Polymorphism Malik Jahan Khan Polymorphism One of the
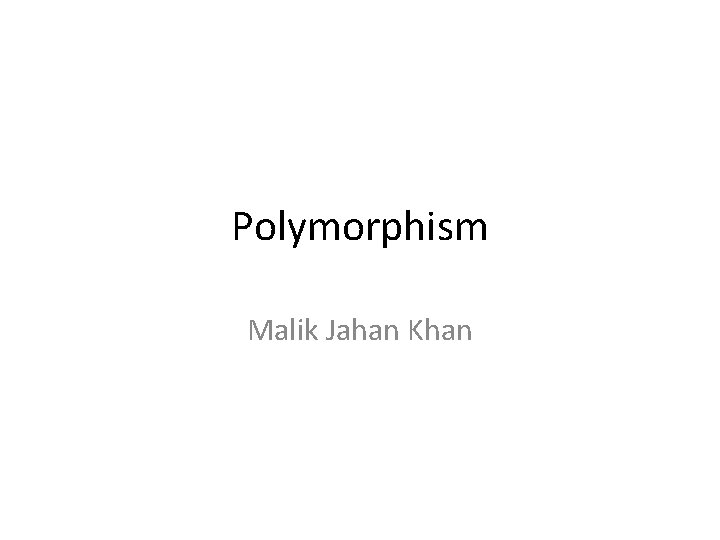
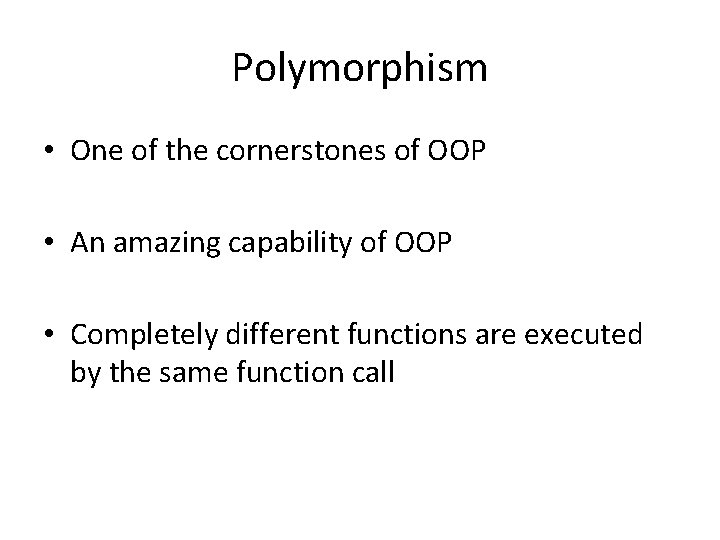
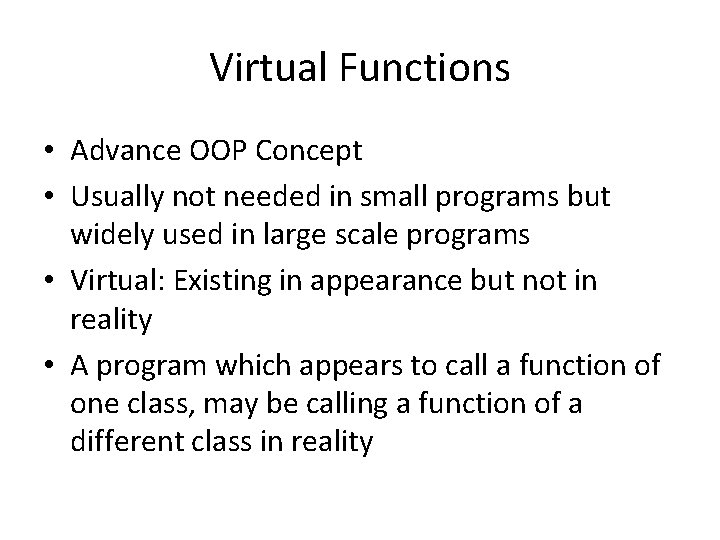
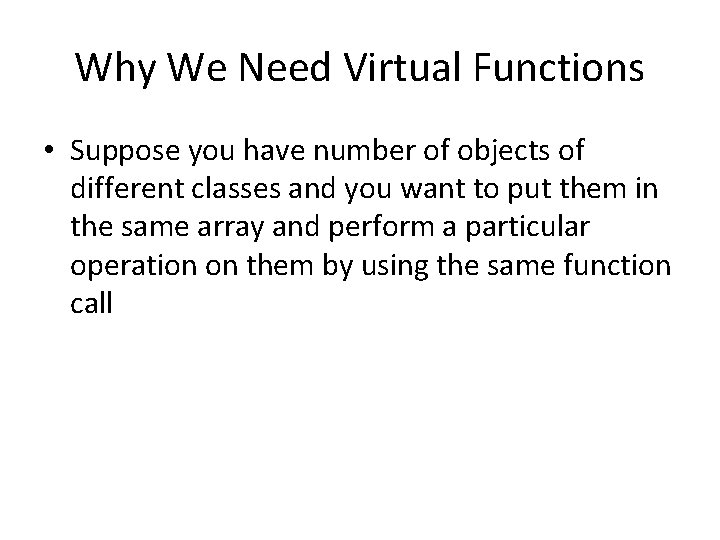
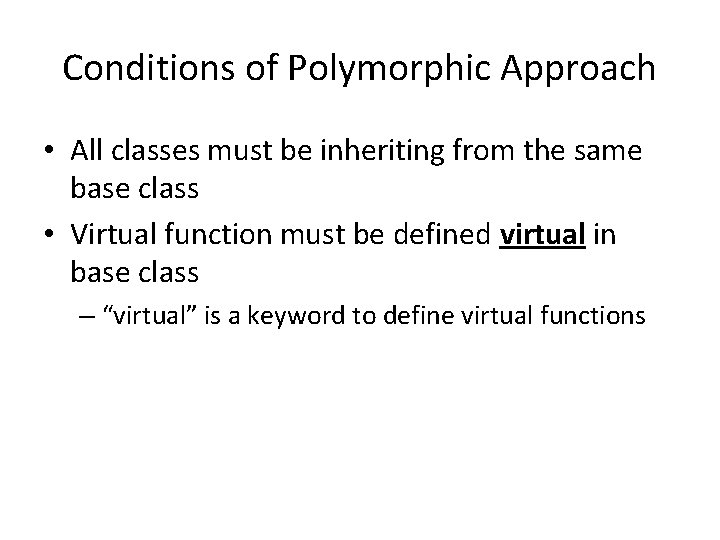
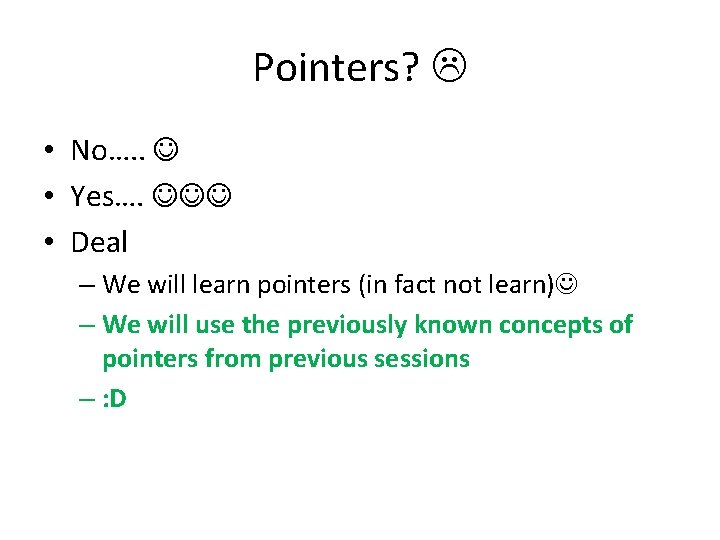
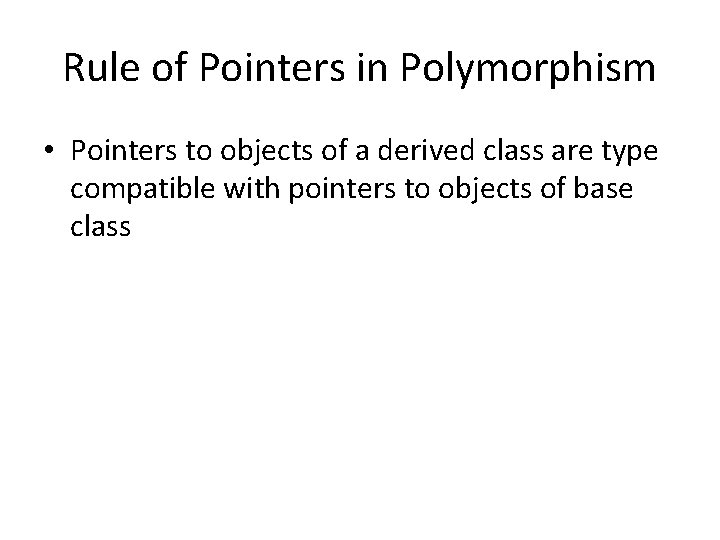
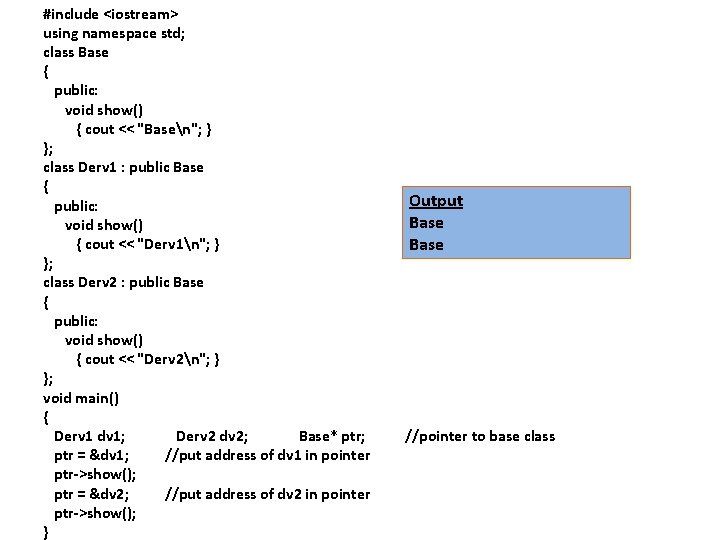
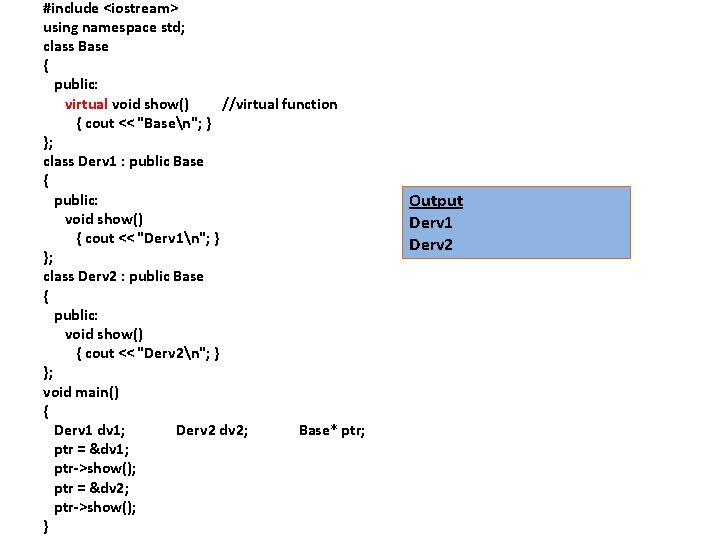
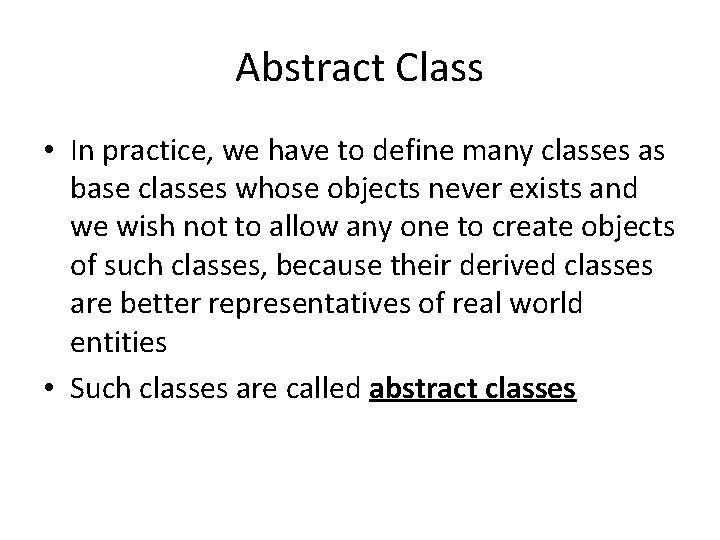
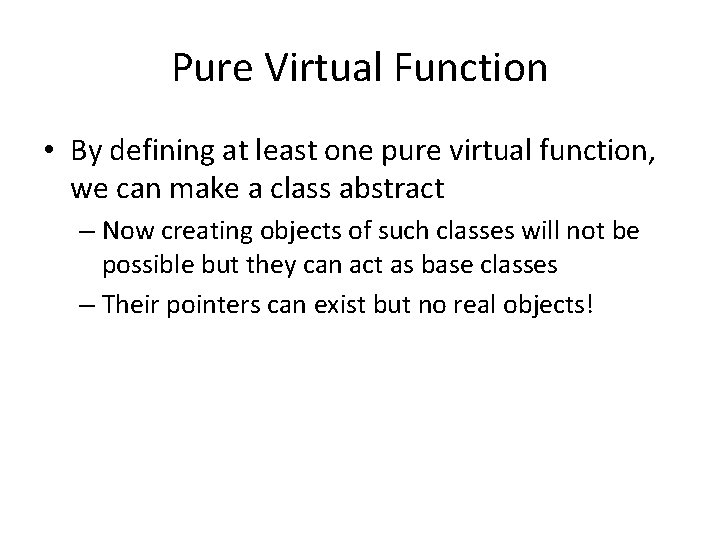
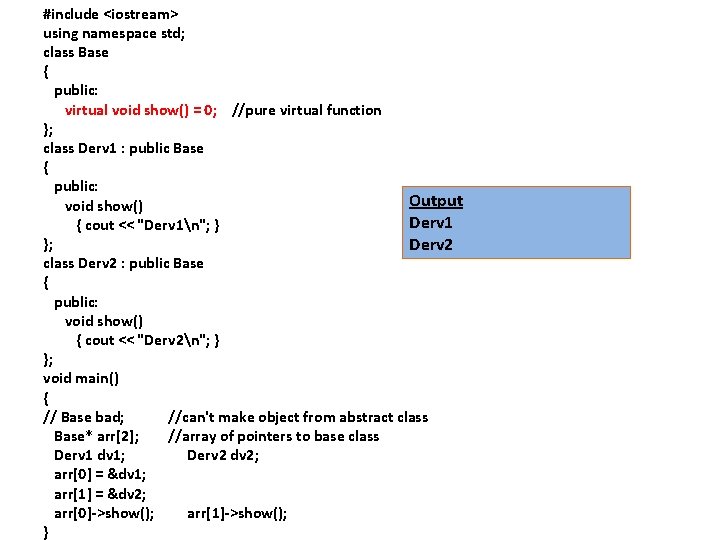
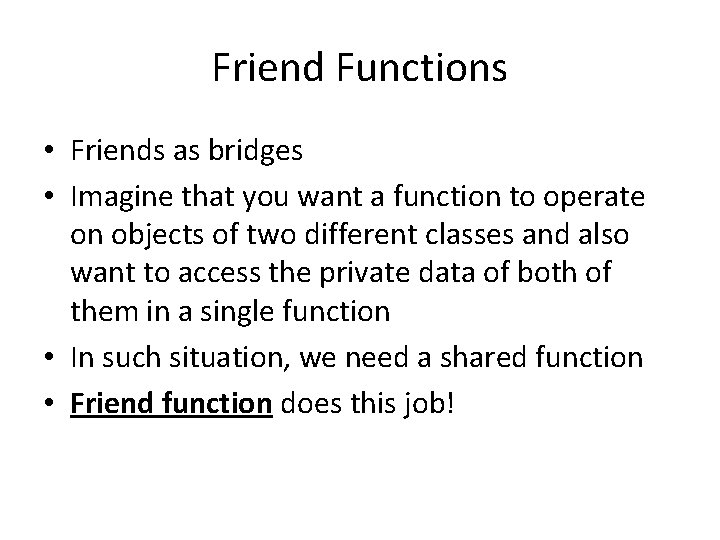
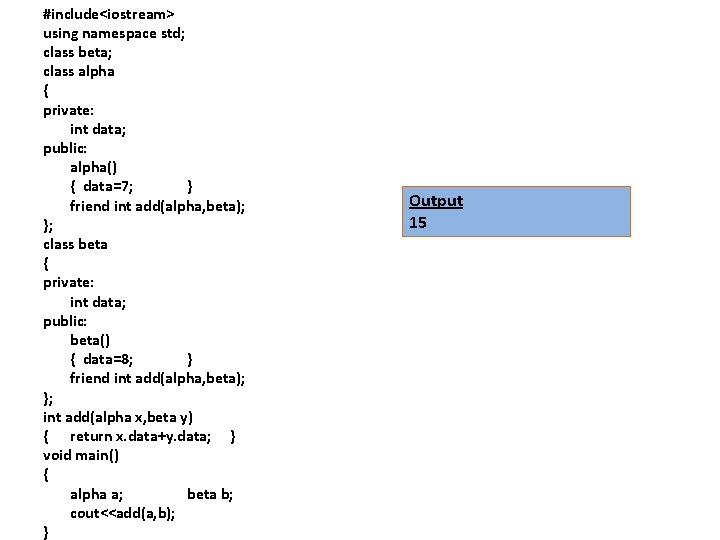
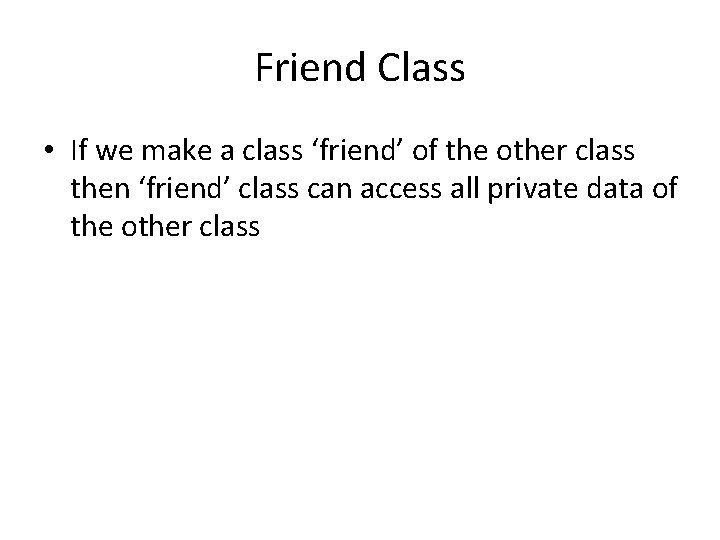
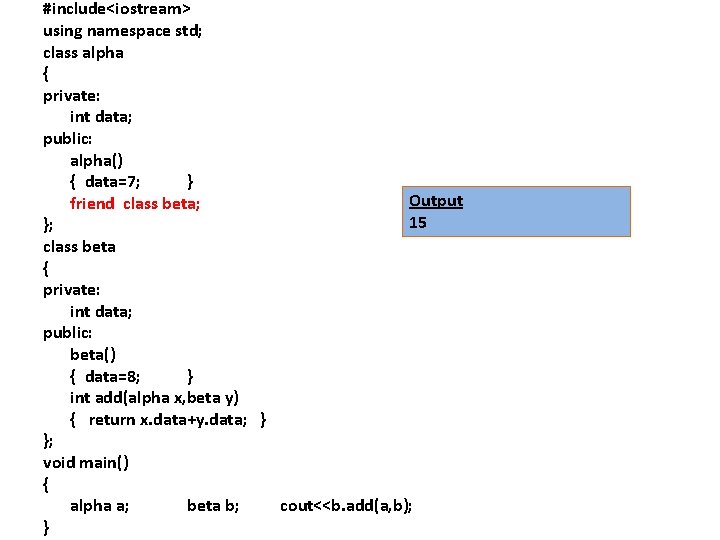
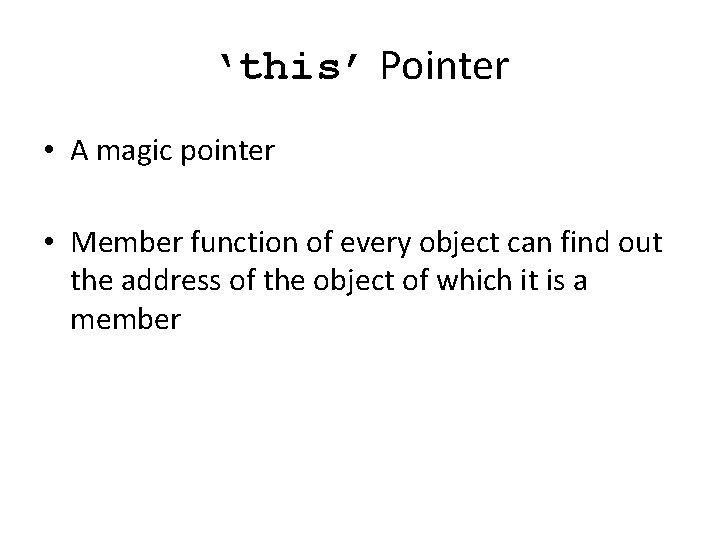
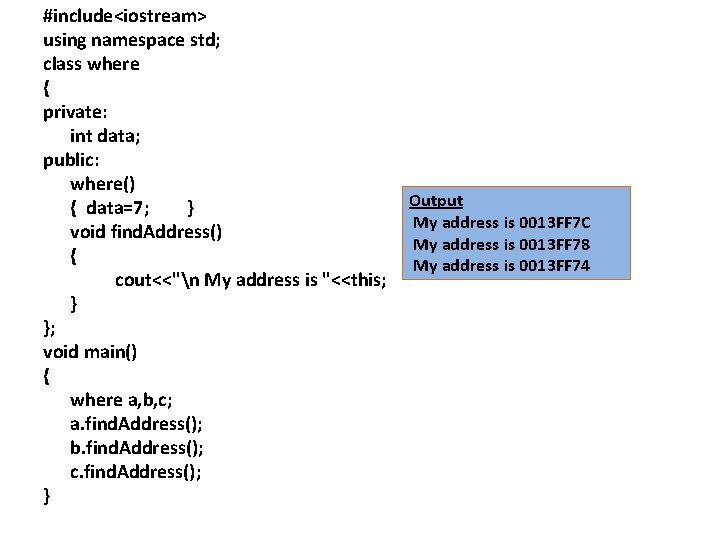
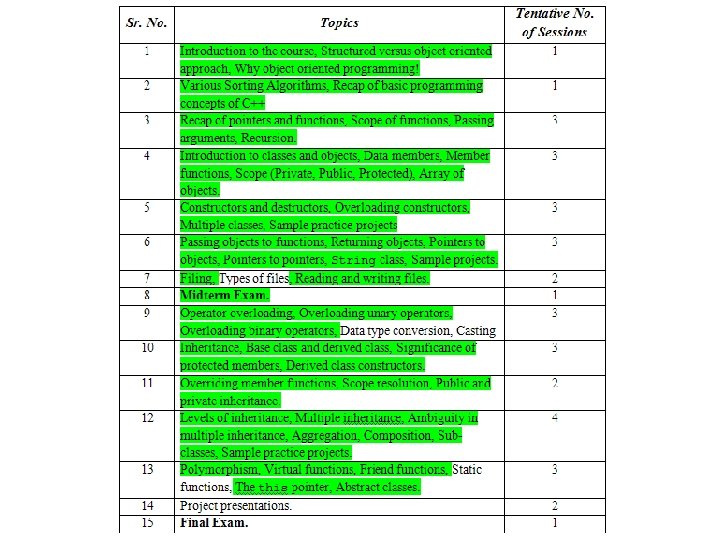
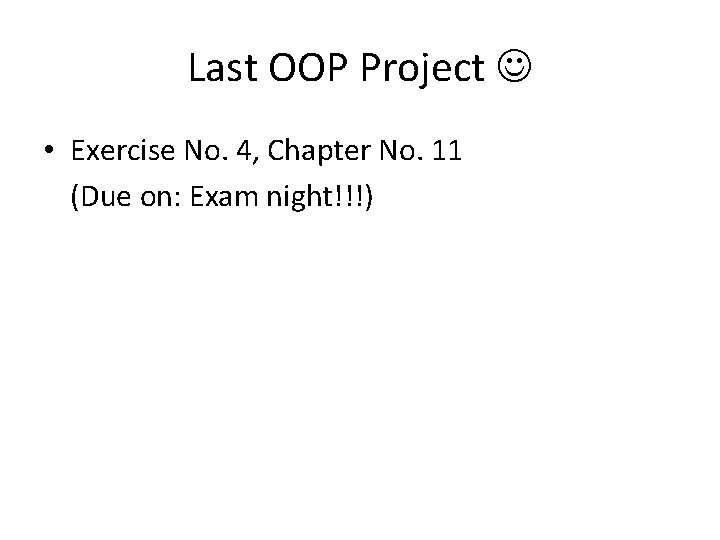
- Slides: 20
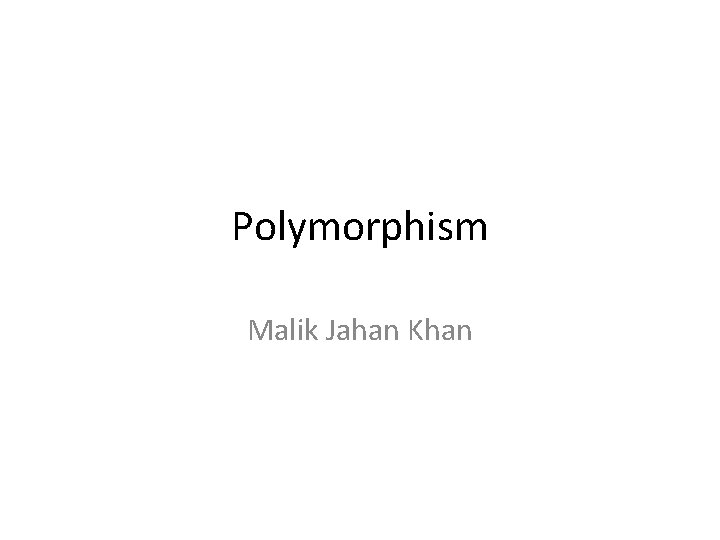
Polymorphism Malik Jahan Khan
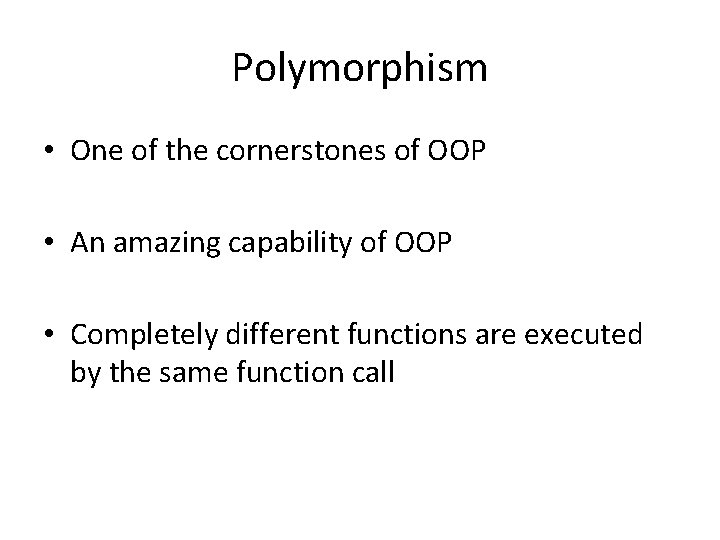
Polymorphism • One of the cornerstones of OOP • An amazing capability of OOP • Completely different functions are executed by the same function call
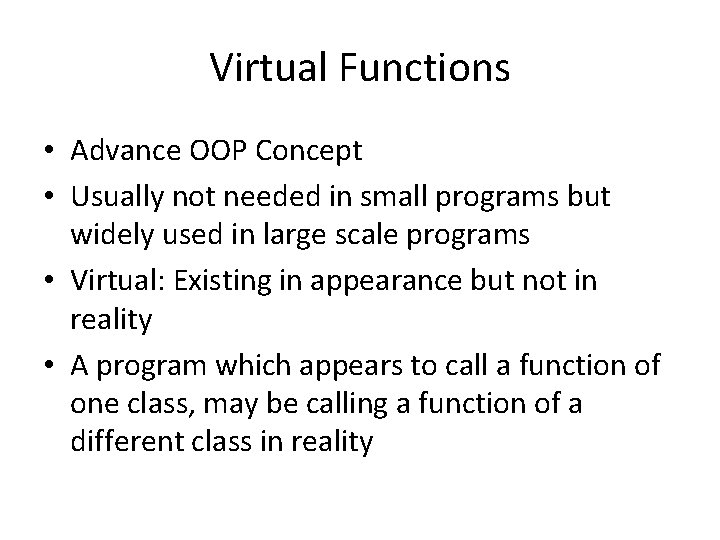
Virtual Functions • Advance OOP Concept • Usually not needed in small programs but widely used in large scale programs • Virtual: Existing in appearance but not in reality • A program which appears to call a function of one class, may be calling a function of a different class in reality
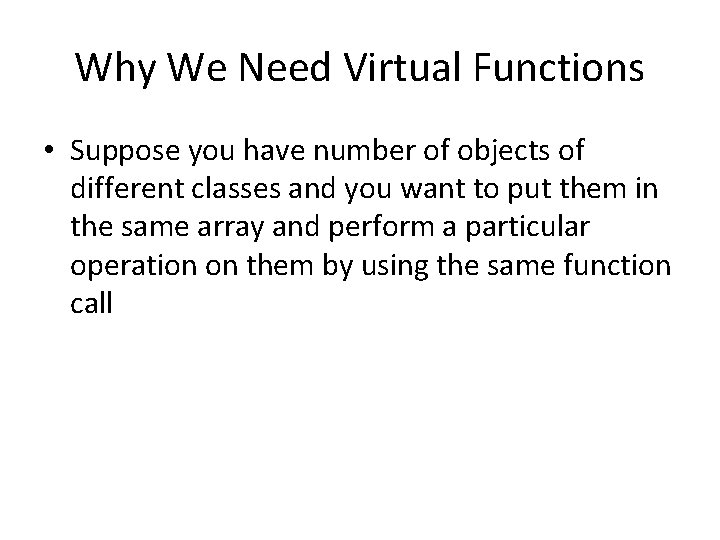
Why We Need Virtual Functions • Suppose you have number of objects of different classes and you want to put them in the same array and perform a particular operation on them by using the same function call
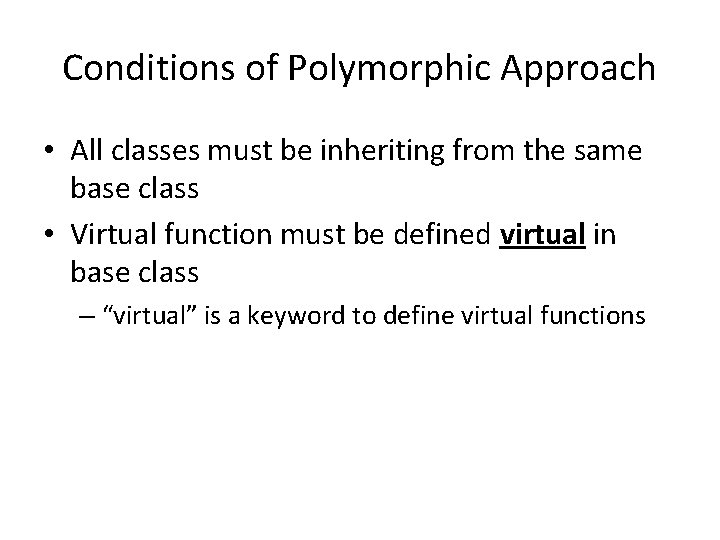
Conditions of Polymorphic Approach • All classes must be inheriting from the same base class • Virtual function must be defined virtual in base class – “virtual” is a keyword to define virtual functions
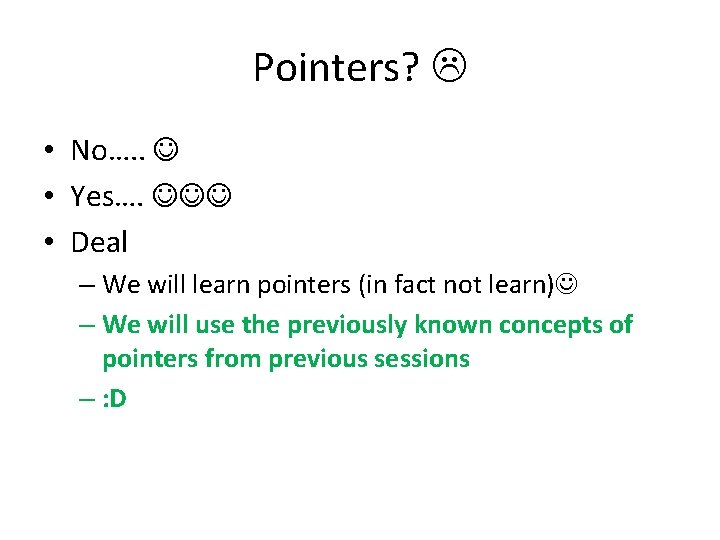
Pointers? • No…. . • Yes…. • Deal – We will learn pointers (in fact not learn) – We will use the previously known concepts of pointers from previous sessions – : D
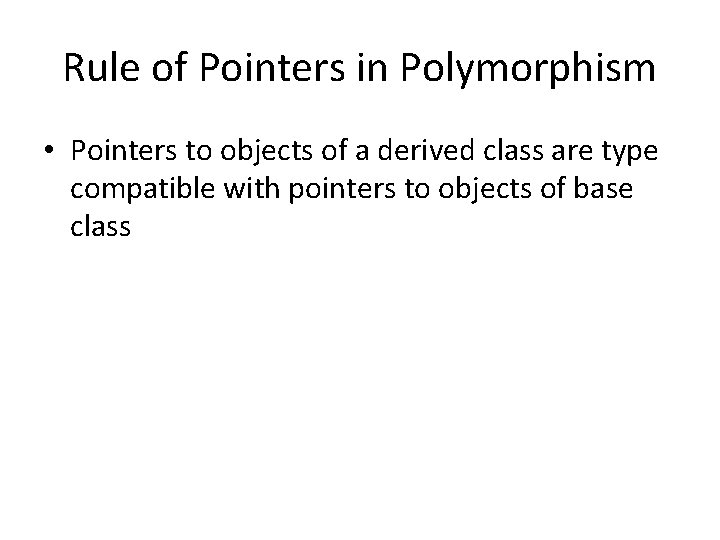
Rule of Pointers in Polymorphism • Pointers to objects of a derived class are type compatible with pointers to objects of base class
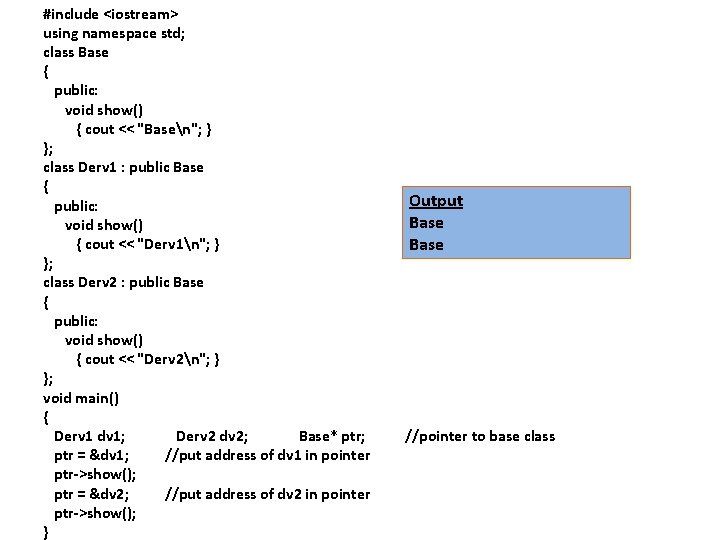
#include <iostream> using namespace std; class Base { public: void show() { cout << "Basen"; } }; class Derv 1 : public Base { public: void show() { cout << "Derv 1n"; } }; class Derv 2 : public Base { public: void show() { cout << "Derv 2n"; } }; void main() { Derv 1 dv 1; Derv 2 dv 2; Base* ptr; ptr = &dv 1; //put address of dv 1 in pointer ptr->show(); ptr = &dv 2; //put address of dv 2 in pointer ptr->show(); } Output Base //pointer to base class
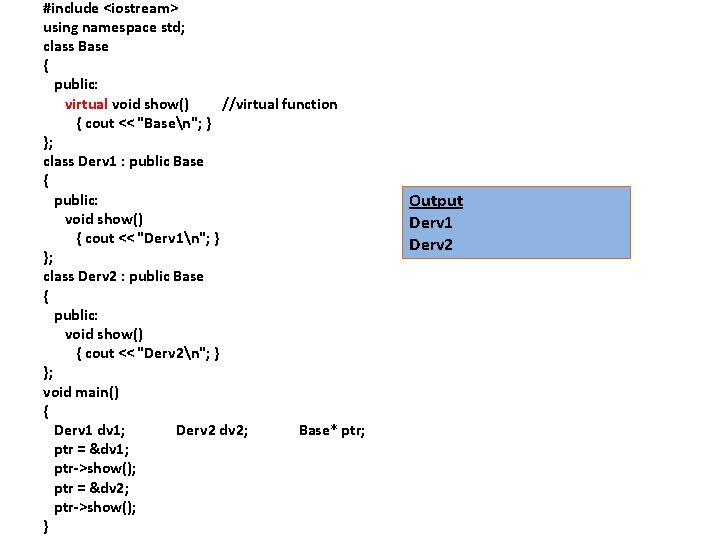
#include <iostream> using namespace std; class Base { public: virtual void show() //virtual function { cout << "Basen"; } }; class Derv 1 : public Base { public: void show() { cout << "Derv 1n"; } }; class Derv 2 : public Base { public: void show() { cout << "Derv 2n"; } }; void main() { Derv 1 dv 1; Derv 2 dv 2; Base* ptr; ptr = &dv 1; ptr->show(); ptr = &dv 2; ptr->show(); } Output Derv 1 Derv 2
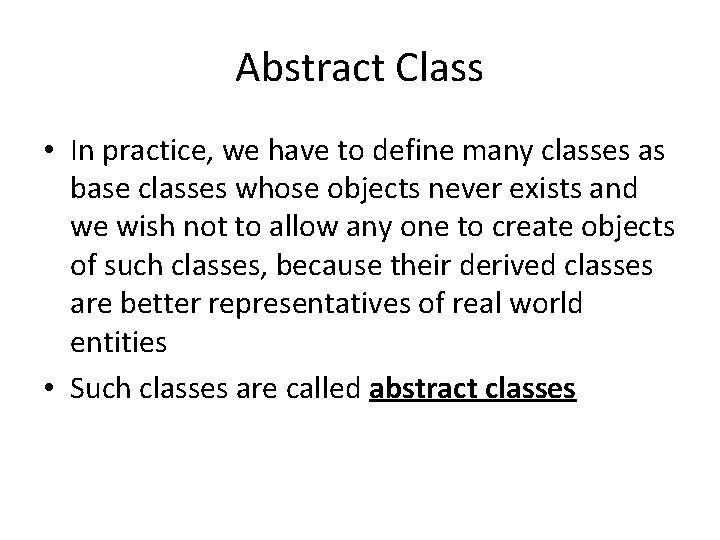
Abstract Class • In practice, we have to define many classes as base classes whose objects never exists and we wish not to allow any one to create objects of such classes, because their derived classes are better representatives of real world entities • Such classes are called abstract classes
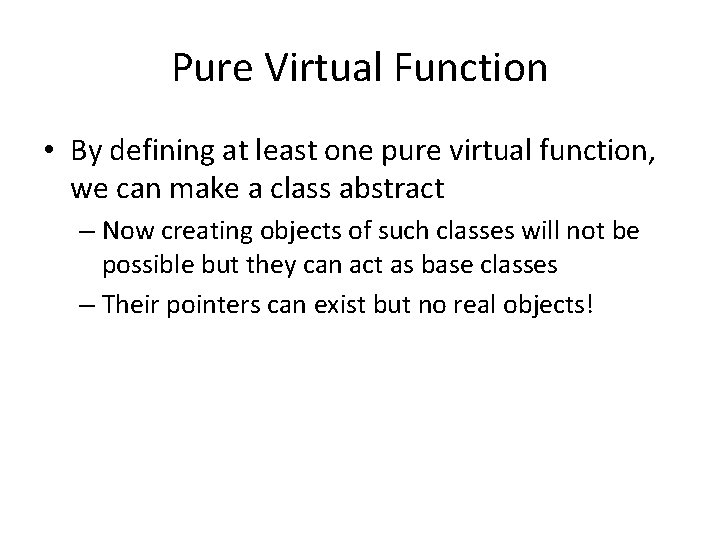
Pure Virtual Function • By defining at least one pure virtual function, we can make a class abstract – Now creating objects of such classes will not be possible but they can act as base classes – Their pointers can exist but no real objects!
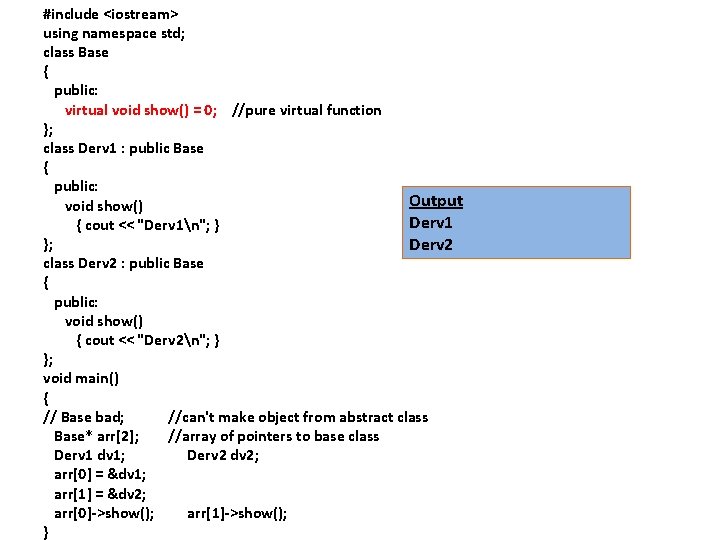
#include <iostream> using namespace std; class Base { public: virtual void show() = 0; //pure virtual function }; class Derv 1 : public Base { public: Output void show() Derv 1 { cout << "Derv 1n"; } }; Derv 2 class Derv 2 : public Base { public: void show() { cout << "Derv 2n"; } }; void main() { // Base bad; //can't make object from abstract class Base* arr[2]; //array of pointers to base class Derv 1 dv 1; Derv 2 dv 2; arr[0] = &dv 1; arr[1] = &dv 2; arr[0]->show(); arr[1]->show(); }
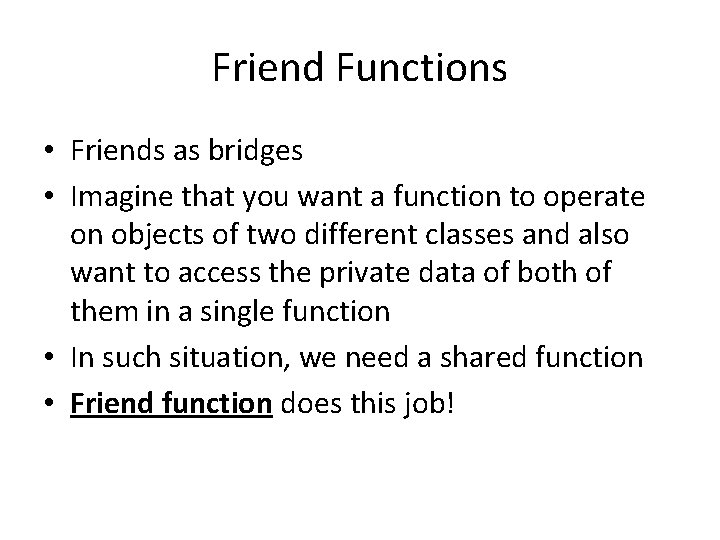
Friend Functions • Friends as bridges • Imagine that you want a function to operate on objects of two different classes and also want to access the private data of both of them in a single function • In such situation, we need a shared function • Friend function does this job!
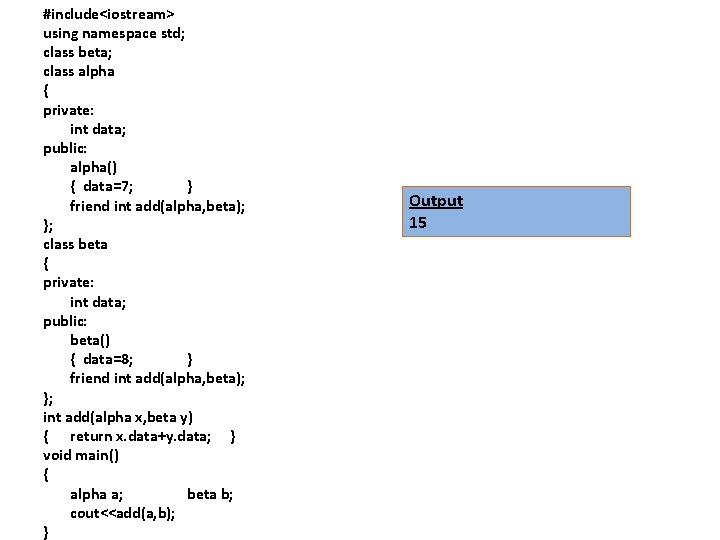
#include<iostream> using namespace std; class beta; class alpha { private: int data; public: alpha() { data=7; } friend int add(alpha, beta); }; class beta { private: int data; public: beta() { data=8; } friend int add(alpha, beta); }; int add(alpha x, beta y) { return x. data+y. data; } void main() { alpha a; beta b; cout<<add(a, b); } Output 15
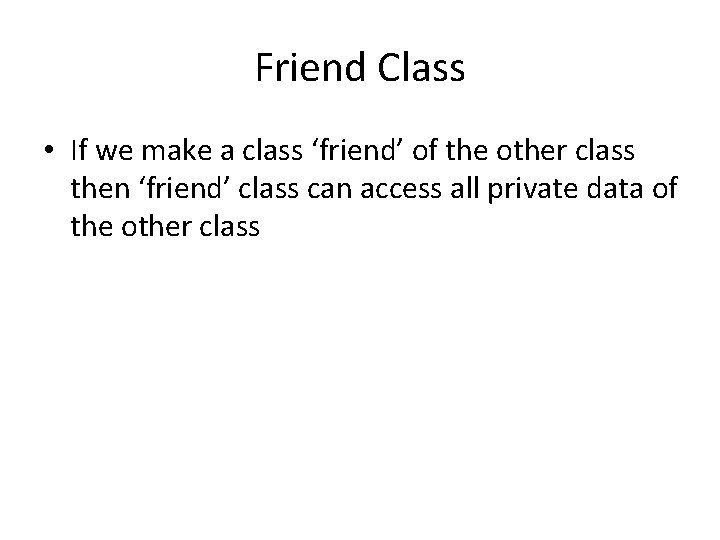
Friend Class • If we make a class ‘friend’ of the other class then ‘friend’ class can access all private data of the other class
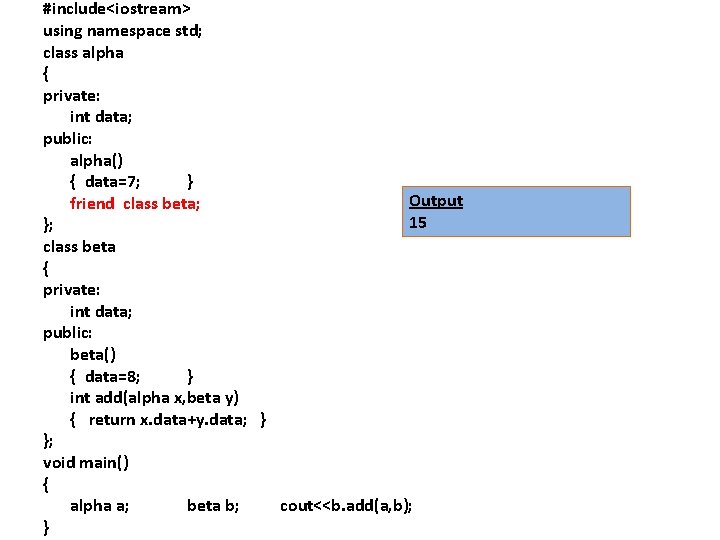
#include<iostream> using namespace std; class alpha { private: int data; public: alpha() { data=7; } Output friend class beta; 15 }; class beta { private: int data; public: beta() { data=8; } int add(alpha x, beta y) { return x. data+y. data; } }; void main() { alpha a; beta b; cout<<b. add(a, b); }
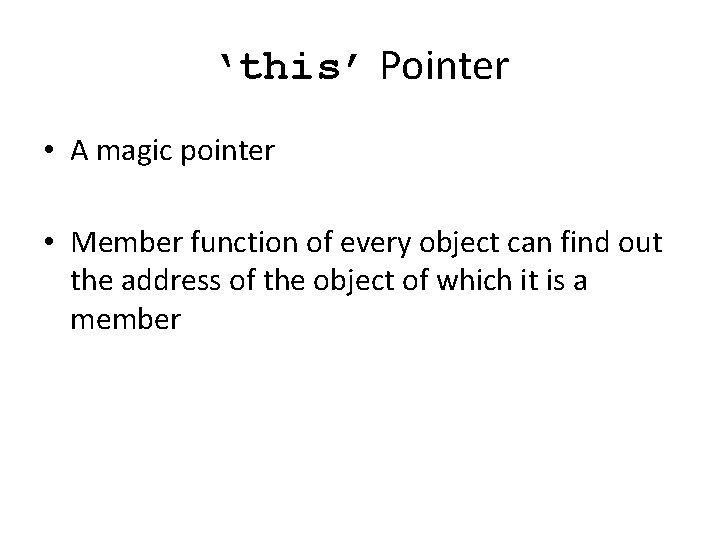
‘this’ Pointer • A magic pointer • Member function of every object can find out the address of the object of which it is a member
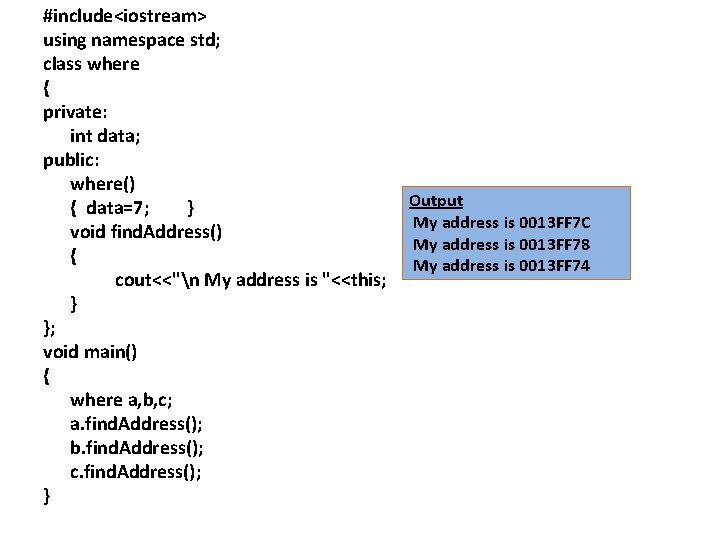
#include<iostream> using namespace std; class where { private: int data; public: where() { data=7; } void find. Address() { cout<<"n My address is "<<this; } }; void main() { where a, b, c; a. find. Address(); b. find. Address(); c. find. Address(); } Output My address is 0013 FF 7 C My address is 0013 FF 78 My address is 0013 FF 74
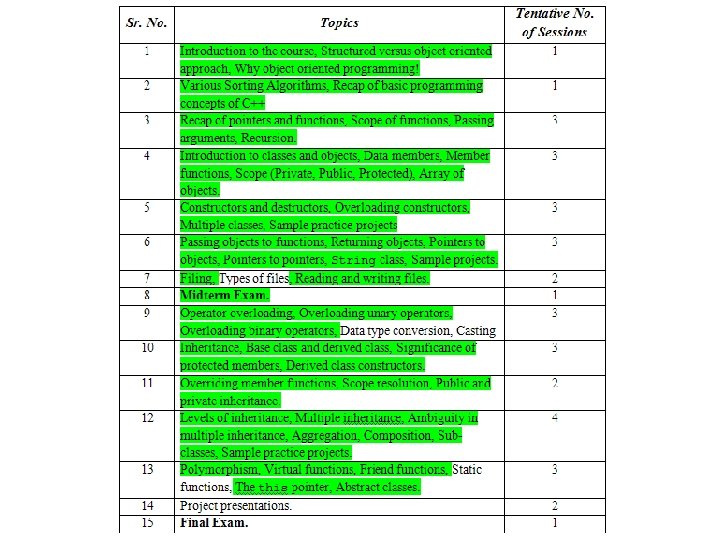
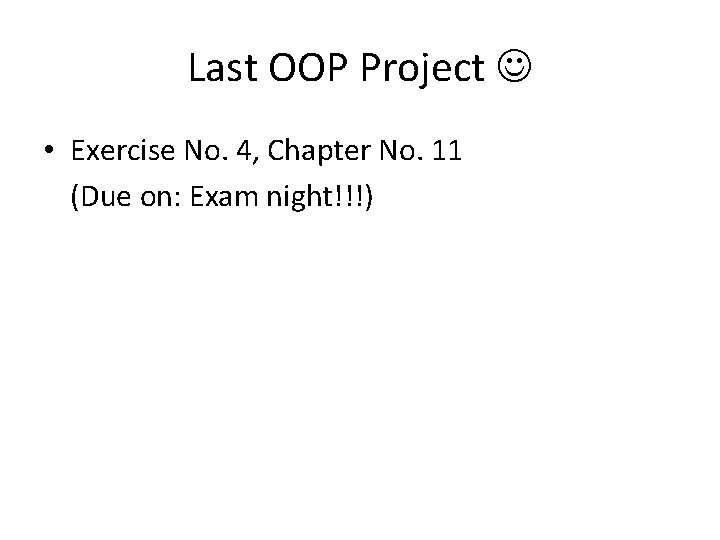
Last OOP Project • Exercise No. 4, Chapter No. 11 (Due on: Exam night!!!)
Malik jahan khan
Khan el destructor
Tanu malik depaul
Taj mahal poem line by line explanation
Bpp ultrasound score
Dr jahan
Shah jahan accomplishments
Hasin jahan wateraid
One empire one god one emperor
One one one little dogs run
One king one law one faith
One god one empire one emperor
One team one plan one goal
See one do one teach one
One price policy
One face one voice one habit and two persons
See one do one teach one
Asean tourism strategic plan
Graphic organizer with the aims of la liga filipina
Dr aqsa malik
Ali afzal malik