EGR 2261 Unit 3 InputOutput Read Malik Chapter
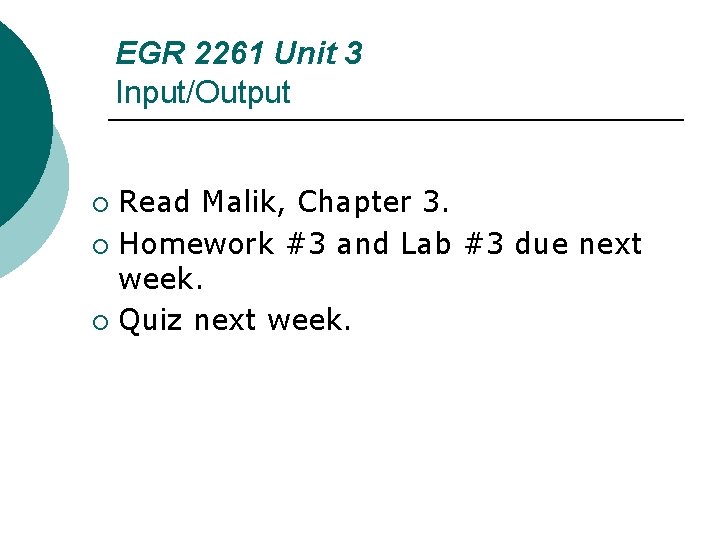
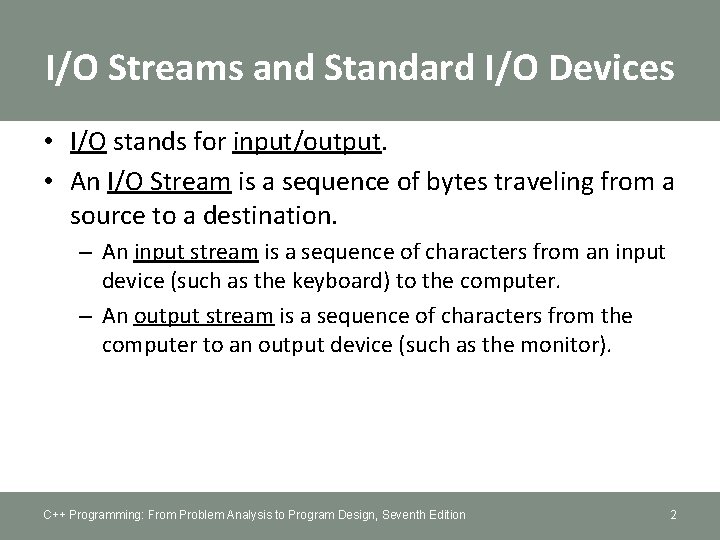
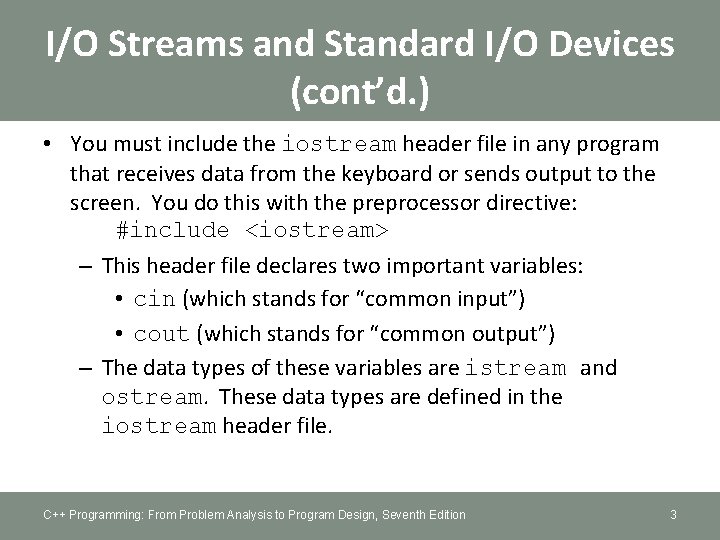
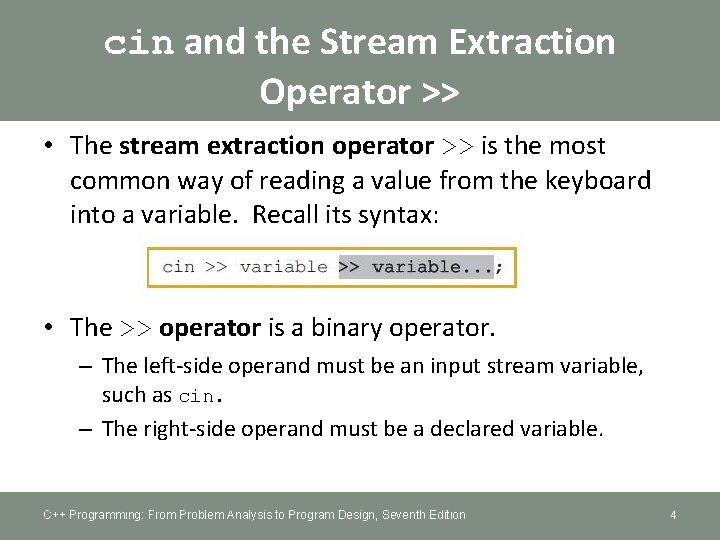
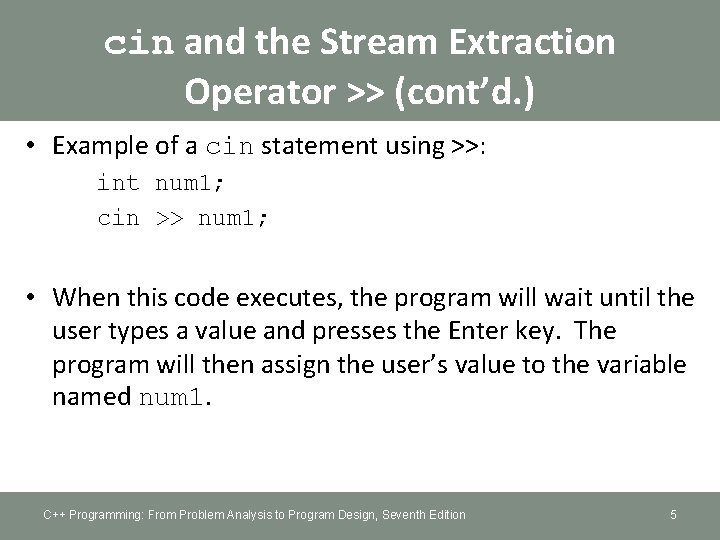
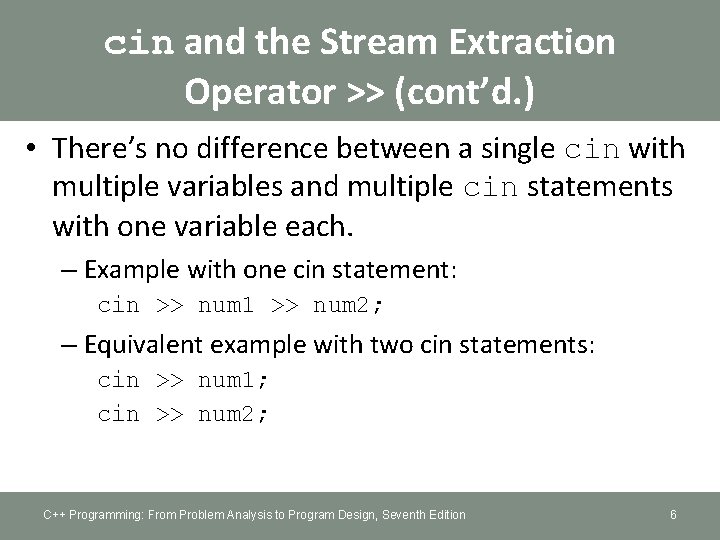
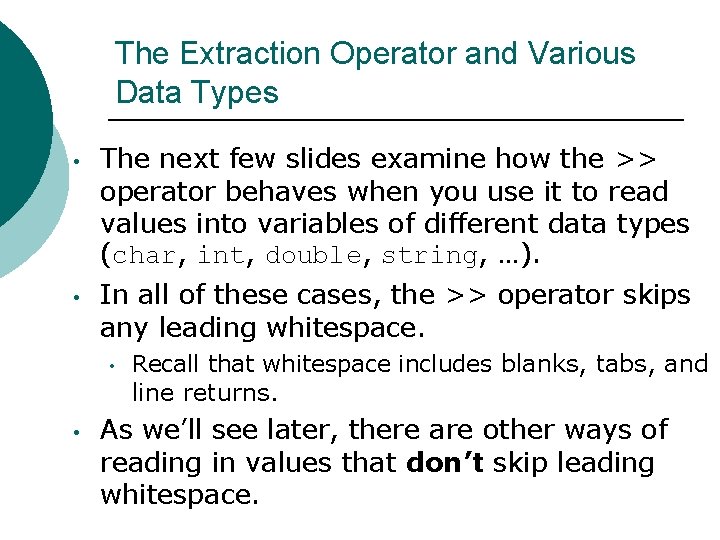
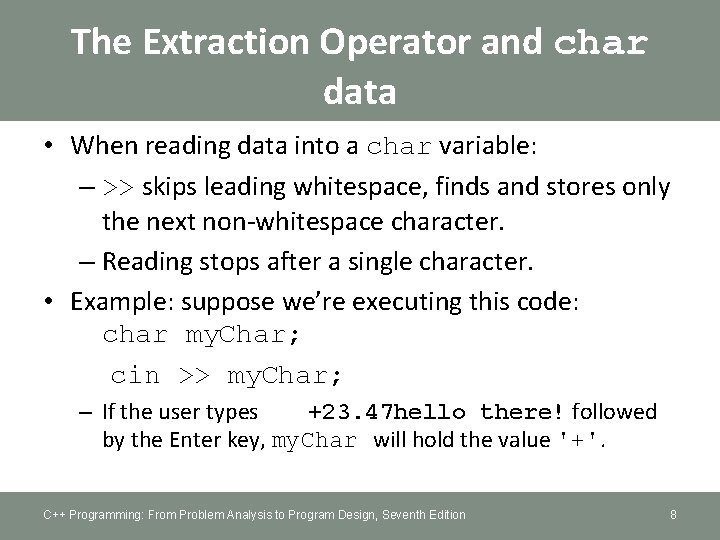
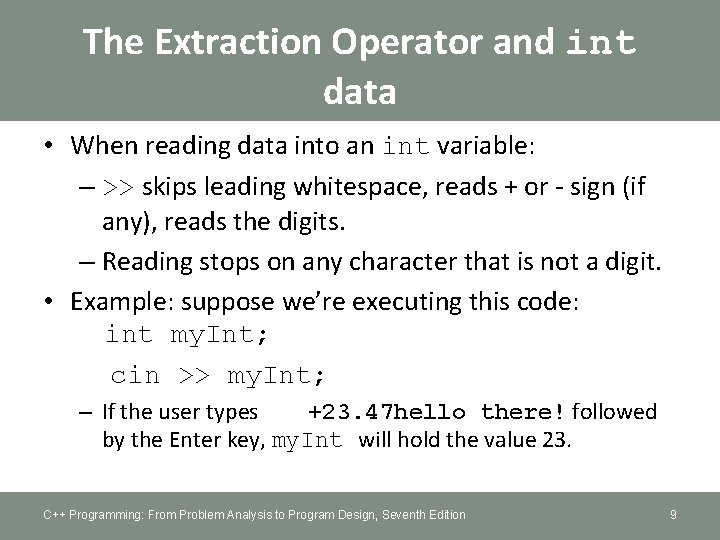
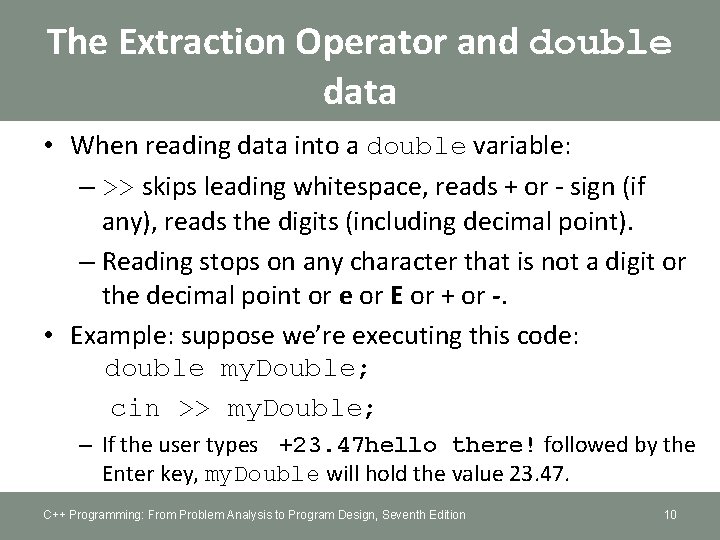
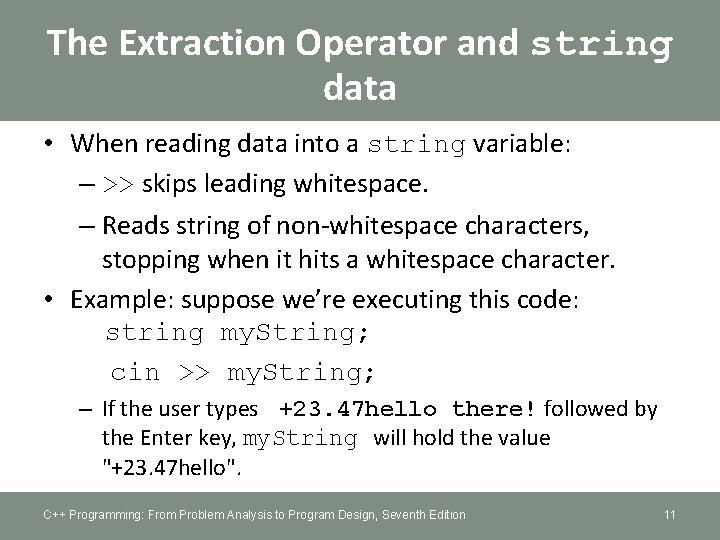
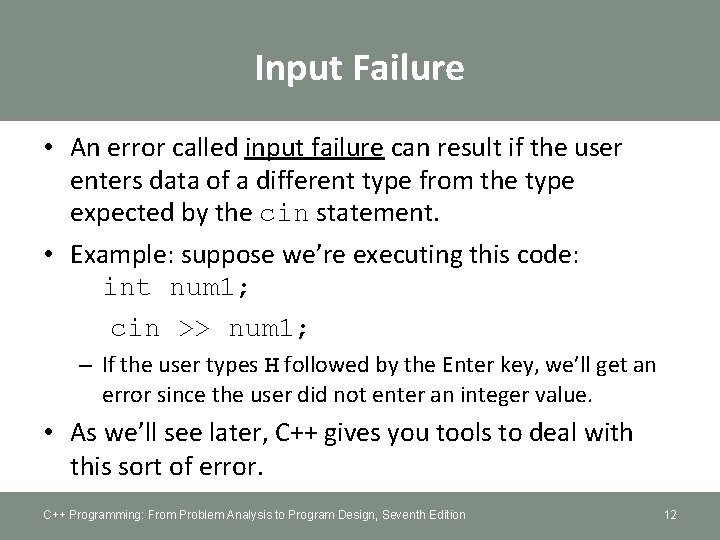
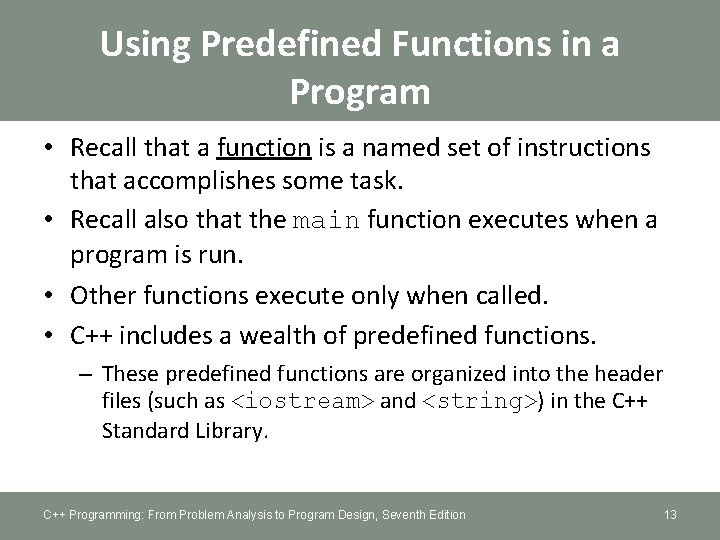
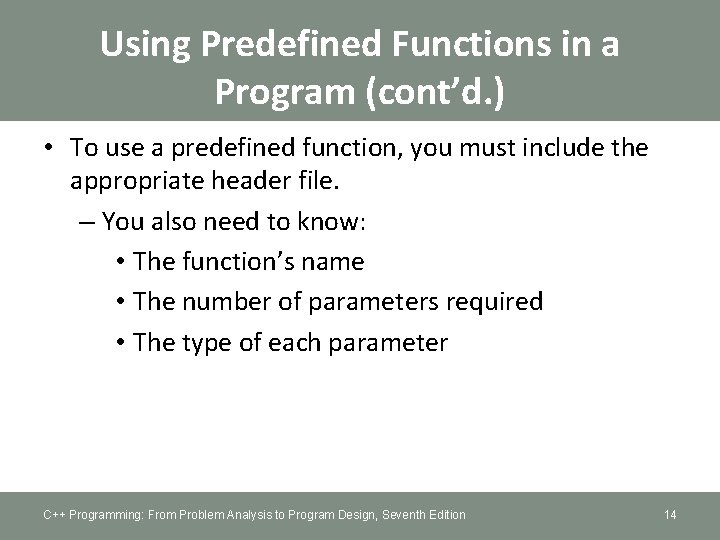
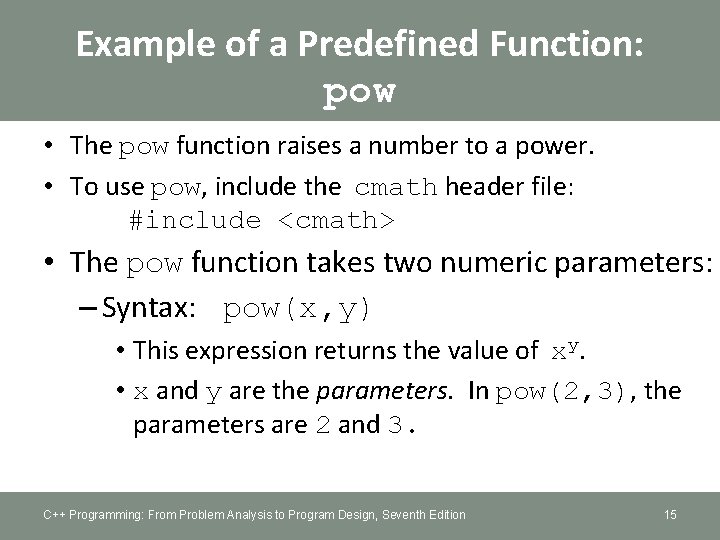
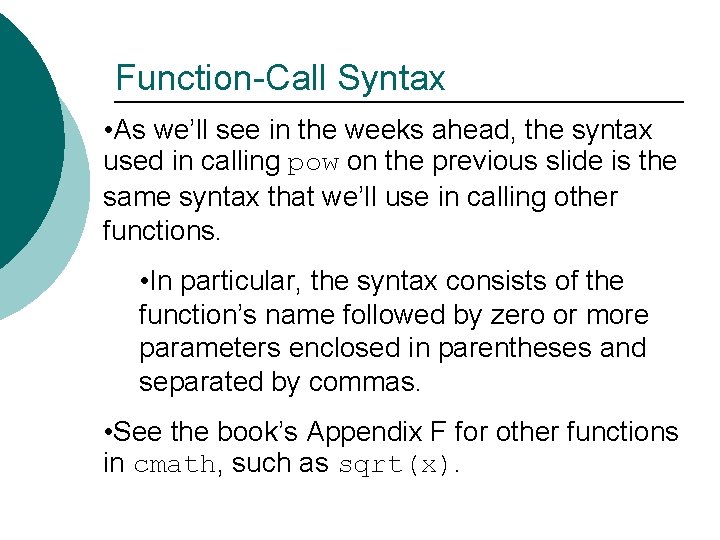
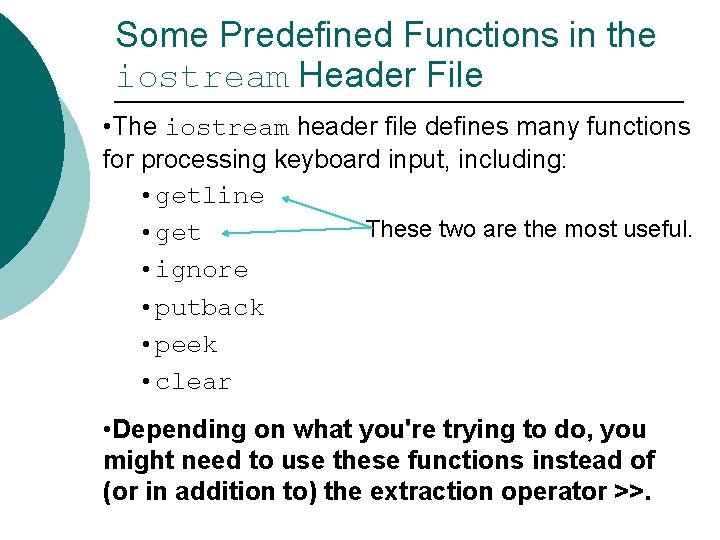
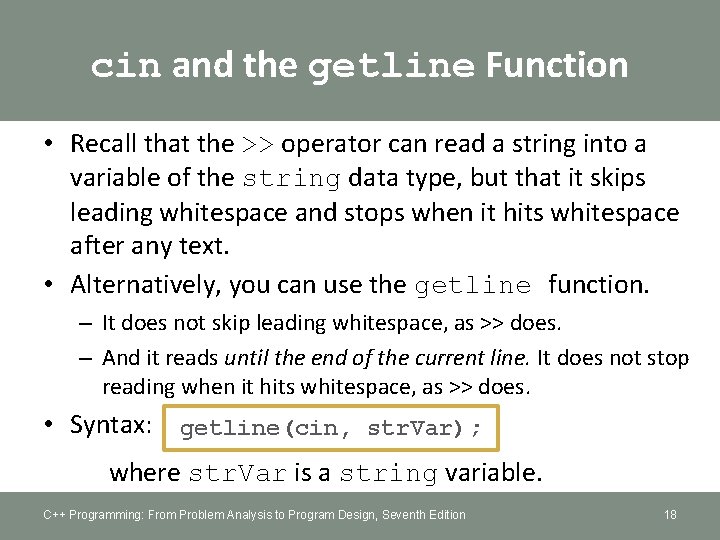
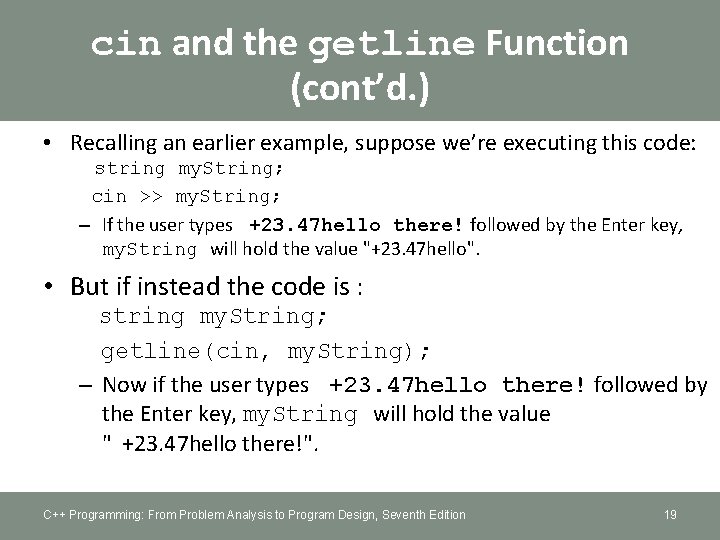
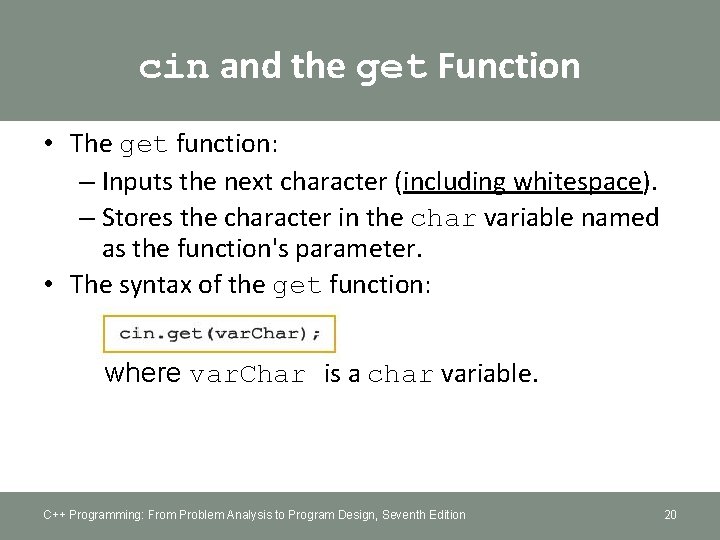
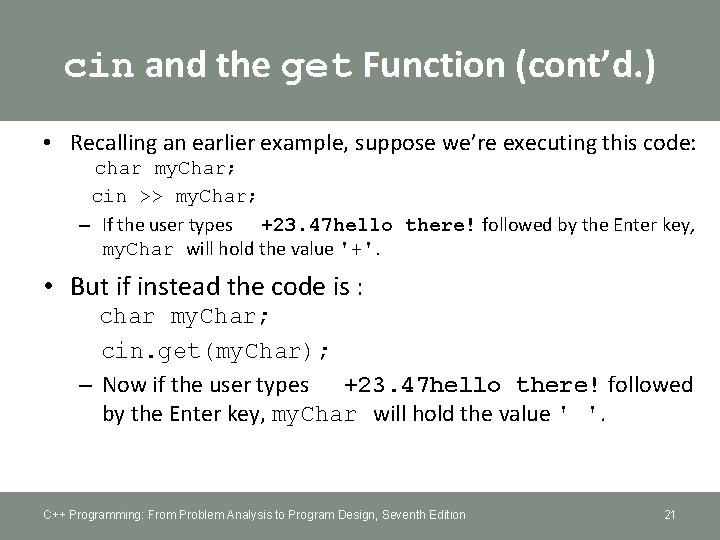
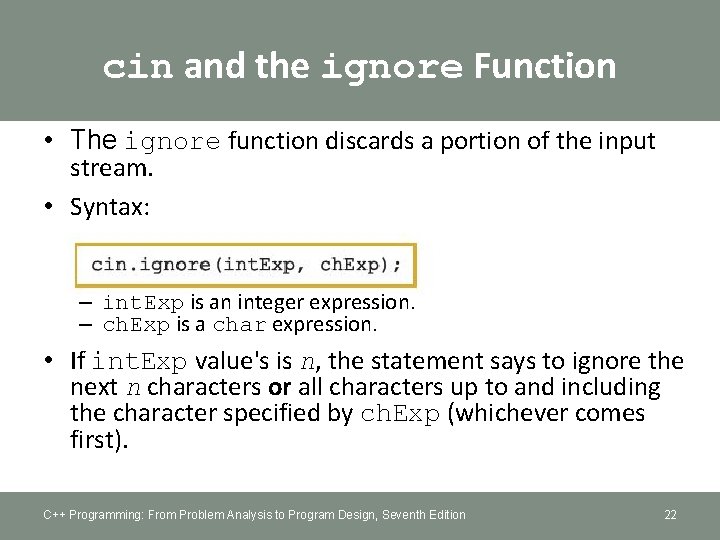
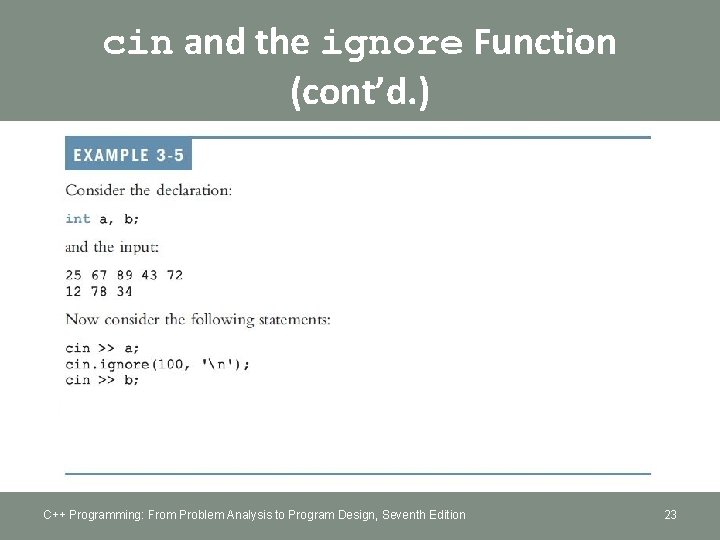
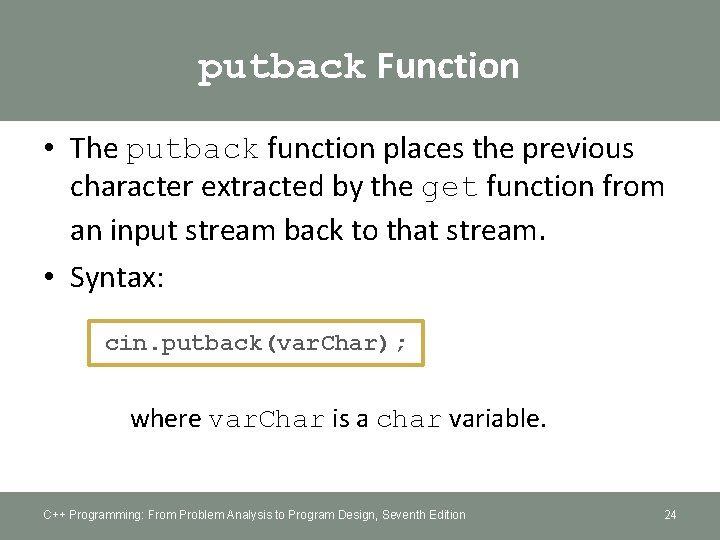
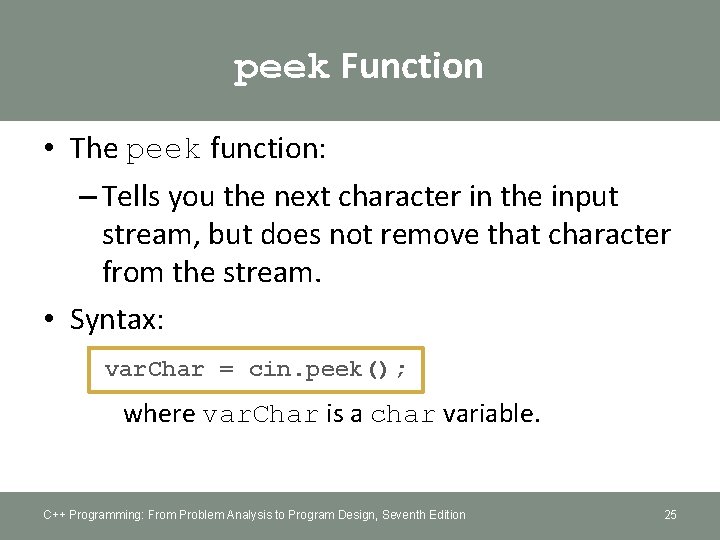
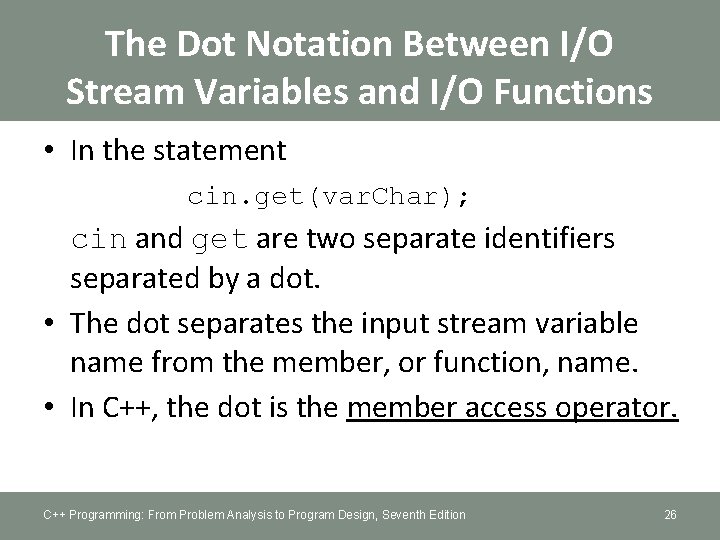
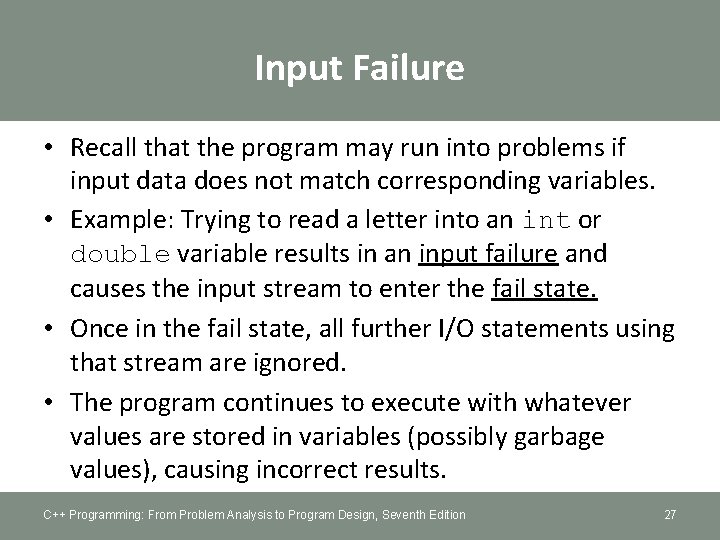
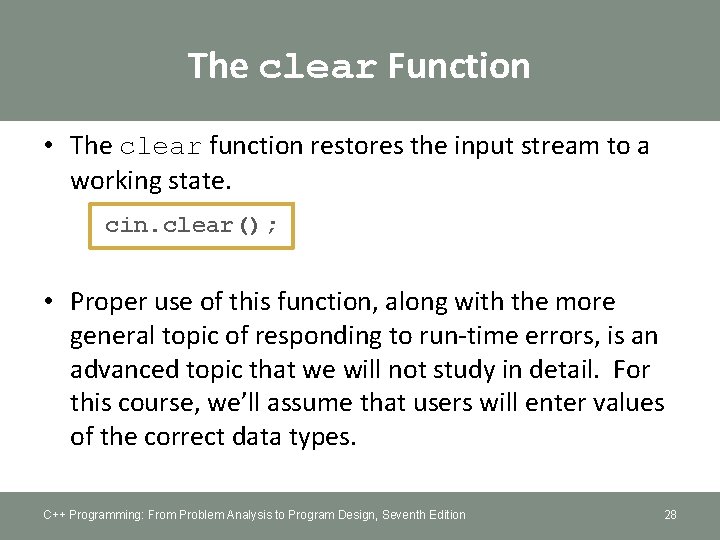
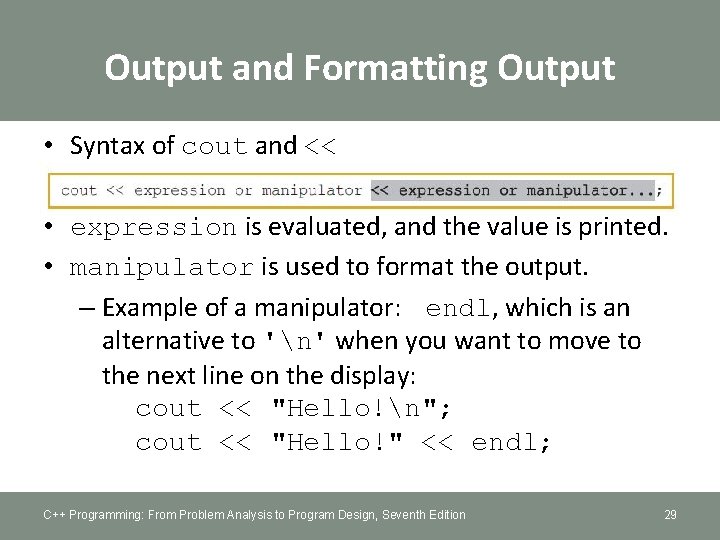
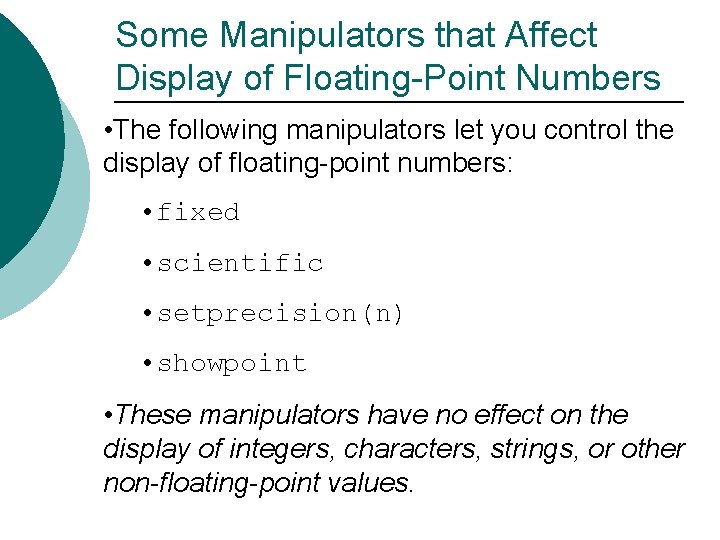
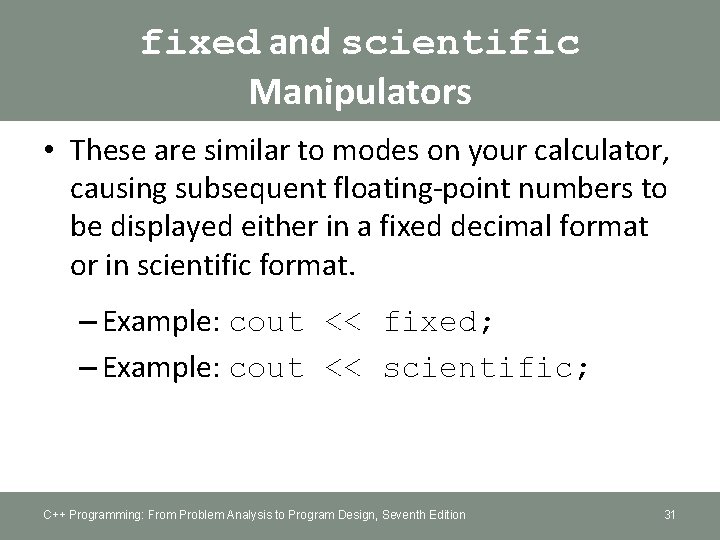
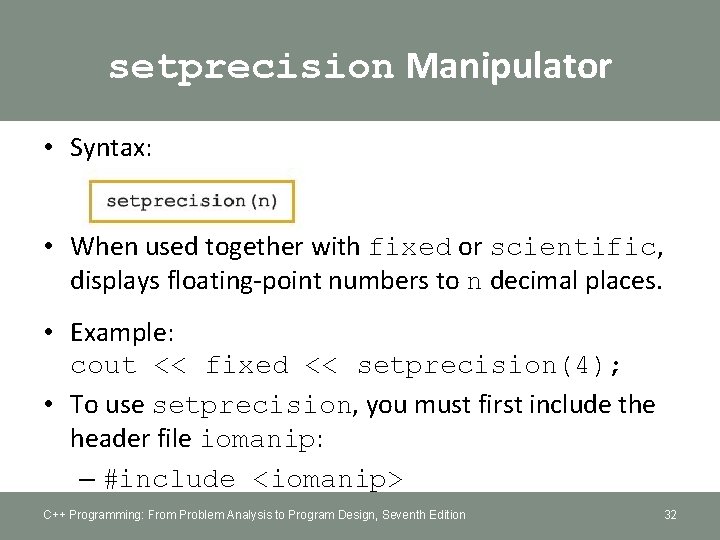
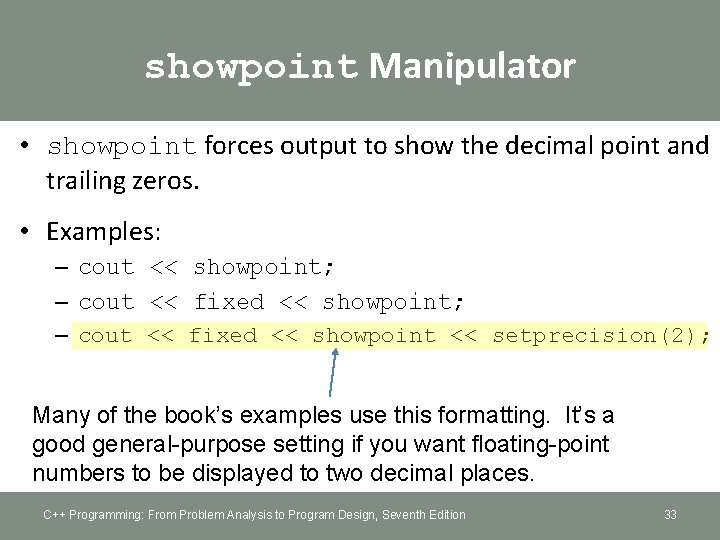
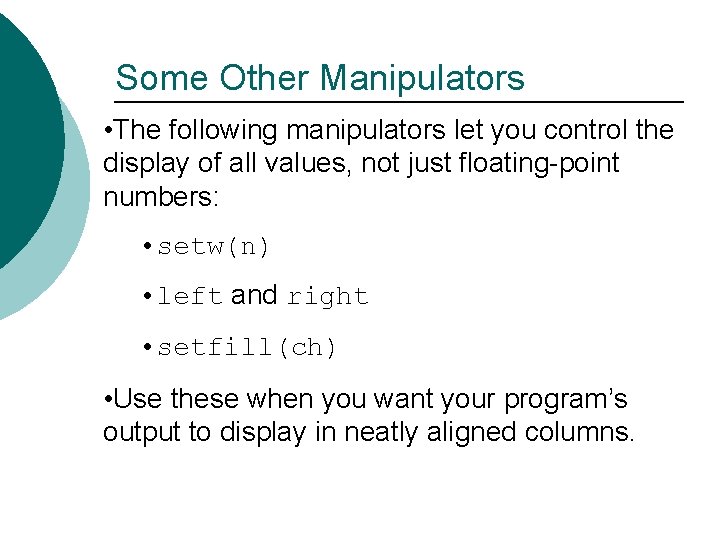
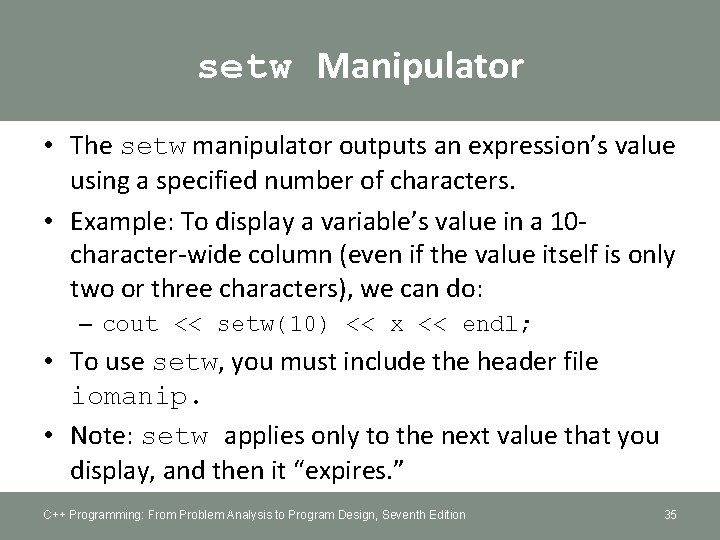
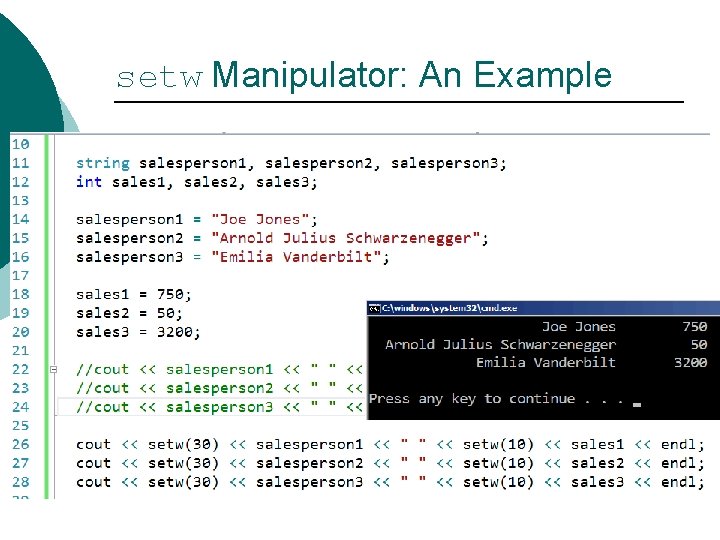
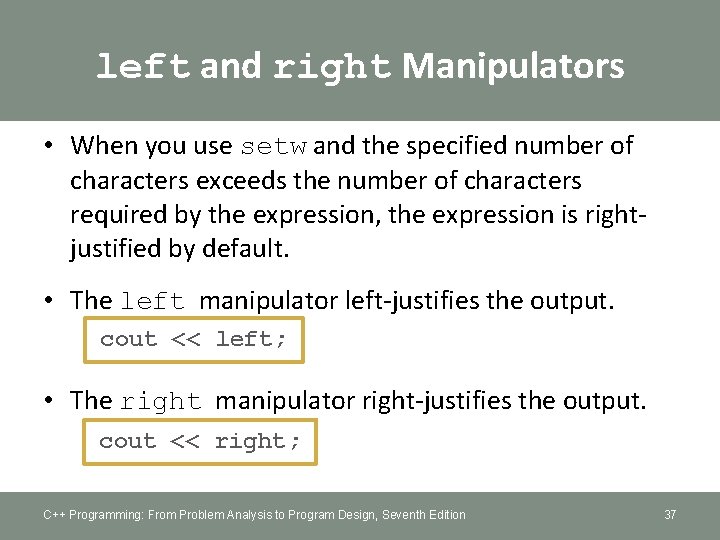
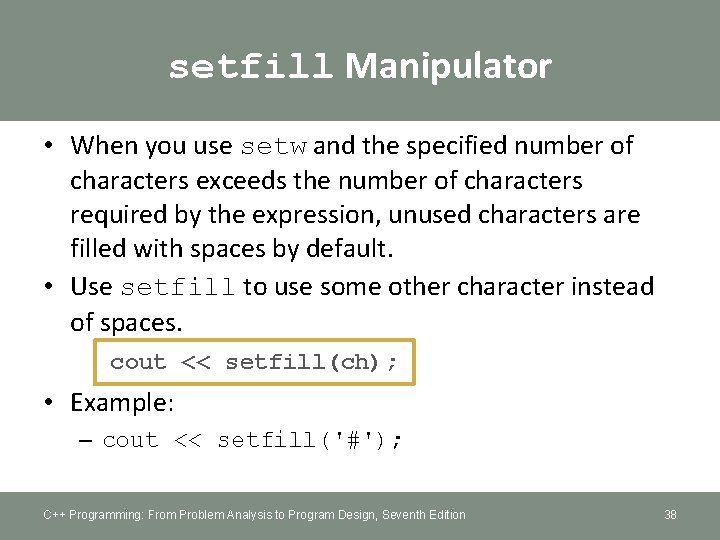
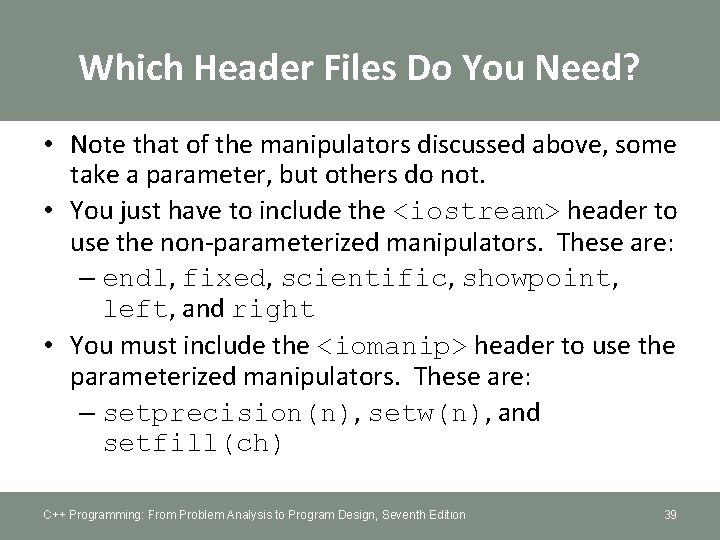
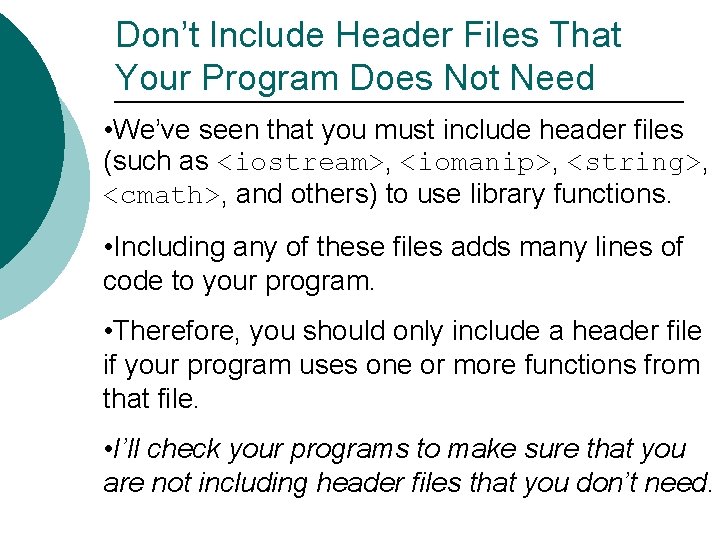
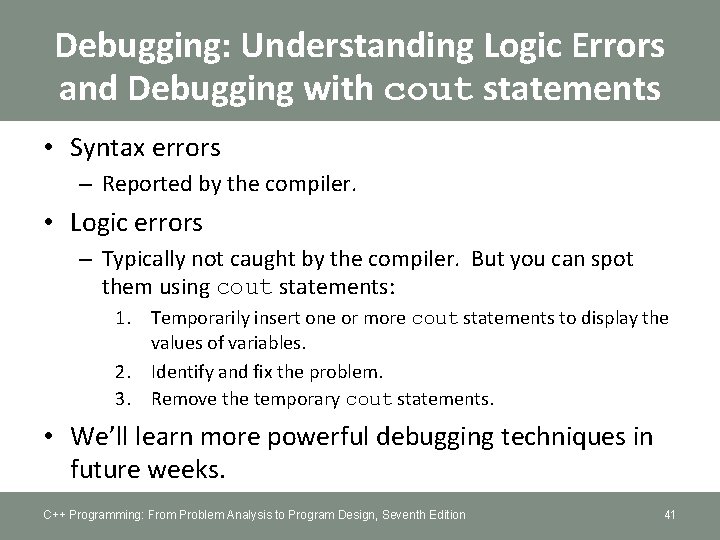
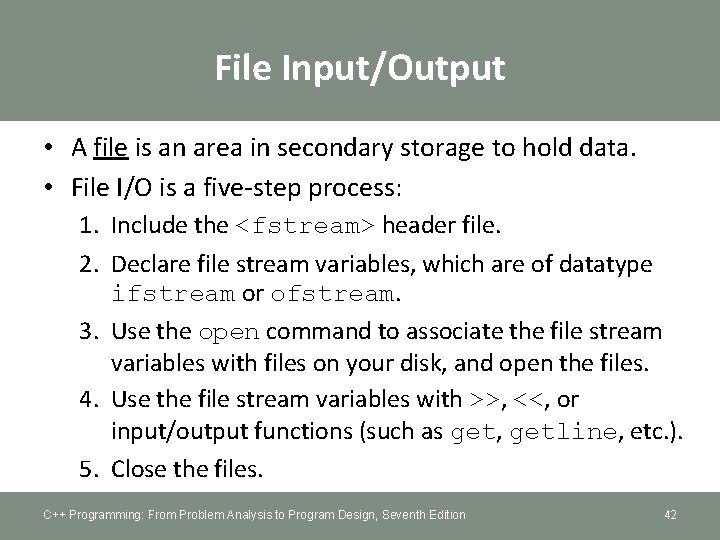
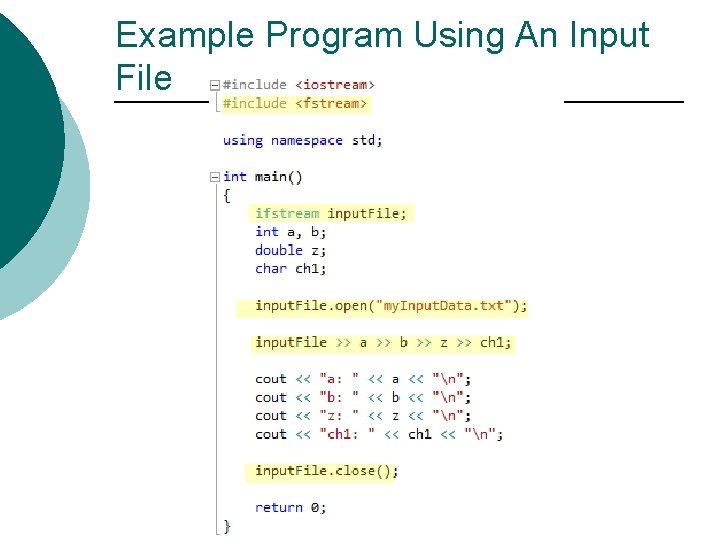
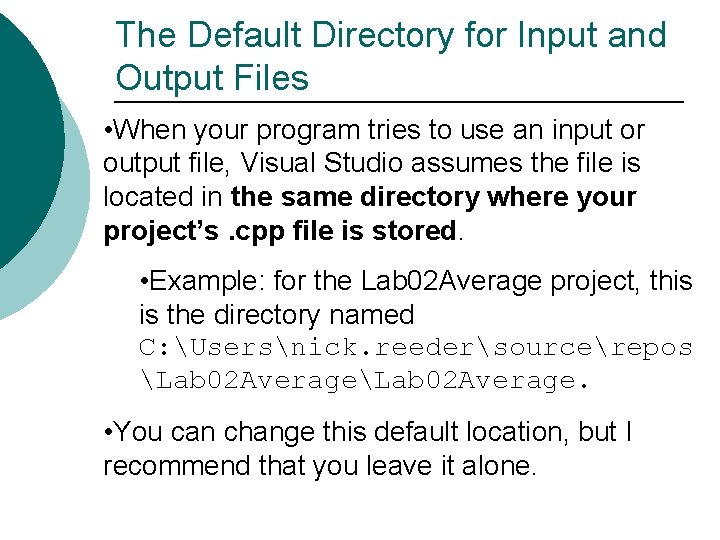
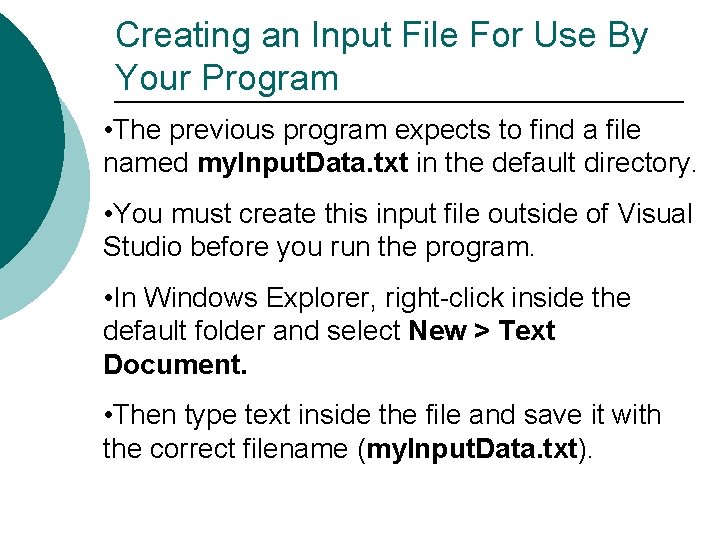
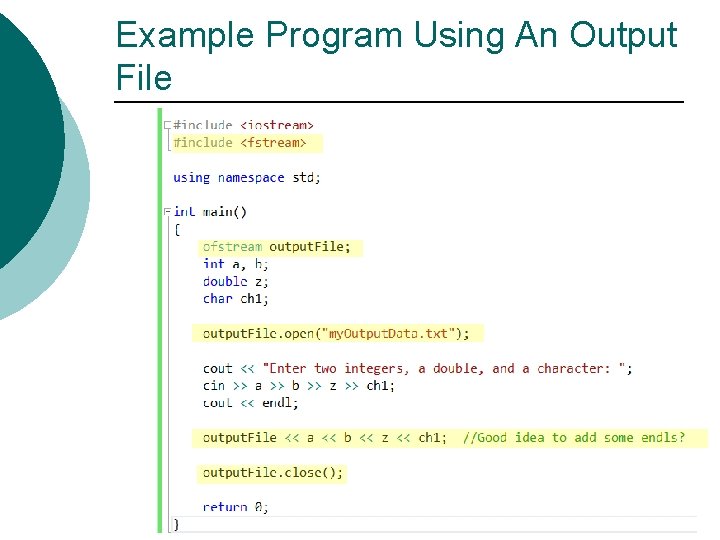
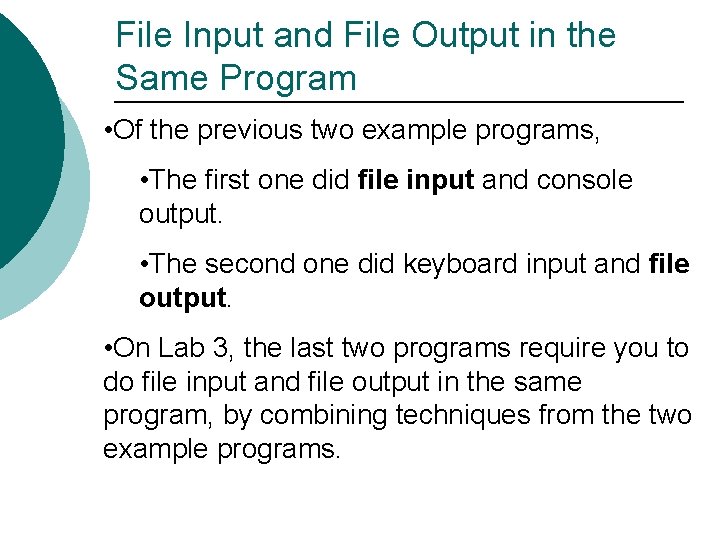
- Slides: 47
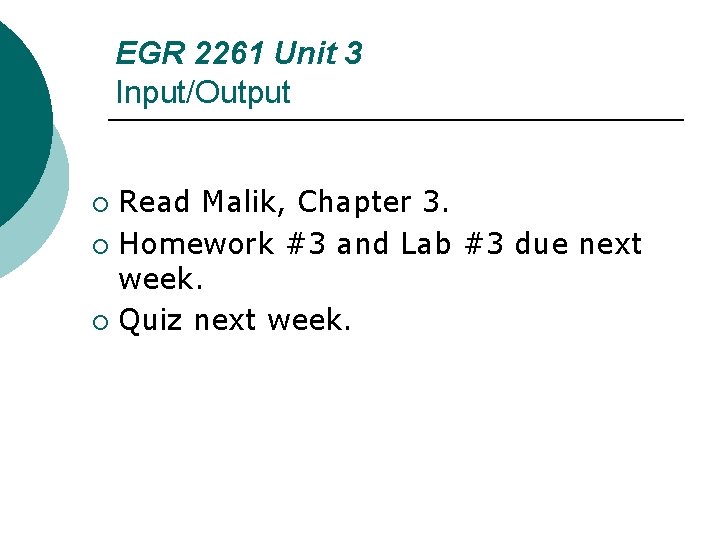
EGR 2261 Unit 3 Input/Output Read Malik, Chapter 3. ¡ Homework #3 and Lab #3 due next week. ¡ Quiz next week. ¡
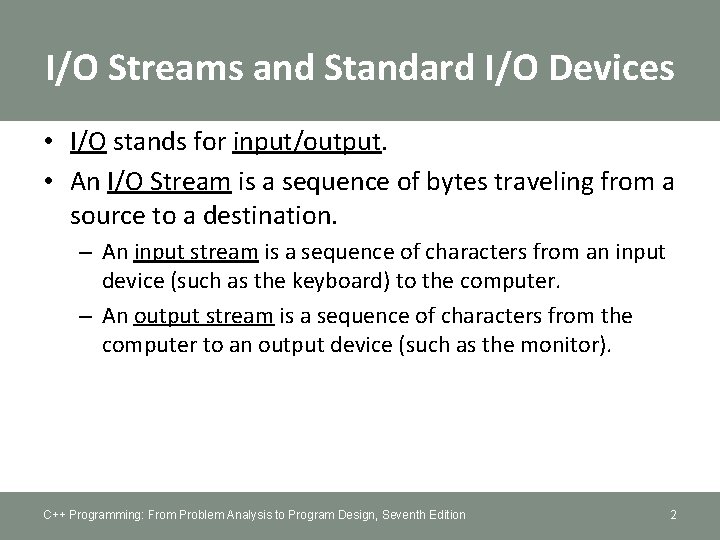
I/O Streams and Standard I/O Devices • I/O stands for input/output. • An I/O Stream is a sequence of bytes traveling from a source to a destination. – An input stream is a sequence of characters from an input device (such as the keyboard) to the computer. – An output stream is a sequence of characters from the computer to an output device (such as the monitor). C++ Programming: From Problem Analysis to Program Design, Seventh Edition 2
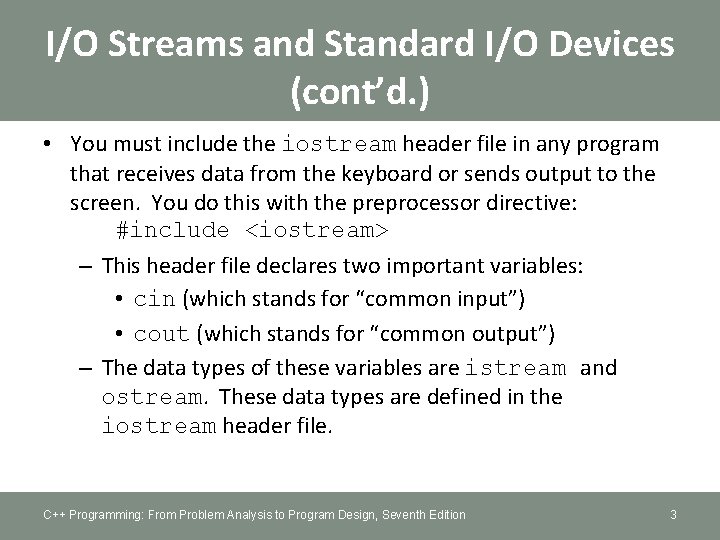
I/O Streams and Standard I/O Devices (cont’d. ) • You must include the iostream header file in any program that receives data from the keyboard or sends output to the screen. You do this with the preprocessor directive: #include <iostream> – This header file declares two important variables: • cin (which stands for “common input”) • cout (which stands for “common output”) – The data types of these variables are istream and ostream. These data types are defined in the iostream header file. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 3
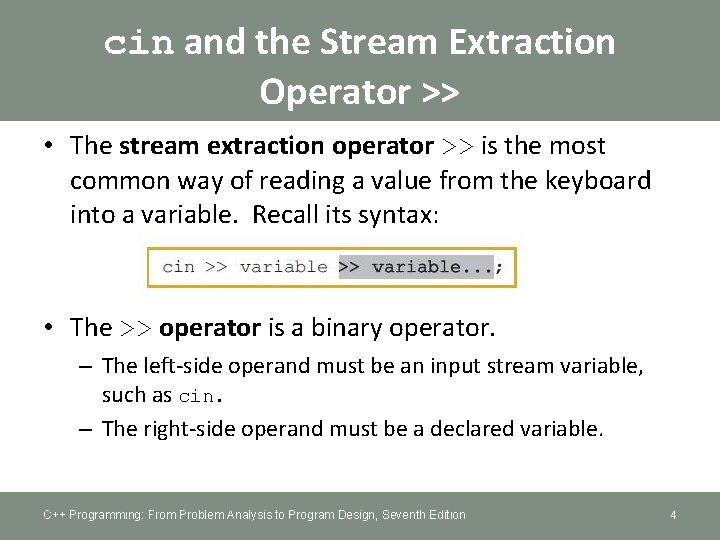
cin and the Stream Extraction Operator >> • The stream extraction operator >> is the most common way of reading a value from the keyboard into a variable. Recall its syntax: • The >> operator is a binary operator. – The left-side operand must be an input stream variable, such as cin. – The right-side operand must be a declared variable. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 4
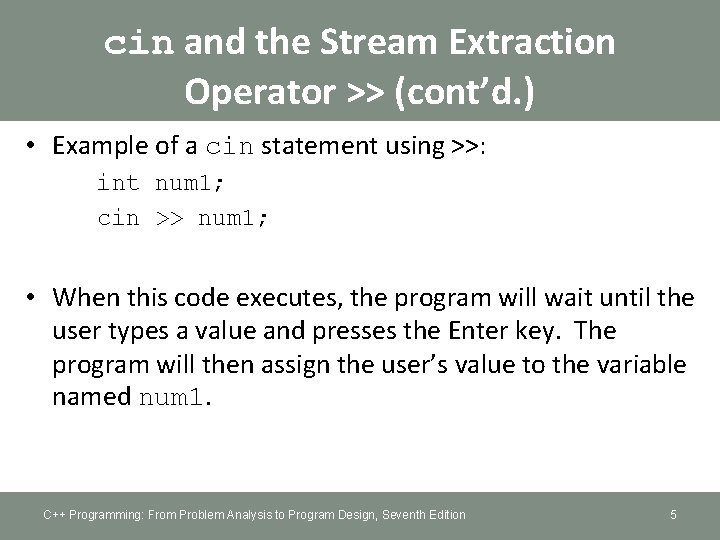
cin and the Stream Extraction Operator >> (cont’d. ) • Example of a cin statement using >>: int num 1; cin >> num 1; • When this code executes, the program will wait until the user types a value and presses the Enter key. The program will then assign the user’s value to the variable named num 1. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 5
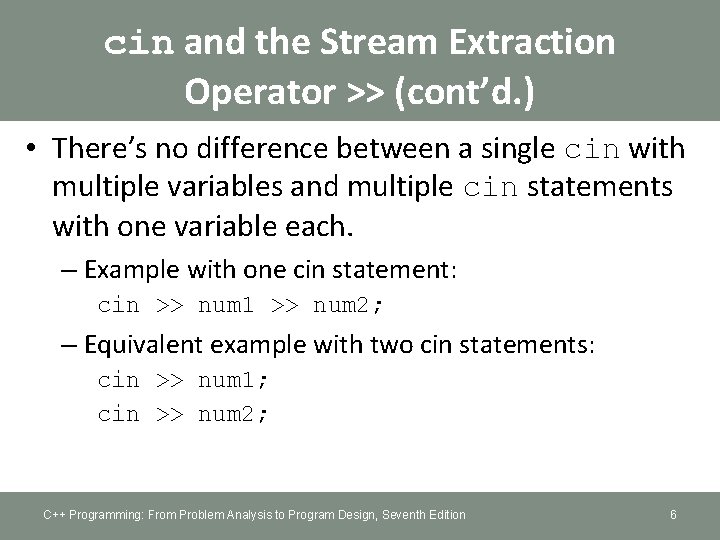
cin and the Stream Extraction Operator >> (cont’d. ) • There’s no difference between a single cin with multiple variables and multiple cin statements with one variable each. – Example with one cin statement: cin >> num 1 >> num 2; – Equivalent example with two cin statements: cin >> num 1; cin >> num 2; C++ Programming: From Problem Analysis to Program Design, Seventh Edition 6
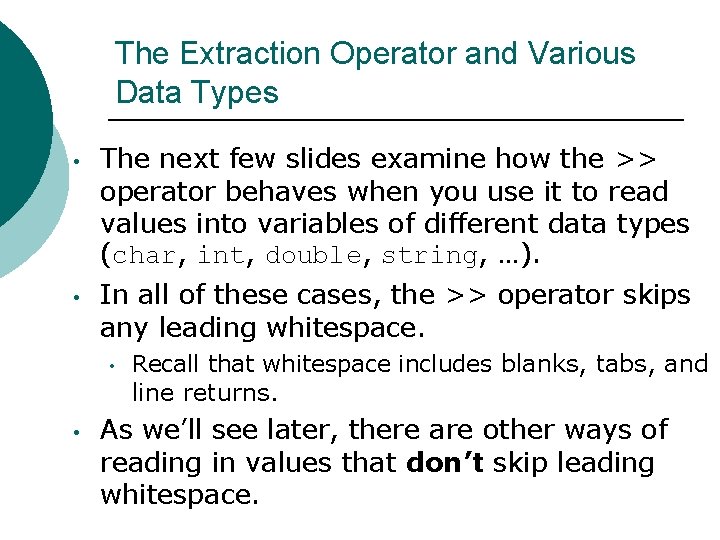
The Extraction Operator and Various Data Types • The next few slides examine how the >> operator behaves when you use it to read values into variables of different data types (char, int, double, string, …). • In all of these cases, the >> operator skips any leading whitespace. • • Recall that whitespace includes blanks, tabs, and line returns. As we’ll see later, there are other ways of reading in values that don’t skip leading whitespace.
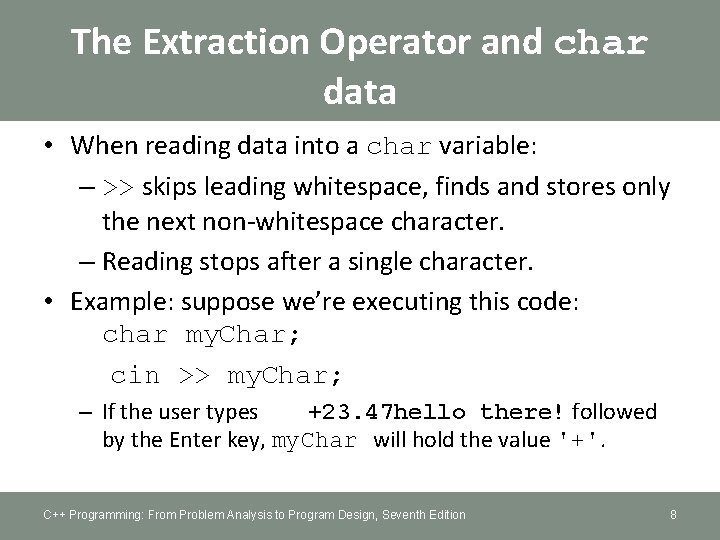
The Extraction Operator and char data • When reading data into a char variable: – >> skips leading whitespace, finds and stores only the next non-whitespace character. – Reading stops after a single character. • Example: suppose we’re executing this code: char my. Char; cin >> my. Char; – If the user types +23. 47 hello there! followed by the Enter key, my. Char will hold the value '+'. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 8
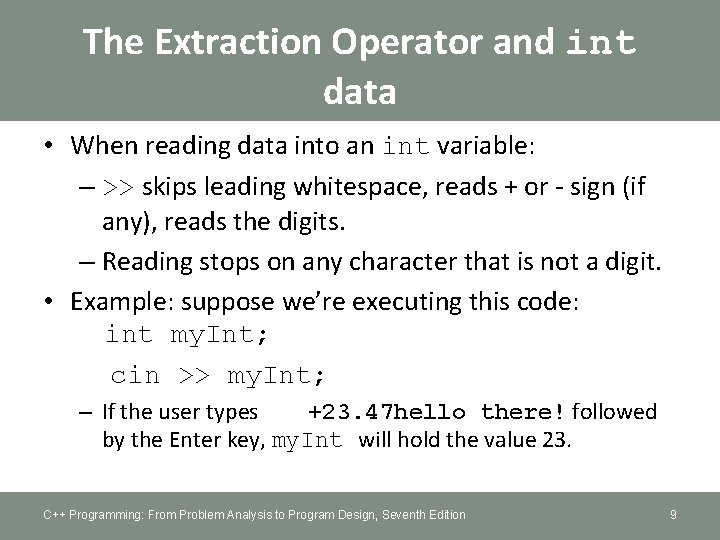
The Extraction Operator and int data • When reading data into an int variable: – >> skips leading whitespace, reads + or - sign (if any), reads the digits. – Reading stops on any character that is not a digit. • Example: suppose we’re executing this code: int my. Int; cin >> my. Int; – If the user types +23. 47 hello there! followed by the Enter key, my. Int will hold the value 23. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 9
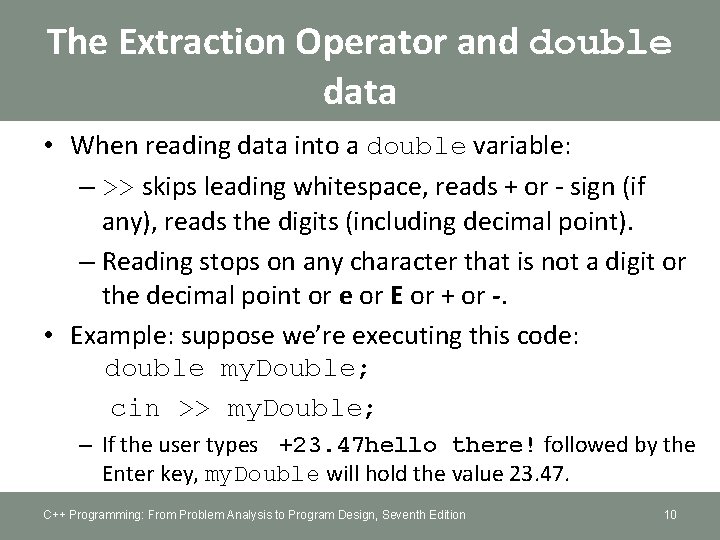
The Extraction Operator and double data • When reading data into a double variable: – >> skips leading whitespace, reads + or - sign (if any), reads the digits (including decimal point). – Reading stops on any character that is not a digit or the decimal point or e or E or + or -. • Example: suppose we’re executing this code: double my. Double; cin >> my. Double; – If the user types +23. 47 hello there! followed by the Enter key, my. Double will hold the value 23. 47. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 10
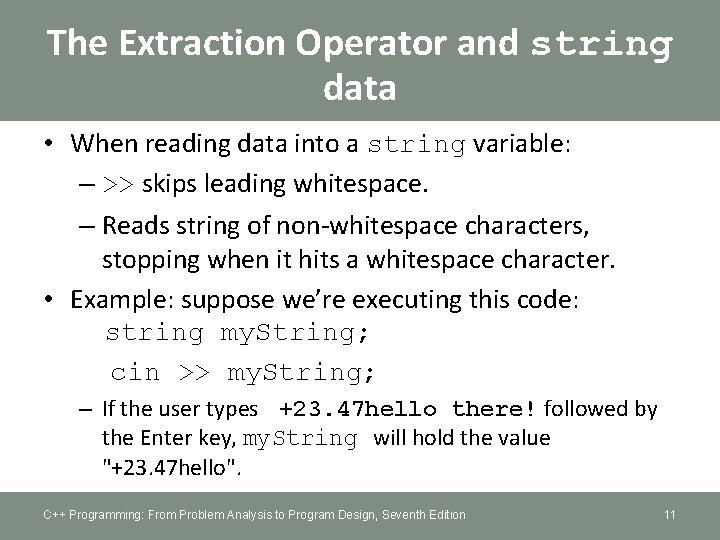
The Extraction Operator and string data • When reading data into a string variable: – >> skips leading whitespace. – Reads string of non-whitespace characters, stopping when it hits a whitespace character. • Example: suppose we’re executing this code: string my. String; cin >> my. String; – If the user types +23. 47 hello there! followed by the Enter key, my. String will hold the value "+23. 47 hello". C++ Programming: From Problem Analysis to Program Design, Seventh Edition 11
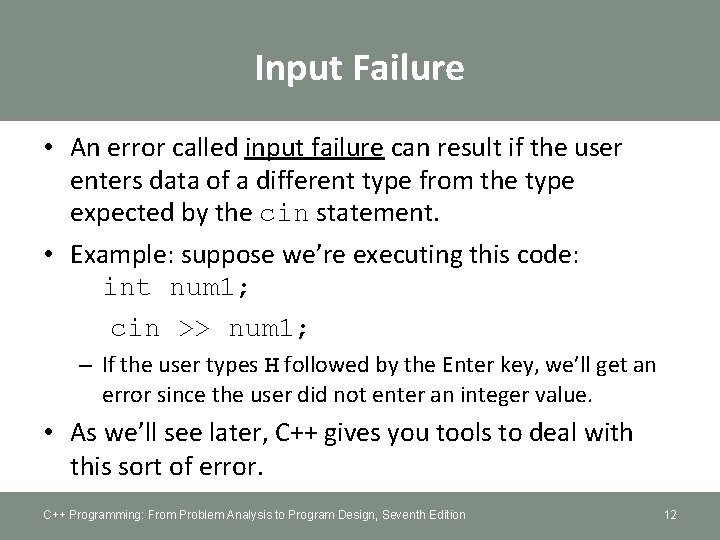
Input Failure • An error called input failure can result if the user enters data of a different type from the type expected by the cin statement. • Example: suppose we’re executing this code: int num 1; cin >> num 1; – If the user types H followed by the Enter key, we’ll get an error since the user did not enter an integer value. • As we’ll see later, C++ gives you tools to deal with this sort of error. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 12
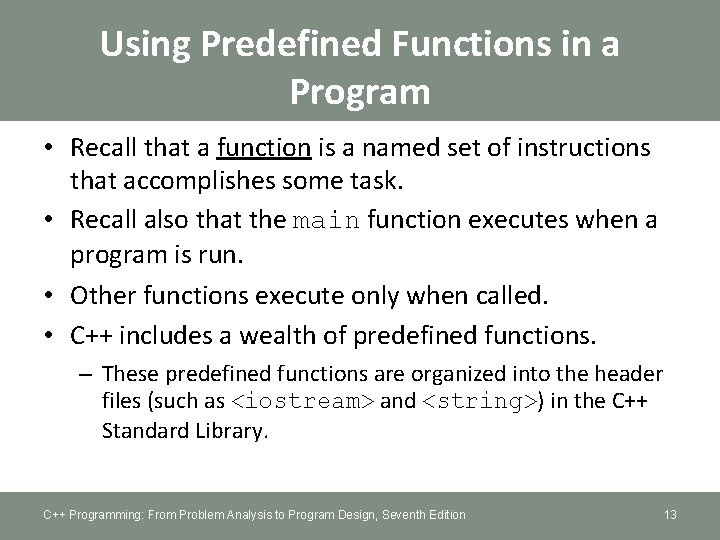
Using Predefined Functions in a Program • Recall that a function is a named set of instructions that accomplishes some task. • Recall also that the main function executes when a program is run. • Other functions execute only when called. • C++ includes a wealth of predefined functions. – These predefined functions are organized into the header files (such as <iostream> and <string>) in the C++ Standard Library. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 13
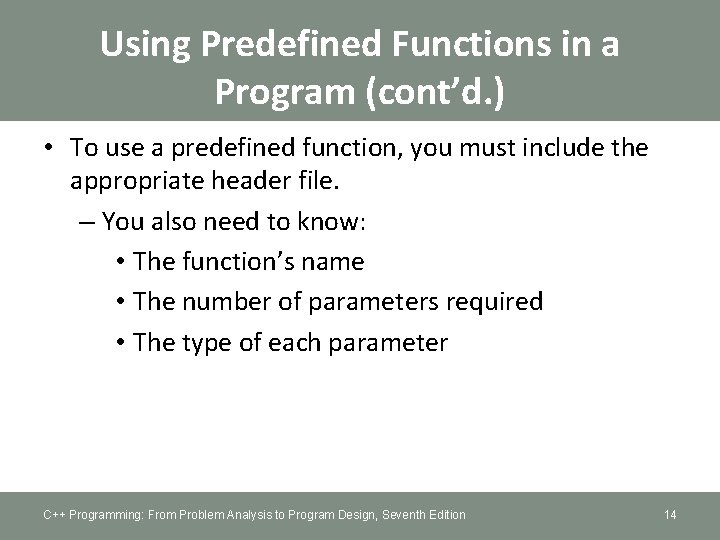
Using Predefined Functions in a Program (cont’d. ) • To use a predefined function, you must include the appropriate header file. – You also need to know: • The function’s name • The number of parameters required • The type of each parameter C++ Programming: From Problem Analysis to Program Design, Seventh Edition 14
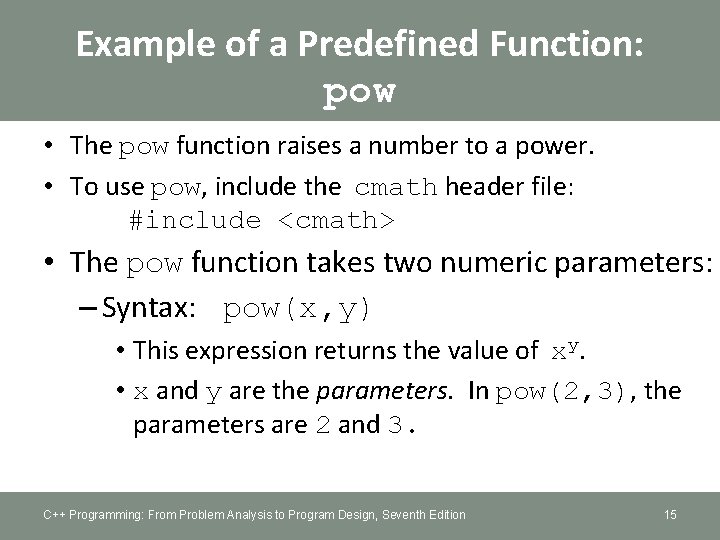
Example of a Predefined Function: pow • The pow function raises a number to a power. • To use pow, include the cmath header file: #include <cmath> • The pow function takes two numeric parameters: – Syntax: pow(x, y) • This expression returns the value of xy. • x and y are the parameters. In pow(2, 3), the parameters are 2 and 3. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 15
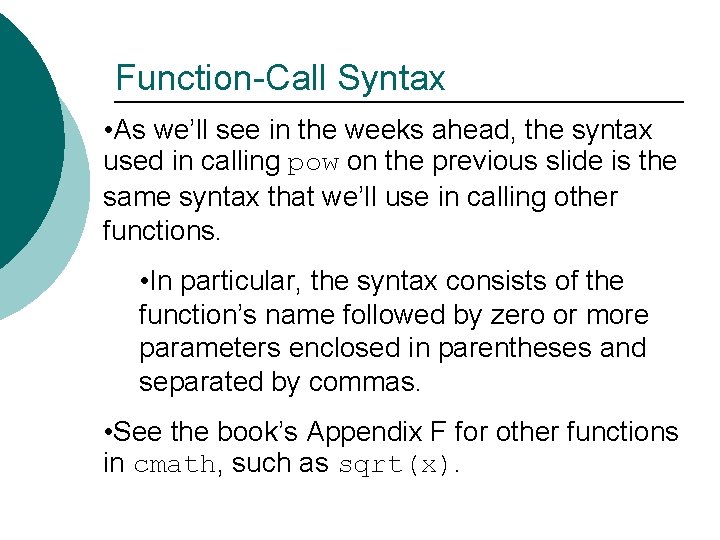
Function-Call Syntax • As we’ll see in the weeks ahead, the syntax used in calling pow on the previous slide is the same syntax that we’ll use in calling other functions. • In particular, the syntax consists of the function’s name followed by zero or more parameters enclosed in parentheses and separated by commas. • See the book’s Appendix F for other functions in cmath, such as sqrt(x).
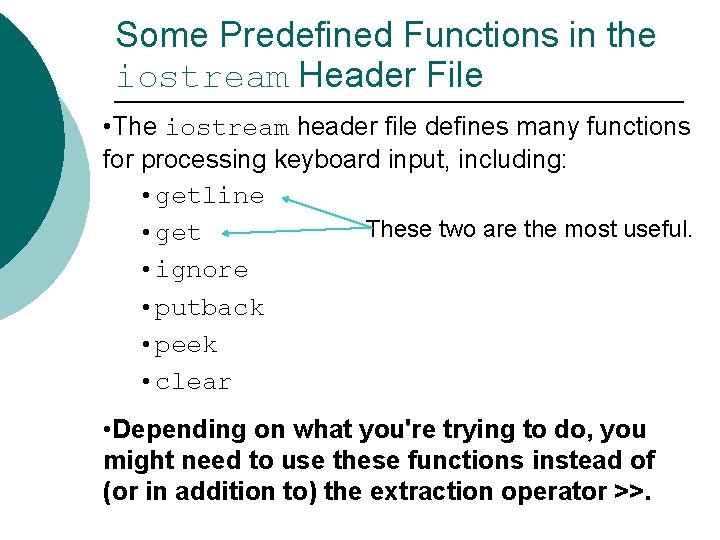
Some Predefined Functions in the iostream Header File • The iostream header file defines many functions for processing keyboard input, including: • getline These two are the most useful. • get • ignore • putback • peek • clear • Depending on what you're trying to do, you might need to use these functions instead of (or in addition to) the extraction operator >>.
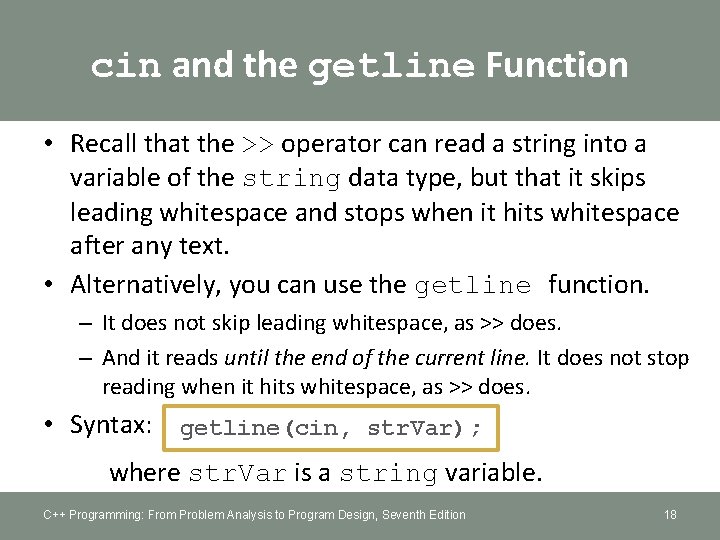
cin and the getline Function • Recall that the >> operator can read a string into a variable of the string data type, but that it skips leading whitespace and stops when it hits whitespace after any text. • Alternatively, you can use the getline function. – It does not skip leading whitespace, as >> does. – And it reads until the end of the current line. It does not stop reading when it hits whitespace, as >> does. • Syntax: getline(cin, str. Var); where str. Var is a string variable. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 18
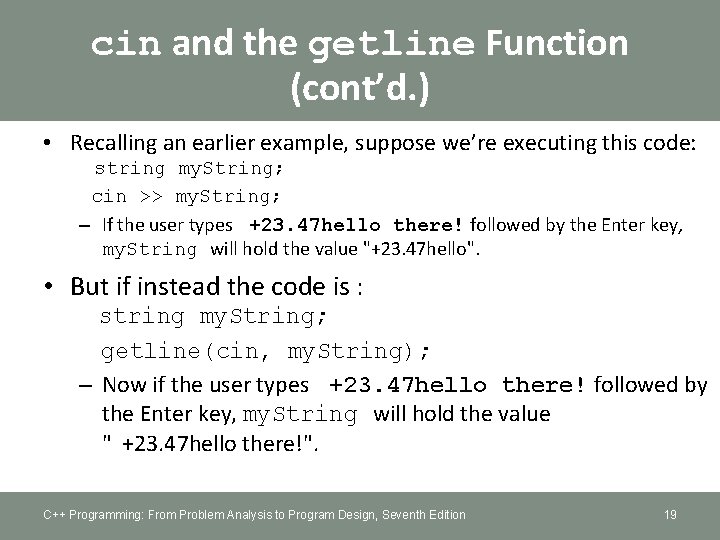
cin and the getline Function (cont’d. ) • Recalling an earlier example, suppose we’re executing this code: string my. String; cin >> my. String; – If the user types +23. 47 hello there! followed by the Enter key, my. String will hold the value "+23. 47 hello". • But if instead the code is : string my. String; getline(cin, my. String); – Now if the user types +23. 47 hello there! followed by the Enter key, my. String will hold the value " +23. 47 hello there!". C++ Programming: From Problem Analysis to Program Design, Seventh Edition 19
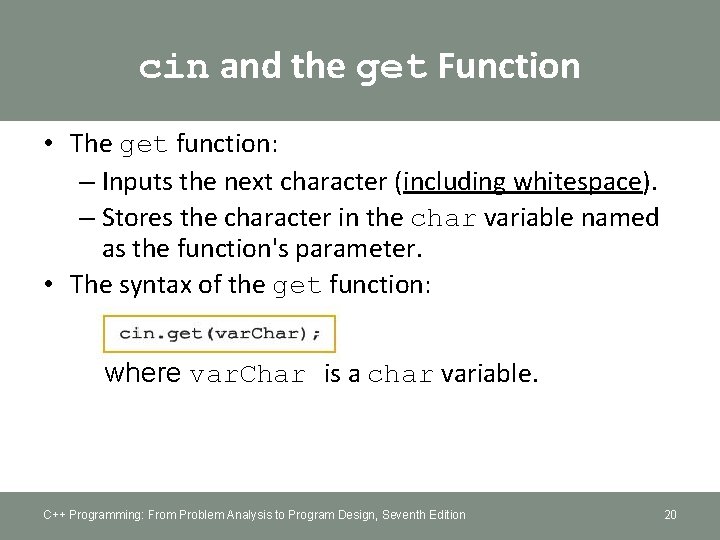
cin and the get Function • The get function: – Inputs the next character (including whitespace). – Stores the character in the char variable named as the function's parameter. • The syntax of the get function: where var. Char is a char variable. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 20
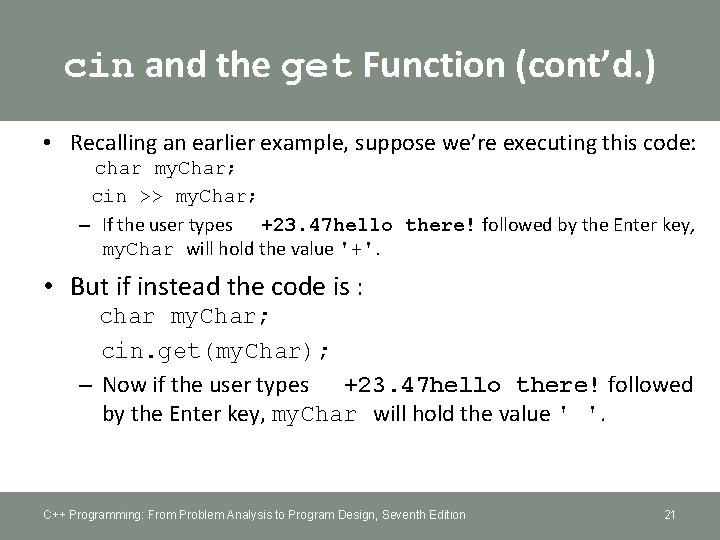
cin and the get Function (cont’d. ) • Recalling an earlier example, suppose we’re executing this code: char my. Char; cin >> my. Char; – If the user types +23. 47 hello there! followed by the Enter key, my. Char will hold the value '+'. • But if instead the code is : char my. Char; cin. get(my. Char); – Now if the user types +23. 47 hello there! followed by the Enter key, my. Char will hold the value ' '. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 21
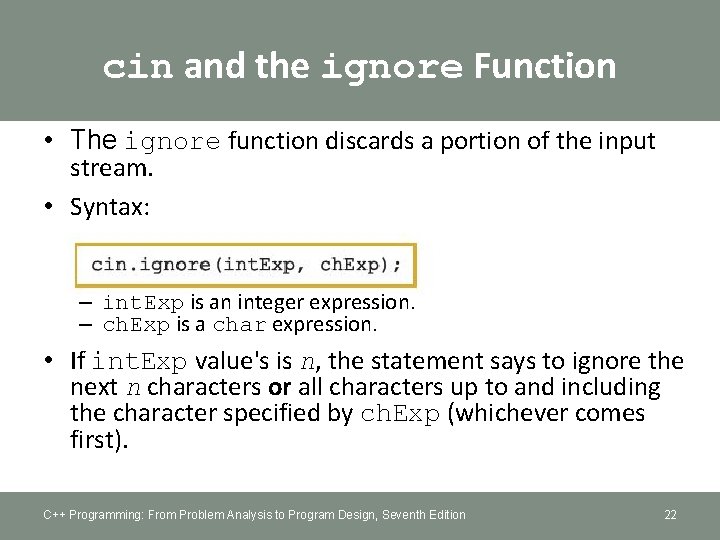
cin and the ignore Function • The ignore function discards a portion of the input stream. • Syntax: – int. Exp is an integer expression. – ch. Exp is a char expression. • If int. Exp value's is n, the statement says to ignore the next n characters or all characters up to and including the character specified by ch. Exp (whichever comes first). C++ Programming: From Problem Analysis to Program Design, Seventh Edition 22
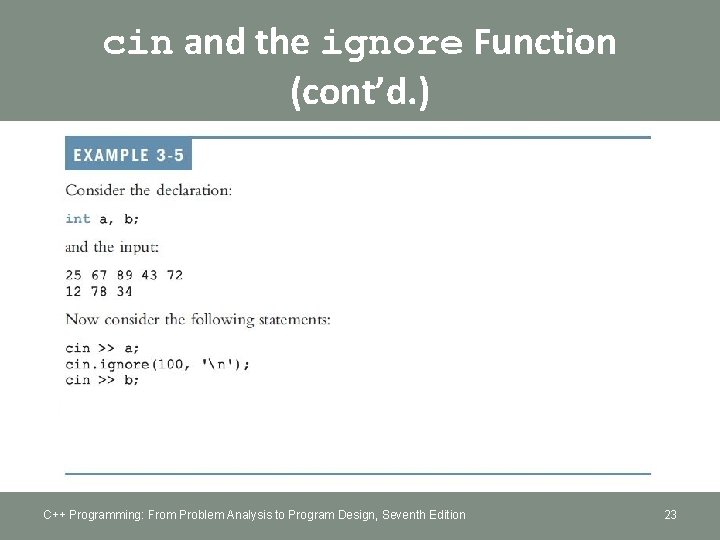
cin and the ignore Function (cont’d. ) C++ Programming: From Problem Analysis to Program Design, Seventh Edition 23
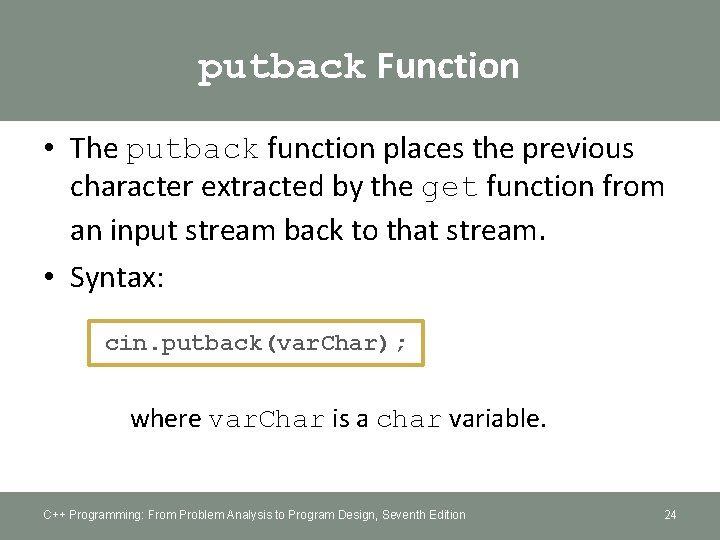
putback Function • The putback function places the previous character extracted by the get function from an input stream back to that stream. • Syntax: cin. putback(var. Char); where var. Char is a char variable. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 24
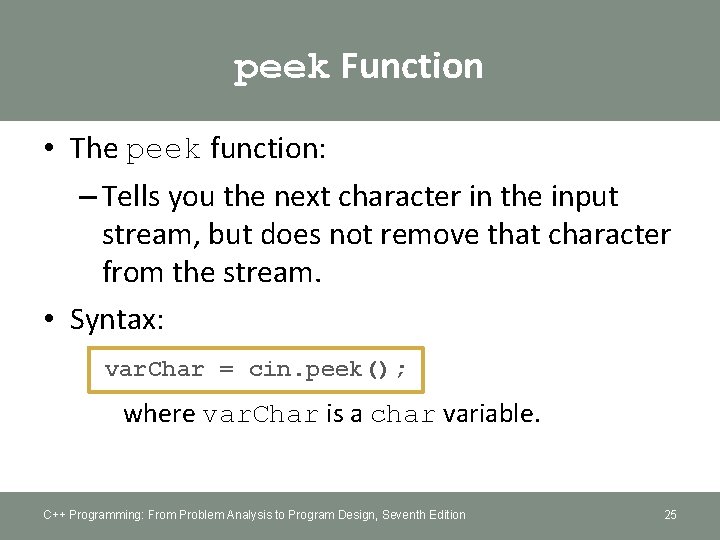
peek Function • The peek function: – Tells you the next character in the input stream, but does not remove that character from the stream. • Syntax: var. Char = cin. peek(); where var. Char is a char variable. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 25
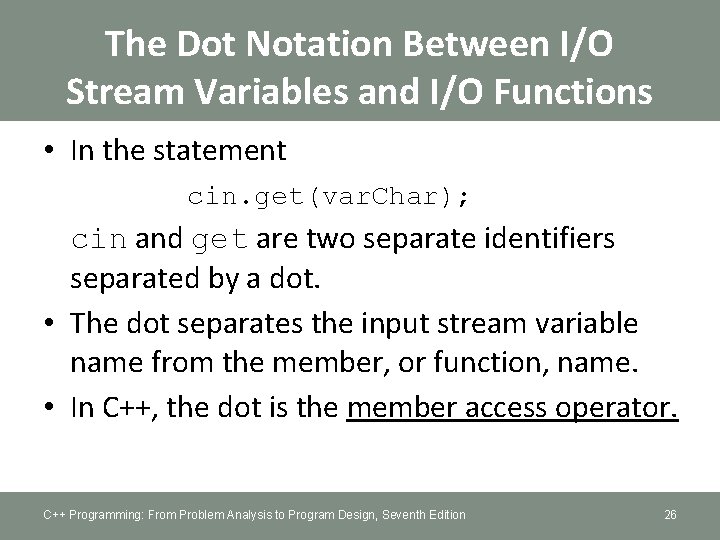
The Dot Notation Between I/O Stream Variables and I/O Functions • In the statement cin. get(var. Char); cin and get are two separate identifiers separated by a dot. • The dot separates the input stream variable name from the member, or function, name. • In C++, the dot is the member access operator. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 26
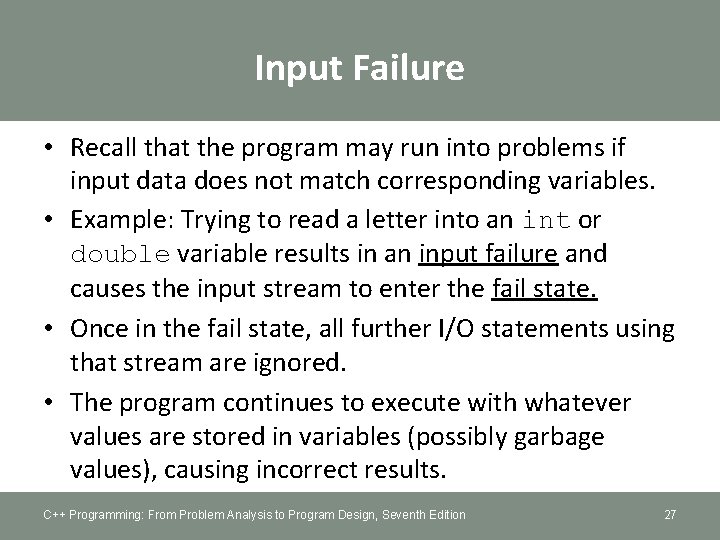
Input Failure • Recall that the program may run into problems if input data does not match corresponding variables. • Example: Trying to read a letter into an int or double variable results in an input failure and causes the input stream to enter the fail state. • Once in the fail state, all further I/O statements using that stream are ignored. • The program continues to execute with whatever values are stored in variables (possibly garbage values), causing incorrect results. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 27
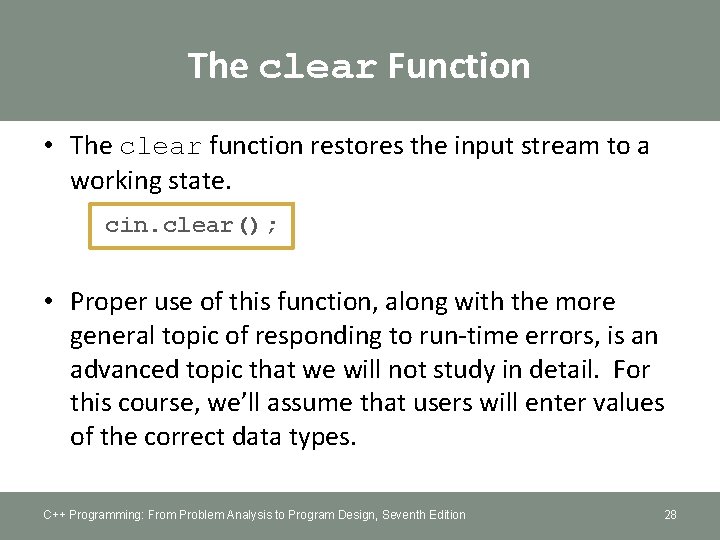
The clear Function • The clear function restores the input stream to a working state. cin. clear(); • Proper use of this function, along with the more general topic of responding to run-time errors, is an advanced topic that we will not study in detail. For this course, we’ll assume that users will enter values of the correct data types. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 28
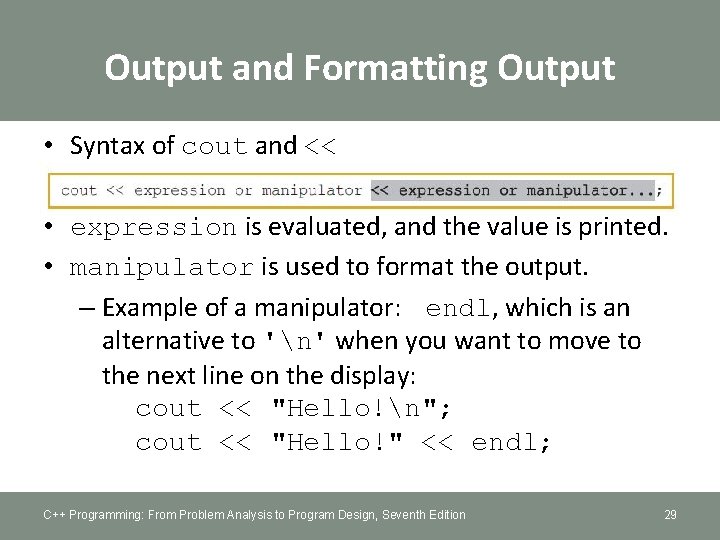
Output and Formatting Output • Syntax of cout and << • expression is evaluated, and the value is printed. • manipulator is used to format the output. – Example of a manipulator: endl, which is an alternative to 'n' when you want to move to the next line on the display: cout << "Hello!n"; cout << "Hello!" << endl; C++ Programming: From Problem Analysis to Program Design, Seventh Edition 29
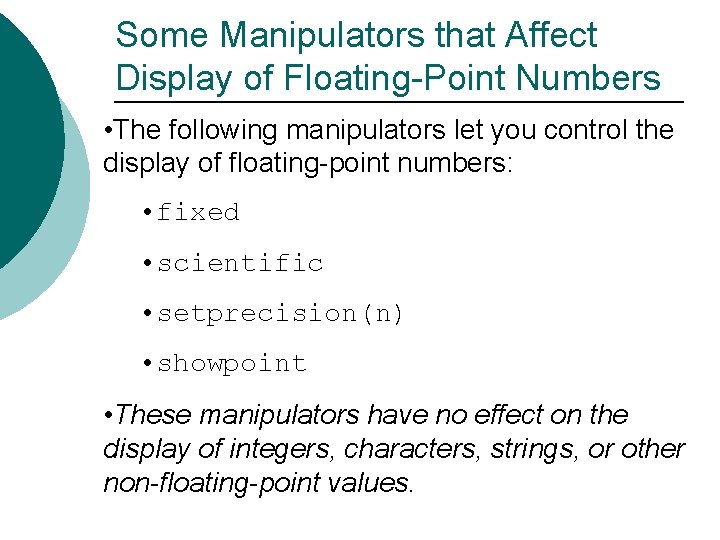
Some Manipulators that Affect Display of Floating-Point Numbers • The following manipulators let you control the display of floating-point numbers: • fixed • scientific • setprecision(n) • showpoint • These manipulators have no effect on the display of integers, characters, strings, or other non-floating-point values.
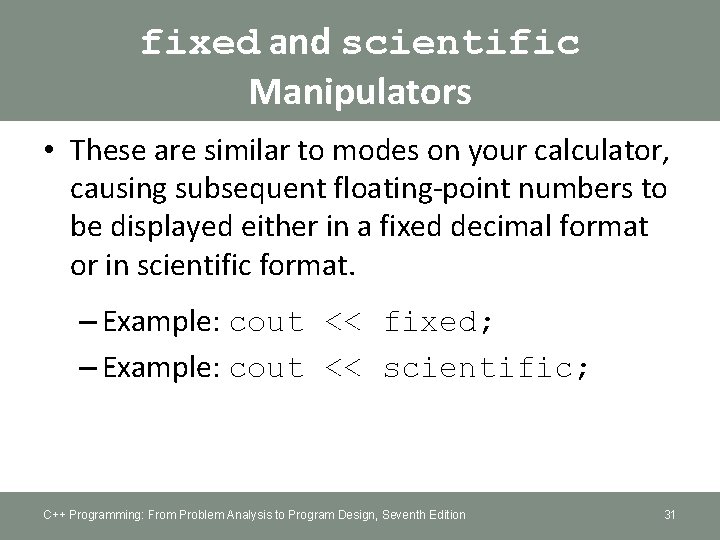
fixed and scientific Manipulators • These are similar to modes on your calculator, causing subsequent floating-point numbers to be displayed either in a fixed decimal format or in scientific format. – Example: cout << fixed; – Example: cout << scientific; C++ Programming: From Problem Analysis to Program Design, Seventh Edition 31
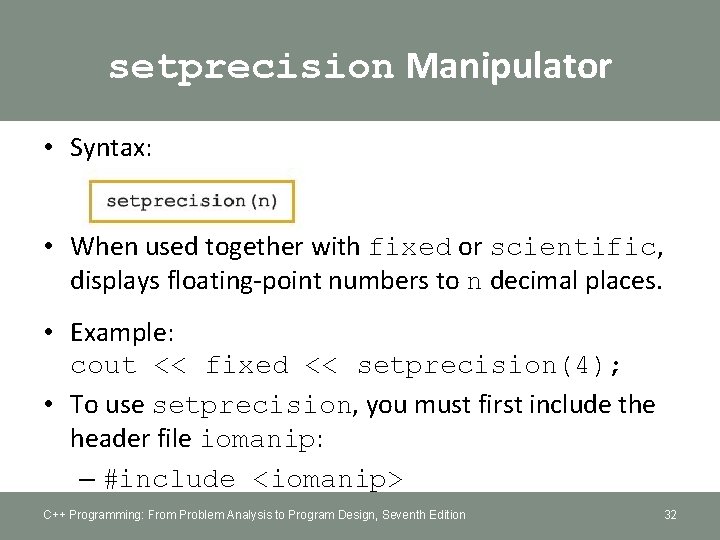
setprecision Manipulator • Syntax: • When used together with fixed or scientific, displays floating-point numbers to n decimal places. • Example: cout << fixed << setprecision(4); • To use setprecision, you must first include the header file iomanip: – #include <iomanip> C++ Programming: From Problem Analysis to Program Design, Seventh Edition 32
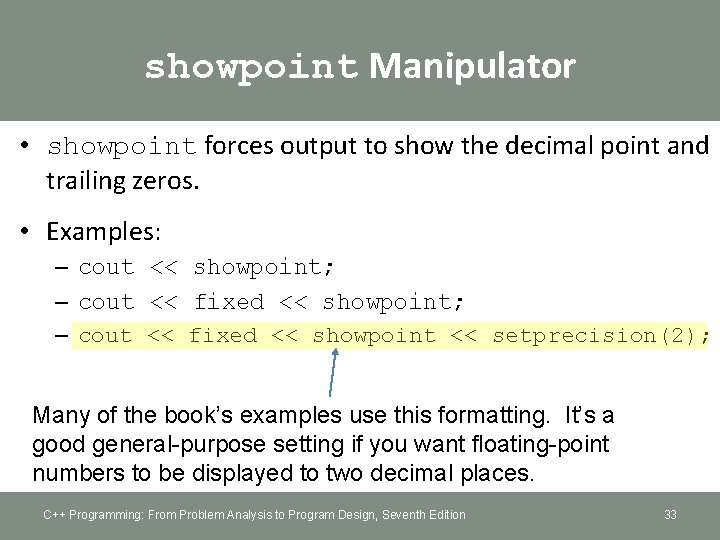
showpoint Manipulator • showpoint forces output to show the decimal point and trailing zeros. • Examples: – cout << showpoint; – cout << fixed << showpoint << setprecision(2); Many of the book’s examples use this formatting. It’s a good general-purpose setting if you want floating-point numbers to be displayed to two decimal places. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 33
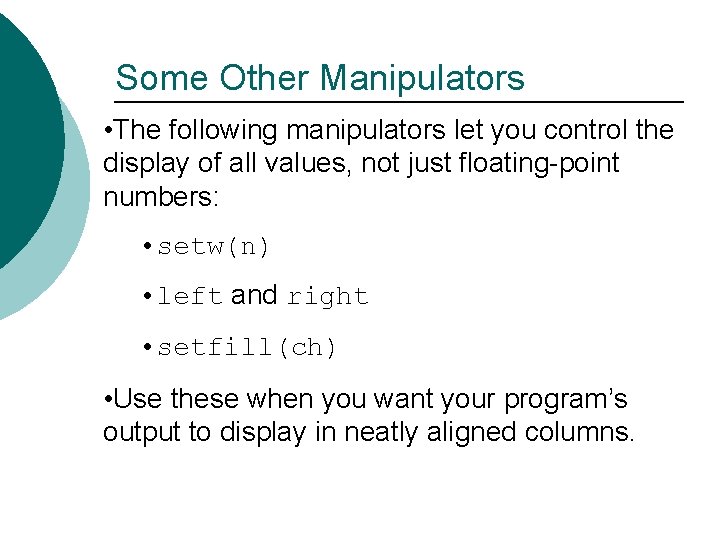
Some Other Manipulators • The following manipulators let you control the display of all values, not just floating-point numbers: • setw(n) • left and right • setfill(ch) • Use these when you want your program’s output to display in neatly aligned columns.
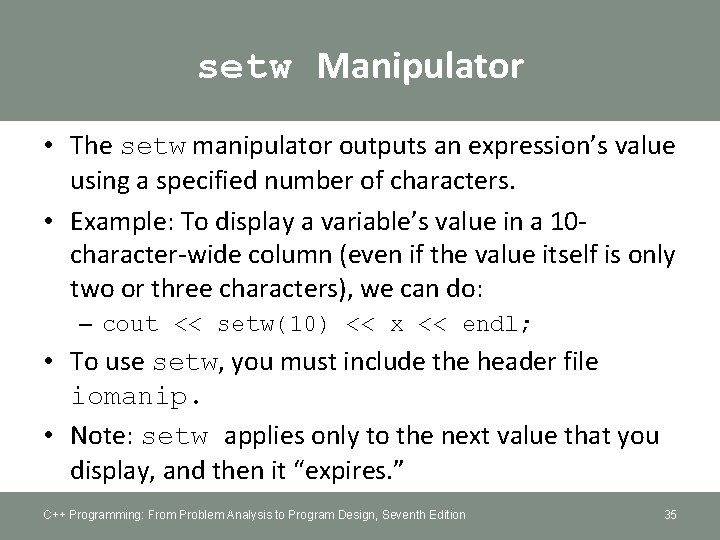
setw Manipulator • The setw manipulator outputs an expression’s value using a specified number of characters. • Example: To display a variable’s value in a 10 character-wide column (even if the value itself is only two or three characters), we can do: – cout << setw(10) << x << endl; • To use setw, you must include the header file iomanip. • Note: setw applies only to the next value that you display, and then it “expires. ” C++ Programming: From Problem Analysis to Program Design, Seventh Edition 35
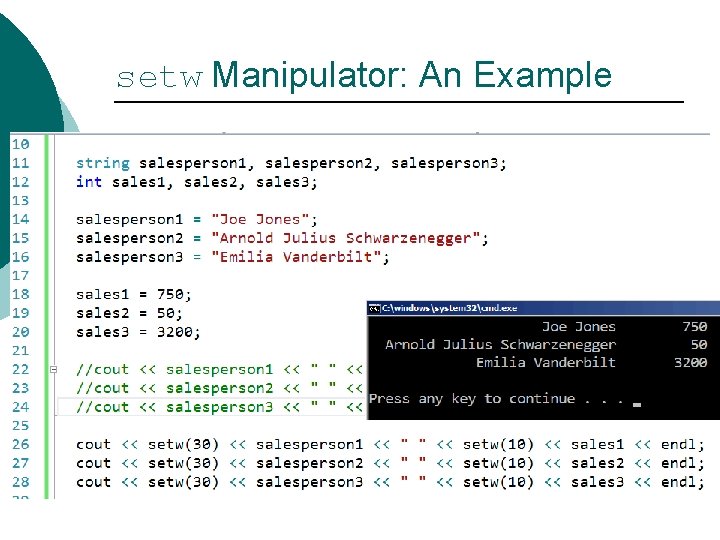
setw Manipulator: An Example
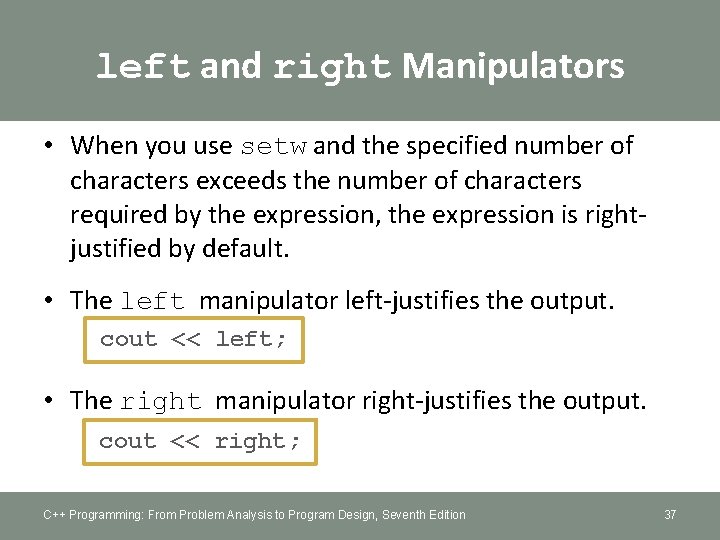
left and right Manipulators • When you use setw and the specified number of characters exceeds the number of characters required by the expression, the expression is rightjustified by default. • The left manipulator left-justifies the output. cout << left; • The right manipulator right-justifies the output. cout << right; C++ Programming: From Problem Analysis to Program Design, Seventh Edition 37
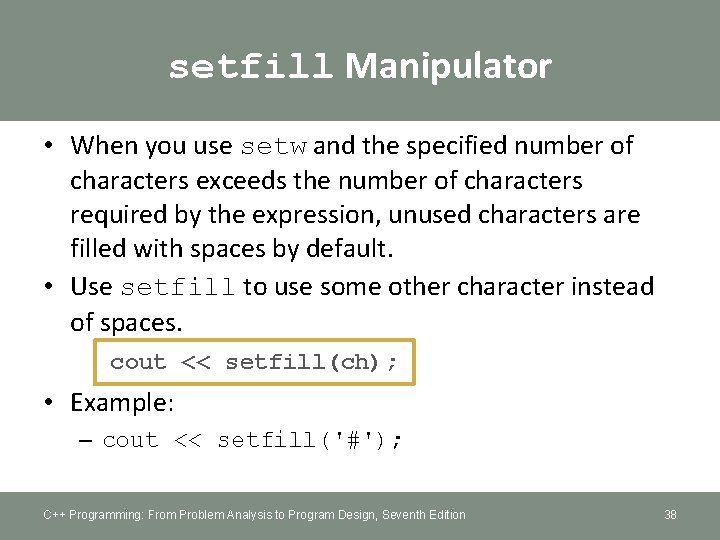
setfill Manipulator • When you use setw and the specified number of characters exceeds the number of characters required by the expression, unused characters are filled with spaces by default. • Use setfill to use some other character instead of spaces. cout << setfill(ch); • Example: – cout << setfill('#'); C++ Programming: From Problem Analysis to Program Design, Seventh Edition 38
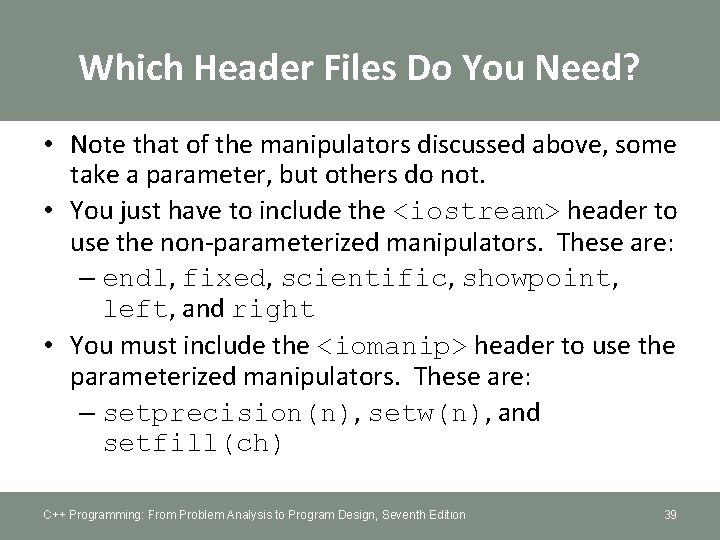
Which Header Files Do You Need? • Note that of the manipulators discussed above, some take a parameter, but others do not. • You just have to include the <iostream> header to use the non-parameterized manipulators. These are: – endl, fixed, scientific, showpoint, left, and right • You must include the <iomanip> header to use the parameterized manipulators. These are: – setprecision(n), setw(n), and setfill(ch) C++ Programming: From Problem Analysis to Program Design, Seventh Edition 39
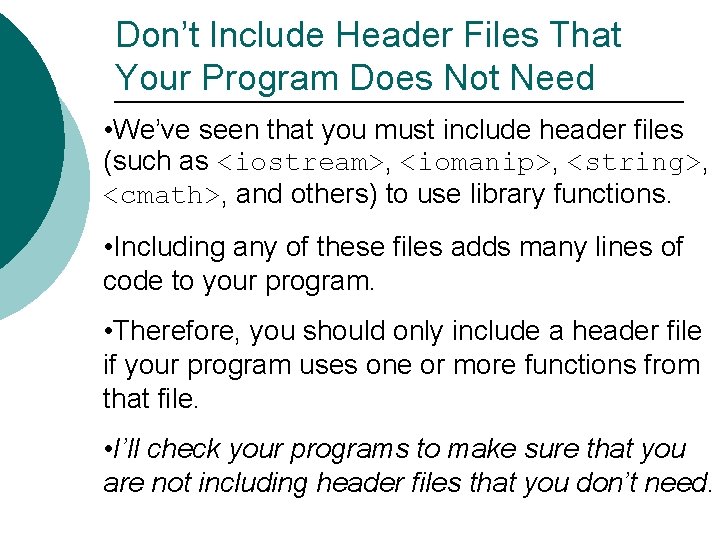
Don’t Include Header Files That Your Program Does Not Need • We’ve seen that you must include header files (such as <iostream>, <iomanip>, <string>, <cmath>, and others) to use library functions. • Including any of these files adds many lines of code to your program. • Therefore, you should only include a header file if your program uses one or more functions from that file. • I’ll check your programs to make sure that you are not including header files that you don’t need.
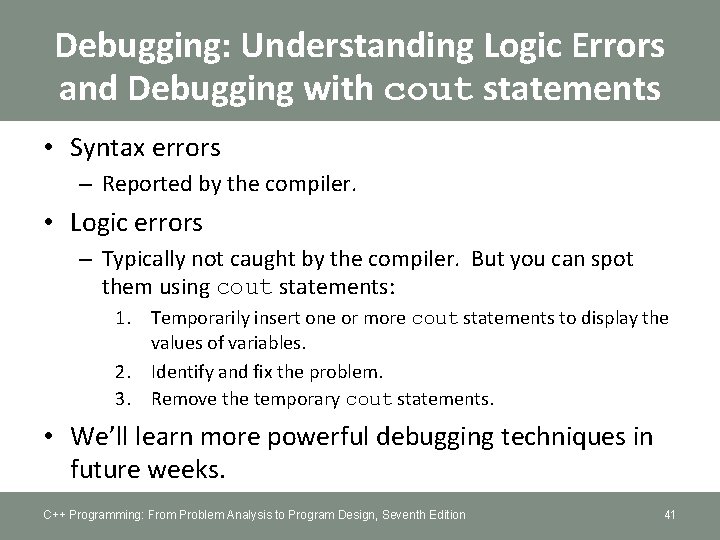
Debugging: Understanding Logic Errors and Debugging with cout statements • Syntax errors – Reported by the compiler. • Logic errors – Typically not caught by the compiler. But you can spot them using cout statements: 1. Temporarily insert one or more cout statements to display the values of variables. 2. Identify and fix the problem. 3. Remove the temporary cout statements. • We’ll learn more powerful debugging techniques in future weeks. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 41
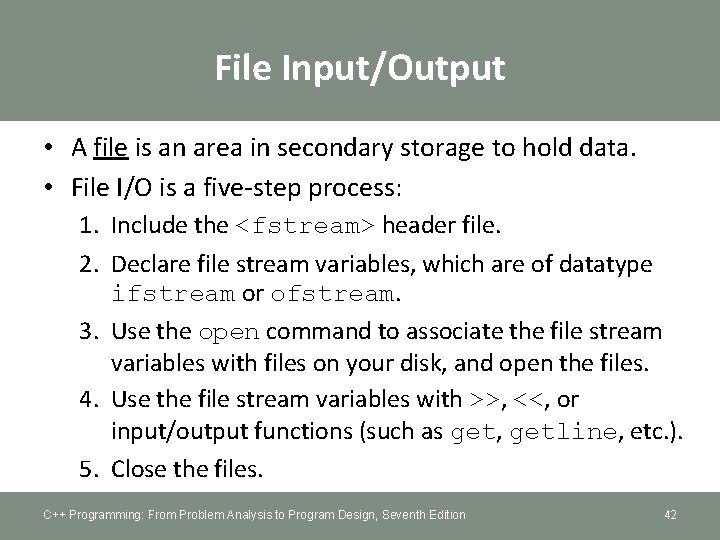
File Input/Output • A file is an area in secondary storage to hold data. • File I/O is a five-step process: 1. Include the <fstream> header file. 2. Declare file stream variables, which are of datatype ifstream or ofstream. 3. Use the open command to associate the file stream variables with files on your disk, and open the files. 4. Use the file stream variables with >>, <<, or input/output functions (such as get, getline, etc. ). 5. Close the files. C++ Programming: From Problem Analysis to Program Design, Seventh Edition 42
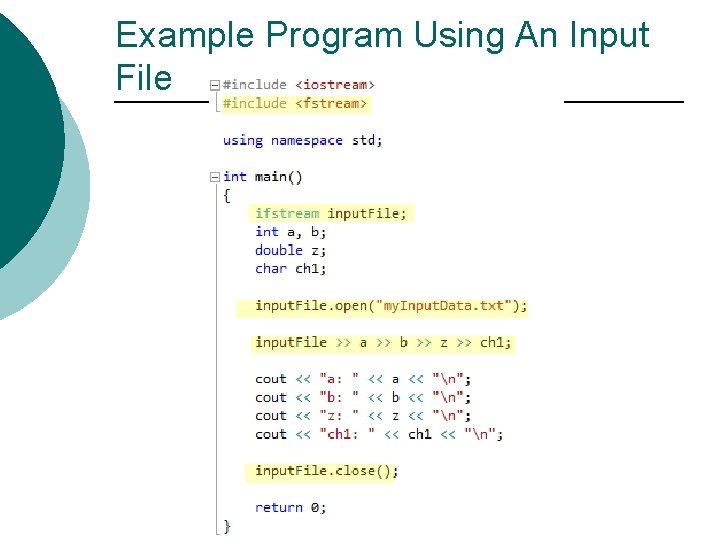
Example Program Using An Input File
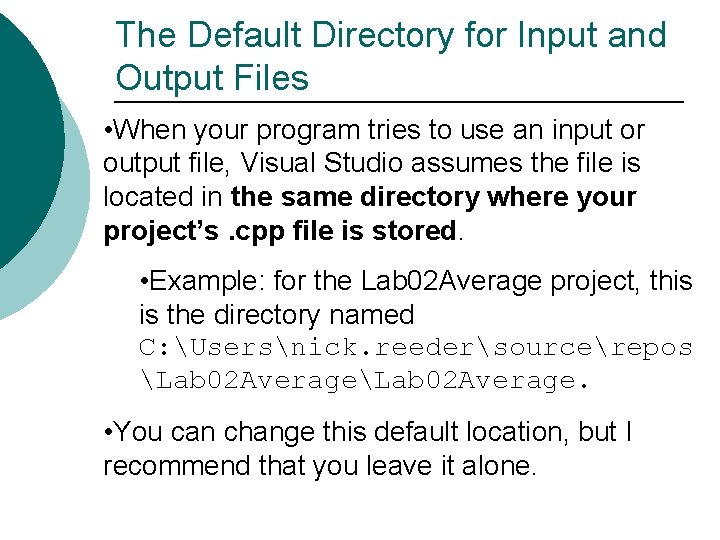
The Default Directory for Input and Output Files • When your program tries to use an input or output file, Visual Studio assumes the file is located in the same directory where your project’s. cpp file is stored. • Example: for the Lab 02 Average project, this is the directory named C: Usersnick. reedersourcerepos Lab 02 Average. • You can change this default location, but I recommend that you leave it alone.
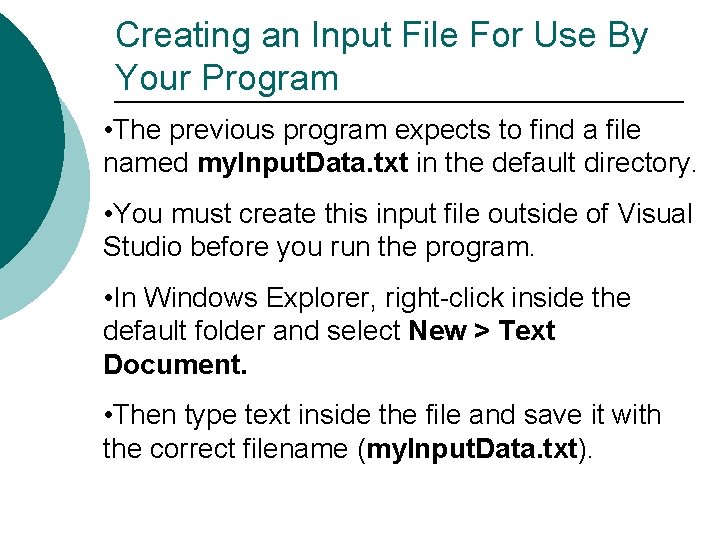
Creating an Input File For Use By Your Program • The previous program expects to find a file named my. Input. Data. txt in the default directory. • You must create this input file outside of Visual Studio before you run the program. • In Windows Explorer, right-click inside the default folder and select New > Text Document. • Then type text inside the file and save it with the correct filename (my. Input. Data. txt).
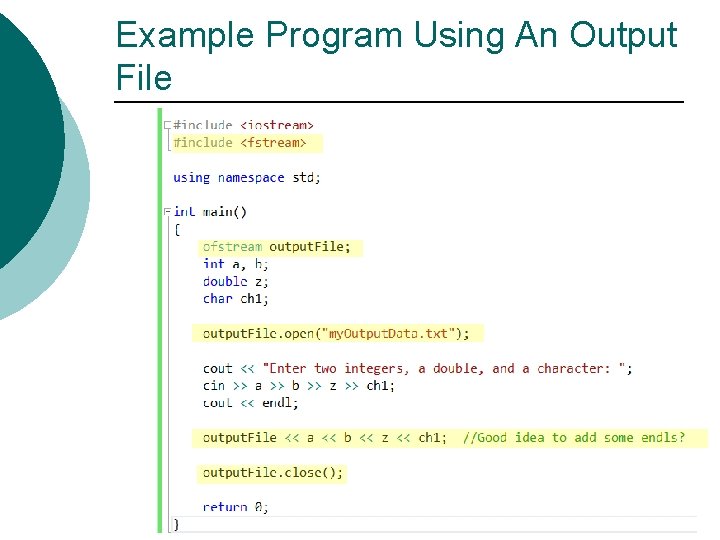
Example Program Using An Output File
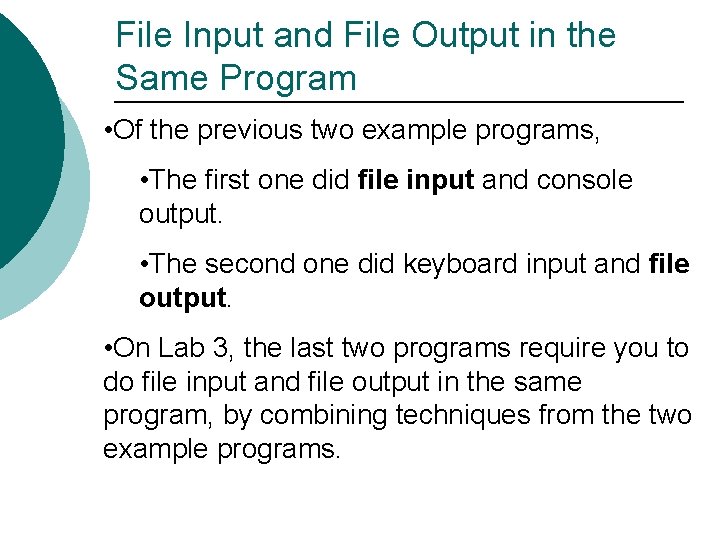
File Input and File Output in the Same Program • Of the previous two example programs, • The first one did file input and console output. • The second one did keyboard input and file output. • On Lab 3, the last two programs require you to do file input and file output in the same program, by combining techniques from the two example programs.
Astm d 3775
Inputoutput devices
Priya malik tanu malik
Two technicians are discussing back pressure
Bme egr
Bme egr
Egr 101
Egr 102
Unit 6 review questions
Dr aqsa malik
Dr ali afzal
Saadat malik
Dr atif malik
Dr aqsa malik
Saadat malik
Pola metropolis menyebar
Russlan boukerou
Meekaaeel angel
Tanu malik depaul
Abdul malik asmai contribution
Christoph malik
Kisah suraqah bin malik
Age niall horan
Dr waqar malik
Dr aqsa malik
"shaip" -"shaip kamberi"
Malik bin enes mezhebi
Zaid malik md
Avinash lakshman
Ana maria malik
Dr aqsa malik
Riena malik
Safarina g malik
Patrycja malik
Dr aqsa malik
Site:slidetodoc.com
Bob marley born place
Gapurosukolilo gresik
Malik jahan khan
Deja and malik
Dr aqsa malik
"malik nd"
Sanjiv malik
Jessup malik abkommen
Humara malik
"malik nd"
Malik jahan khan
Rimsha malik