Polymorphism Agenda What is Why Polymorphism Polymorphism Examples
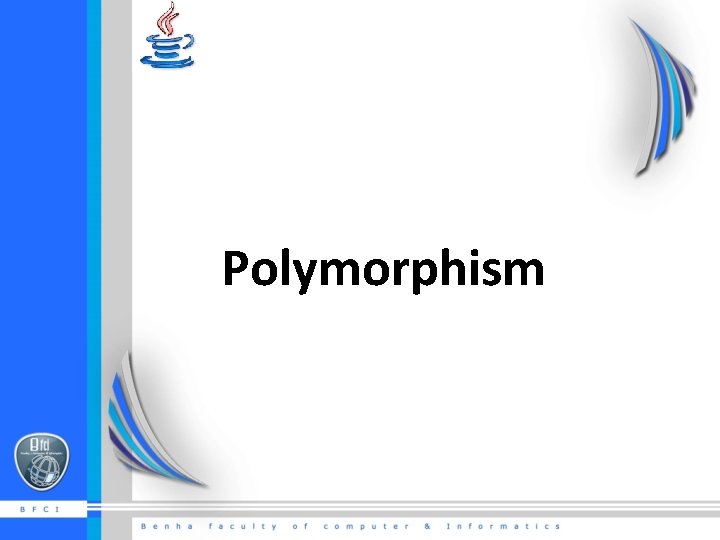
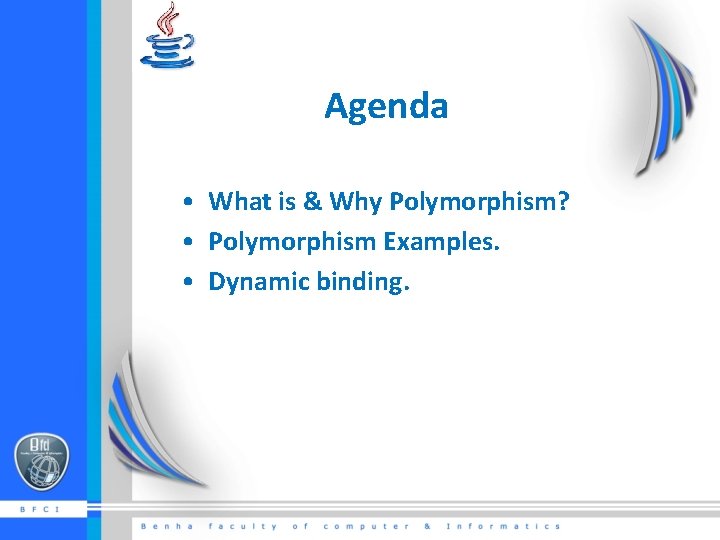
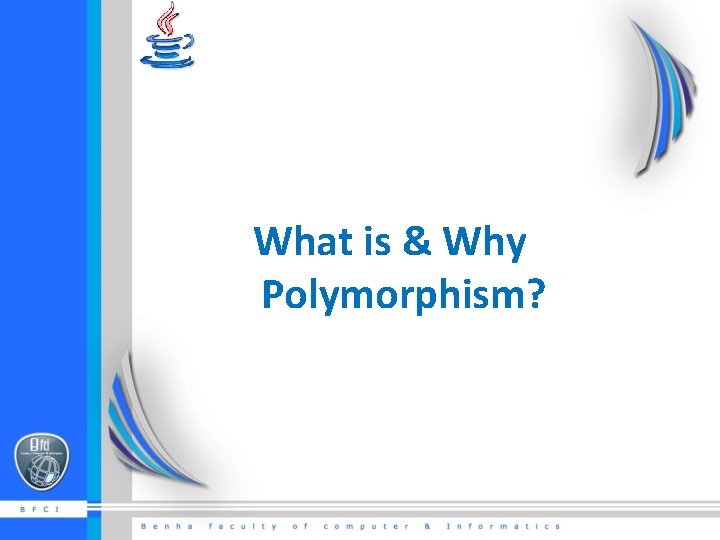
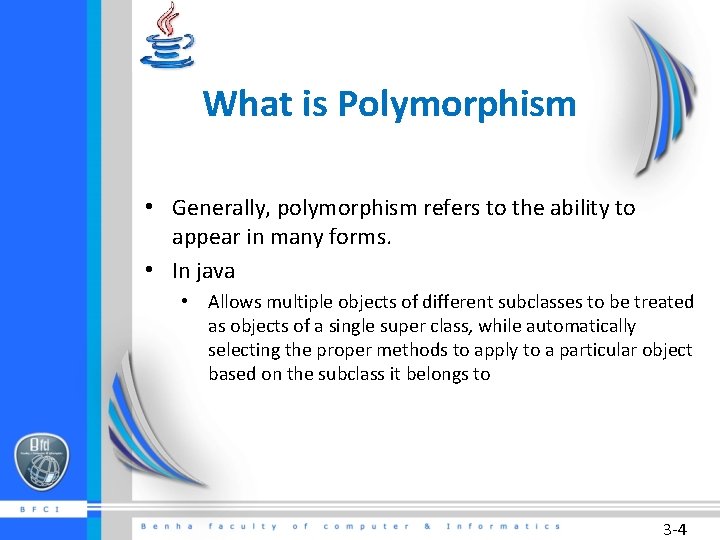
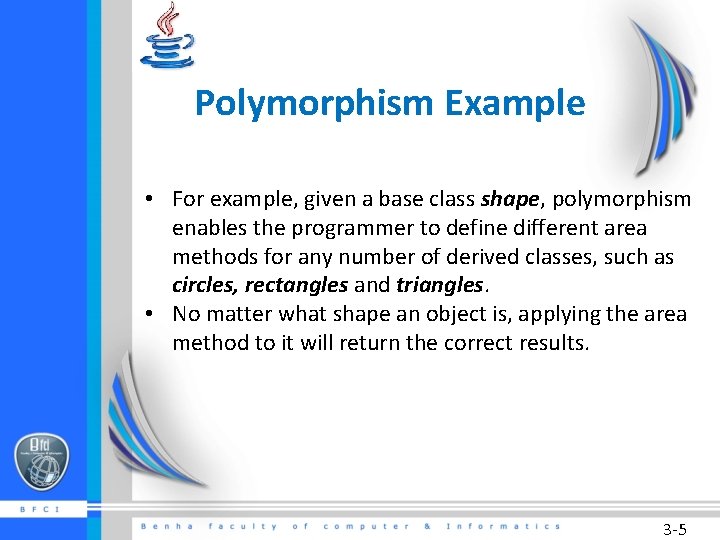
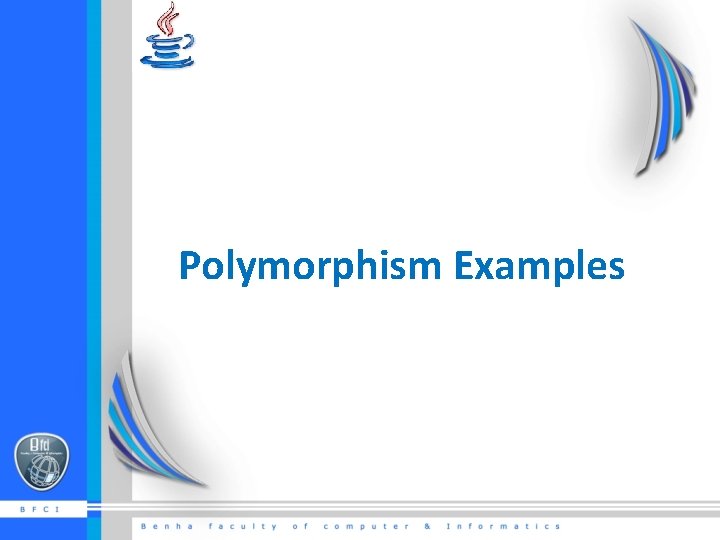
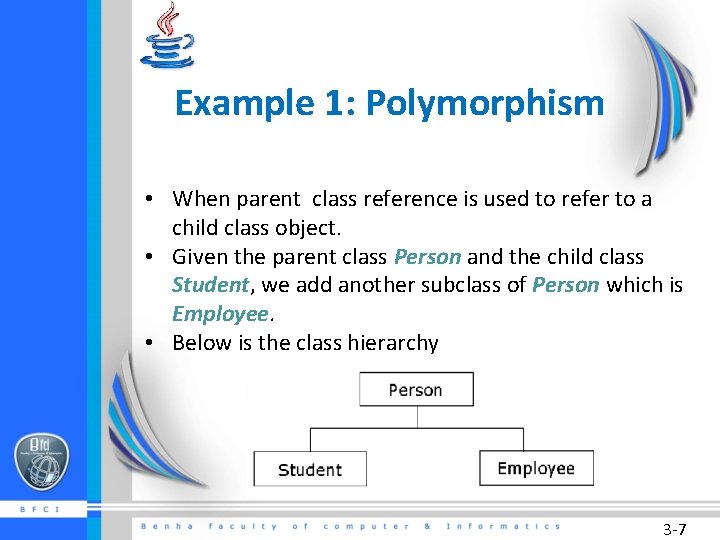
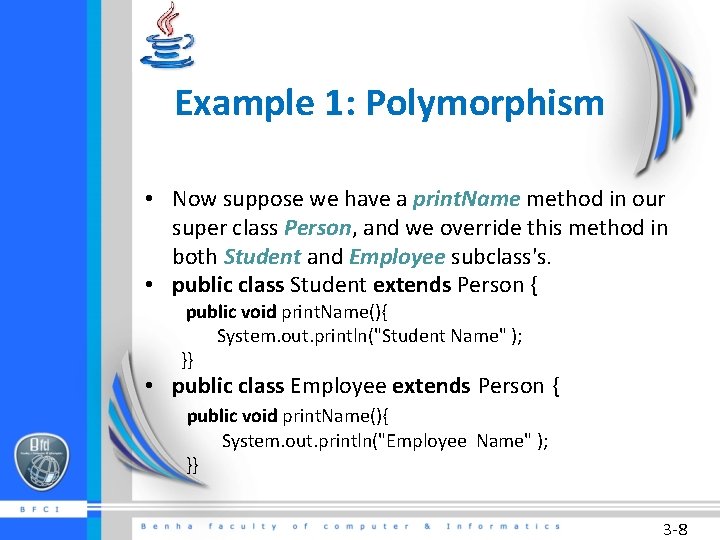
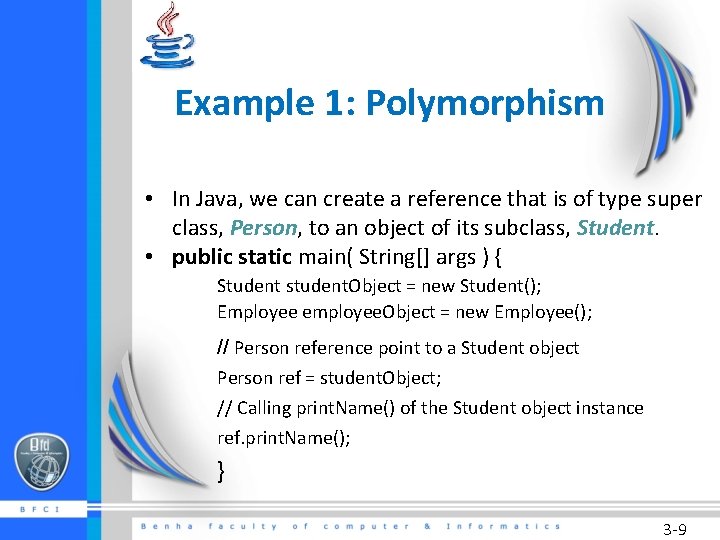
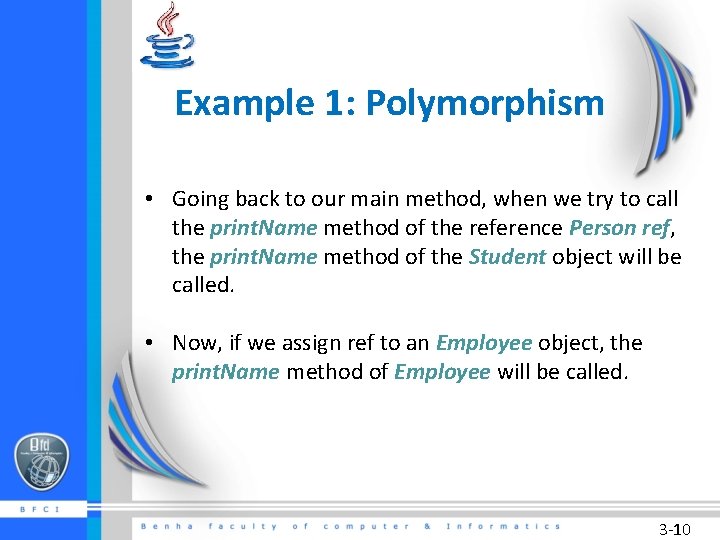
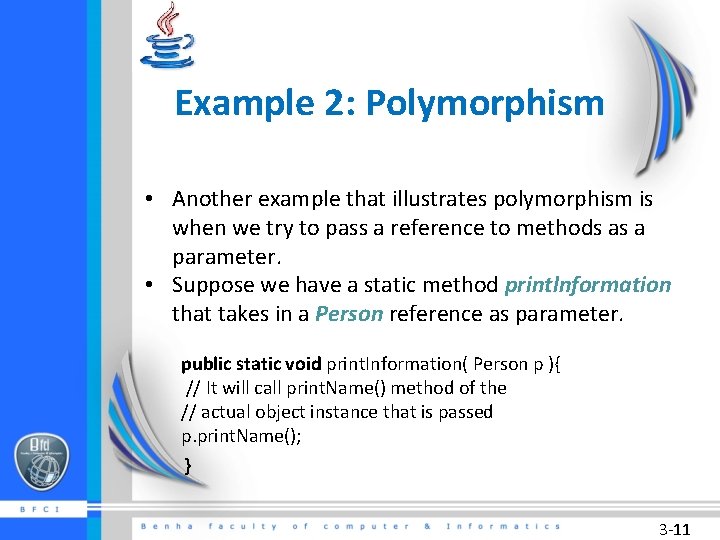
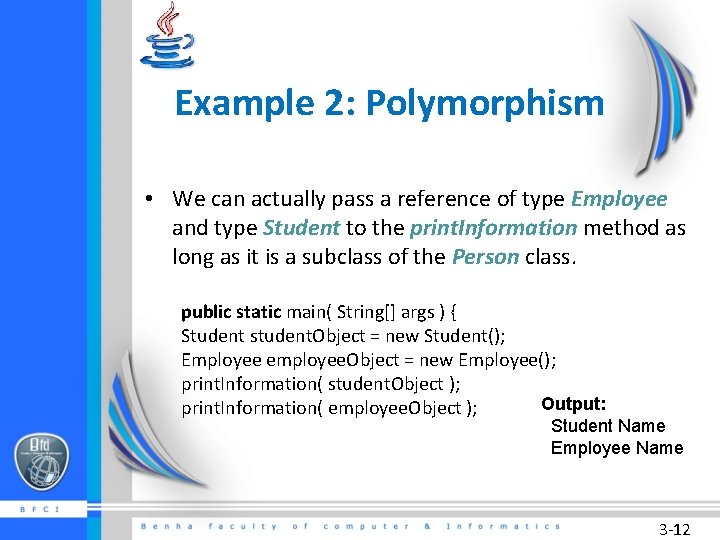
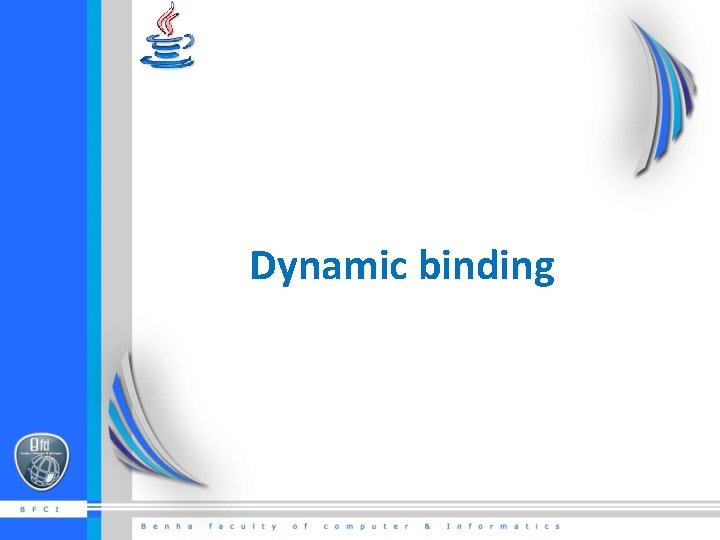
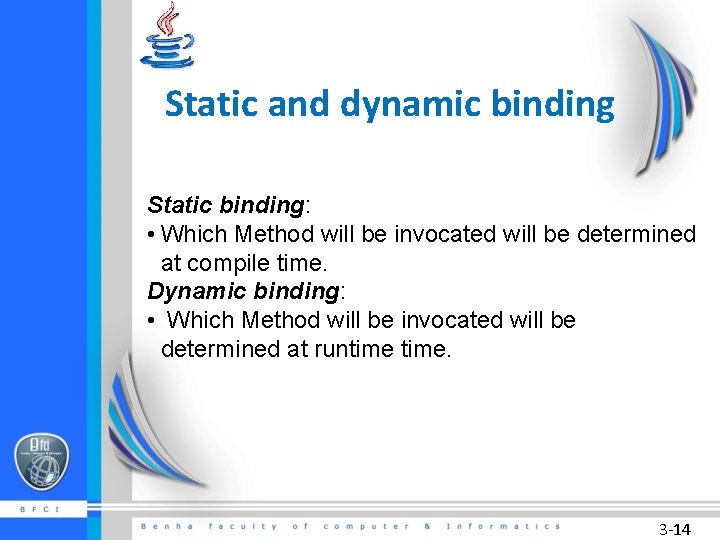
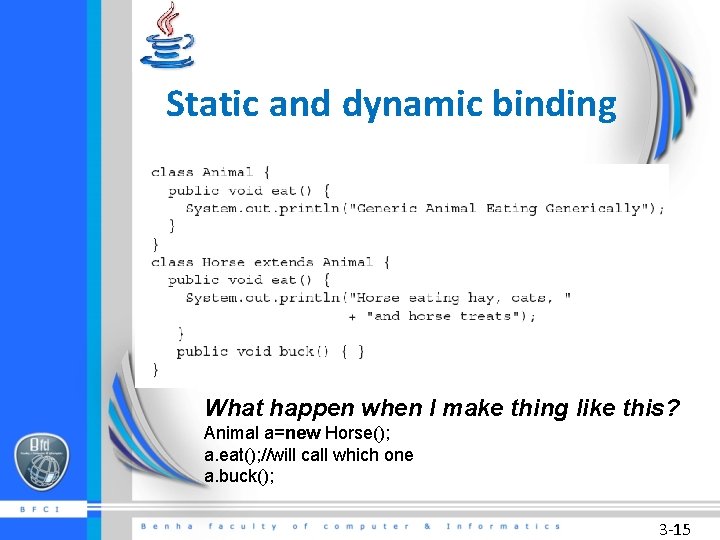
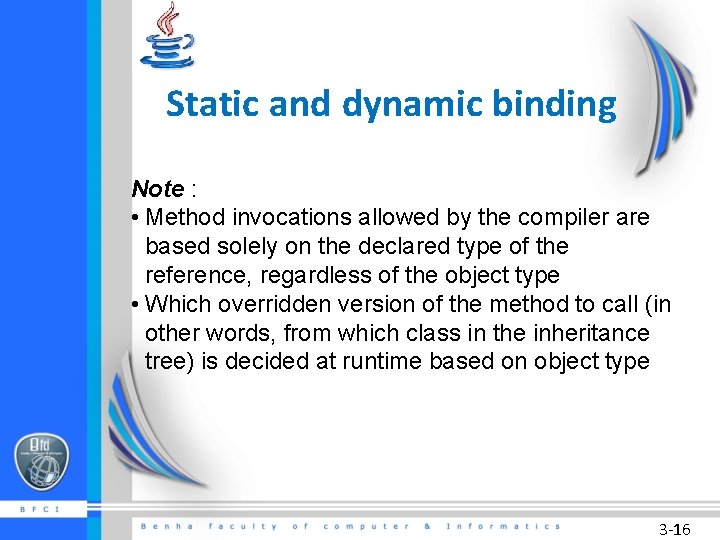
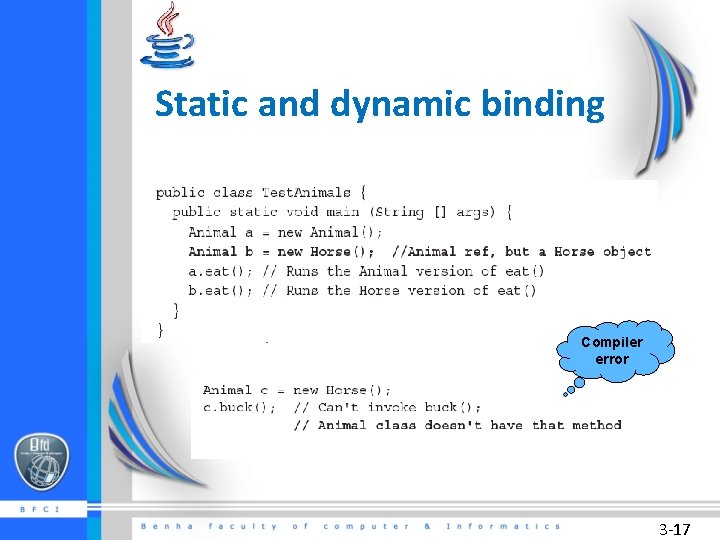
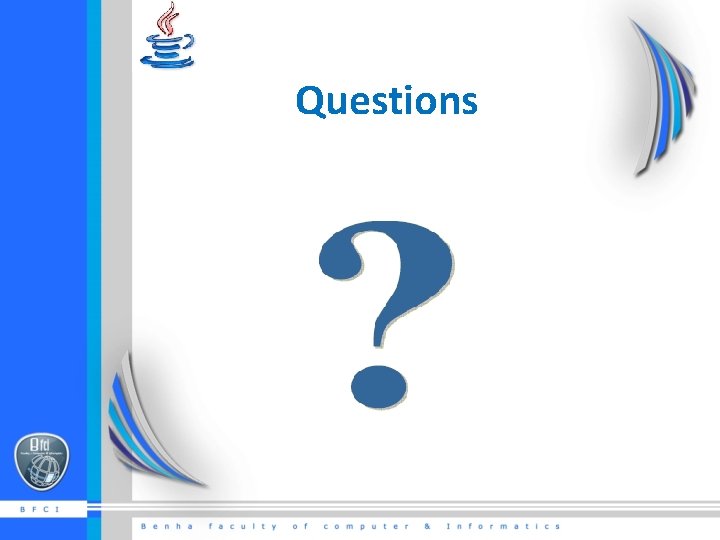
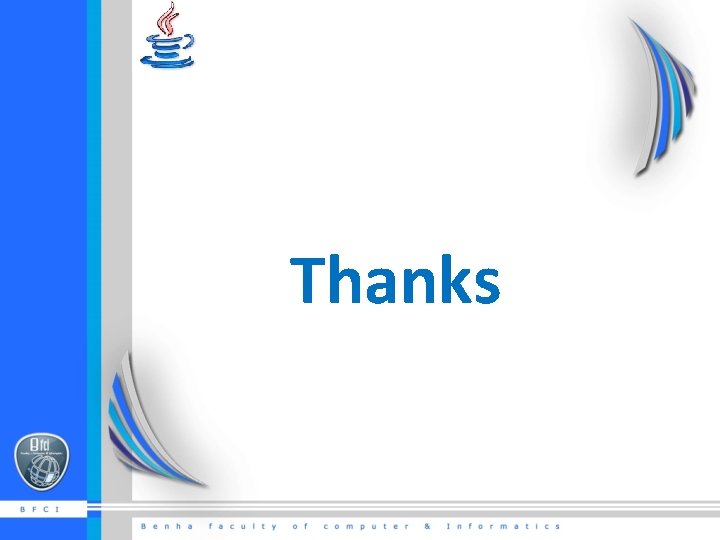
- Slides: 19
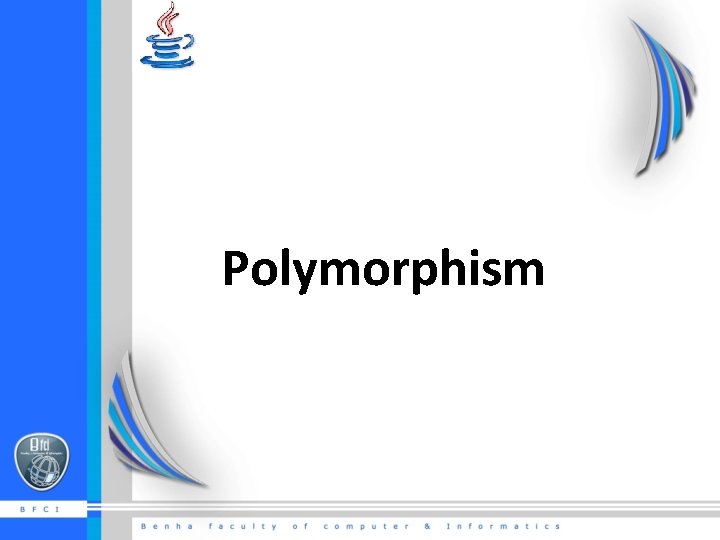
Polymorphism
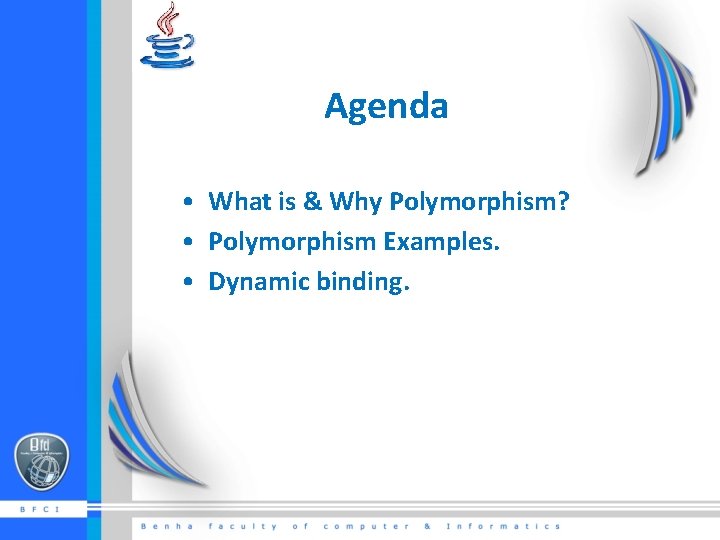
Agenda • What is & Why Polymorphism? • Polymorphism Examples. • Dynamic binding.
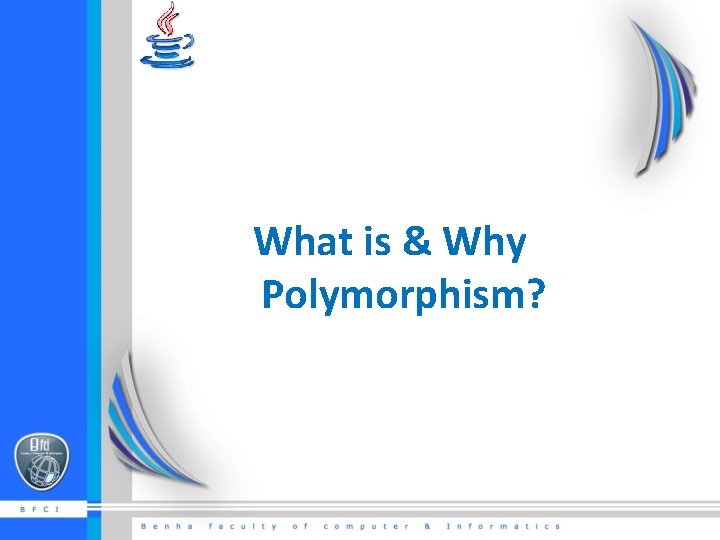
What is & Why Polymorphism?
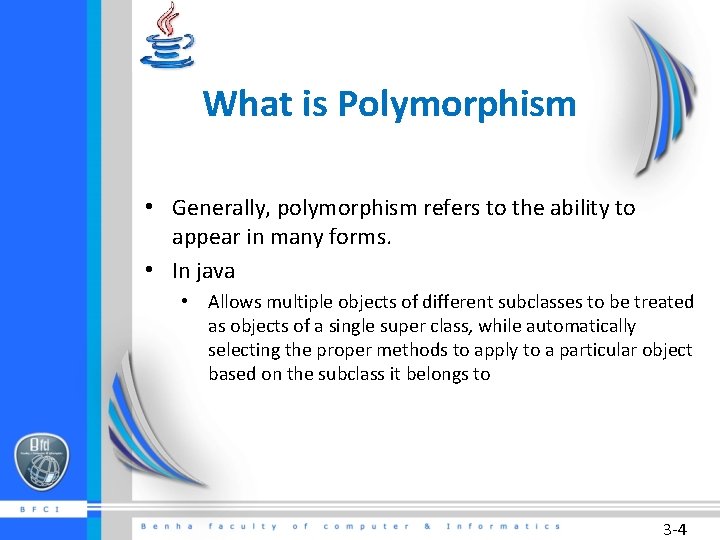
What is Polymorphism • Generally, polymorphism refers to the ability to appear in many forms. • In java • Allows multiple objects of different subclasses to be treated as objects of a single super class, while automatically selecting the proper methods to apply to a particular object based on the subclass it belongs to 3 -4
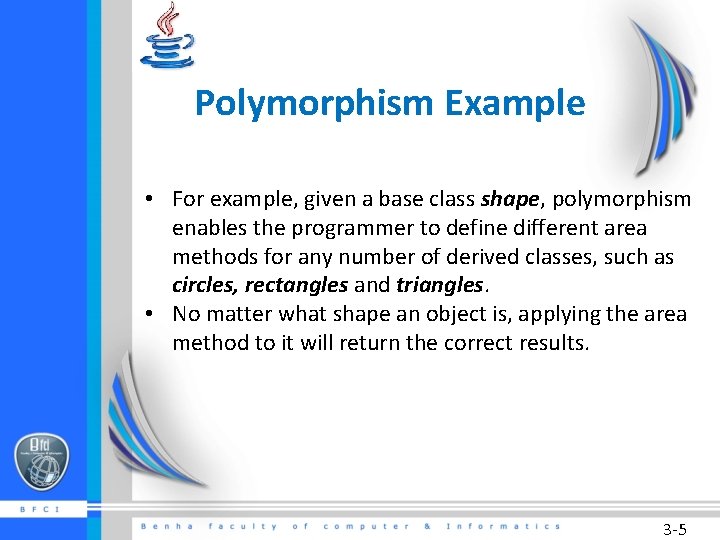
Polymorphism Example • For example, given a base class shape, polymorphism enables the programmer to define different area methods for any number of derived classes, such as circles, rectangles and triangles. • No matter what shape an object is, applying the area method to it will return the correct results. 3 -5
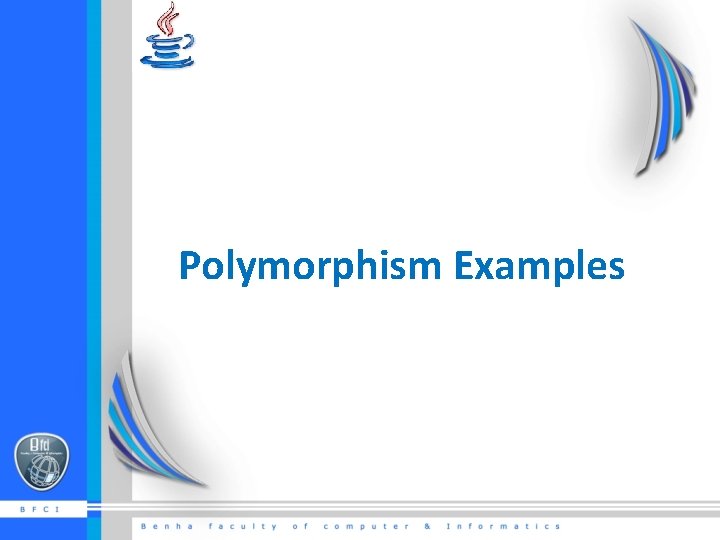
Polymorphism Examples
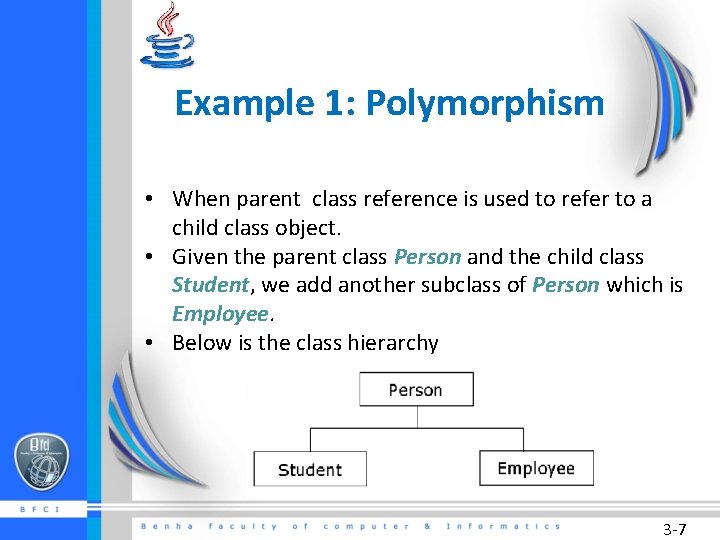
Example 1: Polymorphism • When parent class reference is used to refer to a child class object. • Given the parent class Person and the child class Student, we add another subclass of Person which is Employee. • Below is the class hierarchy 3 -7
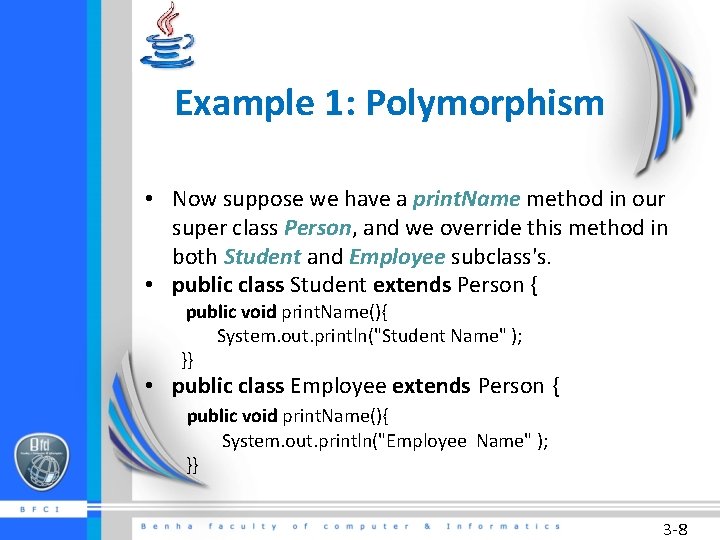
Example 1: Polymorphism • Now suppose we have a print. Name method in our super class Person, and we override this method in both Student and Employee subclass's. • public class Student extends Person { public void print. Name(){ System. out. println("Student Name" ); }} • public class Employee extends Person { public void print. Name(){ System. out. println("Employee Name" ); }} 3 -8
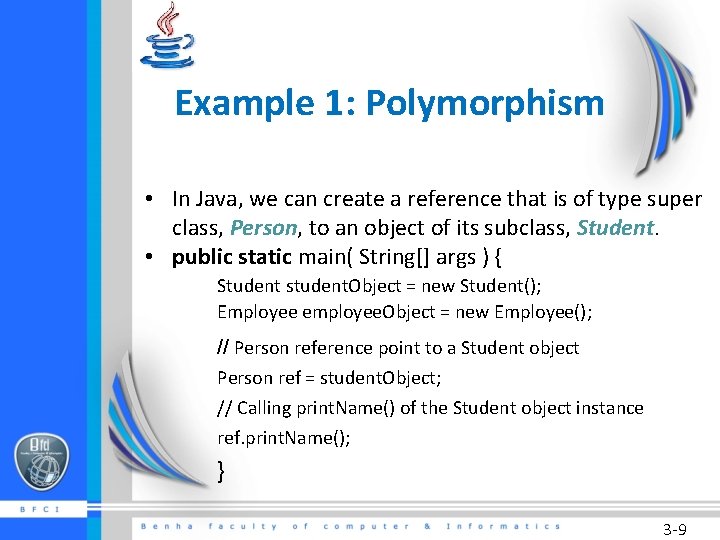
Example 1: Polymorphism • In Java, we can create a reference that is of type super class, Person, to an object of its subclass, Student. • public static main( String[] args ) { Student student. Object = new Student(); Employee employee. Object = new Employee(); // Person reference point to a Student object Person ref = student. Object; // Calling print. Name() of the Student object instance ref. print. Name(); } 3 -9
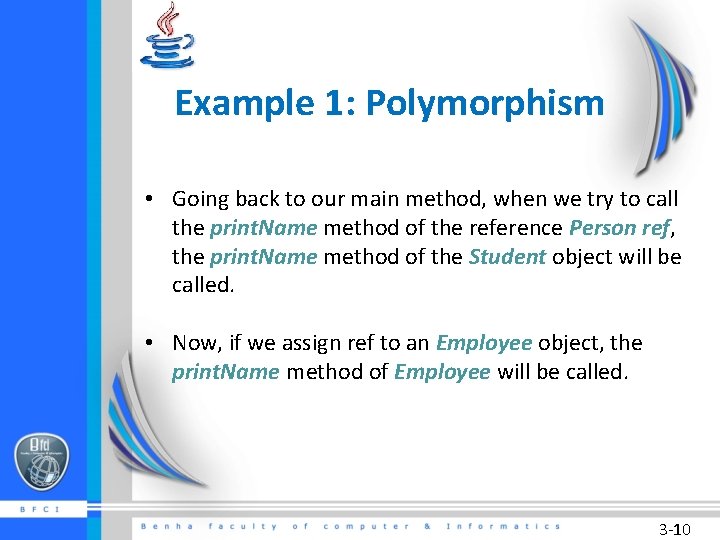
Example 1: Polymorphism • Going back to our main method, when we try to call the print. Name method of the reference Person ref, the print. Name method of the Student object will be called. • Now, if we assign ref to an Employee object, the print. Name method of Employee will be called. 3 -10
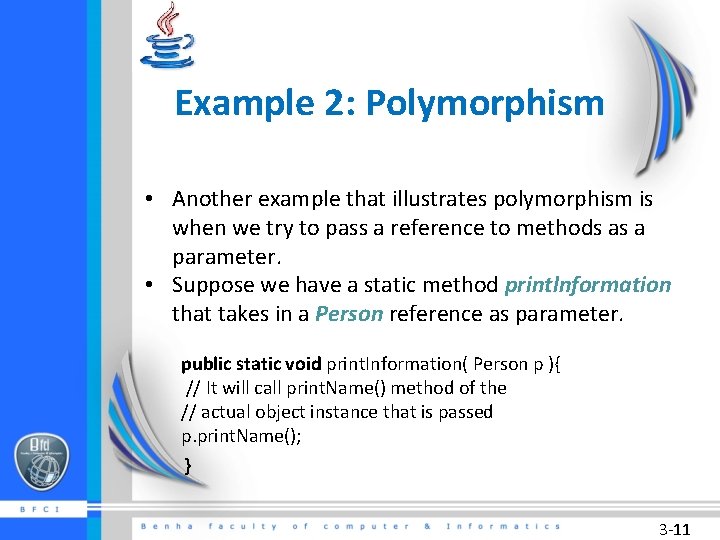
Example 2: Polymorphism • Another example that illustrates polymorphism is when we try to pass a reference to methods as a parameter. • Suppose we have a static method printlnformation that takes in a Person reference as parameter. public static void print. Information( Person p ){ // It will call print. Name() method of the // actual object instance that is passed p. print. Name(); } 3 -11
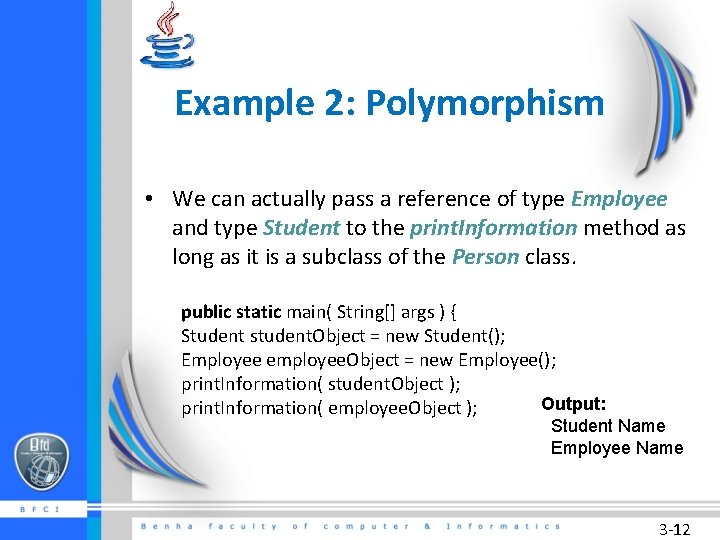
Example 2: Polymorphism • We can actually pass a reference of type Employee and type Student to the print. Information method as long as it is a subclass of the Person class. public static main( String[] args ) { Student student. Object = new Student(); Employee employee. Object = new Employee(); print. Information( student. Object ); Output: print. Information( employee. Object ); Student Name Employee Name 3 -12
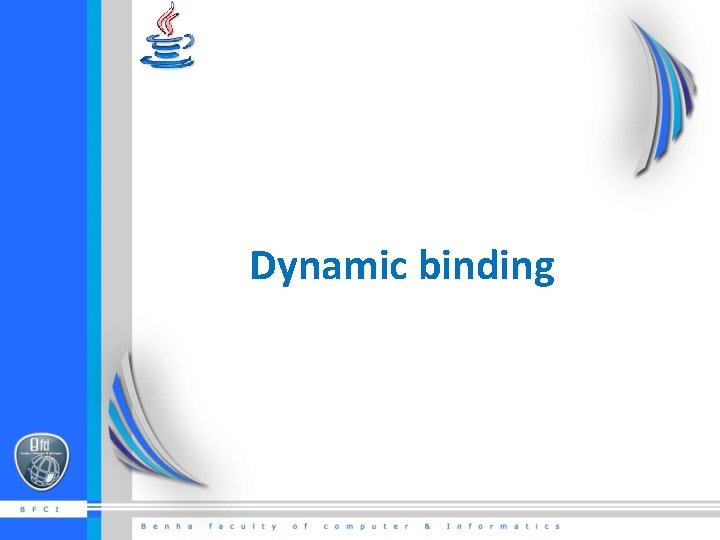
Dynamic binding
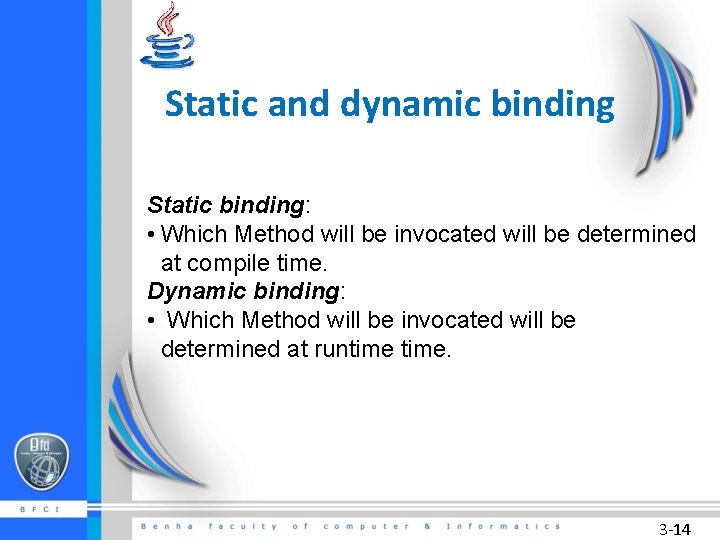
Static and dynamic binding Static binding: • Which Method will be invocated will be determined at compile time. Dynamic binding: • Which Method will be invocated will be determined at runtime. 3 -14
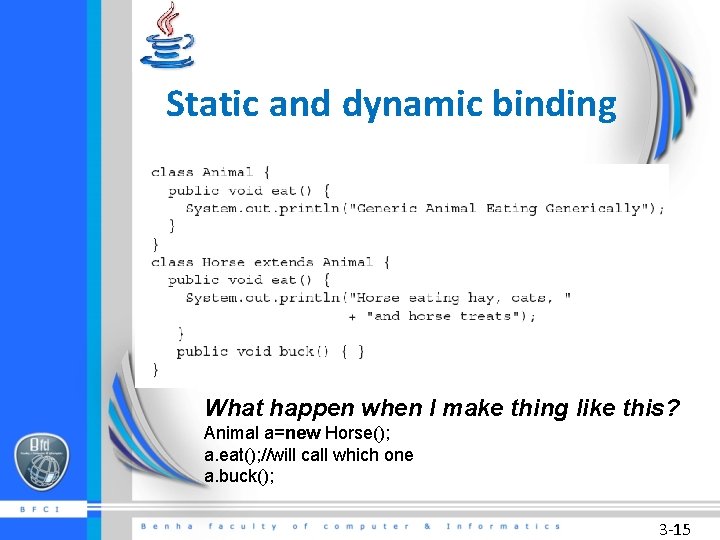
Static and dynamic binding What happen when I make thing like this? Animal a=new Horse(); a. eat(); //will call which one a. buck(); 3 -15
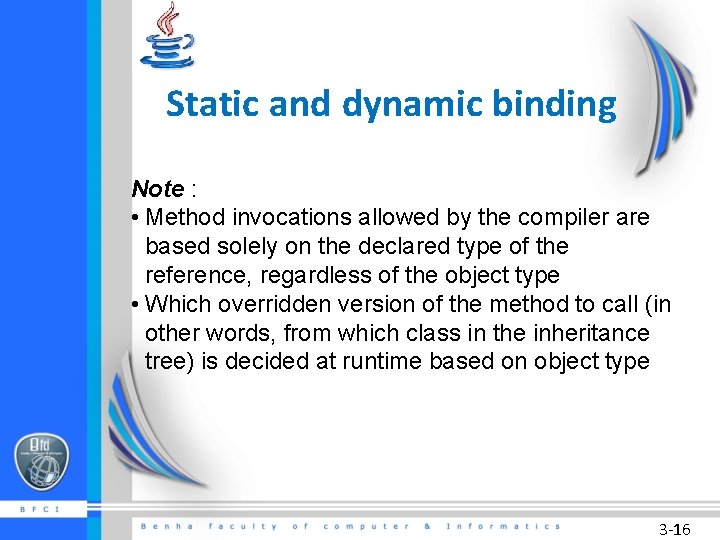
Static and dynamic binding Note : • Method invocations allowed by the compiler are based solely on the declared type of the reference, regardless of the object type • Which overridden version of the method to call (in other words, from which class in the inheritance tree) is decided at runtime based on object type 3 -16
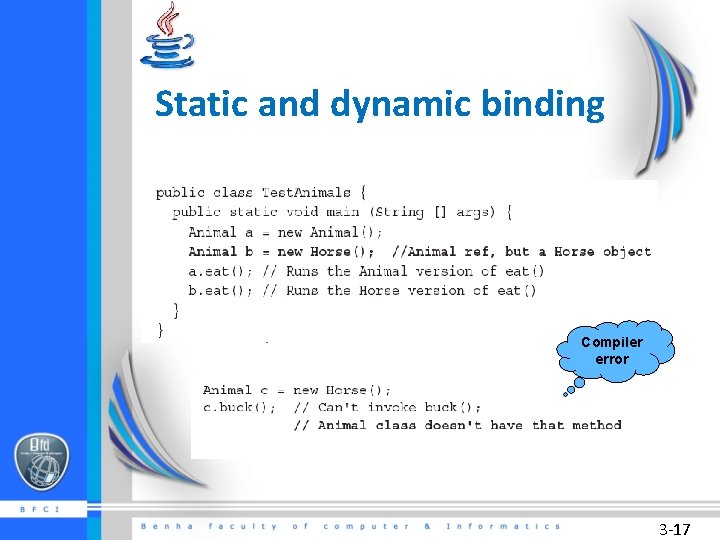
Static and dynamic binding Compiler error 3 -17
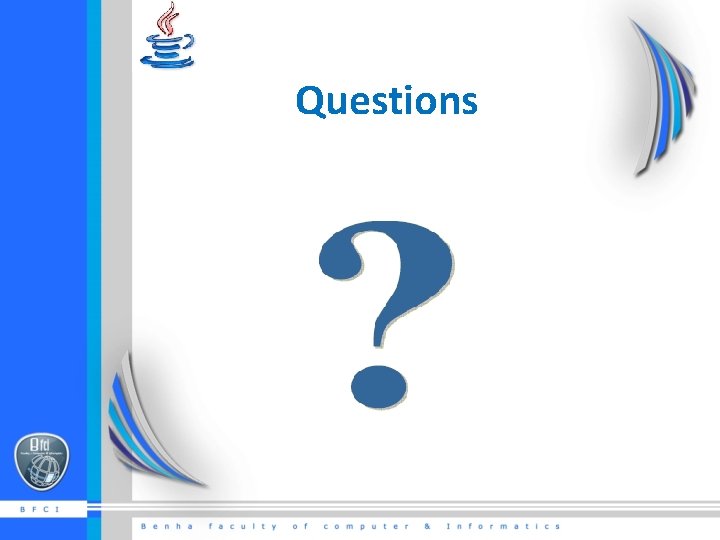
Questions
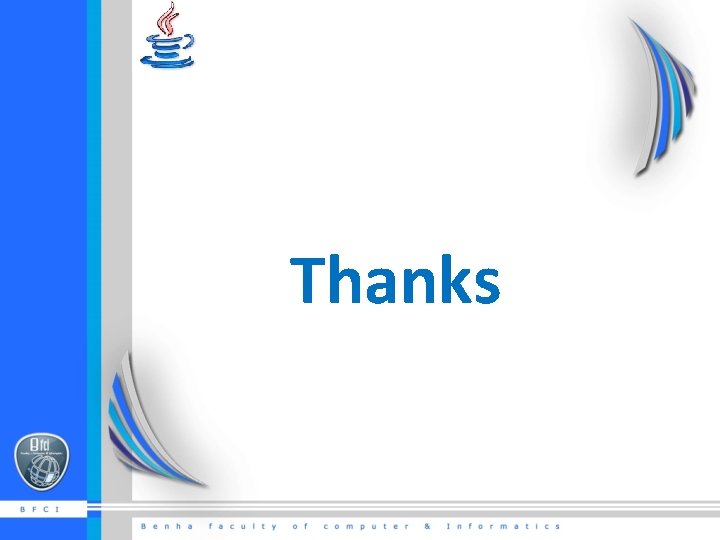
Thanks