COMP 110 Introduction to Programming Tyler Johnson Mar
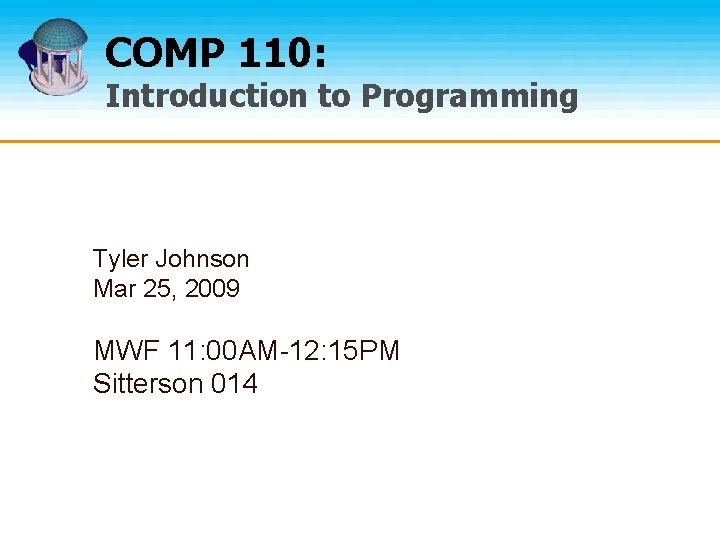
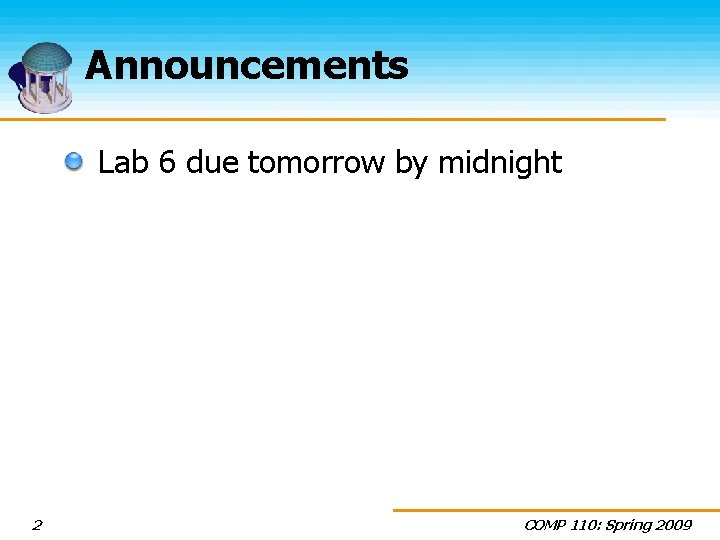
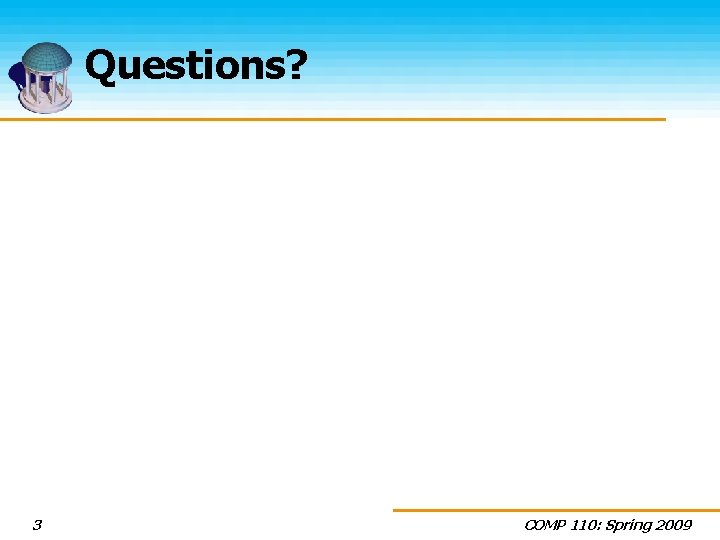
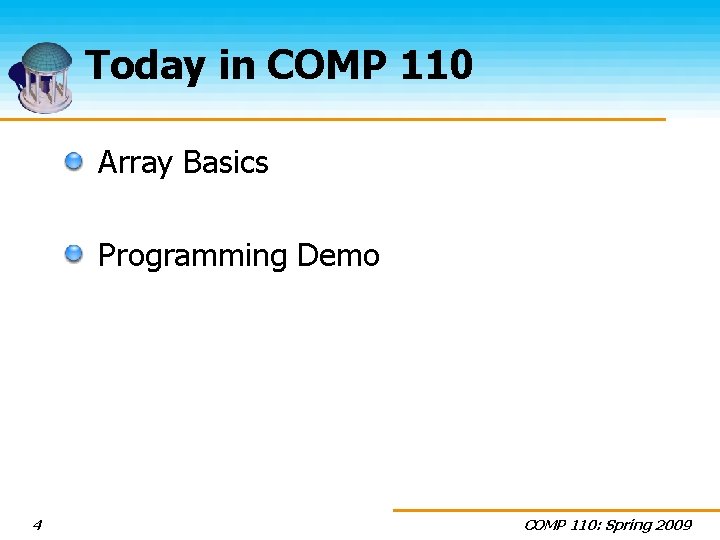
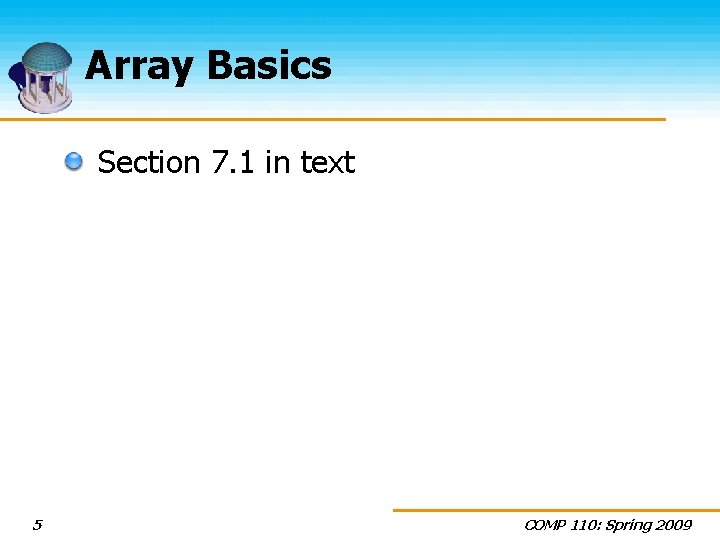
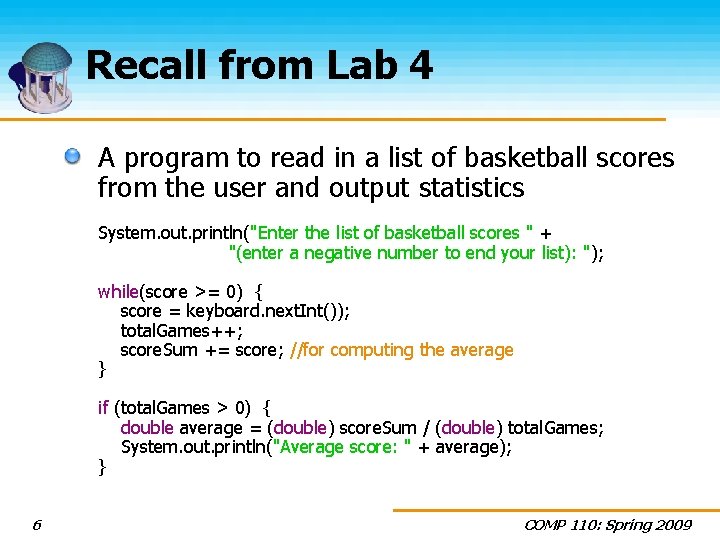
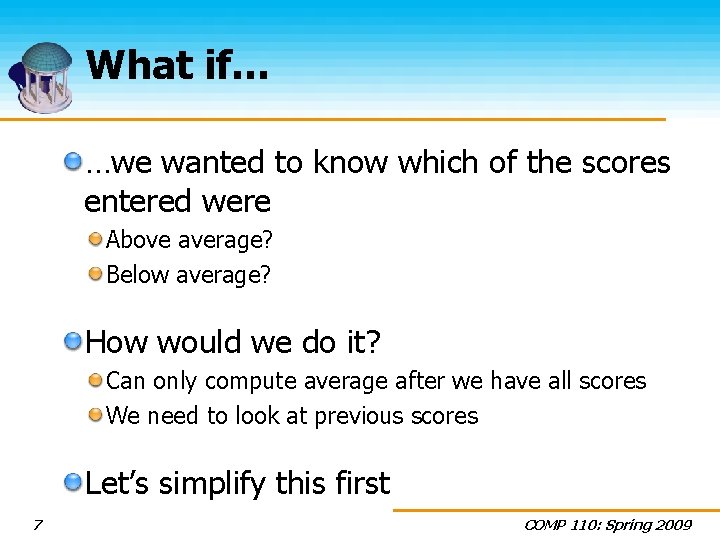
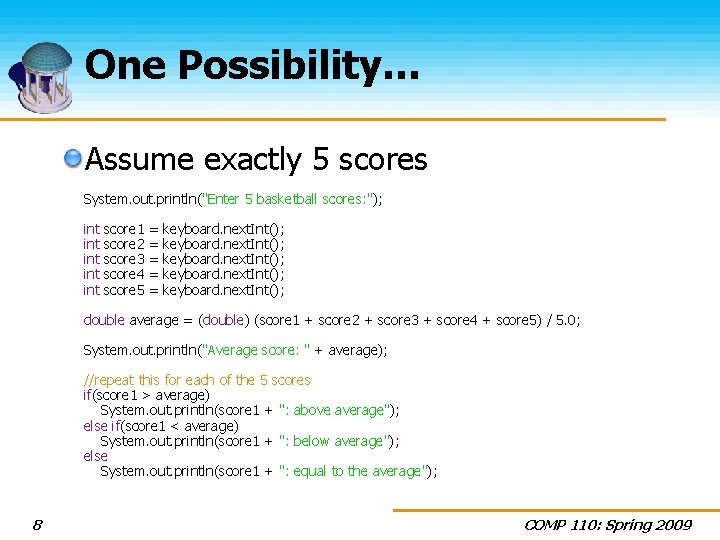
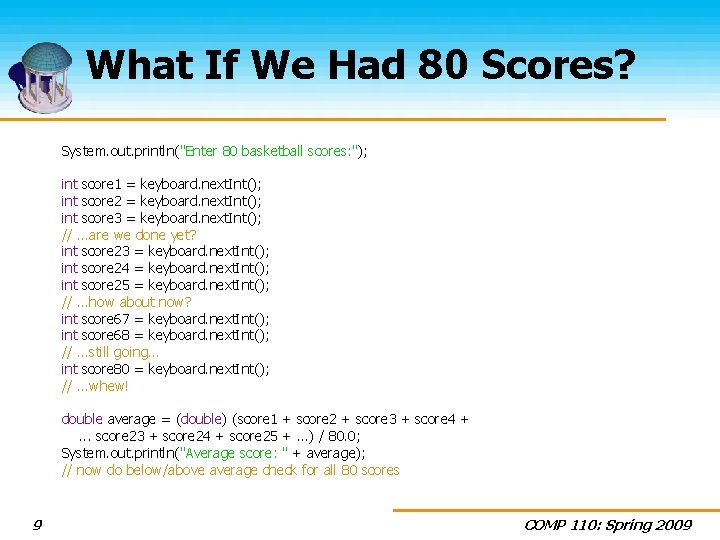
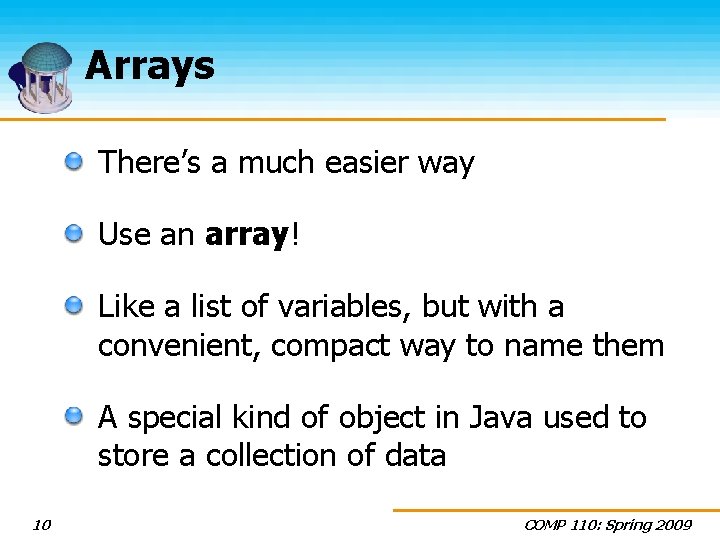
![Creating an Array int[] scores = new int[5]; //create 5 ints This creates an Creating an Array int[] scores = new int[5]; //create 5 ints This creates an](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-11.jpg)
![Indexing Variables such as scores[0] and scores[1] that have an integer expression in square Indexing Variables such as scores[0] and scores[1] that have an integer expression in square](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-12.jpg)
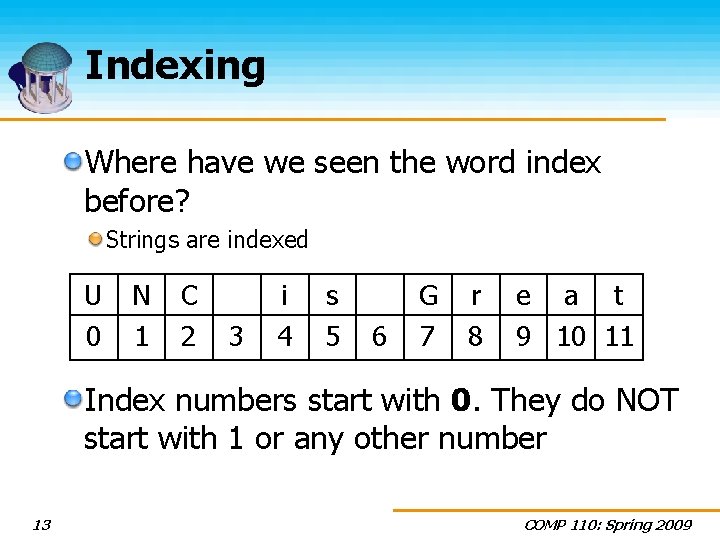
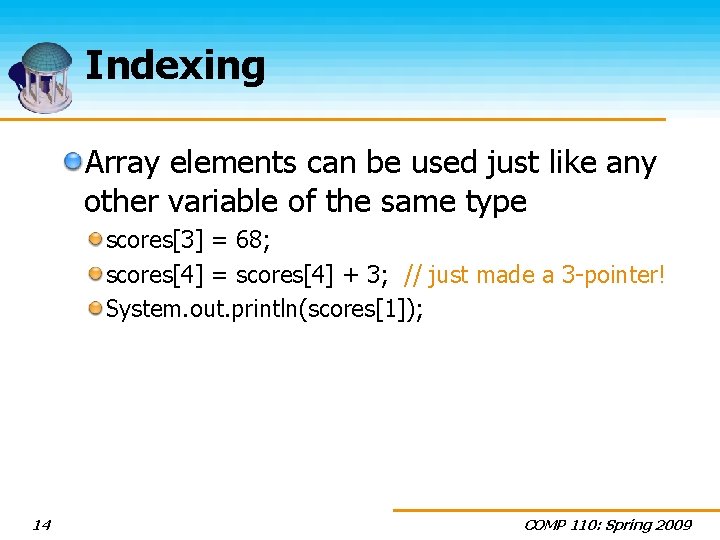
![Vector Example double[] vector = new double[3]; //an array of 3 doubles (x, y, Vector Example double[] vector = new double[3]; //an array of 3 doubles (x, y,](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-15.jpg)
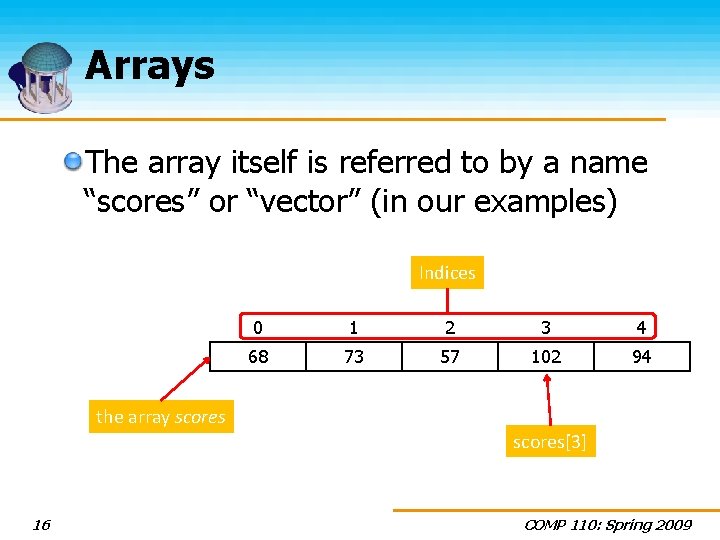
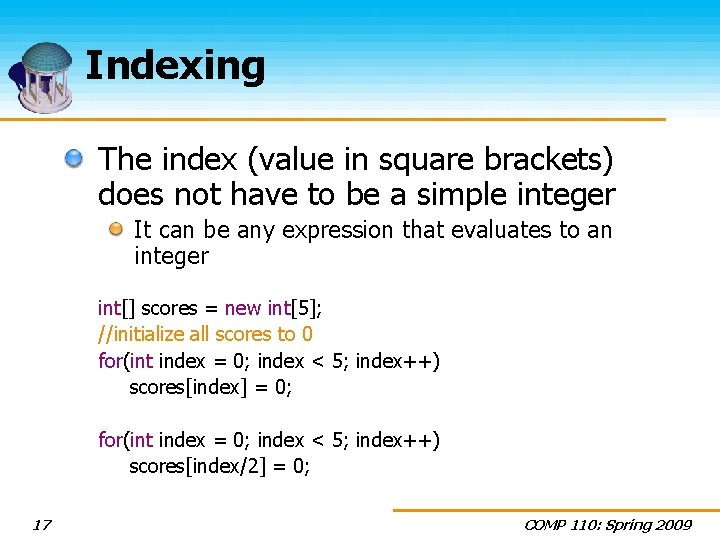
![Array Terminology Indexed Variable scores[index/2] = 0; Array name 18 Index COMP 110: Spring Array Terminology Indexed Variable scores[index/2] = 0; Array name 18 Index COMP 110: Spring](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-18.jpg)
![Reading in Scores System. out. println("Enter 5 basketball scores: "); int[] scores = new Reading in Scores System. out. println("Enter 5 basketball scores: "); int[] scores = new](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-19.jpg)
![Array Details Syntax for creating an array: Base_Type[] Array_Name = new Base_Type[Length]; Example: int[] Array Details Syntax for creating an array: Base_Type[] Array_Name = new Base_Type[Length]; Example: int[]](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-20.jpg)
![Array Details The base type can be any type double[] temperature = new double[7]; Array Details The base type can be any type double[] temperature = new double[7];](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-21.jpg)
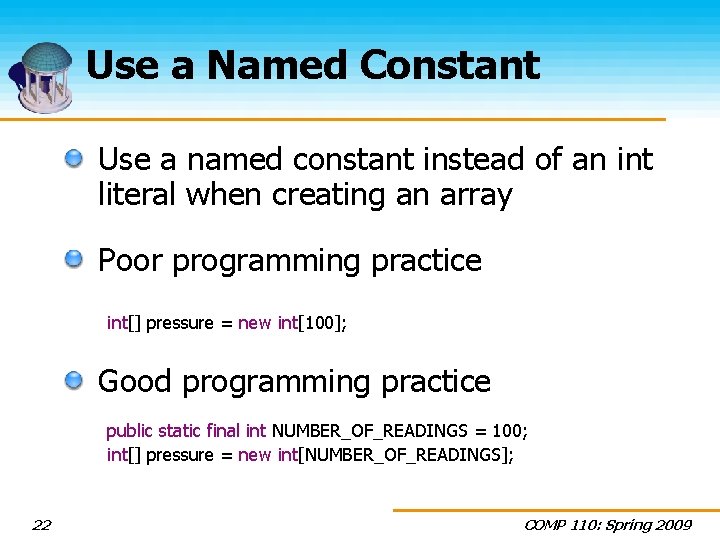
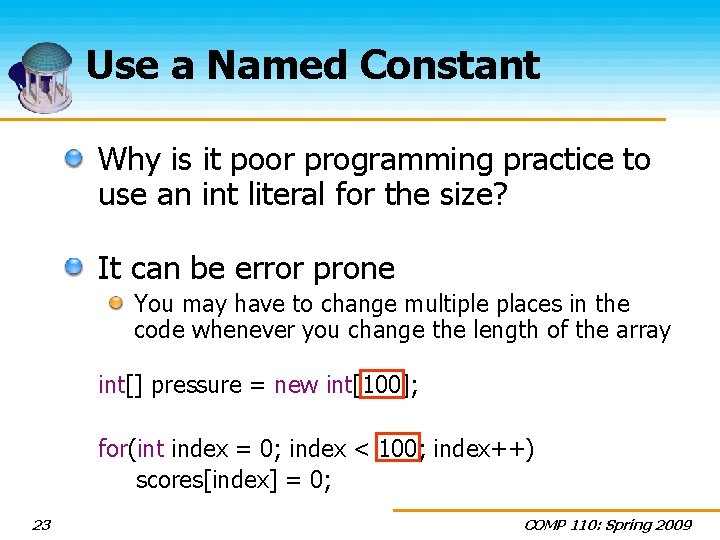
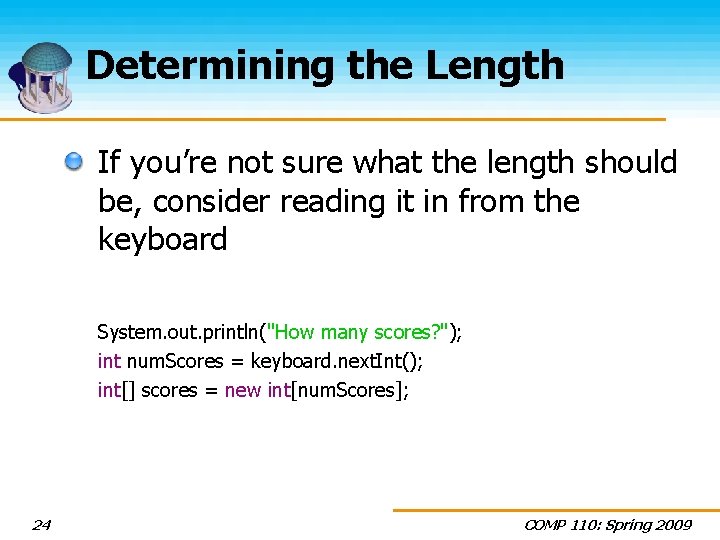
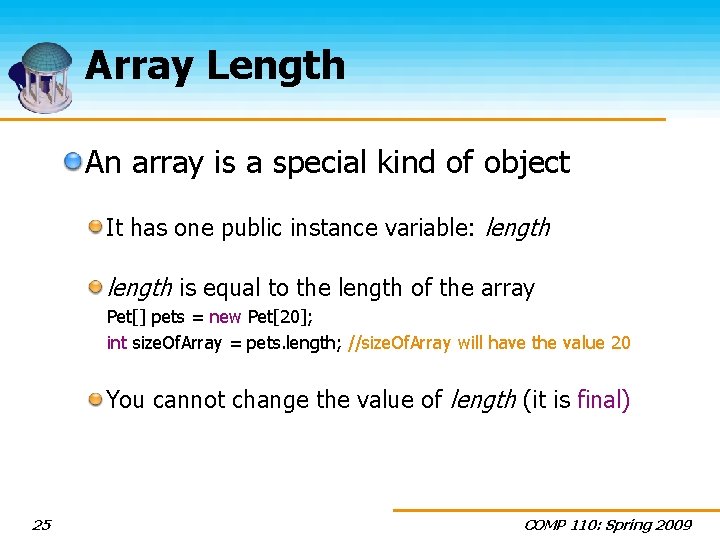
![Our Previous Example System. out. println("Enter 5 basketball scores: "); int[] scores = new Our Previous Example System. out. println("Enter 5 basketball scores: "); int[] scores = new](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-26.jpg)
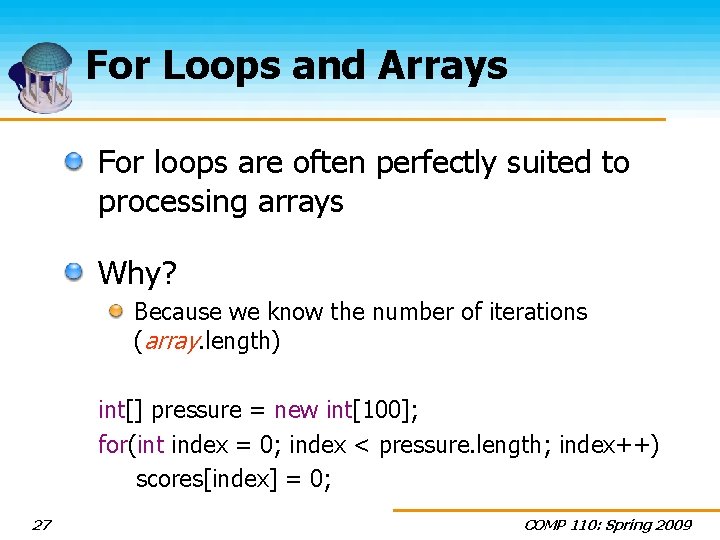
![Be Careful with Indices MUST be in bounds double[] entries = new double[5]; entries[5] Be Careful with Indices MUST be in bounds double[] entries = new double[5]; entries[5]](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-28.jpg)
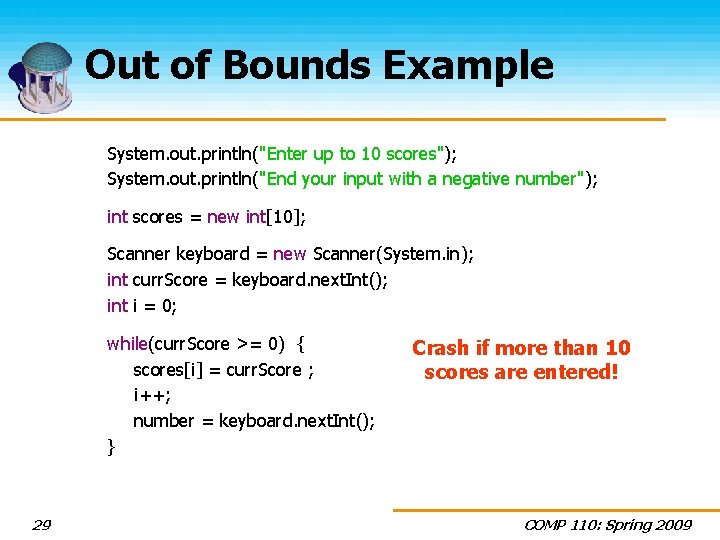
![Initializing Arrays You can initialize arrays when you declare them int[] scores = {68, Initializing Arrays You can initialize arrays when you declare them int[] scores = {68,](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-30.jpg)
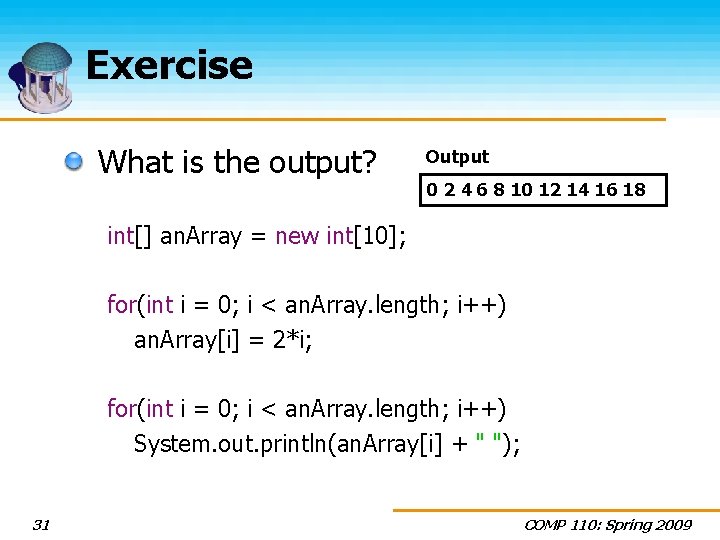
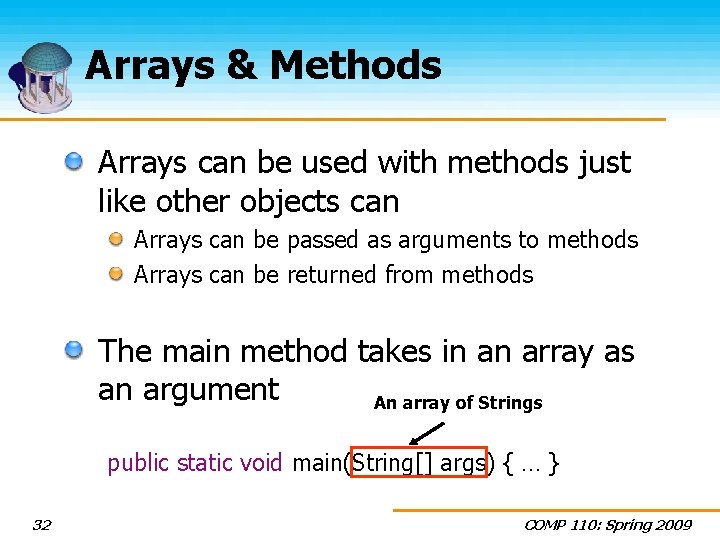
![Arrays as Arguments Example //init all array elements to 0 public void init. Array(int[] Arrays as Arguments Example //init all array elements to 0 public void init. Array(int[]](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-33.jpg)
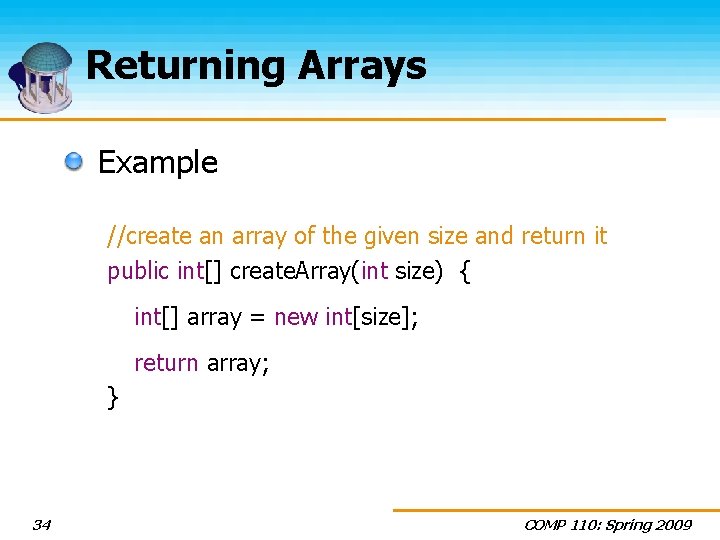
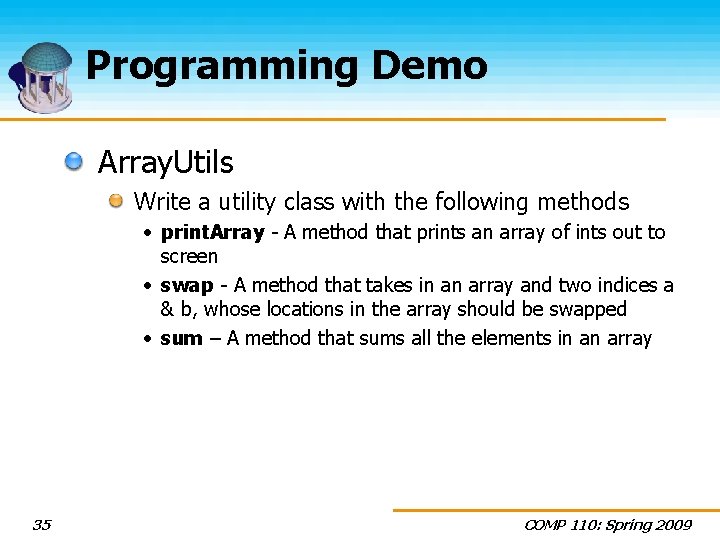
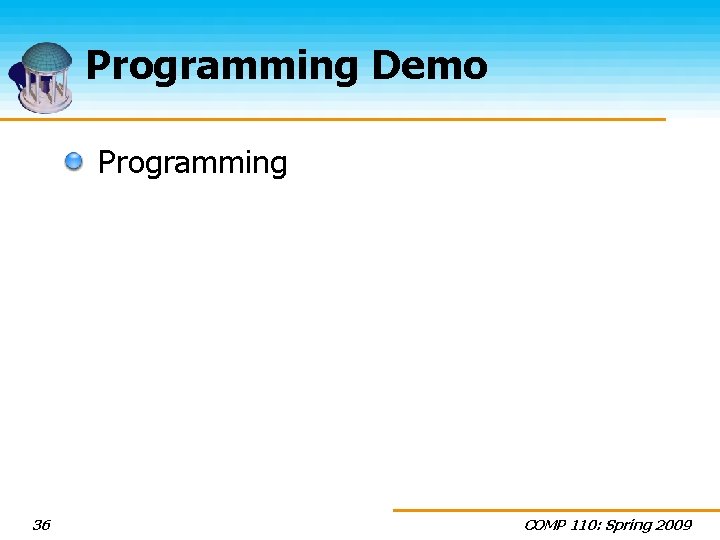
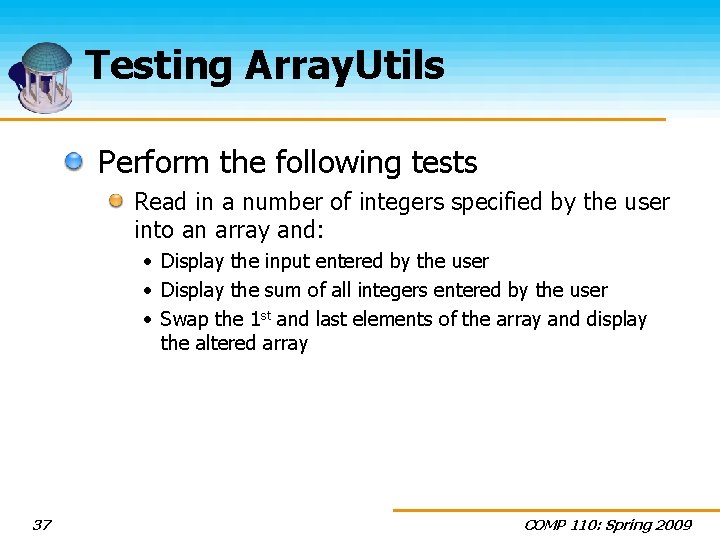
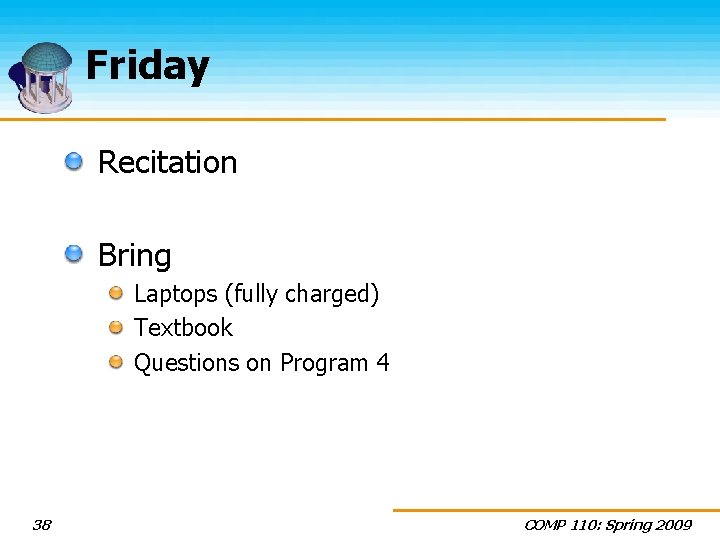
- Slides: 38
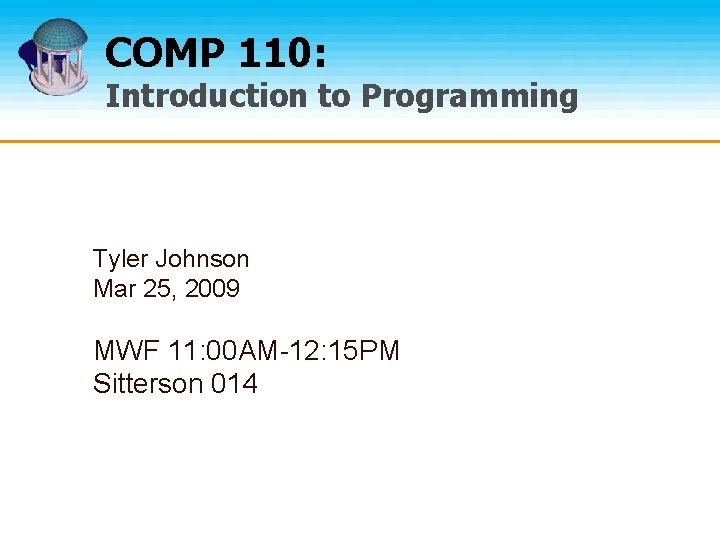
COMP 110: Introduction to Programming Tyler Johnson Mar 25, 2009 MWF 11: 00 AM-12: 15 PM Sitterson 014
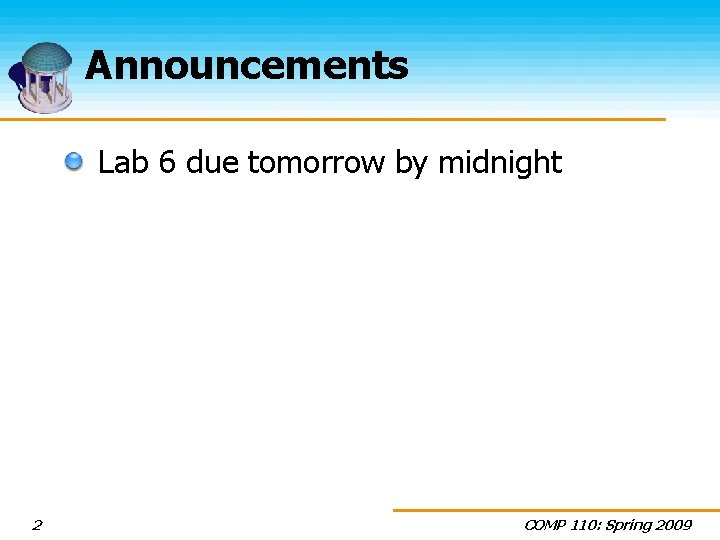
Announcements Lab 6 due tomorrow by midnight 2 COMP 110: Spring 2009
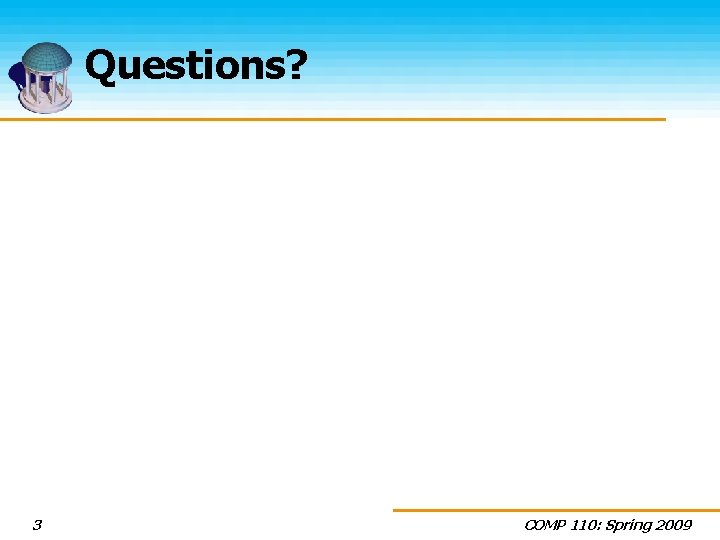
Questions? 3 COMP 110: Spring 2009
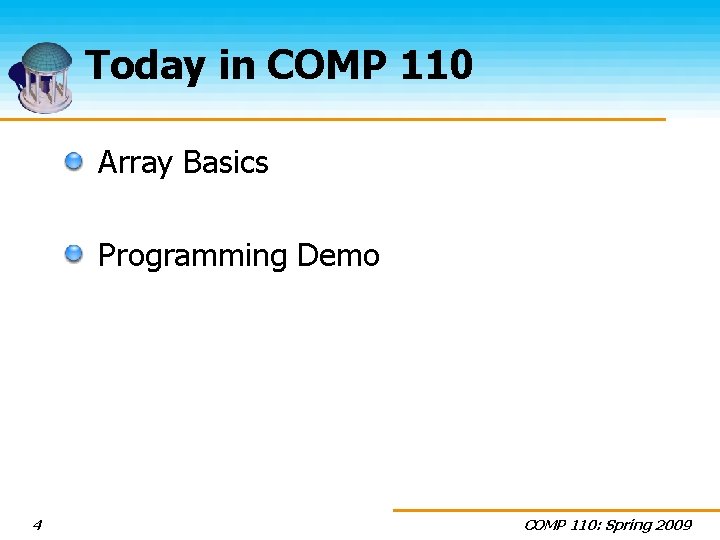
Today in COMP 110 Array Basics Programming Demo 4 COMP 110: Spring 2009
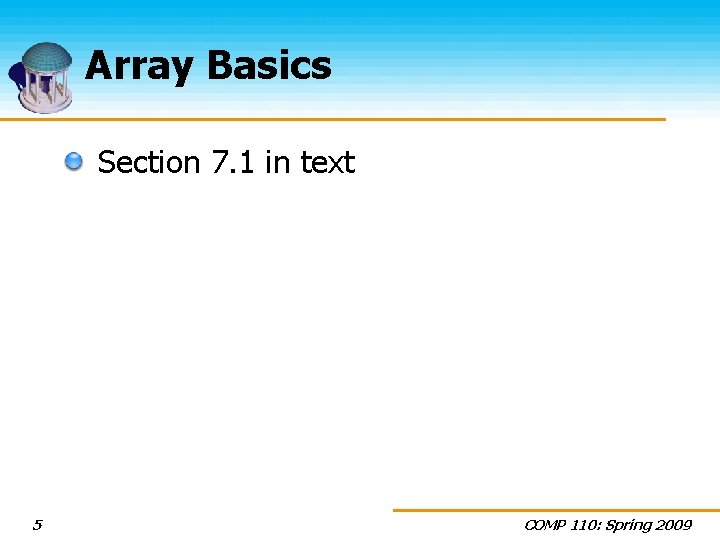
Array Basics Section 7. 1 in text 5 COMP 110: Spring 2009
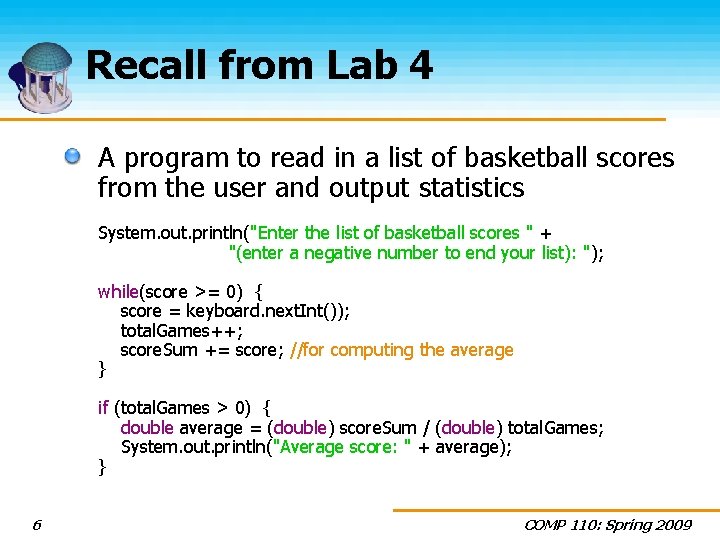
Recall from Lab 4 A program to read in a list of basketball scores from the user and output statistics System. out. println("Enter the list of basketball scores " + "(enter a negative number to end your list): "); while(score >= 0) { score = keyboard. next. Int()); total. Games++; score. Sum += score; //for computing the average } if (total. Games > 0) { double average = (double) score. Sum / (double) total. Games; System. out. println("Average score: " + average); } 6 COMP 110: Spring 2009
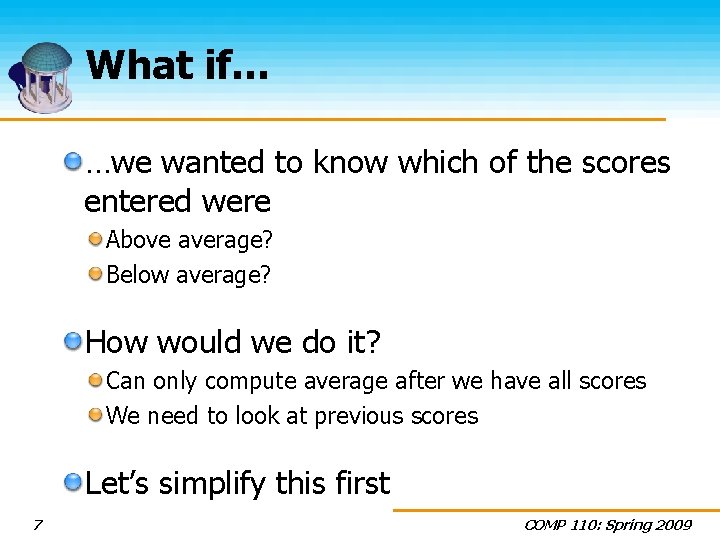
What if… …we wanted to know which of the scores entered were Above average? Below average? How would we do it? Can only compute average after we have all scores We need to look at previous scores Let’s simplify this first 7 COMP 110: Spring 2009
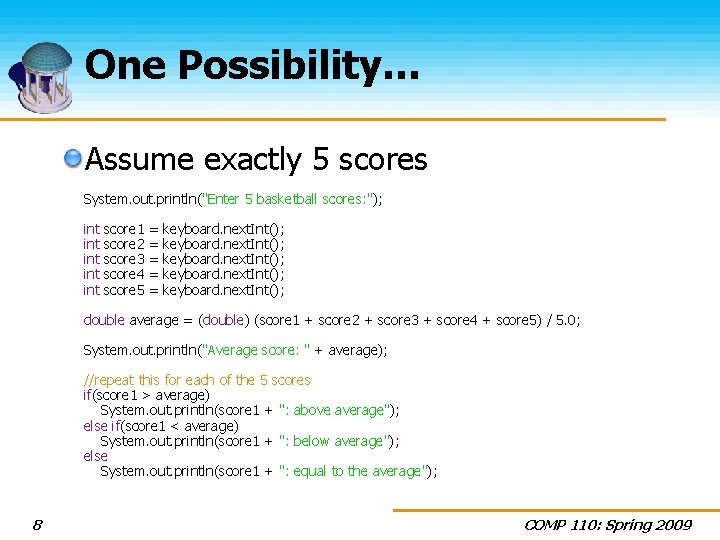
One Possibility… Assume exactly 5 scores System. out. println("Enter 5 basketball scores: "); int int int score 1 score 2 score 3 score 4 score 5 = = = keyboard. next. Int(); double average = (double) (score 1 + score 2 + score 3 + score 4 + score 5) / 5. 0; System. out. println("Average score: " + average); //repeat this for each of the 5 scores if(score 1 > average) System. out. println(score 1 + ": above average"); else if(score 1 < average) System. out. println(score 1 + ": below average"); else System. out. println(score 1 + ": equal to the average"); 8 COMP 110: Spring 2009
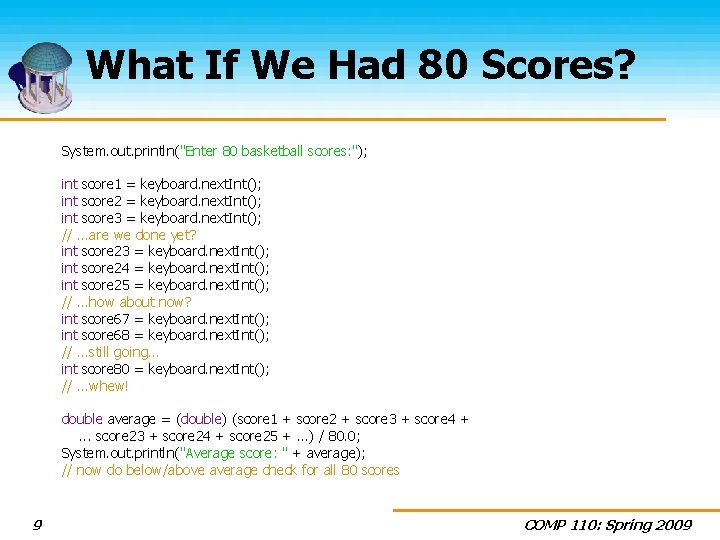
What If We Had 80 Scores? System. out. println("Enter 80 basketball scores: "); int score 1 = keyboard. next. Int(); int score 2 = keyboard. next. Int(); int score 3 = keyboard. next. Int(); //. . . are we done yet? int score 23 = keyboard. next. Int(); int score 24 = keyboard. next. Int(); int score 25 = keyboard. next. Int(); //. . . how about now? int score 67 = keyboard. next. Int(); int score 68 = keyboard. next. Int(); //. . . still going… int score 80 = keyboard. next. Int(); //. . . whew! double average = (double) (score 1 + score 2 + score 3 + score 4 +. . . score 23 + score 24 + score 25 +. . . ) / 80. 0; System. out. println("Average score: " + average); // now do below/above average check for all 80 scores 9 COMP 110: Spring 2009
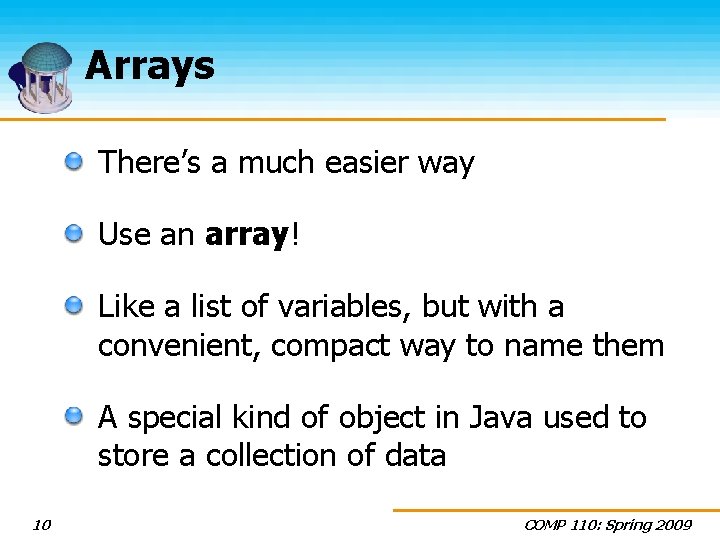
Arrays There’s a much easier way Use an array! Like a list of variables, but with a convenient, compact way to name them A special kind of object in Java used to store a collection of data 10 COMP 110: Spring 2009
![Creating an Array int scores new int5 create 5 ints This creates an Creating an Array int[] scores = new int[5]; //create 5 ints This creates an](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-11.jpg)
Creating an Array int[] scores = new int[5]; //create 5 ints This creates an array called “scores” that holds five integer values scores[0] //a single integer, the 1 st integer in the array scores[1] //a single integer, the 2 nd integer in the array scores[2] scores[3] scores[4] //a single integer, the 5 th and last integer in the array 11 COMP 110: Spring 2009
![Indexing Variables such as scores0 and scores1 that have an integer expression in square Indexing Variables such as scores[0] and scores[1] that have an integer expression in square](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-12.jpg)
Indexing Variables such as scores[0] and scores[1] that have an integer expression in square brackets are known as: indexed variables, subscripted variables, array elements, or simply elements An index or subscript is an integer expression inside the square brackets that indicates an array element 12 COMP 110: Spring 2009
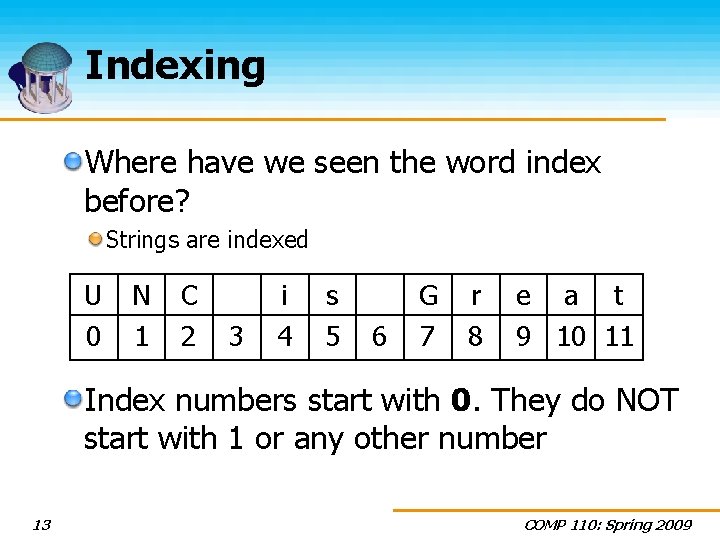
Indexing Where have we seen the word index before? Strings are indexed U 0 N 1 C 2 3 i 4 s 5 6 G 7 r 8 e a t 9 10 11 Index numbers start with 0. They do NOT start with 1 or any other number 13 COMP 110: Spring 2009
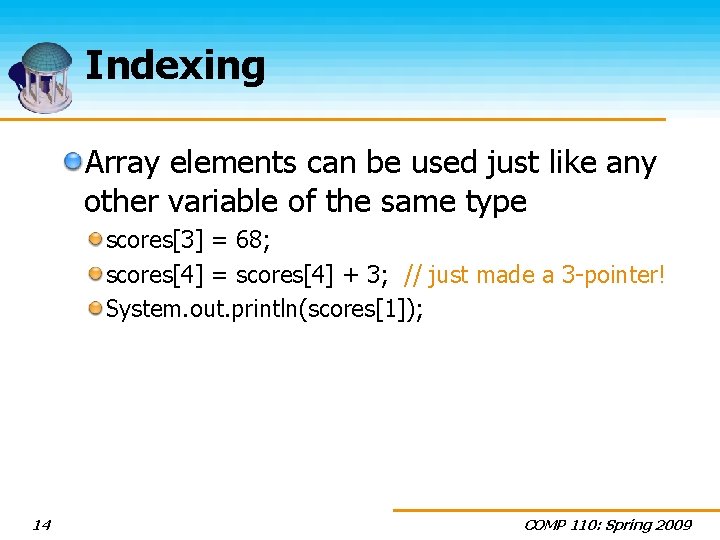
Indexing Array elements can be used just like any other variable of the same type scores[3] = 68; scores[4] = scores[4] + 3; // just made a 3 -pointer! System. out. println(scores[1]); 14 COMP 110: Spring 2009
![Vector Example double vector new double3 an array of 3 doubles x y Vector Example double[] vector = new double[3]; //an array of 3 doubles (x, y,](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-15.jpg)
Vector Example double[] vector = new double[3]; //an array of 3 doubles (x, y, z) vector[0] = 1. ; //set the x value vector[1] = 56. ; //set the y value vector[2] = 101. ; //set the z value (x, y, z) //compute the length of the vector double length = Math. sqrt(vector[0]*vector [0] + vector [1]*vector [1] + vector [2]*vector [2]); //normalize the vector double[] vector. N = new double[3]; vector. N[0] = vector[0]/length; vector. N[1] = vector[1]/length; vector. N[2] = vector[2]/length; 15 COMP 110: Spring 2009
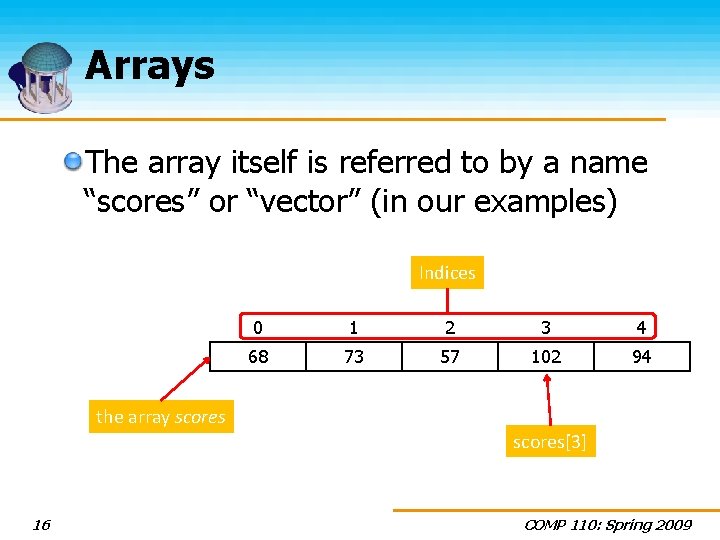
Arrays The array itself is referred to by a name “scores” or “vector” (in our examples) Indices 0 1 2 3 4 68 73 57 102 94 the array scores[3] 16 COMP 110: Spring 2009
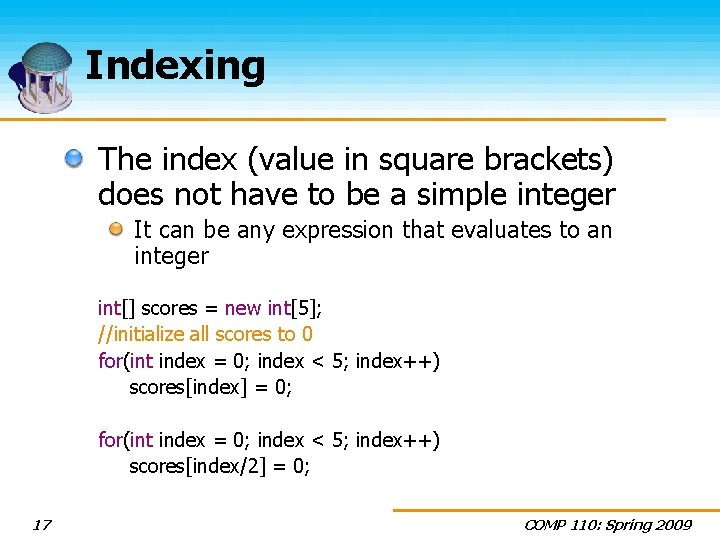
Indexing The index (value in square brackets) does not have to be a simple integer It can be any expression that evaluates to an integer int[] scores = new int[5]; //initialize all scores to 0 for(int index = 0; index < 5; index++) scores[index] = 0; for(int index = 0; index < 5; index++) scores[index/2] = 0; 17 COMP 110: Spring 2009
![Array Terminology Indexed Variable scoresindex2 0 Array name 18 Index COMP 110 Spring Array Terminology Indexed Variable scores[index/2] = 0; Array name 18 Index COMP 110: Spring](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-18.jpg)
Array Terminology Indexed Variable scores[index/2] = 0; Array name 18 Index COMP 110: Spring 2009
![Reading in Scores System out printlnEnter 5 basketball scores int scores new Reading in Scores System. out. println("Enter 5 basketball scores: "); int[] scores = new](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-19.jpg)
Reading in Scores System. out. println("Enter 5 basketball scores: "); int[] scores = new int[5]; int score. Sum = 0; for(int i = 0; i < 5; i++) { scores[i] = keyboard. next. Int(); score. Sum += scores[i]; } double average = (double) score. Sum / 5; System. out. println("Average score: " + average); for(int i = 0; i < 5; i++) { if(scores[i] > average) System. out. println(scores[i] + ": above average"); else if(scores[i] < average) System. out. println(scores[i] + ": below average"); else System. out. println(scores[i] + ": equal to the average"); } 19 COMP 110: Spring 2009
![Array Details Syntax for creating an array BaseType ArrayName new BaseTypeLength Example int Array Details Syntax for creating an array: Base_Type[] Array_Name = new Base_Type[Length]; Example: int[]](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-20.jpg)
Array Details Syntax for creating an array: Base_Type[] Array_Name = new Base_Type[Length]; Example: int[] pressure = new int[100]; //create 100 variables of type int that can //be referred to collectively Alternatively: int[] pressure; //declare an integer array called pressure = new int[100]; //allocate memory for the array to hold 100 ints 20 COMP 110: Spring 2009
![Array Details The base type can be any type double temperature new double7 Array Details The base type can be any type double[] temperature = new double[7];](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-21.jpg)
Array Details The base type can be any type double[] temperature = new double[7]; Student[] students = new Student[35]; Pet[] my. Pets = new Pet[3]; The number of elements in an array is its length, size, or capacity temperature has 7 elements, temperature[0] through temperature[6] students has 35 elements, students[0] through students[34] my. Pets has 3 elements, my. Pets[0] through my. Pets[2] 21 COMP 110: Spring 2009
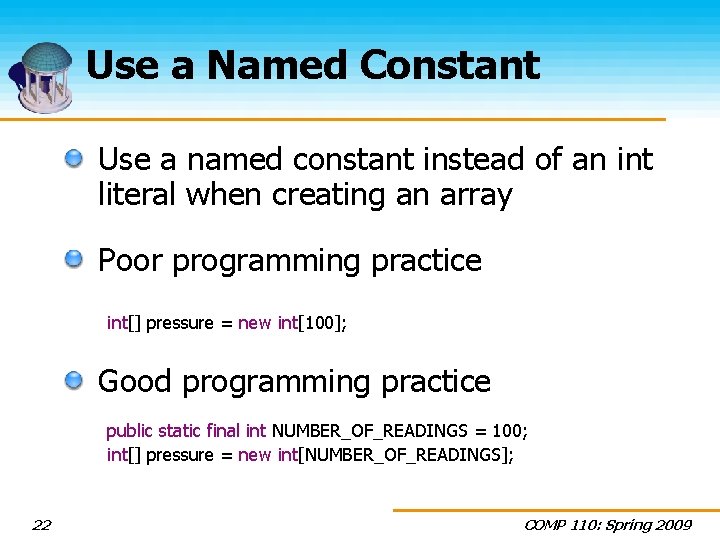
Use a Named Constant Use a named constant instead of an int literal when creating an array Poor programming practice int[] pressure = new int[100]; Good programming practice public static final int NUMBER_OF_READINGS = 100; int[] pressure = new int[NUMBER_OF_READINGS]; 22 COMP 110: Spring 2009
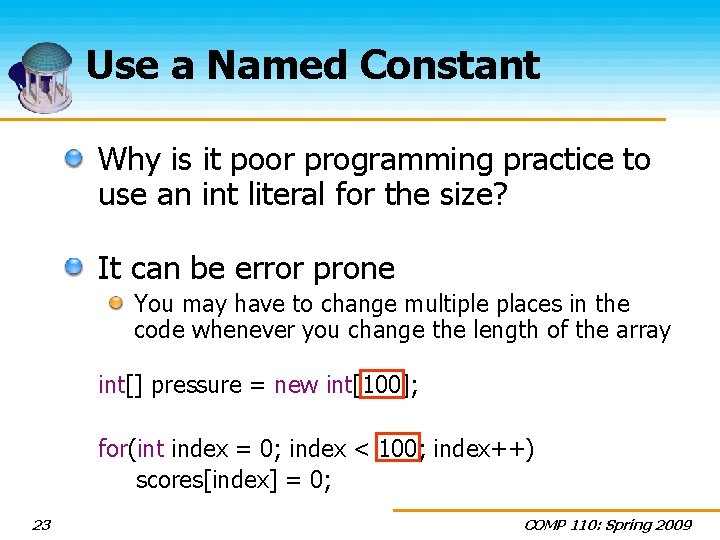
Use a Named Constant Why is it poor programming practice to use an int literal for the size? It can be error prone You may have to change multiple places in the code whenever you change the length of the array int[] pressure = new int[100]; for(int index = 0; index < 100; index++) scores[index] = 0; 23 COMP 110: Spring 2009
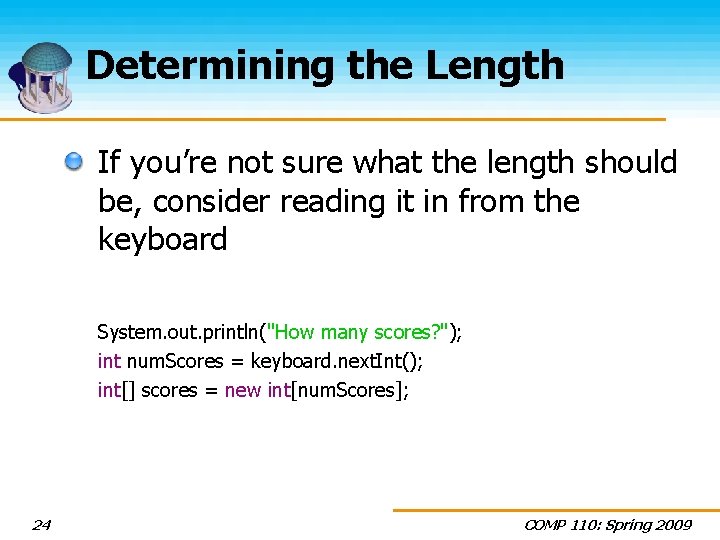
Determining the Length If you’re not sure what the length should be, consider reading it in from the keyboard System. out. println("How many scores? "); int num. Scores = keyboard. next. Int(); int[] scores = new int[num. Scores]; 24 COMP 110: Spring 2009
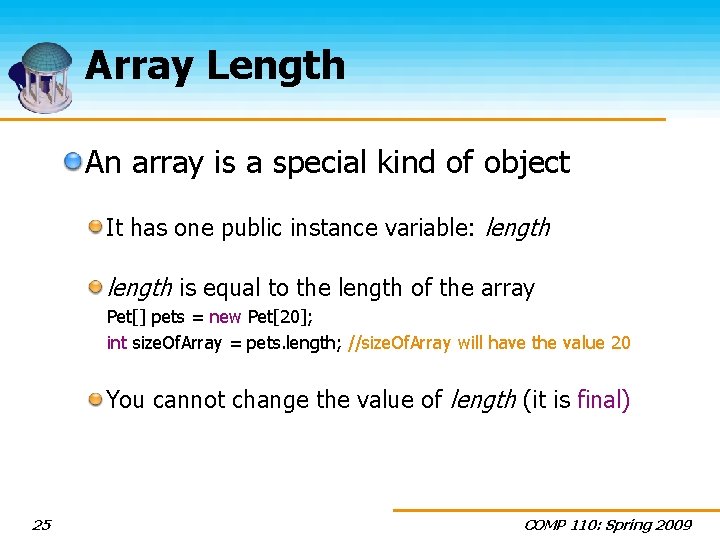
Array Length An array is a special kind of object It has one public instance variable: length is equal to the length of the array Pet[] pets = new Pet[20]; int size. Of. Array = pets. length; //size. Of. Array will have the value 20 You cannot change the value of length (it is final) 25 COMP 110: Spring 2009
![Our Previous Example System out printlnEnter 5 basketball scores int scores new Our Previous Example System. out. println("Enter 5 basketball scores: "); int[] scores = new](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-26.jpg)
Our Previous Example System. out. println("Enter 5 basketball scores: "); int[] scores = new int[5]; int score. Sum = 0; for(int i = 0; i < scores. length; i++) { scores[i] = keyboard. next. Int(); score. Sum += scores[i]; } Better than using the value 5 everywhere because it’s not obvious where it comes from and its value may change! double average = (double) score. Sum / scores. length; System. out. println("Average score: " + average); for(int i = 0; i < scores. length; i++) { if(scores[i] > average) System. out. println(scores[i] + ": above average"); else if(scores[i] < average) System. out. println(scores[i] + ": below average"); else System. out. println(scores[i] + ": equal to the average"); } 26 COMP 110: Spring 2009
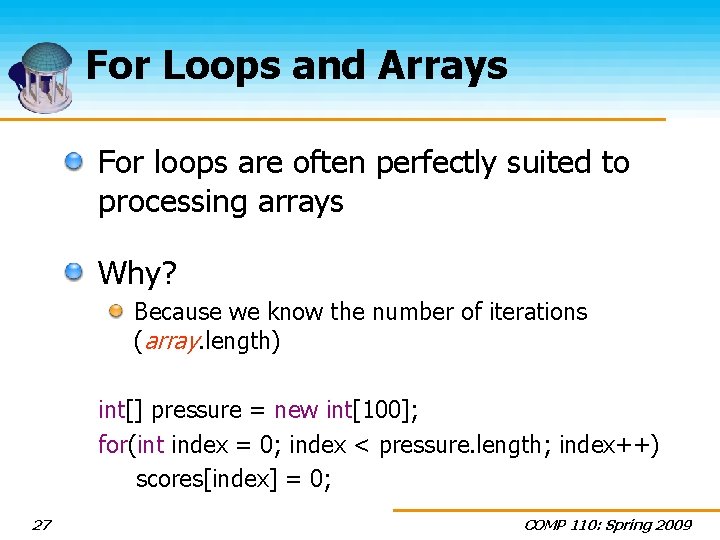
For Loops and Arrays For loops are often perfectly suited to processing arrays Why? Because we know the number of iterations (array. length) int[] pressure = new int[100]; for(int index = 0; index < pressure. length; index++) scores[index] = 0; 27 COMP 110: Spring 2009
![Be Careful with Indices MUST be in bounds double entries new double5 entries5 Be Careful with Indices MUST be in bounds double[] entries = new double[5]; entries[5]](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-28.jpg)
Be Careful with Indices MUST be in bounds double[] entries = new double[5]; entries[5] = 3. 7; //RUN-TIME ERROR! Index out of bounds Your code WILL compile with an out-ofbounds index But it will result in a run-time error (crash) 28 COMP 110: Spring 2009
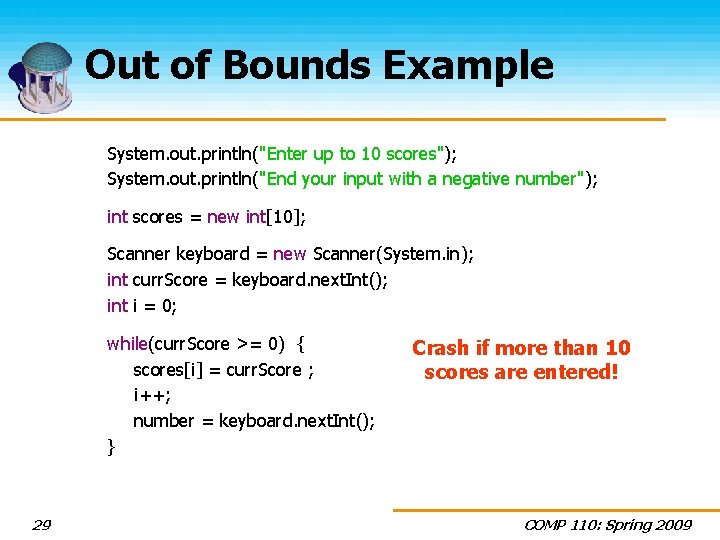
Out of Bounds Example System. out. println("Enter up to 10 scores"); System. out. println("End your input with a negative number"); int scores = new int[10]; Scanner keyboard = new Scanner(System. in); int curr. Score = keyboard. next. Int(); int i = 0; while(curr. Score >= 0) { scores[i] = curr. Score ; i++; number = keyboard. next. Int(); } 29 Crash if more than 10 scores are entered! COMP 110: Spring 2009
![Initializing Arrays You can initialize arrays when you declare them int scores 68 Initializing Arrays You can initialize arrays when you declare them int[] scores = {68,](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-30.jpg)
Initializing Arrays You can initialize arrays when you declare them int[] scores = {68, 97, 102}; Equivalent to int[] scores = new int[3]; scores[0] = 68; scores[1] = 97; scores[2] = 102; 30 COMP 110: Spring 2009
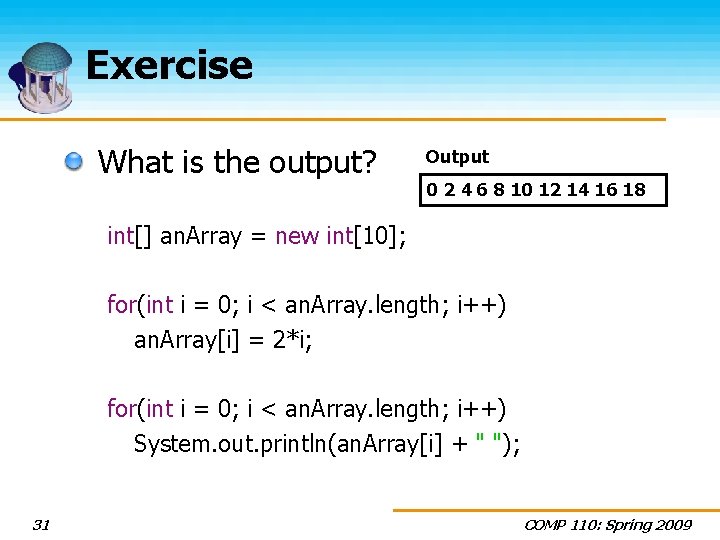
Exercise What is the output? Output 0 2 4 6 8 10 12 14 16 18 int[] an. Array = new int[10]; for(int i = 0; i < an. Array. length; i++) an. Array[i] = 2*i; for(int i = 0; i < an. Array. length; i++) System. out. println(an. Array[i] + " "); 31 COMP 110: Spring 2009
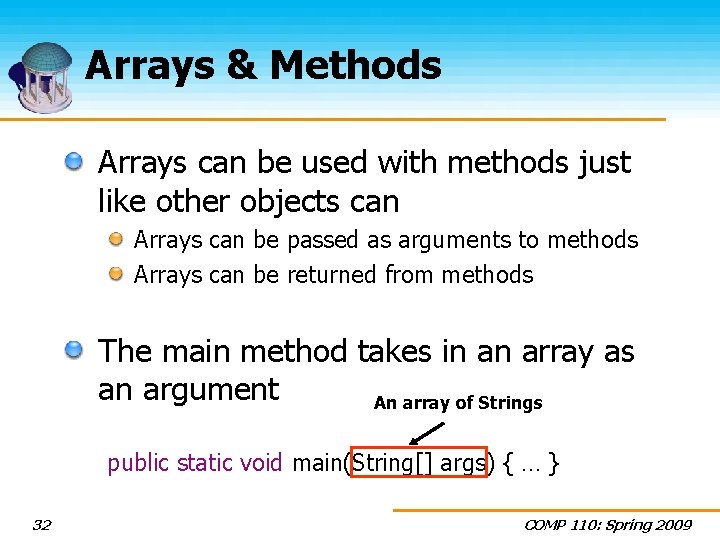
Arrays & Methods Arrays can be used with methods just like other objects can Arrays can be passed as arguments to methods Arrays can be returned from methods The main method takes in an array as an argument An array of Strings public static void main(String[] args) { … } 32 COMP 110: Spring 2009
![Arrays as Arguments Example init all array elements to 0 public void init Arrayint Arrays as Arguments Example //init all array elements to 0 public void init. Array(int[]](https://slidetodoc.com/presentation_image_h2/73675463f8f3955ee8cf9c735130b136/image-33.jpg)
Arrays as Arguments Example //init all array elements to 0 public void init. Array(int[] array) { for(int i = 0; i < array. length; i++) array[i] = 0; } 33 COMP 110: Spring 2009
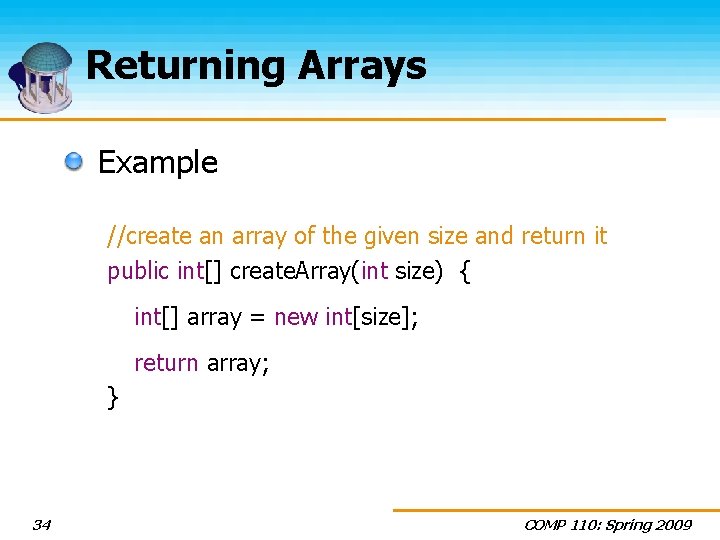
Returning Arrays Example //create an array of the given size and return it public int[] create. Array(int size) { int[] array = new int[size]; return array; } 34 COMP 110: Spring 2009
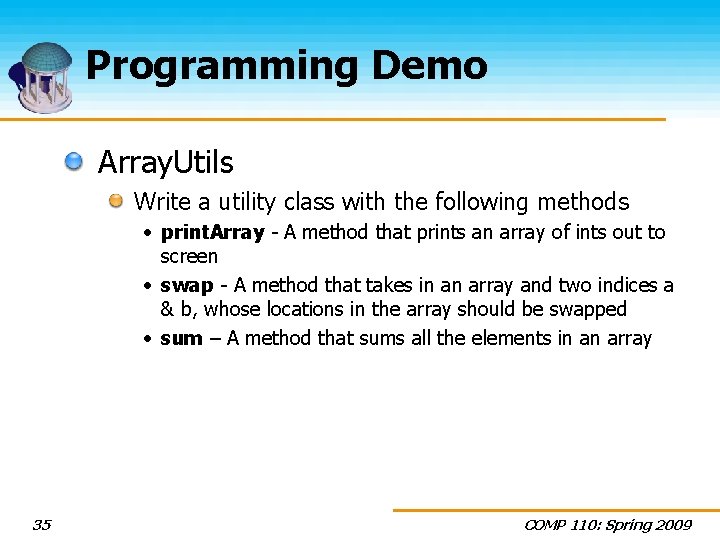
Programming Demo Array. Utils Write a utility class with the following methods • print. Array - A method that prints an array of ints out to screen • swap - A method that takes in an array and two indices a & b, whose locations in the array should be swapped • sum – A method that sums all the elements in an array 35 COMP 110: Spring 2009
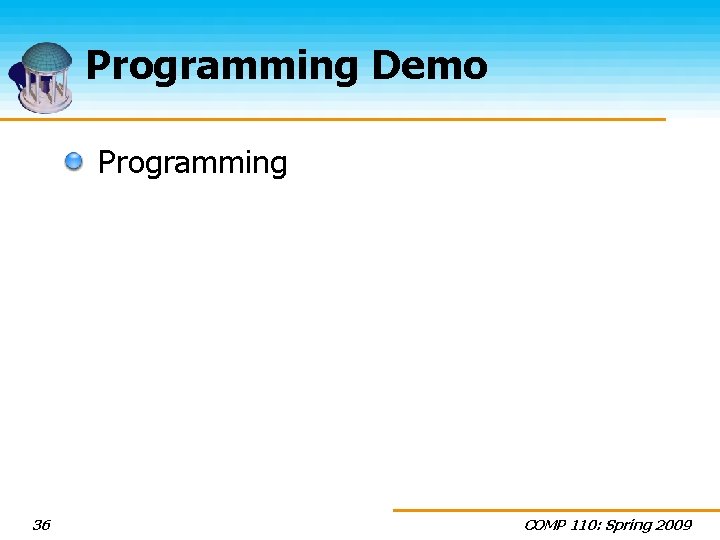
Programming Demo Programming 36 COMP 110: Spring 2009
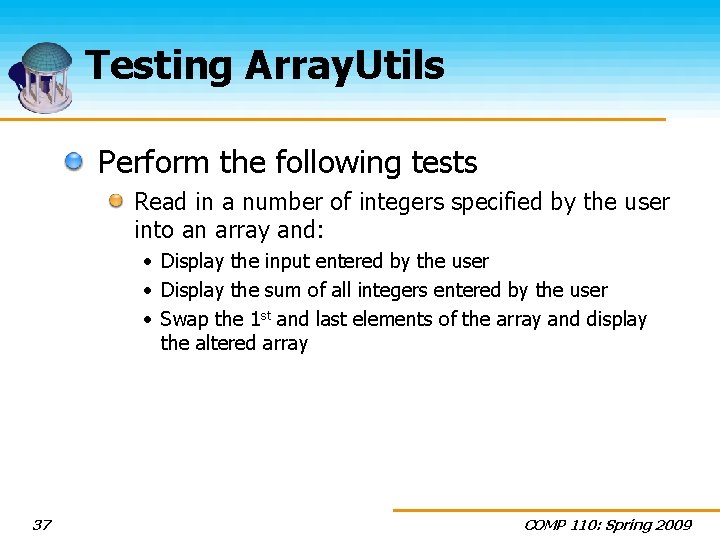
Testing Array. Utils Perform the following tests Read in a number of integers specified by the user into an array and: • Display the input entered by the user • Display the sum of all integers entered by the user • Swap the 1 st and last elements of the array and display the altered array 37 COMP 110: Spring 2009
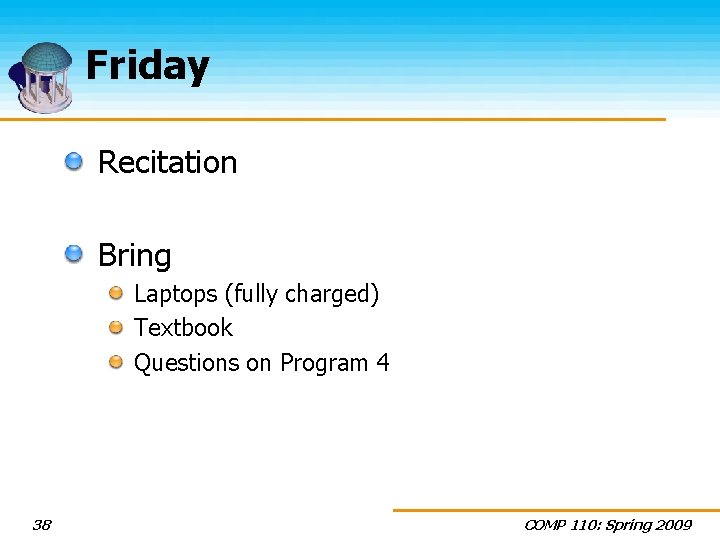
Friday Recitation Bring Laptops (fully charged) Textbook Questions on Program 4 38 COMP 110: Spring 2009
Où se trouve le numéro d'affiliation mutuelle vignette ?
110-000-110 & 111-000-111
Comp sci 110 northwestern
Swot johnson and johnson
Johnson and johnson bcg matrix
Brad and laurie johnson
Our credo johnson & johnson
Johnson and johnson organizational structure
Understanding the mirai botnet
Johnson and johnson swot
Jjeds johnson and johnson
Dreikurs classroom management theory
Johnson and johnson md&d
Introduction to physics 110
Perbedaan linear programming dan integer programming
Greedy vs dynamic programming
Windows 10 system programming, part 1
Integer programming vs linear programming
Definisi linear
Introduction to server side programming
Problem solving
Introduction to programming languages
Daniel liang introduction to java programming
An introduction to parallel programming peter pacheco
Visual basic overview
Introduction to programming
Programming and problem solving with java
Console programming
Introduction to programming
Csc102
A web based introduction to programming
Sic programming examples
Chapter 1 introduction to computers and programming
C programming and numerical analysis an introduction
Introduction to visual basic
Scratch programming concepts
Python programming an introduction to computer science
Java introduction to problem solving and programming
Chapter 1 introduction to computers and programming