Graphics CSCI 343 Fall 2019 Lecture 6 Viewing
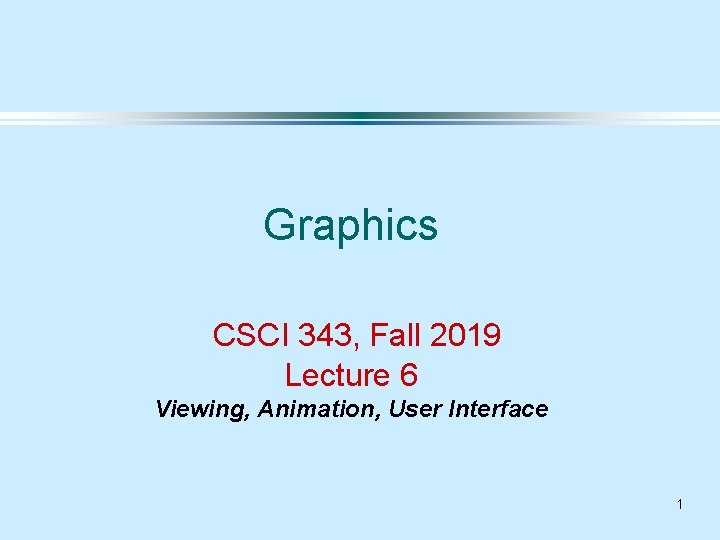
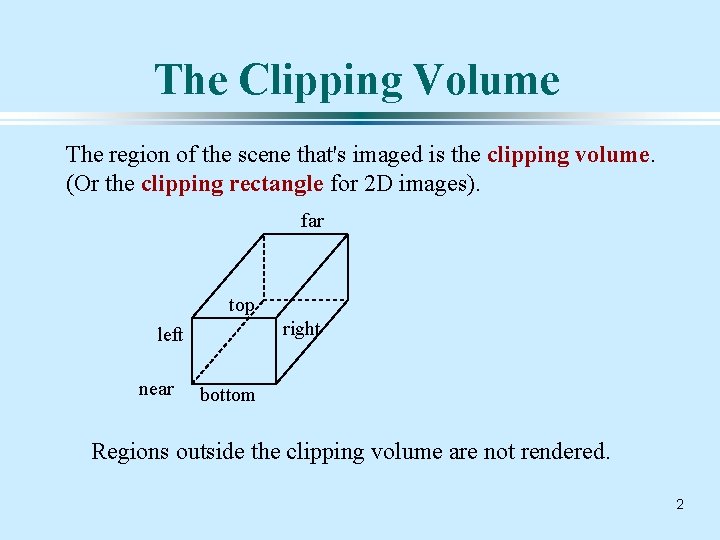
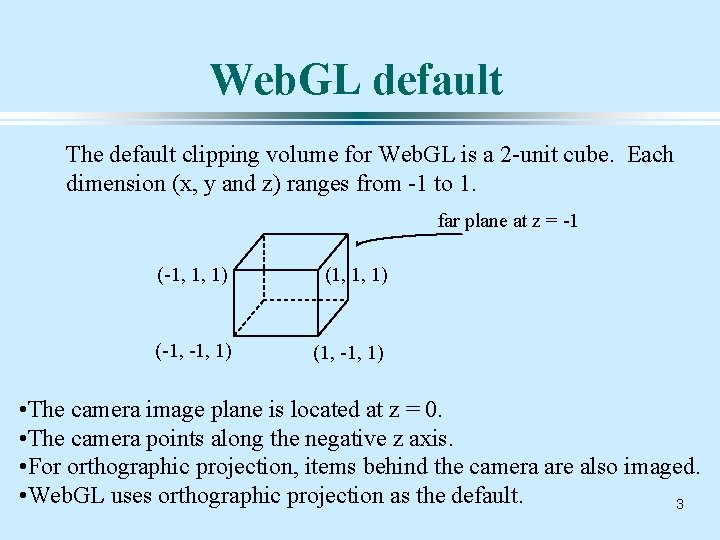
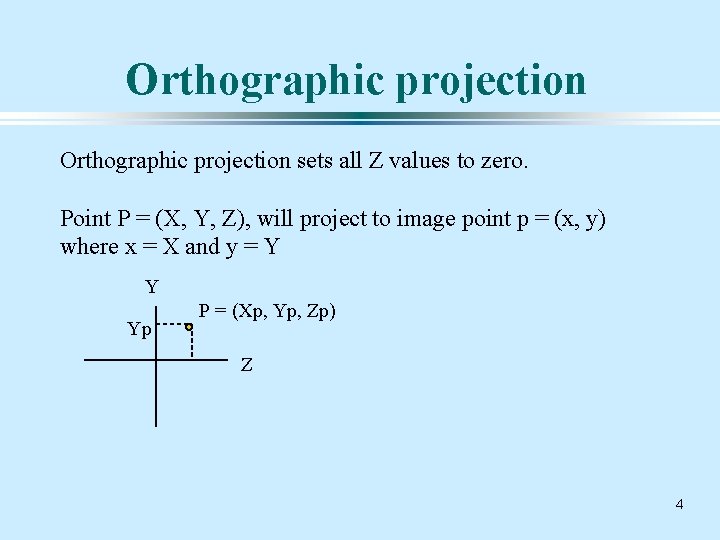
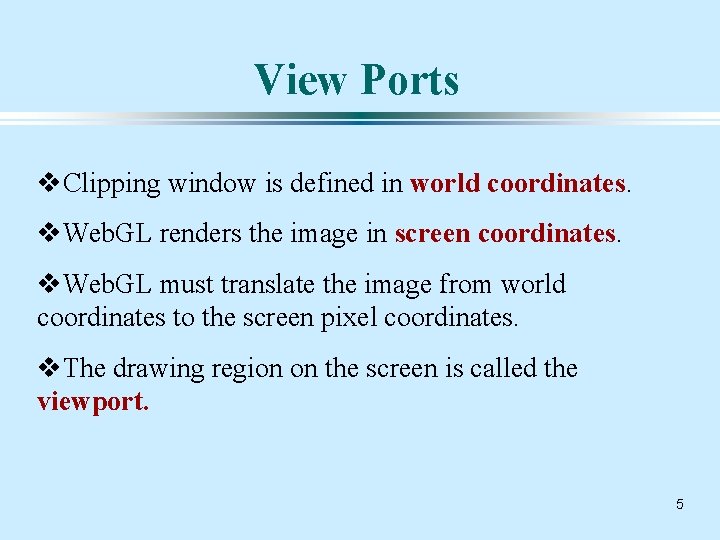
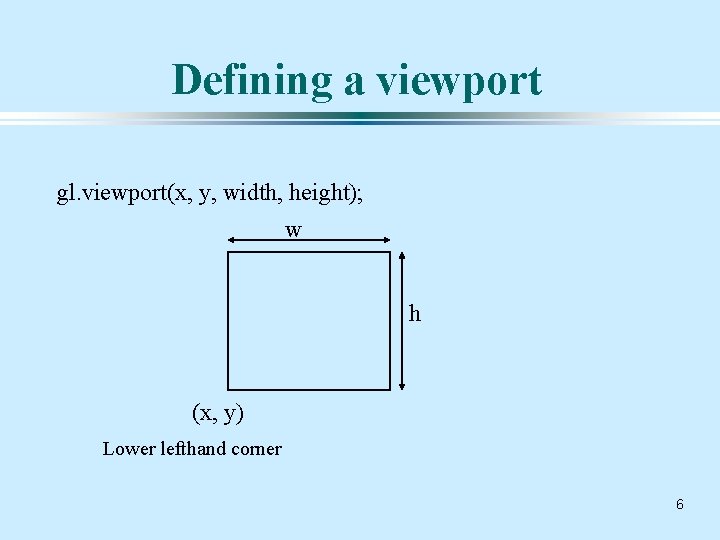
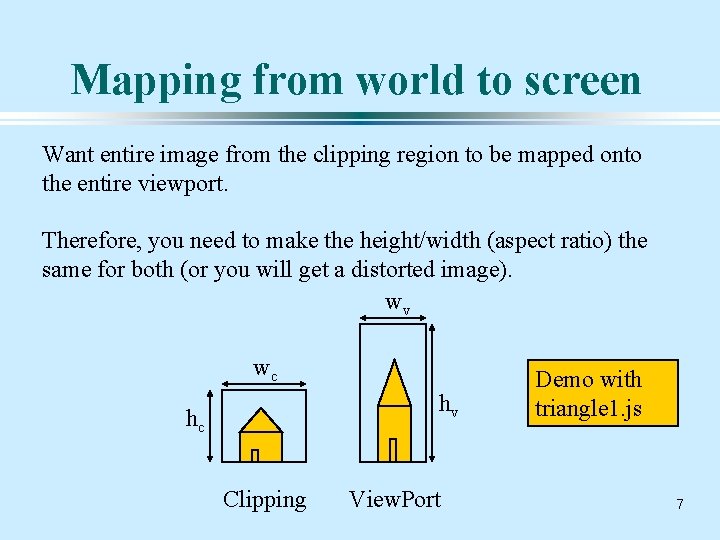
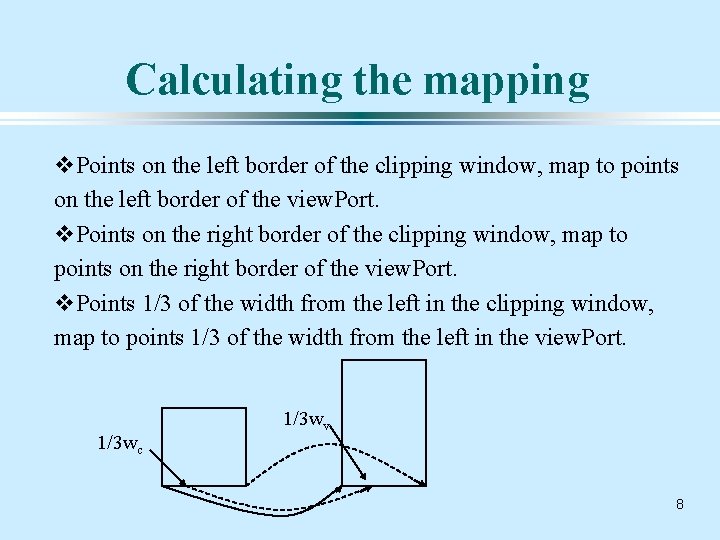
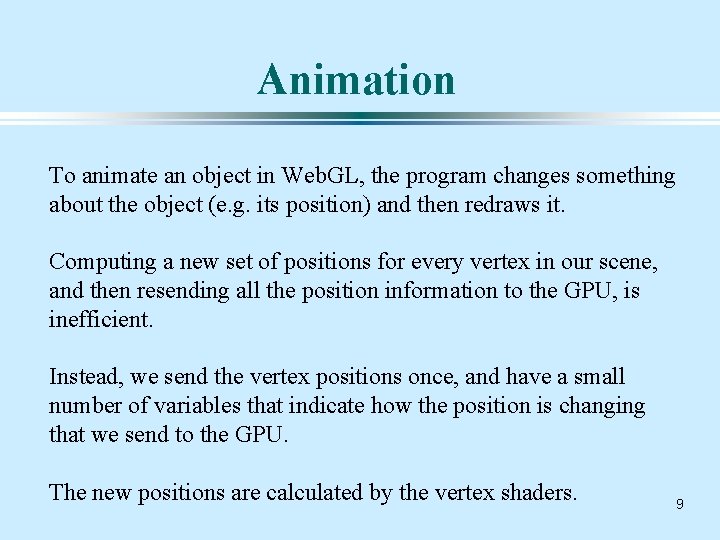
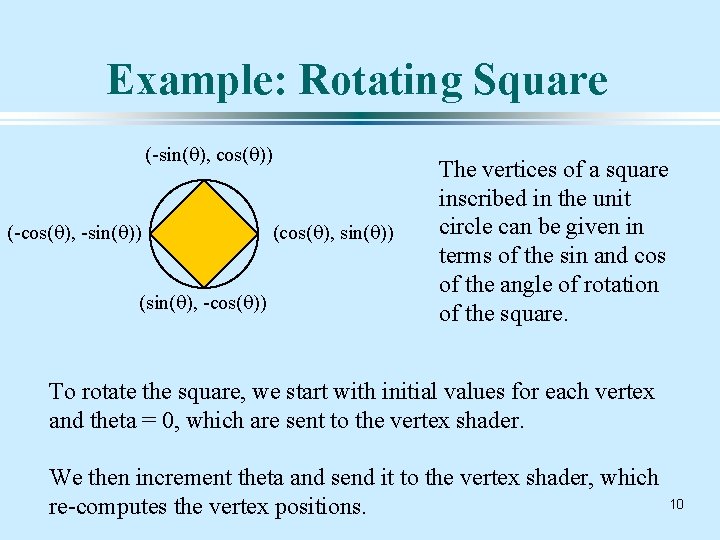
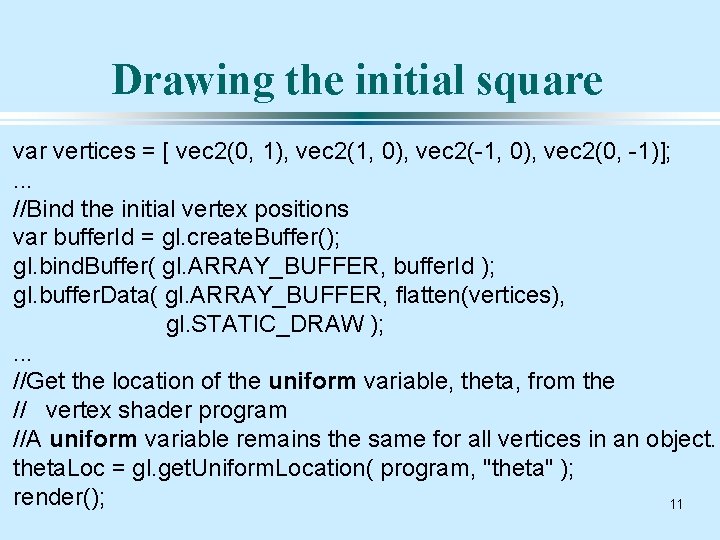
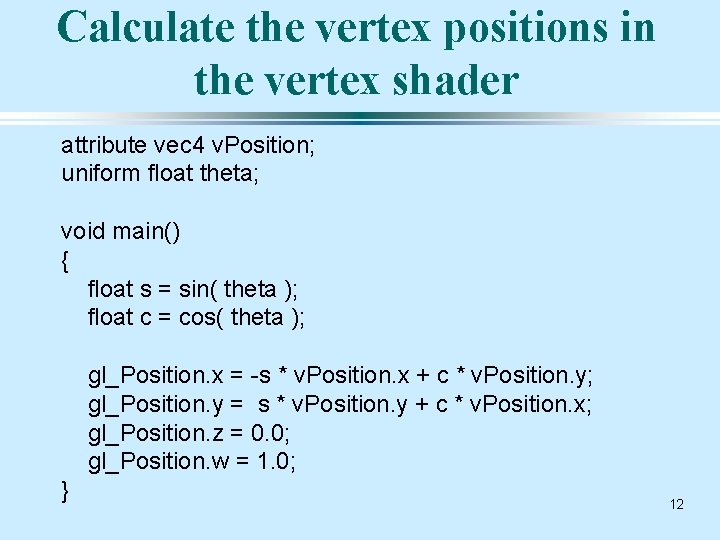
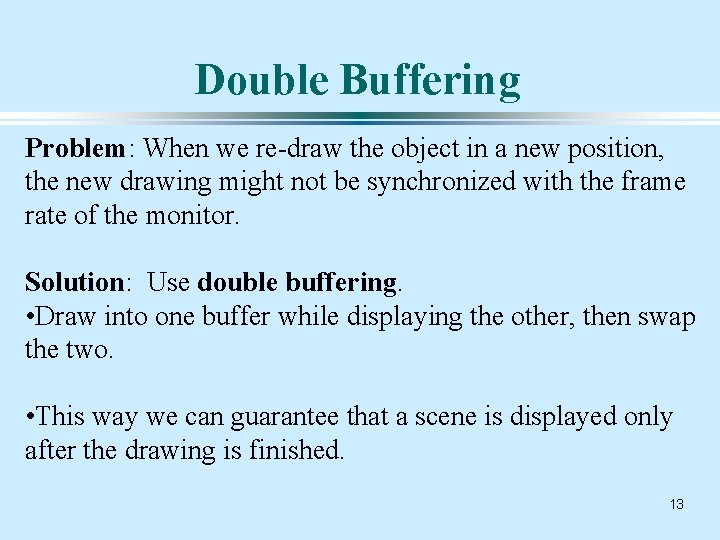
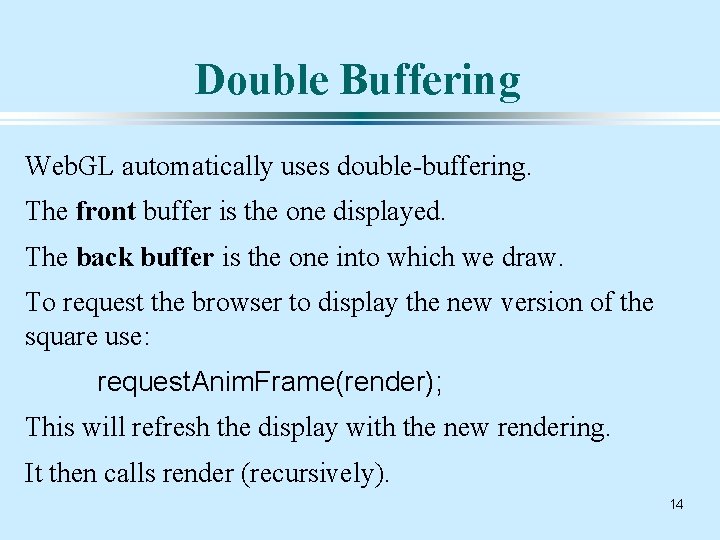
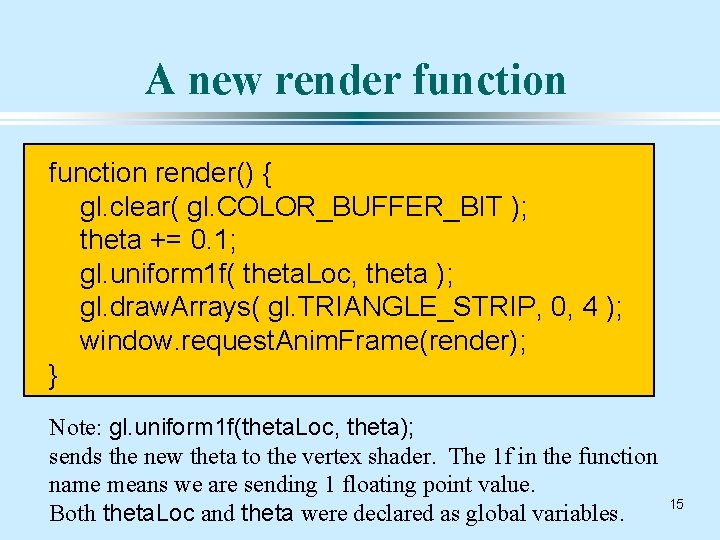
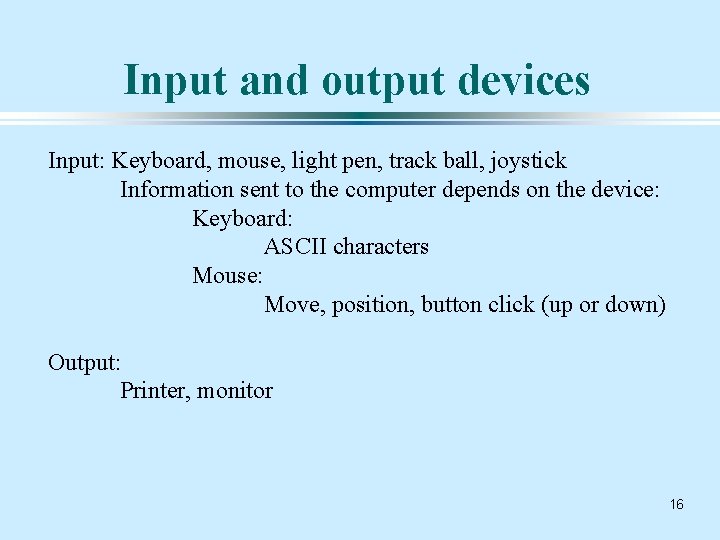
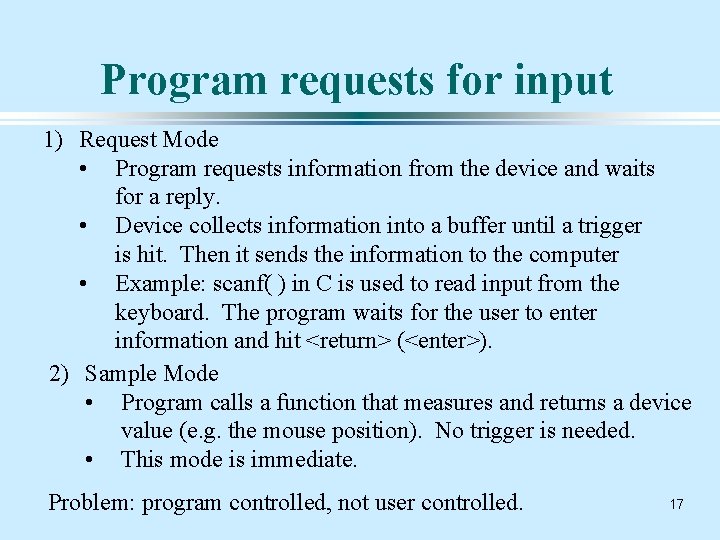
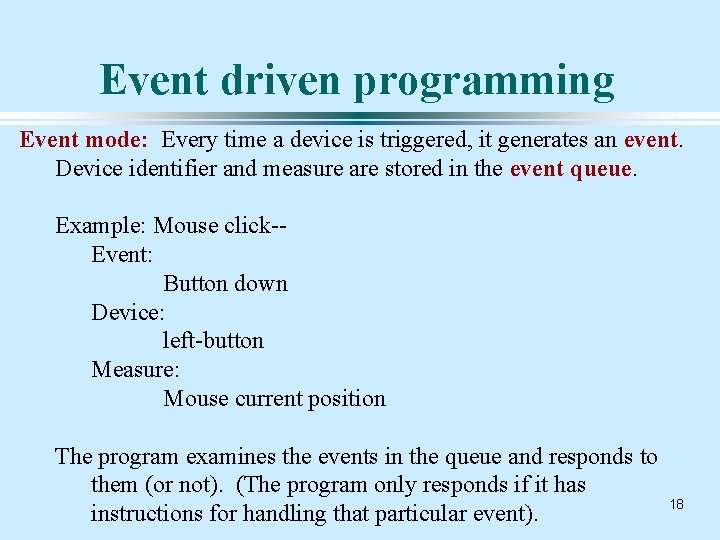
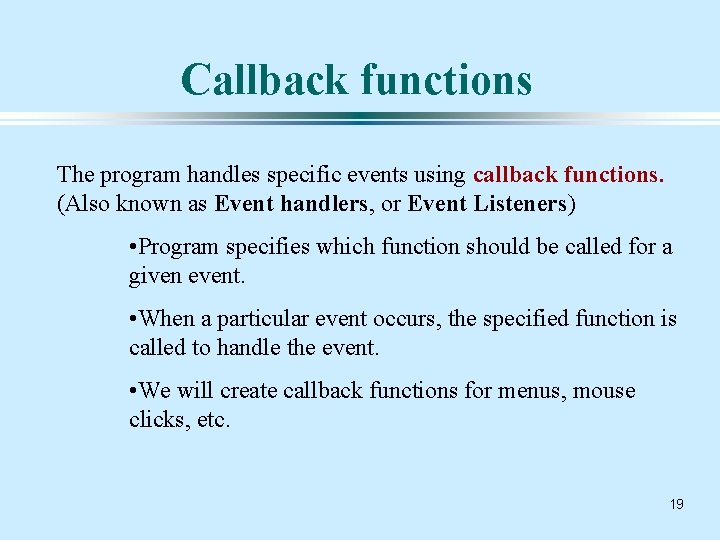
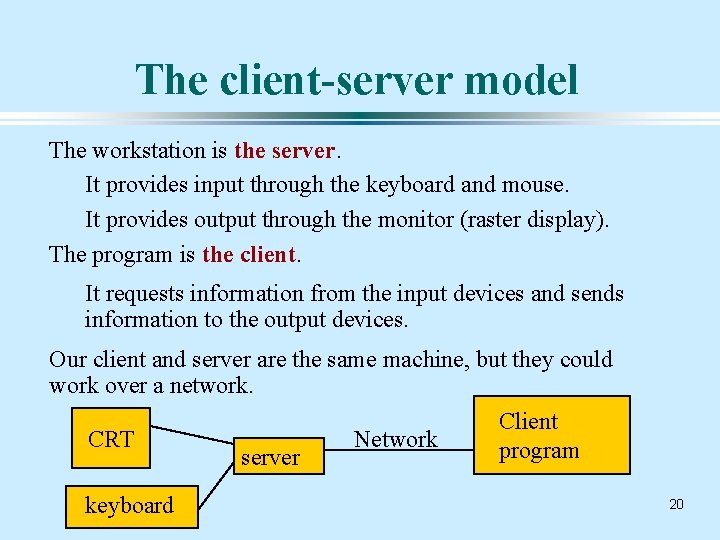
- Slides: 20
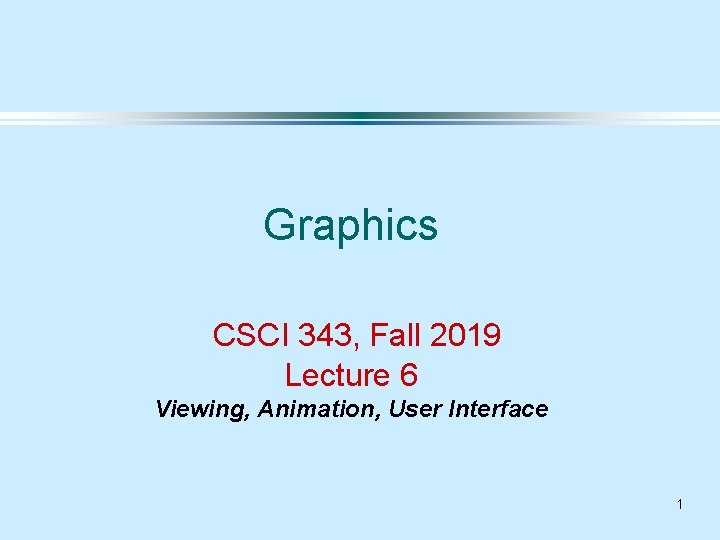
Graphics CSCI 343, Fall 2019 Lecture 6 Viewing, Animation, User Interface 1
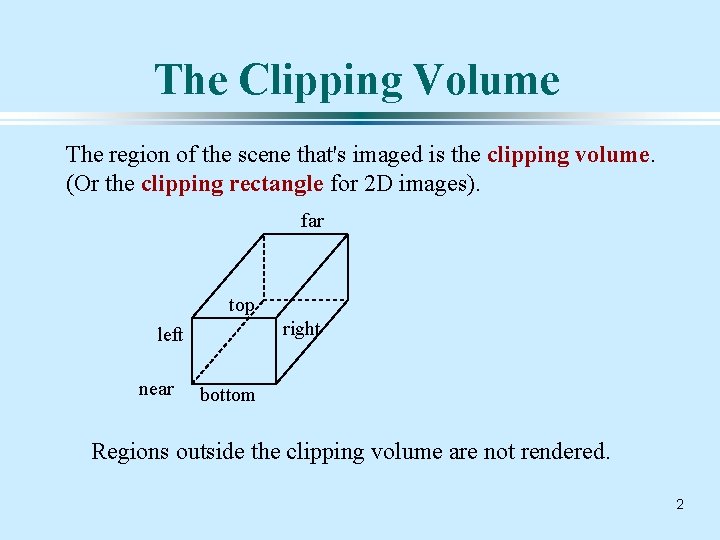
The Clipping Volume The region of the scene that's imaged is the clipping volume. (Or the clipping rectangle for 2 D images). far top right left near bottom Regions outside the clipping volume are not rendered. 2
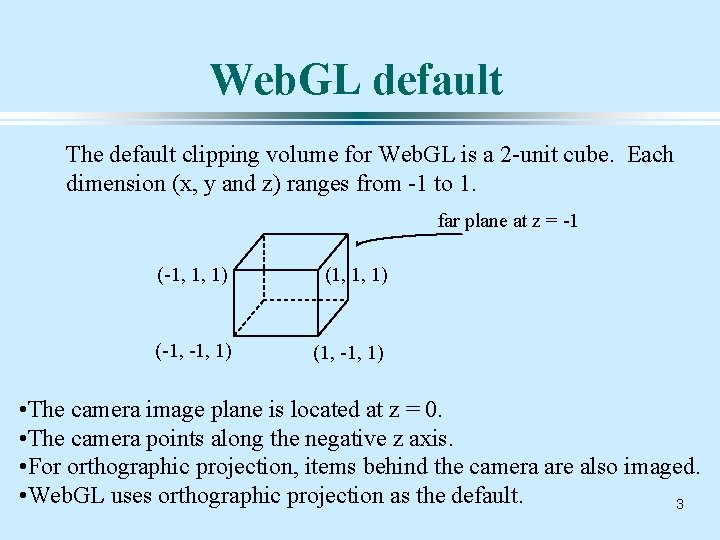
Web. GL default The default clipping volume for Web. GL is a 2 -unit cube. Each dimension (x, y and z) ranges from -1 to 1. far plane at z = -1 (-1, 1, 1) (-1, 1) (1, -1, 1) • The camera image plane is located at z = 0. • The camera points along the negative z axis. • For orthographic projection, items behind the camera are also imaged. • Web. GL uses orthographic projection as the default. 3
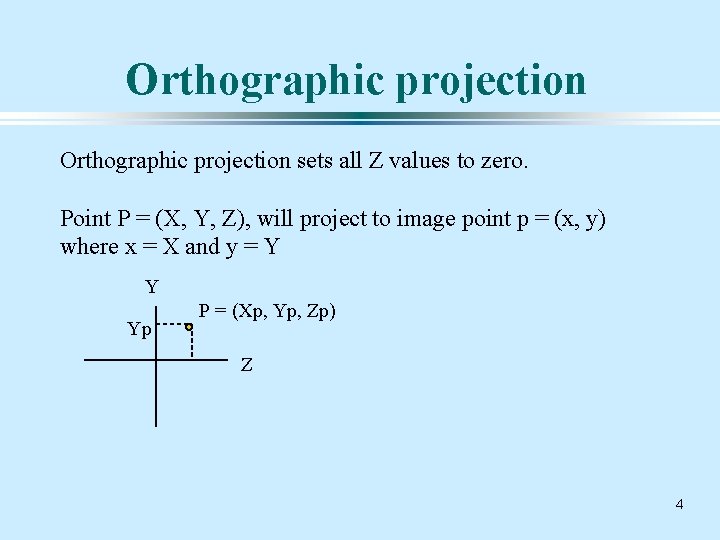
Orthographic projection sets all Z values to zero. Point P = (X, Y, Z), will project to image point p = (x, y) where x = X and y = Y Y Yp P = (Xp, Yp, Zp) Z 4
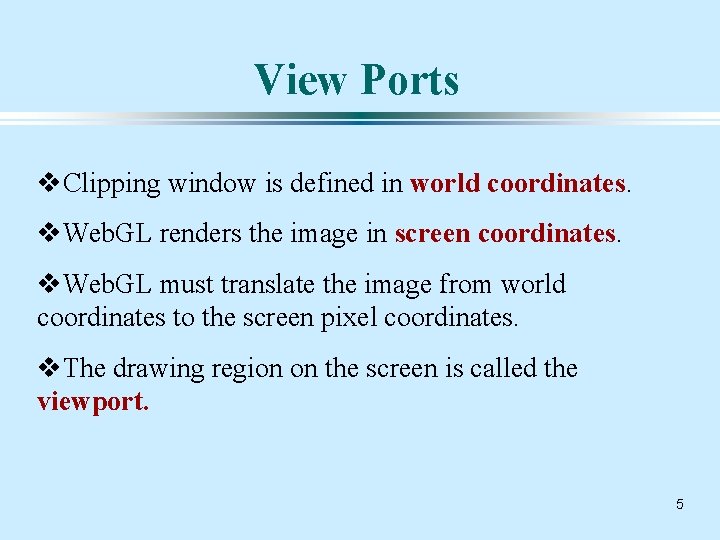
View Ports v. Clipping window is defined in world coordinates. v. Web. GL renders the image in screen coordinates. v. Web. GL must translate the image from world coordinates to the screen pixel coordinates. v. The drawing region on the screen is called the viewport. 5
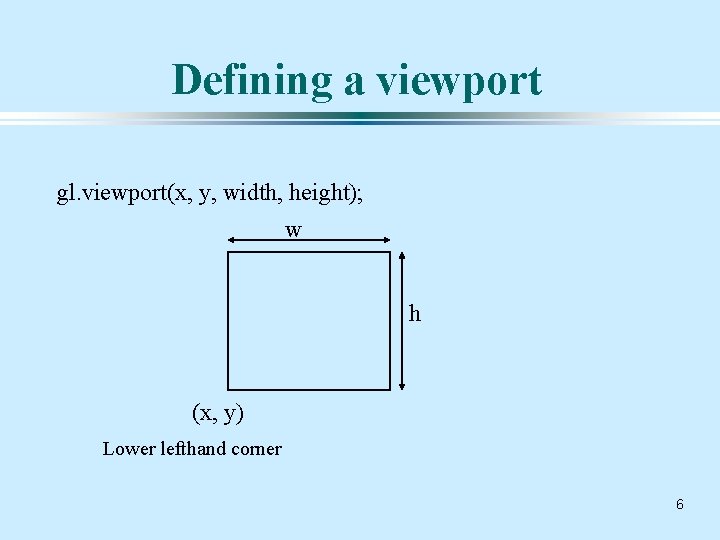
Defining a viewport gl. viewport(x, y, width, height); w h (x, y) Lower lefthand corner 6
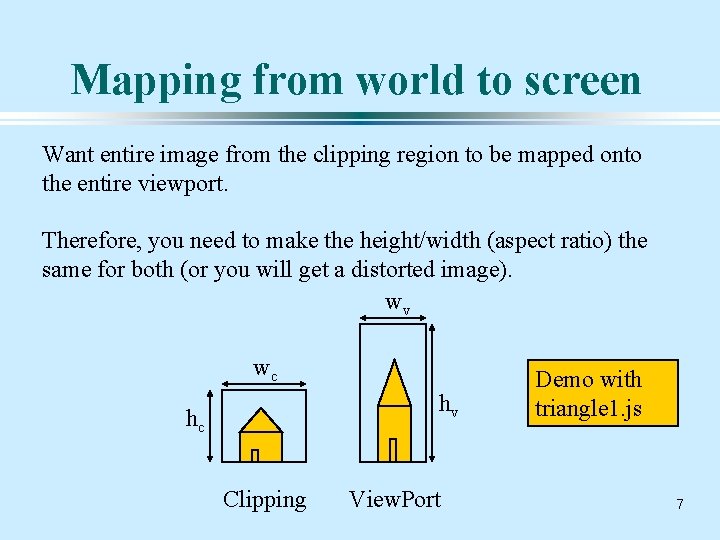
Mapping from world to screen Want entire image from the clipping region to be mapped onto the entire viewport. Therefore, you need to make the height/width (aspect ratio) the same for both (or you will get a distorted image). wv wc hv hc Clipping View. Port Demo with triangle 1. js 7
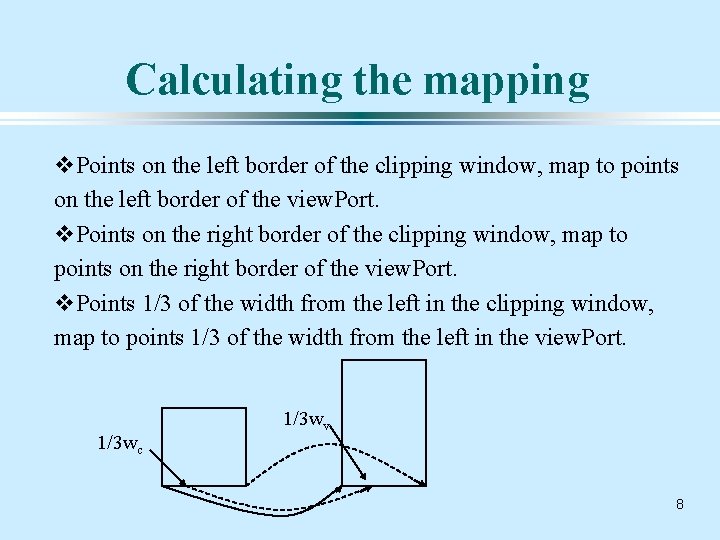
Calculating the mapping v. Points on the left border of the clipping window, map to points on the left border of the view. Port. v. Points on the right border of the clipping window, map to points on the right border of the view. Port. v. Points 1/3 of the width from the left in the clipping window, map to points 1/3 of the width from the left in the view. Port. 1/3 wc 1/3 wv 8
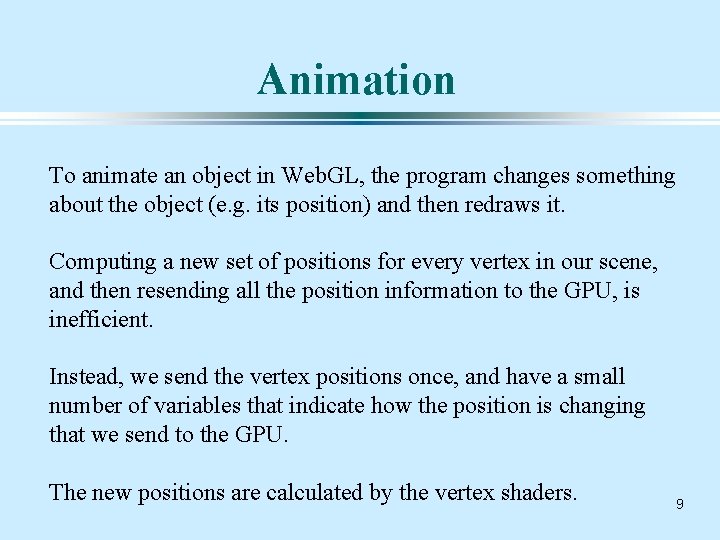
Animation To animate an object in Web. GL, the program changes something about the object (e. g. its position) and then redraws it. Computing a new set of positions for every vertex in our scene, and then resending all the position information to the GPU, is inefficient. Instead, we send the vertex positions once, and have a small number of variables that indicate how the position is changing that we send to the GPU. The new positions are calculated by the vertex shaders. 9
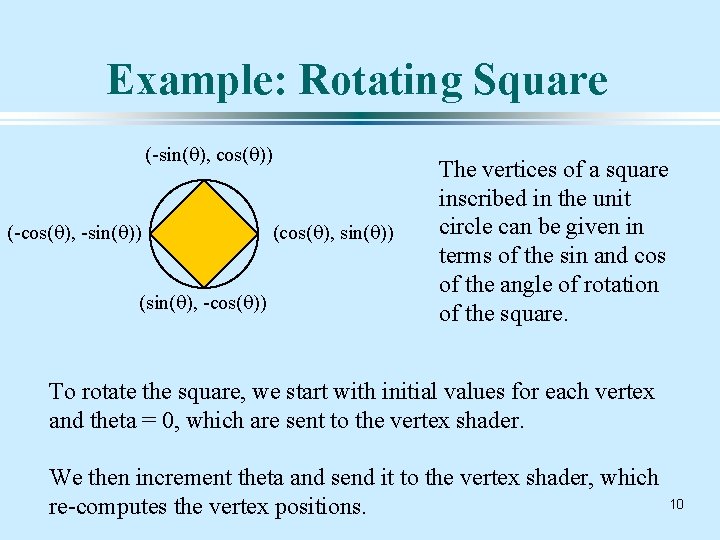
Example: Rotating Square (-sin(q), cos(q)) (-cos(q), -sin(q)) (sin(q), -cos(q)) (cos(q), sin(q)) The vertices of a square inscribed in the unit circle can be given in terms of the sin and cos of the angle of rotation of the square. To rotate the square, we start with initial values for each vertex and theta = 0, which are sent to the vertex shader. We then increment theta and send it to the vertex shader, which re-computes the vertex positions. 10
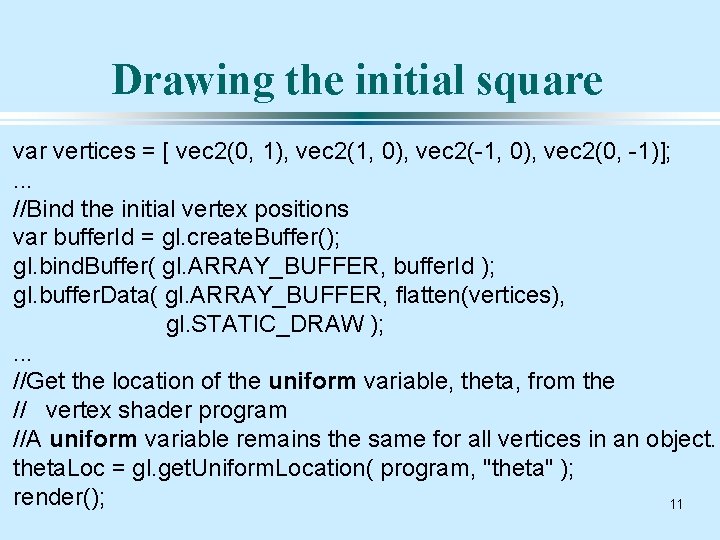
Drawing the initial square var vertices = [ vec 2(0, 1), vec 2(1, 0), vec 2(-1, 0), vec 2(0, -1)]; . . . //Bind the initial vertex positions var buffer. Id = gl. create. Buffer(); gl. bind. Buffer( gl. ARRAY_BUFFER, buffer. Id ); gl. buffer. Data( gl. ARRAY_BUFFER, flatten(vertices), gl. STATIC_DRAW ); . . . //Get the location of the uniform variable, theta, from the // vertex shader program //A uniform variable remains the same for all vertices in an object. theta. Loc = gl. get. Uniform. Location( program, "theta" ); render(); 11
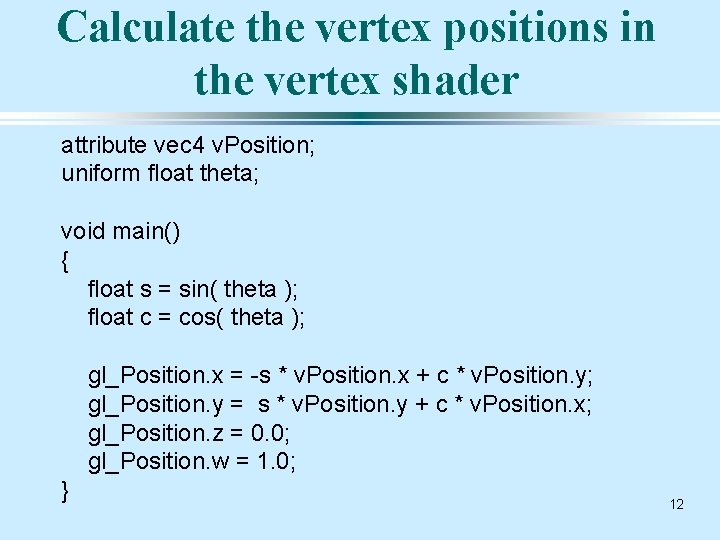
Calculate the vertex positions in the vertex shader attribute vec 4 v. Position; uniform float theta; void main() { float s = sin( theta ); float c = cos( theta ); gl_Position. x = -s * v. Position. x + c * v. Position. y; gl_Position. y = s * v. Position. y + c * v. Position. x; gl_Position. z = 0. 0; gl_Position. w = 1. 0; } 12
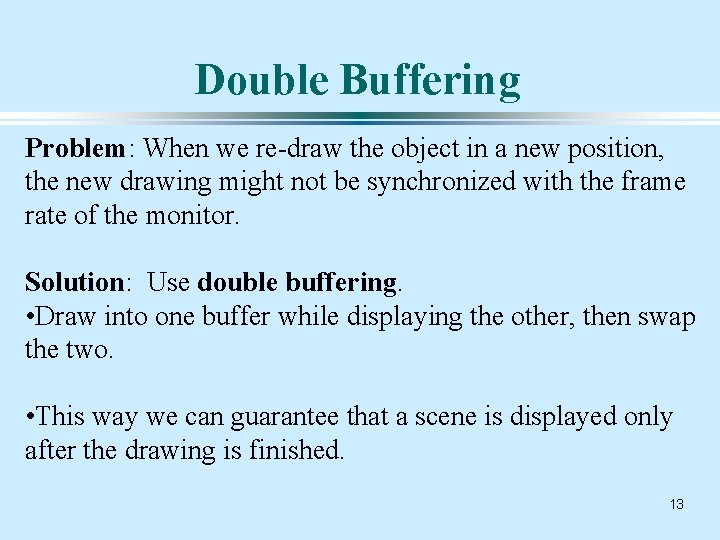
Double Buffering Problem: When we re-draw the object in a new position, the new drawing might not be synchronized with the frame rate of the monitor. Solution: Use double buffering. • Draw into one buffer while displaying the other, then swap the two. • This way we can guarantee that a scene is displayed only after the drawing is finished. 13
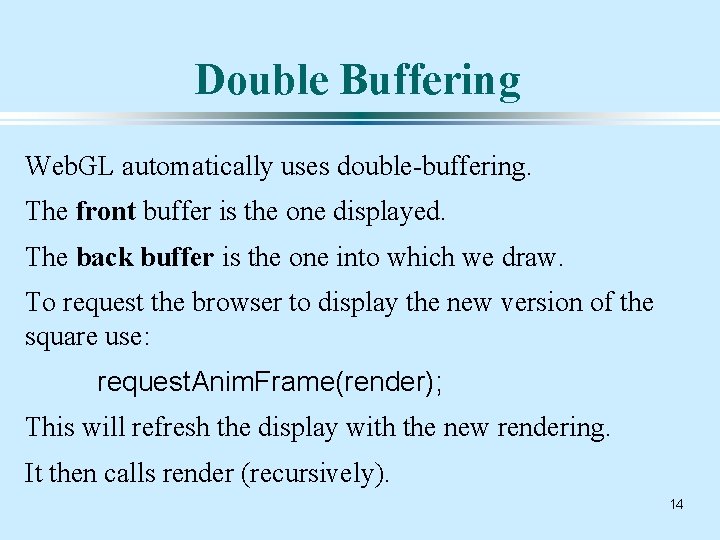
Double Buffering Web. GL automatically uses double-buffering. The front buffer is the one displayed. The back buffer is the one into which we draw. To request the browser to display the new version of the square use: request. Anim. Frame(render); This will refresh the display with the new rendering. It then calls render (recursively). 14
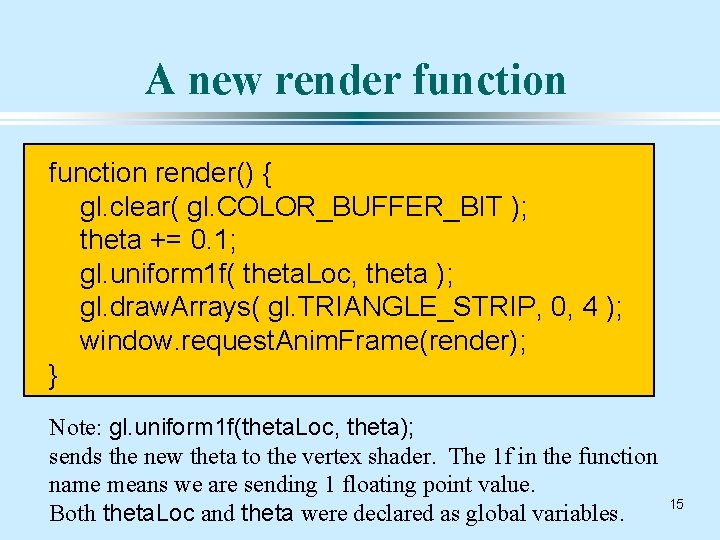
A new render function render() { gl. clear( gl. COLOR_BUFFER_BIT ); theta += 0. 1; gl. uniform 1 f( theta. Loc, theta ); gl. draw. Arrays( gl. TRIANGLE_STRIP, 0, 4 ); window. request. Anim. Frame(render); } Note: gl. uniform 1 f(theta. Loc, theta); sends the new theta to the vertex shader. The 1 f in the function name means we are sending 1 floating point value. Both theta. Loc and theta were declared as global variables. 15
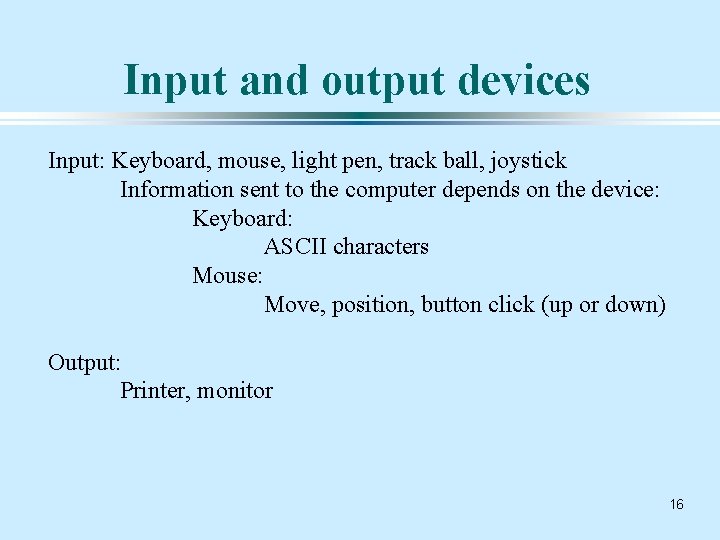
Input and output devices Input: Keyboard, mouse, light pen, track ball, joystick Information sent to the computer depends on the device: Keyboard: ASCII characters Mouse: Move, position, button click (up or down) Output: Printer, monitor 16
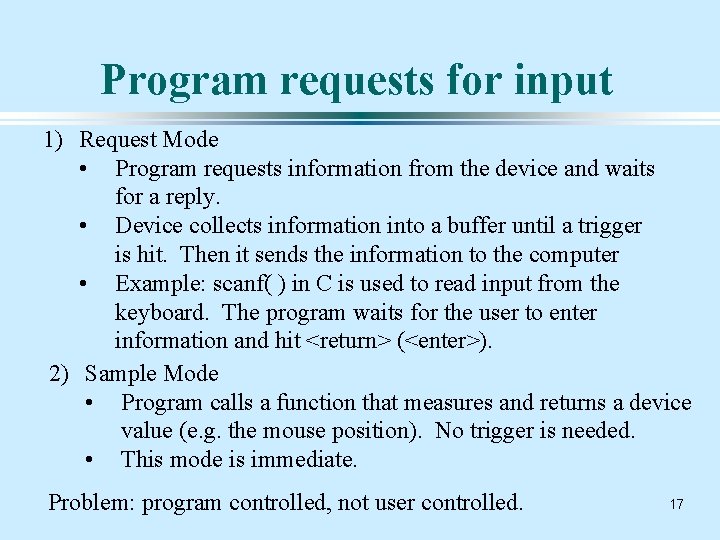
Program requests for input 1) Request Mode • Program requests information from the device and waits for a reply. • Device collects information into a buffer until a trigger is hit. Then it sends the information to the computer • Example: scanf( ) in C is used to read input from the keyboard. The program waits for the user to enter information and hit <return> (<enter>). 2) Sample Mode • Program calls a function that measures and returns a device value (e. g. the mouse position). No trigger is needed. • This mode is immediate. Problem: program controlled, not user controlled. 17
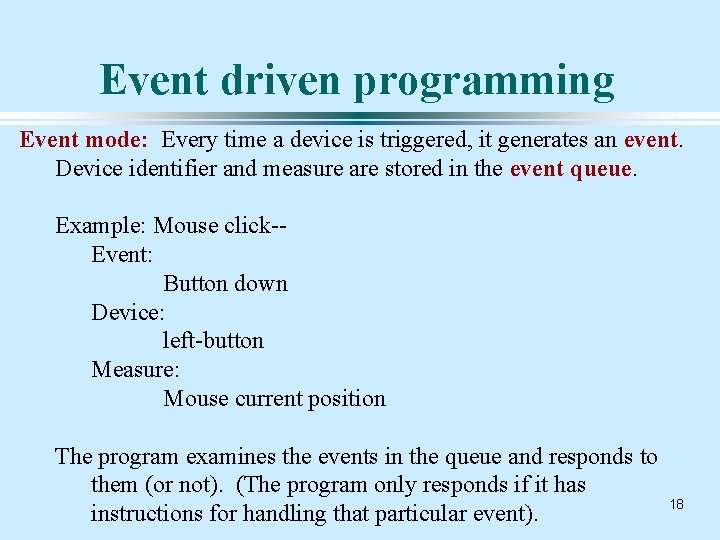
Event driven programming Event mode: Every time a device is triggered, it generates an event. Device identifier and measure are stored in the event queue. Example: Mouse click-Event: Button down Device: left-button Measure: Mouse current position The program examines the events in the queue and responds to them (or not). (The program only responds if it has instructions for handling that particular event). 18
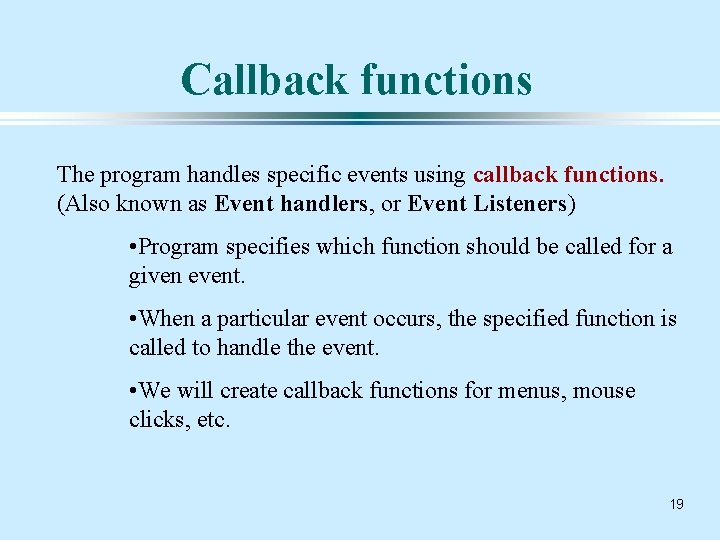
Callback functions The program handles specific events using callback functions. (Also known as Event handlers, or Event Listeners) • Program specifies which function should be called for a given event. • When a particular event occurs, the specified function is called to handle the event. • We will create callback functions for menus, mouse clicks, etc. 19
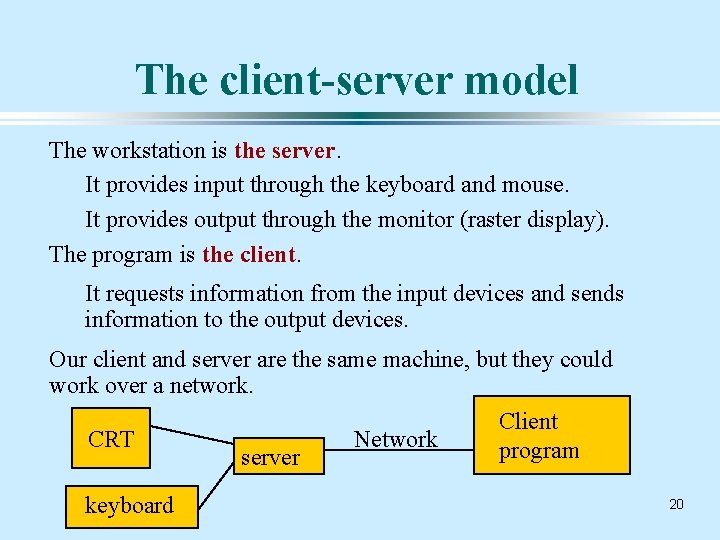
The client-server model The workstation is the server. It provides input through the keyboard and mouse. It provides output through the monitor (raster display). The program is the client. It requests information from the input devices and sends information to the output devices. Our client and server are the same machine, but they could work over a network. Client CRT Network program server keyboard 20
Define viewing in computer graphics
Graphics hardware in computer graphics ppt
Viewing transformation pipeline
01:640:244 lecture notes - lecture 15: plat, idah, farad
Hand held computer
Opkins
Log 343
Experiment 343
Ds renkema
343 descompus
Csc 343
Csc343
343 pro session
Soen 343
Soen 343
X3=64/343
125x^3-64
Texas health and safety code 343
343 jesus
Cecs 343
582 x 4