Graphics CSCI 343 Fall 2019 Lecture 25 Texture
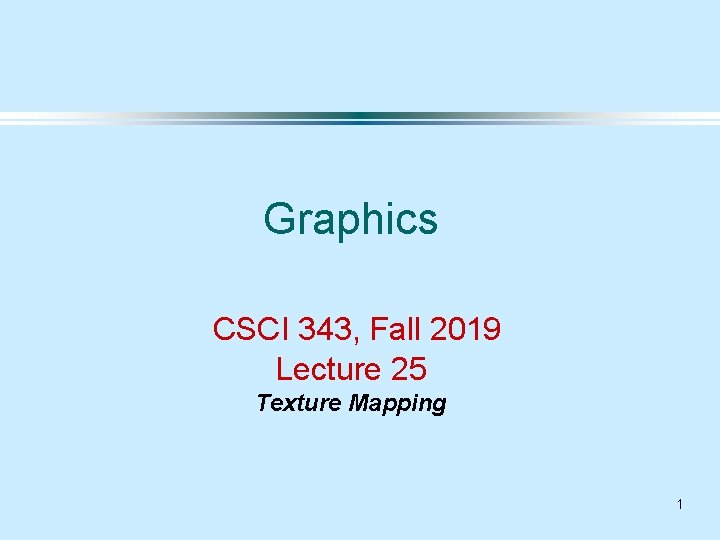
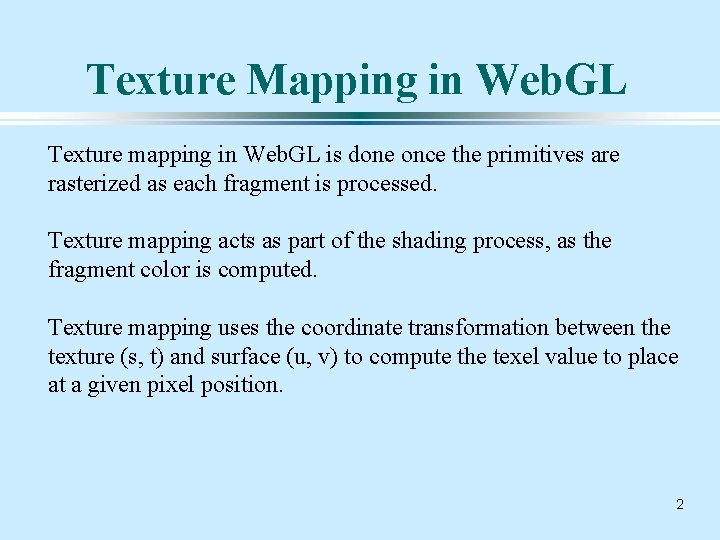
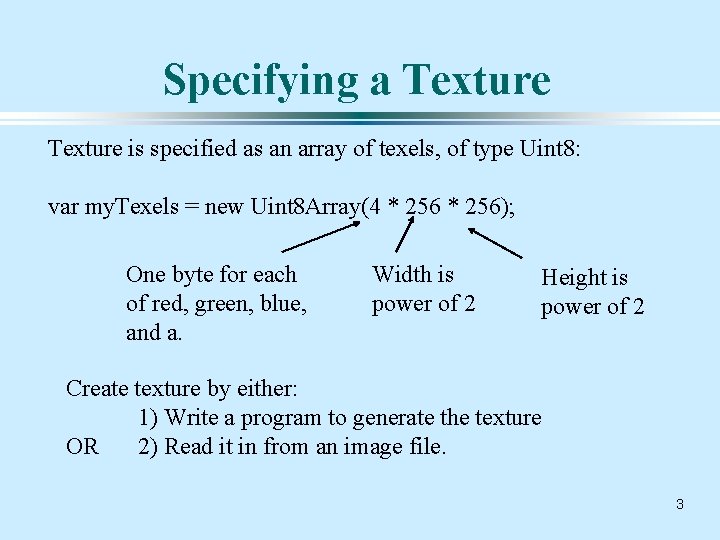
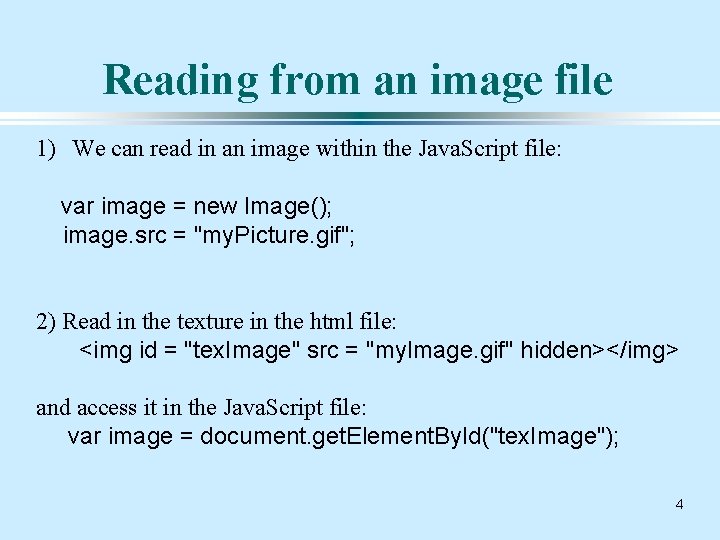
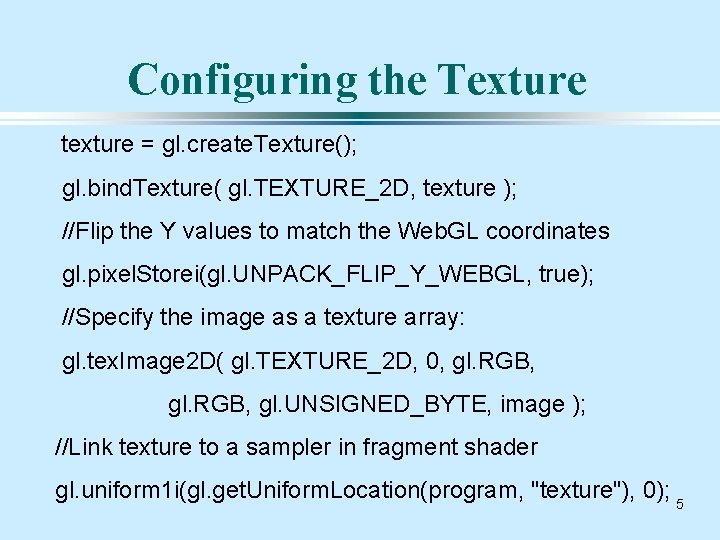
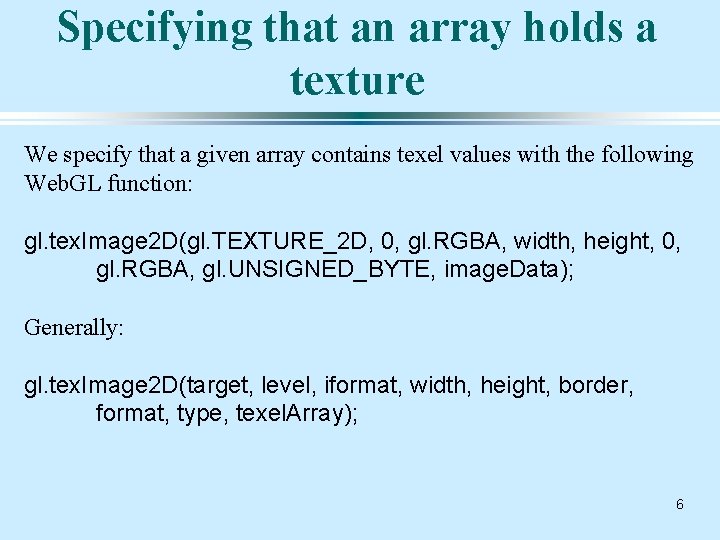
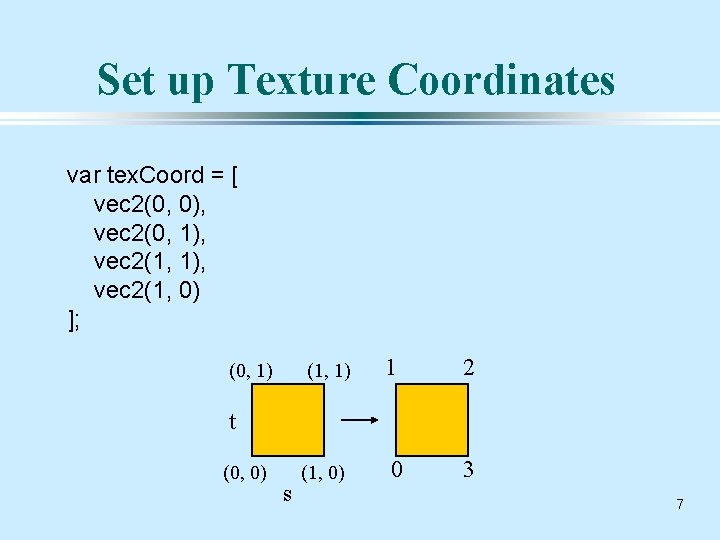
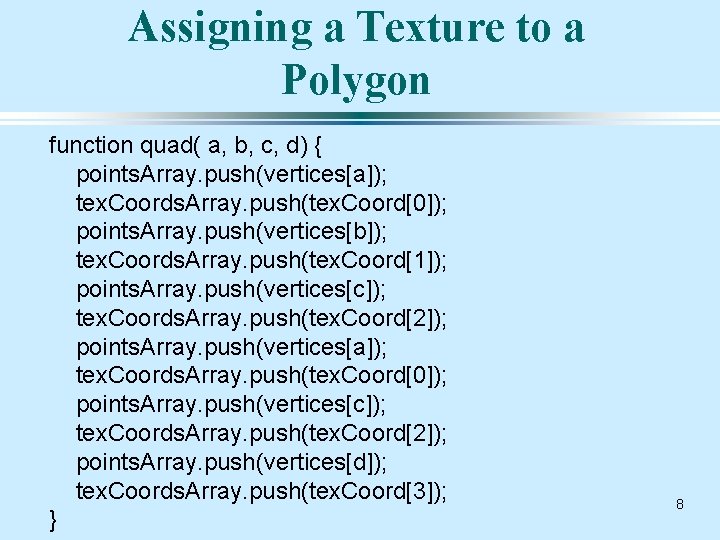
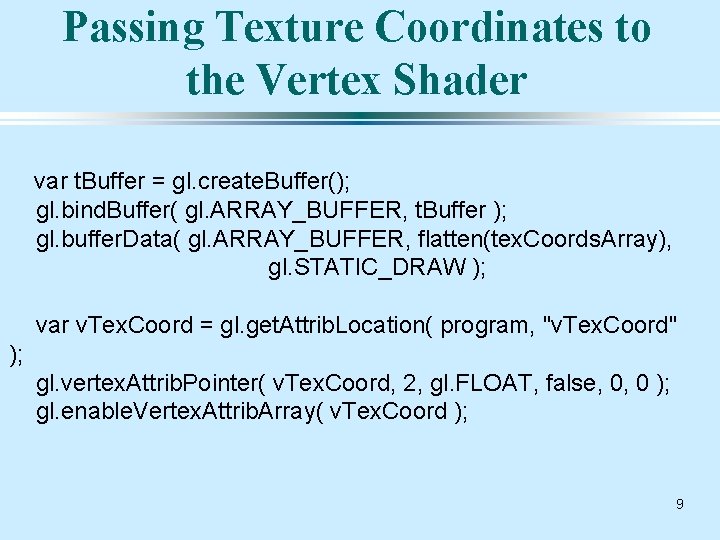
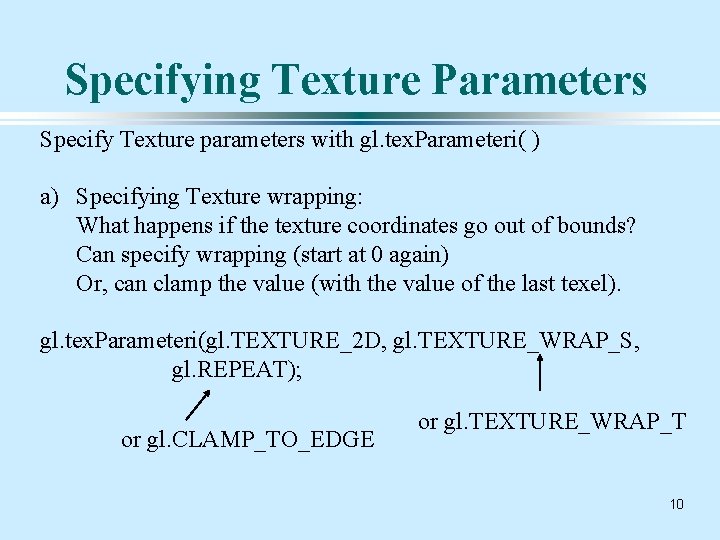
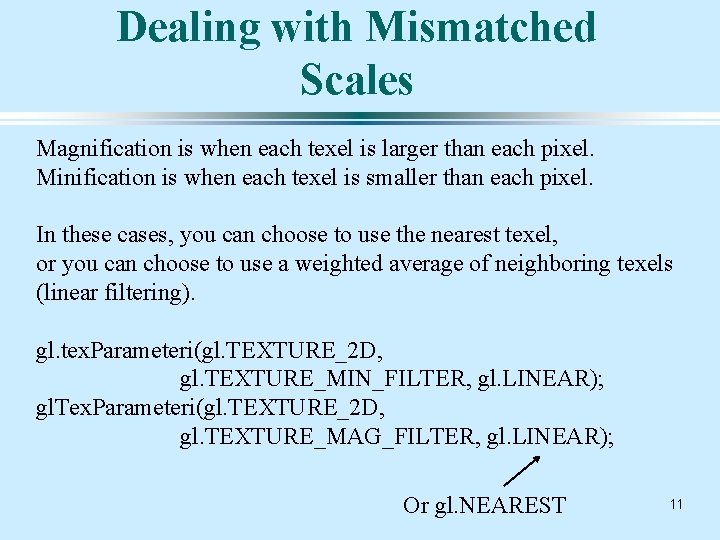
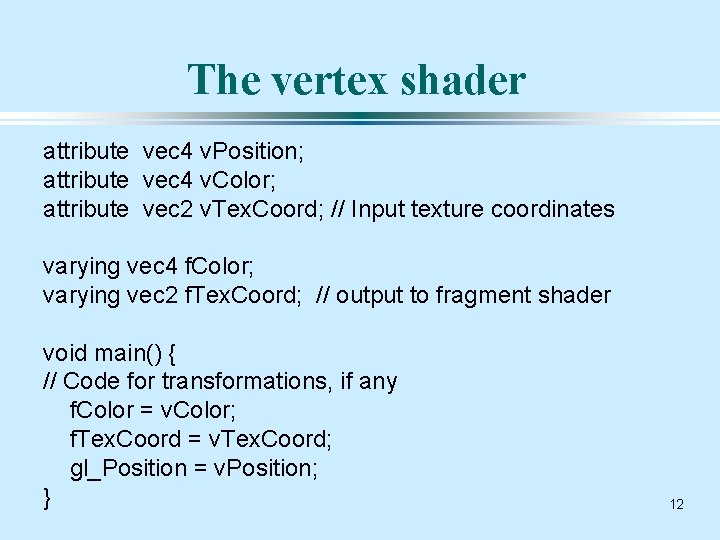
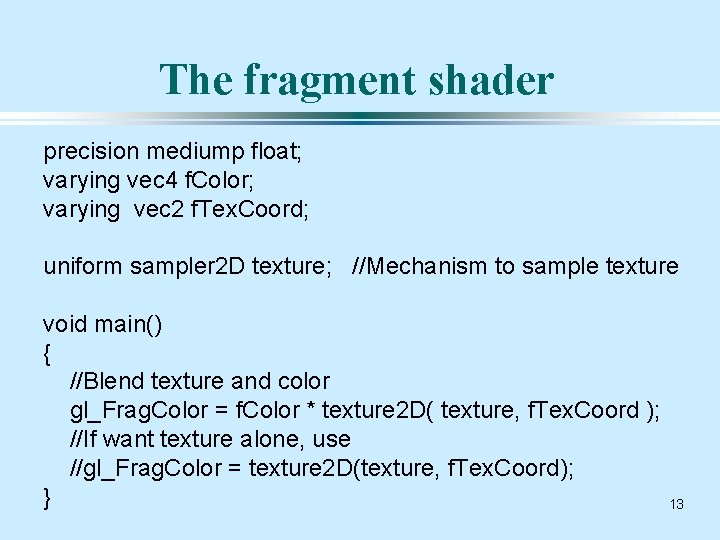
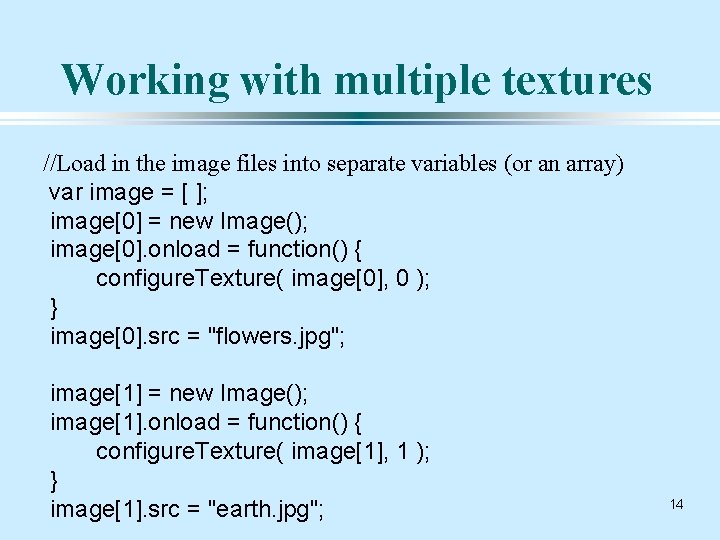
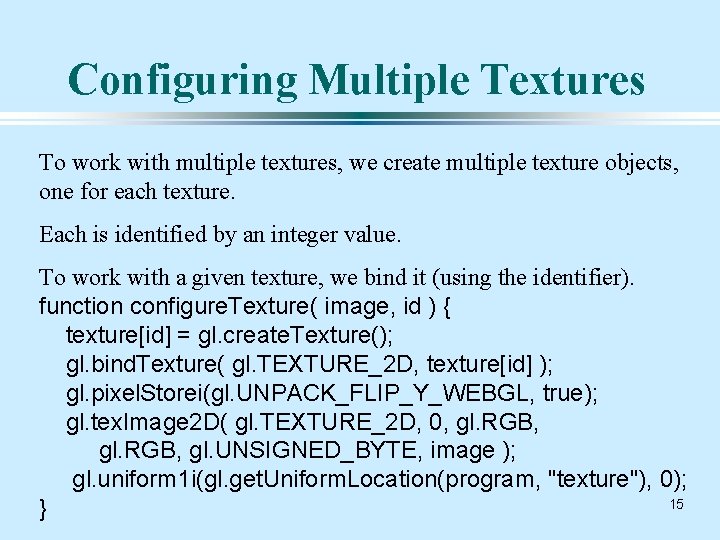
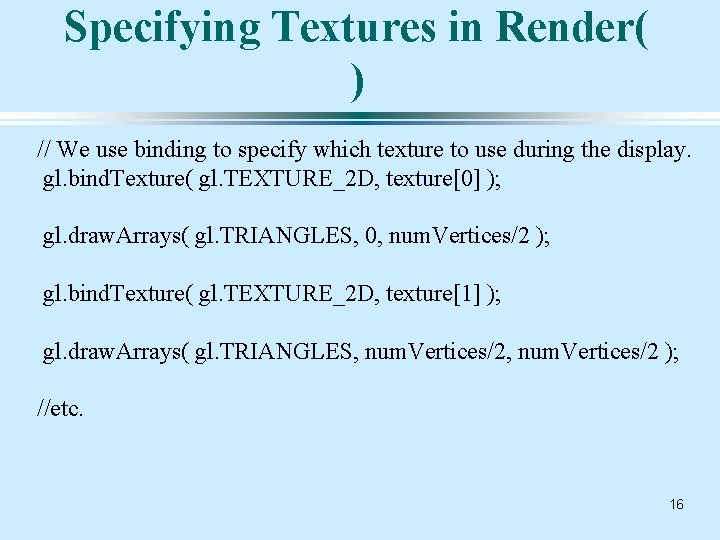
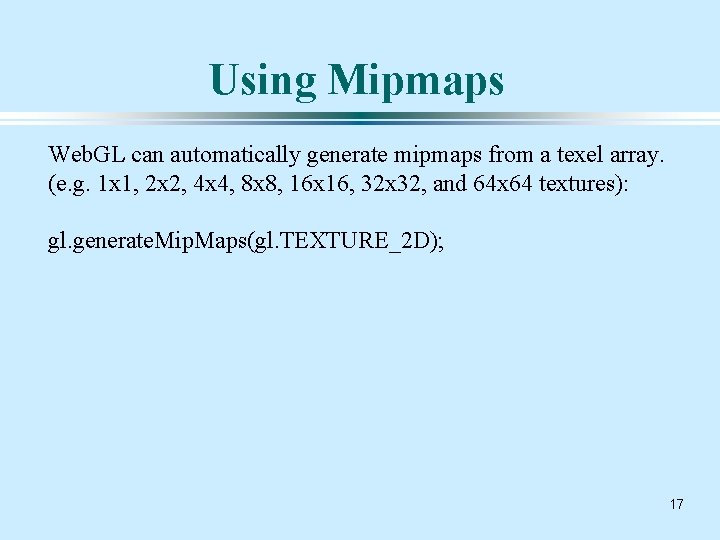
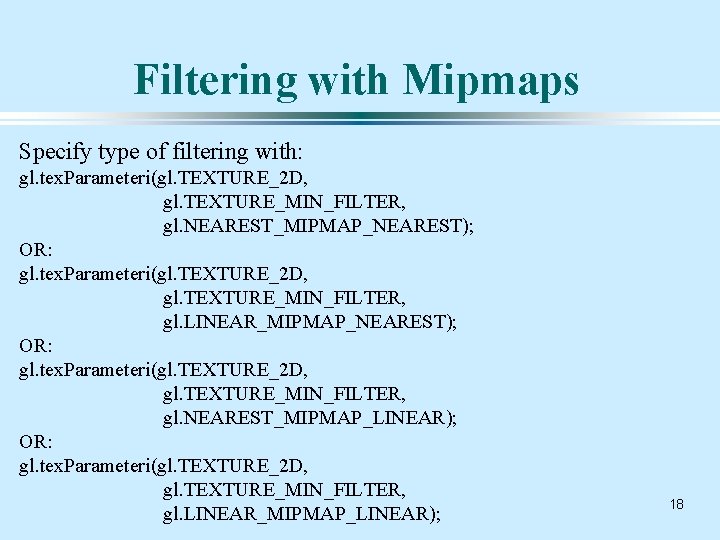
- Slides: 18
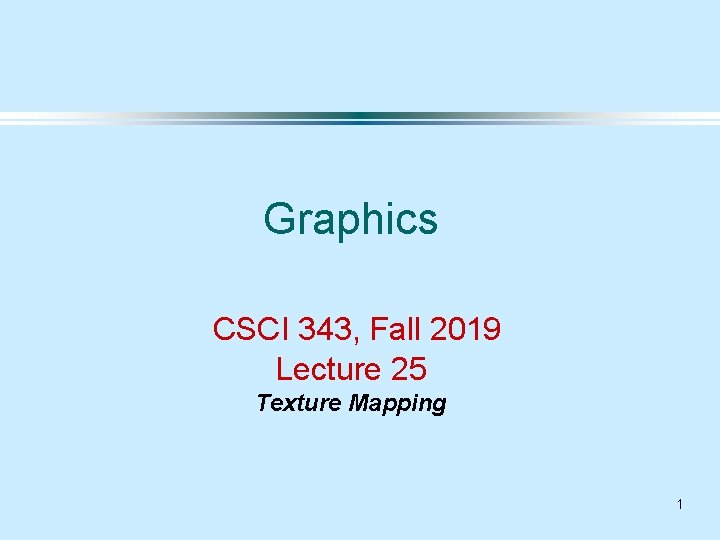
Graphics CSCI 343, Fall 2019 Lecture 25 Texture Mapping 1
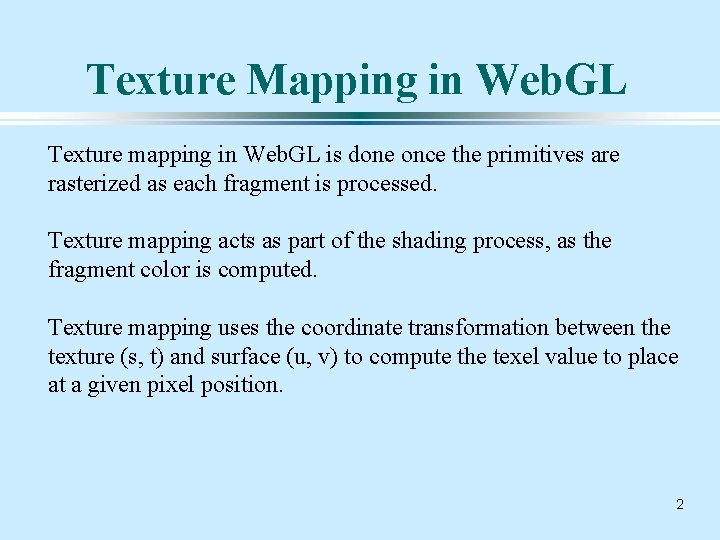
Texture Mapping in Web. GL Texture mapping in Web. GL is done once the primitives are rasterized as each fragment is processed. Texture mapping acts as part of the shading process, as the fragment color is computed. Texture mapping uses the coordinate transformation between the texture (s, t) and surface (u, v) to compute the texel value to place at a given pixel position. 2
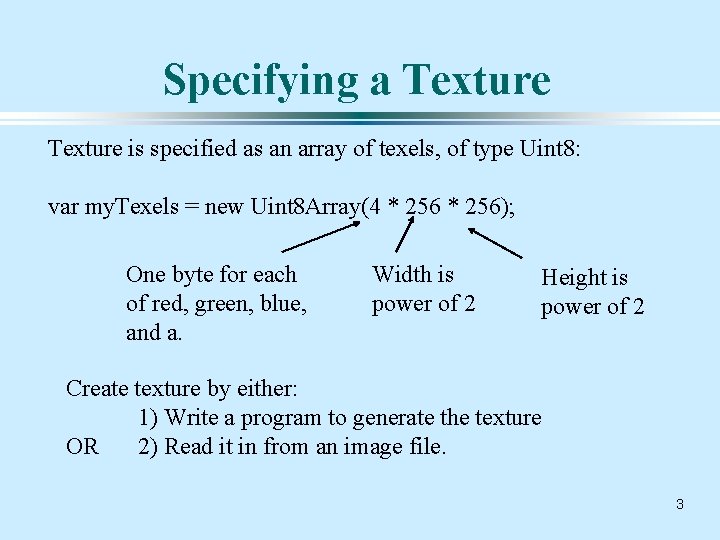
Specifying a Texture is specified as an array of texels, of type Uint 8: var my. Texels = new Uint 8 Array(4 * 256); One byte for each of red, green, blue, and a. Width is power of 2 Height is power of 2 Create texture by either: 1) Write a program to generate the texture OR 2) Read it in from an image file. 3
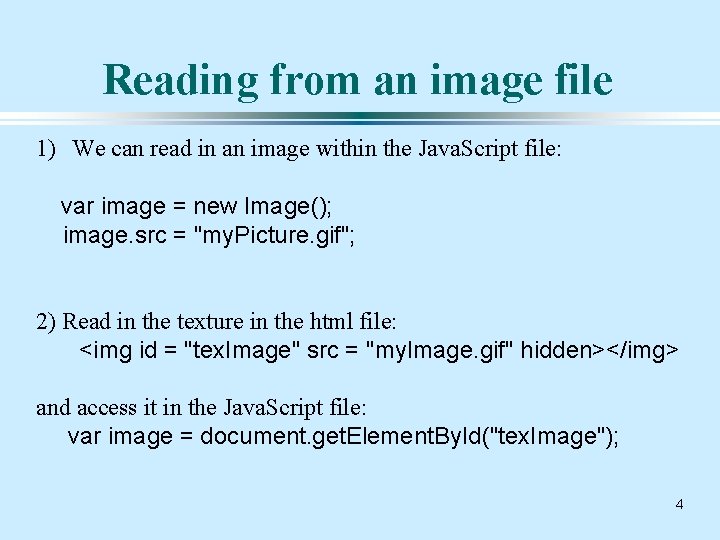
Reading from an image file 1) We can read in an image within the Java. Script file: var image = new Image(); image. src = "my. Picture. gif"; 2) Read in the texture in the html file: <img id = "tex. Image" src = "my. Image. gif" hidden></img> and access it in the Java. Script file: var image = document. get. Element. By. Id("tex. Image"); 4
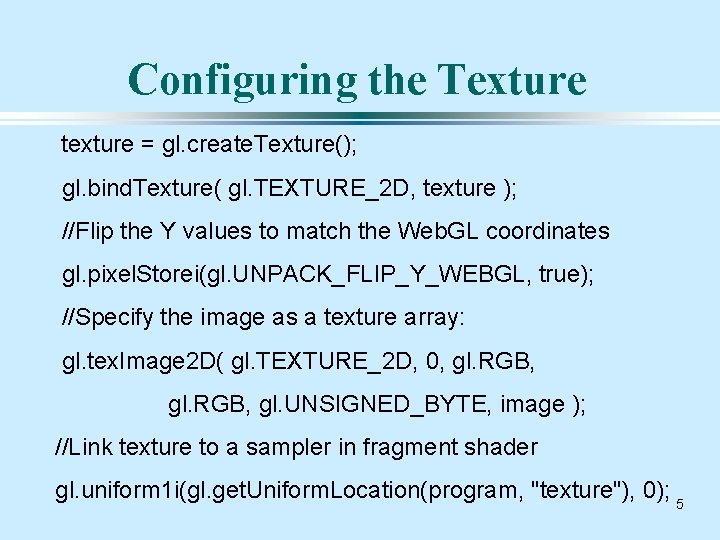
Configuring the Texture texture = gl. create. Texture(); gl. bind. Texture( gl. TEXTURE_2 D, texture ); //Flip the Y values to match the Web. GL coordinates gl. pixel. Storei(gl. UNPACK_FLIP_Y_WEBGL, true); //Specify the image as a texture array: gl. tex. Image 2 D( gl. TEXTURE_2 D, 0, gl. RGB, gl. UNSIGNED_BYTE, image ); //Link texture to a sampler in fragment shader gl. uniform 1 i(gl. get. Uniform. Location(program, "texture"), 0); 5
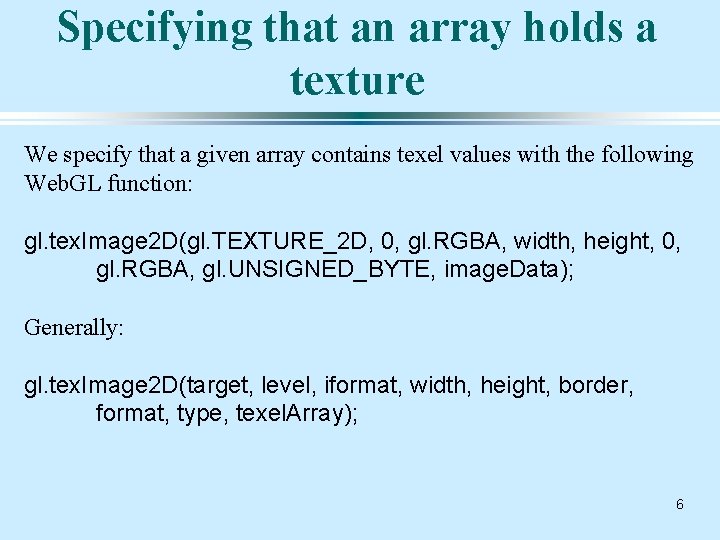
Specifying that an array holds a texture We specify that a given array contains texel values with the following Web. GL function: gl. tex. Image 2 D(gl. TEXTURE_2 D, 0, gl. RGBA, width, height, 0, gl. RGBA, gl. UNSIGNED_BYTE, image. Data); Generally: gl. tex. Image 2 D(target, level, iformat, width, height, border, format, type, texel. Array); 6
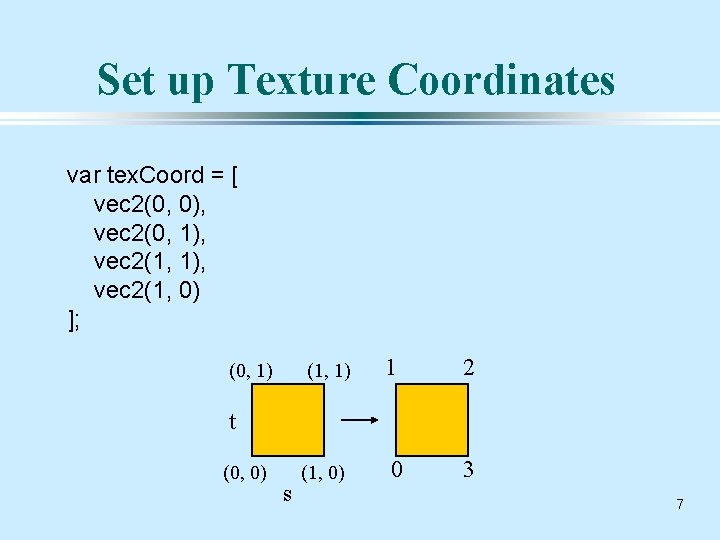
Set up Texture Coordinates var tex. Coord = [ vec 2(0, 0), vec 2(0, 1), vec 2(1, 0) ]; (0, 1) (1, 1) 1 2 (1, 0) 0 3 t (0, 0) s 7
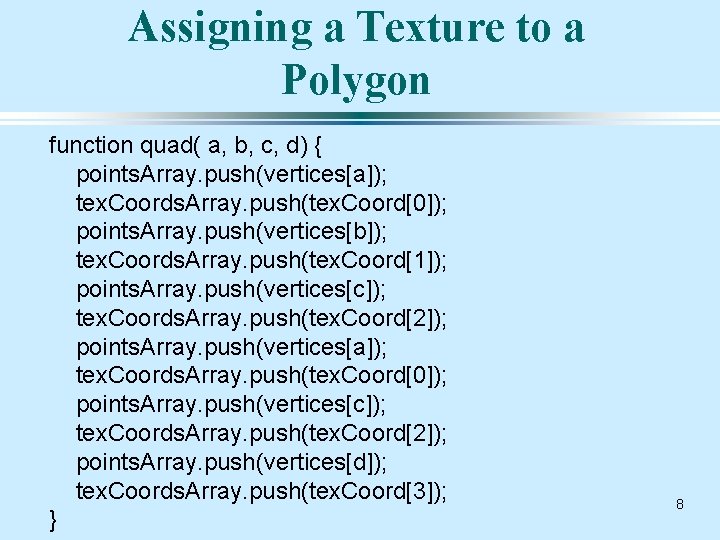
Assigning a Texture to a Polygon function quad( a, b, c, d) { points. Array. push(vertices[a]); tex. Coords. Array. push(tex. Coord[0]); points. Array. push(vertices[b]); tex. Coords. Array. push(tex. Coord[1]); points. Array. push(vertices[c]); tex. Coords. Array. push(tex. Coord[2]); points. Array. push(vertices[a]); tex. Coords. Array. push(tex. Coord[0]); points. Array. push(vertices[c]); tex. Coords. Array. push(tex. Coord[2]); points. Array. push(vertices[d]); tex. Coords. Array. push(tex. Coord[3]); } 8
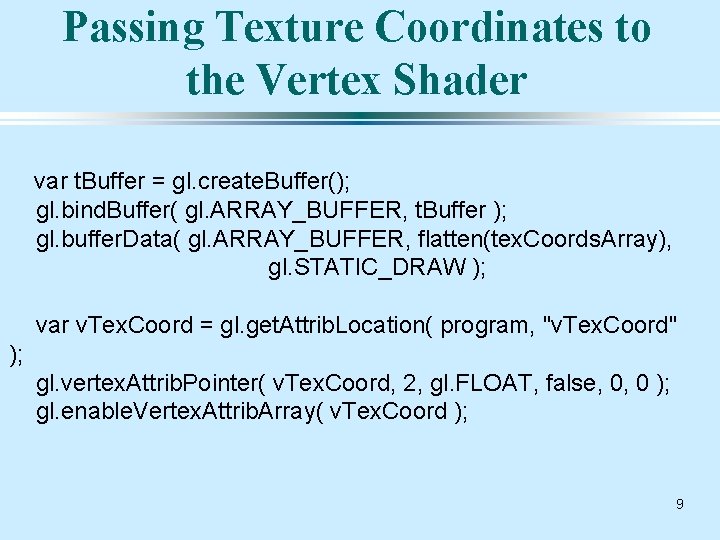
Passing Texture Coordinates to the Vertex Shader var t. Buffer = gl. create. Buffer(); gl. bind. Buffer( gl. ARRAY_BUFFER, t. Buffer ); gl. buffer. Data( gl. ARRAY_BUFFER, flatten(tex. Coords. Array), gl. STATIC_DRAW ); var v. Tex. Coord = gl. get. Attrib. Location( program, "v. Tex. Coord" ); gl. vertex. Attrib. Pointer( v. Tex. Coord, 2, gl. FLOAT, false, 0, 0 ); gl. enable. Vertex. Attrib. Array( v. Tex. Coord ); 9
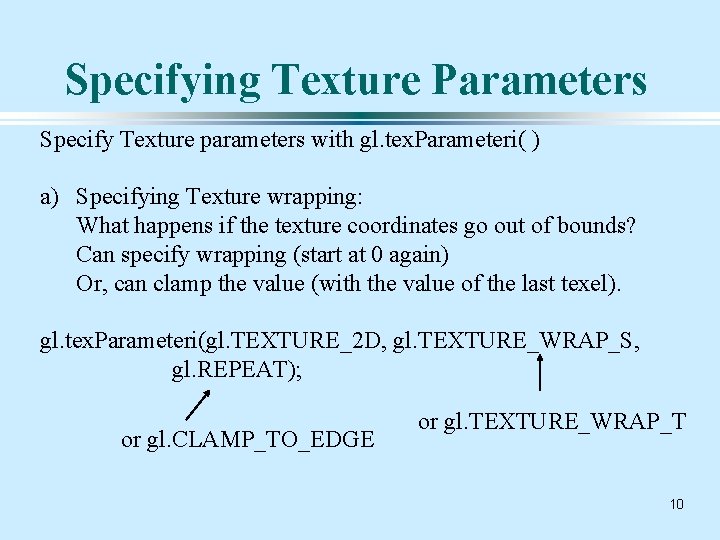
Specifying Texture Parameters Specify Texture parameters with gl. tex. Parameteri( ) a) Specifying Texture wrapping: What happens if the texture coordinates go out of bounds? Can specify wrapping (start at 0 again) Or, can clamp the value (with the value of the last texel). gl. tex. Parameteri(gl. TEXTURE_2 D, gl. TEXTURE_WRAP_S, gl. REPEAT); or gl. CLAMP_TO_EDGE or gl. TEXTURE_WRAP_T 10
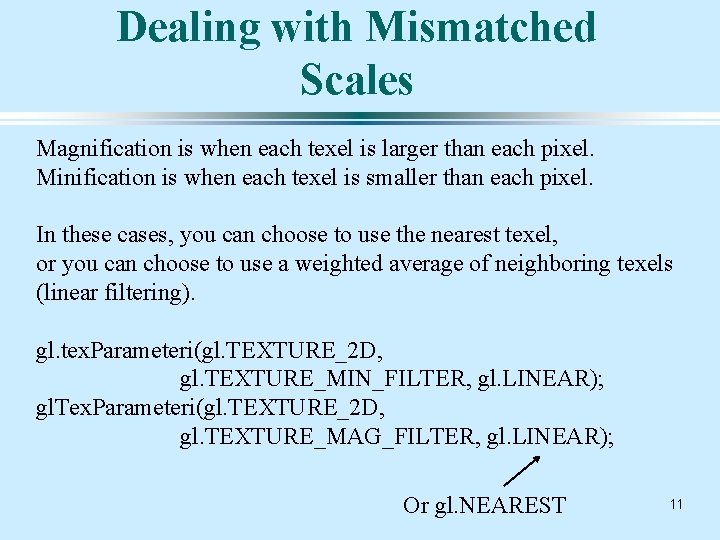
Dealing with Mismatched Scales Magnification is when each texel is larger than each pixel. Minification is when each texel is smaller than each pixel. In these cases, you can choose to use the nearest texel, or you can choose to use a weighted average of neighboring texels (linear filtering). gl. tex. Parameteri(gl. TEXTURE_2 D, gl. TEXTURE_MIN_FILTER, gl. LINEAR); gl. Tex. Parameteri(gl. TEXTURE_2 D, gl. TEXTURE_MAG_FILTER, gl. LINEAR); Or gl. NEAREST 11
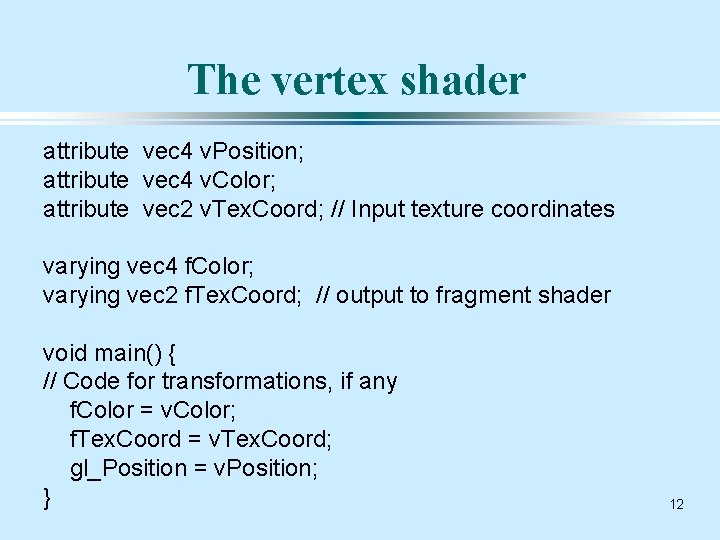
The vertex shader attribute vec 4 v. Position; attribute vec 4 v. Color; attribute vec 2 v. Tex. Coord; // Input texture coordinates varying vec 4 f. Color; varying vec 2 f. Tex. Coord; // output to fragment shader void main() { // Code for transformations, if any f. Color = v. Color; f. Tex. Coord = v. Tex. Coord; gl_Position = v. Position; } 12
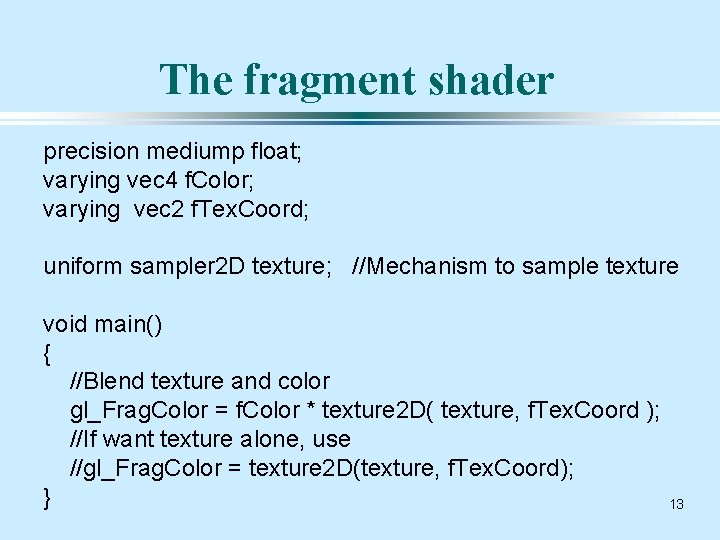
The fragment shader precision mediump float; varying vec 4 f. Color; varying vec 2 f. Tex. Coord; uniform sampler 2 D texture; //Mechanism to sample texture void main() { //Blend texture and color gl_Frag. Color = f. Color * texture 2 D( texture, f. Tex. Coord ); //If want texture alone, use //gl_Frag. Color = texture 2 D(texture, f. Tex. Coord); } 13
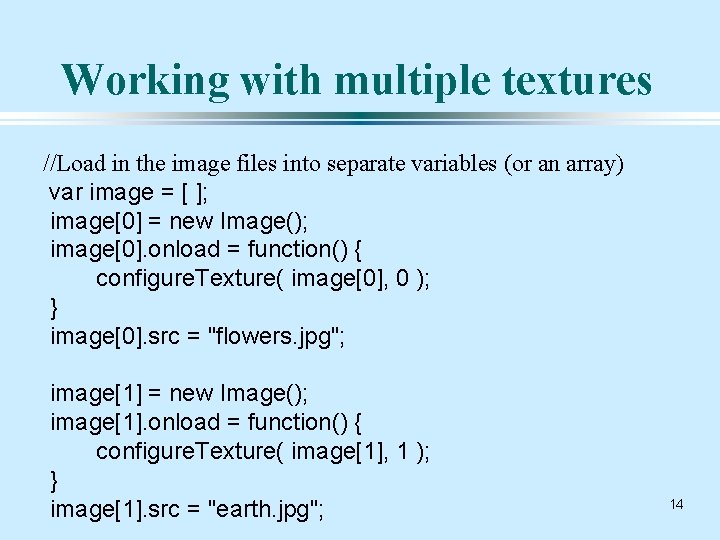
Working with multiple textures //Load in the image files into separate variables (or an array) var image = [ ]; image[0] = new Image(); image[0]. onload = function() { configure. Texture( image[0], 0 ); } image[0]. src = "flowers. jpg"; image[1] = new Image(); image[1]. onload = function() { configure. Texture( image[1], 1 ); } image[1]. src = "earth. jpg"; 14
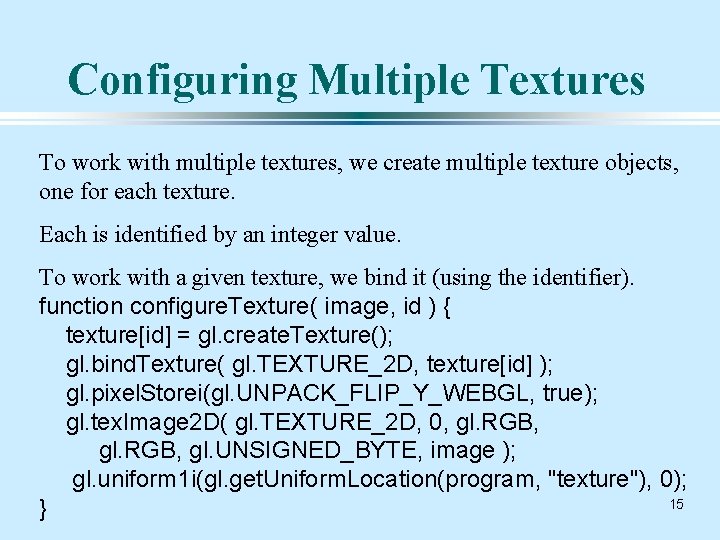
Configuring Multiple Textures To work with multiple textures, we create multiple texture objects, one for each texture. Each is identified by an integer value. To work with a given texture, we bind it (using the identifier). function configure. Texture( image, id ) { texture[id] = gl. create. Texture(); gl. bind. Texture( gl. TEXTURE_2 D, texture[id] ); gl. pixel. Storei(gl. UNPACK_FLIP_Y_WEBGL, true); gl. tex. Image 2 D( gl. TEXTURE_2 D, 0, gl. RGB, gl. UNSIGNED_BYTE, image ); gl. uniform 1 i(gl. get. Uniform. Location(program, "texture"), 0); 15 }
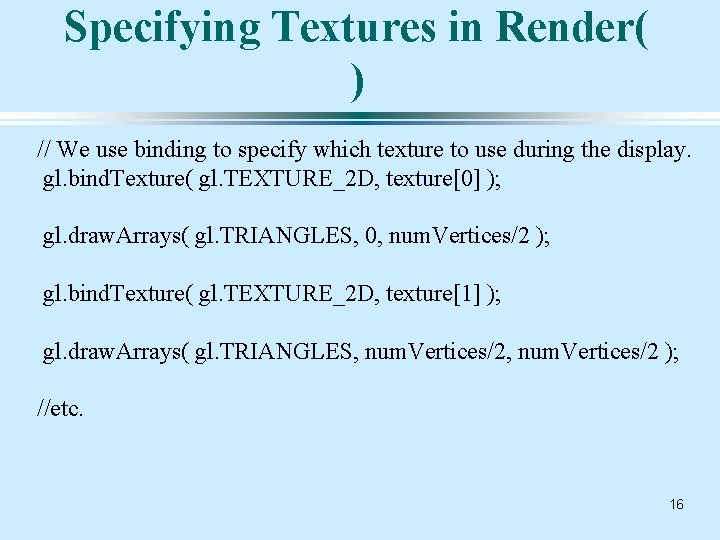
Specifying Textures in Render( ) // We use binding to specify which texture to use during the display. gl. bind. Texture( gl. TEXTURE_2 D, texture[0] ); gl. draw. Arrays( gl. TRIANGLES, 0, num. Vertices/2 ); gl. bind. Texture( gl. TEXTURE_2 D, texture[1] ); gl. draw. Arrays( gl. TRIANGLES, num. Vertices/2 ); //etc. 16
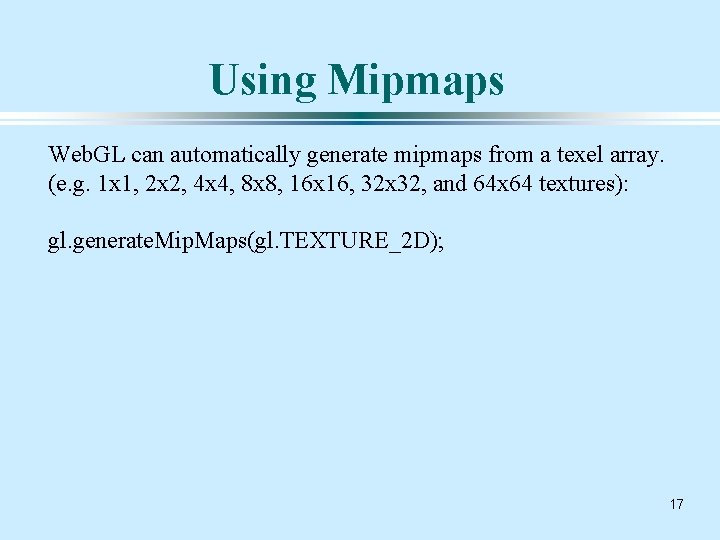
Using Mipmaps Web. GL can automatically generate mipmaps from a texel array. (e. g. 1 x 1, 2 x 2, 4 x 4, 8 x 8, 16 x 16, 32 x 32, and 64 x 64 textures): gl. generate. Mip. Maps(gl. TEXTURE_2 D); 17
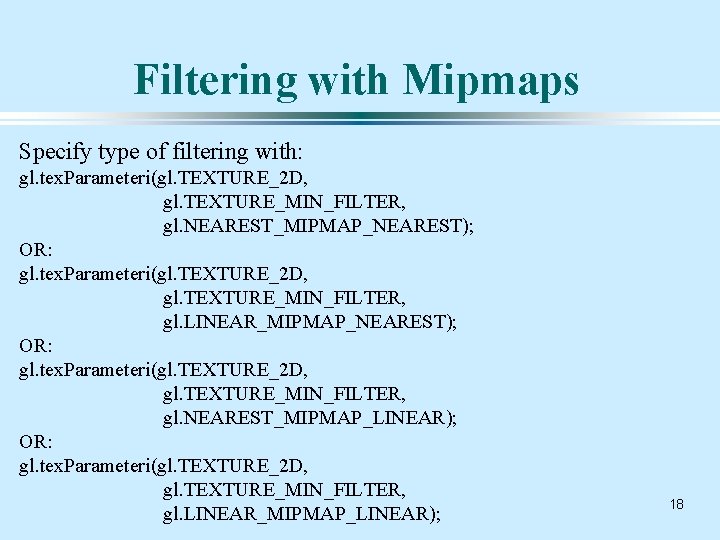
Filtering with Mipmaps Specify type of filtering with: gl. tex. Parameteri(gl. TEXTURE_2 D, gl. TEXTURE_MIN_FILTER, gl. NEAREST_MIPMAP_NEAREST); OR: gl. tex. Parameteri(gl. TEXTURE_2 D, gl. TEXTURE_MIN_FILTER, gl. LINEAR_MIPMAP_NEAREST); OR: gl. tex. Parameteri(gl. TEXTURE_2 D, gl. TEXTURE_MIN_FILTER, gl. NEAREST_MIPMAP_LINEAR); OR: gl. tex. Parameteri(gl. TEXTURE_2 D, gl. TEXTURE_MIN_FILTER, gl. LINEAR_MIPMAP_LINEAR); 18