Searching CS 105 See Section 14 6 of
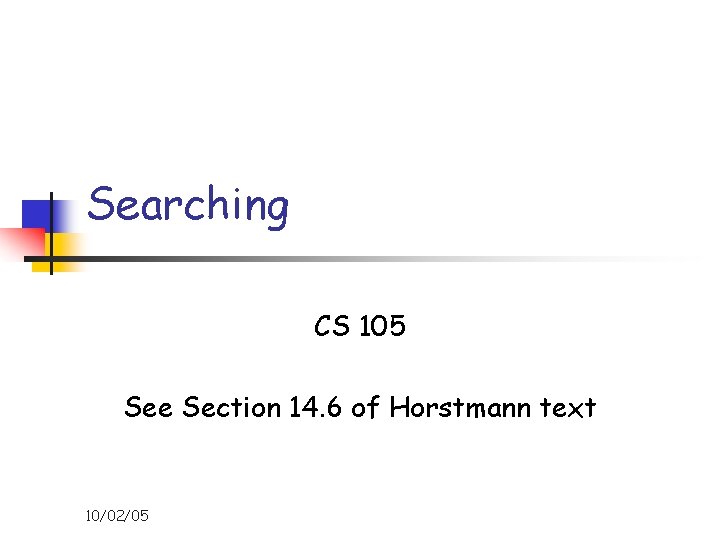
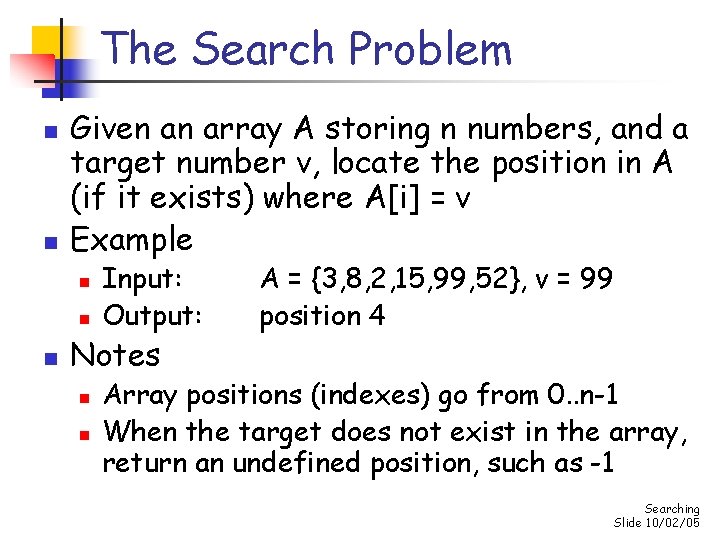
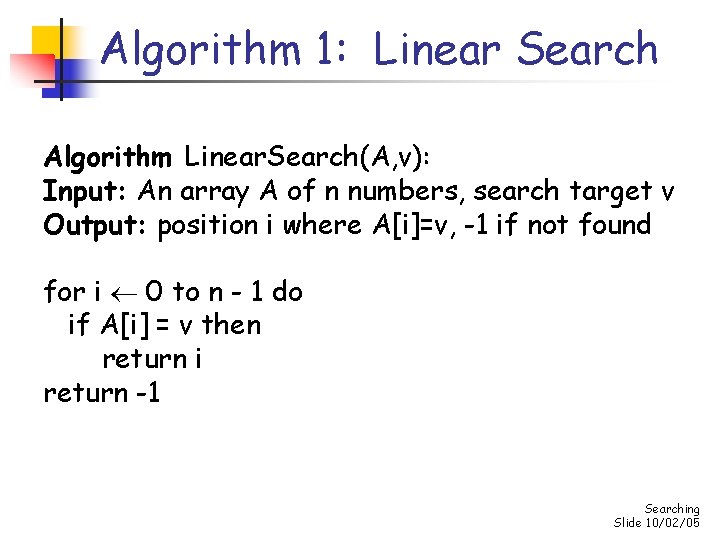
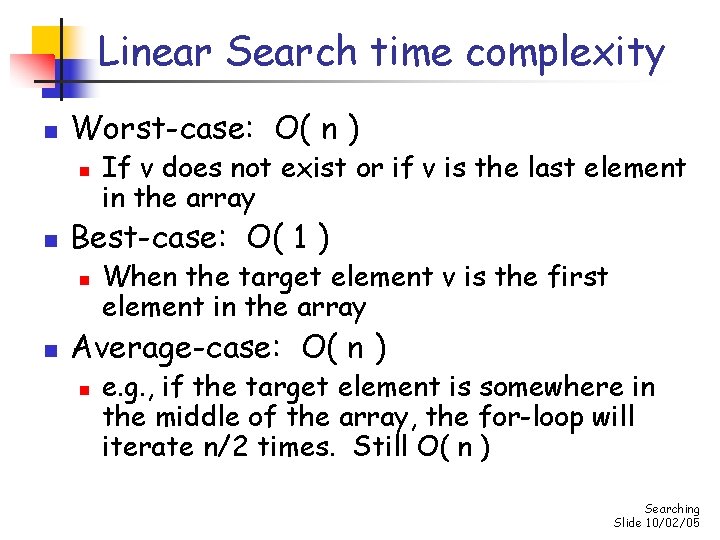
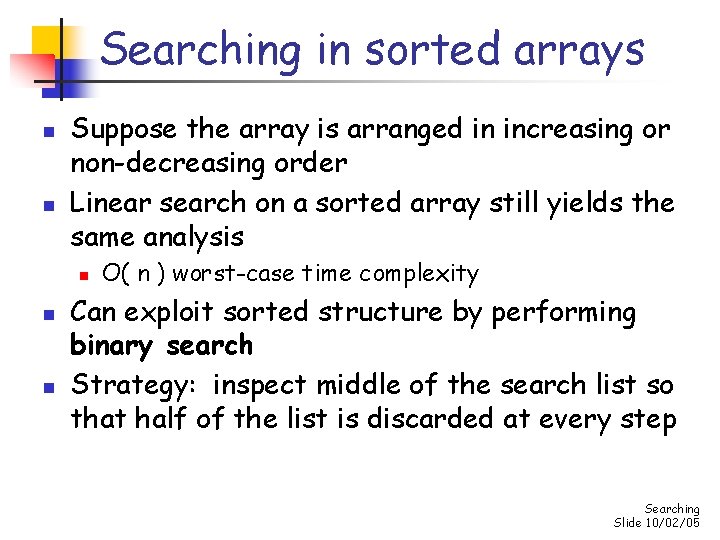
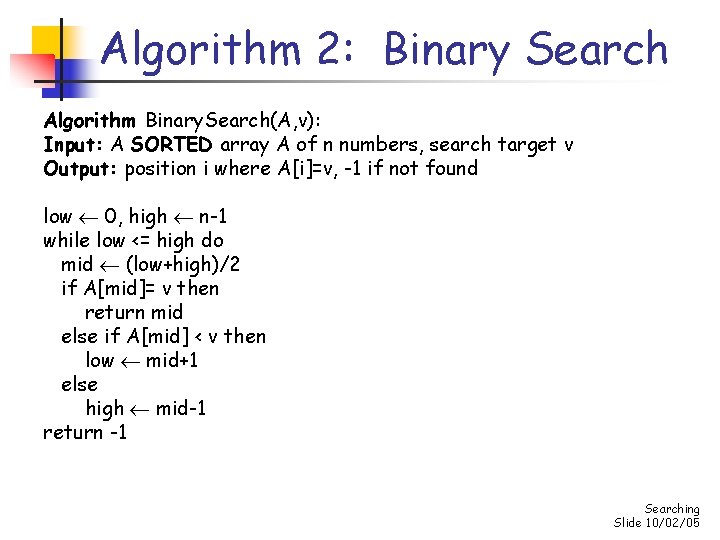
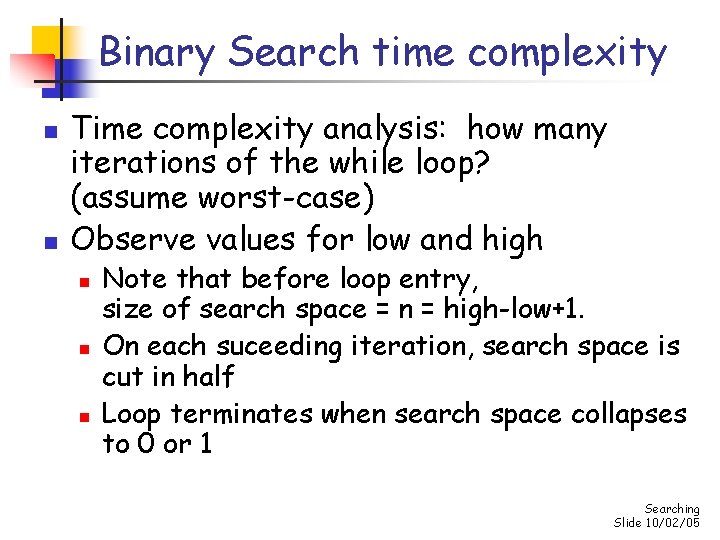
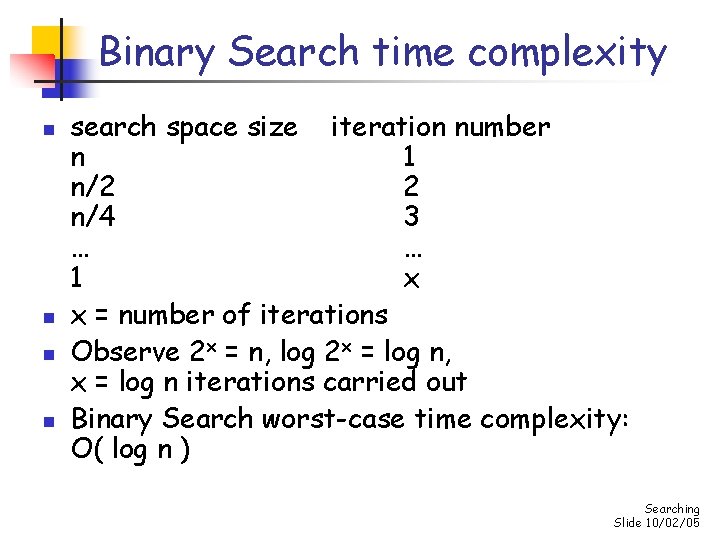
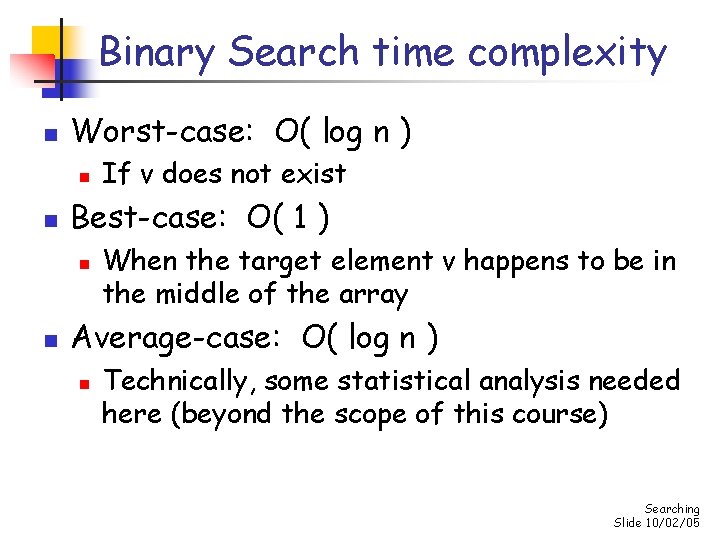
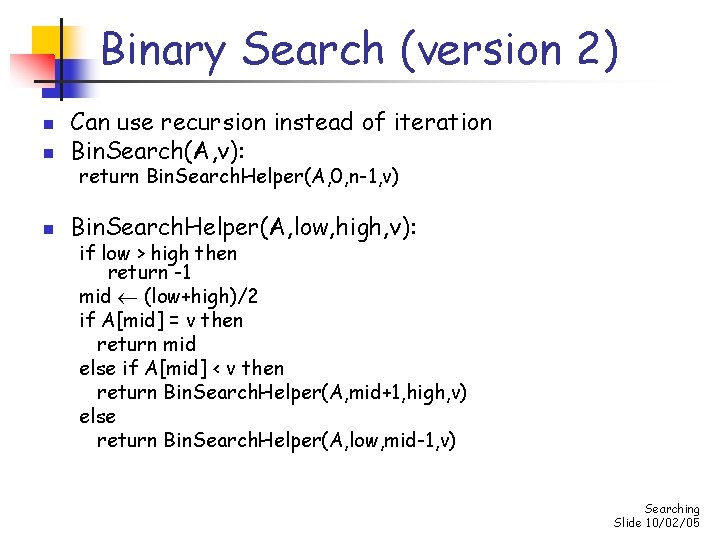
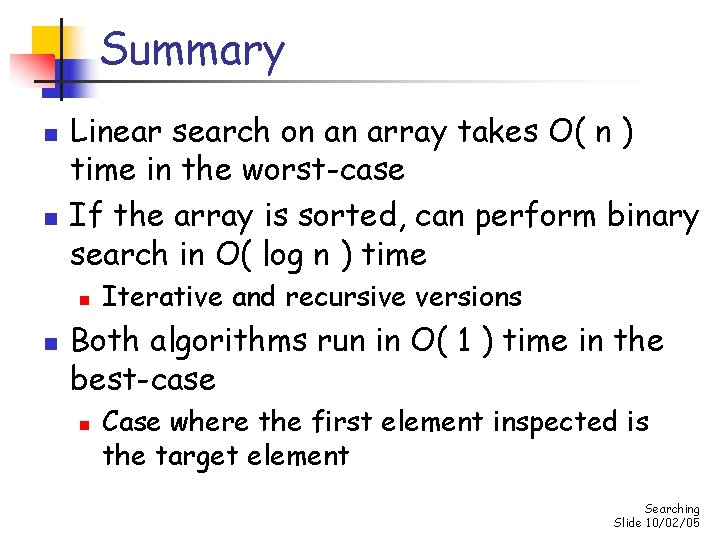
- Slides: 11
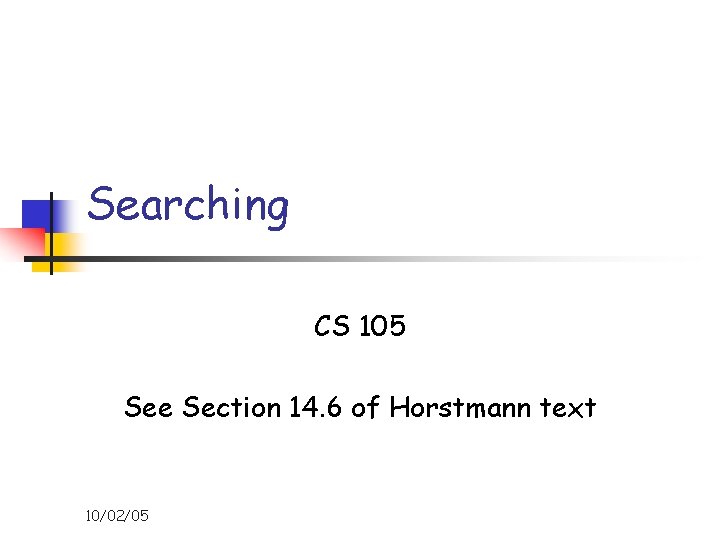
Searching CS 105 See Section 14. 6 of Horstmann text 10/02/05
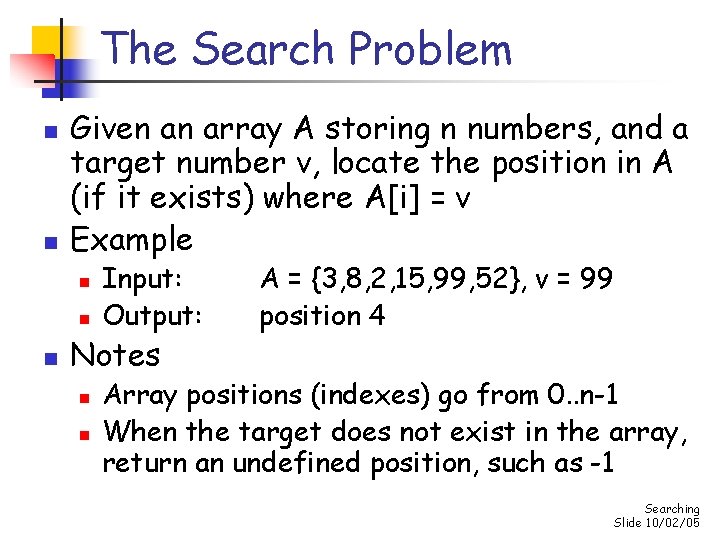
The Search Problem n n Given an array A storing n numbers, and a target number v, locate the position in A (if it exists) where A[i] = v Example n n n Input: Output: Notes n n A = {3, 8, 2, 15, 99, 52}, v = 99 position 4 Array positions (indexes) go from 0. . n-1 When the target does not exist in the array, return an undefined position, such as -1 Searching Slide 10/02/05
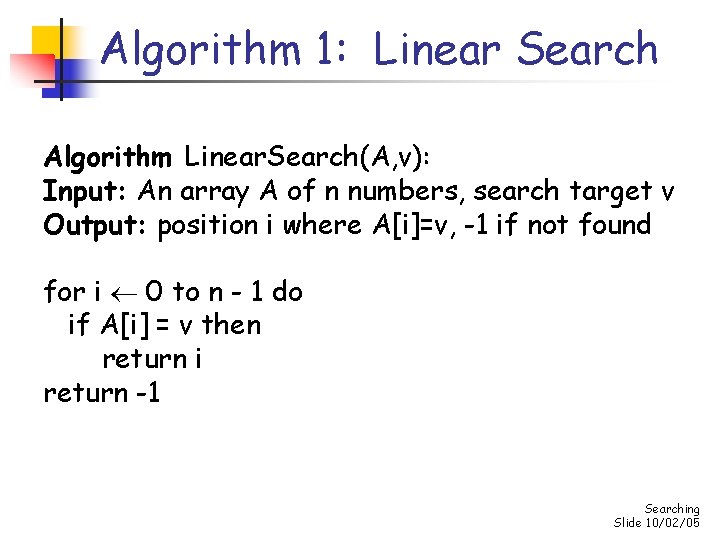
Algorithm 1: Linear Search Algorithm Linear. Search(A, v): Input: An array A of n numbers, search target v Output: position i where A[i]=v, -1 if not found for i 0 to n - 1 do if A[i] = v then return i return -1 Searching Slide 10/02/05
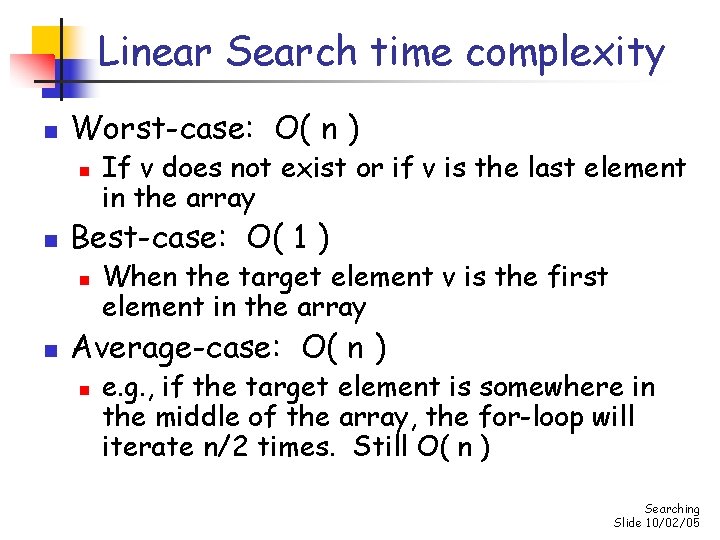
Linear Search time complexity n Worst-case: O( n ) n n Best-case: O( 1 ) n n If v does not exist or if v is the last element in the array When the target element v is the first element in the array Average-case: O( n ) n e. g. , if the target element is somewhere in the middle of the array, the for-loop will iterate n/2 times. Still O( n ) Searching Slide 10/02/05
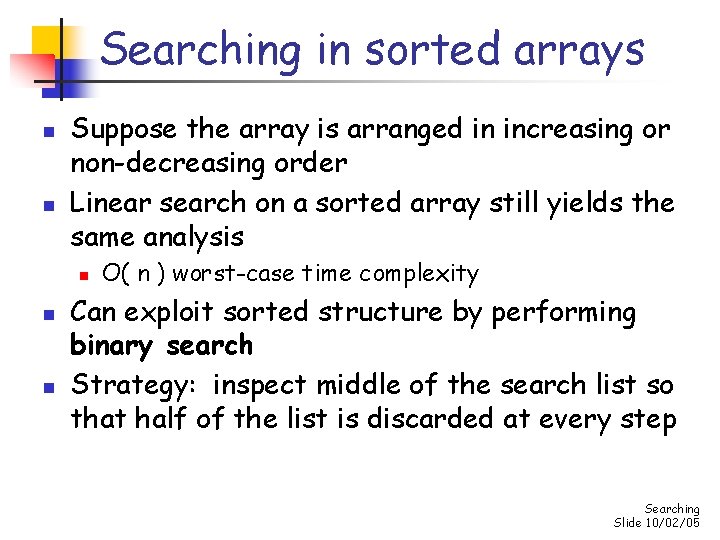
Searching in sorted arrays n n Suppose the array is arranged in increasing or non-decreasing order Linear search on a sorted array still yields the same analysis n n n O( n ) worst-case time complexity Can exploit sorted structure by performing binary search Strategy: inspect middle of the search list so that half of the list is discarded at every step Searching Slide 10/02/05
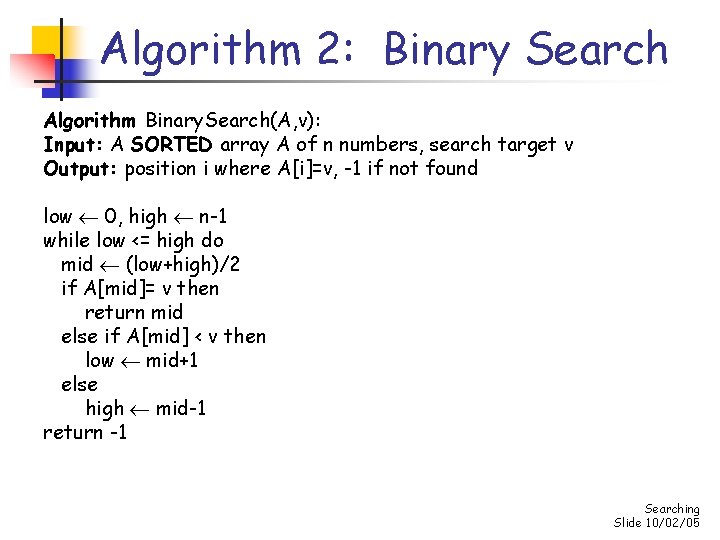
Algorithm 2: Binary Search Algorithm Binary. Search(A, v): Input: A SORTED array A of n numbers, search target v Output: position i where A[i]=v, -1 if not found low 0, high n-1 while low <= high do mid (low+high)/2 if A[mid]= v then return mid else if A[mid] < v then low mid+1 else high mid-1 return -1 Searching Slide 10/02/05
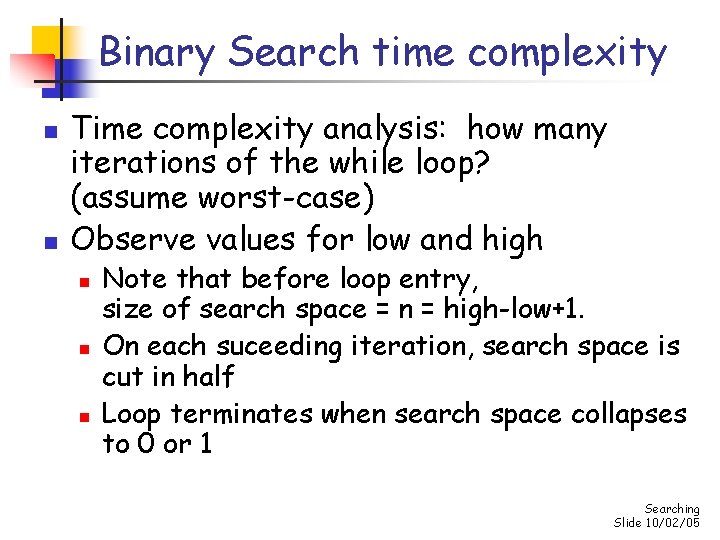
Binary Search time complexity n n Time complexity analysis: how many iterations of the while loop? (assume worst-case) Observe values for low and high n n n Note that before loop entry, size of search space = n = high-low+1. On each suceeding iteration, search space is cut in half Loop terminates when search space collapses to 0 or 1 Searching Slide 10/02/05
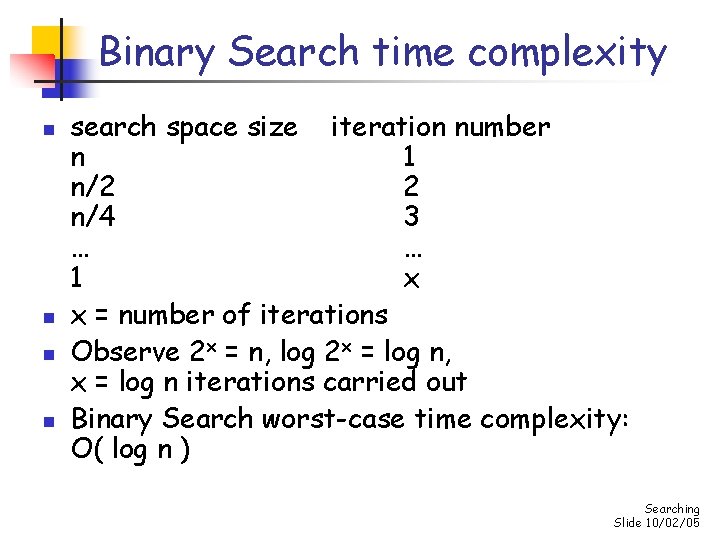
Binary Search time complexity n n search space size iteration number n 1 n/2 2 n/4 3 … … 1 x x = number of iterations Observe 2 x = n, log 2 x = log n, x = log n iterations carried out Binary Search worst-case time complexity: O( log n ) Searching Slide 10/02/05
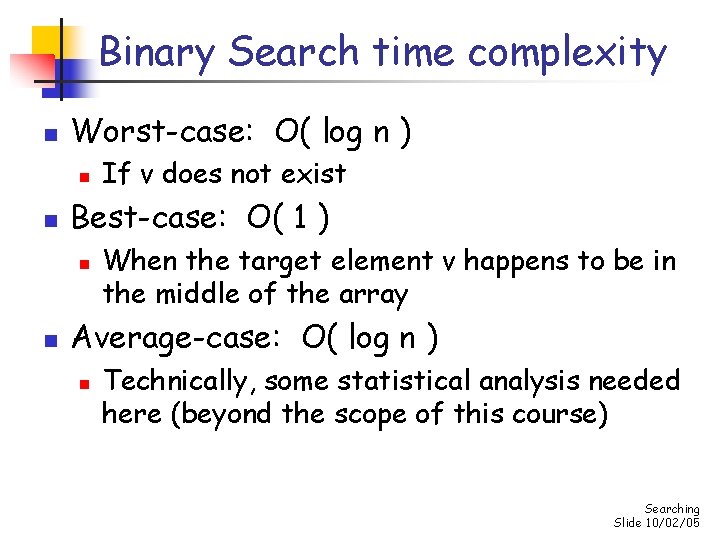
Binary Search time complexity n Worst-case: O( log n ) n n Best-case: O( 1 ) n n If v does not exist When the target element v happens to be in the middle of the array Average-case: O( log n ) n Technically, some statistical analysis needed here (beyond the scope of this course) Searching Slide 10/02/05
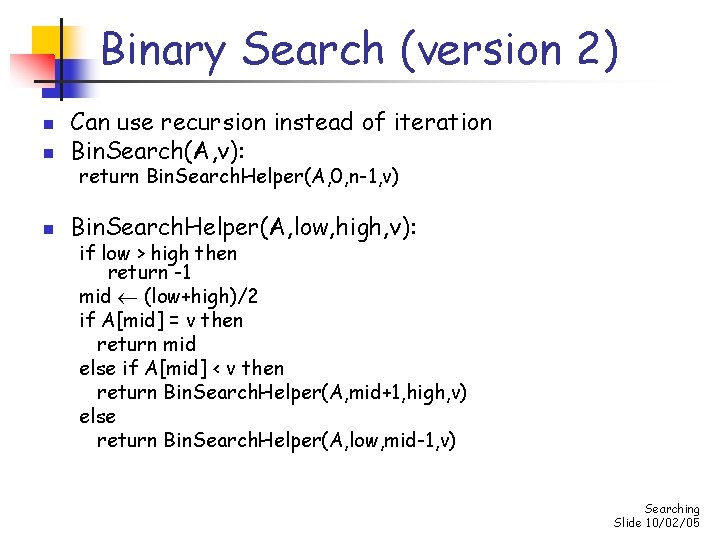
Binary Search (version 2) n Can use recursion instead of iteration Bin. Search(A, v): n Bin. Search. Helper(A, low, high, v): n return Bin. Search. Helper(A, 0, n-1, v) if low > high then return -1 mid (low+high)/2 if A[mid] = v then return mid else if A[mid] < v then return Bin. Search. Helper(A, mid+1, high, v) else return Bin. Search. Helper(A, low, mid-1, v) Searching Slide 10/02/05
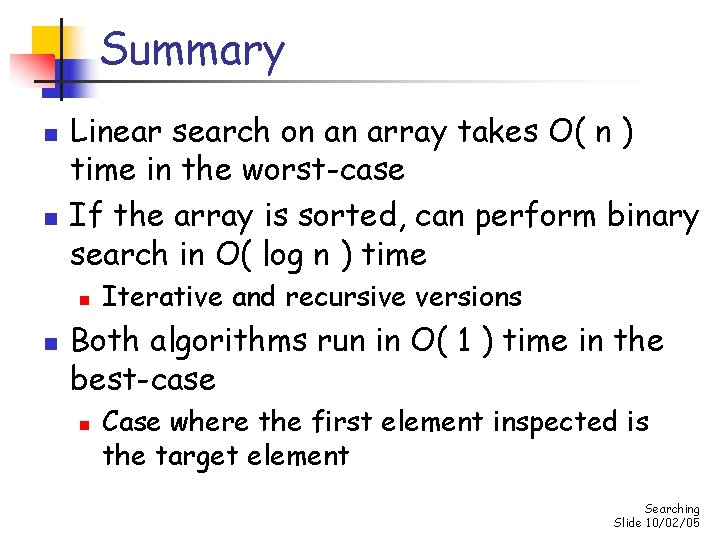
Summary n n Linear search on an array takes O( n ) time in the worst-case If the array is sorted, can perform binary search in O( log n ) time n n Iterative and recursive versions Both algorithms run in O( 1 ) time in the best-case n Case where the first element inspected is the target element Searching Slide 10/02/05
Sectional views
Sequential search algorithm
Searching for solutions in artificial intelligence
Pencarian elemen dalam array disebut juga dengan
Keyword search techniques
Orthogonal range searching
Solving problem by searching
While the csi team is searching the crime scene, _____.
Algoritma searching
Kekurangan binary search
Sorting dan searching
A bear searching for food wanders 35 meters east