Function User defined function is a code segment
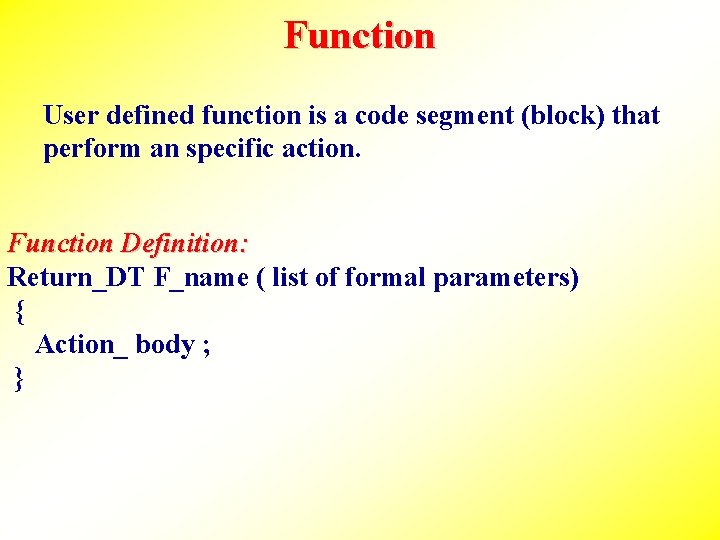
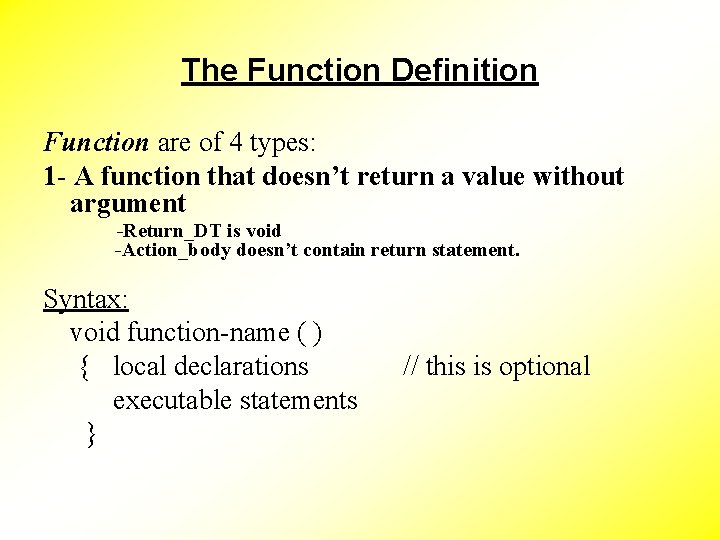
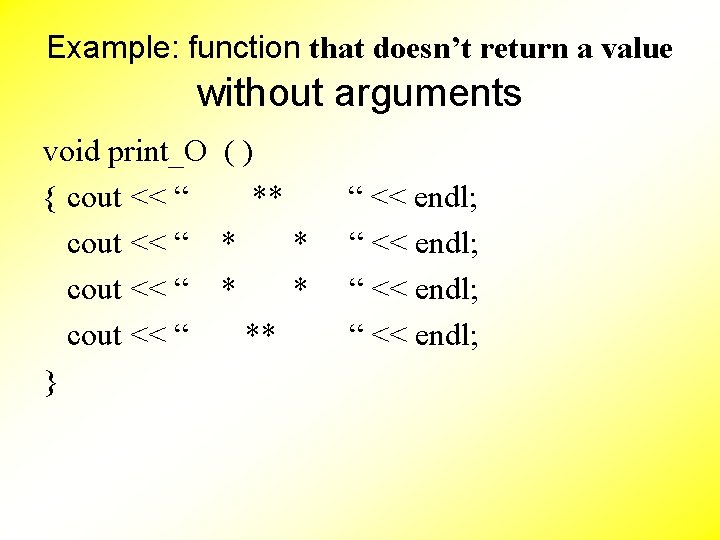
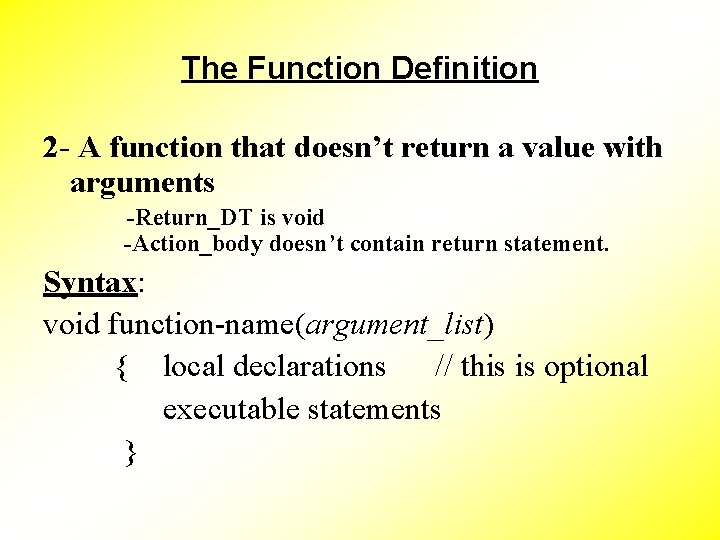
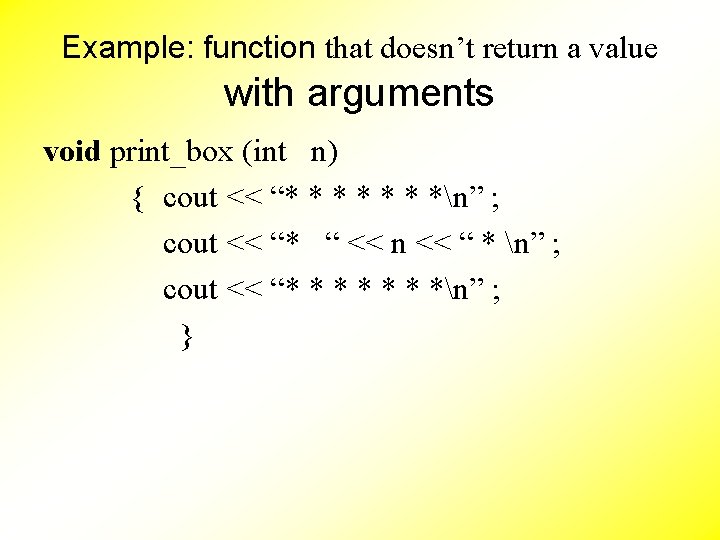
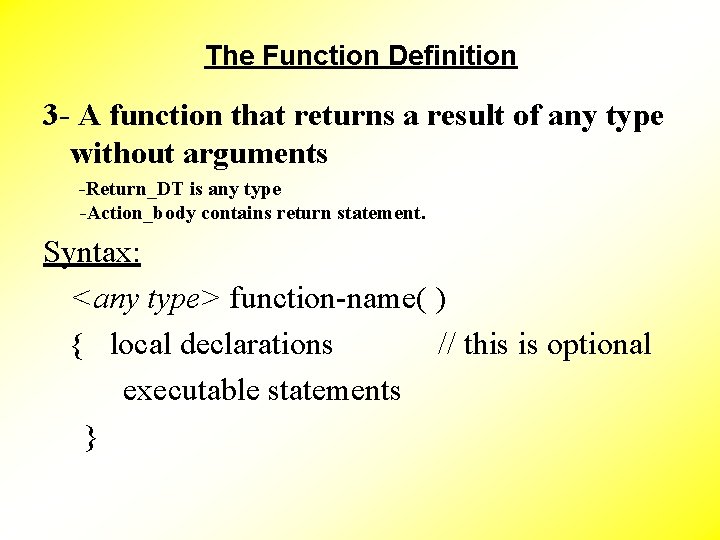
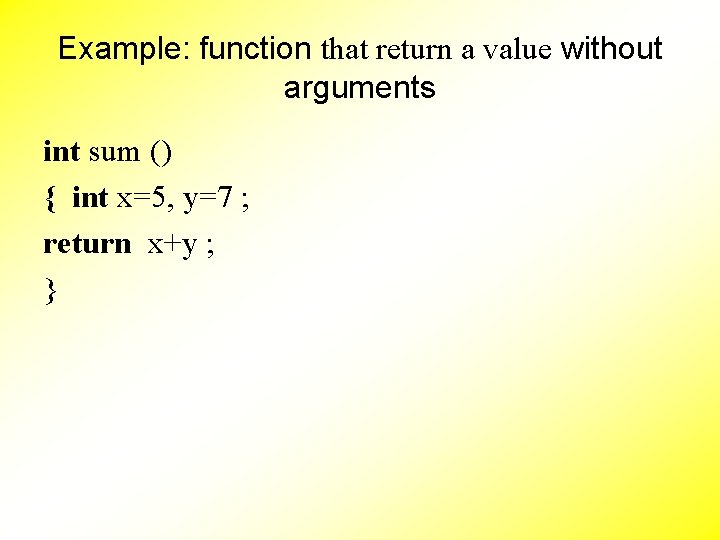
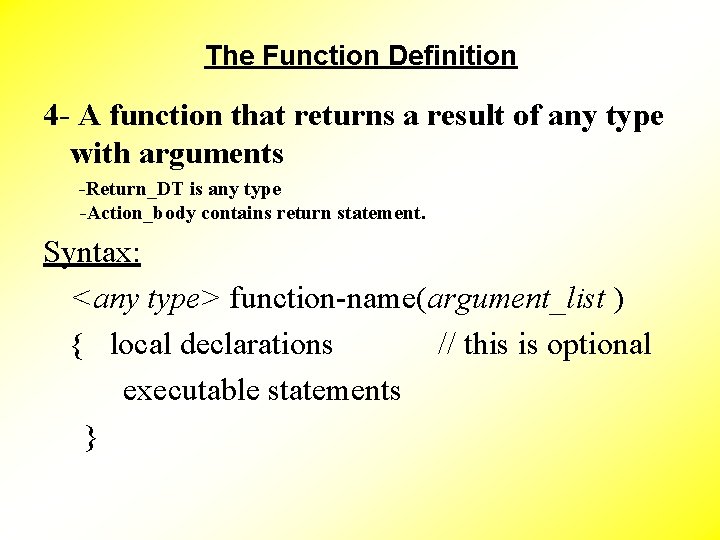
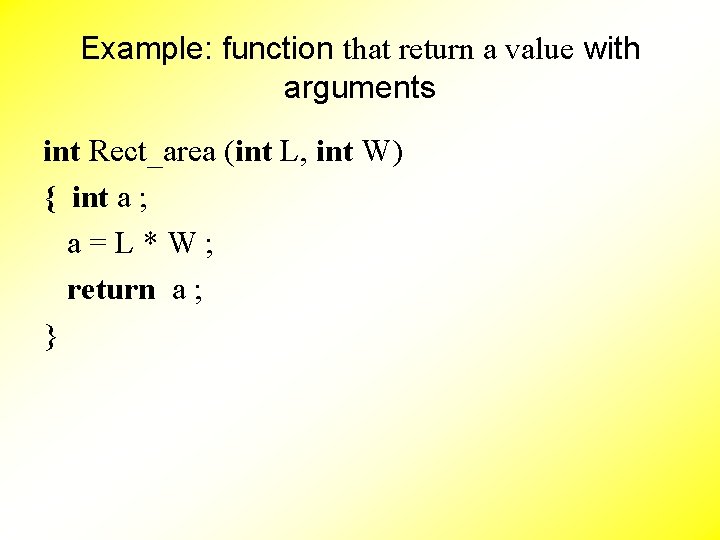
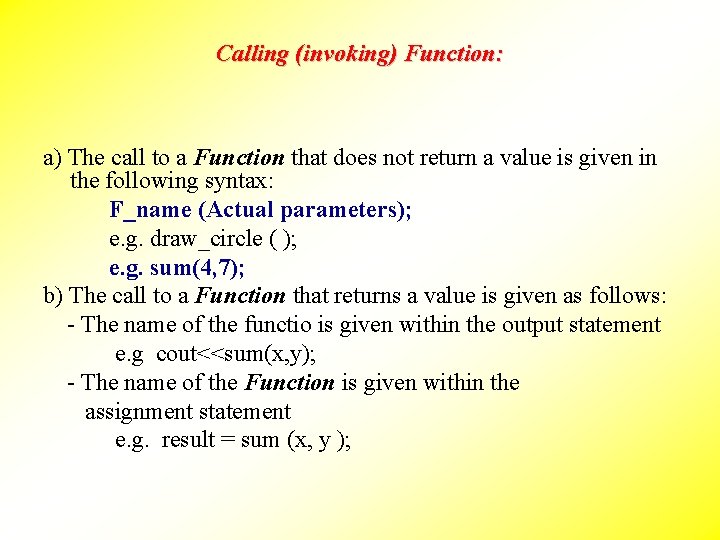
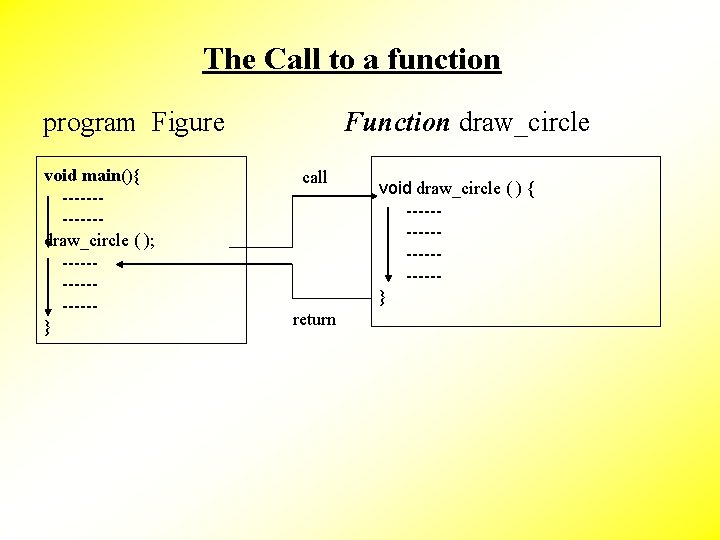
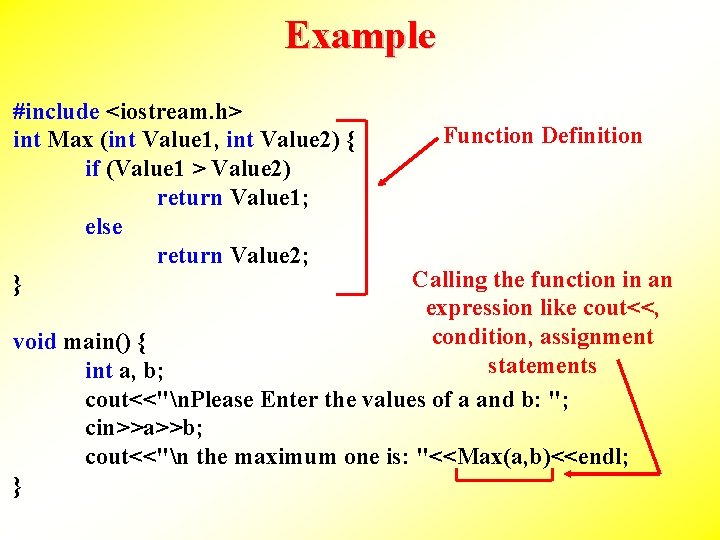
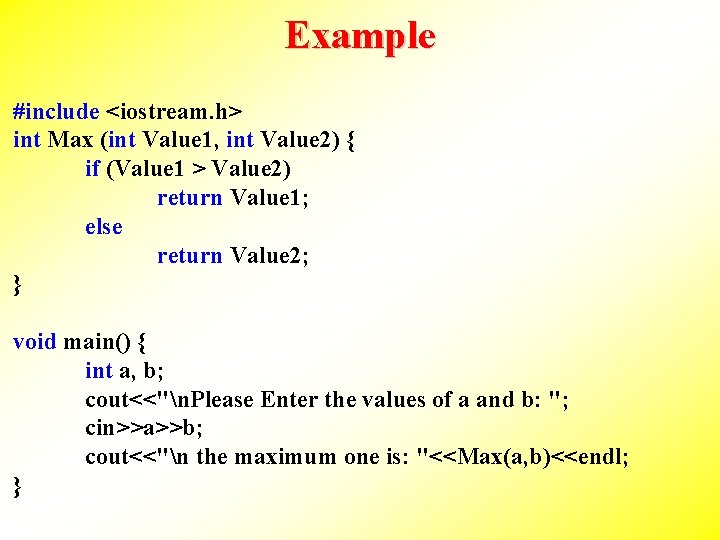
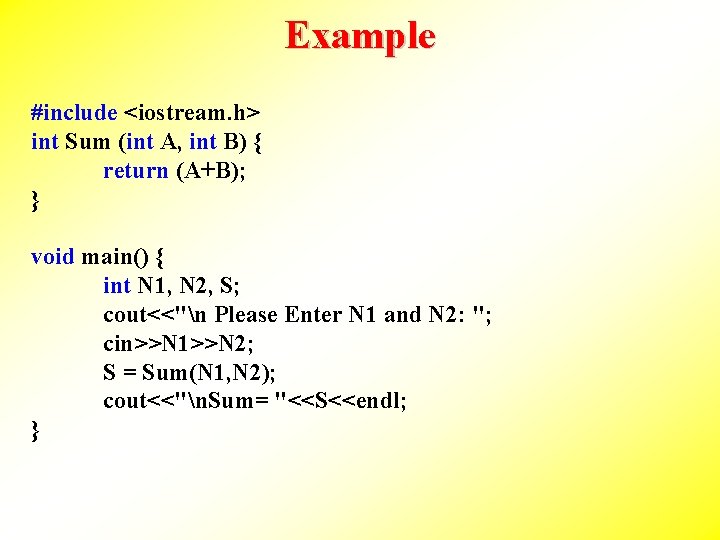
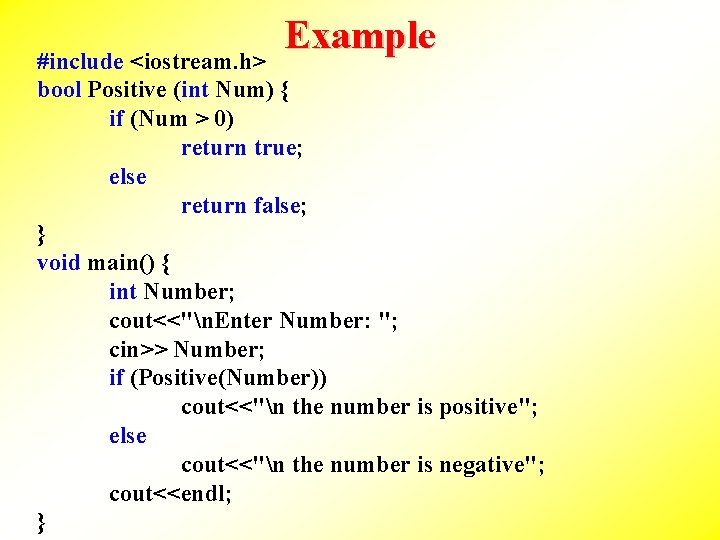
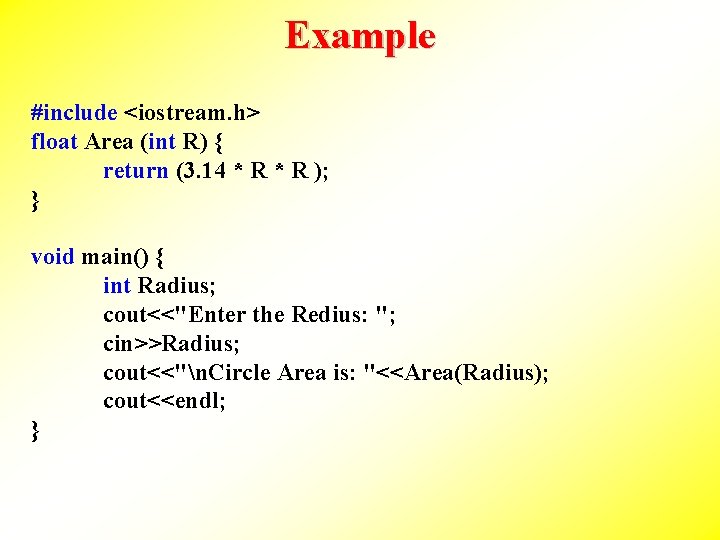
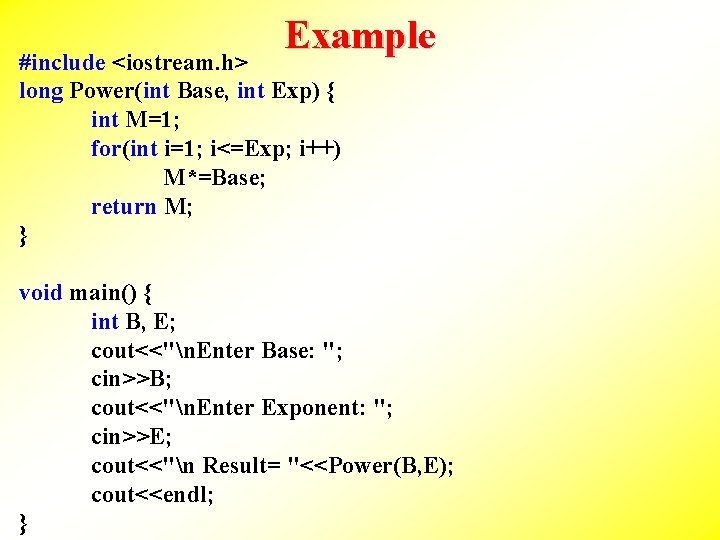
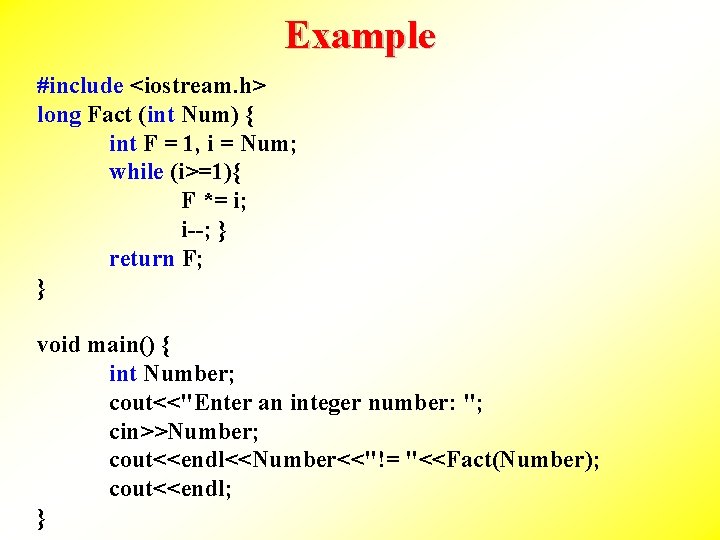
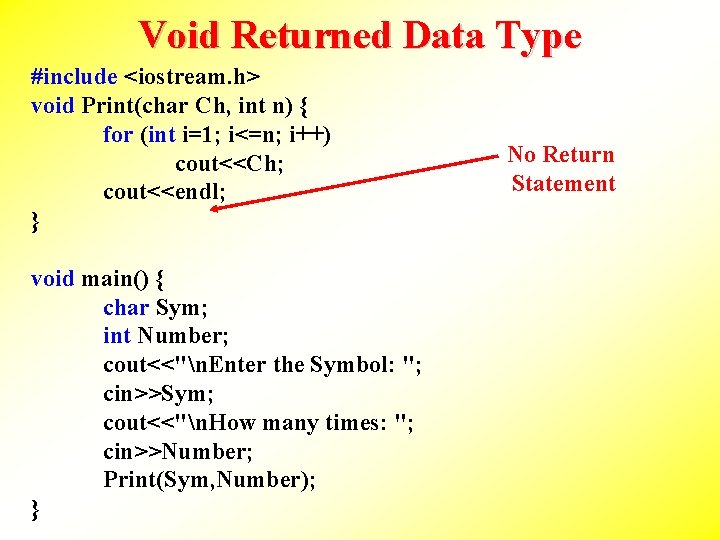
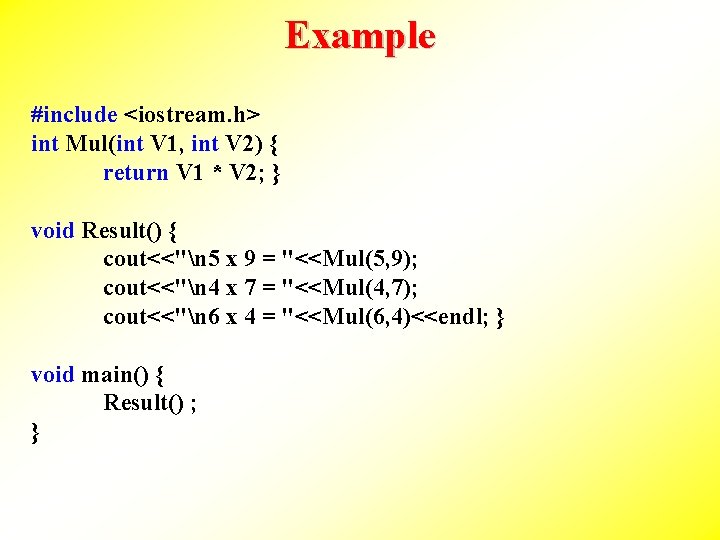
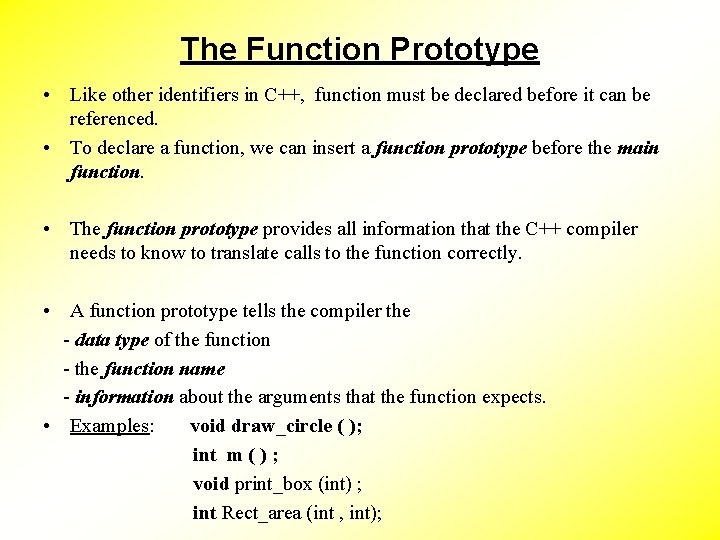
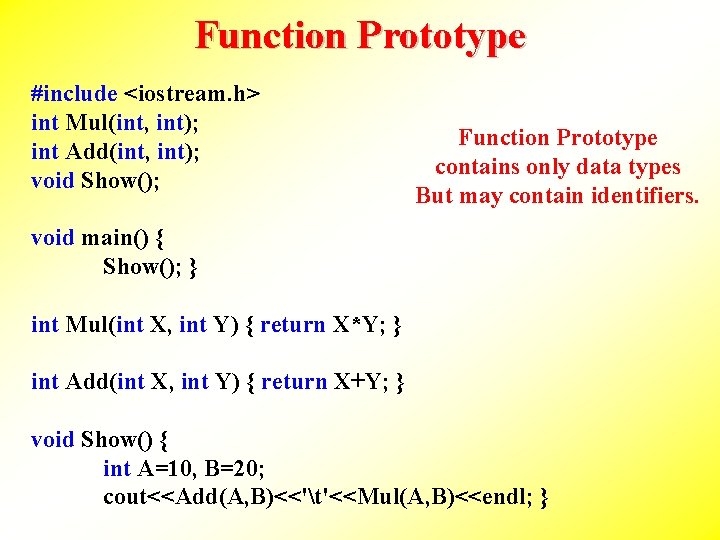
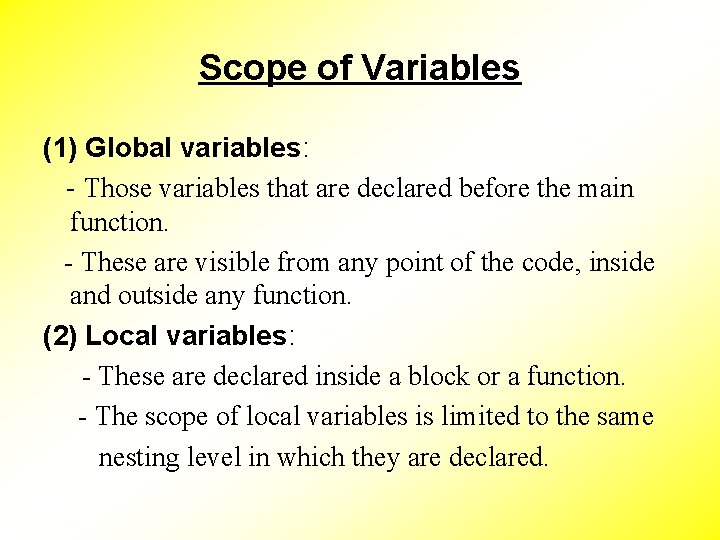
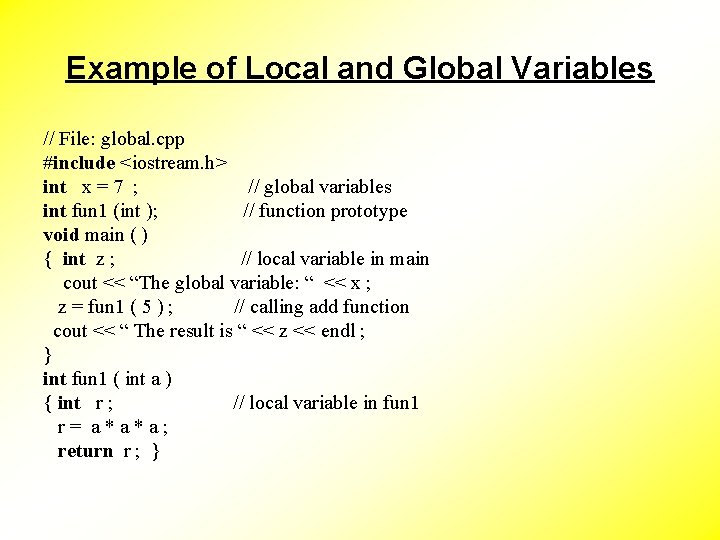
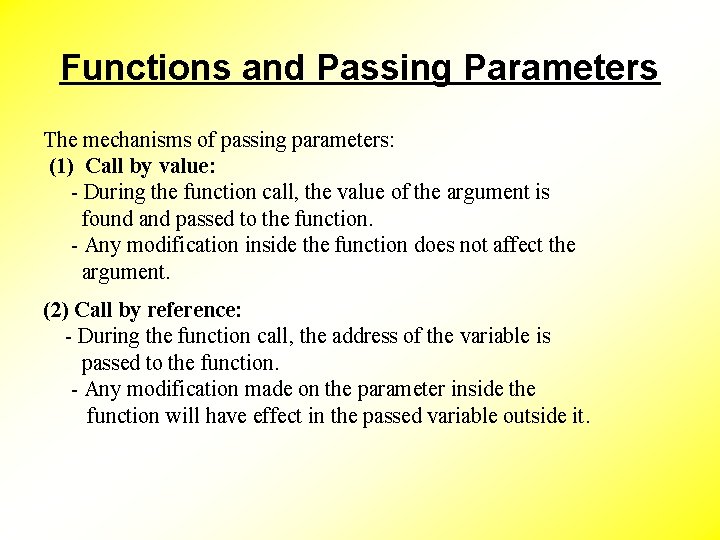
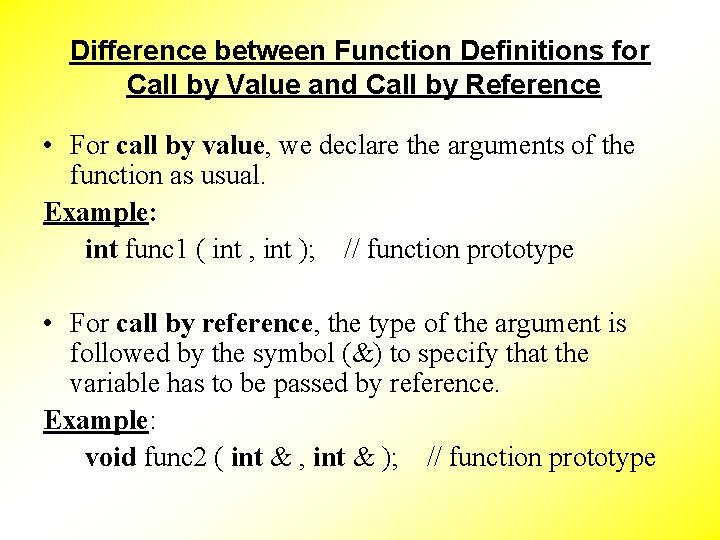
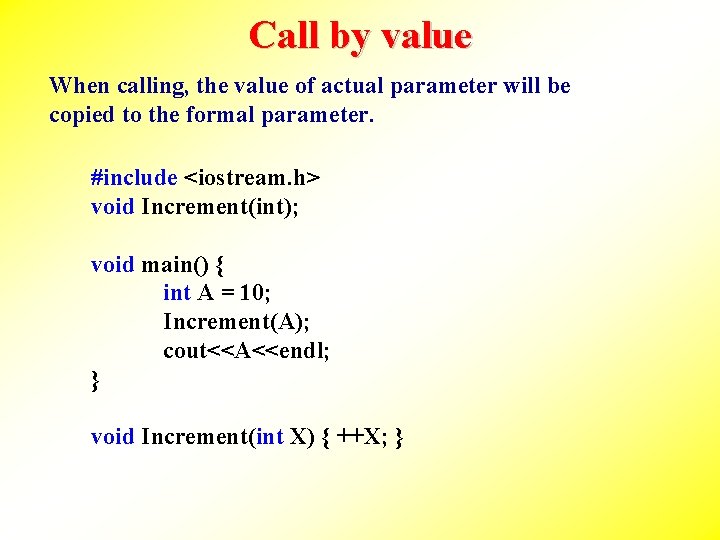
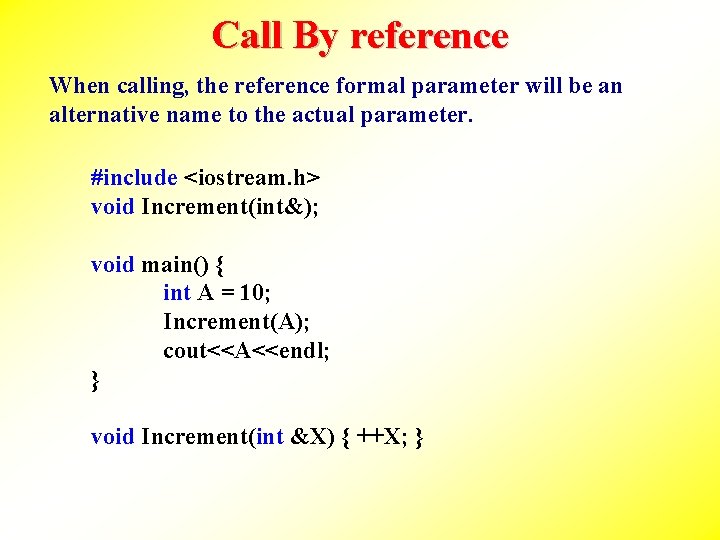
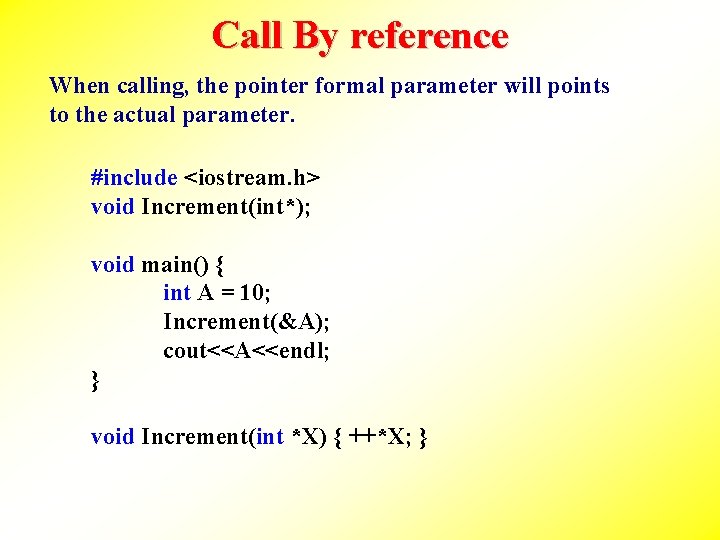
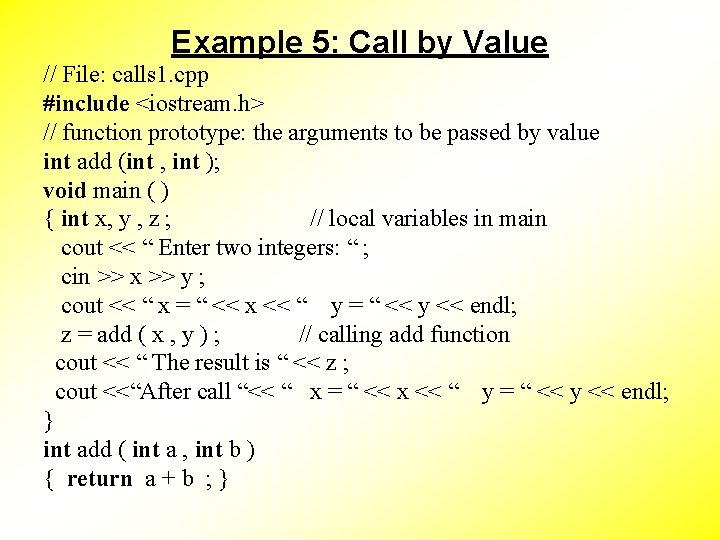
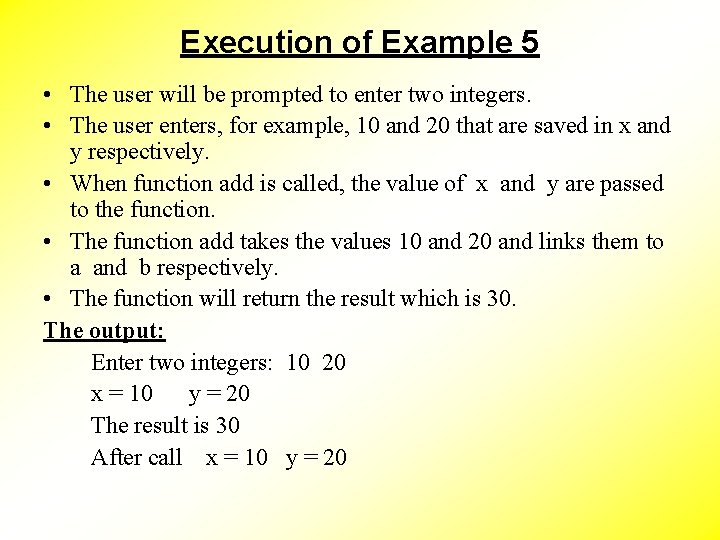
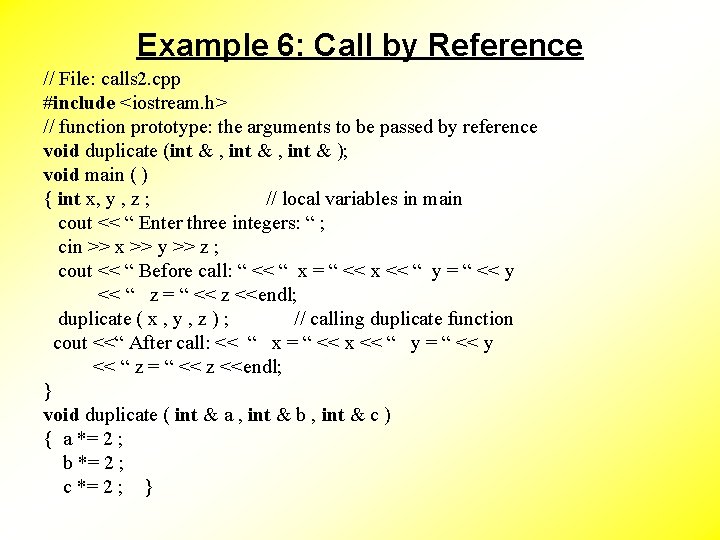
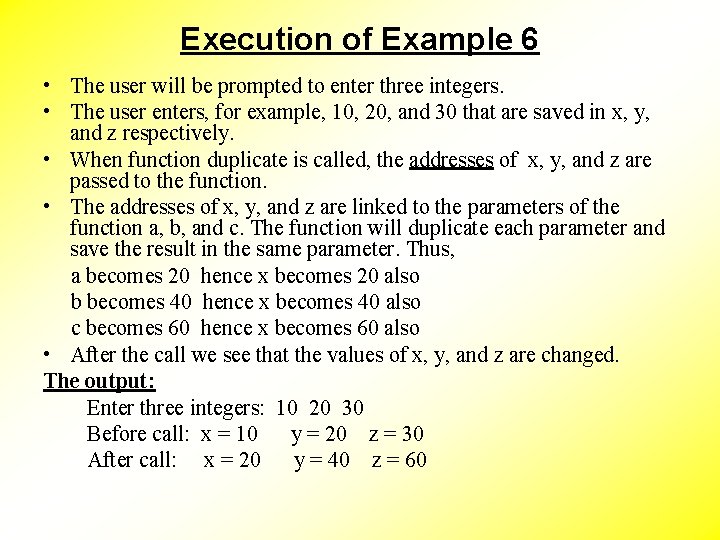
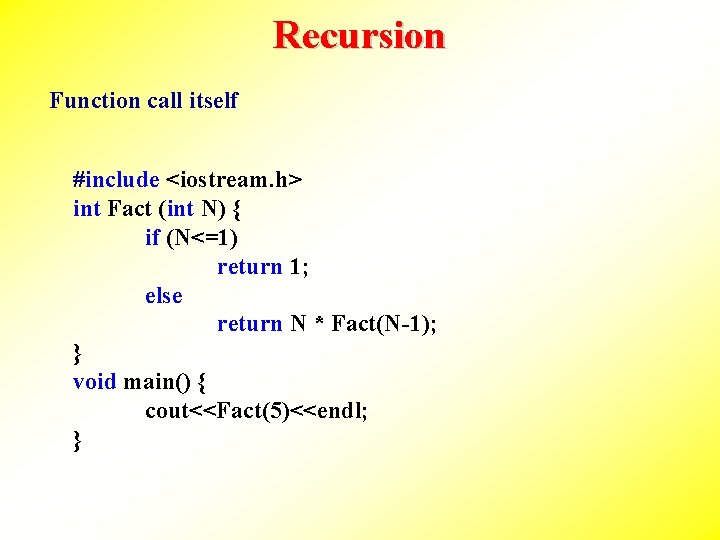
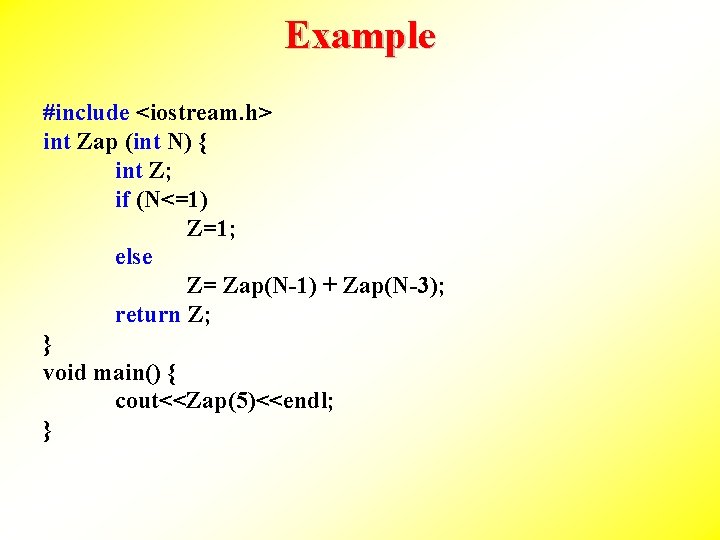
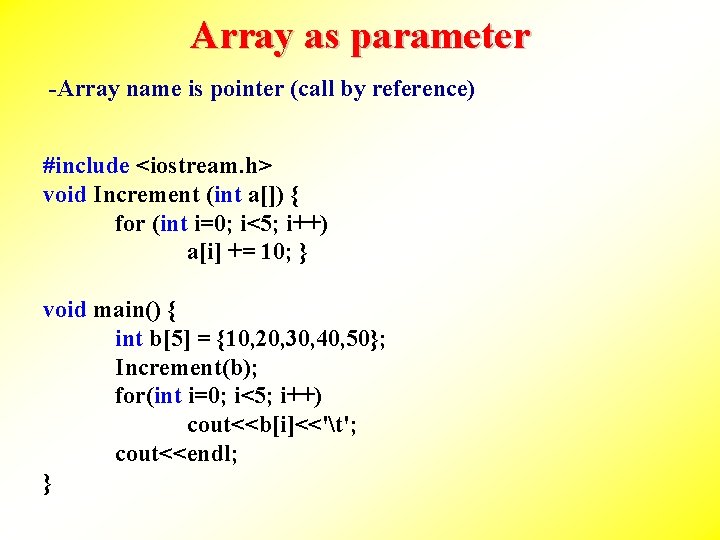
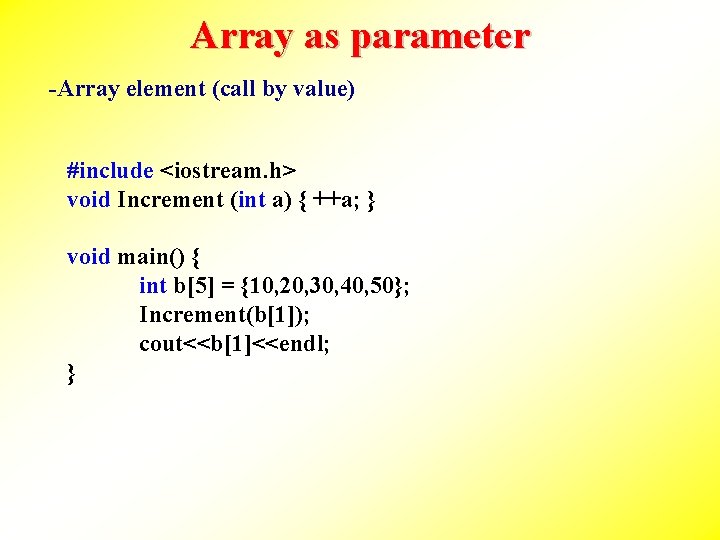
- Slides: 37
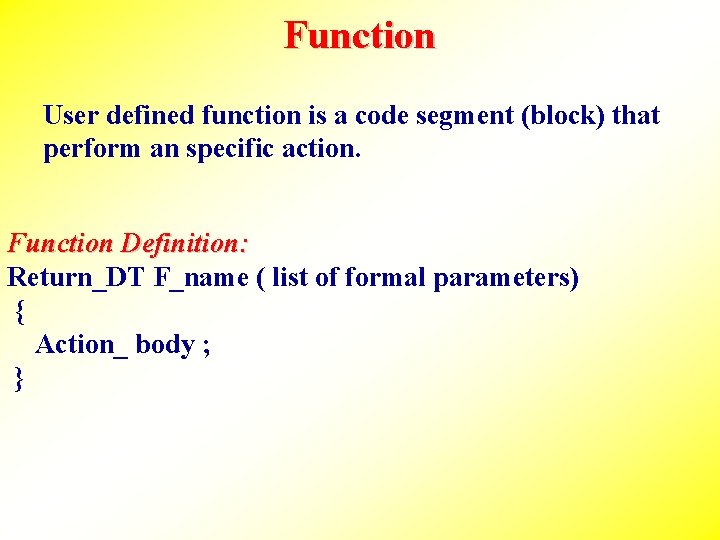
Function User defined function is a code segment (block) that perform an specific action. Function Definition: Return_DT F_name ( list of formal parameters) { Action_ body ; }
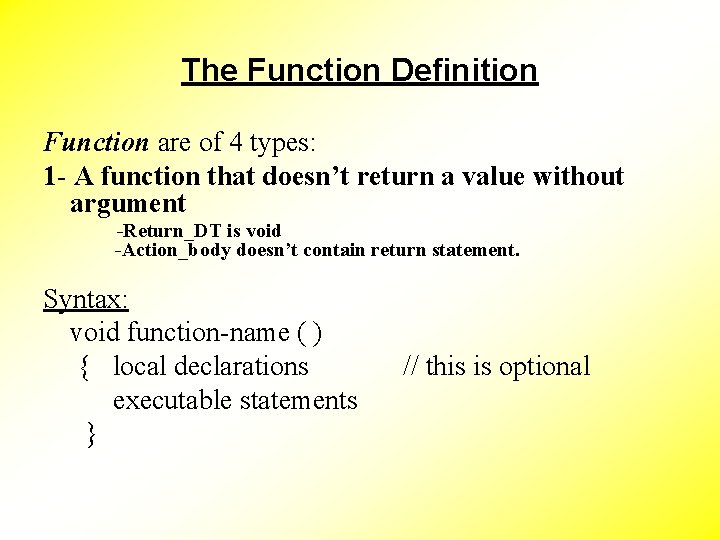
The Function Definition Function are of 4 types: 1 - A function that doesn’t return a value without argument -Return_DT is void -Action_body doesn’t contain return statement. Syntax: void function-name ( ) { local declarations executable statements } // this is optional
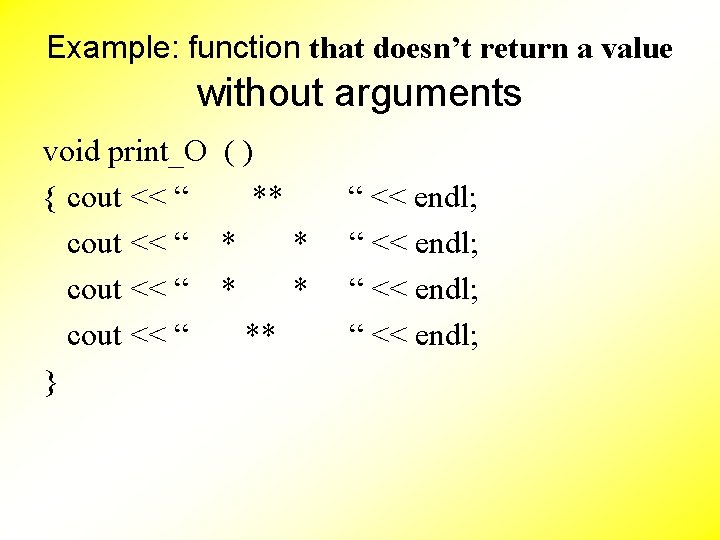
Example: function that doesn’t return a value without arguments void print_O ( ) { cout << “ ** cout << “ ** } “ << endl;
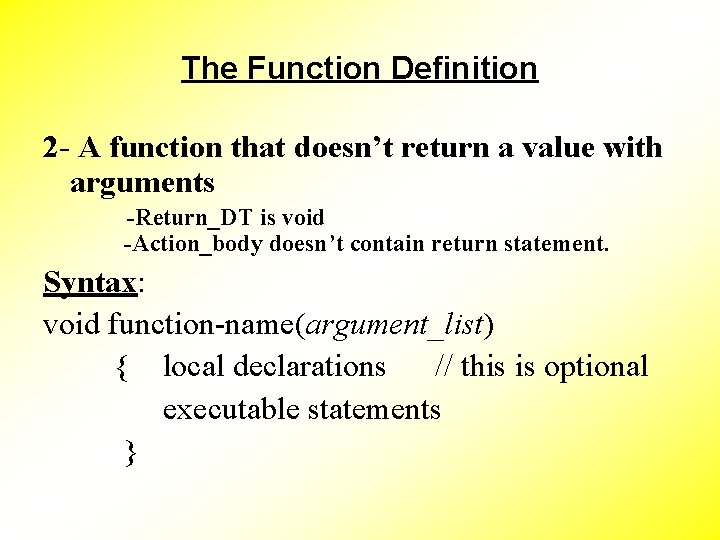
The Function Definition 2 - A function that doesn’t return a value with arguments -Return_DT is void -Action_body doesn’t contain return statement. Syntax: void function-name(argument_list) { local declarations // this is optional executable statements }
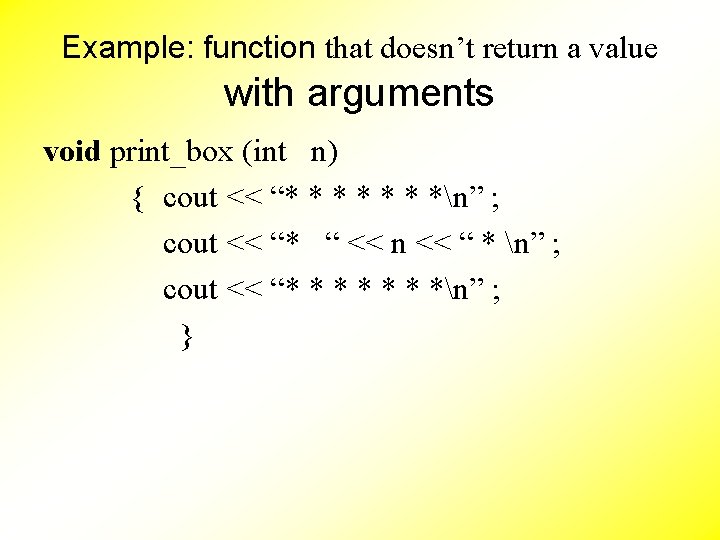
Example: function that doesn’t return a value with arguments void print_box (int n) { cout << “* * * *n” ; cout << “* “ << n << “ * n” ; cout << “* * * *n” ; }
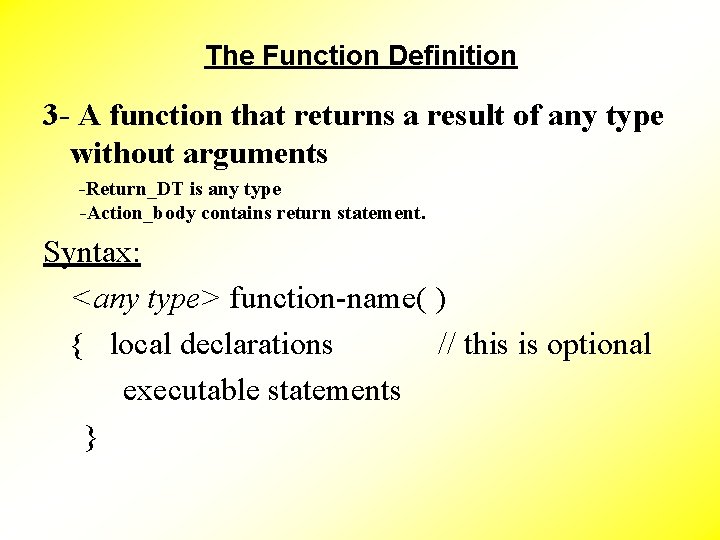
The Function Definition 3 - A function that returns a result of any type without arguments -Return_DT is any type -Action_body contains return statement. Syntax: <any type> function-name( ) { local declarations // this is optional executable statements }
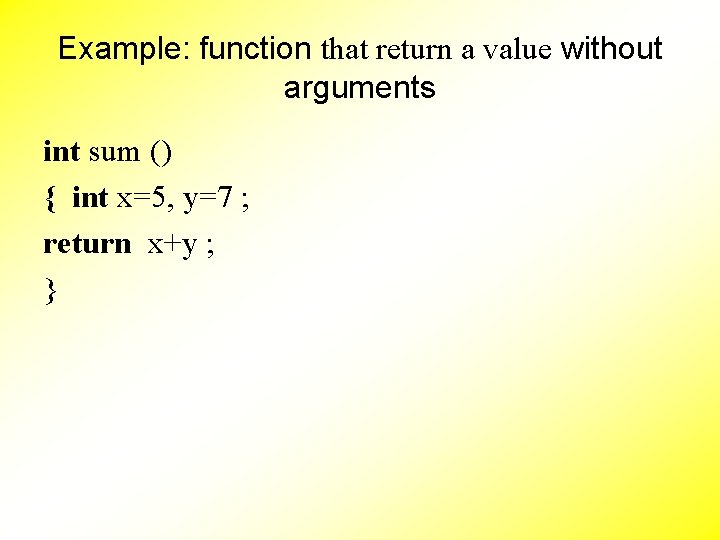
Example: function that return a value without arguments int sum () { int x=5, y=7 ; return x+y ; }
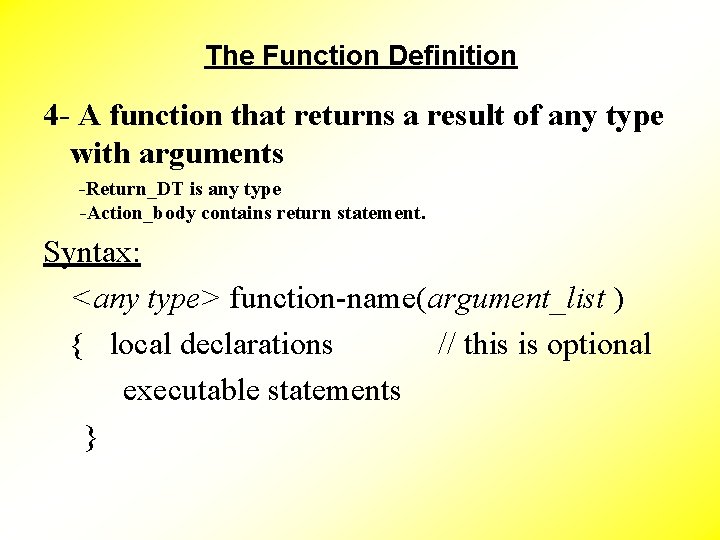
The Function Definition 4 - A function that returns a result of any type with arguments -Return_DT is any type -Action_body contains return statement. Syntax: <any type> function-name(argument_list ) { local declarations // this is optional executable statements }
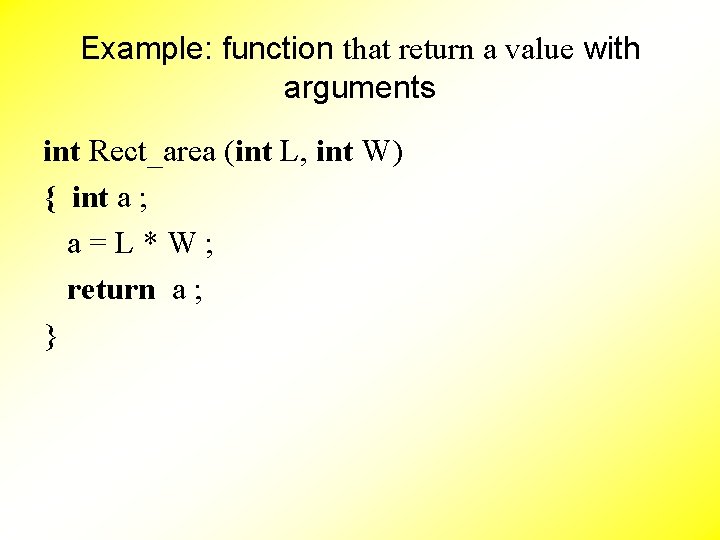
Example: function that return a value with arguments int Rect_area (int L, int W) { int a ; a=L*W; return a ; }
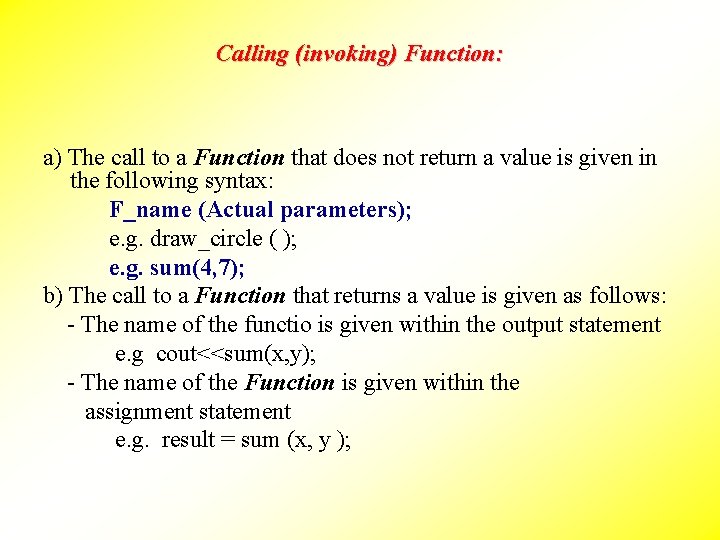
Calling (invoking) Function: a) The call to a Function that does not return a value is given in the following syntax: F_name (Actual parameters); e. g. draw_circle ( ); e. g. sum(4, 7); b) The call to a Function that returns a value is given as follows: - The name of the functio is given within the output statement e. g cout<<sum(x, y); - The name of the Function is given within the assignment statement e. g. result = sum (x, y );
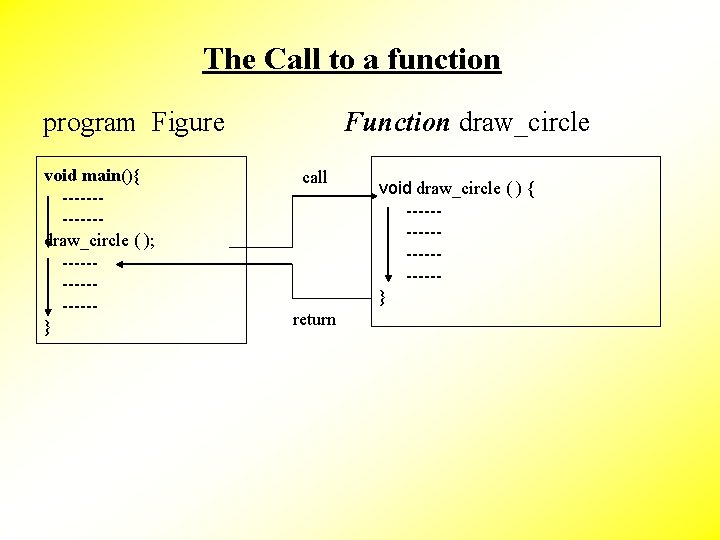
The Call to a function program Figure void main(){ ------draw_circle ( ); --------} Function draw_circle call return void draw_circle ( ) { ----------}
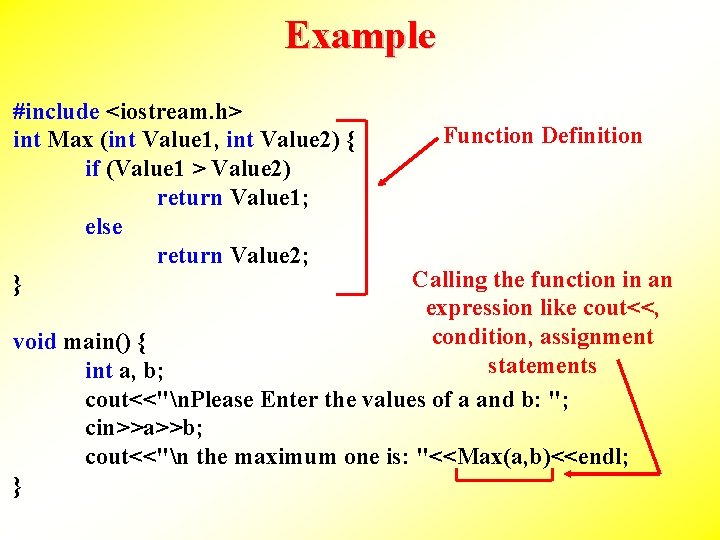
Example #include <iostream. h> int Max (int Value 1, int Value 2) { if (Value 1 > Value 2) return Value 1; else return Value 2; } Function Definition Calling the function in an expression like cout<<, condition, assignment void main() { statements int a, b; cout<<"n. Please Enter the values of a and b: "; cin>>a>>b; cout<<"n the maximum one is: "<<Max(a, b)<<endl; }
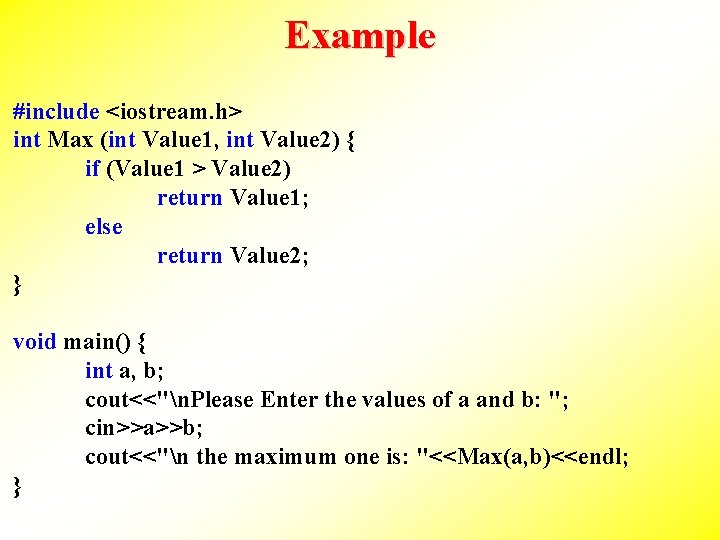
Example #include <iostream. h> int Max (int Value 1, int Value 2) { if (Value 1 > Value 2) return Value 1; else return Value 2; } void main() { int a, b; cout<<"n. Please Enter the values of a and b: "; cin>>a>>b; cout<<"n the maximum one is: "<<Max(a, b)<<endl; }
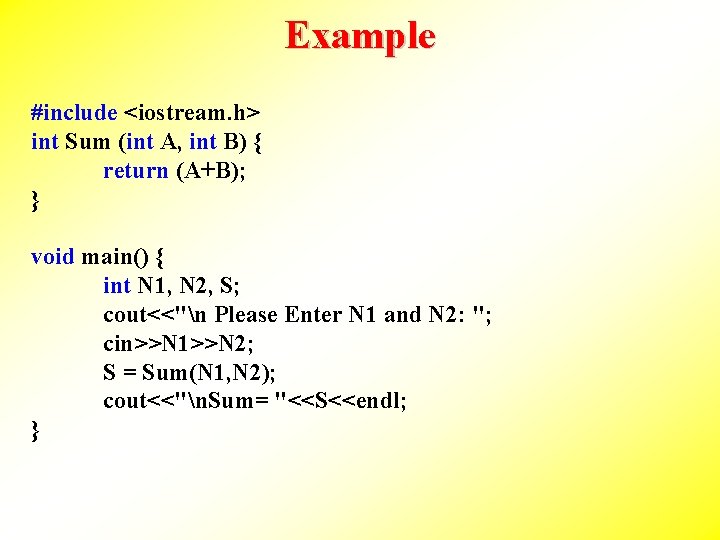
Example #include <iostream. h> int Sum (int A, int B) { return (A+B); } void main() { int N 1, N 2, S; cout<<"n Please Enter N 1 and N 2: "; cin>>N 1>>N 2; S = Sum(N 1, N 2); cout<<"n. Sum= "<<S<<endl; }
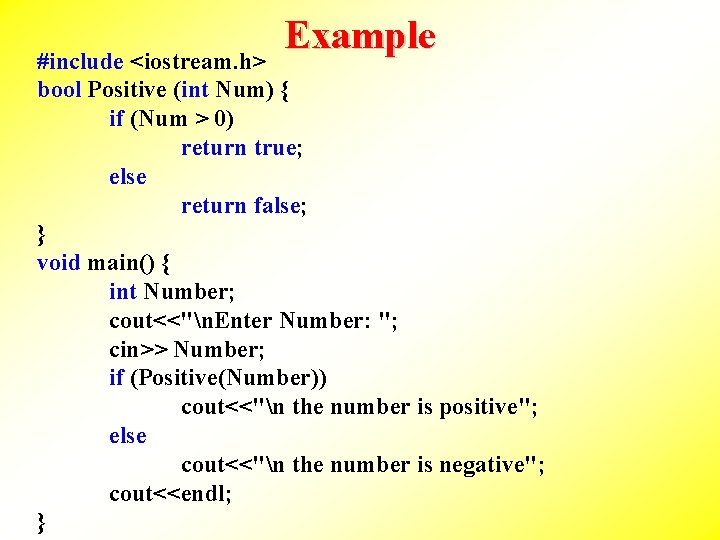
Example #include <iostream. h> bool Positive (int Num) { if (Num > 0) return true; else return false; } void main() { int Number; cout<<"n. Enter Number: "; cin>> Number; if (Positive(Number)) cout<<"n the number is positive"; else cout<<"n the number is negative"; cout<<endl; }
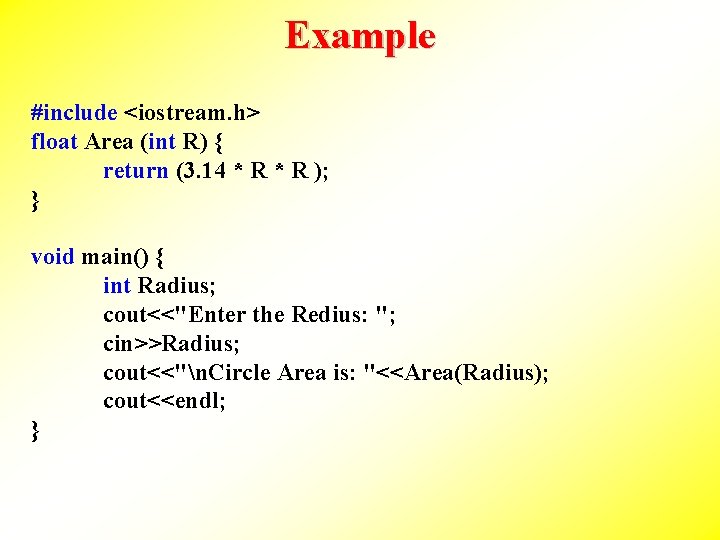
Example #include <iostream. h> float Area (int R) { return (3. 14 * R ); } void main() { int Radius; cout<<"Enter the Redius: "; cin>>Radius; cout<<"n. Circle Area is: "<<Area(Radius); cout<<endl; }
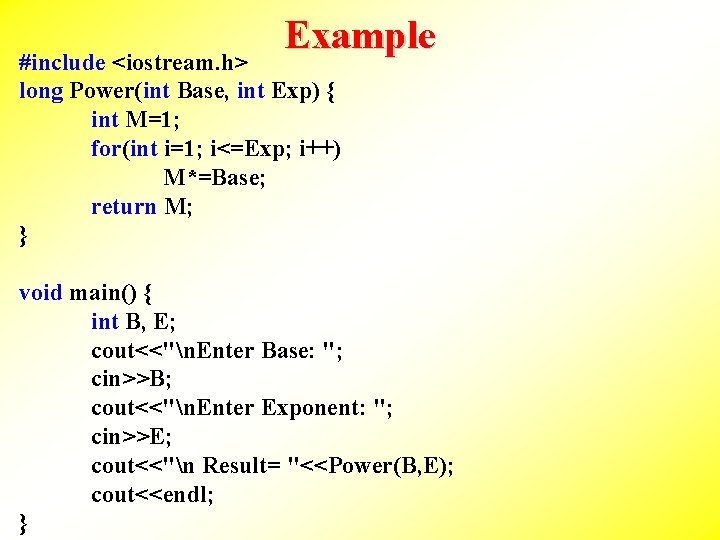
Example #include <iostream. h> long Power(int Base, int Exp) { int M=1; for(int i=1; i<=Exp; i++) M*=Base; return M; } void main() { int B, E; cout<<"n. Enter Base: "; cin>>B; cout<<"n. Enter Exponent: "; cin>>E; cout<<"n Result= "<<Power(B, E); cout<<endl; }
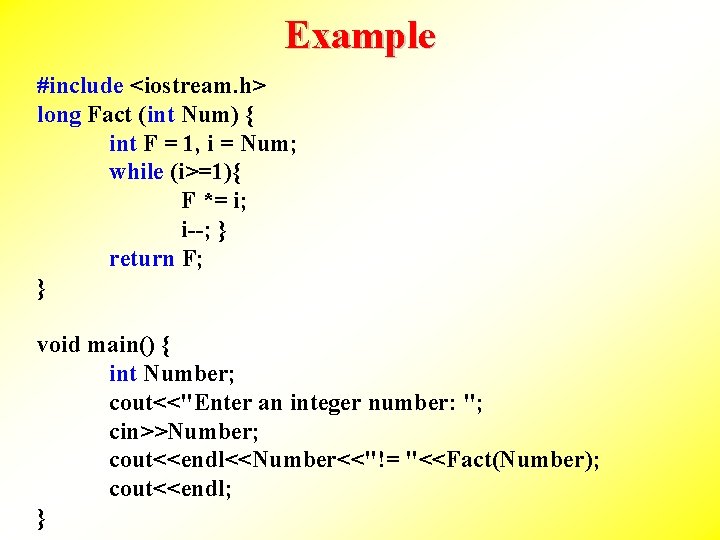
Example #include <iostream. h> long Fact (int Num) { int F = 1, i = Num; while (i>=1){ F *= i; i--; } return F; } void main() { int Number; cout<<"Enter an integer number: "; cin>>Number; cout<<endl<<Number<<"!= "<<Fact(Number); cout<<endl; }
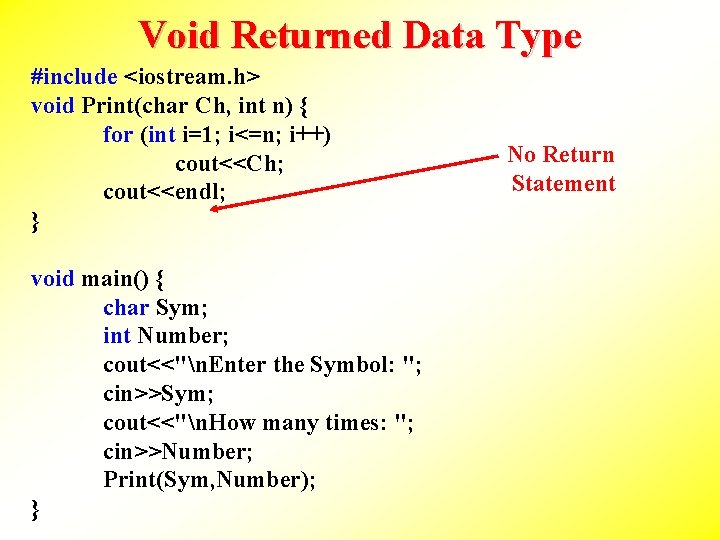
Void Returned Data Type #include <iostream. h> void Print(char Ch, int n) { for (int i=1; i<=n; i++) cout<<Ch; cout<<endl; } void main() { char Sym; int Number; cout<<"n. Enter the Symbol: "; cin>>Sym; cout<<"n. How many times: "; cin>>Number; Print(Sym, Number); } No Return Statement
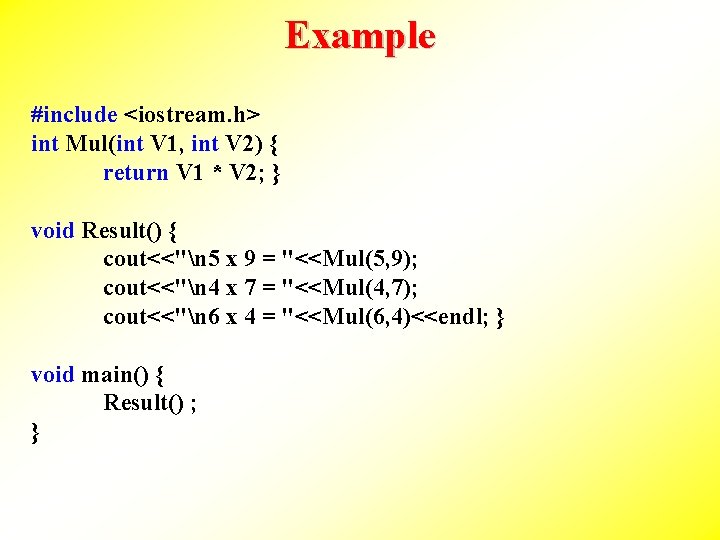
Example #include <iostream. h> int Mul(int V 1, int V 2) { return V 1 * V 2; } void Result() { cout<<"n 5 x 9 = "<<Mul(5, 9); cout<<"n 4 x 7 = "<<Mul(4, 7); cout<<"n 6 x 4 = "<<Mul(6, 4)<<endl; } void main() { Result() ; }
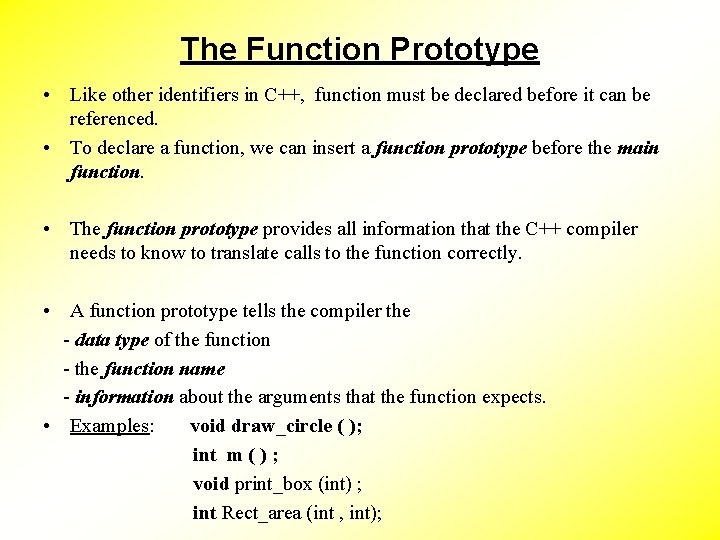
The Function Prototype • Like other identifiers in C++, function must be declared before it can be referenced. • To declare a function, we can insert a function prototype before the main function. • The function prototype provides all information that the C++ compiler needs to know to translate calls to the function correctly. • A function prototype tells the compiler the - data type of the function - the function name - information about the arguments that the function expects. • Examples: void draw_circle ( ); int m ( ) ; void print_box (int) ; int Rect_area (int , int);
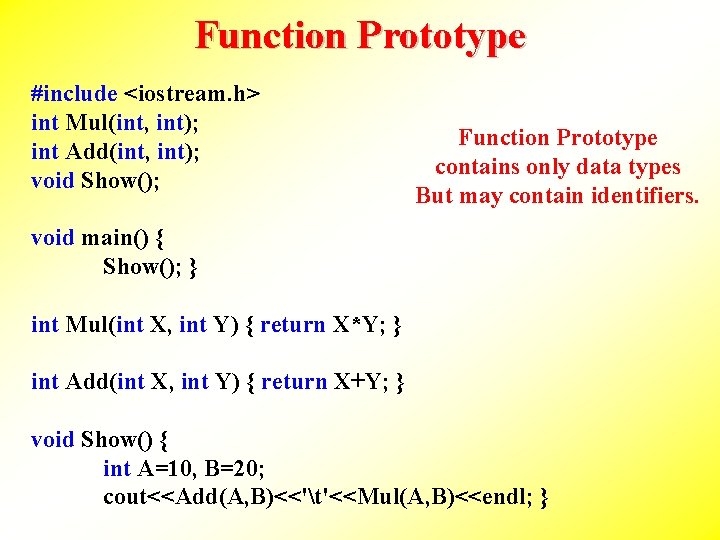
Function Prototype #include <iostream. h> int Mul(int, int); int Add(int, int); void Show(); Function Prototype contains only data types But may contain identifiers. void main() { Show(); } int Mul(int X, int Y) { return X*Y; } int Add(int X, int Y) { return X+Y; } void Show() { int A=10, B=20; cout<<Add(A, B)<<'t'<<Mul(A, B)<<endl; }
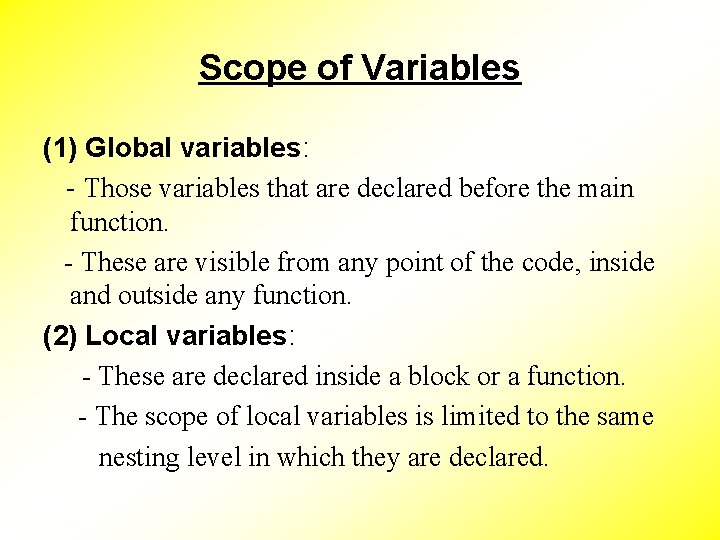
Scope of Variables (1) Global variables: - Those variables that are declared before the main function. - These are visible from any point of the code, inside and outside any function. (2) Local variables: - These are declared inside a block or a function. - The scope of local variables is limited to the same nesting level in which they are declared.
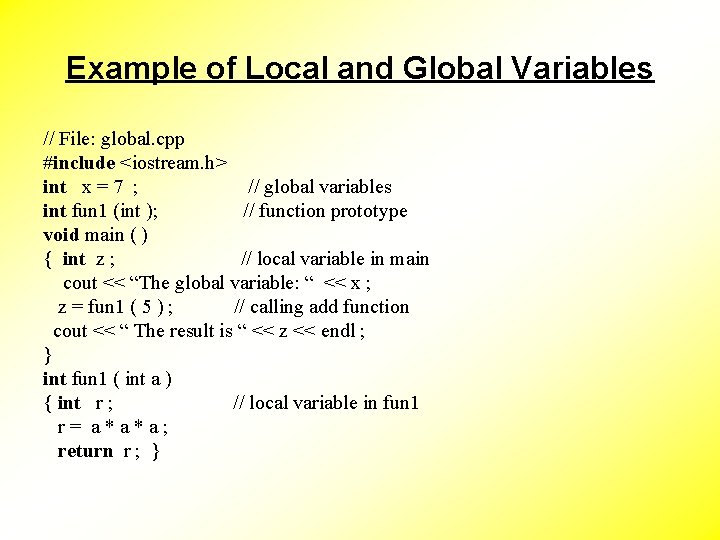
Example of Local and Global Variables // File: global. cpp #include <iostream. h> int x = 7 ; // global variables int fun 1 (int ); // function prototype void main ( ) { int z ; // local variable in main cout << “The global variable: “ << x ; z = fun 1 ( 5 ) ; // calling add function cout << “ The result is “ << z << endl ; } int fun 1 ( int a ) { int r ; // local variable in fun 1 r= a*a*a; return r ; }
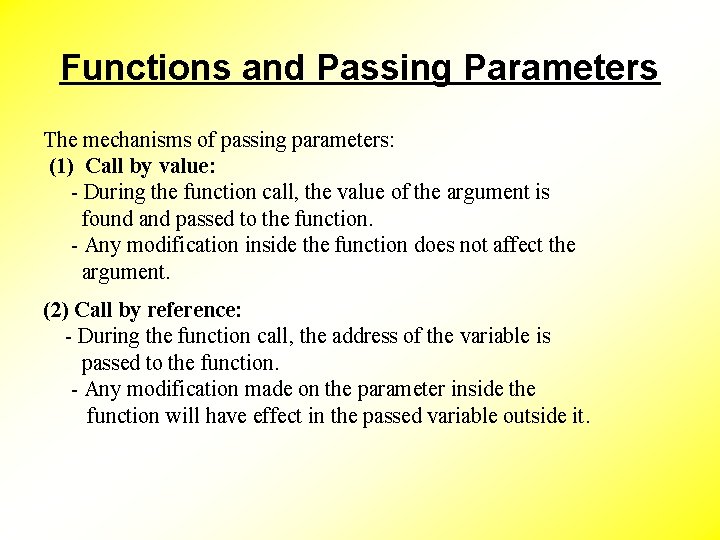
Functions and Passing Parameters The mechanisms of passing parameters: (1) Call by value: - During the function call, the value of the argument is found and passed to the function. - Any modification inside the function does not affect the argument. (2) Call by reference: - During the function call, the address of the variable is passed to the function. - Any modification made on the parameter inside the function will have effect in the passed variable outside it.
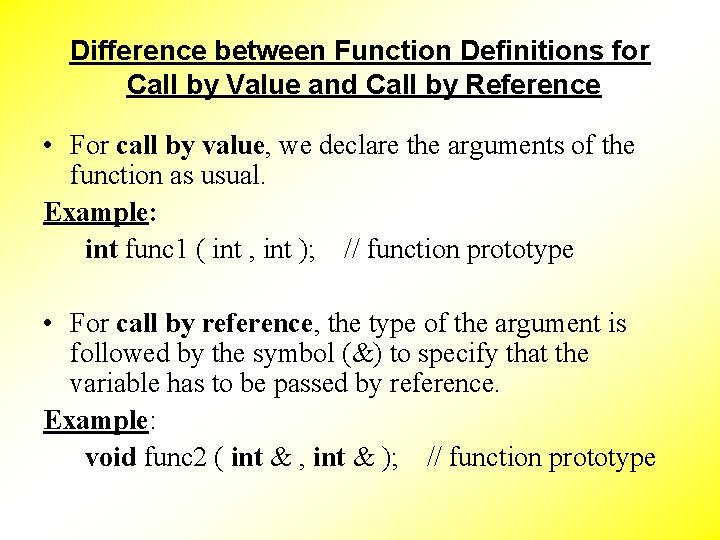
Difference between Function Definitions for Call by Value and Call by Reference • For call by value, we declare the arguments of the function as usual. Example: int func 1 ( int , int ); // function prototype • For call by reference, the type of the argument is followed by the symbol (&) to specify that the variable has to be passed by reference. Example: void func 2 ( int & , int & ); // function prototype
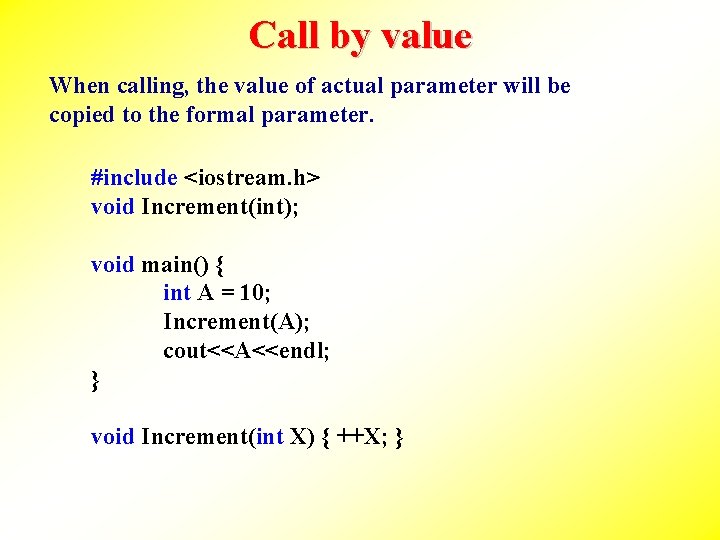
Call by value When calling, the value of actual parameter will be copied to the formal parameter. #include <iostream. h> void Increment(int); void main() { int A = 10; Increment(A); cout<<A<<endl; } void Increment(int X) { ++X; }
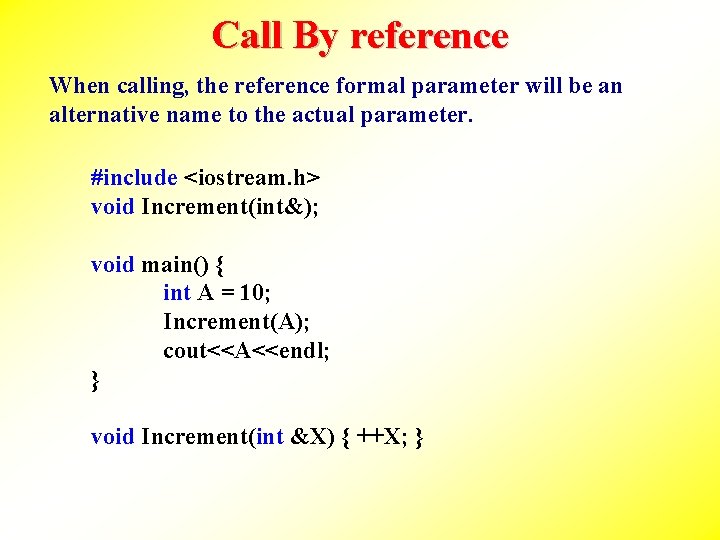
Call By reference When calling, the reference formal parameter will be an alternative name to the actual parameter. #include <iostream. h> void Increment(int&); void main() { int A = 10; Increment(A); cout<<A<<endl; } void Increment(int &X) { ++X; }
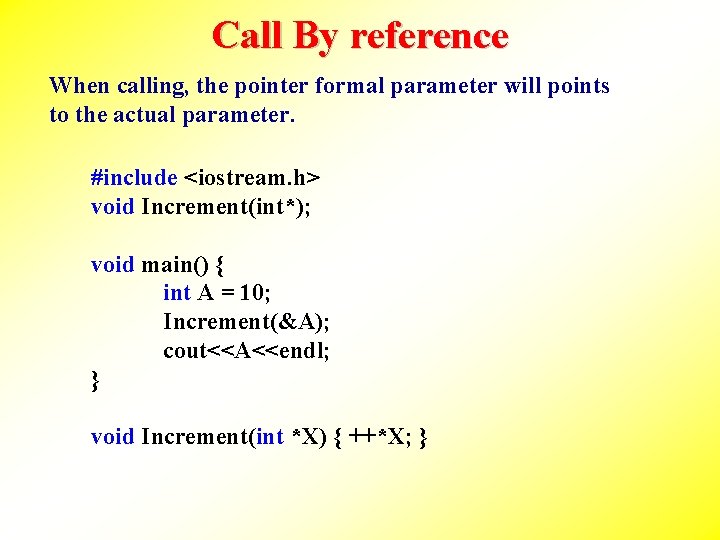
Call By reference When calling, the pointer formal parameter will points to the actual parameter. #include <iostream. h> void Increment(int*); void main() { int A = 10; Increment(&A); cout<<A<<endl; } void Increment(int *X) { ++*X; }
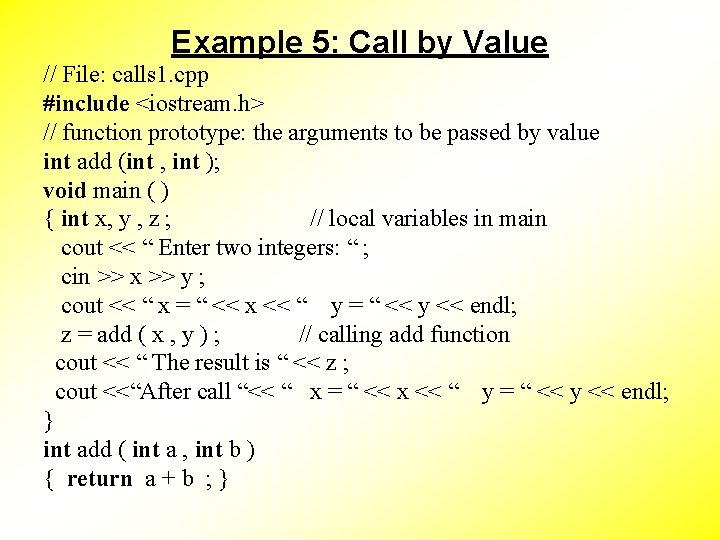
Example 5: Call by Value // File: calls 1. cpp #include <iostream. h> // function prototype: the arguments to be passed by value int add (int , int ); void main ( ) { int x, y , z ; // local variables in main cout << “ Enter two integers: “ ; cin >> x >> y ; cout << “ x = “ << x << “ y = “ << y << endl; z = add ( x , y ) ; // calling add function cout << “ The result is “ << z ; cout <<“After call “<< “ x = “ << x << “ y = “ << y << endl; } int add ( int a , int b ) { return a + b ; }
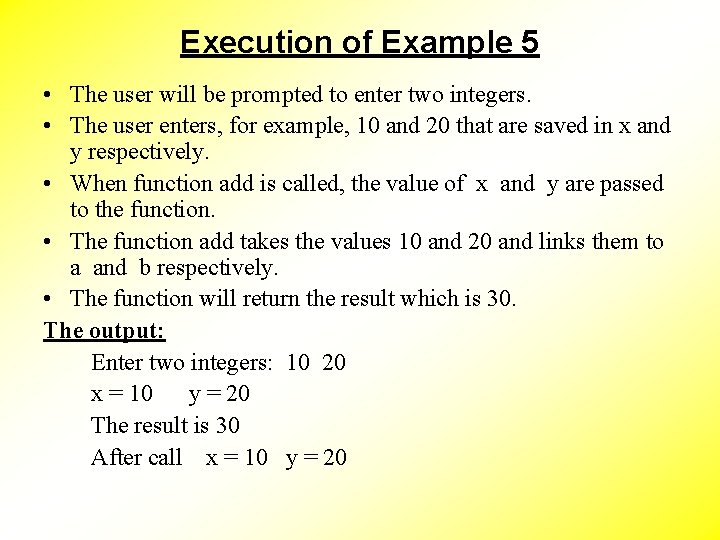
Execution of Example 5 • The user will be prompted to enter two integers. • The user enters, for example, 10 and 20 that are saved in x and y respectively. • When function add is called, the value of x and y are passed to the function. • The function add takes the values 10 and 20 and links them to a and b respectively. • The function will return the result which is 30. The output: Enter two integers: 10 20 x = 10 y = 20 The result is 30 After call x = 10 y = 20
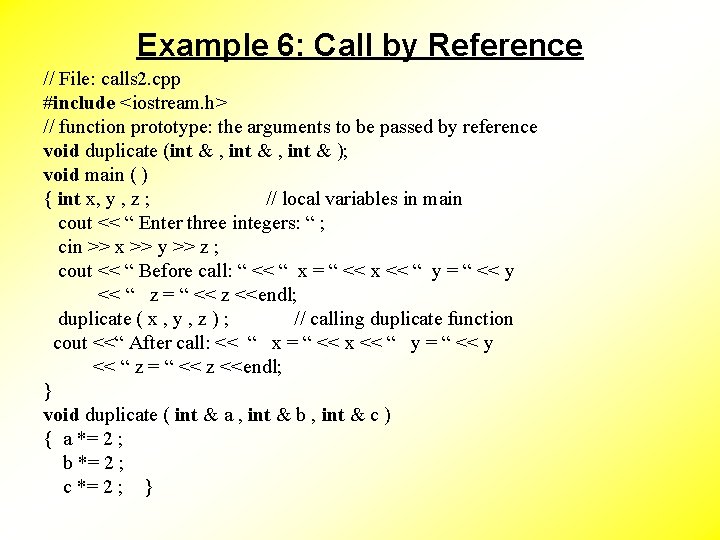
Example 6: Call by Reference // File: calls 2. cpp #include <iostream. h> // function prototype: the arguments to be passed by reference void duplicate (int & , int & ); void main ( ) { int x, y , z ; // local variables in main cout << “ Enter three integers: “ ; cin >> x >> y >> z ; cout << “ Before call: “ << “ x = “ << x << “ y = “ << y << “ z = “ << z <<endl; duplicate ( x , y , z ) ; // calling duplicate function cout <<“ After call: << “ x = “ << x << “ y = “ << y << “ z = “ << z <<endl; } void duplicate ( int & a , int & b , int & c ) { a *= 2 ; b *= 2 ; c *= 2 ; }
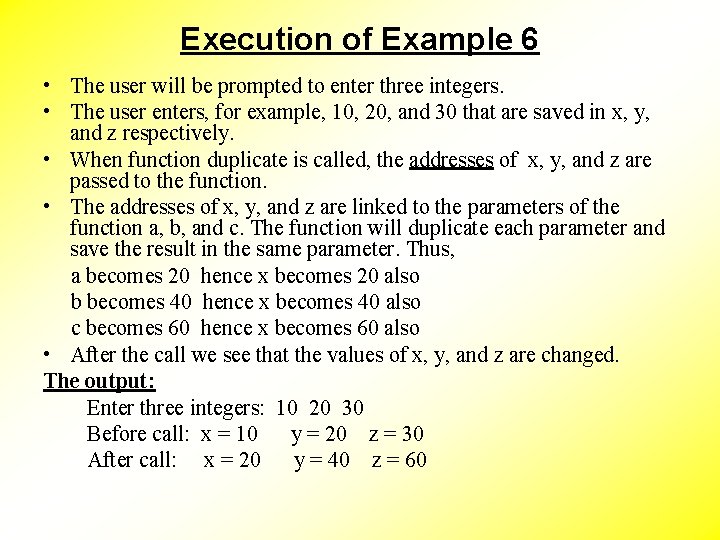
Execution of Example 6 • The user will be prompted to enter three integers. • The user enters, for example, 10, 20, and 30 that are saved in x, y, and z respectively. • When function duplicate is called, the addresses of x, y, and z are passed to the function. • The addresses of x, y, and z are linked to the parameters of the function a, b, and c. The function will duplicate each parameter and save the result in the same parameter. Thus, a becomes 20 hence x becomes 20 also b becomes 40 hence x becomes 40 also c becomes 60 hence x becomes 60 also • After the call we see that the values of x, y, and z are changed. The output: Enter three integers: 10 20 30 Before call: x = 10 y = 20 z = 30 After call: x = 20 y = 40 z = 60
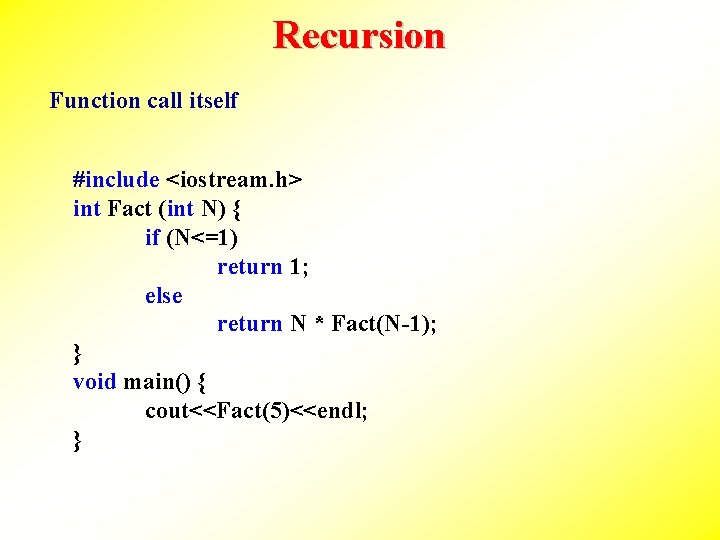
Recursion Function call itself #include <iostream. h> int Fact (int N) { if (N<=1) return 1; else return N * Fact(N-1); } void main() { cout<<Fact(5)<<endl; }
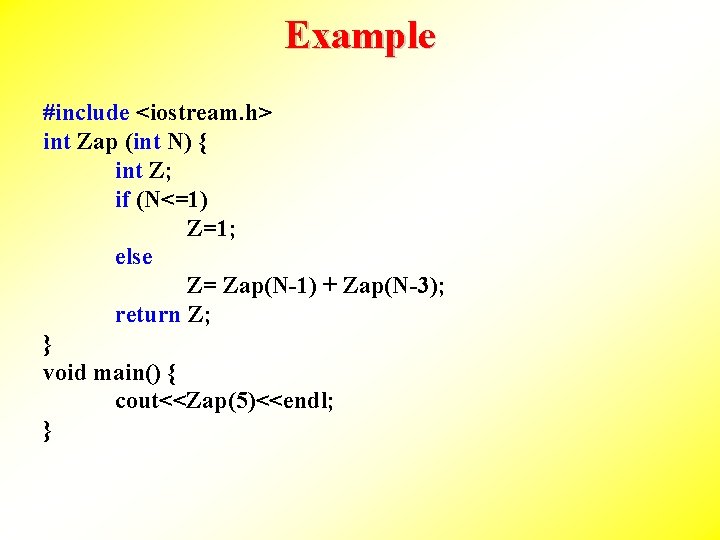
Example #include <iostream. h> int Zap (int N) { int Z; if (N<=1) Z=1; else Z= Zap(N-1) + Zap(N-3); return Z; } void main() { cout<<Zap(5)<<endl; }
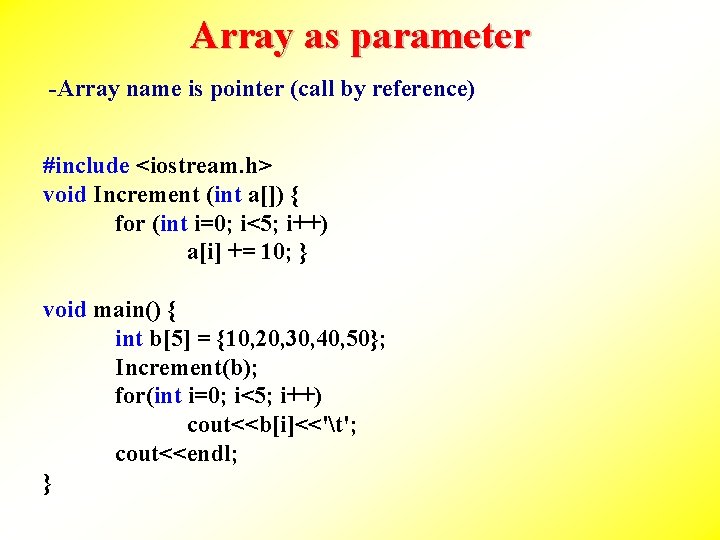
Array as parameter -Array name is pointer (call by reference) #include <iostream. h> void Increment (int a[]) { for (int i=0; i<5; i++) a[i] += 10; } void main() { int b[5] = {10, 20, 30, 40, 50}; Increment(b); for(int i=0; i<5; i++) cout<<b[i]<<'t'; cout<<endl; }
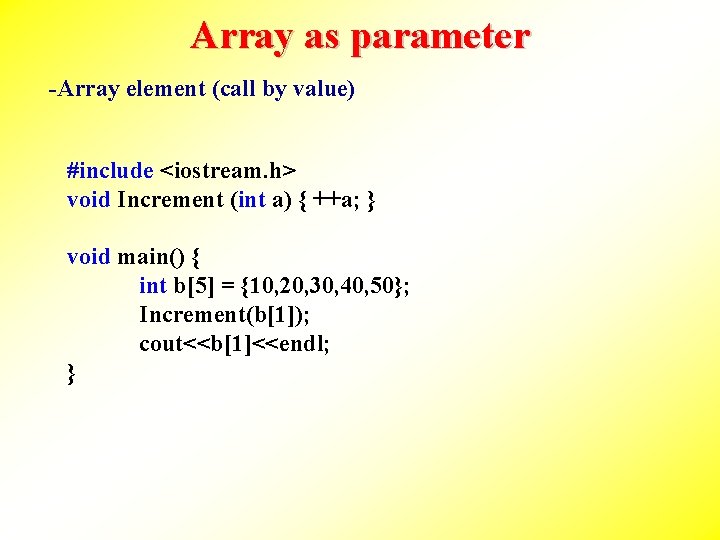
Array as parameter -Array element (call by value) #include <iostream. h> void Increment (int a) { ++a; } void main() { int b[5] = {10, 20, 30, 40, 50}; Increment(b[1]); cout<<b[1]<<endl; }
User-defined functions matlab
Circumferencce
Ansys fluent user defined function
A collection of well defined objects
Segment by segment invasion
Arsitektur komunikasi satelit
Sql user defined table
Java user defined methods
Tipe data user defined
User-defined functions
Busceral
Control segment of gps
Single user and multi user operating system
Multi user operating system
7 segment display vhdl
Proc far
Segment code
Code segment
Parity generator
Piecewise functions in real life situations
Nesting of member function in c++
Define piecewise function
Description of exponential function
Discrete numeric functions
What is function of a user diagram
What is gui
Code élaboré code restreint
Managed and unmanaged code
Object code vs assembly code
Difference between source code and machine code
Code switching and code mixing
Trace the code genetic code table
Hash separate chaining
Separate chaining hash table
What is work in physics
Finance can be defined as
Mandoline definition
Web usability definition