Class specification Member function definition nested member function
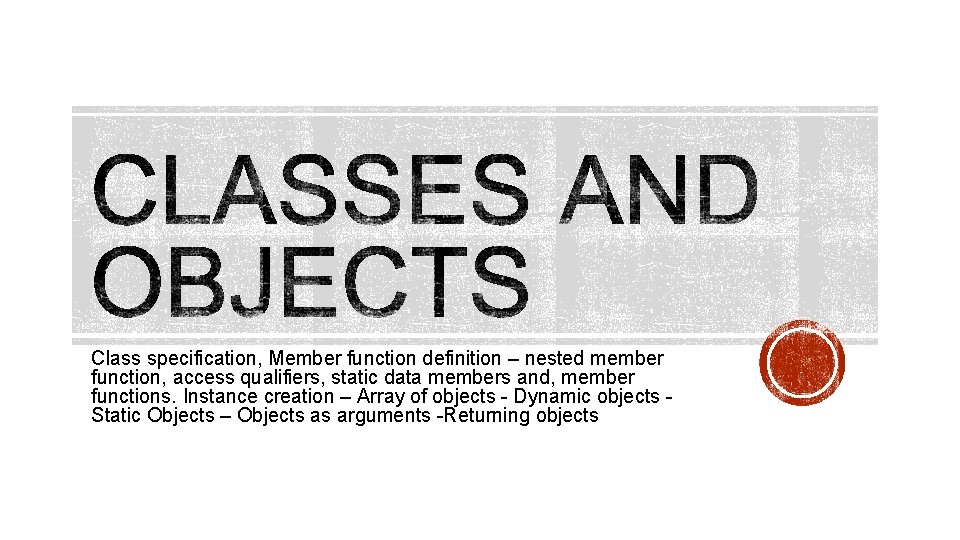
Class specification, Member function definition – nested member function, access qualifiers, static data members and, member functions. Instance creation – Array of objects - Dynamic objects Static Objects – Objects as arguments -Returning objects
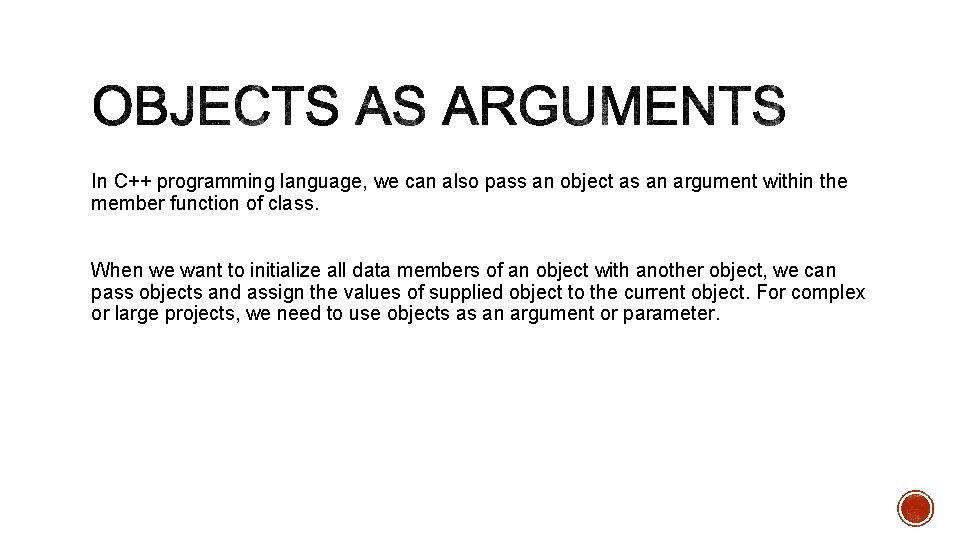
In C++ programming language, we can also pass an object as an argument within the member function of class. When we want to initialize all data members of an object with another object, we can pass objects and assign the values of supplied object to the current object. For complex or large projects, we need to use objects as an argument or parameter.
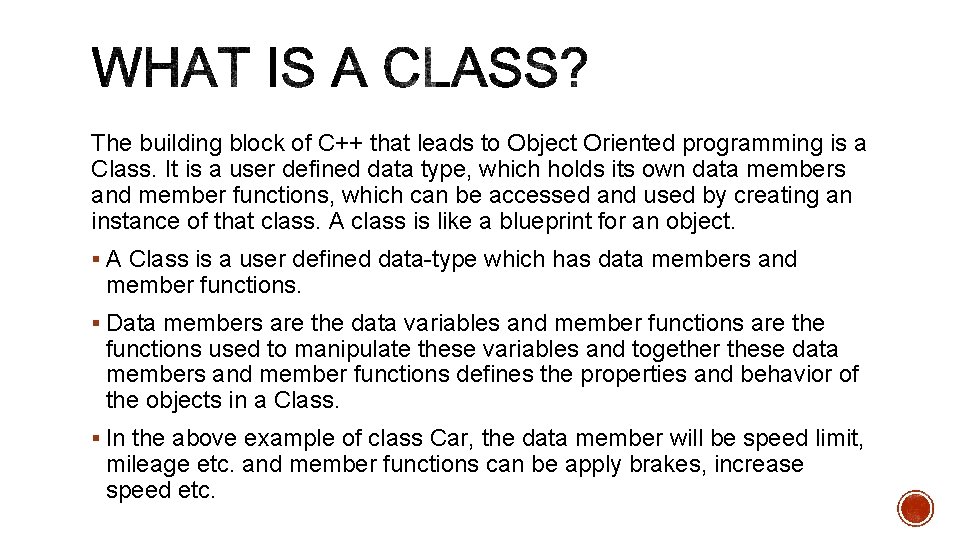
The building block of C++ that leads to Object Oriented programming is a Class. It is a user defined data type, which holds its own data members and member functions, which can be accessed and used by creating an instance of that class. A class is like a blueprint for an object. § A Class is a user defined data-type which has data members and member functions. § Data members are the data variables and member functions are the functions used to manipulate these variables and together these data members and member functions defines the properties and behavior of the objects in a Class. § In the above example of class Car, the data member will be speed limit, mileage etc. and member functions can be apply brakes, increase speed etc.
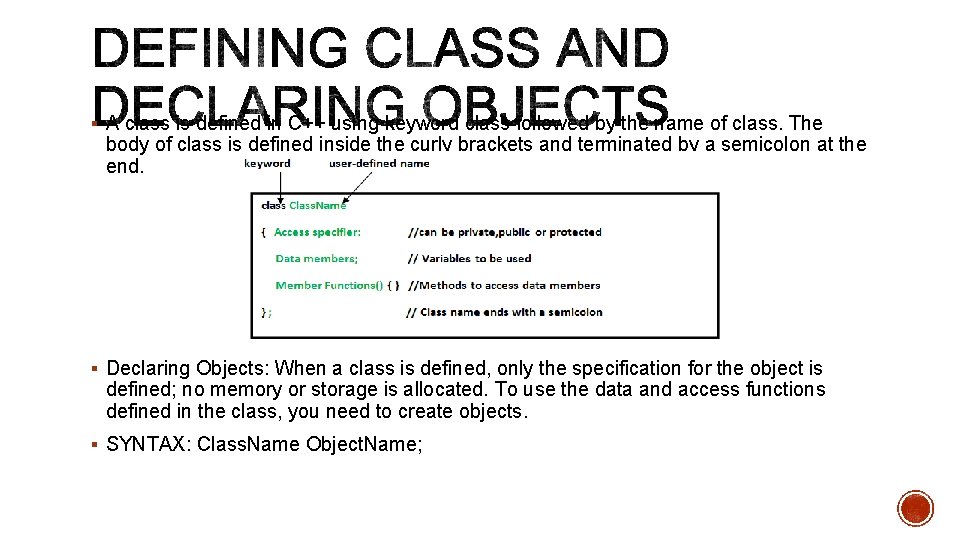
§ A class is defined in C++ using keyword class followed by the name of class. The body of class is defined inside the curly brackets and terminated by a semicolon at the end. § Declaring Objects: When a class is defined, only the specification for the object is defined; no memory or storage is allocated. To use the data and access functions defined in the class, you need to create objects. § SYNTAX: Class. Name Object. Name;
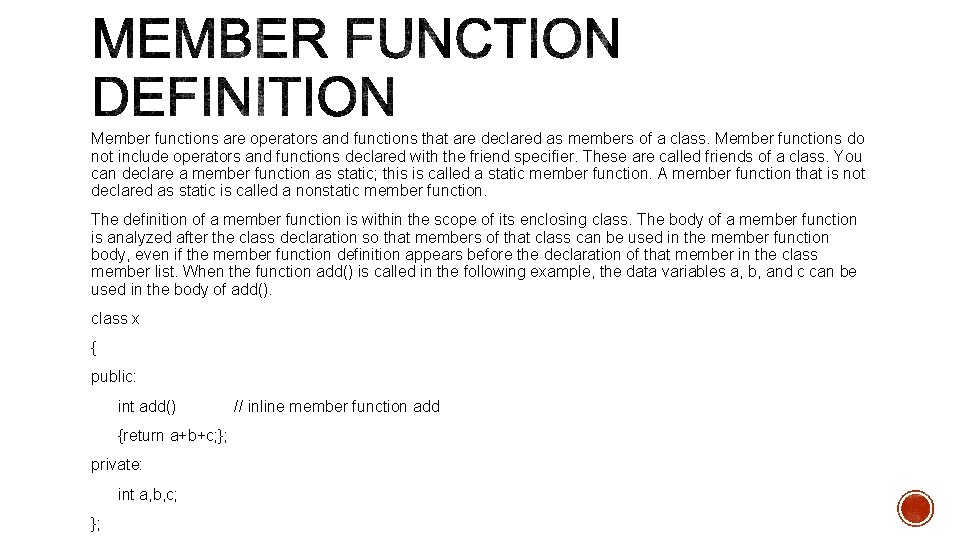
Member functions are operators and functions that are declared as members of a class. Member functions do not include operators and functions declared with the friend specifier. These are called friends of a class. You can declare a member function as static; this is called a static member function. A member function that is not declared as static is called a nonstatic member function. The definition of a member function is within the scope of its enclosing class. The body of a member function is analyzed after the class declaration so that members of that class can be used in the member function body, even if the member function definition appears before the declaration of that member in the class member list. When the function add() is called in the following example, the data variables a, b, and c can be used in the body of add(). class x { public: int add() {return a+b+c; }; private: int a, b, c; }; // inline member function add
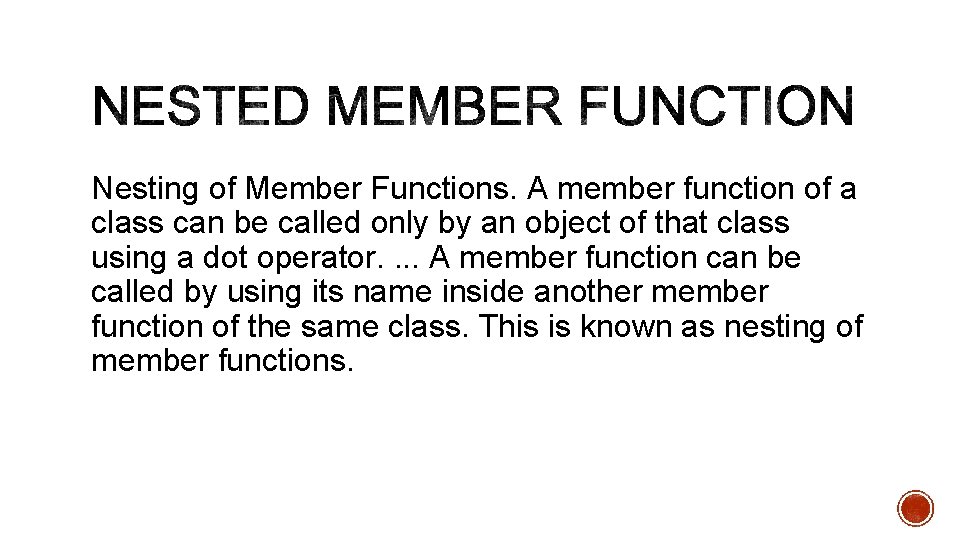
Nesting of Member Functions. A member function of a class can be called only by an object of that class using a dot operator. . A member function can be called by using its name inside another member function of the same class. This is known as nesting of member functions.
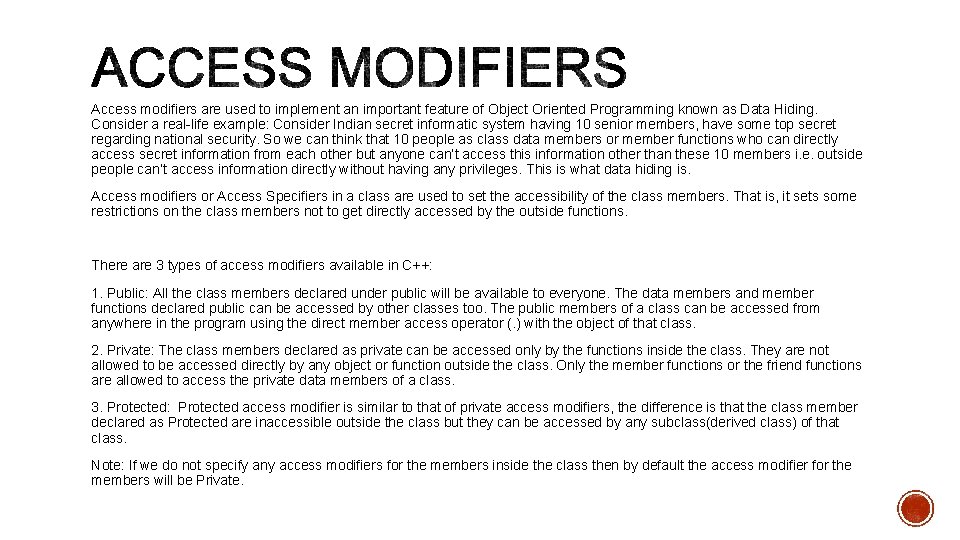
Access modifiers are used to implement an important feature of Object Oriented Programming known as Data Hiding. Consider a real-life example: Consider Indian secret informatic system having 10 senior members, have some top secret regarding national security. So we can think that 10 people as class data members or member functions who can directly access secret information from each other but anyone can’t access this information other than these 10 members i. e. outside people can’t access information directly without having any privileges. This is what data hiding is. Access modifiers or Access Specifiers in a class are used to set the accessibility of the class members. That is, it sets some restrictions on the class members not to get directly accessed by the outside functions. There are 3 types of access modifiers available in C++: 1. Public: All the class members declared under public will be available to everyone. The data members and member functions declared public can be accessed by other classes too. The public members of a class can be accessed from anywhere in the program using the direct member access operator (. ) with the object of that class. 2. Private: The class members declared as private can be accessed only by the functions inside the class. They are not allowed to be accessed directly by any object or function outside the class. Only the member functions or the friend functions are allowed to access the private data members of a class. 3. Protected: Protected access modifier is similar to that of private access modifiers, the difference is that the class member declared as Protected are inaccessible outside the class but they can be accessed by any subclass(derived class) of that class. Note: If we do not specify any access modifiers for the members inside the class then by default the access modifier for the members will be Private.
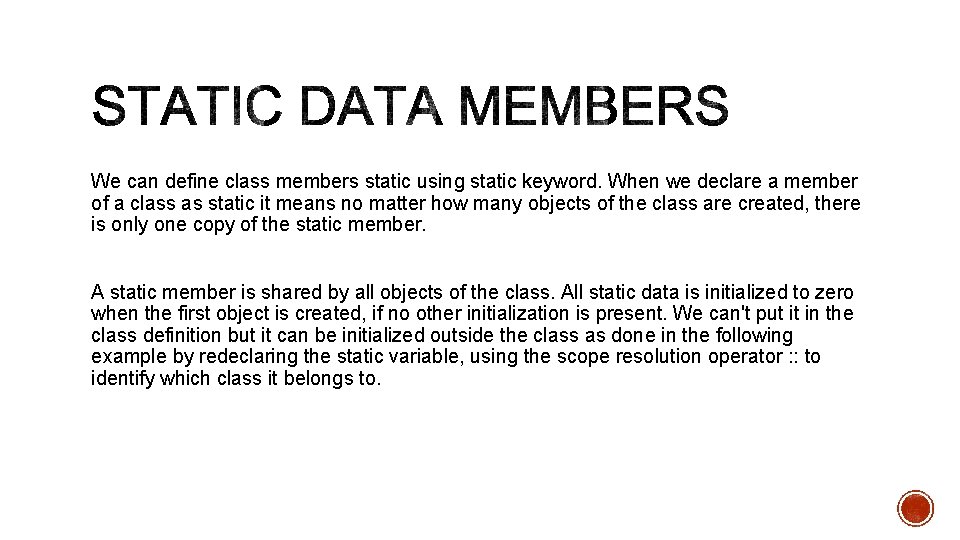
We can define class members static using static keyword. When we declare a member of a class as static it means no matter how many objects of the class are created, there is only one copy of the static member. A static member is shared by all objects of the class. All static data is initialized to zero when the first object is created, if no other initialization is present. We can't put it in the class definition but it can be initialized outside the class as done in the following example by redeclaring the static variable, using the scope resolution operator : : to identify which class it belongs to.
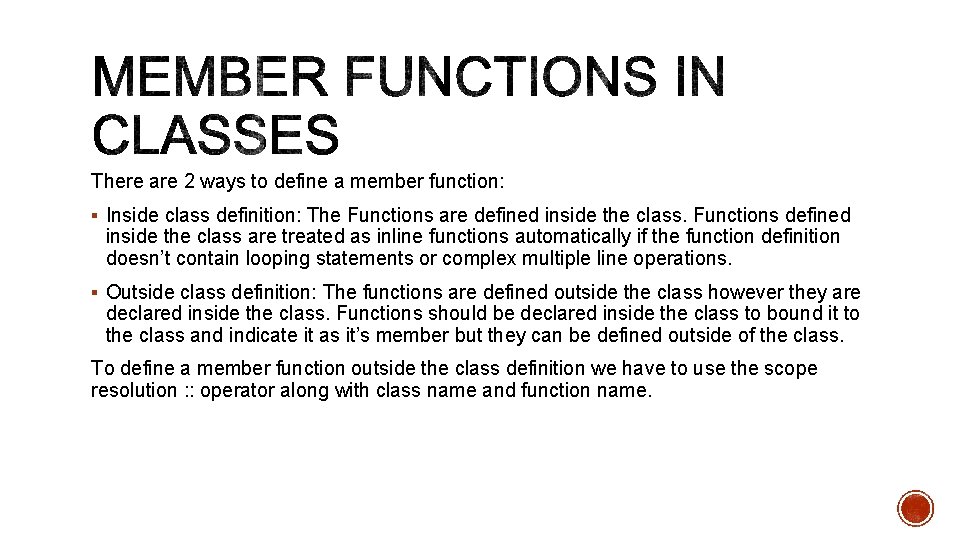
There are 2 ways to define a member function: § Inside class definition: The Functions are defined inside the class. Functions defined inside the class are treated as inline functions automatically if the function definition doesn’t contain looping statements or complex multiple line operations. § Outside class definition: The functions are defined outside the class however they are declared inside the class. Functions should be declared inside the class to bound it to the class and indicate it as it’s member but they can be defined outside of the class. To define a member function outside the class definition we have to use the scope resolution : : operator along with class name and function name.
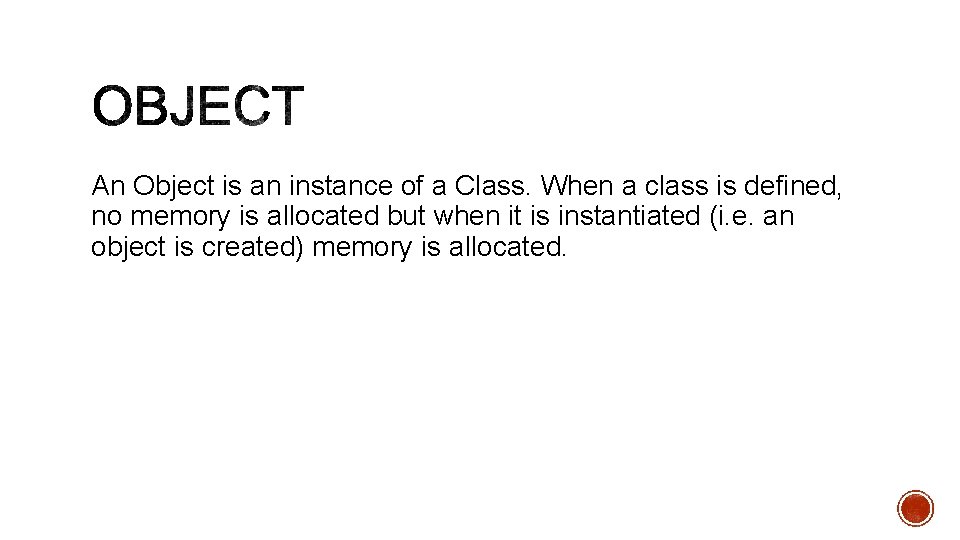
An Object is an instance of a Class. When a class is defined, no memory is allocated but when it is instantiated (i. e. an object is created) memory is allocated.
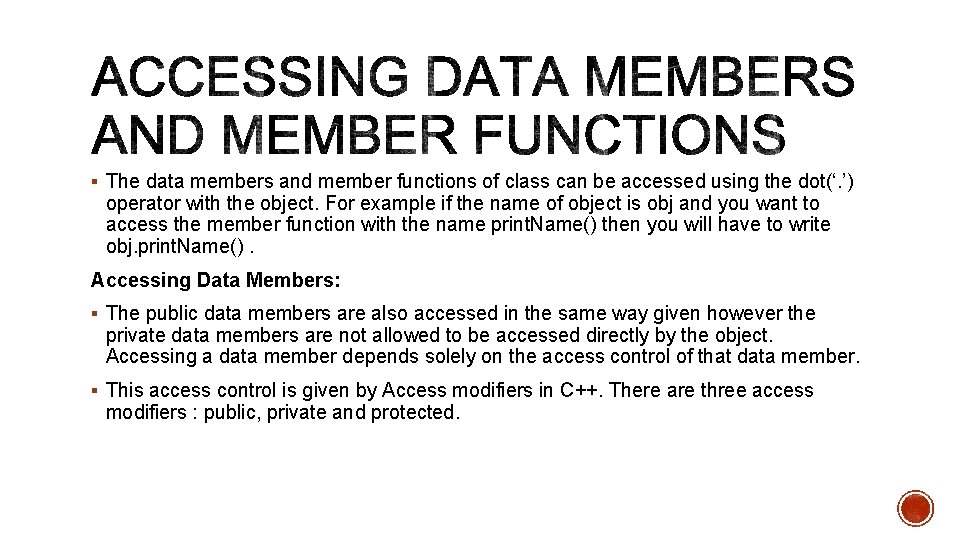
§ The data members and member functions of class can be accessed using the dot(‘. ’) operator with the object. For example if the name of object is obj and you want to access the member function with the name print. Name() then you will have to write obj. print. Name(). Accessing Data Members: § The public data members are also accessed in the same way given however the private data members are not allowed to be accessed directly by the object. Accessing a data member depends solely on the access control of that data member. § This access control is given by Access modifiers in C++. There are three access modifiers : public, private and protected.
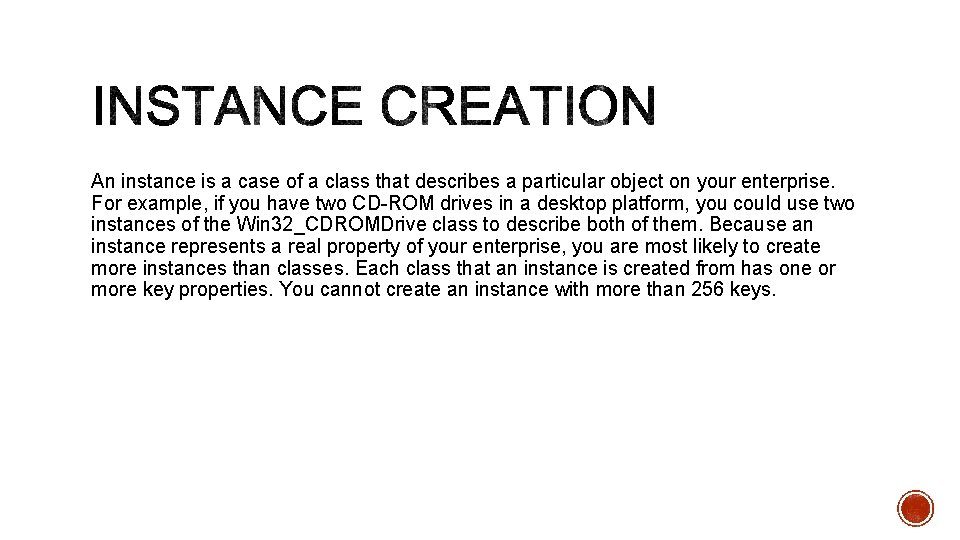
An instance is a case of a class that describes a particular object on your enterprise. For example, if you have two CD-ROM drives in a desktop platform, you could use two instances of the Win 32_CDROMDrive class to describe both of them. Because an instance represents a real property of your enterprise, you are most likely to create more instances than classes. Each class that an instance is created from has one or more key properties. You cannot create an instance with more than 256 keys.
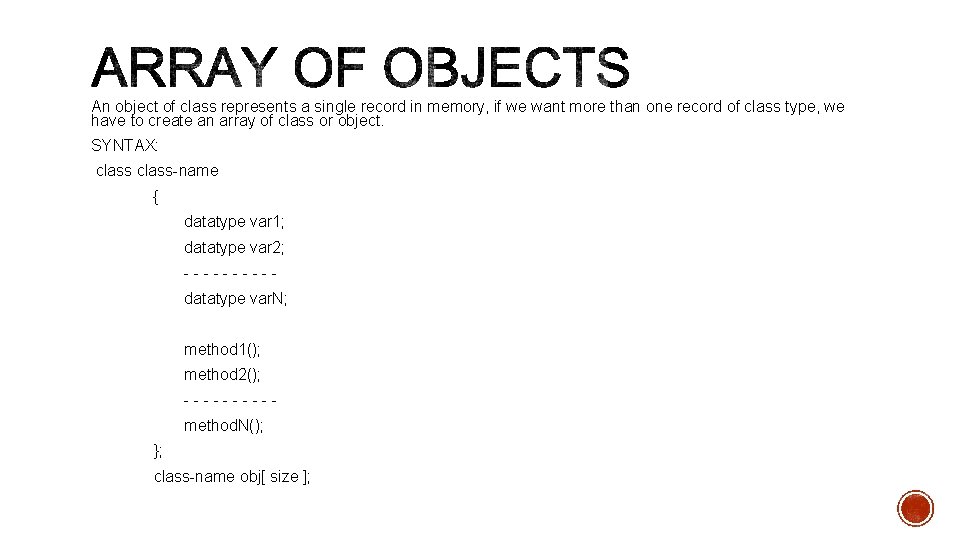
An object of class represents a single record in memory, if we want more than one record of class type, we have to create an array of class or object. SYNTAX: class-name { datatype var 1; datatype var 2; -----datatype var. N; method 1(); method 2(); -----method. N(); }; class-name obj[ size ];
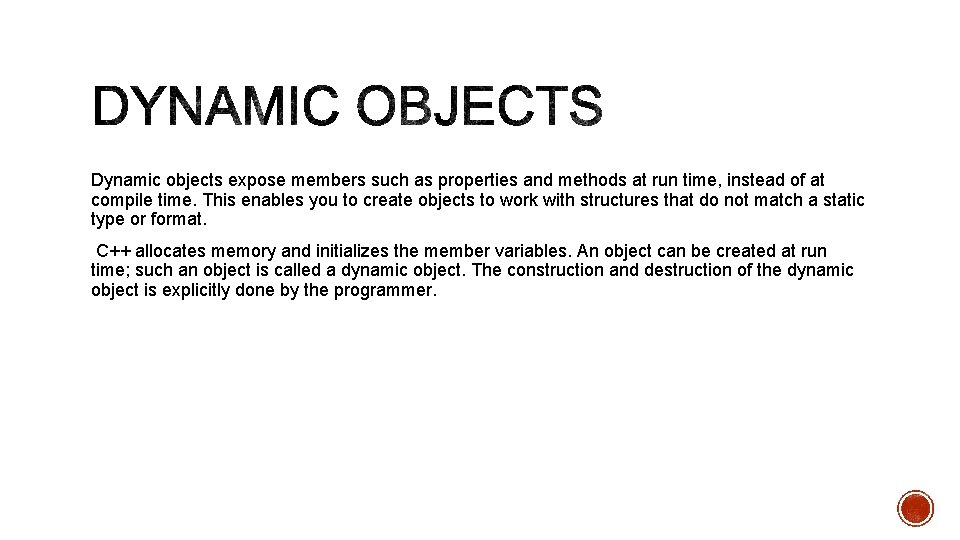
Dynamic objects expose members such as properties and methods at run time, instead of at compile time. This enables you to create objects to work with structures that do not match a static type or format. C++ allocates memory and initializes the member variables. An object can be created at run time; such an object is called a dynamic object. The construction and destruction of the dynamic object is explicitly done by the programmer.
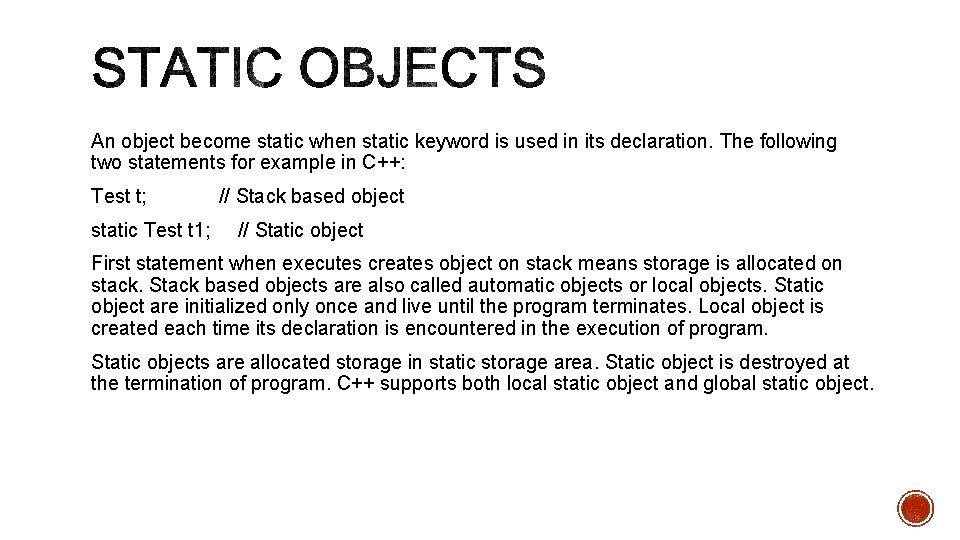
An object become static when static keyword is used in its declaration. The following two statements for example in C++: Test t; static Test t 1; // Stack based object // Static object First statement when executes creates object on stack means storage is allocated on stack. Stack based objects are also called automatic objects or local objects. Static object are initialized only once and live until the program terminates. Local object is created each time its declaration is encountered in the execution of program. Static objects are allocated storage in static storage area. Static object is destroyed at the termination of program. C++ supports both local static object and global static object.
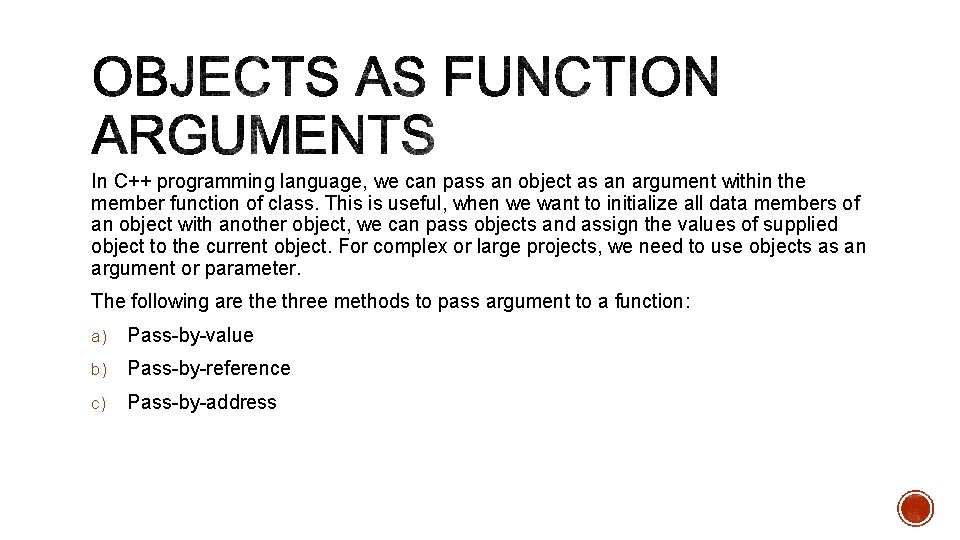
In C++ programming language, we can pass an object as an argument within the member function of class. This is useful, when we want to initialize all data members of an object with another object, we can pass objects and assign the values of supplied object to the current object. For complex or large projects, we need to use objects as an argument or parameter. The following are three methods to pass argument to a function: a) Pass-by-value b) Pass-by-reference c) Pass-by-address
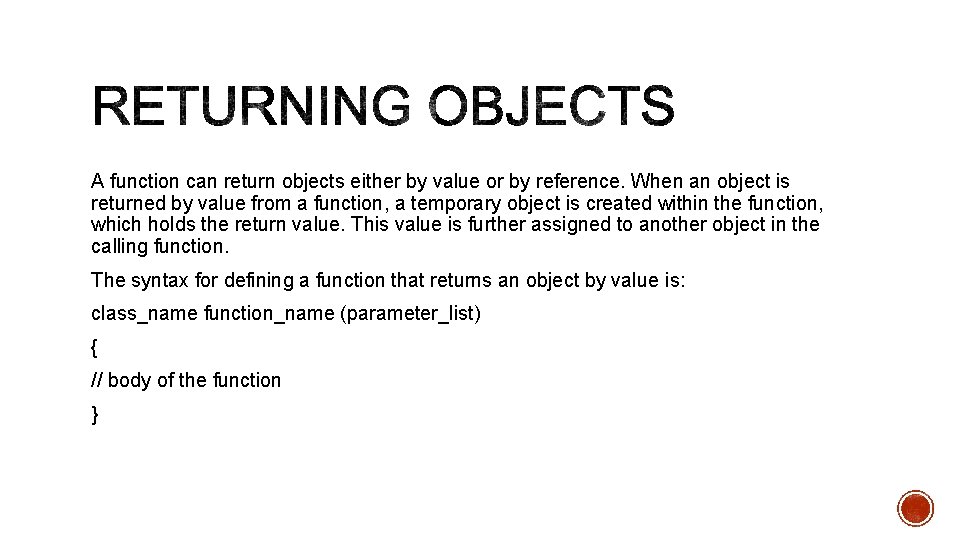
A function can return objects either by value or by reference. When an object is returned by value from a function, a temporary object is created within the function, which holds the return value. This value is further assigned to another object in the calling function. The syntax for defining a function that returns an object by value is: class_name function_name (parameter_list) { // body of the function }
- Slides: 17