Concordia University Department of Computer Science and Software
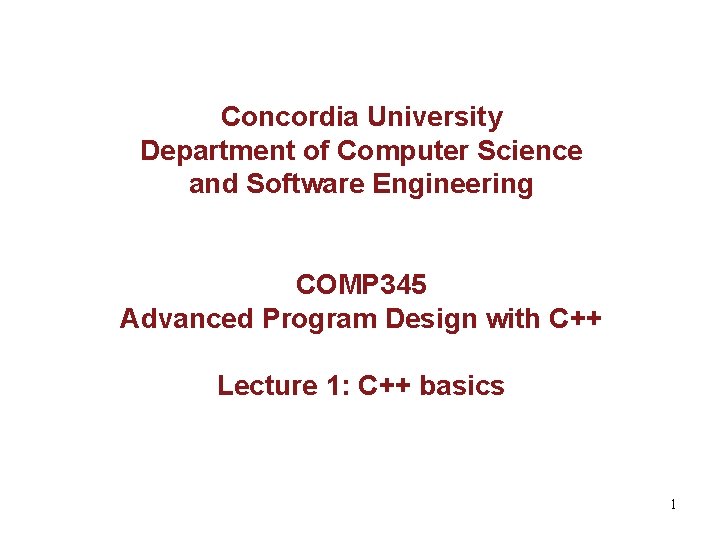
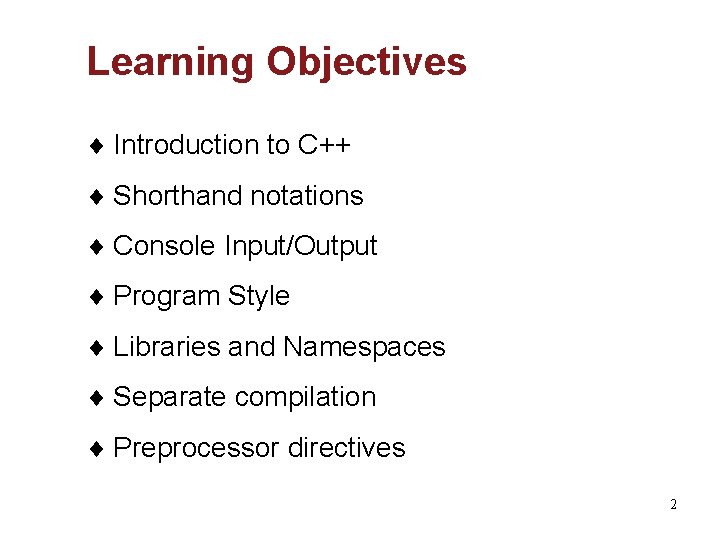
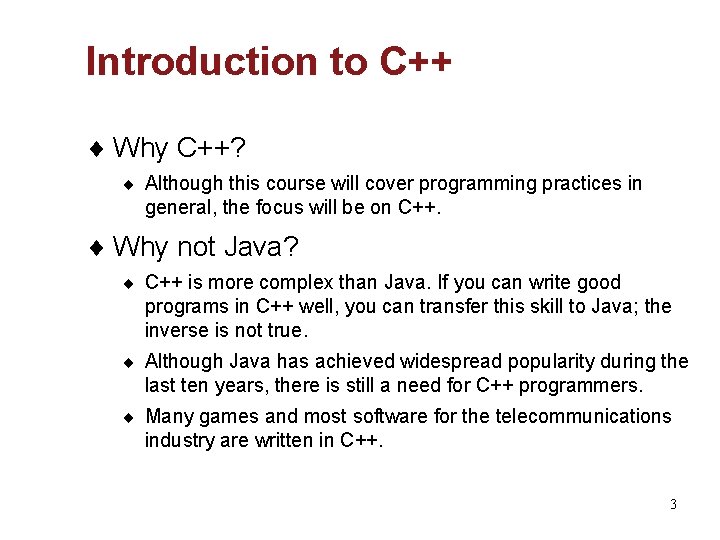
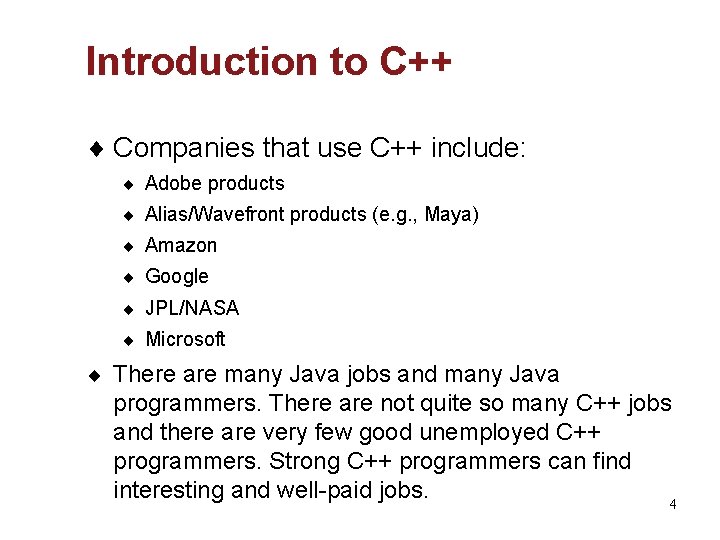
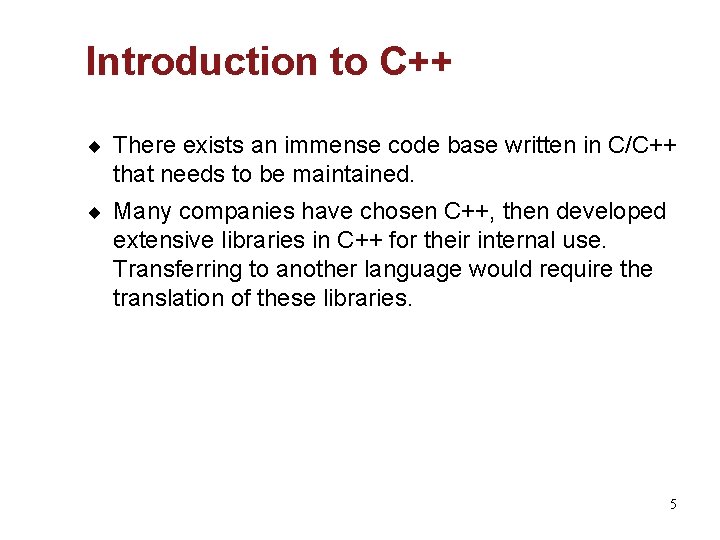
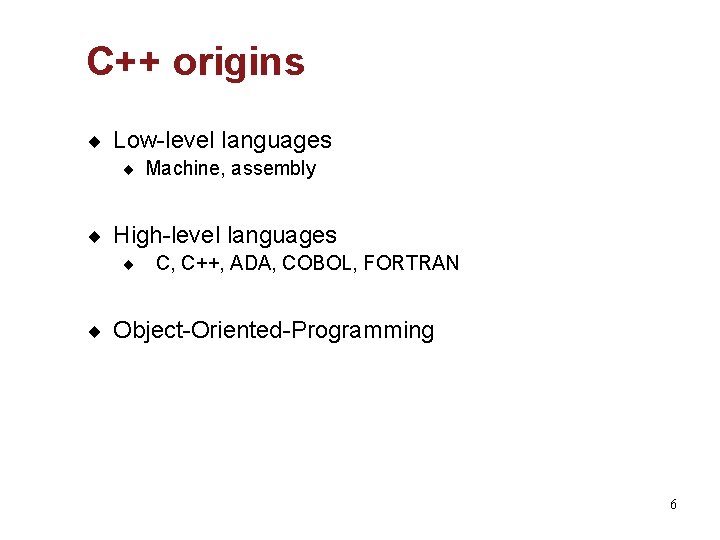
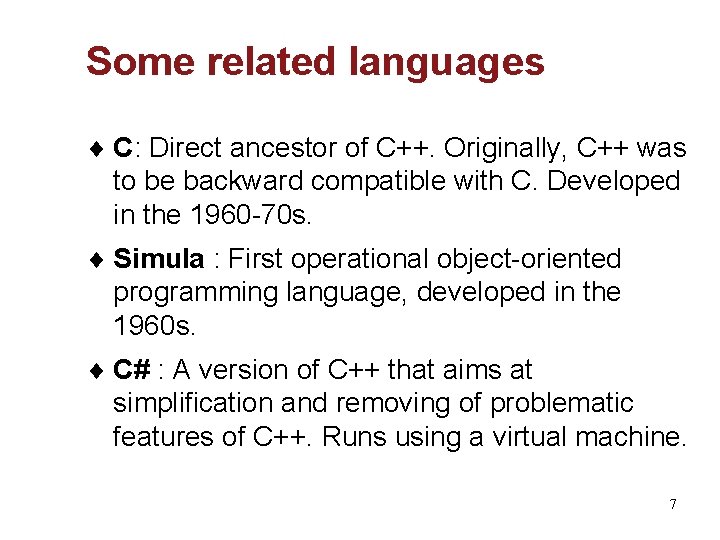
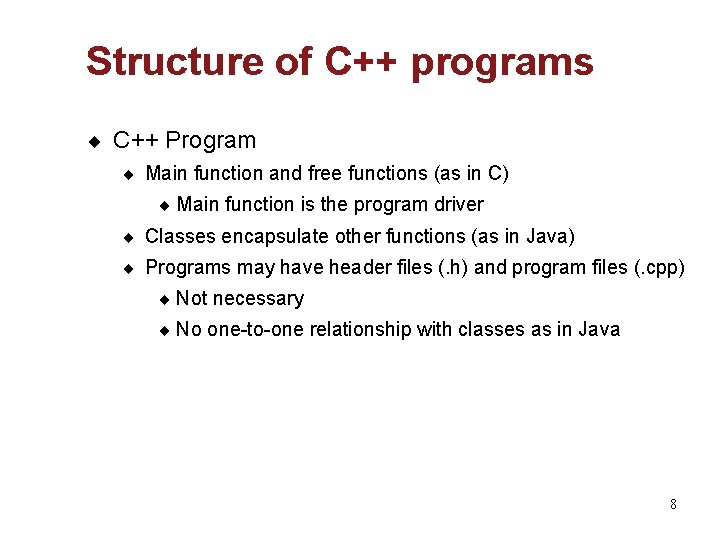
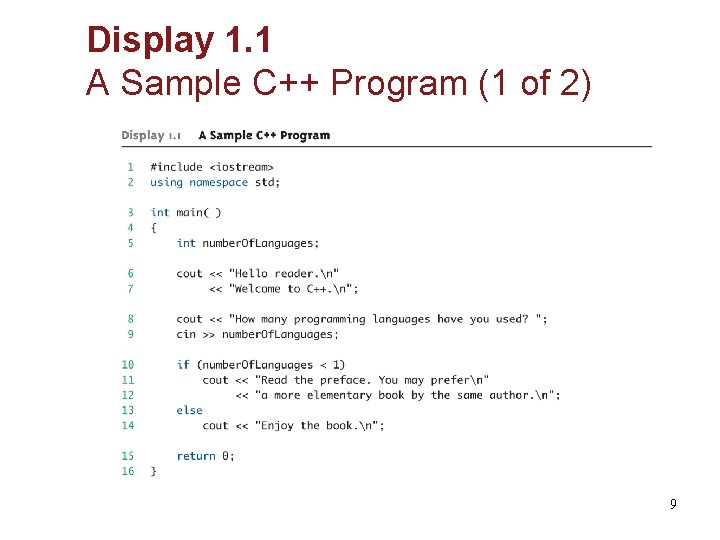
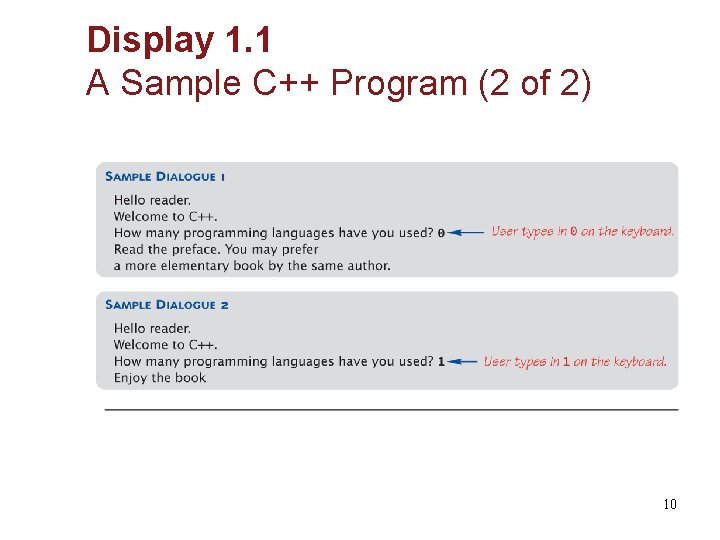
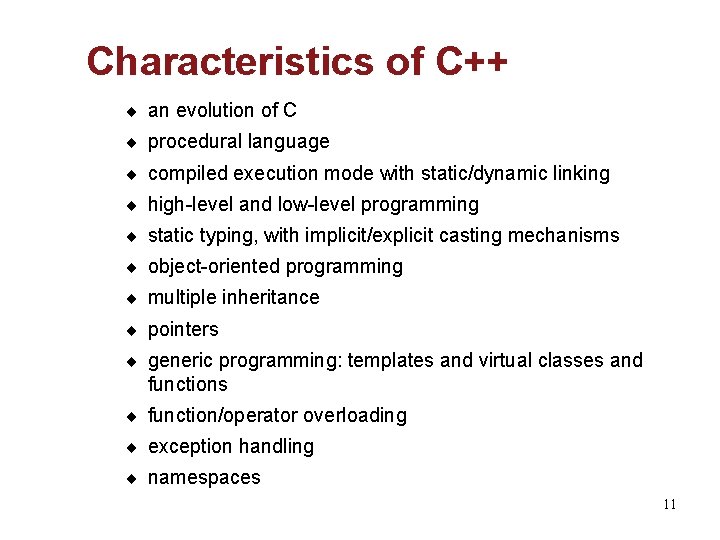
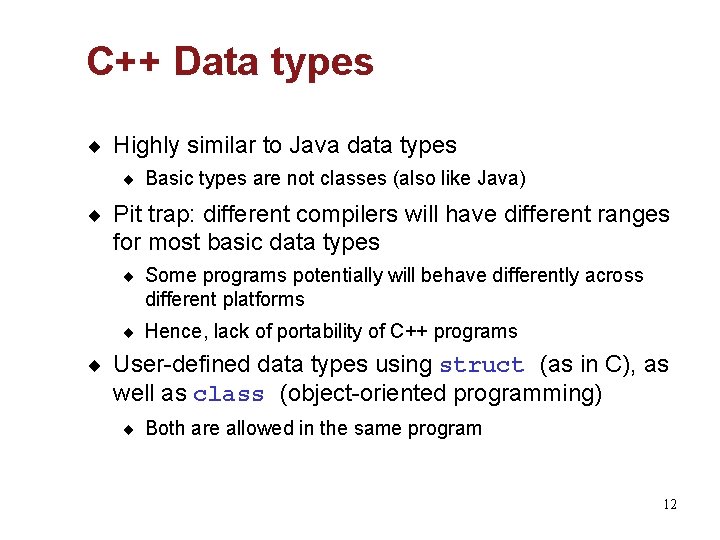
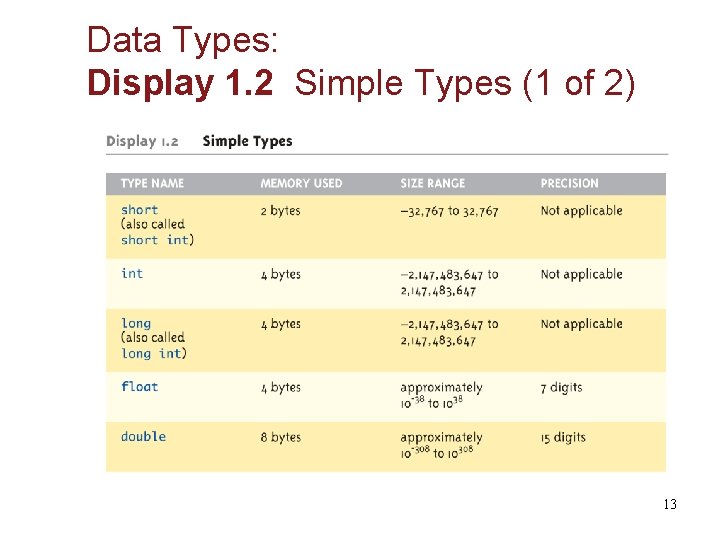
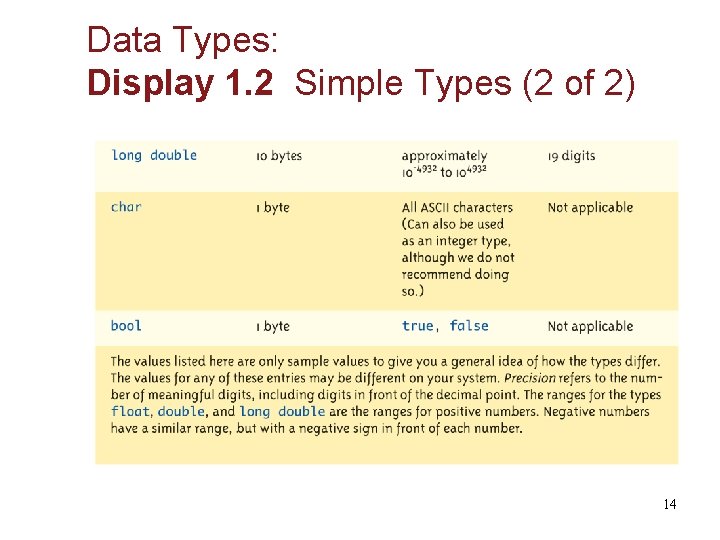
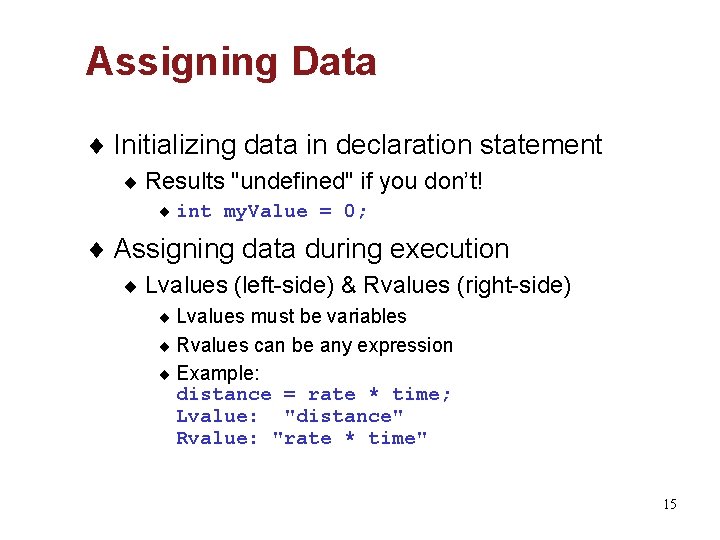
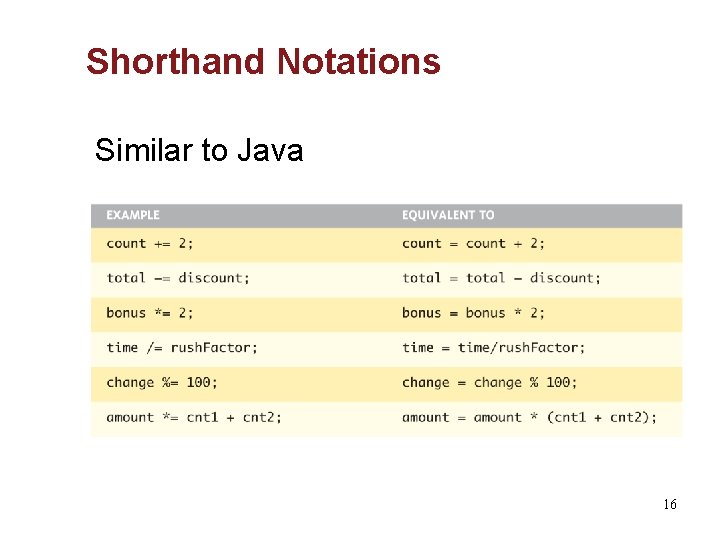
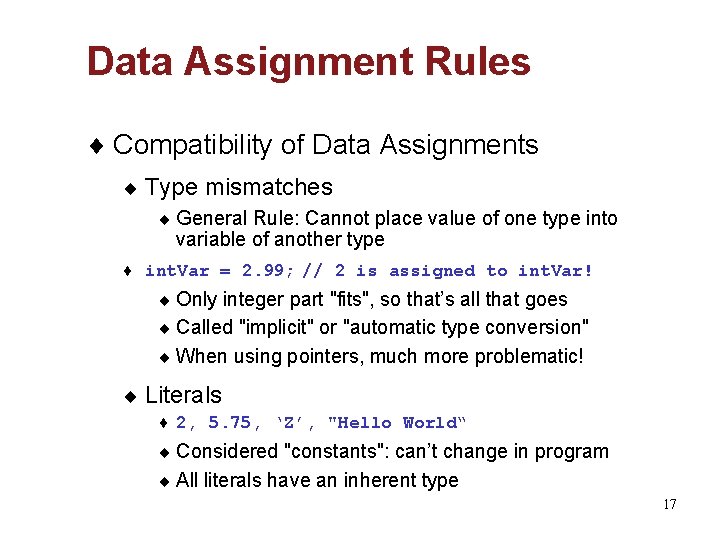
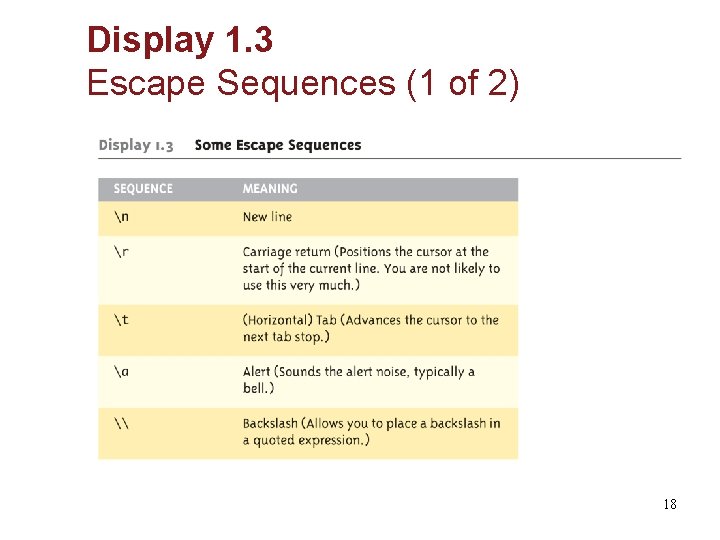
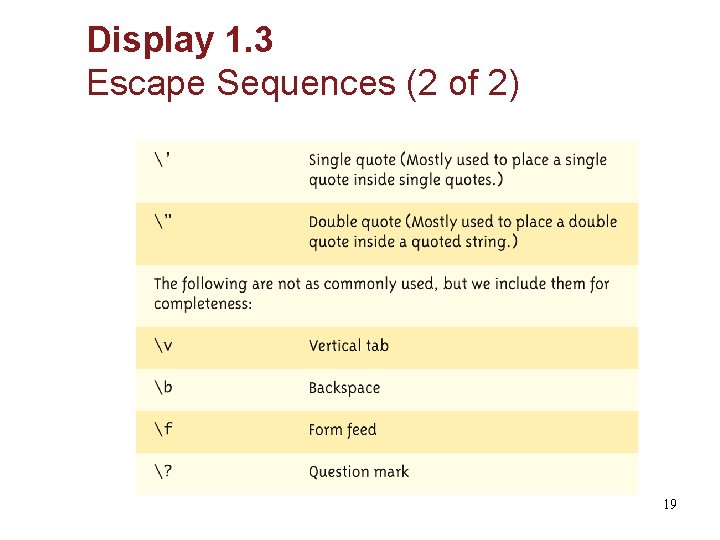
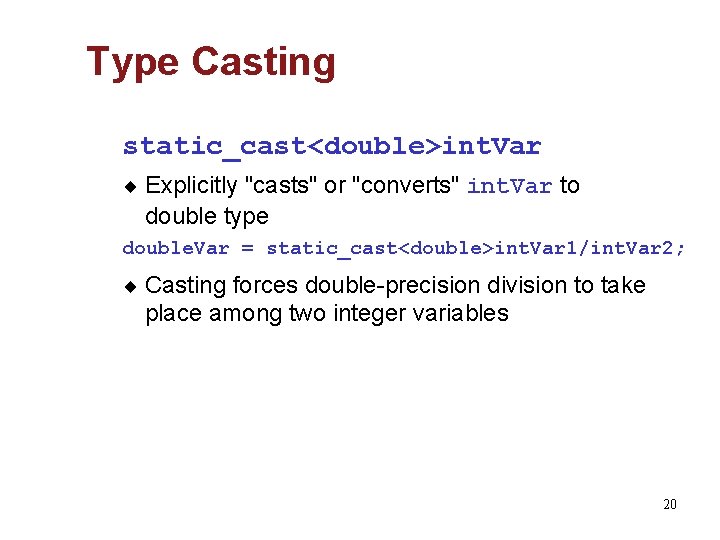
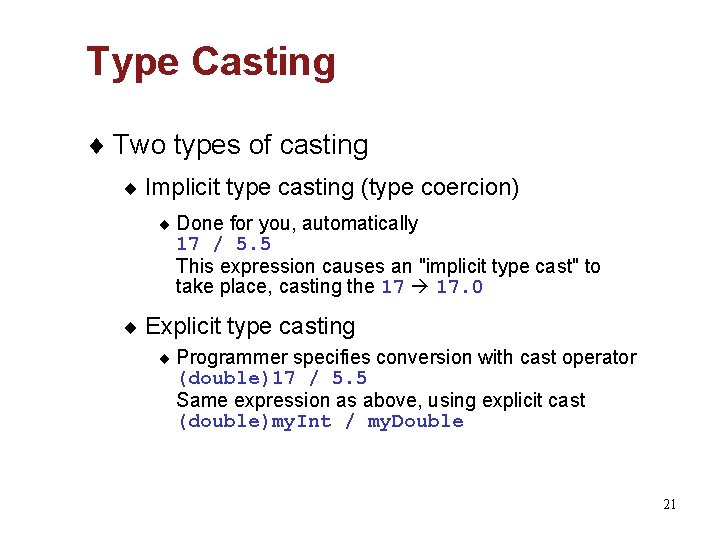
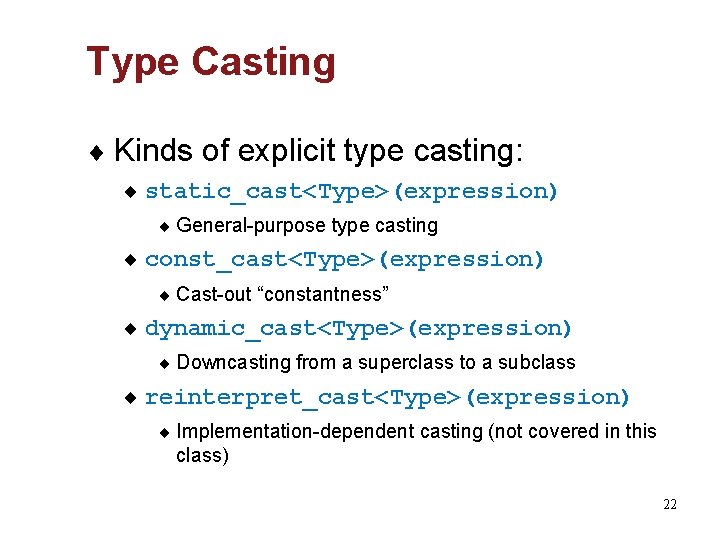
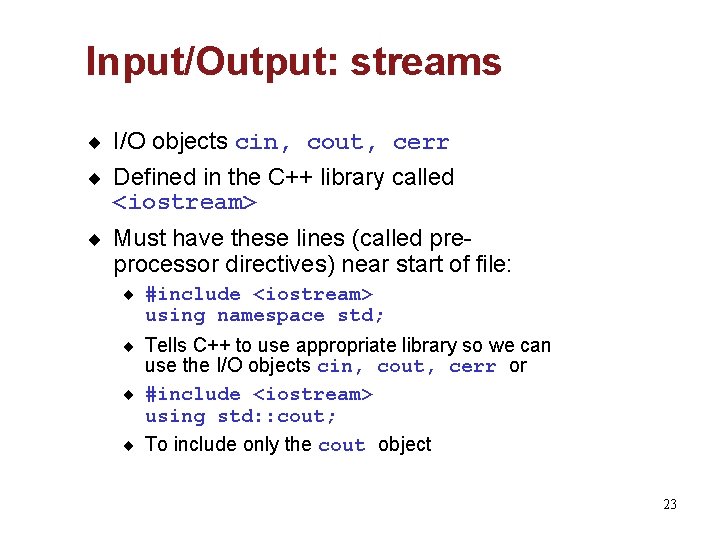
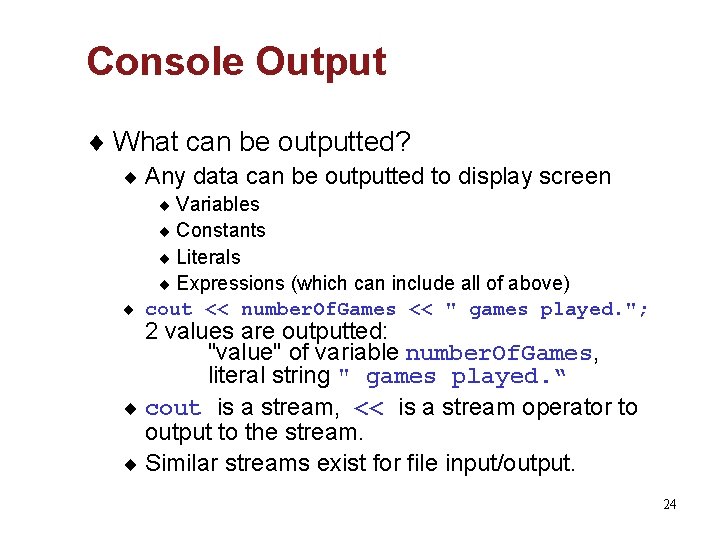
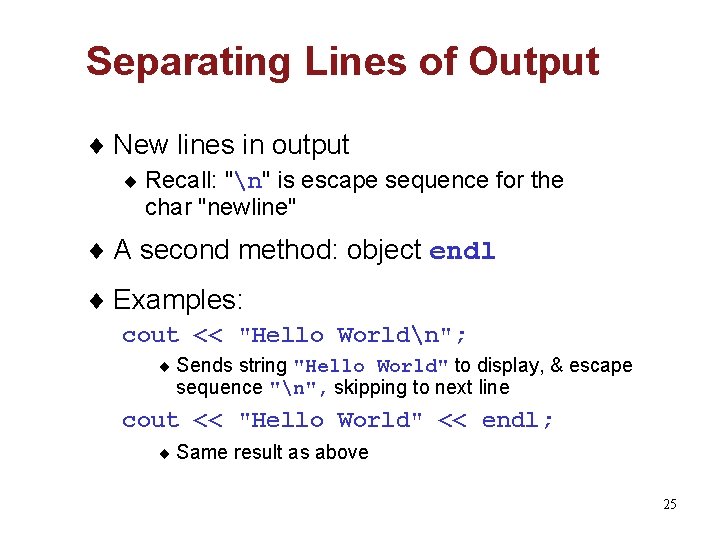
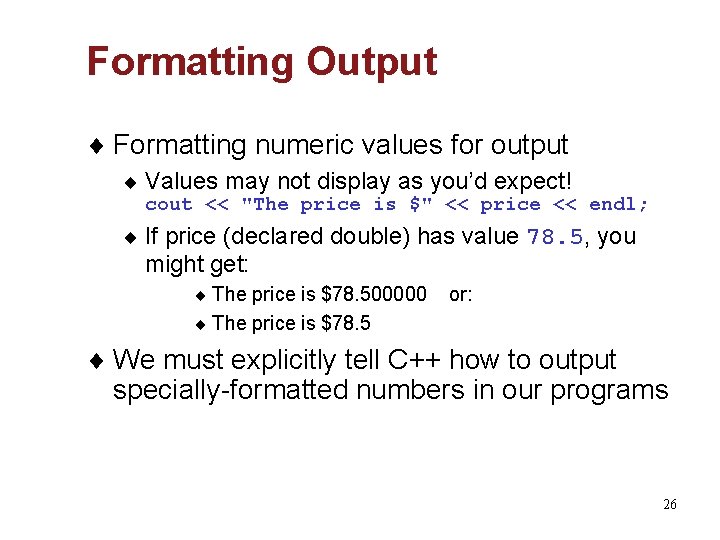
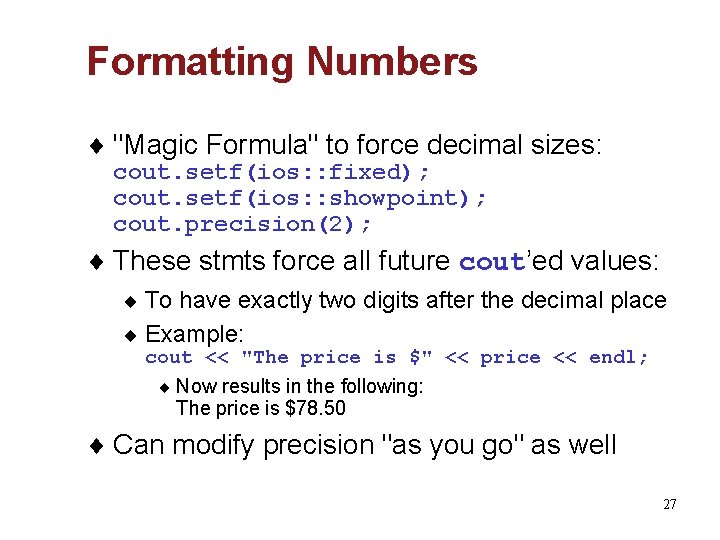
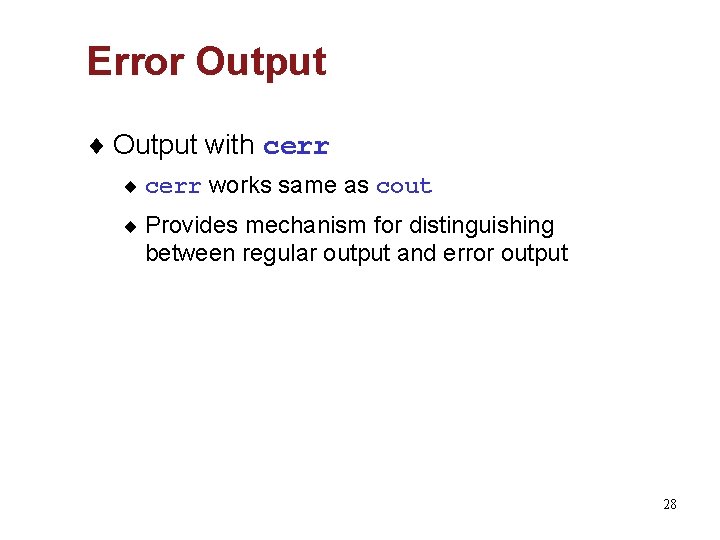
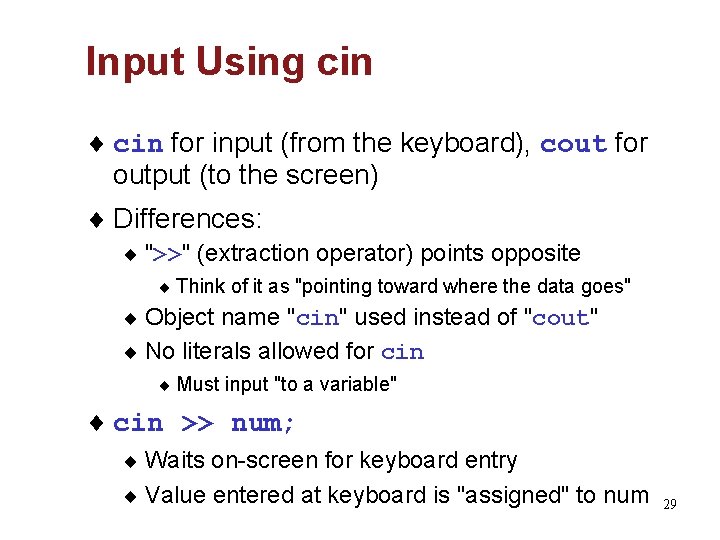
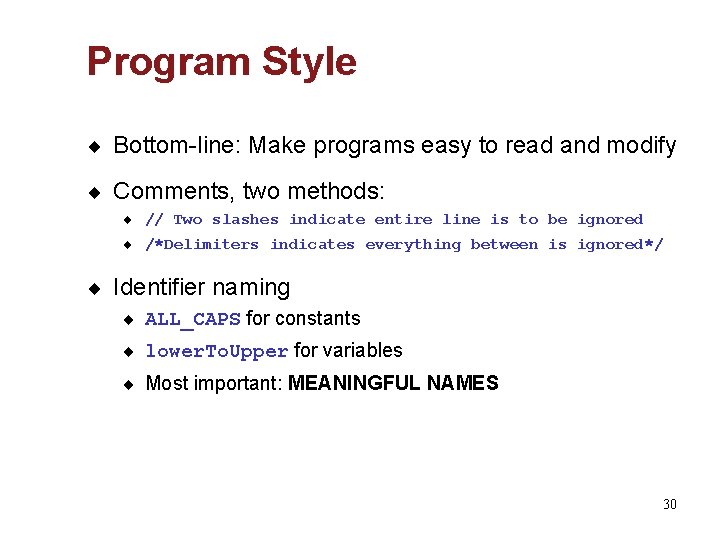
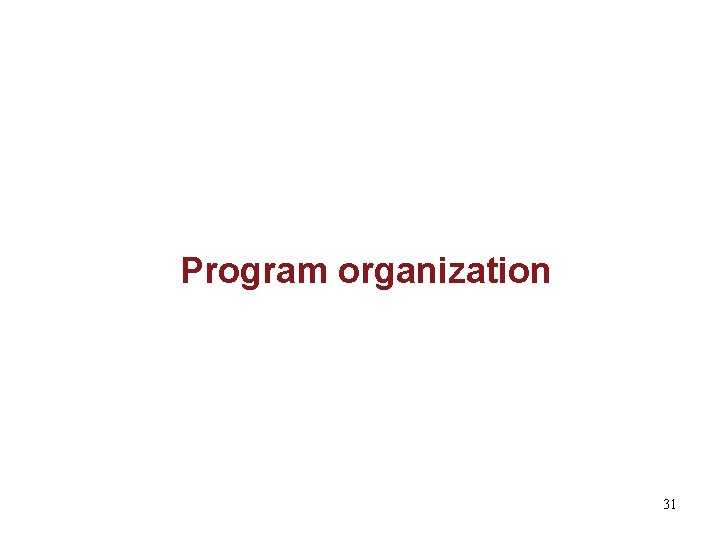
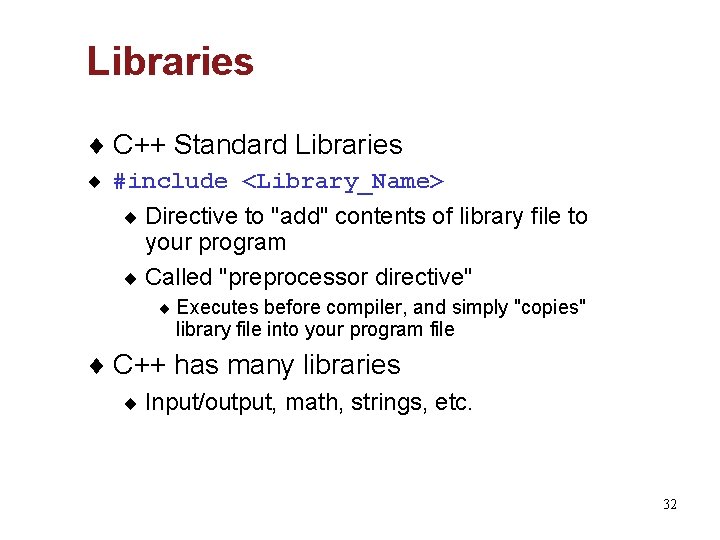
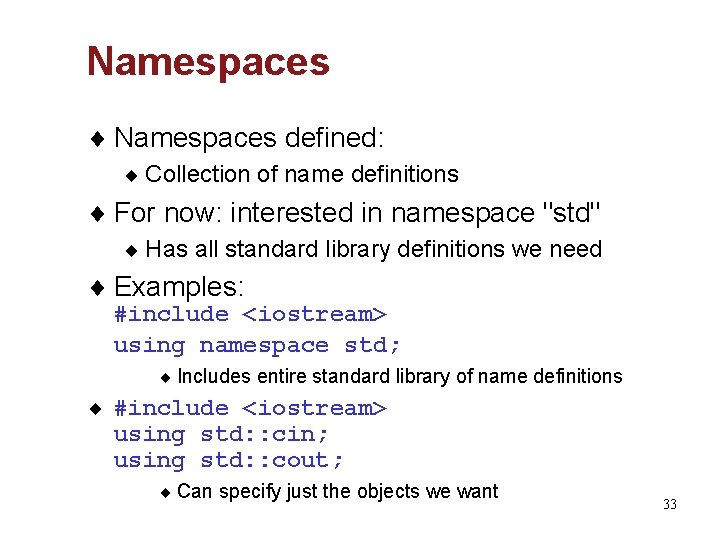
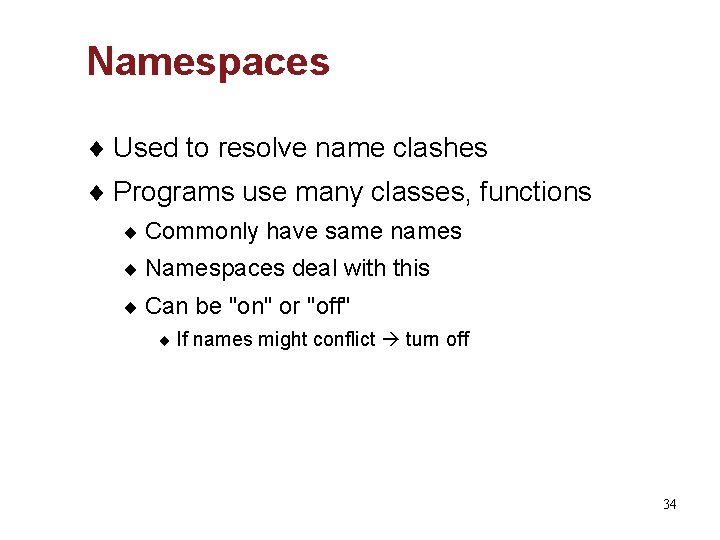
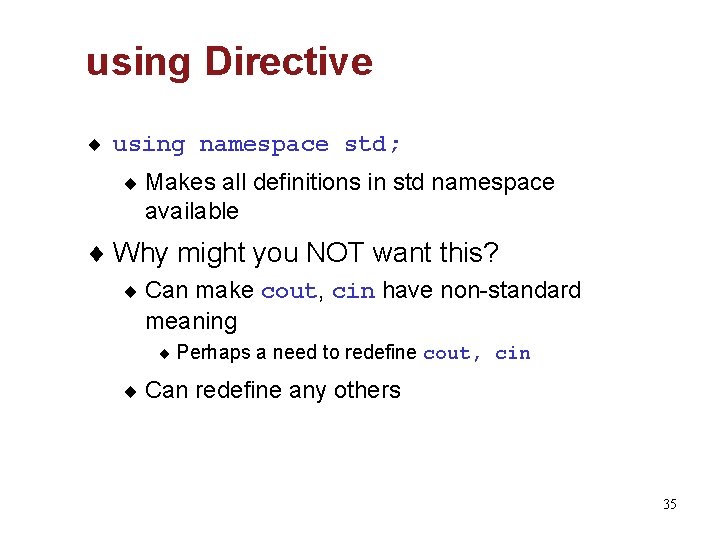
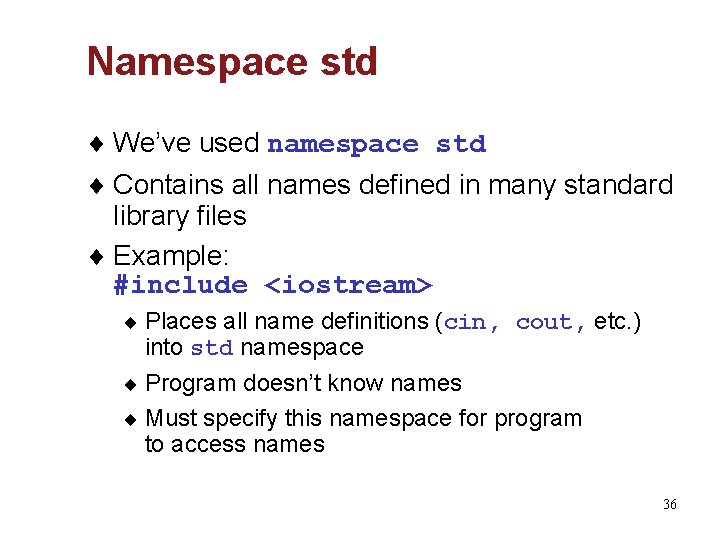
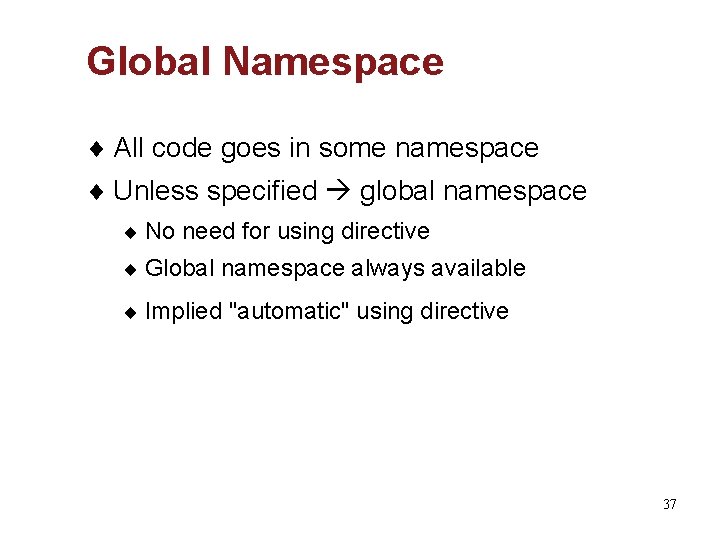
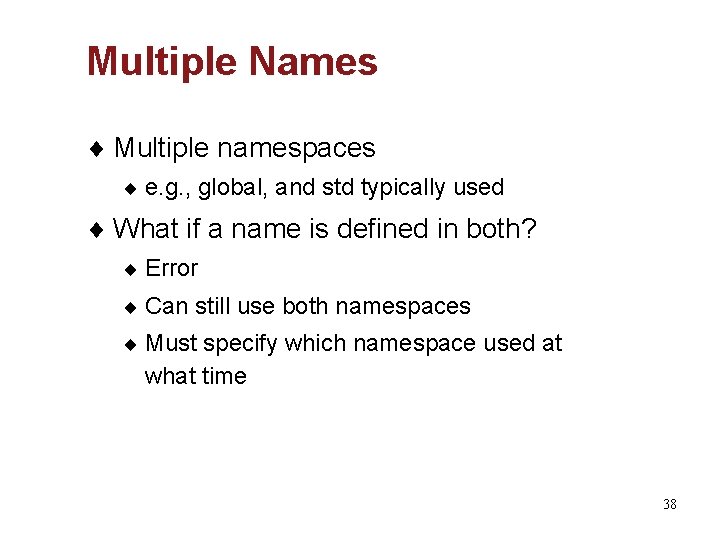
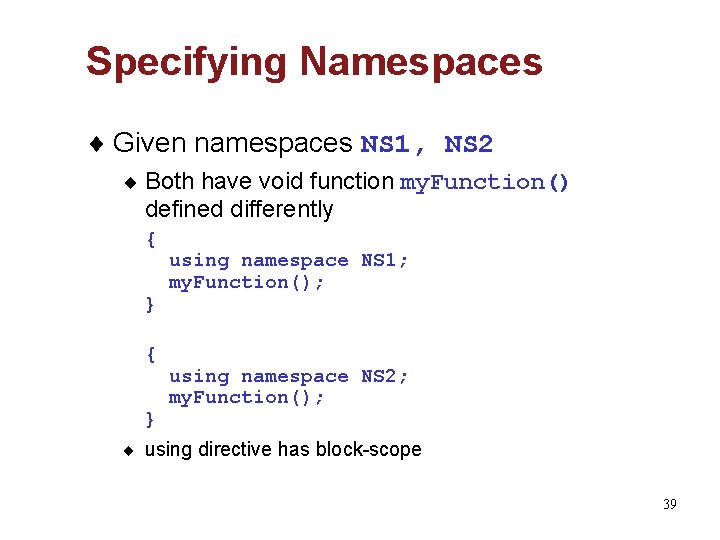
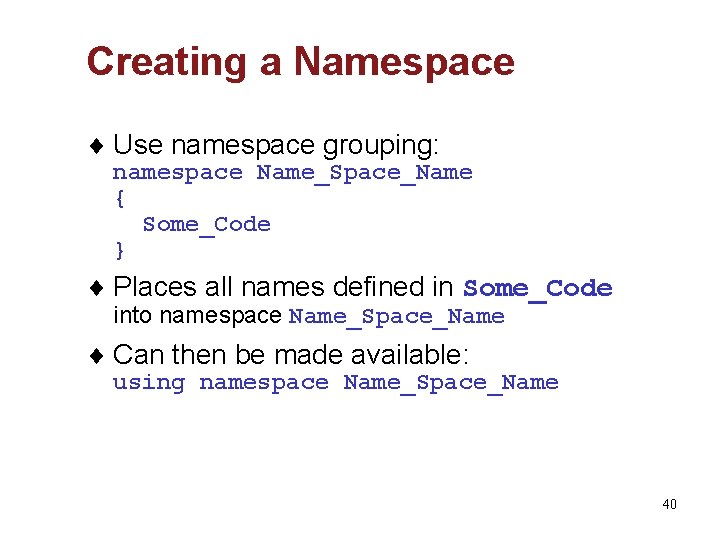
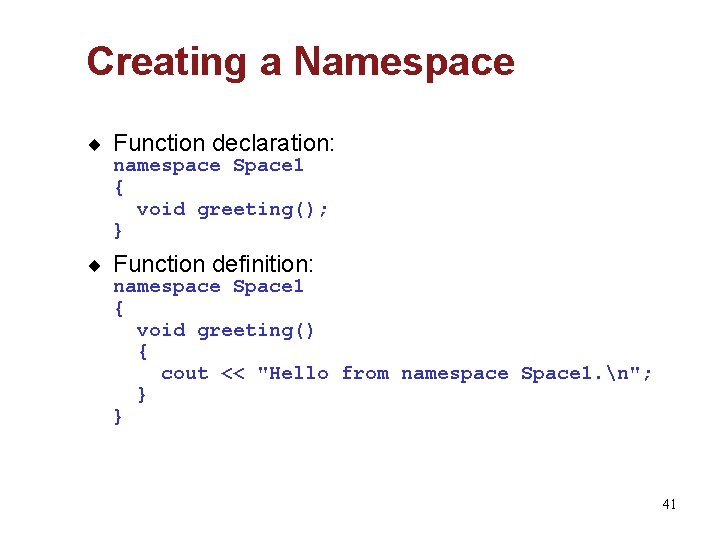
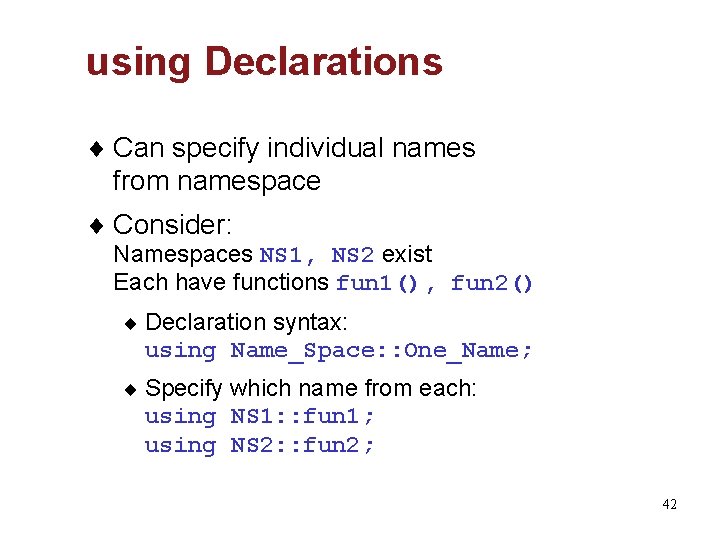
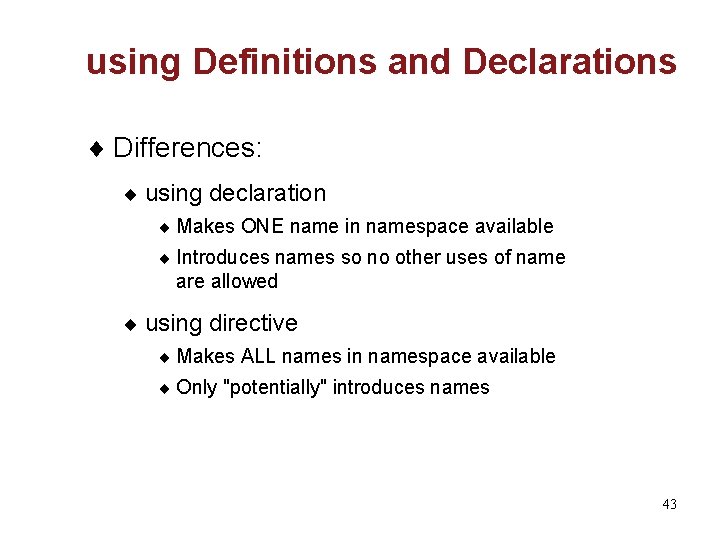
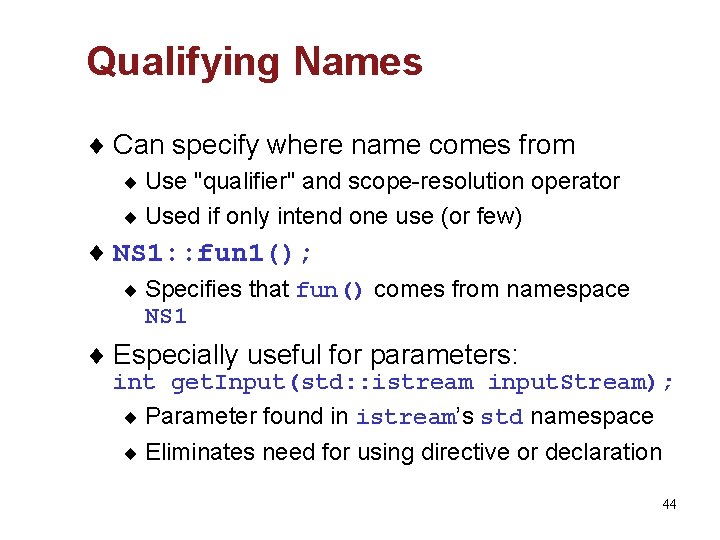
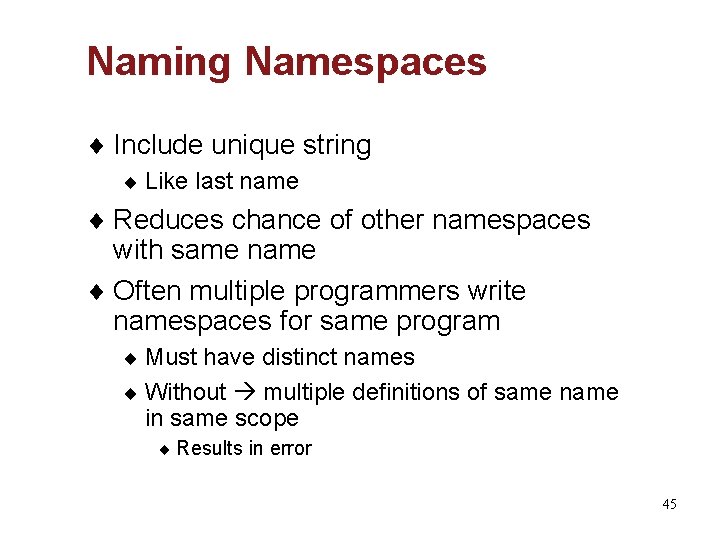
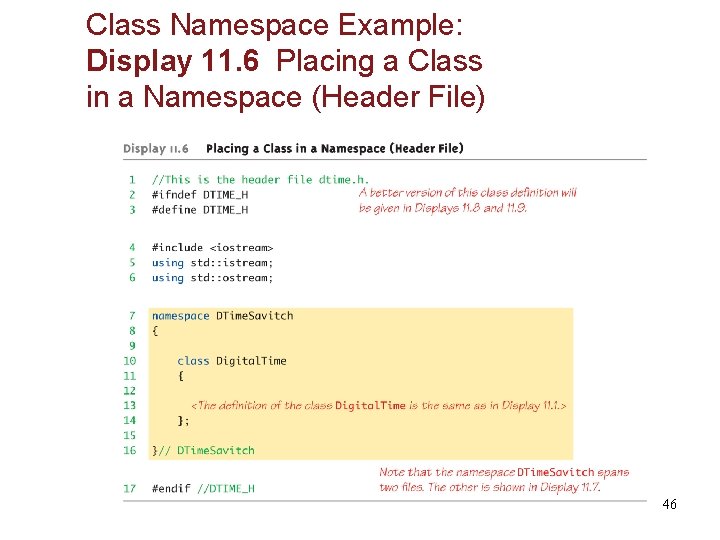
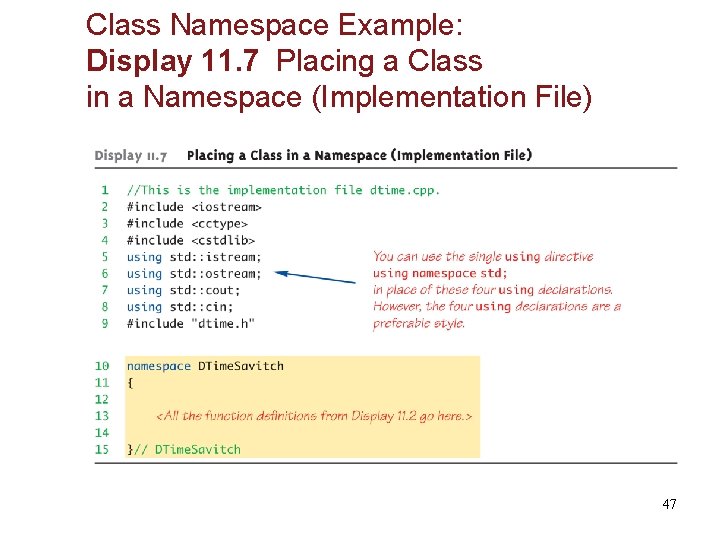
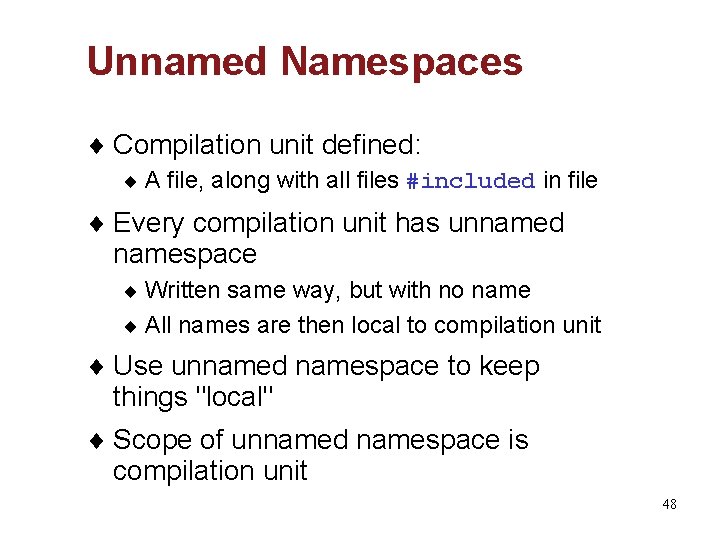
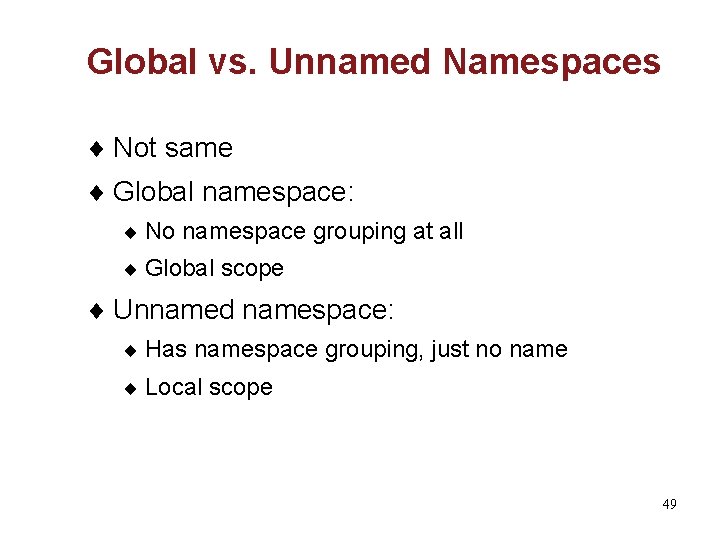
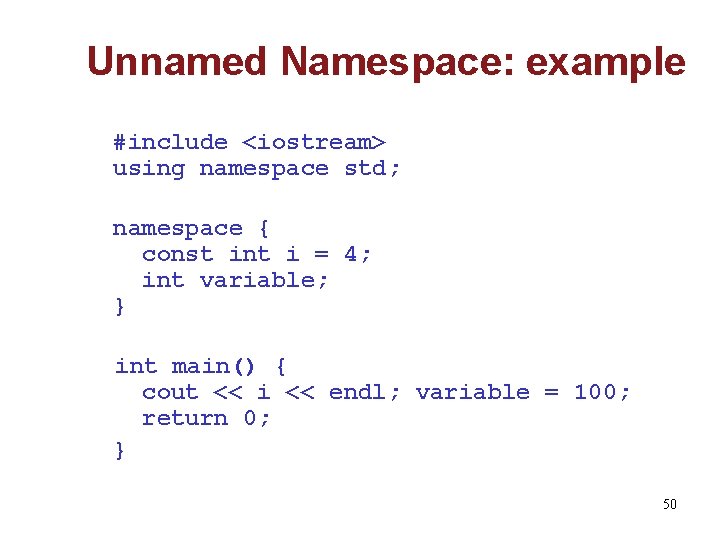
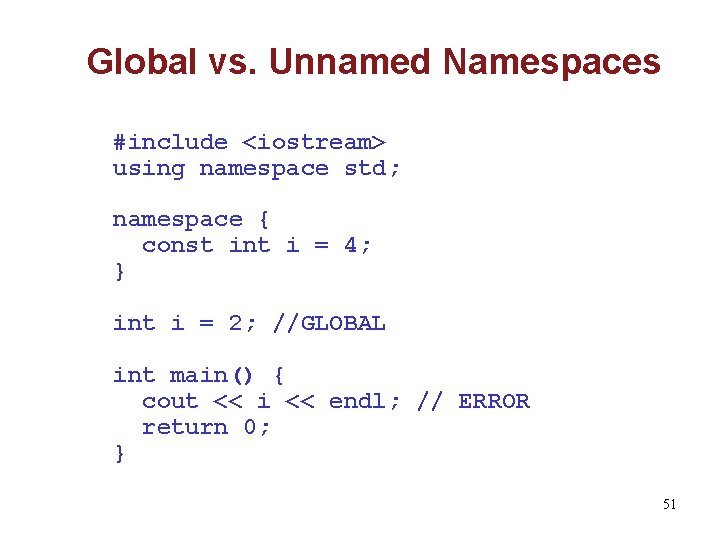
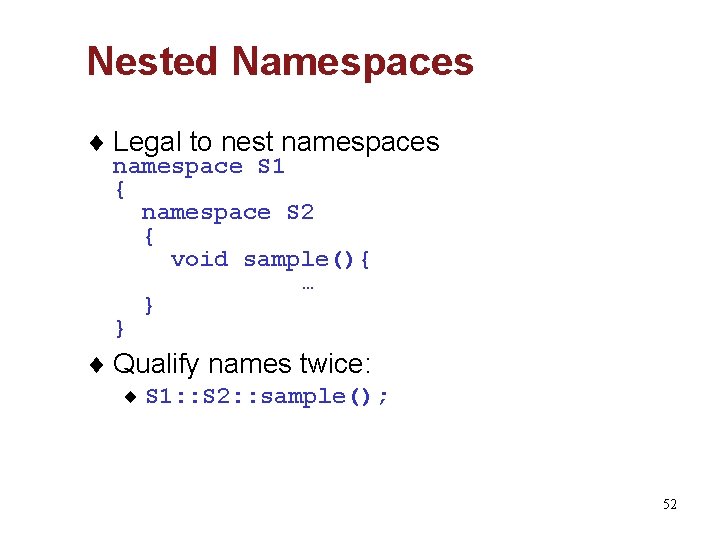
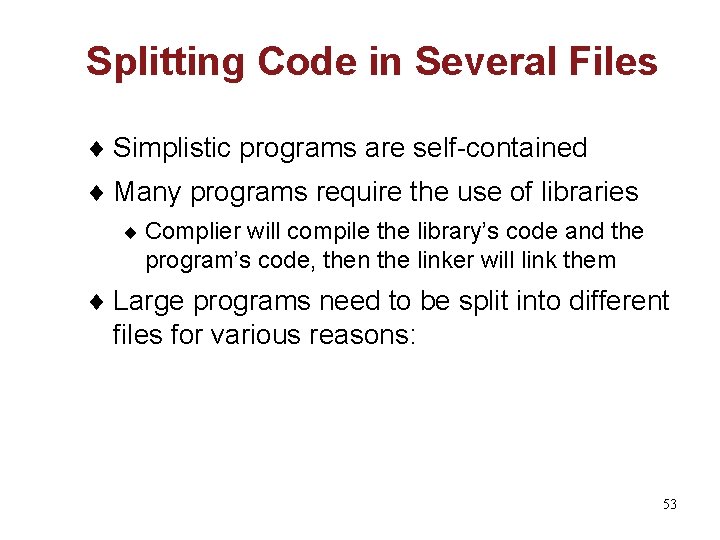
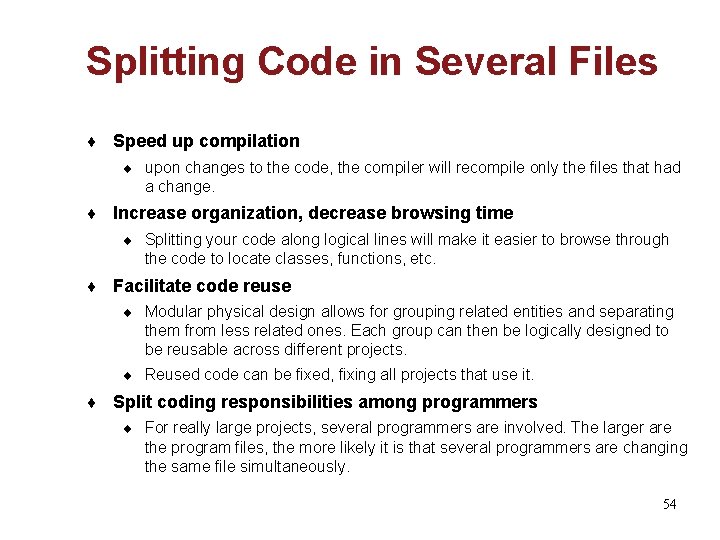
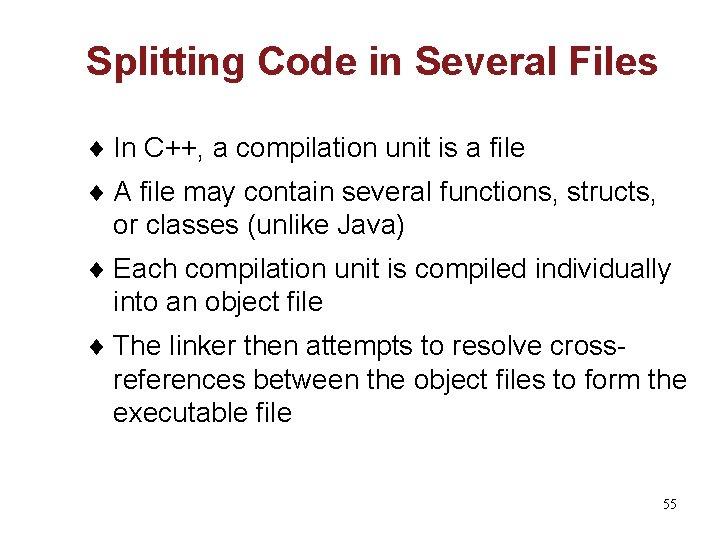
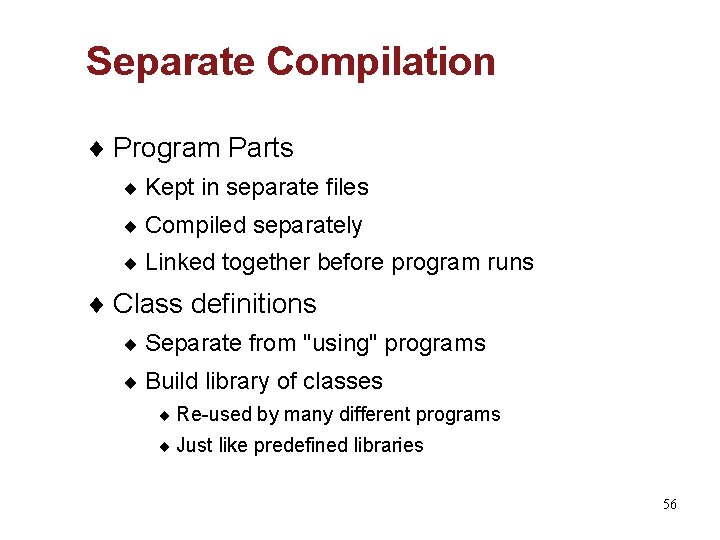
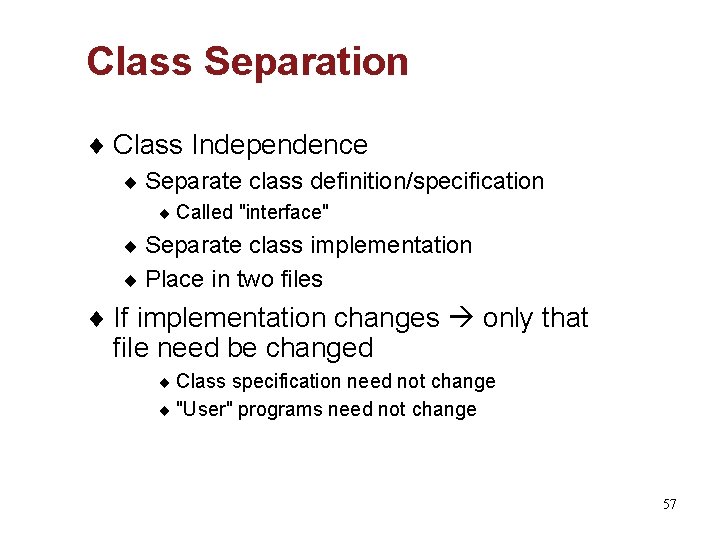
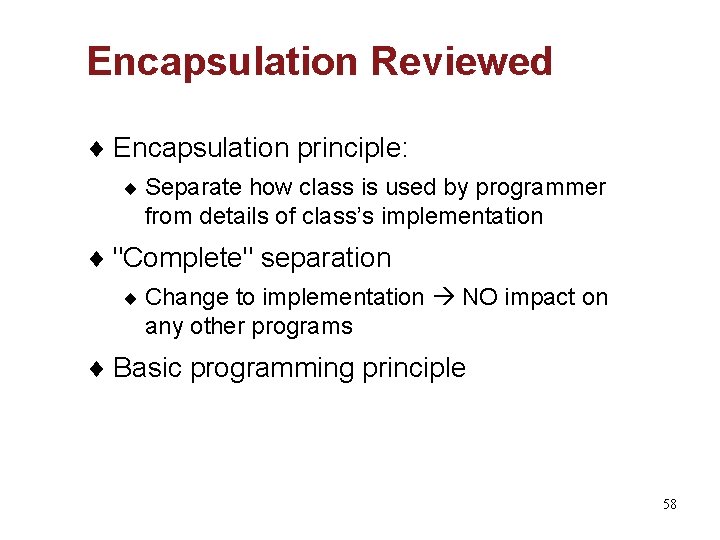
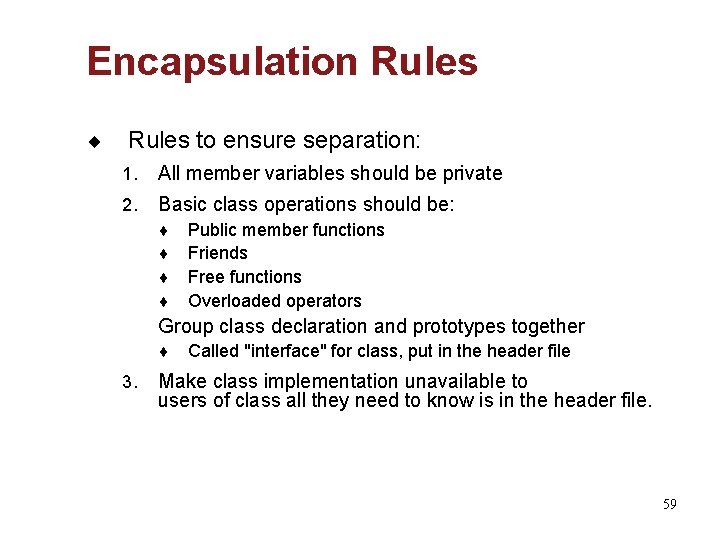
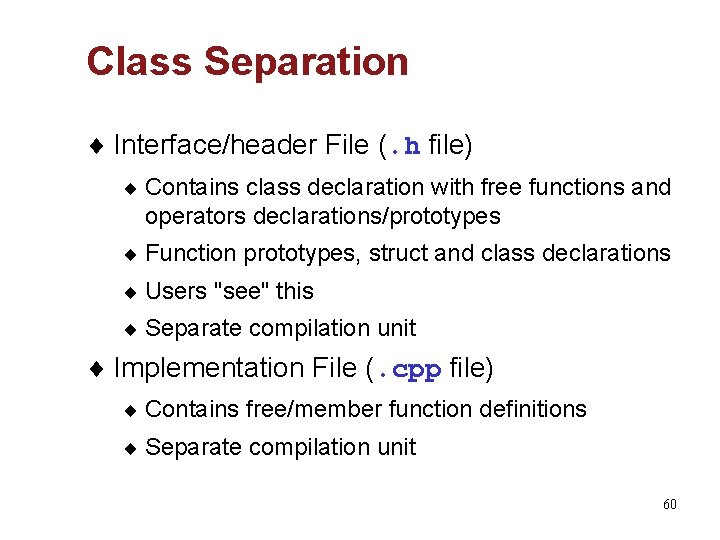
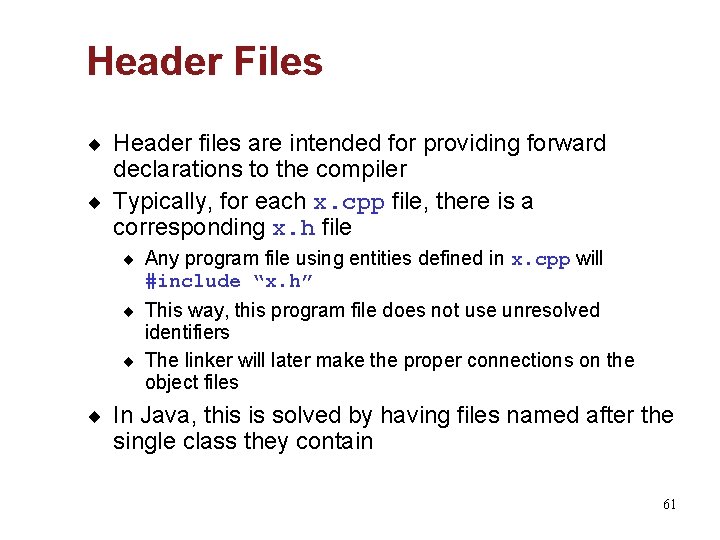
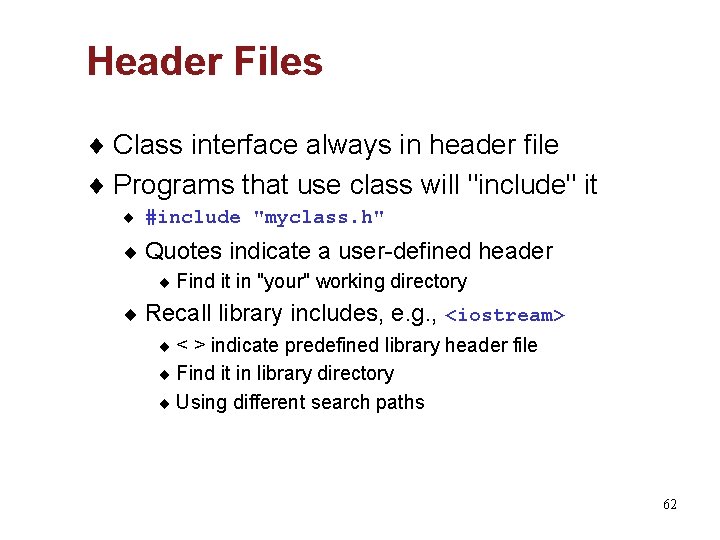
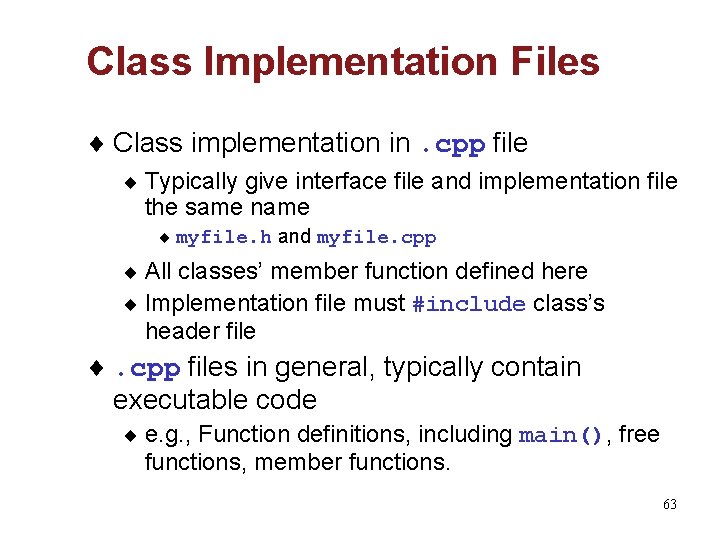
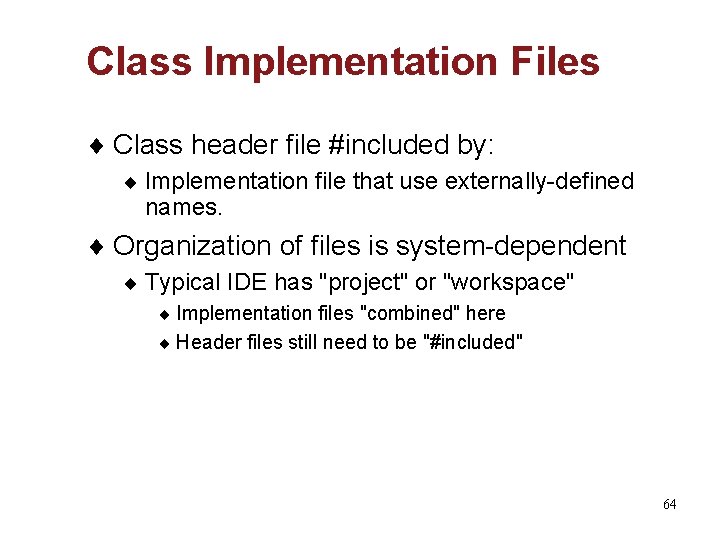
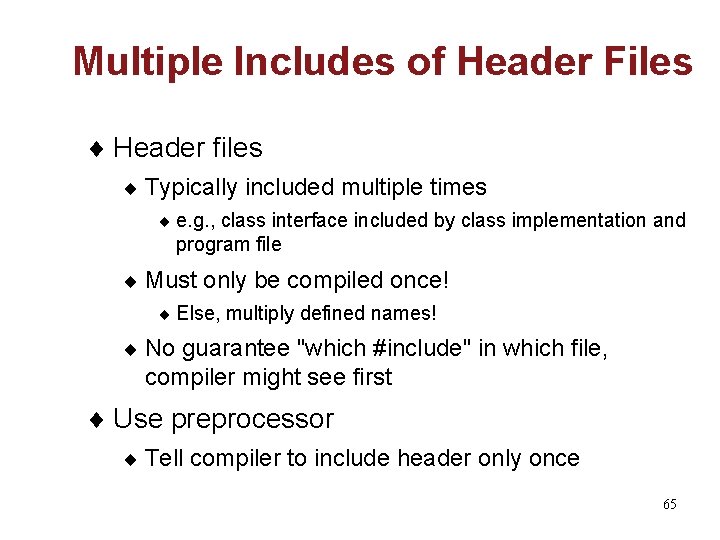
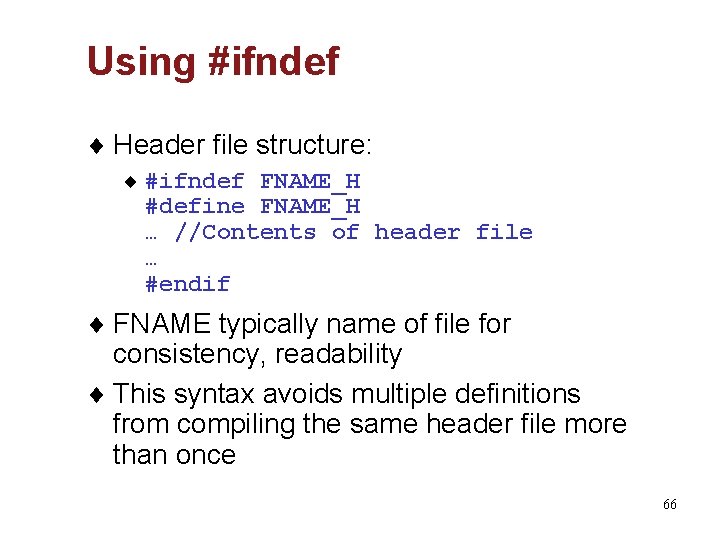
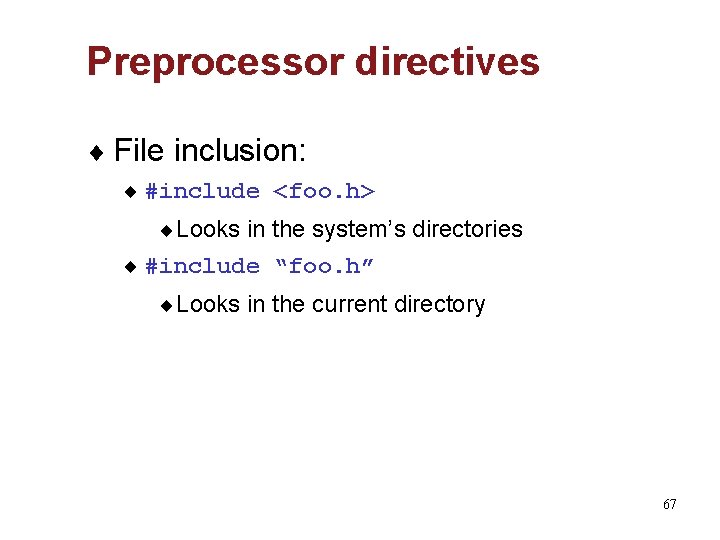
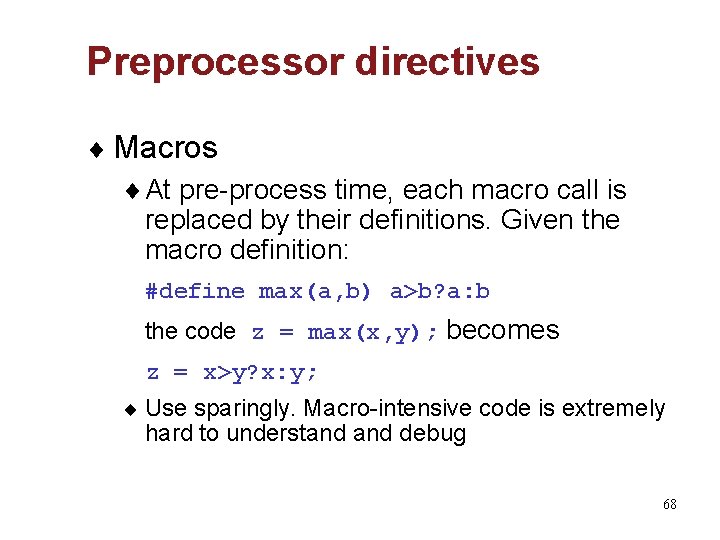
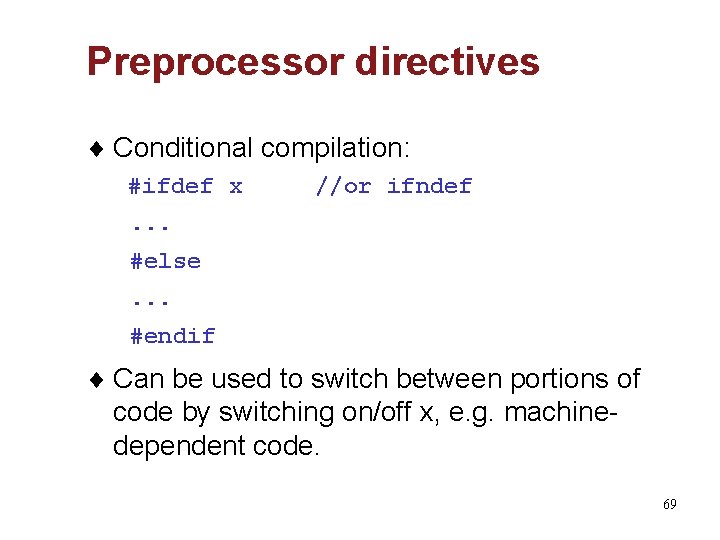
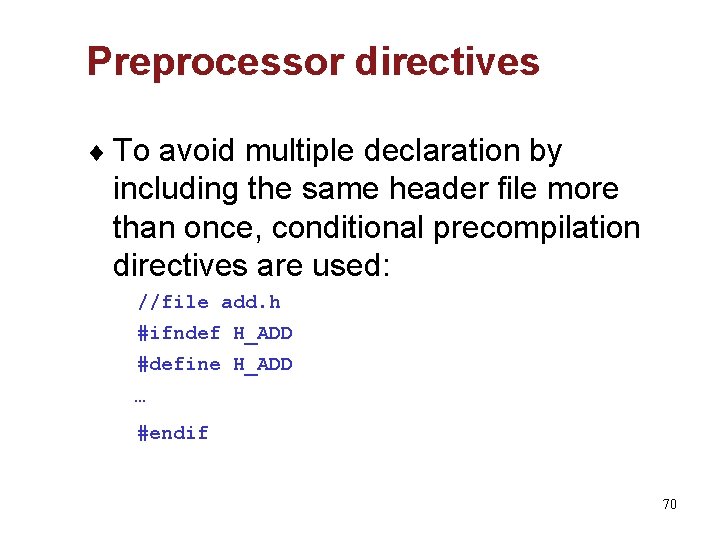
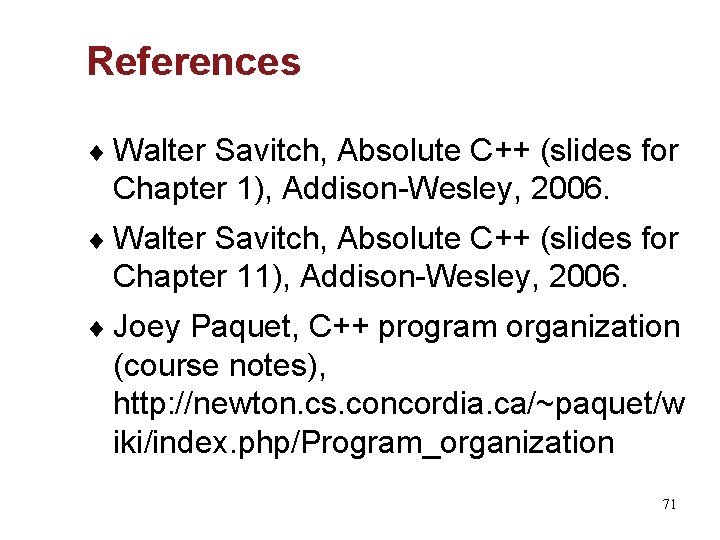
- Slides: 71
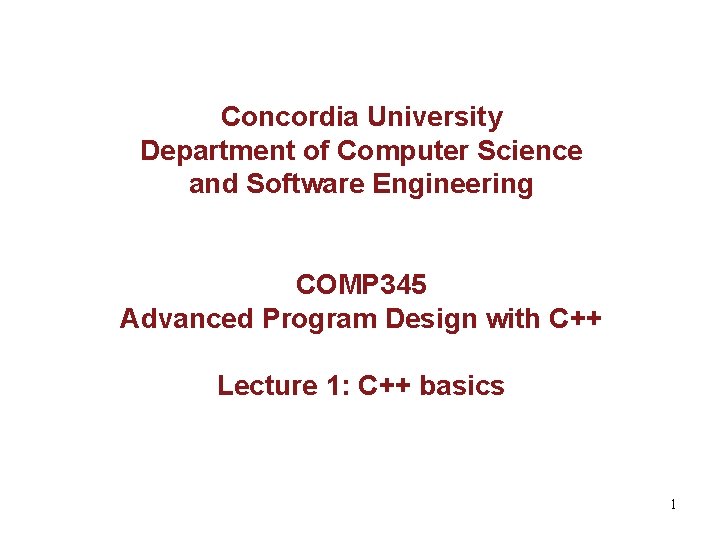
Concordia University Department of Computer Science and Software Engineering COMP 345 Advanced Program Design with C++ Lecture 1: C++ basics 1
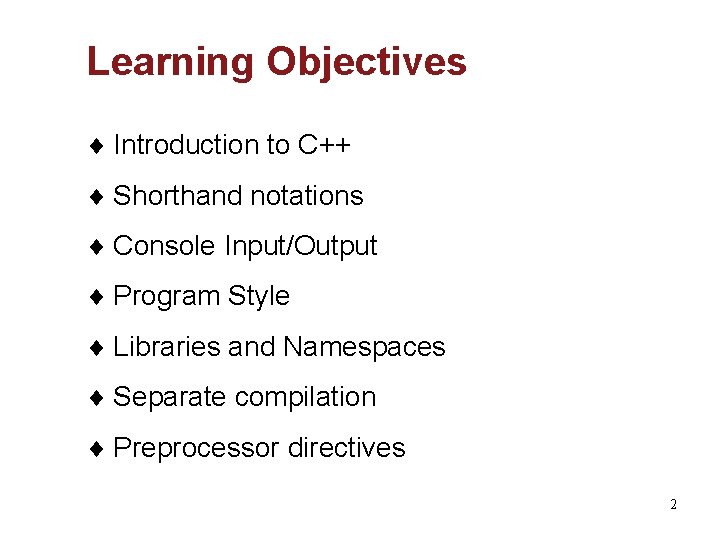
Learning Objectives ¨ Introduction to C++ ¨ Shorthand notations ¨ Console Input/Output ¨ Program Style ¨ Libraries and Namespaces ¨ Separate compilation ¨ Preprocessor directives 2
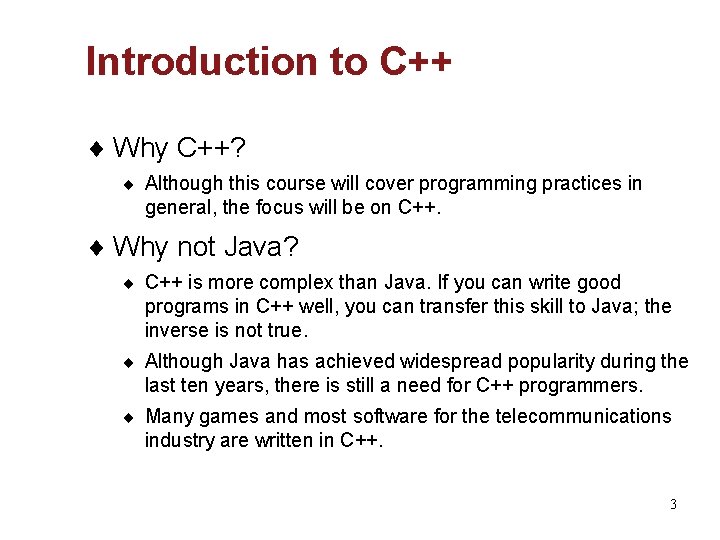
Introduction to C++ ¨ Why C++? ¨ Although this course will cover programming practices in general, the focus will be on C++. ¨ Why not Java? ¨ C++ is more complex than Java. If you can write good programs in C++ well, you can transfer this skill to Java; the inverse is not true. ¨ Although Java has achieved widespread popularity during the last ten years, there is still a need for C++ programmers. ¨ Many games and most software for the telecommunications industry are written in C++. 3
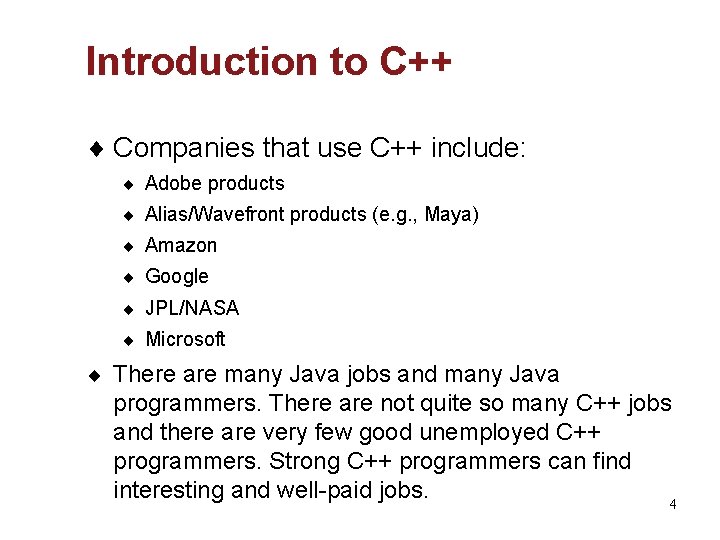
Introduction to C++ ¨ Companies that use C++ include: ¨ Adobe products ¨ Alias/Wavefront products (e. g. , Maya) ¨ Amazon ¨ Google ¨ JPL/NASA ¨ Microsoft ¨ There are many Java jobs and many Java programmers. There are not quite so many C++ jobs and there are very few good unemployed C++ programmers. Strong C++ programmers can find interesting and well-paid jobs. 4
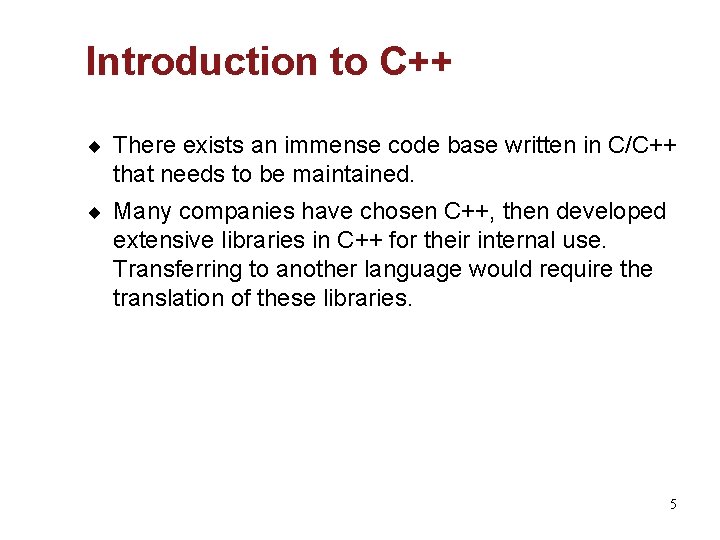
Introduction to C++ ¨ There exists an immense code base written in C/C++ that needs to be maintained. ¨ Many companies have chosen C++, then developed extensive libraries in C++ for their internal use. Transferring to another language would require the translation of these libraries. 5
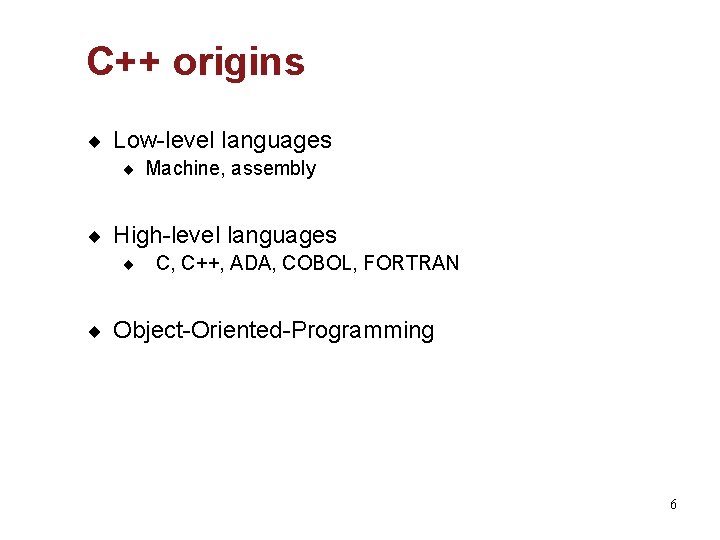
C++ origins ¨ Low-level languages ¨ Machine, assembly ¨ High-level languages ¨ C, C++, ADA, COBOL, FORTRAN ¨ Object-Oriented-Programming 6
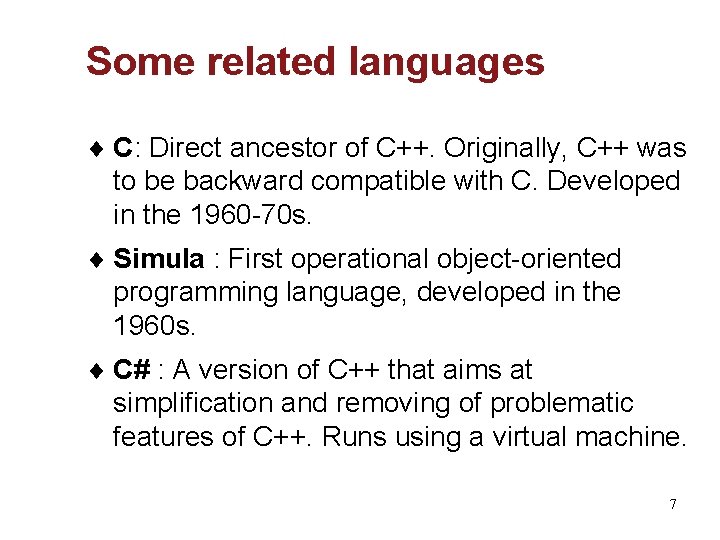
Some related languages ¨ C: Direct ancestor of C++. Originally, C++ was to be backward compatible with C. Developed in the 1960 -70 s. ¨ Simula : First operational object-oriented programming language, developed in the 1960 s. ¨ C# : A version of C++ that aims at simplification and removing of problematic features of C++. Runs using a virtual machine. 7
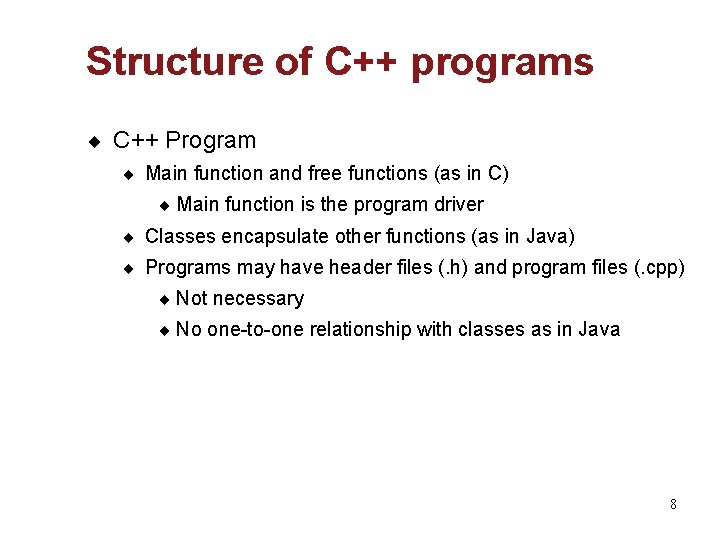
Structure of C++ programs ¨ C++ Program ¨ Main function and free functions (as in C) ¨ Main function is the program driver ¨ Classes encapsulate other functions (as in Java) ¨ Programs may have header files (. h) and program files (. cpp) ¨ Not necessary ¨ No one-to-one relationship with classes as in Java 8
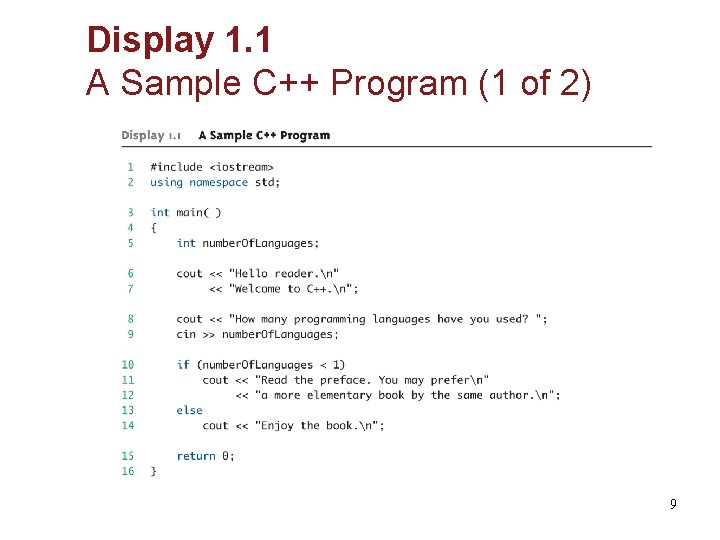
Display 1. 1 A Sample C++ Program (1 of 2) 9
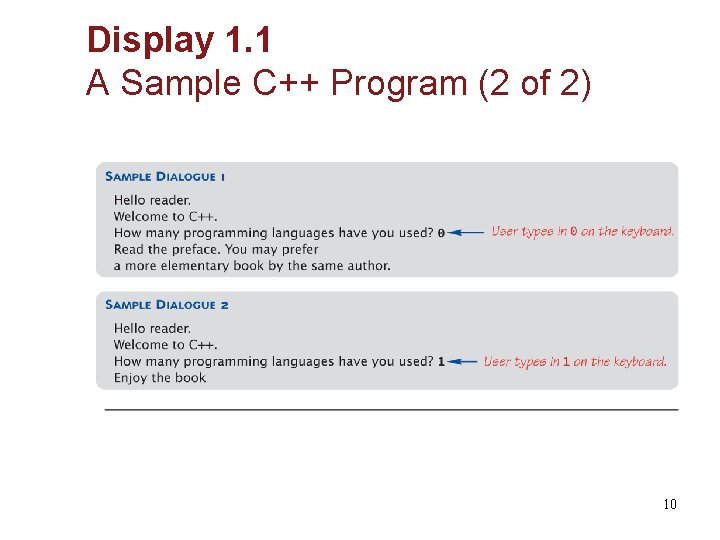
Display 1. 1 A Sample C++ Program (2 of 2) 10
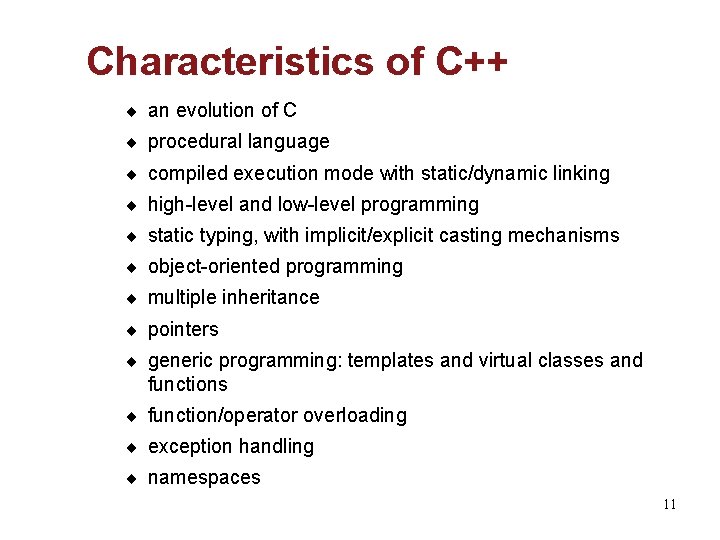
Characteristics of C++ ¨ an evolution of C ¨ procedural language ¨ compiled execution mode with static/dynamic linking ¨ high-level and low-level programming ¨ static typing, with implicit/explicit casting mechanisms ¨ object-oriented programming ¨ multiple inheritance ¨ pointers ¨ generic programming: templates and virtual classes and functions ¨ function/operator overloading ¨ exception handling ¨ namespaces 11
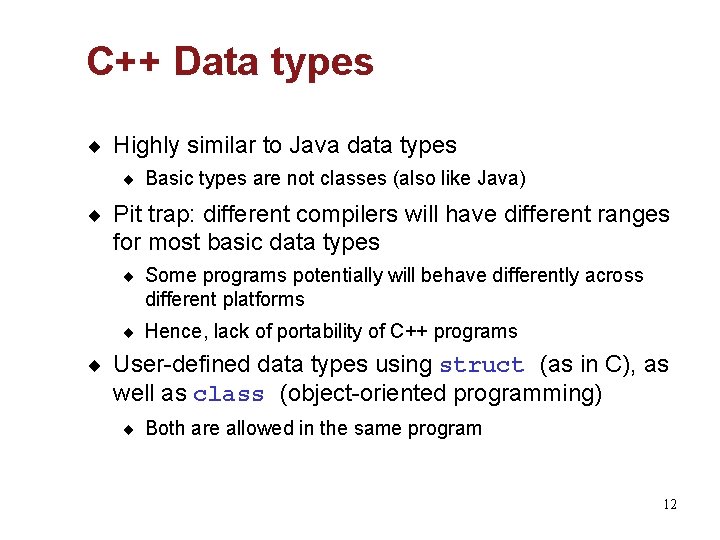
C++ Data types ¨ Highly similar to Java data types ¨ Basic types are not classes (also like Java) ¨ Pit trap: different compilers will have different ranges for most basic data types ¨ Some programs potentially will behave differently across different platforms ¨ Hence, lack of portability of C++ programs ¨ User-defined data types using struct (as in C), as well as class (object-oriented programming) ¨ Both are allowed in the same program 12
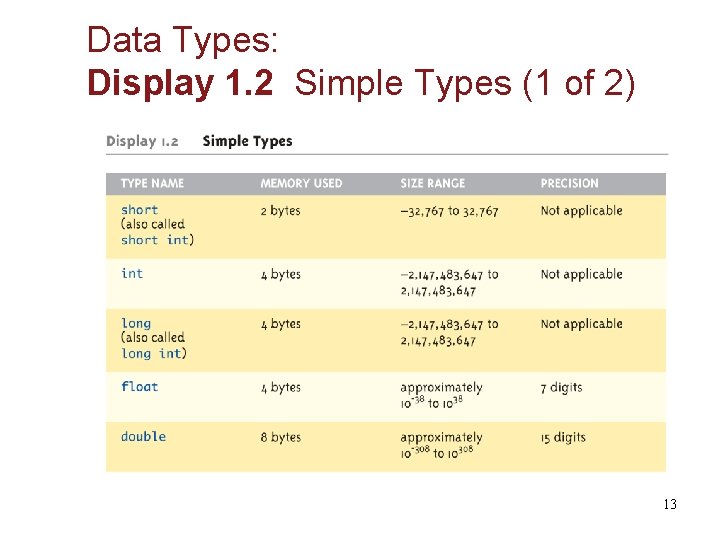
Data Types: Display 1. 2 Simple Types (1 of 2) 13
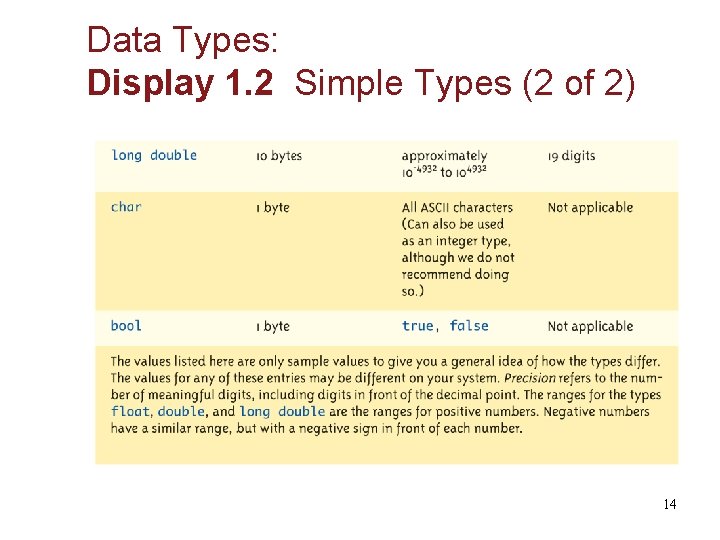
Data Types: Display 1. 2 Simple Types (2 of 2) 14
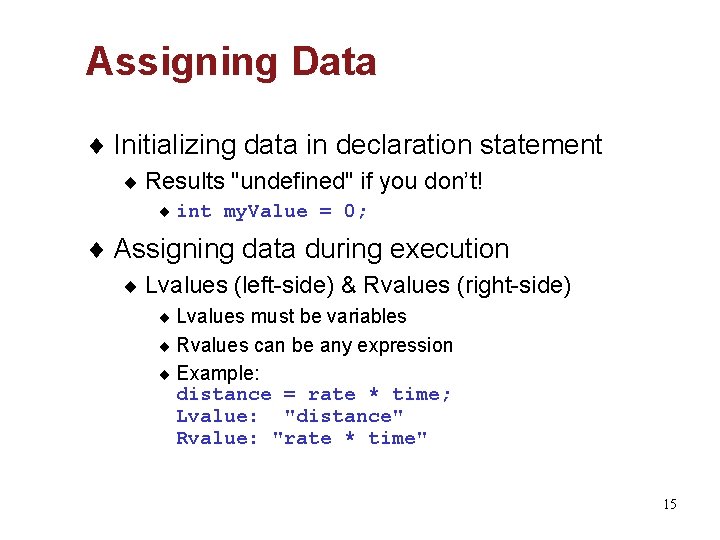
Assigning Data ¨ Initializing data in declaration statement ¨ Results "undefined" if you don’t! ¨ int my. Value = 0; ¨ Assigning data during execution ¨ Lvalues (left-side) & Rvalues (right-side) ¨ Lvalues must be variables ¨ Rvalues can be any expression ¨ Example: distance = rate * time; Lvalue: "distance" Rvalue: "rate * time" 15
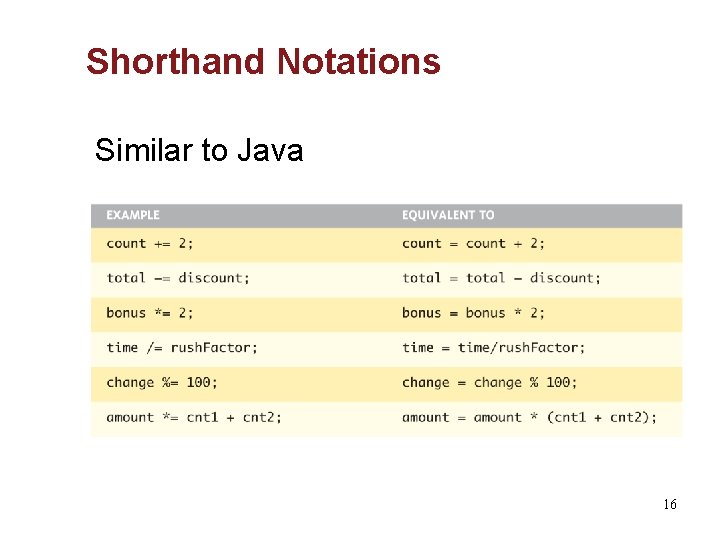
Shorthand Notations Similar to Java 16
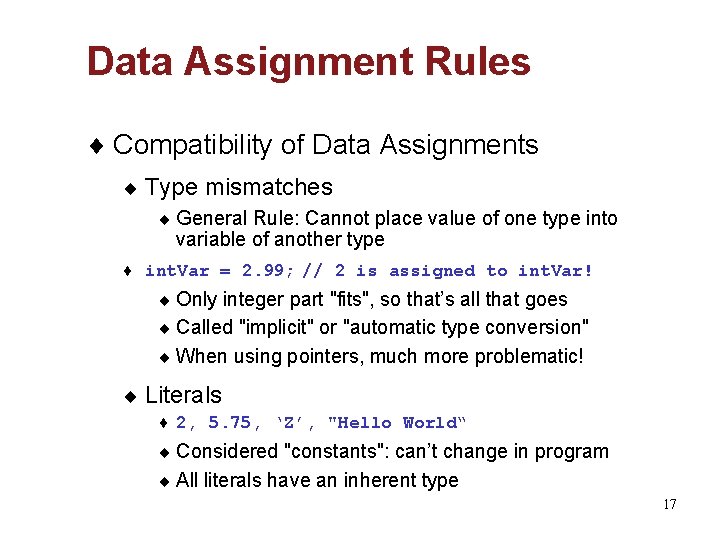
Data Assignment Rules ¨ Compatibility of Data Assignments ¨ Type mismatches ¨ General Rule: Cannot place value of one type into variable of another type ¨ int. Var = 2. 99; // 2 is assigned to int. Var! ¨ Only integer part "fits", so that’s all that goes ¨ Called "implicit" or "automatic type conversion" ¨ When using pointers, much more problematic! ¨ Literals ¨ 2, 5. 75, ‘Z’, "Hello World“ ¨ Considered "constants": can’t change in program ¨ All literals have an inherent type 17
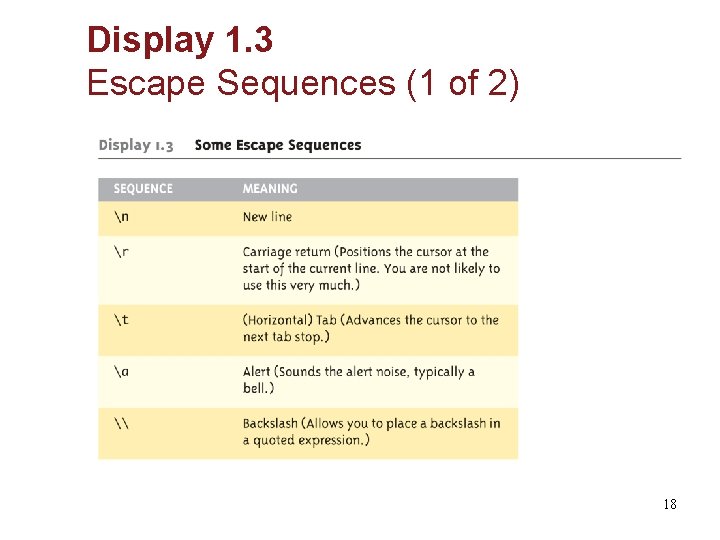
Display 1. 3 Escape Sequences (1 of 2) 18
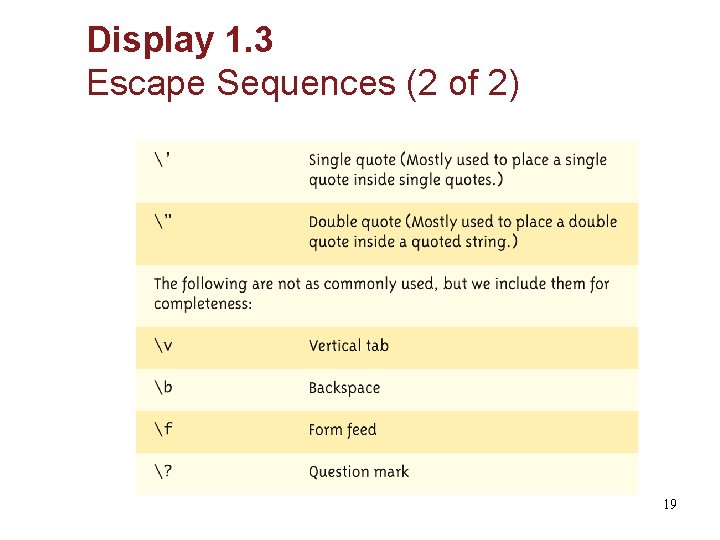
Display 1. 3 Escape Sequences (2 of 2) 19
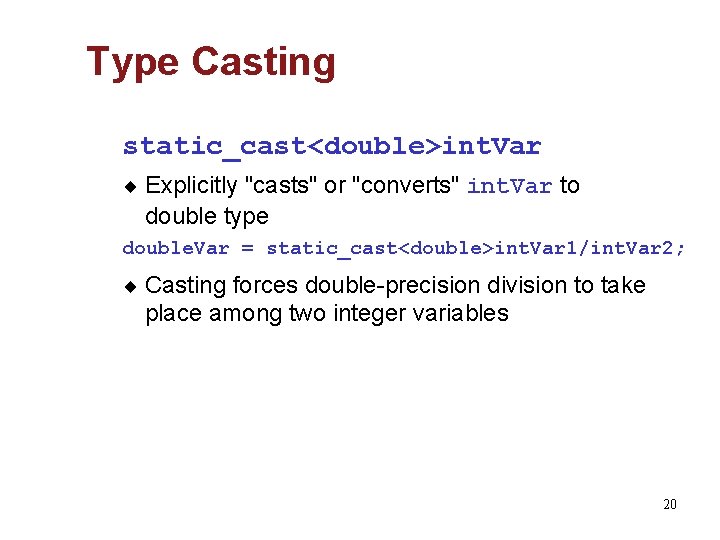
Type Casting static_cast<double>int. Var ¨ Explicitly "casts" or "converts" int. Var to double type double. Var = static_cast<double>int. Var 1/int. Var 2; ¨ Casting forces double-precision division to take place among two integer variables 20
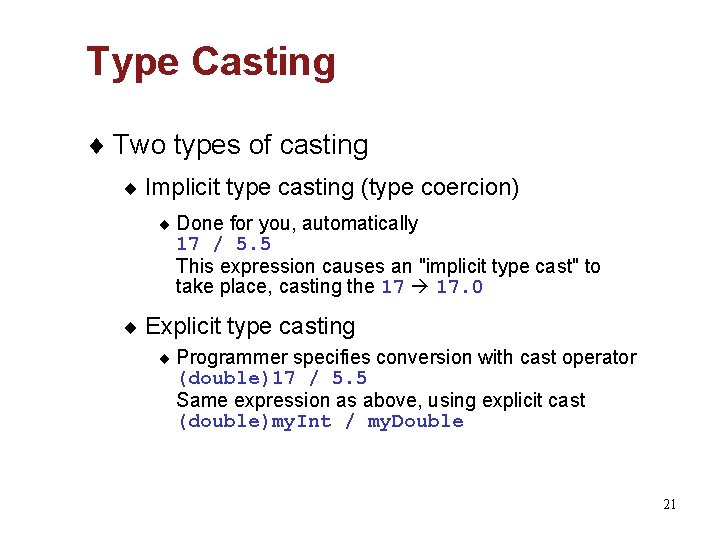
Type Casting ¨ Two types of casting ¨ Implicit type casting (type coercion) ¨ Done for you, automatically 17 / 5. 5 This expression causes an "implicit type cast" to take place, casting the 17 17. 0 ¨ Explicit type casting ¨ Programmer specifies conversion with cast operator (double)17 / 5. 5 Same expression as above, using explicit cast (double)my. Int / my. Double 21
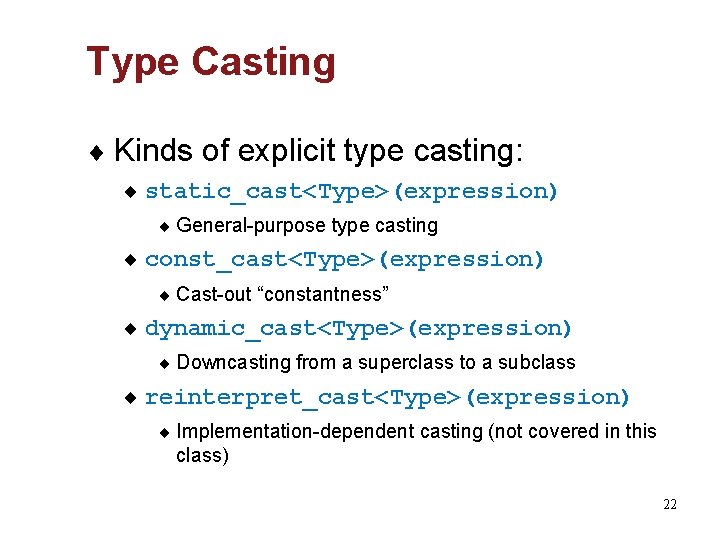
Type Casting ¨ Kinds of explicit type casting: ¨ static_cast<Type>(expression) ¨ General-purpose type casting ¨ const_cast<Type>(expression) ¨ Cast-out “constantness” ¨ dynamic_cast<Type>(expression) ¨ Downcasting from a superclass to a subclass ¨ reinterpret_cast<Type>(expression) ¨ Implementation-dependent casting (not covered in this class) 22
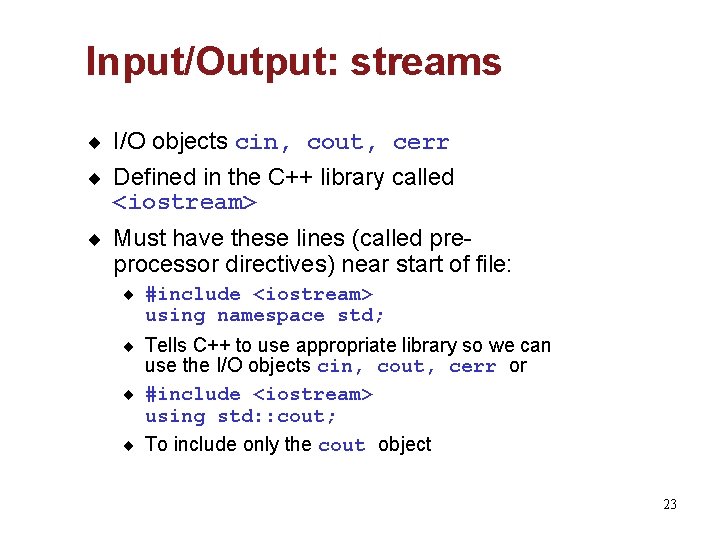
Input/Output: streams ¨ I/O objects cin, cout, cerr ¨ Defined in the C++ library called <iostream> ¨ Must have these lines (called pre- processor directives) near start of file: ¨ #include <iostream> using namespace std; ¨ Tells C++ to use appropriate library so we can use the I/O objects cin, cout, cerr or ¨ #include <iostream> using std: : cout; ¨ To include only the cout object 23
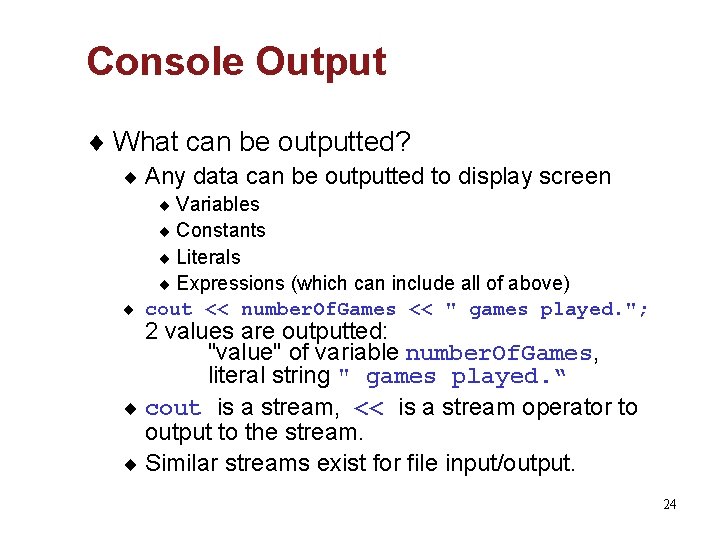
Console Output ¨ What can be outputted? ¨ Any data can be outputted to display screen ¨ Variables ¨ Constants ¨ Literals ¨ Expressions (which can include all of above) ¨ cout << number. Of. Games << " games played. "; 2 values are outputted: "value" of variable number. Of. Games, literal string " games played. “ ¨ cout is a stream, << is a stream operator to output to the stream. ¨ Similar streams exist for file input/output. 24
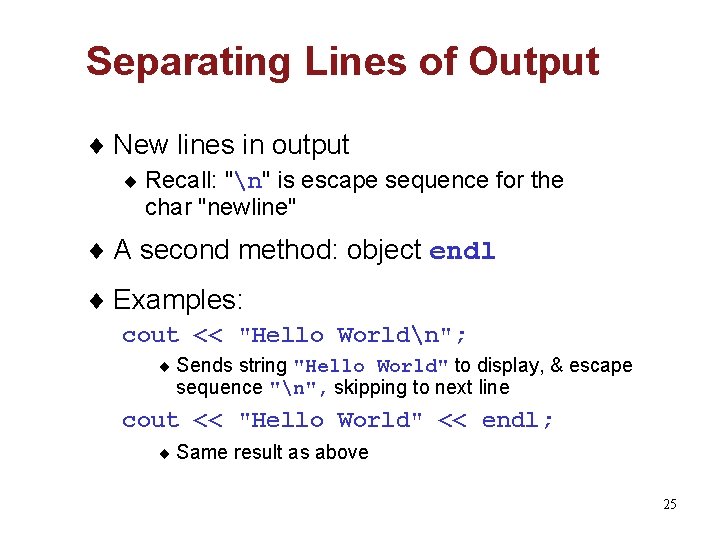
Separating Lines of Output ¨ New lines in output ¨ Recall: "n" is escape sequence for the char "newline" ¨ A second method: object endl ¨ Examples: cout << "Hello Worldn"; ¨ Sends string "Hello World" to display, & escape sequence "n", skipping to next line cout << "Hello World" << endl; ¨ Same result as above 25
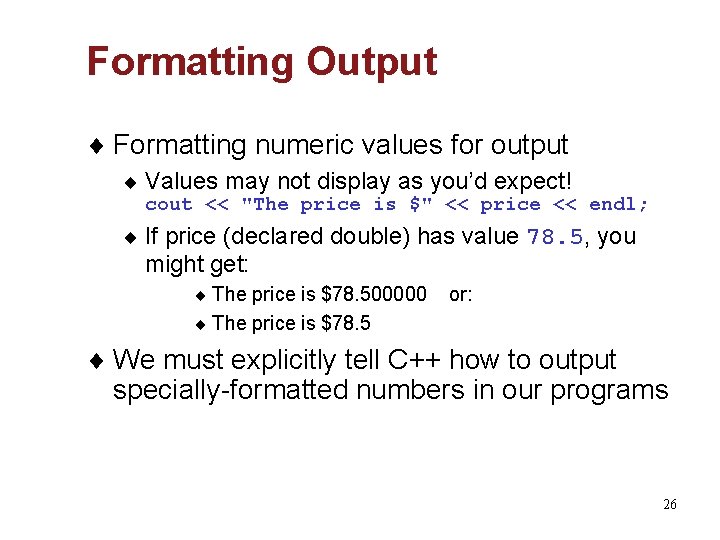
Formatting Output ¨ Formatting numeric values for output ¨ Values may not display as you’d expect! cout << "The price is $" << price << endl; ¨ If price (declared double) has value 78. 5, you might get: ¨ The price is $78. 500000 or: ¨ The price is $78. 5 ¨ We must explicitly tell C++ how to output specially-formatted numbers in our programs 26
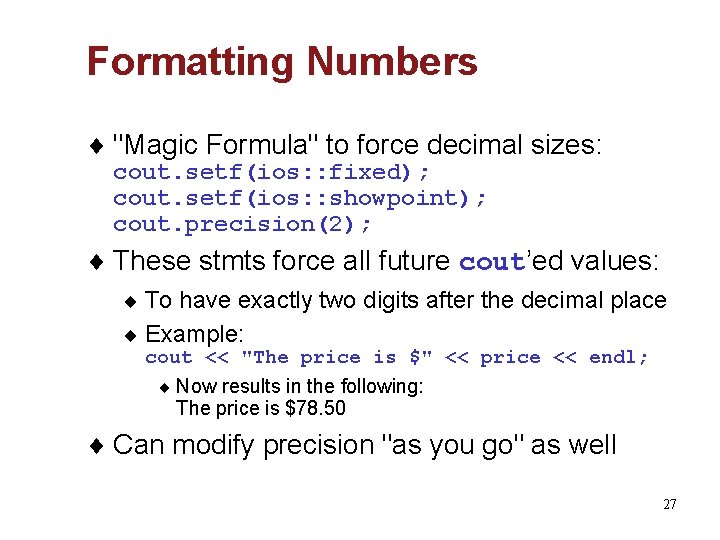
Formatting Numbers ¨ "Magic Formula" to force decimal sizes: cout. setf(ios: : fixed); cout. setf(ios: : showpoint); cout. precision(2); ¨ These stmts force all future cout’ed values: ¨ To have exactly two digits after the decimal place ¨ Example: cout << "The price is $" << price << endl; ¨ Now results in the following: The price is $78. 50 ¨ Can modify precision "as you go" as well 27
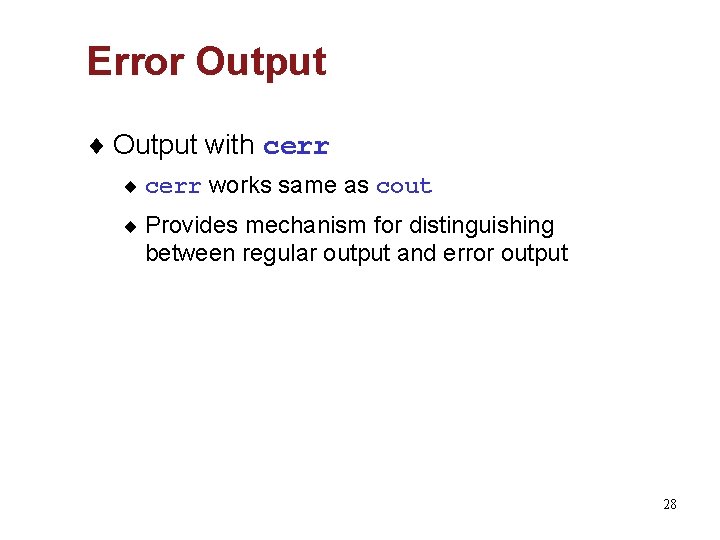
Error Output ¨ Output with cerr ¨ cerr works same as cout ¨ Provides mechanism for distinguishing between regular output and error output 28
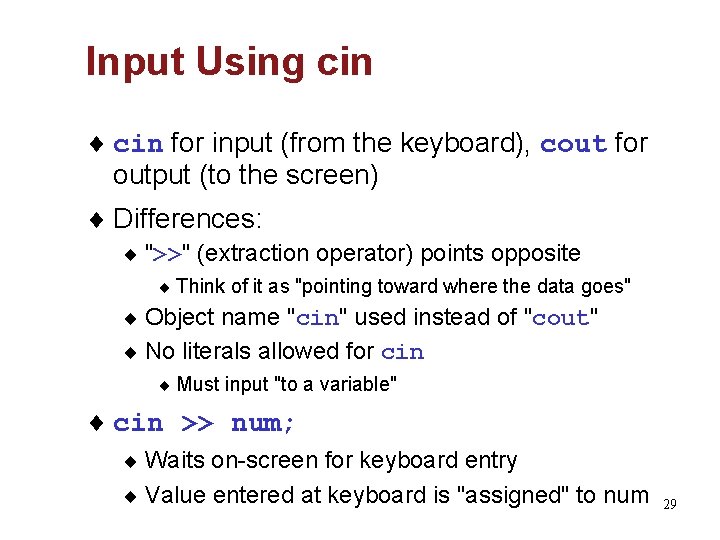
Input Using cin ¨ cin for input (from the keyboard), cout for output (to the screen) ¨ Differences: ¨ ">>" (extraction operator) points opposite ¨ Think of it as "pointing toward where the data goes" ¨ Object name "cin" used instead of "cout" ¨ No literals allowed for cin ¨ Must input "to a variable" ¨ cin >> num; ¨ Waits on-screen for keyboard entry ¨ Value entered at keyboard is "assigned" to num 29
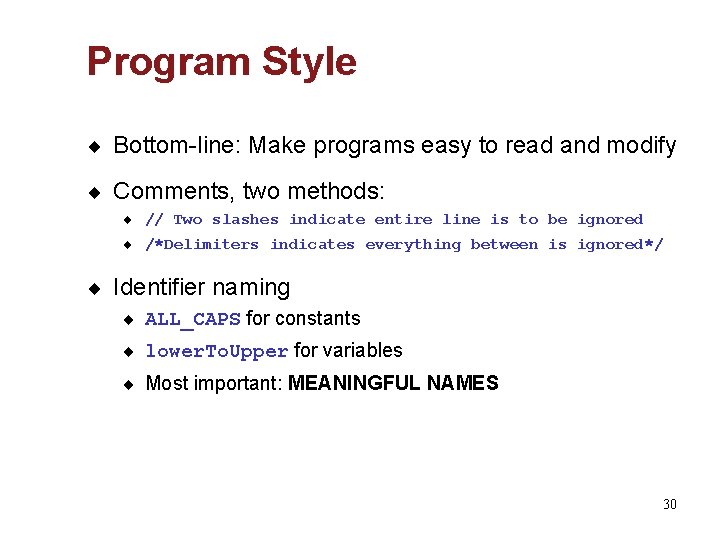
Program Style ¨ Bottom-line: Make programs easy to read and modify ¨ Comments, two methods: ¨ // Two slashes indicate entire line is to be ignored ¨ /*Delimiters indicates everything between is ignored*/ ¨ Identifier naming ¨ ALL_CAPS for constants ¨ lower. To. Upper for variables ¨ Most important: MEANINGFUL NAMES 30
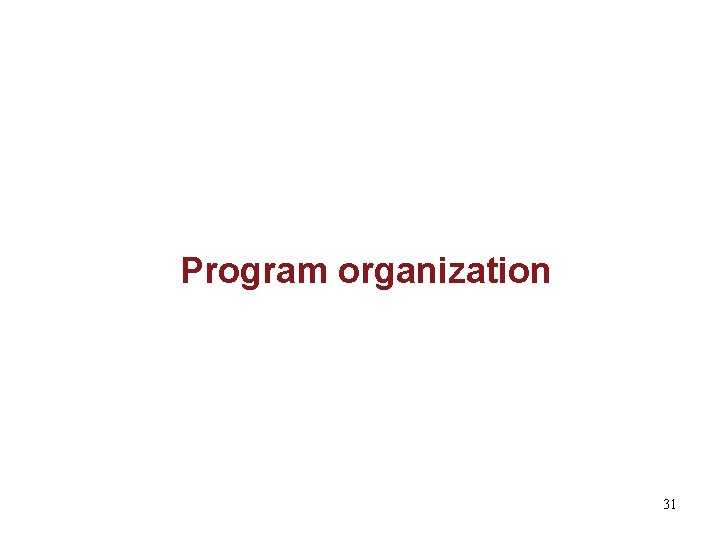
Program organization 31
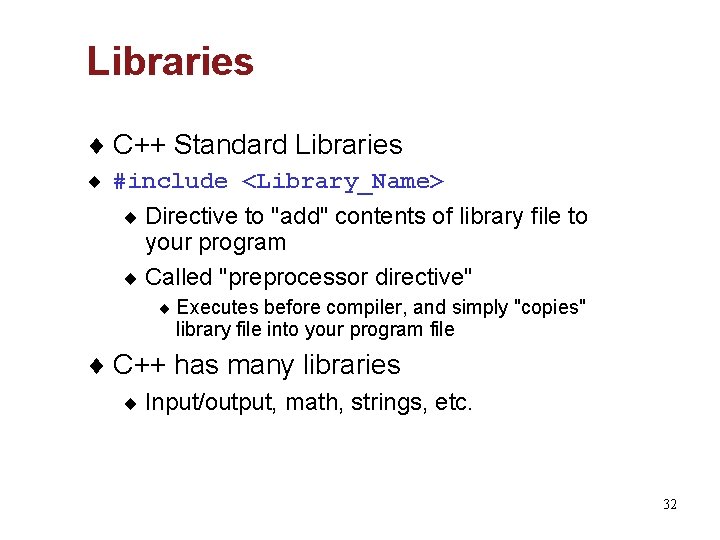
Libraries ¨ C++ Standard Libraries ¨ #include <Library_Name> ¨ Directive to "add" contents of library file to your program ¨ Called "preprocessor directive" ¨ Executes before compiler, and simply "copies" library file into your program file ¨ C++ has many libraries ¨ Input/output, math, strings, etc. 32
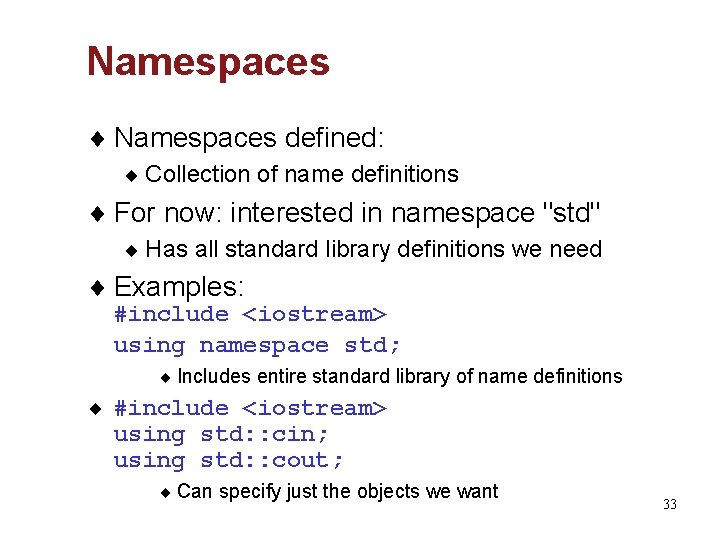
Namespaces ¨ Namespaces defined: ¨ Collection of name definitions ¨ For now: interested in namespace "std" ¨ Has all standard library definitions we need ¨ Examples: #include <iostream> using namespace std; ¨ Includes entire standard library of name definitions ¨ #include <iostream> using std: : cin; using std: : cout; ¨ Can specify just the objects we want 33
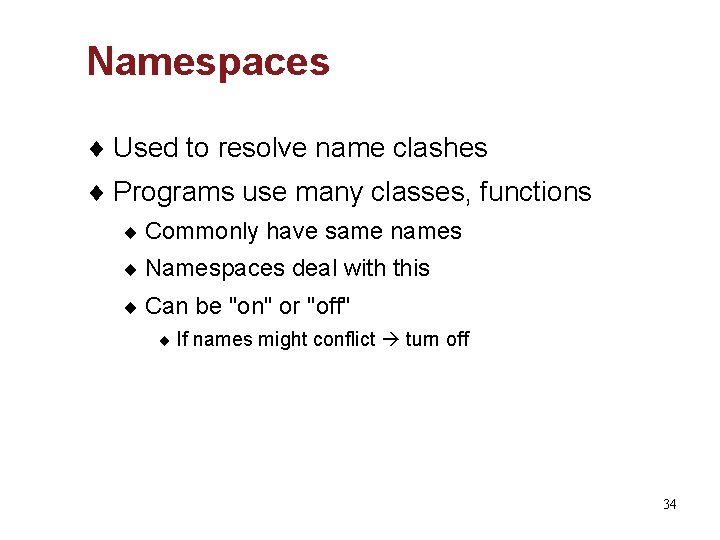
Namespaces ¨ Used to resolve name clashes ¨ Programs use many classes, functions ¨ Commonly have same names ¨ Namespaces deal with this ¨ Can be "on" or "off" ¨ If names might conflict turn off 34
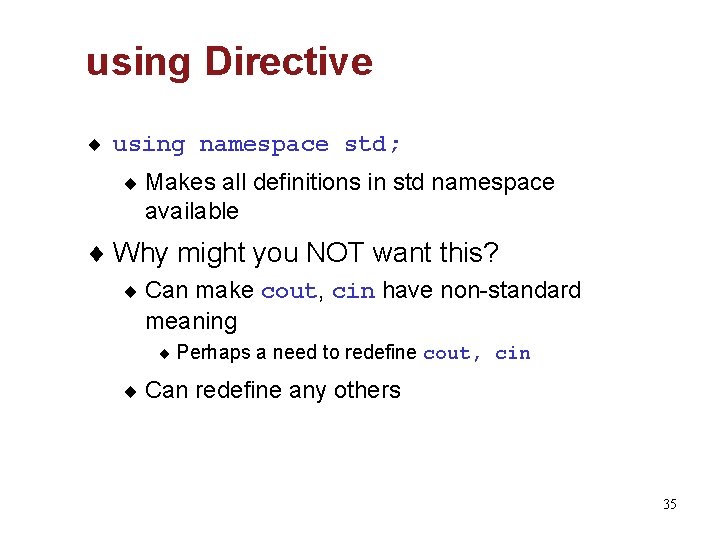
using Directive ¨ using namespace std; ¨ Makes all definitions in std namespace available ¨ Why might you NOT want this? ¨ Can make cout, cin have non-standard meaning ¨ Perhaps a need to redefine cout, cin ¨ Can redefine any others 35
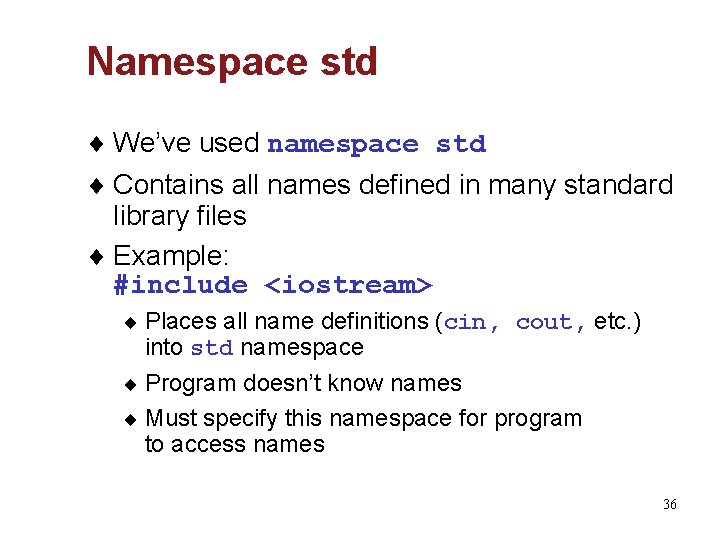
Namespace std ¨ We’ve used namespace std ¨ Contains all names defined in many standard library files ¨ Example: #include <iostream> ¨ Places all name definitions (cin, cout, etc. ) into std namespace ¨ Program doesn’t know names ¨ Must specify this namespace for program to access names 36
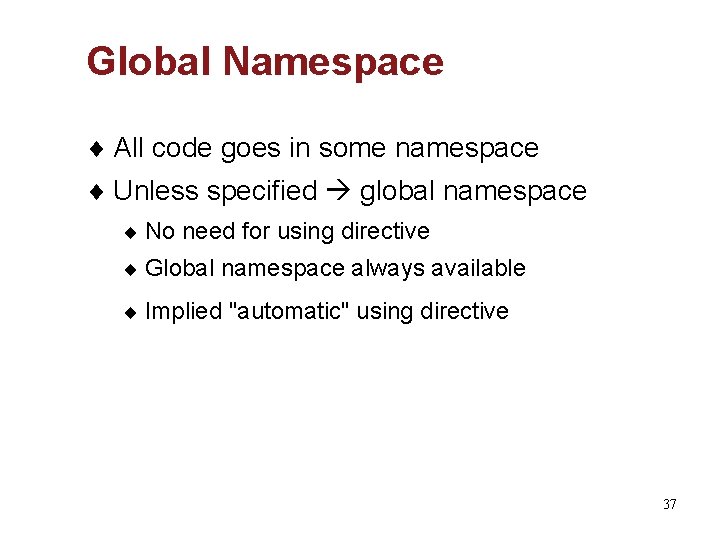
Global Namespace ¨ All code goes in some namespace ¨ Unless specified global namespace ¨ No need for using directive ¨ Global namespace always available ¨ Implied "automatic" using directive 37
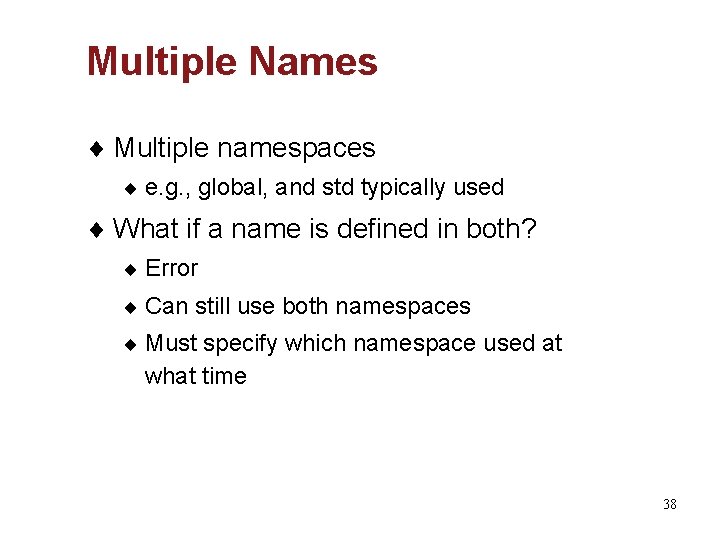
Multiple Names ¨ Multiple namespaces ¨ e. g. , global, and std typically used ¨ What if a name is defined in both? ¨ Error ¨ Can still use both namespaces ¨ Must specify which namespace used at what time 38
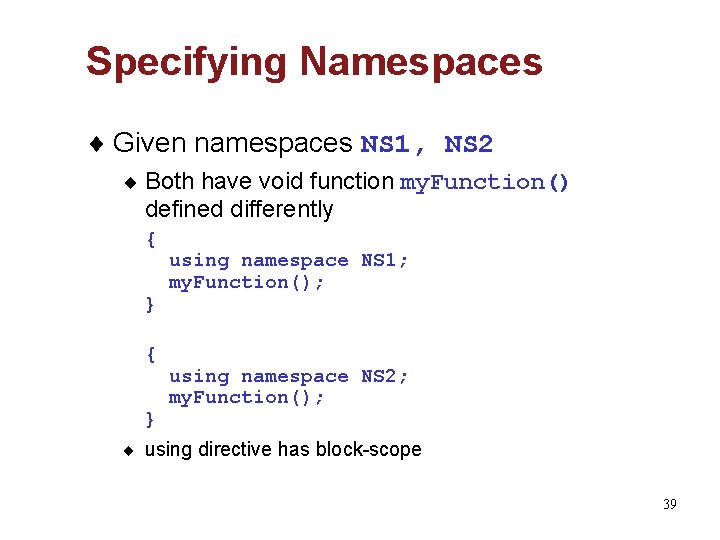
Specifying Namespaces ¨ Given namespaces NS 1, NS 2 ¨ Both have void function my. Function() defined differently { } using namespace NS 1; my. Function(); using namespace NS 2; my. Function(); ¨ using directive has block-scope 39
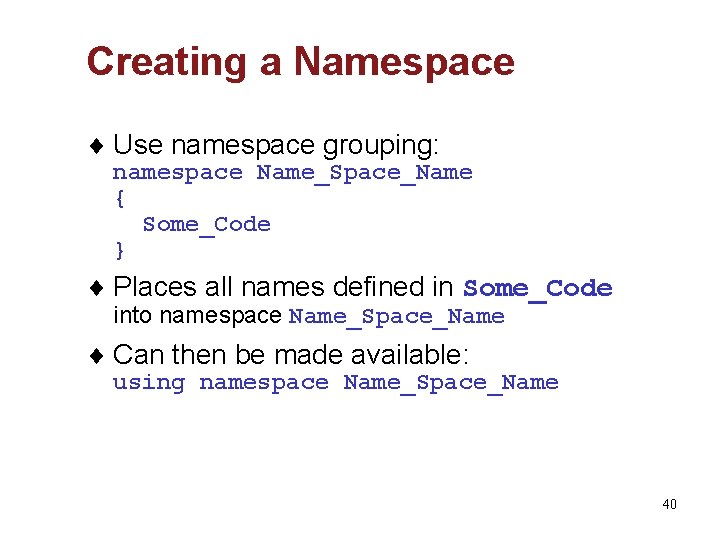
Creating a Namespace ¨ Use namespace grouping: namespace Name_Space_Name { Some_Code } ¨ Places all names defined in Some_Code into namespace Name_Space_Name ¨ Can then be made available: using namespace Name_Space_Name 40
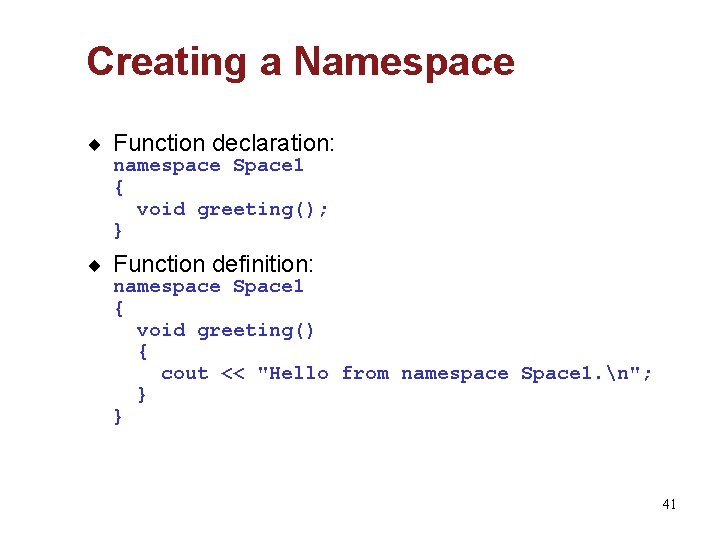
Creating a Namespace ¨ Function declaration: namespace Space 1 { void greeting(); } ¨ Function definition: namespace Space 1 { void greeting() { cout << "Hello from namespace Space 1. n"; } } 41
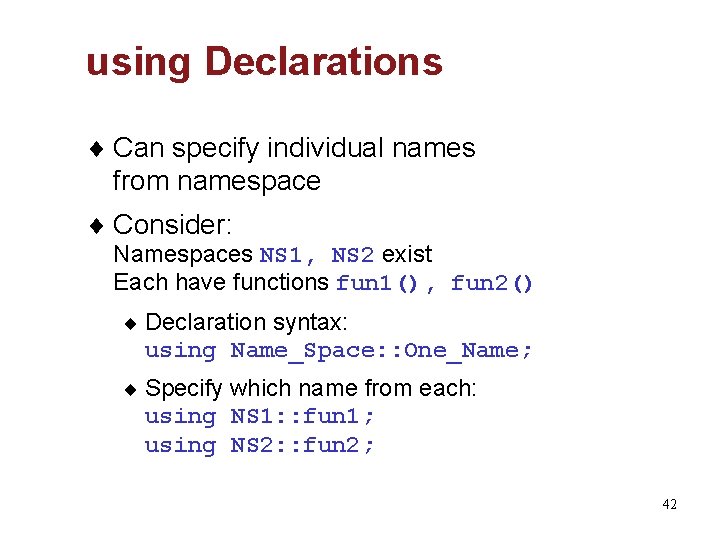
using Declarations ¨ Can specify individual names from namespace ¨ Consider: Namespaces NS 1, NS 2 exist Each have functions fun 1(), fun 2() ¨ Declaration syntax: using Name_Space: : One_Name; ¨ Specify which name from each: using NS 1: : fun 1; using NS 2: : fun 2; 42
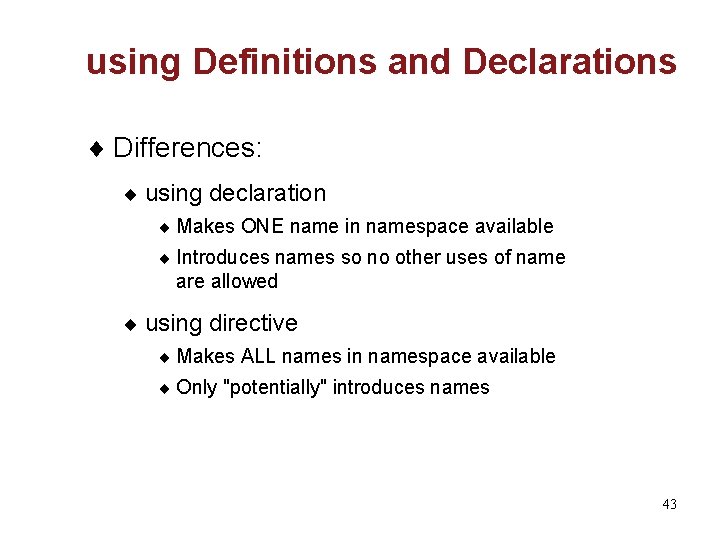
using Definitions and Declarations ¨ Differences: ¨ using declaration ¨ Makes ONE name in namespace available ¨ Introduces names so no other uses of name are allowed ¨ using directive ¨ Makes ALL names in namespace available ¨ Only "potentially" introduces names 43
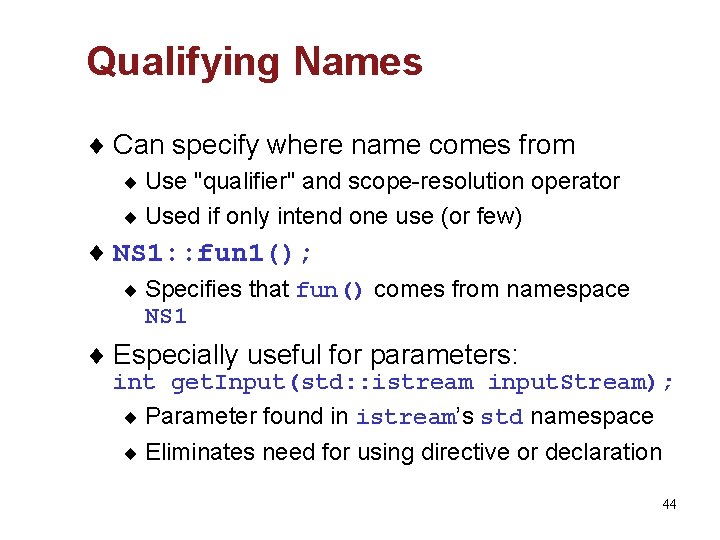
Qualifying Names ¨ Can specify where name comes from ¨ Use "qualifier" and scope-resolution operator ¨ Used if only intend one use (or few) ¨ NS 1: : fun 1(); ¨ Specifies that fun() comes from namespace NS 1 ¨ Especially useful for parameters: int get. Input(std: : istream input. Stream); ¨ Parameter found in istream’s std namespace ¨ Eliminates need for using directive or declaration 44
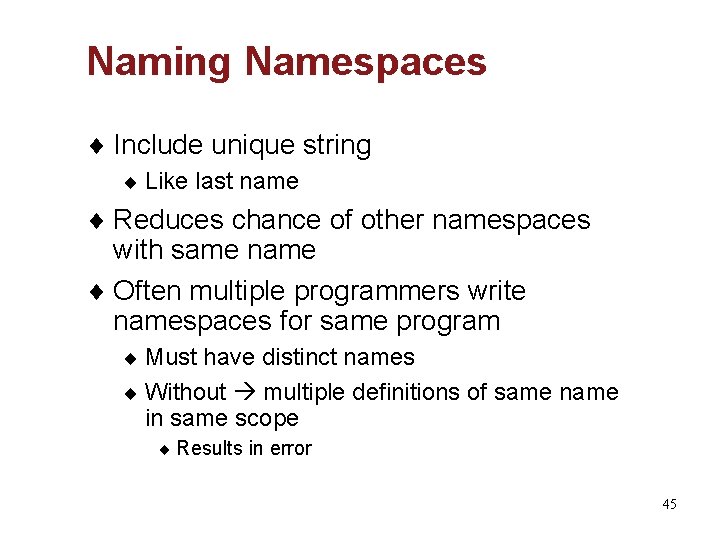
Naming Namespaces ¨ Include unique string ¨ Like last name ¨ Reduces chance of other namespaces with same name ¨ Often multiple programmers write namespaces for same program ¨ Must have distinct names ¨ Without multiple definitions of same name in same scope ¨ Results in error 45
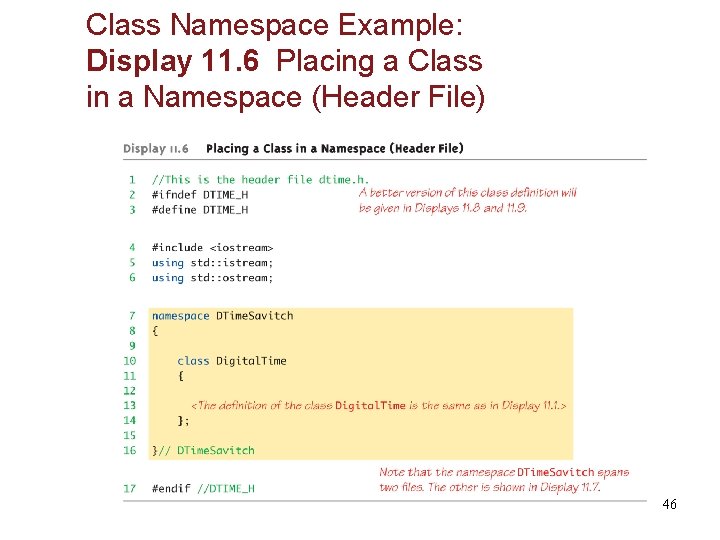
Class Namespace Example: Display 11. 6 Placing a Class in a Namespace (Header File) 46
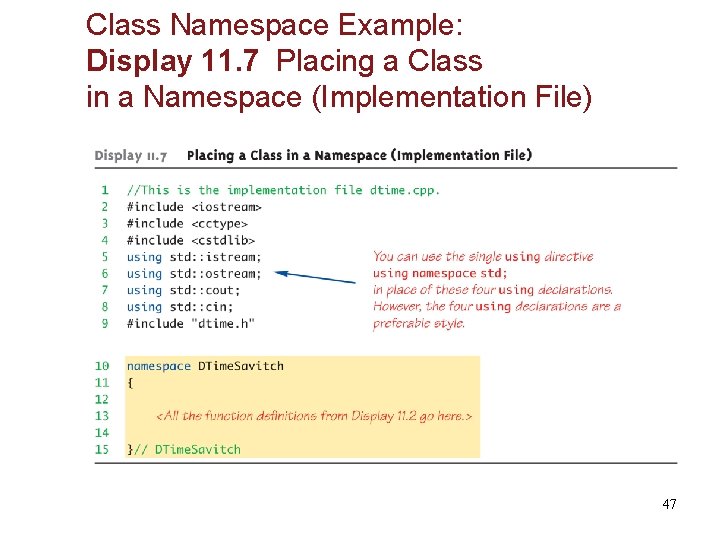
Class Namespace Example: Display 11. 7 Placing a Class in a Namespace (Implementation File) 47
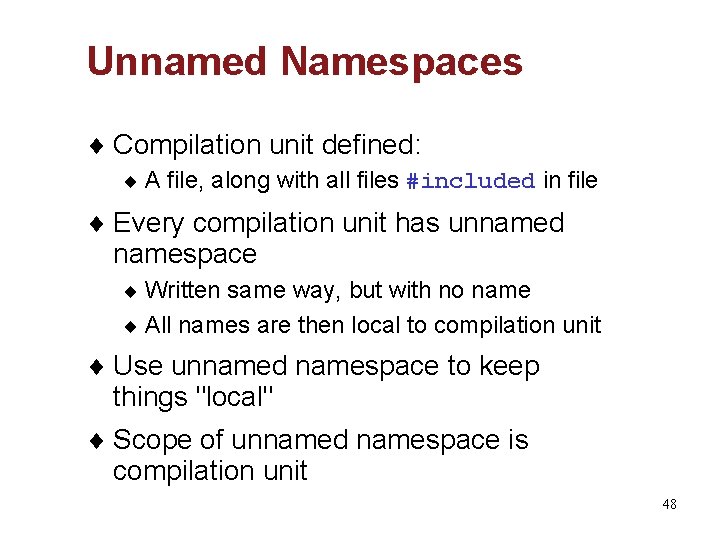
Unnamed Namespaces ¨ Compilation unit defined: ¨ A file, along with all files #included in file ¨ Every compilation unit has unnamed namespace ¨ Written same way, but with no name ¨ All names are then local to compilation unit ¨ Use unnamed namespace to keep things "local" ¨ Scope of unnamed namespace is compilation unit 48
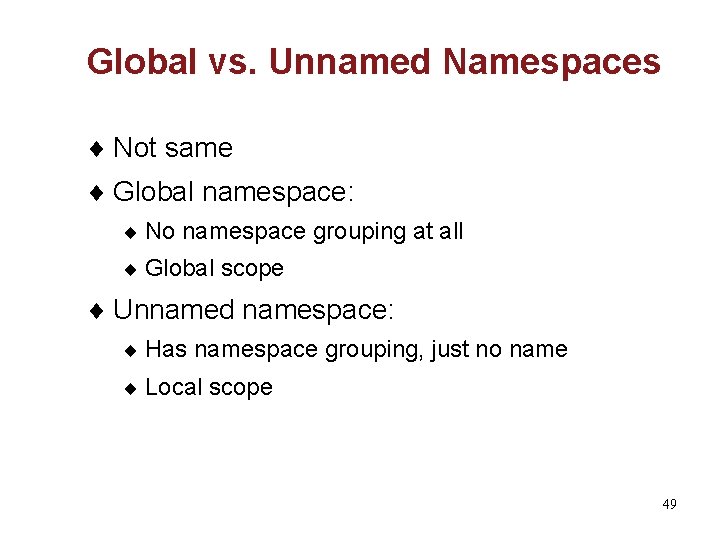
Global vs. Unnamed Namespaces ¨ Not same ¨ Global namespace: ¨ No namespace grouping at all ¨ Global scope ¨ Unnamed namespace: ¨ Has namespace grouping, just no name ¨ Local scope 49
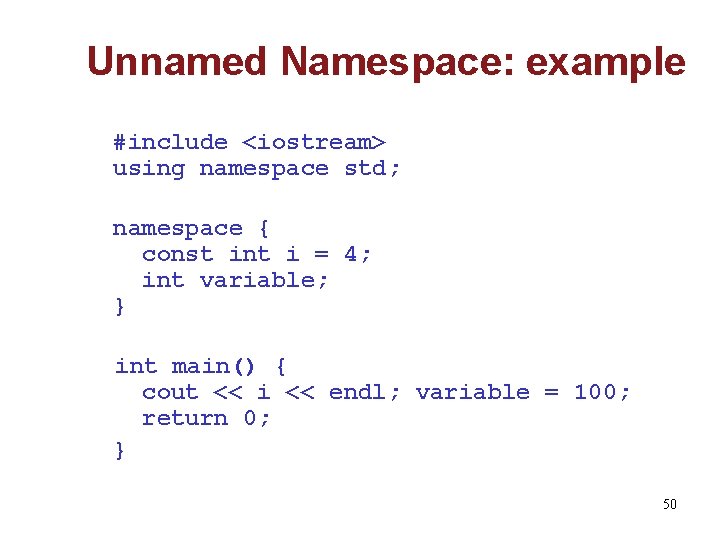
Unnamed Namespace: example #include <iostream> using namespace std; namespace { const int i = 4; int variable; } int main() { cout << i << endl; variable = 100; return 0; } 50
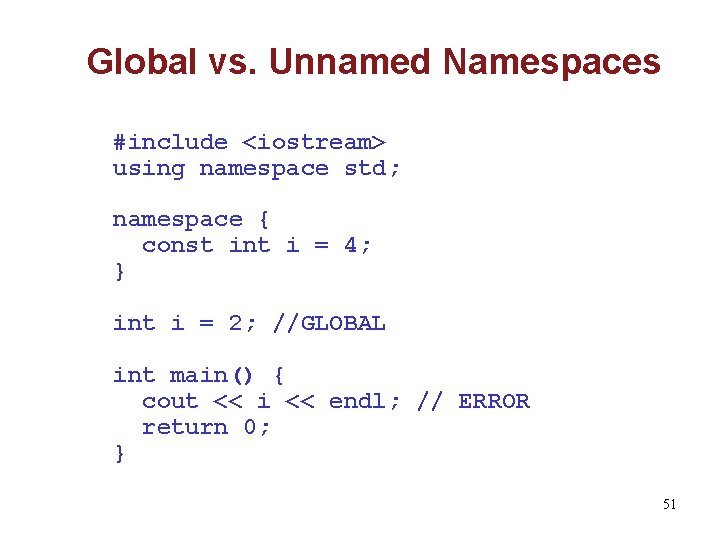
Global vs. Unnamed Namespaces #include <iostream> using namespace std; namespace { const int i = 4; } int i = 2; //GLOBAL int main() { cout << i << endl; // ERROR return 0; } 51
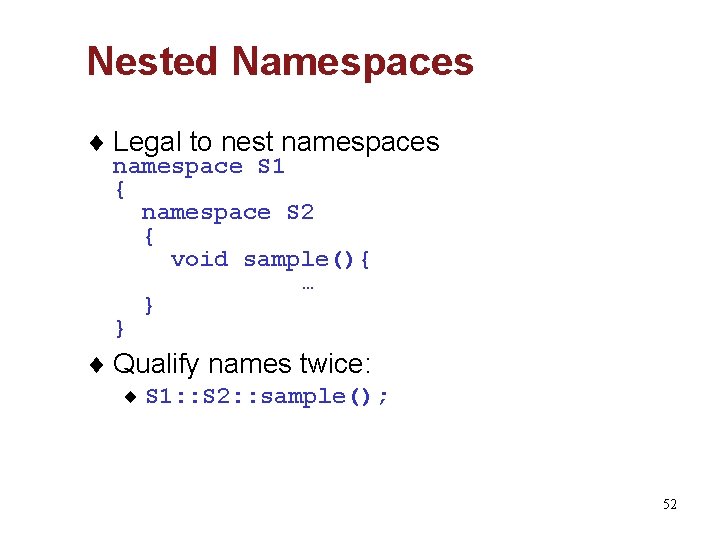
Nested Namespaces ¨ Legal to nest namespaces namespace S 1 { namespace S 2 { void sample(){ … } } ¨ Qualify names twice: ¨ S 1: : S 2: : sample(); 52
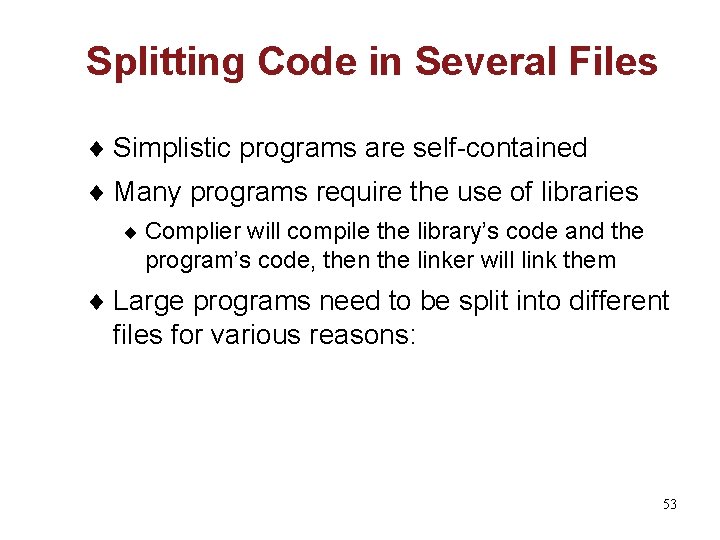
Splitting Code in Several Files ¨ Simplistic programs are self-contained ¨ Many programs require the use of libraries ¨ Complier will compile the library’s code and the program’s code, then the linker will link them ¨ Large programs need to be split into different files for various reasons: 53
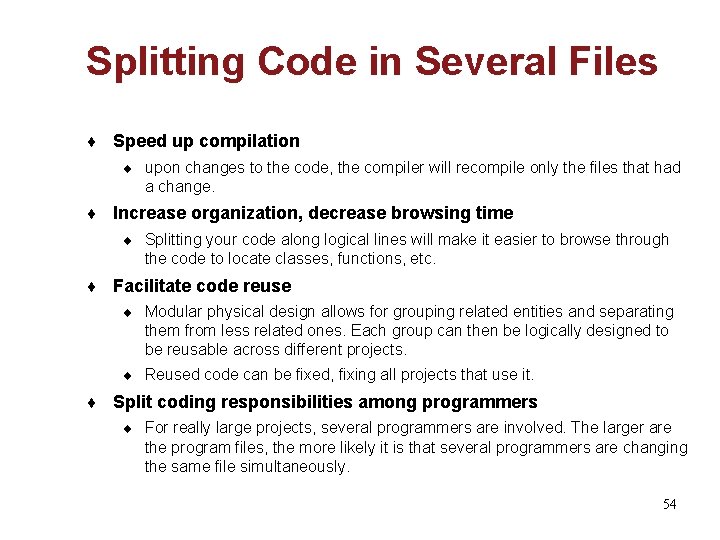
Splitting Code in Several Files ¨ Speed up compilation ¨ upon changes to the code, the compiler will recompile only the files that had a change. ¨ Increase organization, decrease browsing time ¨ Splitting your code along logical lines will make it easier to browse through the code to locate classes, functions, etc. ¨ Facilitate code reuse ¨ Modular physical design allows for grouping related entities and separating them from less related ones. Each group can then be logically designed to be reusable across different projects. ¨ Reused code can be fixed, fixing all projects that use it. ¨ Split coding responsibilities among programmers ¨ For really large projects, several programmers are involved. The larger are the program files, the more likely it is that several programmers are changing the same file simultaneously. 54
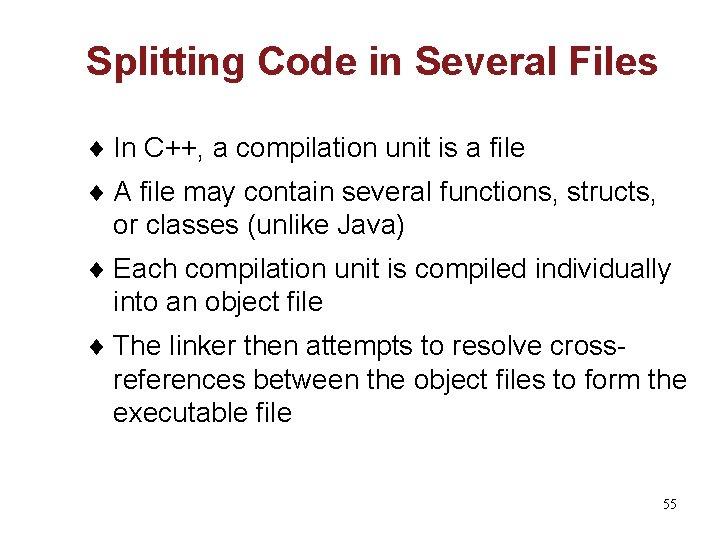
Splitting Code in Several Files ¨ In C++, a compilation unit is a file ¨ A file may contain several functions, structs, or classes (unlike Java) ¨ Each compilation unit is compiled individually into an object file ¨ The linker then attempts to resolve cross- references between the object files to form the executable file 55
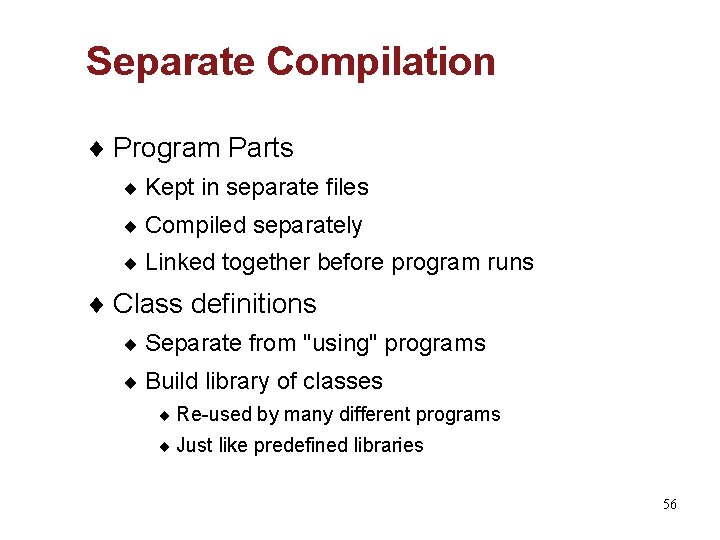
Separate Compilation ¨ Program Parts ¨ Kept in separate files ¨ Compiled separately ¨ Linked together before program runs ¨ Class definitions ¨ Separate from "using" programs ¨ Build library of classes ¨ Re-used by many different programs ¨ Just like predefined libraries 56
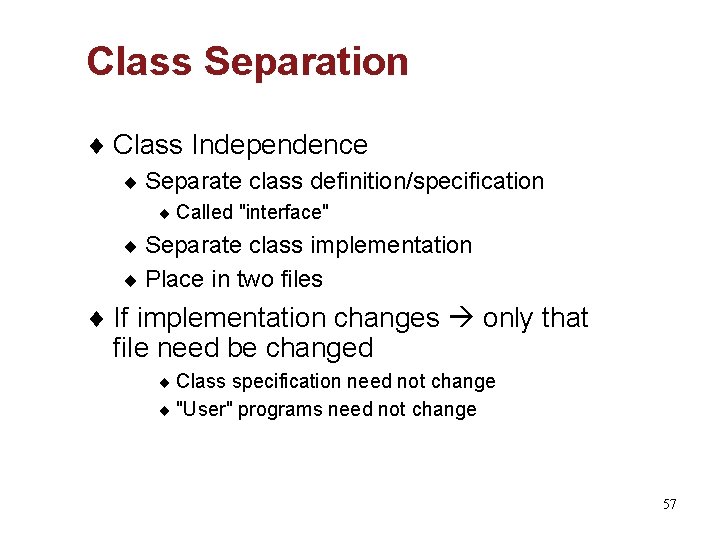
Class Separation ¨ Class Independence ¨ Separate class definition/specification ¨ Called "interface" ¨ Separate class implementation ¨ Place in two files ¨ If implementation changes only that file need be changed ¨ Class specification need not change ¨ "User" programs need not change 57
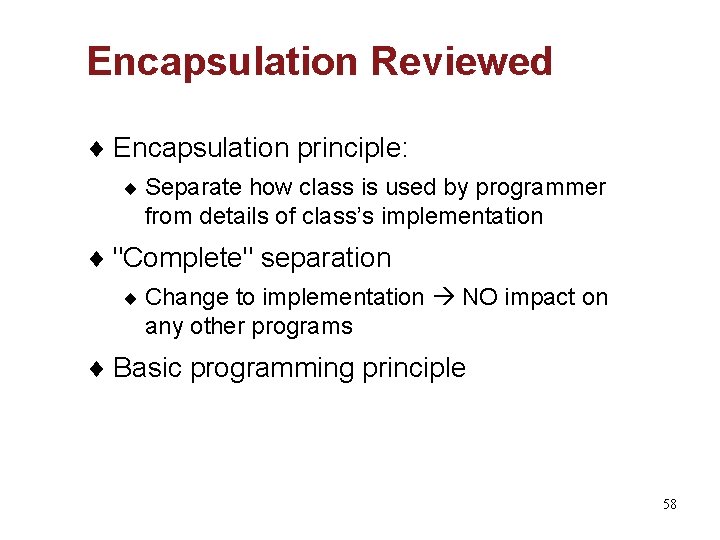
Encapsulation Reviewed ¨ Encapsulation principle: ¨ Separate how class is used by programmer from details of class’s implementation ¨ "Complete" separation ¨ Change to implementation NO impact on any other programs ¨ Basic programming principle 58
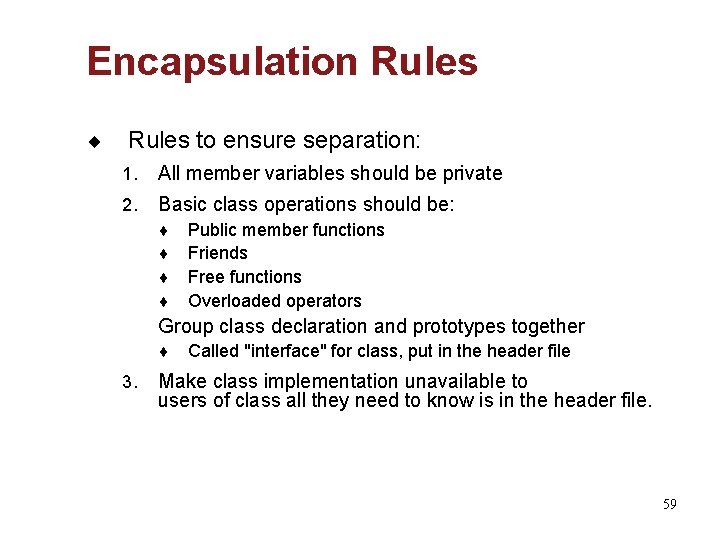
Encapsulation Rules ¨ Rules to ensure separation: 1. All member variables should be private 2. Basic class operations should be: ¨ ¨ Public member functions Friends Free functions Overloaded operators Group class declaration and prototypes together ¨ 3. Called "interface" for class, put in the header file Make class implementation unavailable to users of class all they need to know is in the header file. 59
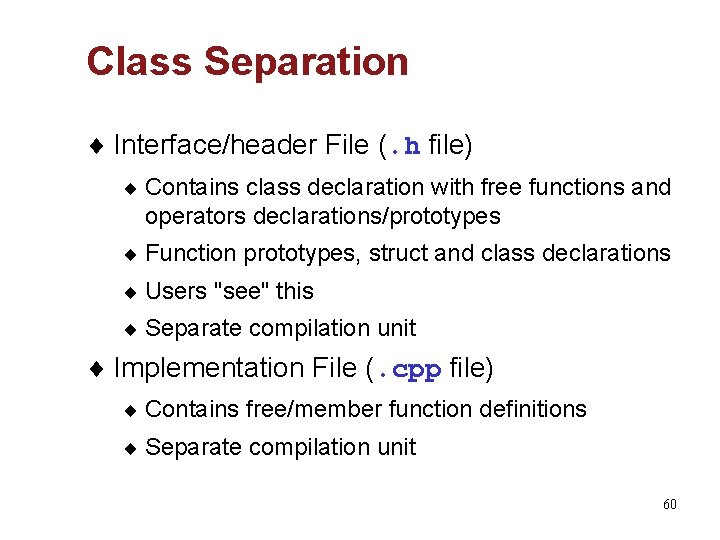
Class Separation ¨ Interface/header File (. h file) ¨ Contains class declaration with free functions and operators declarations/prototypes ¨ Function prototypes, struct and class declarations ¨ Users "see" this ¨ Separate compilation unit ¨ Implementation File (. cpp file) ¨ Contains free/member function definitions ¨ Separate compilation unit 60
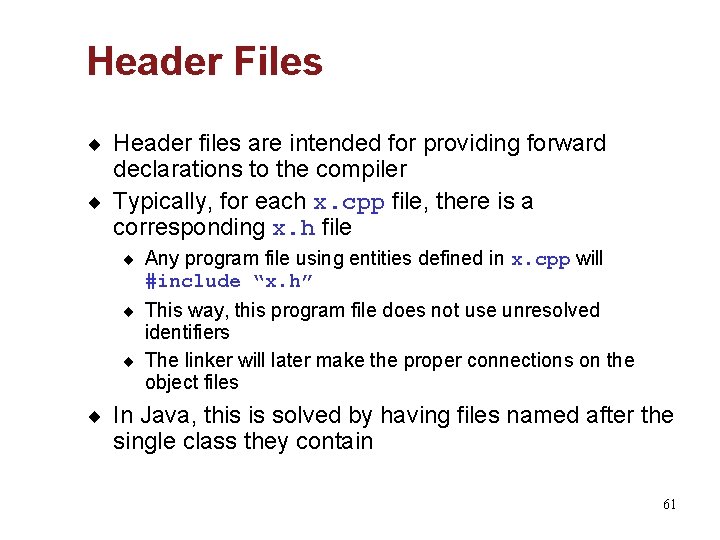
Header Files ¨ Header files are intended for providing forward declarations to the compiler ¨ Typically, for each x. cpp file, there is a corresponding x. h file ¨ Any program file using entities defined in x. cpp will #include “x. h” ¨ This way, this program file does not use unresolved identifiers ¨ The linker will later make the proper connections on the object files ¨ In Java, this is solved by having files named after the single class they contain 61
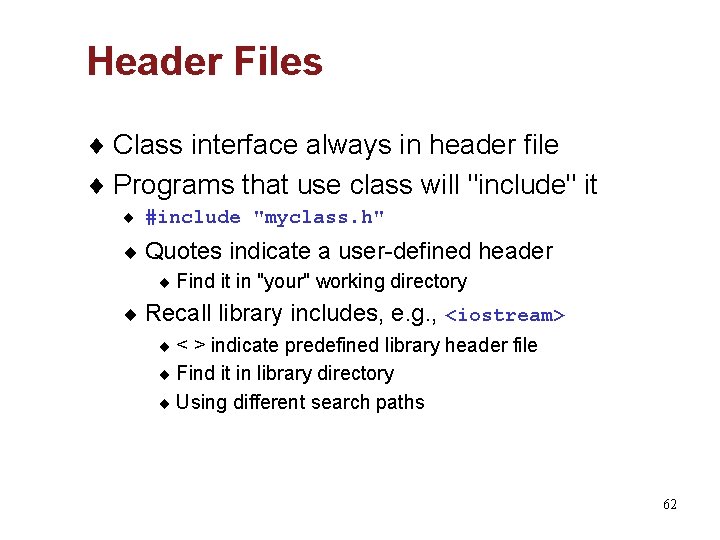
Header Files ¨ Class interface always in header file ¨ Programs that use class will "include" it ¨ #include "myclass. h" ¨ Quotes indicate a user-defined header ¨ Find it in "your" working directory ¨ Recall library includes, e. g. , <iostream> ¨ < > indicate predefined library header file ¨ Find it in library directory ¨ Using different search paths 62
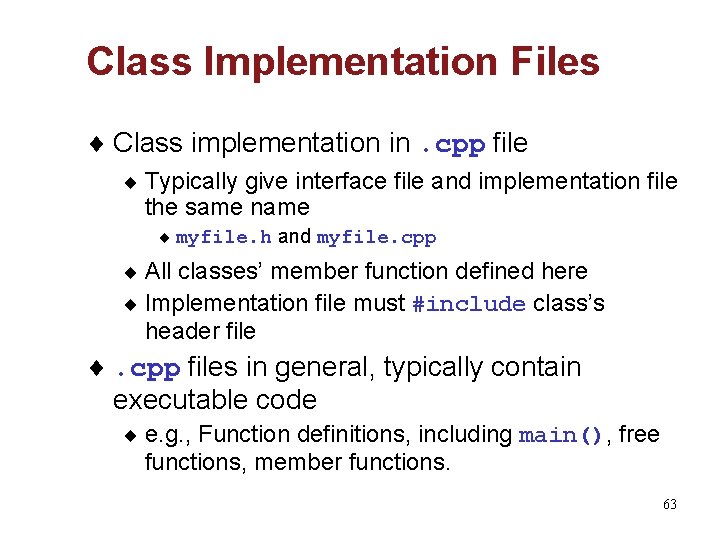
Class Implementation Files ¨ Class implementation in. cpp file ¨ Typically give interface file and implementation file the same name ¨ myfile. h and myfile. cpp ¨ All classes’ member function defined here ¨ Implementation file must #include class’s header file ¨. cpp files in general, typically contain executable code ¨ e. g. , Function definitions, including main(), free functions, member functions. 63
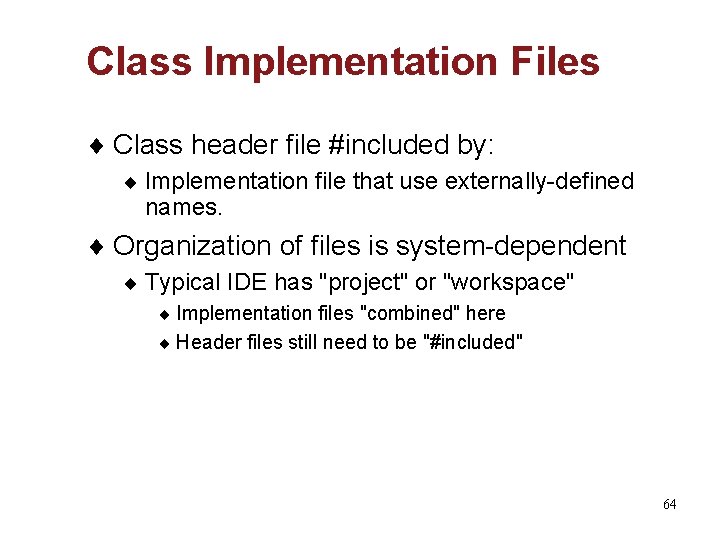
Class Implementation Files ¨ Class header file #included by: ¨ Implementation file that use externally-defined names. ¨ Organization of files is system-dependent ¨ Typical IDE has "project" or "workspace" ¨ Implementation files "combined" here ¨ Header files still need to be "#included" 64
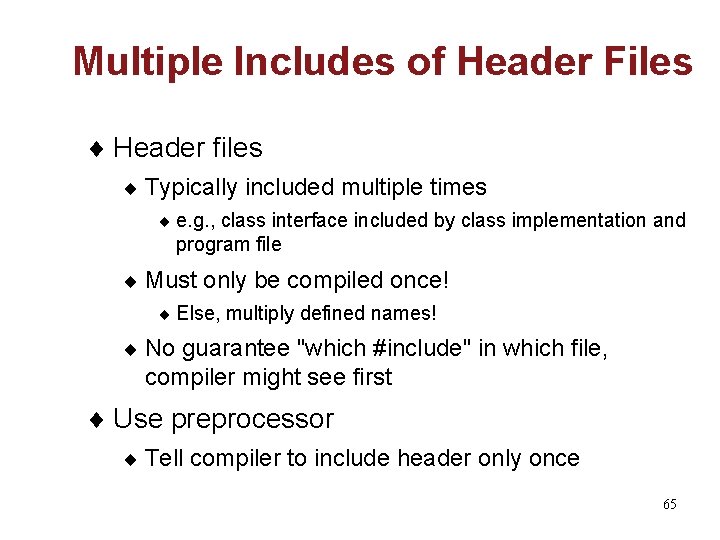
Multiple Includes of Header Files ¨ Header files ¨ Typically included multiple times ¨ e. g. , class interface included by class implementation and program file ¨ Must only be compiled once! ¨ Else, multiply defined names! ¨ No guarantee "which #include" in which file, compiler might see first ¨ Use preprocessor ¨ Tell compiler to include header only once 65
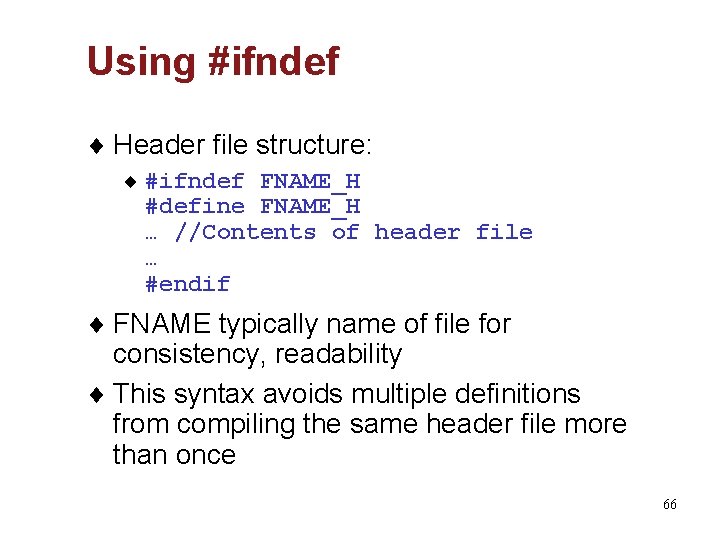
Using #ifndef ¨ Header file structure: ¨ #ifndef FNAME_H #define FNAME_H … //Contents of header file … #endif ¨ FNAME typically name of file for consistency, readability ¨ This syntax avoids multiple definitions from compiling the same header file more than once 66
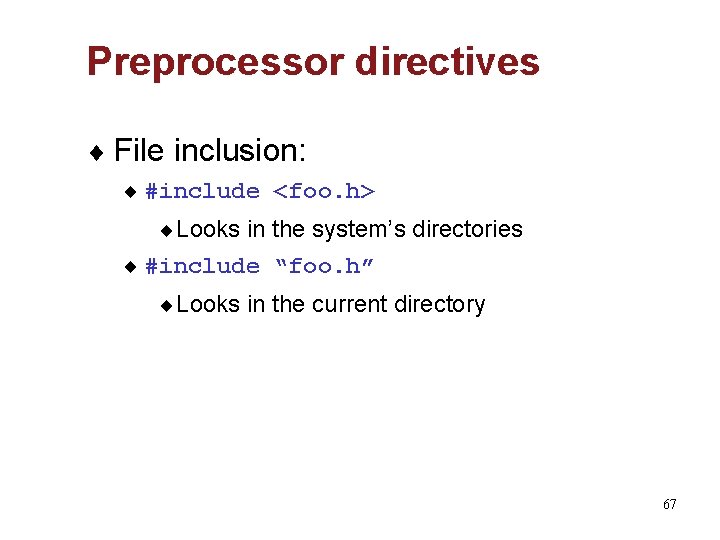
Preprocessor directives ¨ File inclusion: ¨ #include <foo. h> ¨ Looks in the system’s directories ¨ #include “foo. h” ¨ Looks in the current directory 67
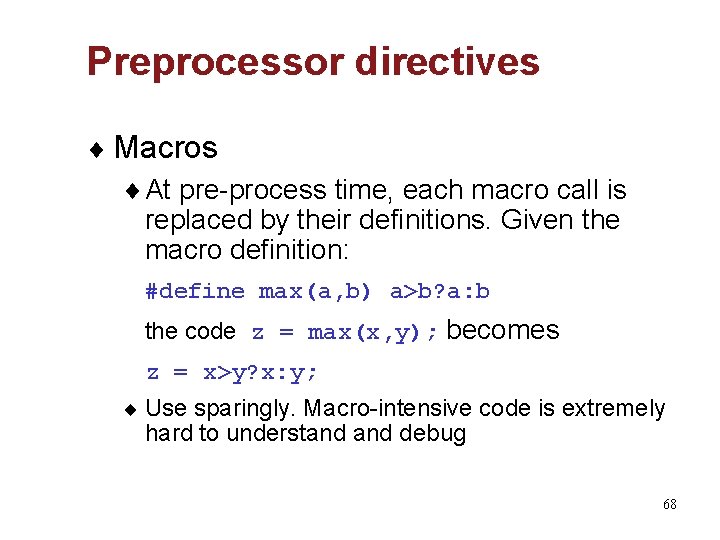
Preprocessor directives ¨ Macros ¨ At pre-process time, each macro call is replaced by their definitions. Given the macro definition: #define max(a, b) a>b? a: b the code z = max(x, y); becomes z = x>y? x: y; ¨ Use sparingly. Macro-intensive code is extremely hard to understand debug 68
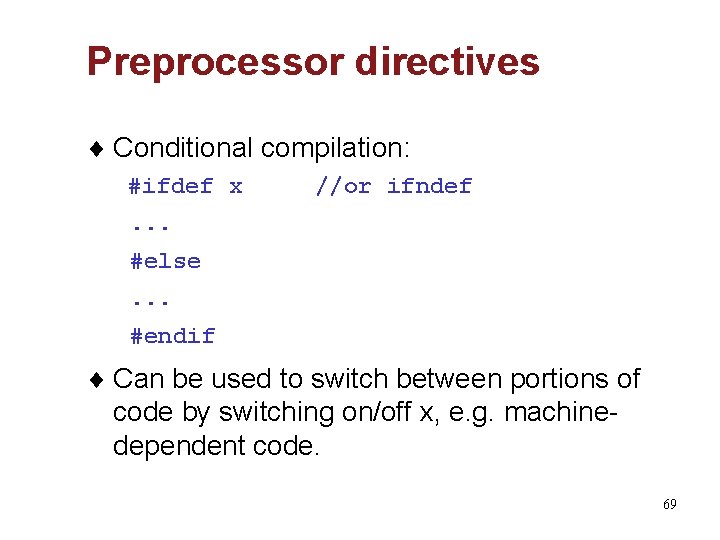
Preprocessor directives ¨ Conditional compilation: #ifdef x //or ifndef . . . #else. . . #endif ¨ Can be used to switch between portions of code by switching on/off x, e. g. machinedependent code. 69
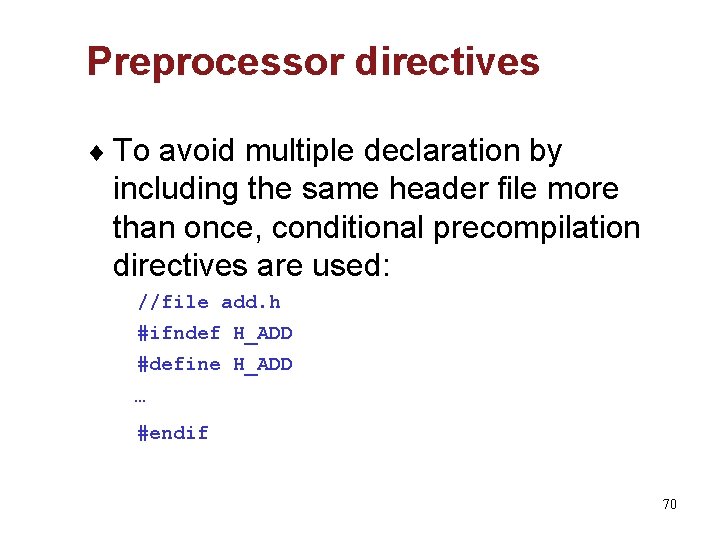
Preprocessor directives ¨ To avoid multiple declaration by including the same header file more than once, conditional precompilation directives are used: //file add. h #ifndef H_ADD #define H_ADD … #endif 70
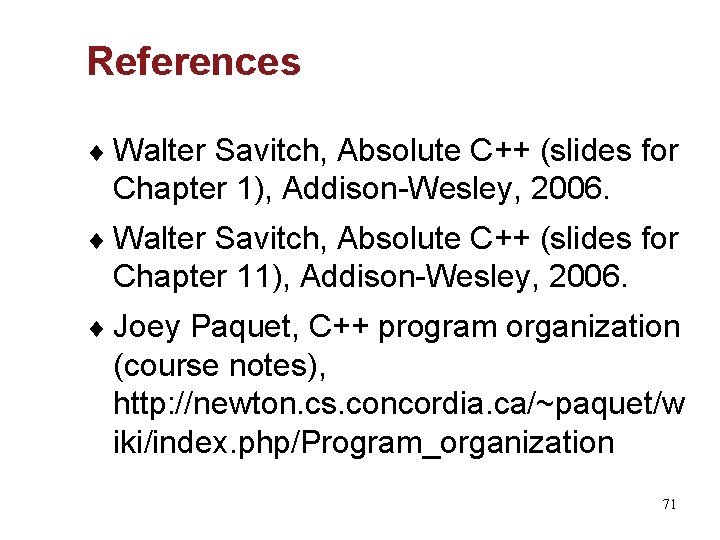
References ¨ Walter Savitch, Absolute C++ (slides for Chapter 1), Addison-Wesley, 2006. ¨ Walter Savitch, Absolute C++ (slides for Chapter 11), Addison-Wesley, 2006. ¨ Joey Paquet, C++ program organization (course notes), http: //newton. cs. concordia. ca/~paquet/w iki/index. php/Program_organization 71
Columbia university department of computer science
Concordia university mechanical engineering
Software engineering concordia
Software engineering concordia
Software engineering concordia
Ucl computer science
Electrical engineering northwestern
Computer science department rutgers
Computer science department stanford
Florida state university masters in computer science
Ubc computer science department
Bhargavi goswami
My favourite science
Computer science university of phoenix
University of bridgeport computer science
University of bridgeport computer science
Yonsei university computer science
York university computer science
Unc chapel hill computer science
Seoul national university computer science
Osaka university computer science
Computer science columbia university
Towson university computer science
Kstate computer science
Brown university computer science faculty
Trinity university computer science
Brandeis university computer science
University
Kotebe metropolitan university
Software engineering vs computer science
Computer science software engineering
Computer science software engineering
Sofiene tahar
Iso concordia drop in
Soen 6441
Cameron skinner concordia
Concordia international school hong kong
Aiman hanna concordia
Titanic vs costa concordia
Diocesi concordia pordenone ufficio catechistico
Anjali agarwal concordia
Machine learning project presentation
Concordia r2
Banweb concordia
Graziano diritto canonico
Hay quienes pretenden ser ricos
Grad pro skills
Concordia originality form
Iit delhi material science faculty
Tum department of electrical and computer engineering
Department of law university of jammu
Department of geology university of dhaka
Mechanicistic
University of bridgeport it department
University of iowa math
Department of physics university of tokyo
Texas state university psychology
Department of information engineering university of padova
Information engineering padova
Manipal university chemistry department
Syracuse university psychology department
Jackson state university finance department
Michigan state astronomy
Columbia university cs department
University of sargodha engineering department
Stanford university philosophy department
Department of forensic science dc
Ohio tpes
Computer engineering department
University of science and technology of hanoi
Spike unist
Science technology university yemen