COMP 110 Introduction to Programming Tyler Johnson Apr
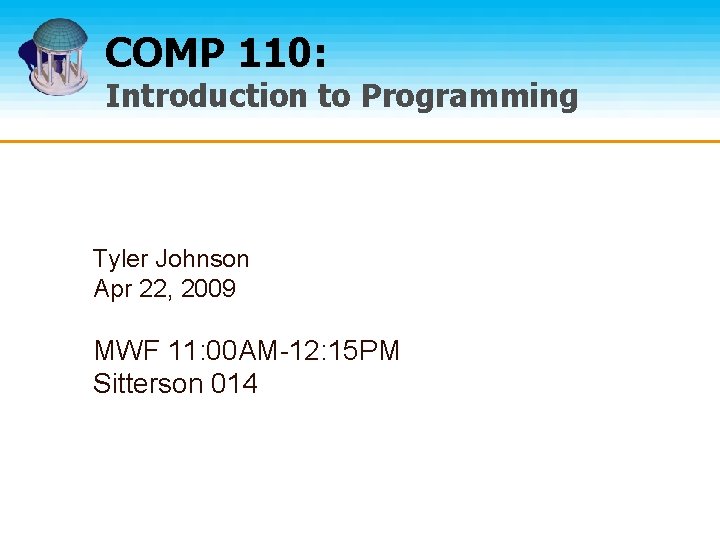
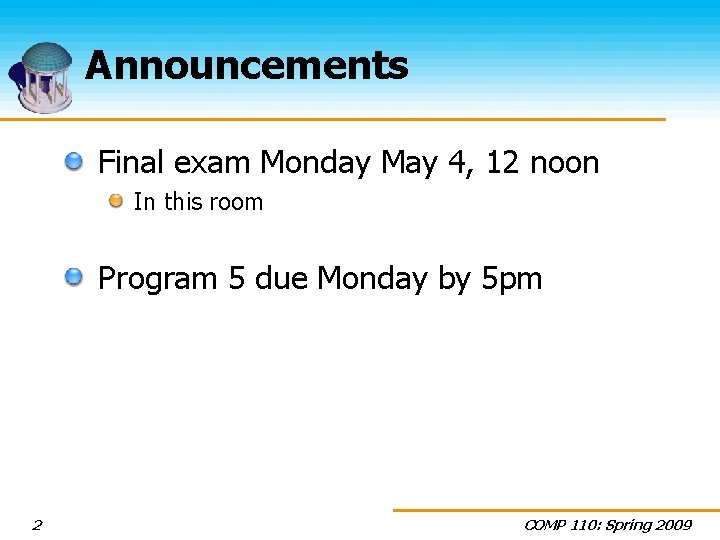
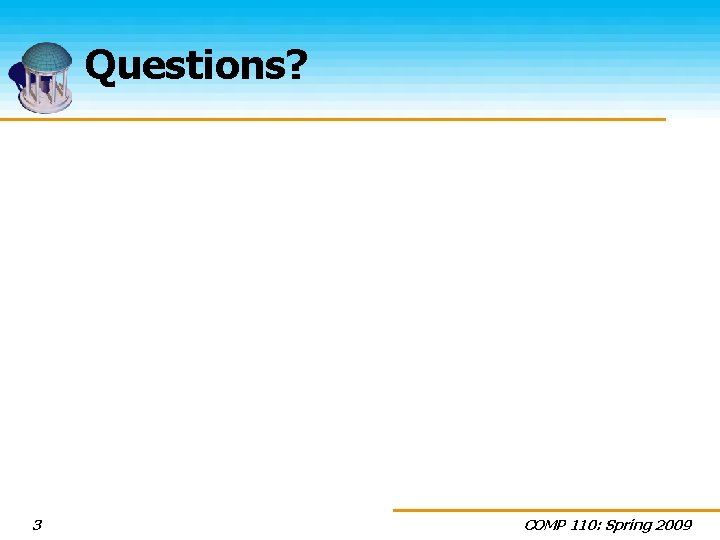
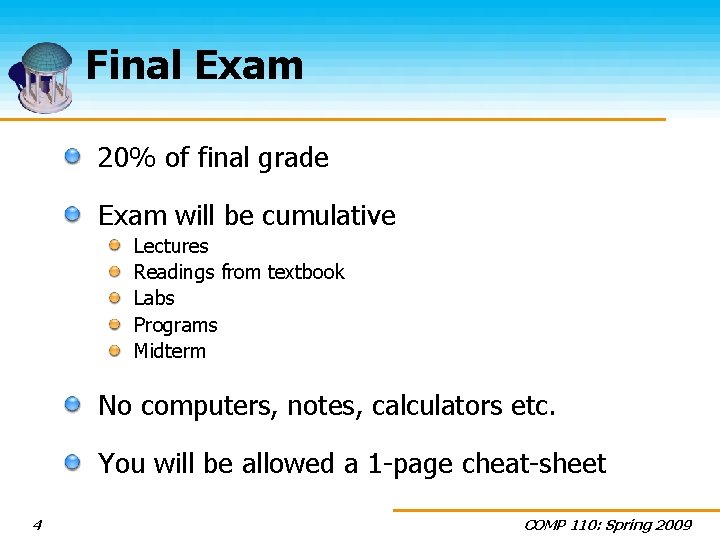
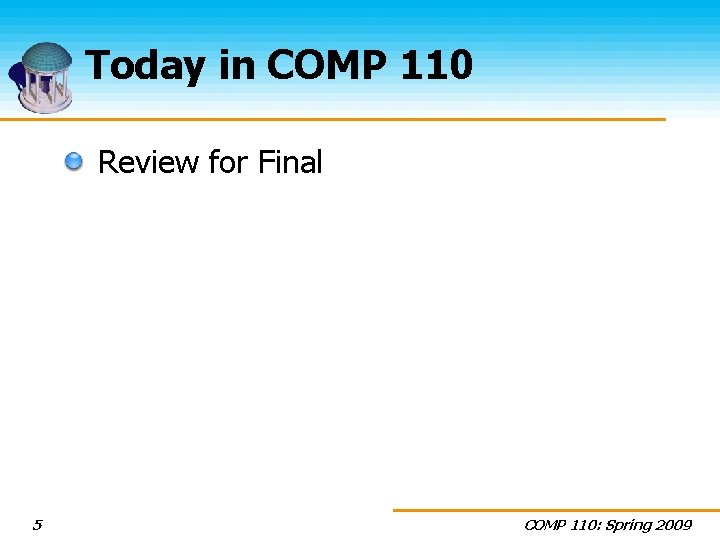
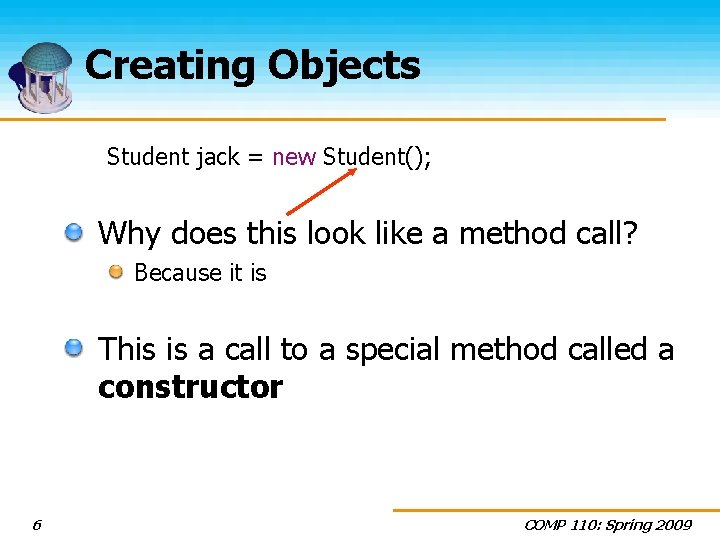
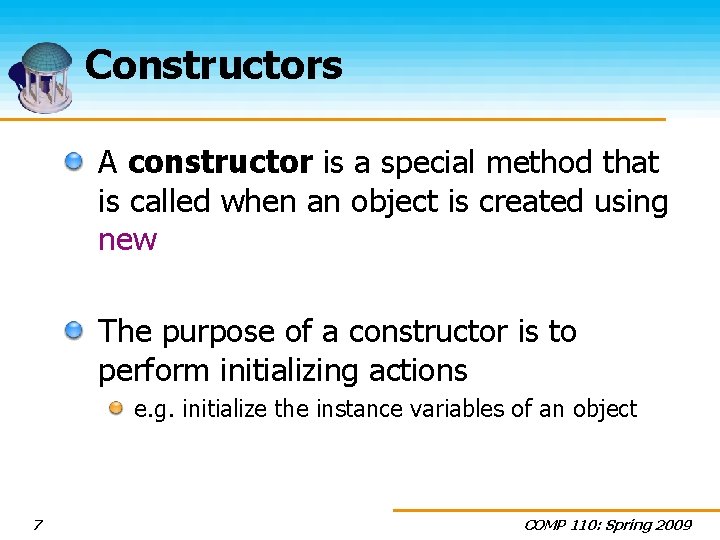
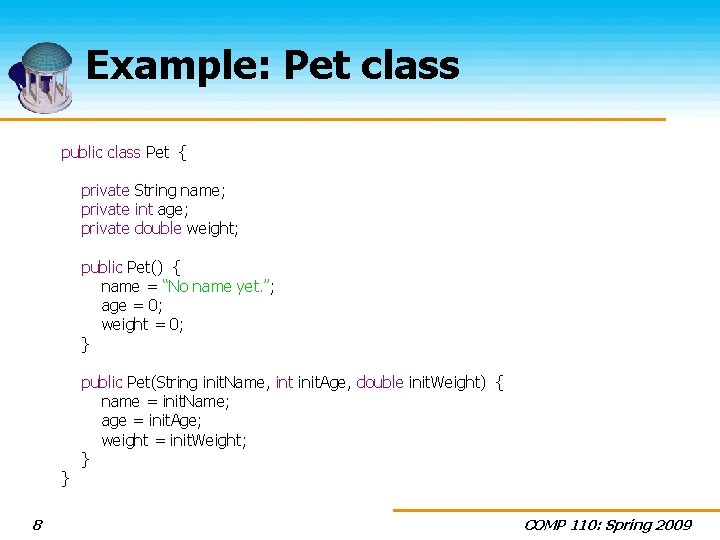
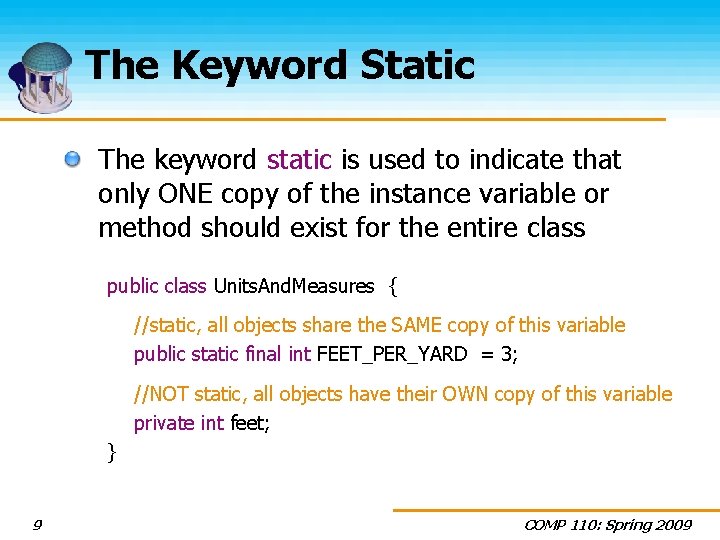
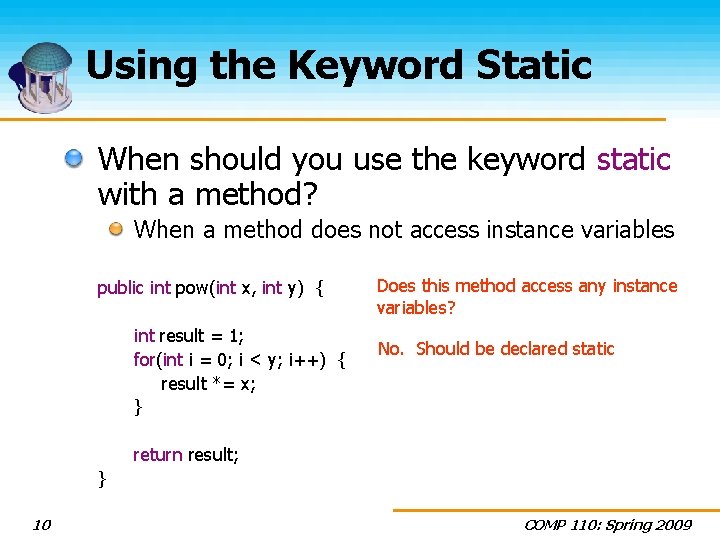
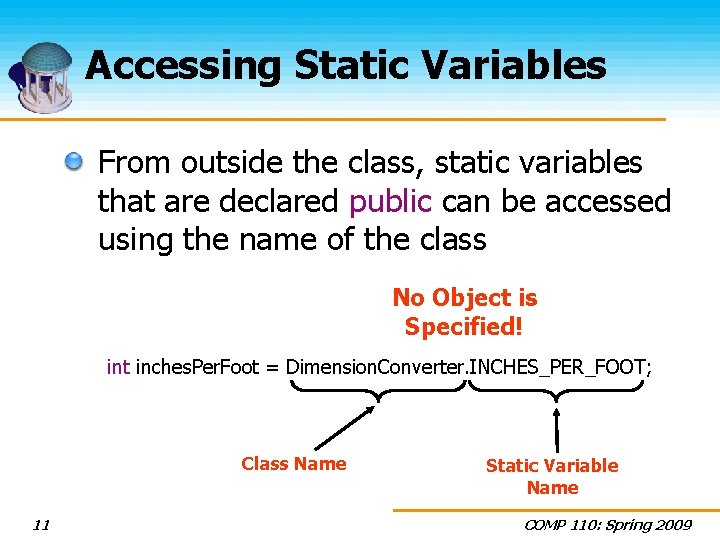
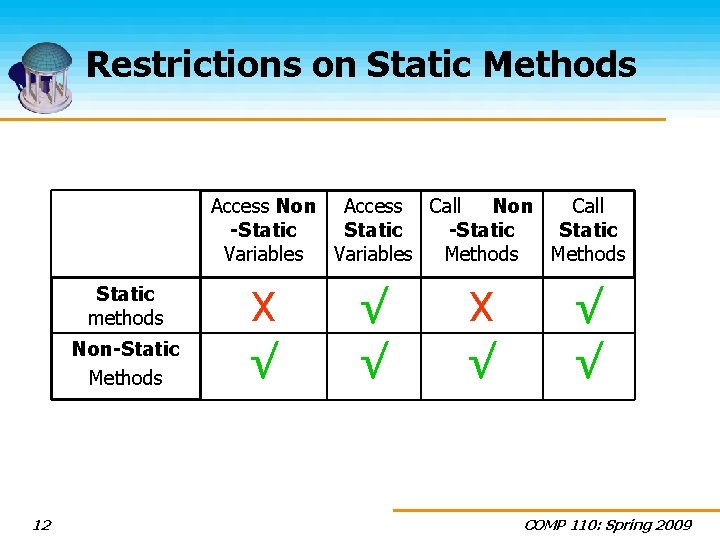
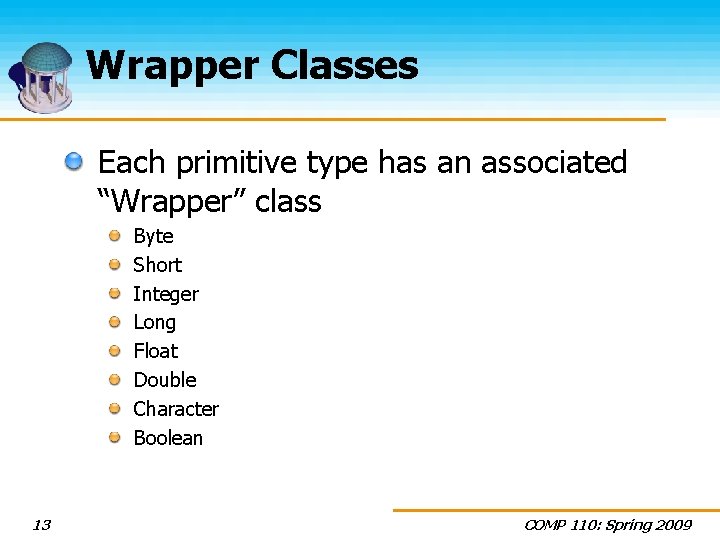
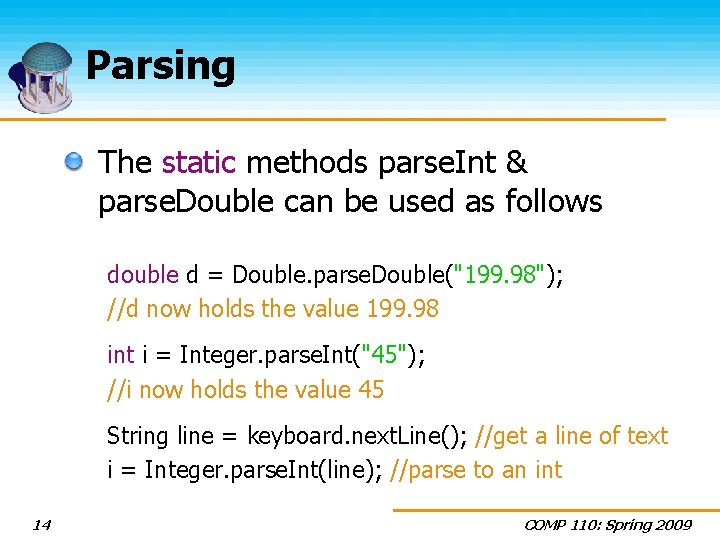
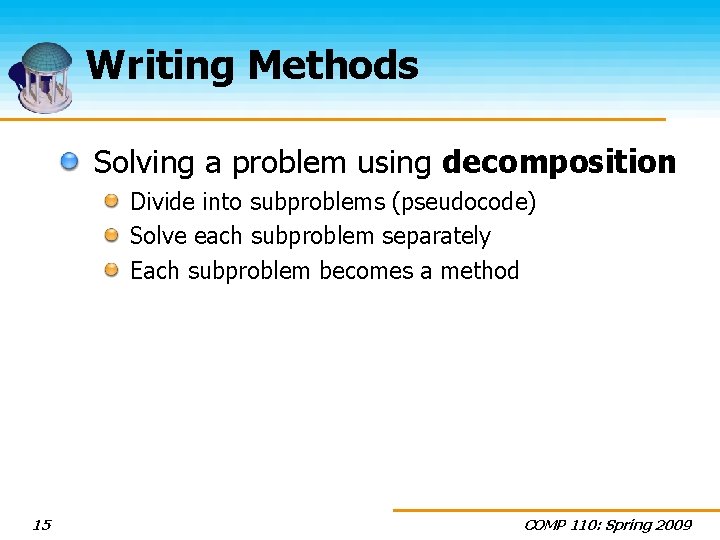
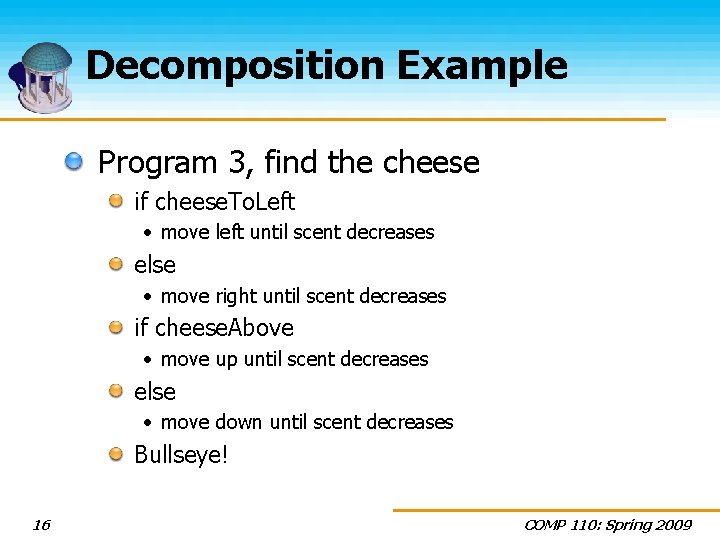
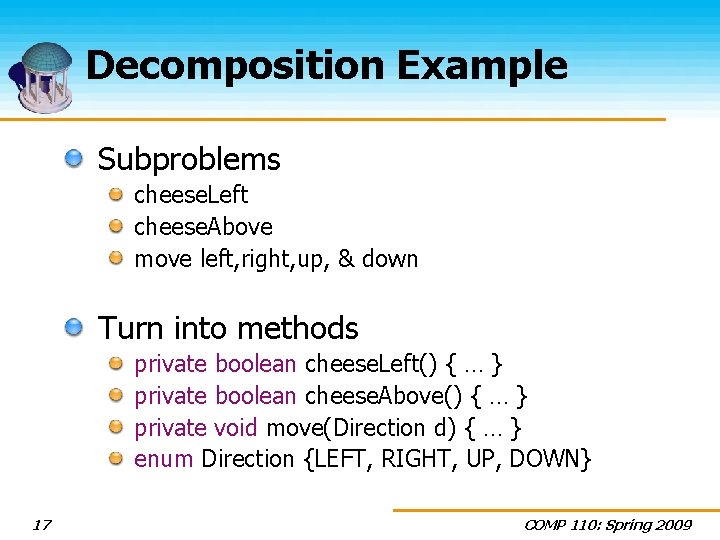
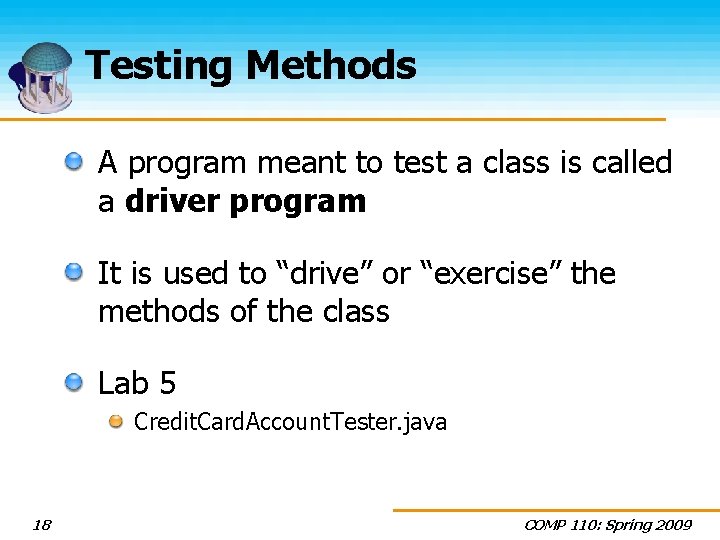
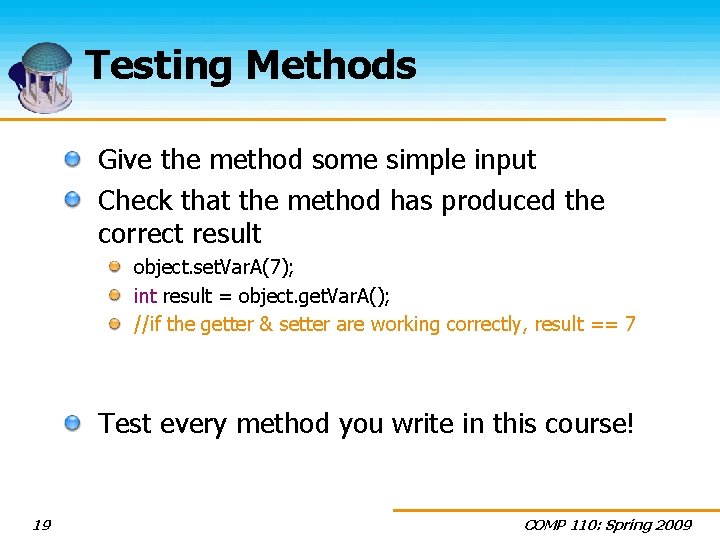
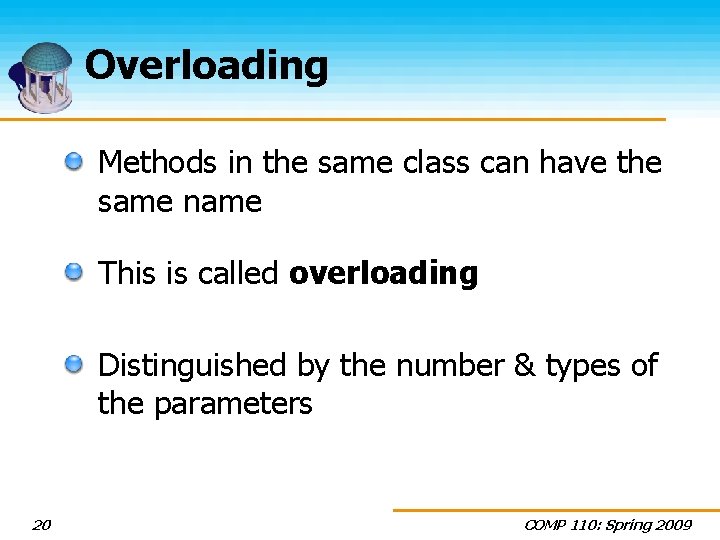
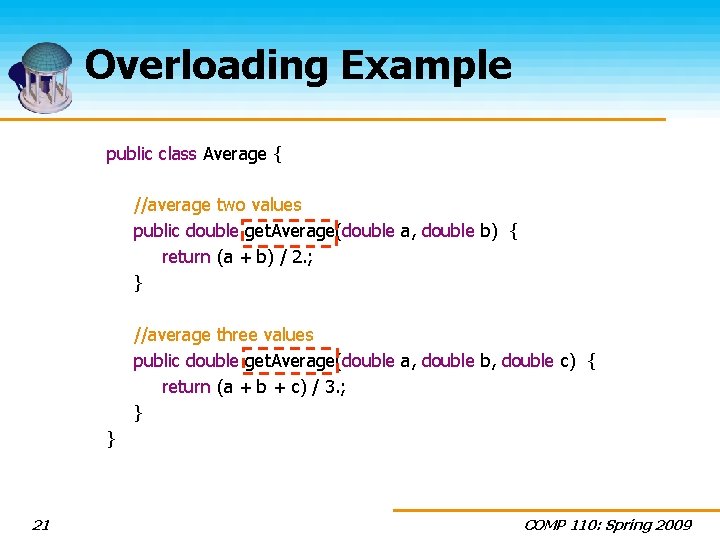
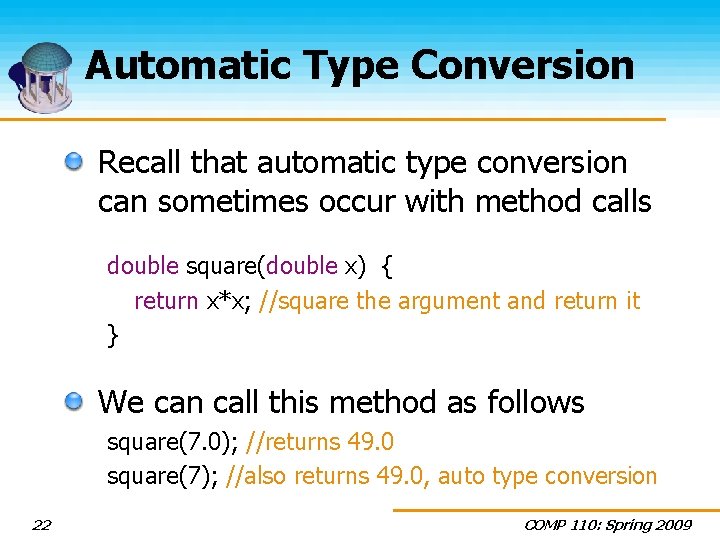
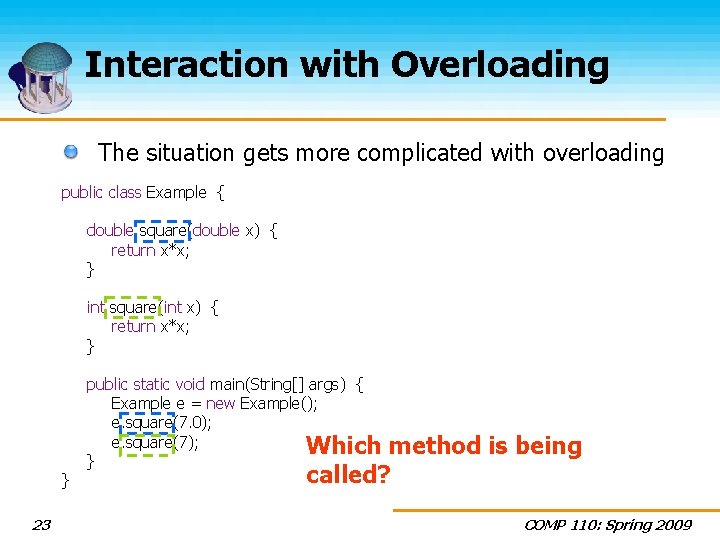
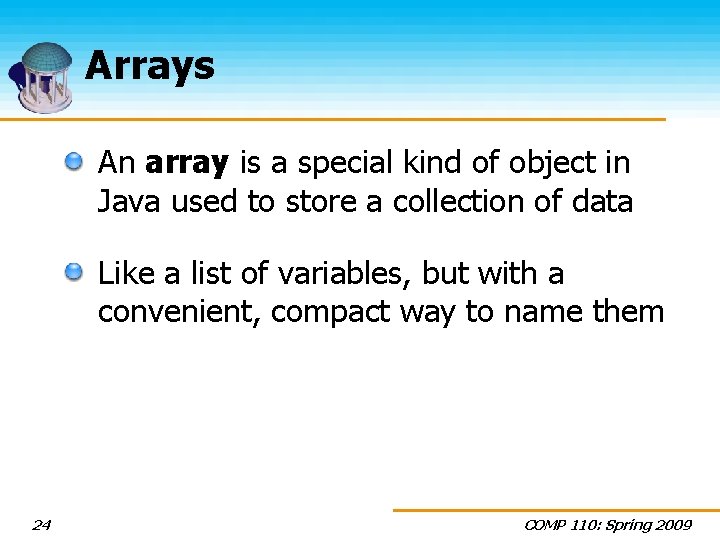
![Creating an Array int[] scores = new int[5]; //create 5 ints This creates an Creating an Array int[] scores = new int[5]; //create 5 ints This creates an](https://slidetodoc.com/presentation_image_h2/4fd40fd9b43e2d20d21169efb1ac904b/image-25.jpg)
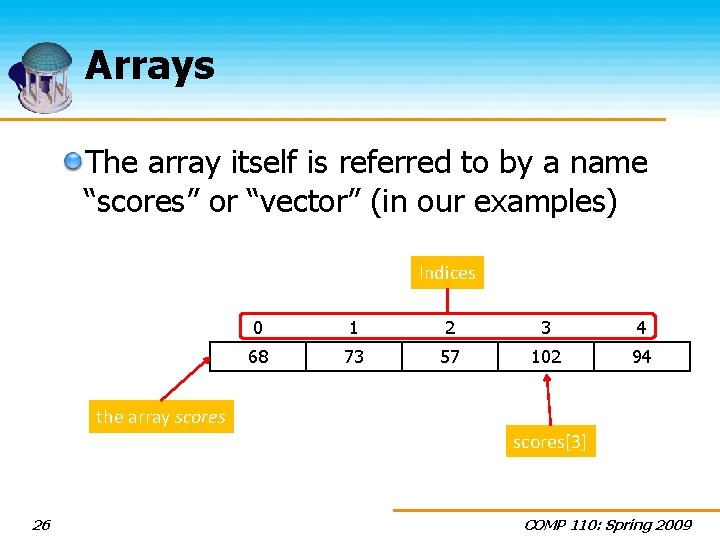
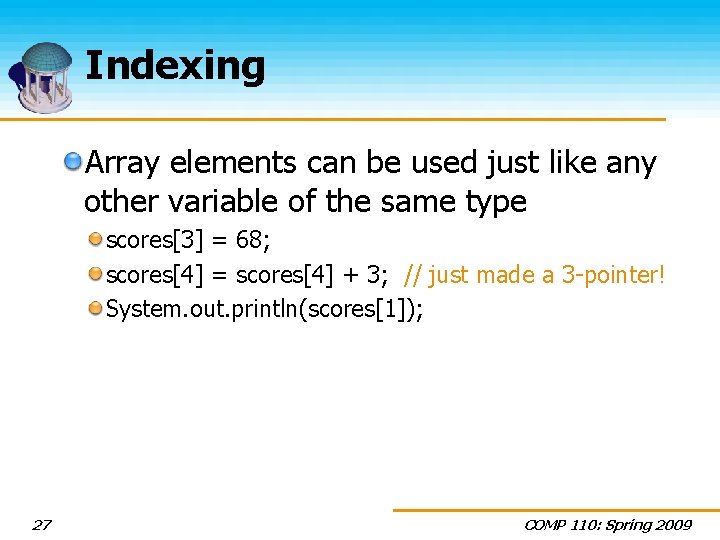
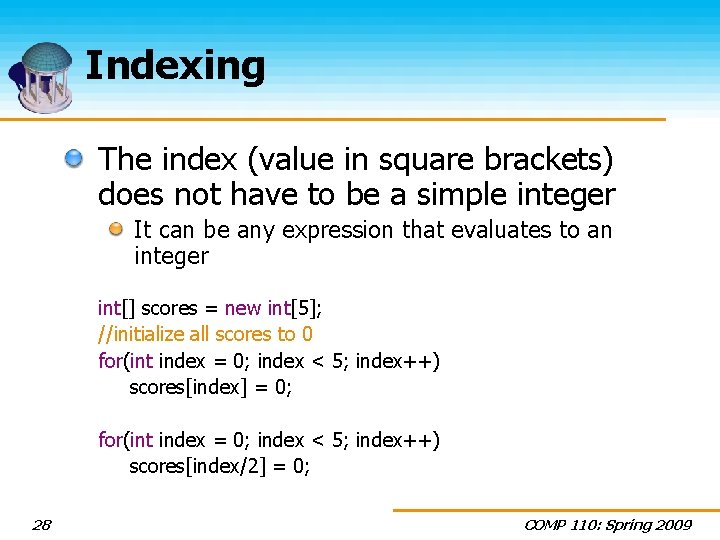
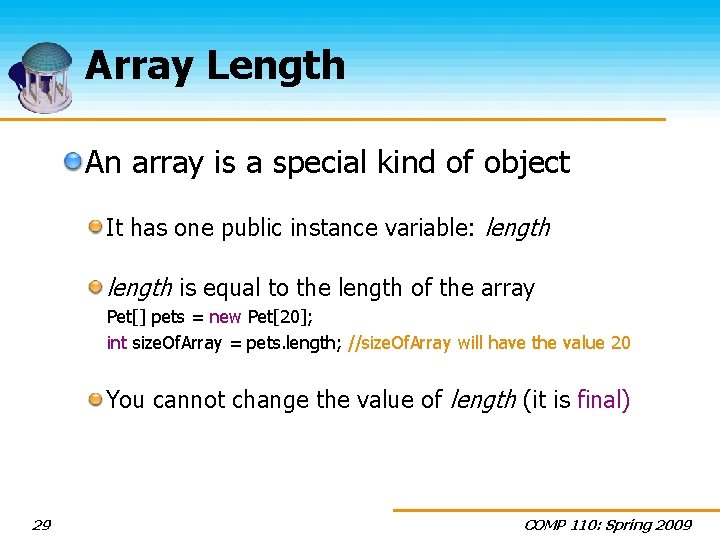
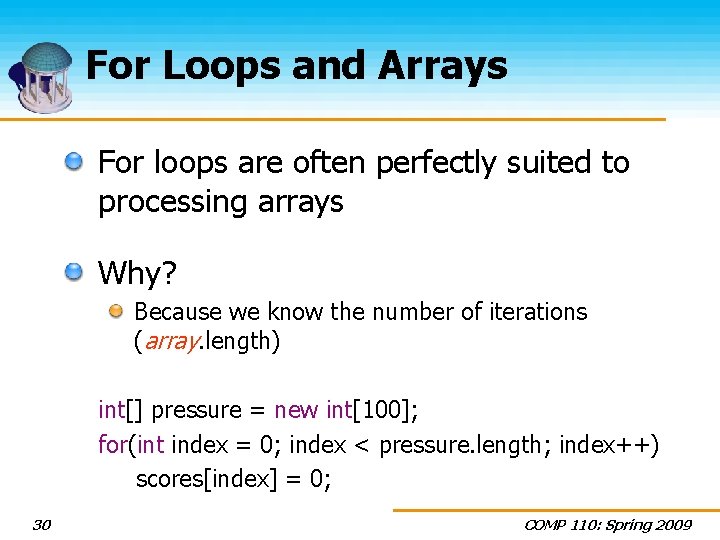
![Be Careful with Indices MUST be in bounds double[] entries = new double[5]; entries[5] Be Careful with Indices MUST be in bounds double[] entries = new double[5]; entries[5]](https://slidetodoc.com/presentation_image_h2/4fd40fd9b43e2d20d21169efb1ac904b/image-31.jpg)
![Initializing Arrays You can initialize arrays when you declare them int[] scores = {68, Initializing Arrays You can initialize arrays when you declare them int[] scores = {68,](https://slidetodoc.com/presentation_image_h2/4fd40fd9b43e2d20d21169efb1ac904b/image-32.jpg)
![Arrays as Arguments Example //init all array elements to 0 public void init. Array(int[] Arrays as Arguments Example //init all array elements to 0 public void init. Array(int[]](https://slidetodoc.com/presentation_image_h2/4fd40fd9b43e2d20d21169efb1ac904b/image-33.jpg)
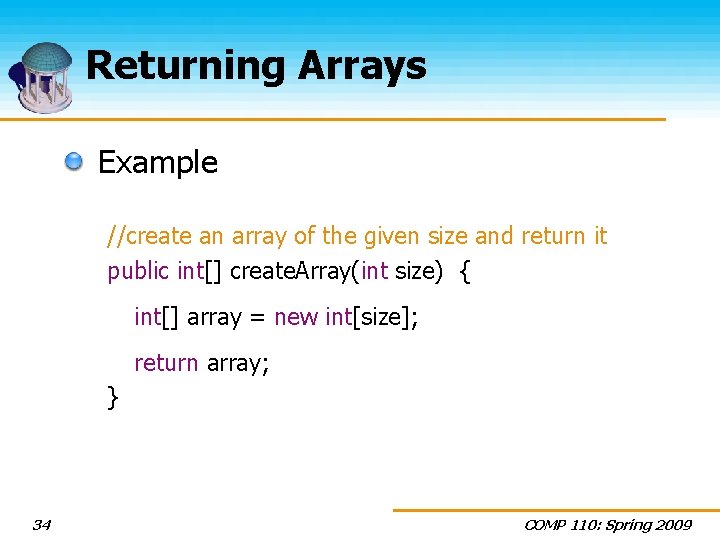
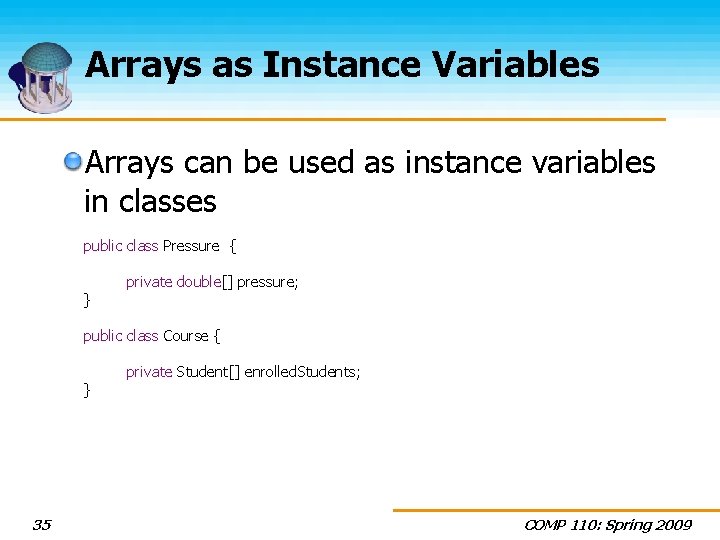
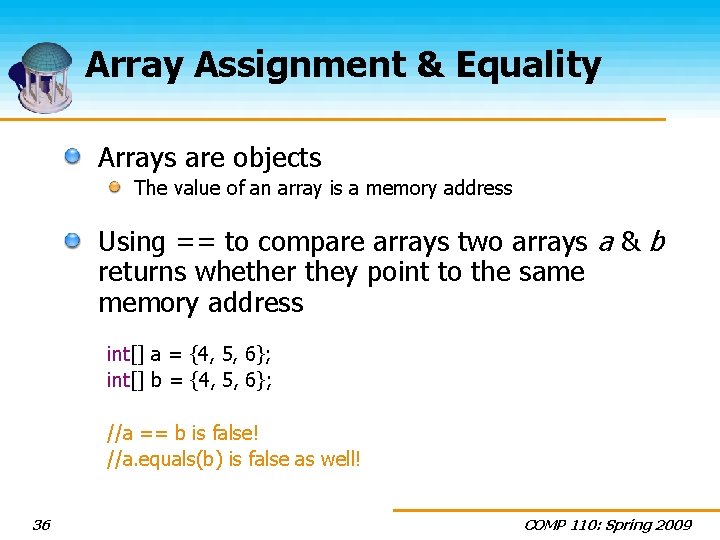
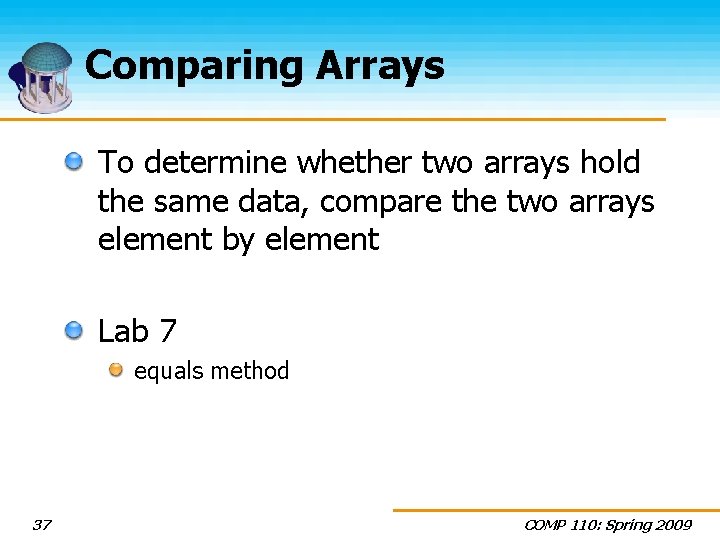
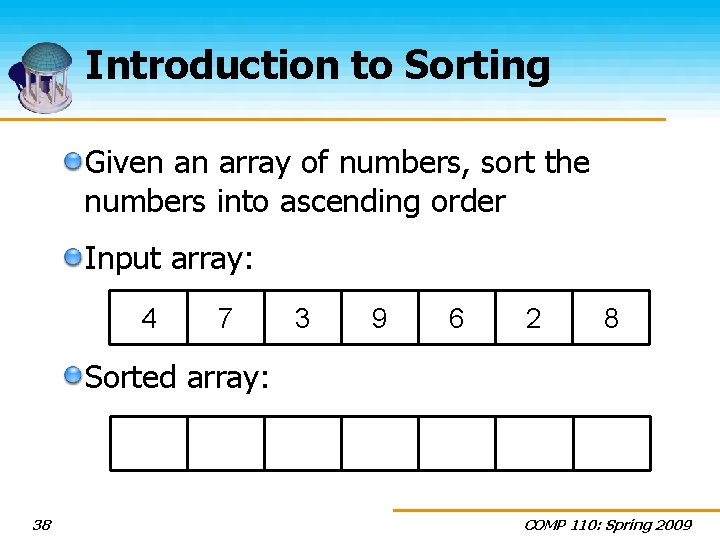
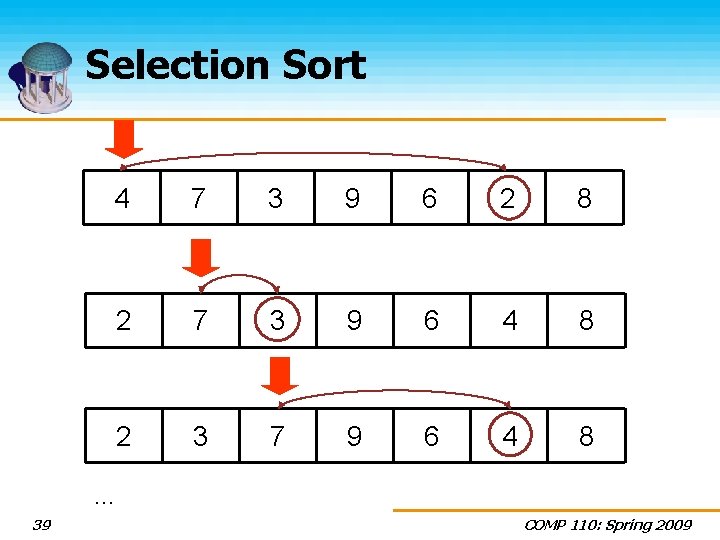
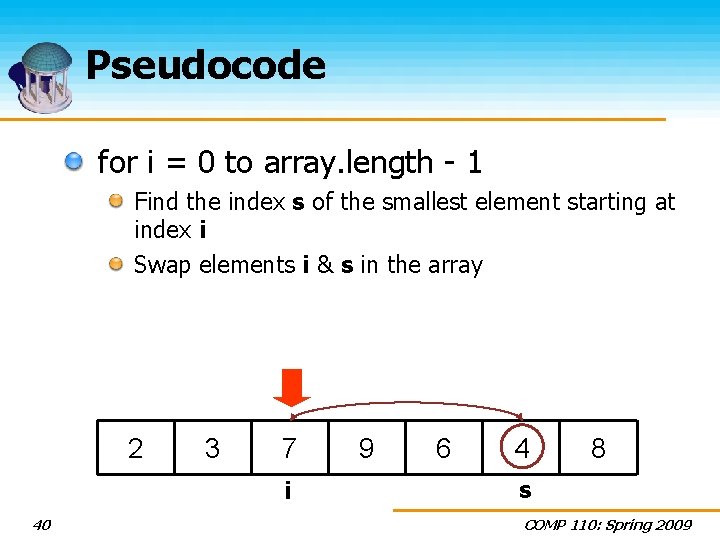
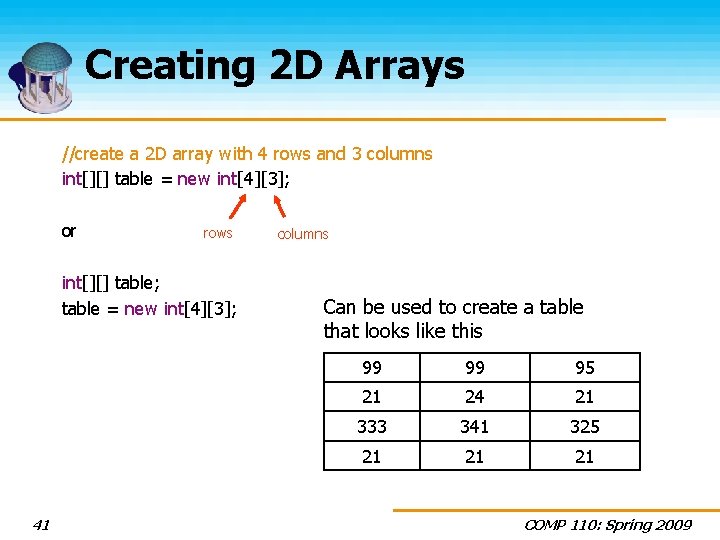
![Creating 2 D Arrays int[][] table = new int[4][3]; gives you access to table[0][0] Creating 2 D Arrays int[][] table = new int[4][3]; gives you access to table[0][0]](https://slidetodoc.com/presentation_image_h2/4fd40fd9b43e2d20d21169efb1ac904b/image-42.jpg)
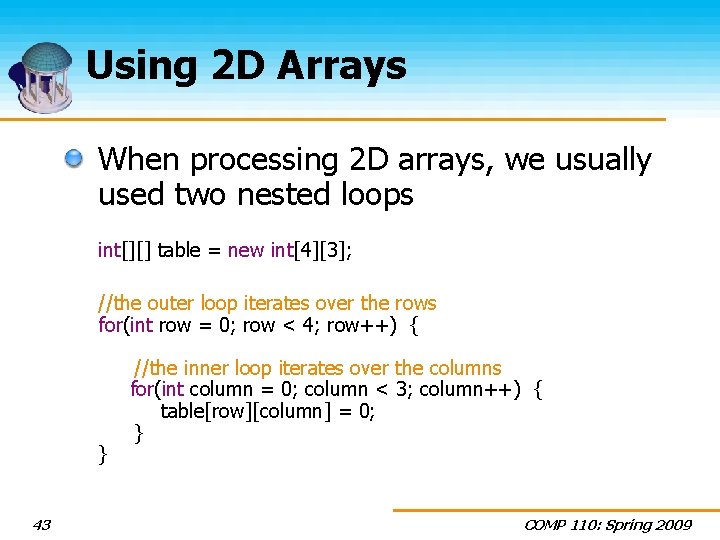
![Representation of 2 D Arrays int[][] table = new int[4][3]; table 1 2 2 Representation of 2 D Arrays int[][] table = new int[4][3]; table 1 2 2](https://slidetodoc.com/presentation_image_h2/4fd40fd9b43e2d20d21169efb1ac904b/image-44.jpg)
![Length field for 2 D Arrays int[][] table = new int[4][3]; table. length gives Length field for 2 D Arrays int[][] table = new int[4][3]; table. length gives](https://slidetodoc.com/presentation_image_h2/4fd40fd9b43e2d20d21169efb1ac904b/image-45.jpg)
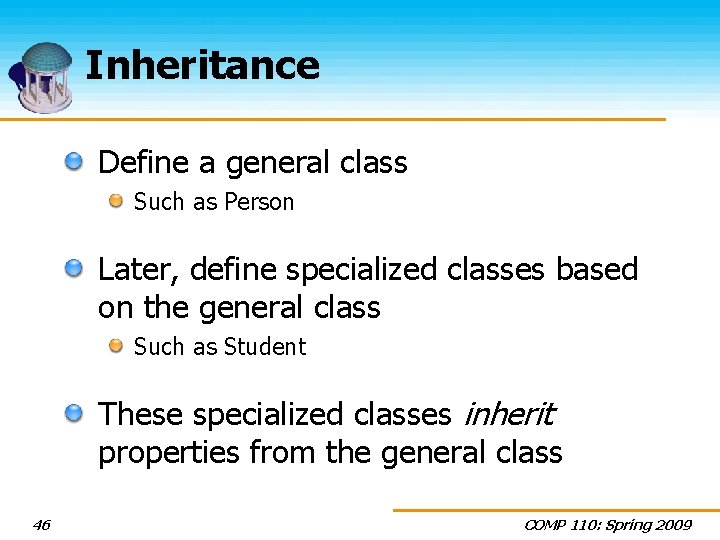
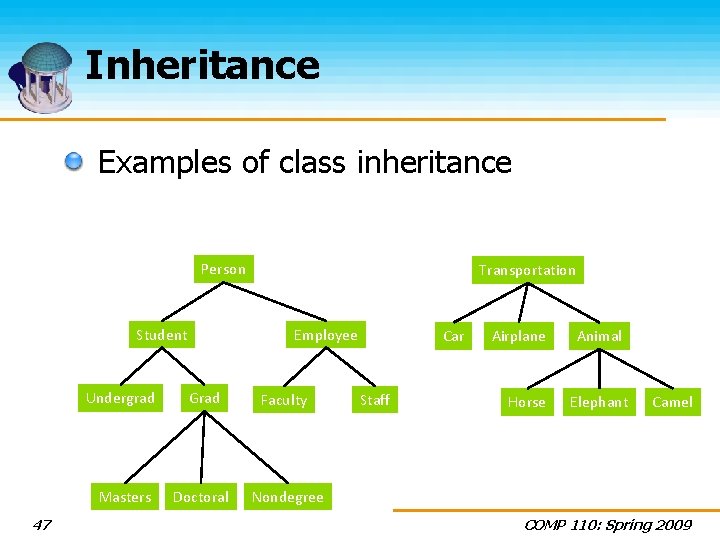
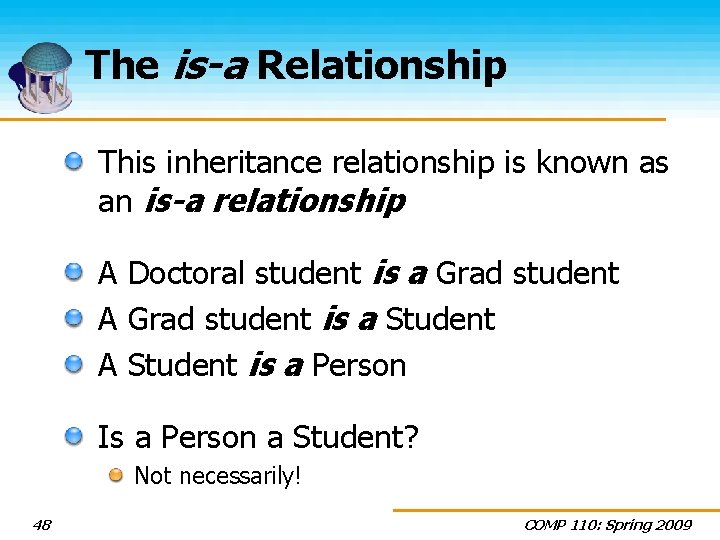
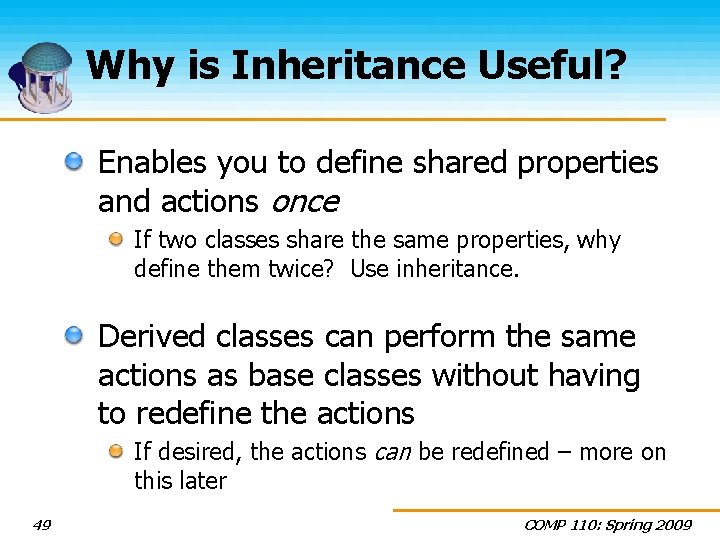
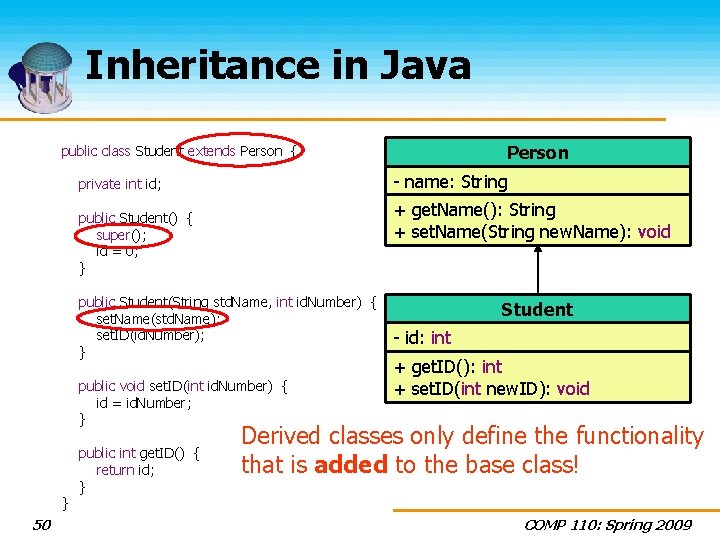
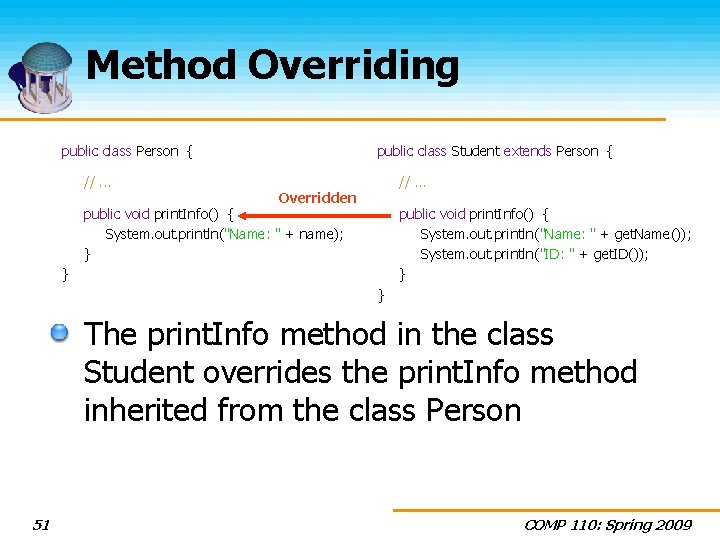
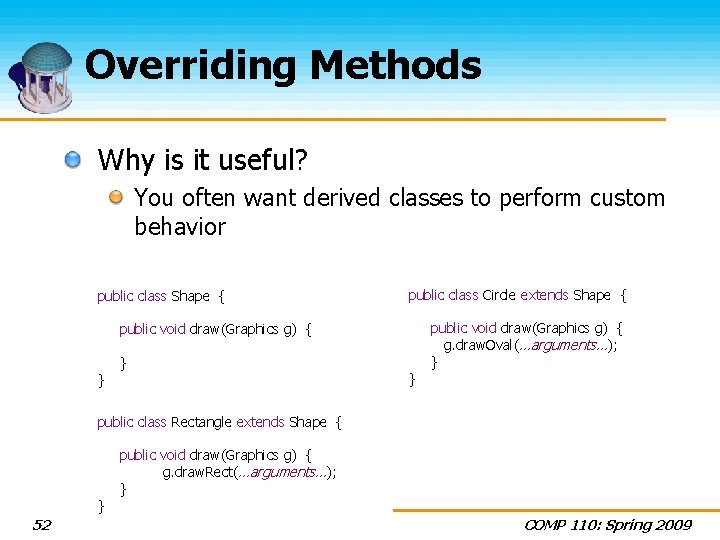
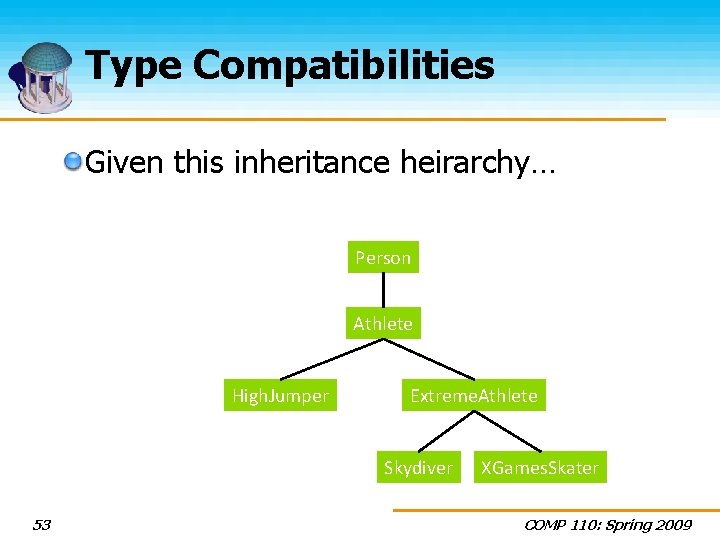
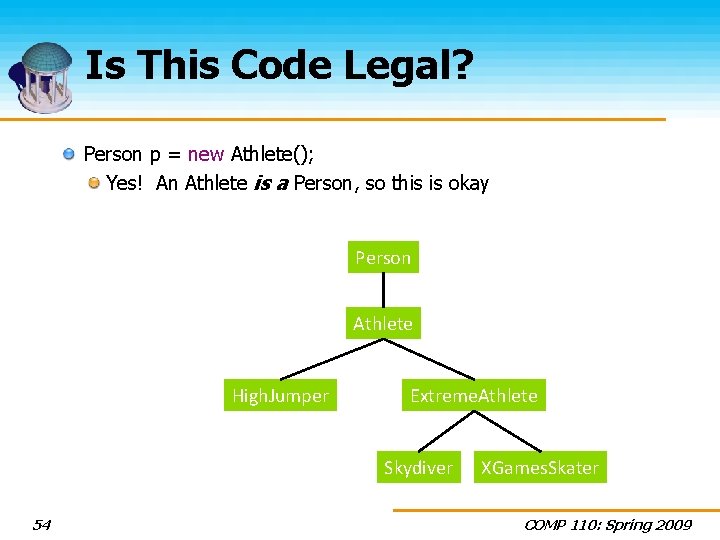
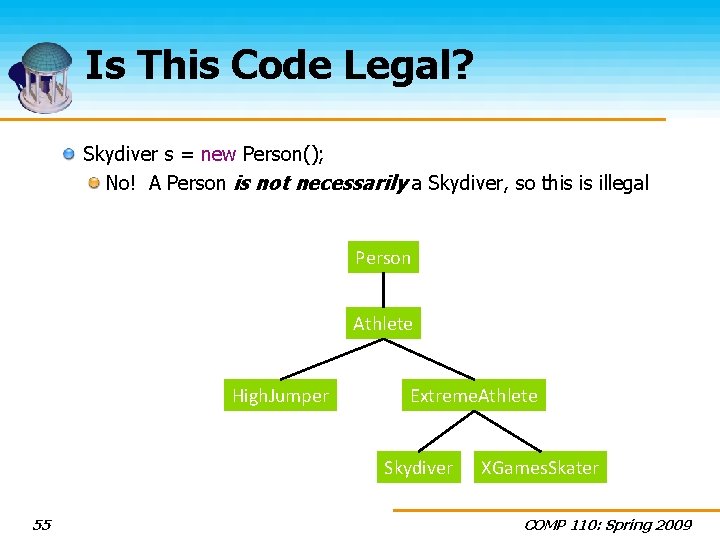
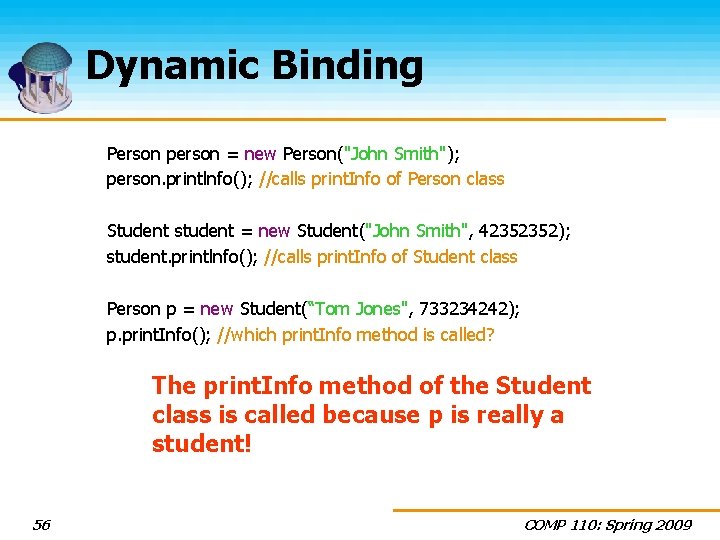
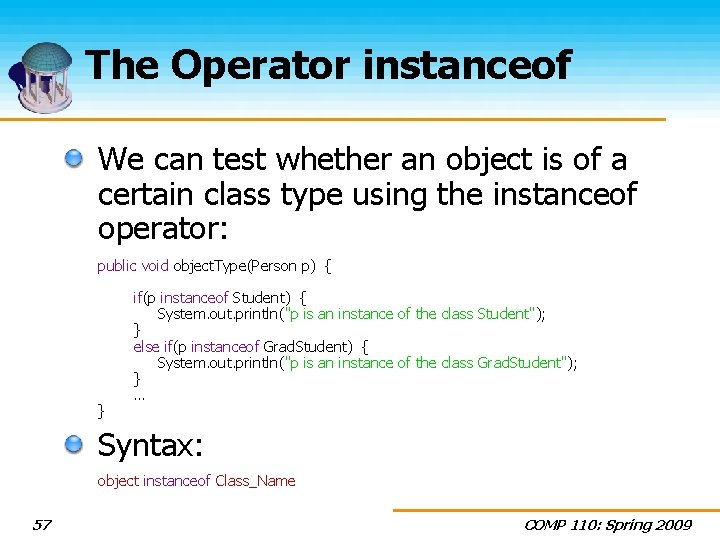
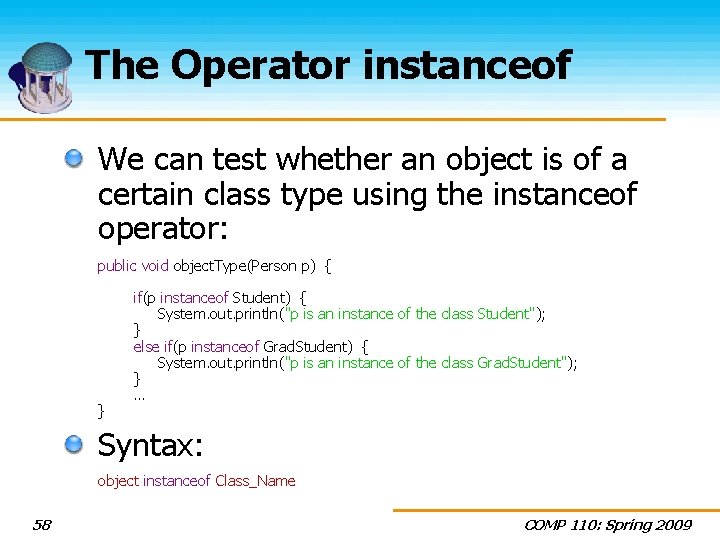
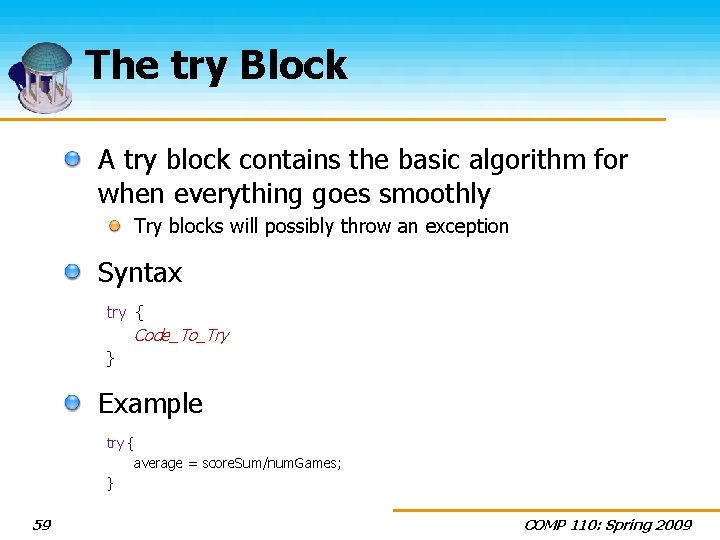
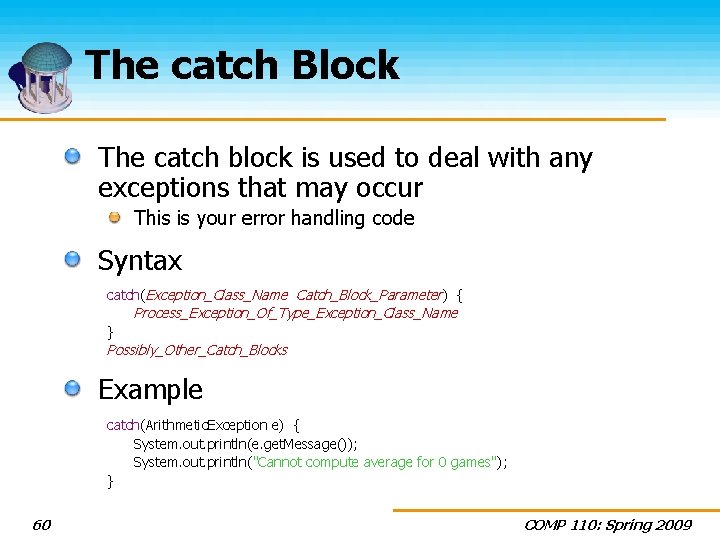
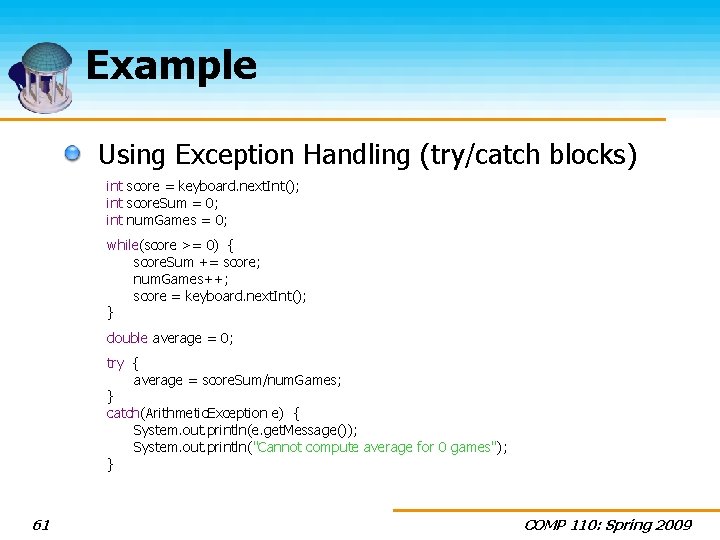
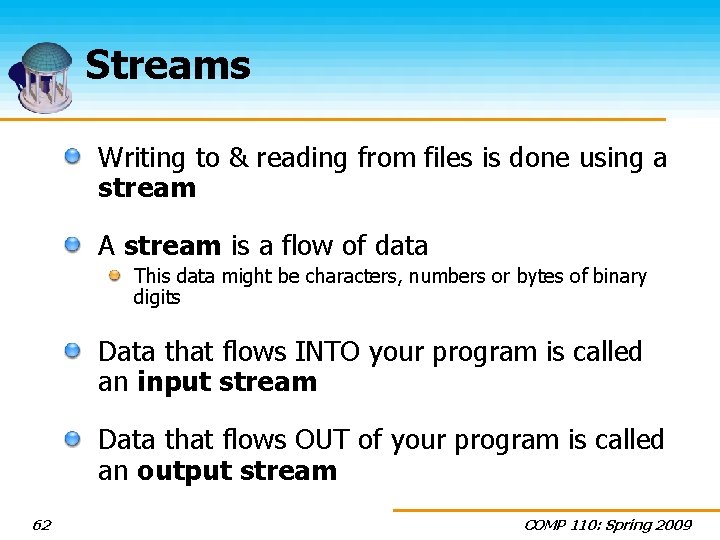
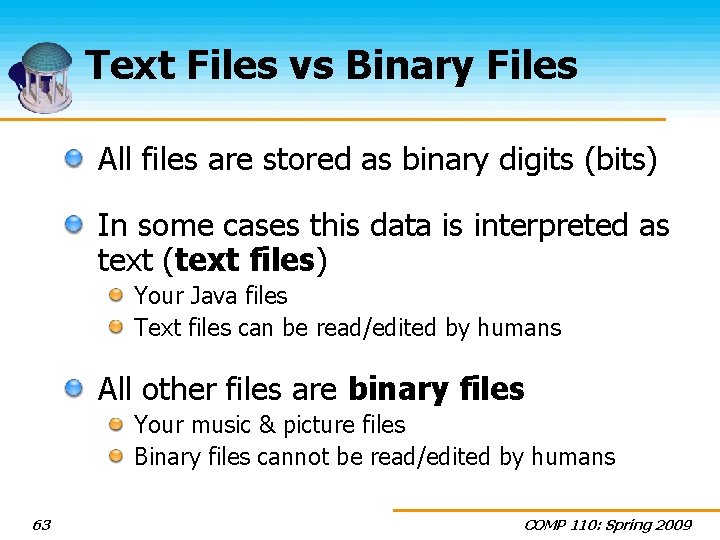
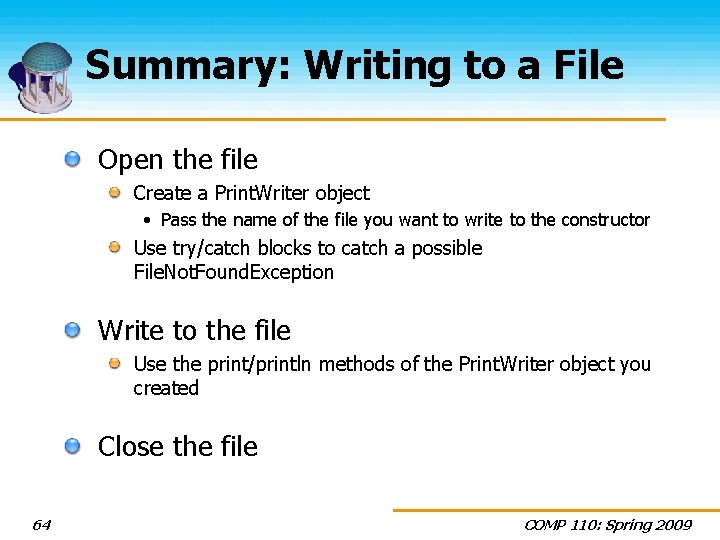
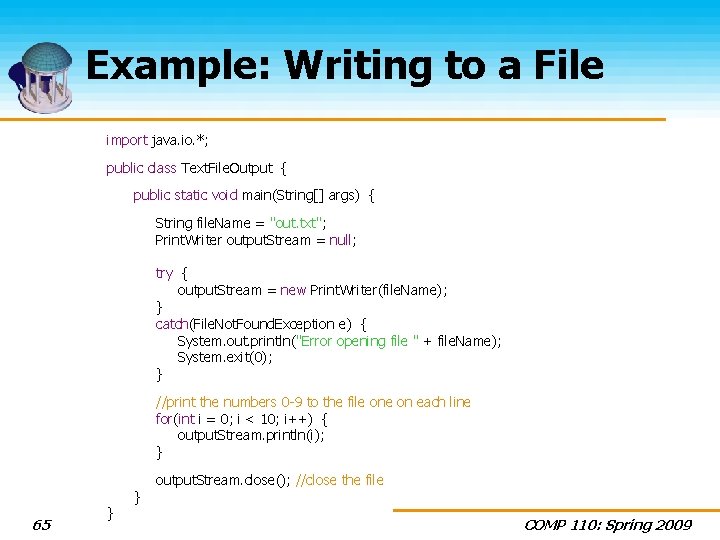
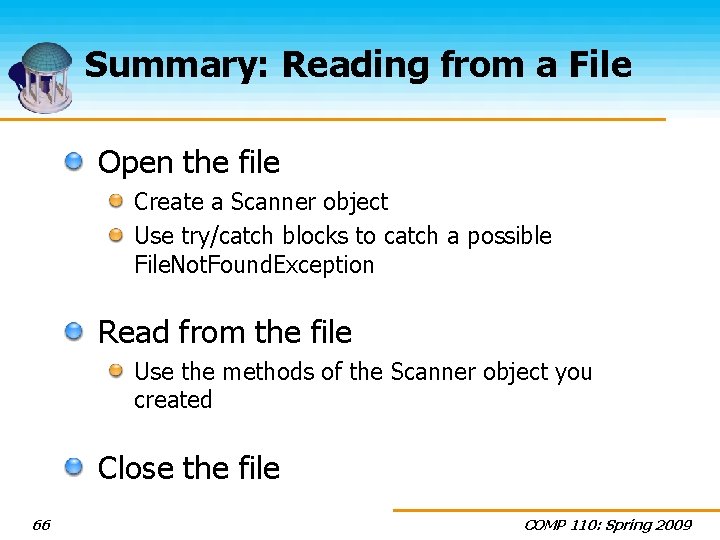
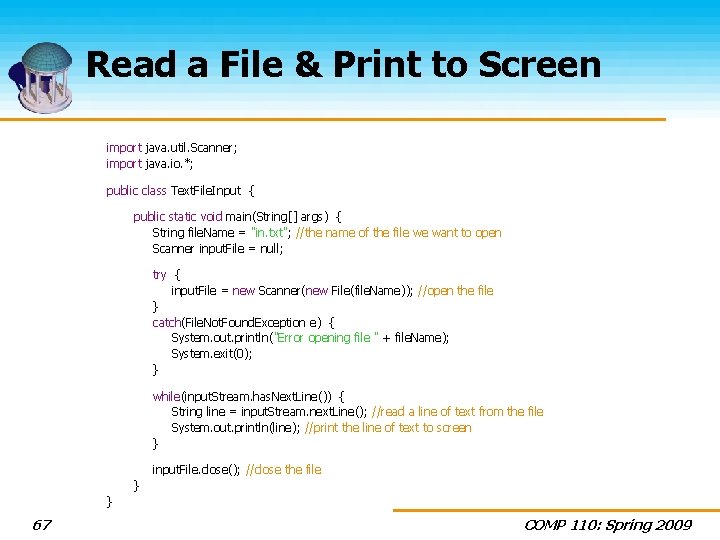
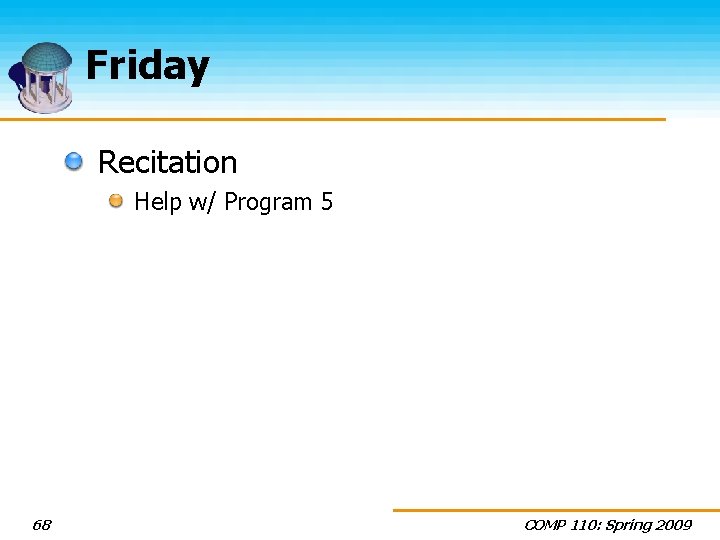
- Slides: 68
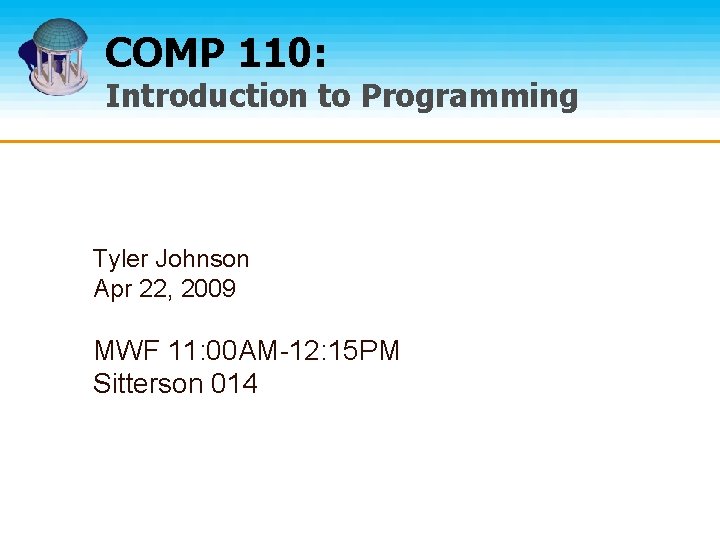
COMP 110: Introduction to Programming Tyler Johnson Apr 22, 2009 MWF 11: 00 AM-12: 15 PM Sitterson 014
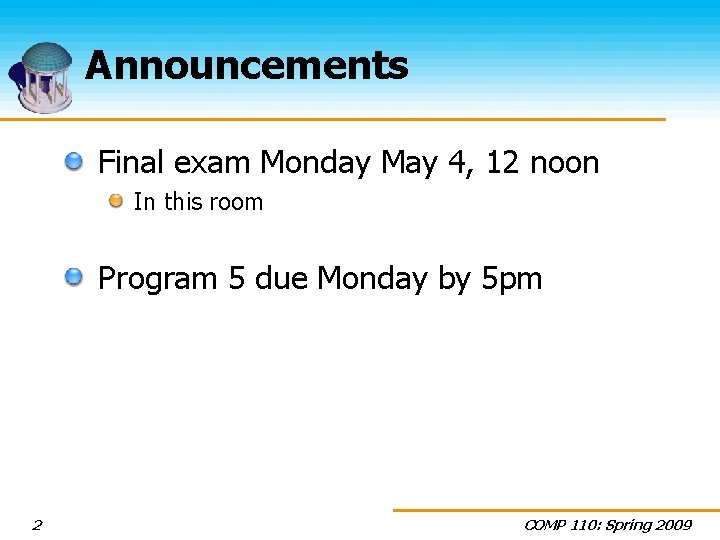
Announcements Final exam Monday May 4, 12 noon In this room Program 5 due Monday by 5 pm 2 COMP 110: Spring 2009
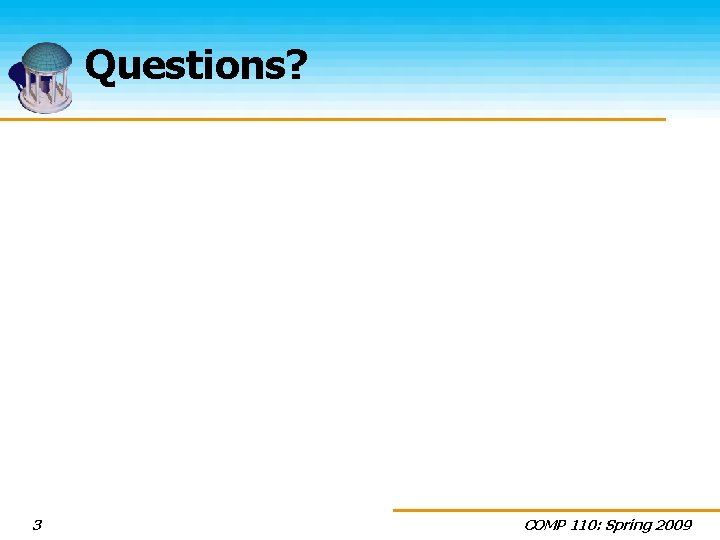
Questions? 3 COMP 110: Spring 2009
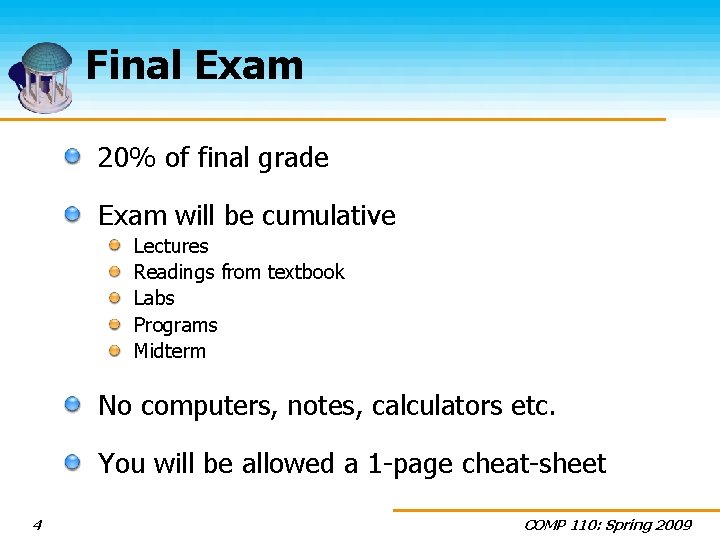
Final Exam 20% of final grade Exam will be cumulative Lectures Readings from textbook Labs Programs Midterm No computers, notes, calculators etc. You will be allowed a 1 -page cheat-sheet 4 COMP 110: Spring 2009
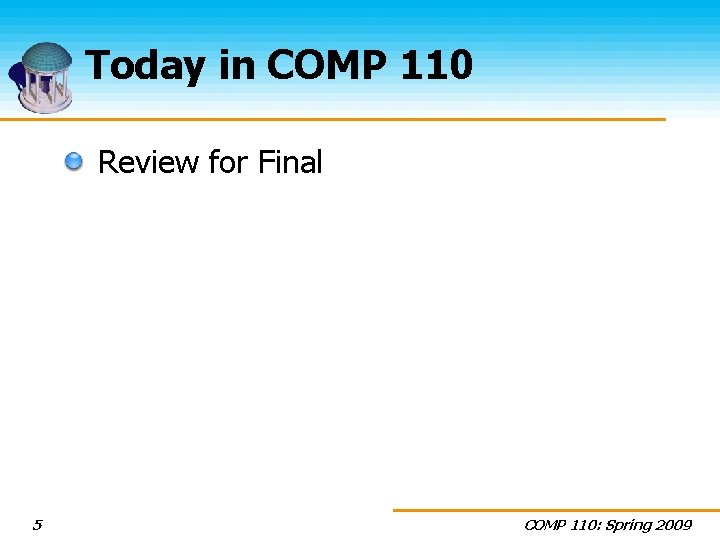
Today in COMP 110 Review for Final 5 COMP 110: Spring 2009
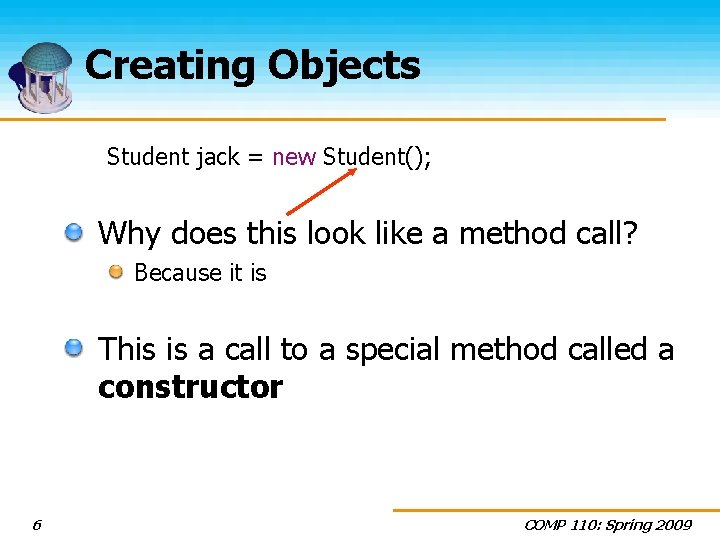
Creating Objects Student jack = new Student(); Why does this look like a method call? Because it is This is a call to a special method called a constructor 6 COMP 110: Spring 2009
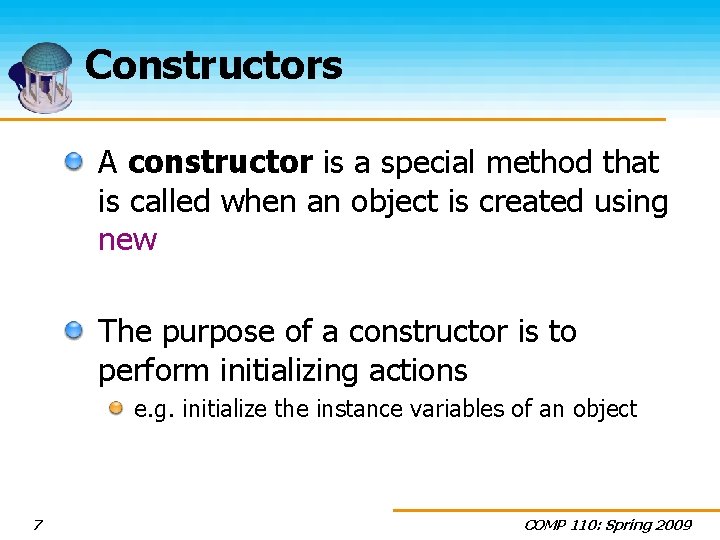
Constructors A constructor is a special method that is called when an object is created using new The purpose of a constructor is to perform initializing actions e. g. initialize the instance variables of an object 7 COMP 110: Spring 2009
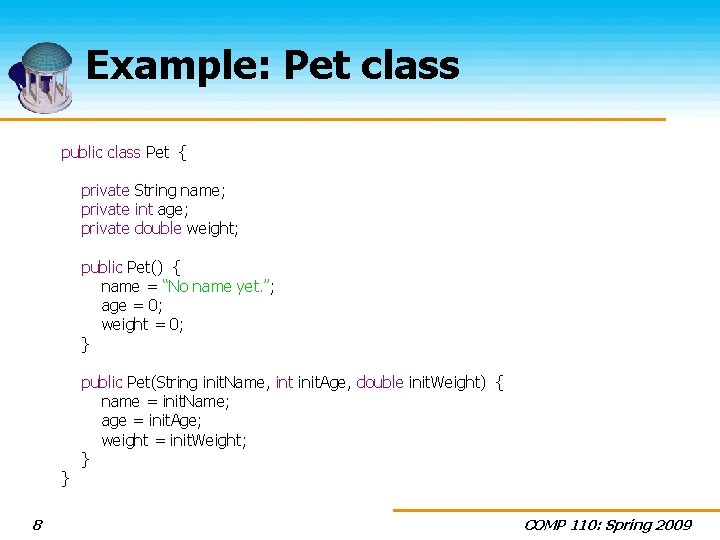
Example: Pet class public class Pet { private String name; private int age; private double weight; public Pet() { name = “No name yet. ”; age = 0; weight = 0; } } 8 public Pet(String init. Name, int init. Age, double init. Weight) { name = init. Name; age = init. Age; weight = init. Weight; } COMP 110: Spring 2009
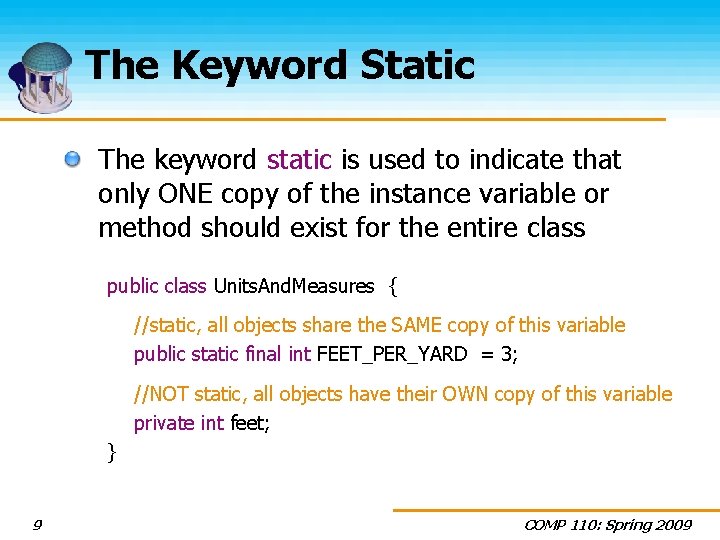
The Keyword Static The keyword static is used to indicate that only ONE copy of the instance variable or method should exist for the entire class public class Units. And. Measures { //static, all objects share the SAME copy of this variable public static final int FEET_PER_YARD = 3; //NOT static, all objects have their OWN copy of this variable private int feet; } 9 COMP 110: Spring 2009
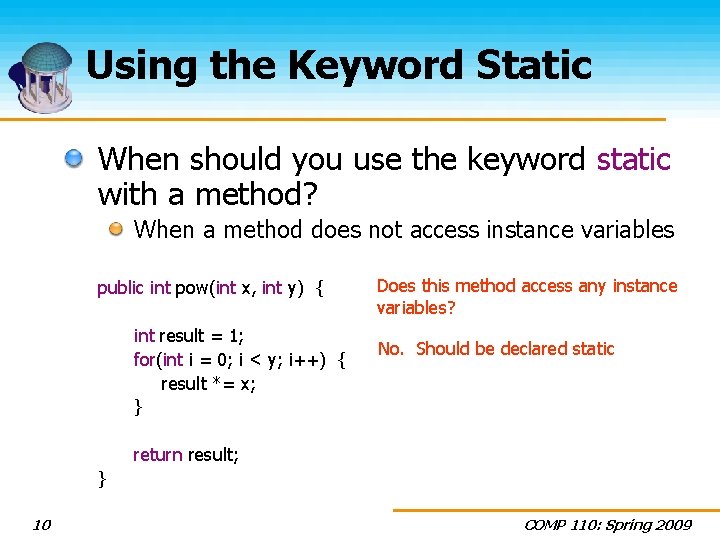
Using the Keyword Static When should you use the keyword static with a method? When a method does not access instance variables public int pow(int x, int y) { int result = 1; for(int i = 0; i < y; i++) { result *= x; } Does this method access any instance variables? No. Should be declared static return result; } 10 COMP 110: Spring 2009
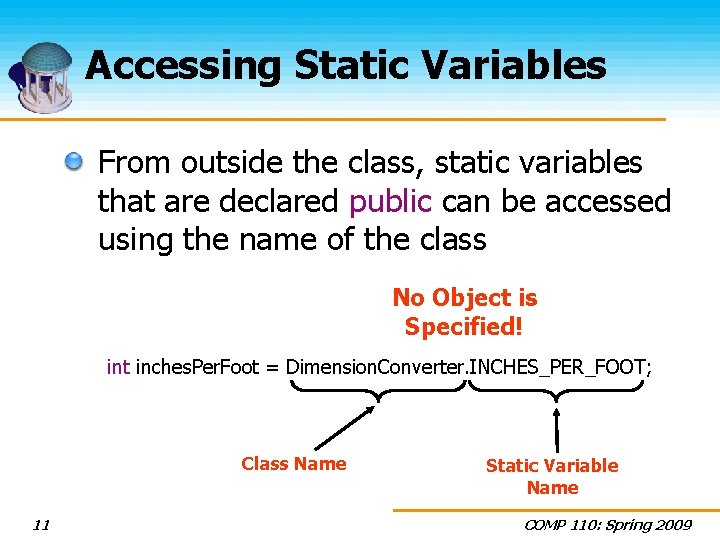
Accessing Static Variables From outside the class, static variables that are declared public can be accessed using the name of the class No Object is Specified! int inches. Per. Foot = Dimension. Converter. INCHES_PER_FOOT; Class Name 11 Static Variable Name COMP 110: Spring 2009
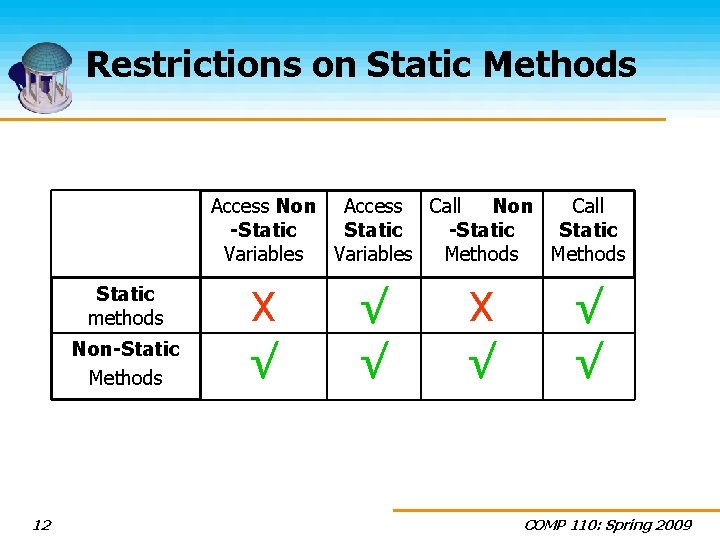
Restrictions on Static Methods Access Non Access Call Non Call -Static Variables Methods Static methods Non-Static Methods 12 X √ √ √ COMP 110: Spring 2009
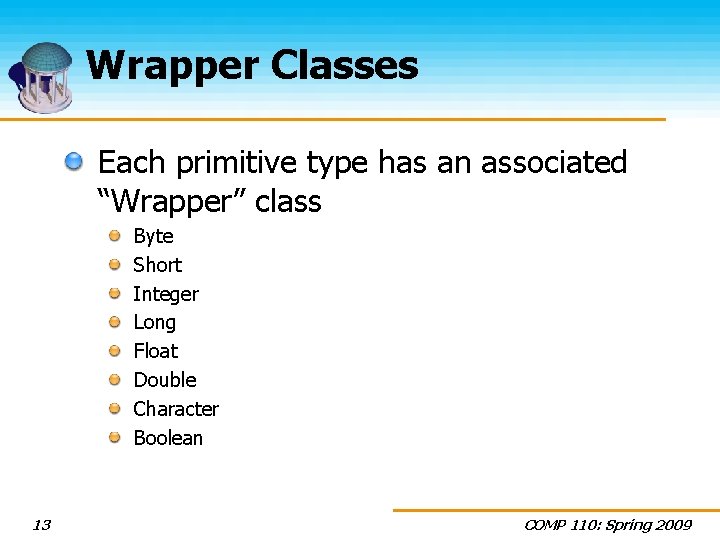
Wrapper Classes Each primitive type has an associated “Wrapper” class Byte Short Integer Long Float Double Character Boolean 13 COMP 110: Spring 2009
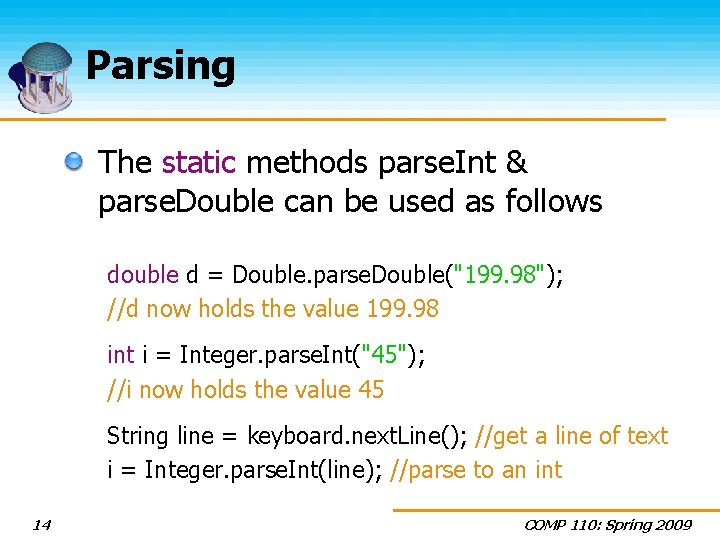
Parsing The static methods parse. Int & parse. Double can be used as follows double d = Double. parse. Double("199. 98"); //d now holds the value 199. 98 int i = Integer. parse. Int("45"); //i now holds the value 45 String line = keyboard. next. Line(); //get a line of text i = Integer. parse. Int(line); //parse to an int 14 COMP 110: Spring 2009
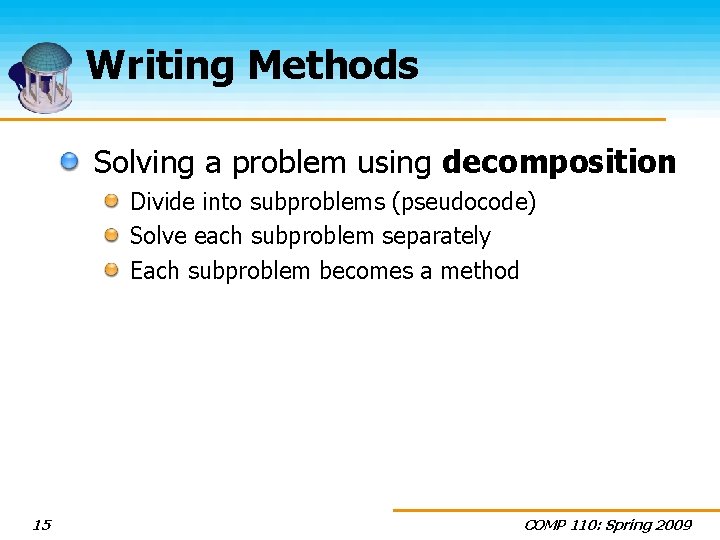
Writing Methods Solving a problem using decomposition Divide into subproblems (pseudocode) Solve each subproblem separately Each subproblem becomes a method 15 COMP 110: Spring 2009
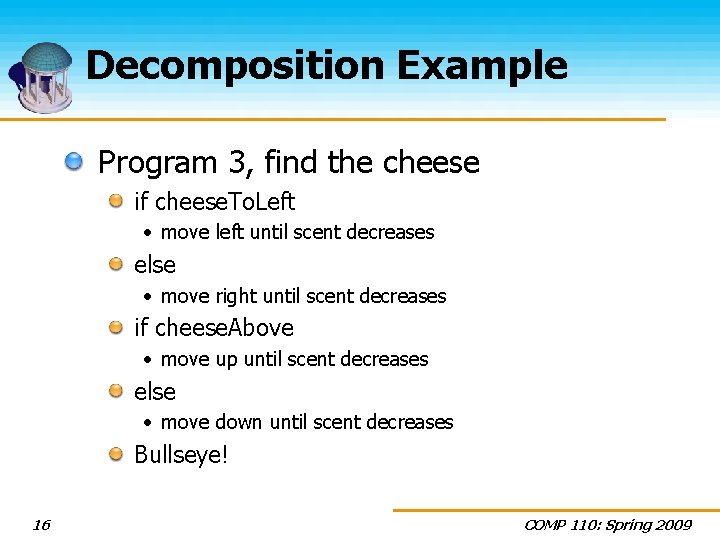
Decomposition Example Program 3, find the cheese if cheese. To. Left • move left until scent decreases else • move right until scent decreases if cheese. Above • move up until scent decreases else • move down until scent decreases Bullseye! 16 COMP 110: Spring 2009
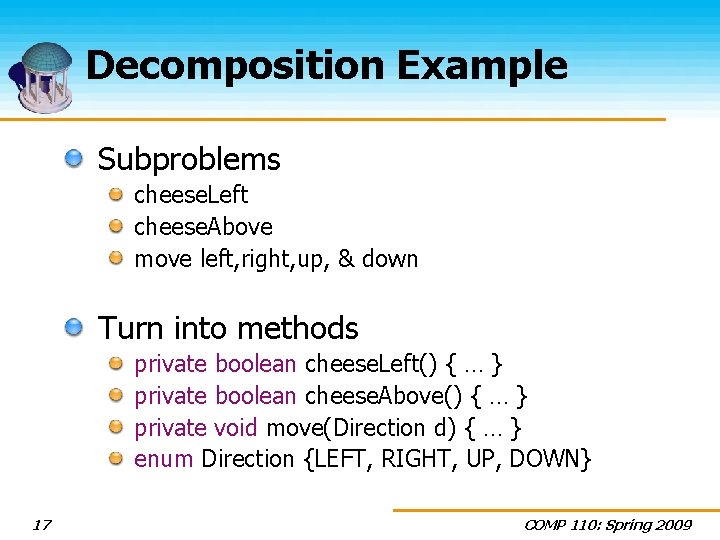
Decomposition Example Subproblems cheese. Left cheese. Above move left, right, up, & down Turn into methods private boolean cheese. Left() { … } private boolean cheese. Above() { … } private void move(Direction d) { … } enum Direction {LEFT, RIGHT, UP, DOWN} 17 COMP 110: Spring 2009
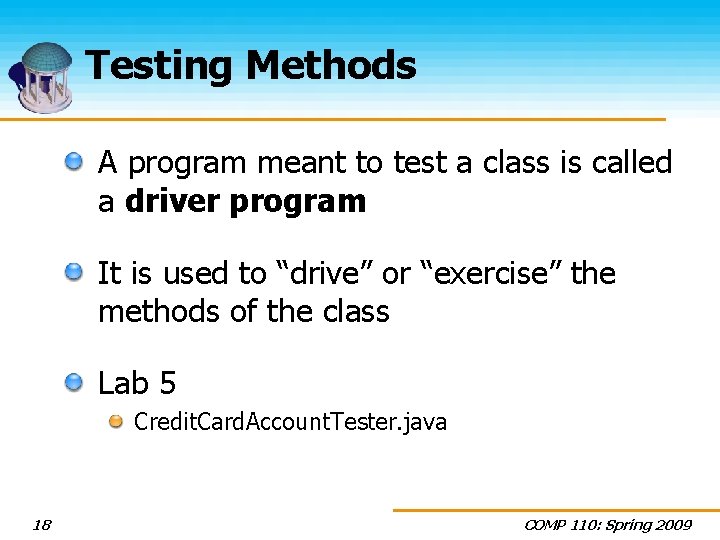
Testing Methods A program meant to test a class is called a driver program It is used to “drive” or “exercise” the methods of the class Lab 5 Credit. Card. Account. Tester. java 18 COMP 110: Spring 2009
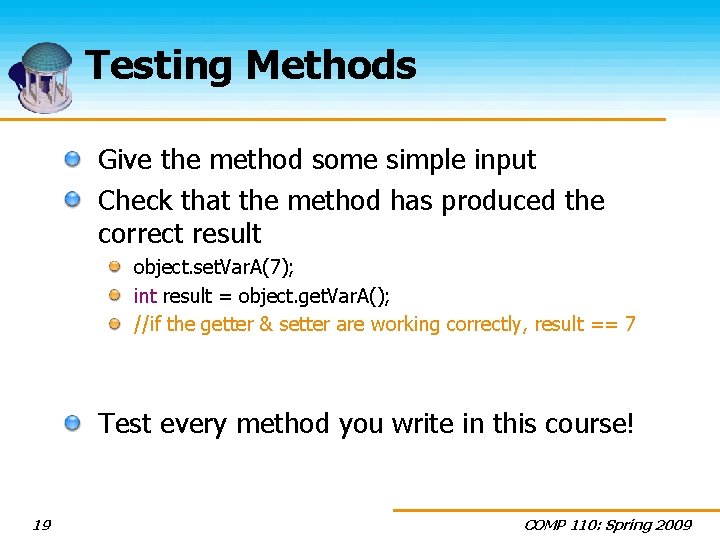
Testing Methods Give the method some simple input Check that the method has produced the correct result object. set. Var. A(7); int result = object. get. Var. A(); //if the getter & setter are working correctly, result == 7 Test every method you write in this course! 19 COMP 110: Spring 2009
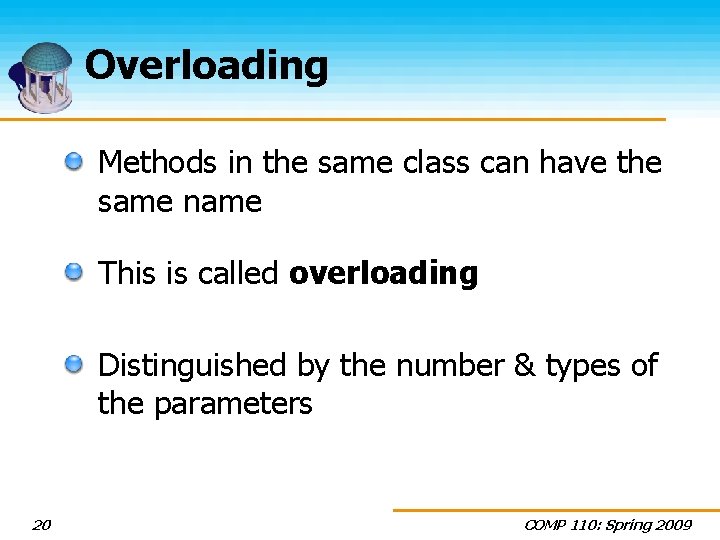
Overloading Methods in the same class can have the same name This is called overloading Distinguished by the number & types of the parameters 20 COMP 110: Spring 2009
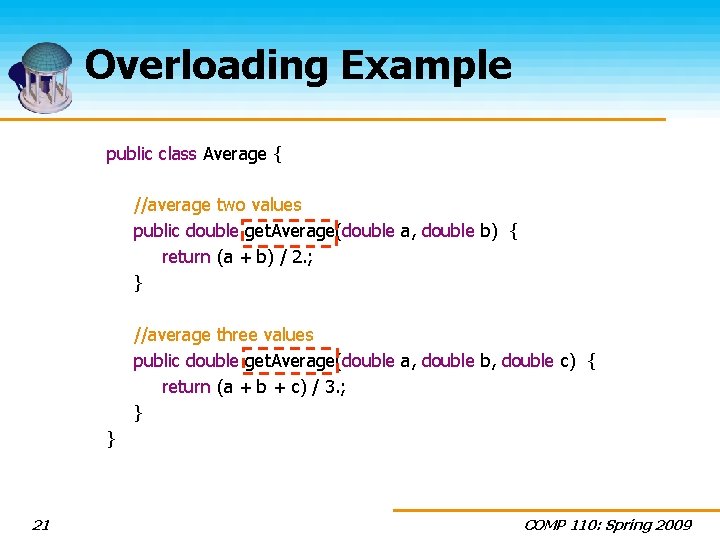
Overloading Example public class Average { //average two values public double get. Average(double a, double b) { return (a + b) / 2. ; } //average three values public double get. Average(double a, double b, double c) { return (a + b + c) / 3. ; } } 21 COMP 110: Spring 2009
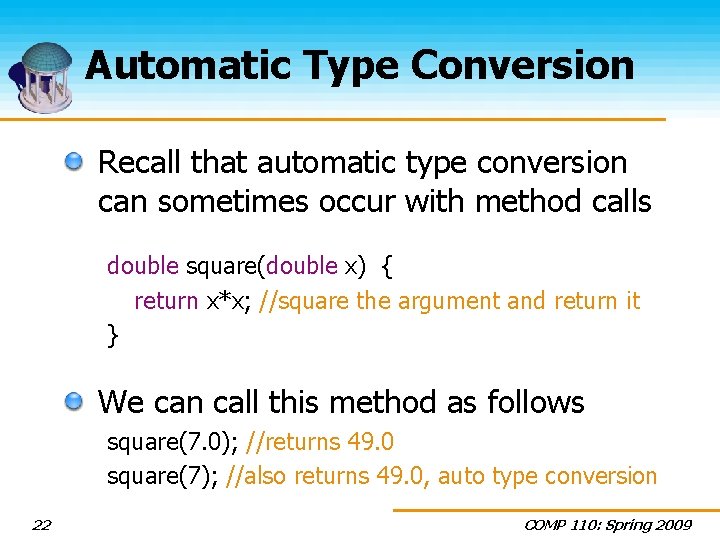
Automatic Type Conversion Recall that automatic type conversion can sometimes occur with method calls double square(double x) { return x*x; //square the argument and return it } We can call this method as follows square(7. 0); //returns 49. 0 square(7); //also returns 49. 0, auto type conversion 22 COMP 110: Spring 2009
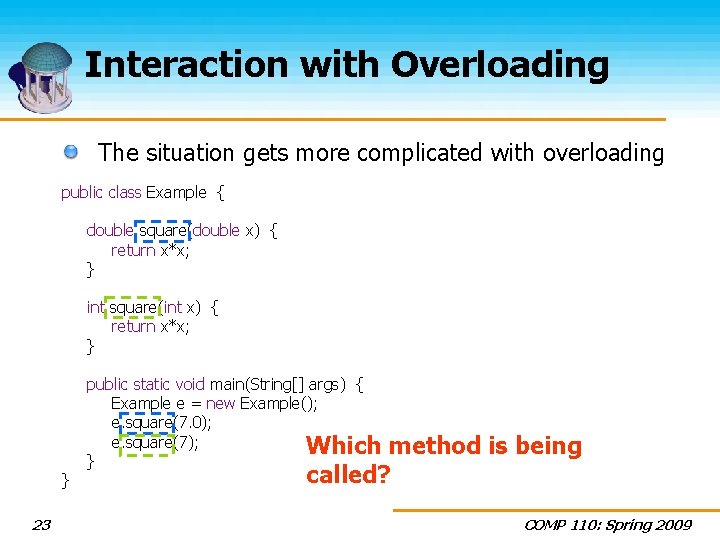
Interaction with Overloading The situation gets more complicated with overloading public class Example { double square(double x) { return x*x; } int square(int x) { return x*x; } } 23 public static void main(String[] args) { Example e = new Example(); e. square(7. 0); e. square(7); Which } method is being called? COMP 110: Spring 2009
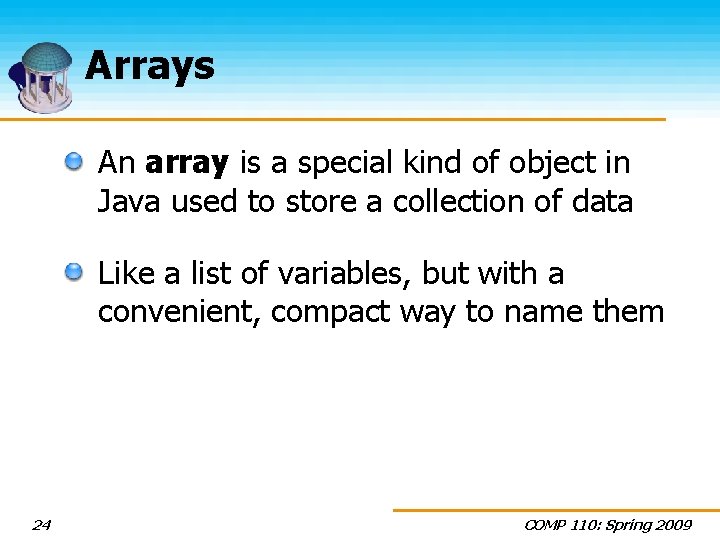
Arrays An array is a special kind of object in Java used to store a collection of data Like a list of variables, but with a convenient, compact way to name them 24 COMP 110: Spring 2009
![Creating an Array int scores new int5 create 5 ints This creates an Creating an Array int[] scores = new int[5]; //create 5 ints This creates an](https://slidetodoc.com/presentation_image_h2/4fd40fd9b43e2d20d21169efb1ac904b/image-25.jpg)
Creating an Array int[] scores = new int[5]; //create 5 ints This creates an array called “scores” that holds five integer values scores[0] //a single integer, the 1 st integer in the array scores[1] //a single integer, the 2 nd integer in the array scores[2] scores[3] scores[4] //a single integer, the 5 th and last integer in the array 25 COMP 110: Spring 2009
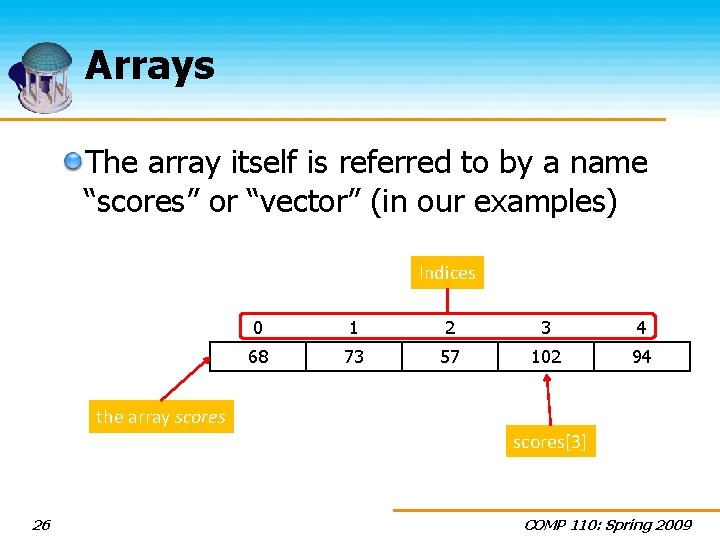
Arrays The array itself is referred to by a name “scores” or “vector” (in our examples) Indices 0 1 2 3 4 68 73 57 102 94 the array scores[3] 26 COMP 110: Spring 2009
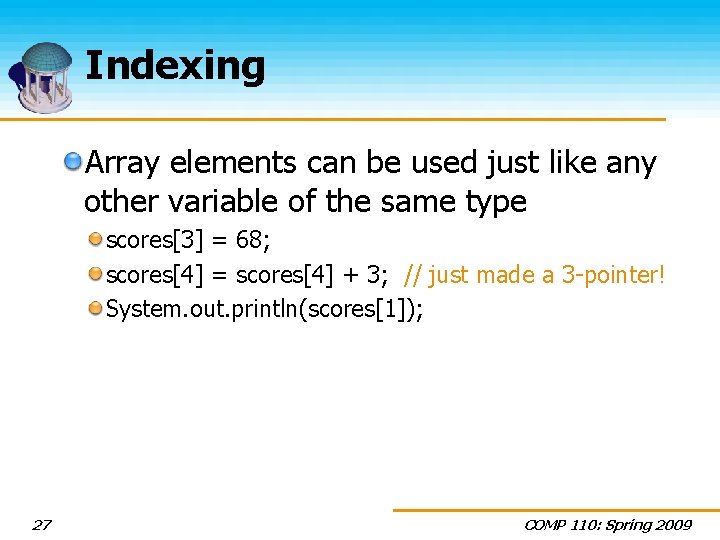
Indexing Array elements can be used just like any other variable of the same type scores[3] = 68; scores[4] = scores[4] + 3; // just made a 3 -pointer! System. out. println(scores[1]); 27 COMP 110: Spring 2009
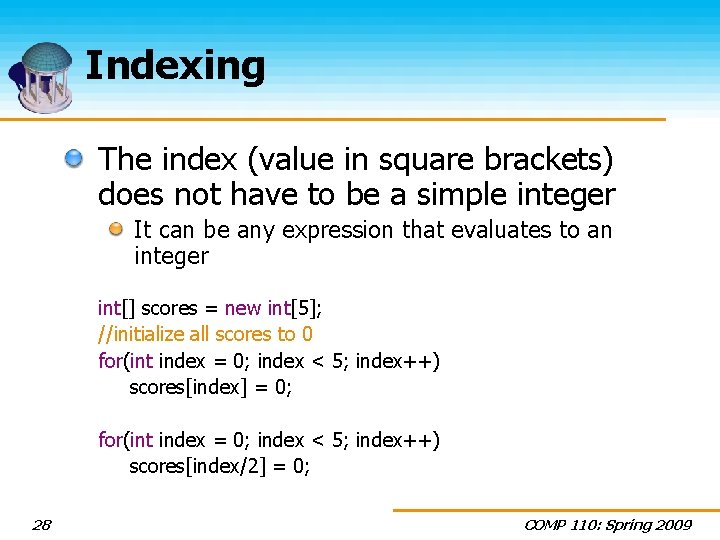
Indexing The index (value in square brackets) does not have to be a simple integer It can be any expression that evaluates to an integer int[] scores = new int[5]; //initialize all scores to 0 for(int index = 0; index < 5; index++) scores[index] = 0; for(int index = 0; index < 5; index++) scores[index/2] = 0; 28 COMP 110: Spring 2009
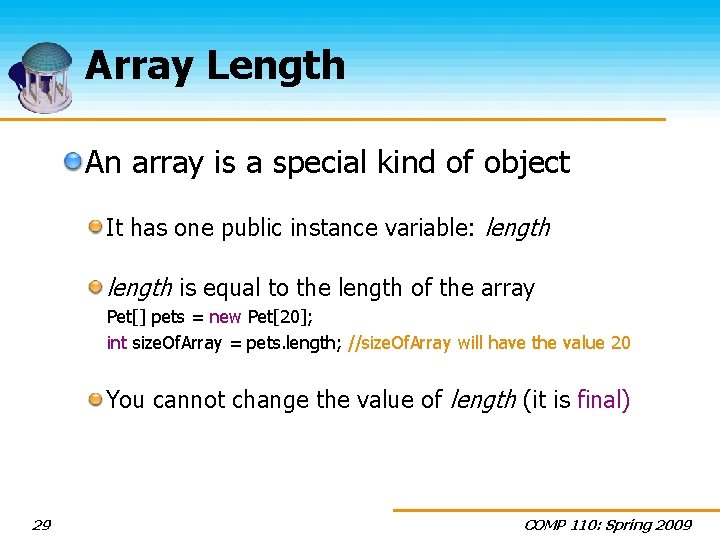
Array Length An array is a special kind of object It has one public instance variable: length is equal to the length of the array Pet[] pets = new Pet[20]; int size. Of. Array = pets. length; //size. Of. Array will have the value 20 You cannot change the value of length (it is final) 29 COMP 110: Spring 2009
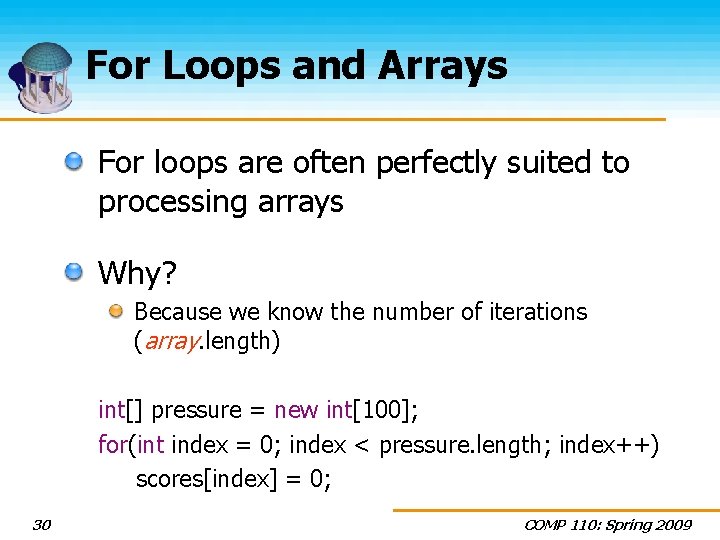
For Loops and Arrays For loops are often perfectly suited to processing arrays Why? Because we know the number of iterations (array. length) int[] pressure = new int[100]; for(int index = 0; index < pressure. length; index++) scores[index] = 0; 30 COMP 110: Spring 2009
![Be Careful with Indices MUST be in bounds double entries new double5 entries5 Be Careful with Indices MUST be in bounds double[] entries = new double[5]; entries[5]](https://slidetodoc.com/presentation_image_h2/4fd40fd9b43e2d20d21169efb1ac904b/image-31.jpg)
Be Careful with Indices MUST be in bounds double[] entries = new double[5]; entries[5] = 3. 7; //RUN-TIME ERROR! Index out of bounds Your code WILL compile with an out-ofbounds index But it will result in a run-time error (crash) 31 COMP 110: Spring 2009
![Initializing Arrays You can initialize arrays when you declare them int scores 68 Initializing Arrays You can initialize arrays when you declare them int[] scores = {68,](https://slidetodoc.com/presentation_image_h2/4fd40fd9b43e2d20d21169efb1ac904b/image-32.jpg)
Initializing Arrays You can initialize arrays when you declare them int[] scores = {68, 97, 102}; Equivalent to int[] scores = new int[3]; scores[0] = 68; scores[1] = 97; scores[2] = 102; 32 COMP 110: Spring 2009
![Arrays as Arguments Example init all array elements to 0 public void init Arrayint Arrays as Arguments Example //init all array elements to 0 public void init. Array(int[]](https://slidetodoc.com/presentation_image_h2/4fd40fd9b43e2d20d21169efb1ac904b/image-33.jpg)
Arrays as Arguments Example //init all array elements to 0 public void init. Array(int[] array) { for(int i = 0; i < array. length; i++) array[i] = 0; } 33 COMP 110: Spring 2009
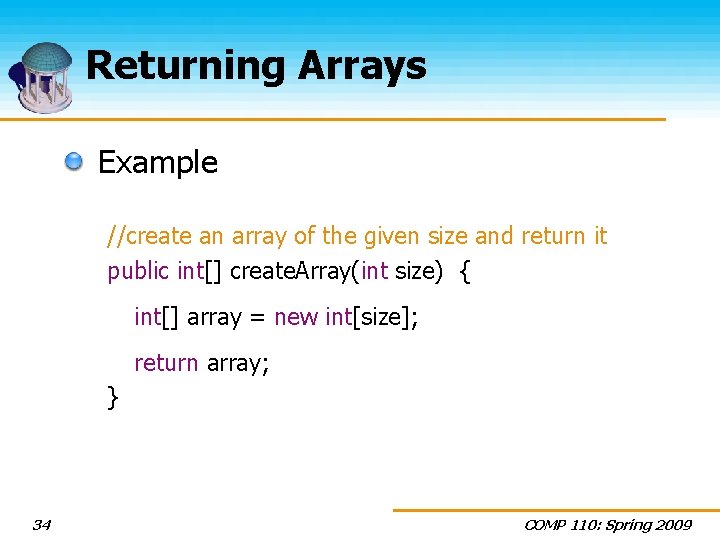
Returning Arrays Example //create an array of the given size and return it public int[] create. Array(int size) { int[] array = new int[size]; return array; } 34 COMP 110: Spring 2009
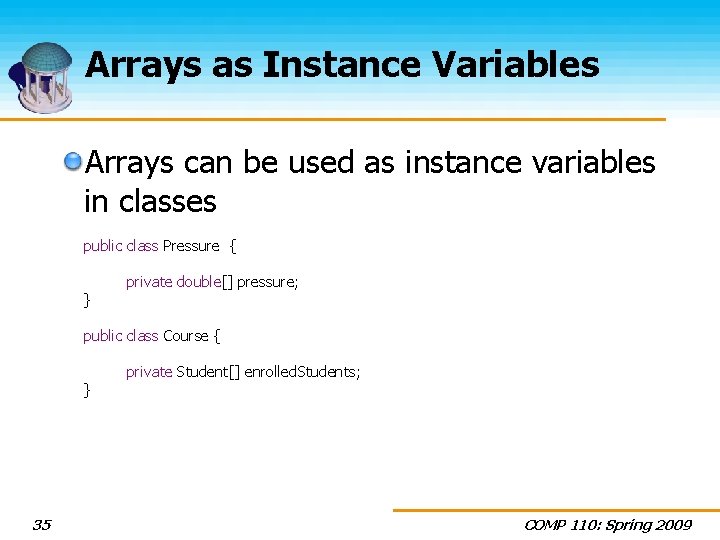
Arrays as Instance Variables Arrays can be used as instance variables in classes public class Pressure { } private double[] pressure; public class Course { } 35 private Student[] enrolled. Students; COMP 110: Spring 2009
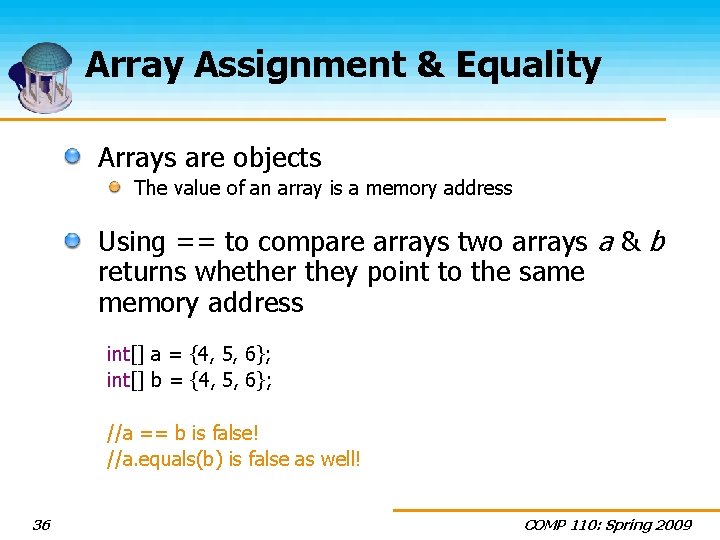
Array Assignment & Equality Arrays are objects The value of an array is a memory address Using == to compare arrays two arrays a & b returns whether they point to the same memory address int[] a = {4, 5, 6}; int[] b = {4, 5, 6}; //a == b is false! //a. equals(b) is false as well! 36 COMP 110: Spring 2009
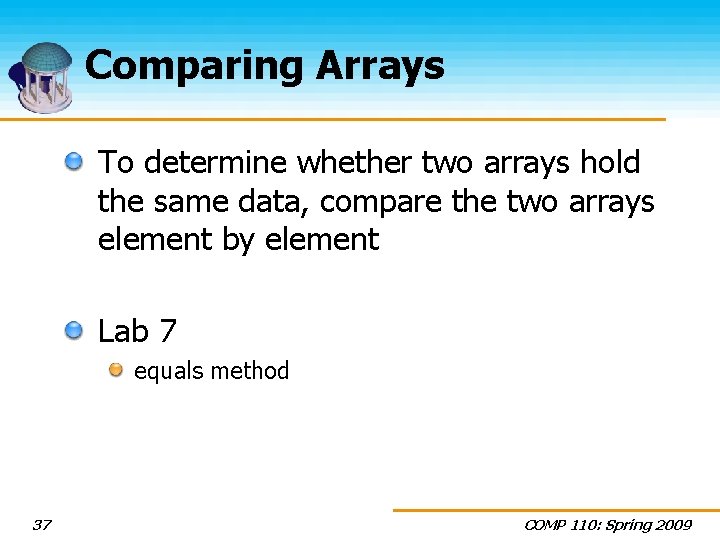
Comparing Arrays To determine whether two arrays hold the same data, compare the two arrays element by element Lab 7 equals method 37 COMP 110: Spring 2009
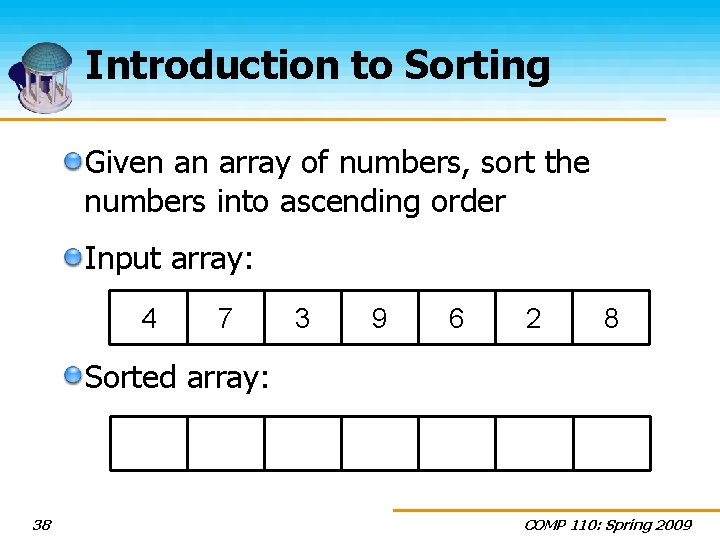
Introduction to Sorting Given an array of numbers, sort the numbers into ascending order Input array: 4 7 3 9 6 2 8 4 6 7 8 9 Sorted array: 2 38 3 COMP 110: Spring 2009
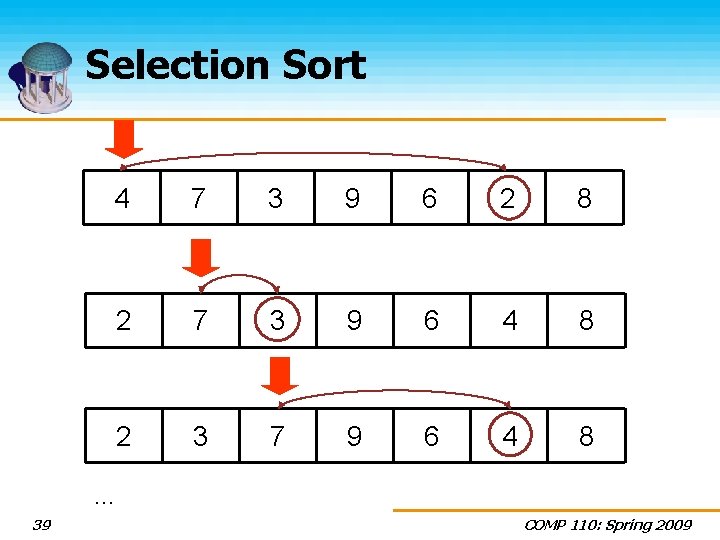
Selection Sort 4 7 3 9 6 2 8 2 7 3 9 6 4 8 2 3 7 9 6 4 8 … 39 COMP 110: Spring 2009
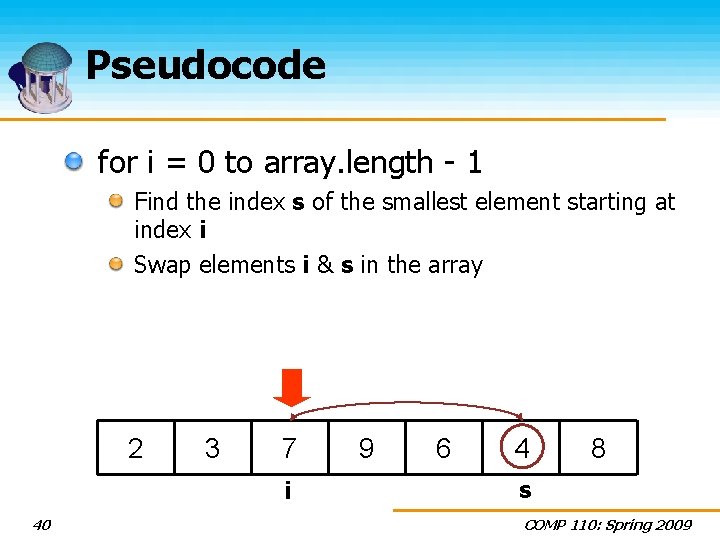
Pseudocode for i = 0 to array. length - 1 Find the index s of the smallest element starting at index i Swap elements i & s in the array 2 3 7 i 40 9 6 4 8 s COMP 110: Spring 2009
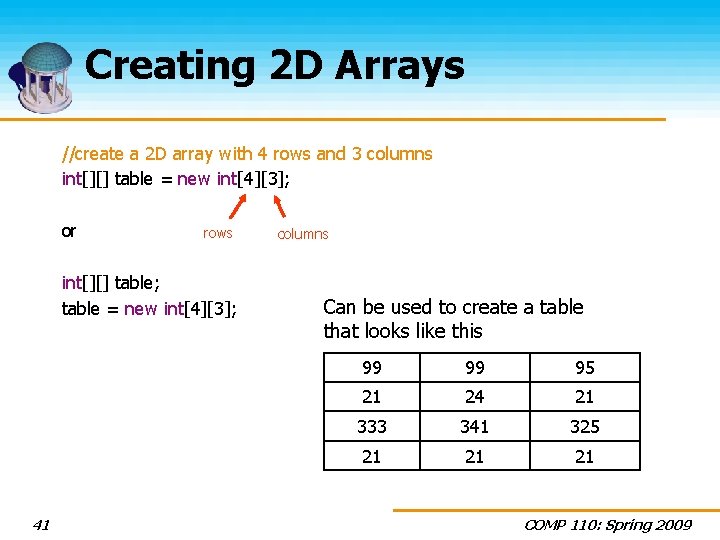
Creating 2 D Arrays //create a 2 D array with 4 rows and 3 columns int[][] table = new int[4][3]; or rows int[][] table; table = new int[4][3]; 41 columns Can be used to create a table that looks like this 99 99 95 21 24 21 333 341 325 21 21 21 COMP 110: Spring 2009
![Creating 2 D Arrays int table new int43 gives you access to table00 Creating 2 D Arrays int[][] table = new int[4][3]; gives you access to table[0][0]](https://slidetodoc.com/presentation_image_h2/4fd40fd9b43e2d20d21169efb1ac904b/image-42.jpg)
Creating 2 D Arrays int[][] table = new int[4][3]; gives you access to table[0][0] table[0][1] table[0][2] table[1][0] table[1][1] table[1][2] table[2][0] table[2][1] table[2][2] table[3][0] table[3][1] table[3][2] 42 //1 st row, 1 st column //1 st row, 2 nd column //1 st row, 3 rd column //4 th row, 1 st column //4 th row, 2 nd column //4 th row, 3 rd column 99 99 95 21 24 21 333 341 325 21 21 21 table[0][0] table[0][1] table[0][2] table[1][0] table[1][1] table[1][2] table[2][0] table[2][1] table[2][2] table[3][0] table[3][1] table[3][2] COMP 110: Spring 2009
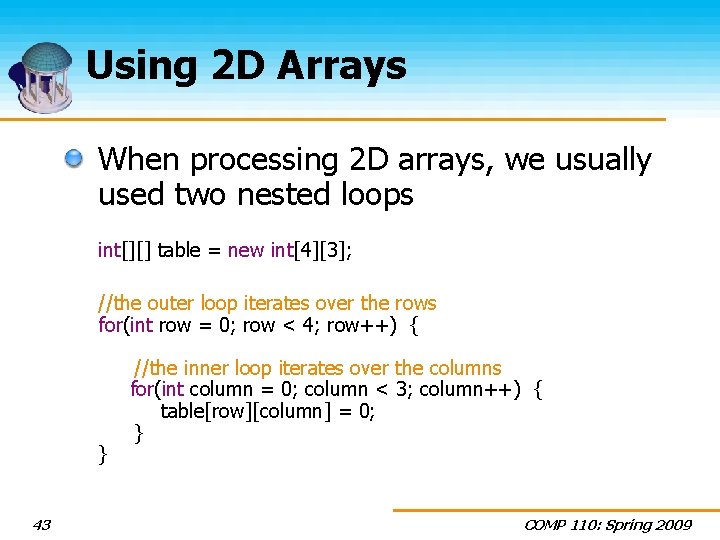
Using 2 D Arrays When processing 2 D arrays, we usually used two nested loops int[][] table = new int[4][3]; //the outer loop iterates over the rows for(int row = 0; row < 4; row++) { } 43 //the inner loop iterates over the columns for(int column = 0; column < 3; column++) { table[row][column] = 0; } COMP 110: Spring 2009
![Representation of 2 D Arrays int table new int43 table 1 2 2 Representation of 2 D Arrays int[][] table = new int[4][3]; table 1 2 2](https://slidetodoc.com/presentation_image_h2/4fd40fd9b43e2d20d21169efb1ac904b/image-44.jpg)
Representation of 2 D Arrays int[][] table = new int[4][3]; table 1 2 2 table[0] 2 7 6 5 7 9 9 7 5 table[1] table[2] table[3] 44 COMP 110: Spring 2009
![Length field for 2 D Arrays int table new int43 table length gives Length field for 2 D Arrays int[][] table = new int[4][3]; table. length gives](https://slidetodoc.com/presentation_image_h2/4fd40fd9b43e2d20d21169efb1ac904b/image-45.jpg)
Length field for 2 D Arrays int[][] table = new int[4][3]; table. length gives the number of rows (4 in this case) table[0]. length, table[1]. length etc gives the number of columns (3 in this case) 45 COMP 110: Spring 2009
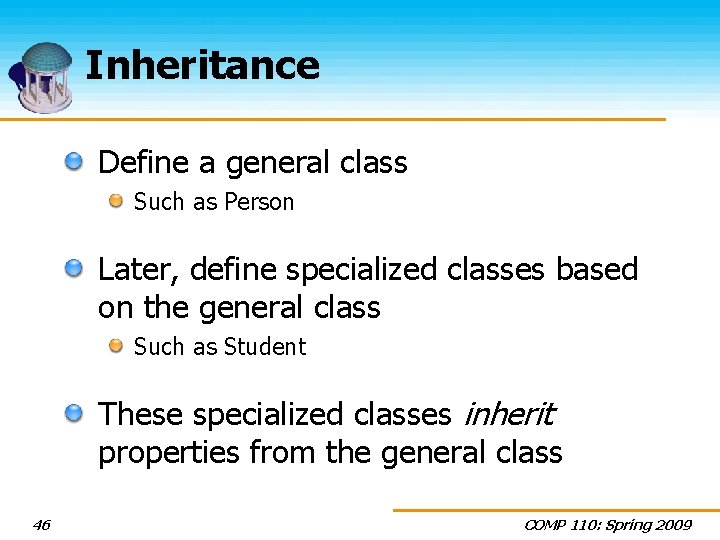
Inheritance Define a general class Such as Person Later, define specialized classes based on the general class Such as Student These specialized classes inherit properties from the general class 46 COMP 110: Spring 2009
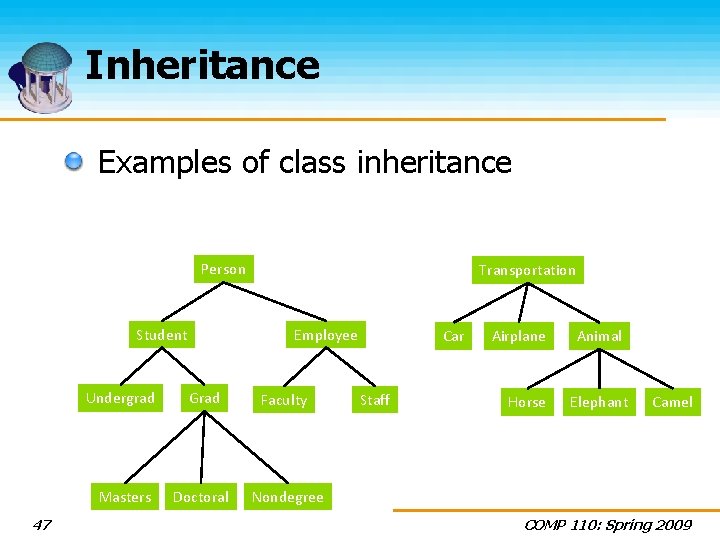
Inheritance Examples of class inheritance Person Student 47 Transportation Employee Undergrad Grad Faculty Masters Doctoral Nondegree Car Staff Airplane Horse Animal Elephant Camel COMP 110: Spring 2009
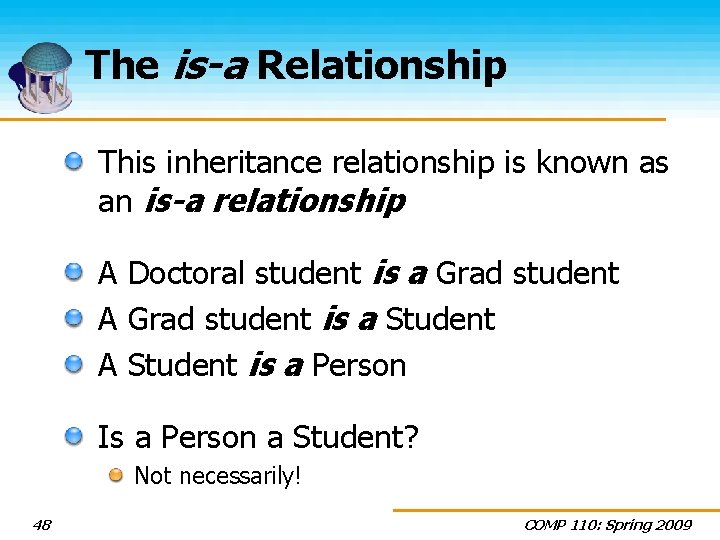
The is-a Relationship This inheritance relationship is known as an is-a relationship A Doctoral student is a Grad student A Grad student is a Student A Student is a Person Is a Person a Student? Not necessarily! 48 COMP 110: Spring 2009
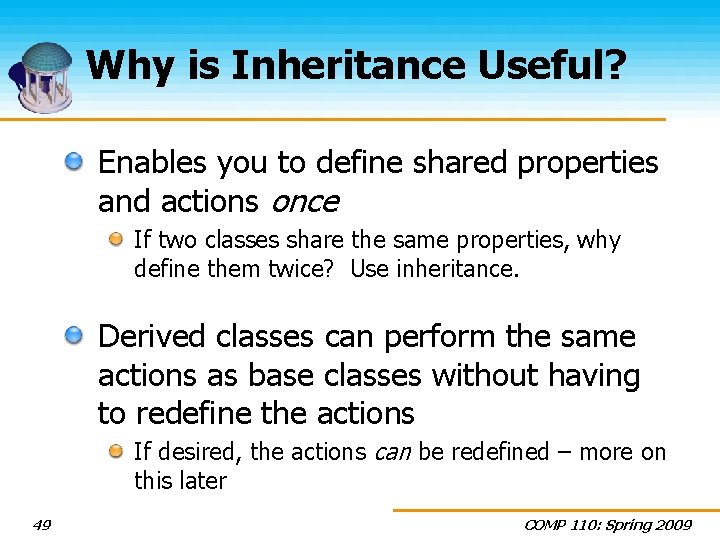
Why is Inheritance Useful? Enables you to define shared properties and actions once If two classes share the same properties, why define them twice? Use inheritance. Derived classes can perform the same actions as base classes without having to redefine the actions If desired, the actions can be redefined – more on this later 49 COMP 110: Spring 2009
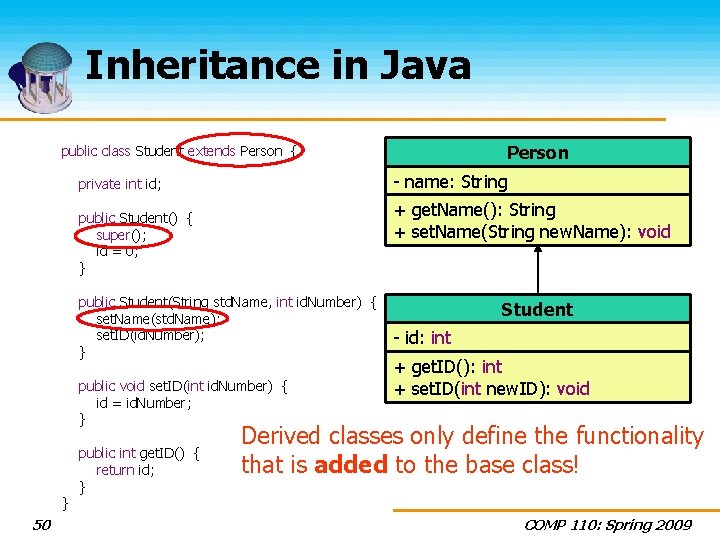
Inheritance in Java Person public class Student extends Person { - name: String private int id; + get. Name(): String + set. Name(String new. Name): void public Student() { super(); id = 0; } public Student(String std. Name, int id. Number) { set. Name(std. Name); set. ID(id. Number); } public void set. ID(int id. Number) { id = id. Number; } } 50 public int get. ID() { return id; } Student - id: int + get. ID(): int + set. ID(int new. ID): void Derived classes only define the functionality that is added to the base class! COMP 110: Spring 2009
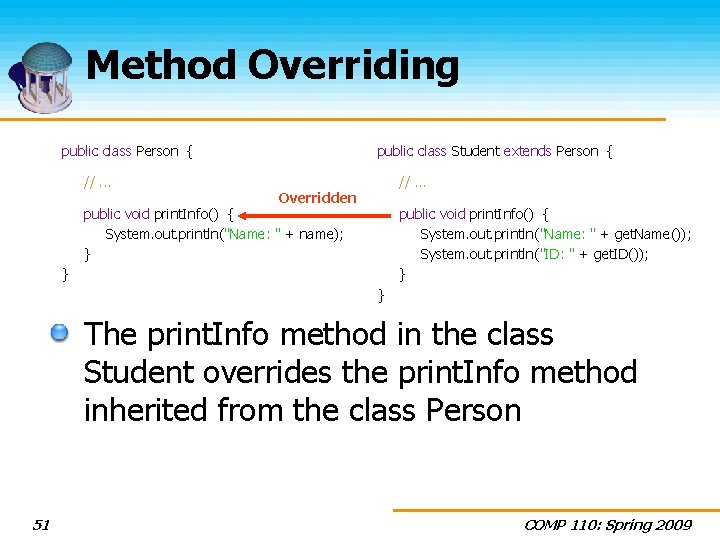
Method Overriding public class Person { //. . . public class Student extends Person { //. . . Overridden public void print. Info() { System. out. println("Name: " + name); } public void print. Info() { System. out. println("Name: " + get. Name()); System. out. println("ID: " + get. ID()); } } } The print. Info method in the class Student overrides the print. Info method inherited from the class Person 51 COMP 110: Spring 2009
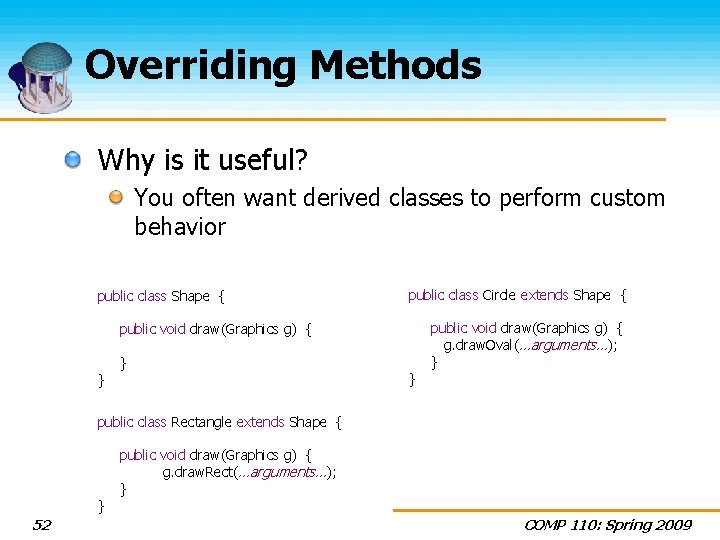
Overriding Methods Why is it useful? You often want derived classes to perform custom behavior public class Shape { public class Circle extends Shape { public void draw(Graphics g) { } } } public void draw(Graphics g) { g. draw. Oval(…arguments…); } public class Rectangle extends Shape { } 52 public void draw(Graphics g) { g. draw. Rect(…arguments…); } COMP 110: Spring 2009
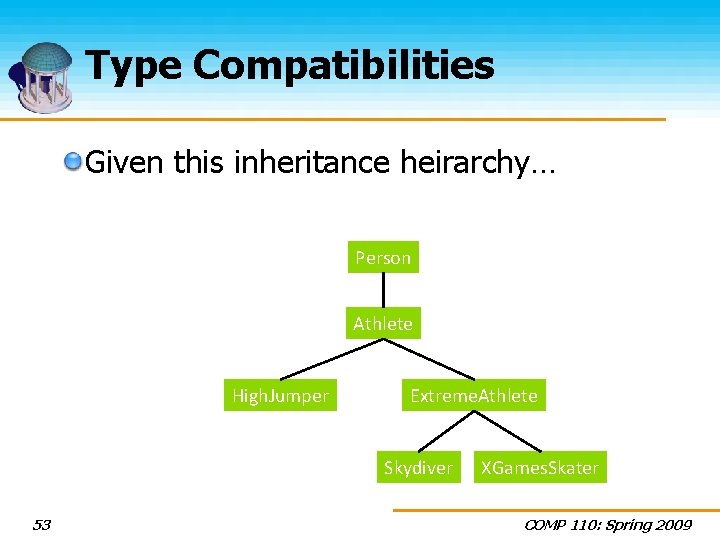
Type Compatibilities Given this inheritance heirarchy… Person Athlete High. Jumper Extreme. Athlete Skydiver 53 XGames. Skater COMP 110: Spring 2009
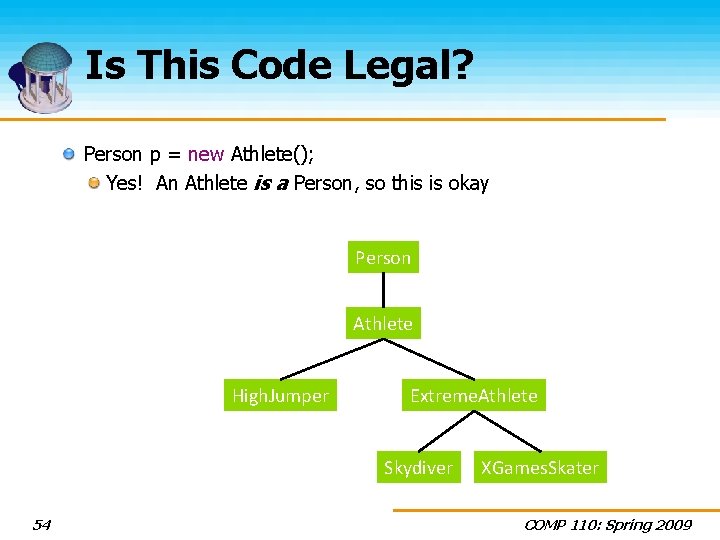
Is This Code Legal? Person p = new Athlete(); Yes! An Athlete is a Person, so this is okay Person Athlete High. Jumper Extreme. Athlete Skydiver 54 XGames. Skater COMP 110: Spring 2009
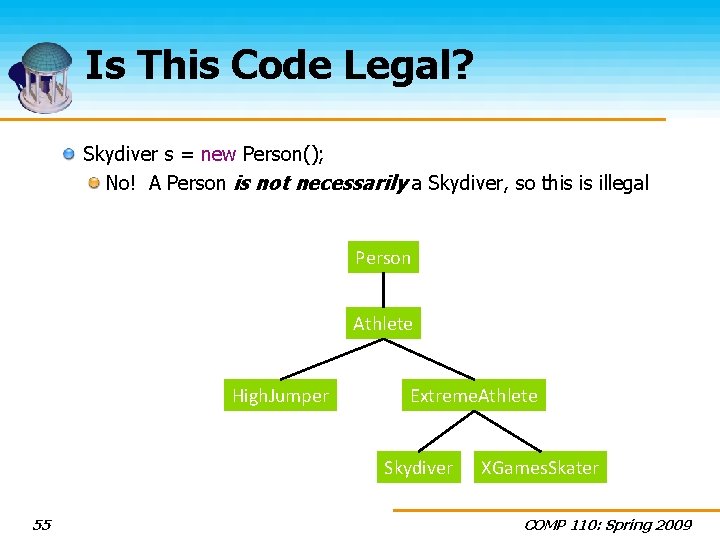
Is This Code Legal? Skydiver s = new Person(); No! A Person is not necessarily a Skydiver, so this is illegal Person Athlete High. Jumper Extreme. Athlete Skydiver 55 XGames. Skater COMP 110: Spring 2009
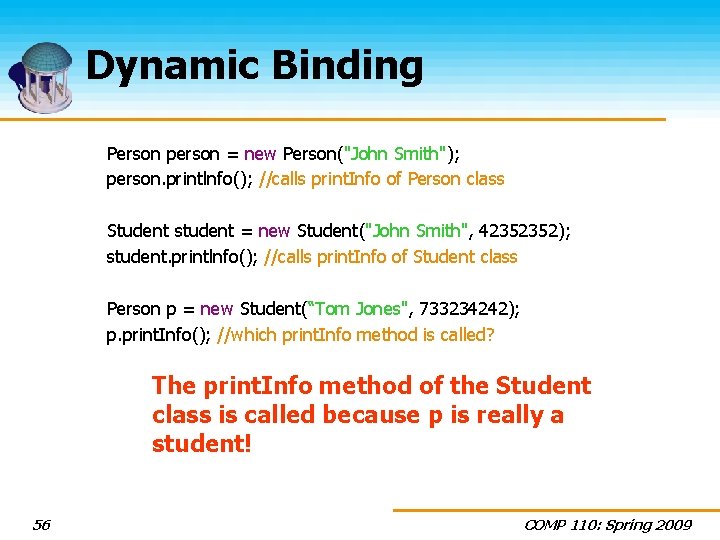
Dynamic Binding Person person = new Person("John Smith"); person. printlnfo(); //calls print. Info of Person class Student student = new Student("John Smith", 42352352); student. printlnfo(); //calls print. Info of Student class Person p = new Student(“Tom Jones", 733234242); p. print. Info(); //which print. Info method is called? The print. Info method of the Student class is called because p is really a student! 56 COMP 110: Spring 2009
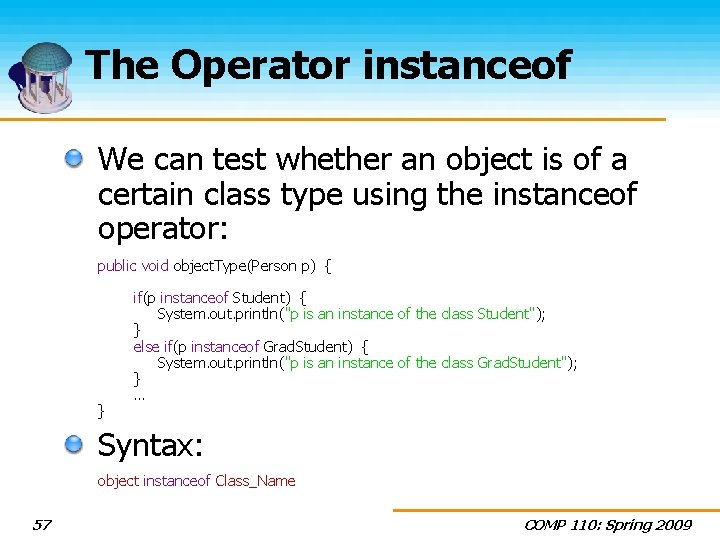
The Operator instanceof We can test whether an object is of a certain class type using the instanceof operator: public void object. Type(Person p) { } if(p instanceof Student) { System. out. println("p is an instance of the class Student"); } else if(p instanceof Grad. Student) { System. out. println("p is an instance of the class Grad. Student"); } … Syntax: object instanceof Class_Name 57 COMP 110: Spring 2009
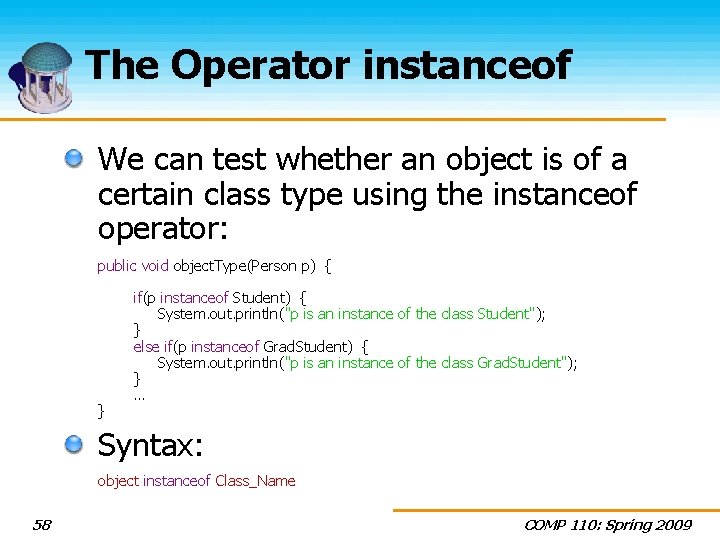
The Operator instanceof We can test whether an object is of a certain class type using the instanceof operator: public void object. Type(Person p) { } if(p instanceof Student) { System. out. println("p is an instance of the class Student"); } else if(p instanceof Grad. Student) { System. out. println("p is an instance of the class Grad. Student"); } … Syntax: object instanceof Class_Name 58 COMP 110: Spring 2009
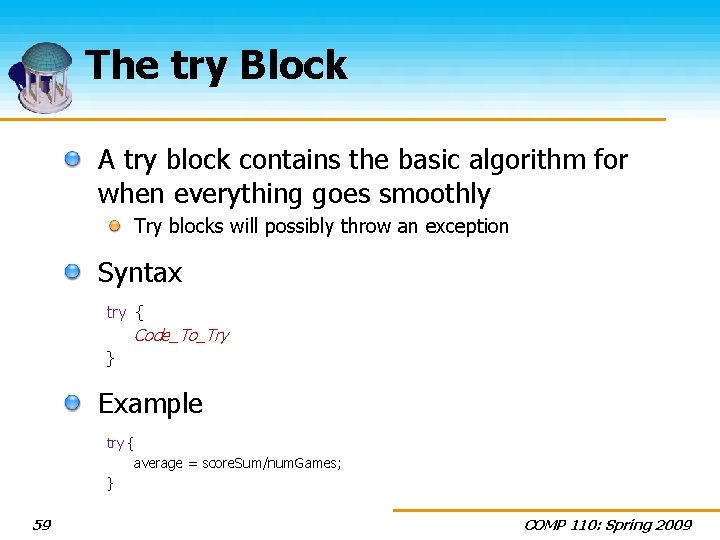
The try Block A try block contains the basic algorithm for when everything goes smoothly Try blocks will possibly throw an exception Syntax try { Code_To_Try } Example try { average = score. Sum/num. Games; } 59 COMP 110: Spring 2009
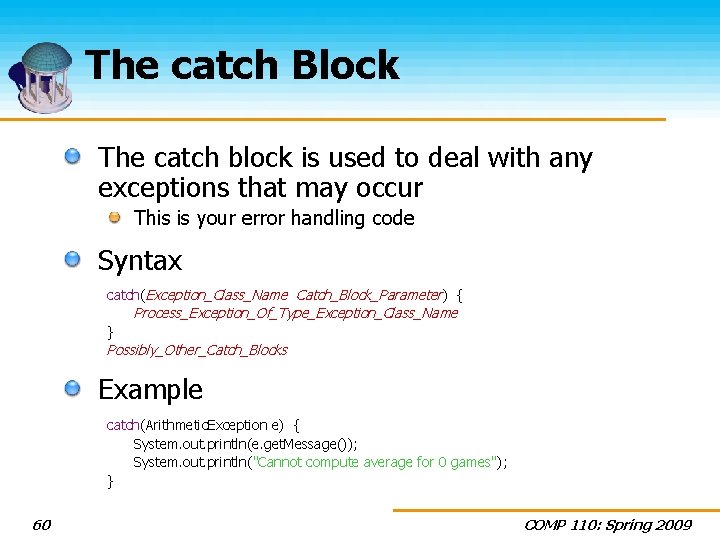
The catch Block The catch block is used to deal with any exceptions that may occur This is your error handling code Syntax catch(Exception_Class_Name Catch_Block_Parameter) { Process_Exception_Of_Type_Exception_Class_Name } Possibly_Other_Catch_Blocks Example catch(Arithmetic. Exception e) { System. out. println(e. get. Message()); System. out. println("Cannot compute average for 0 games"); } 60 COMP 110: Spring 2009
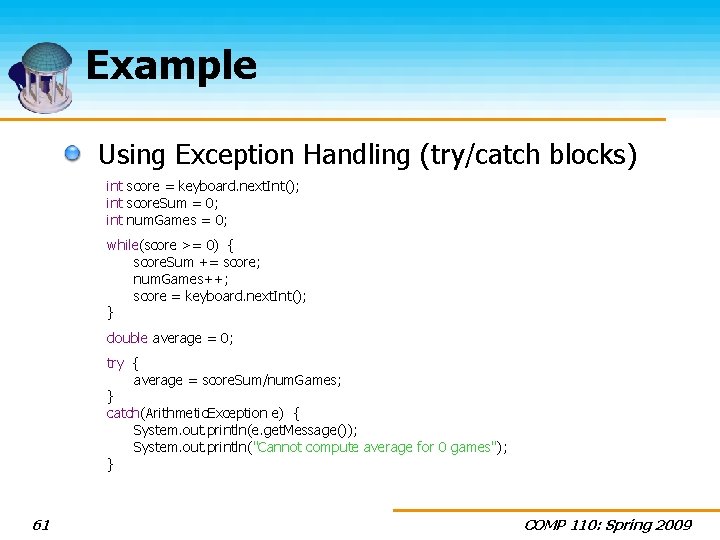
Example Using Exception Handling (try/catch blocks) int score = keyboard. next. Int(); int score. Sum = 0; int num. Games = 0; while(score >= 0) { score. Sum += score; num. Games++; score = keyboard. next. Int(); } double average = 0; try { average = score. Sum/num. Games; } catch(Arithmetic. Exception e) { System. out. println(e. get. Message()); System. out. println("Cannot compute average for 0 games"); } 61 COMP 110: Spring 2009
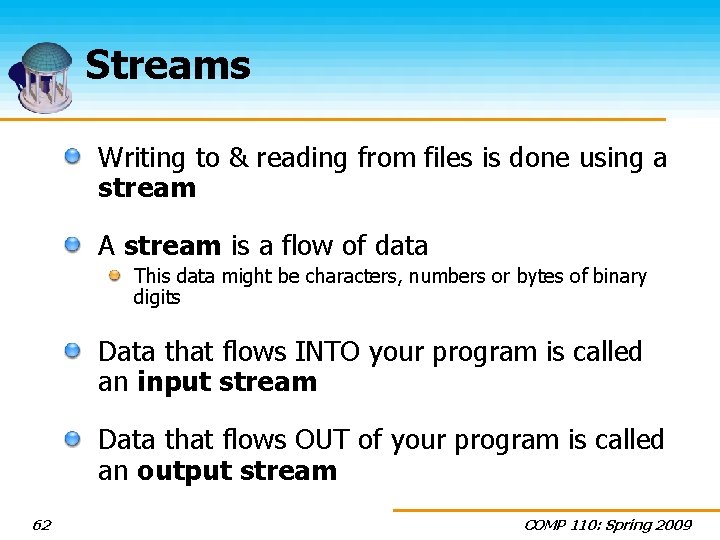
Streams Writing to & reading from files is done using a stream A stream is a flow of data This data might be characters, numbers or bytes of binary digits Data that flows INTO your program is called an input stream Data that flows OUT of your program is called an output stream 62 COMP 110: Spring 2009
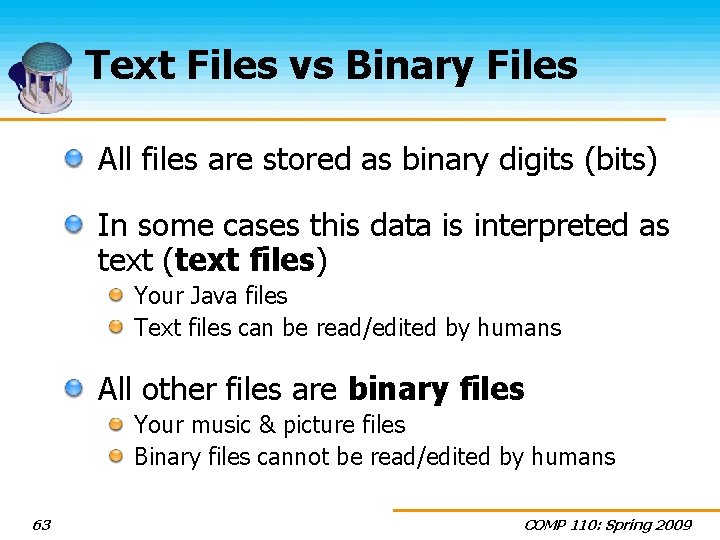
Text Files vs Binary Files All files are stored as binary digits (bits) In some cases this data is interpreted as text (text files) Your Java files Text files can be read/edited by humans All other files are binary files Your music & picture files Binary files cannot be read/edited by humans 63 COMP 110: Spring 2009
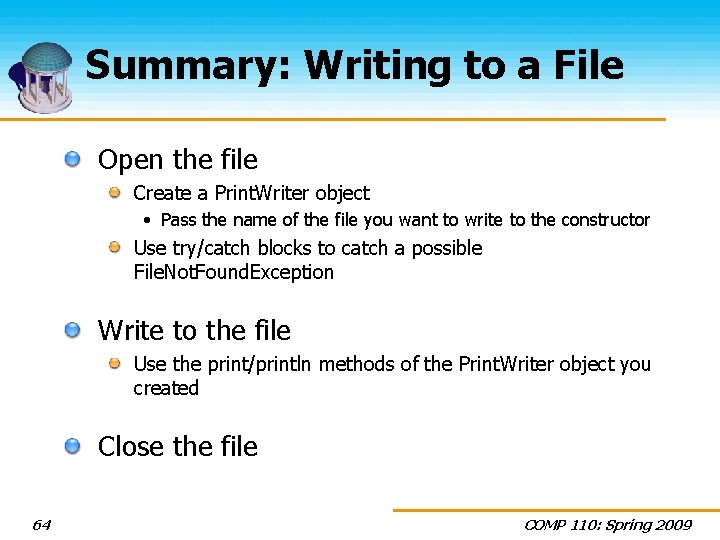
Summary: Writing to a File Open the file Create a Print. Writer object • Pass the name of the file you want to write to the constructor Use try/catch blocks to catch a possible File. Not. Found. Exception Write to the file Use the print/println methods of the Print. Writer object you created Close the file 64 COMP 110: Spring 2009
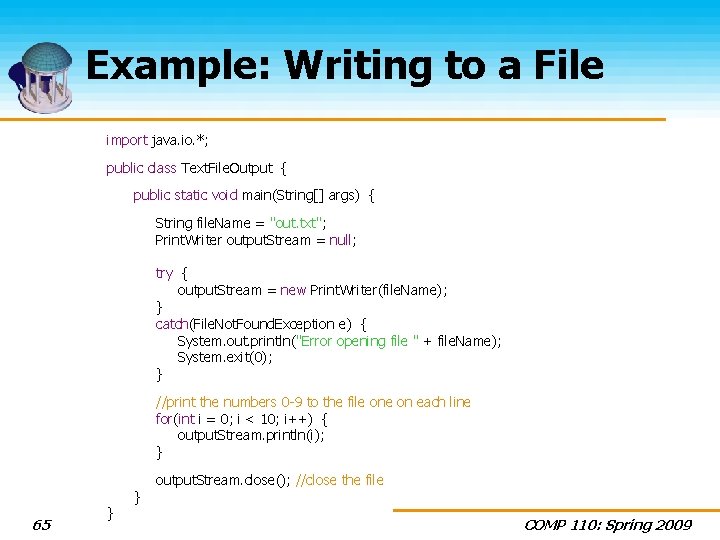
Example: Writing to a File import java. io. *; public class Text. File. Output { public static void main(String[] args) { String file. Name = "out. txt"; Print. Writer output. Stream = null; try { output. Stream = new Print. Writer(file. Name); } catch(File. Not. Found. Exception e) { System. out. println("Error opening file " + file. Name); System. exit(0); } //print the numbers 0 -9 to the file on each line for(int i = 0; i < 10; i++) { output. Stream. println(i); } 65 } } output. Stream. close(); //close the file COMP 110: Spring 2009
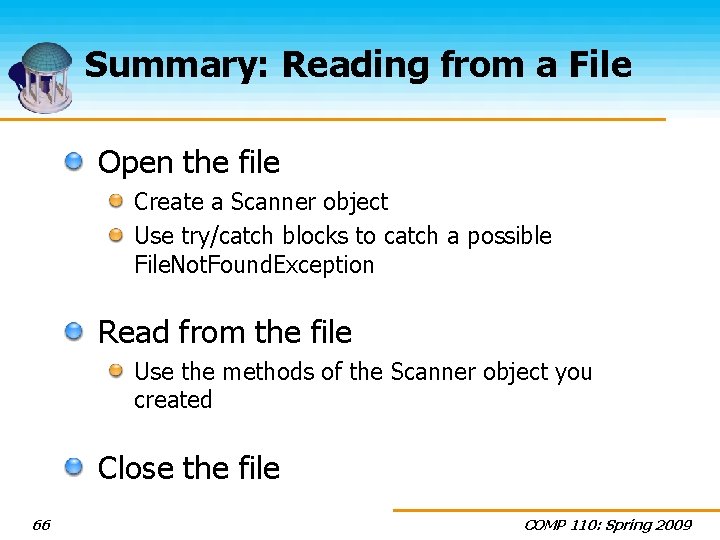
Summary: Reading from a File Open the file Create a Scanner object Use try/catch blocks to catch a possible File. Not. Found. Exception Read from the file Use the methods of the Scanner object you created Close the file 66 COMP 110: Spring 2009
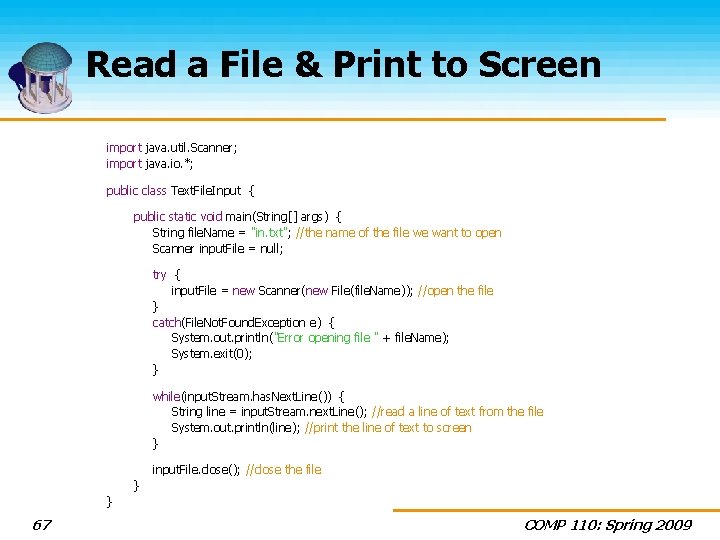
Read a File & Print to Screen import java. util. Scanner; import java. io. *; public class Text. File. Input { public static void main(String[] args) { String file. Name = "in. txt"; //the name of the file we want to open Scanner input. File = null; try { input. File = new Scanner(new File(file. Name)); //open the file } catch(File. Not. Found. Exception e) { System. out. println("Error opening file " + file. Name); System. exit(0); } while(input. Stream. has. Next. Line()) { String line = input. Stream. next. Line(); //read a line of text from the file System. out. println(line); //print the line of text to screen } input. File. close(); //close the file } } 67 COMP 110: Spring 2009
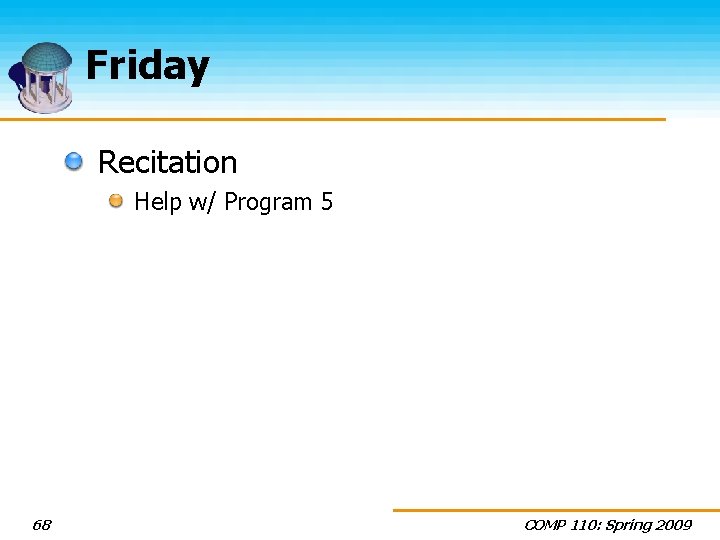
Friday Recitation Help w/ Program 5 68 COMP 110: Spring 2009