Graphics Drawing Canvas canvas new Drawing Canvas Note
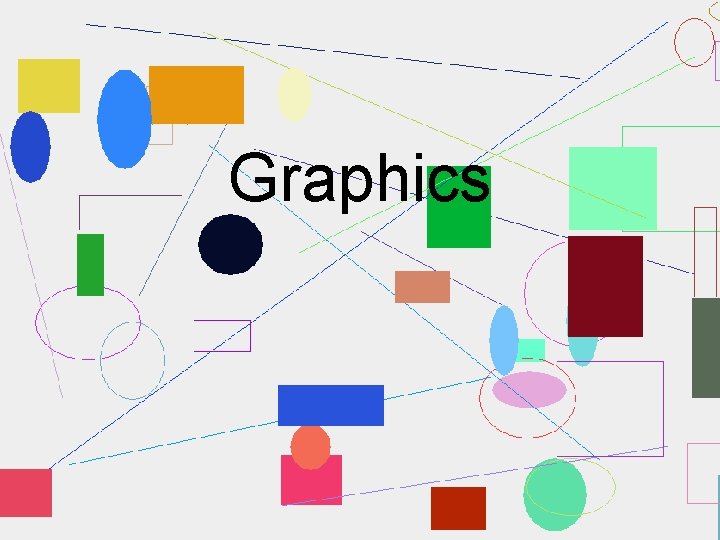
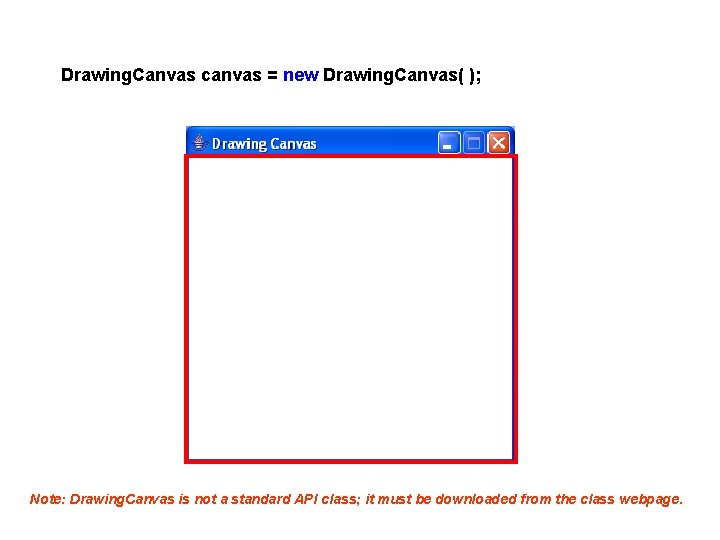
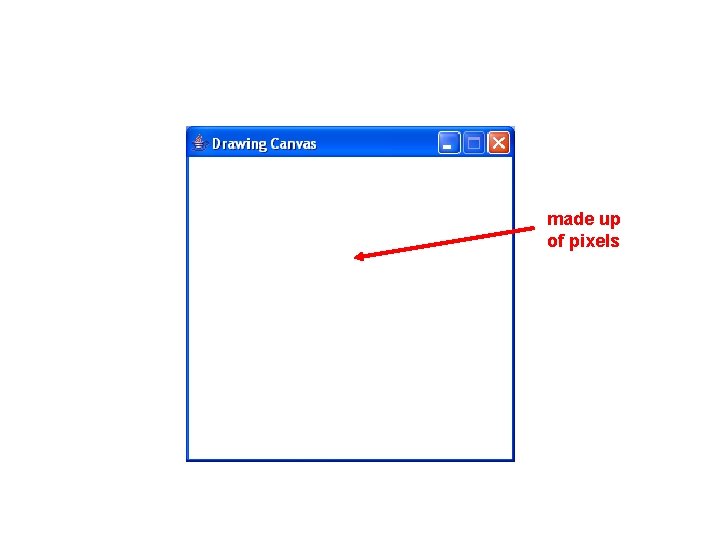
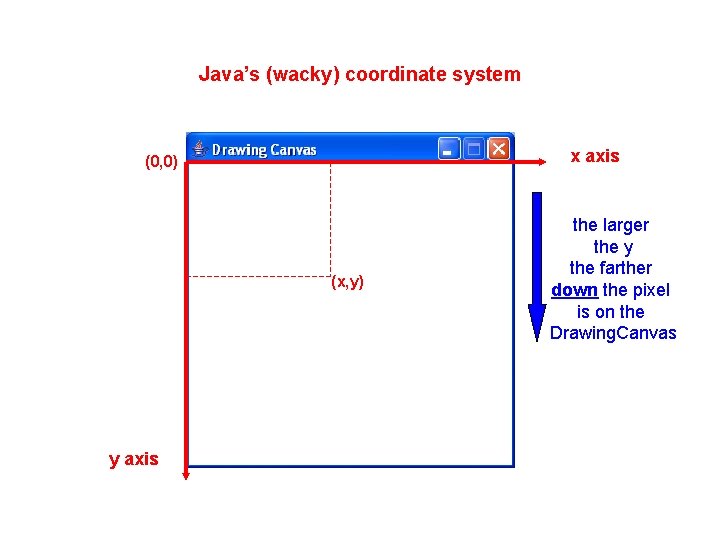
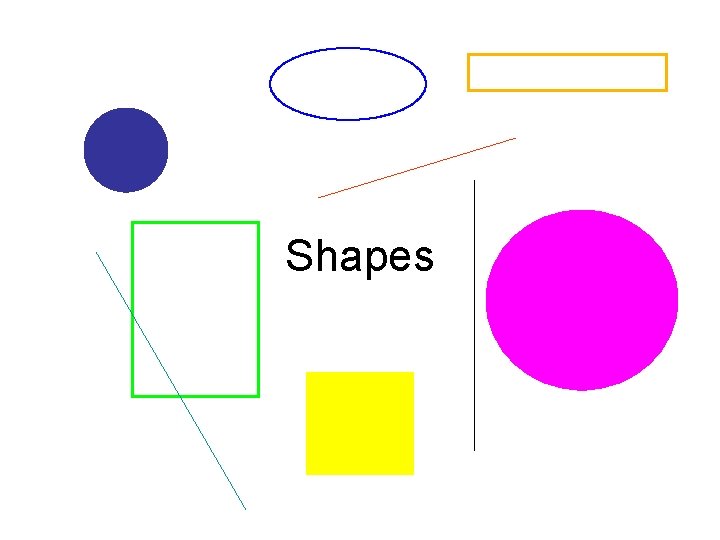
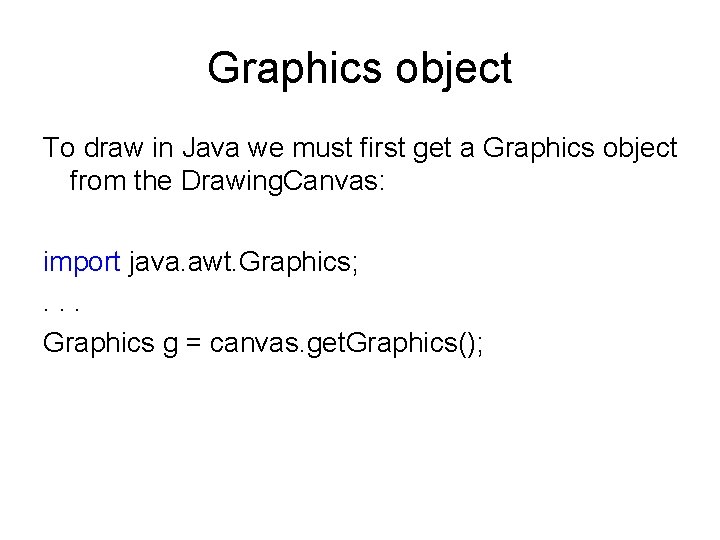
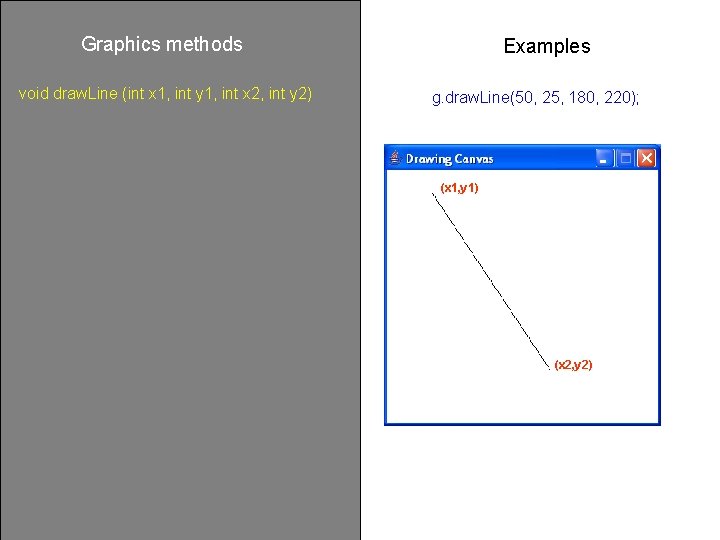
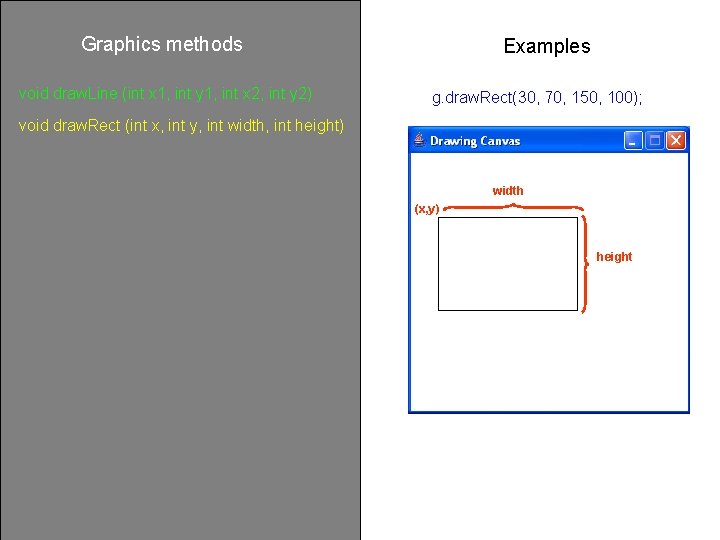
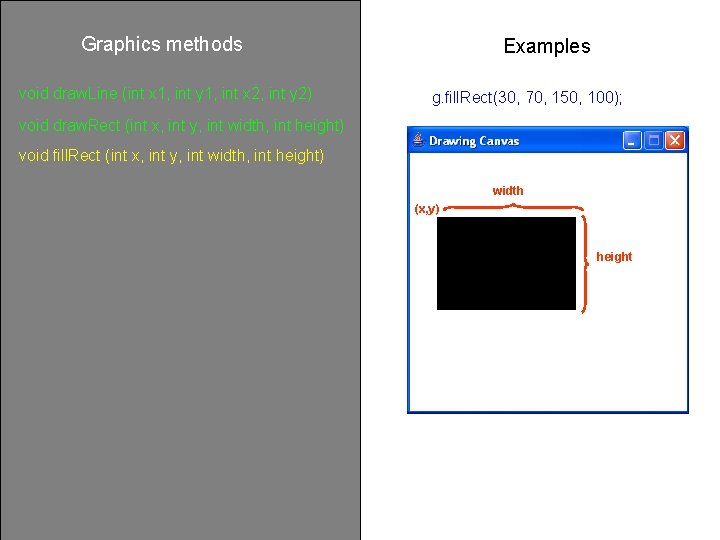
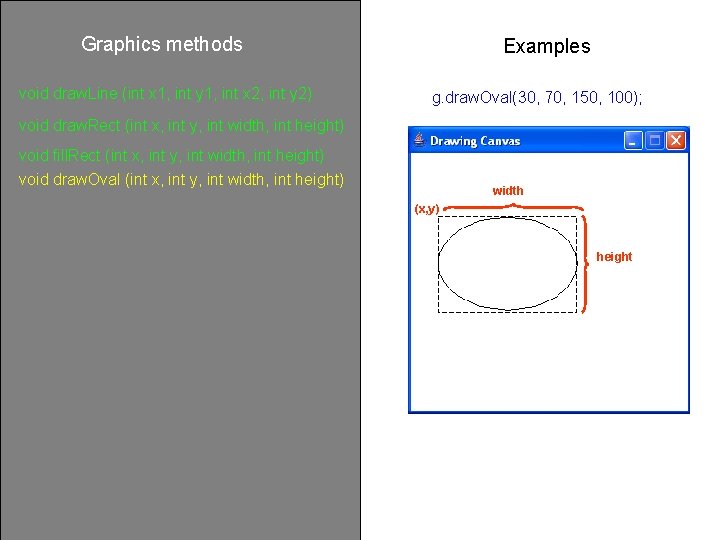
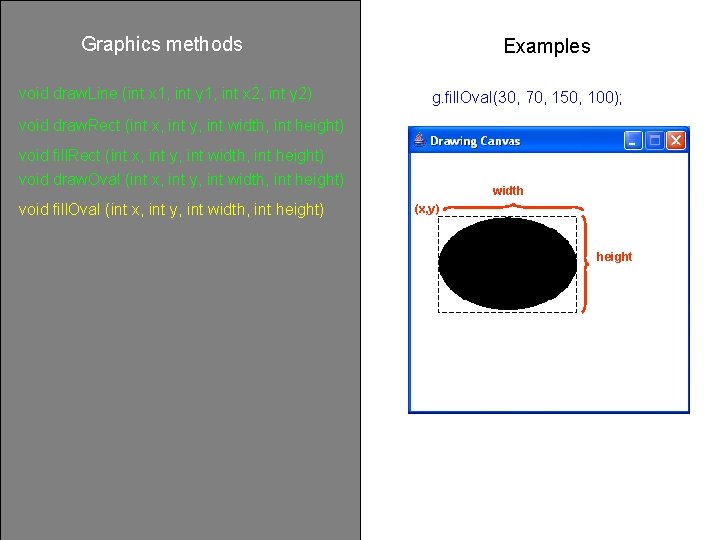
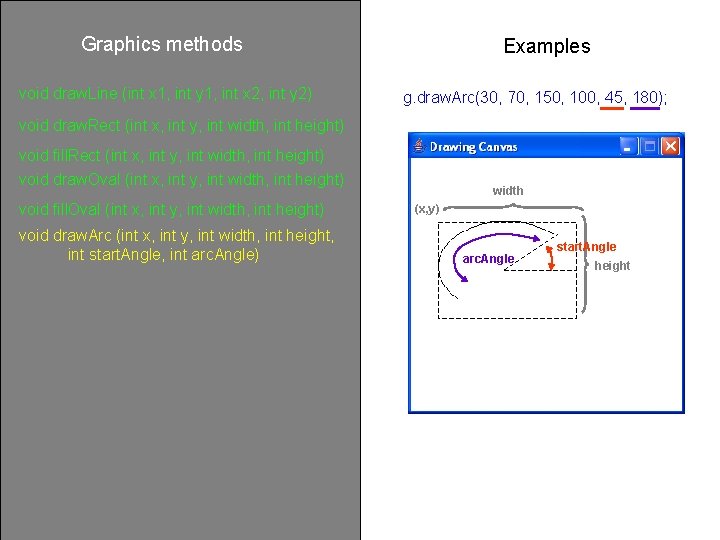
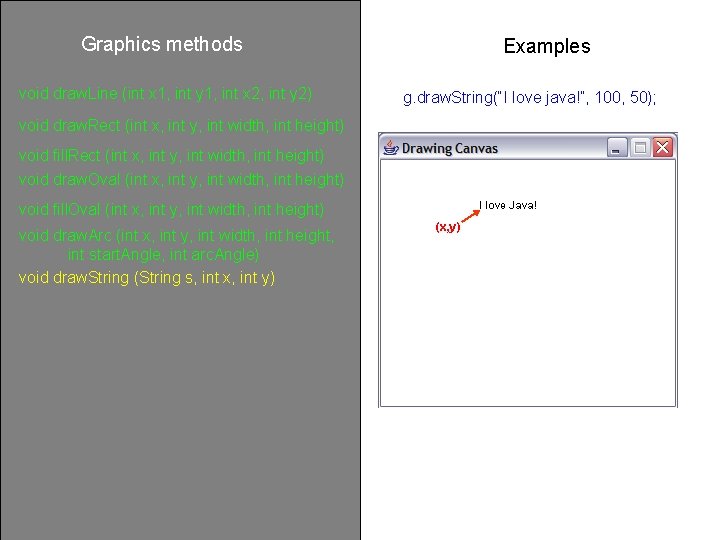
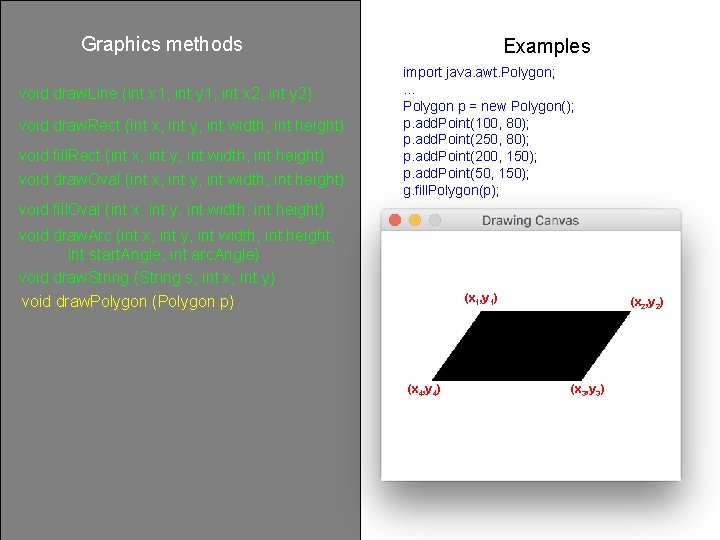
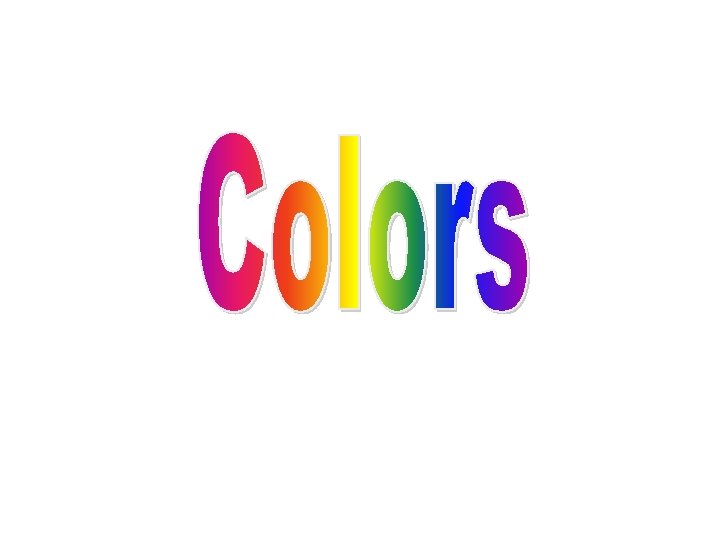
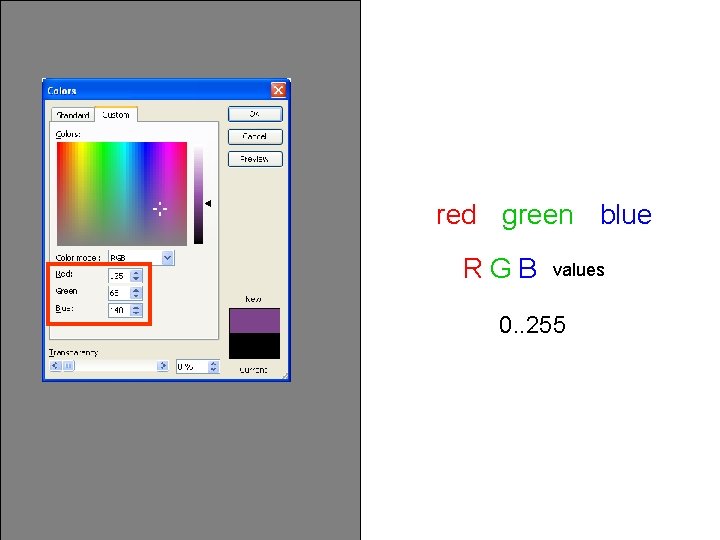
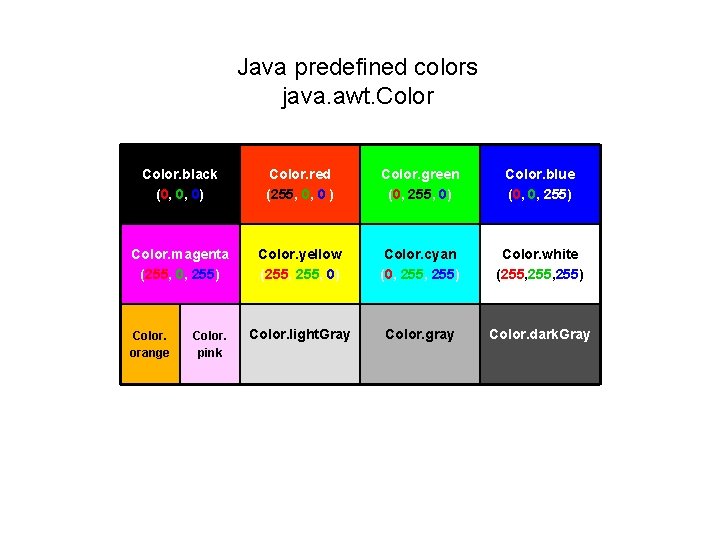
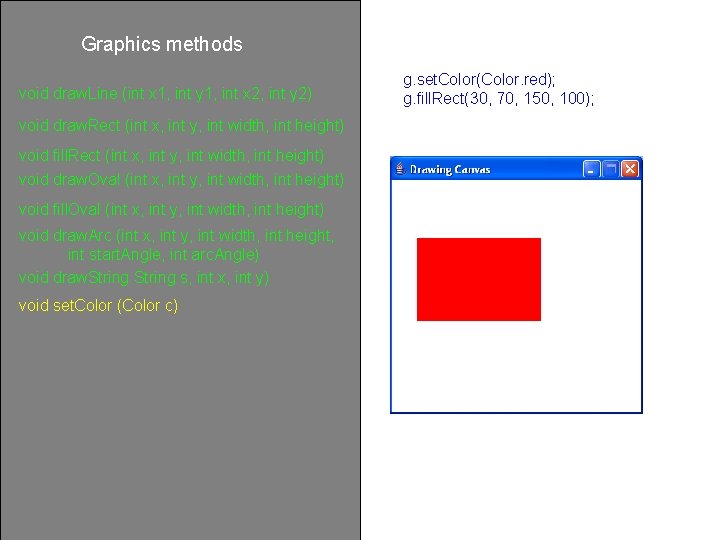
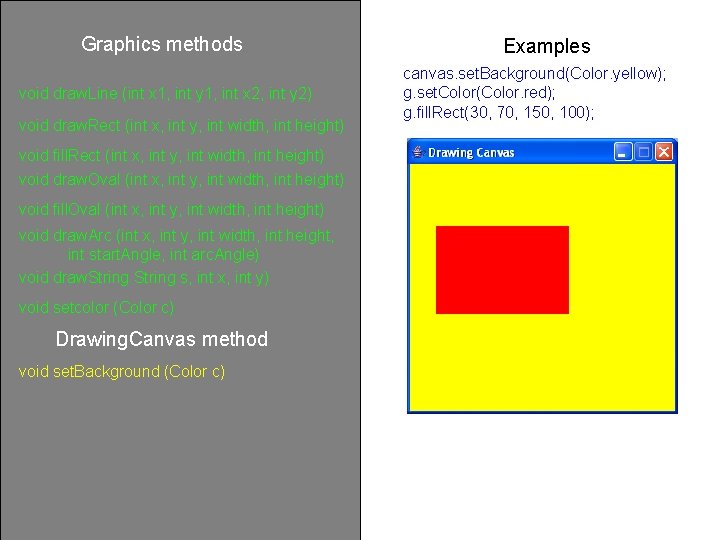
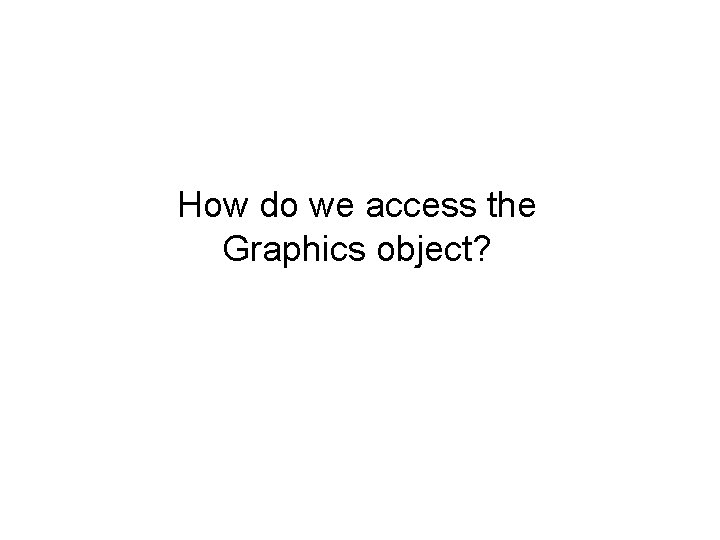
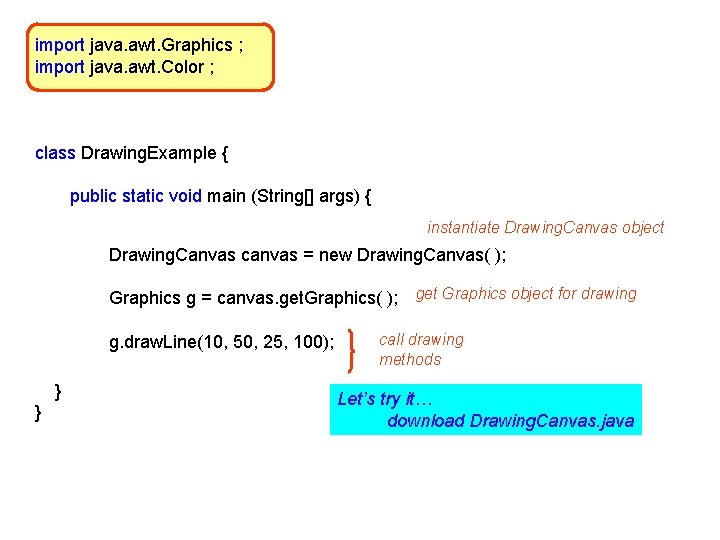
- Slides: 21
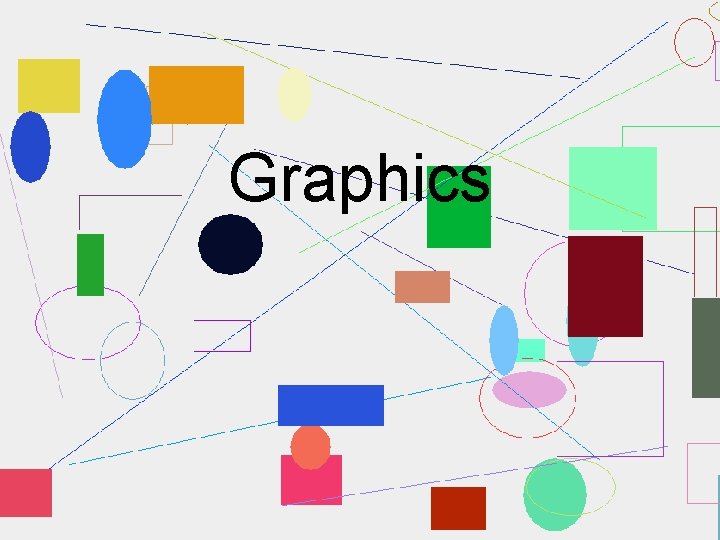
Graphics
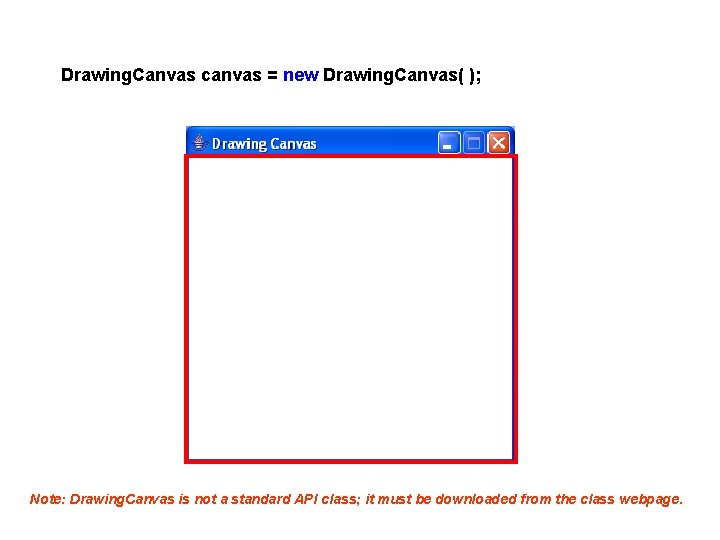
Drawing. Canvas canvas = new Drawing. Canvas( ); Note: Drawing. Canvas is not a standard API class; it must be downloaded from the class webpage.
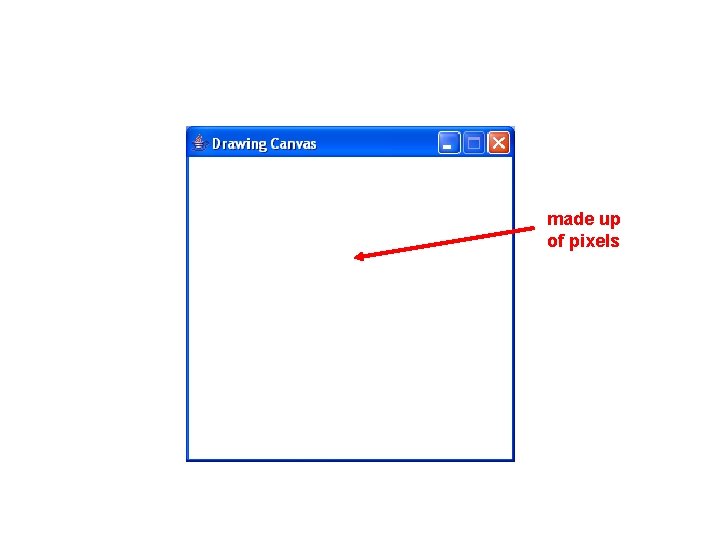
made up of pixels
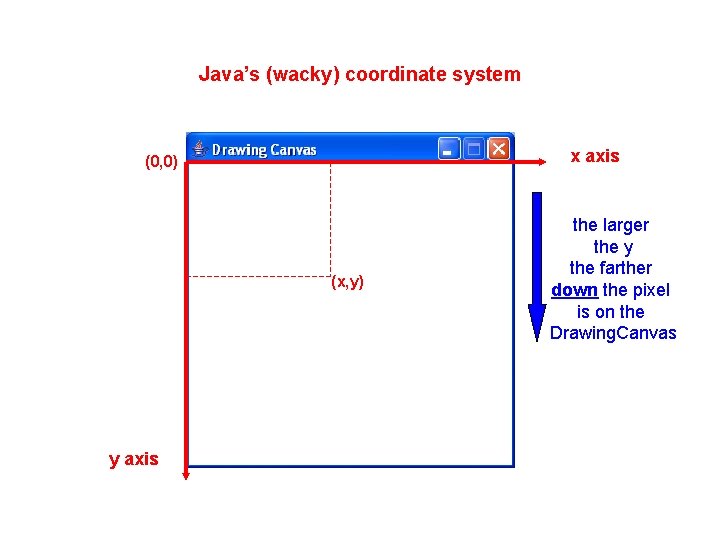
Java’s (wacky) coordinate system x axis (0, 0) (x, y) y axis the larger the y the farther down the pixel is on the Drawing. Canvas
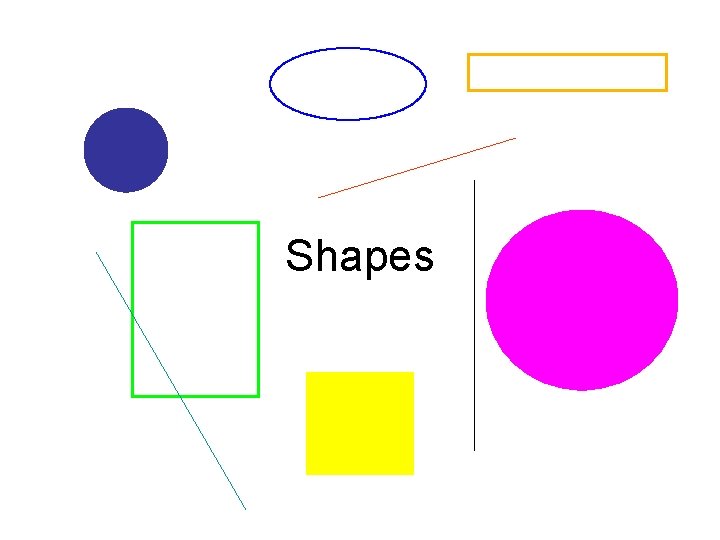
Shapes
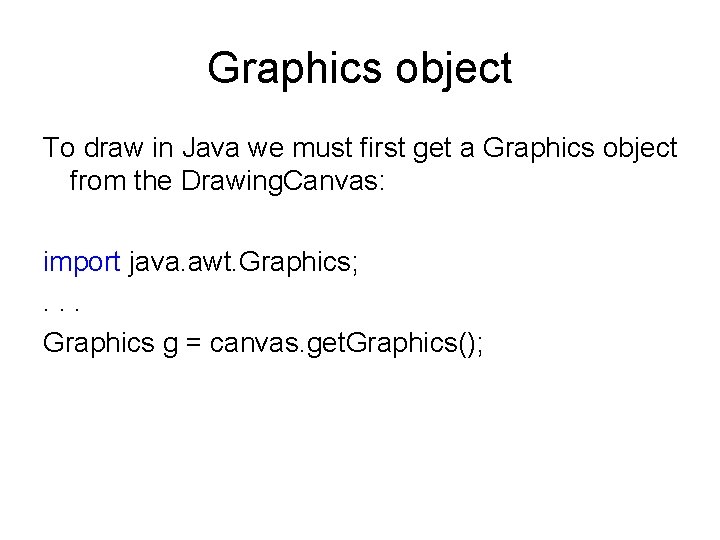
Graphics object To draw in Java we must first get a Graphics object from the Drawing. Canvas: import java. awt. Graphics; . . . Graphics g = canvas. get. Graphics();
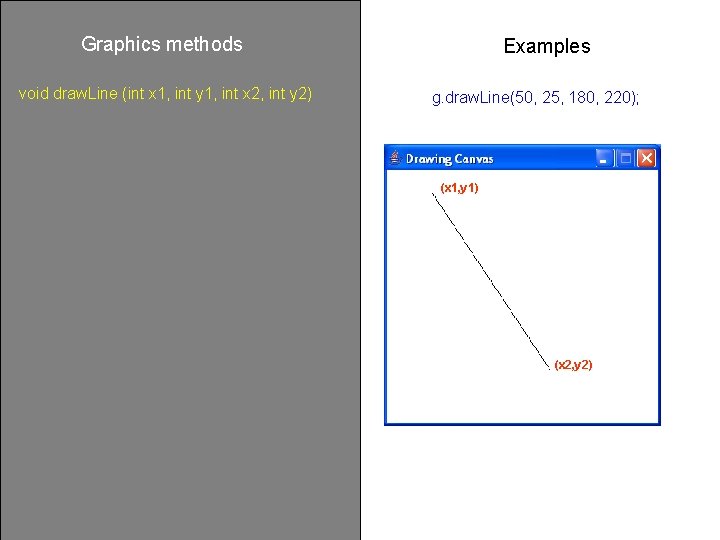
Graphics methods void draw. Line (int x 1, int y 1, int x 2, int y 2) Examples g. draw. Line(50, 25, 180, 220); (x 1, y 1) (x 2, y 2)
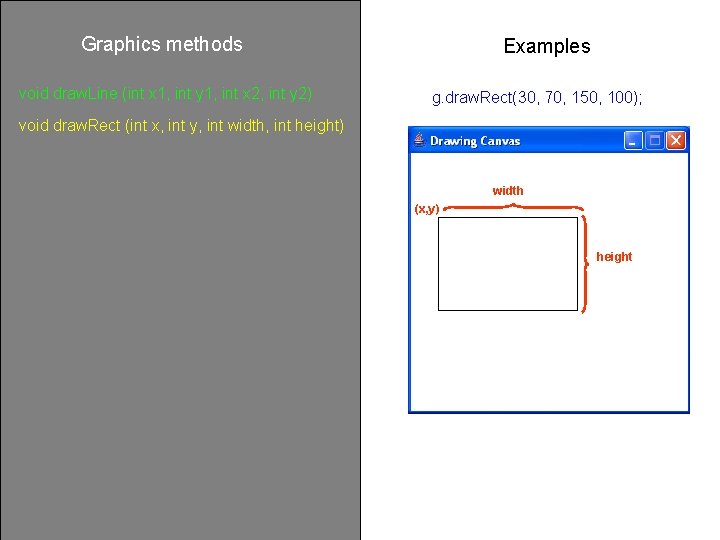
Graphics methods void draw. Line (int x 1, int y 1, int x 2, int y 2) Examples g. draw. Rect(30, 70, 150, 100); void draw. Rect (int x, int y, int width, int height) width (x, y) height
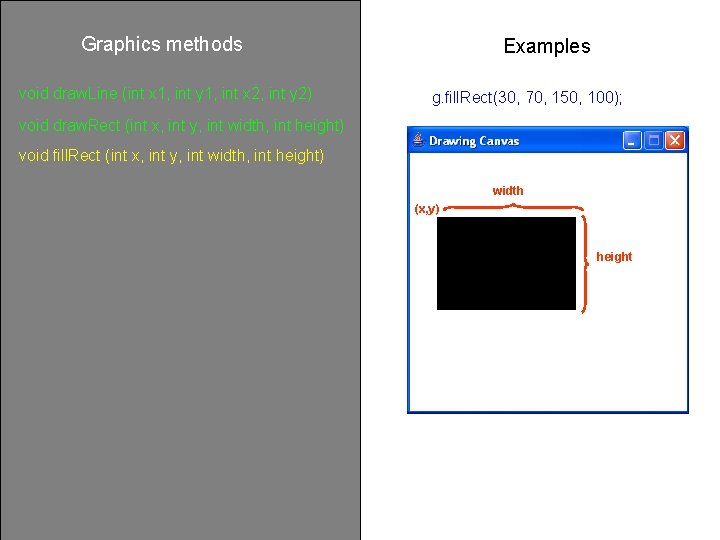
Graphics methods void draw. Line (int x 1, int y 1, int x 2, int y 2) Examples g. fill. Rect(30, 70, 150, 100); void draw. Rect (int x, int y, int width, int height) void fill. Rect (int x, int y, int width, int height) width (x, y) height
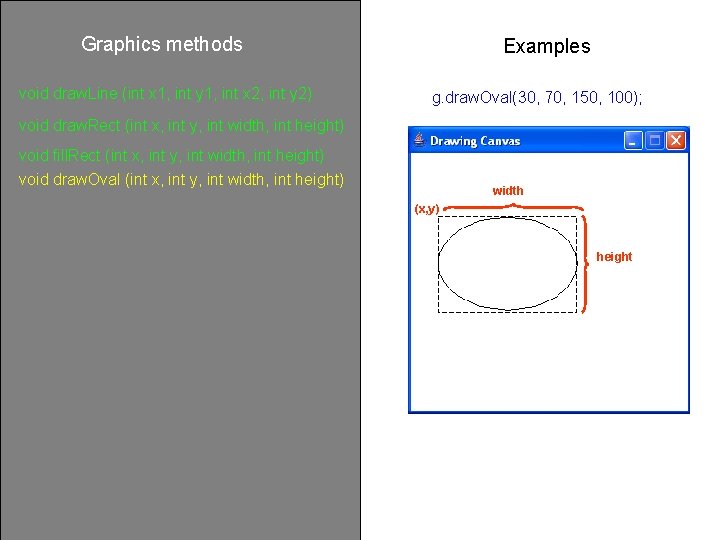
Graphics methods void draw. Line (int x 1, int y 1, int x 2, int y 2) Examples g. draw. Oval(30, 70, 150, 100); void draw. Rect (int x, int y, int width, int height) void fill. Rect (int x, int y, int width, int height) void draw. Oval (int x, int y, int width, int height) width (x, y) height
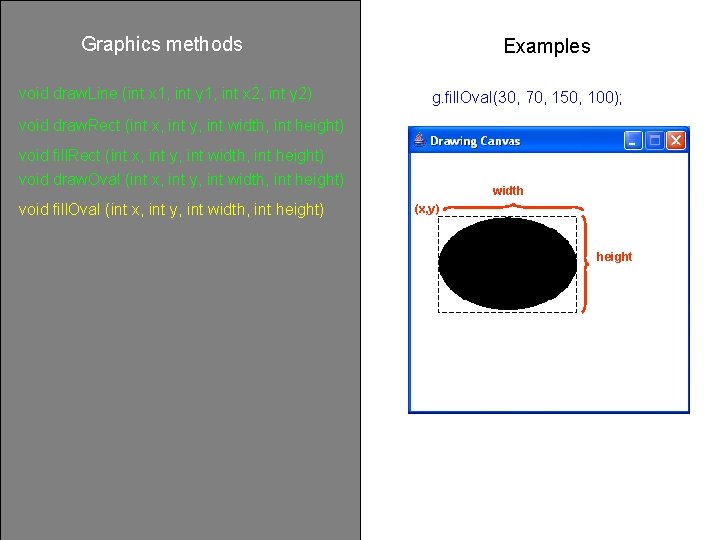
Graphics methods void draw. Line (int x 1, int y 1, int x 2, int y 2) Examples g. fill. Oval(30, 70, 150, 100); void draw. Rect (int x, int y, int width, int height) void fill. Rect (int x, int y, int width, int height) void draw. Oval (int x, int y, int width, int height) void fill. Oval (int x, int y, int width, int height) width (x, y) height
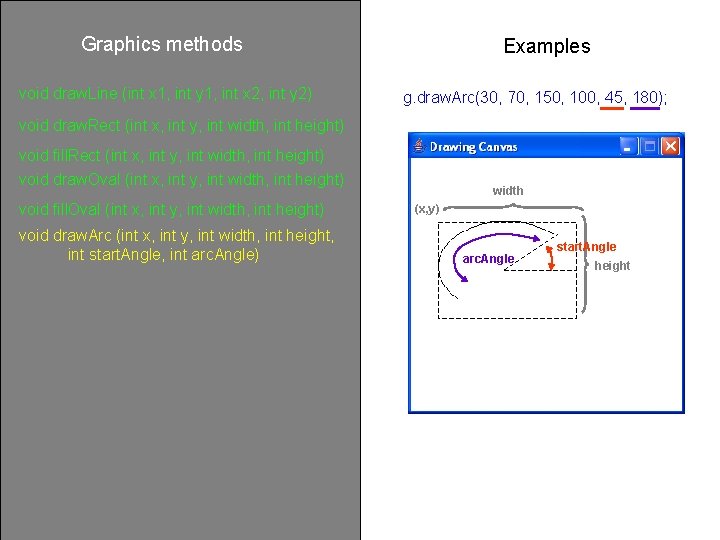
Graphics methods void draw. Line (int x 1, int y 1, int x 2, int y 2) Examples g. draw. Arc(30, 70, 150, 100, 45, 180); void draw. Rect (int x, int y, int width, int height) void fill. Rect (int x, int y, int width, int height) void draw. Oval (int x, int y, int width, int height) void fill. Oval (int x, int y, int width, int height) void draw. Arc (int x, int y, int width, int height, int start. Angle, int arc. Angle) width (x, y) arc. Angle start. Angle height
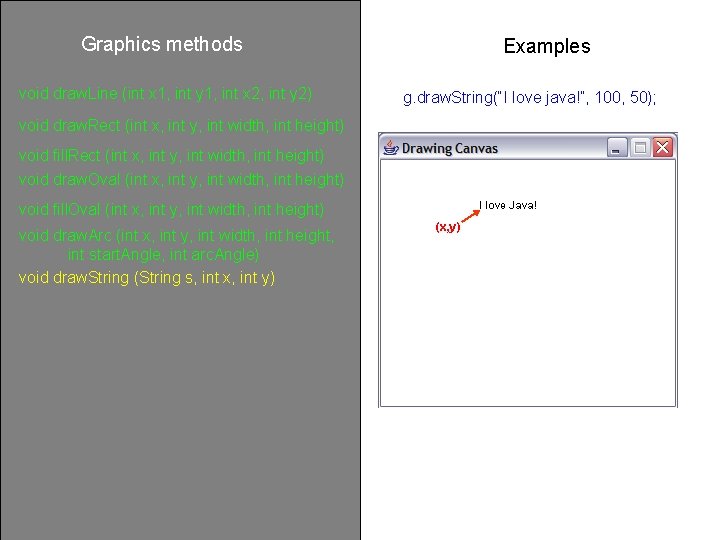
Graphics methods void draw. Line (int x 1, int y 1, int x 2, int y 2) Examples g. draw. String(“I love java!”, 100, 50); void draw. Rect (int x, int y, int width, int height) void fill. Rect (int x, int y, int width, int height) void draw. Oval (int x, int y, int width, int height) void fill. Oval (int x, int y, int width, int height) void draw. Arc (int x, int y, int width, int height, int start. Angle, int arc. Angle) void draw. String (String s, int x, int y) (x, y)
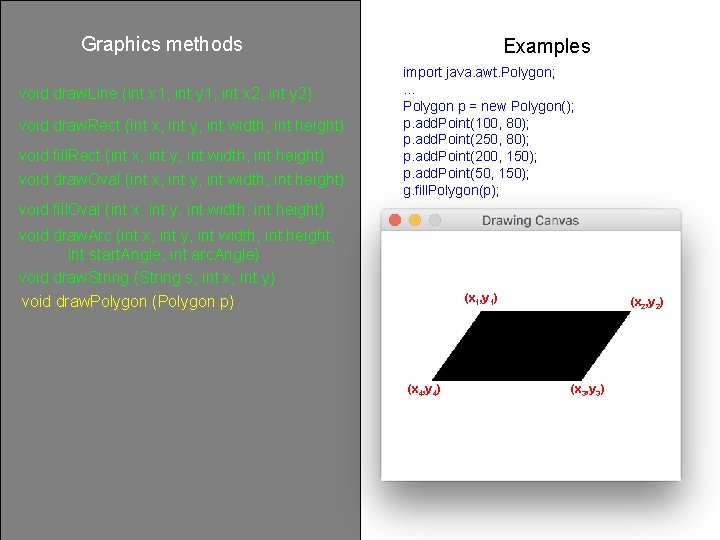
Graphics methods void draw. Line (int x 1, int y 1, int x 2, int y 2) void draw. Rect (int x, int y, int width, int height) void fill. Rect (int x, int y, int width, int height) void draw. Oval (int x, int y, int width, int height) Examples import java. awt. Polygon; … Polygon p = new Polygon(); p. add. Point(100, 80); p. add. Point(250, 80); p. add. Point(200, 150); p. add. Point(50, 150); g. fill. Polygon(p); void fill. Oval (int x, int y, int width, int height) void draw. Arc (int x, int y, int width, int height, int start. Angle, int arc. Angle) void draw. String (String s, int x, int y) void draw. Polygon (Polygon p) (x 1, y 1) (x 4, y 4) (x 2, y 2) (x 3, y 3)
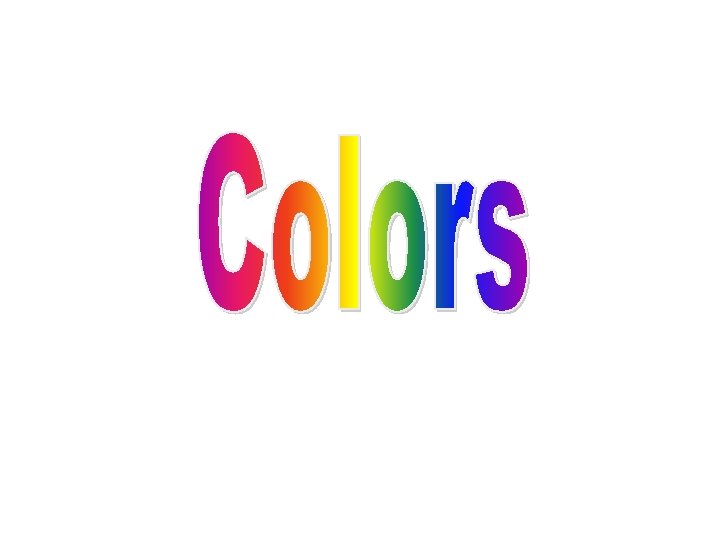
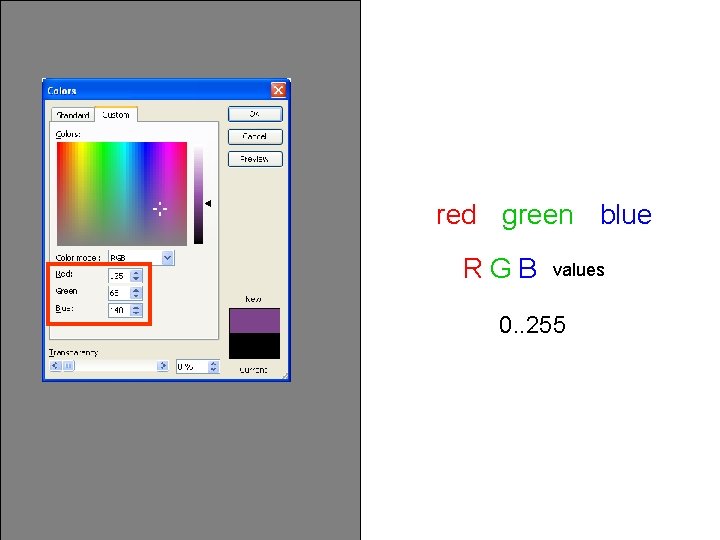
red green blue RGB values 0. . 255
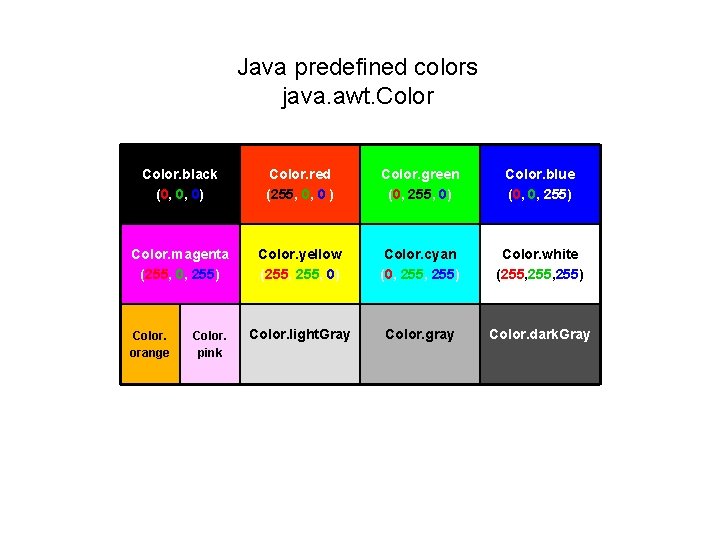
Java predefined colors java. awt. Color. black (0, 0, 0) Color. red (255, 0, 0 ) Color. green (0, 255, 0) Color. blue (0, 0, 255) Color. magenta (255, 0, 255) Color. yellow (255, 0) Color. cyan (0, 255) Color. white (255, 255) Color. light. Gray Color. gray Color. dark. Gray Color. orange Color. pink
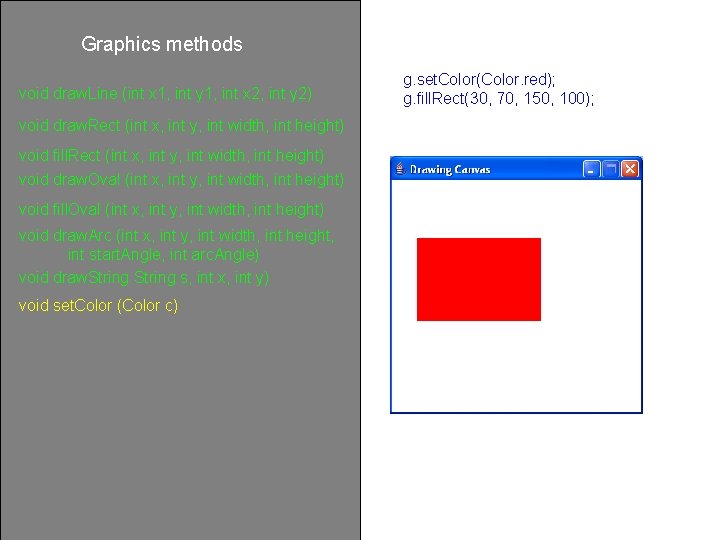
Graphics methods void draw. Line (int x 1, int y 1, int x 2, int y 2) void draw. Rect (int x, int y, int width, int height) void fill. Rect (int x, int y, int width, int height) void draw. Oval (int x, int y, int width, int height) void fill. Oval (int x, int y, int width, int height) void draw. Arc (int x, int y, int width, int height, int start. Angle, int arc. Angle) void draw. String s, int x, int y) void set. Color (Color c) g. set. Color(Color. red); g. fill. Rect(30, 70, 150, 100);
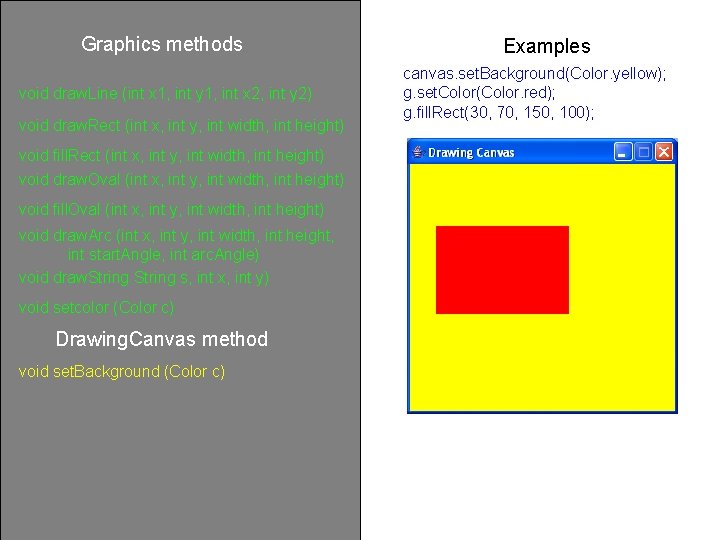
Graphics methods void draw. Line (int x 1, int y 1, int x 2, int y 2) void draw. Rect (int x, int y, int width, int height) void fill. Rect (int x, int y, int width, int height) void draw. Oval (int x, int y, int width, int height) void fill. Oval (int x, int y, int width, int height) void draw. Arc (int x, int y, int width, int height, int start. Angle, int arc. Angle) void draw. String s, int x, int y) void setcolor (Color c) Drawing. Canvas method void set. Background (Color c) Examples canvas. set. Background(Color. yellow); g. set. Color(Color. red); g. fill. Rect(30, 70, 150, 100);
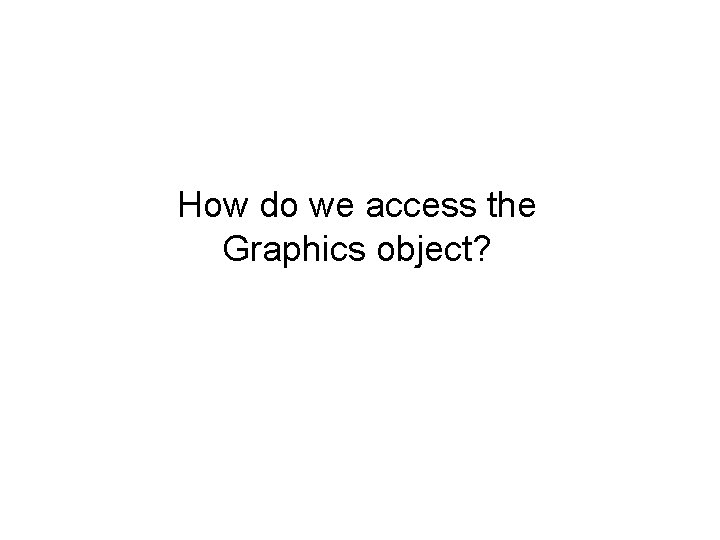
How do we access the Graphics object?
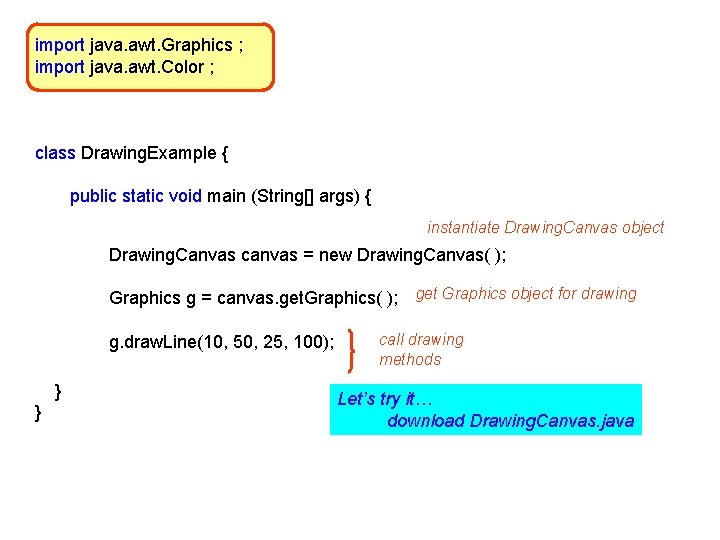
import java. awt. Graphics ; import java. awt. Color ; class Drawing. Example { public static void main (String[] args) { instantiate Drawing. Canvas object Drawing. Canvas canvas = new Drawing. Canvas( ); Graphics g = canvas. get. Graphics( ); get Graphics object for drawing g. draw. Line(10, 50, 25, 100); } } call drawing methods Let’s try it… download Drawing. Canvas. java
Difference between note making and note taking
What are signal words
Difference between note making and note taking
Goods received note
Debit note example
What is a debit memo
Note taking and note making
Simple discount example
Graphics monitors and workstations
Computer graphics introduction ppt
Note three provisions in japan’s new constitution
Line in computer graphics
Korea
Pixel addressing in computer graphics
Direct use of line equation in computer graphics
Find the decision variable (d) for midpoint algorithm.
How to draw a line in computer graphics
This is the midpoint inside the ellipse
Circle in computer graphics
Lean model canvas vs business model canvas
Split direct speech
New york pennsylvania new jersey delaware