The Canvas Introducing the Canvas The canvas is
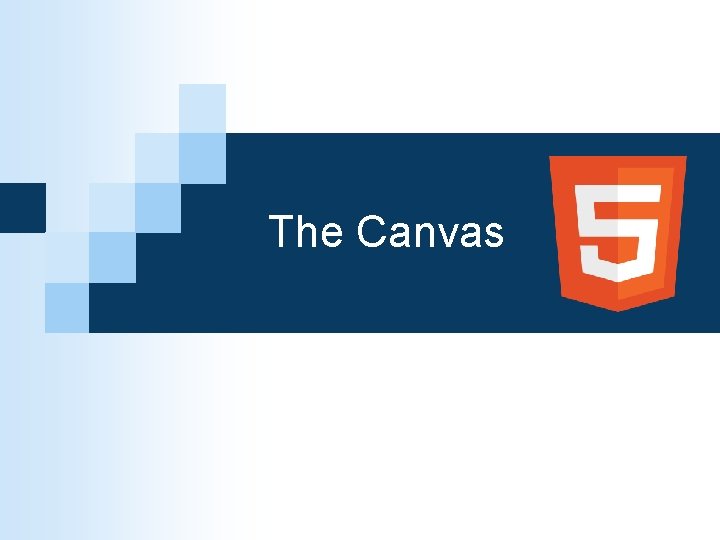
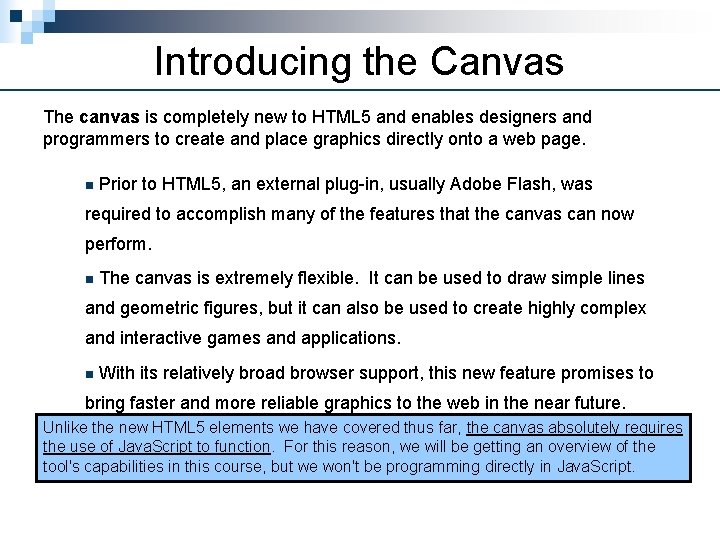
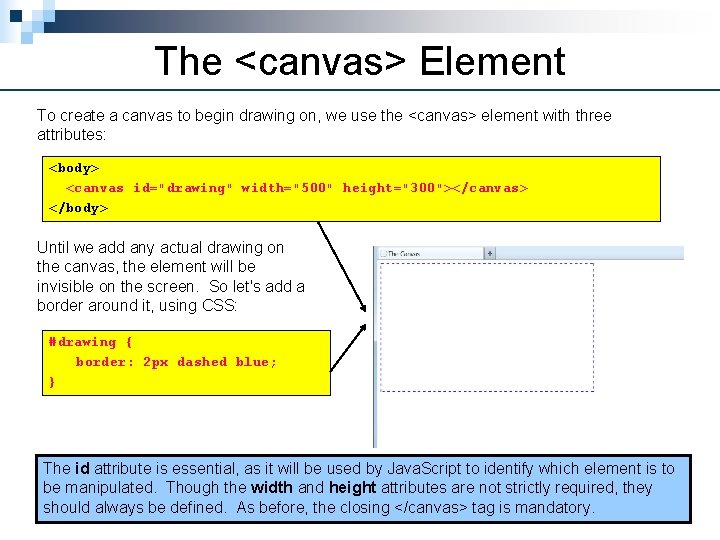
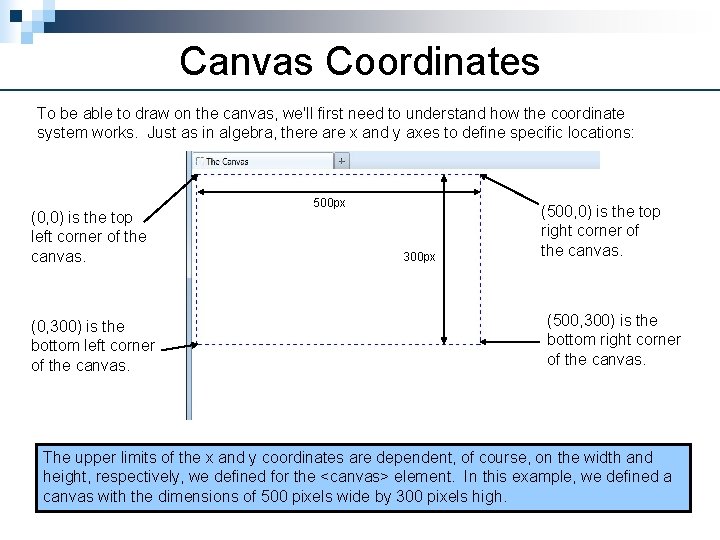
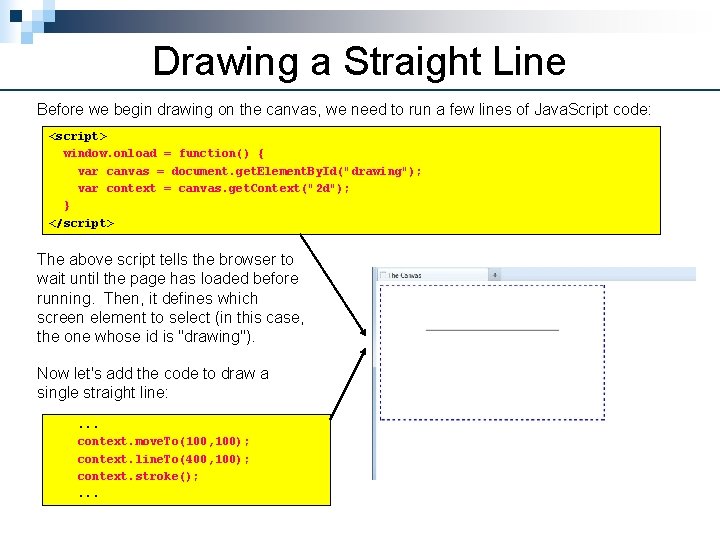
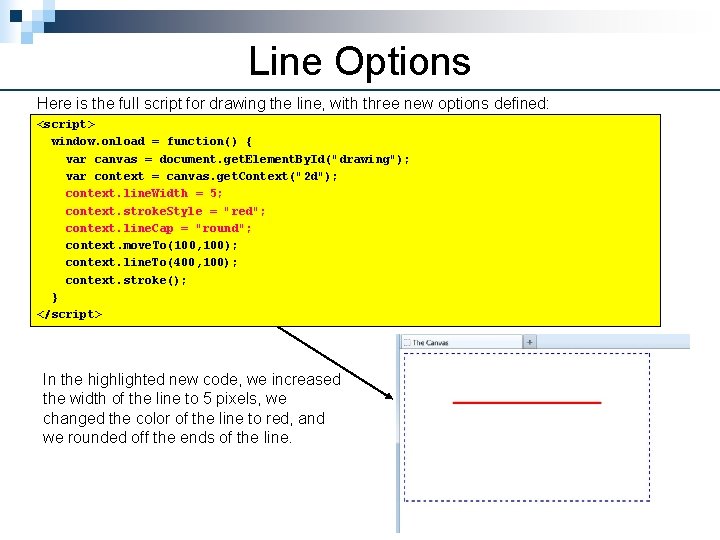
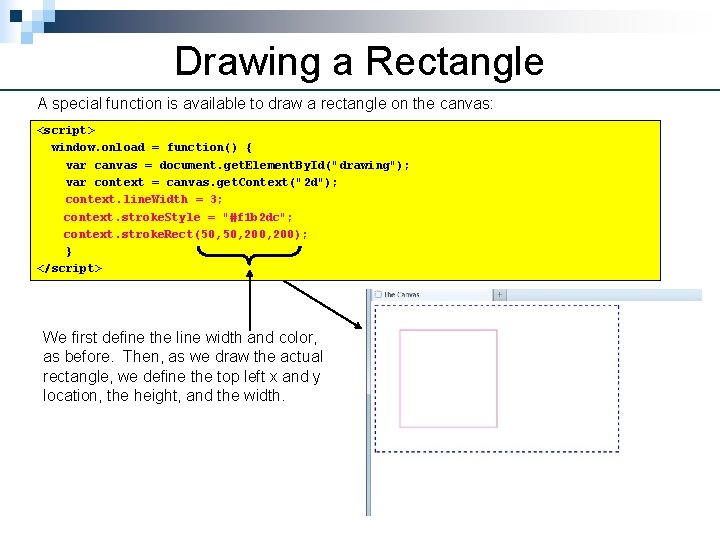
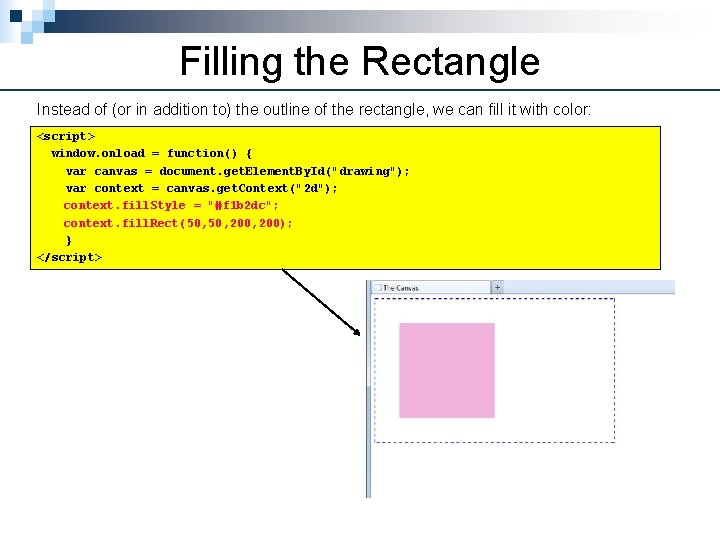
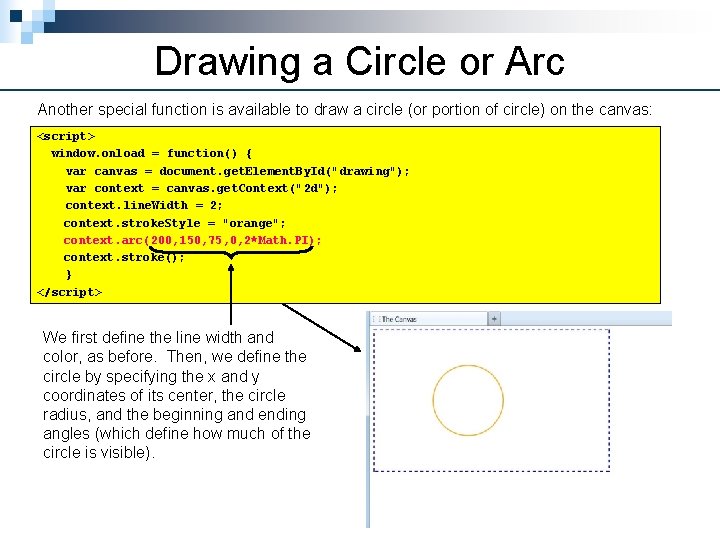
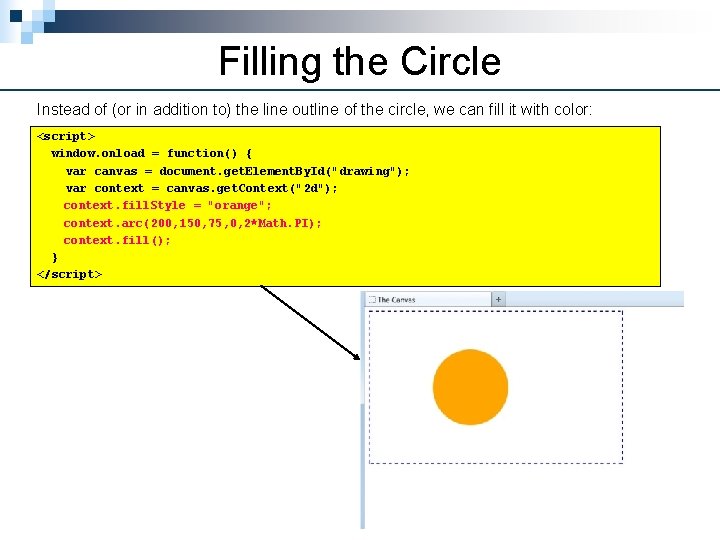
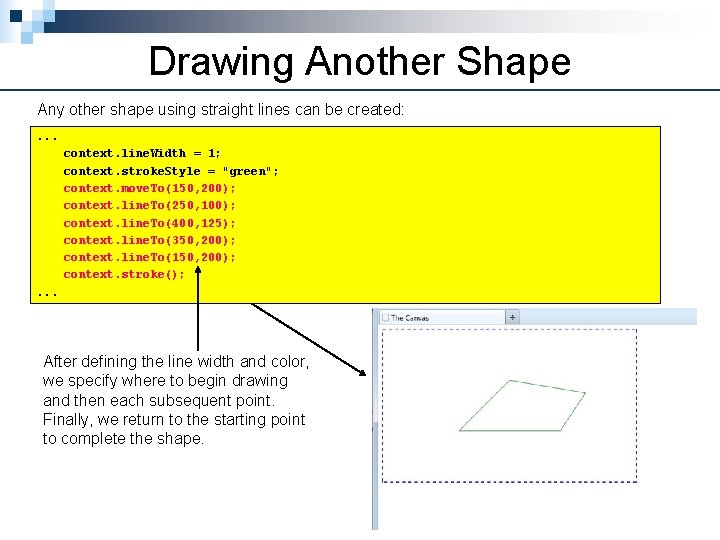
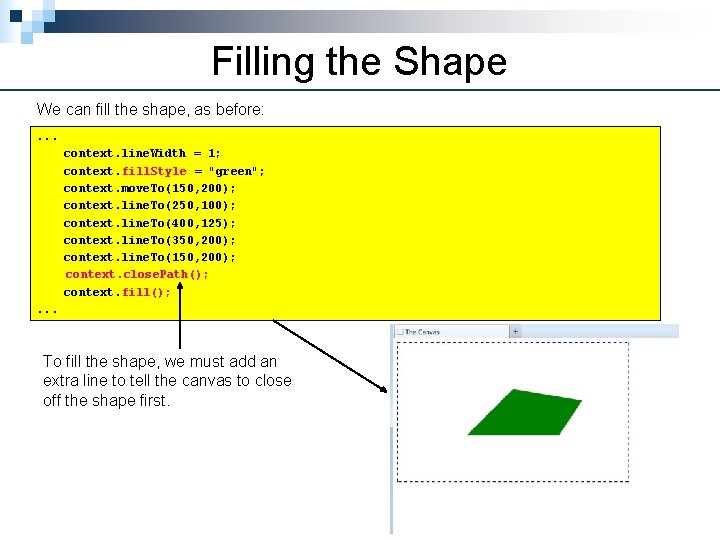
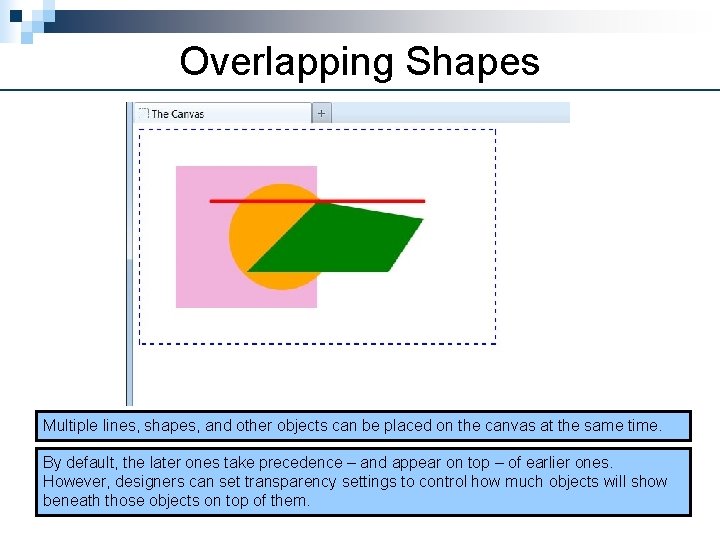
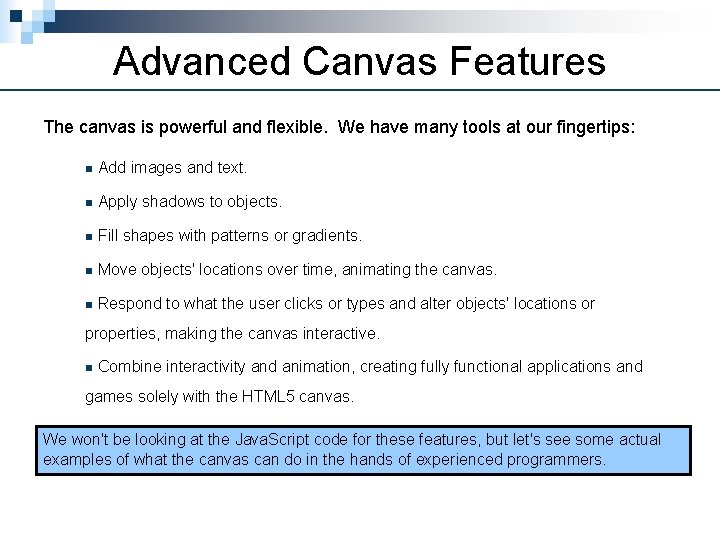
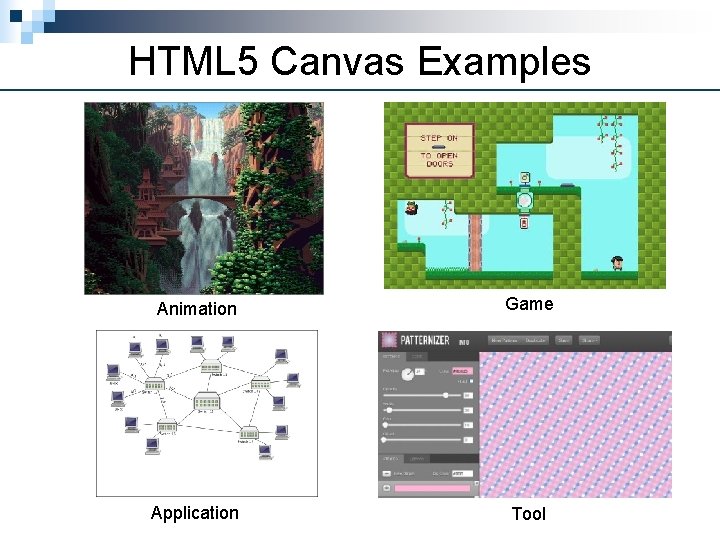
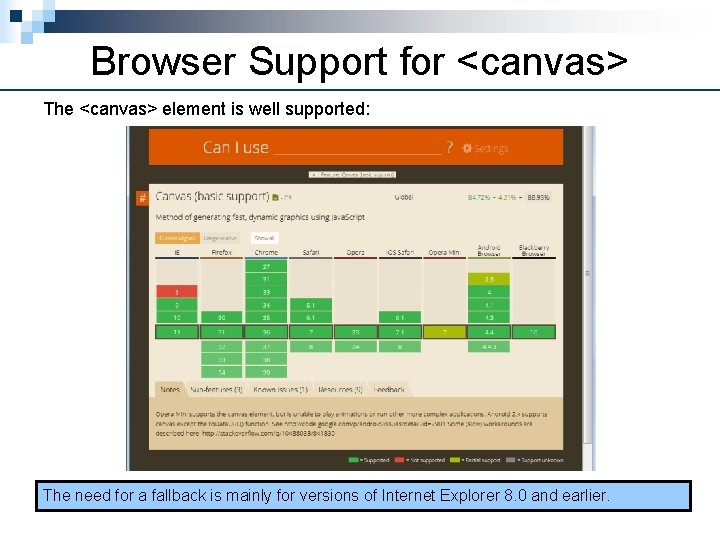
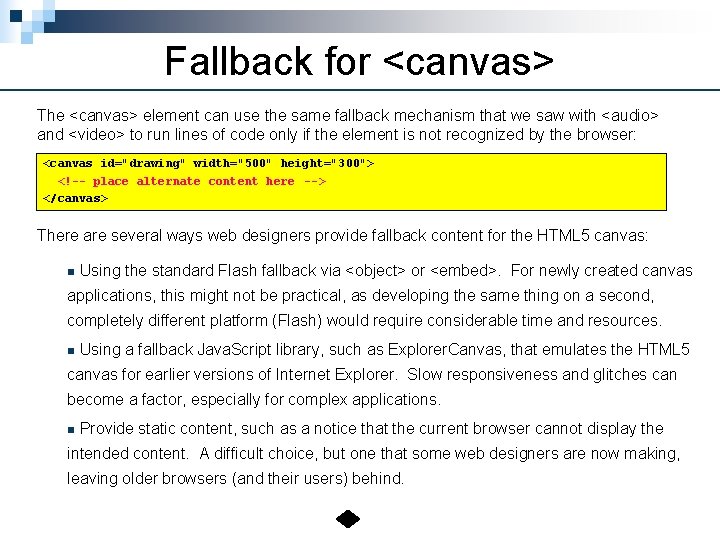
- Slides: 17
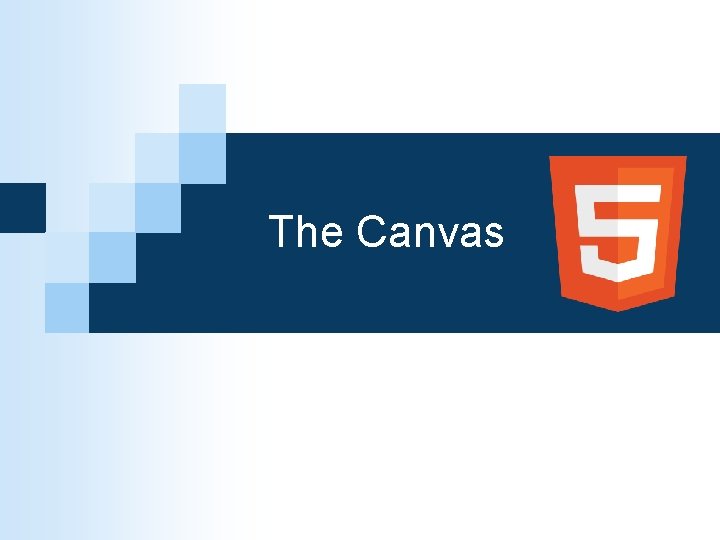
The Canvas
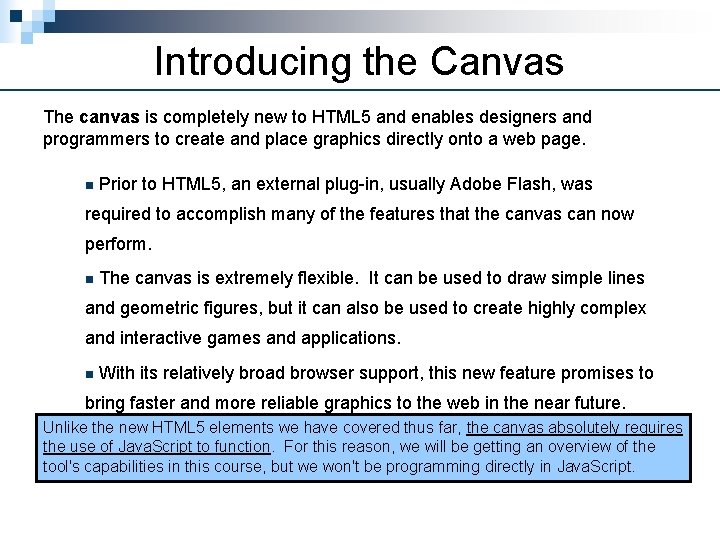
Introducing the Canvas The canvas is completely new to HTML 5 and enables designers and programmers to create and place graphics directly onto a web page. n Prior to HTML 5, an external plug-in, usually Adobe Flash, was required to accomplish many of the features that the canvas can now perform. n The canvas is extremely flexible. It can be used to draw simple lines and geometric figures, but it can also be used to create highly complex and interactive games and applications. n With its relatively broad browser support, this new feature promises to bring faster and more reliable graphics to the web in the near future. Unlike the new HTML 5 elements we have covered thus far, the canvas absolutely requires the use of Java. Script to function. For this reason, we will be getting an overview of the tool's capabilities in this course, but we won't be programming directly in Java. Script.
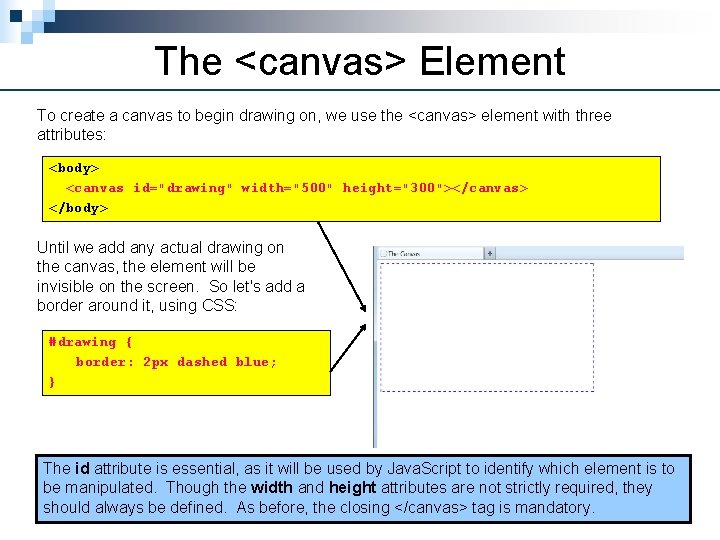
The <canvas> Element To create a canvas to begin drawing on, we use the <canvas> element with three attributes: <body> <canvas id="drawing" width="500" height="300"></canvas> </body> Until we add any actual drawing on the canvas, the element will be invisible on the screen. So let's add a border around it, using CSS: #drawing { border: 2 px dashed blue; } The id attribute is essential, as it will be used by Java. Script to identify which element is to be manipulated. Though the width and height attributes are not strictly required, they should always be defined. As before, the closing </canvas> tag is mandatory.
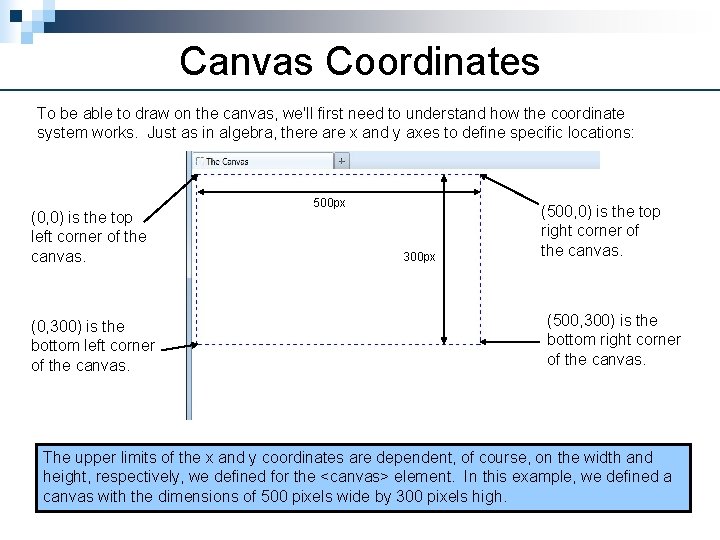
Canvas Coordinates To be able to draw on the canvas, we'll first need to understand how the coordinate system works. Just as in algebra, there are x and y axes to define specific locations: (0, 0) is the top left corner of the canvas. (0, 300) is the bottom left corner of the canvas. 500 px 300 px (500, 0) is the top right corner of the canvas. (500, 300) is the bottom right corner of the canvas. The upper limits of the x and y coordinates are dependent, of course, on the width and height, respectively, we defined for the <canvas> element. In this example, we defined a canvas with the dimensions of 500 pixels wide by 300 pixels high.
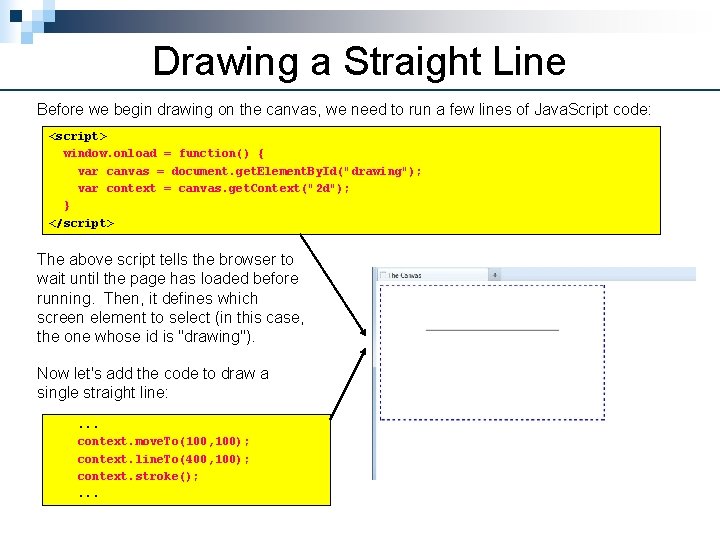
Drawing a Straight Line Before we begin drawing on the canvas, we need to run a few lines of Java. Script code: <script> window. onload = function() { var canvas = document. get. Element. By. Id("drawing"); var context = canvas. get. Context("2 d"); } </script> The above script tells the browser to wait until the page has loaded before running. Then, it defines which screen element to select (in this case, the one whose id is "drawing"). Now let's add the code to draw a single straight line: . . . context. move. To(100, 100); context. line. To(400, 100); context. stroke(); . . .
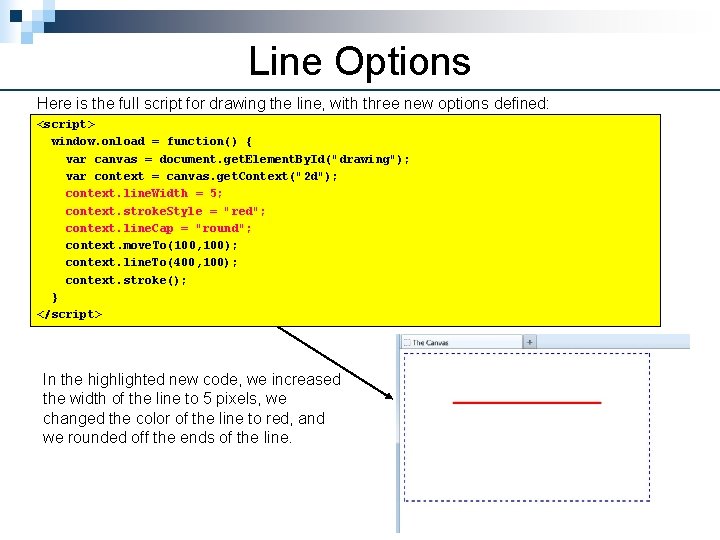
Line Options Here is the full script for drawing the line, with three new options defined: <script> window. onload = function() { var canvas = document. get. Element. By. Id("drawing"); var context = canvas. get. Context("2 d"); context. line. Width = 5; context. stroke. Style = "red"; context. line. Cap = "round"; context. move. To(100, 100); context. line. To(400, 100); context. stroke(); } </script> In the highlighted new code, we increased the width of the line to 5 pixels, we changed the color of the line to red, and we rounded off the ends of the line.
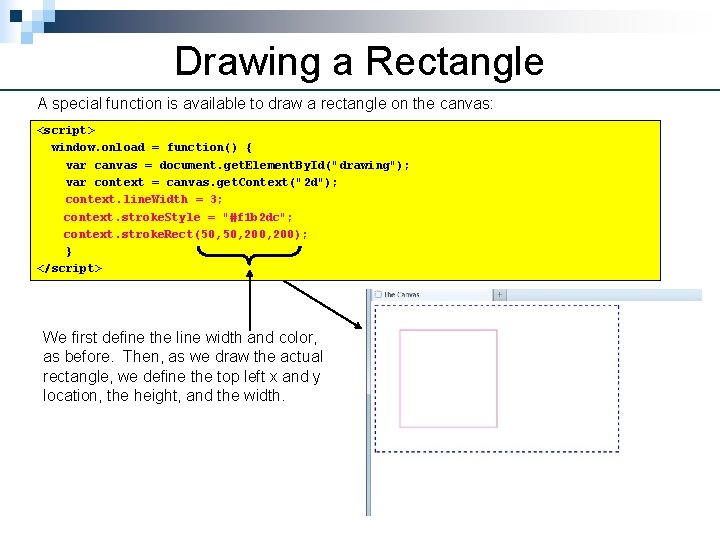
Drawing a Rectangle A special function is available to draw a rectangle on the canvas: <script> window. onload = function() { var canvas = document. get. Element. By. Id("drawing"); var context = canvas. get. Context("2 d"); context. line. Width = 3; context. stroke. Style = "#f 1 b 2 dc"; context. stroke. Rect(50, 200, 200); } </script> We first define the line width and color, as before. Then, as we draw the actual rectangle, we define the top left x and y location, the height, and the width.
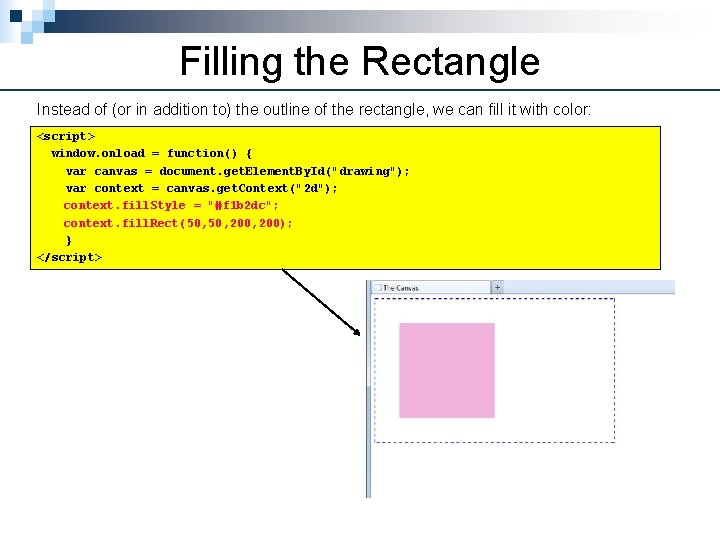
Filling the Rectangle Instead of (or in addition to) the outline of the rectangle, we can fill it with color: <script> window. onload = function() { var canvas = document. get. Element. By. Id("drawing"); var context = canvas. get. Context("2 d"); context. fill. Style = "#f 1 b 2 dc"; context. fill. Rect(50, 200, 200); } </script>
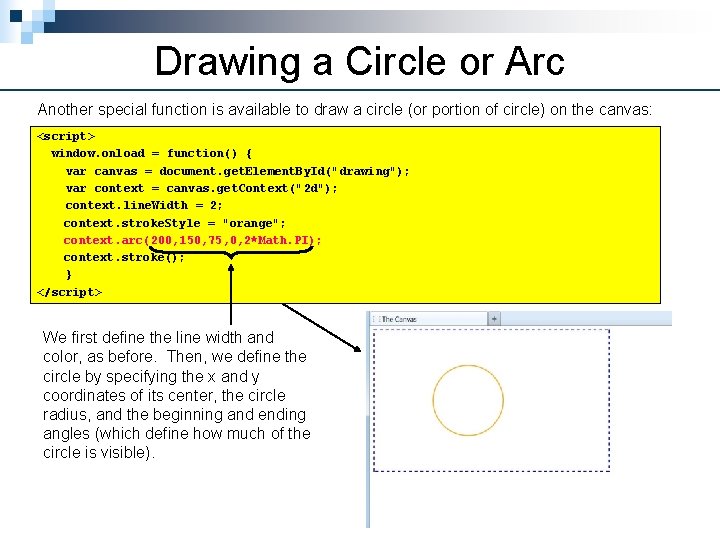
Drawing a Circle or Arc Another special function is available to draw a circle (or portion of circle) on the canvas: <script> window. onload = function() { var canvas = document. get. Element. By. Id("drawing"); var context = canvas. get. Context("2 d"); context. line. Width = 2; context. stroke. Style = "orange"; context. arc(200, 150, 75, 0, 2*Math. PI); context. stroke(); } </script> We first define the line width and color, as before. Then, we define the circle by specifying the x and y coordinates of its center, the circle radius, and the beginning and ending angles (which define how much of the circle is visible).
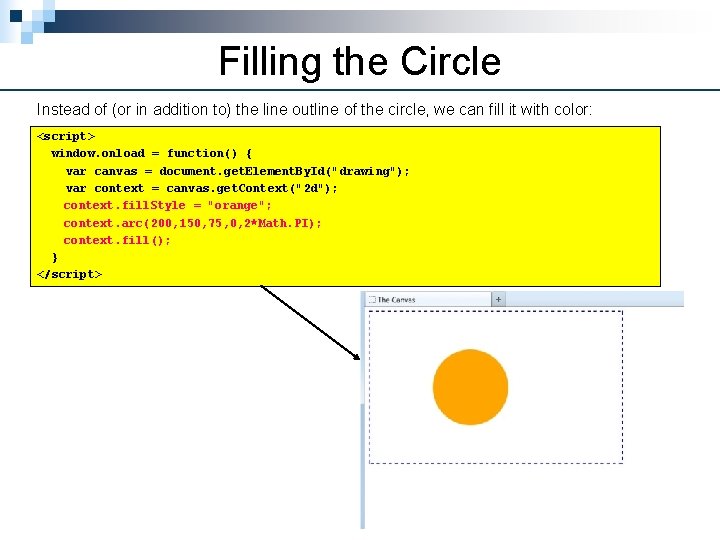
Filling the Circle Instead of (or in addition to) the line outline of the circle, we can fill it with color: <script> window. onload = function() { var canvas = document. get. Element. By. Id("drawing"); var context = canvas. get. Context("2 d"); context. fill. Style = "orange"; context. arc(200, 150, 75, 0, 2*Math. PI); context. fill(); } </script>
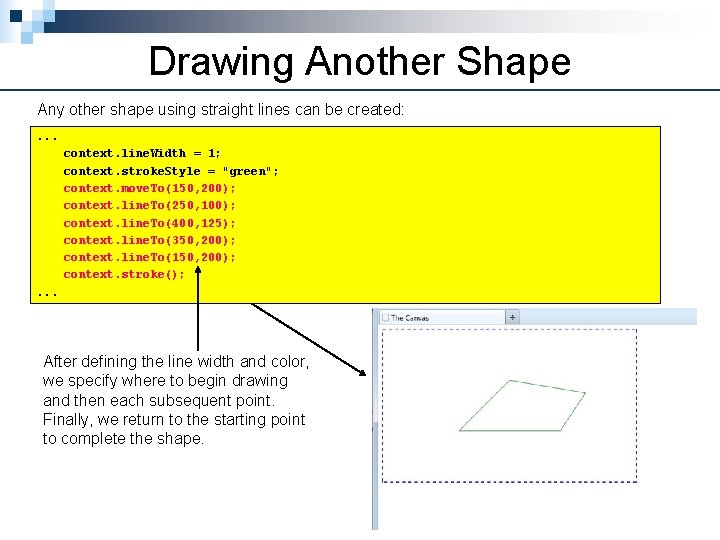
Drawing Another Shape Any other shape using straight lines can be created: . . . context. line. Width = 1; context. stroke. Style = "green"; context. move. To(150, 200); context. line. To(250, 100); context. line. To(400, 125); context. line. To(350, 200); context. line. To(150, 200); context. stroke(); . . . After defining the line width and color, we specify where to begin drawing and then each subsequent point. Finally, we return to the starting point to complete the shape.
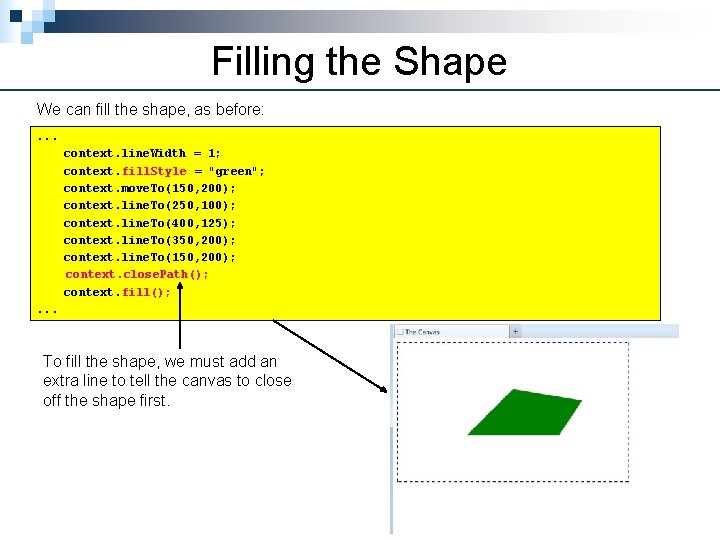
Filling the Shape We can fill the shape, as before: . . . context. line. Width = 1; context. fill. Style = "green"; context. move. To(150, 200); context. line. To(250, 100); context. line. To(400, 125); context. line. To(350, 200); context. line. To(150, 200); context. close. Path(); context. fill(); . . . To fill the shape, we must add an extra line to tell the canvas to close off the shape first.
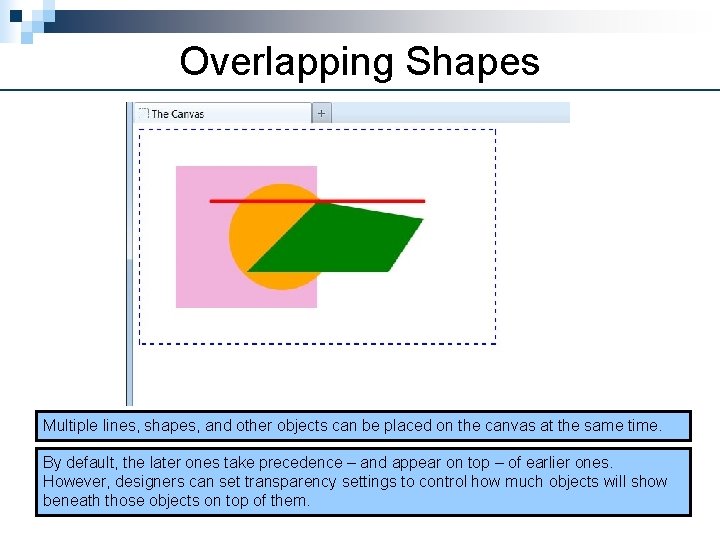
Overlapping Shapes Multiple lines, shapes, and other objects can be placed on the canvas at the same time. By default, the later ones take precedence – and appear on top – of earlier ones. However, designers can set transparency settings to control how much objects will show beneath those objects on top of them.
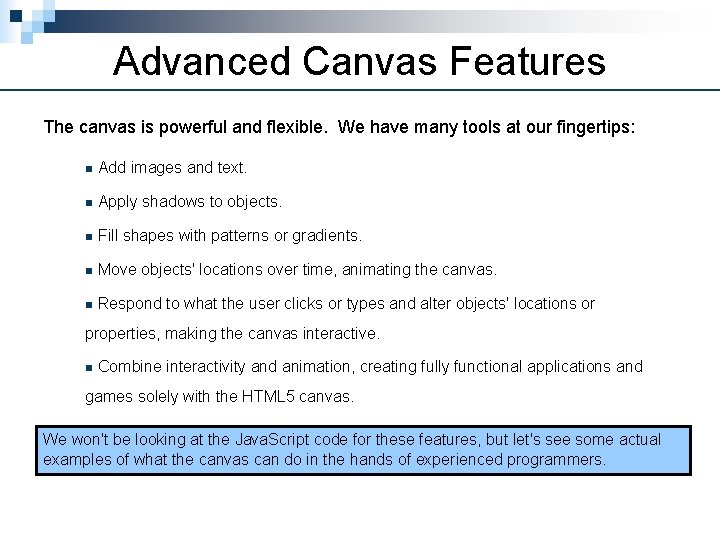
Advanced Canvas Features The canvas is powerful and flexible. We have many tools at our fingertips: n Add images and text. n Apply shadows to objects. n Fill shapes with patterns or gradients. n Move objects' locations over time, animating the canvas. n Respond to what the user clicks or types and alter objects' locations or properties, making the canvas interactive. n Combine interactivity and animation, creating fully functional applications and games solely with the HTML 5 canvas. We won't be looking at the Java. Script code for these features, but let's see some actual examples of what the canvas can do in the hands of experienced programmers.
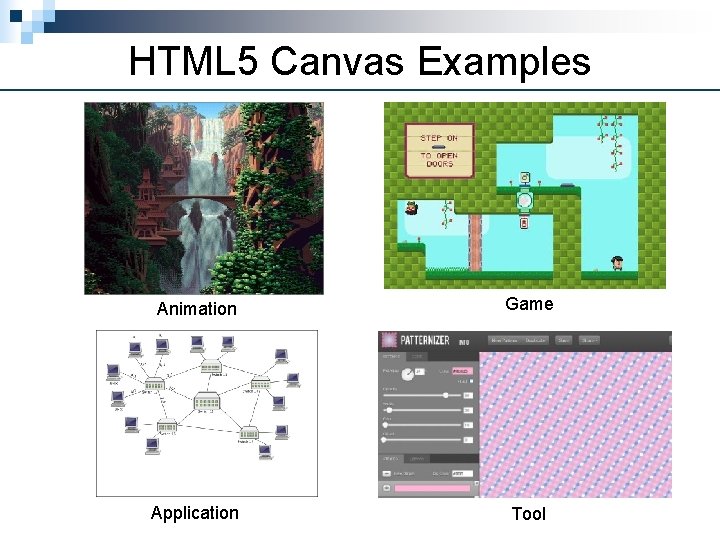
HTML 5 Canvas Examples Animation Game Application Tool
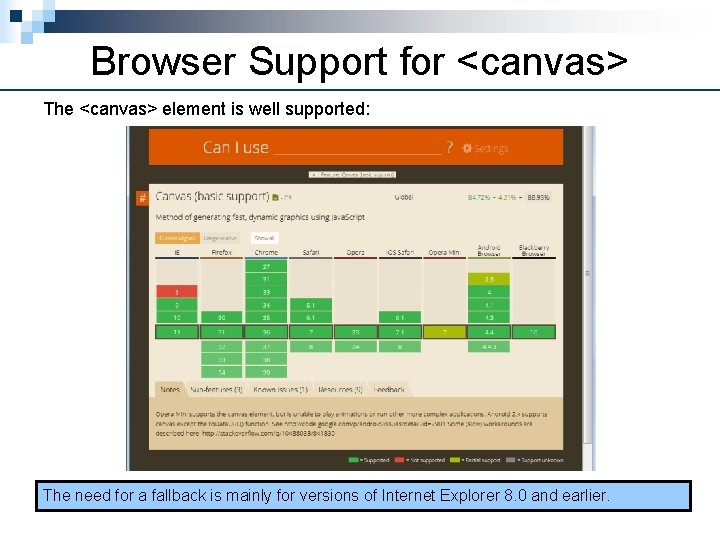
Browser Support for <canvas> The <canvas> element is well supported: The need for a fallback is mainly for versions of Internet Explorer 8. 0 and earlier.
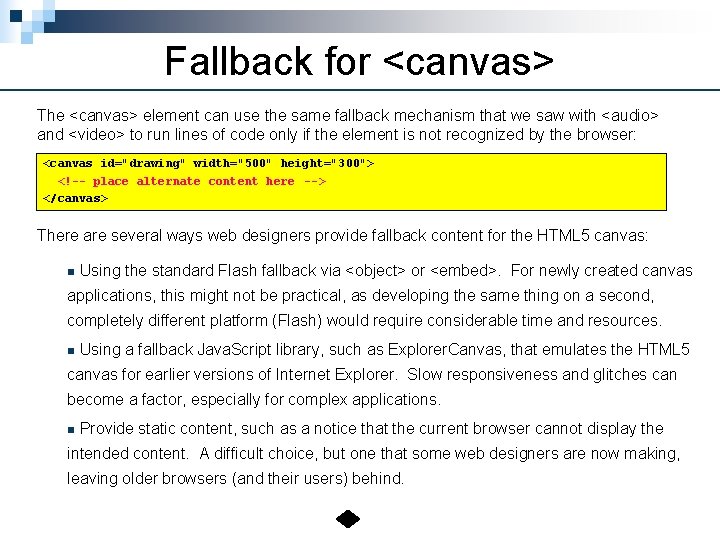
Fallback for <canvas> The <canvas> element can use the same fallback mechanism that we saw with <audio> and <video> to run lines of code only if the element is not recognized by the browser: <canvas id="drawing" width="500" height="300"> <!-- place alternate content here --> </canvas> There are several ways web designers provide fallback content for the HTML 5 canvas: n Using the standard Flash fallback via <object> or <embed>. For newly created canvas applications, this might not be practical, as developing the same thing on a second, completely different platform (Flash) would require considerable time and resources. n Using a fallback Java. Script library, such as Explorer. Canvas, that emulates the HTML 5 canvas for earlier versions of Internet Explorer. Slow responsiveness and glitches can become a factor, especially for complex applications. n Provide static content, such as a notice that the current browser cannot display the intended content. A difficult choice, but one that some web designers are now making, leaving older browsers (and their users) behind.
Introducing neeta anil said
Kfc founded
Templates for introducing quotations
Informal letter exchange student
Quote introduction sentence starters
Templates for introducing quotations
Introducing new market offerings ppt
Chapter 1 introducing psychology
Definition of politics
Ma
Relocation diffusion definition
Signal phrases for quotes
Introducing and naming new products and brand extensions
What are integers
Metric system basics
Carrying broker
Introducing counterclaim
Define upgrade advisor