { return (Point. Model) contents. element. At(index); } public int size() { return contents. size(); } }
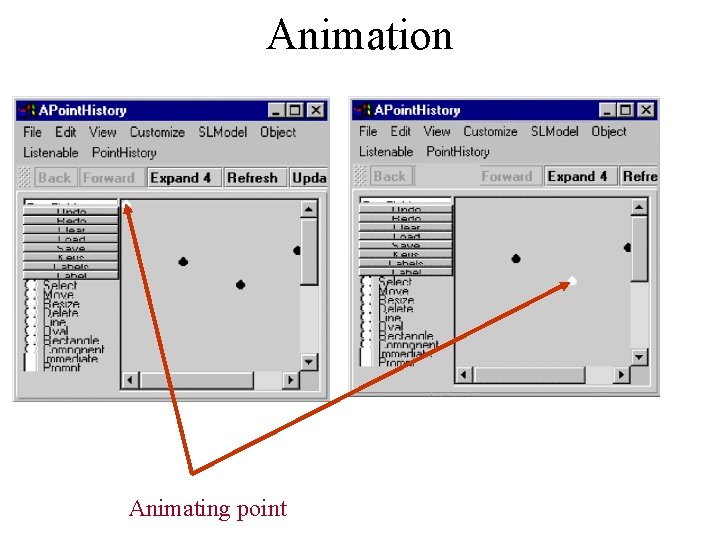
Animation Animating point
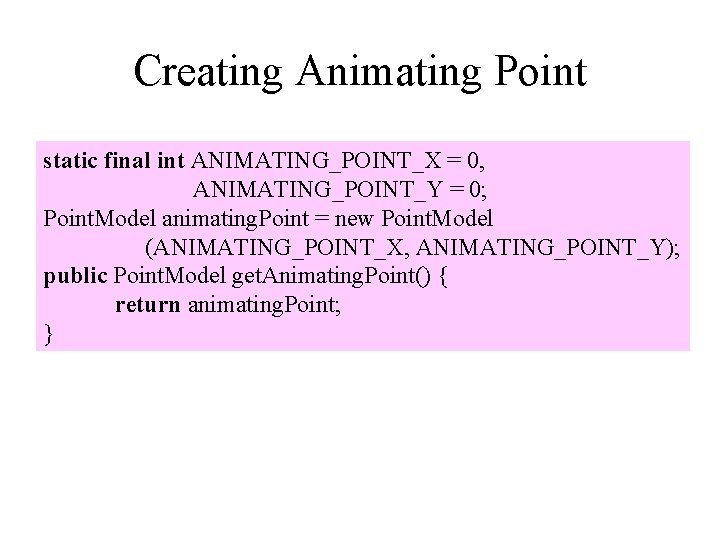
Creating Animating Point static final int ANIMATING_POINT_X = 0, ANIMATING_POINT_Y = 0; Point. Model animating. Point = new Point. Model (ANIMATING_POINT_X, ANIMATING_POINT_Y); public Point. Model get. Animating. Point() { return animating. Point; }
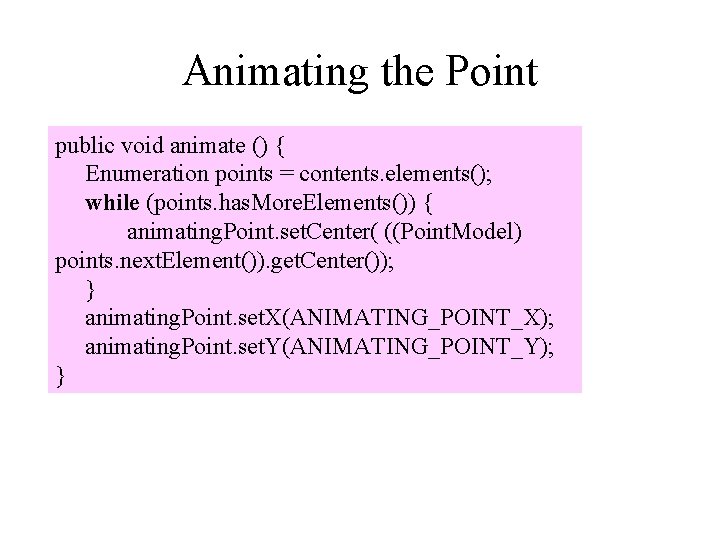
Animating the Point public void animate () { Enumeration points = contents. elements(); while (points. has. More. Elements()) { animating. Point. set. Center( ((Point. Model) points. next. Element()). get. Center()); } animating. Point. set. X(ANIMATING_POINT_X); animating. Point. set. Y(ANIMATING_POINT_Y); }
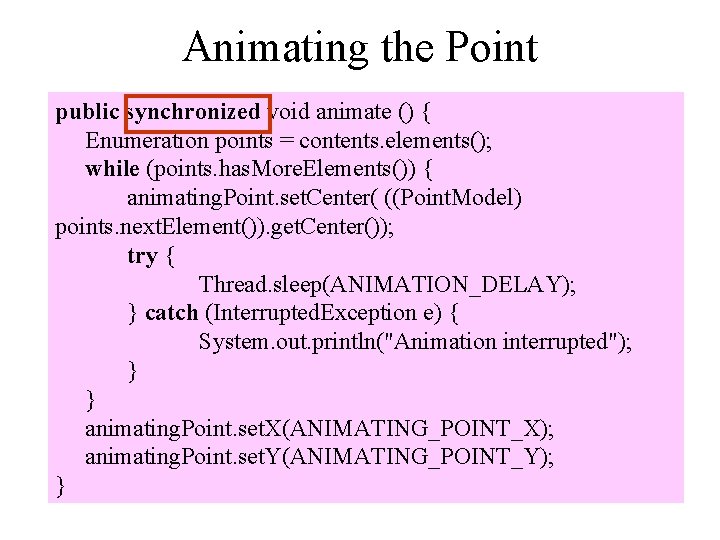
Animating the Point public synchronized void animate () { Enumeration points = contents. elements(); while (points. has. More. Elements()) { animating. Point. set. Center( ((Point. Model) points. next. Element()). get. Center()); try { Thread. sleep(ANIMATION_DELAY); } catch (Interrupted. Exception e) { System. out. println("Animation interrupted"); } } animating. Point. set. X(ANIMATING_POINT_X); animating. Point. set. Y(ANIMATING_POINT_Y); }
The scratch stage is 480 pixels wide and 360 pixels high
What is scrath
800 pixels wide and 200 pixels tall pictures
The scratch stage is 480 pixels wide and 360 pixels high
The scratch stage is 480 pixels wide and 360 pixels high
Mixed adjacency in image processing
The scratch stage is 480 pixels wide and 360 pixels high
Post coordinate indexing system
What is spreadsheet modeling
Coordinate covalent bond vs covalent bond
Spreadsheet vs database
Spreadsheets
Facts about spreadsheets
Introduction to management science with spreadsheets
What is an electronic spreadsheet?
What are the uses of spreadsheet
Management science the art of modeling with spreadsheets
A collection of spreadsheets
Input of graphical data in computer graphics
Java user interface
Coordinate system in computer graphics
Adjacency in image processing examples
Graphic monitor and workstation in computer graphics
Basic relationships between pixels
Ratio math
Hot pixels