Sorting Sorting refers to arranging data in a
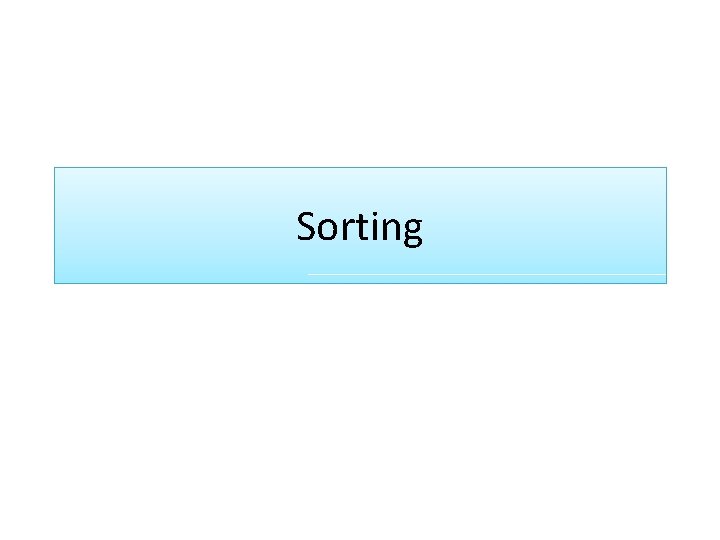
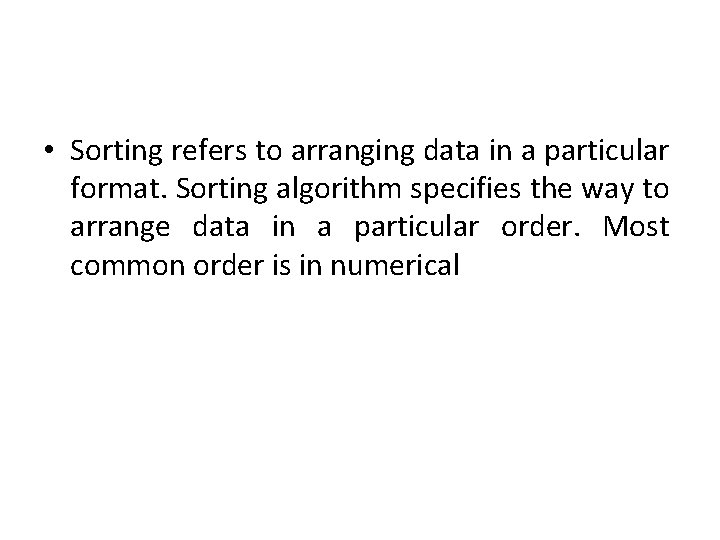
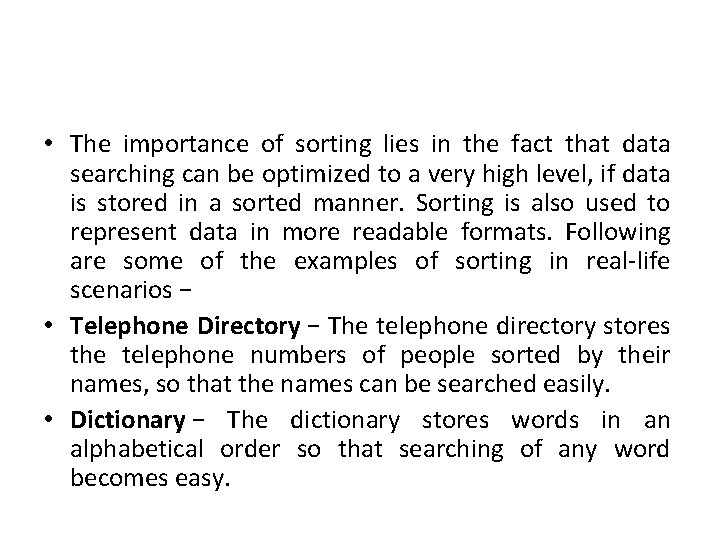
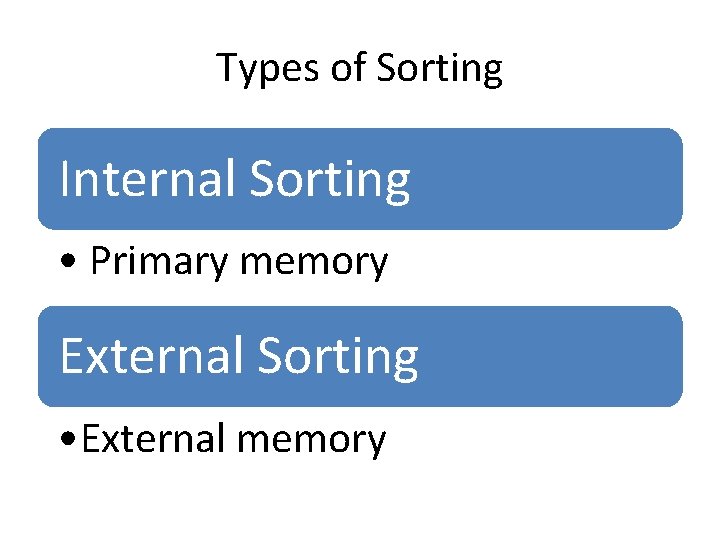
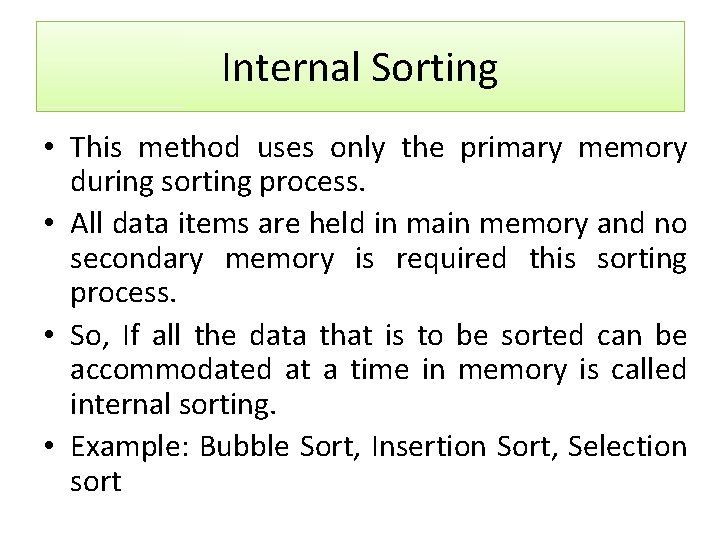
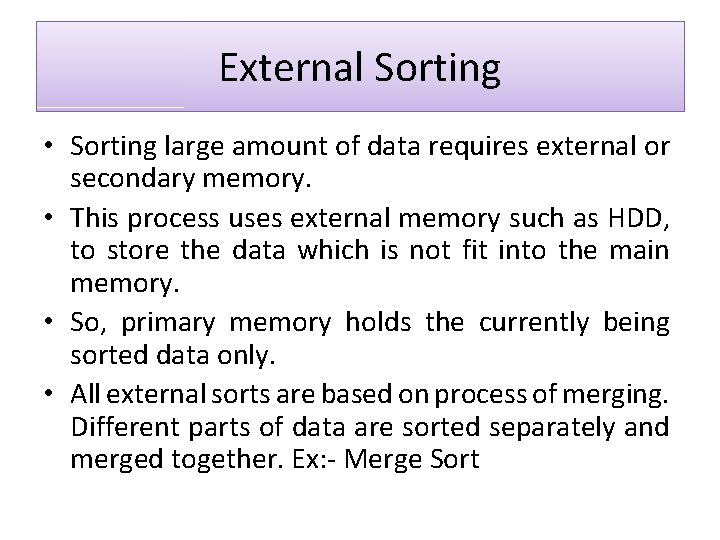
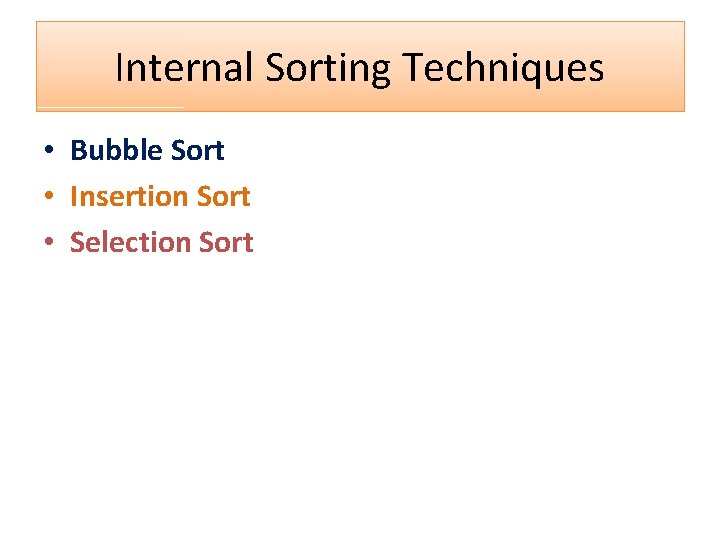
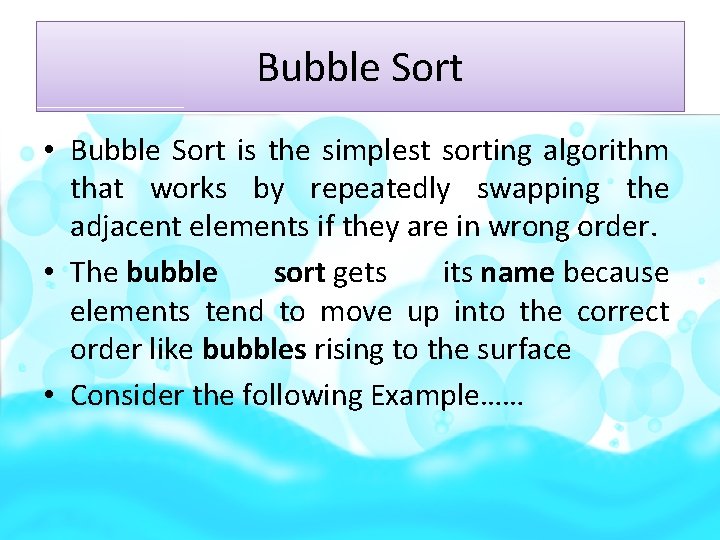
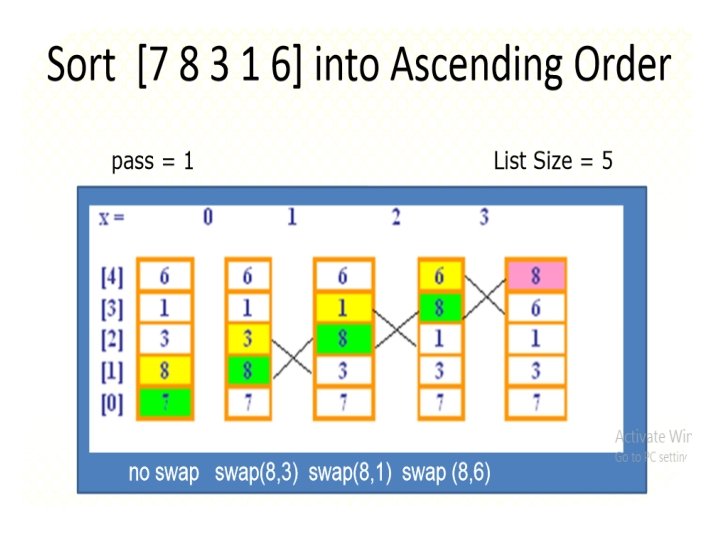
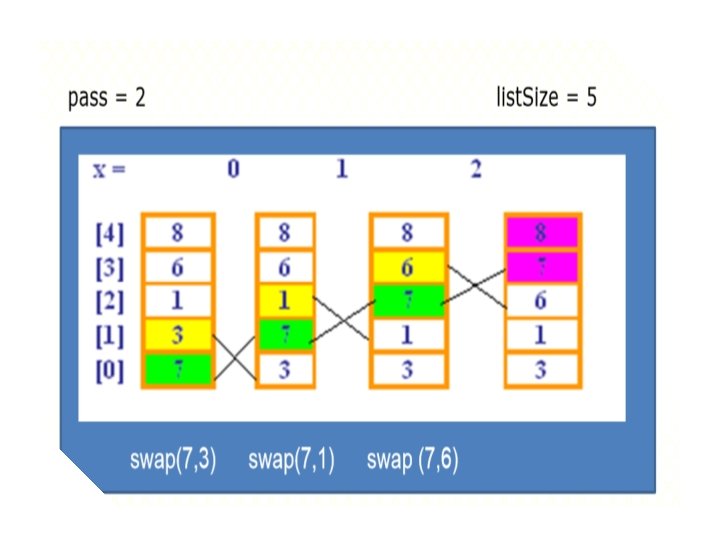
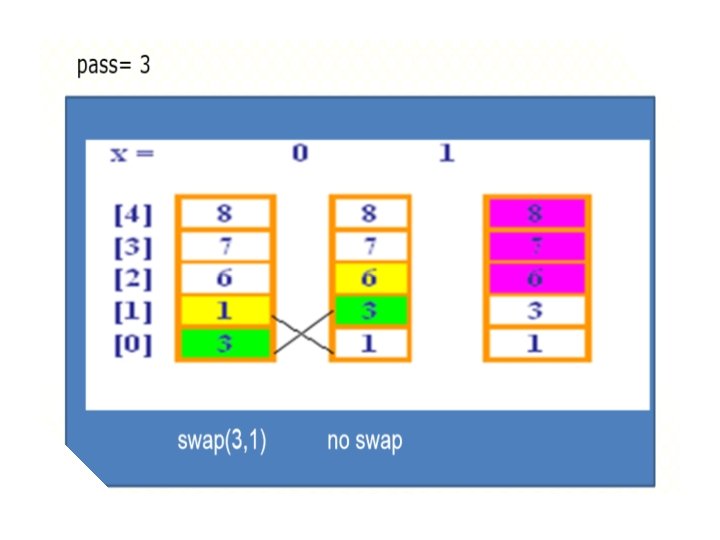
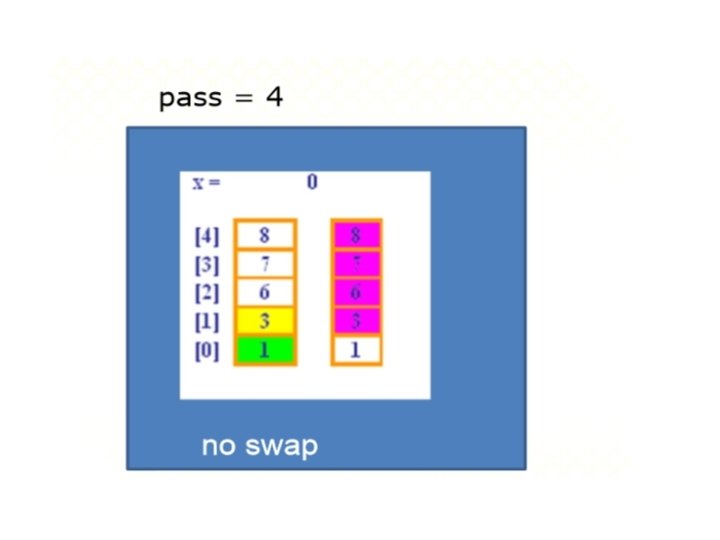
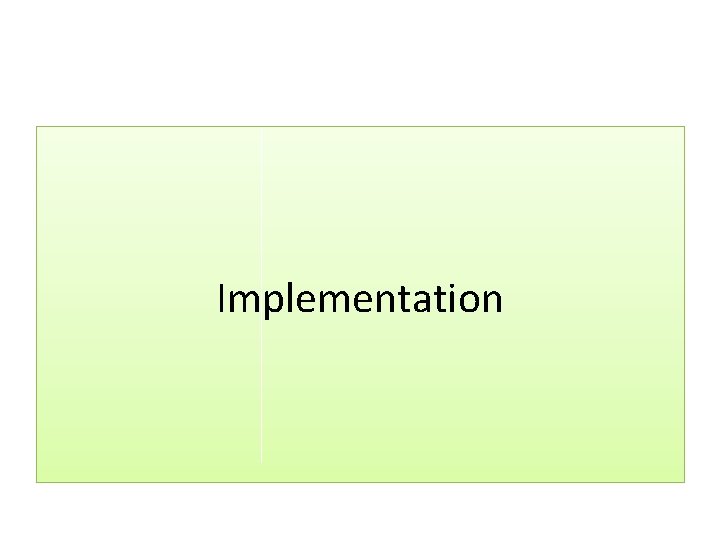
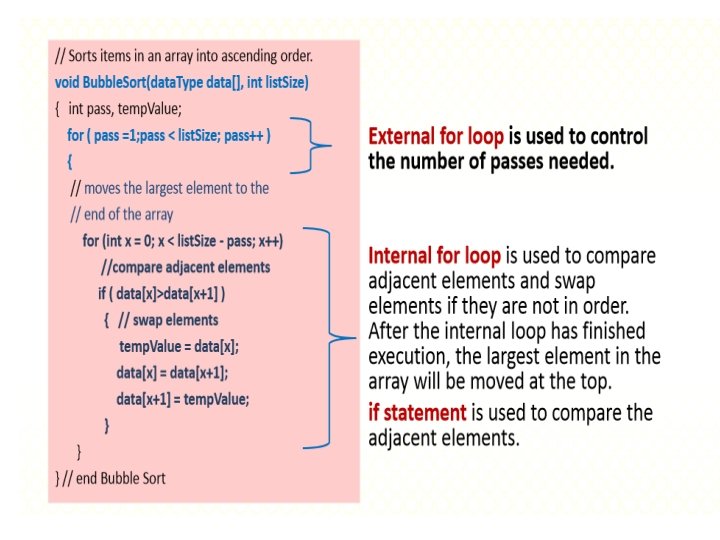
![A function to implement bubble sort • void bubble. Sort(int arr[], int n) { A function to implement bubble sort • void bubble. Sort(int arr[], int n) {](https://slidetodoc.com/presentation_image_h2/cb65a621f99cd599681a4afa22726137/image-15.jpg)
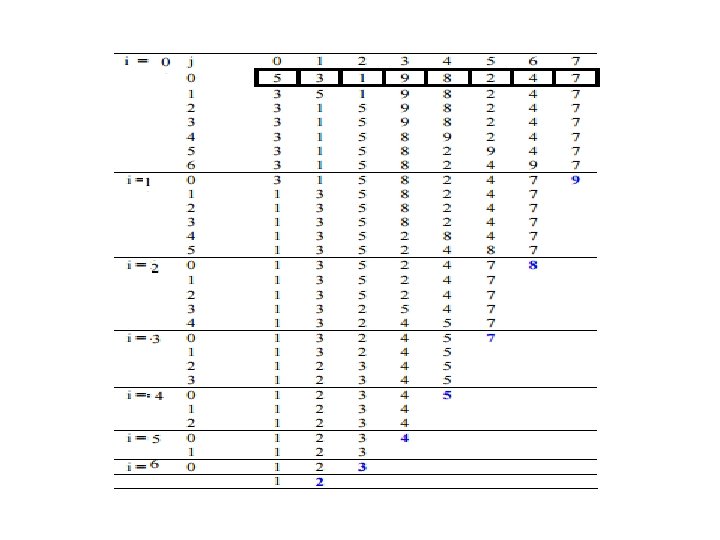
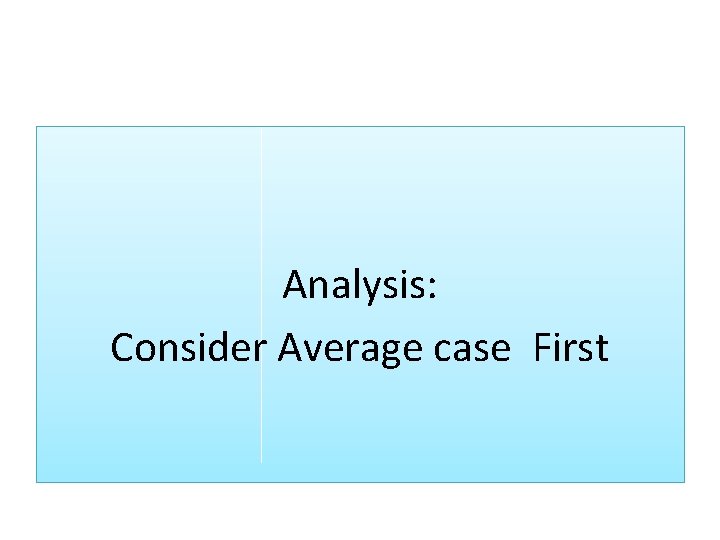
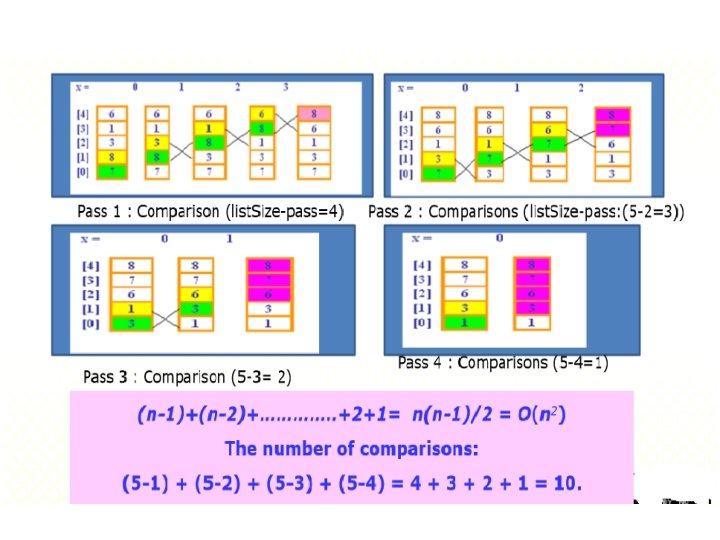
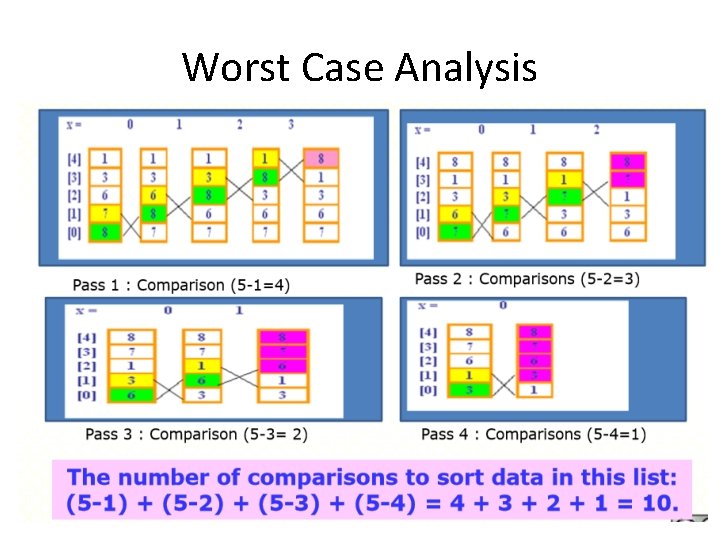
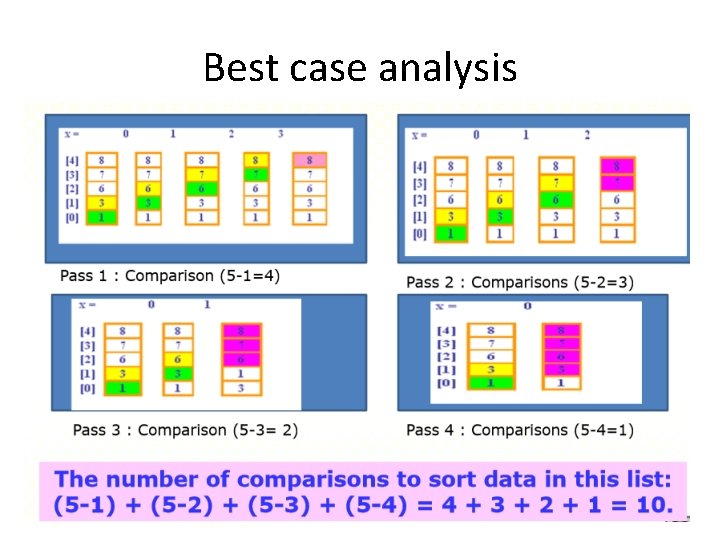
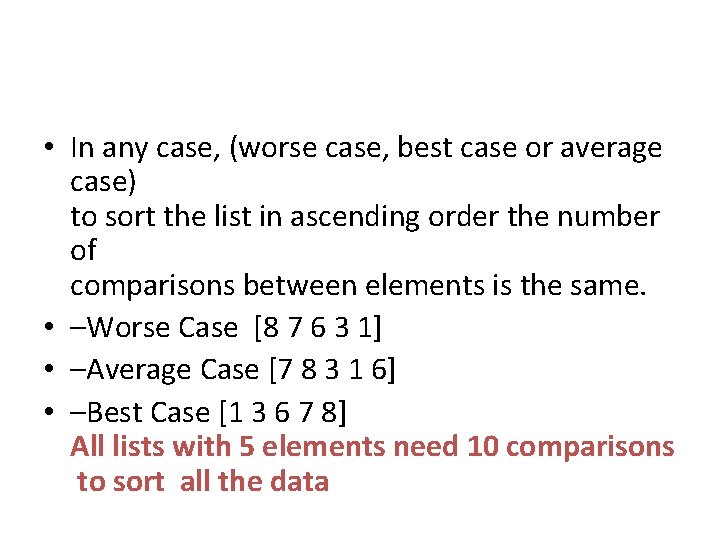
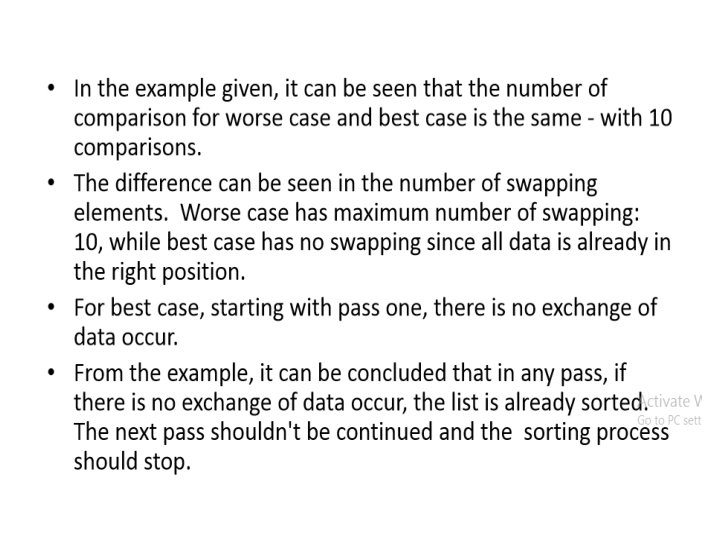
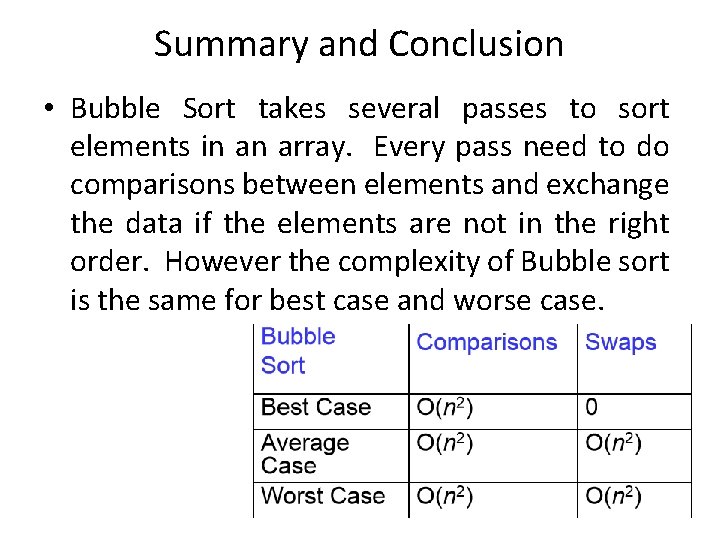
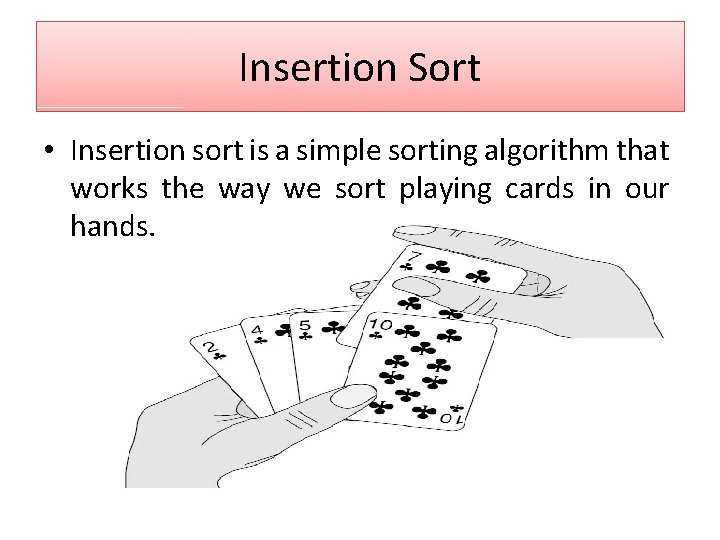
![• We start with an empty left hand [sorted array] and the cards • We start with an empty left hand [sorted array] and the cards](https://slidetodoc.com/presentation_image_h2/cb65a621f99cd599681a4afa22726137/image-25.jpg)
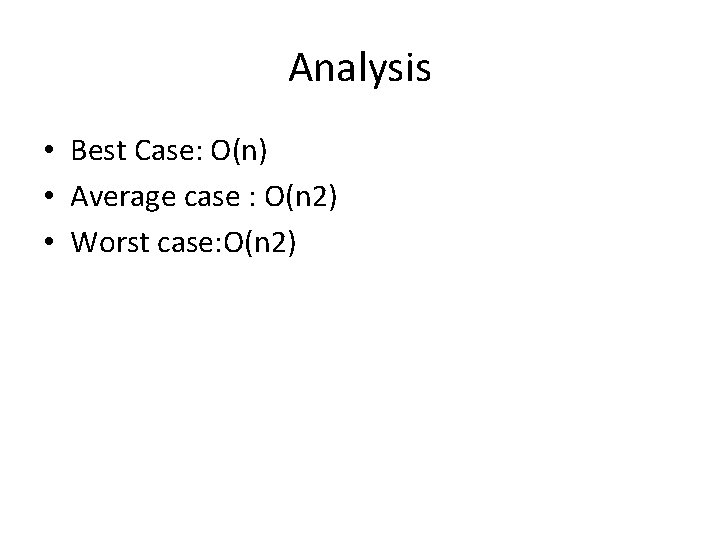
- Slides: 26
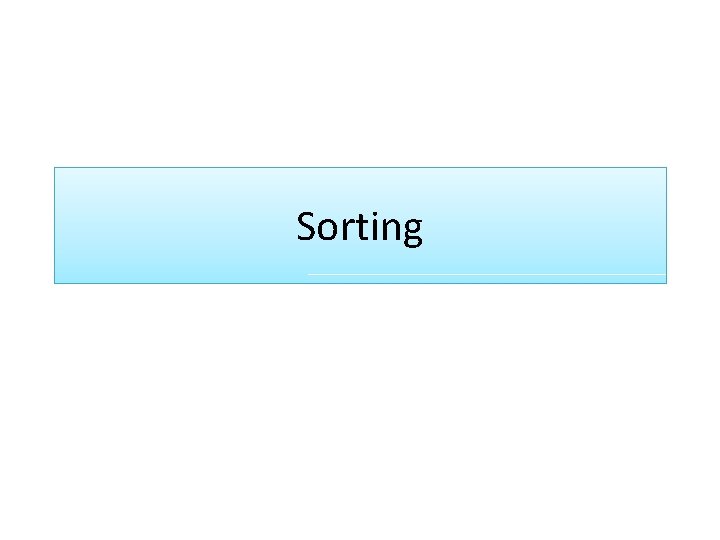
Sorting
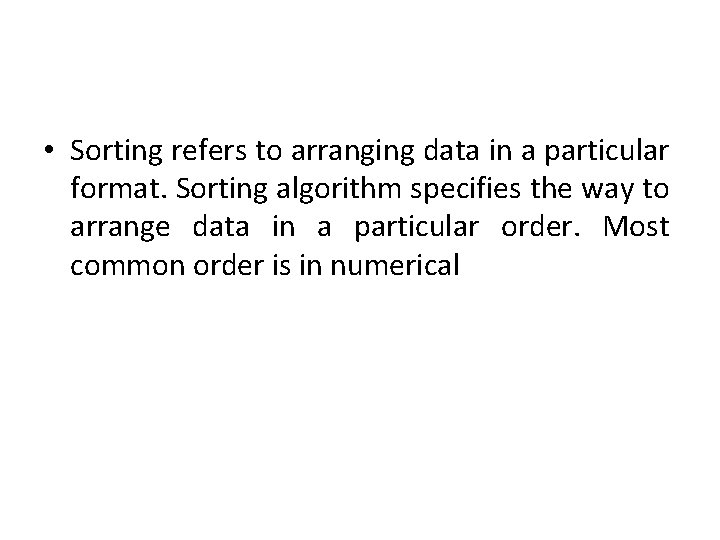
• Sorting refers to arranging data in a particular format. Sorting algorithm specifies the way to arrange data in a particular order. Most common order is in numerical
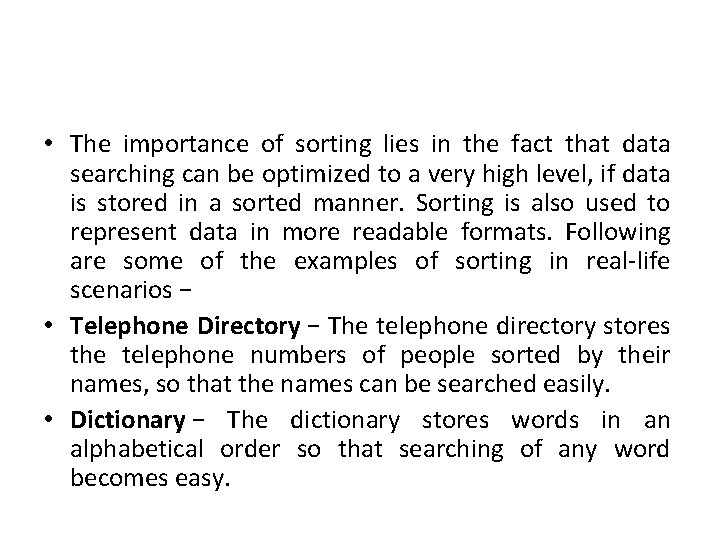
• The importance of sorting lies in the fact that data searching can be optimized to a very high level, if data is stored in a sorted manner. Sorting is also used to represent data in more readable formats. Following are some of the examples of sorting in real-life scenarios − • Telephone Directory − The telephone directory stores the telephone numbers of people sorted by their names, so that the names can be searched easily. • Dictionary − The dictionary stores words in an alphabetical order so that searching of any word becomes easy.
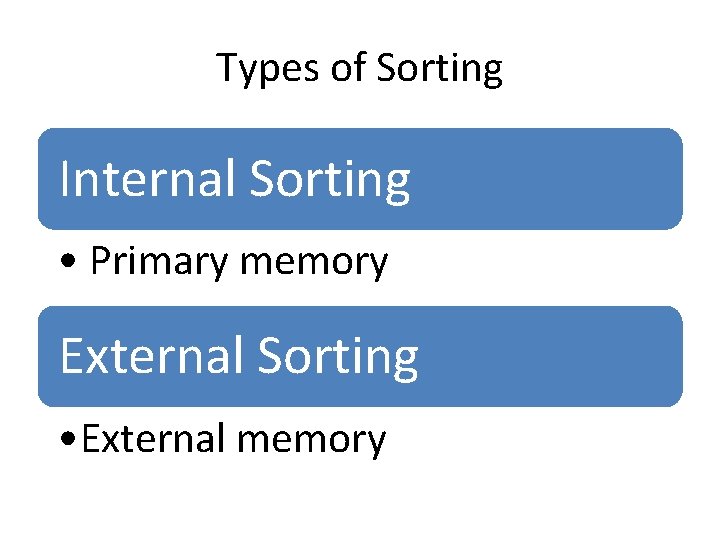
Types of Sorting Internal Sorting • Primary memory External Sorting • External memory
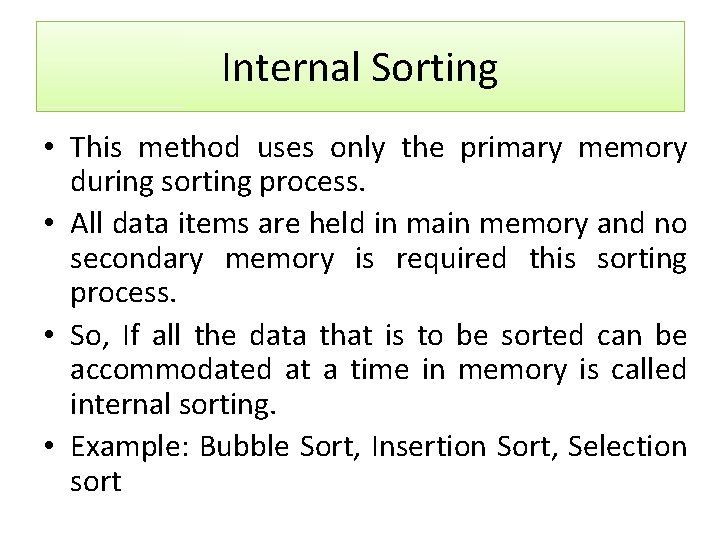
Internal Sorting • This method uses only the primary memory during sorting process. • All data items are held in main memory and no secondary memory is required this sorting process. • So, If all the data that is to be sorted can be accommodated at a time in memory is called internal sorting. • Example: Bubble Sort, Insertion Sort, Selection sort
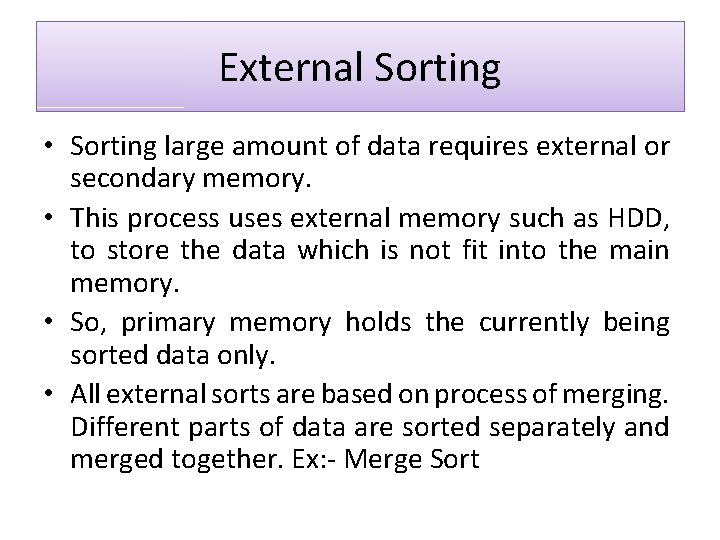
External Sorting • Sorting large amount of data requires external or secondary memory. • This process uses external memory such as HDD, to store the data which is not fit into the main memory. • So, primary memory holds the currently being sorted data only. • All external sorts are based on process of merging. Different parts of data are sorted separately and merged together. Ex: - Merge Sort
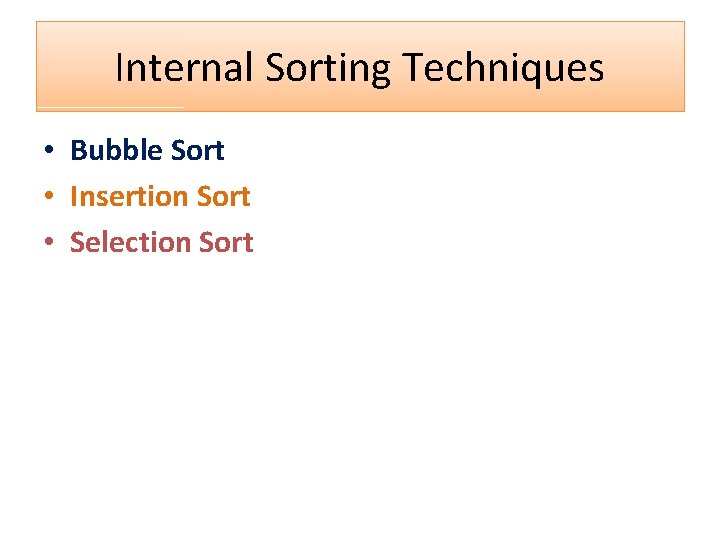
Internal Sorting Techniques • Bubble Sort • Insertion Sort • Selection Sort
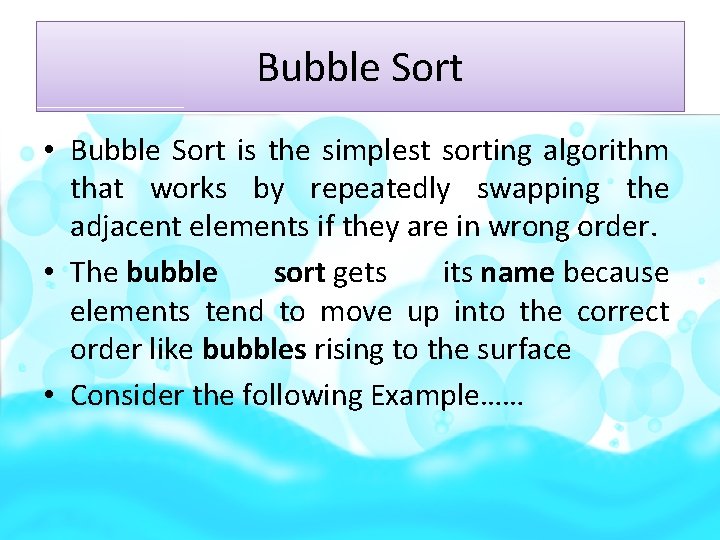
Bubble Sort • Bubble Sort is the simplest sorting algorithm that works by repeatedly swapping the adjacent elements if they are in wrong order. • The bubble sort gets its name because elements tend to move up into the correct order like bubbles rising to the surface • Consider the following Example……
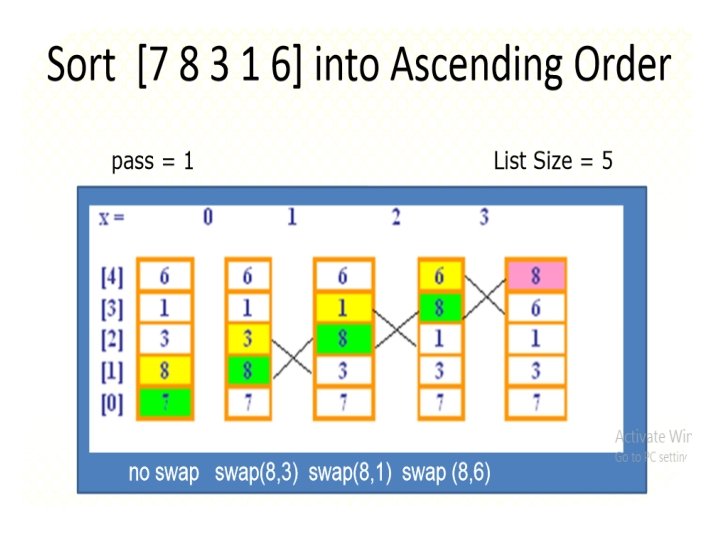
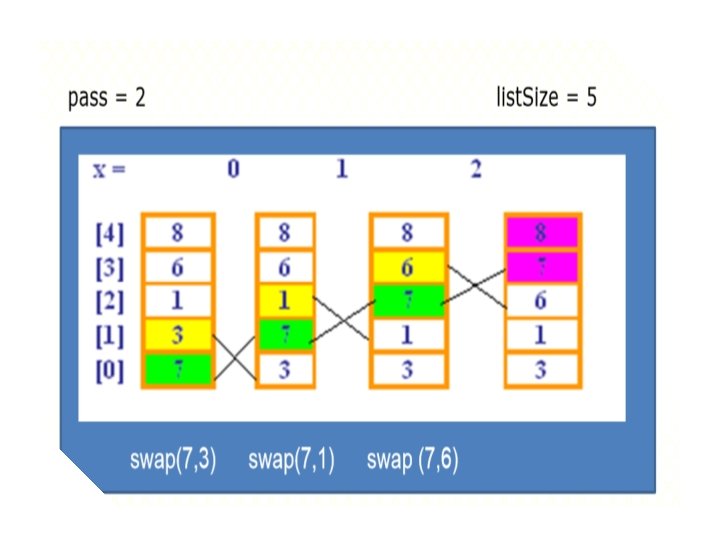
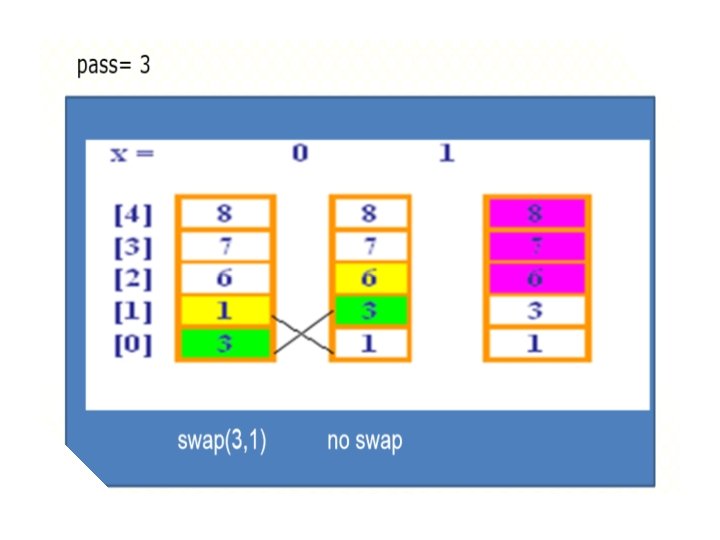
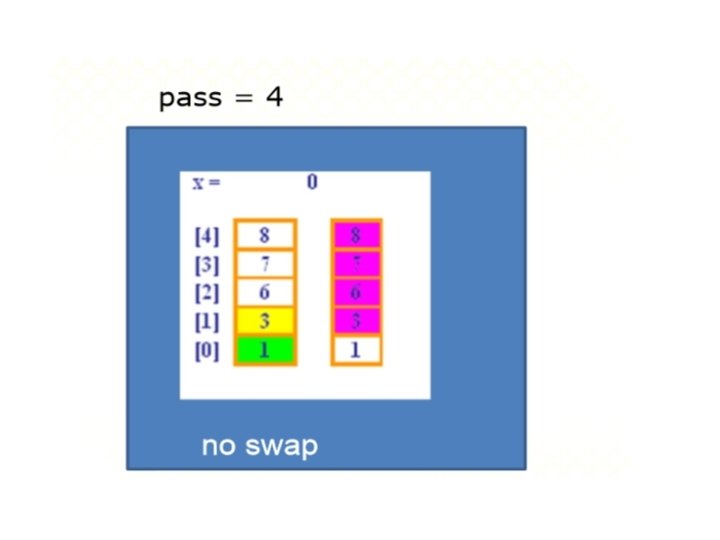
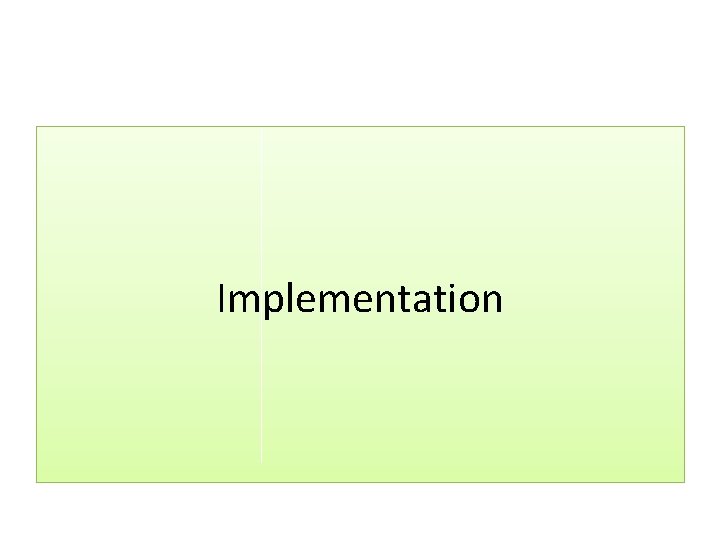
Implementation
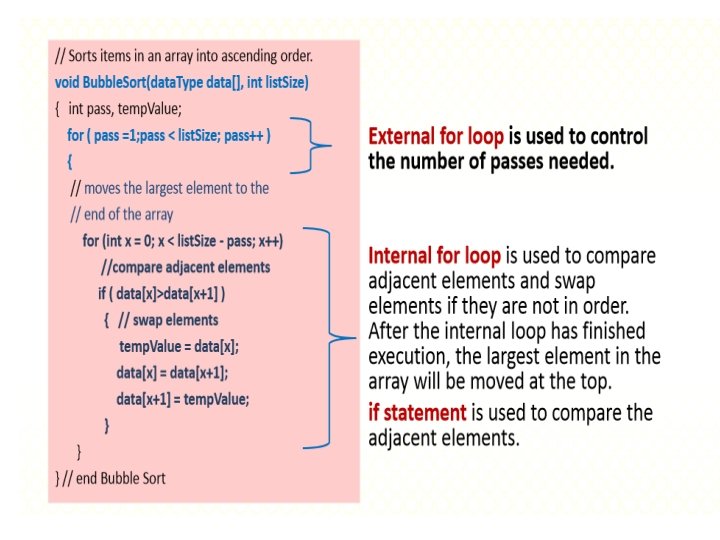
![A function to implement bubble sort void bubble Sortint arr int n A function to implement bubble sort • void bubble. Sort(int arr[], int n) {](https://slidetodoc.com/presentation_image_h2/cb65a621f99cd599681a4afa22726137/image-15.jpg)
A function to implement bubble sort • void bubble. Sort(int arr[], int n) { int i, j; for (i = 0; i < n-1; i++) // external for loop for (j = 0; j < n-i-1; j++) Internal for loop if (arr[j] > arr[j+1]) swap(&arr[j], &arr[j+1]); }
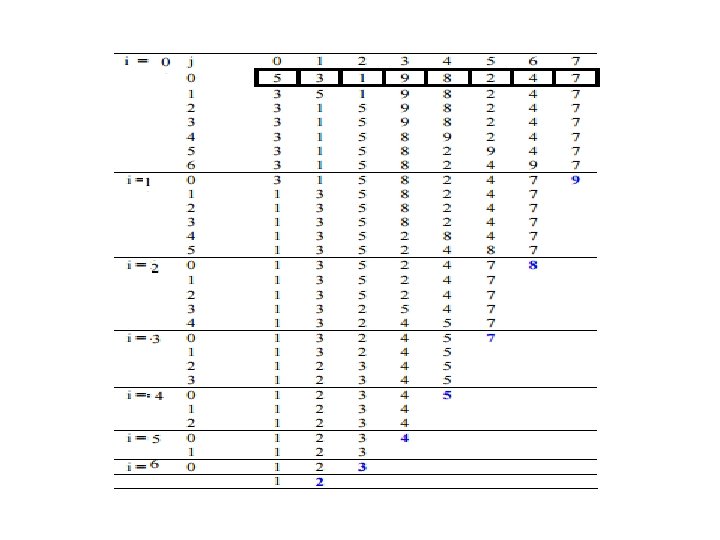
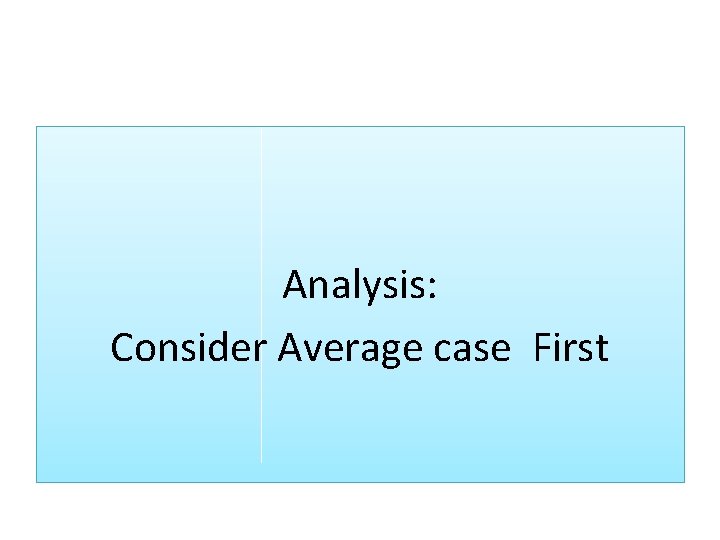
Analysis: Consider Average case First
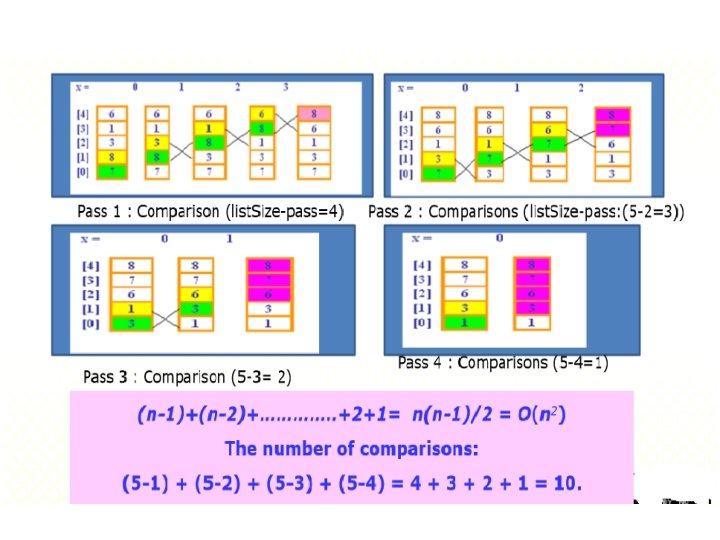
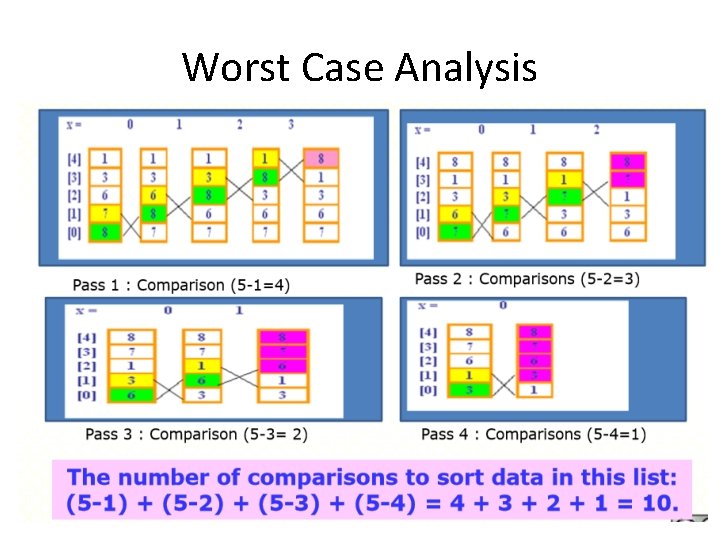
Worst Case Analysis
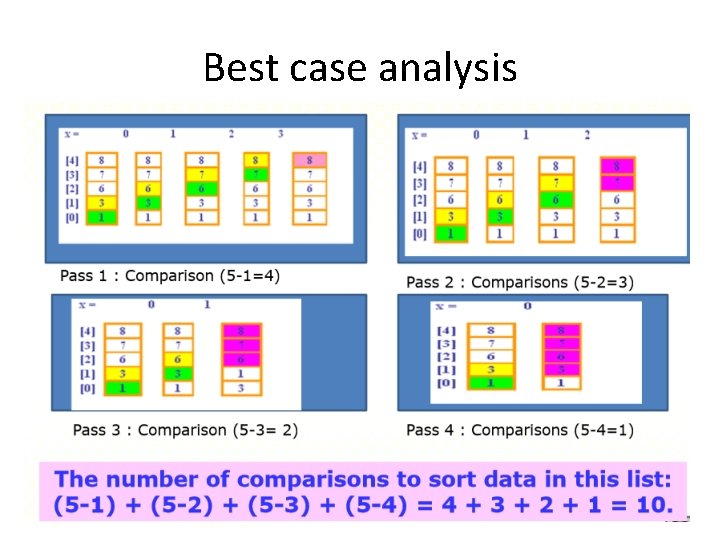
Best case analysis
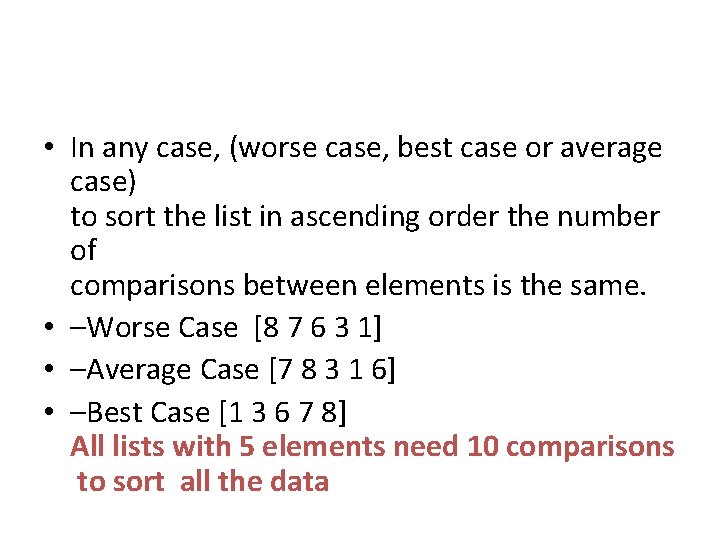
• In any case, (worse case, best case or average case) to sort the list in ascending order the number of comparisons between elements is the same. • –Worse Case [8 7 6 3 1] • –Average Case [7 8 3 1 6] • –Best Case [1 3 6 7 8] All lists with 5 elements need 10 comparisons to sort all the data
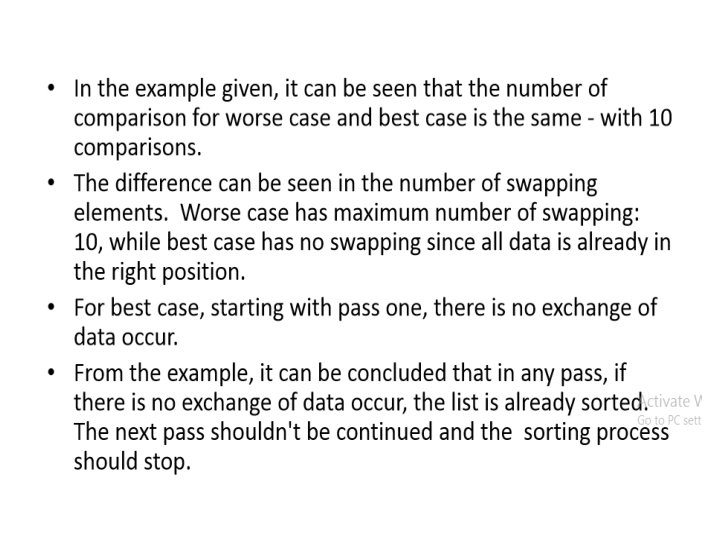
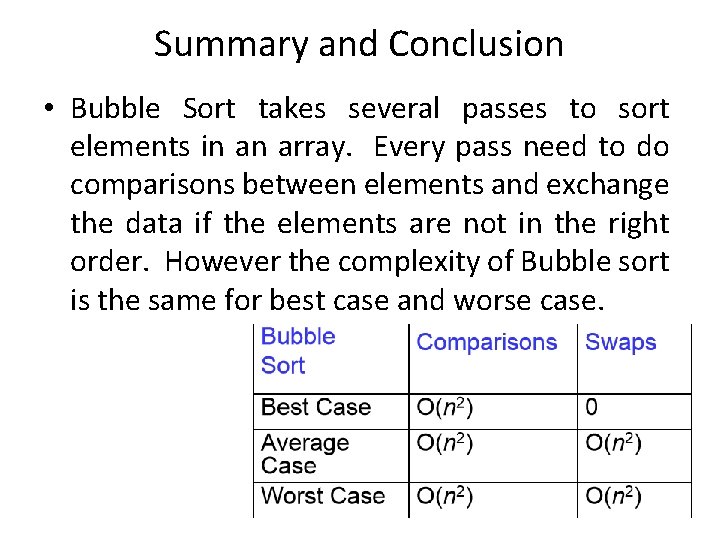
Summary and Conclusion • Bubble Sort takes several passes to sort elements in an array. Every pass need to do comparisons between elements and exchange the data if the elements are not in the right order. However the complexity of Bubble sort is the same for best case and worse case.
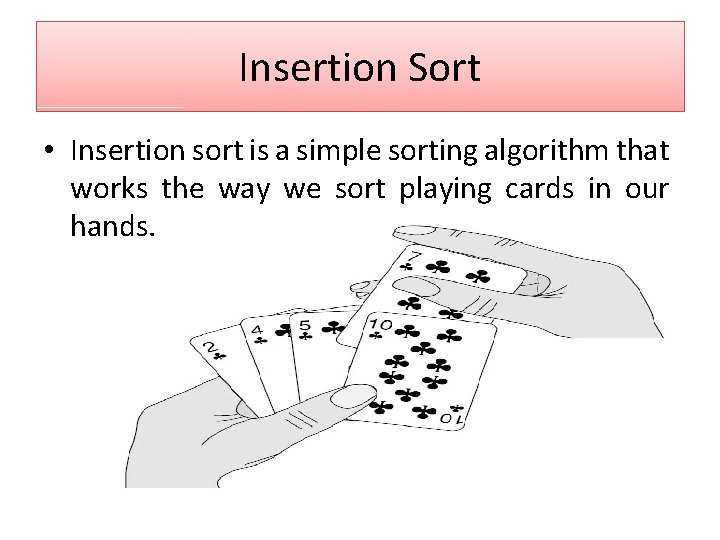
Insertion Sort • Insertion sort is a simple sorting algorithm that works the way we sort playing cards in our hands.
![We start with an empty left hand sorted array and the cards • We start with an empty left hand [sorted array] and the cards](https://slidetodoc.com/presentation_image_h2/cb65a621f99cd599681a4afa22726137/image-25.jpg)
• We start with an empty left hand [sorted array] and the cards face down on the table [unsorted array]. • Then remove one card [key] at a time from the table [unsorted array], and insert it into the correct position in the left hand [sorted array]. • To find the correct position for the card, we compare it with each of the cards already in the hand, from right to left.
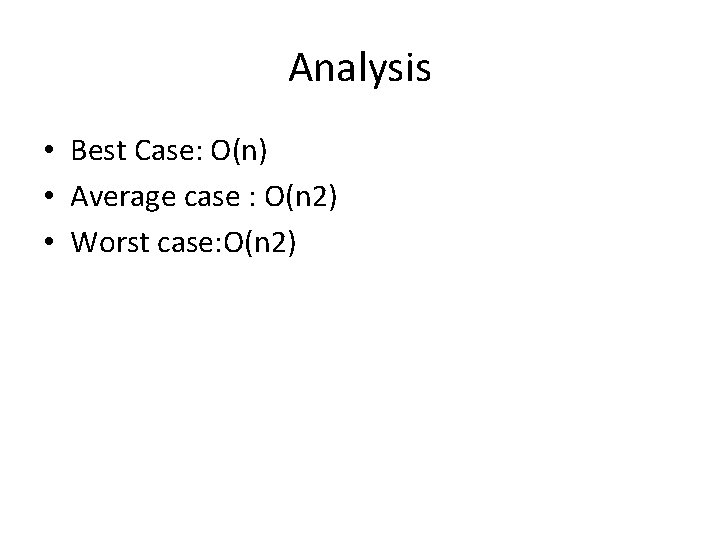
Analysis • Best Case: O(n) • Average case : O(n 2) • Worst case: O(n 2)
What is internal and external sorting
Data refers to
Preparing for a client meeting
Arranging sentences into paragraph
Arrange the following sentence:
Rearrange the following sentences in weren't order
Style occidental
Diploma in funeral arranging and administration
Diploma in funeral arranging and administration
Diploma in funeral arranging and administration
Design is
Spike flower arrangement
Domain kingdom phylum mnemonic
Apa tujuan dari hasil proses pengurutan data
Orale charakterstruktur
Tujuan dari mengurutkan data adalah
Searching dan sorting
Pengurutan data
Data refers to
Data conditioning refers to
A grouping of characters into a word
Lego sorting robot
What is stable sorting
Evaporation mixtures examples
How is chert formed
Principles of mineral processing
Grain size sorting formula