User defined data types Classes Classes Programmer defined
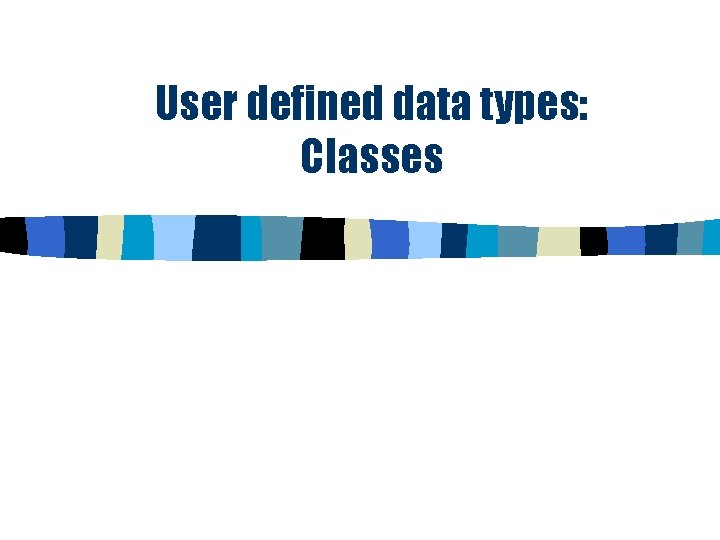
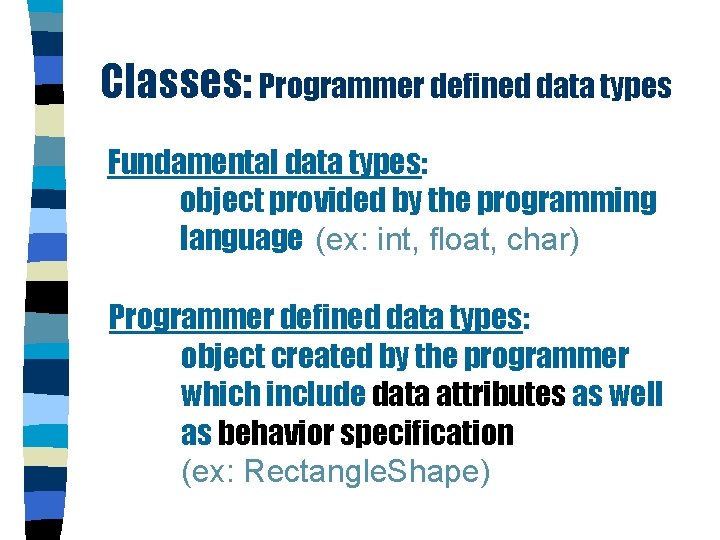
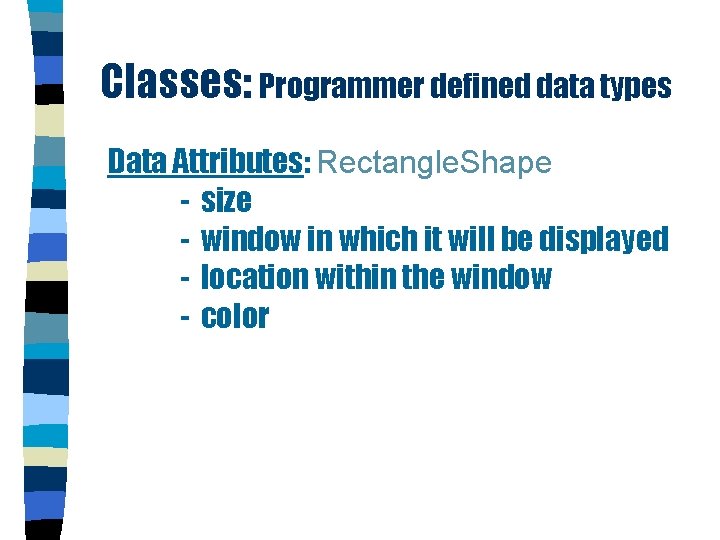
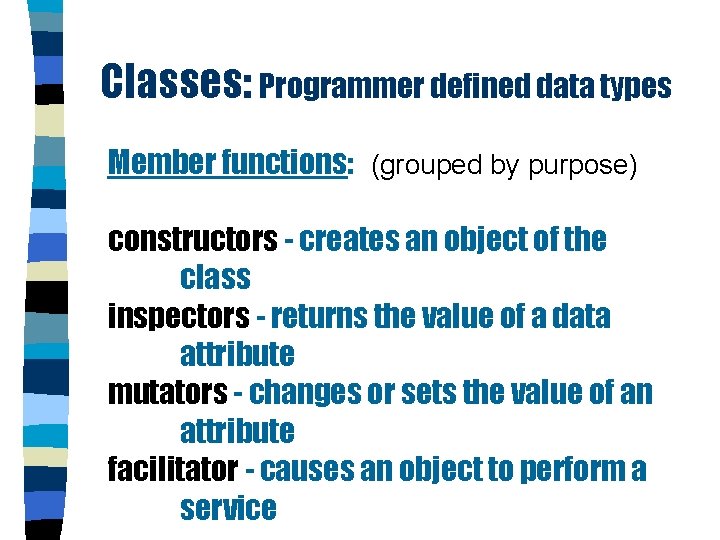
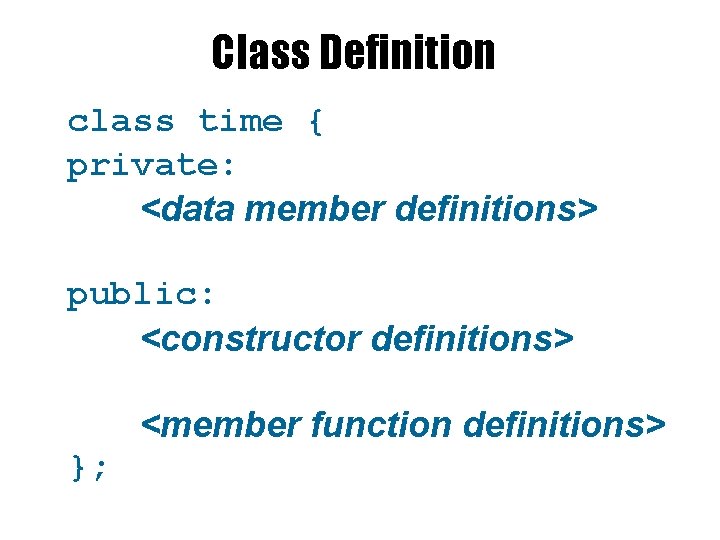
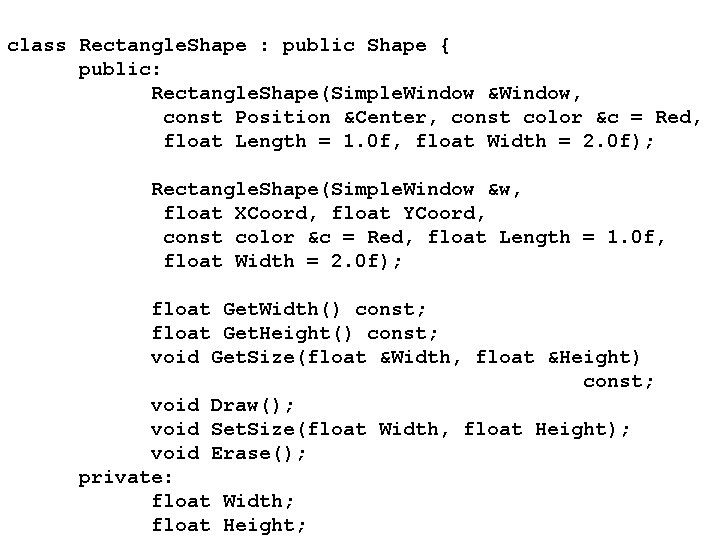
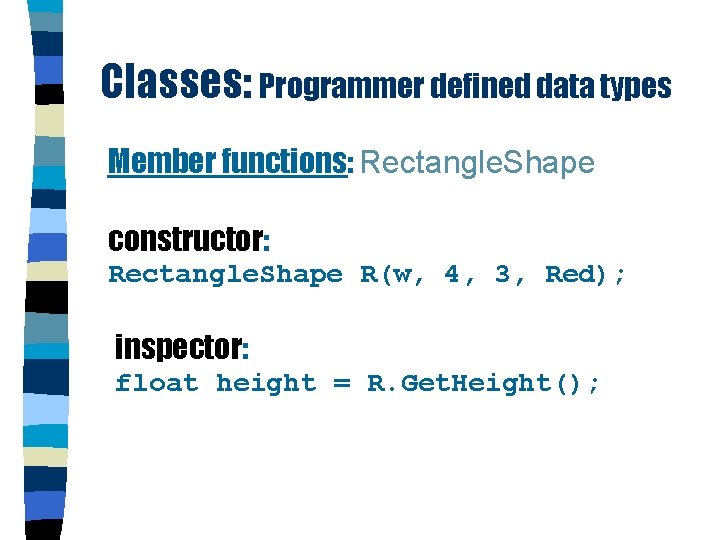
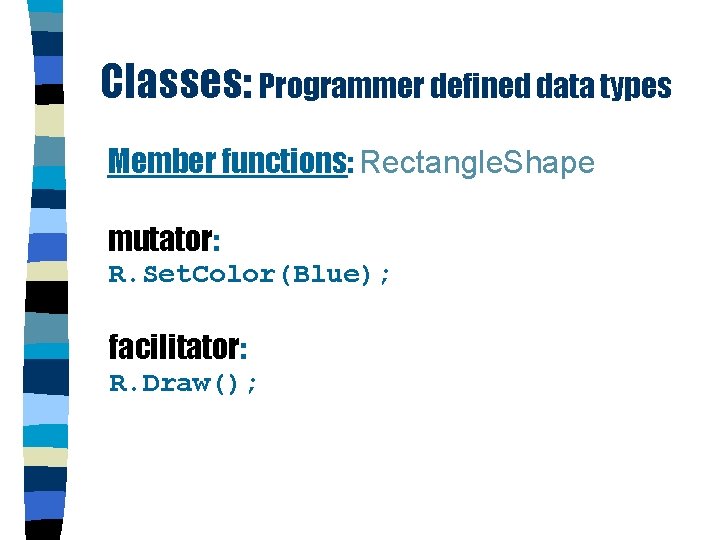
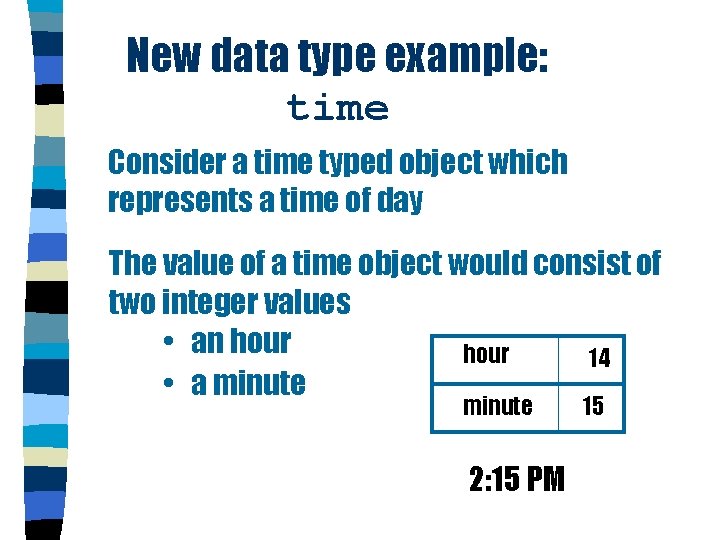
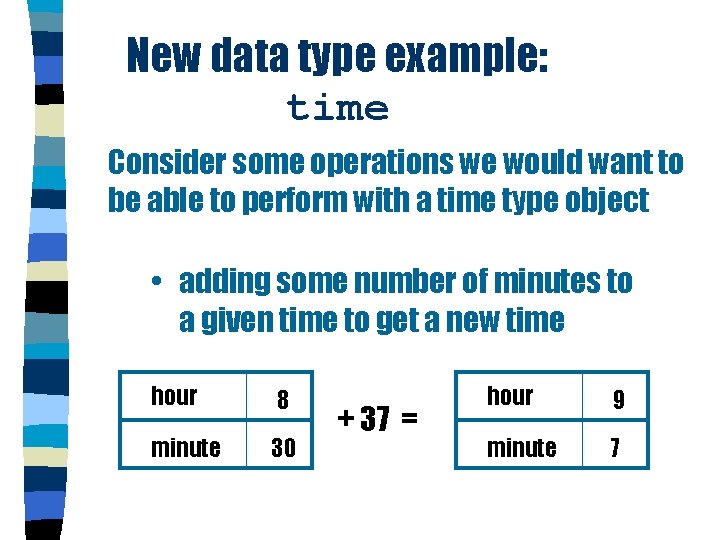
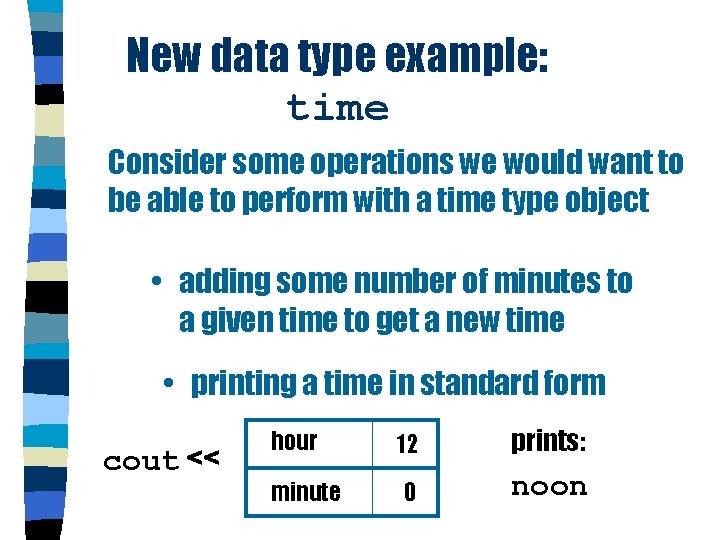
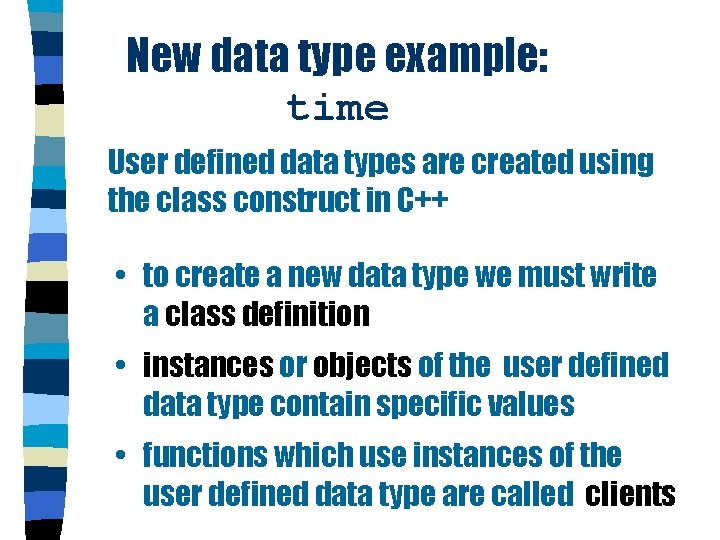
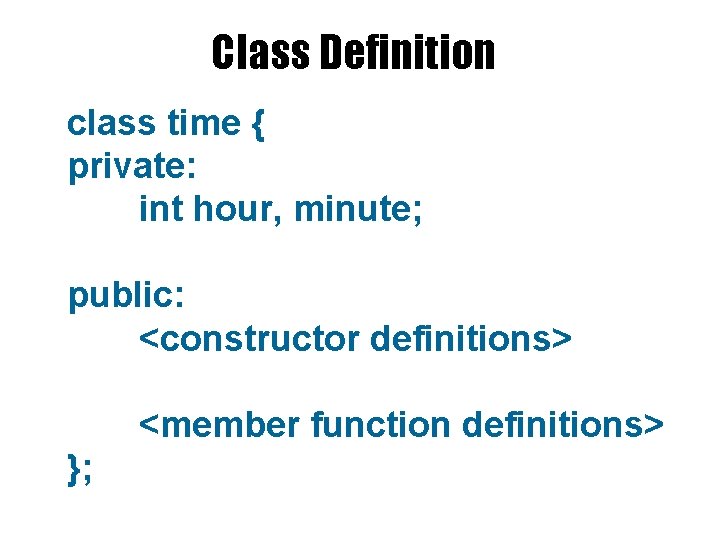
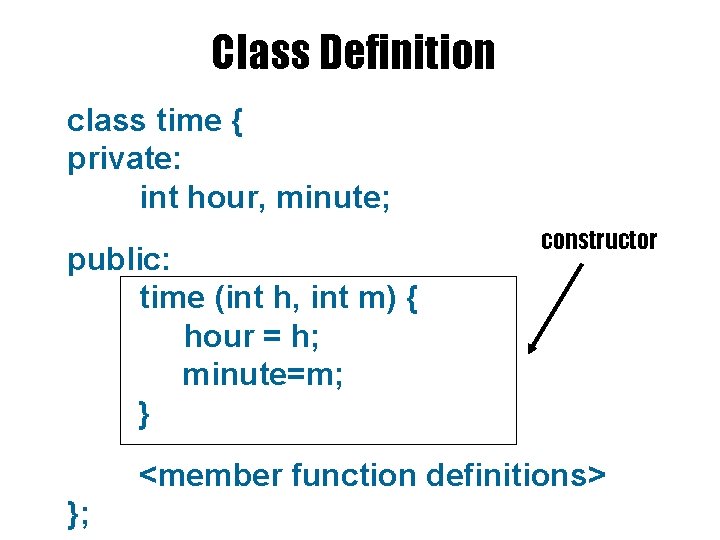
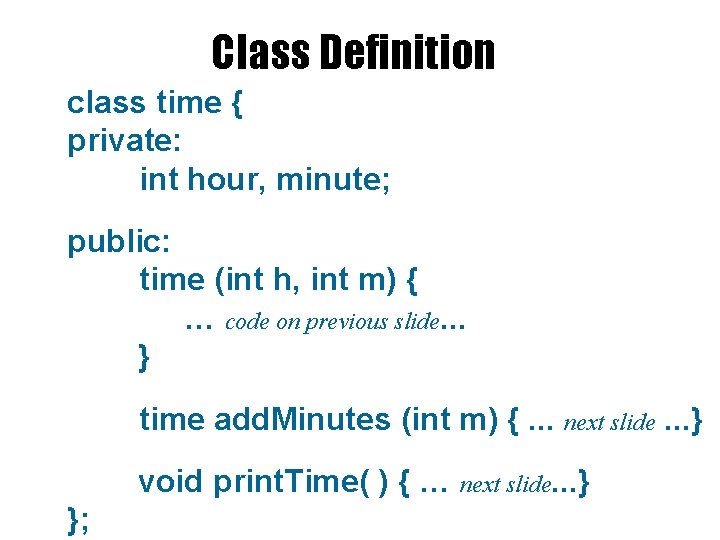
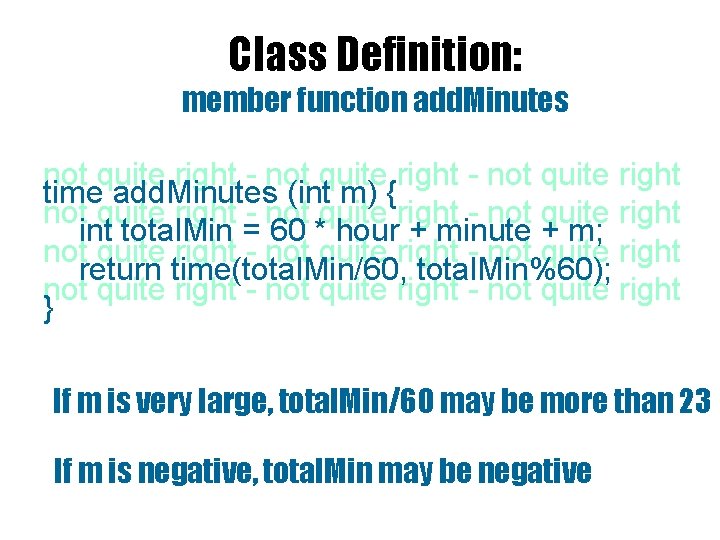
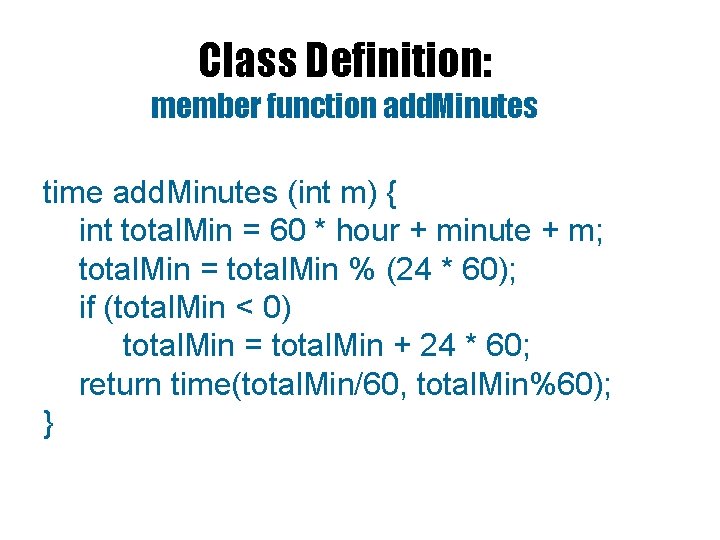
- Slides: 17
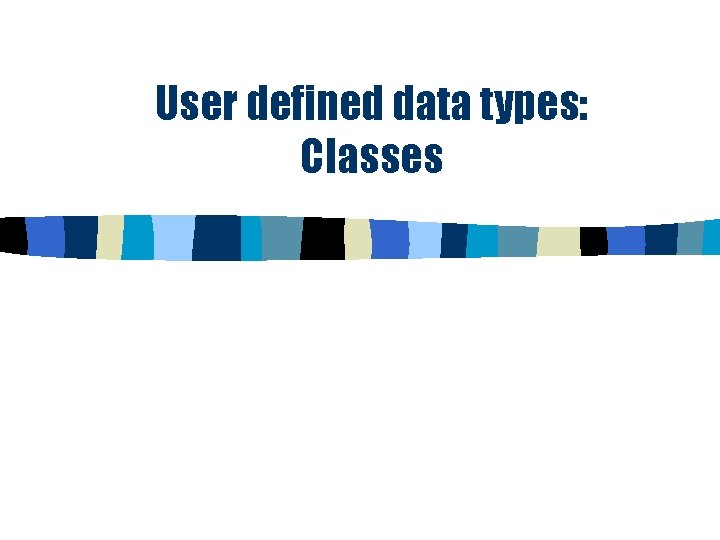
User defined data types: Classes
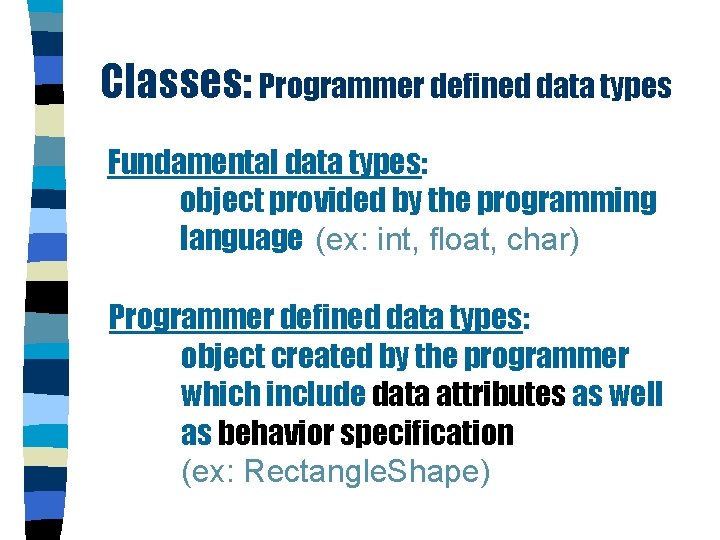
Classes: Programmer defined data types Fundamental data types: object provided by the programming language (ex: int, float, char) Programmer defined data types: object created by the programmer which include data attributes as well as behavior specification (ex: Rectangle. Shape)
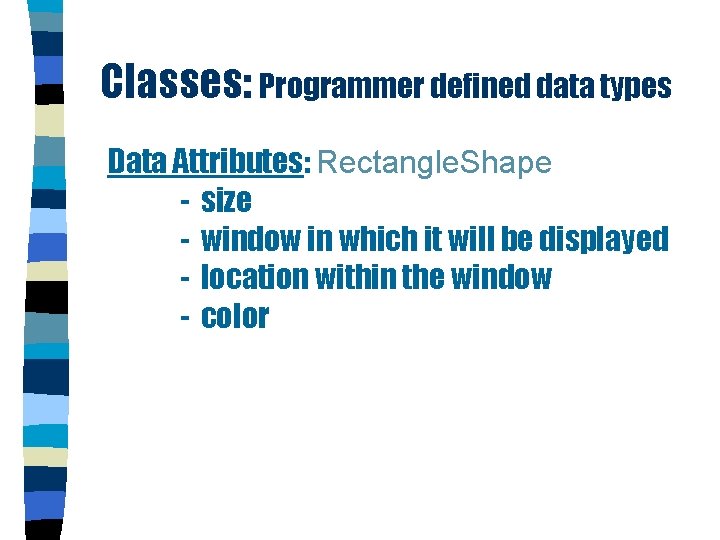
Classes: Programmer defined data types Data Attributes: Rectangle. Shape - size - window in which it will be displayed - location within the window - color
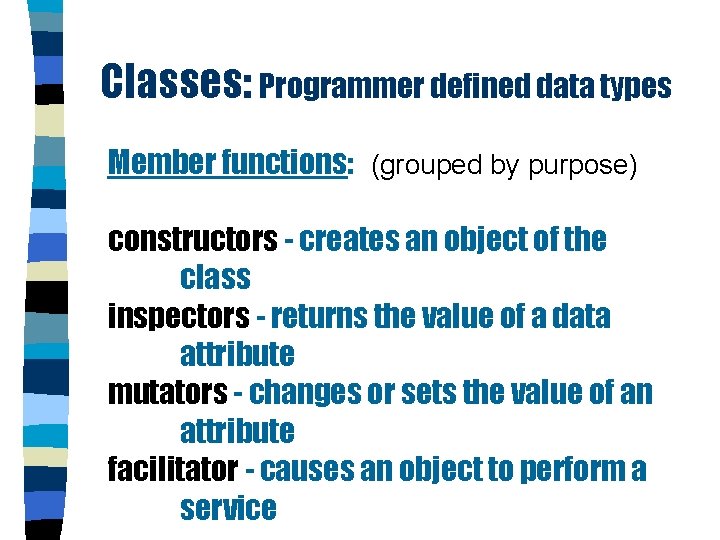
Classes: Programmer defined data types Member functions: (grouped by purpose) constructors - creates an object of the class inspectors - returns the value of a data attribute mutators - changes or sets the value of an attribute facilitator - causes an object to perform a service
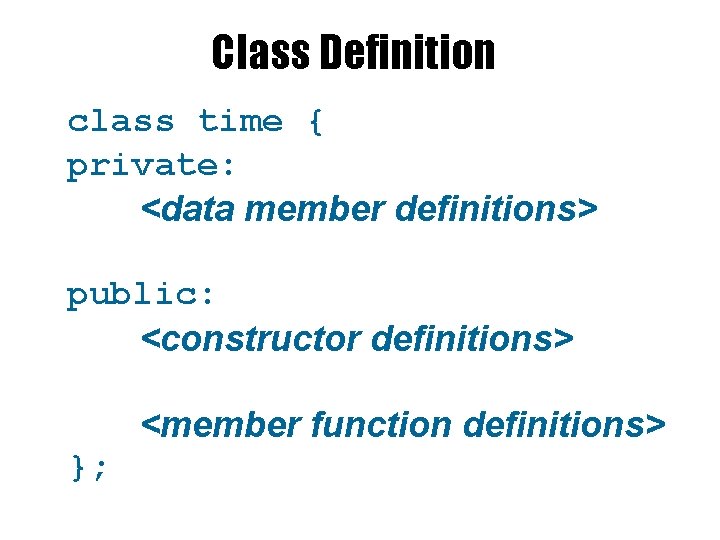
Class Definition class time {{ class <name> private: <data members> definitions> the attributes of a class-type object public: <constructor definitions> used to create a new instance of an object <member function definitions> }; }; defines how an instance can be used
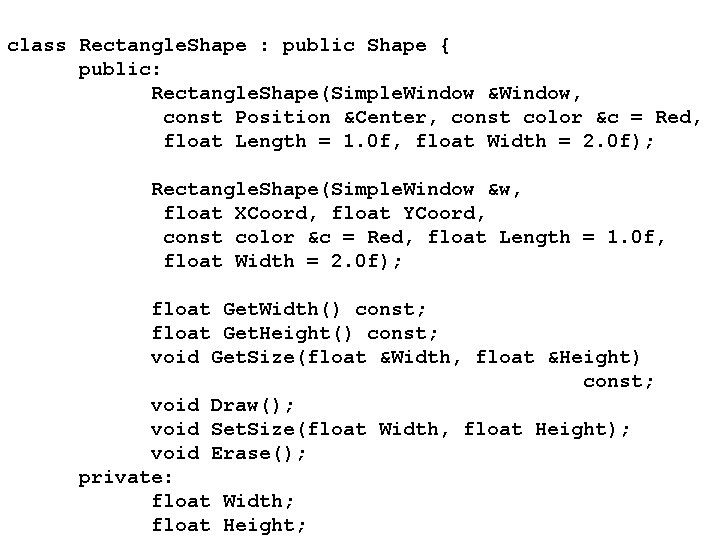
class Rectangle. Shape : public Shape { public: Rectangle. Shape(Simple. Window &Window, const Position &Center, const color &c = Red, float Length = 1. 0 f, float Width = 2. 0 f); Rectangle. Shape(Simple. Window &w, float XCoord, float YCoord, const color &c = Red, float Length = 1. 0 f, float Width = 2. 0 f); float Get. Width() const; float Get. Height() const; void Get. Size(float &Width, float &Height) const; void Draw(); void Set. Size(float Width, float Height); void Erase(); private: float Width; float Height;
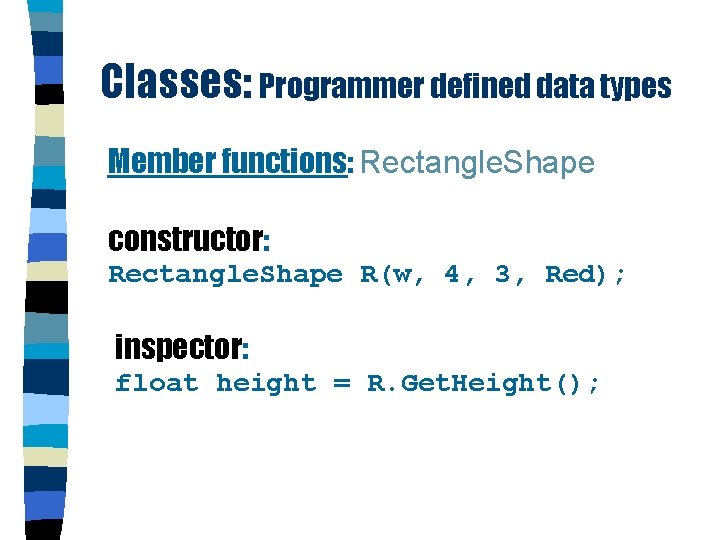
Classes: Programmer defined data types Member functions: Rectangle. Shape constructor: Rectangle. Shape R(w, 4, 3, Red); inspector: float height = R. Get. Height();
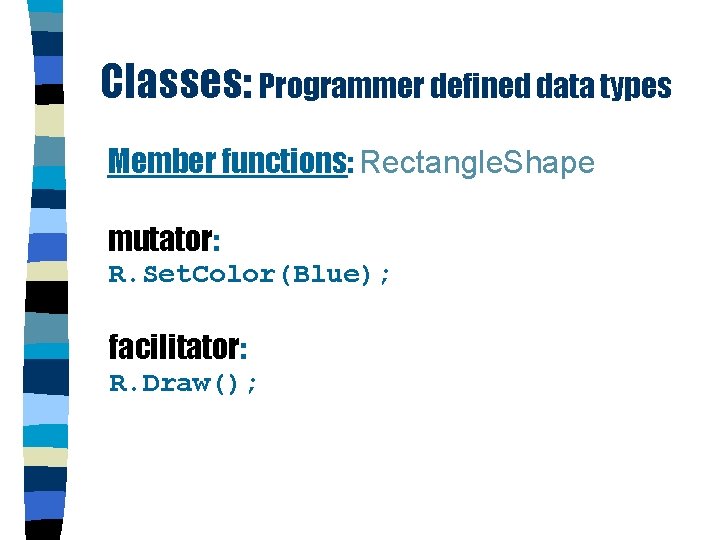
Classes: Programmer defined data types Member functions: Rectangle. Shape mutator: R. Set. Color(Blue); facilitator: R. Draw();
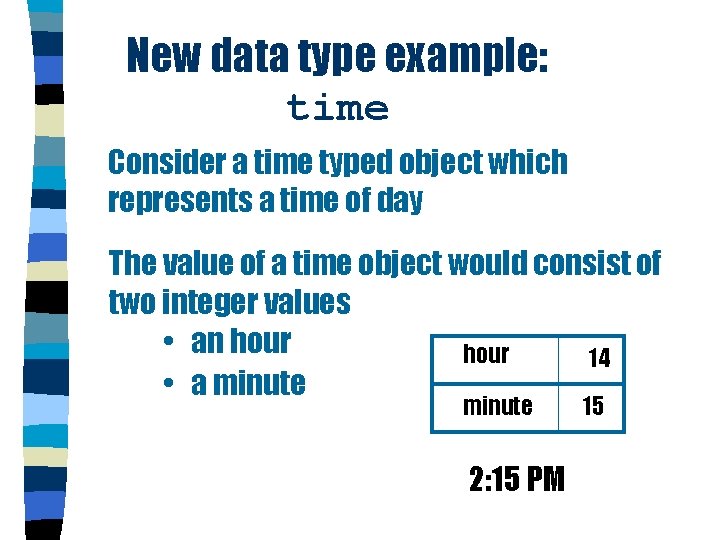
New data type example: time Consider a time typed object which represents a time of day The value of a time object would consist of two integer values • an hour 914 • a minute 15 30 2: 15 9: 30 PM AM
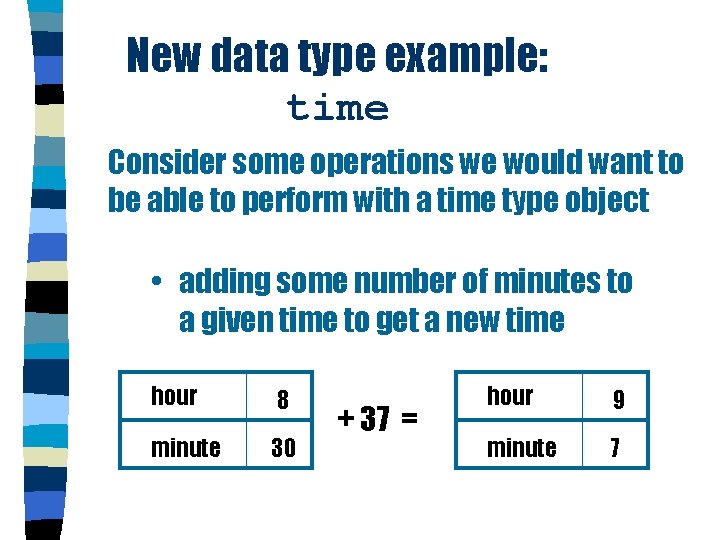
New data type example: time Consider some operations we would want to be able to perform with a time type object • adding some number of minutes to a given time to get a new time hour 8 minute 30 + 37 = hour 9 minute 7
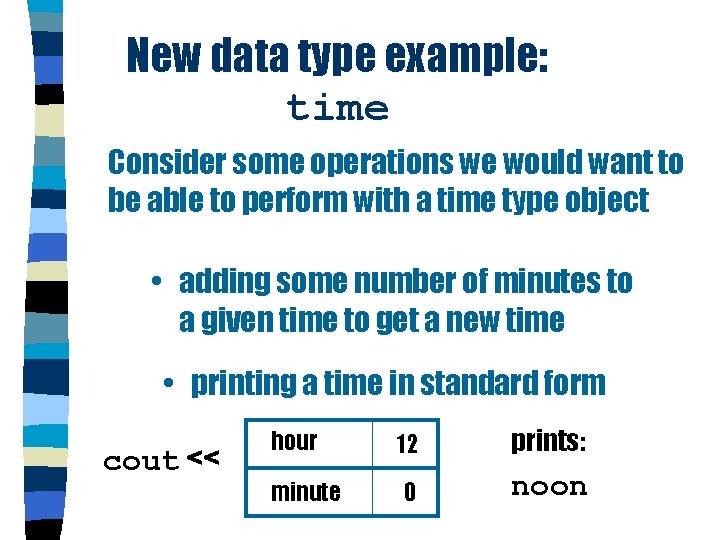
New data type example: time Consider some operations we would want to be able to perform with a time type object • adding some number of minutes to a given time to get a new time • printing a time in standard form cout << hour 9 12 prints: minute 30 0 9: 30 AM noon
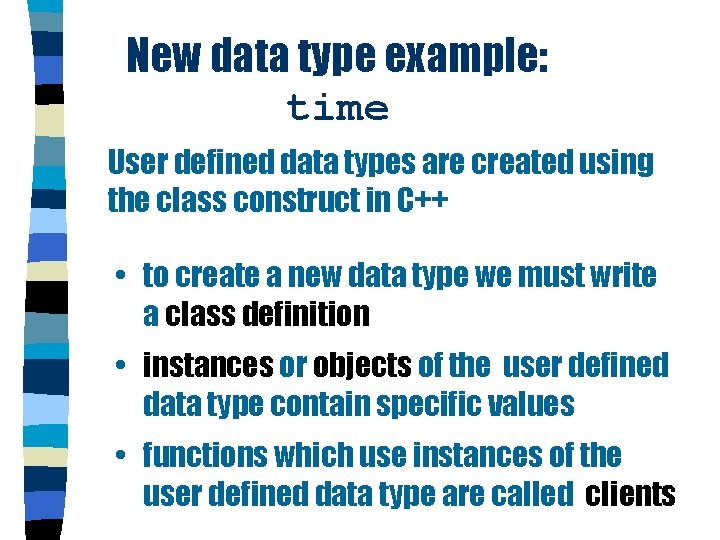
New data type example: time User defined data types are created using the class construct in C++ • to create a new data type we must write a class definition • instances or objects of the user defined data type contain specific values • functions which use instances of the user defined data type are called clients
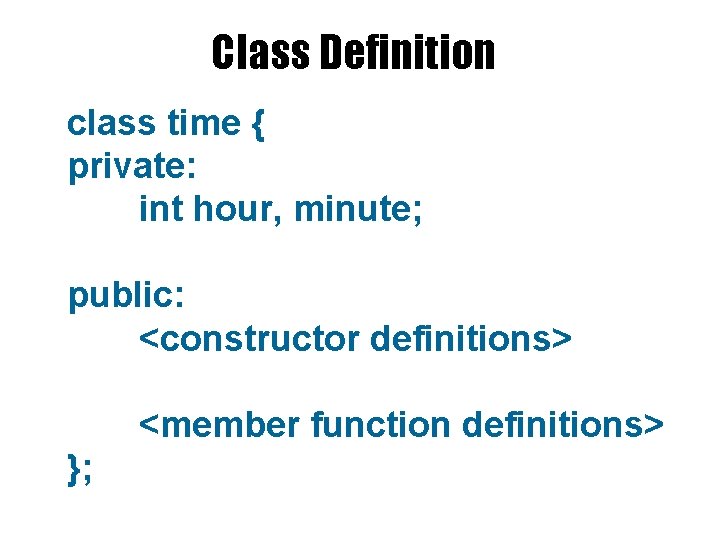
Class Definition class time <name> { { private: <data int hour, members> minute; the attributes of a class-type object public: <constructor definitions> used to create a new instance of an object <member function definitions> }; defines how an instance can be used
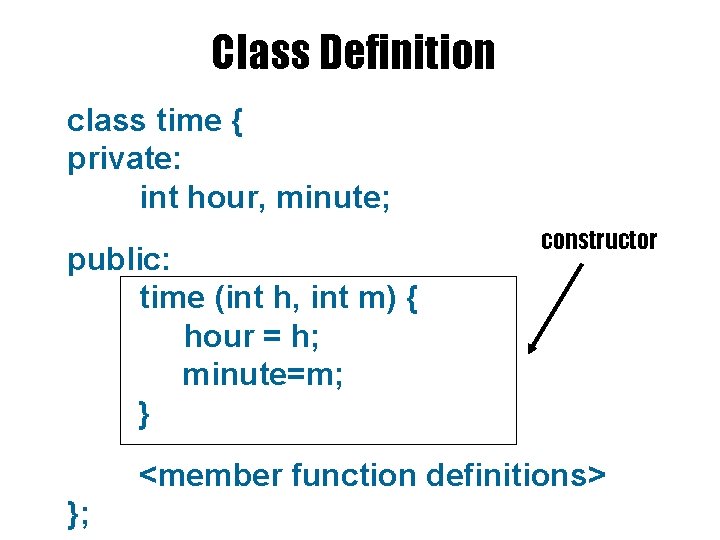
Class Definition class time { private: int hour, minute; public: time (int h, int m) { hour = h; minute=m; } constructor <member function definitions> };
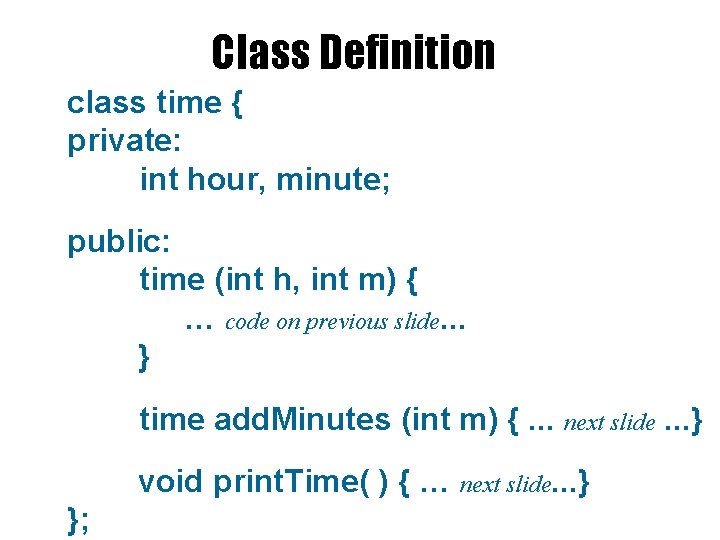
Class Definition class time { private: int hour, minute; public: time (int h, int m) { … code on previous slide. . . } time add. Minutes (int m) {. . . next slide. . . } void print. Time( ) { … next slide. . . } };
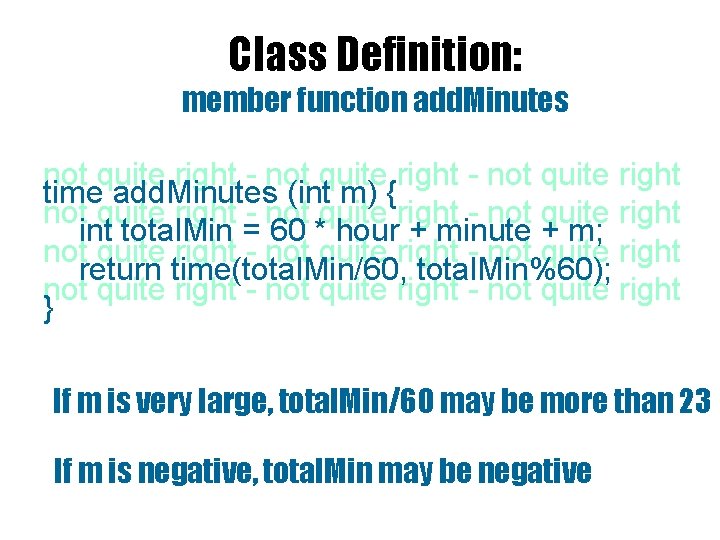
Class Definition: member function add. Minutes not quite right - not quite right time add. Minutes (int m) { not quite right - not quite right int total. Min = 60 * hour + minute + m; not quite right - not quite right return time(total. Min/60, total. Min%60); not quite right - not quite right } If m is very large, total. Min/60 may be more than 23 If m is negative, total. Min may be negative
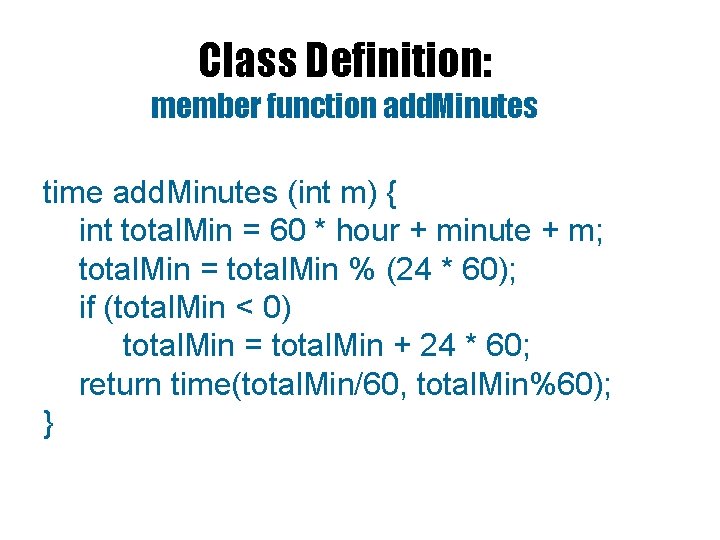
Class Definition: member function add. Minutes time add. Minutes (int m) { int total. Min = 60 * hour + minute + m; total. Min = total. Min % (24 * 60); if (total. Min < 0) total. Min = total. Min + 24 * 60; return time(total. Min/60, total. Min%60); }