Best case Worst case Average case Spacetime 5
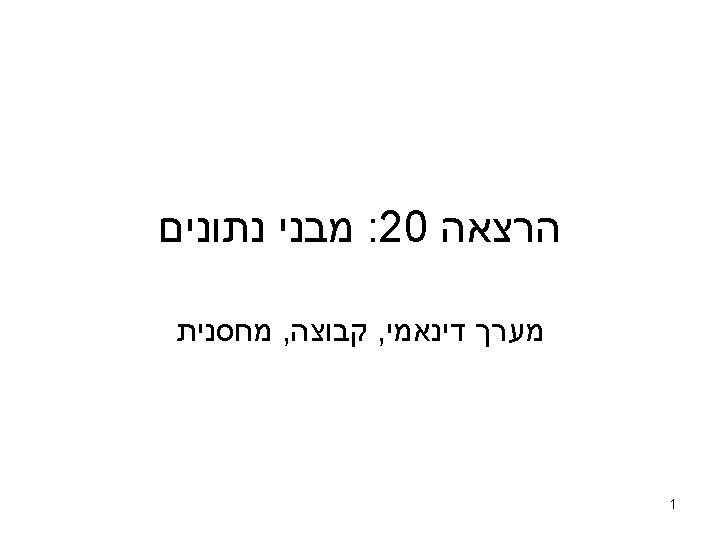
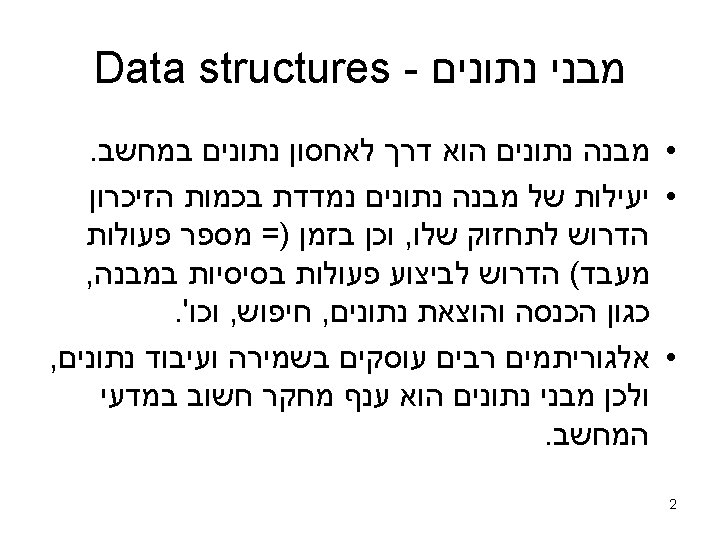
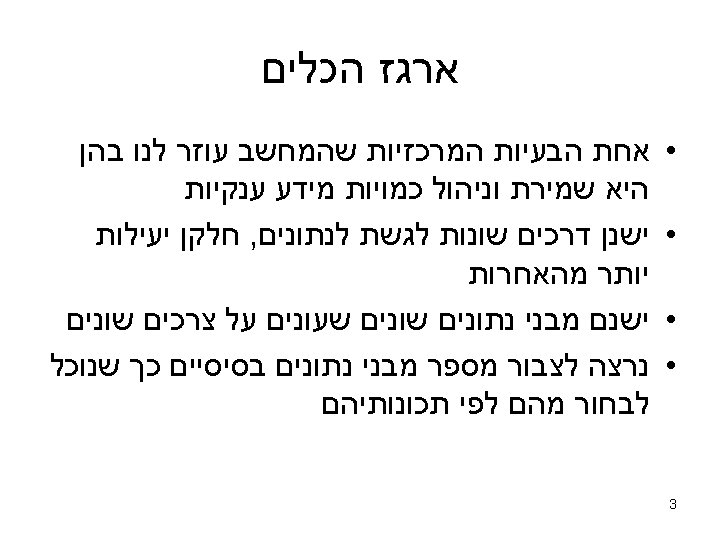
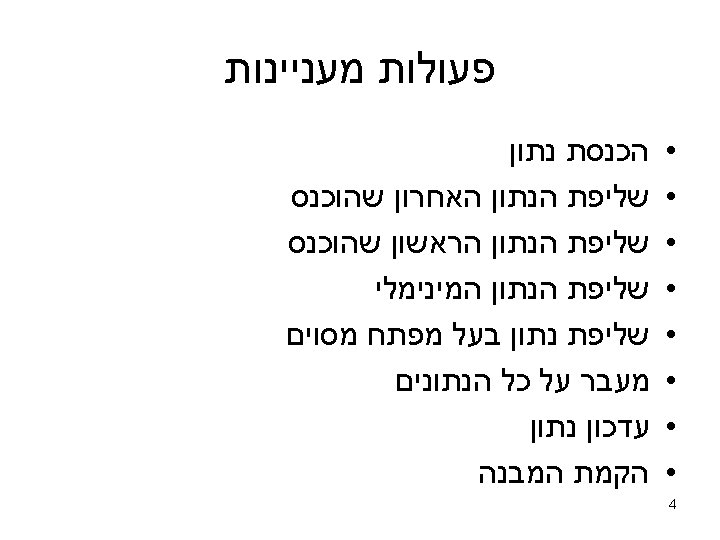
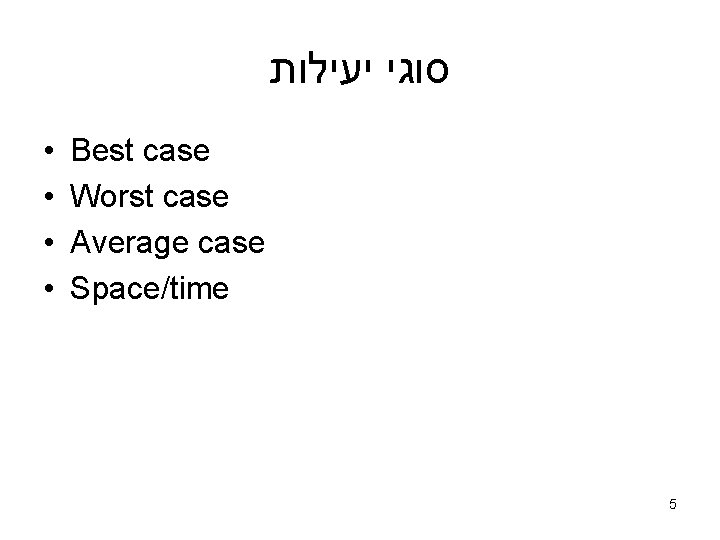
סוגי יעילות • • Best case Worst case Average case Space/time 5
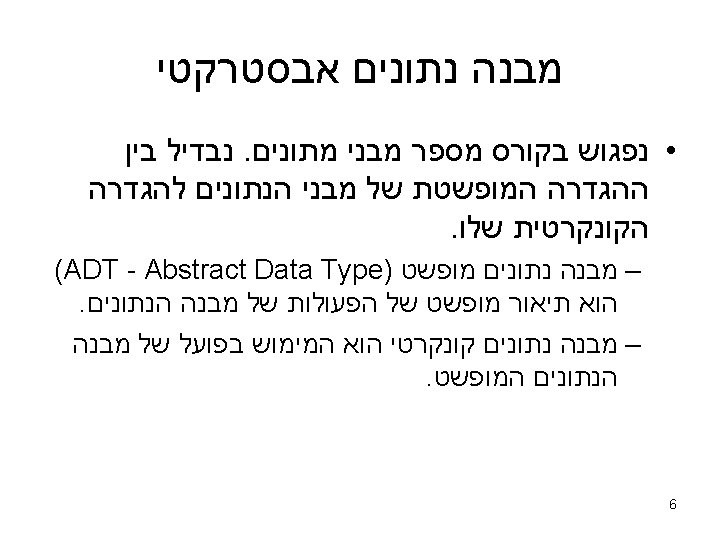
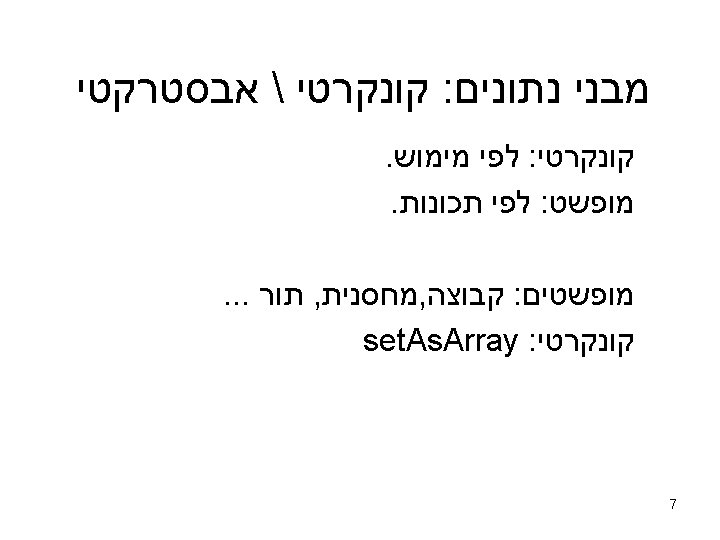
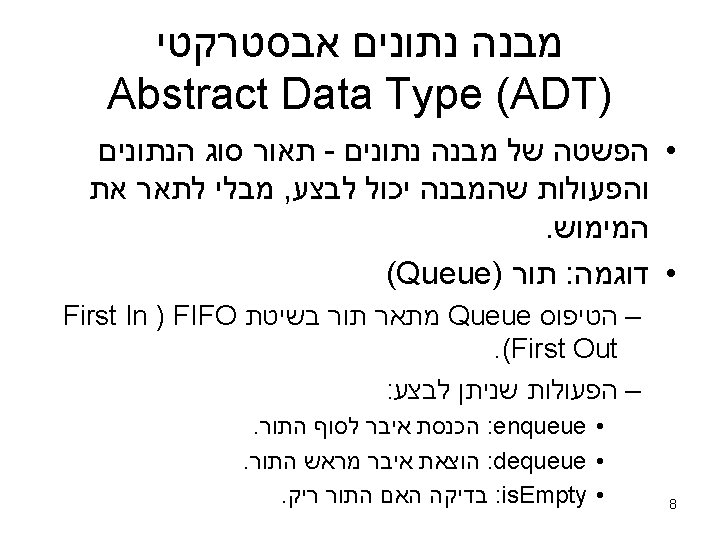
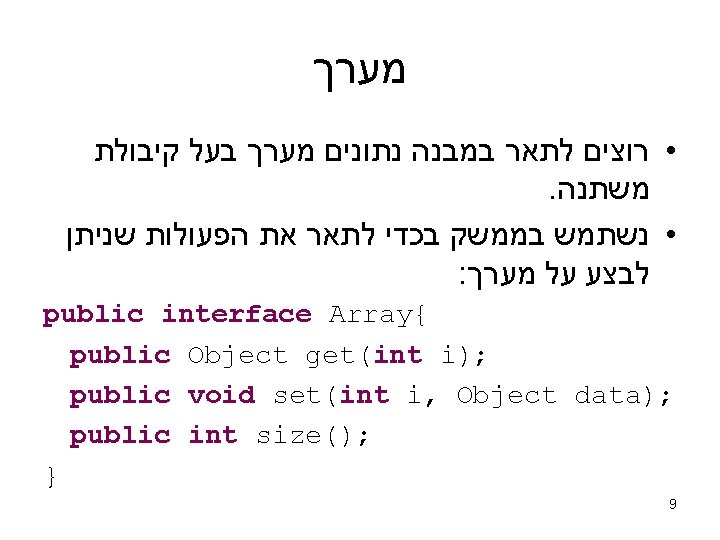
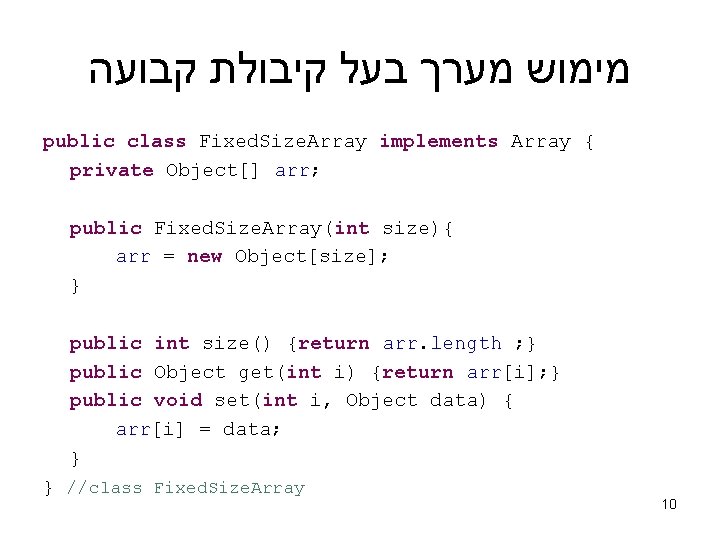
מימוש מערך בעל קיבולת קבועה public class Fixed. Size. Array implements Array { private Object[] arr; public Fixed. Size. Array(int size){ arr = new Object[size]; } public int size() {return arr. length ; } public Object get(int i) {return arr[i]; } public void set(int i, Object data) { arr[i] = data; } } //class Fixed. Size. Array 10
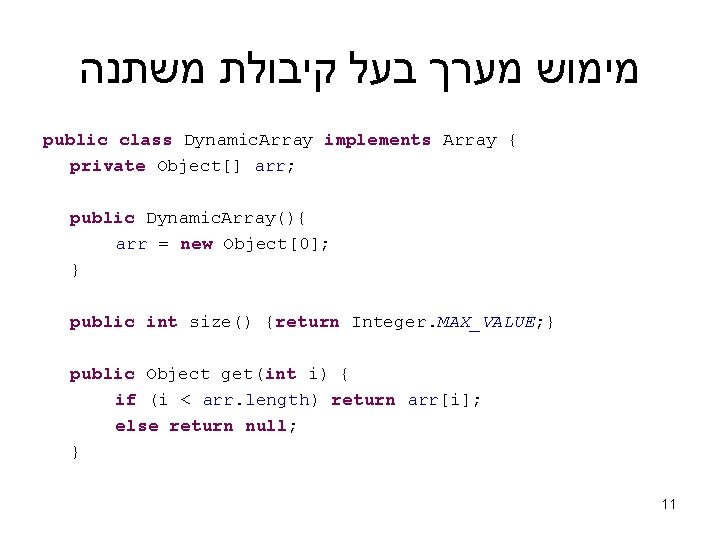
מימוש מערך בעל קיבולת משתנה public class Dynamic. Array implements Array { private Object[] arr; public Dynamic. Array(){ arr = new Object[0]; } public int size() {return Integer. MAX_VALUE; } public Object get(int i) { if (i < arr. length) return arr[i]; else return null; } 11
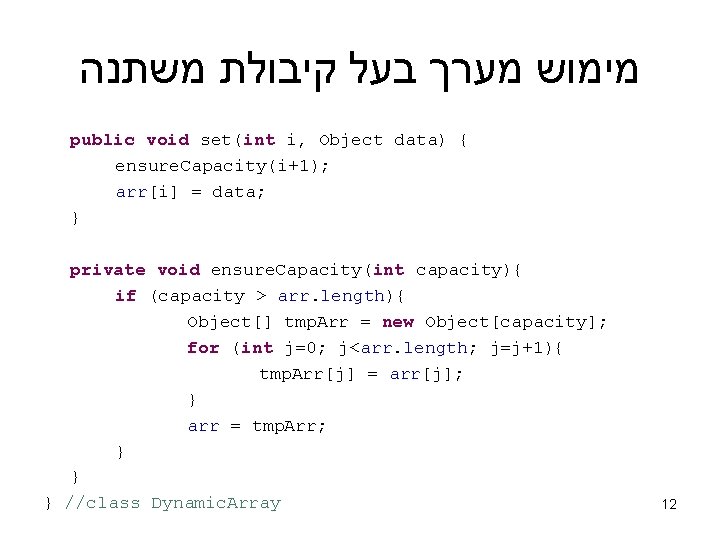
מימוש מערך בעל קיבולת משתנה public void set(int i, Object data) { ensure. Capacity(i+1); arr[i] = data; } private void ensure. Capacity(int capacity){ if (capacity > arr. length){ Object[] tmp. Arr = new Object[capacity]; for (int j=0; j<arr. length; j=j+1){ tmp. Arr[j] = arr[j]; } arr = tmp. Arr; } } } //class Dynamic. Array 12
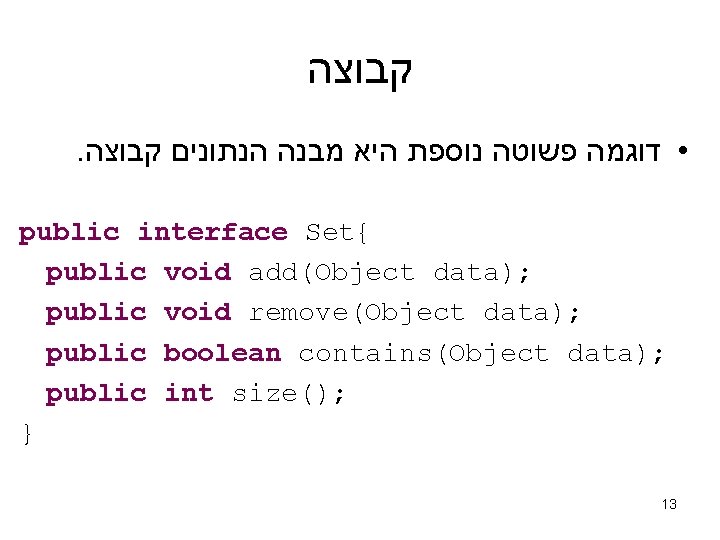
קבוצה . • דוגמה פשוטה נוספת היא מבנה הנתונים קבוצה public interface Set{ public void add(Object data); public void remove(Object data); public boolean contains(Object data); public int size(); } 13
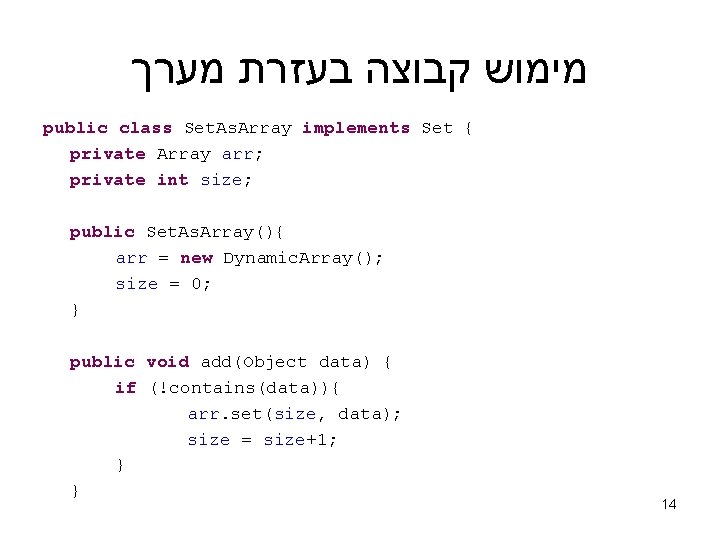
מימוש קבוצה בעזרת מערך public class Set. As. Array implements Set { private Array arr; private int size; public Set. As. Array(){ arr = new Dynamic. Array(); size = 0; } public void add(Object data) { if (!contains(data)){ arr. set(size, data); size = size+1; } } 14
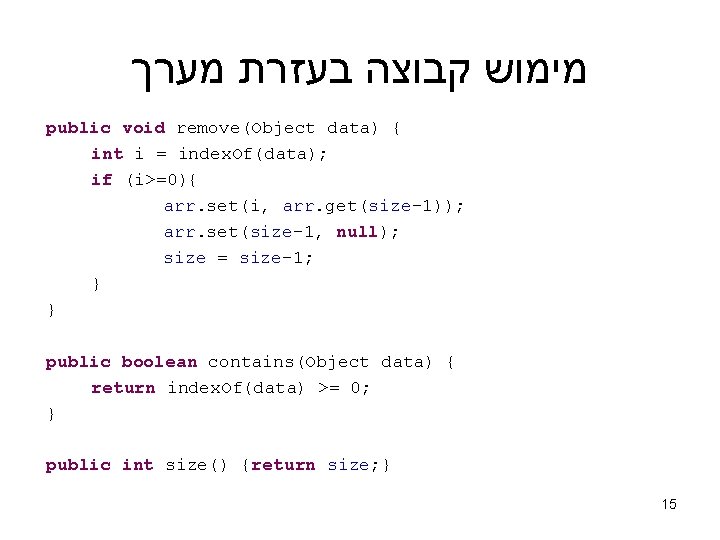
מימוש קבוצה בעזרת מערך public void remove(Object data) { int i = index. Of(data); if (i>=0){ arr. set(i, arr. get(size-1)); arr. set(size-1, null); size = size-1; } } public boolean contains(Object data) { return index. Of(data) >= 0; } public int size() {return size; } 15
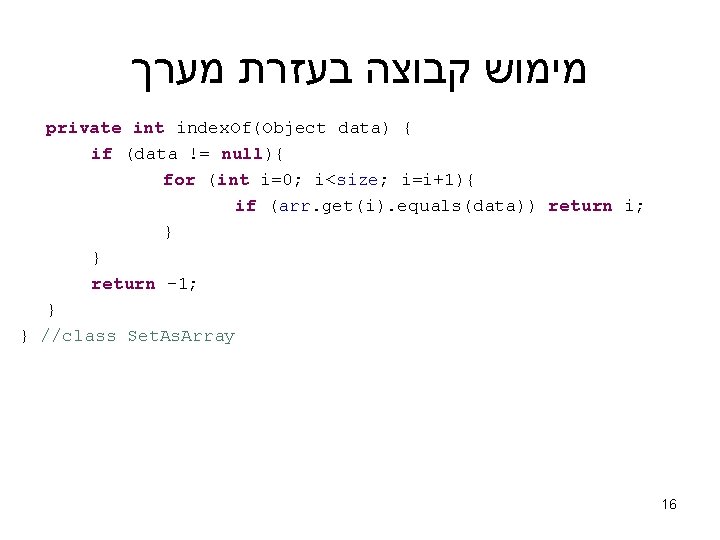
מימוש קבוצה בעזרת מערך private int index. Of(Object data) { if (data != null){ for (int i=0; i<size; i=i+1){ if (arr. get(i). equals(data)) return i; } } return -1; } } //class Set. As. Array 16
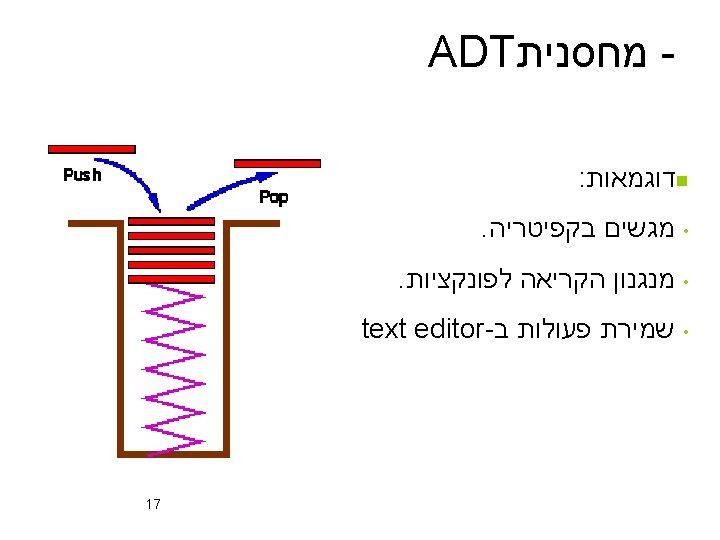
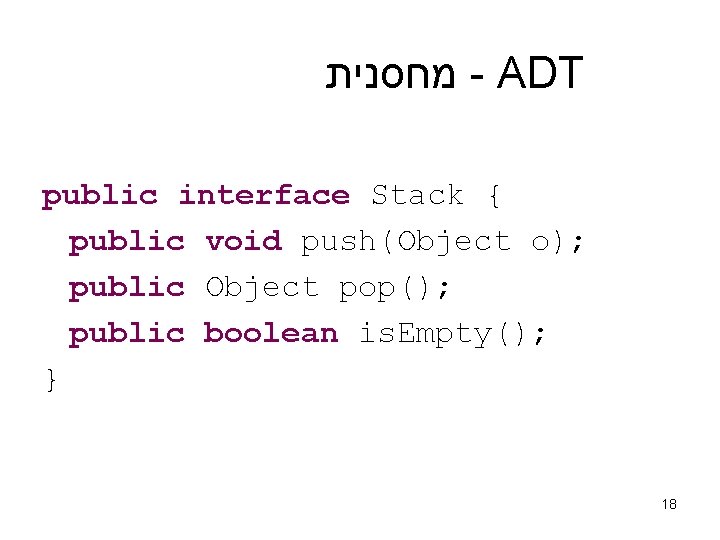
מחסנית - ADT public interface Stack { public void push(Object o); public Object pop(); public boolean is. Empty(); } 18
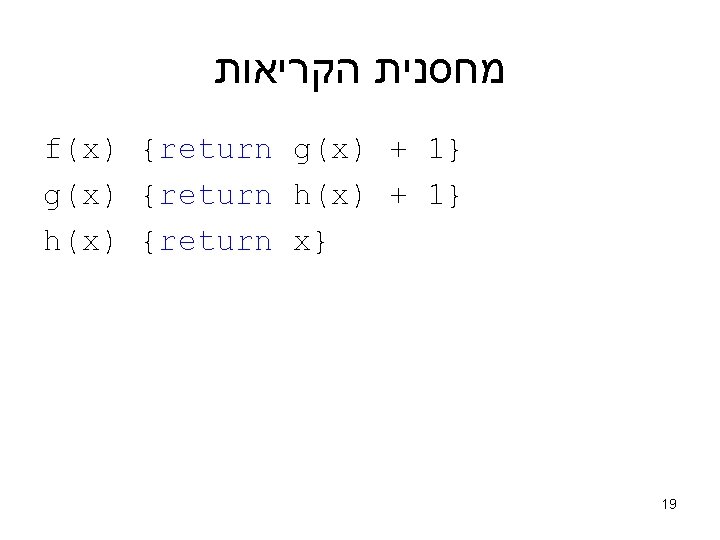
מחסנית הקריאות f(x) {return g(x) + 1} g(x) {return h(x) + 1} h(x) {return x} 19
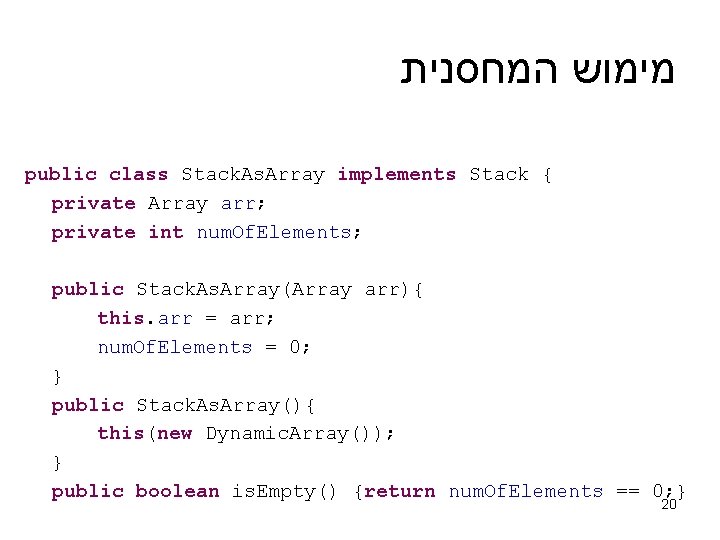
מימוש המחסנית public class Stack. As. Array implements Stack { private Array arr; private int num. Of. Elements; public Stack. As. Array(Array arr){ this. arr = arr; num. Of. Elements = 0; } public Stack. As. Array(){ this(new Dynamic. Array()); } public boolean is. Empty() {return num. Of. Elements == 0; } 20
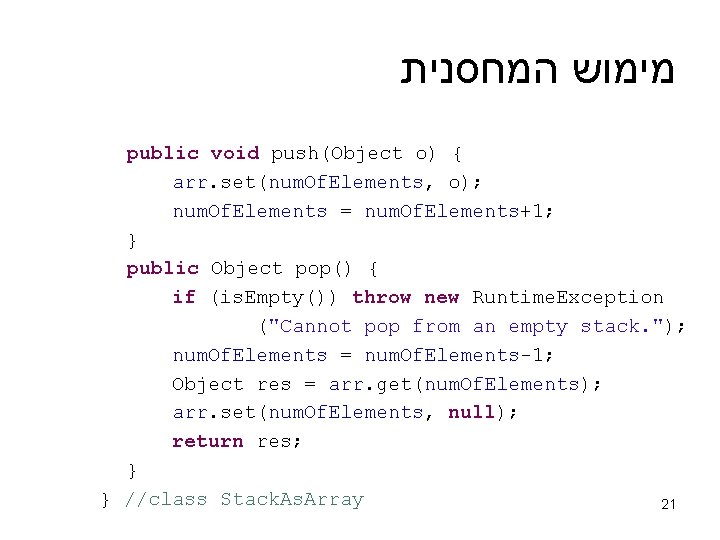
מימוש המחסנית public void push(Object o) { arr. set(num. Of. Elements, o); num. Of. Elements = num. Of. Elements+1; } public Object pop() { if (is. Empty()) throw new Runtime. Exception ("Cannot pop from an empty stack. "); num. Of. Elements = num. Of. Elements-1; Object res = arr. get(num. Of. Elements); arr. set(num. Of. Elements, null); return res; } } //class Stack. As. Array 21
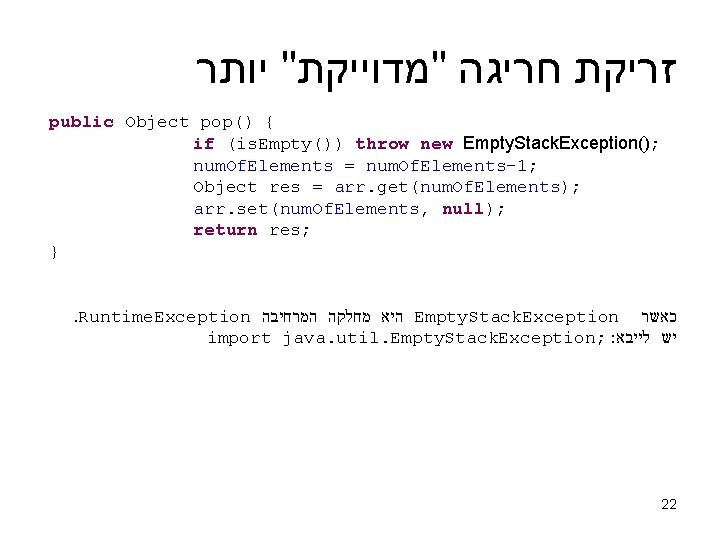
זריקת חריגה "מדוייקת" יותר public Object pop() { if (is. Empty()) throw new Empty. Stack. Exception(); num. Of. Elements = num. Of. Elements-1; Object res = arr. get(num. Of. Elements); arr. set(num. Of. Elements, null); return res; }. Runtime. Exception המרחיבה מחלקה היא Empty. Stack. Exception כאשר import java. util. Empty. Stack. Exception; : לייבא יש 22
![דוגמת שימוש I! מאסטר יודה : public static String yoda(String[] sentence){ String res דוגמת שימוש I! מאסטר יודה : public static String yoda(String[] sentence){ String res](http://slidetodoc.com/presentation_image_h/e5a52659a8bf053e60d3baca5c50c93c/image-23.jpg)
דוגמת שימוש I! מאסטר יודה : public static String yoda(String[] sentence){ String res = ""; Stack st = new stack. As. Array(); for(int i=0; i<sentence. length; i++){ st. push(sentence[i]); } while(!st. is. Empty()){ res = res + " " + st. pop(); } res = res + "? "; return res; } 23
![דוגמת שימוש I! מאסטר יודה : public static void main(String[] args) { String[] דוגמת שימוש I! מאסטר יודה : public static void main(String[] args) { String[]](http://slidetodoc.com/presentation_image_h/e5a52659a8bf053e60d3baca5c50c93c/image-24.jpg)
דוגמת שימוש I! מאסטר יודה : public static void main(String[] args) { String[] sentence = {"it", "isn't", "funny!"}; System. out. println(yoda(sentence)); } funny! isn't it? 24
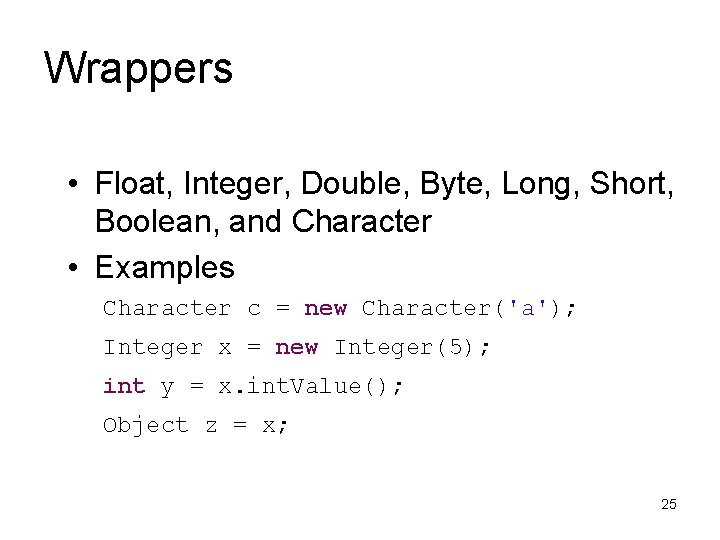
Wrappers • Float, Integer, Double, Byte, Long, Short, Boolean, and Character • Examples Character c = new Character('a'); Integer x = new Integer(5); int y = x. int. Value(); Object z = x; 25
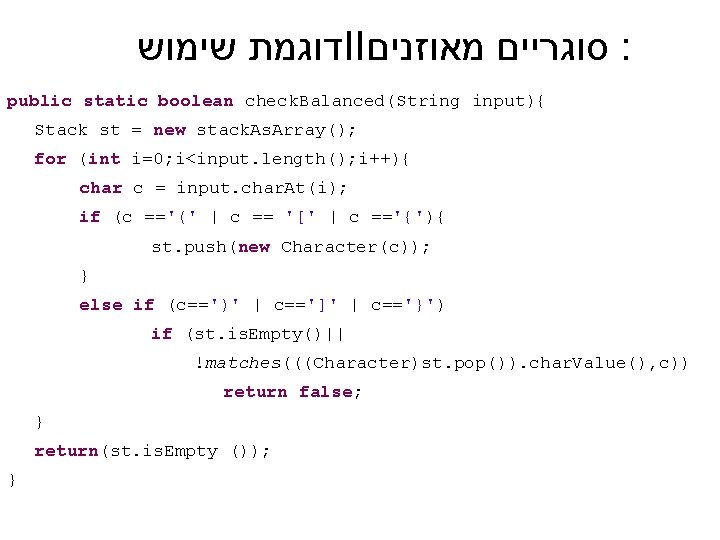
דוגמת שימוש II סוגריים מאוזנים : public static boolean check. Balanced(String input){ Stack st = new stack. As. Array(); for (int i=0; i<input. length(); i++){ char c = input. char. At(i); if (c =='(' | c == '[' | c =='{'){ st. push(new Character(c)); } else if (c==')' | c==']' | c=='}') if (st. is. Empty()|| !matches(((Character)st. pop()). char. Value(), c)) return false; } return(st. is. Empty ()); }
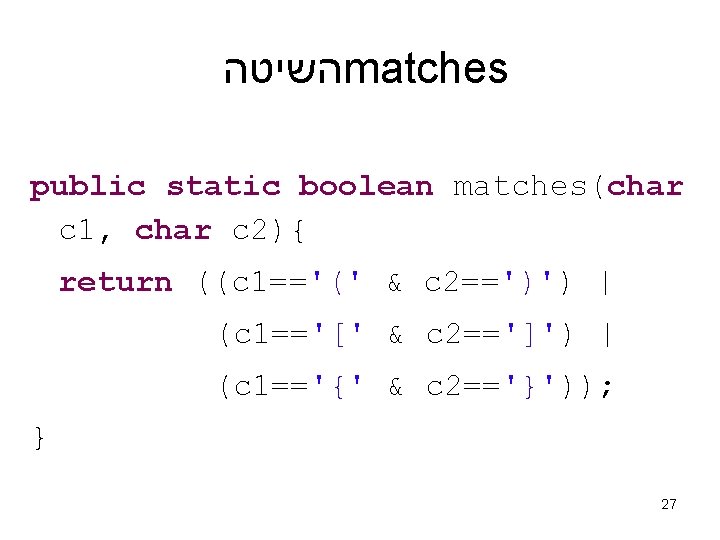
השיטה matches public static boolean matches(char c 1, char c 2){ return ((c 1=='(' & c 2==')') | (c 1=='[' & c 2==']') | (c 1=='{' & c 2=='}')); } 27
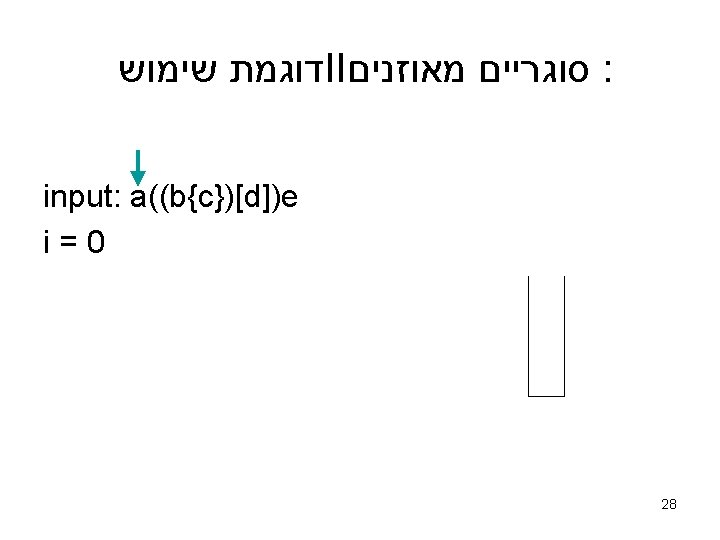
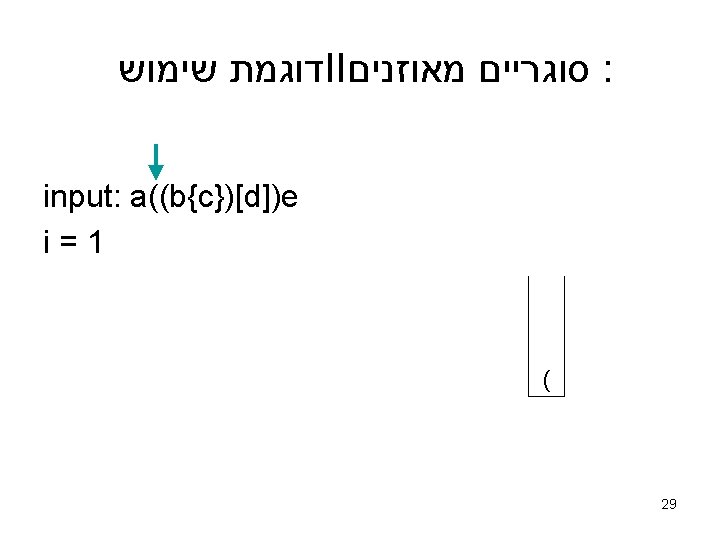
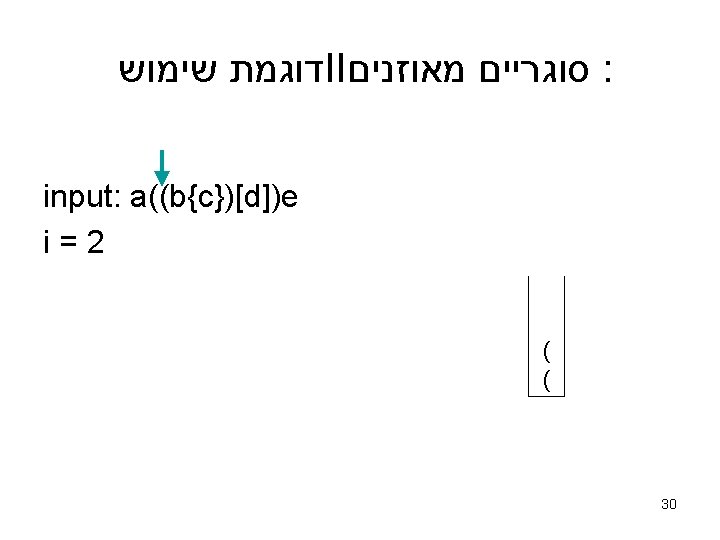
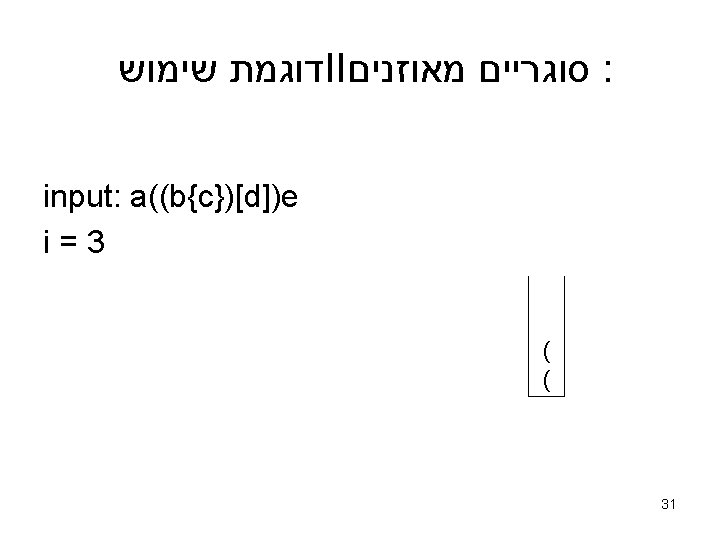
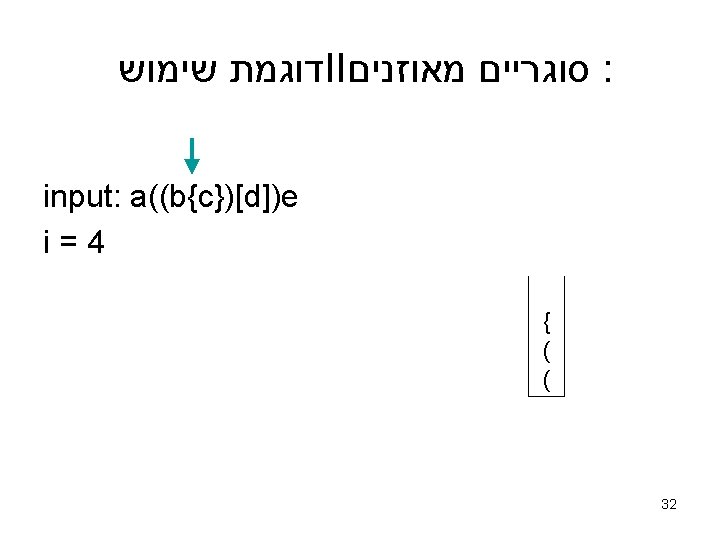
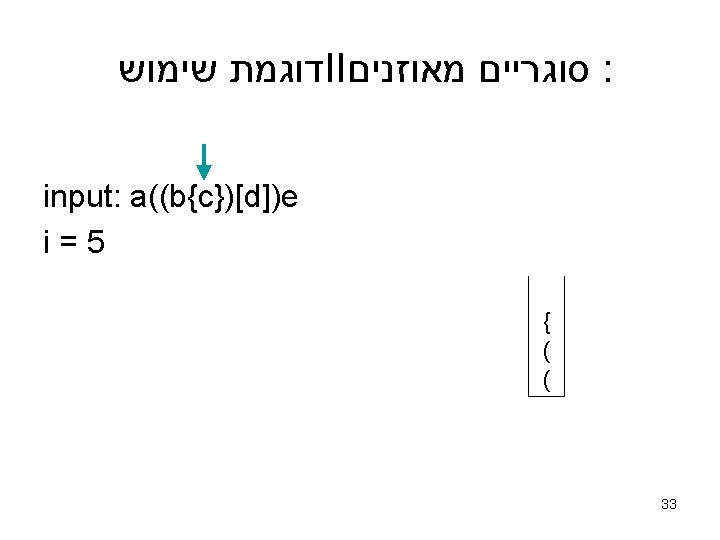
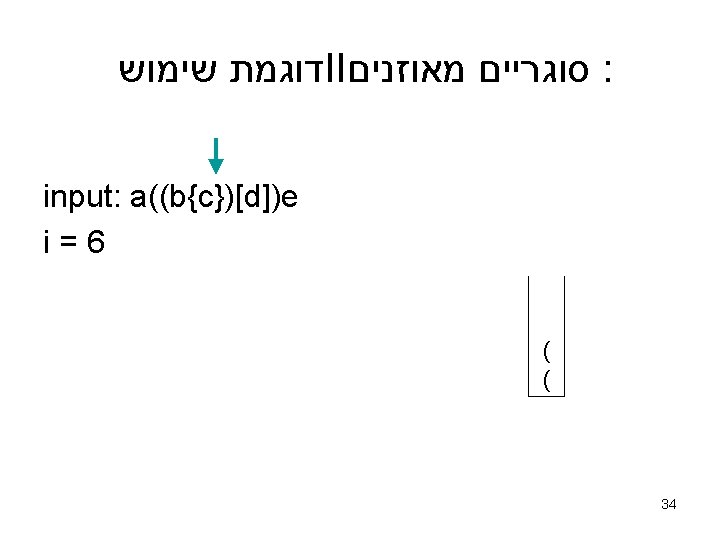
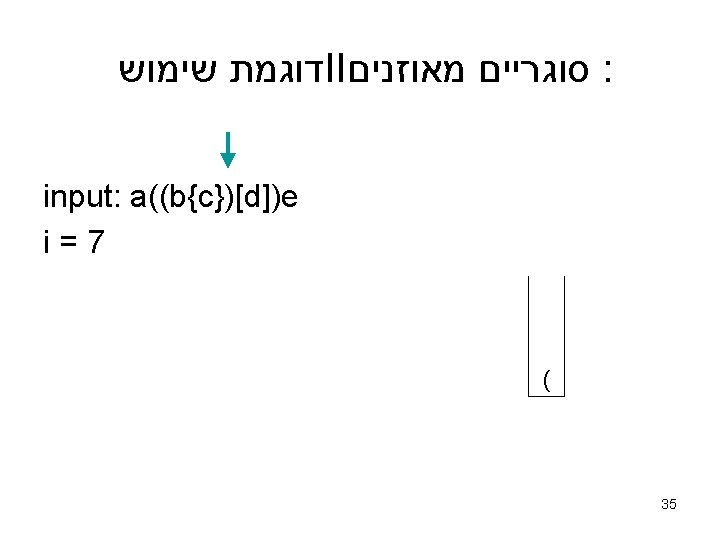
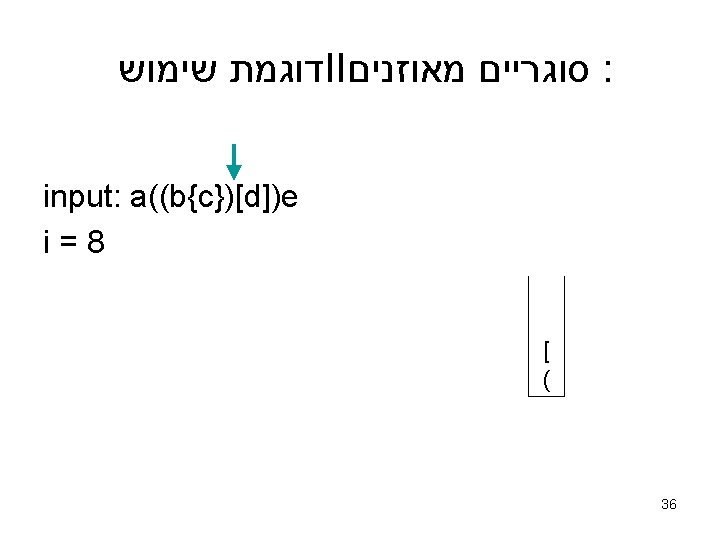
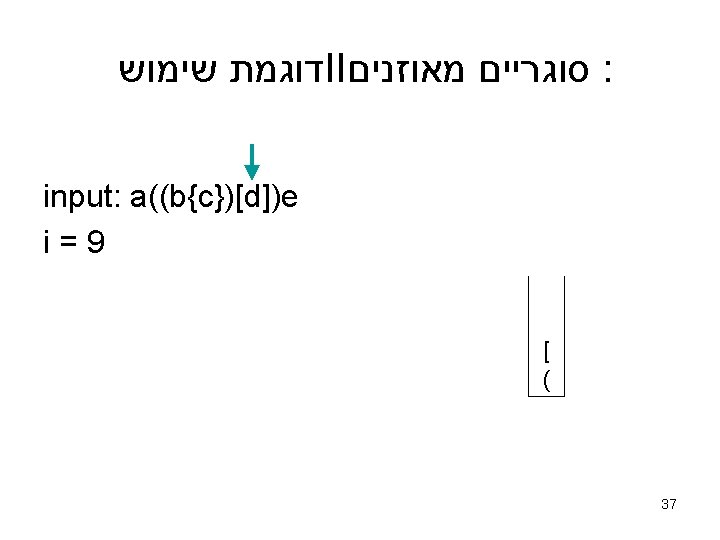
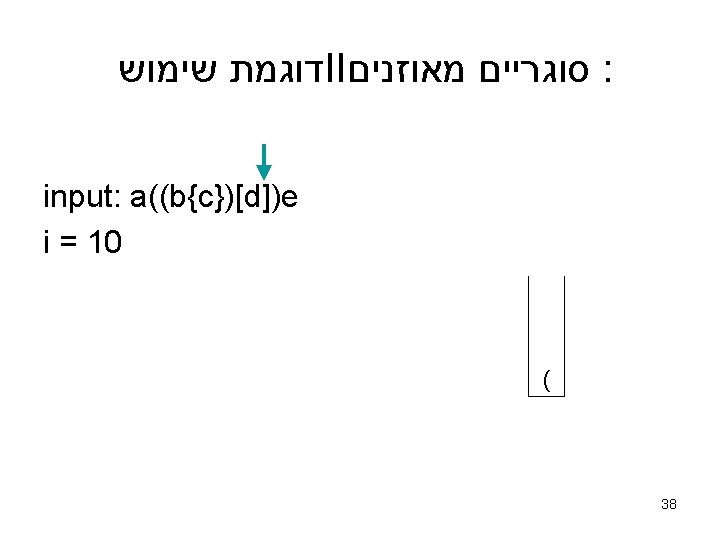
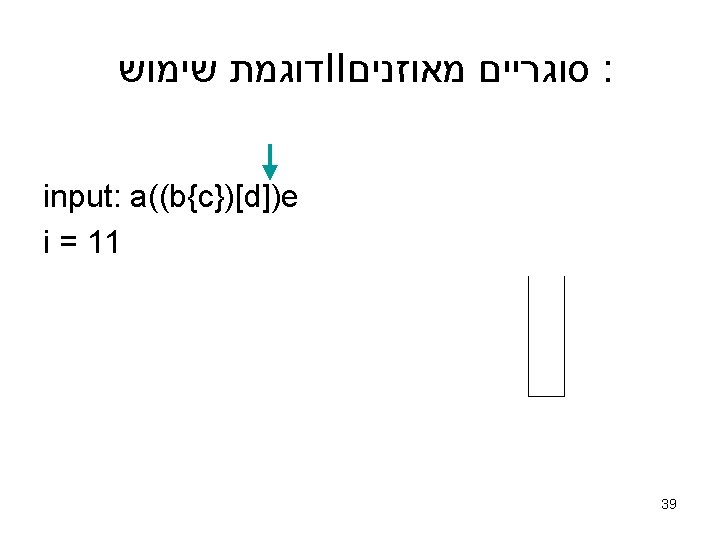
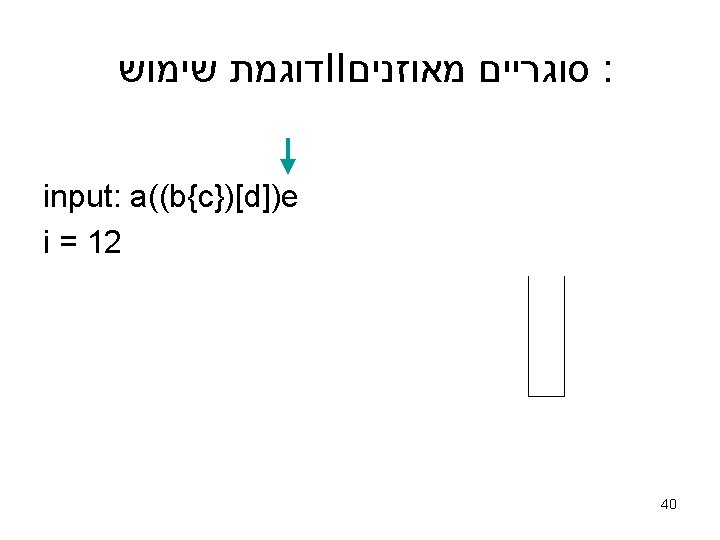
- Slides: 40