Chapter 6 Polymorphism What is polymorphism Polymorphism means
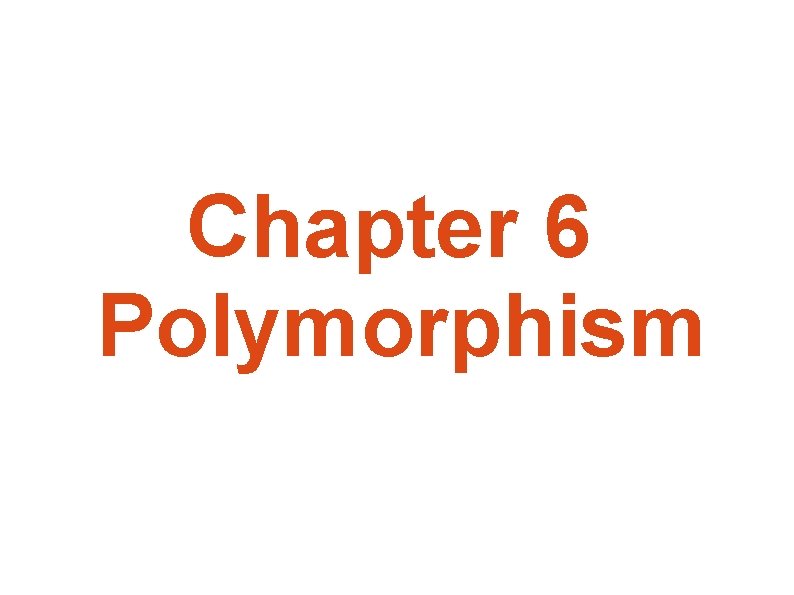
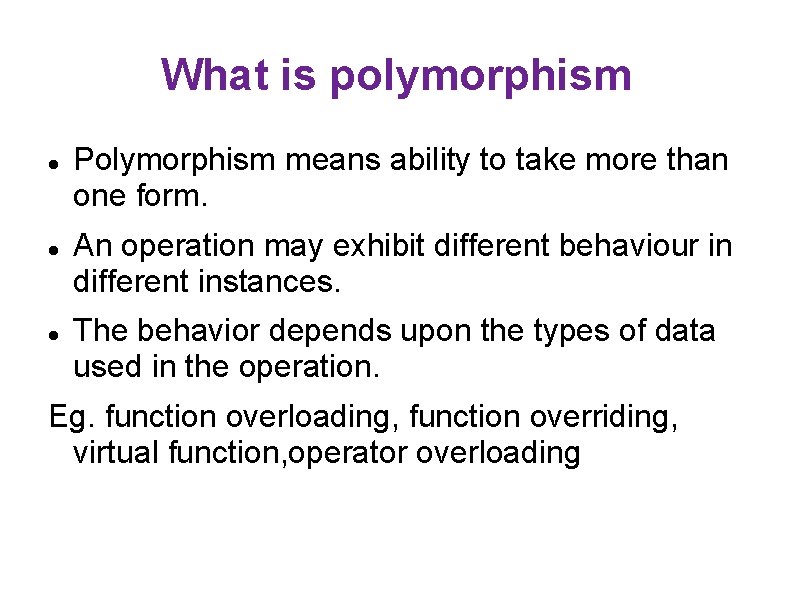
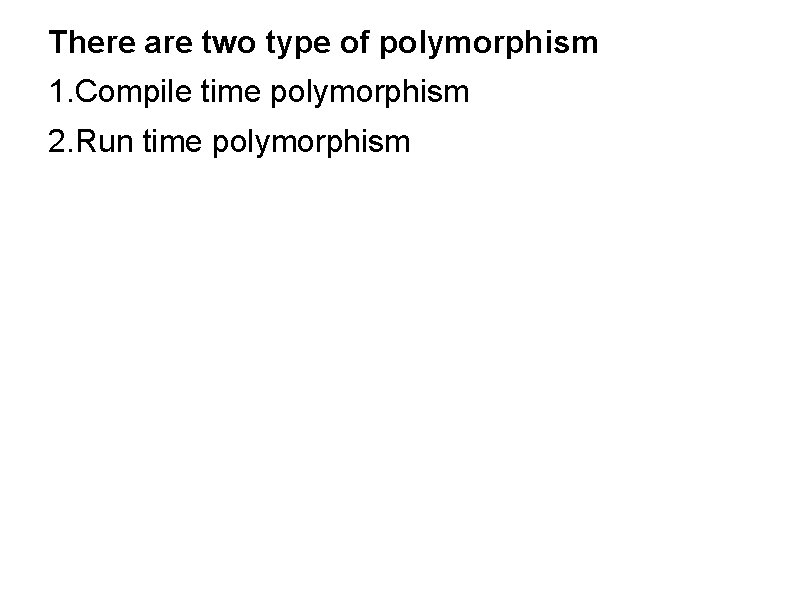
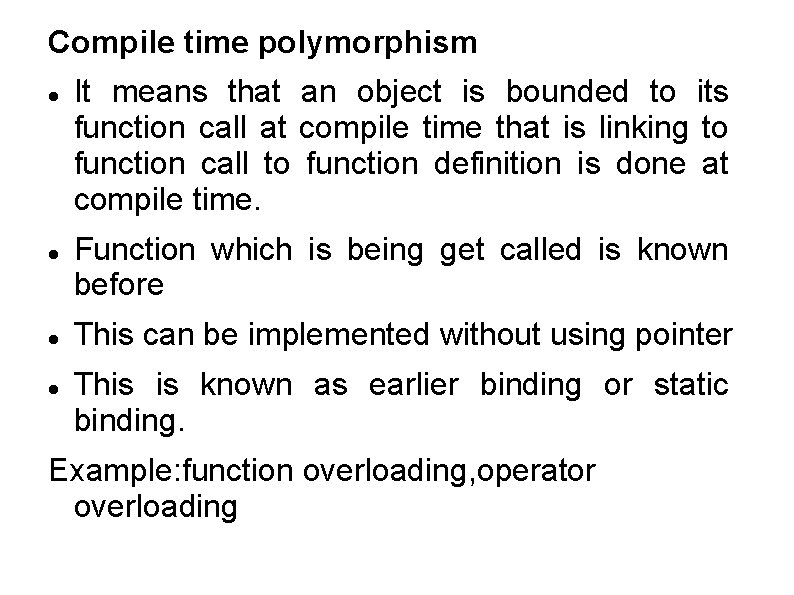
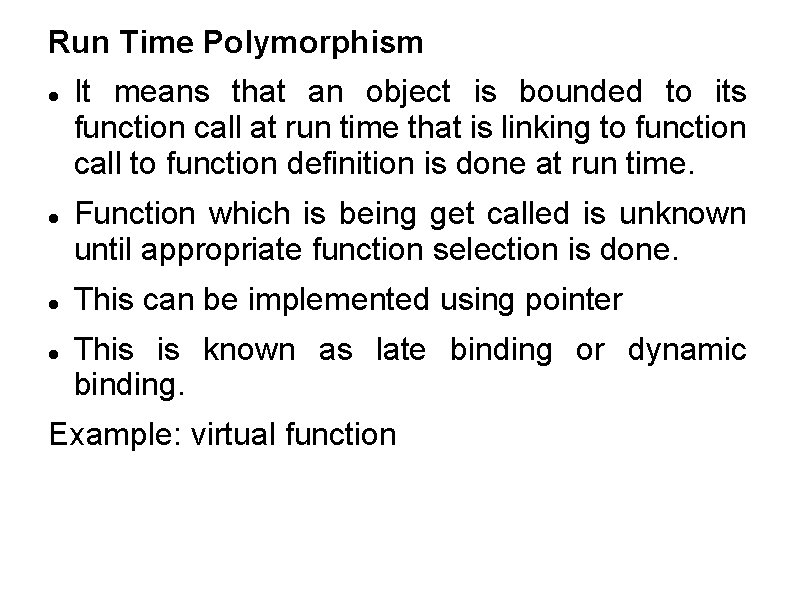
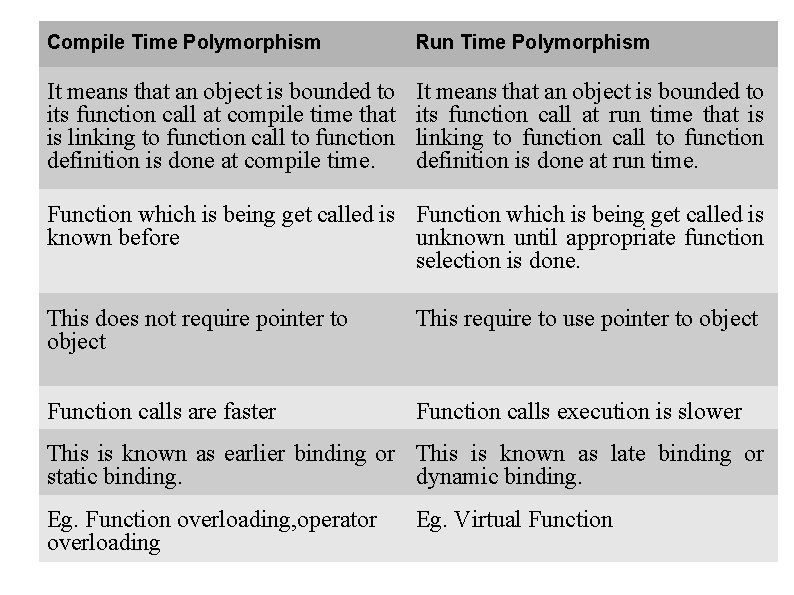
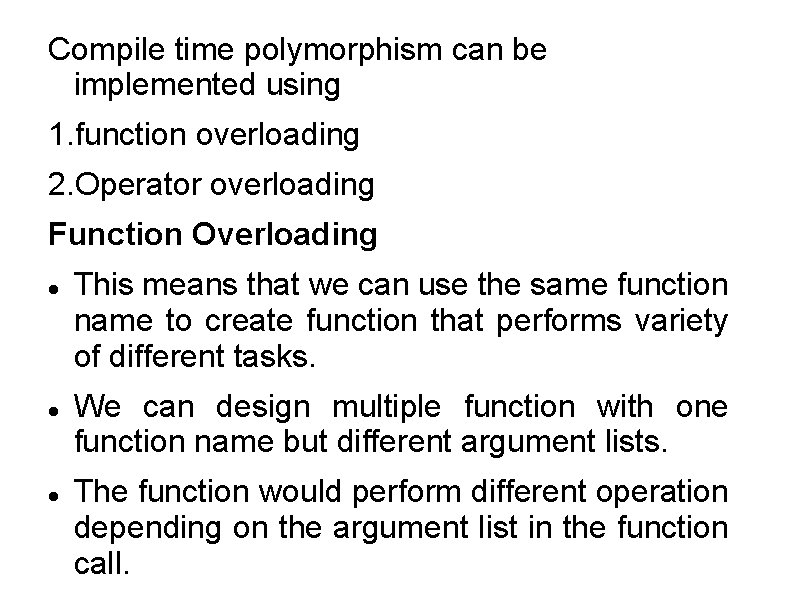
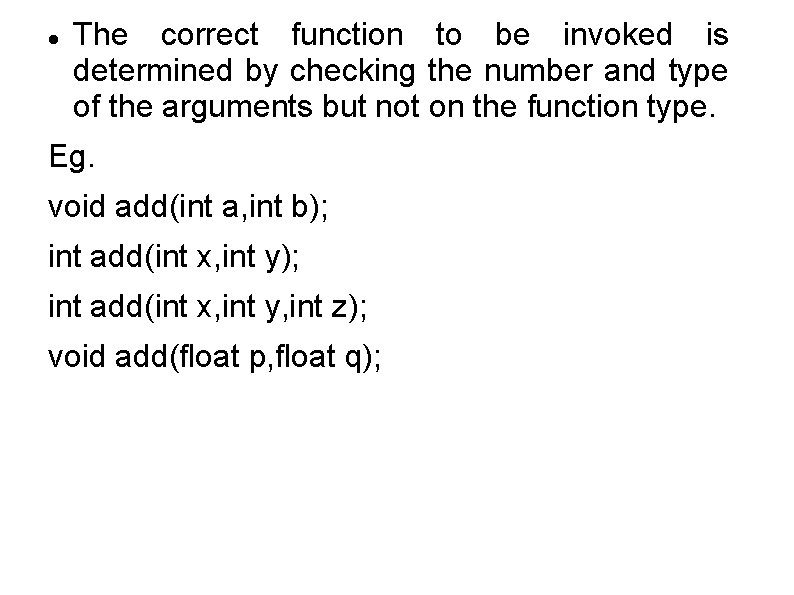
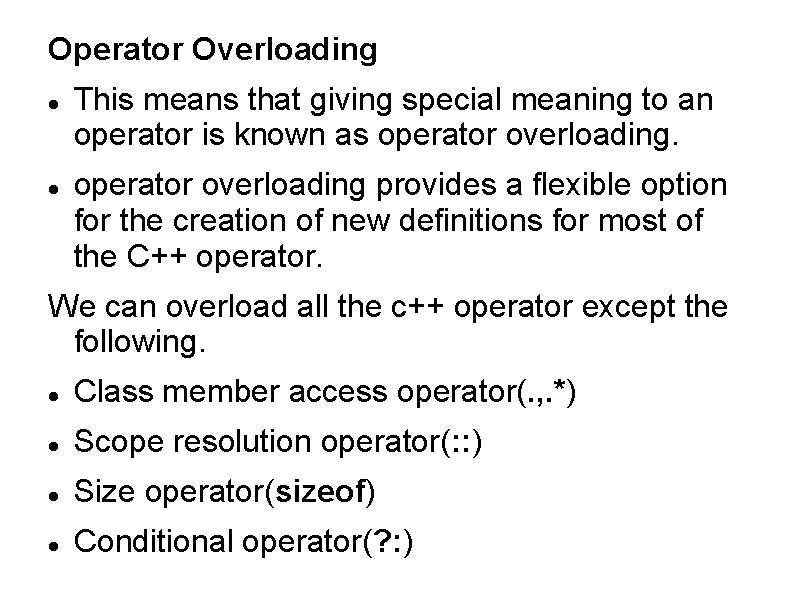
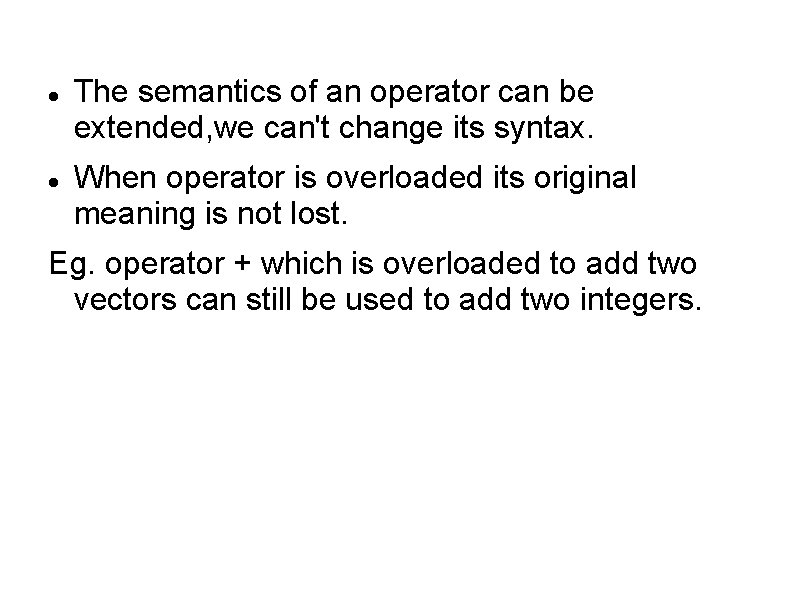
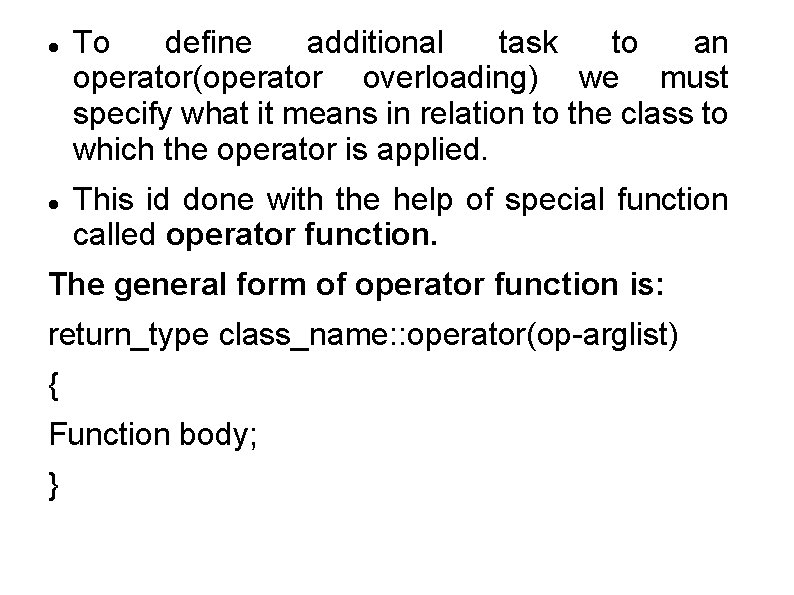
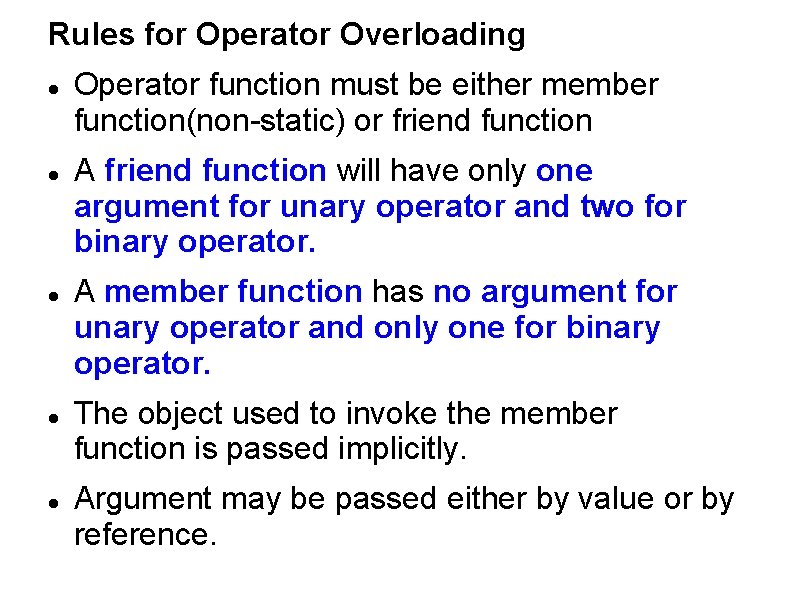
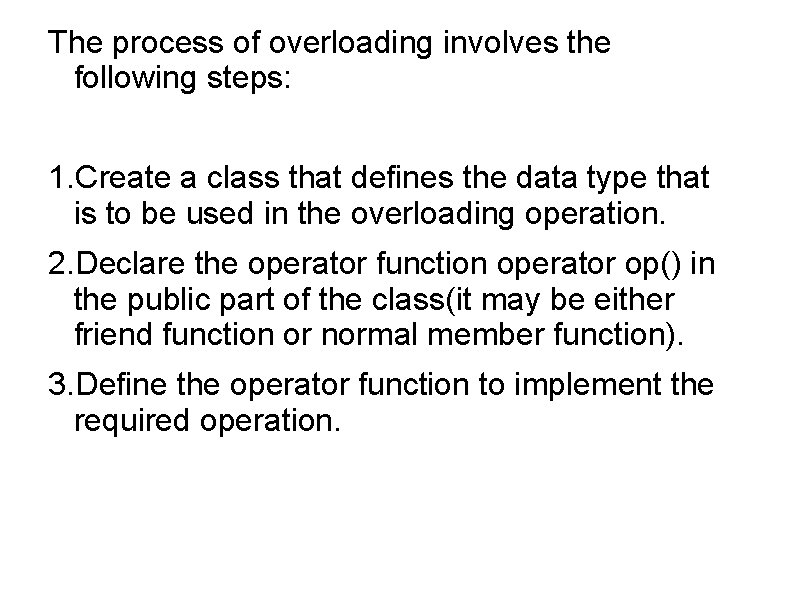
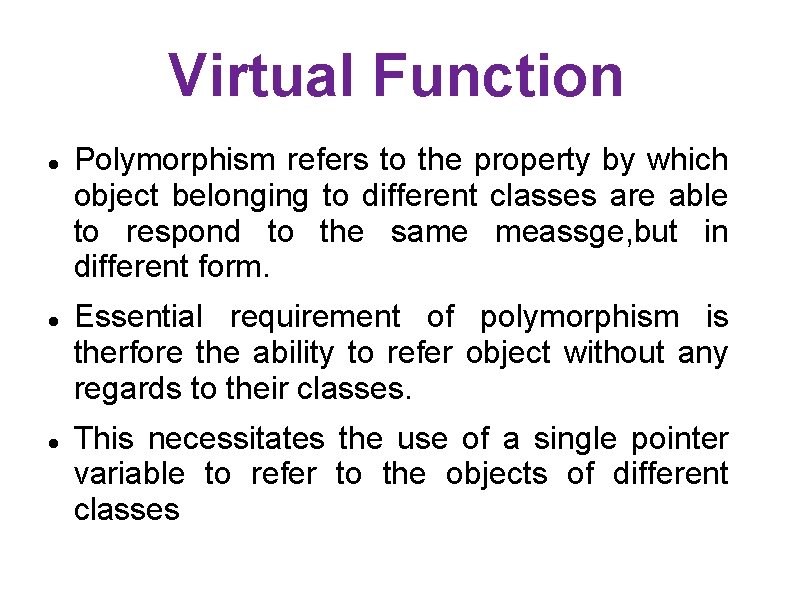
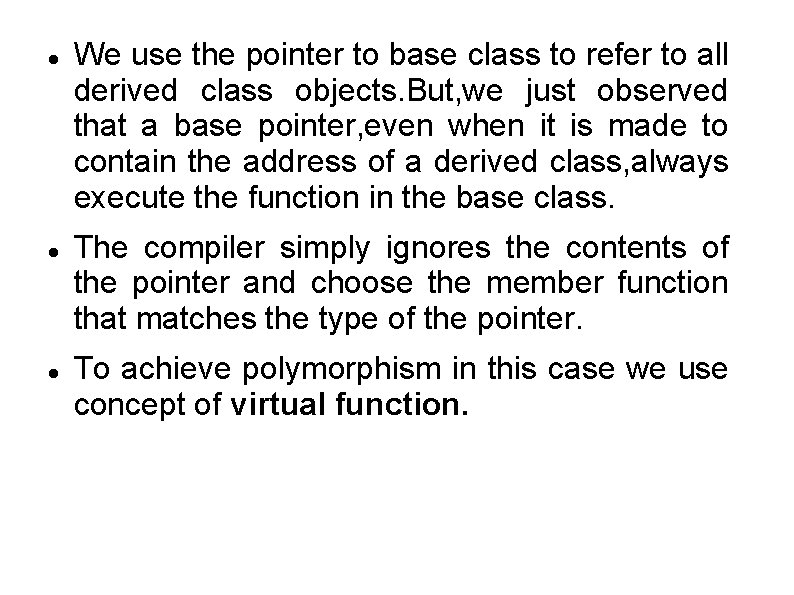
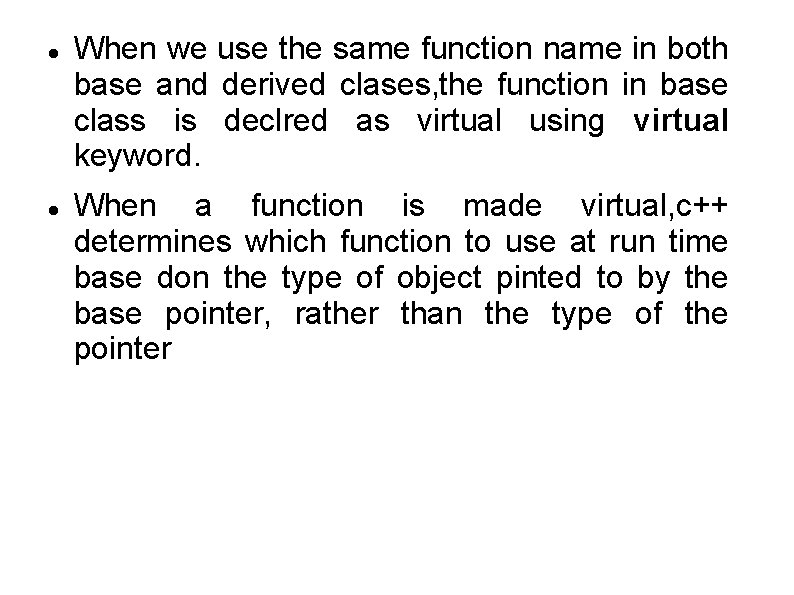
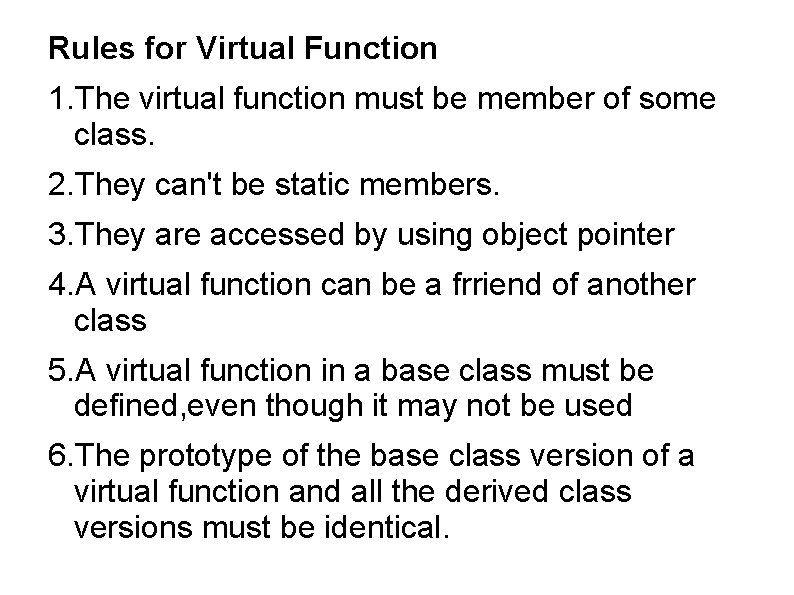
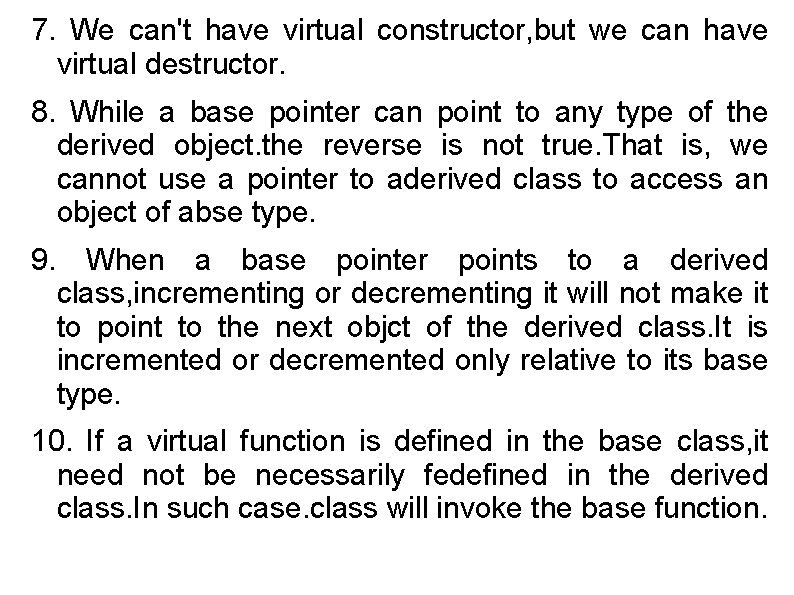
- Slides: 18
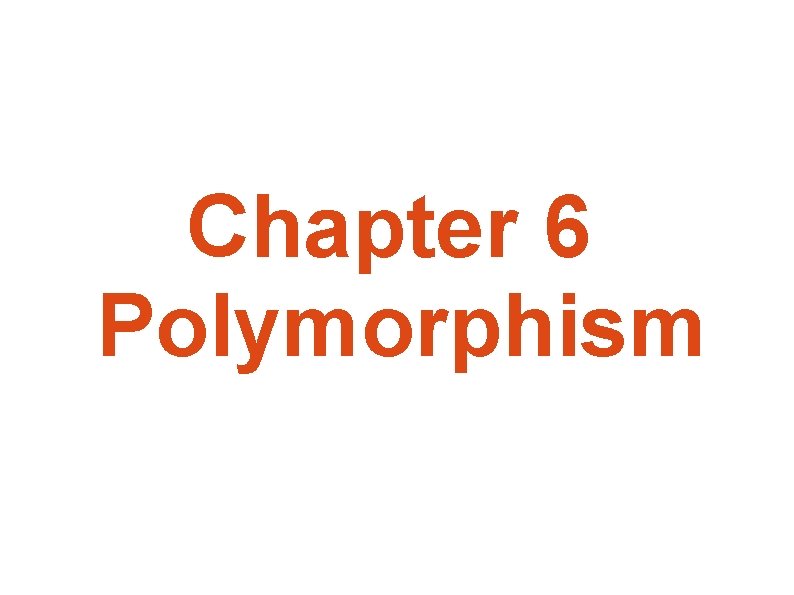
Chapter 6 Polymorphism
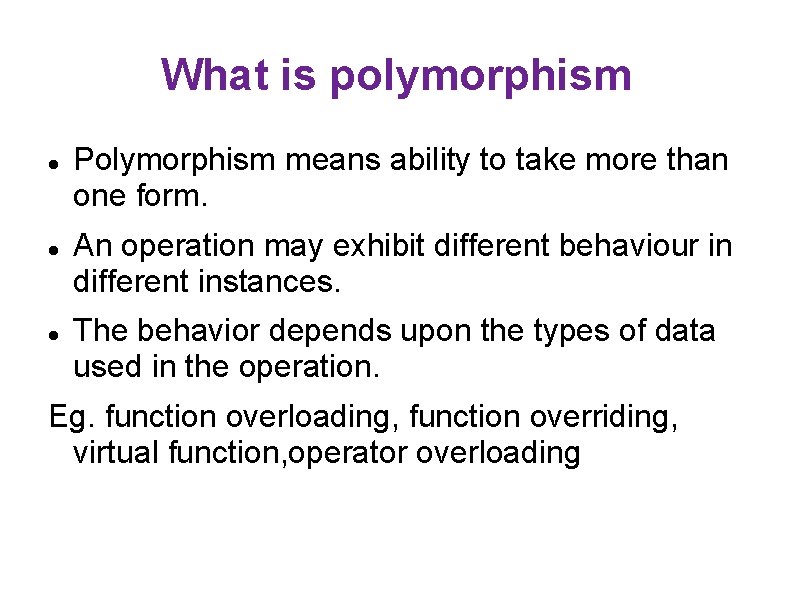
What is polymorphism Polymorphism means ability to take more than one form. An operation may exhibit different behaviour in different instances. The behavior depends upon the types of data used in the operation. Eg. function overloading, function overriding, virtual function, operator overloading
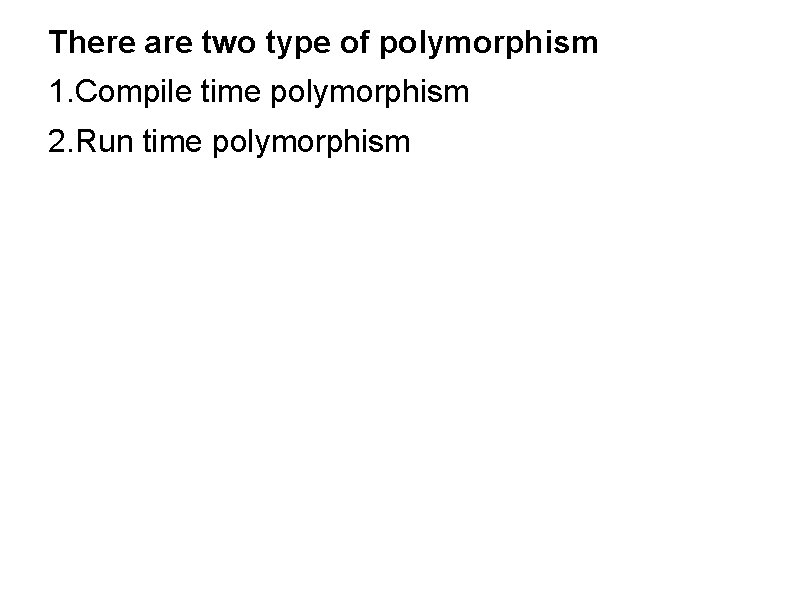
There are two type of polymorphism 1. Compile time polymorphism 2. Run time polymorphism
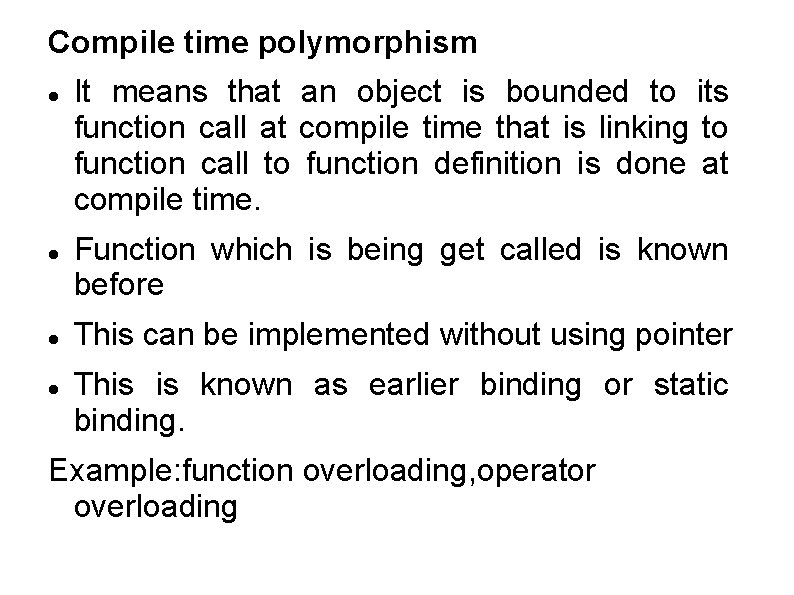
Compile time polymorphism It means that an object is bounded to its function call at compile time that is linking to function call to function definition is done at compile time. Function which is being get called is known before This can be implemented without using pointer This is known as earlier binding or static binding. Example: function overloading, operator overloading
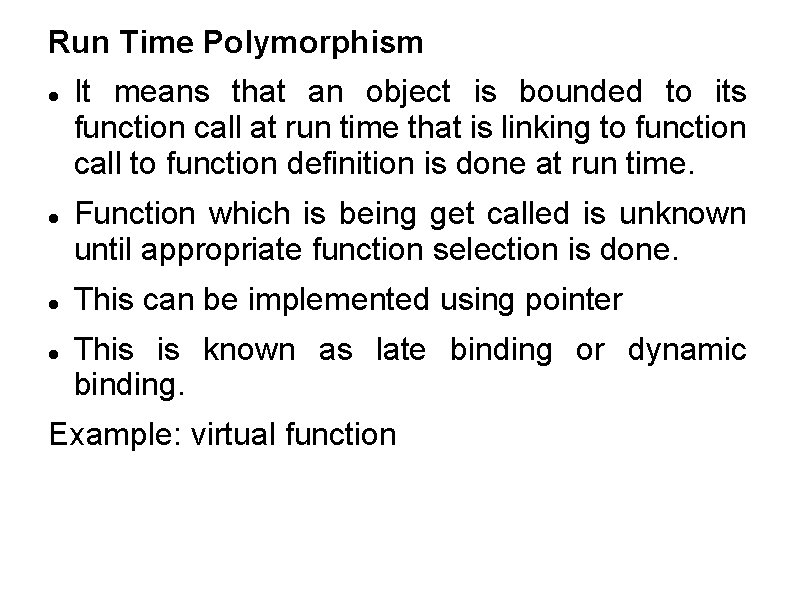
Run Time Polymorphism It means that an object is bounded to its function call at run time that is linking to function call to function definition is done at run time. Function which is being get called is unknown until appropriate function selection is done. This can be implemented using pointer This is known as late binding or dynamic binding. Example: virtual function
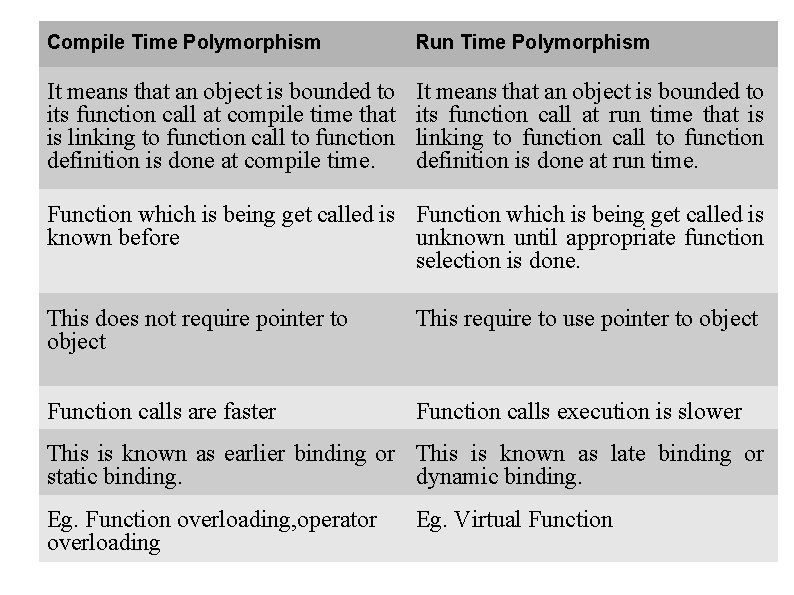
Compile Time Polymorphism Run Time Polymorphism It means that an object is bounded to its function call at compile time that is linking to function call to function definition is done at compile time. It means that an object is bounded to its function call at run time that is linking to function call to function definition is done at run time. Function which is being get called is known before unknown until appropriate function selection is done. This does not require pointer to object This require to use pointer to object Function calls are faster Function calls execution is slower This is known as earlier binding or This is known as late binding or static binding. dynamic binding. Eg. Function overloading, operator overloading Eg. Virtual Function
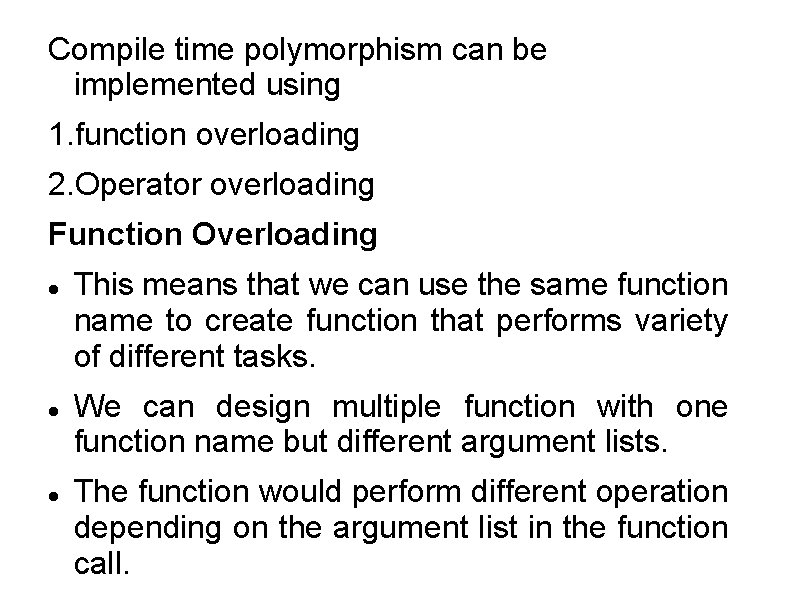
Compile time polymorphism can be implemented using 1. function overloading 2. Operator overloading Function Overloading This means that we can use the same function name to create function that performs variety of different tasks. We can design multiple function with one function name but different argument lists. The function would perform different operation depending on the argument list in the function call.
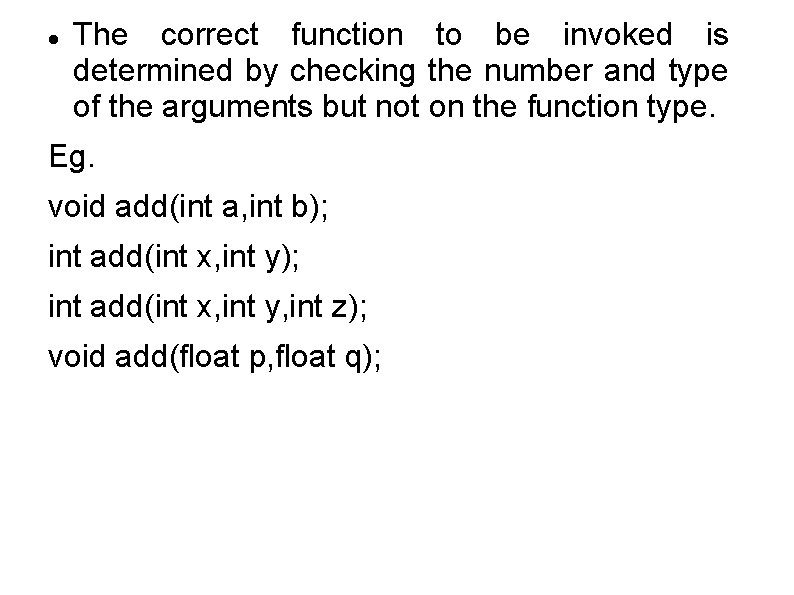
The correct function to be invoked is determined by checking the number and type of the arguments but not on the function type. Eg. void add(int a, int b); int add(int x, int y, int z); void add(float p, float q);
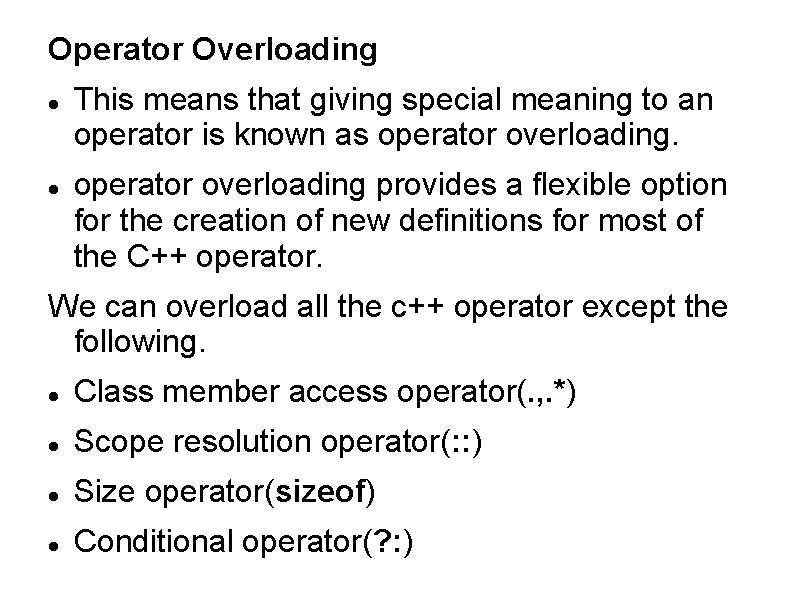
Operator Overloading This means that giving special meaning to an operator is known as operator overloading provides a flexible option for the creation of new definitions for most of the C++ operator. We can overload all the c++ operator except the following. Class member access operator(. , . *) Scope resolution operator(: : ) Size operator(sizeof) Conditional operator(? : )
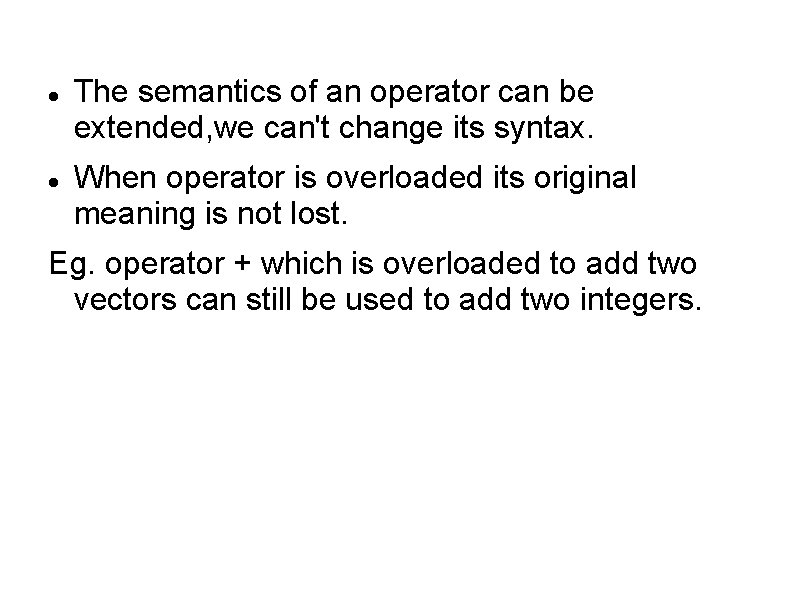
The semantics of an operator can be extended, we can't change its syntax. When operator is overloaded its original meaning is not lost. Eg. operator + which is overloaded to add two vectors can still be used to add two integers.
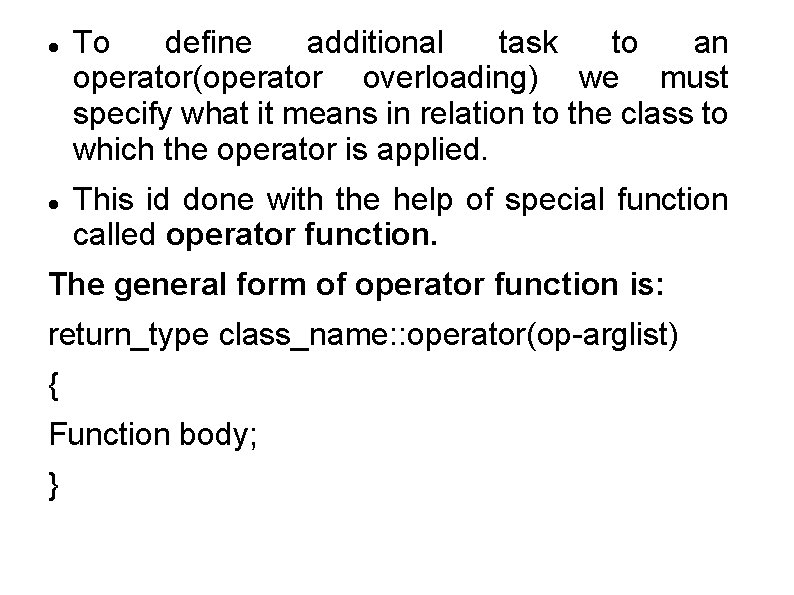
To define additional task to an operator(operator overloading) we must specify what it means in relation to the class to which the operator is applied. This id done with the help of special function called operator function. The general form of operator function is: return_type class_name: : operator(op-arglist) { Function body; }
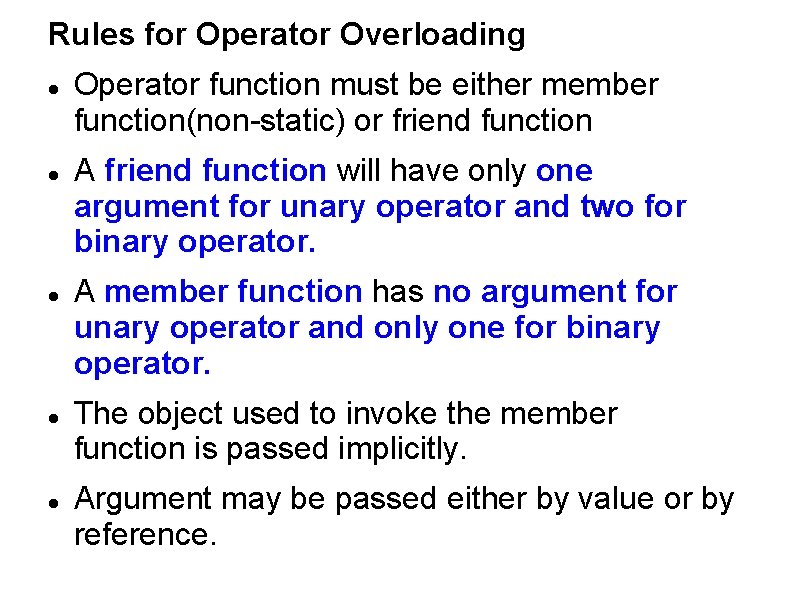
Rules for Operator Overloading Operator function must be either member function(non-static) or friend function A friend function will have only one argument for unary operator and two for binary operator. A member function has no argument for unary operator and only one for binary operator. The object used to invoke the member function is passed implicitly. Argument may be passed either by value or by reference.
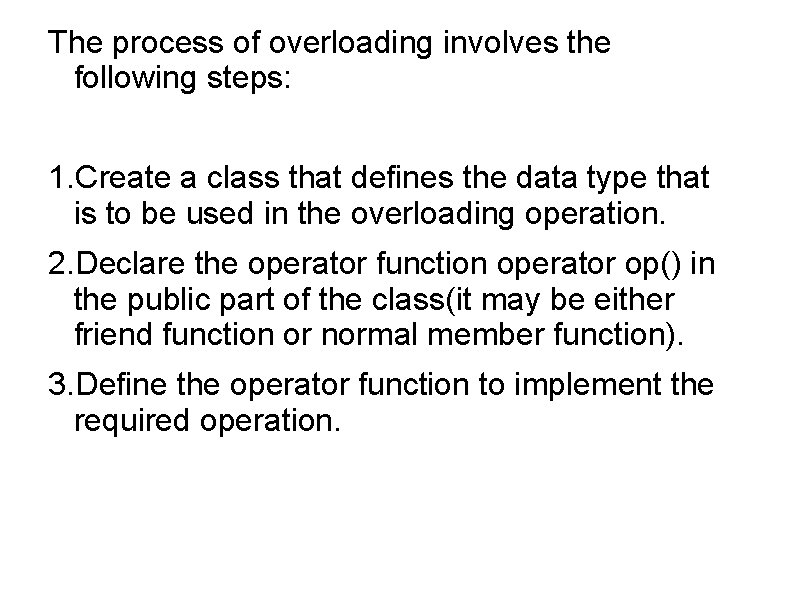
The process of overloading involves the following steps: 1. Create a class that defines the data type that is to be used in the overloading operation. 2. Declare the operator function operator op() in the public part of the class(it may be either friend function or normal member function). 3. Define the operator function to implement the required operation.
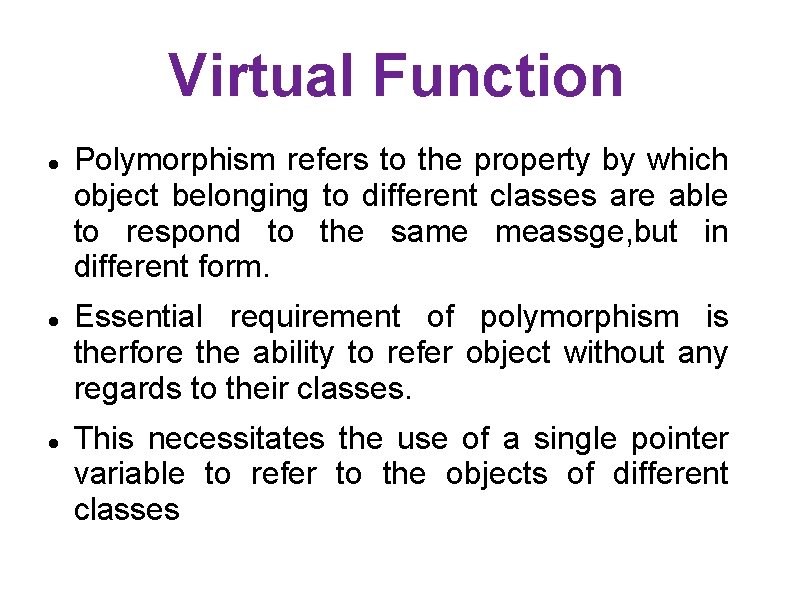
Virtual Function Polymorphism refers to the property by which object belonging to different classes are able to respond to the same meassge, but in different form. Essential requirement of polymorphism is therfore the ability to refer object without any regards to their classes. This necessitates the use of a single pointer variable to refer to the objects of different classes
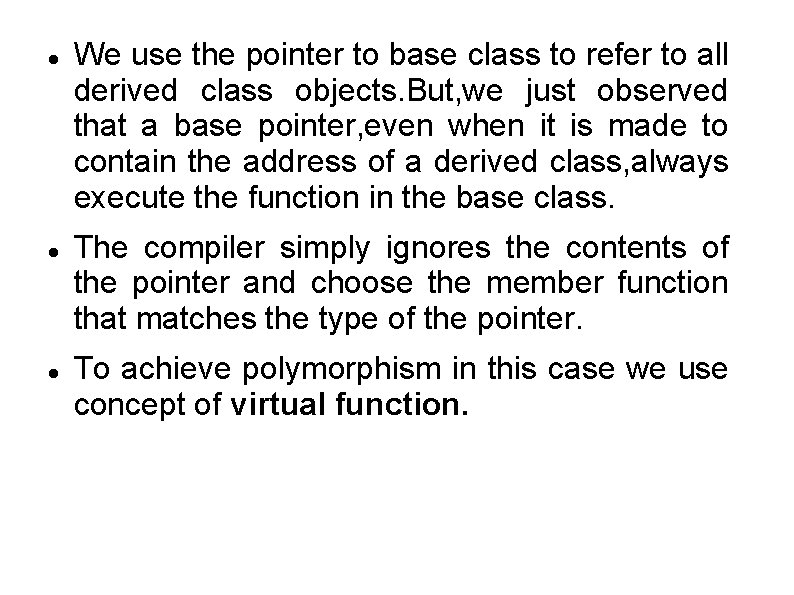
We use the pointer to base class to refer to all derived class objects. But, we just observed that a base pointer, even when it is made to contain the address of a derived class, always execute the function in the base class. The compiler simply ignores the contents of the pointer and choose the member function that matches the type of the pointer. To achieve polymorphism in this case we use concept of virtual function.
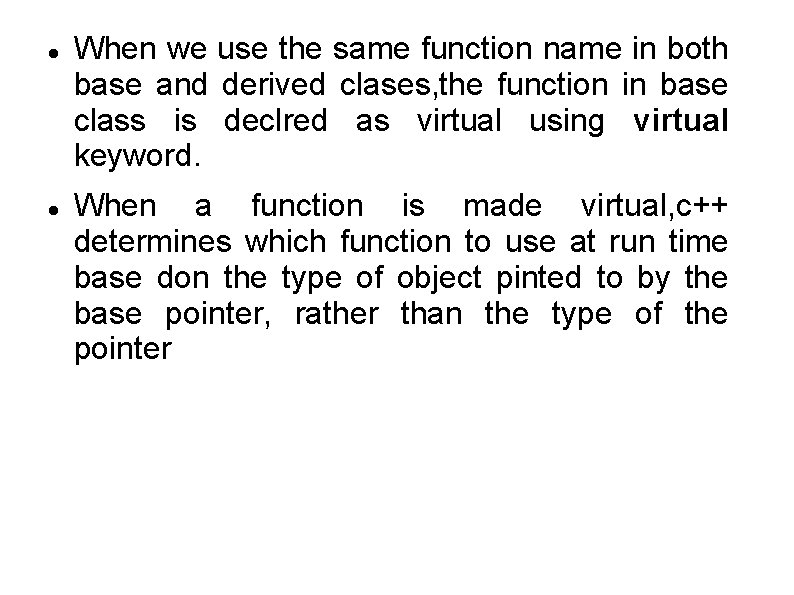
When we use the same function name in both base and derived clases, the function in base class is declred as virtual using virtual keyword. When a function is made virtual, c++ determines which function to use at run time base don the type of object pinted to by the base pointer, rather than the type of the pointer
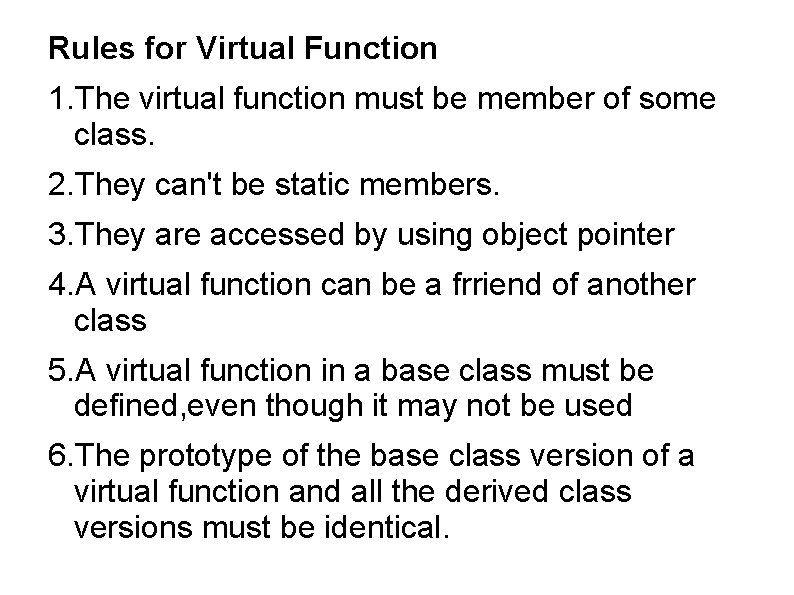
Rules for Virtual Function 1. The virtual function must be member of some class. 2. They can't be static members. 3. They are accessed by using object pointer 4. A virtual function can be a frriend of another class 5. A virtual function in a base class must be defined, even though it may not be used 6. The prototype of the base class version of a virtual function and all the derived class versions must be identical.
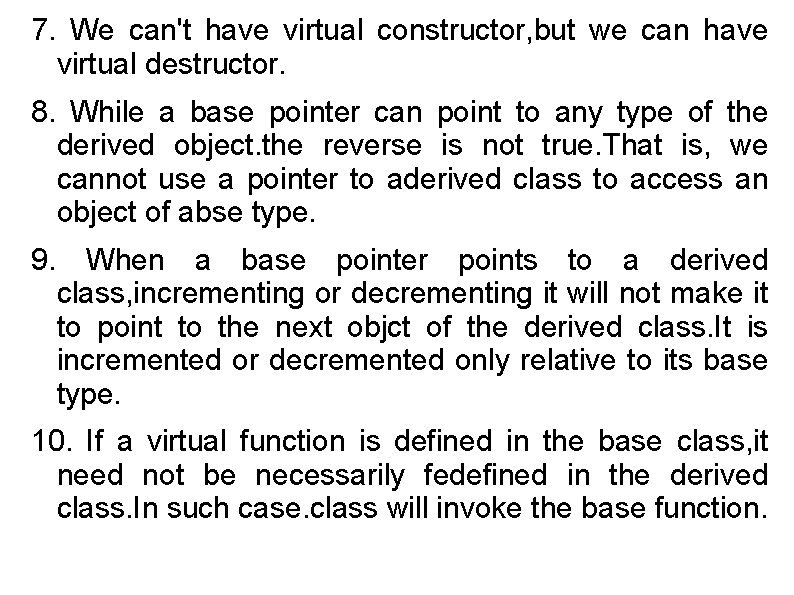
7. We can't have virtual constructor, but we can have virtual destructor. 8. While a base pointer can point to any type of the derived object. the reverse is not true. That is, we cannot use a pointer to aderived class to access an object of abse type. 9. When a base pointer points to a derived class, incrementing or decrementing it will not make it to point to the next objct of the derived class. It is incremented or decremented only relative to its base type. 10. If a virtual function is defined in the base class, it need not be necessarily fedefined in the derived class. In such case. class will invoke the base function.
If poly means many, what is gon?
Meta'' means morphe'' means
Meta means change and morph means heat
Ex situ conservation definition
Bio means life logy means
Physalia is a polymorphic colony
What is polymorphism
Ad-hoc polymorphism
Polymorphism in oops concept
Compile time polymorphism in c++
Subtype polymorphism
Polymorphism computer science
Subtype polymorphism
Difference between allotropy and polymorphism
Definition of polymorphism
Polymorphism car example
Payroll system using polymorphism in java
Oop inheritance polymorphism encapsulation abstraction
Compile time polymorphism in c++