Functions Important concept in programming languages function represents
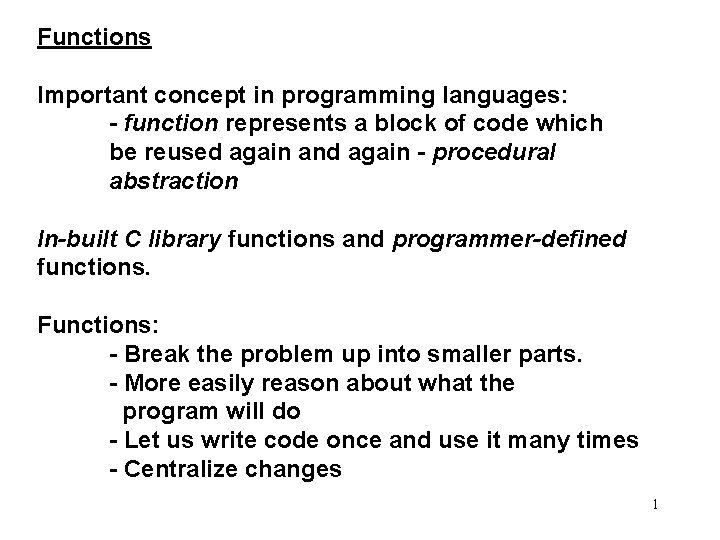
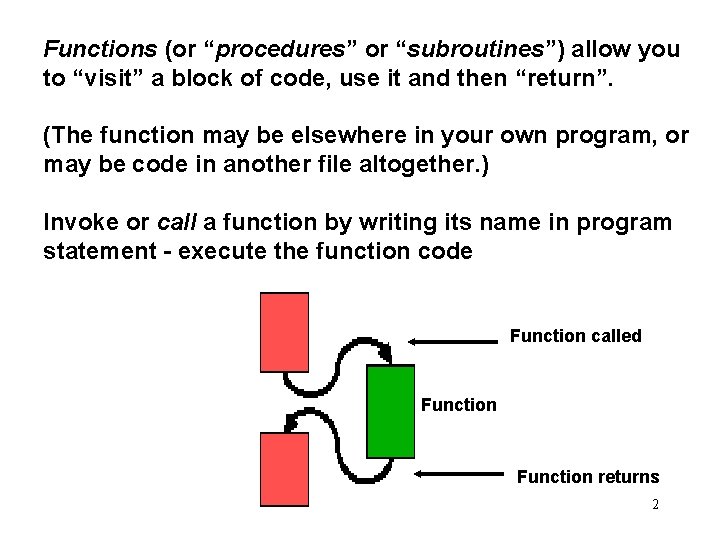
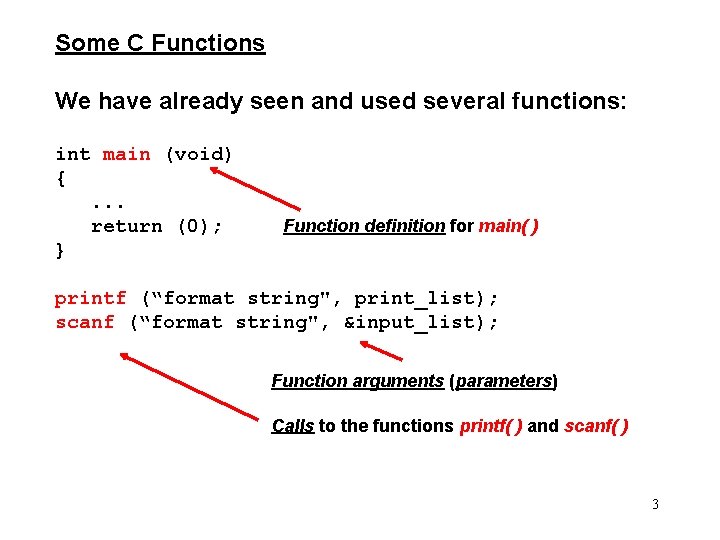
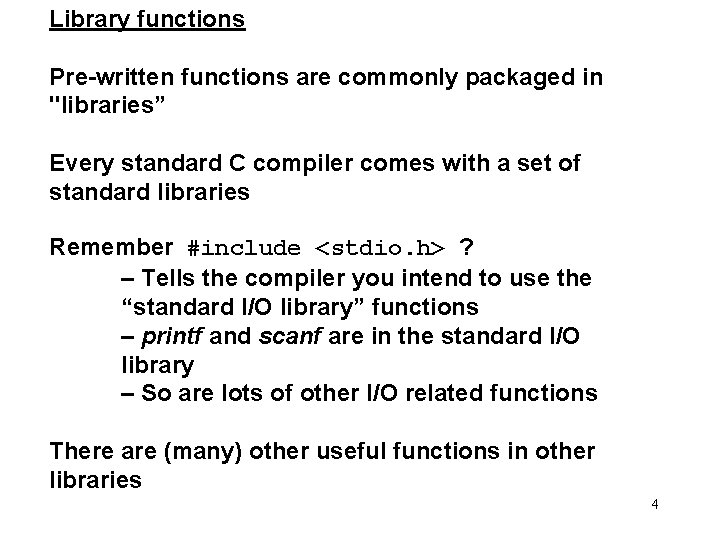
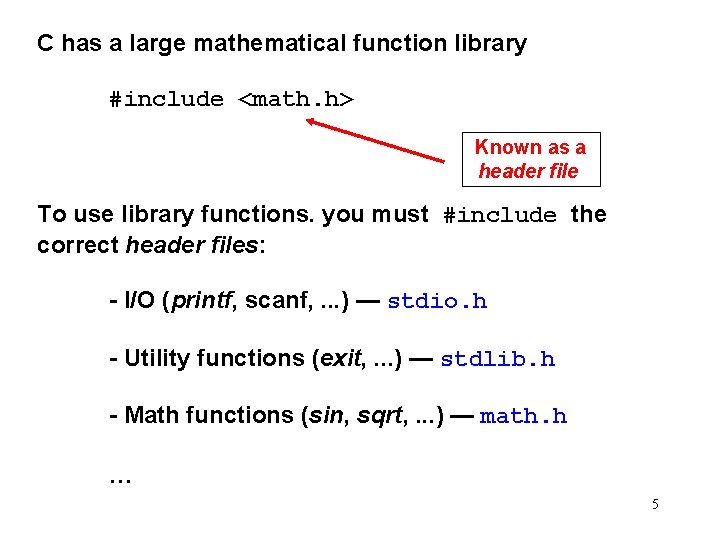
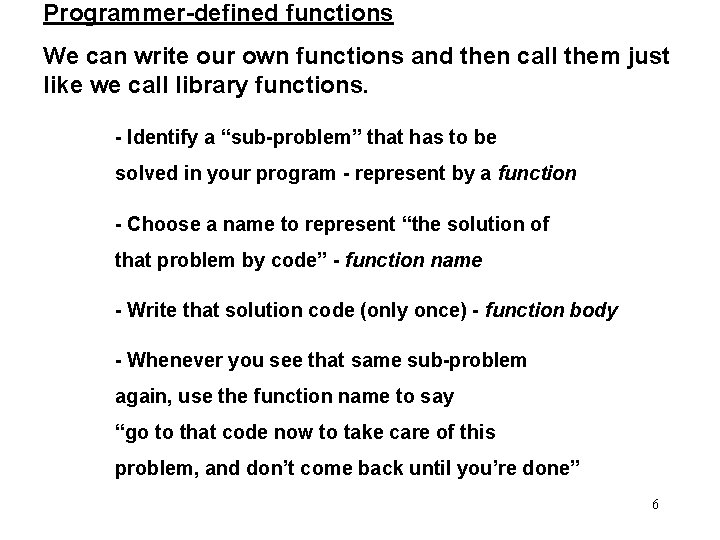
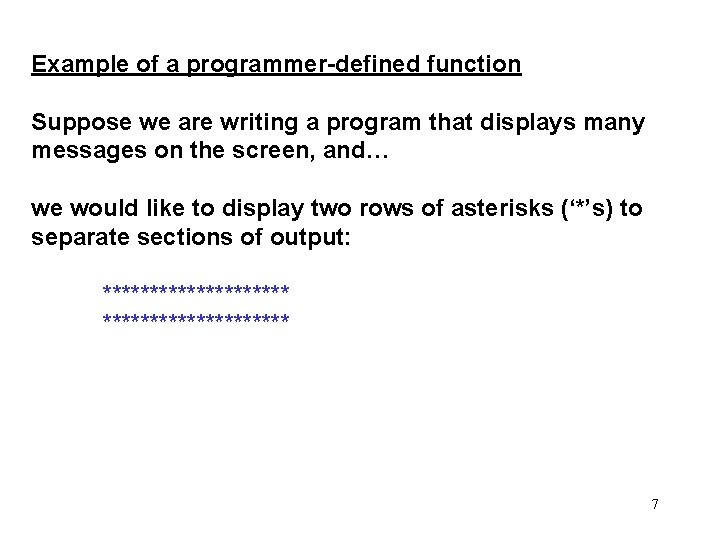
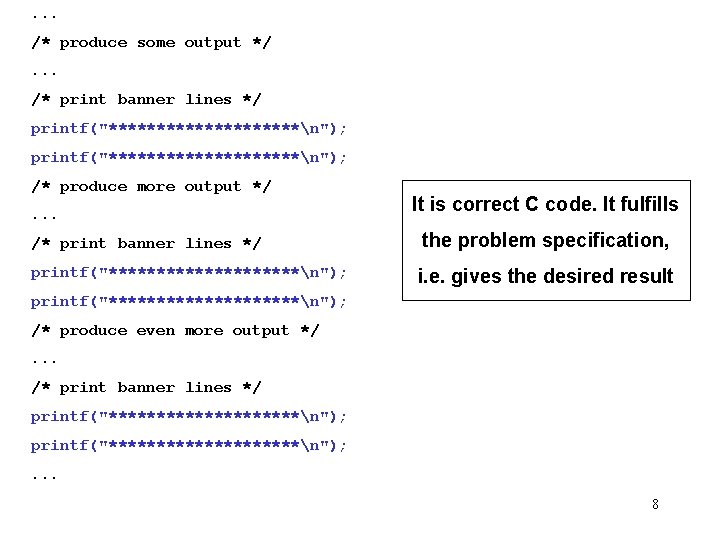
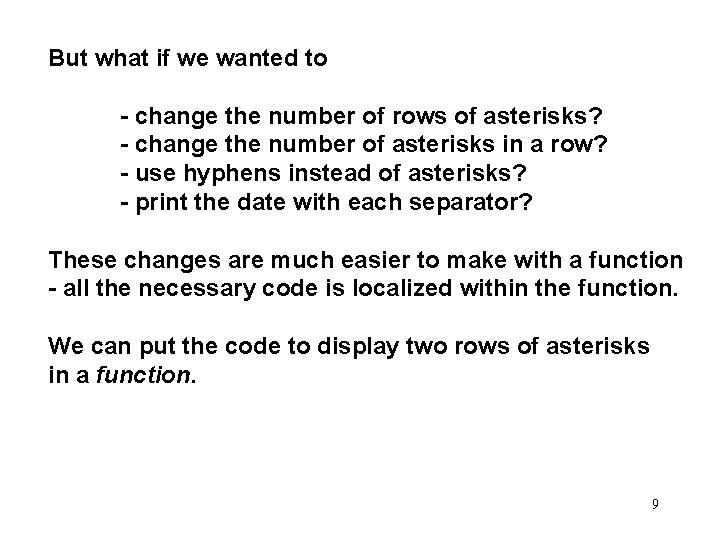
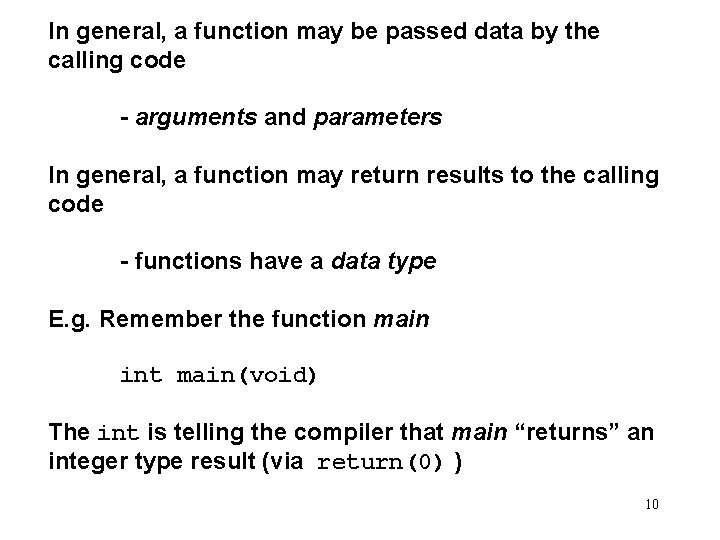
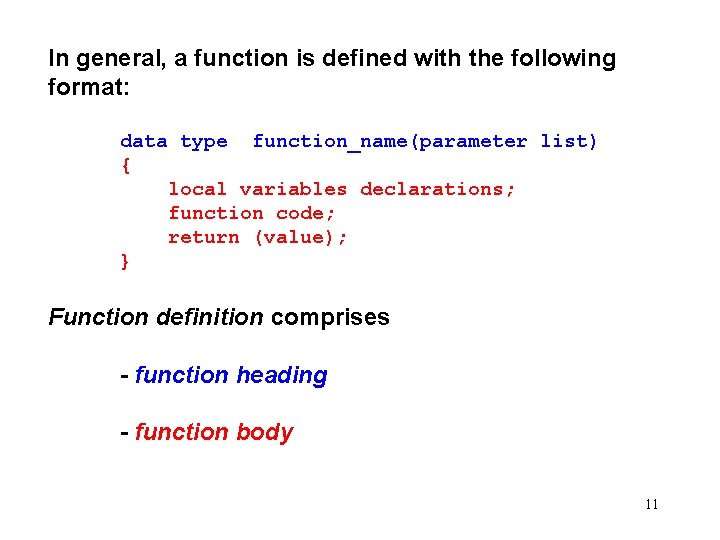
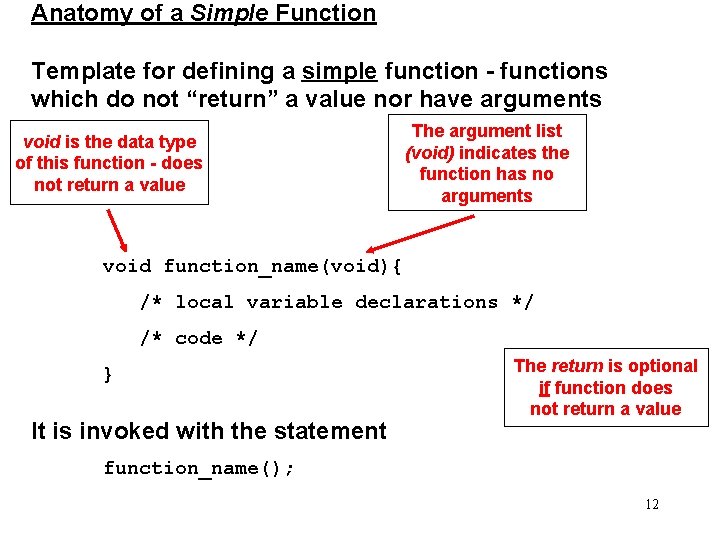
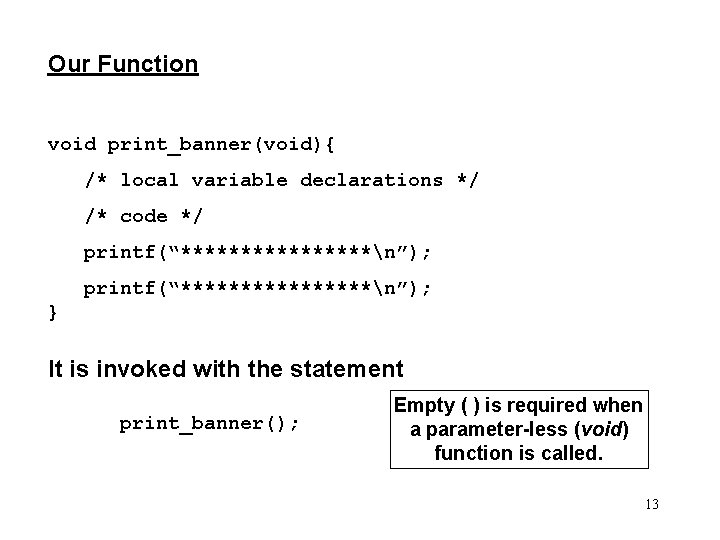
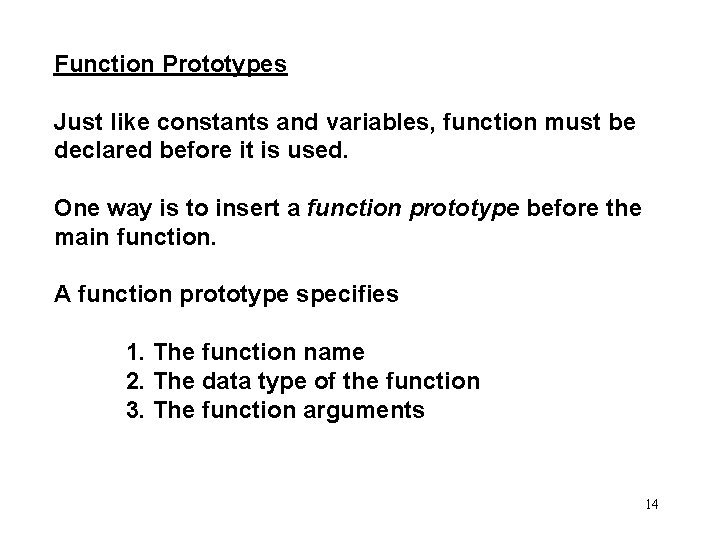
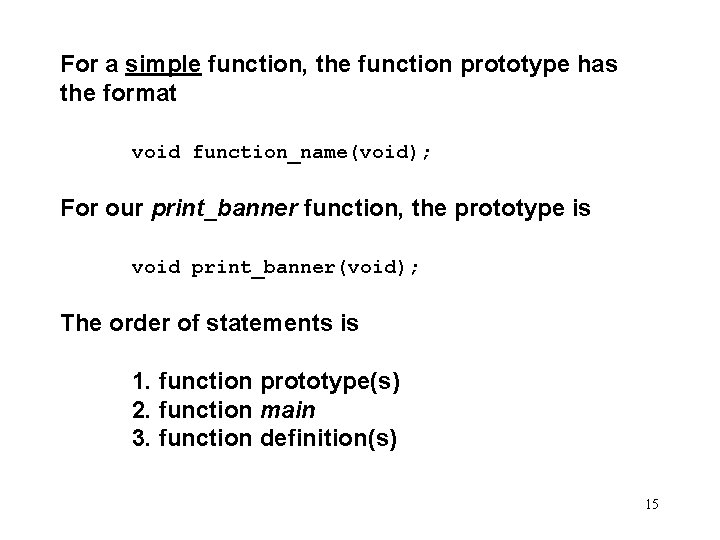
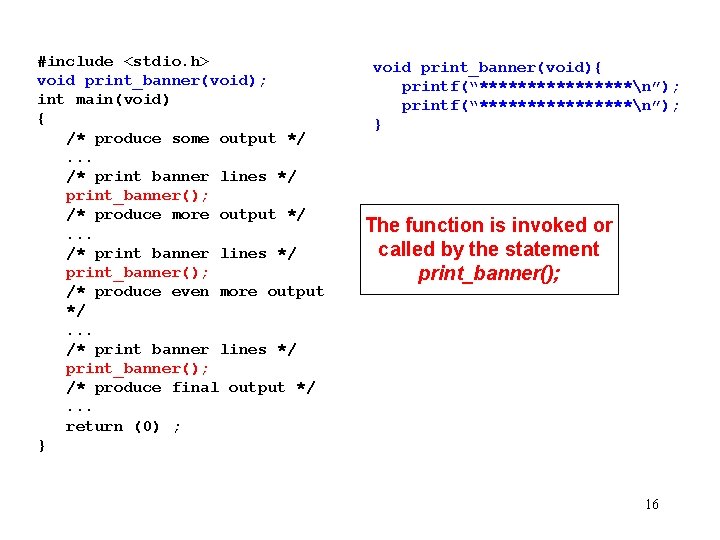
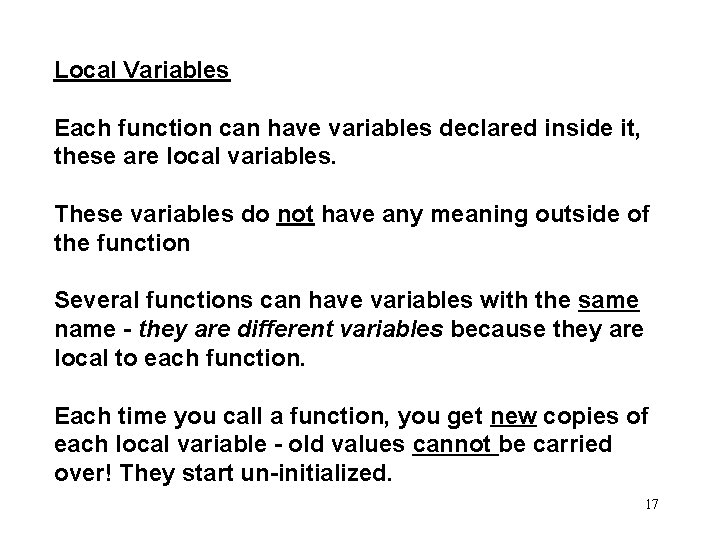
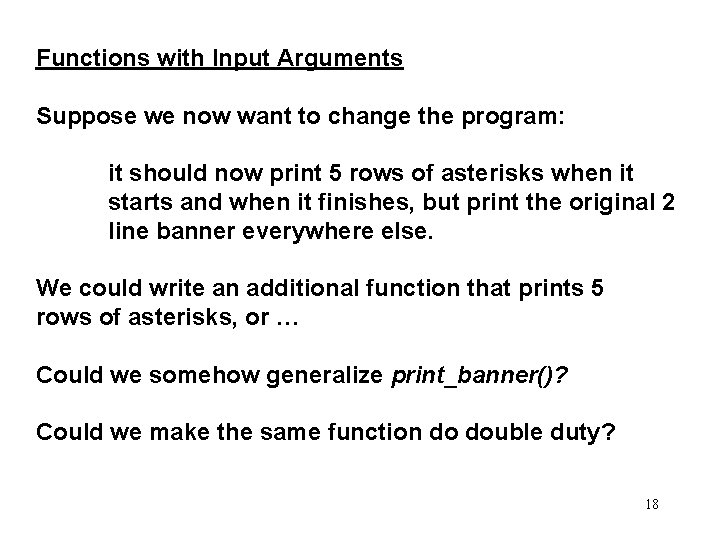
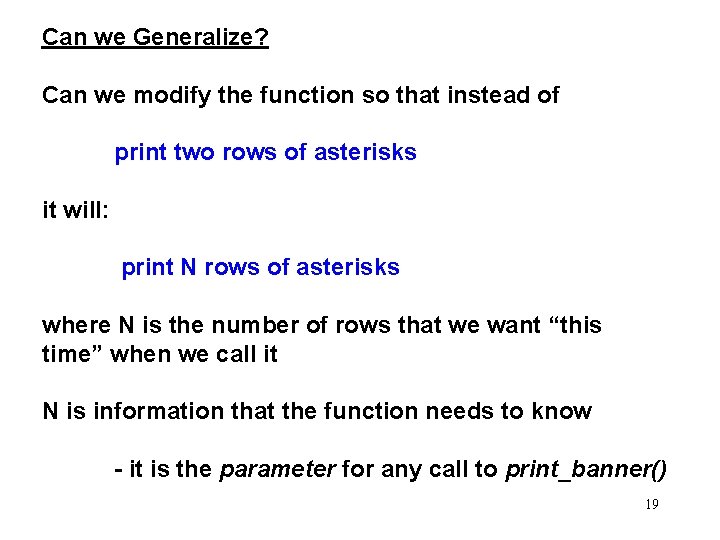
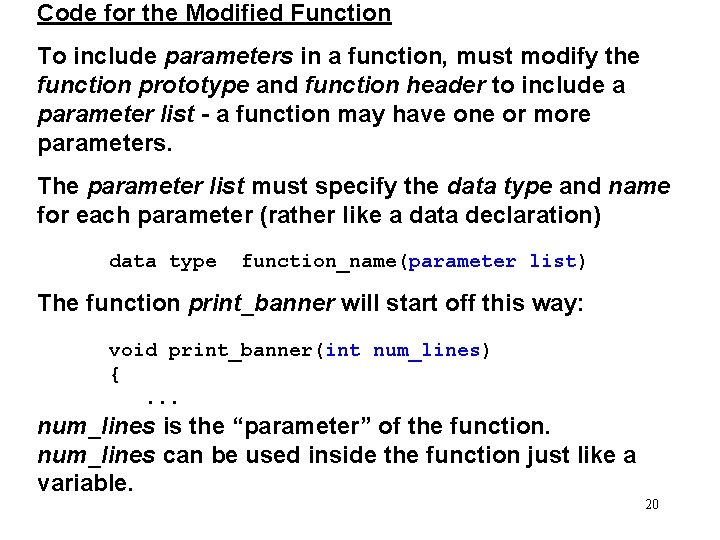
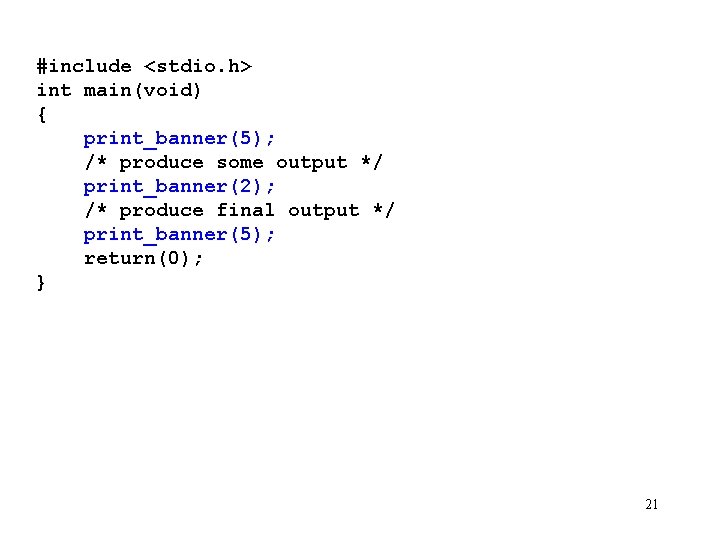
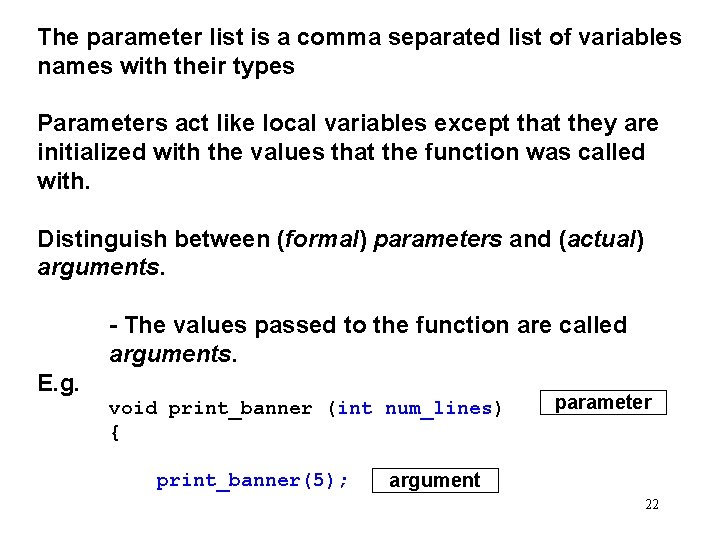
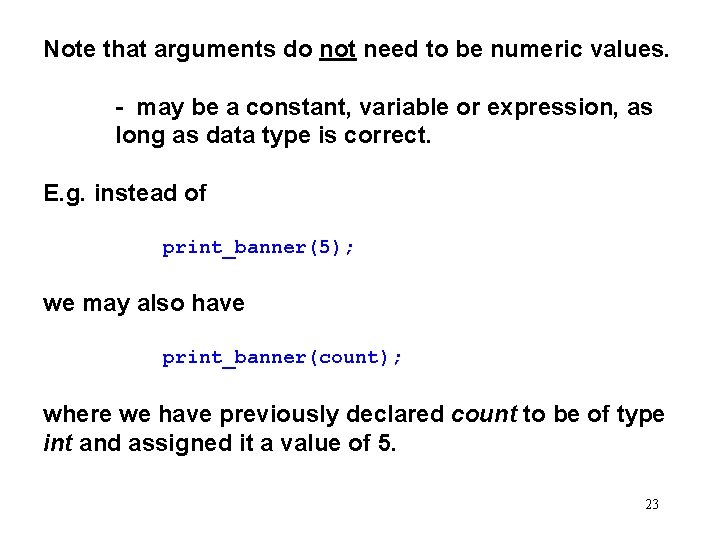
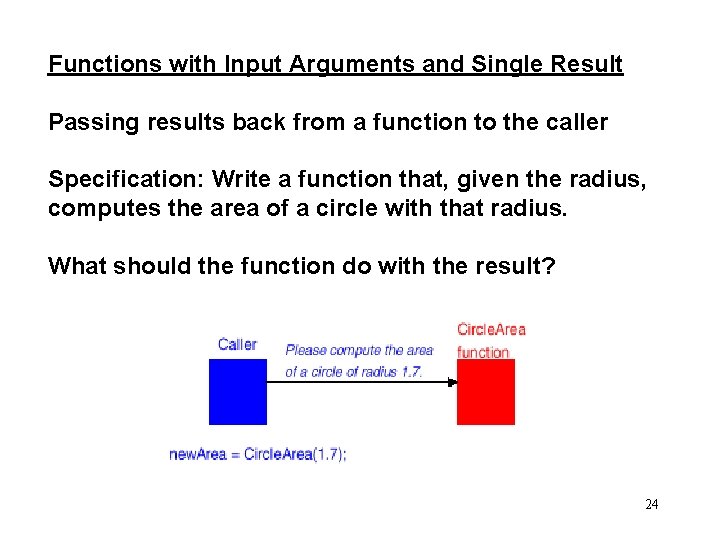
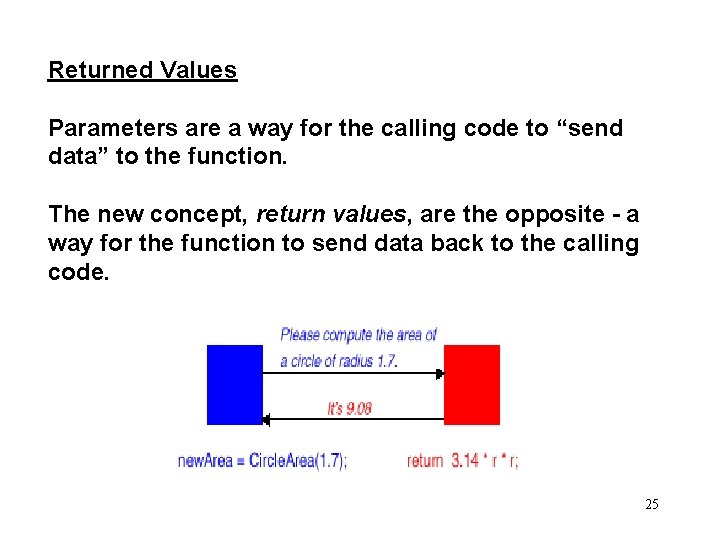
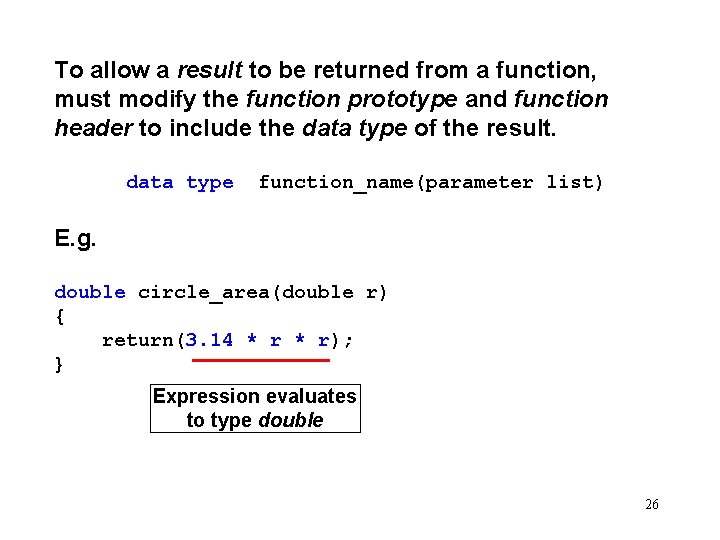
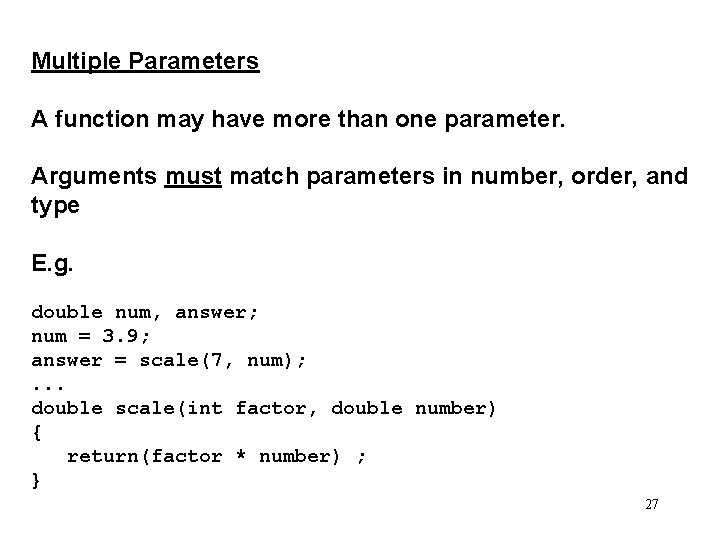
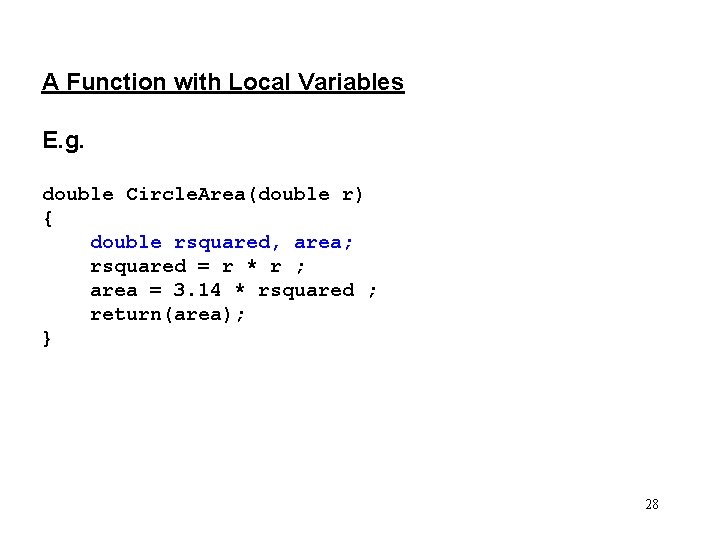
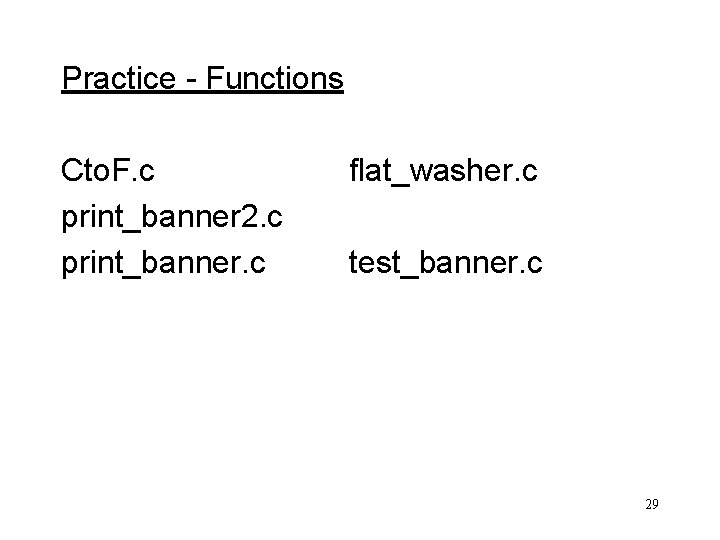
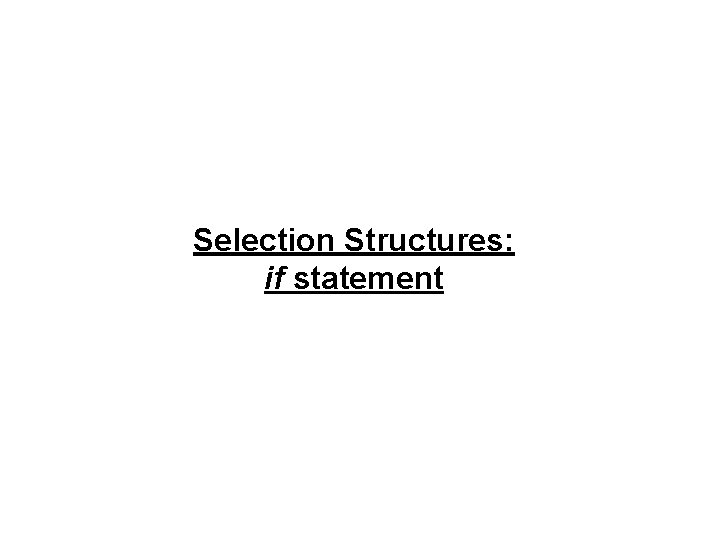
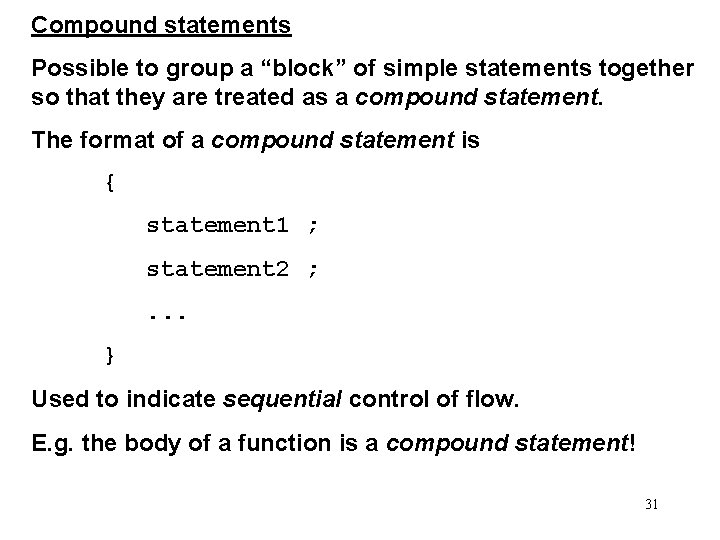
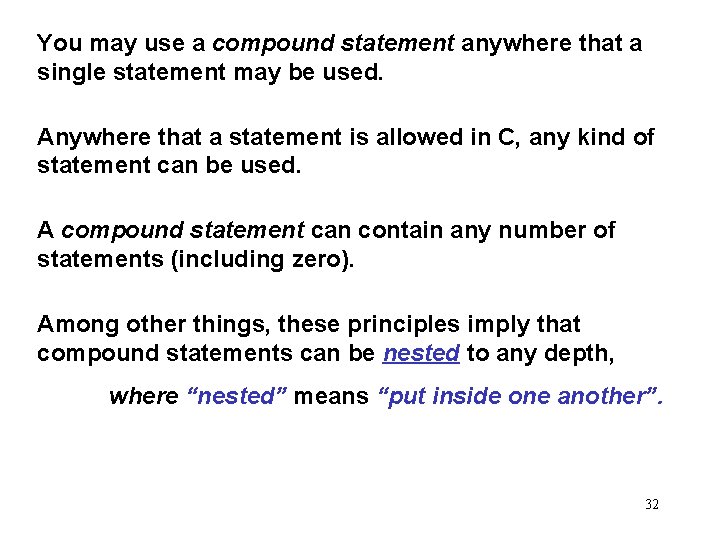
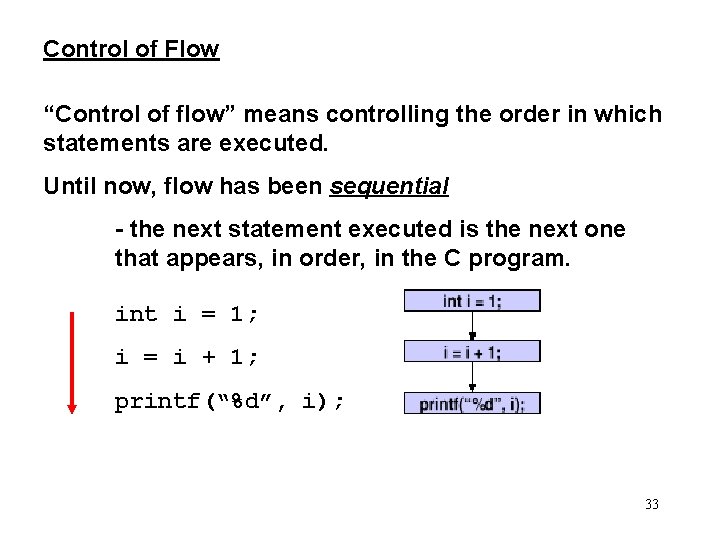
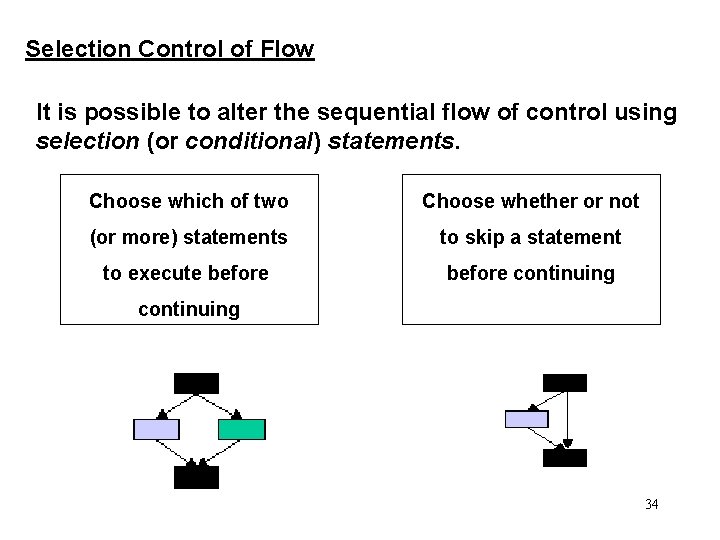
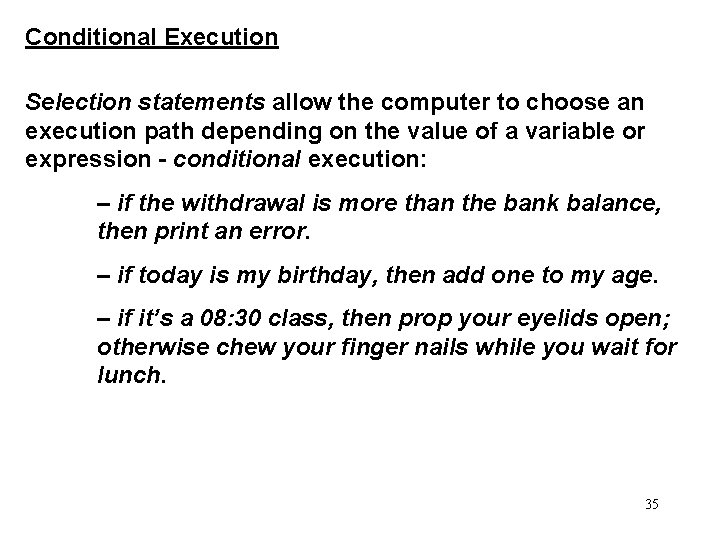
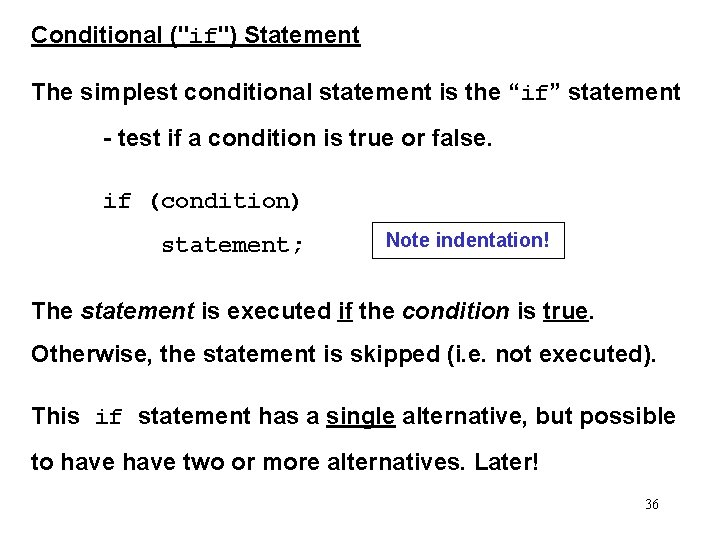
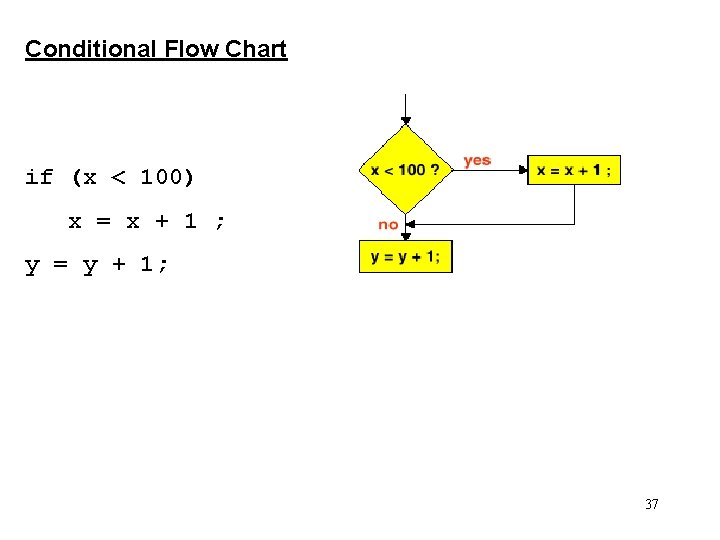
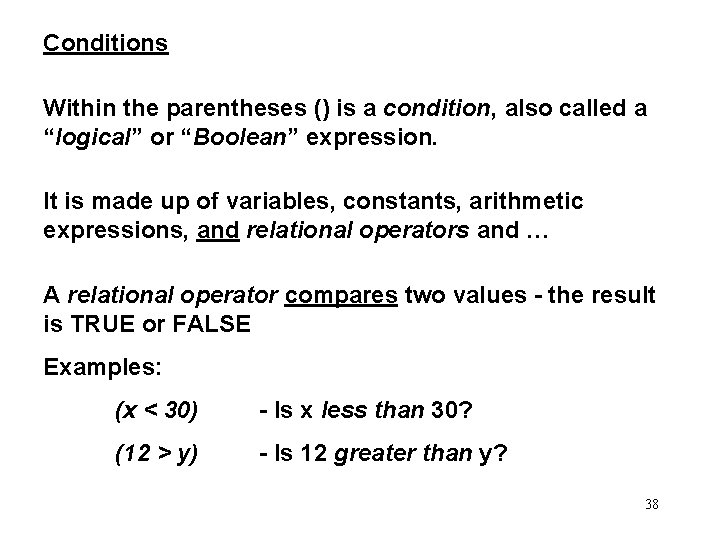
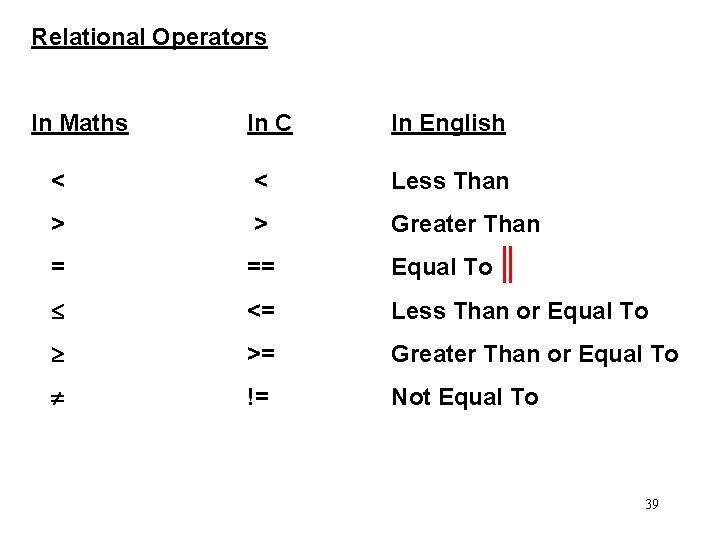
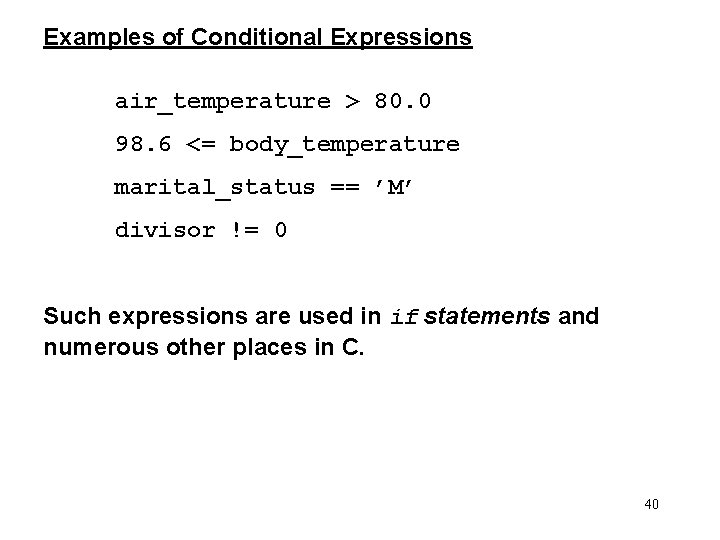
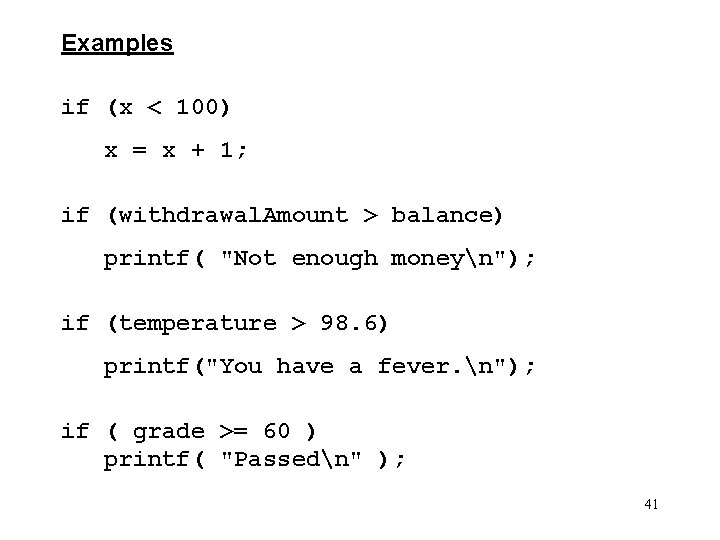
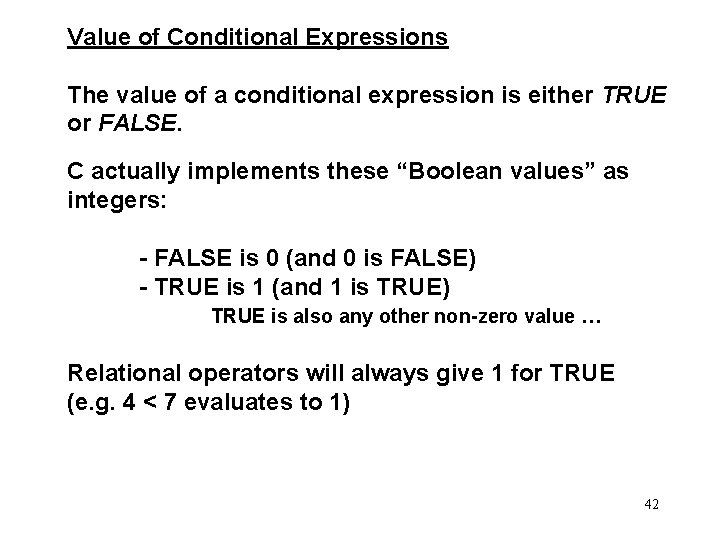
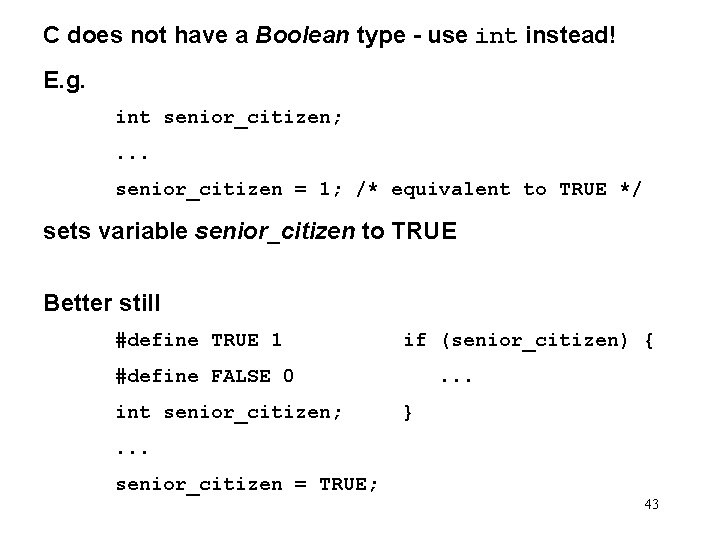
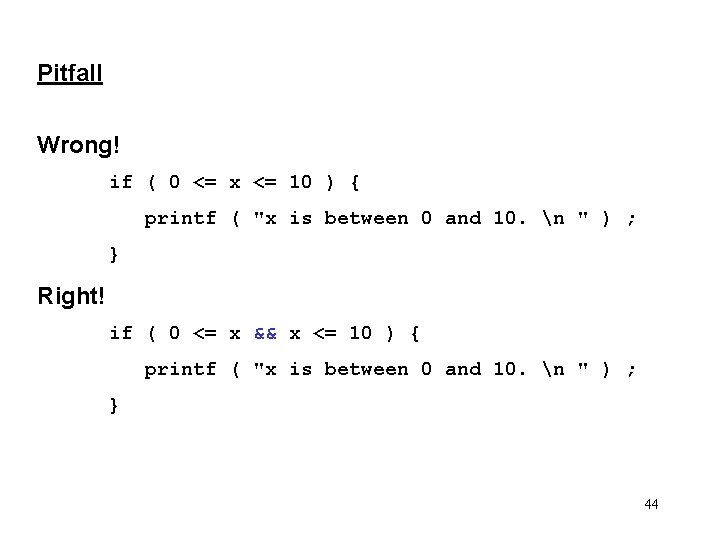
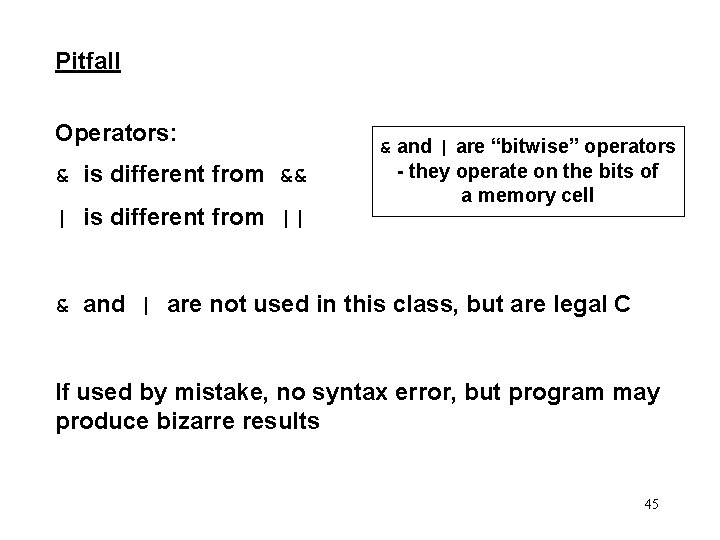
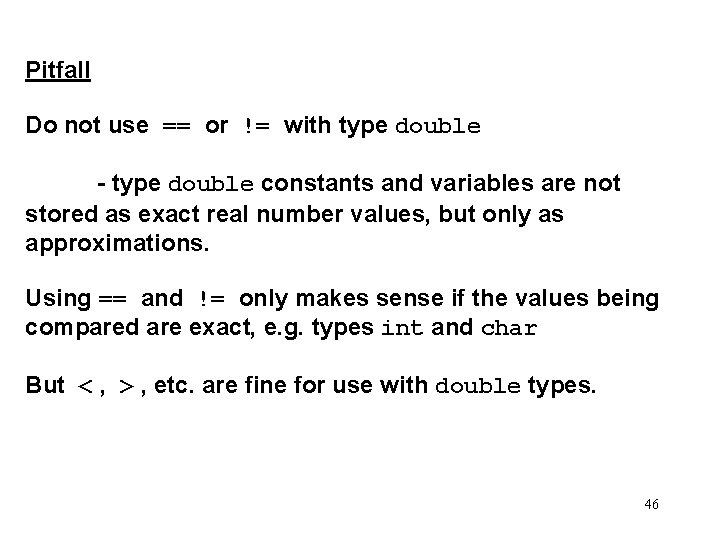
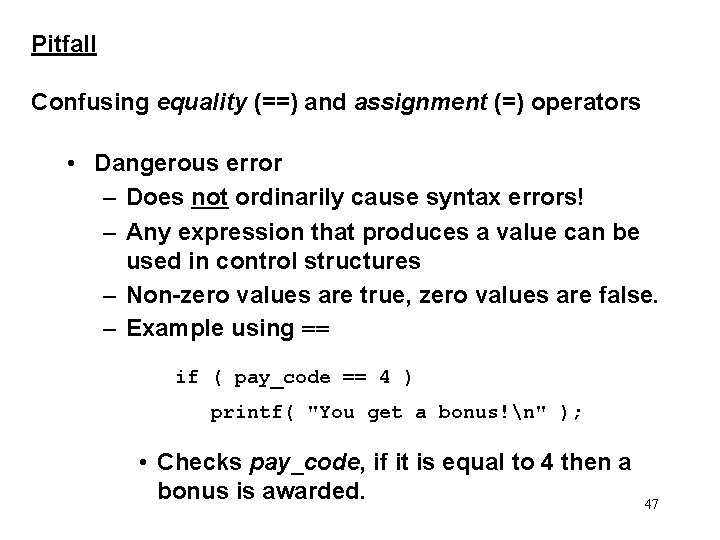
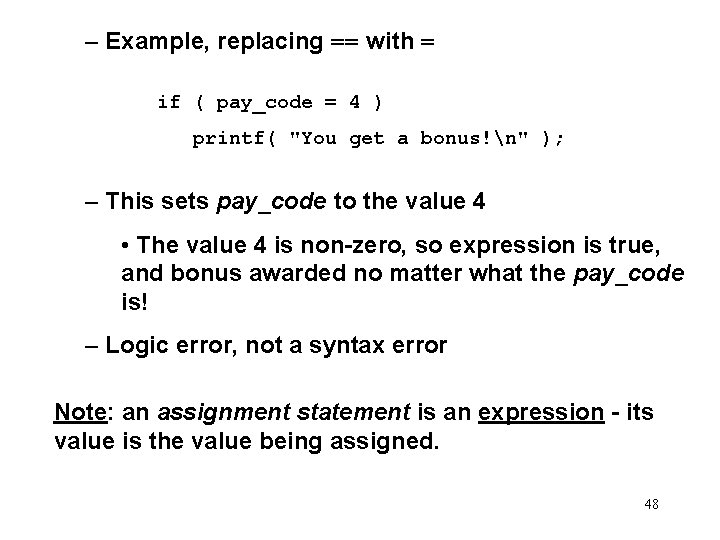
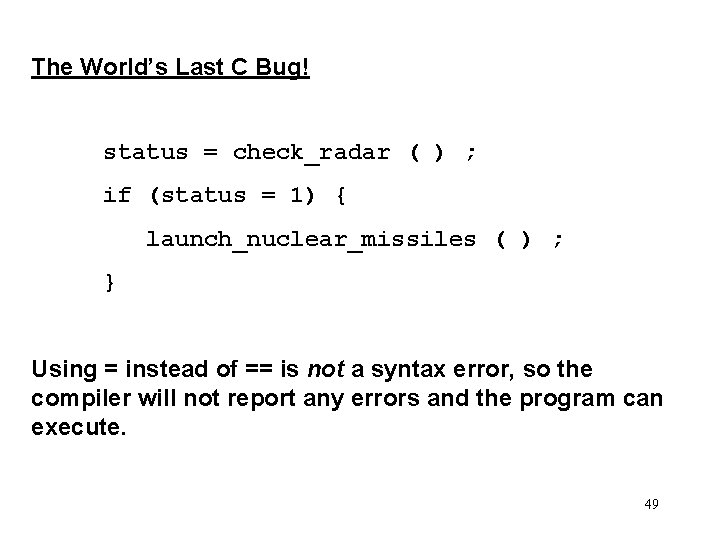
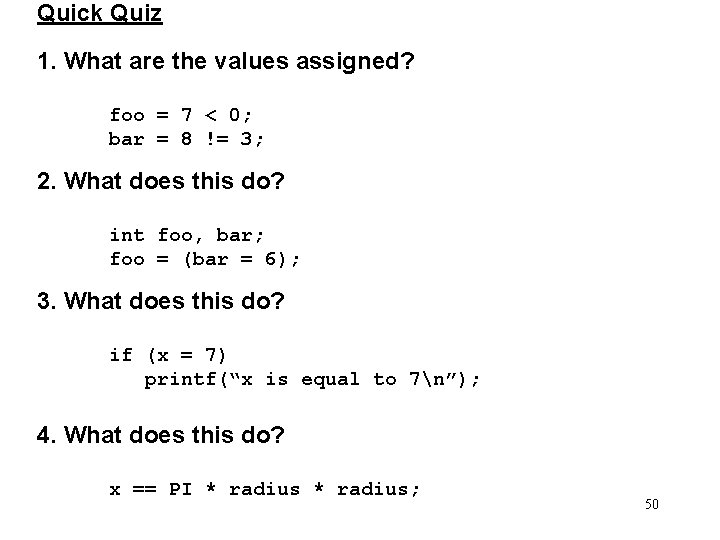
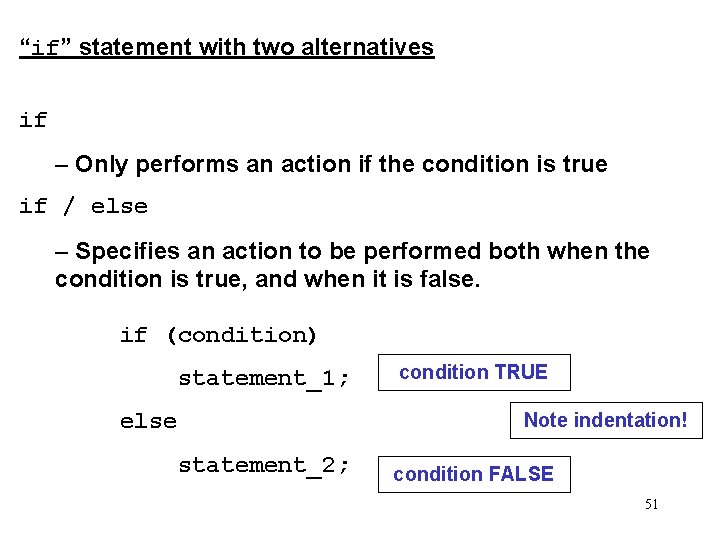
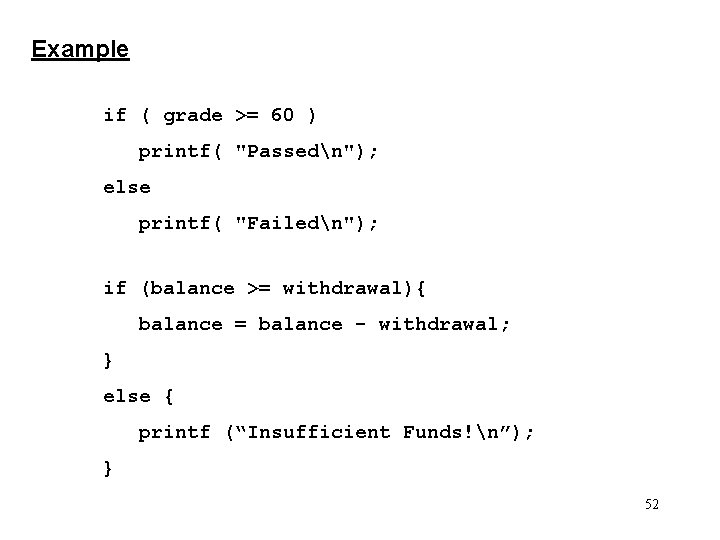
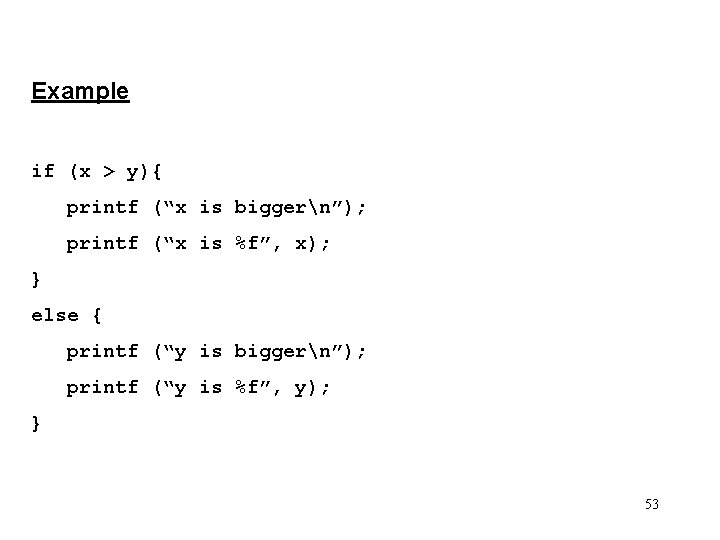
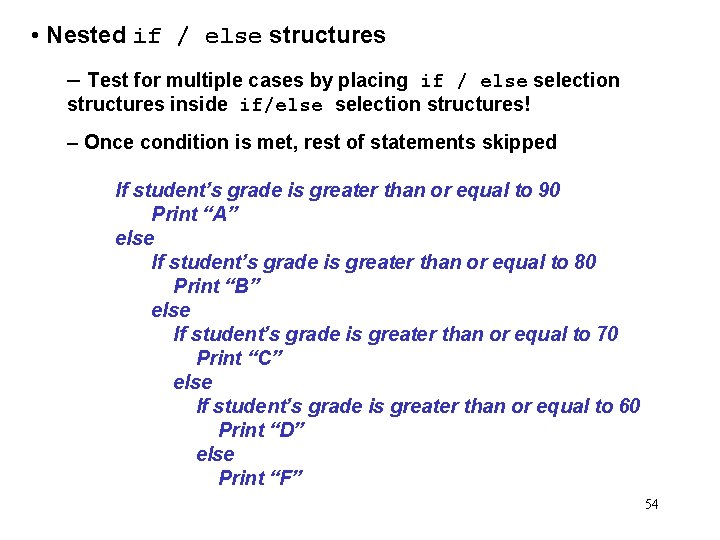
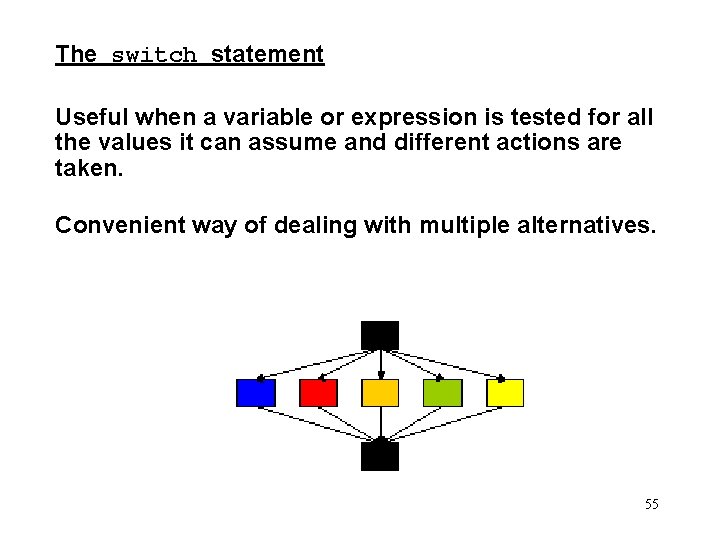
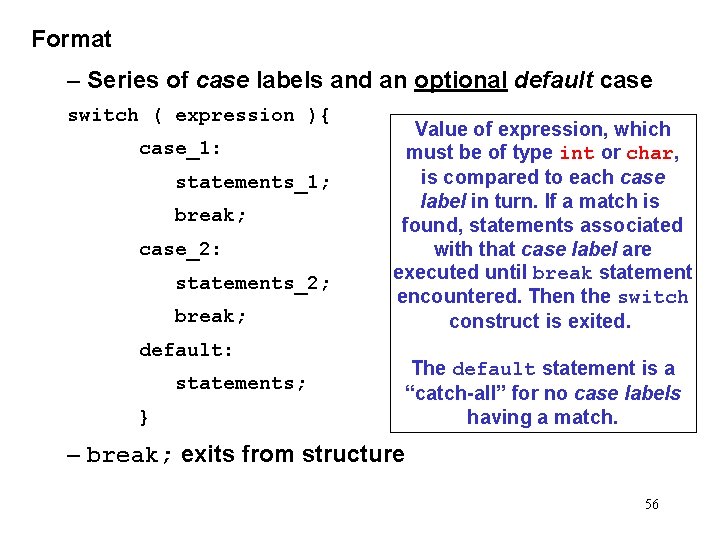
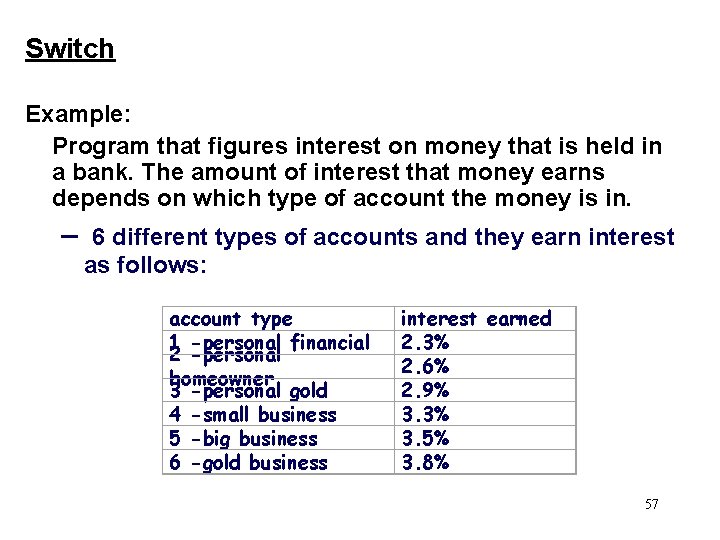
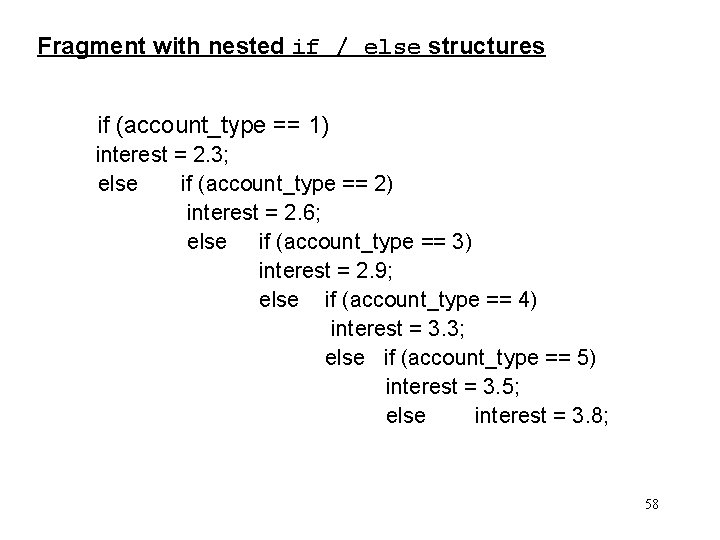
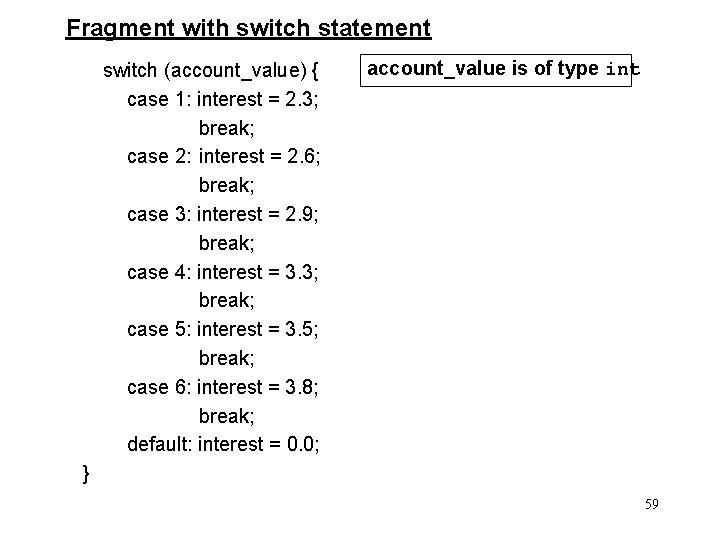
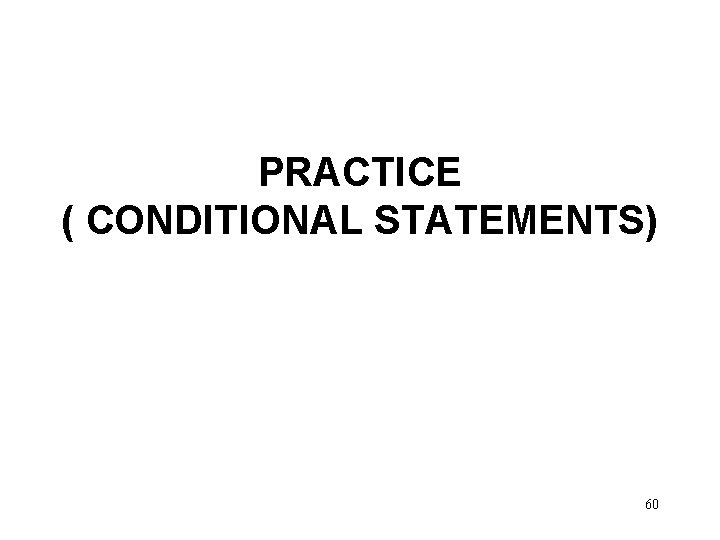
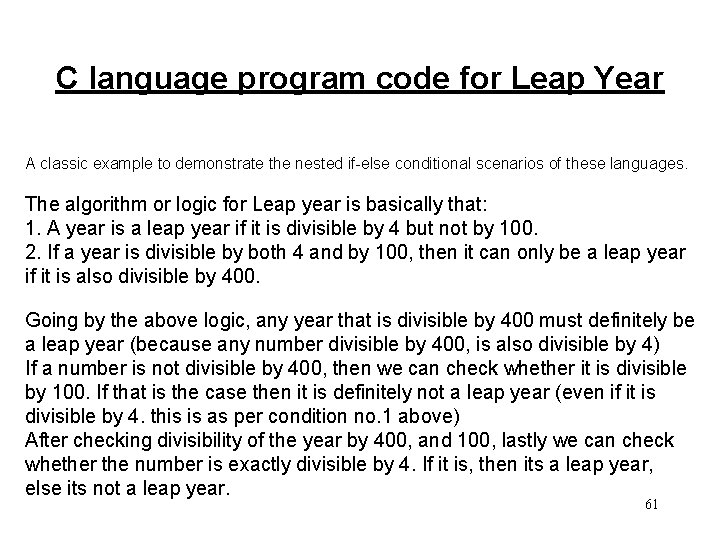
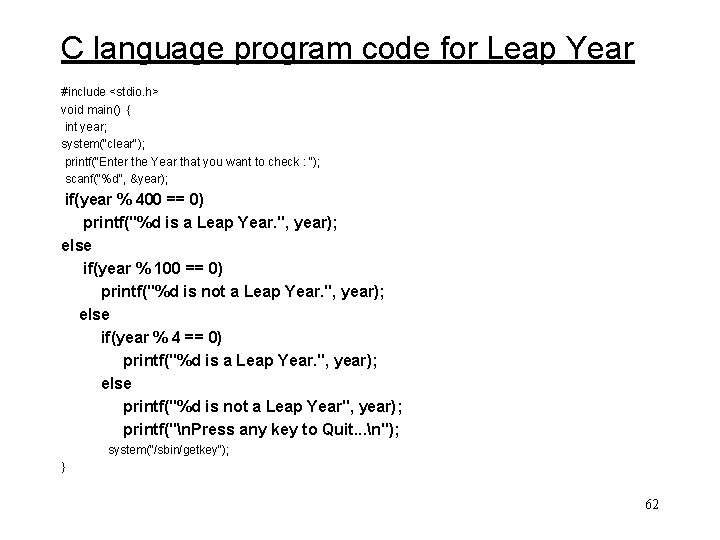
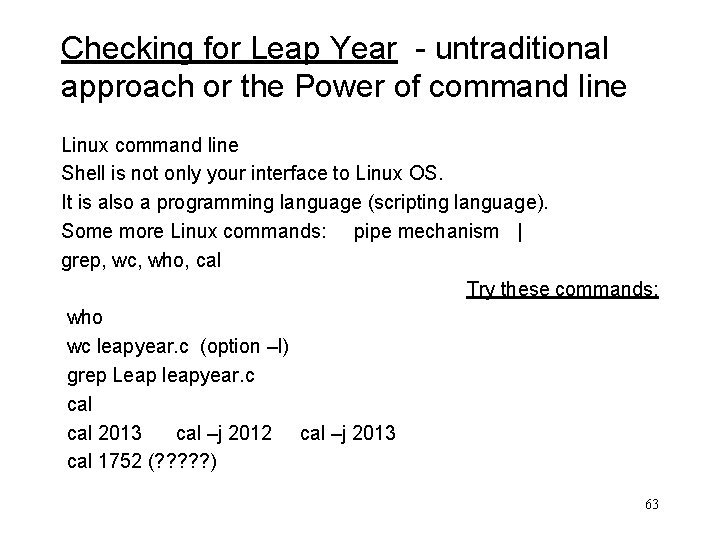
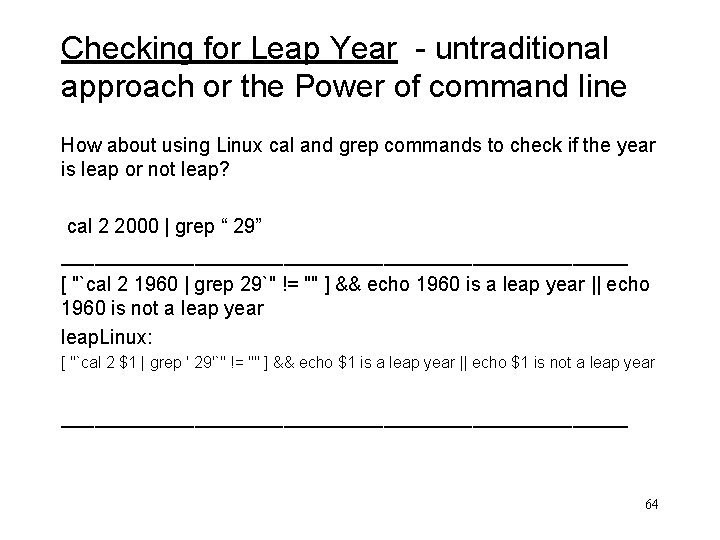
- Slides: 64
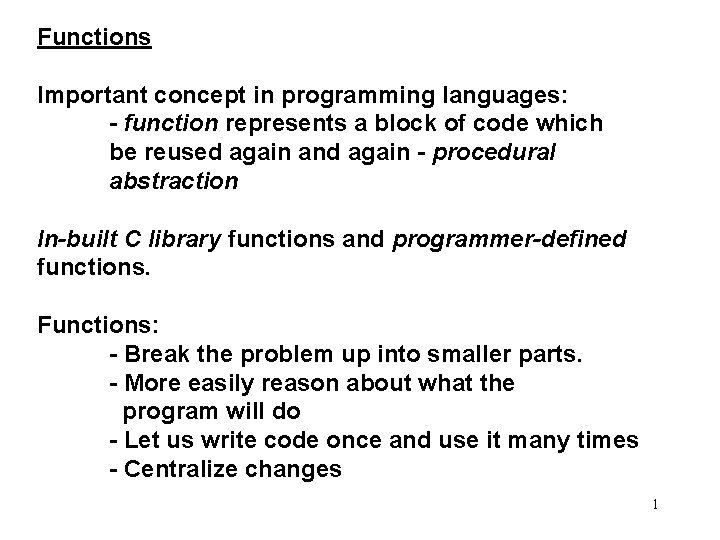
Functions Important concept in programming languages: - function represents a block of code which be reused again and again - procedural abstraction In-built C library functions and programmer-defined functions. Functions: - Break the problem up into smaller parts. - More easily reason about what the program will do - Let us write code once and use it many times - Centralize changes 1
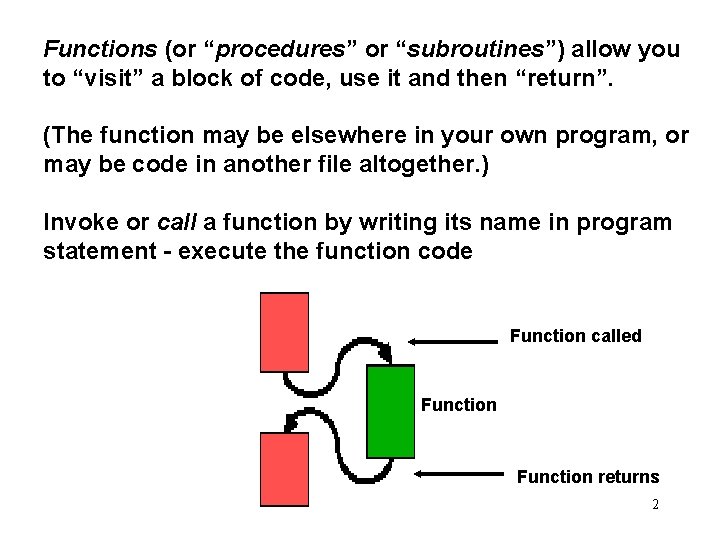
Functions (or “procedures” or “subroutines”) allow you to “visit” a block of code, use it and then “return”. (The function may be elsewhere in your own program, or may be code in another file altogether. ) Invoke or call a function by writing its name in program statement - execute the function code Function called Function returns 2
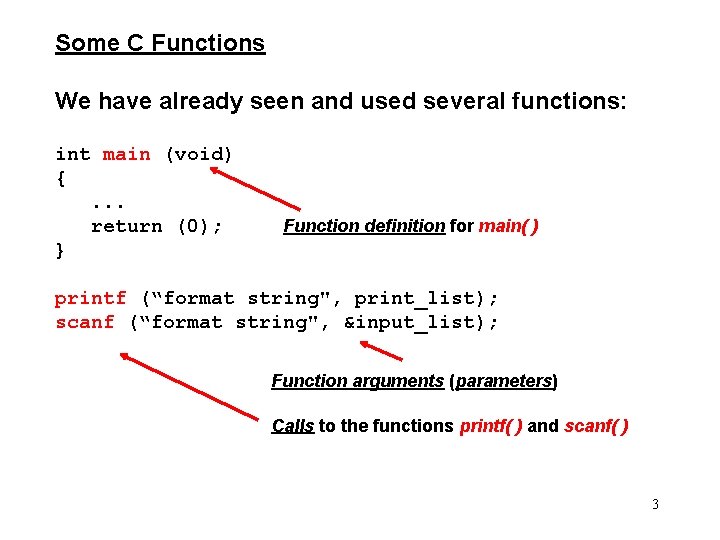
Some C Functions We have already seen and used several functions: int main (void) {. . . return (0); } Function definition for main( ) printf (“format string", print_list); scanf (“format string", &input_list); Function arguments (parameters) Calls to the functions printf( ) and scanf( ) 3
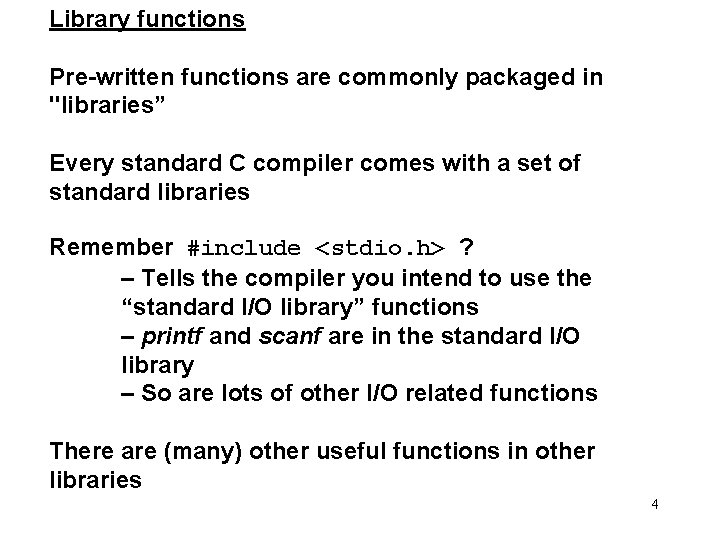
Library functions Pre-written functions are commonly packaged in "libraries” Every standard C compiler comes with a set of standard libraries Remember #include <stdio. h> ? – Tells the compiler you intend to use the “standard I/O library” functions – printf and scanf are in the standard I/O library – So are lots of other I/O related functions There are (many) other useful functions in other libraries 4
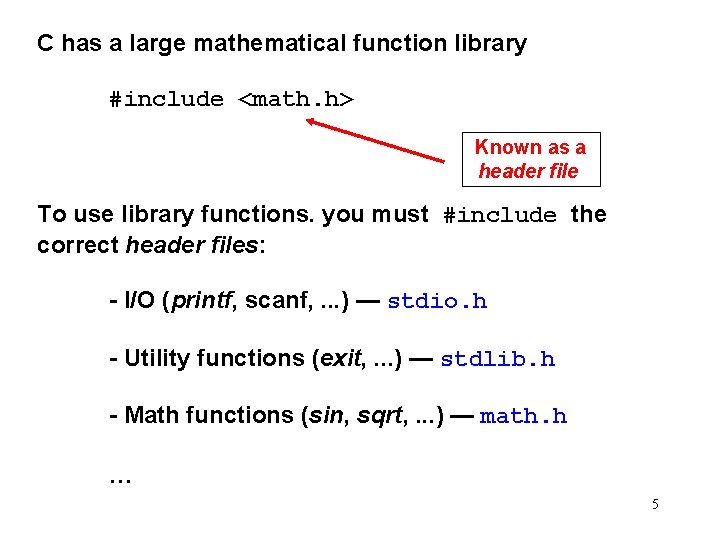
C has a large mathematical function library #include <math. h> Known as a header file To use library functions. you must #include the correct header files: - I/O (printf, scanf, . . . ) — stdio. h - Utility functions (exit, . . . ) — stdlib. h - Math functions (sin, sqrt, . . . ) — math. h … 5
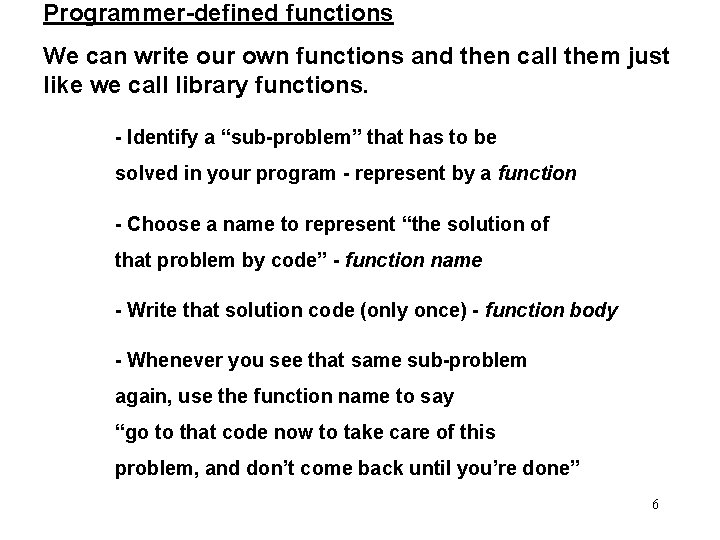
Programmer-defined functions We can write our own functions and then call them just like we call library functions. - Identify a “sub-problem” that has to be solved in your program - represent by a function - Choose a name to represent “the solution of that problem by code” - function name - Write that solution code (only once) - function body - Whenever you see that same sub-problem again, use the function name to say “go to that code now to take care of this problem, and don’t come back until you’re done” 6
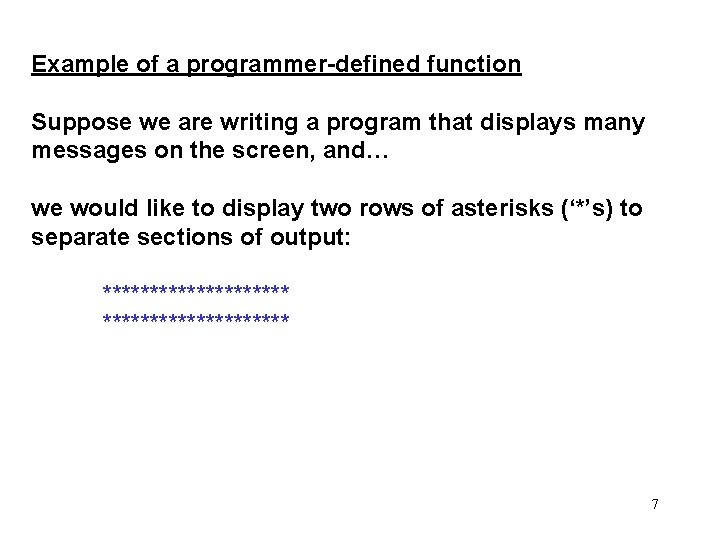
Example of a programmer-defined function Suppose we are writing a program that displays many messages on the screen, and… we would like to display two rows of asterisks (‘*’s) to separate sections of output: ******************** 7
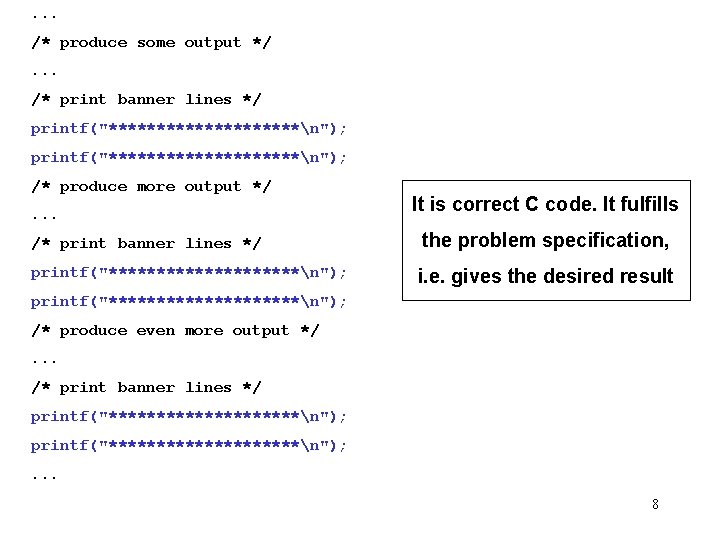
. . . /* produce some output */. . . /* print banner lines */ printf("********************n"); /* produce more output */. . . It is correct C code. It fulfills /* print banner lines */ the problem specification, printf("**********n"); i. e. gives the desired result printf("**********n"); /* produce even more output */. . . /* print banner lines */ printf("********************n"); . . . 8
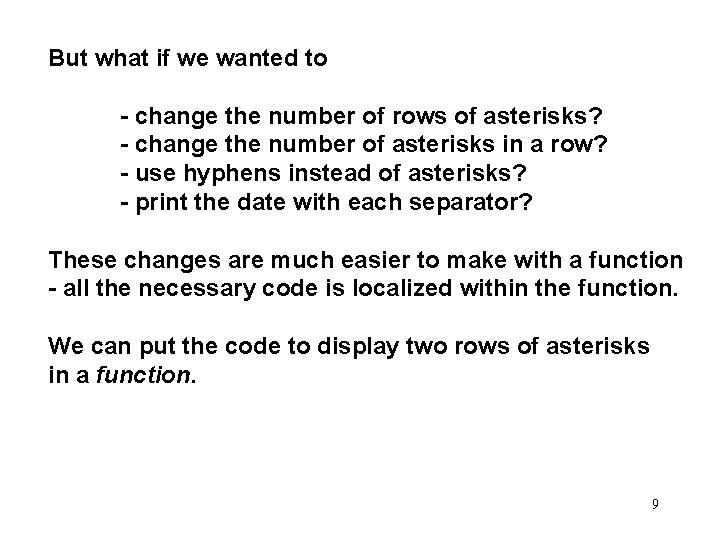
But what if we wanted to - change the number of rows of asterisks? - change the number of asterisks in a row? - use hyphens instead of asterisks? - print the date with each separator? These changes are much easier to make with a function - all the necessary code is localized within the function. We can put the code to display two rows of asterisks in a function. 9
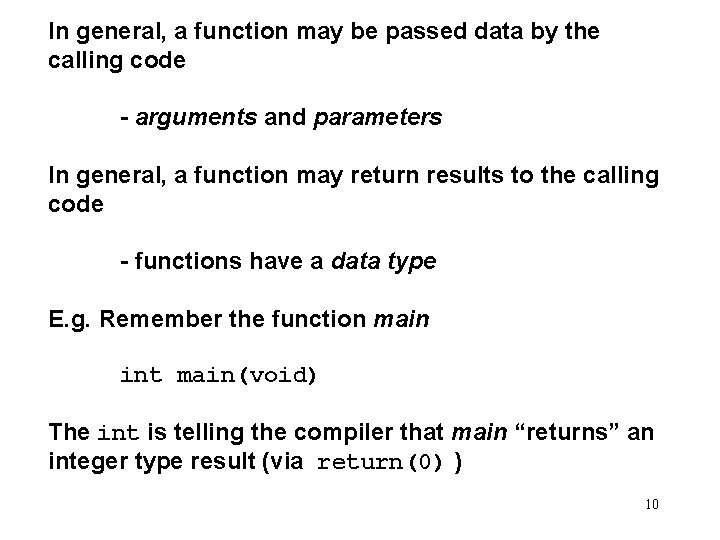
In general, a function may be passed data by the calling code - arguments and parameters In general, a function may return results to the calling code - functions have a data type E. g. Remember the function main int main(void) The int is telling the compiler that main “returns” an integer type result (via return(0) ) 10
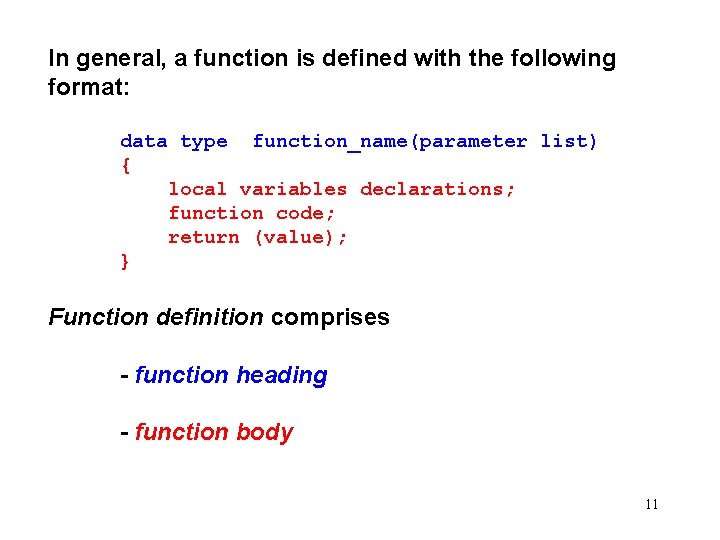
In general, a function is defined with the following format: data type function_name(parameter list) { local variables declarations; function code; return (value); } Function definition comprises - function heading - function body 11
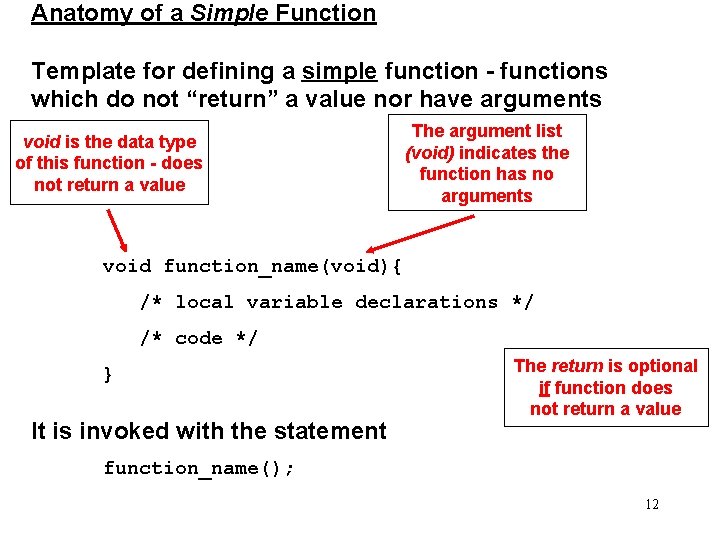
Anatomy of a Simple Function Template for defining a simple function - functions which do not “return” a value nor have arguments void is the data type of this function - does not return a value The argument list (void) indicates the function has no arguments void function_name(void){ /* local variable declarations */ /* code */ } It is invoked with the statement The return is optional if function does not return a value function_name(); 12
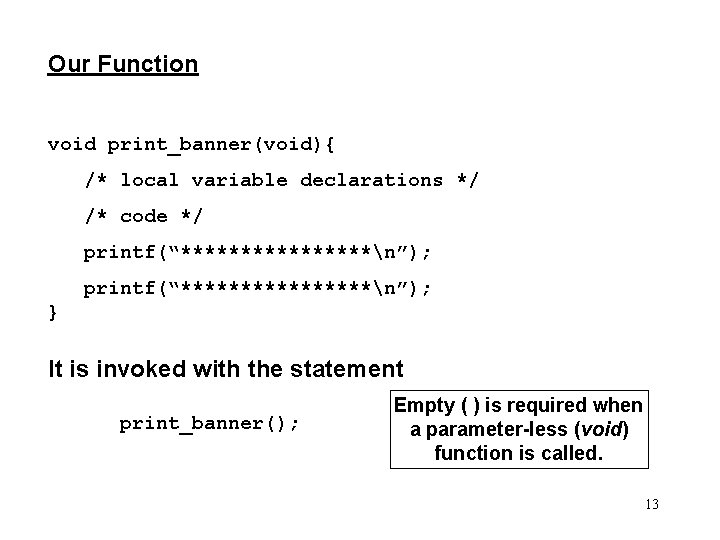
Our Function void print_banner(void){ /* local variable declarations */ /* code */ printf(“****************n”); } It is invoked with the statement print_banner(); Empty ( ) is required when a parameter-less (void) function is called. 13
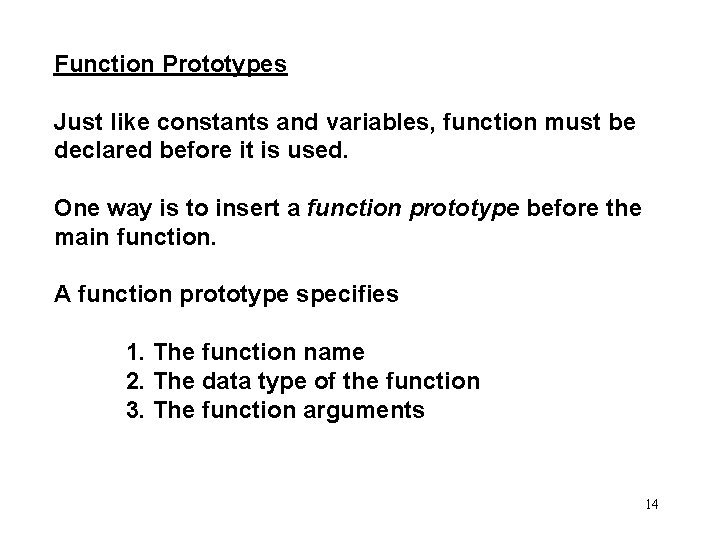
Function Prototypes Just like constants and variables, function must be declared before it is used. One way is to insert a function prototype before the main function. A function prototype specifies 1. The function name 2. The data type of the function 3. The function arguments 14
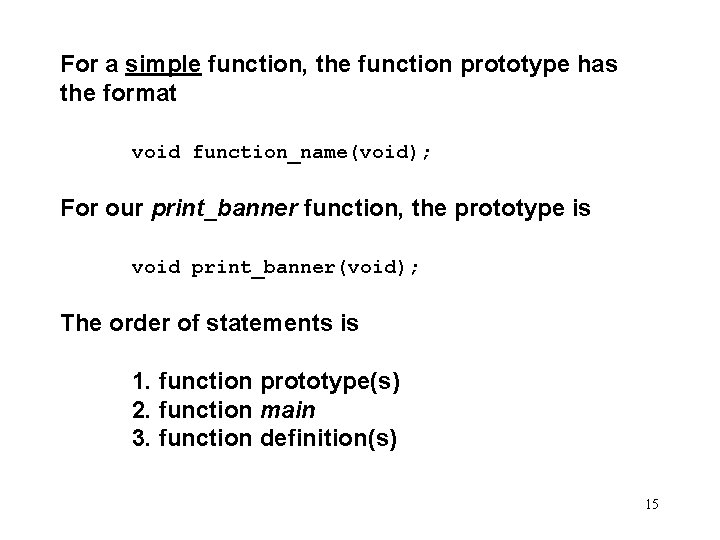
For a simple function, the function prototype has the format void function_name(void); For our print_banner function, the prototype is void print_banner(void); The order of statements is 1. function prototype(s) 2. function main 3. function definition(s) 15
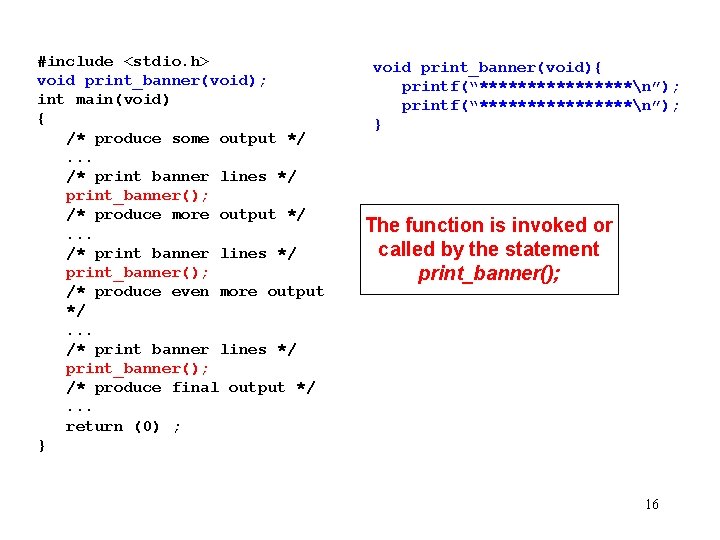
#include <stdio. h> void print_banner(void); int main(void) { /* produce some output */. . . /* print banner lines */ print_banner(); /* produce more output */. . . /* print banner lines */ print_banner(); /* produce even more output */. . . /* print banner lines */ print_banner(); /* produce final output */. . . return (0) ; } void print_banner(void){ printf(“****************n”); } The function is invoked or called by the statement print_banner(); 16
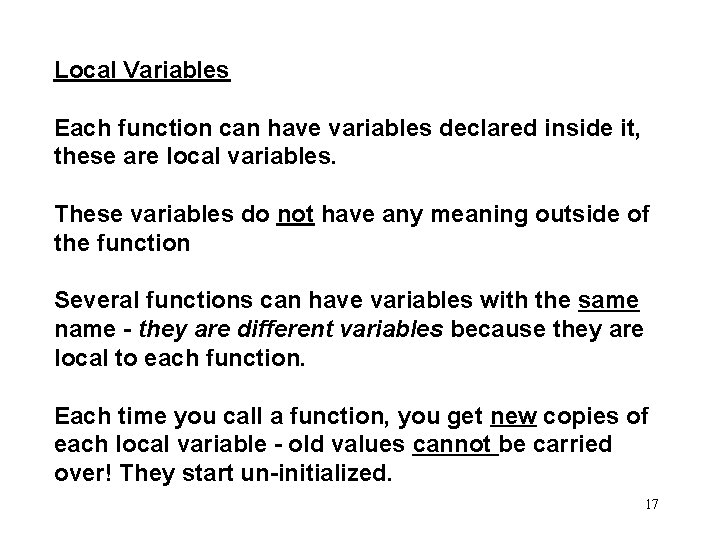
Local Variables Each function can have variables declared inside it, these are local variables. These variables do not have any meaning outside of the function Several functions can have variables with the same name - they are different variables because they are local to each function. Each time you call a function, you get new copies of each local variable - old values cannot be carried over! They start un-initialized. 17
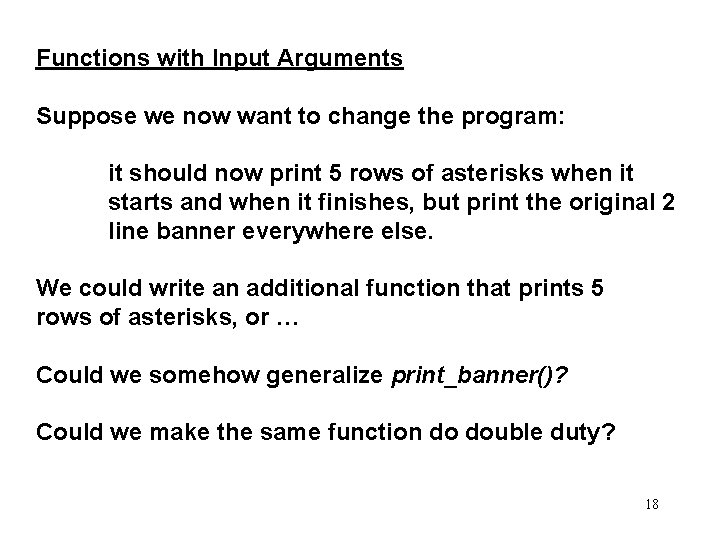
Functions with Input Arguments Suppose we now want to change the program: it should now print 5 rows of asterisks when it starts and when it finishes, but print the original 2 line banner everywhere else. We could write an additional function that prints 5 rows of asterisks, or … Could we somehow generalize print_banner()? Could we make the same function do double duty? 18
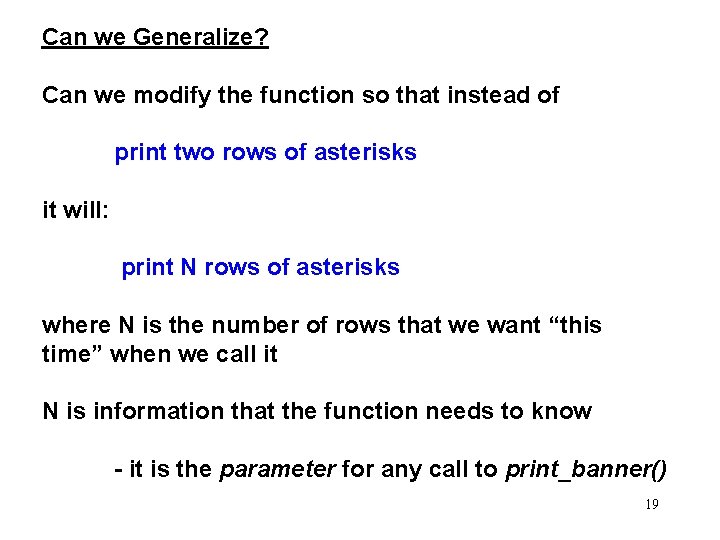
Can we Generalize? Can we modify the function so that instead of print two rows of asterisks it will: print N rows of asterisks where N is the number of rows that we want “this time” when we call it N is information that the function needs to know - it is the parameter for any call to print_banner() 19
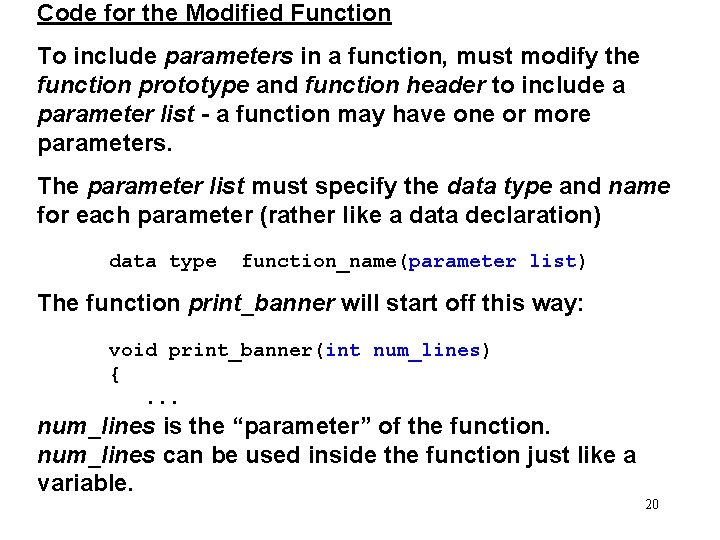
Code for the Modified Function To include parameters in a function, must modify the function prototype and function header to include a parameter list - a function may have one or more parameters. The parameter list must specify the data type and name for each parameter (rather like a data declaration) data type function_name(parameter list) The function print_banner will start off this way: void print_banner(int num_lines) {. . . num_lines is the “parameter” of the function. num_lines can be used inside the function just like a variable. 20
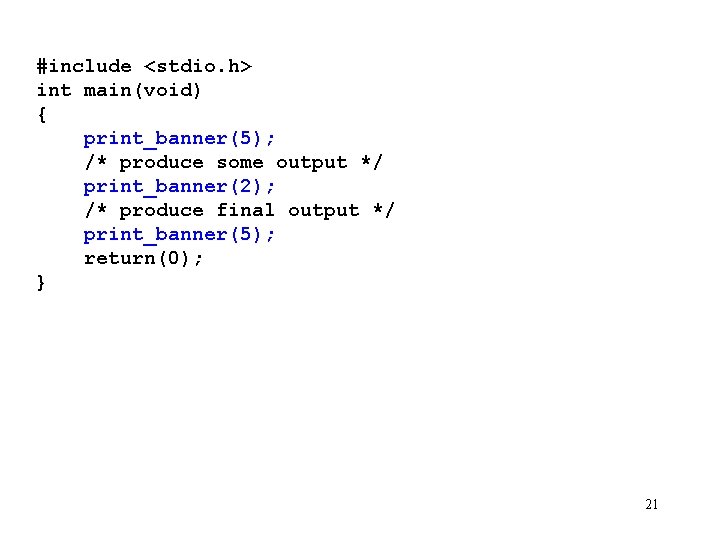
#include <stdio. h> int main(void) { print_banner(5); /* produce some output */ print_banner(2); /* produce final output */ print_banner(5); return(0); } 21
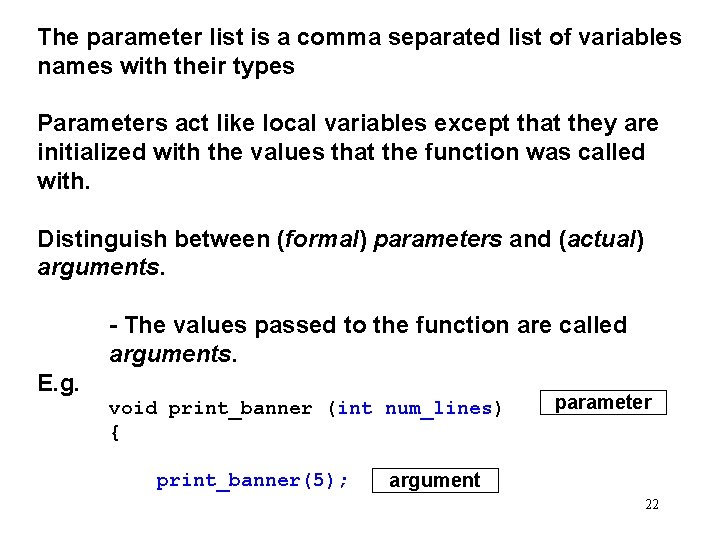
The parameter list is a comma separated list of variables names with their types Parameters act like local variables except that they are initialized with the values that the function was called with. Distinguish between (formal) parameters and (actual) arguments. - The values passed to the function are called arguments. E. g. void print_banner (int num_lines) { print_banner(5); parameter argument 22
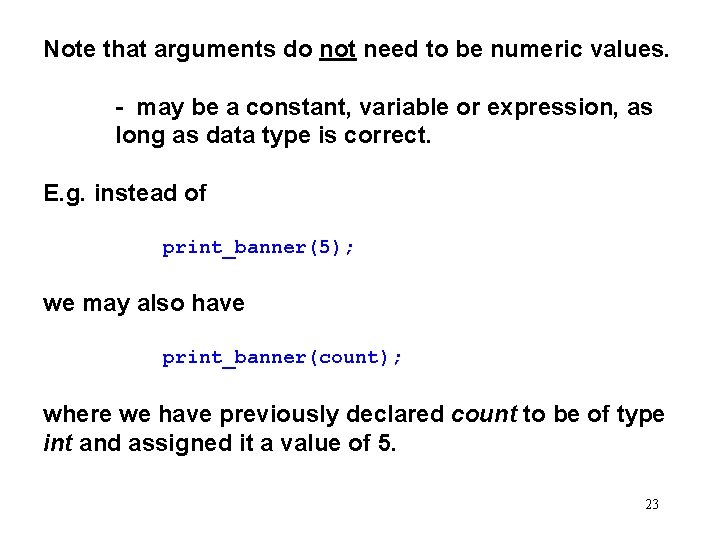
Note that arguments do not need to be numeric values. - may be a constant, variable or expression, as long as data type is correct. E. g. instead of print_banner(5); we may also have print_banner(count); where we have previously declared count to be of type int and assigned it a value of 5. 23
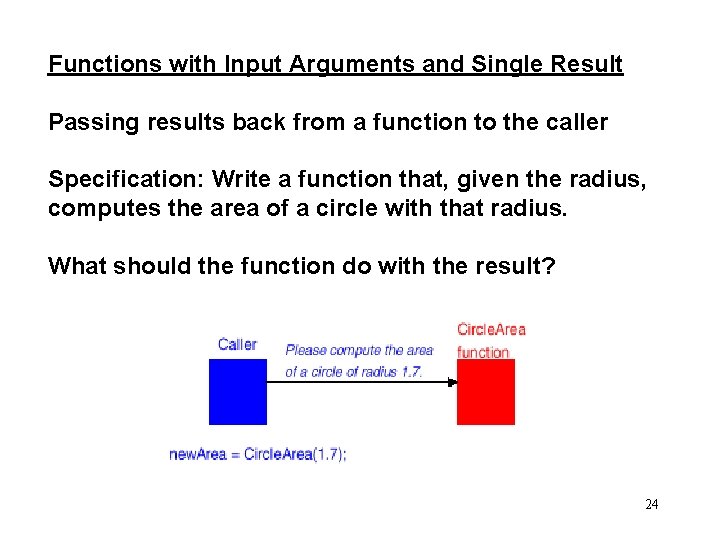
Functions with Input Arguments and Single Result Passing results back from a function to the caller Specification: Write a function that, given the radius, computes the area of a circle with that radius. What should the function do with the result? 24
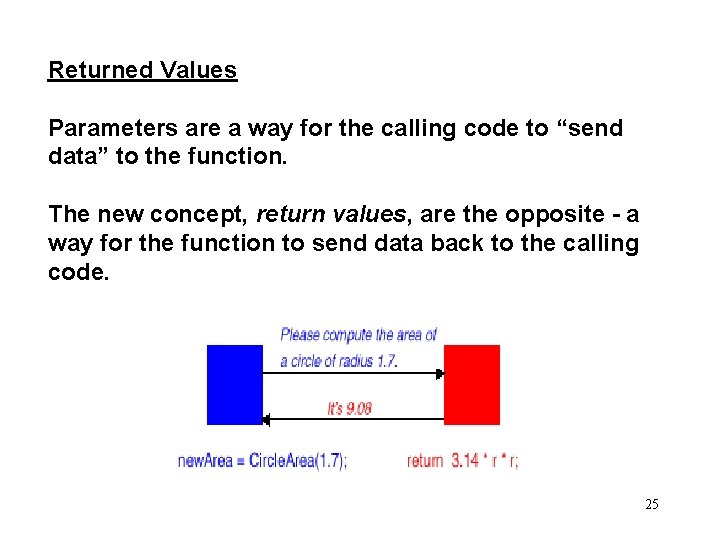
Returned Values Parameters are a way for the calling code to “send data” to the function. The new concept, return values, are the opposite - a way for the function to send data back to the calling code. 25
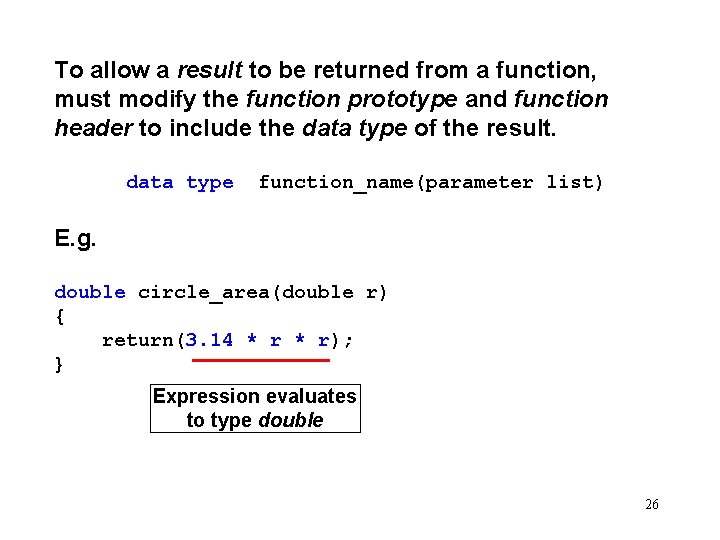
To allow a result to be returned from a function, must modify the function prototype and function header to include the data type of the result. data type function_name(parameter list) E. g. double circle_area(double r) { return(3. 14 * r); } Expression evaluates to type double 26
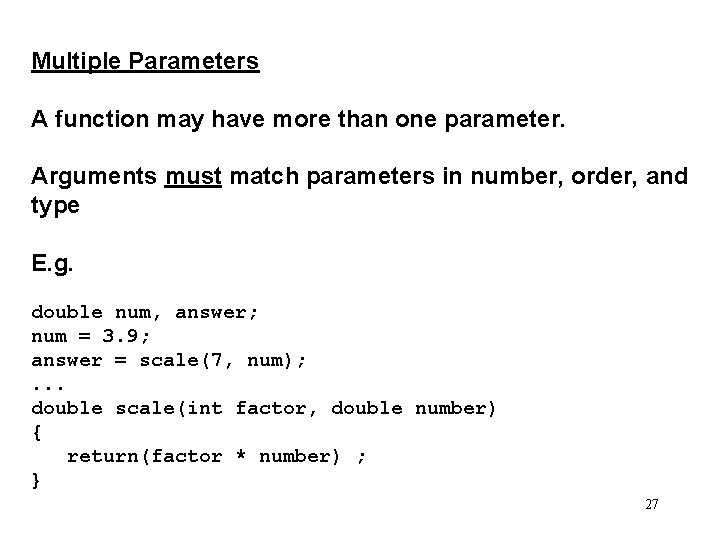
Multiple Parameters A function may have more than one parameter. Arguments must match parameters in number, order, and type E. g. double num, answer; num = 3. 9; answer = scale(7, num); . . . double scale(int factor, double number) { return(factor * number) ; } 27
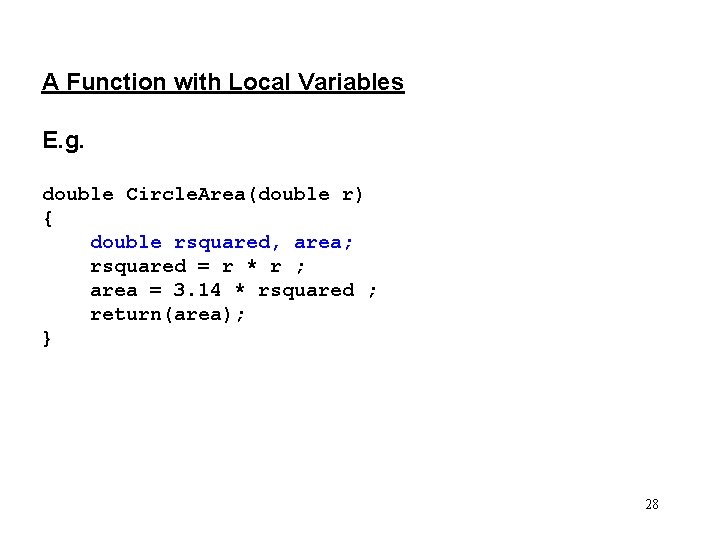
A Function with Local Variables E. g. double Circle. Area(double r) { double rsquared, area; rsquared = r * r ; area = 3. 14 * rsquared ; return(area); } 28
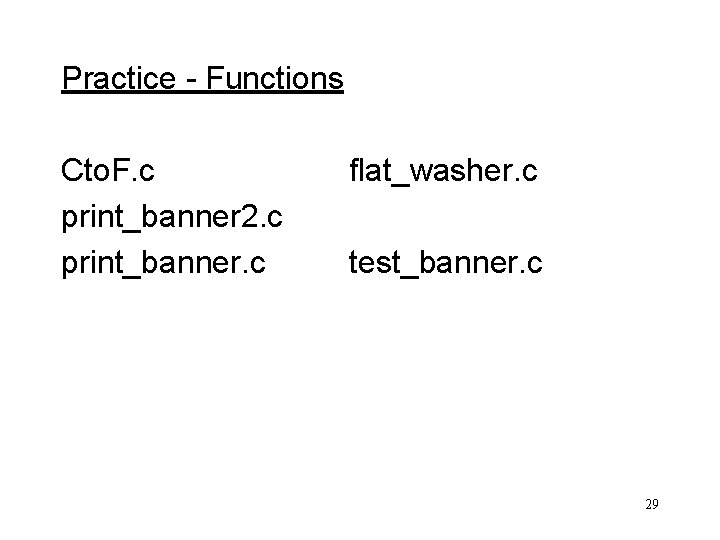
Practice - Functions Cto. F. c print_banner 2. c print_banner. c flat_washer. c test_banner. c 29
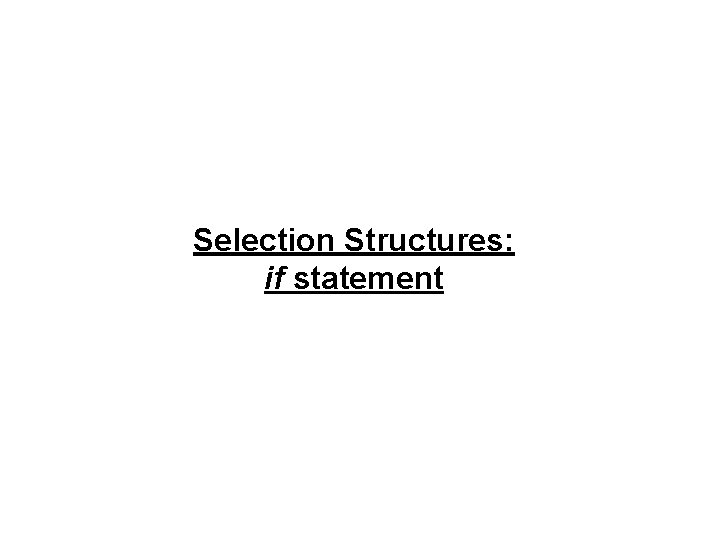
Selection Structures: if statement
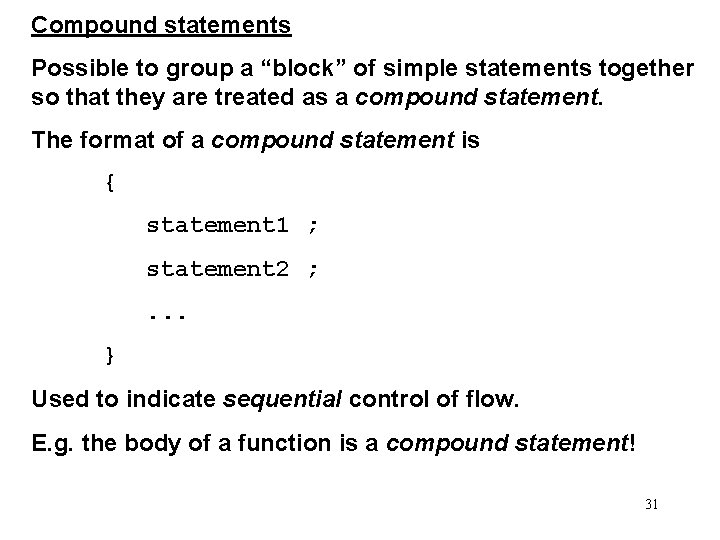
Compound statements Possible to group a “block” of simple statements together so that they are treated as a compound statement. The format of a compound statement is { statement 1 ; statement 2 ; . . . } Used to indicate sequential control of flow. E. g. the body of a function is a compound statement! 31
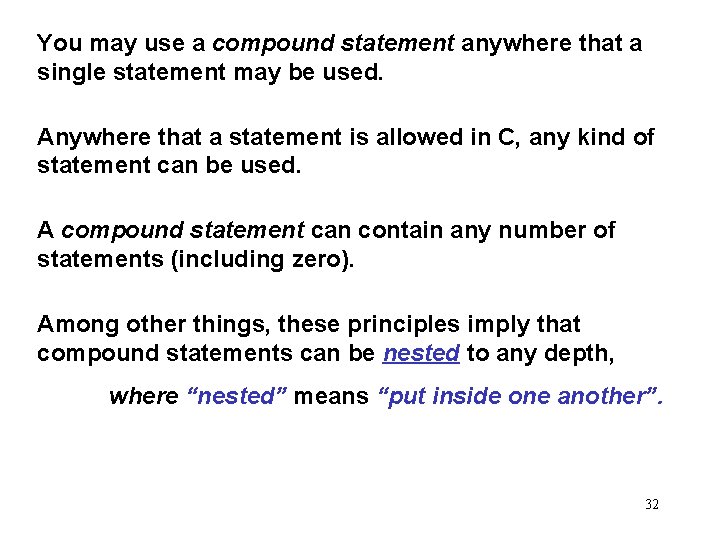
You may use a compound statement anywhere that a single statement may be used. Anywhere that a statement is allowed in C, any kind of statement can be used. A compound statement can contain any number of statements (including zero). Among other things, these principles imply that compound statements can be nested to any depth, where “nested” means “put inside one another”. 32
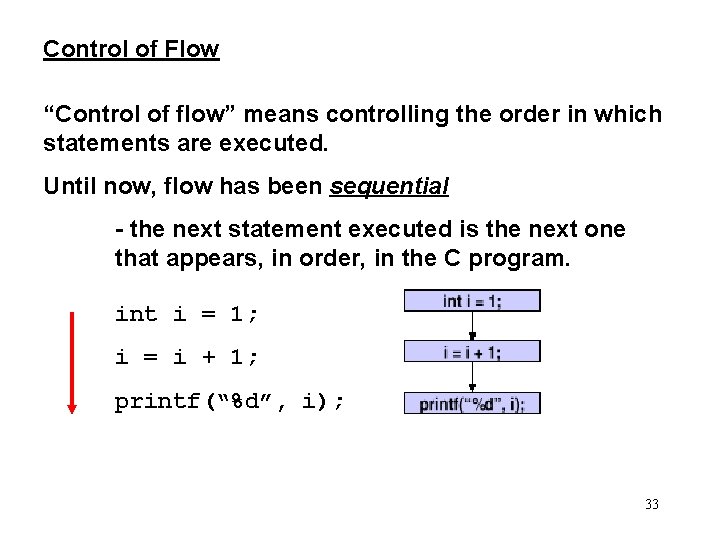
Control of Flow “Control of flow” means controlling the order in which statements are executed. Until now, flow has been sequential - the next statement executed is the next one that appears, in order, in the C program. int i = 1; i = i + 1; printf(“%d”, i); 33
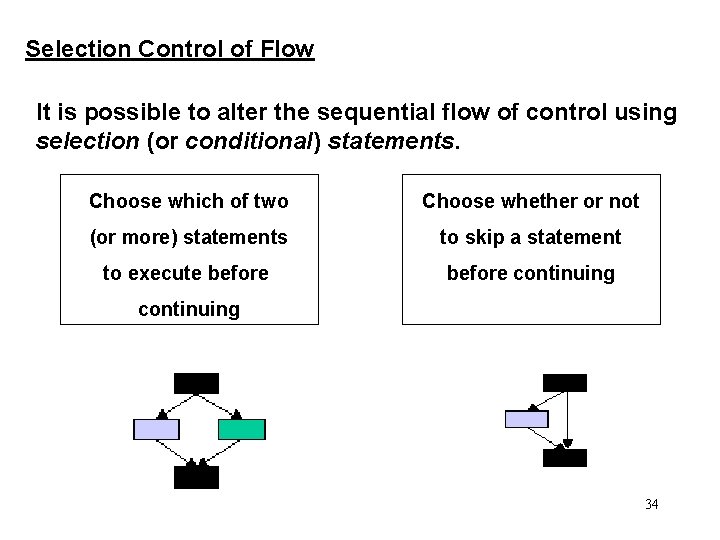
Selection Control of Flow It is possible to alter the sequential flow of control using selection (or conditional) statements. Choose which of two Choose whether or not (or more) statements to skip a statement to execute before continuing 34
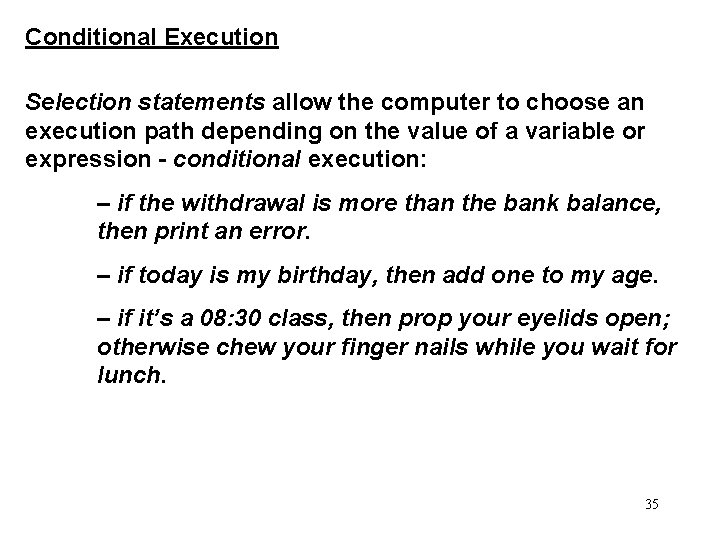
Conditional Execution Selection statements allow the computer to choose an execution path depending on the value of a variable or expression - conditional execution: – if the withdrawal is more than the bank balance, then print an error. – if today is my birthday, then add one to my age. – if it’s a 08: 30 class, then prop your eyelids open; otherwise chew your finger nails while you wait for lunch. 35
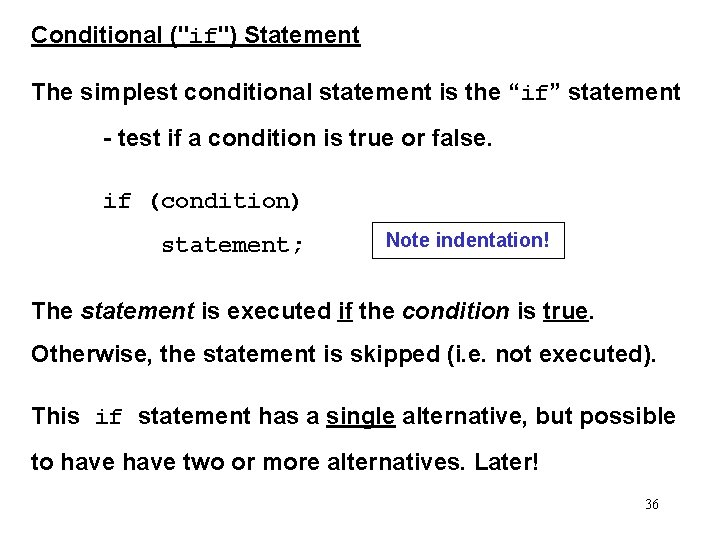
Conditional ("if") Statement The simplest conditional statement is the “if” statement - test if a condition is true or false. if (condition) statement; Note indentation! The statement is executed if the condition is true. Otherwise, the statement is skipped (i. e. not executed). This if statement has a single alternative, but possible to have two or more alternatives. Later! 36
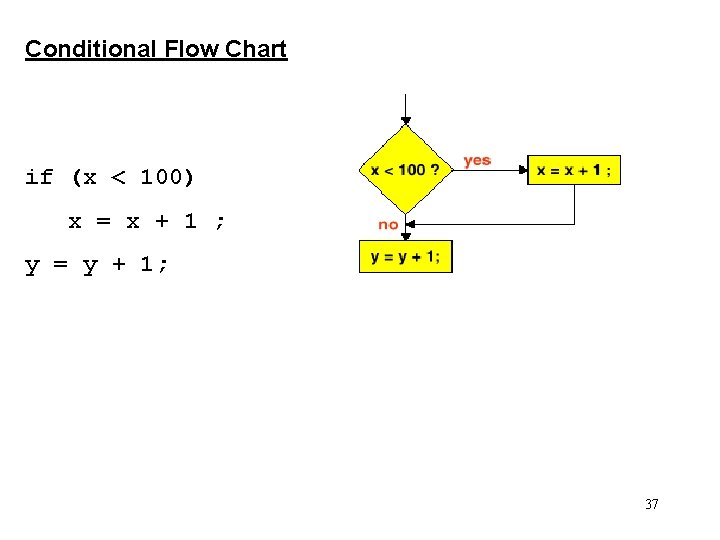
Conditional Flow Chart if (x < 100) x = x + 1 ; y = y + 1; 37
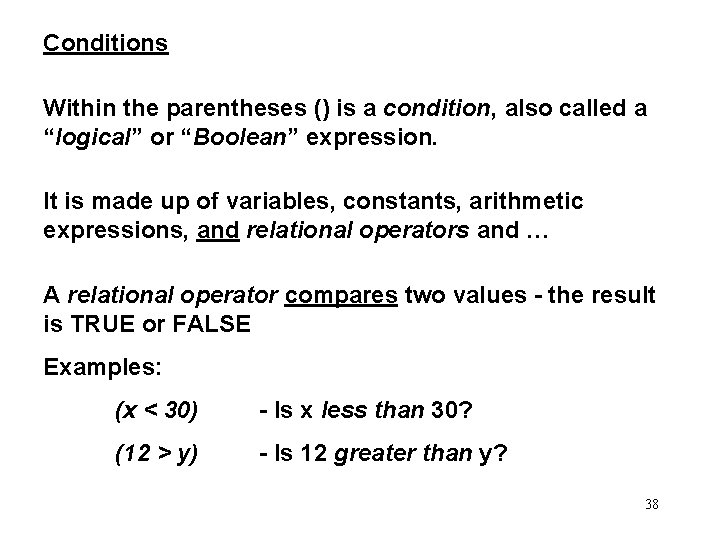
Conditions Within the parentheses () is a condition, also called a “logical” or “Boolean” expression. It is made up of variables, constants, arithmetic expressions, and relational operators and … A relational operator compares two values - the result is TRUE or FALSE Examples: (x < 30) - Is x less than 30? (12 > y) - Is 12 greater than y? 38
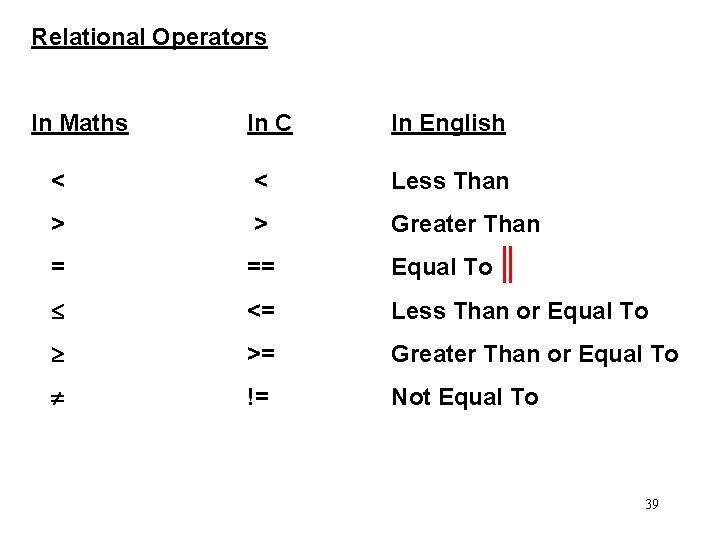
Relational Operators In Maths In C In English < < Less Than > > Greater Than = == Equal To <= Less Than or Equal To >= Greater Than or Equal To != Not Equal To 39
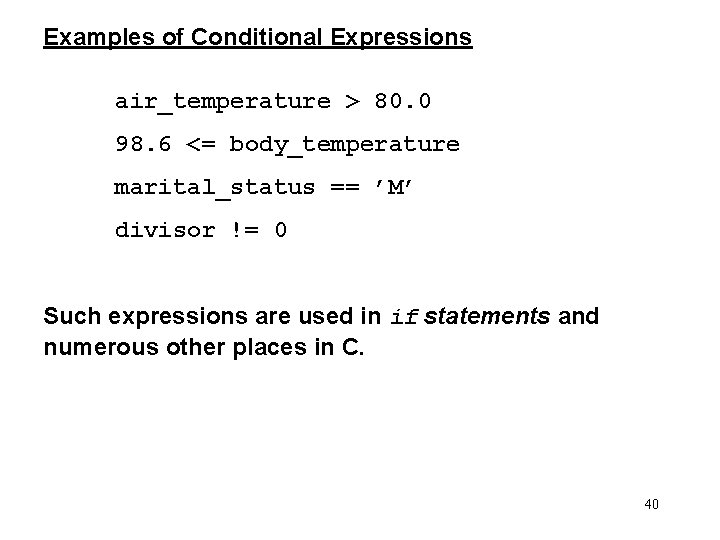
Examples of Conditional Expressions air_temperature > 80. 0 98. 6 <= body_temperature marital_status == ’M’ divisor != 0 Such expressions are used in if statements and numerous other places in C. 40
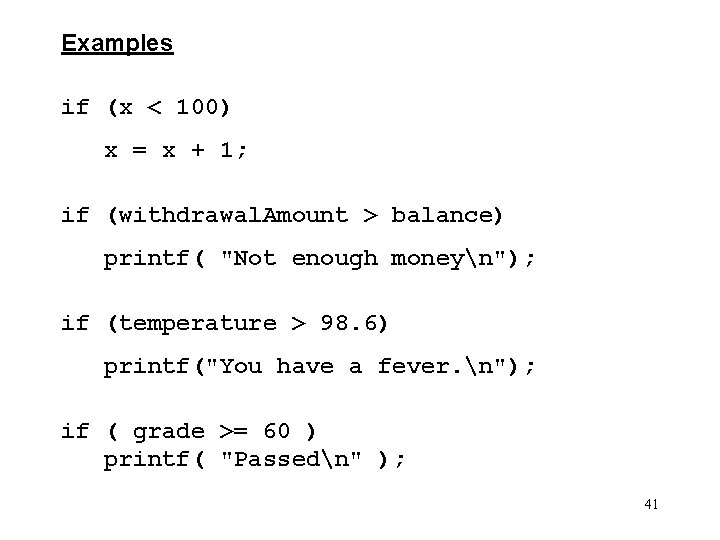
Examples if (x < 100) x = x + 1; if (withdrawal. Amount > balance) printf( "Not enough moneyn"); if (temperature > 98. 6) printf("You have a fever. n"); if ( grade >= 60 ) printf( "Passedn" ); 41
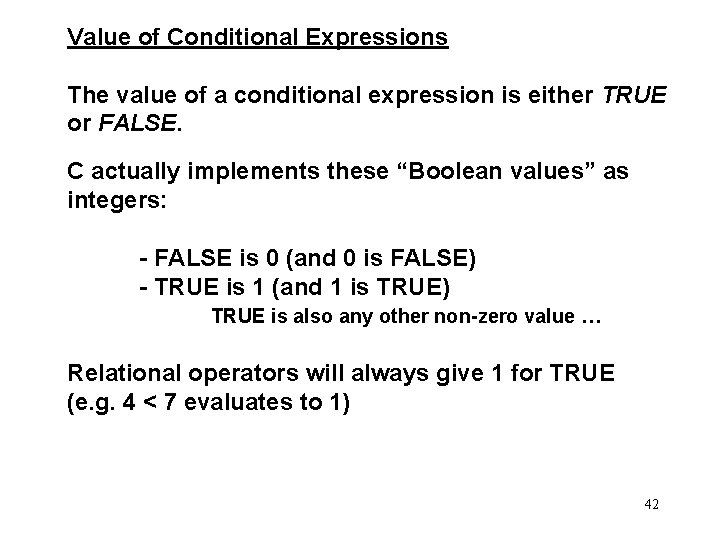
Value of Conditional Expressions The value of a conditional expression is either TRUE or FALSE. C actually implements these “Boolean values” as integers: - FALSE is 0 (and 0 is FALSE) - TRUE is 1 (and 1 is TRUE) TRUE is also any other non-zero value … Relational operators will always give 1 for TRUE (e. g. 4 < 7 evaluates to 1) 42
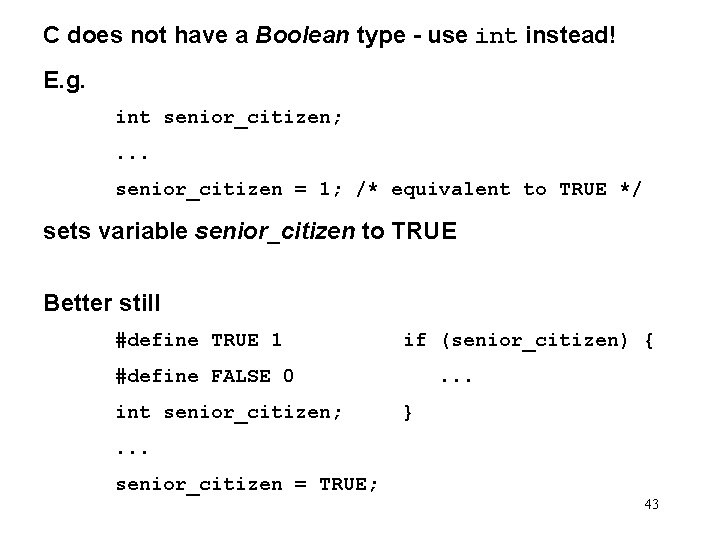
C does not have a Boolean type - use int instead! E. g. int senior_citizen; . . . senior_citizen = 1; /* equivalent to TRUE */ sets variable senior_citizen to TRUE Better still #define TRUE 1 if (senior_citizen) { #define FALSE 0 int senior_citizen; . . . } . . . senior_citizen = TRUE; 43
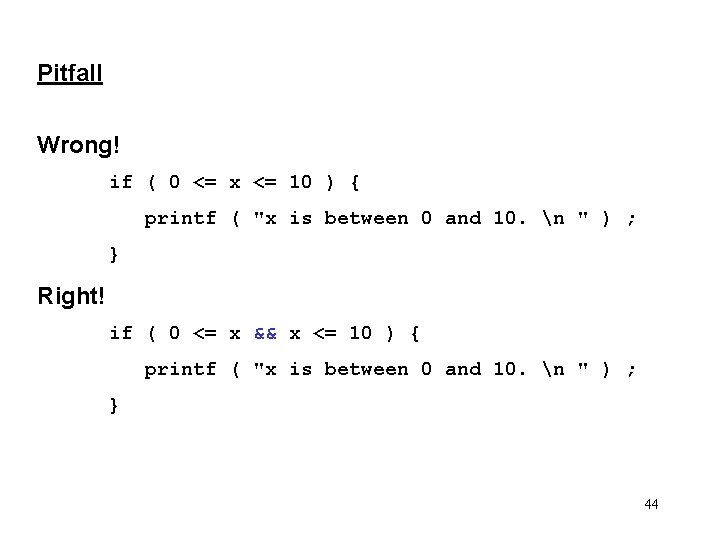
Pitfall Wrong! if ( 0 <= x <= 10 ) { printf ( "x is between 0 and 10. n " ) ; } Right! if ( 0 <= x && x <= 10 ) { printf ( "x is between 0 and 10. n " ) ; } 44
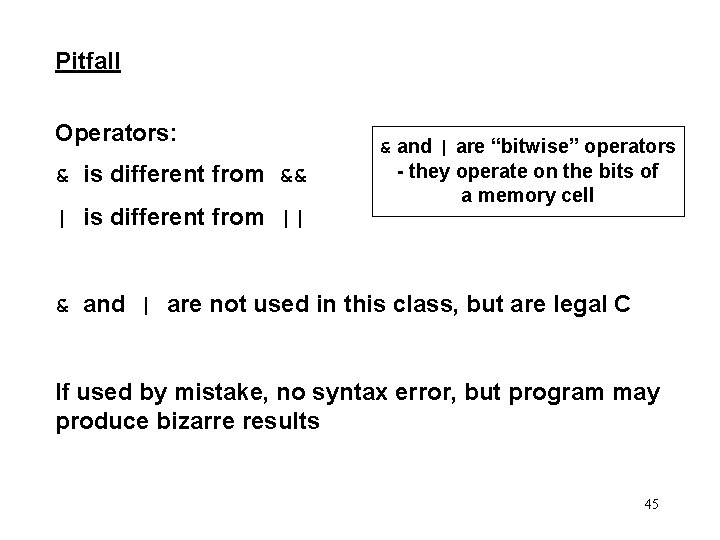
Pitfall Operators: & is different from && | is different from || & and | are “bitwise” operators - they operate on the bits of a memory cell & and | are not used in this class, but are legal C If used by mistake, no syntax error, but program may produce bizarre results 45
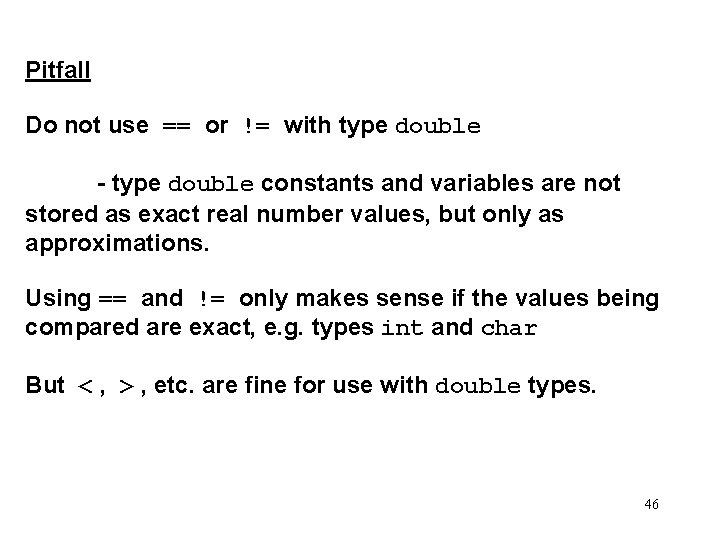
Pitfall Do not use == or != with type double - type double constants and variables are not stored as exact real number values, but only as approximations. Using == and != only makes sense if the values being compared are exact, e. g. types int and char But < , > , etc. are fine for use with double types. 46
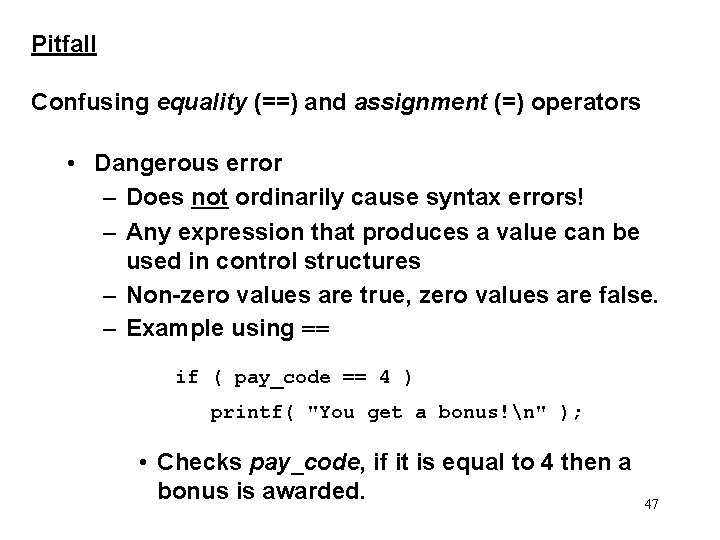
Pitfall Confusing equality (==) and assignment (=) operators • Dangerous error – Does not ordinarily cause syntax errors! – Any expression that produces a value can be used in control structures – Non-zero values are true, zero values are false. – Example using == if ( pay_code == 4 ) printf( "You get a bonus!n" ); • Checks pay_code, if it is equal to 4 then a bonus is awarded. 47
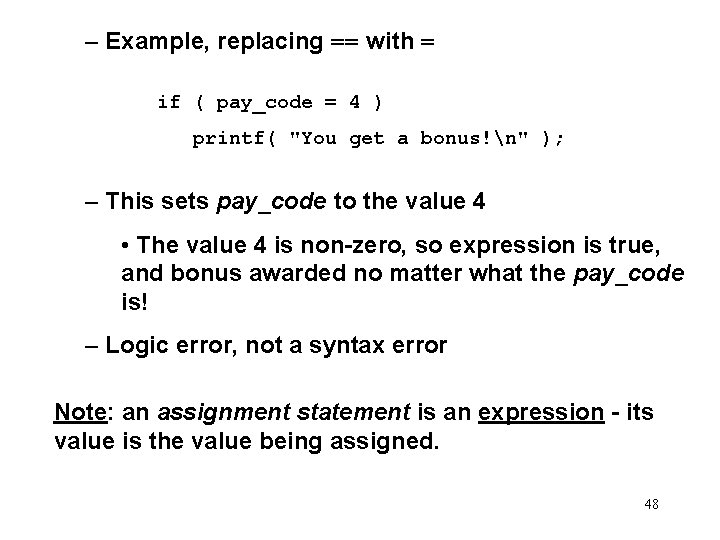
– Example, replacing == with = if ( pay_code = 4 ) printf( "You get a bonus!n" ); – This sets pay_code to the value 4 • The value 4 is non-zero, so expression is true, and bonus awarded no matter what the pay_code is! – Logic error, not a syntax error Note: an assignment statement is an expression - its value is the value being assigned. 48
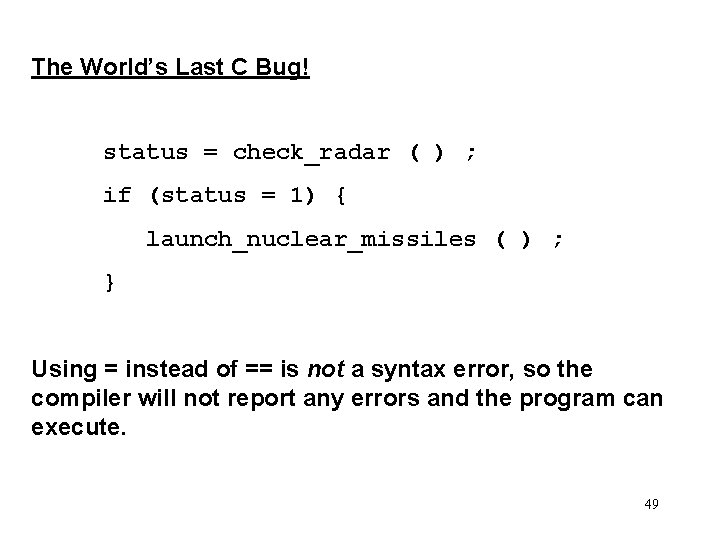
The World’s Last C Bug! status = check_radar ( ) ; if (status = 1) { launch_nuclear_missiles ( ) ; } Using = instead of == is not a syntax error, so the compiler will not report any errors and the program can execute. 49
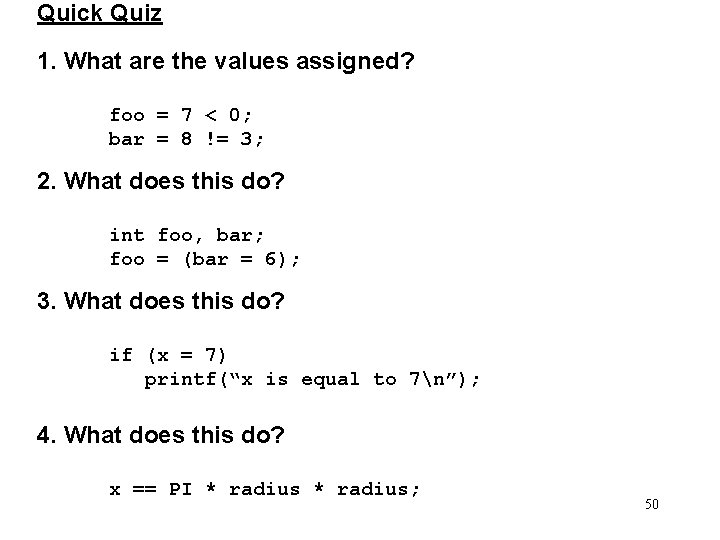
Quick Quiz 1. What are the values assigned? foo = 7 < 0; bar = 8 != 3; 2. What does this do? int foo, bar; foo = (bar = 6); 3. What does this do? if (x = 7) printf(“x is equal to 7n”); 4. What does this do? x == PI * radius; 50
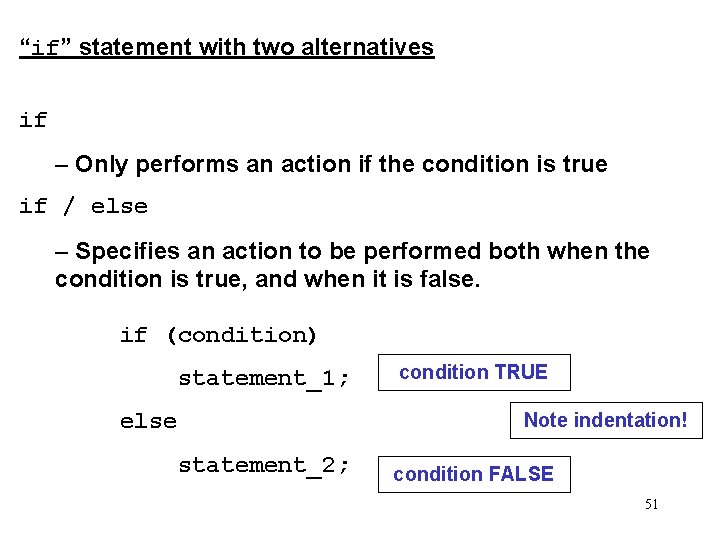
“if” statement with two alternatives if – Only performs an action if the condition is true if / else – Specifies an action to be performed both when the condition is true, and when it is false. if (condition) statement_1; else condition TRUE Note indentation! statement_2; condition FALSE 51
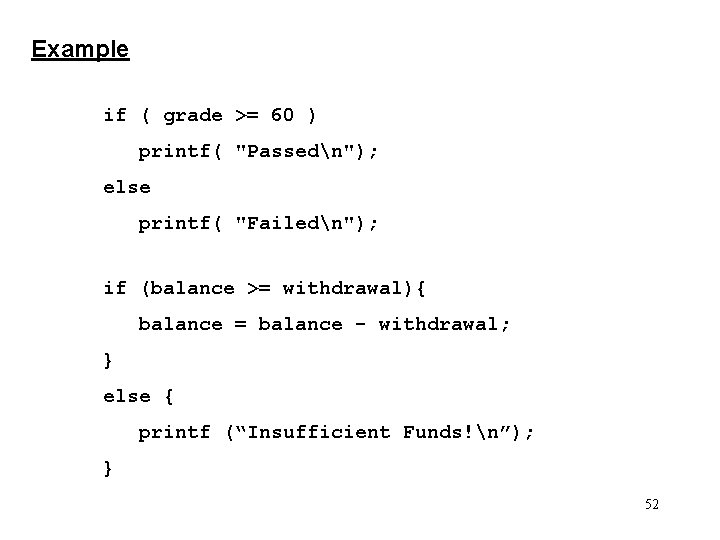
Example if ( grade >= 60 ) printf( "Passedn"); else printf( "Failedn"); if (balance >= withdrawal){ balance = balance - withdrawal; } else { printf (“Insufficient Funds!n”); } 52
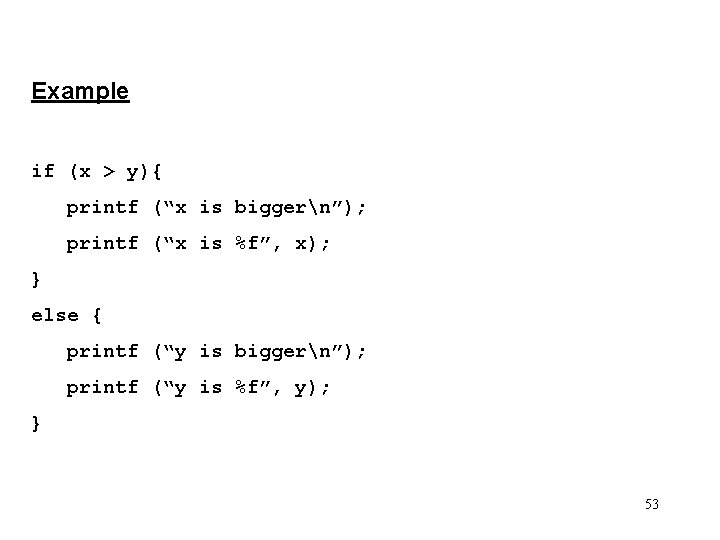
Example if (x > y){ printf (“x is biggern”); printf (“x is %f”, x); } else { printf (“y is biggern”); printf (“y is %f”, y); } 53
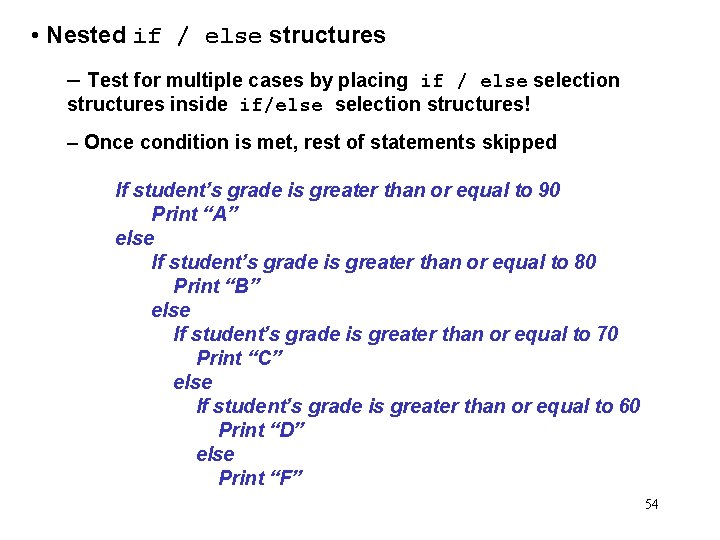
• Nested if / else structures – Test for multiple cases by placing if / else selection structures inside if/else selection structures! – Once condition is met, rest of statements skipped If student’s grade is greater than or equal to 90 Print “A” else If student’s grade is greater than or equal to 80 Print “B” else If student’s grade is greater than or equal to 70 Print “C” else If student’s grade is greater than or equal to 60 Print “D” else Print “F” 54
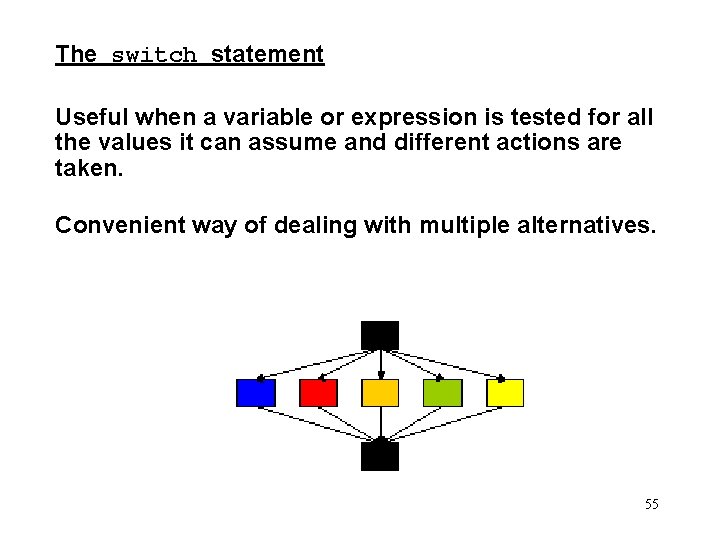
The switch statement Useful when a variable or expression is tested for all the values it can assume and different actions are taken. Convenient way of dealing with multiple alternatives. 55
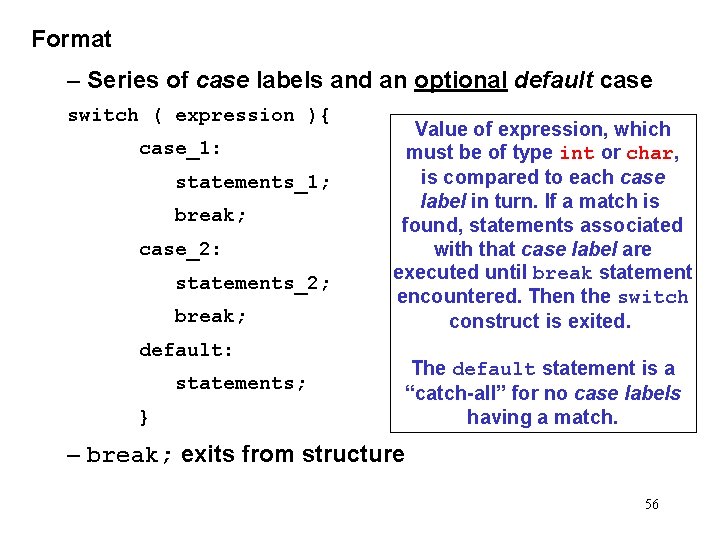
Format – Series of case labels and an optional default case switch ( expression ){ case_1: statements_1; break; case_2: statements_2; break; default: statements; } Value of expression, which must be of type int or char, is compared to each case label in turn. If a match is found, statements associated with that case label are executed until break statement encountered. Then the switch construct is exited. The default statement is a “catch-all” for no case labels having a match. – break; exits from structure 56
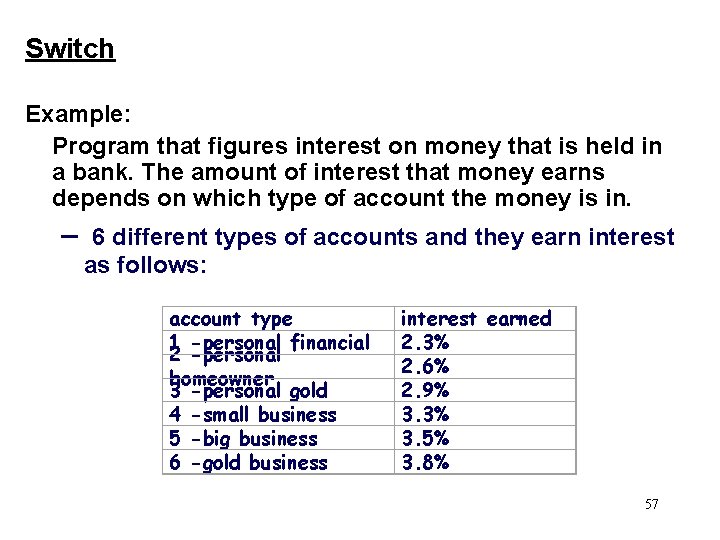
Switch Example: Program that figures interest on money that is held in a bank. The amount of interest that money earns depends on which type of account the money is in. – 6 different types of accounts and they earn interest as follows: account type 1 -personal financial 2 -personal homeowner 3 -personal gold 4 -small business 5 -big business 6 -gold business interest earned 2. 3% 2. 6% 2. 9% 3. 3% 3. 5% 3. 8% 57
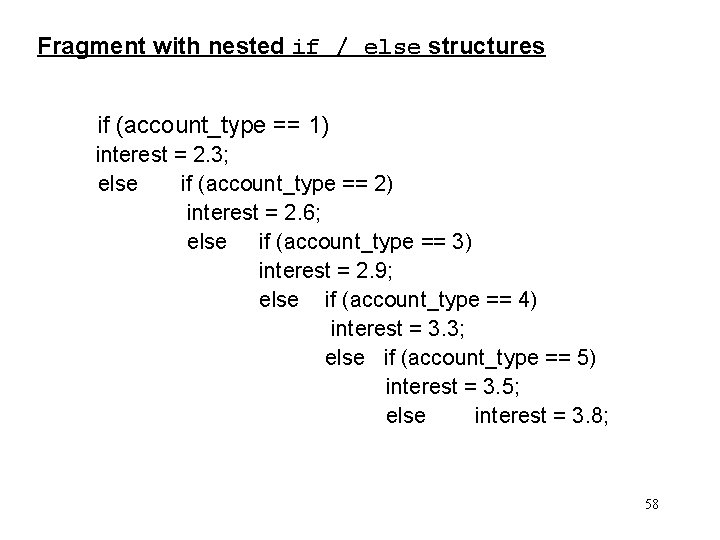
Fragment with nested if / else structures if (account_type == 1) interest = 2. 3; else if (account_type == 2) interest = 2. 6; else if (account_type == 3) interest = 2. 9; else if (account_type == 4) interest = 3. 3; else if (account_type == 5) interest = 3. 5; else interest = 3. 8; 58
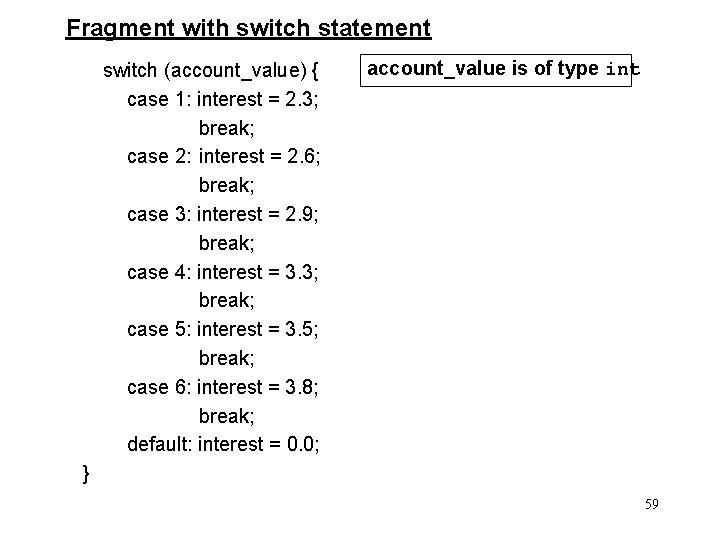
Fragment with switch statement switch (account_value) { account_value is of type int case 1: interest = 2. 3; break; case 2: interest = 2. 6; break; case 3: interest = 2. 9; break; case 4: interest = 3. 3; break; case 5: interest = 3. 5; break; case 6: interest = 3. 8; break; default: interest = 0. 0; } 59
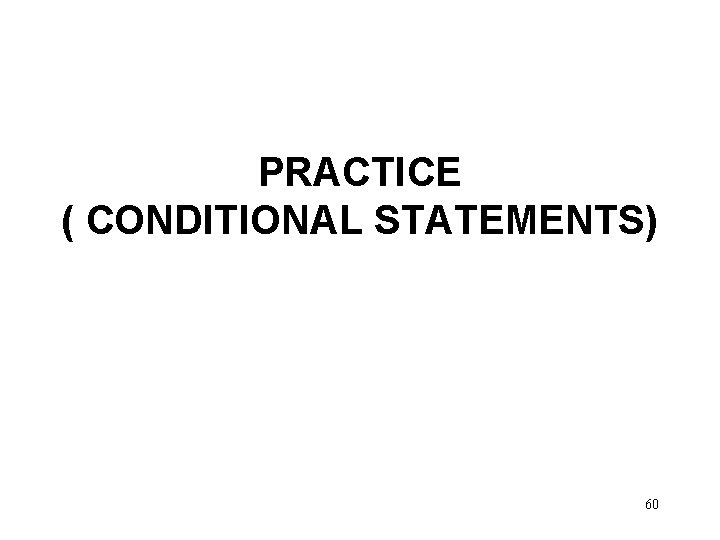
PRACTICE ( CONDITIONAL STATEMENTS) 60
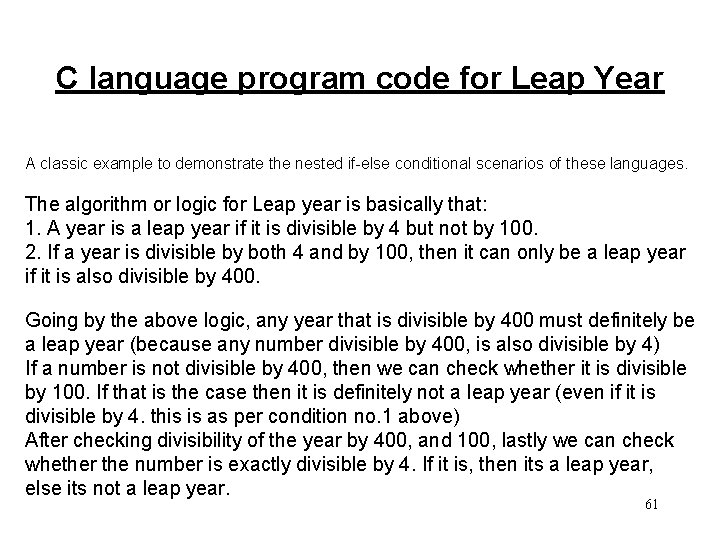
C language program code for Leap Year A classic example to demonstrate the nested if-else conditional scenarios of these languages. The algorithm or logic for Leap year is basically that: 1. A year is a leap year if it is divisible by 4 but not by 100. 2. If a year is divisible by both 4 and by 100, then it can only be a leap year if it is also divisible by 400. Going by the above logic, any year that is divisible by 400 must definitely be a leap year (because any number divisible by 400, is also divisible by 4) If a number is not divisible by 400, then we can check whether it is divisible by 100. If that is the case then it is definitely not a leap year (even if it is divisible by 4. this is as per condition no. 1 above) After checking divisibility of the year by 400, and 100, lastly we can check whether the number is exactly divisible by 4. If it is, then its a leap year, else its not a leap year. 61
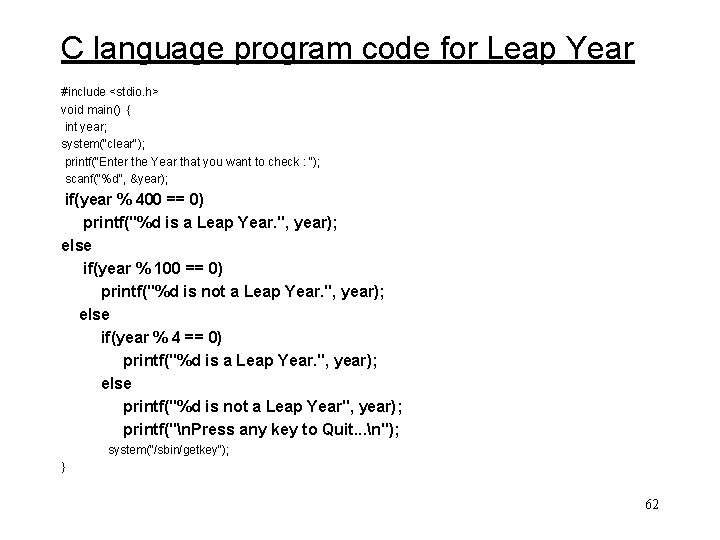
C language program code for Leap Year #include <stdio. h> void main() { int year; system("clear"); printf("Enter the Year that you want to check : "); scanf("%d", &year); if(year % 400 == 0) printf("%d is a Leap Year. ", year); else if(year % 100 == 0) printf("%d is not a Leap Year. ", year); else if(year % 4 == 0) printf("%d is a Leap Year. ", year); else printf("%d is not a Leap Year", year); printf("n. Press any key to Quit. . . n"); system("/sbin/getkey"); } 62
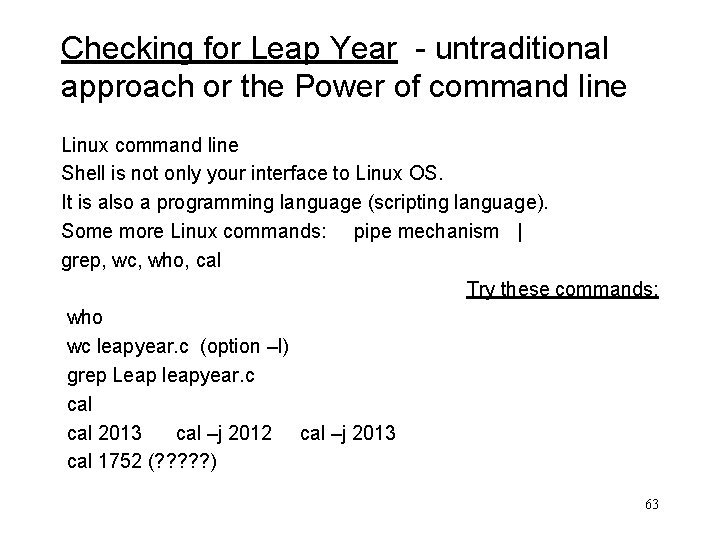
Checking for Leap Year - untraditional approach or the Power of command line Linux command line Shell is not only your interface to Linux OS. It is also a programming language (scripting language). Some more Linux commands: pipe mechanism | grep, wc, who, cal Try these commands: who wc leapyear. c (option –l) grep Leap leapyear. c cal 2013 cal –j 2012 cal –j 2013 cal 1752 (? ? ? ) 63
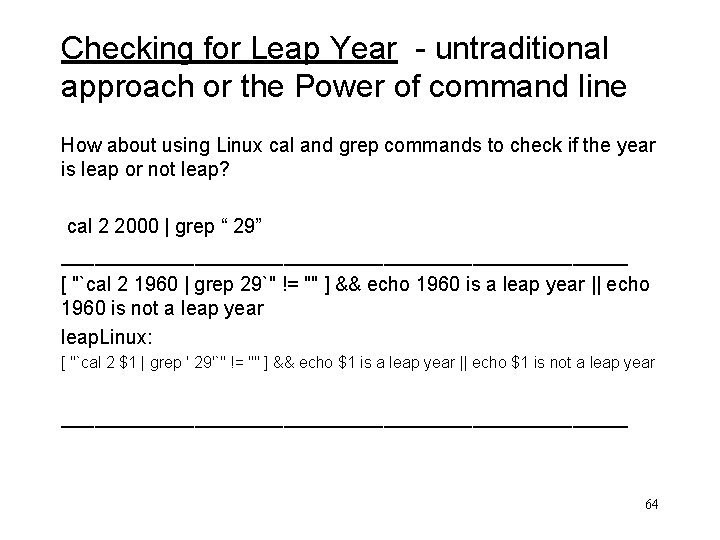
Checking for Leap Year - untraditional approach or the Power of command line How about using Linux cal and grep commands to check if the year is leap or not leap? cal 2 2000 | grep “ 29” __________________________ [ "`cal 2 1960 | grep 29`" != "" ] && echo 1960 is a leap year || echo 1960 is not a leap year leap. Linux: [ "`cal 2 $1 | grep ' 29'`" != "" ] && echo $1 is a leap year || echo $1 is not a leap year __________________________ 64
Real-time systems and programming languages
Cs 421 programming languages and compilers
Real time example of multithreading in java
Programming languages levels
Introduction to programming languages
Plc programming languages
Procedural programming languages
Imperative programming languages
Alternative programming languages
Strongly typed vs weakly typed
Transmission programming languages
Adam doupe cse 340
Integral data type in c
Xenia programming languages
Advantages of high level language
Mainstream programming languages
Vineeth kashyap
Programming languages
Programming languages
Programming languages
Programming languages
Language
Brief history of programming languages
Taxonomy of programming languages
Real time programming language
Xkcd programming languages
If programming languages were cars
Reasons for studying concepts of programming languages
Cornell programming languages
Low level programming languages
Middle level programming languages
The art of programming
Cs 421 programming languages and compilers
Iat 265
Storage management in programming languages
From most important to least important in writing
From most important to least important in writing
Least important to most important
Perbedaan linear programming dan integer programming
Greedy vs dynamic programming
Runtime programming
Integer programming vs linear programming
Perbedaan linear programming dan integer programming
What represents a function
Which graph represents a function with direct variation
Characteristics of linear equations
Special functions algebra 2
Which graph represents a function with direct variation?
Which relation represents a function
Which of the following expressions represents a function
Nano programmed control unit
Concept of duality in linear programming
Adolescence example
Dating serves several important functions that include
Dating serves several important functions that include
Functions of the leaves
Types of functions in programming
Differentiate actual self and ideal self
Perbedaan pemasaran dan penjualan
Mitochondria function
Is the very important function in the radio telex system
Bind function in socket programming
What is a socket
Piecewise function absolute value
Evaluating functions