Recursion Malik Jahan Khan Recursion A function may
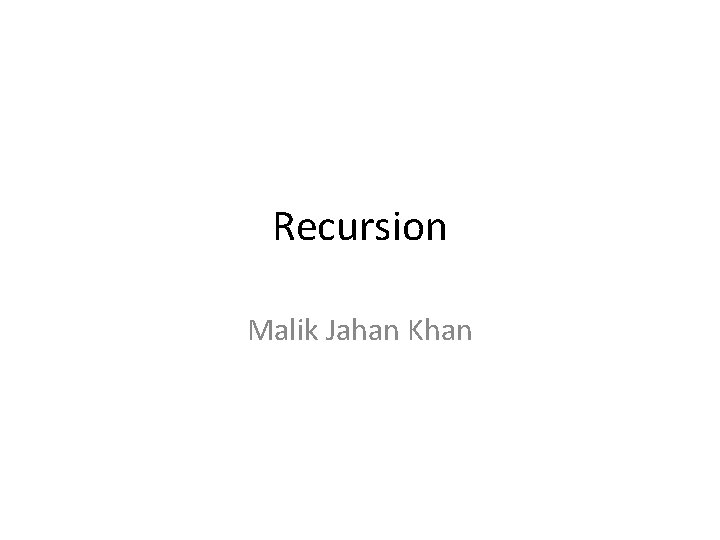
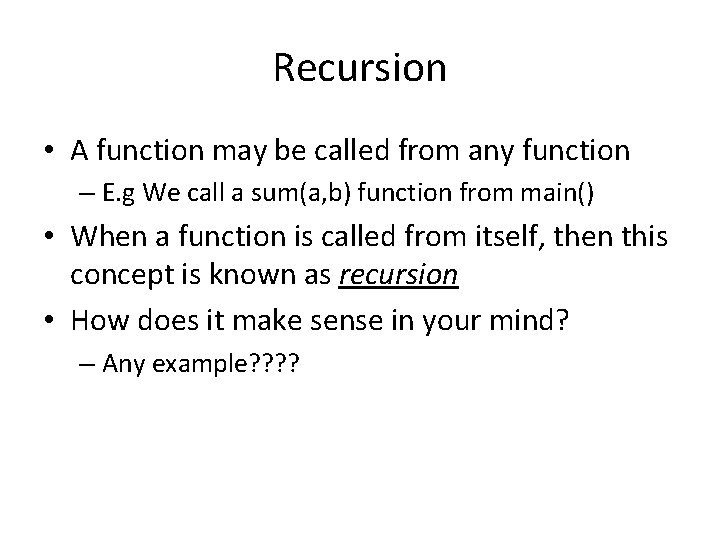
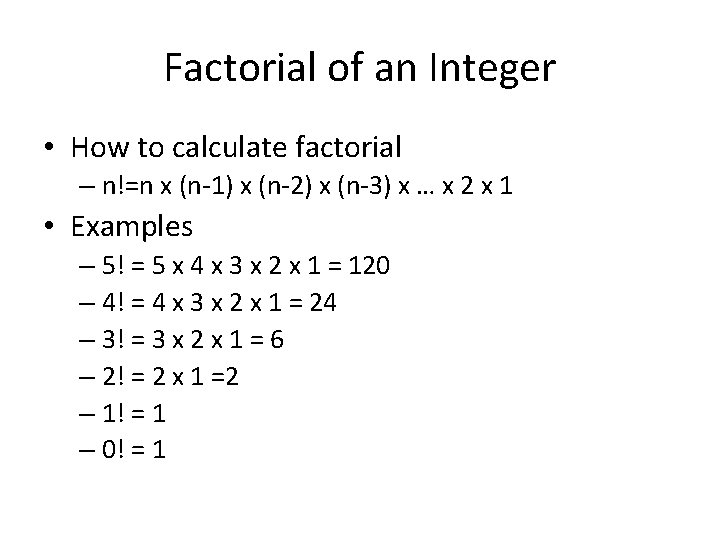
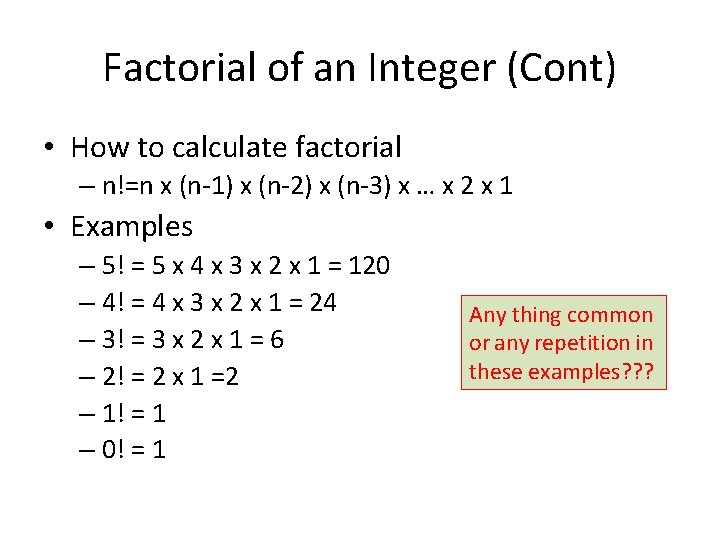
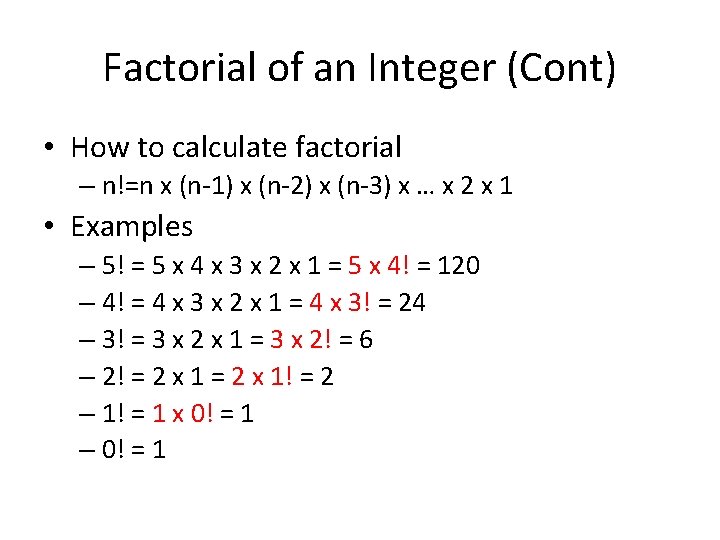
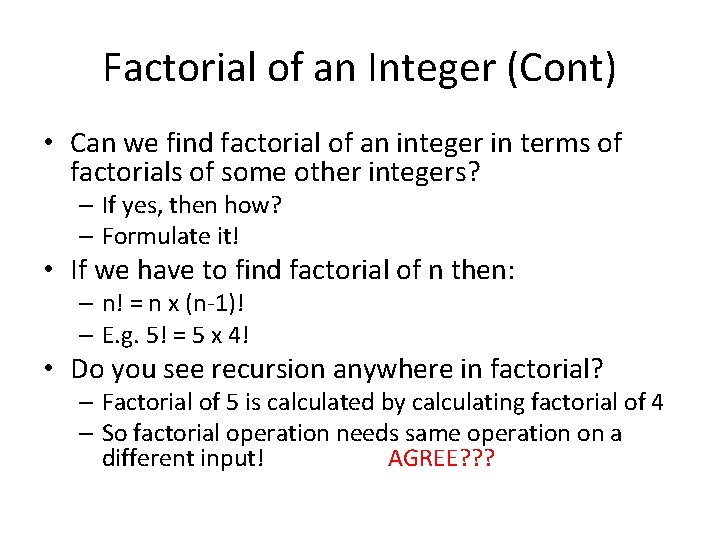
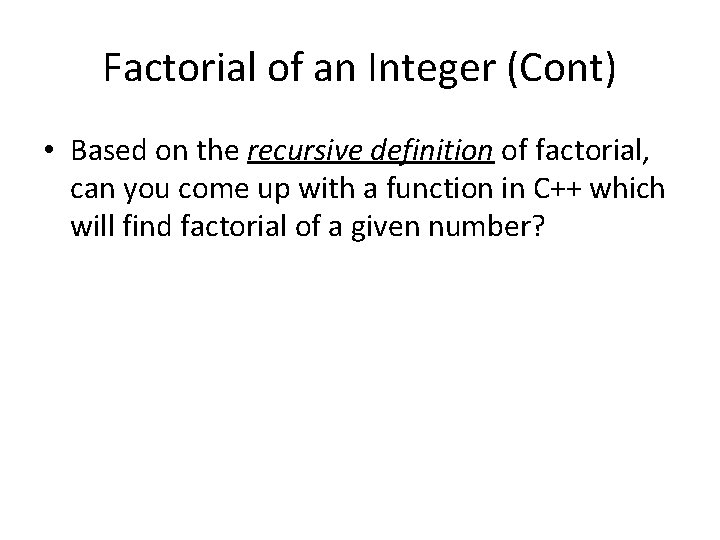
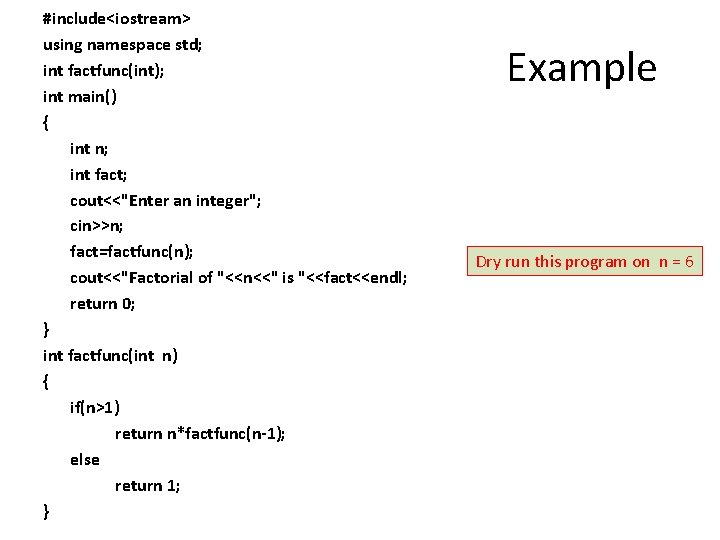
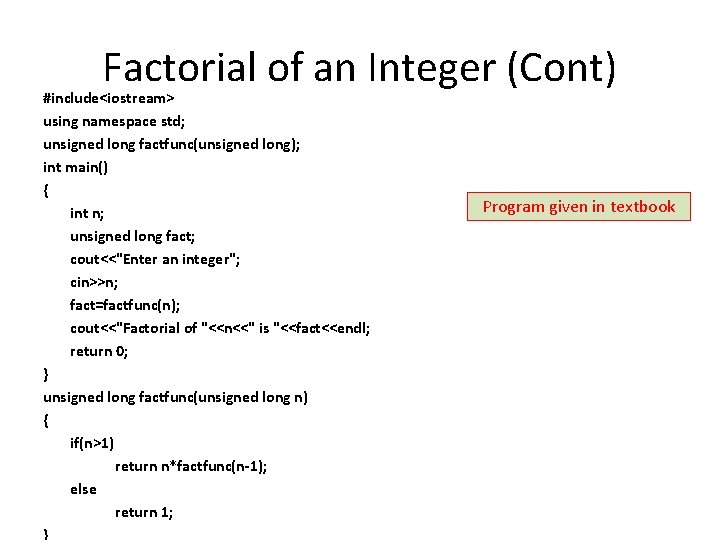
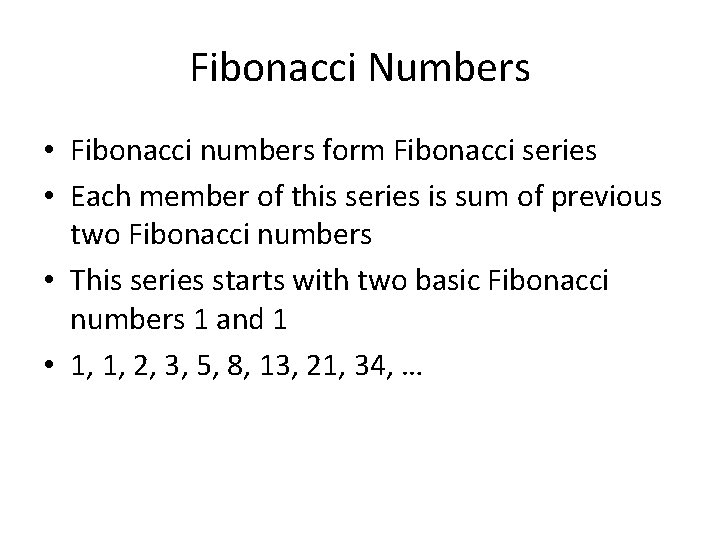
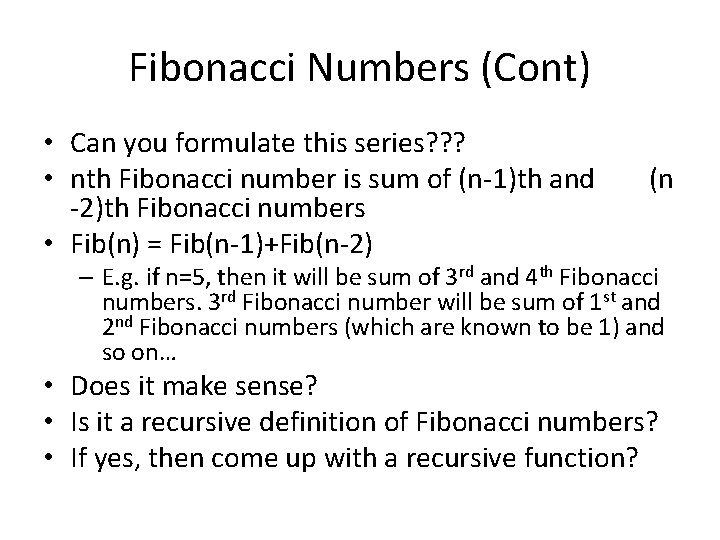
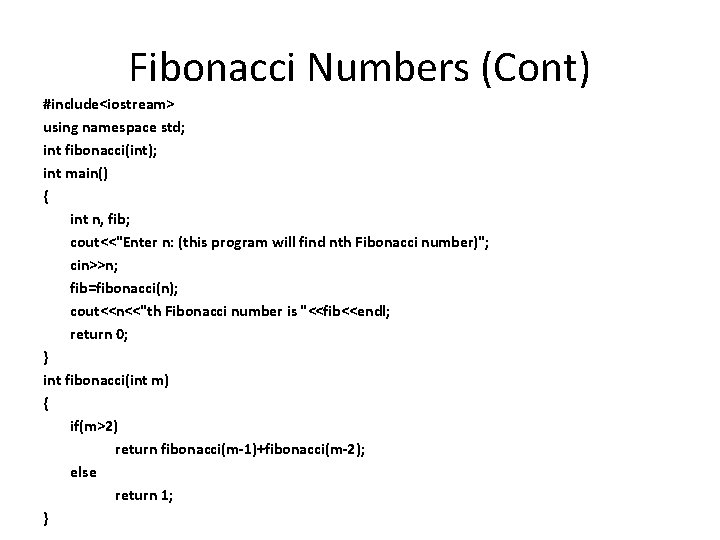
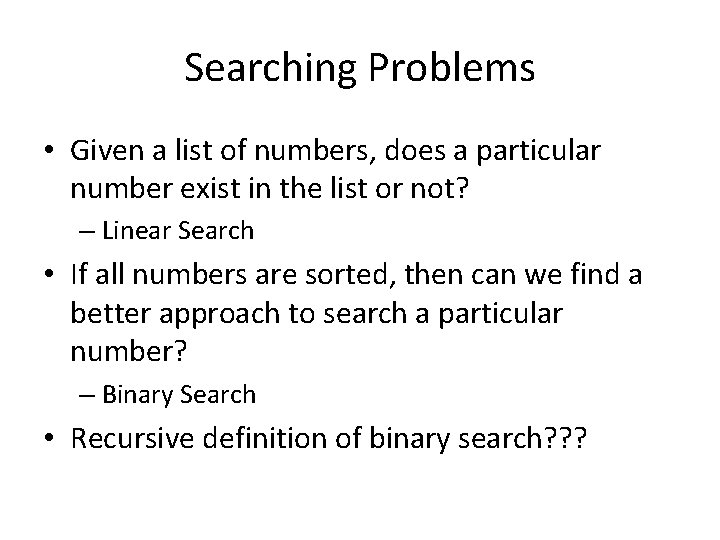
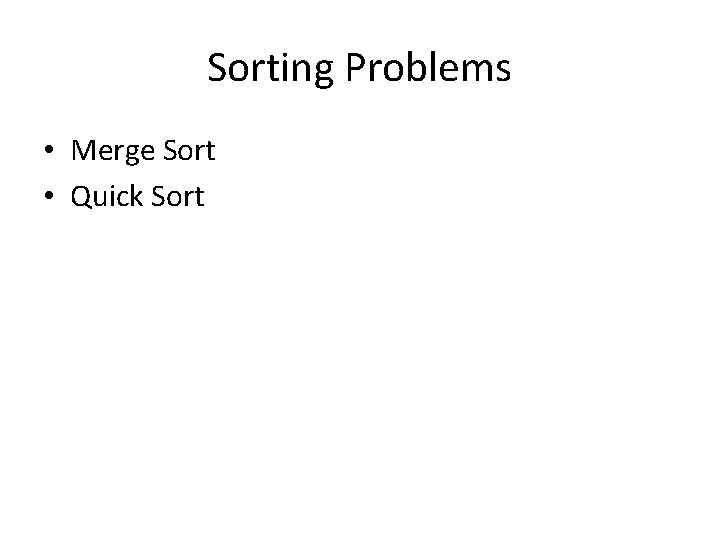
- Slides: 14
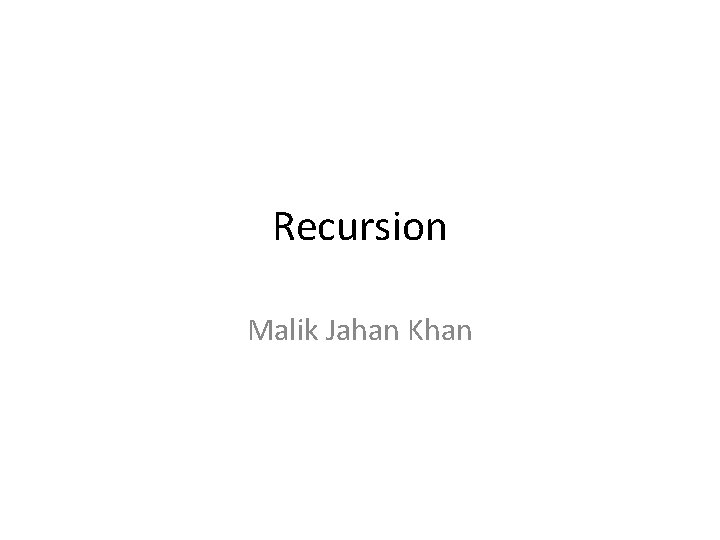
Recursion Malik Jahan Khan
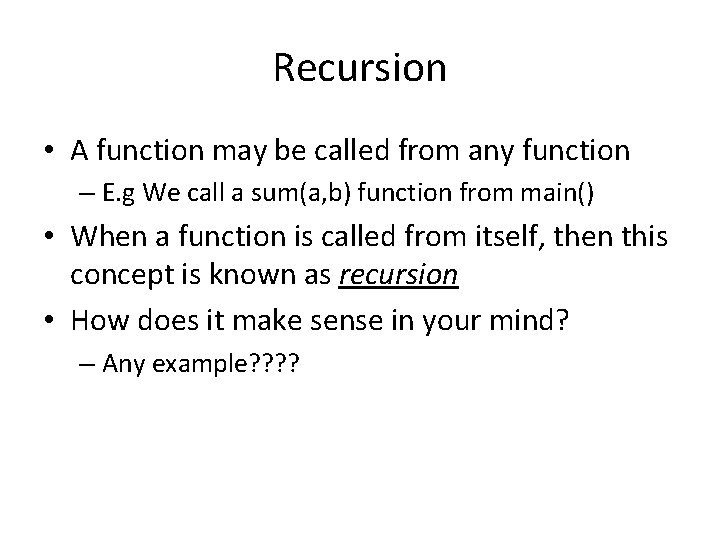
Recursion • A function may be called from any function – E. g We call a sum(a, b) function from main() • When a function is called from itself, then this concept is known as recursion • How does it make sense in your mind? – Any example? ?
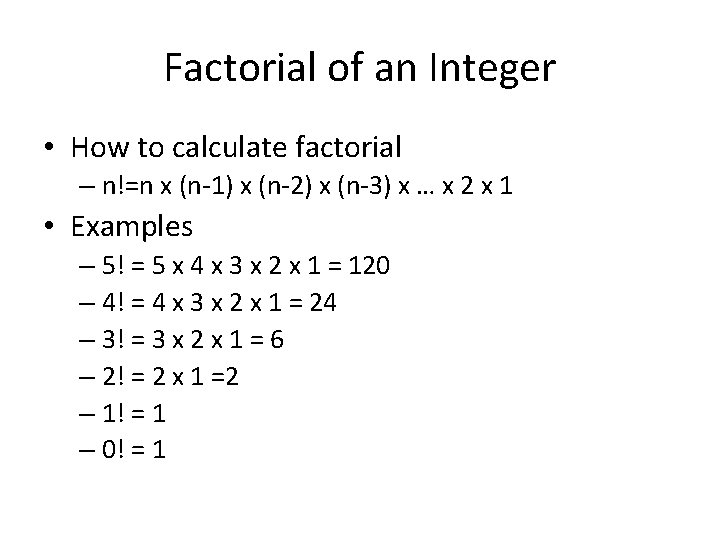
Factorial of an Integer • How to calculate factorial – n!=n x (n-1) x (n-2) x (n-3) x … x 2 x 1 • Examples – 5! = 5 x 4 x 3 x 2 x 1 = 120 – 4! = 4 x 3 x 2 x 1 = 24 – 3! = 3 x 2 x 1 = 6 – 2! = 2 x 1 =2 – 1! = 1 – 0! = 1
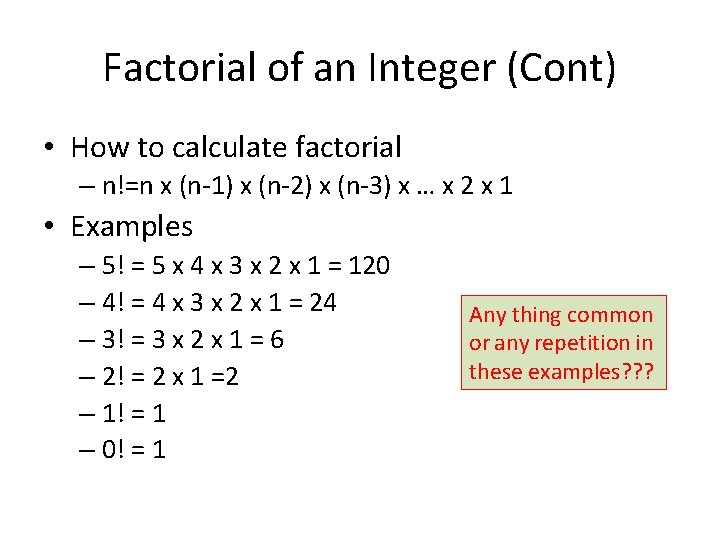
Factorial of an Integer (Cont) • How to calculate factorial – n!=n x (n-1) x (n-2) x (n-3) x … x 2 x 1 • Examples – 5! = 5 x 4 x 3 x 2 x 1 = 120 – 4! = 4 x 3 x 2 x 1 = 24 – 3! = 3 x 2 x 1 = 6 – 2! = 2 x 1 =2 – 1! = 1 – 0! = 1 Any thing common or any repetition in these examples? ? ?
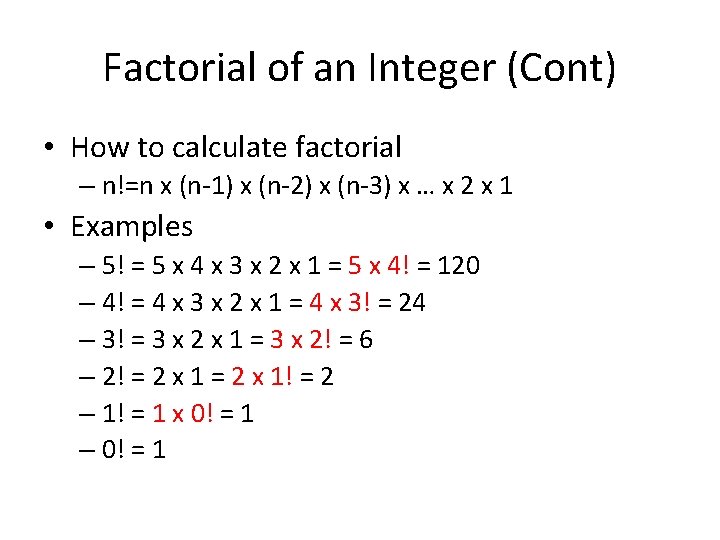
Factorial of an Integer (Cont) • How to calculate factorial – n!=n x (n-1) x (n-2) x (n-3) x … x 2 x 1 • Examples – 5! = 5 x 4 x 3 x 2 x 1 = 5 x 4! = 120 – 4! = 4 x 3 x 2 x 1 = 4 x 3! = 24 – 3! = 3 x 2 x 1 = 3 x 2! = 6 – 2! = 2 x 1! = 2 – 1! = 1 x 0! = 1 – 0! = 1
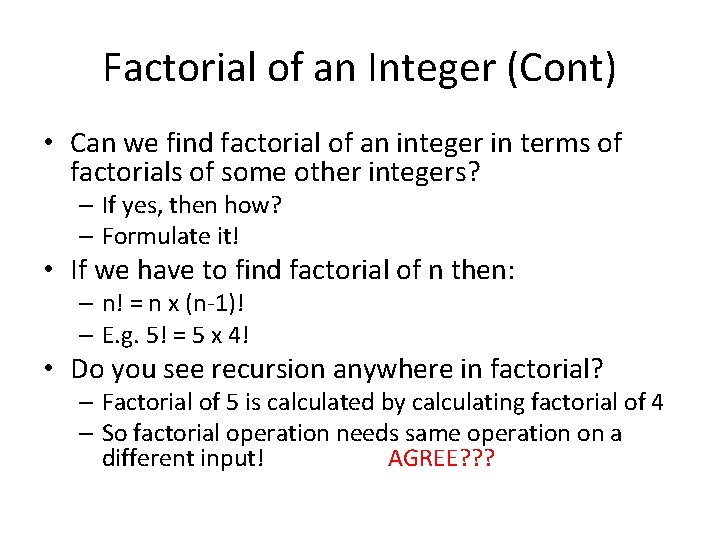
Factorial of an Integer (Cont) • Can we find factorial of an integer in terms of factorials of some other integers? – If yes, then how? – Formulate it! • If we have to find factorial of n then: – n! = n x (n-1)! – E. g. 5! = 5 x 4! • Do you see recursion anywhere in factorial? – Factorial of 5 is calculated by calculating factorial of 4 – So factorial operation needs same operation on a different input! AGREE? ? ?
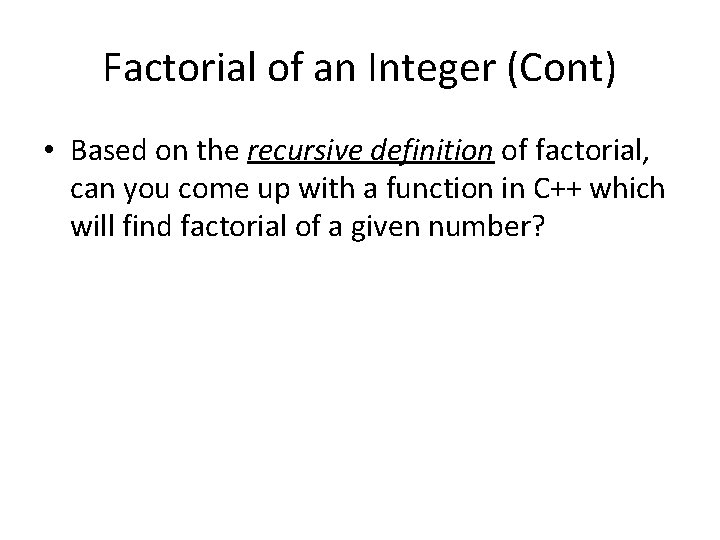
Factorial of an Integer (Cont) • Based on the recursive definition of factorial, can you come up with a function in C++ which will find factorial of a given number?
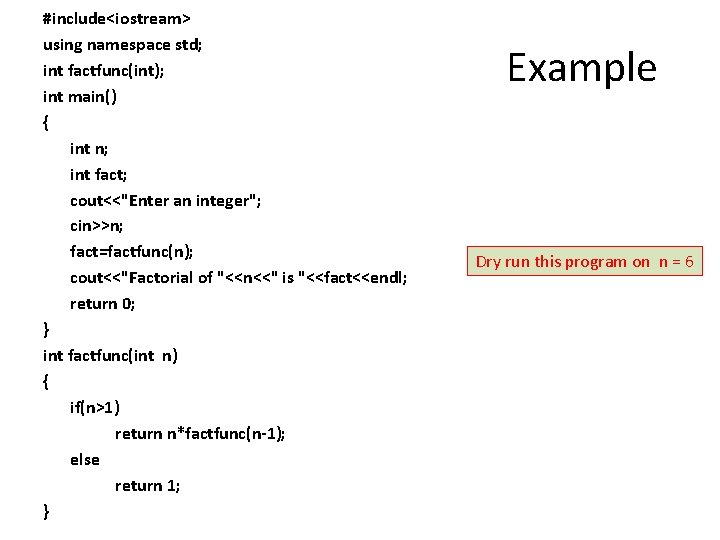
#include<iostream> using namespace std; int factfunc(int); int main() { int n; int fact; cout<<"Enter an integer"; cin>>n; fact=factfunc(n); cout<<"Factorial of "<<n<<" is "<<fact<<endl; return 0; } int factfunc(int n) { if(n>1) return n*factfunc(n-1); else return 1; } Example Dry run this program on n = 6
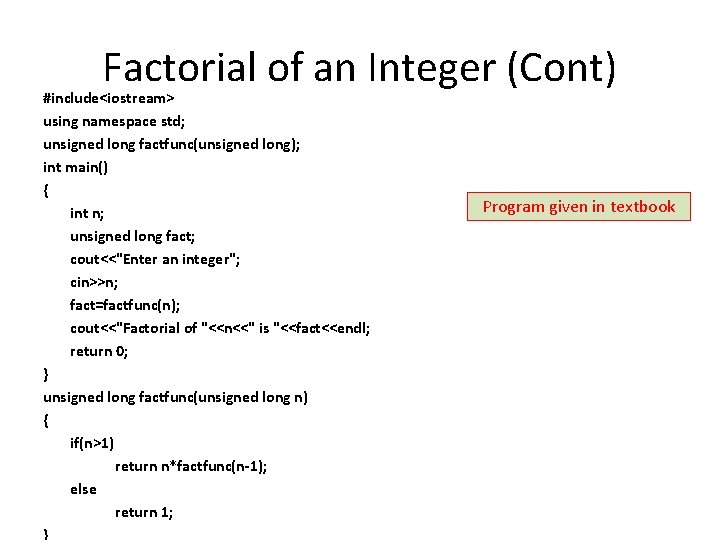
Factorial of an Integer (Cont) #include<iostream> using namespace std; unsigned long factfunc(unsigned long); int main() { int n; unsigned long fact; cout<<"Enter an integer"; cin>>n; fact=factfunc(n); cout<<"Factorial of "<<n<<" is "<<fact<<endl; return 0; } unsigned long factfunc(unsigned long n) { if(n>1) return n*factfunc(n-1); else return 1; Program given in textbook
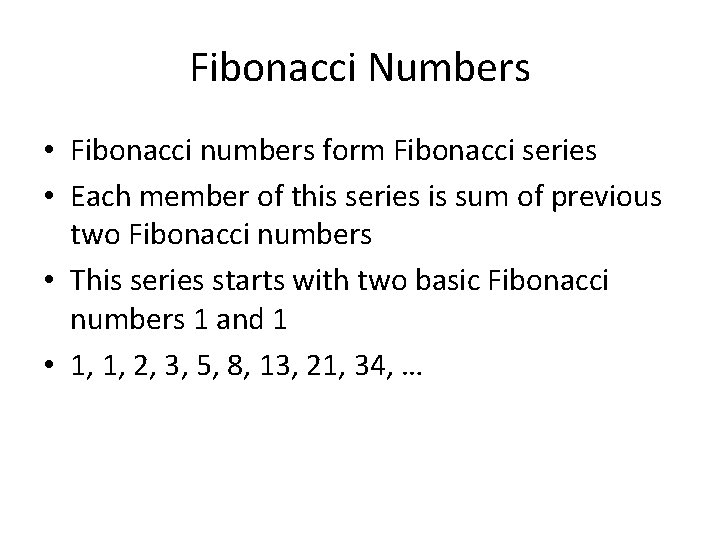
Fibonacci Numbers • Fibonacci numbers form Fibonacci series • Each member of this series is sum of previous two Fibonacci numbers • This series starts with two basic Fibonacci numbers 1 and 1 • 1, 1, 2, 3, 5, 8, 13, 21, 34, …
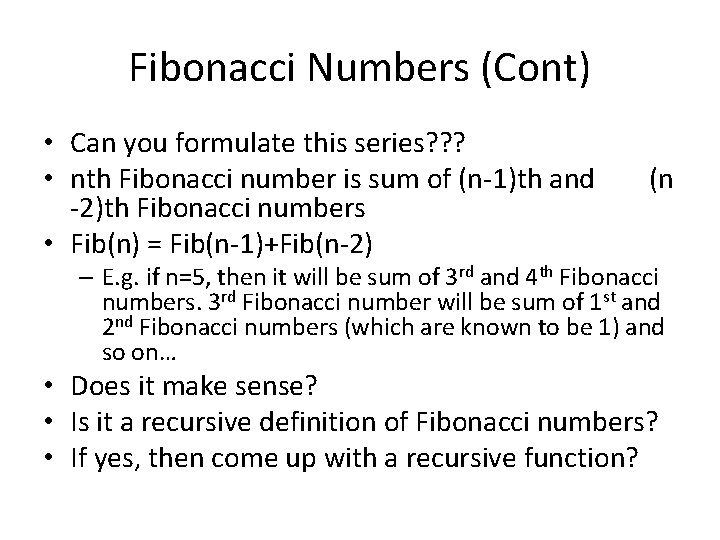
Fibonacci Numbers (Cont) • Can you formulate this series? ? ? • nth Fibonacci number is sum of (n-1)th and -2)th Fibonacci numbers • Fib(n) = Fib(n-1)+Fib(n-2) (n – E. g. if n=5, then it will be sum of 3 rd and 4 th Fibonacci numbers. 3 rd Fibonacci number will be sum of 1 st and 2 nd Fibonacci numbers (which are known to be 1) and so on… • Does it make sense? • Is it a recursive definition of Fibonacci numbers? • If yes, then come up with a recursive function?
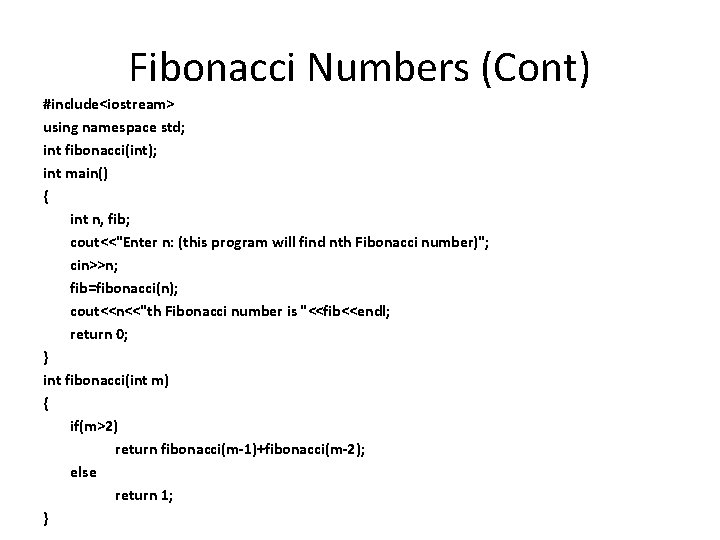
Fibonacci Numbers (Cont) #include<iostream> using namespace std; int fibonacci(int); int main() { int n, fib; cout<<"Enter n: (this program will find nth Fibonacci number)"; cin>>n; fib=fibonacci(n); cout<<n<<"th Fibonacci number is "<<fib<<endl; return 0; } int fibonacci(int m) { if(m>2) return fibonacci(m-1)+fibonacci(m-2); else return 1; }
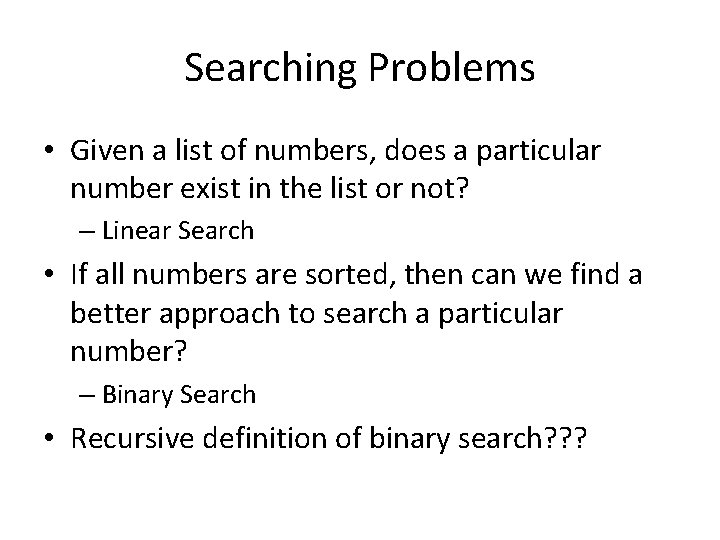
Searching Problems • Given a list of numbers, does a particular number exist in the list or not? – Linear Search • If all numbers are sorted, then can we find a better approach to search a particular number? – Binary Search • Recursive definition of binary search? ? ?
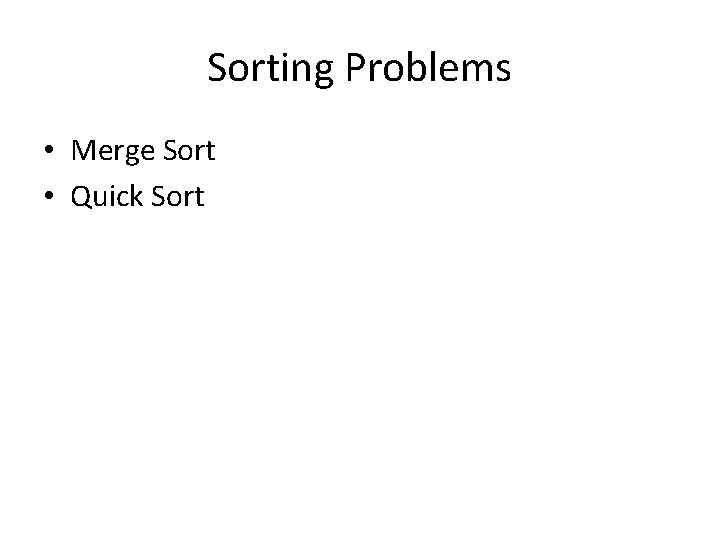
Sorting Problems • Merge Sort • Quick Sort