Chapter 12 Data Structures Outline 12 1 12
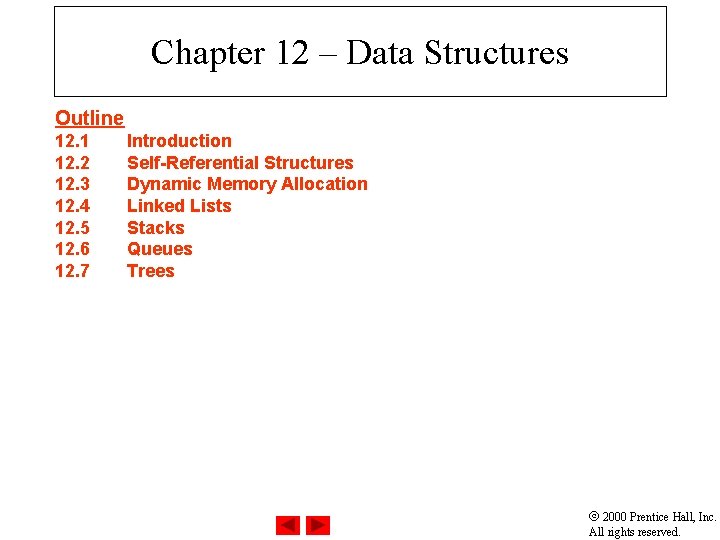
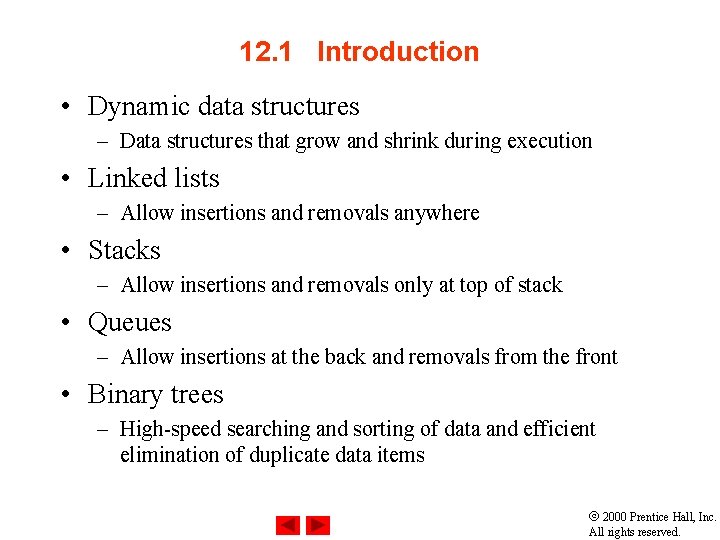
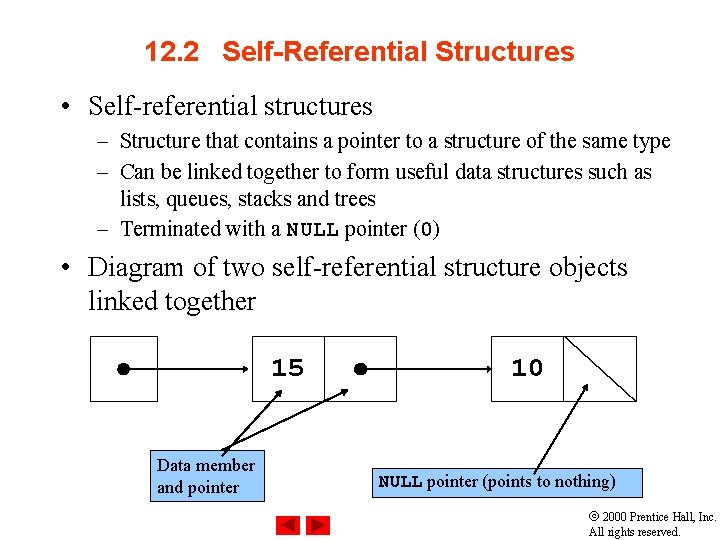
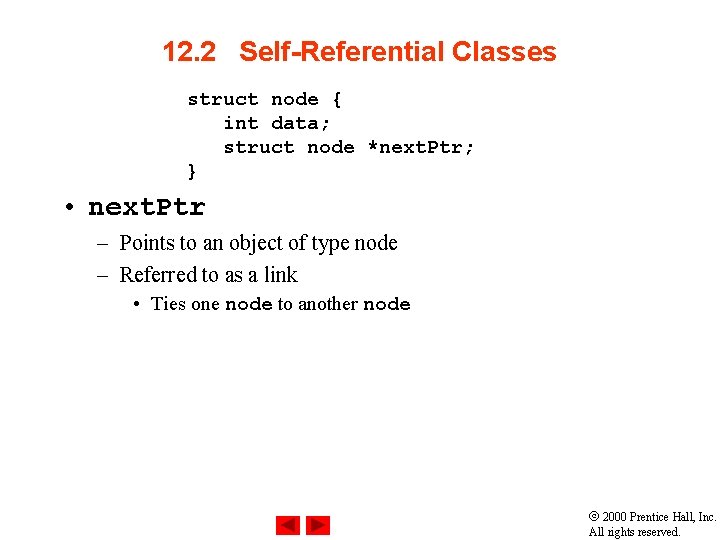
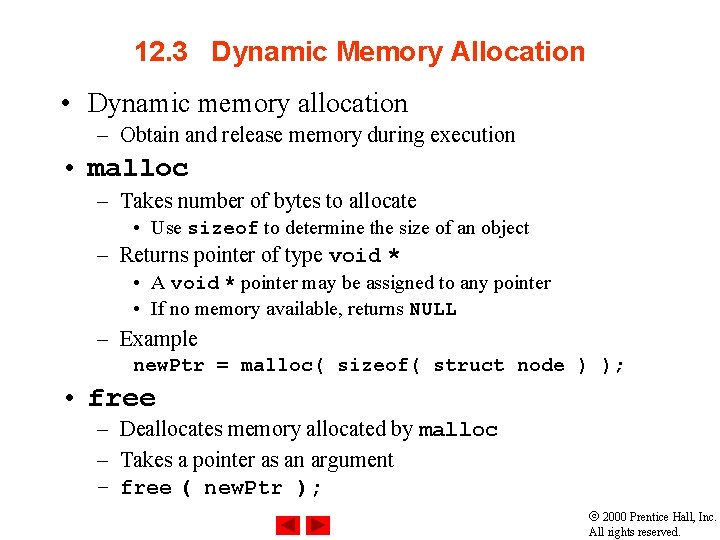
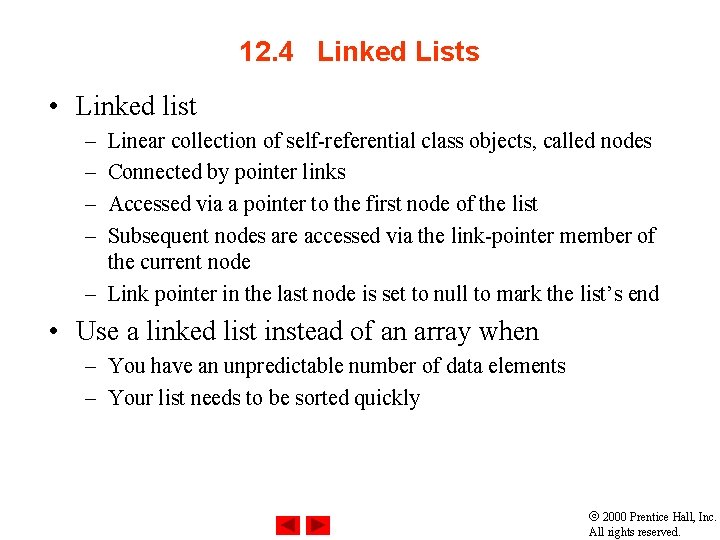
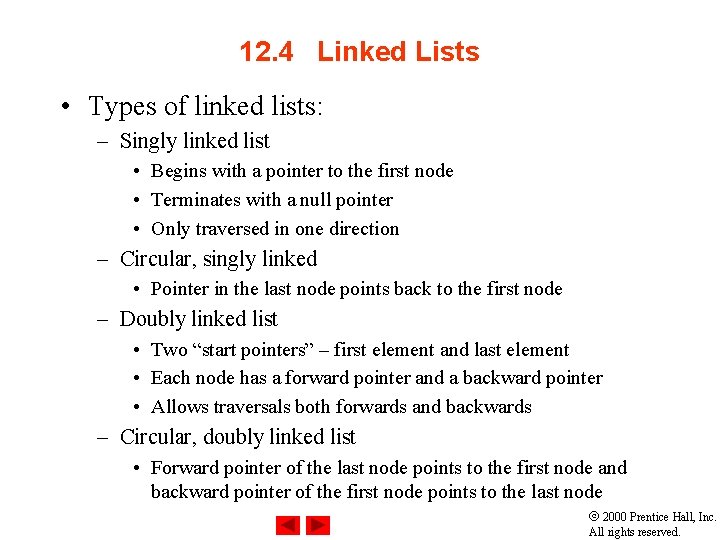
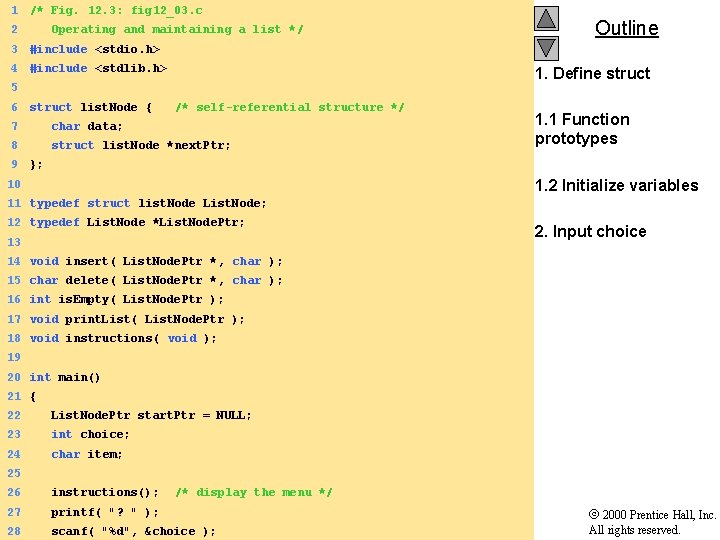
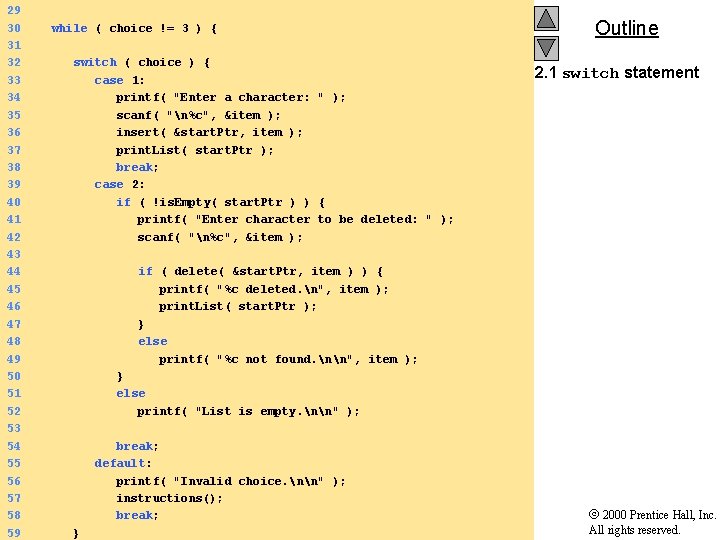
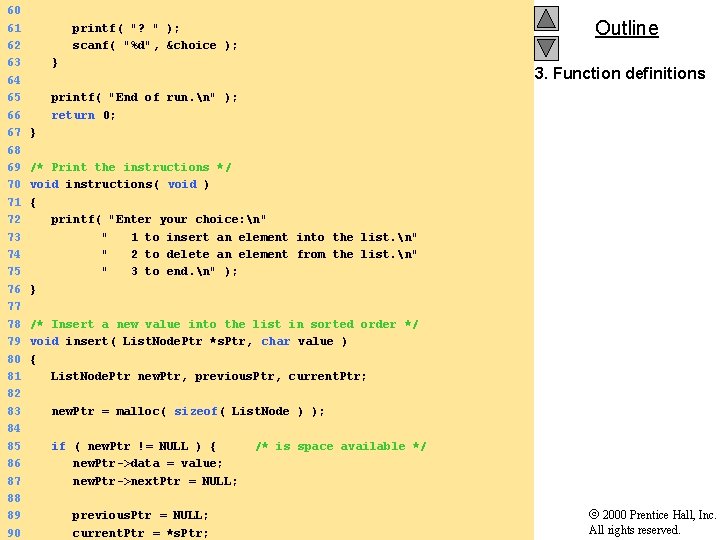
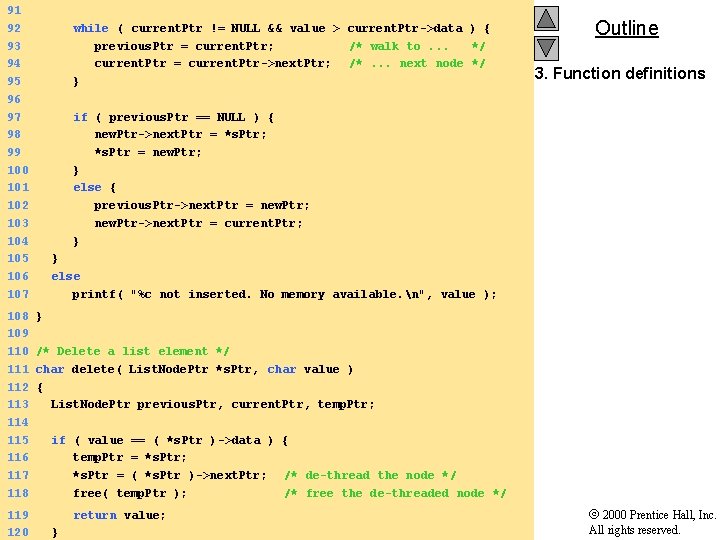
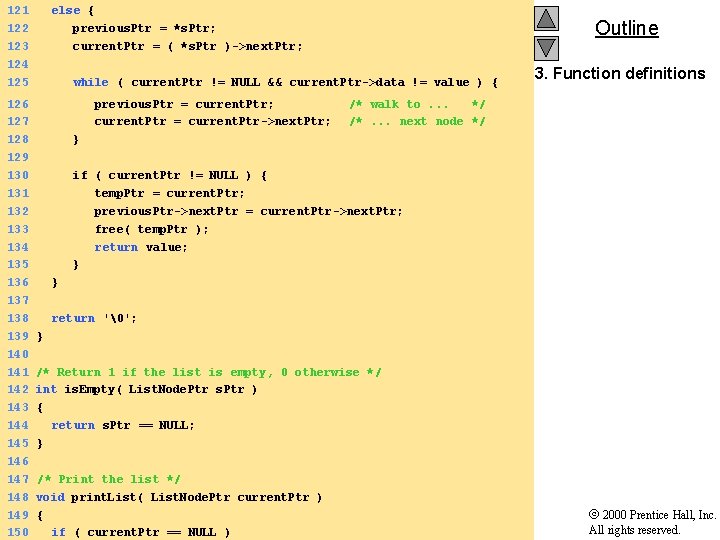
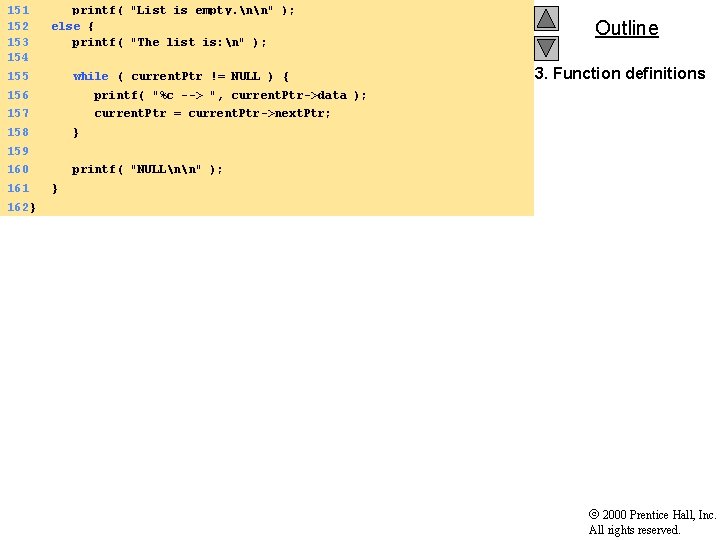
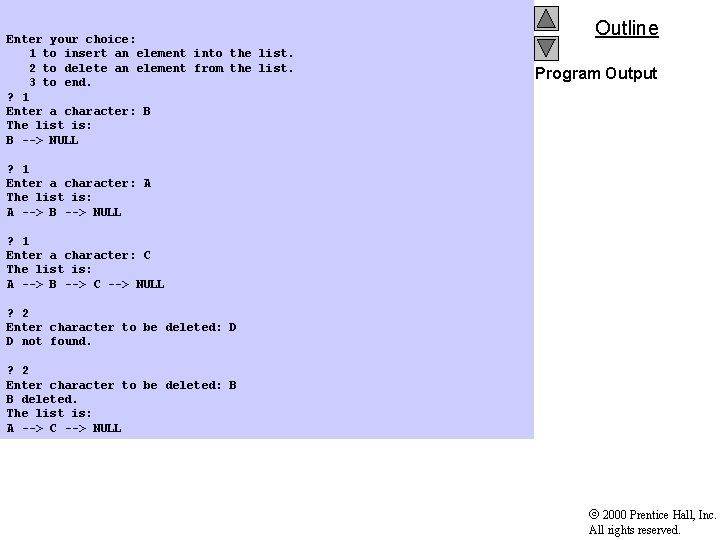
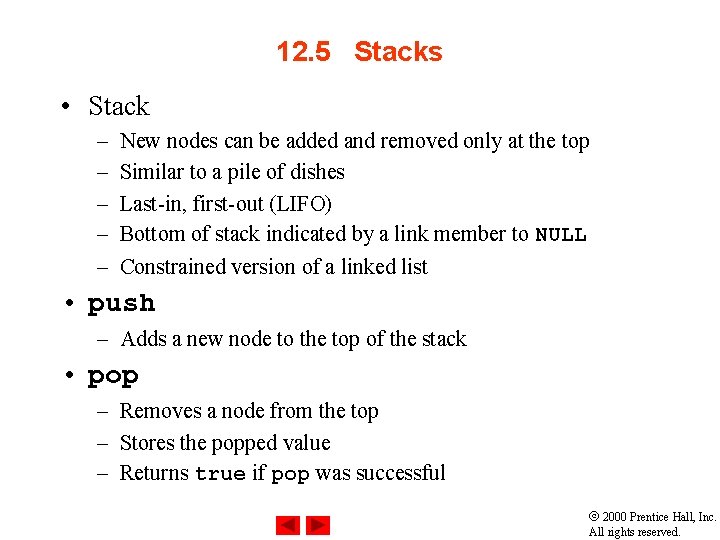
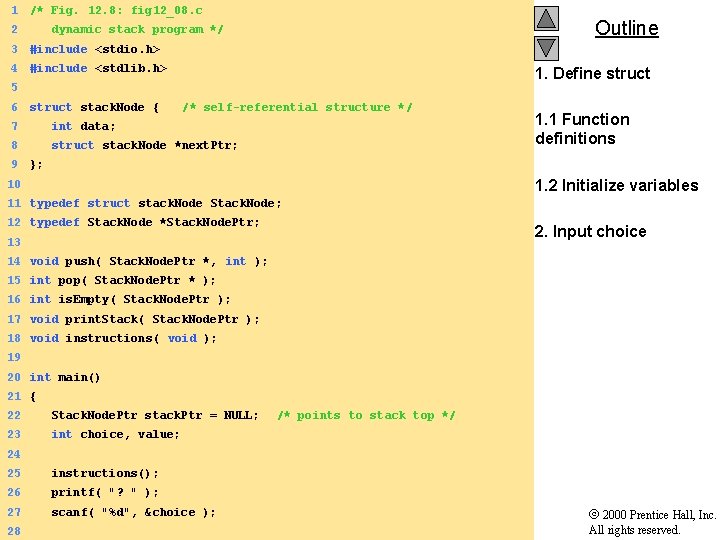
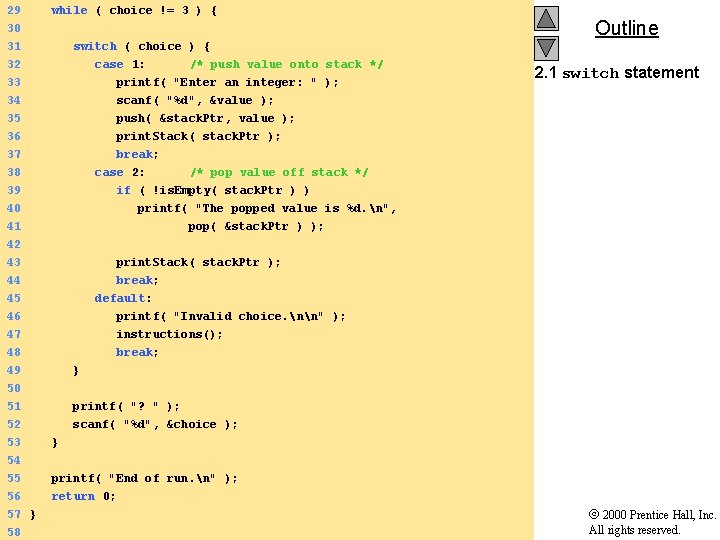
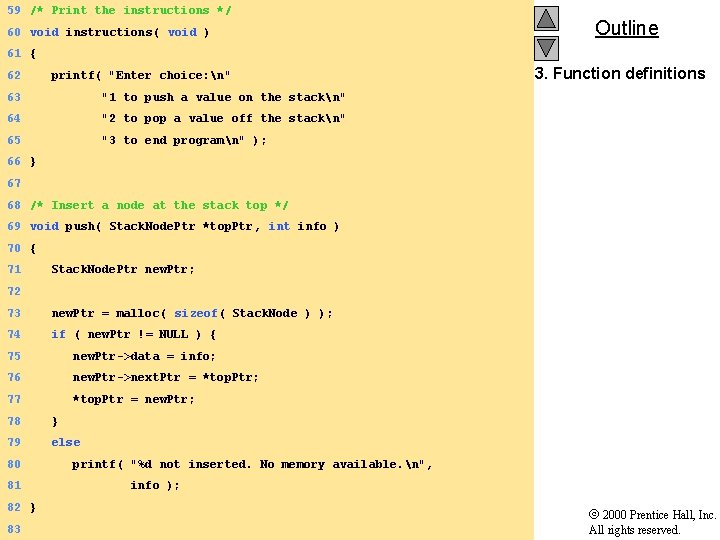
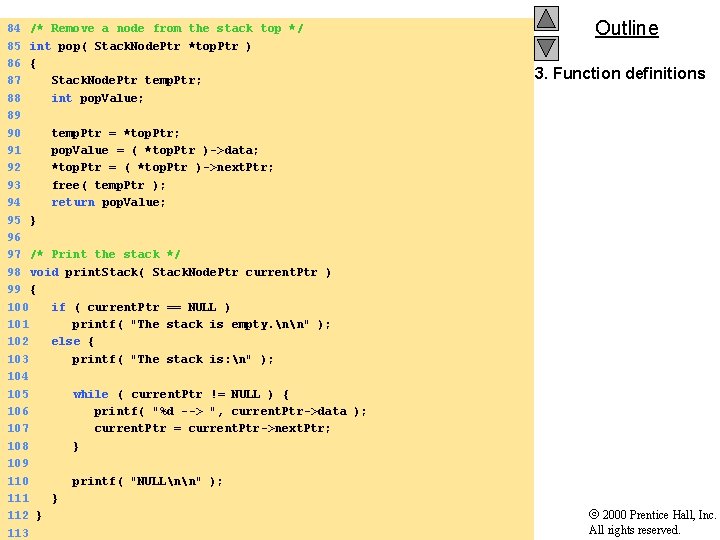
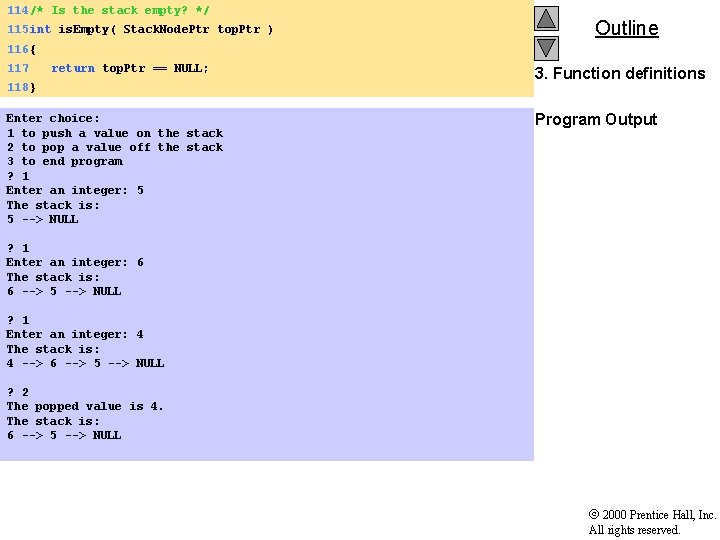
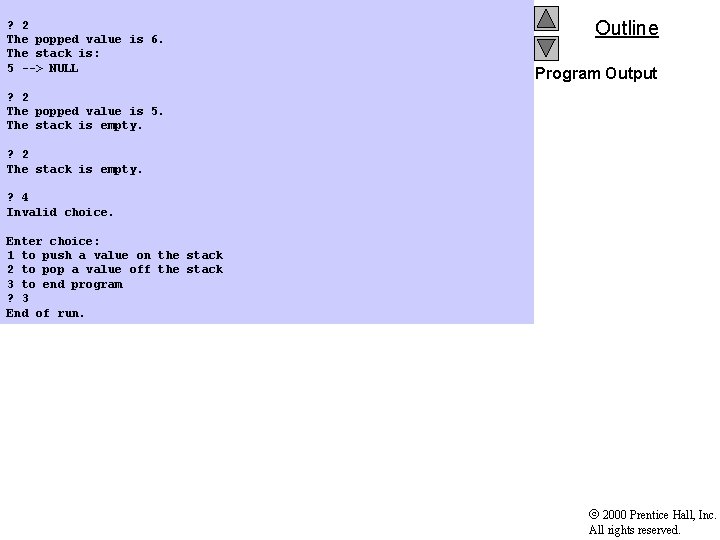
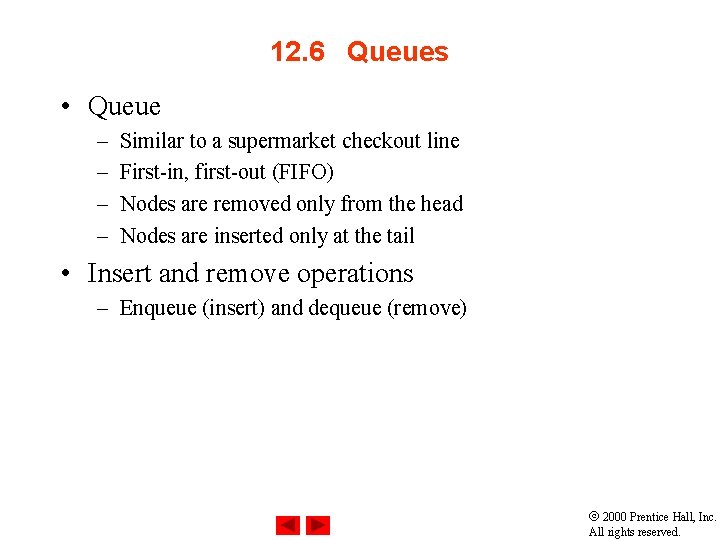
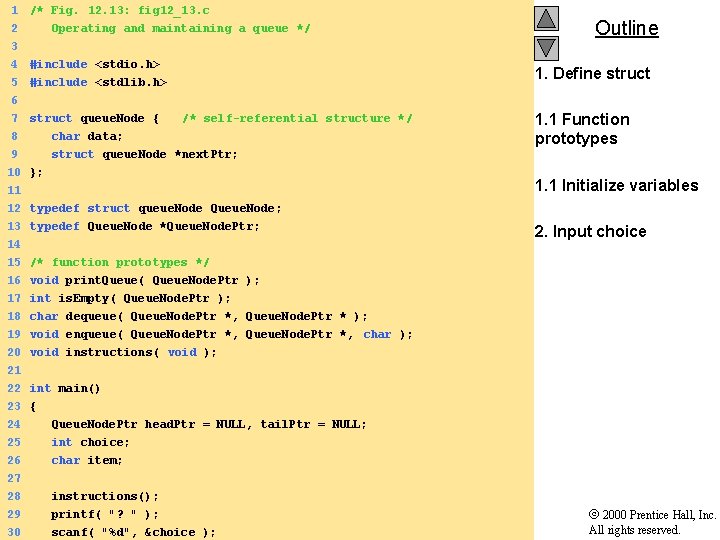
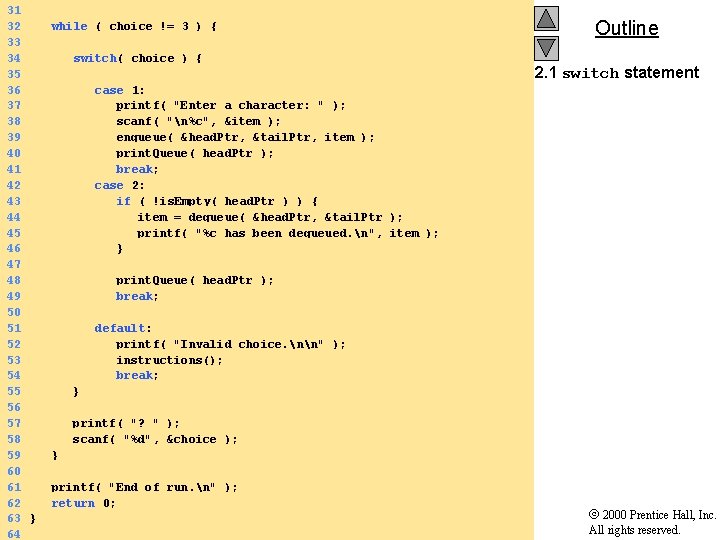
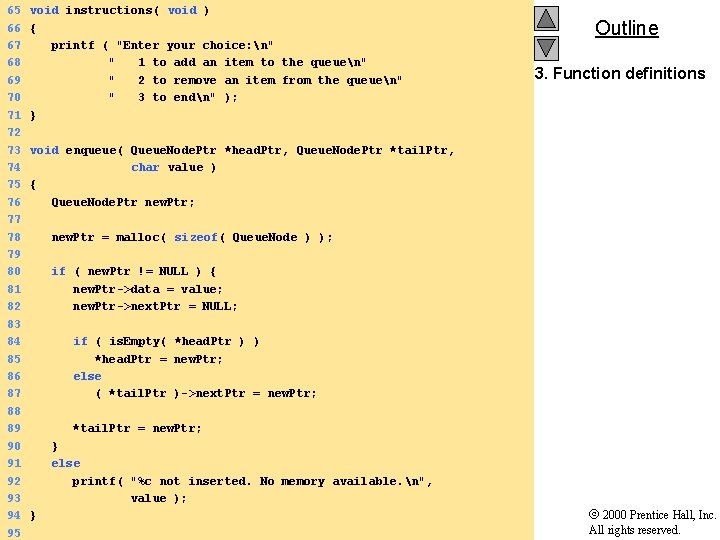
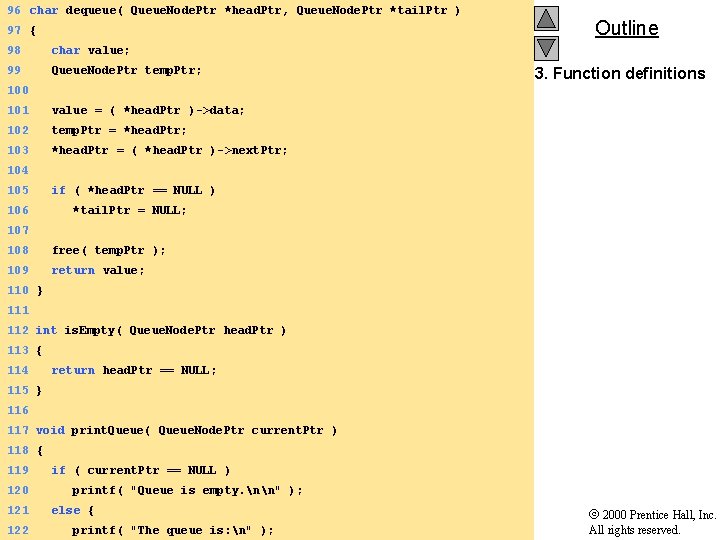
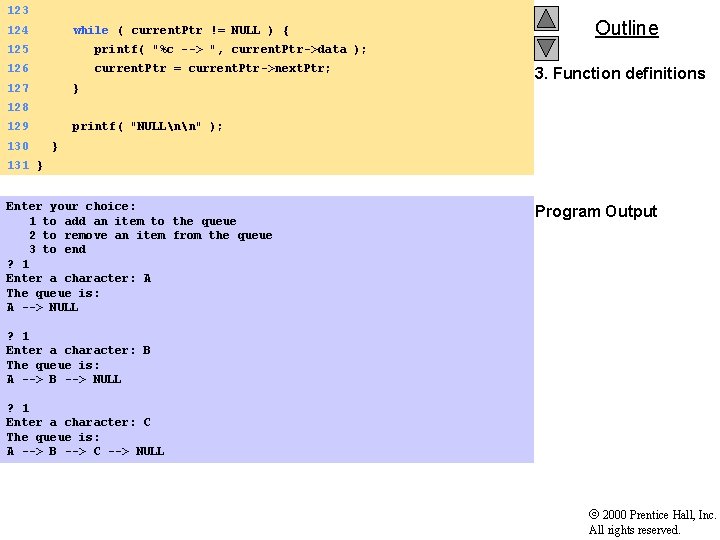
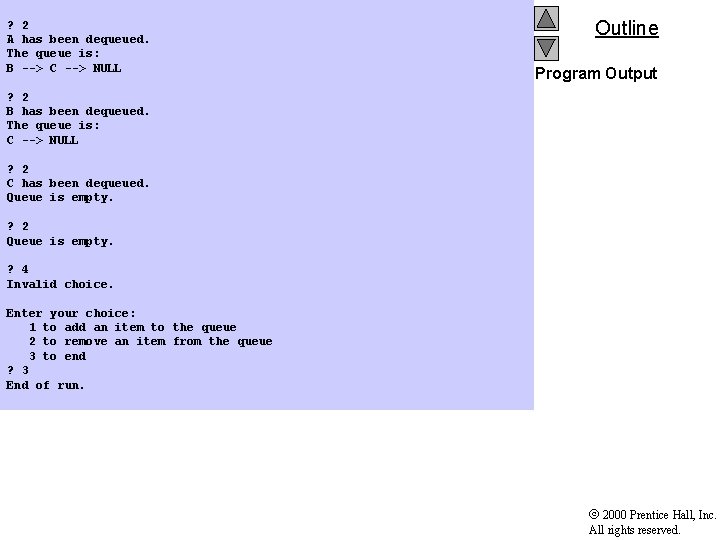
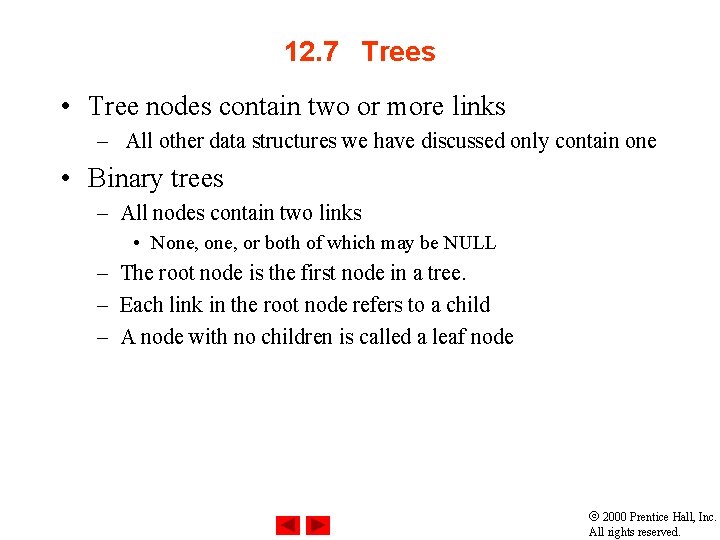
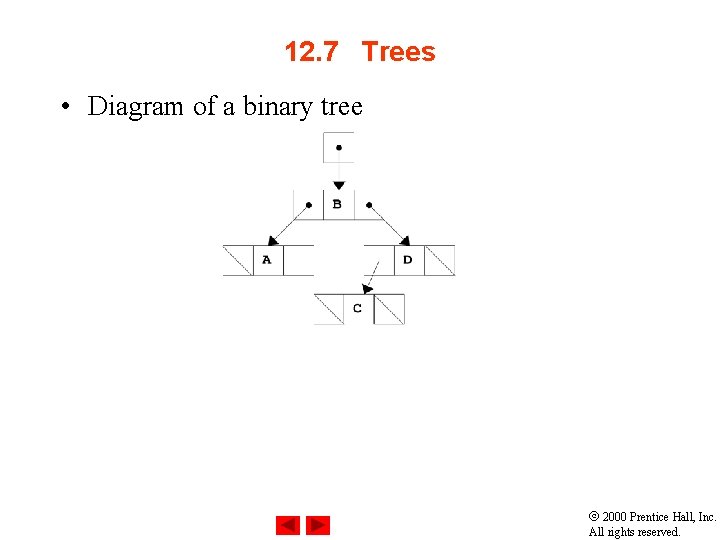
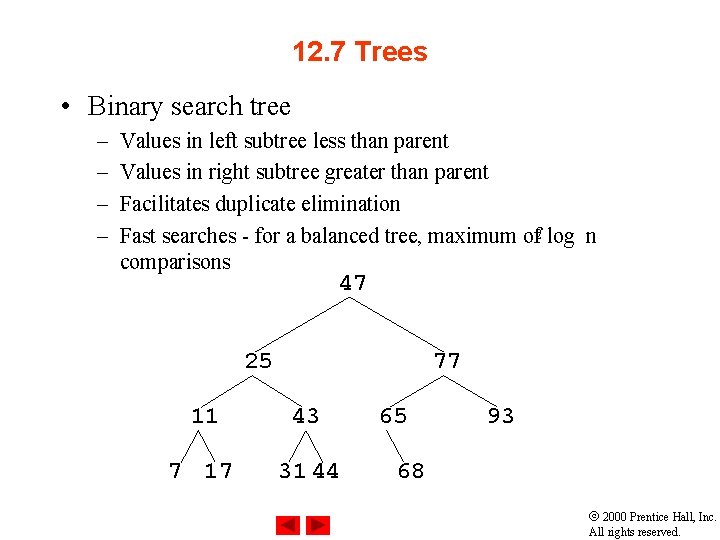
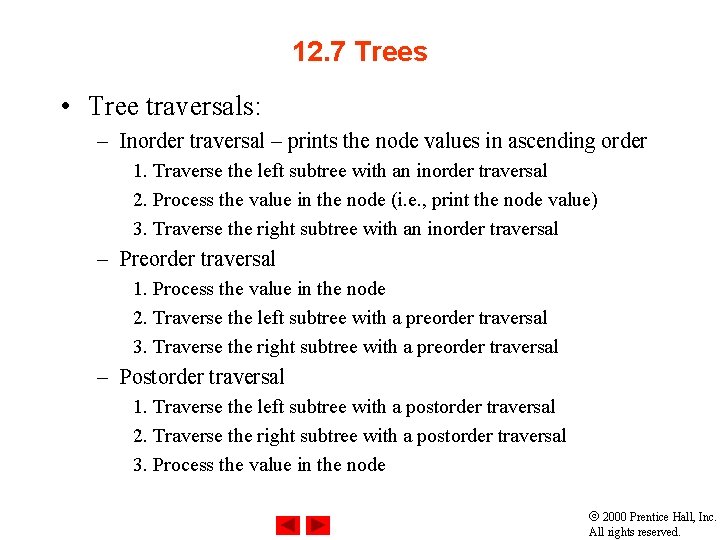
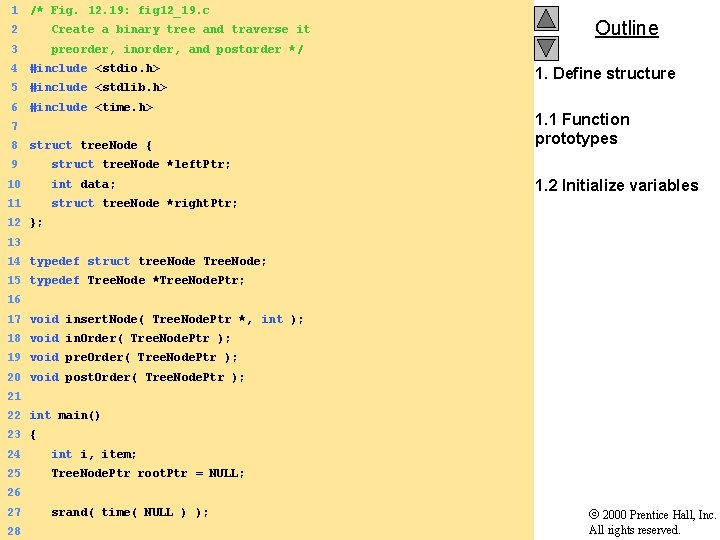
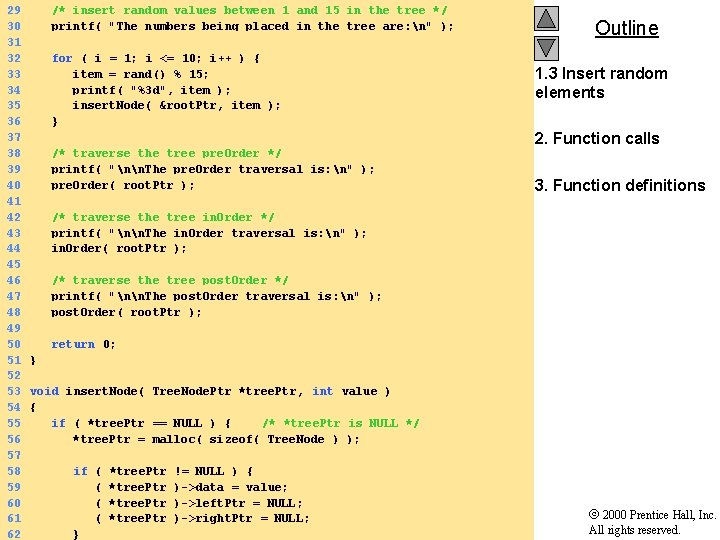
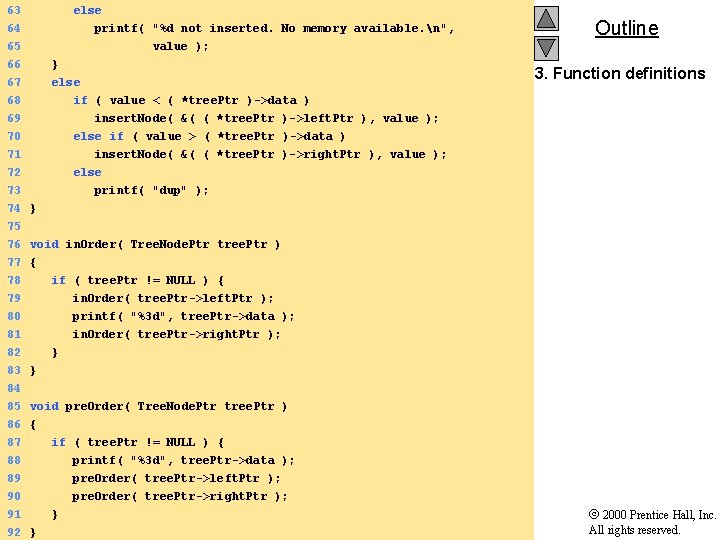
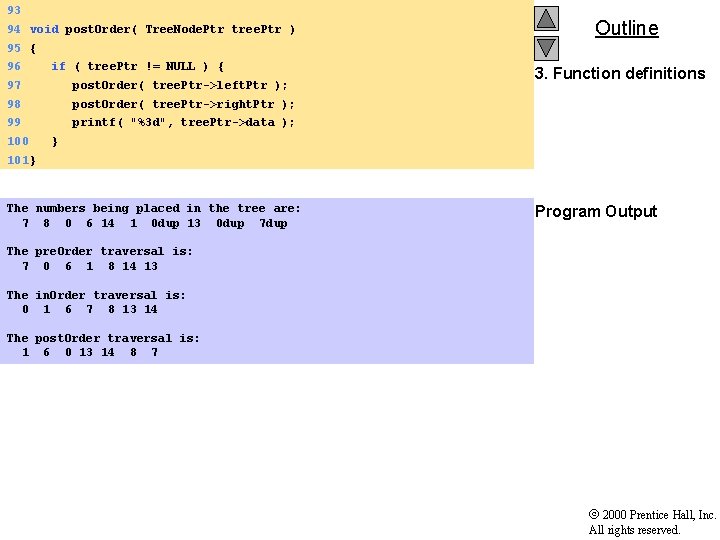
- Slides: 36
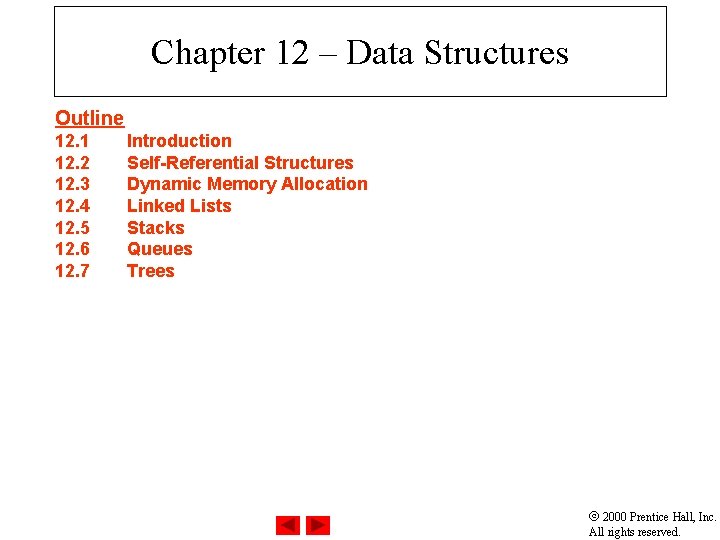
Chapter 12 – Data Structures Outline 12. 1 12. 2 12. 3 12. 4 12. 5 12. 6 12. 7 Introduction Self-Referential Structures Dynamic Memory Allocation Linked Lists Stacks Queues Trees 2000 Prentice Hall, Inc. All rights reserved.
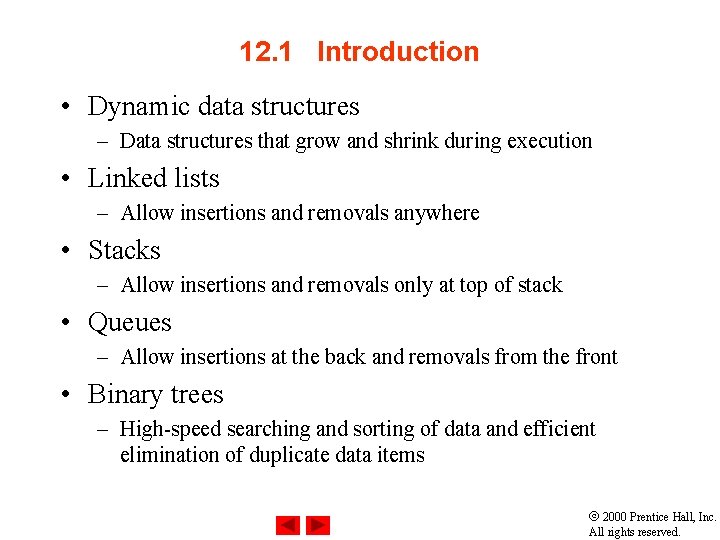
12. 1 Introduction • Dynamic data structures – Data structures that grow and shrink during execution • Linked lists – Allow insertions and removals anywhere • Stacks – Allow insertions and removals only at top of stack • Queues – Allow insertions at the back and removals from the front • Binary trees – High-speed searching and sorting of data and efficient elimination of duplicate data items 2000 Prentice Hall, Inc. All rights reserved.
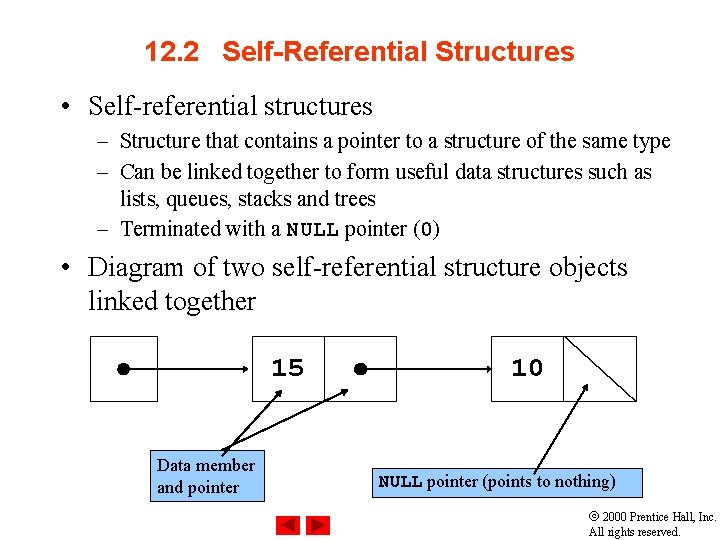
12. 2 Self-Referential Structures • Self-referential structures – Structure that contains a pointer to a structure of the same type – Can be linked together to form useful data structures such as lists, queues, stacks and trees – Terminated with a NULL pointer (0) • Diagram of two self-referential structure objects linked together 15 Data member and pointer 10 NULL pointer (points to nothing) 2000 Prentice Hall, Inc. All rights reserved.
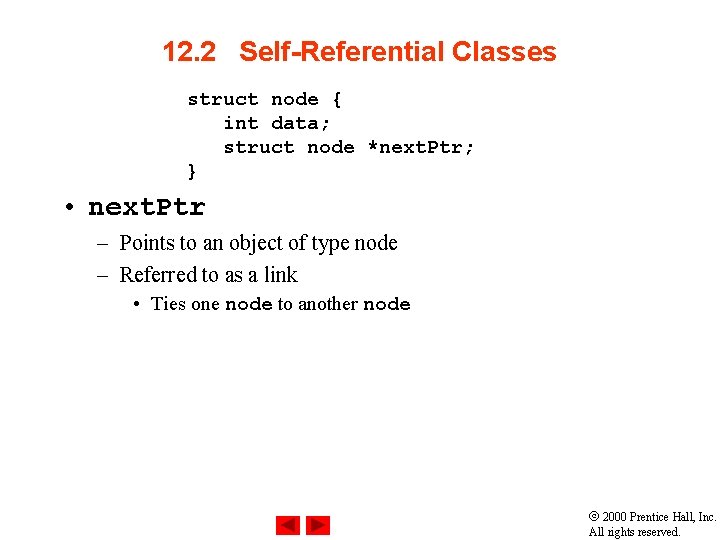
12. 2 Self-Referential Classes struct node { int data; struct node *next. Ptr; } • next. Ptr – Points to an object of type node – Referred to as a link • Ties one node to another node 2000 Prentice Hall, Inc. All rights reserved.
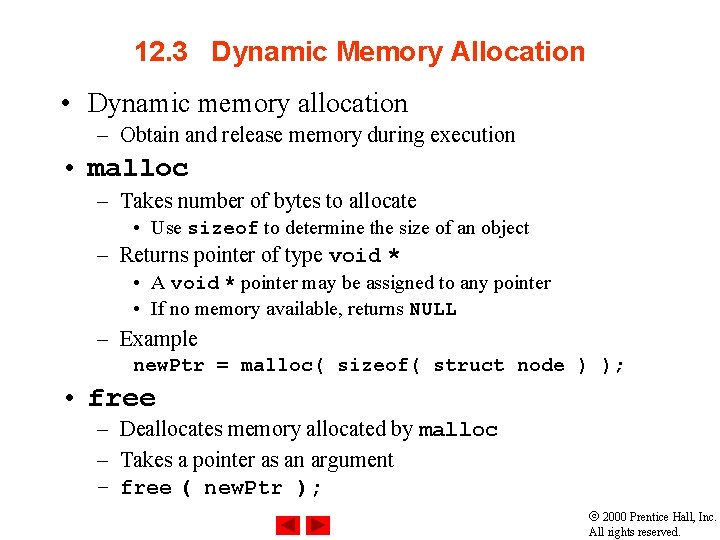
12. 3 Dynamic Memory Allocation • Dynamic memory allocation – Obtain and release memory during execution • malloc – Takes number of bytes to allocate • Use sizeof to determine the size of an object – Returns pointer of type void * • A void * pointer may be assigned to any pointer • If no memory available, returns NULL – Example new. Ptr = malloc( sizeof( struct node ) ); • free – Deallocates memory allocated by malloc – Takes a pointer as an argument – free ( new. Ptr ); 2000 Prentice Hall, Inc. All rights reserved.
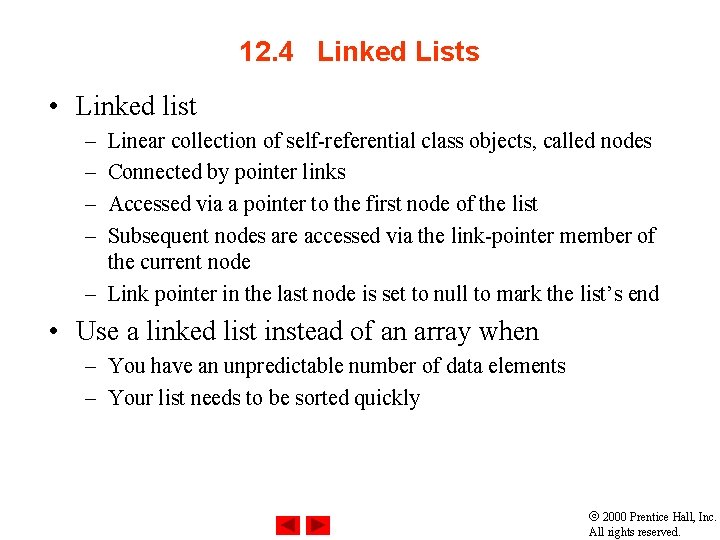
12. 4 Linked Lists • Linked list – – Linear collection of self-referential class objects, called nodes Connected by pointer links Accessed via a pointer to the first node of the list Subsequent nodes are accessed via the link-pointer member of the current node – Link pointer in the last node is set to null to mark the list’s end • Use a linked list instead of an array when – You have an unpredictable number of data elements – Your list needs to be sorted quickly 2000 Prentice Hall, Inc. All rights reserved.
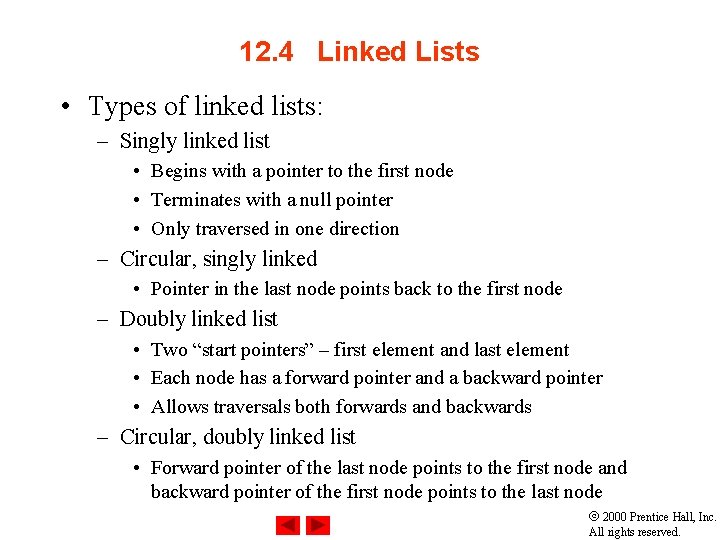
12. 4 Linked Lists • Types of linked lists: – Singly linked list • Begins with a pointer to the first node • Terminates with a null pointer • Only traversed in one direction – Circular, singly linked • Pointer in the last node points back to the first node – Doubly linked list • Two “start pointers” – first element and last element • Each node has a forward pointer and a backward pointer • Allows traversals both forwards and backwards – Circular, doubly linked list • Forward pointer of the last node points to the first node and backward pointer of the first node points to the last node 2000 Prentice Hall, Inc. All rights reserved.
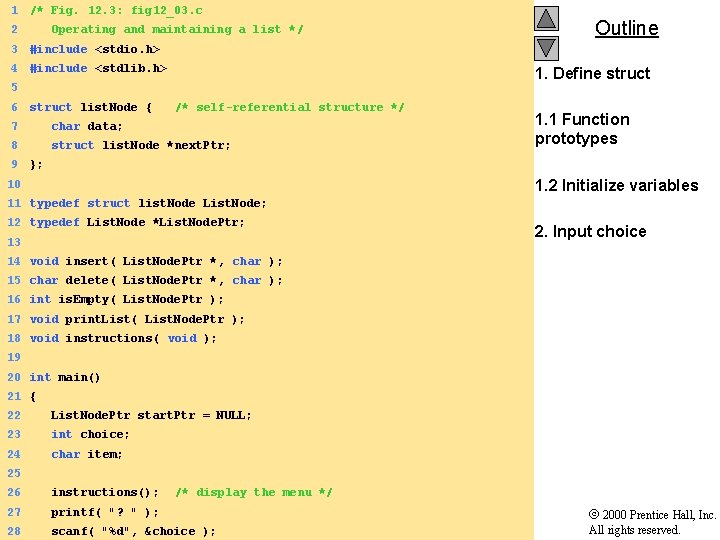
1 /* Fig. 12. 3: fig 12_03. c 2 Operating and maintaining a list */ 3 #include <stdio. h> 4 #include <stdlib. h> 5 6 struct list. Node { /* self-referential structure */ 7 char data; 8 struct list. Node *next. Ptr; 9 }; 10 Outline 1. Define struct 1. 1 Function prototypes 1. 2 Initialize variables 11 typedef struct list. Node List. Node; 12 typedef List. Node *List. Node. Ptr; 13 2. Input choice 14 void insert( List. Node. Ptr *, char ); 15 char delete( List. Node. Ptr *, char ); 16 int is. Empty( List. Node. Ptr ); 17 void print. List( List. Node. Ptr ); 18 void instructions( void ); 19 20 int main() 21 { 22 List. Node. Ptr start. Ptr = NULL; 23 int choice; 24 char item; 25 26 instructions(); /* display the menu */ 27 printf( "? " ); 2000 Prentice Hall, Inc. 28 scanf( "%d", &choice ); All rights reserved.
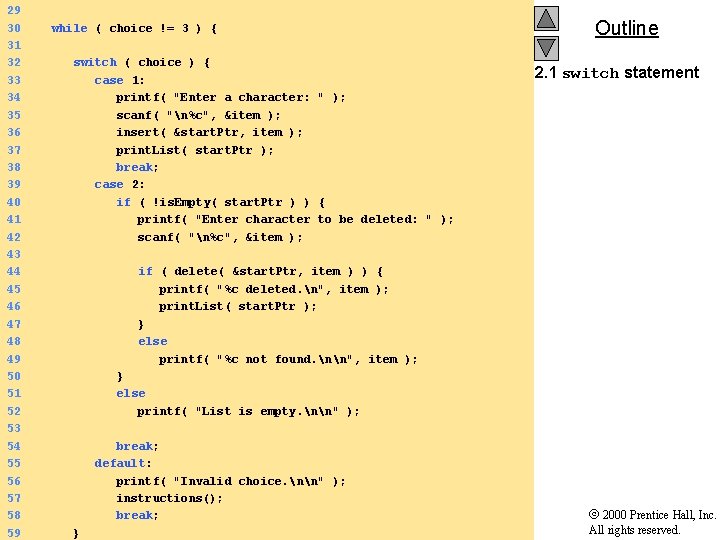
29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 while ( choice != 3 ) { switch ( choice ) { case 1: printf( "Enter a character: " ); scanf( "n%c", &item ); insert( &start. Ptr, item ); print. List( start. Ptr ); break; case 2: if ( !is. Empty( start. Ptr ) ) { printf( "Enter character to be deleted: " ); scanf( "n%c", &item ); Outline 2. 1 switch statement if ( delete( &start. Ptr, item ) ) { printf( "%c deleted. n", item ); print. List( start. Ptr ); } else printf( "%c not found. nn", item ); } else printf( "List is empty. nn" ); break; default: printf( "Invalid choice. nn" ); instructions(); break; } 2000 Prentice Hall, Inc. All rights reserved.
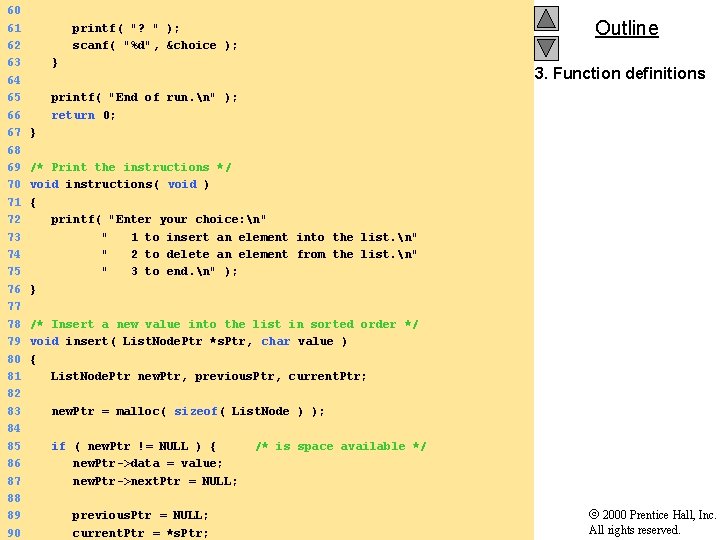
60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 printf( "? " ); scanf( "%d", &choice ); } Outline 3. Function definitions printf( "End of run. n" ); return 0; } /* Print the instructions */ void instructions( void ) { printf( "Enter your choice: n" " 1 to insert an element into the list. n" " 2 to delete an element from the list. n" " 3 to end. n" ); } /* Insert a new value into the list in sorted order */ void insert( List. Node. Ptr *s. Ptr, char value ) { List. Node. Ptr new. Ptr, previous. Ptr, current. Ptr; new. Ptr = malloc( sizeof( List. Node ) ); if ( new. Ptr != NULL ) { /* is space available */ new. Ptr->data = value; new. Ptr->next. Ptr = NULL; previous. Ptr = NULL; current. Ptr = *s. Ptr; 2000 Prentice Hall, Inc. All rights reserved.
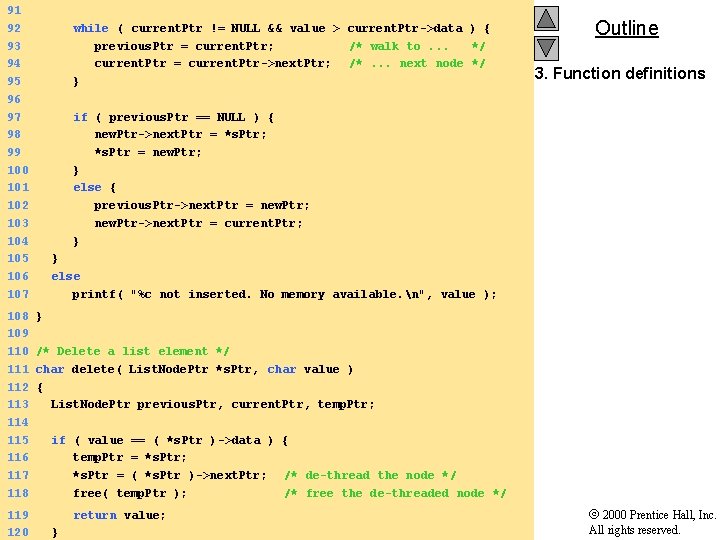
91 92 while ( current. Ptr != NULL && value > current. Ptr->data ) { 93 previous. Ptr = current. Ptr; /* walk to. . . */ 94 current. Ptr = current. Ptr->next. Ptr; /*. . . next node */ 95 } 96 97 if ( previous. Ptr == NULL ) { 98 new. Ptr->next. Ptr = *s. Ptr; 99 *s. Ptr = new. Ptr; 100 } 101 else { 102 previous. Ptr->next. Ptr = new. Ptr; 103 new. Ptr->next. Ptr = current. Ptr; 104 } 105 } 106 else 107 printf( "%c not inserted. No memory available. n", value ); Outline 3. Function definitions 108 } 109 110 /* Delete a list element */ 111 char delete( List. Node. Ptr *s. Ptr, char value ) 112 { 113 List. Node. Ptr previous. Ptr, current. Ptr, temp. Ptr; 114 115 if ( value == ( *s. Ptr )->data ) { 116 temp. Ptr = *s. Ptr; 117 *s. Ptr = ( *s. Ptr )->next. Ptr; /* de-thread the node */ 118 free( temp. Ptr ); /* free the de-threaded node */ 119 return value; 120 } 2000 Prentice Hall, Inc. All rights reserved.
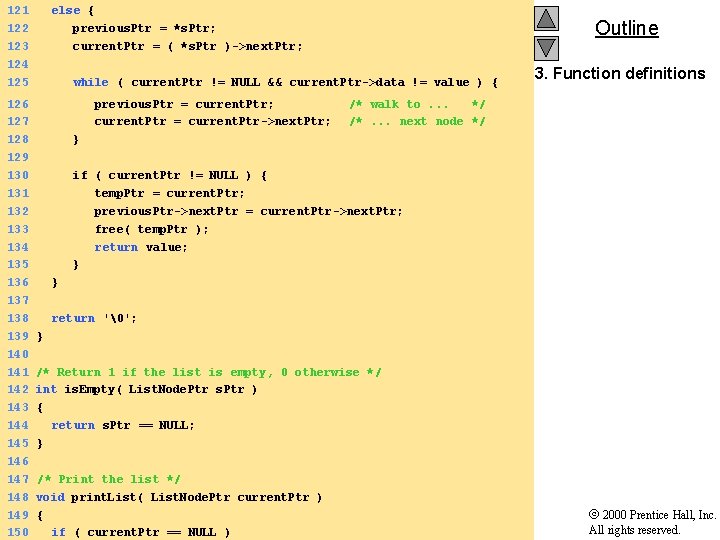
121 else { 122 previous. Ptr = *s. Ptr; 123 current. Ptr = ( *s. Ptr )->next. Ptr; 124 125 while ( current. Ptr != NULL && current. Ptr->data != value ) { 126 previous. Ptr = current. Ptr; /* walk to. . . */ 127 current. Ptr = current. Ptr->next. Ptr; /*. . . next node */ 128 } 129 130 if ( current. Ptr != NULL ) { 131 temp. Ptr = current. Ptr; 132 previous. Ptr->next. Ptr = current. Ptr->next. Ptr; 133 free( temp. Ptr ); 134 return value; 135 } 136 } 137 138 return '