Graphics CSCI 343 Fall 2019 Lecture 13 More
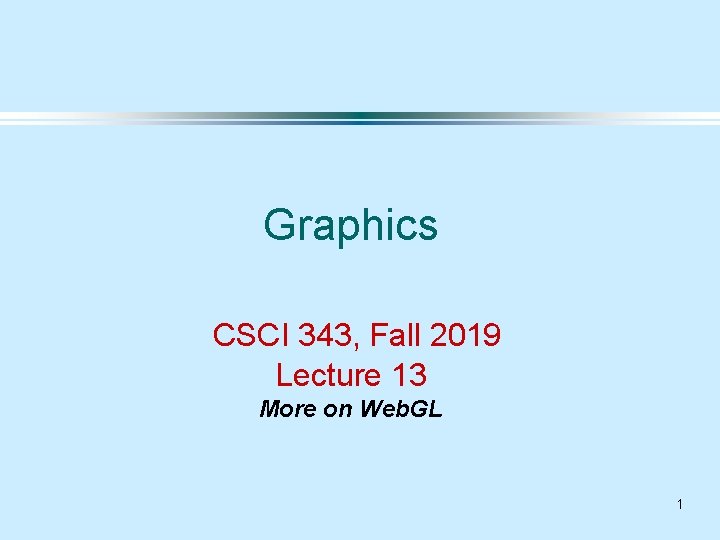
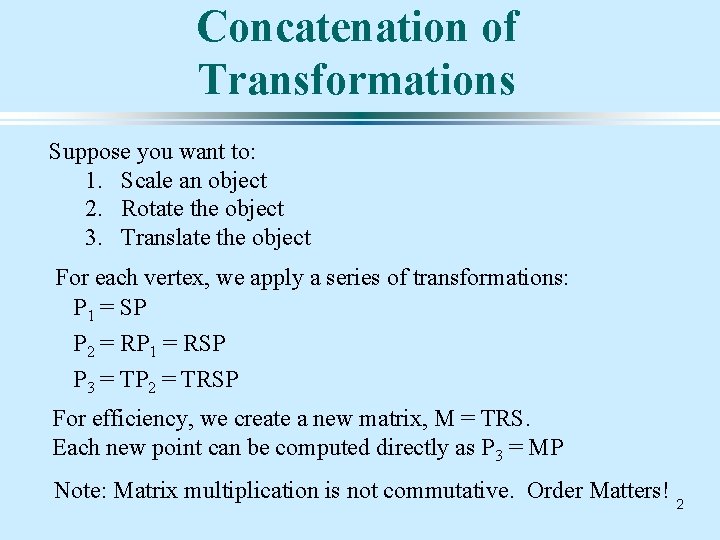
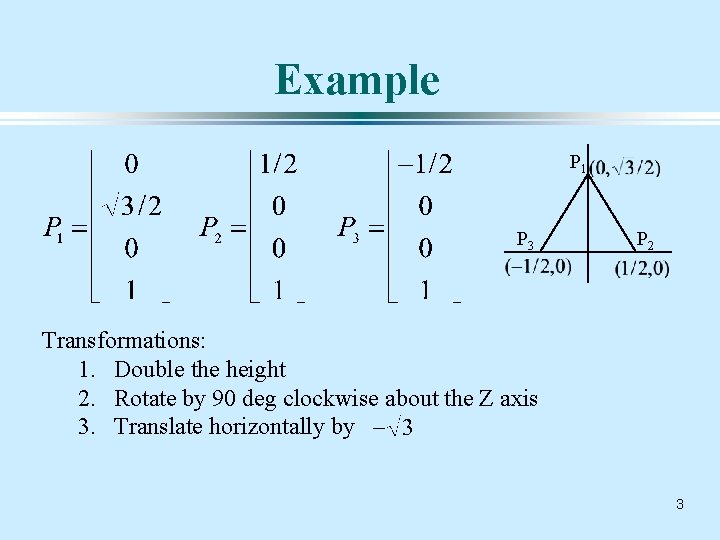
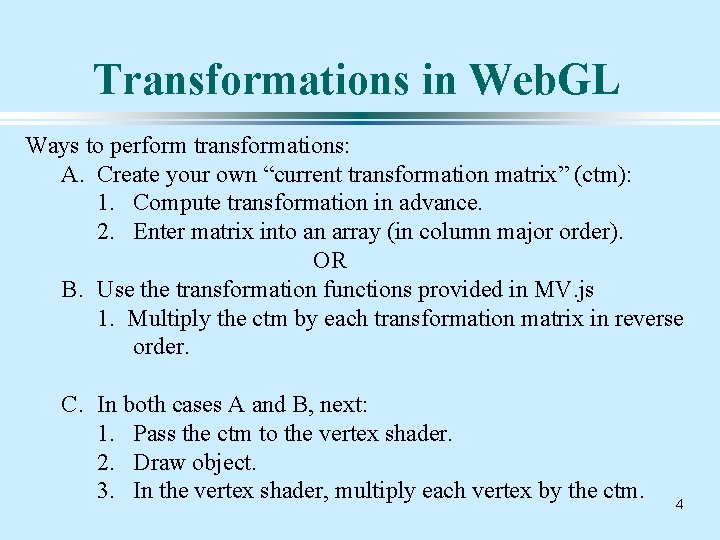
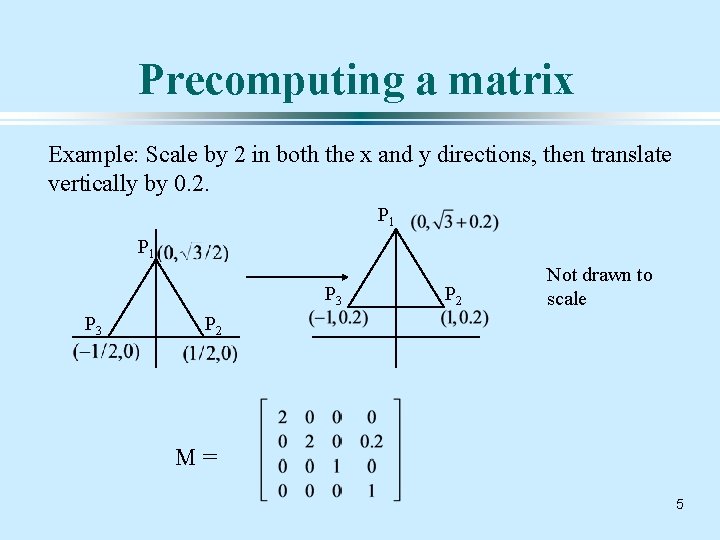
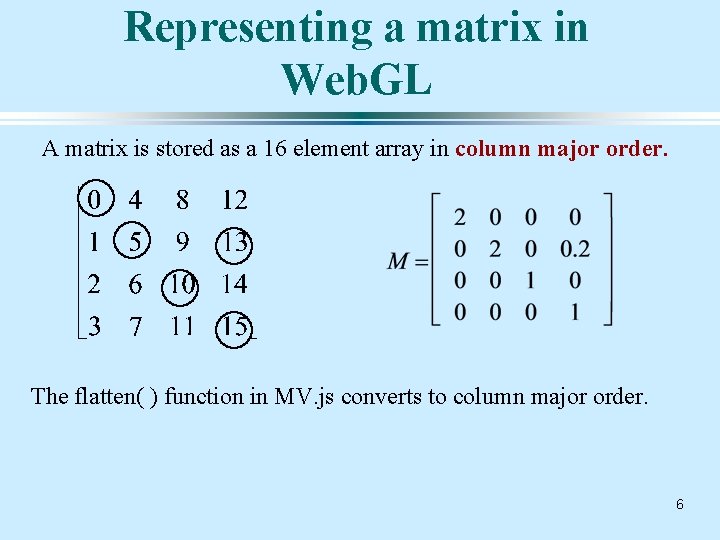
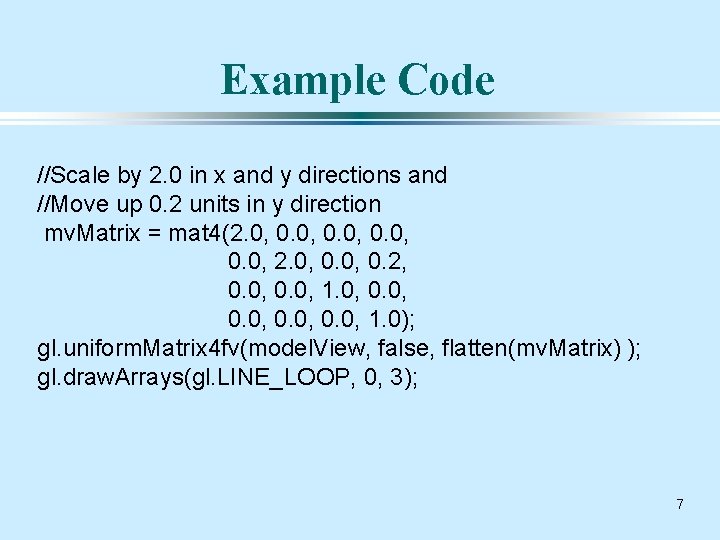
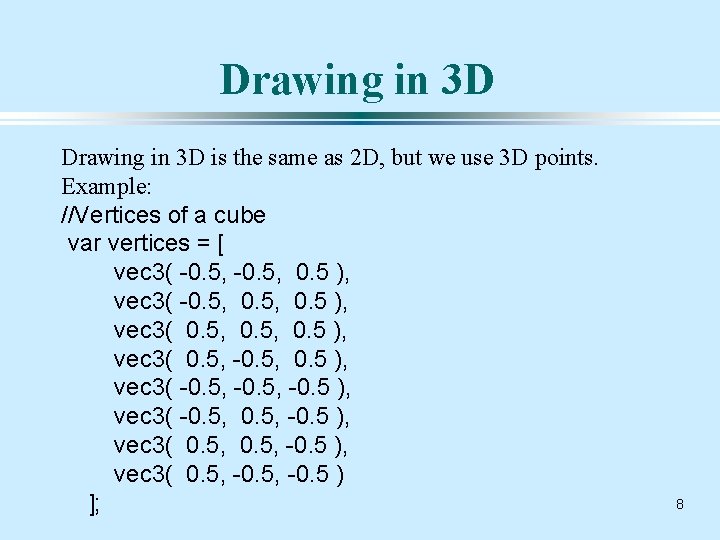
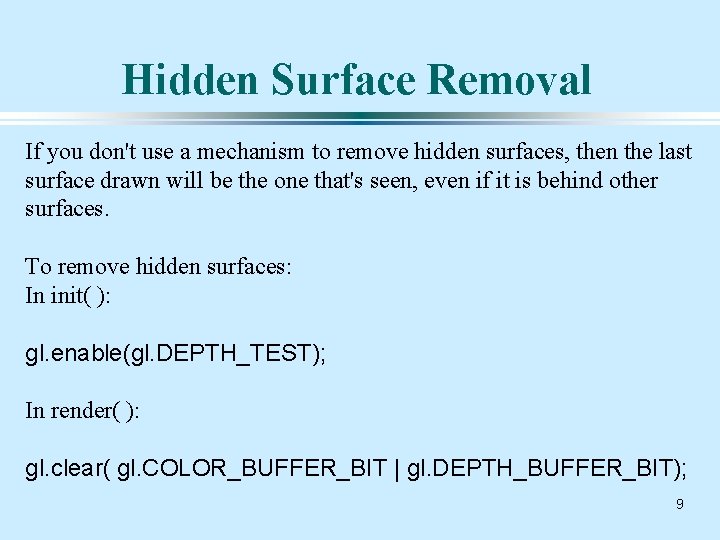
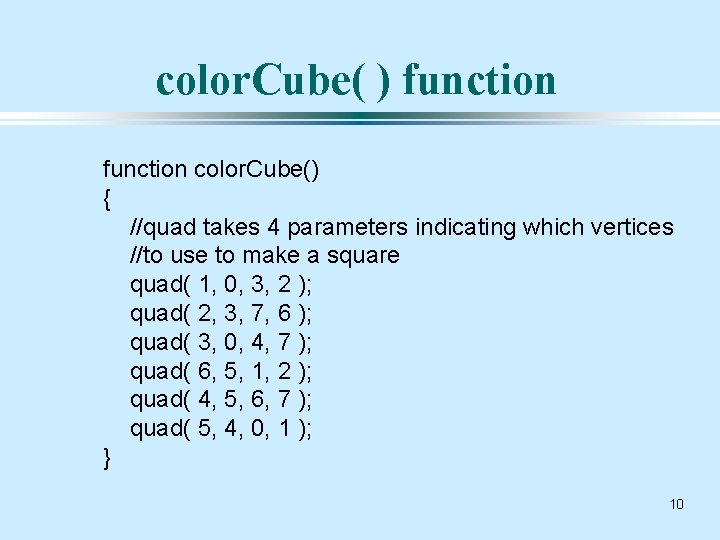
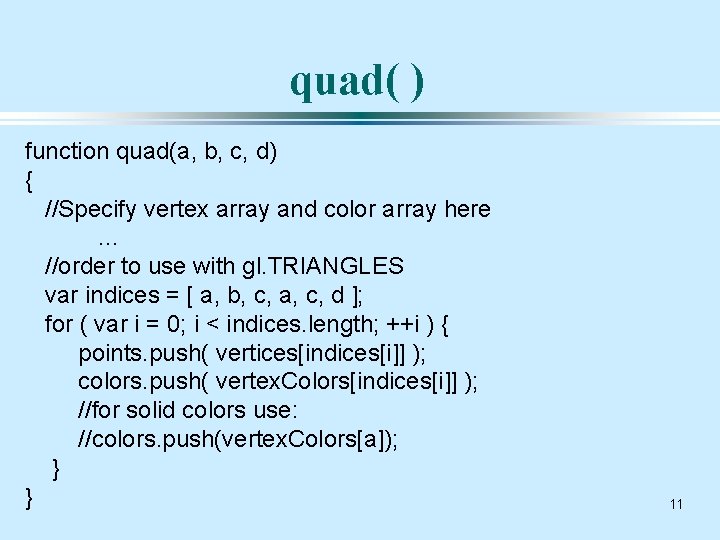
- Slides: 11
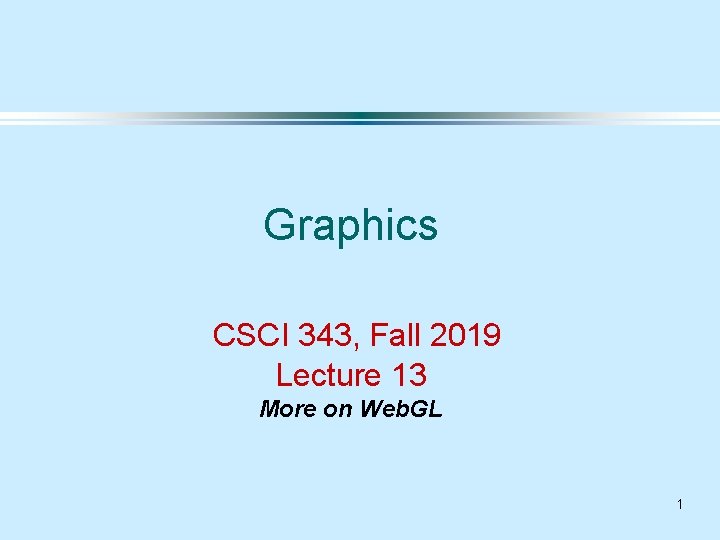
Graphics CSCI 343, Fall 2019 Lecture 13 More on Web. GL 1
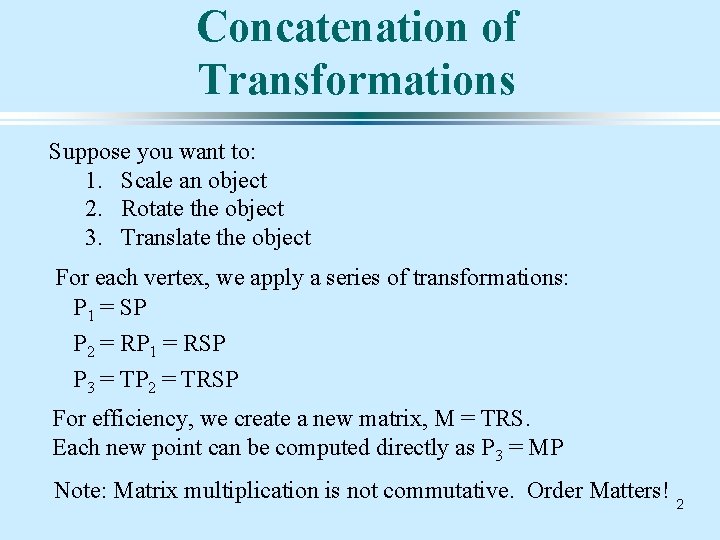
Concatenation of Transformations Suppose you want to: 1. Scale an object 2. Rotate the object 3. Translate the object For each vertex, we apply a series of transformations: P 1 = SP P 2 = RP 1 = RSP P 3 = TP 2 = TRSP For efficiency, we create a new matrix, M = TRS. Each new point can be computed directly as P 3 = MP Note: Matrix multiplication is not commutative. Order Matters! 2
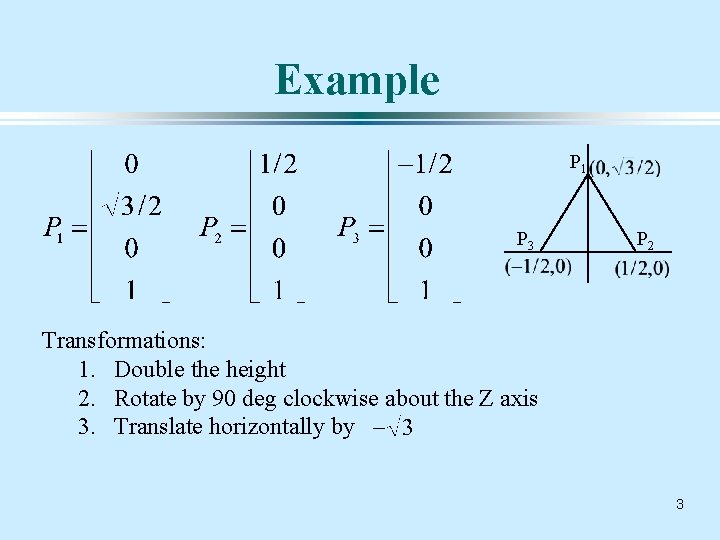
Example P 1 P 3 P 2 Transformations: 1. Double the height 2. Rotate by 90 deg clockwise about the Z axis 3. Translate horizontally by 3
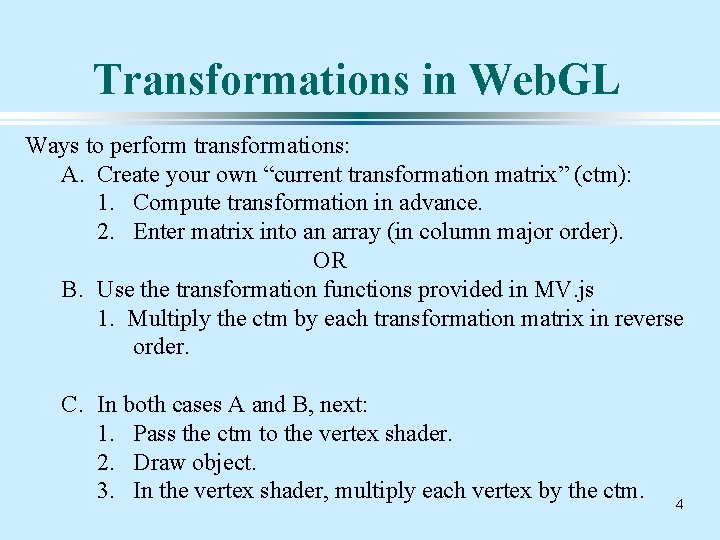
Transformations in Web. GL Ways to perform transformations: A. Create your own “current transformation matrix” (ctm): 1. Compute transformation in advance. 2. Enter matrix into an array (in column major order). OR B. Use the transformation functions provided in MV. js 1. Multiply the ctm by each transformation matrix in reverse order. C. In both cases A and B, next: 1. Pass the ctm to the vertex shader. 2. Draw object. 3. In the vertex shader, multiply each vertex by the ctm. 4
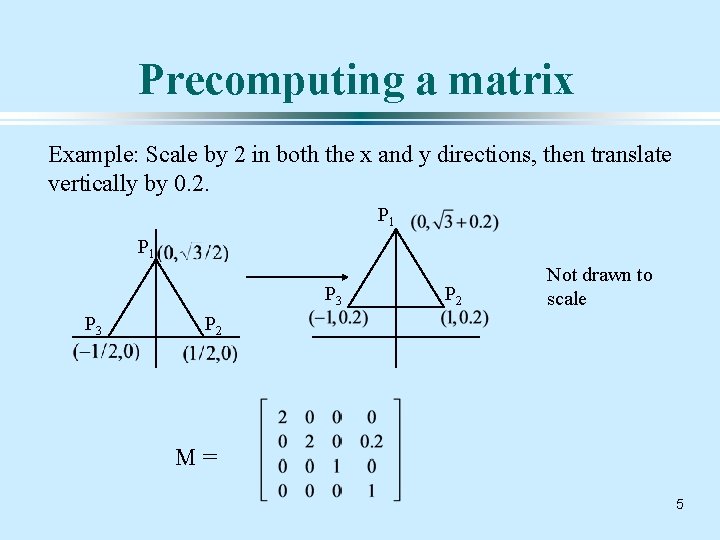
Precomputing a matrix Example: Scale by 2 in both the x and y directions, then translate vertically by 0. 2. P 1 P 3 P 2 Not drawn to scale P 2 M= 5
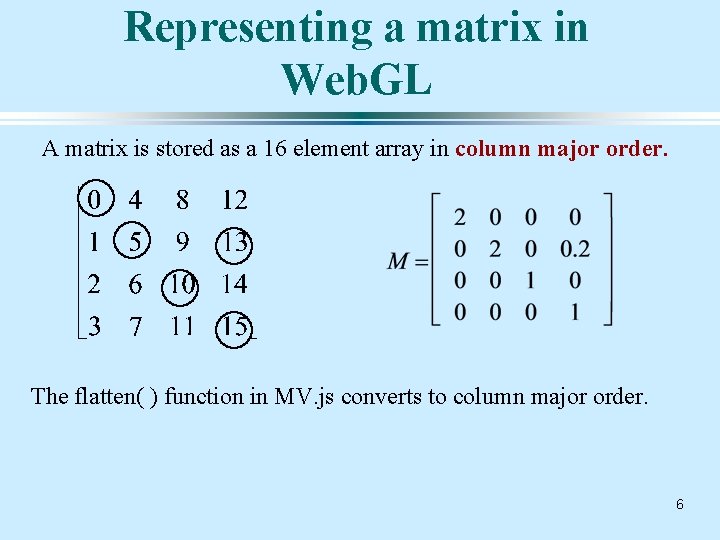
Representing a matrix in Web. GL A matrix is stored as a 16 element array in column major order. The flatten( ) function in MV. js converts to column major order. 6
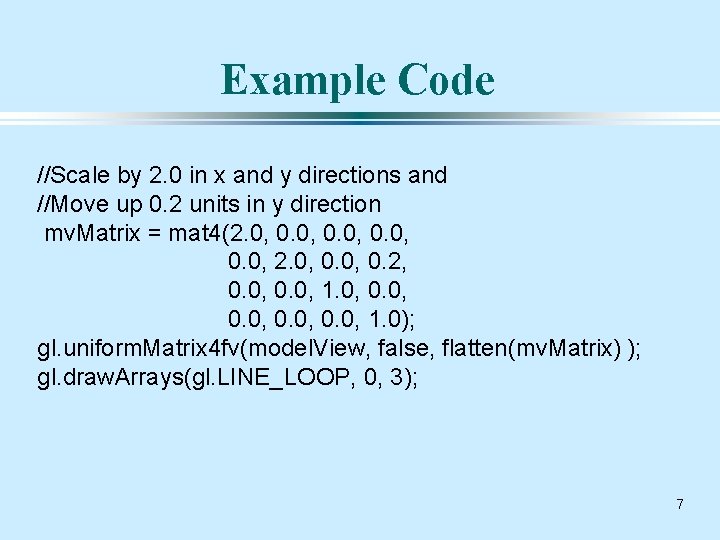
Example Code //Scale by 2. 0 in x and y directions and //Move up 0. 2 units in y direction mv. Matrix = mat 4(2. 0, 0. 0, 2. 0, 0. 2, 0. 0, 1. 0, 0. 0, 1. 0); gl. uniform. Matrix 4 fv(model. View, false, flatten(mv. Matrix) ); gl. draw. Arrays(gl. LINE_LOOP, 0, 3); 7
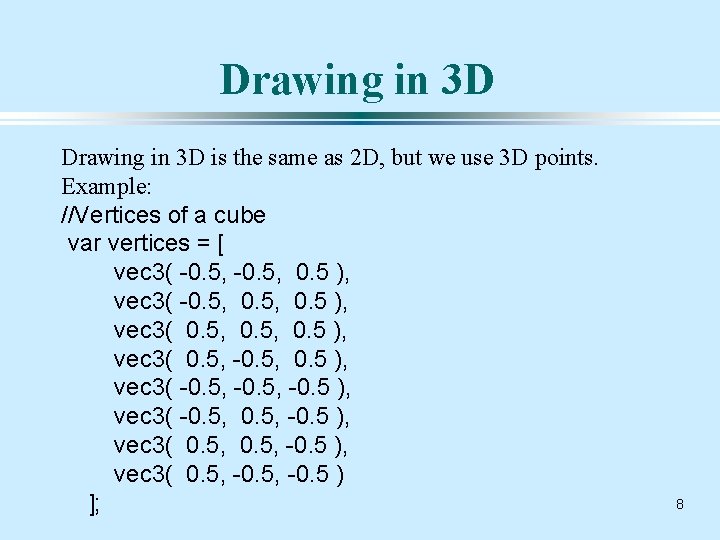
Drawing in 3 D is the same as 2 D, but we use 3 D points. Example: //Vertices of a cube var vertices = [ vec 3( -0. 5, 0. 5 ), vec 3( 0. 5, -0. 5, 0. 5 ), vec 3( -0. 5, -0. 5 ), vec 3( 0. 5, -0. 5 ) ]; 8
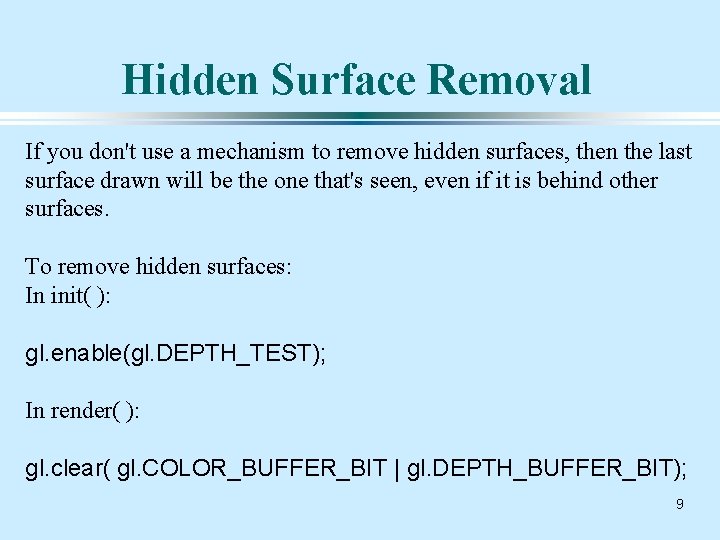
Hidden Surface Removal If you don't use a mechanism to remove hidden surfaces, then the last surface drawn will be the one that's seen, even if it is behind other surfaces. To remove hidden surfaces: In init( ): gl. enable(gl. DEPTH_TEST); In render( ): gl. clear( gl. COLOR_BUFFER_BIT | gl. DEPTH_BUFFER_BIT); 9
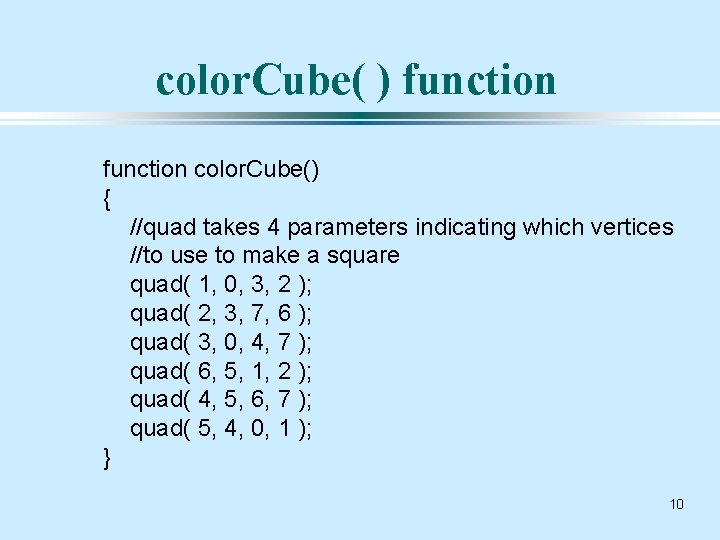
color. Cube( ) function color. Cube() { //quad takes 4 parameters indicating which vertices //to use to make a square quad( 1, 0, 3, 2 ); quad( 2, 3, 7, 6 ); quad( 3, 0, 4, 7 ); quad( 6, 5, 1, 2 ); quad( 4, 5, 6, 7 ); quad( 5, 4, 0, 1 ); } 10
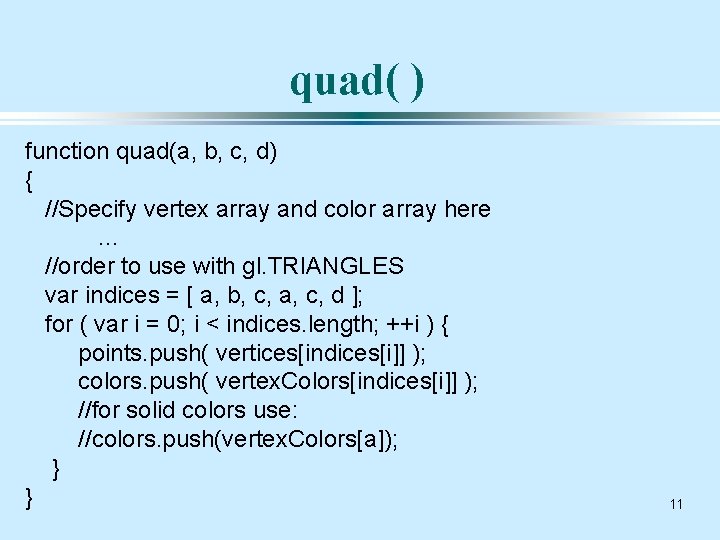
quad( ) function quad(a, b, c, d) { //Specify vertex array and color array here … //order to use with gl. TRIANGLES var indices = [ a, b, c, a, c, d ]; for ( var i = 0; i < indices. length; ++i ) { points. push( vertices[indices[i]] ); colors. push( vertex. Colors[indices[i]] ); //for solid colors use: //colors. push(vertex. Colors[a]); } } 11