Levels of Inheritance Multiple Inheritance Malik Jahan Khan
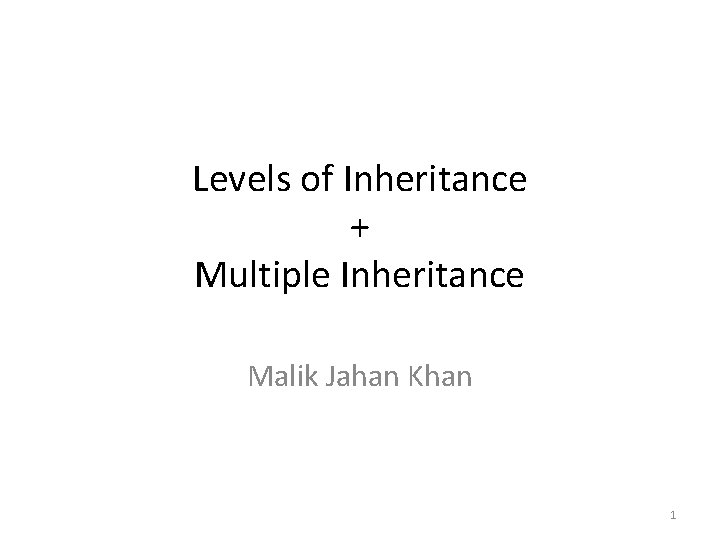
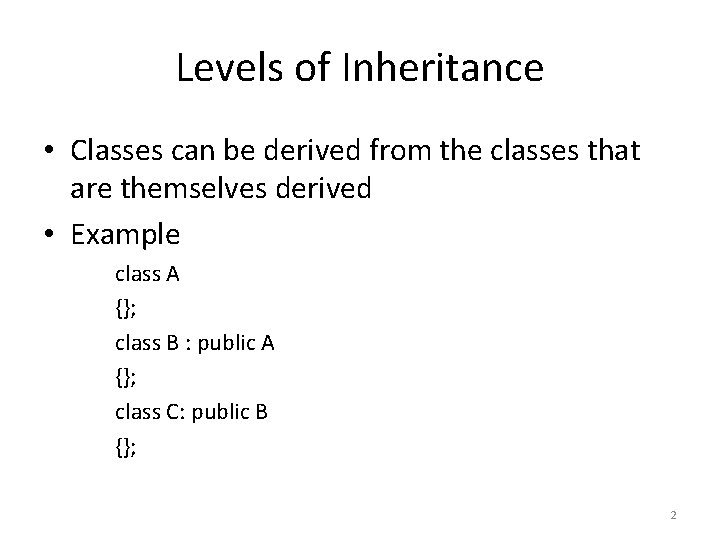
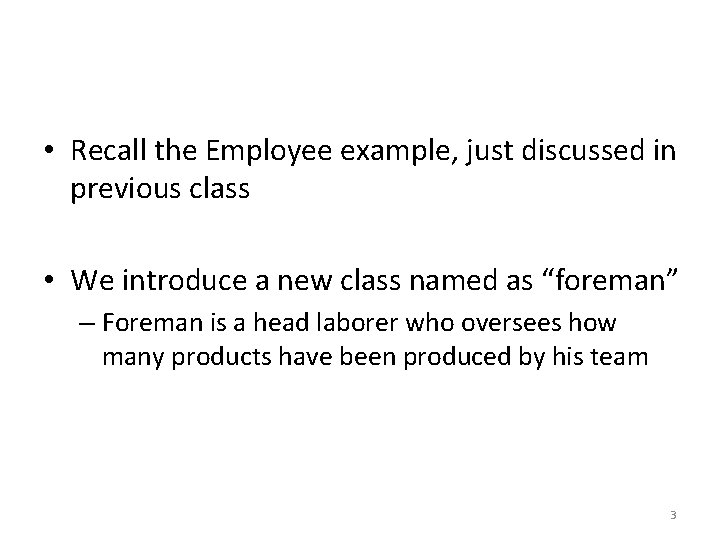
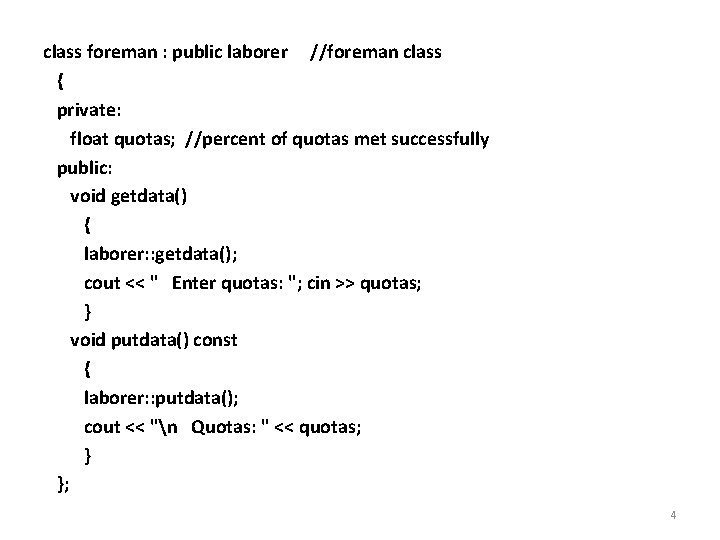
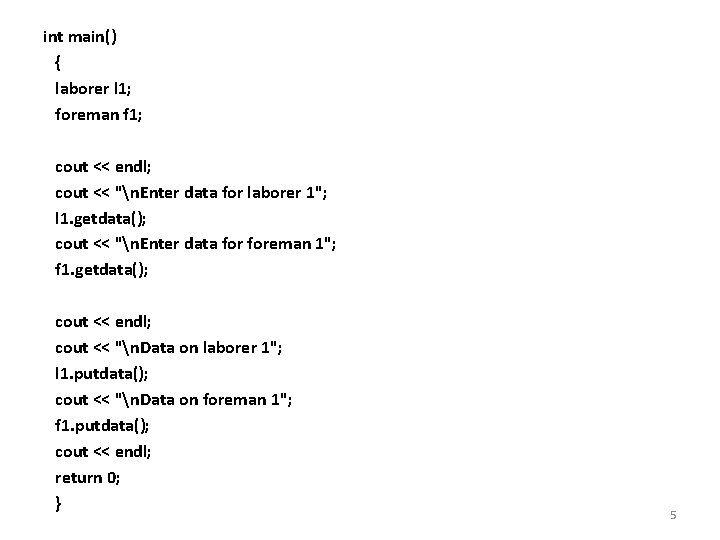
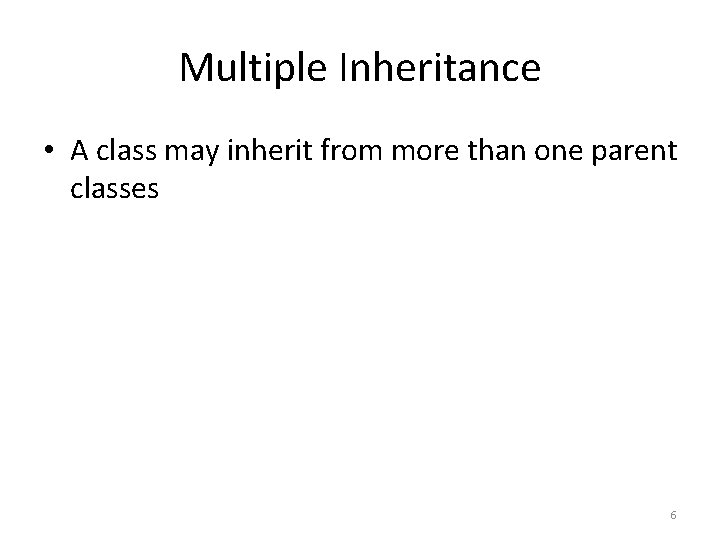
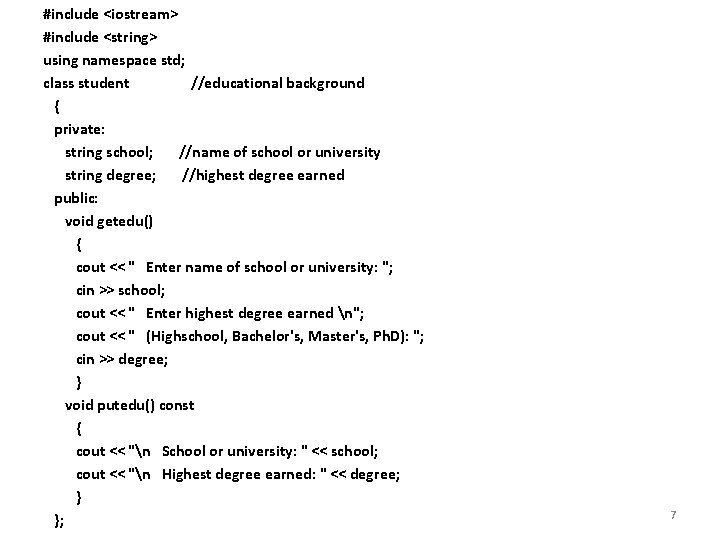
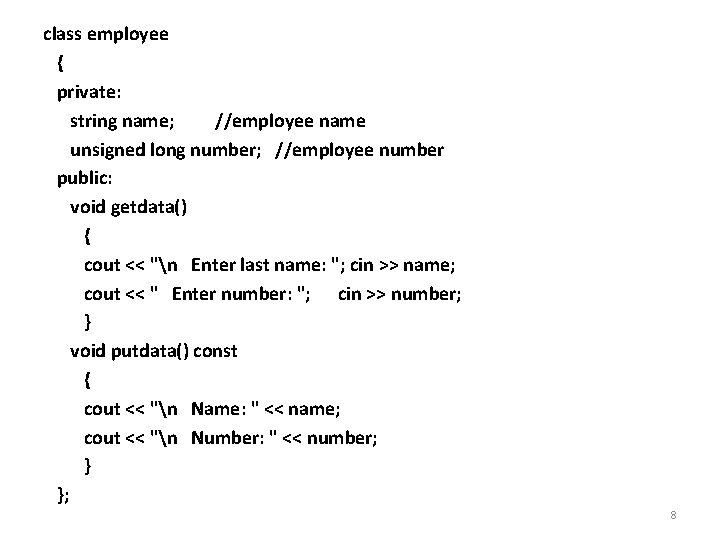
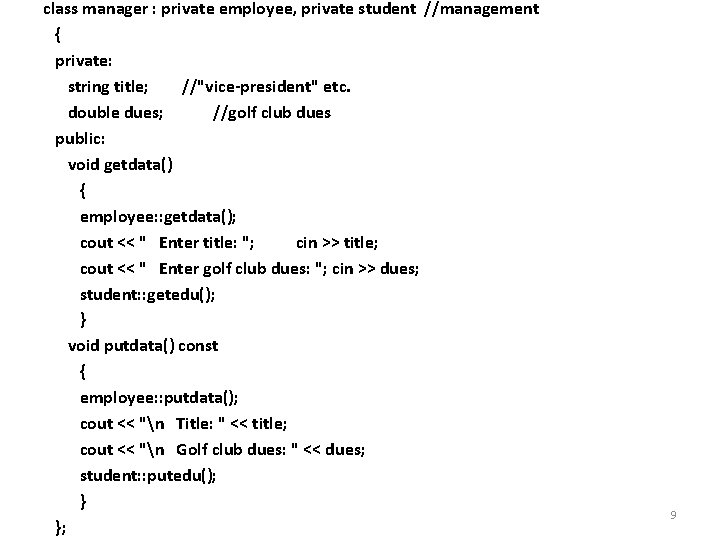
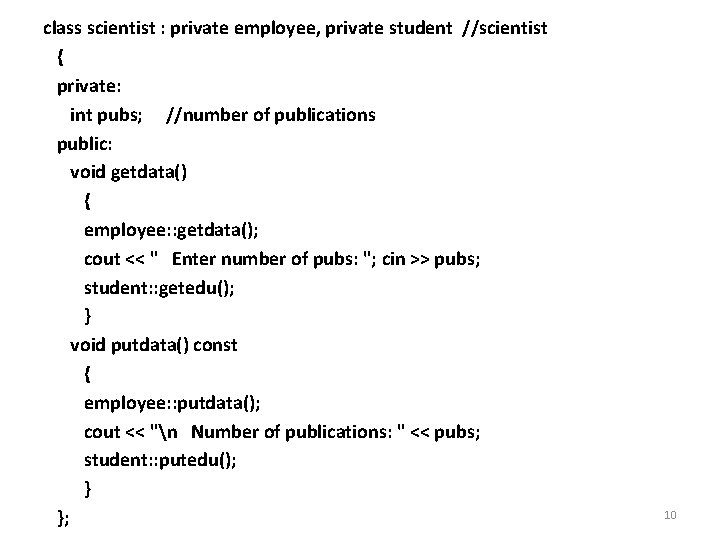
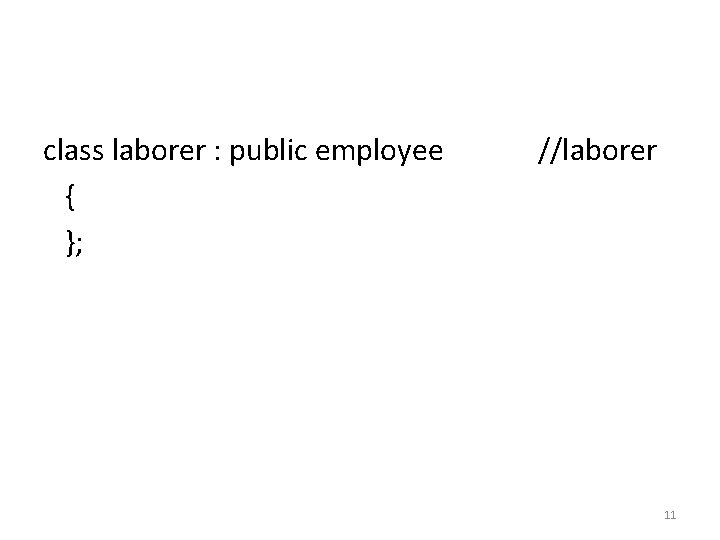
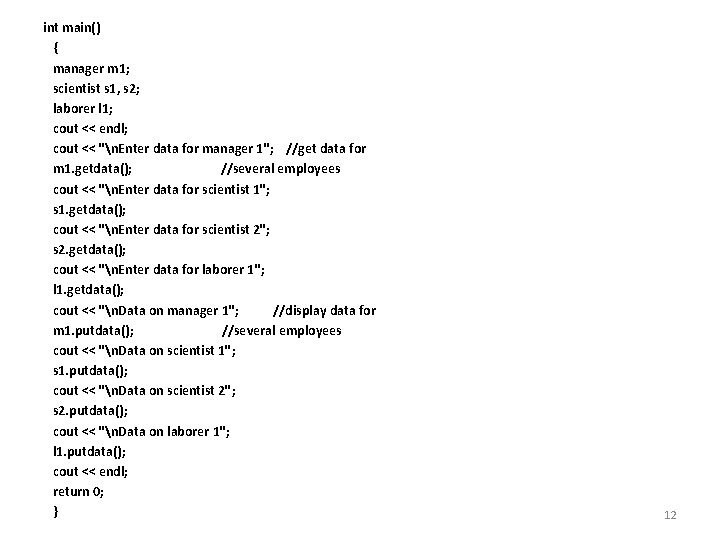
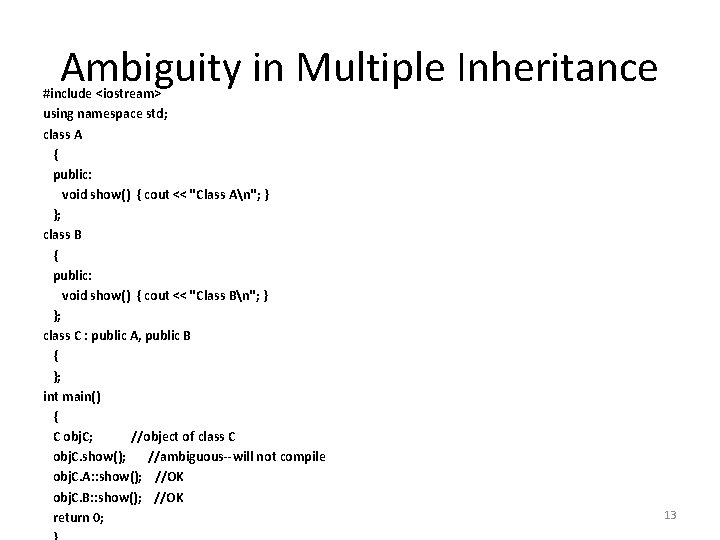
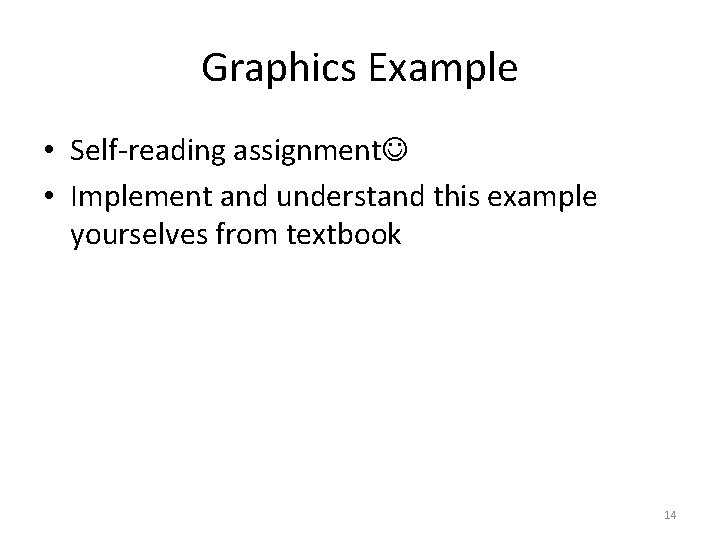
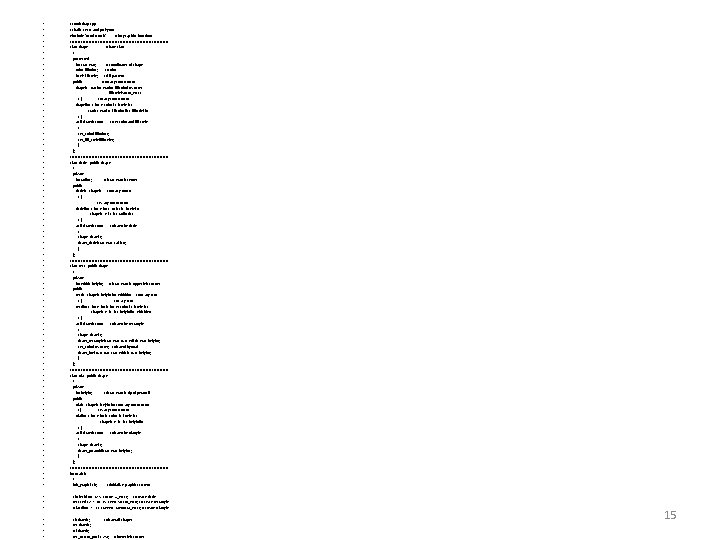
- Slides: 15
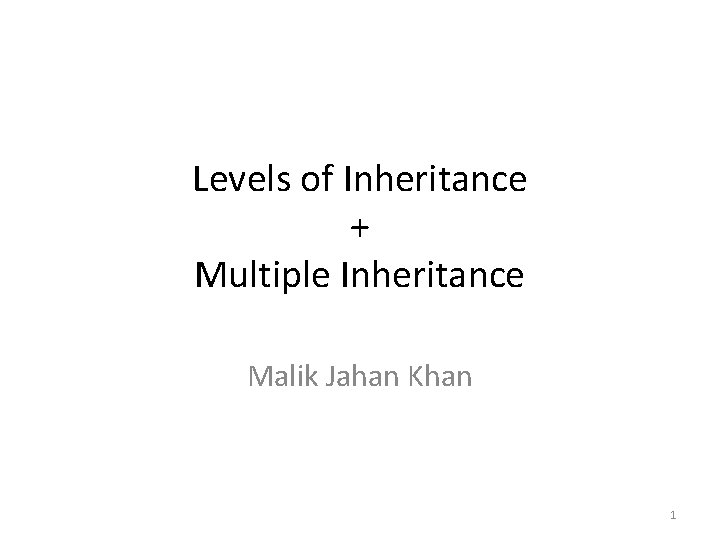
Levels of Inheritance + Multiple Inheritance Malik Jahan Khan 1
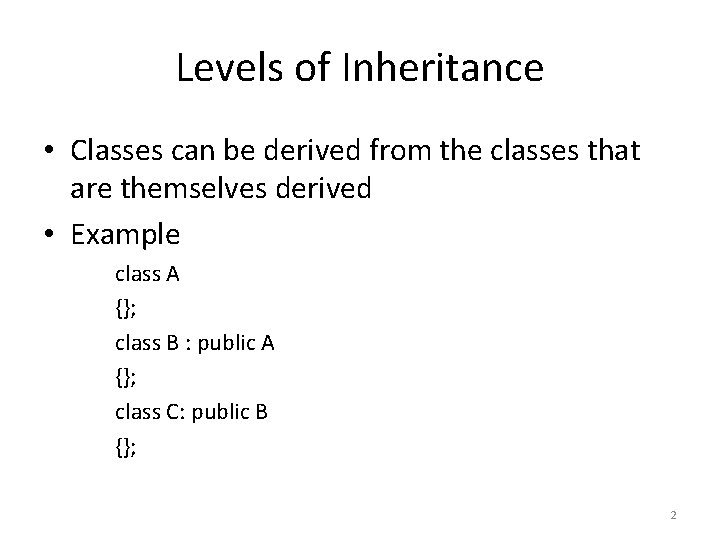
Levels of Inheritance • Classes can be derived from the classes that are themselves derived • Example class A {}; class B : public A {}; class C: public B {}; 2
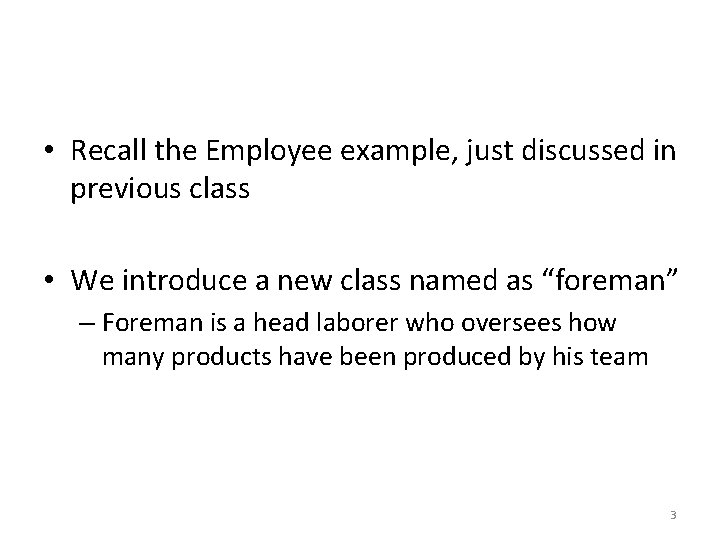
• Recall the Employee example, just discussed in previous class • We introduce a new class named as “foreman” – Foreman is a head laborer who oversees how many products have been produced by his team 3
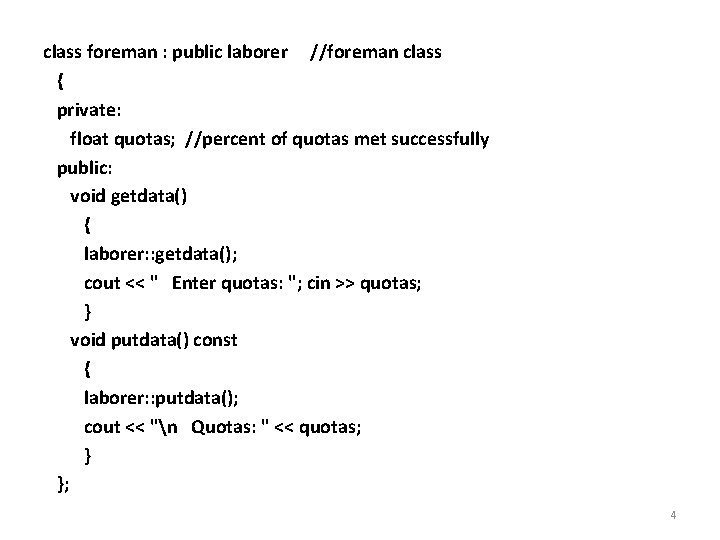
class foreman : public laborer //foreman class { private: float quotas; //percent of quotas met successfully public: void getdata() { laborer: : getdata(); cout << " Enter quotas: "; cin >> quotas; } void putdata() const { laborer: : putdata(); cout << "n Quotas: " << quotas; } }; 4
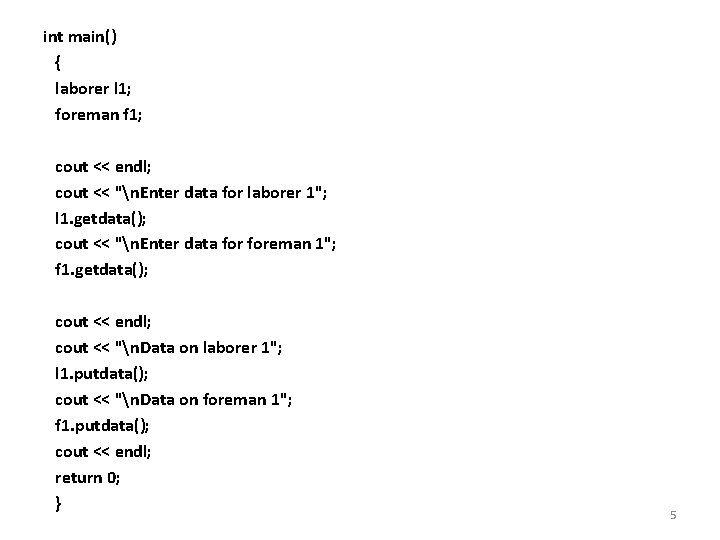
int main() { laborer l 1; foreman f 1; cout << endl; cout << "n. Enter data for laborer 1"; l 1. getdata(); cout << "n. Enter data foreman 1"; f 1. getdata(); cout << endl; cout << "n. Data on laborer 1"; l 1. putdata(); cout << "n. Data on foreman 1"; f 1. putdata(); cout << endl; return 0; } 5
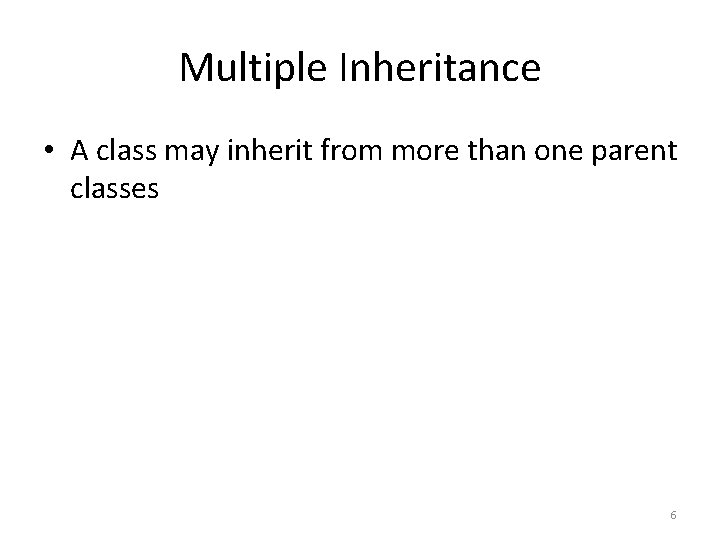
Multiple Inheritance • A class may inherit from more than one parent classes 6
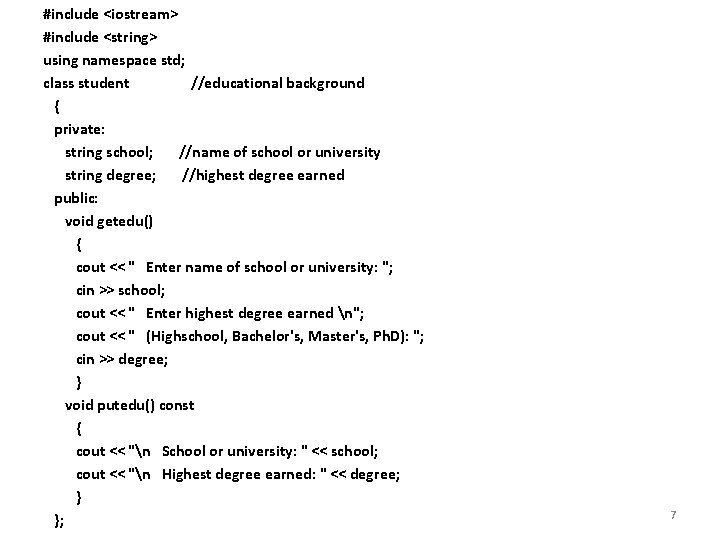
#include <iostream> #include <string> using namespace std; class student //educational background { private: string school; //name of school or university string degree; //highest degree earned public: void getedu() { cout << " Enter name of school or university: "; cin >> school; cout << " Enter highest degree earned n"; cout << " (Highschool, Bachelor's, Master's, Ph. D): "; cin >> degree; } void putedu() const { cout << "n School or university: " << school; cout << "n Highest degree earned: " << degree; } }; 7
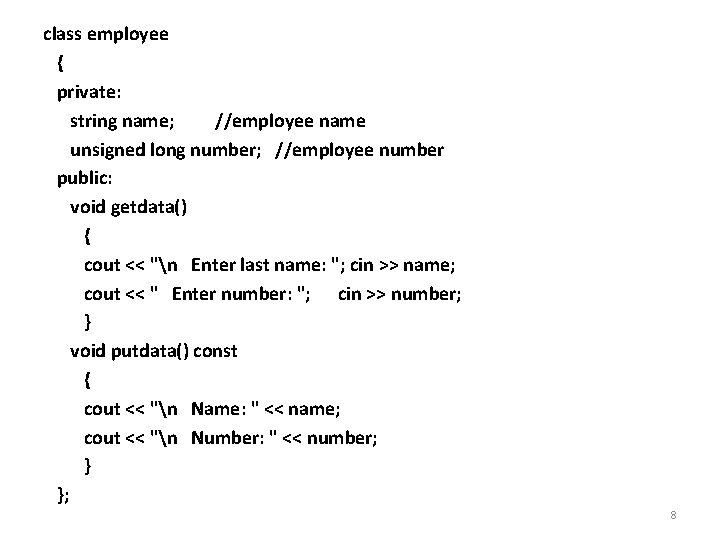
class employee { private: string name; //employee name unsigned long number; //employee number public: void getdata() { cout << "n Enter last name: "; cin >> name; cout << " Enter number: "; cin >> number; } void putdata() const { cout << "n Name: " << name; cout << "n Number: " << number; } }; 8
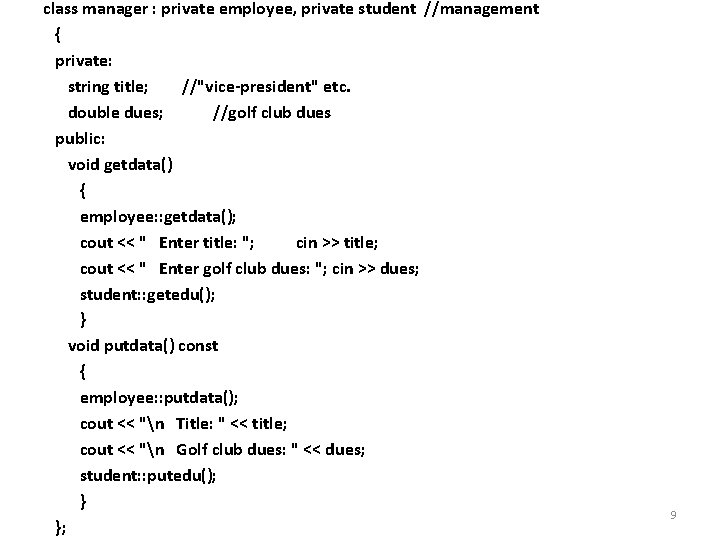
class manager : private employee, private student //management { private: string title; //"vice-president" etc. double dues; //golf club dues public: void getdata() { employee: : getdata(); cout << " Enter title: "; cin >> title; cout << " Enter golf club dues: "; cin >> dues; student: : getedu(); } void putdata() const { employee: : putdata(); cout << "n Title: " << title; cout << "n Golf club dues: " << dues; student: : putedu(); } }; 9
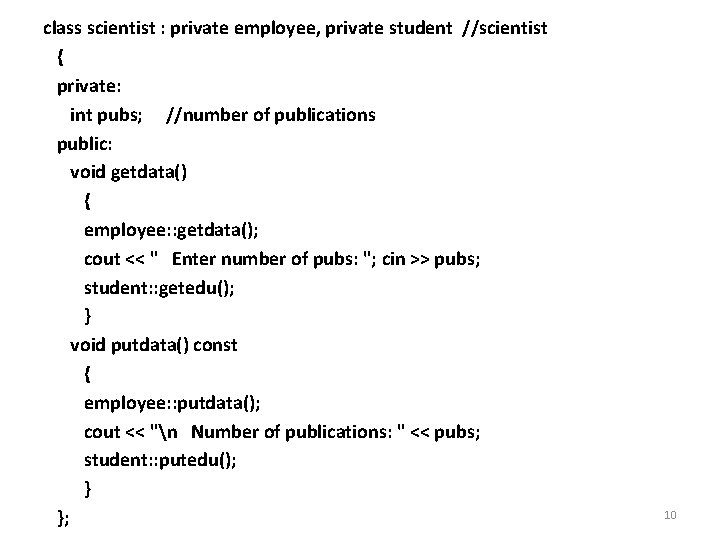
class scientist : private employee, private student //scientist { private: int pubs; //number of publications public: void getdata() { employee: : getdata(); cout << " Enter number of pubs: "; cin >> pubs; student: : getedu(); } void putdata() const { employee: : putdata(); cout << "n Number of publications: " << pubs; student: : putedu(); } }; 10
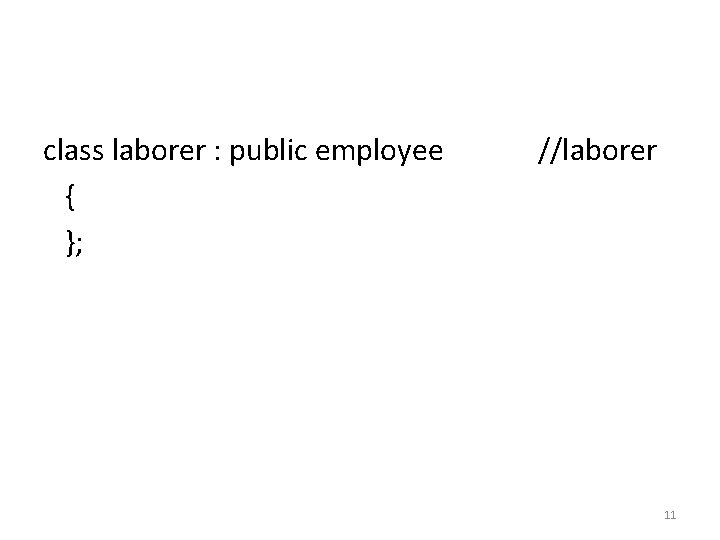
class laborer : public employee { }; //laborer 11
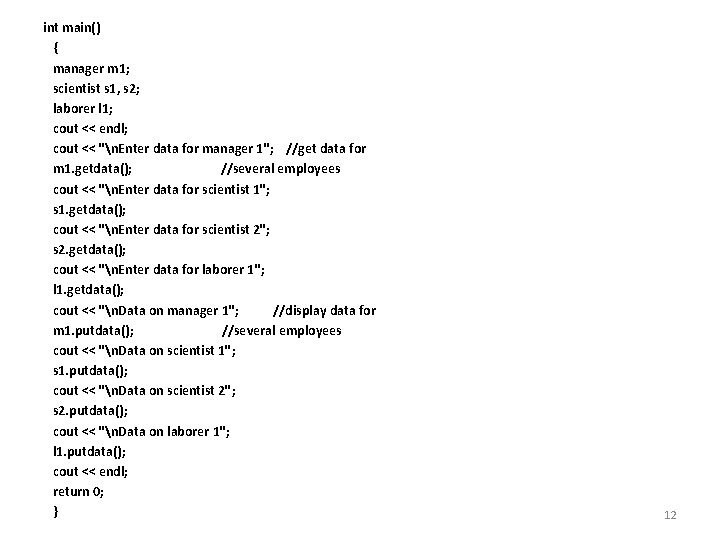
int main() { manager m 1; scientist s 1, s 2; laborer l 1; cout << endl; cout << "n. Enter data for manager 1"; //get data for m 1. getdata(); //several employees cout << "n. Enter data for scientist 1"; s 1. getdata(); cout << "n. Enter data for scientist 2"; s 2. getdata(); cout << "n. Enter data for laborer 1"; l 1. getdata(); cout << "n. Data on manager 1"; //display data for m 1. putdata(); //several employees cout << "n. Data on scientist 1"; s 1. putdata(); cout << "n. Data on scientist 2"; s 2. putdata(); cout << "n. Data on laborer 1"; l 1. putdata(); cout << endl; return 0; } 12
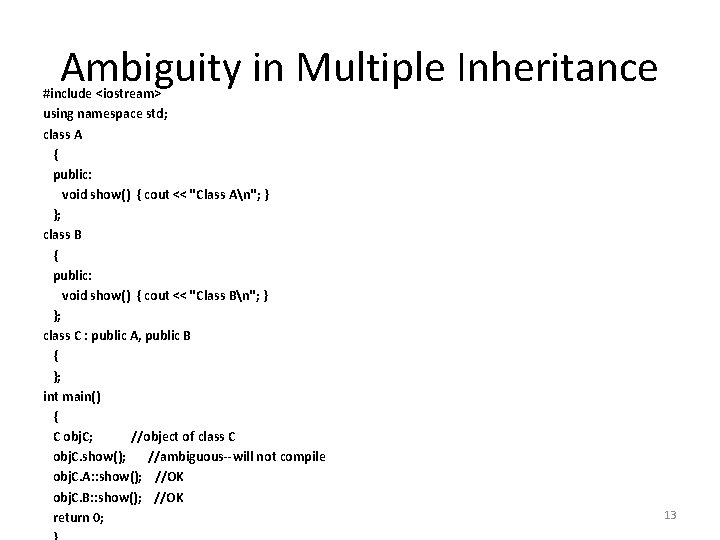
Ambiguity in Multiple Inheritance #include <iostream> using namespace std; class A { public: void show() { cout << "Class An"; } }; class B { public: void show() { cout << "Class Bn"; } }; class C : public A, public B { }; int main() { C obj. C; //object of class C obj. C. show(); //ambiguous--will not compile obj. C. A: : show(); //OK obj. C. B: : show(); //OK return 0; 13
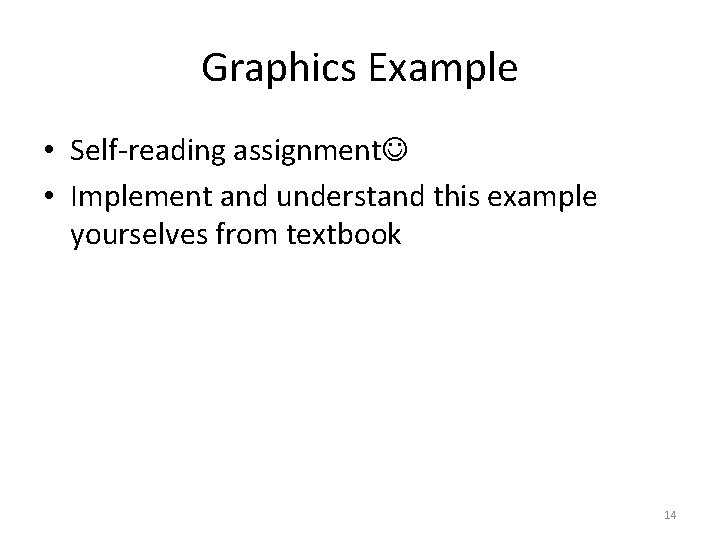
Graphics Example • Self-reading assignment • Implement and understand this example yourselves from textbook 14
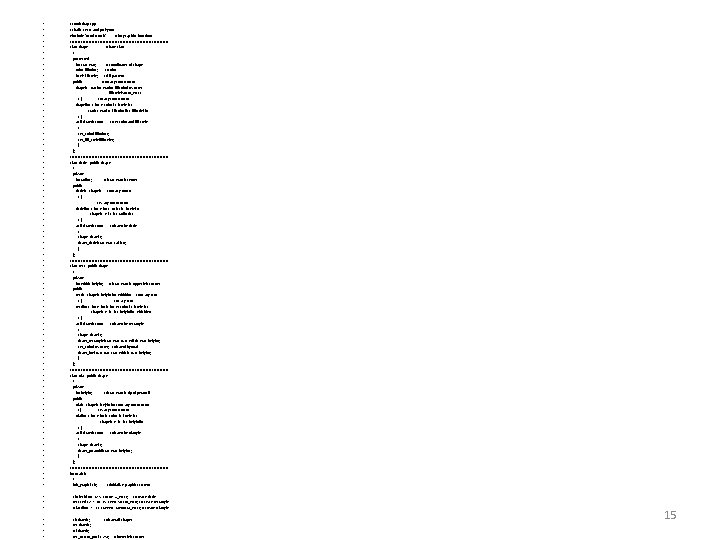
• • • • • • • • • • • • • • • • • • • • • // multshap. cpp // balls, rects, and polygons #include "msoftcon. h" //for graphics functions //////////////////////////////// class shape //base class { protected: int x. Co, y. Co; //coordinates of shape color fillcolor; //color fstyle fillstyle; //fill pattern public: //no-arg constructor shape() : x. Co(0), y. Co(0), fillcolor(c. WHITE), fillstyle(SOLID_FILL) { } //4 -arg constructor shape(int x, int y, color fc, fstyle fs) : x. Co(x), y. Co(y), fillcolor(fc), fillstyle(fs) { } void draw() const //set color and fill style { set_color(fillcolor); set_fill_style(fillstyle); } }; //////////////////////////////// class circle : public shape { private: int radius; //(x. Co, y. Co) is center public: circle() : shape() //no-arg constr { } //5 -arg constructor circle(int x, int y, int r, color fc, fstyle fs) : shape(x, y, fc, fs), radius(r) { } void draw() const //draw the circle { shape: : draw(); draw_circle(x. Co, y. Co, radius); } }; //////////////////////////////// class rect : public shape { private: int width, height; //(x. Co, y. Co) is upper left corner public: rect() : shape(), height(0), width(0) //no-arg ctor { } //6 -arg ctor rect(int x, int y, int h, int w, color fc, fstyle fs) : shape(x, y, fc, fs), height(h), width(w) { } void draw() const //draw the rectangle { shape: : draw(); draw_rectangle(x. Co, y. Co, x. Co+width, y. Co+height); set_color(c. WHITE); //draw diagonal draw_line(x. Co, y. Co, x. Co+width, y. Co+height); } }; //////////////////////////////// class tria : public shape { private: int height; //(x. Co, y. Co) is tip of pyramid public: tria() : shape(), height(0) //no-arg constructor {} //5 -arg constructor tria(int x, int y, int h, color fc, fstyle fs) : shape(x, y, fc, fs), height(h) { } void draw() const //draw the triangle { shape: : draw(); draw_pyramid(x. Co, y. Co, height); } }; //////////////////////////////// int main() { init_graphics(); //initialize graphics system • • • circle cir(40, 12, 5, c. BLUE, X_FILL); //create circle rect rec(12, 7, 10, 15, c. RED, SOLID_FILL); //create rectangle tria tri(60, 7, 11, c. GREEN, MEDIUM_FILL); //create triangle • • cir. draw(); //draw all shapes rec. draw(); tri. draw(); set_cursor_pos(1, 25); //lower left corner 15
Malik jahan khan
Malik jahan khan
Priya malik tanu malik
Dr. parul jahan
Treatment for stridor
Shah jahan accomplishments
Taj mahal simile
Cht water
Least common multiple khan academy
Andrea morichetta
Diamond problem oop
What is a level 3 question example
Multiple independent levels of security
Baseline
Multiple instruction multiple data
Dr aqsa malik