Sorting Techniques Sorting algorithm Sorting refers to arranging
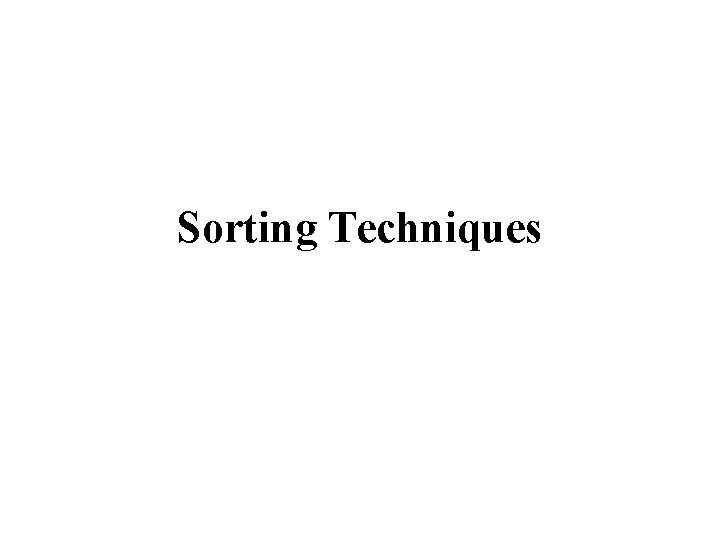
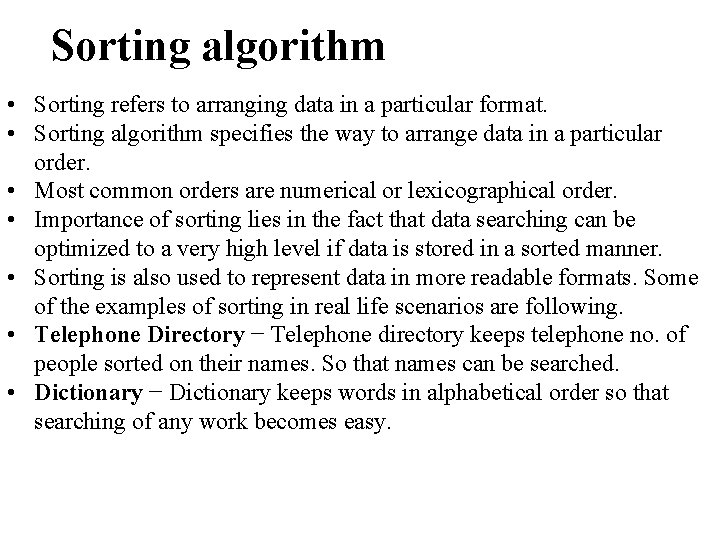
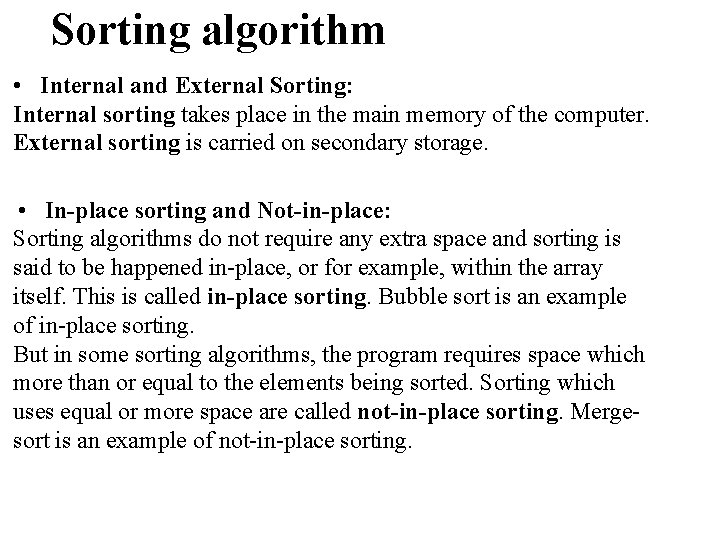
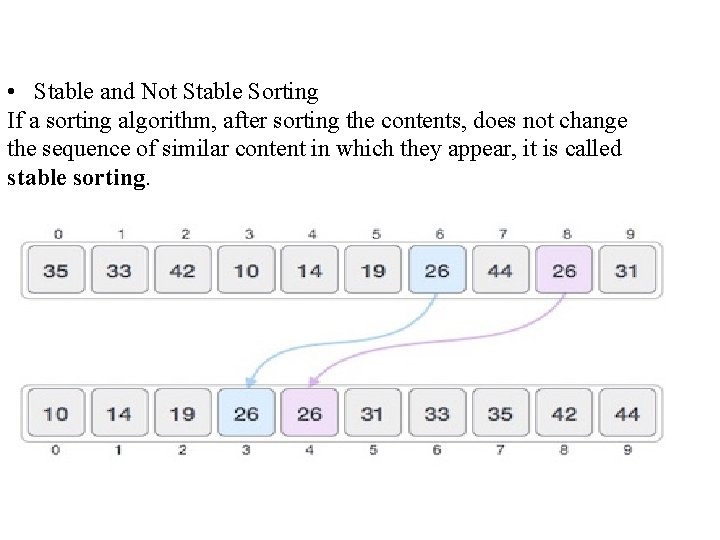
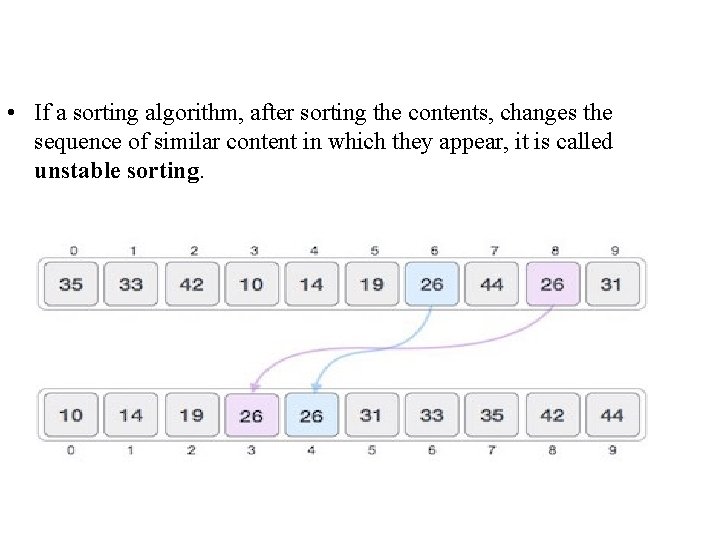
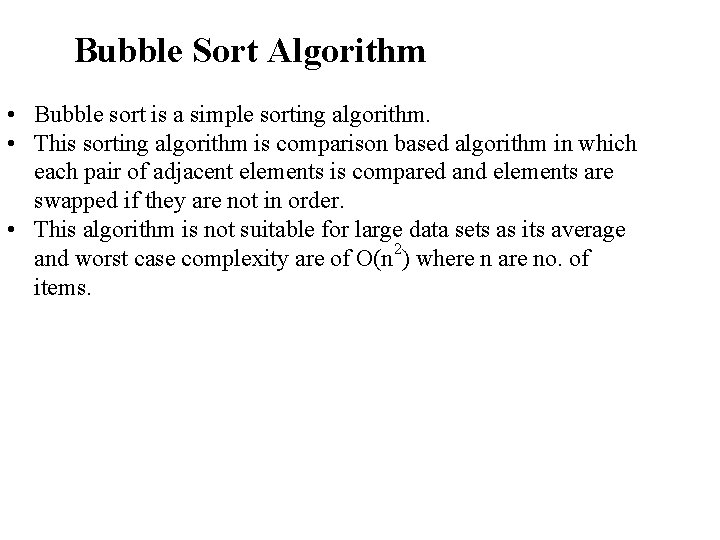
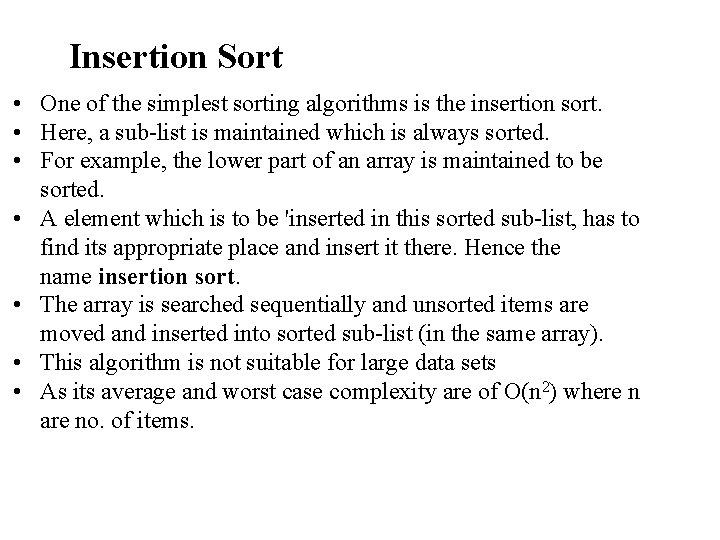
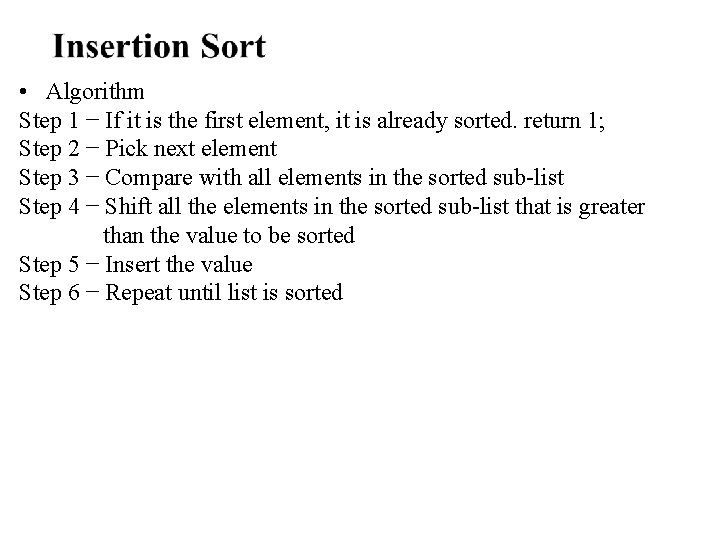
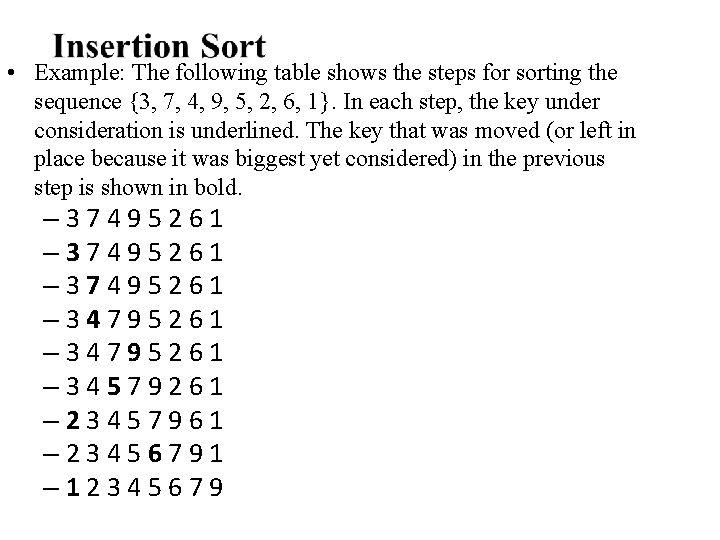
![void insertion_sort( int a[ ], int n ) { int i, j; for (i=1; void insertion_sort( int a[ ], int n ) { int i, j; for (i=1;](https://slidetodoc.com/presentation_image_h2/4e2f988a62f75d764ea7ab27dcef42e3/image-10.jpg)
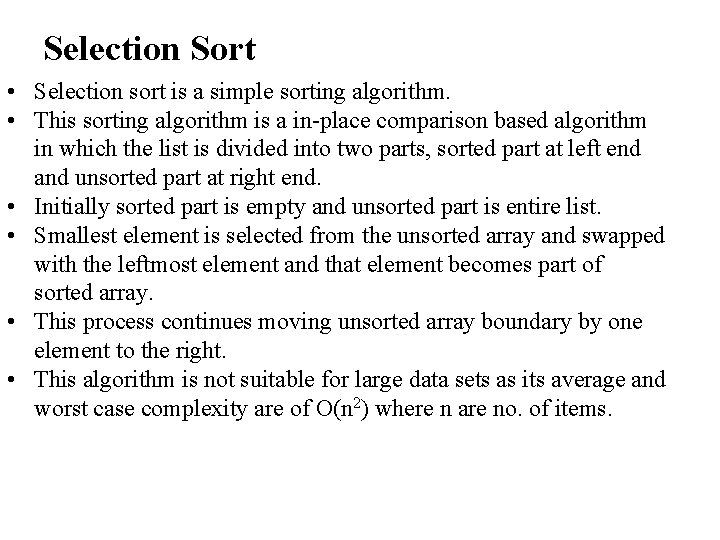
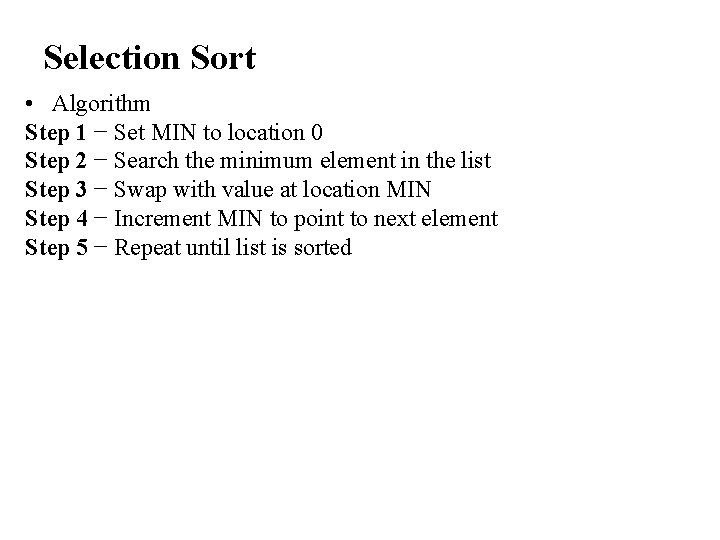
![Selection Sort void selection_sort(int a[], int n) { int i, j, temp; for(i=0; i<n; Selection Sort void selection_sort(int a[], int n) { int i, j, temp; for(i=0; i<n;](https://slidetodoc.com/presentation_image_h2/4e2f988a62f75d764ea7ab27dcef42e3/image-13.jpg)
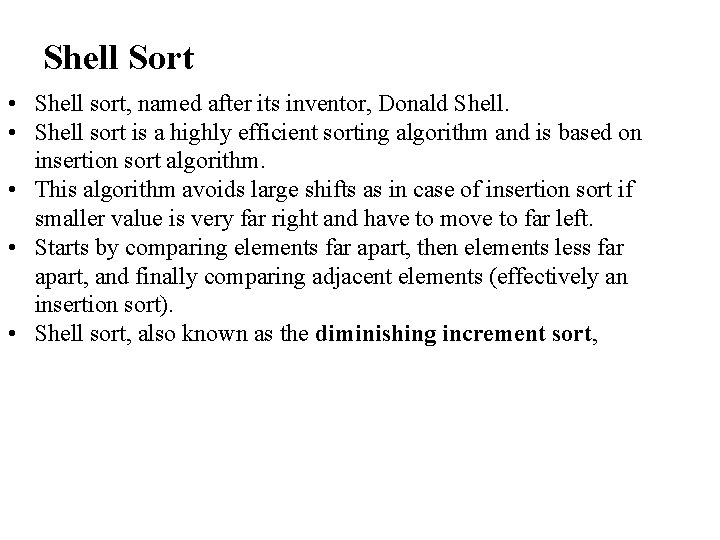
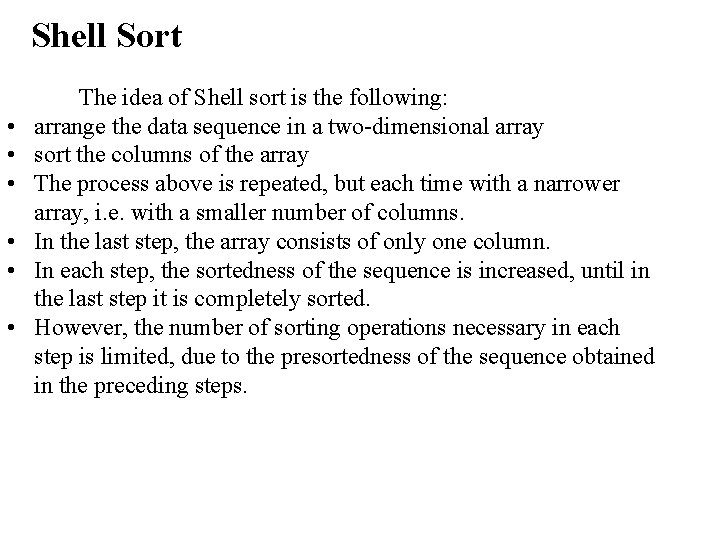
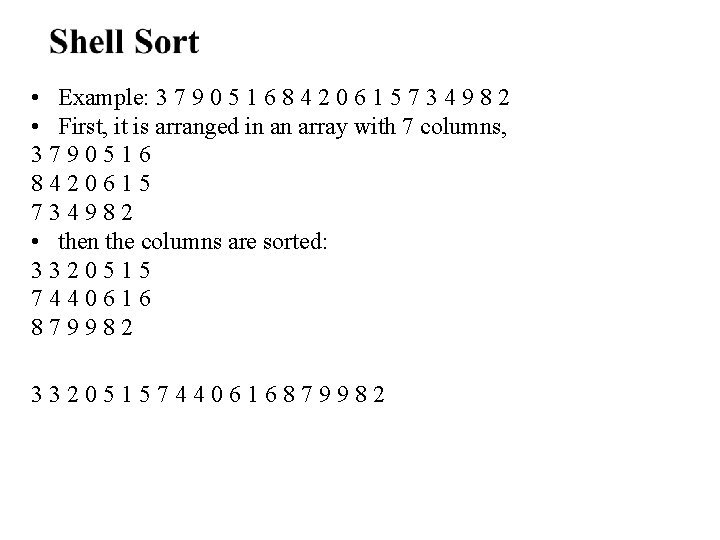
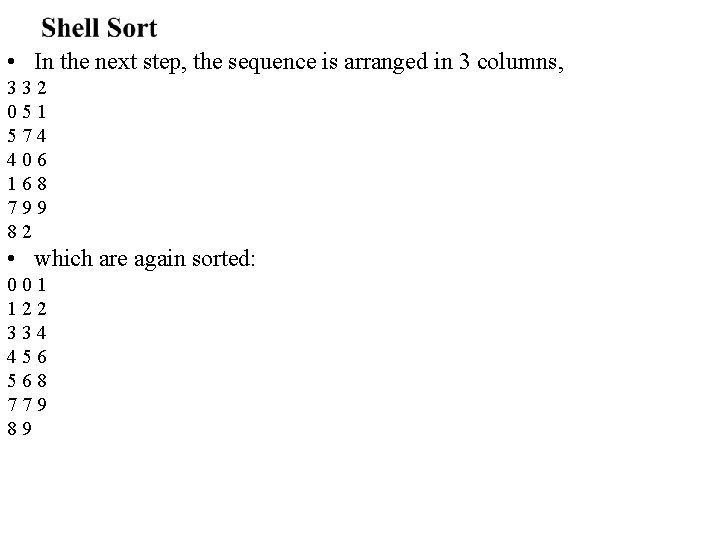
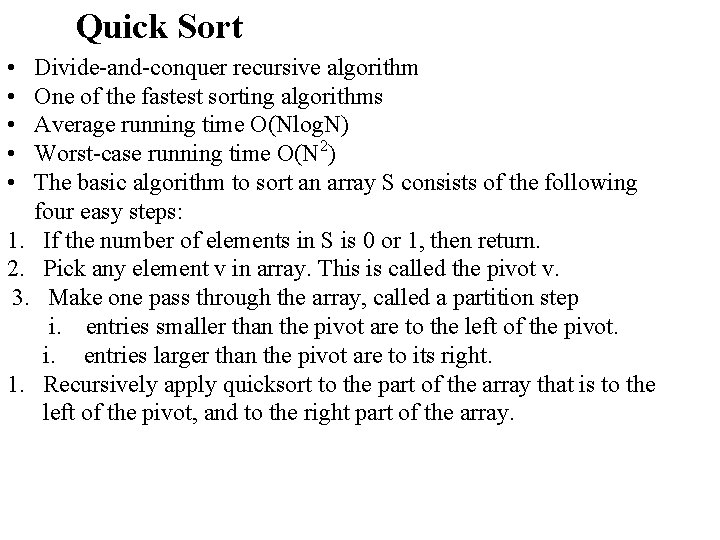
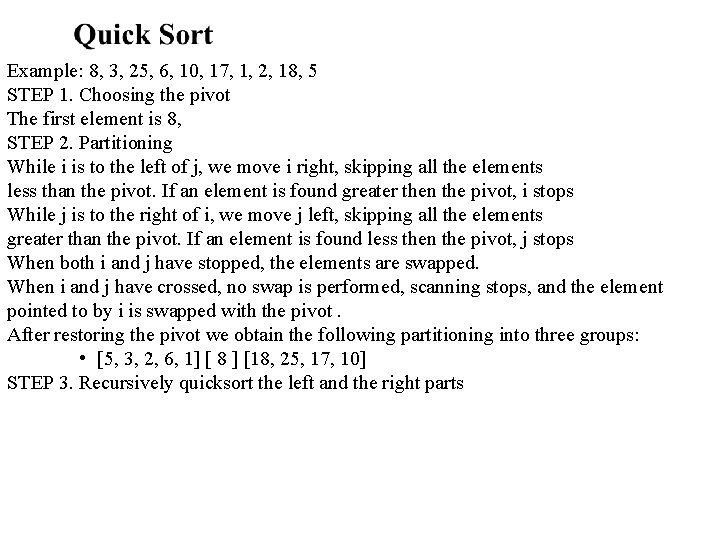
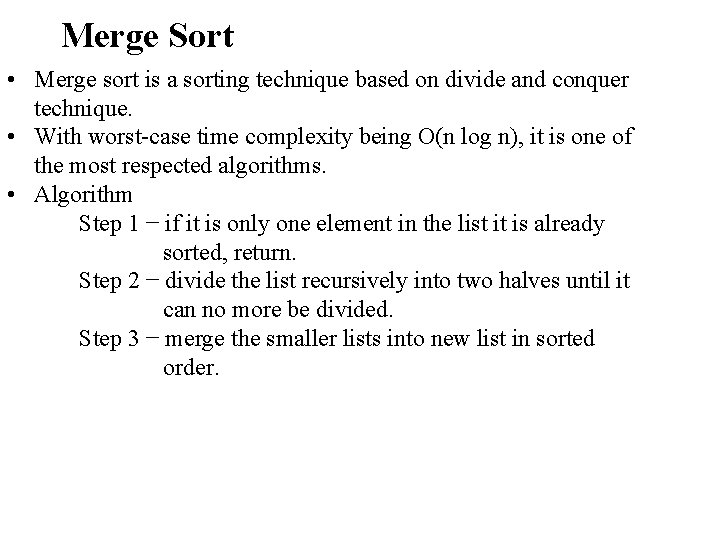
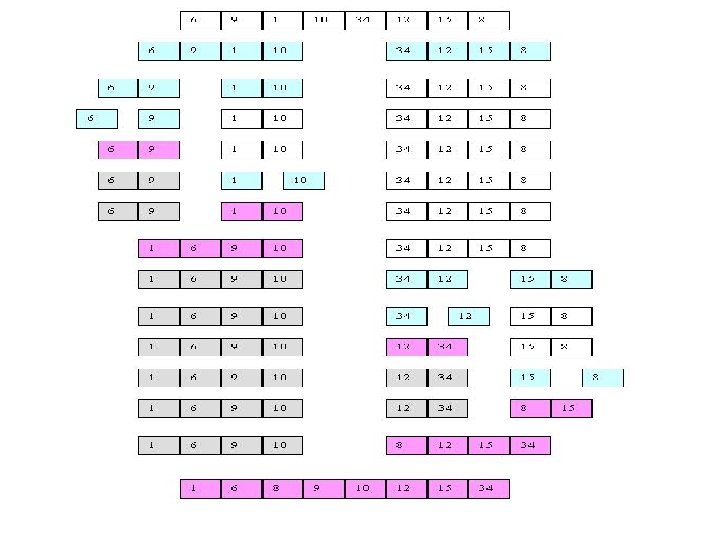
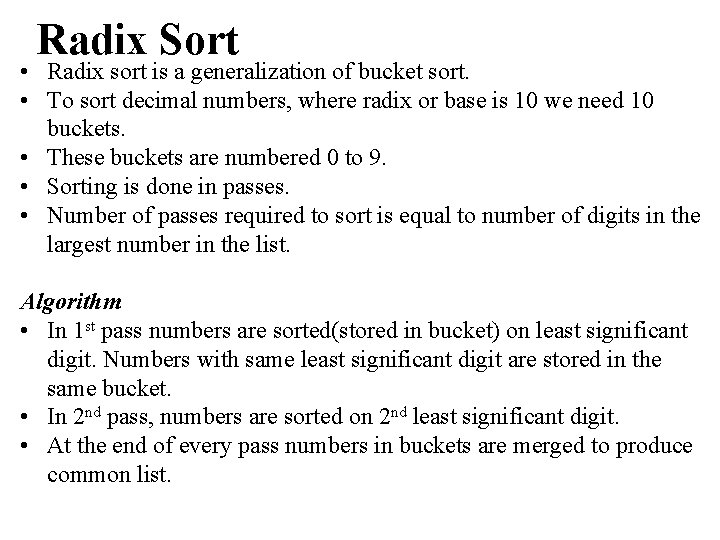
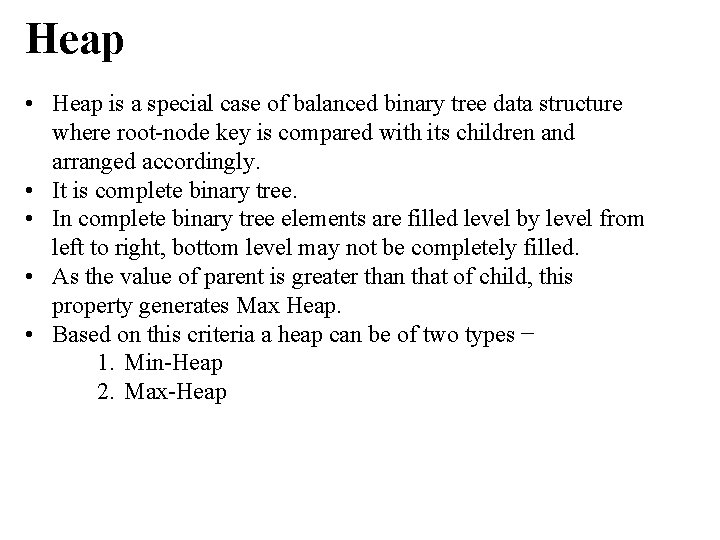
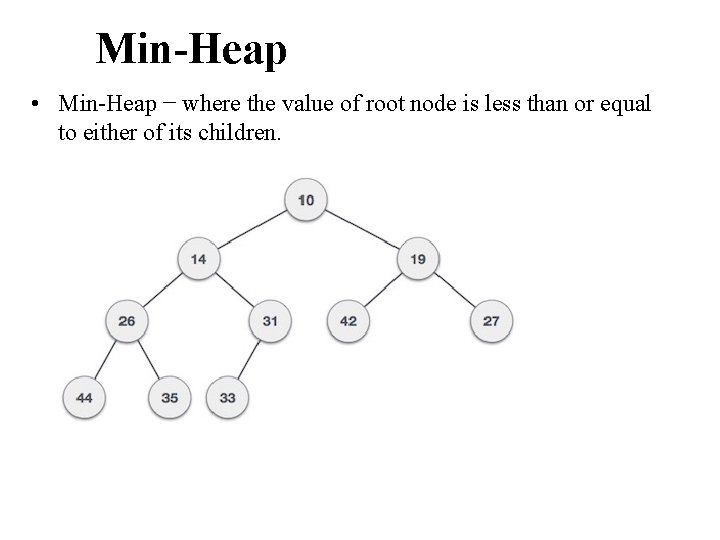
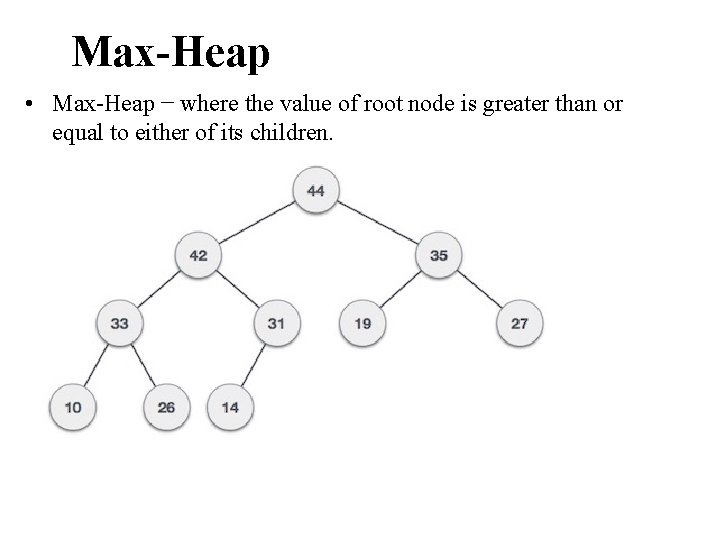
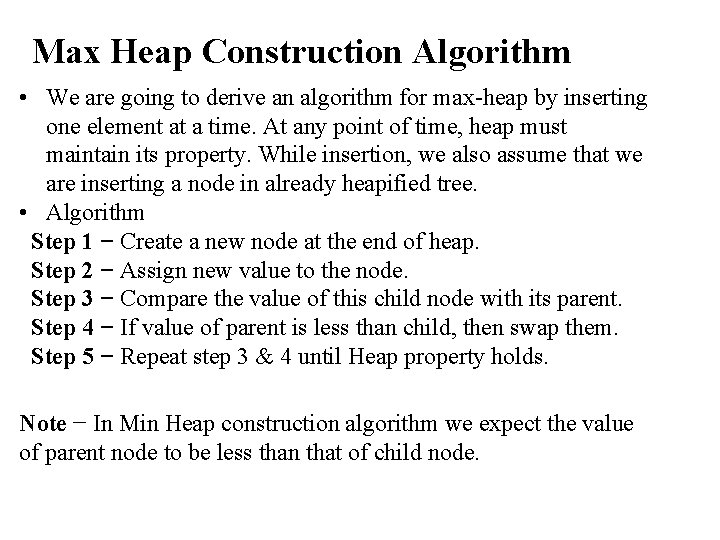
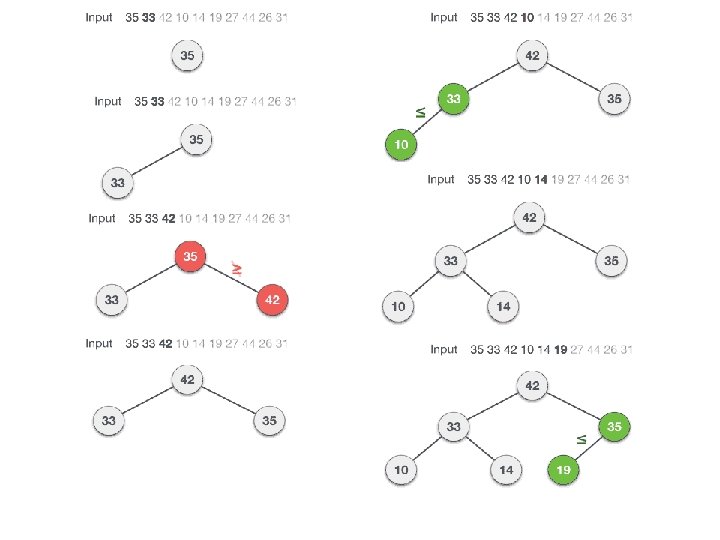
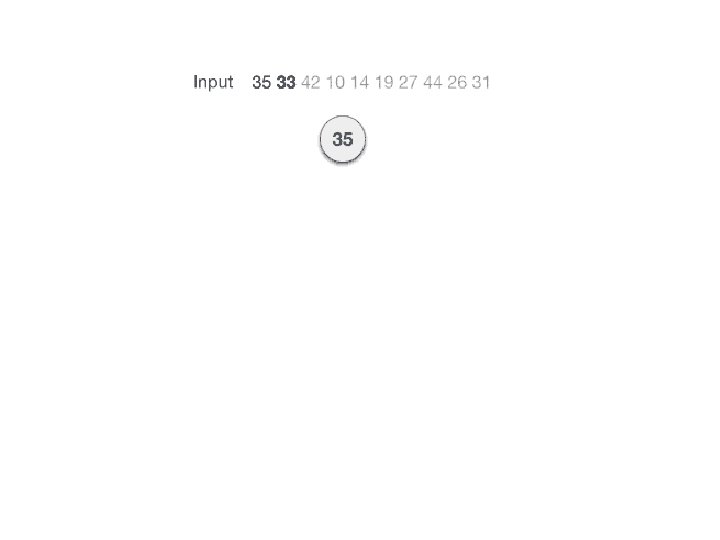
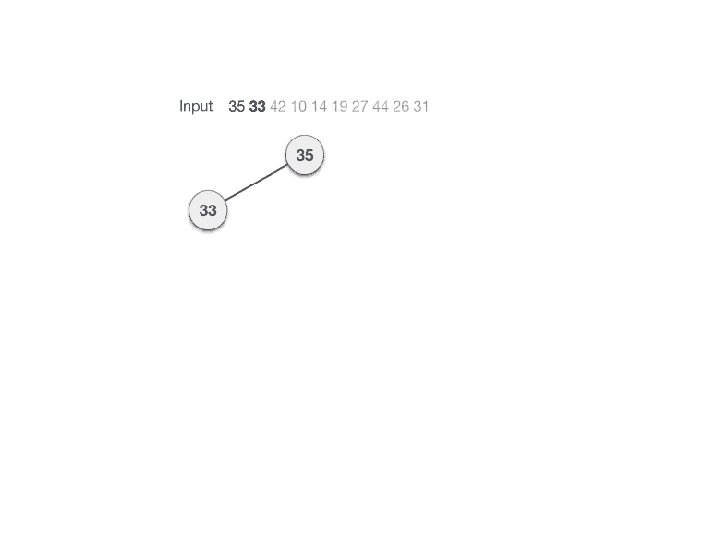
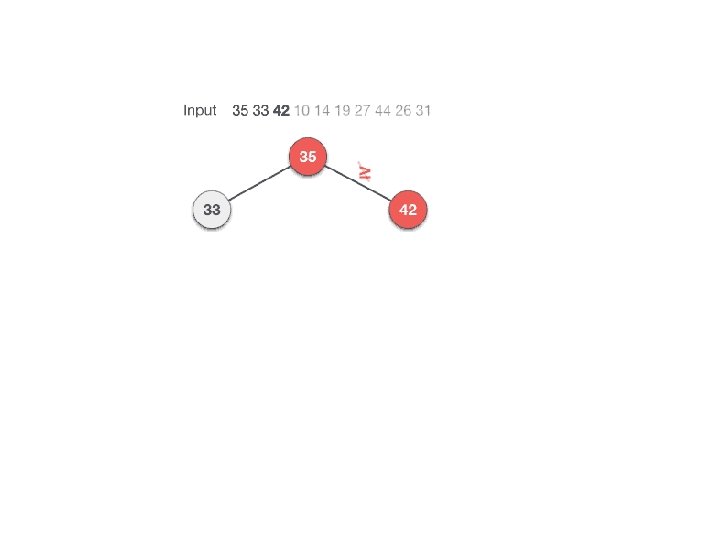
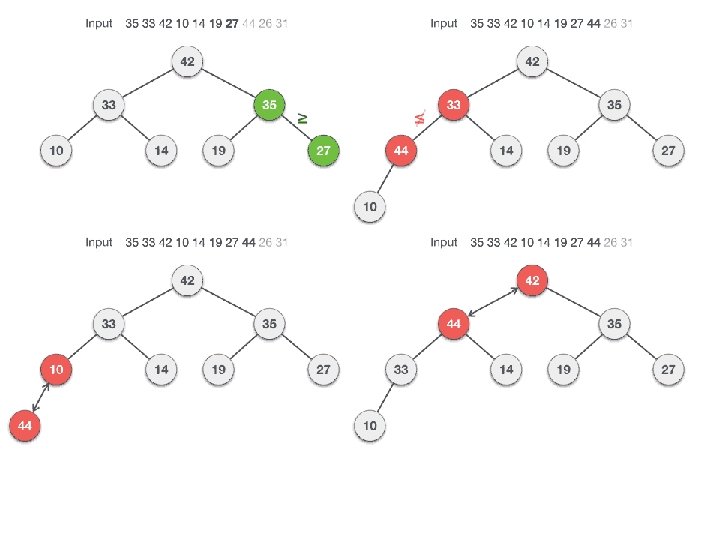
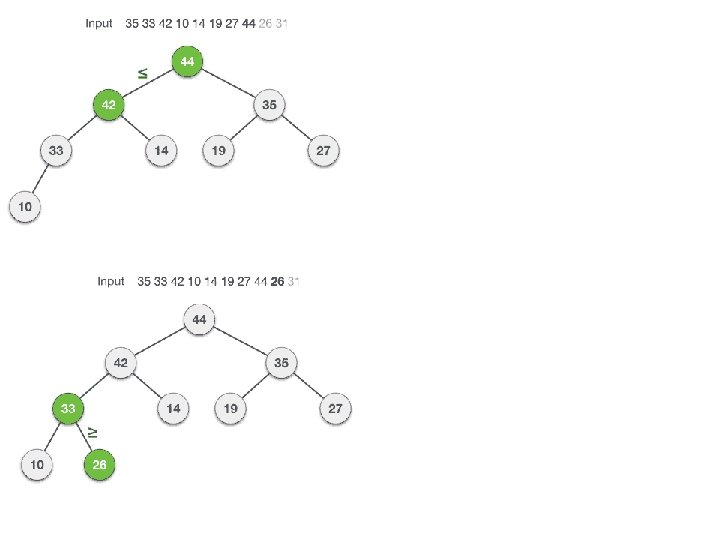
- Slides: 32
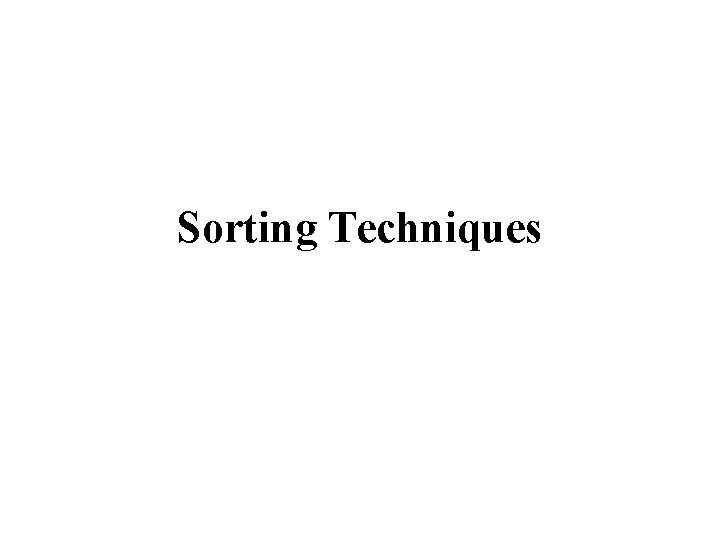
Sorting Techniques
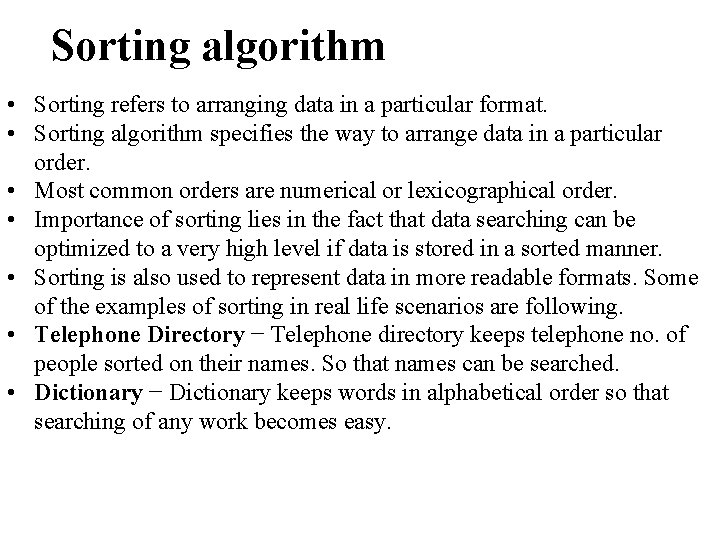
Sorting algorithm • Sorting refers to arranging data in a particular format. • Sorting algorithm specifies the way to arrange data in a particular order. • Most common orders are numerical or lexicographical order. • Importance of sorting lies in the fact that data searching can be optimized to a very high level if data is stored in a sorted manner. • Sorting is also used to represent data in more readable formats. Some of the examples of sorting in real life scenarios are following. • Telephone Directory − Telephone directory keeps telephone no. of people sorted on their names. So that names can be searched. • Dictionary − Dictionary keeps words in alphabetical order so that searching of any work becomes easy.
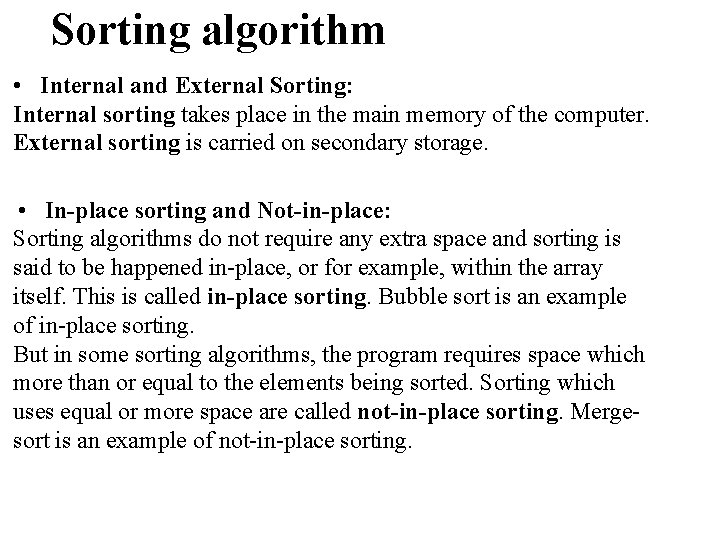
Sorting algorithm • Internal and External Sorting: Internal sorting takes place in the main memory of the computer. External sorting is carried on secondary storage. • In-place sorting and Not-in-place: Sorting algorithms do not require any extra space and sorting is said to be happened in-place, or for example, within the array itself. This is called in-place sorting. Bubble sort is an example of in-place sorting. But in some sorting algorithms, the program requires space which more than or equal to the elements being sorted. Sorting which uses equal or more space are called not-in-place sorting. Mergesort is an example of not-in-place sorting.
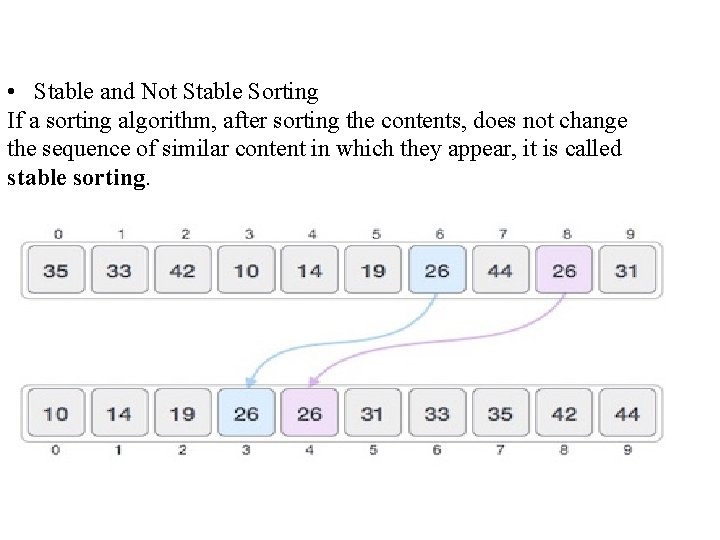
• Stable and Not Stable Sorting If a sorting algorithm, after sorting the contents, does not change the sequence of similar content in which they appear, it is called stable sorting.
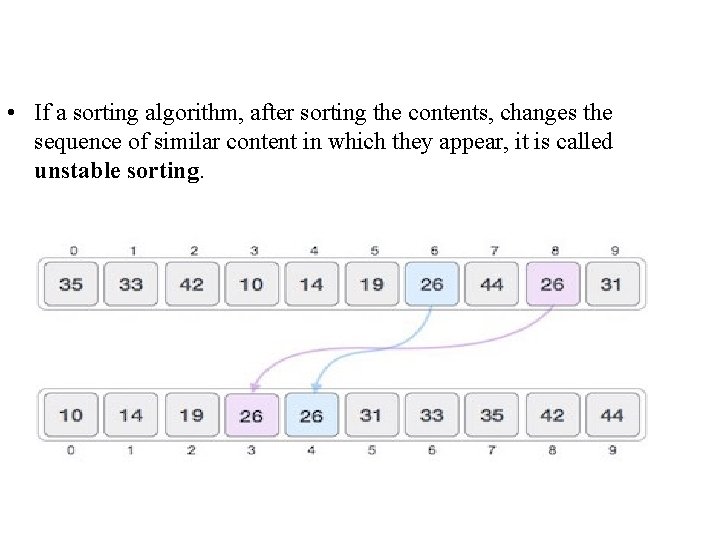
• If a sorting algorithm, after sorting the contents, changes the sequence of similar content in which they appear, it is called unstable sorting.
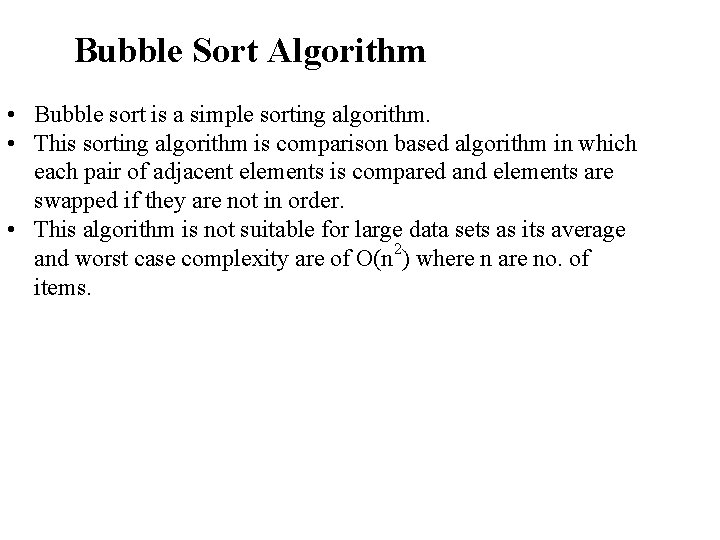
Bubble Sort Algorithm • Bubble sort is a simple sorting algorithm. • This sorting algorithm is comparison based algorithm in which each pair of adjacent elements is compared and elements are swapped if they are not in order. • This algorithm is not suitable for large data sets as its average and worst case complexity are of O(n 2) where n are no. of items.
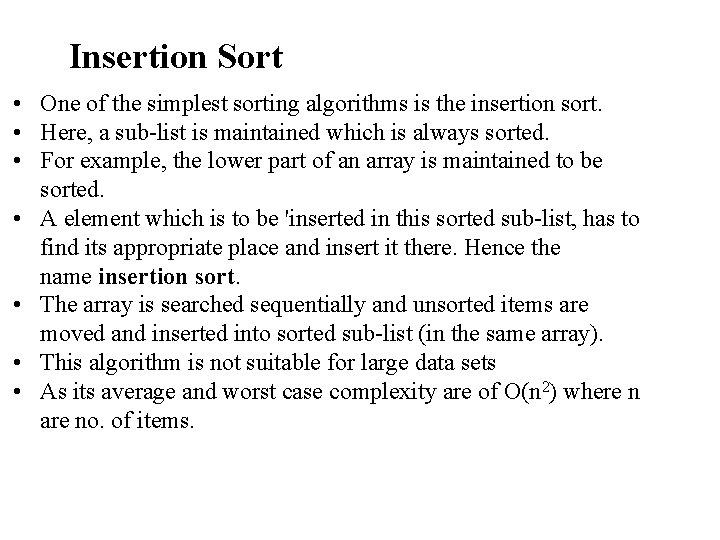
Insertion Sort • One of the simplest sorting algorithms is the insertion sort. • Here, a sub-list is maintained which is always sorted. • For example, the lower part of an array is maintained to be sorted. • A element which is to be 'inserted in this sorted sub-list, has to find its appropriate place and insert it there. Hence the name insertion sort. • The array is searched sequentially and unsorted items are moved and inserted into sorted sub-list (in the same array). • This algorithm is not suitable for large data sets • As its average and worst case complexity are of Ο(n 2) where n are no. of items.
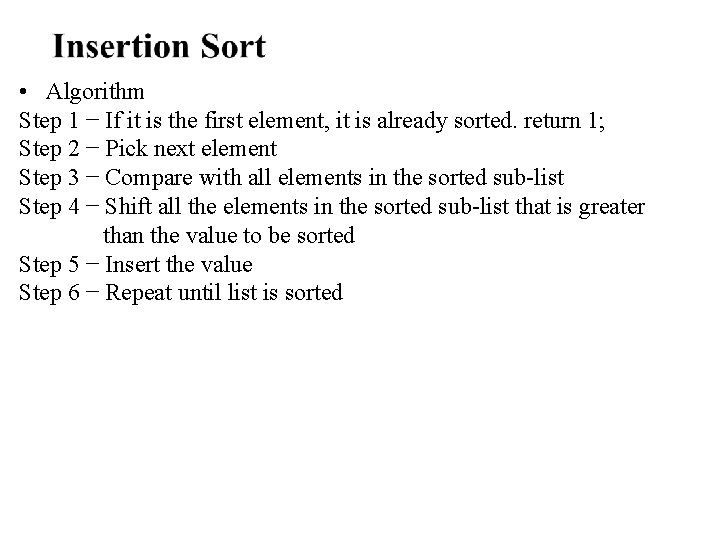
• Algorithm Step 1 − If it is the first element, it is already sorted. return 1; Step 2 − Pick next element Step 3 − Compare with all elements in the sorted sub-list Step 4 − Shift all the elements in the sorted sub-list that is greater than the value to be sorted Step 5 − Insert the value Step 6 − Repeat until list is sorted
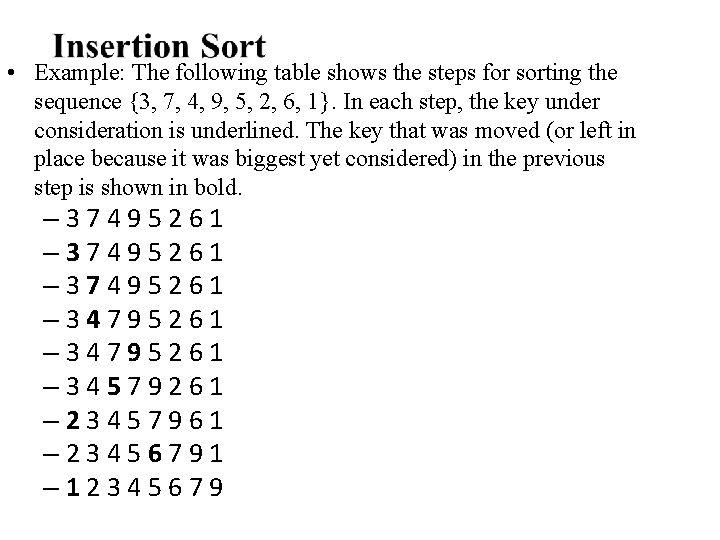
• Example: The following table shows the steps for sorting the sequence {3, 7, 4, 9, 5, 2, 6, 1}. In each step, the key under consideration is underlined. The key that was moved (or left in place because it was biggest yet considered) in the previous step is shown in bold. – 37495261 – 34795261 – 34579261 – 23457961 – 23456791 – 12345679
![void insertionsort int a int n int i j for i1 void insertion_sort( int a[ ], int n ) { int i, j; for (i=1;](https://slidetodoc.com/presentation_image_h2/4e2f988a62f75d764ea7ab27dcef42e3/image-10.jpg)
void insertion_sort( int a[ ], int n ) { int i, j; for (i=1; i<n; i++) { x = a[i]; j = i – 1; while ( j >= 0 && a[j] > x ) { a[j+1] = a[j]; j=j - 1 ; } a[j+1] = x; }
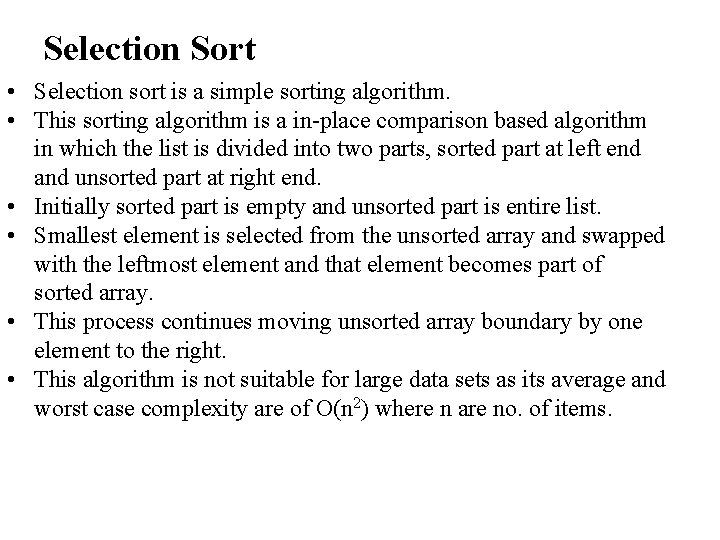
Selection Sort • Selection sort is a simple sorting algorithm. • This sorting algorithm is a in-place comparison based algorithm in which the list is divided into two parts, sorted part at left end and unsorted part at right end. • Initially sorted part is empty and unsorted part is entire list. • Smallest element is selected from the unsorted array and swapped with the leftmost element and that element becomes part of sorted array. • This process continues moving unsorted array boundary by one element to the right. • This algorithm is not suitable for large data sets as its average and worst case complexity are of O(n 2) where n are no. of items.
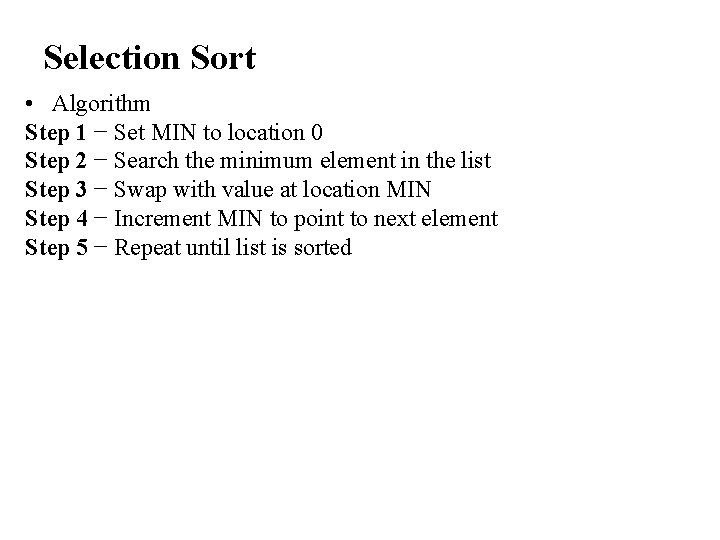
Selection Sort • Algorithm Step 1 − Set MIN to location 0 Step 2 − Search the minimum element in the list Step 3 − Swap with value at location MIN Step 4 − Increment MIN to point to next element Step 5 − Repeat until list is sorted
![Selection Sort void selectionsortint a int n int i j temp fori0 in Selection Sort void selection_sort(int a[], int n) { int i, j, temp; for(i=0; i<n;](https://slidetodoc.com/presentation_image_h2/4e2f988a62f75d764ea7ab27dcef42e3/image-13.jpg)
Selection Sort void selection_sort(int a[], int n) { int i, j, temp; for(i=0; i<n; i++) { for(j=i+1; j<n; j++) { if(a[i]>a[j]) { temp=a[i]; a[i]=a[j]; a[j]=temp; } }
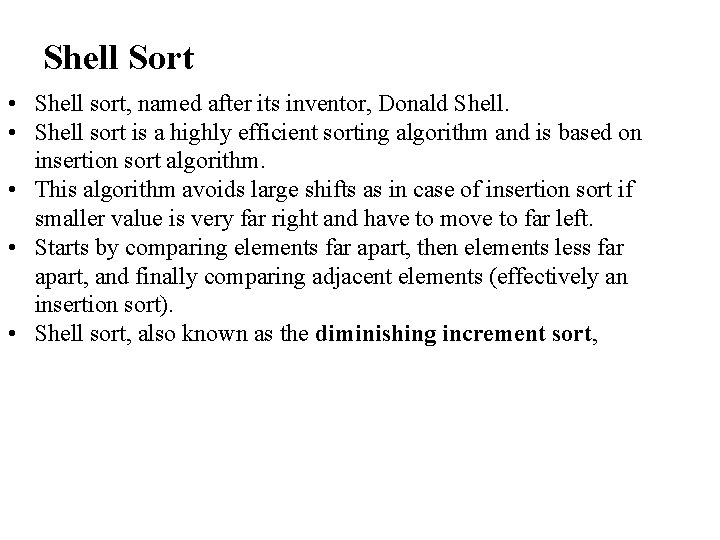
Shell Sort • Shell sort, named after its inventor, Donald Shell. • Shell sort is a highly efficient sorting algorithm and is based on insertion sort algorithm. • This algorithm avoids large shifts as in case of insertion sort if smaller value is very far right and have to move to far left. • Starts by comparing elements far apart, then elements less far apart, and finally comparing adjacent elements (effectively an insertion sort). • Shell sort, also known as the diminishing increment sort,
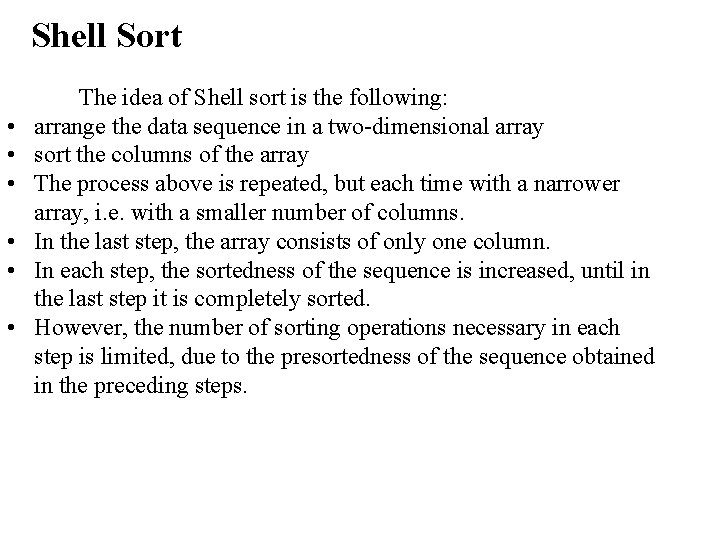
Shell Sort • • • The idea of Shell sort is the following: arrange the data sequence in a two-dimensional array sort the columns of the array The process above is repeated, but each time with a narrower array, i. e. with a smaller number of columns. In the last step, the array consists of only one column. In each step, the sortedness of the sequence is increased, until in the last step it is completely sorted. However, the number of sorting operations necessary in each step is limited, due to the presortedness of the sequence obtained in the preceding steps.
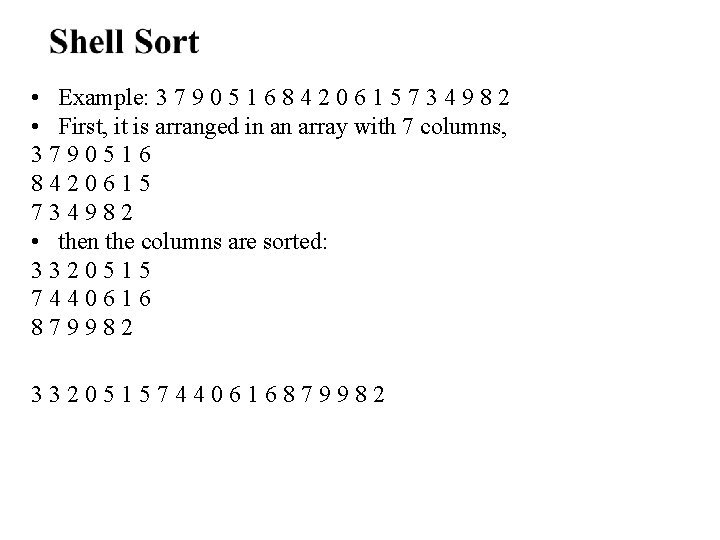
• Example: 3 7 9 0 5 1 6 8 4 2 0 6 1 5 7 3 4 9 8 2 • First, it is arranged in an array with 7 columns, 3790516 8420615 734982 • then the columns are sorted: 3320515 7440616 879982 33205157440616879982
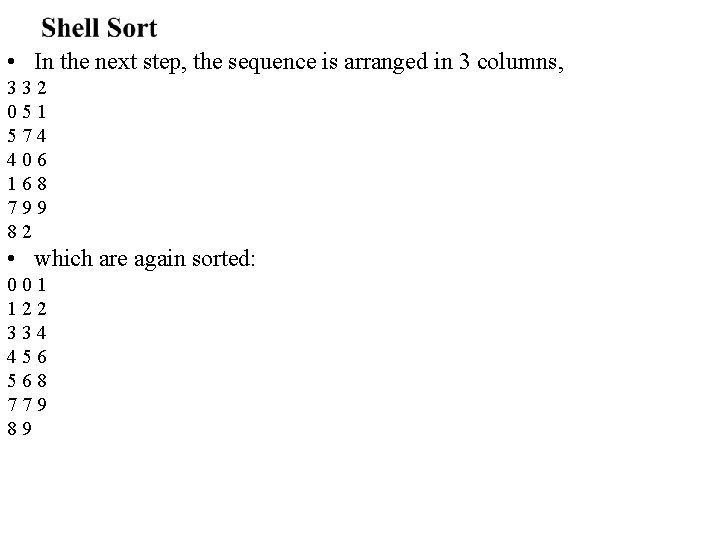
• In the next step, the sequence is arranged in 3 columns, 332 051 574 406 168 799 82 • which are again sorted: 001 122 334 456 568 779 89
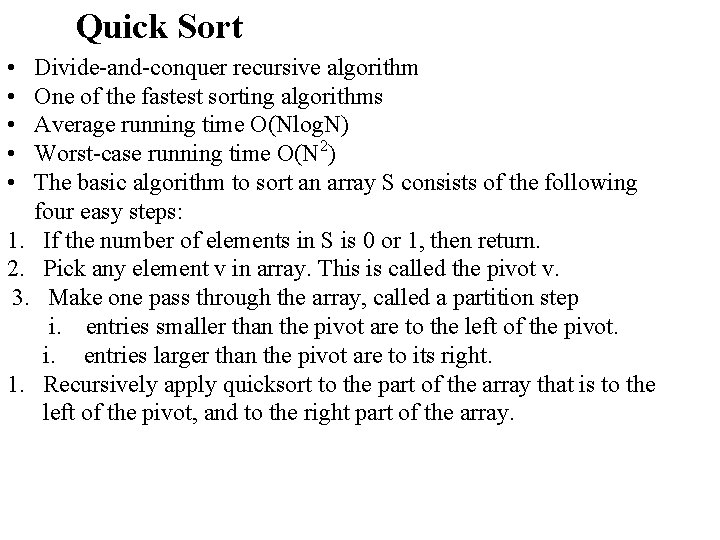
Quick Sort • • • Divide-and-conquer recursive algorithm One of the fastest sorting algorithms Average running time O(Nlog. N) Worst-case running time O(N 2) The basic algorithm to sort an array S consists of the following four easy steps: 1. If the number of elements in S is 0 or 1, then return. 2. Pick any element v in array. This is called the pivot v. 3. Make one pass through the array, called a partition step i. entries smaller than the pivot are to the left of the pivot. i. entries larger than the pivot are to its right. 1. Recursively apply quicksort to the part of the array that is to the left of the pivot, and to the right part of the array.
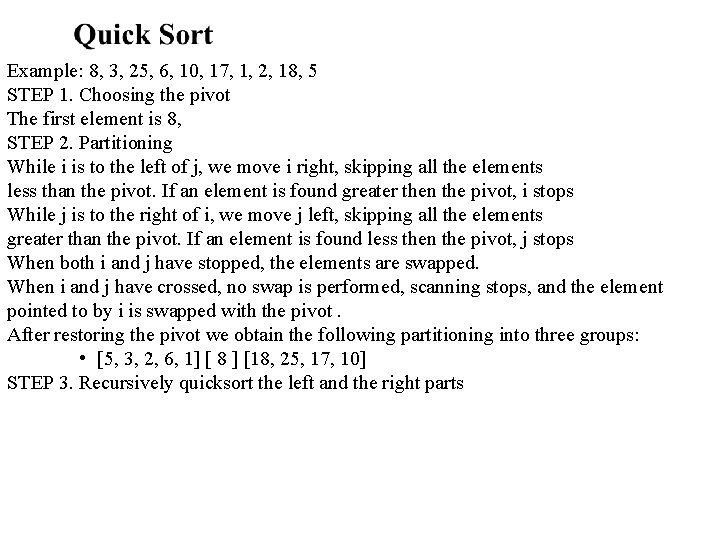
Example: 8, 3, 25, 6, 10, 17, 1, 2, 18, 5 STEP 1. Choosing the pivot The first element is 8, STEP 2. Partitioning While i is to the left of j, we move i right, skipping all the elements less than the pivot. If an element is found greater then the pivot, i stops While j is to the right of i, we move j left, skipping all the elements greater than the pivot. If an element is found less then the pivot, j stops When both i and j have stopped, the elements are swapped. When i and j have crossed, no swap is performed, scanning stops, and the element pointed to by i is swapped with the pivot. After restoring the pivot we obtain the following partitioning into three groups: • [5, 3, 2, 6, 1] [ 8 ] [18, 25, 17, 10] STEP 3. Recursively quicksort the left and the right parts
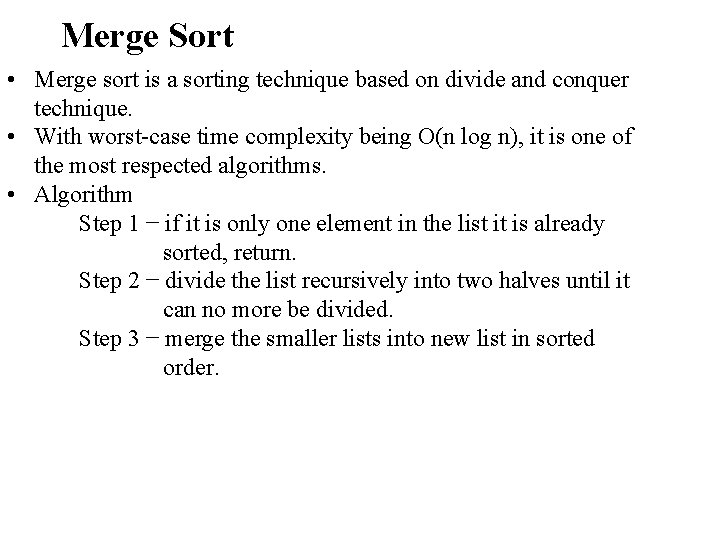
Merge Sort • Merge sort is a sorting technique based on divide and conquer technique. • With worst-case time complexity being Ο(n log n), it is one of the most respected algorithms. • Algorithm Step 1 − if it is only one element in the list it is already sorted, return. Step 2 − divide the list recursively into two halves until it can no more be divided. Step 3 − merge the smaller lists into new list in sorted order.
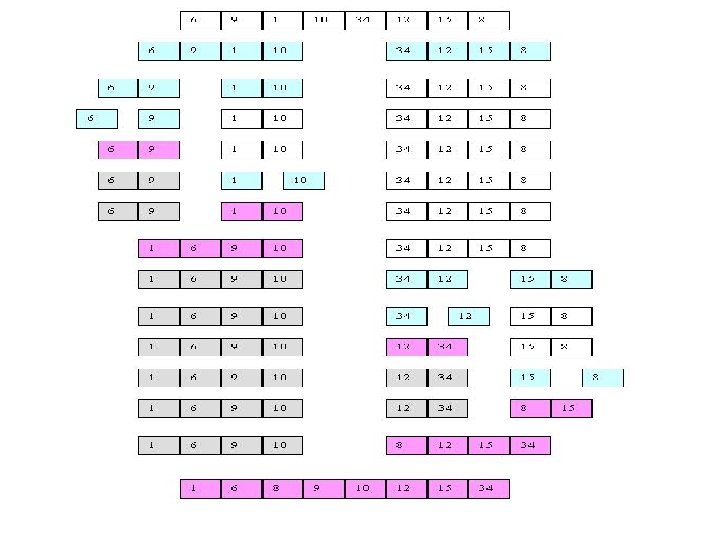
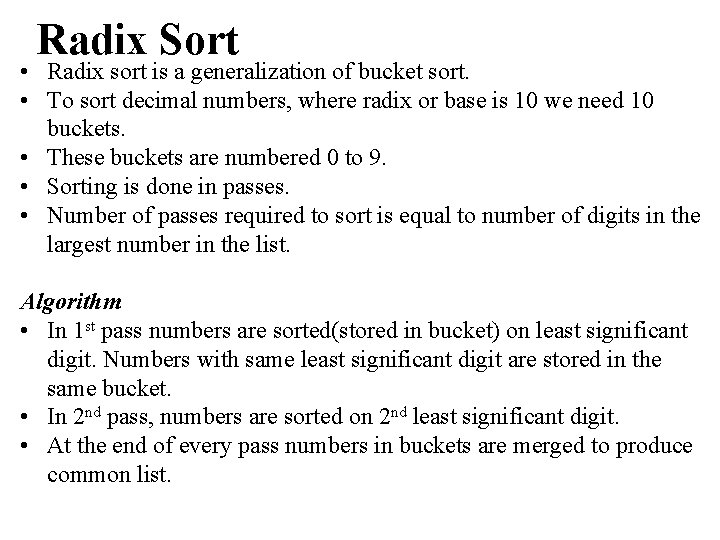
Radix Sort • Radix sort is a generalization of bucket sort. • To sort decimal numbers, where radix or base is 10 we need 10 buckets. • These buckets are numbered 0 to 9. • Sorting is done in passes. • Number of passes required to sort is equal to number of digits in the largest number in the list. Algorithm • In 1 st pass numbers are sorted(stored in bucket) on least significant digit. Numbers with same least significant digit are stored in the same bucket. • In 2 nd pass, numbers are sorted on 2 nd least significant digit. • At the end of every pass numbers in buckets are merged to produce common list.
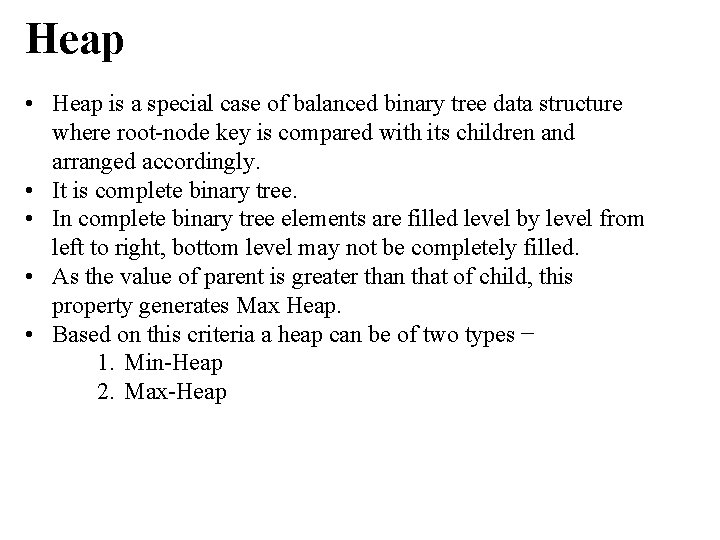
Heap • Heap is a special case of balanced binary tree data structure where root-node key is compared with its children and arranged accordingly. • It is complete binary tree. • In complete binary tree elements are filled level by level from left to right, bottom level may not be completely filled. • As the value of parent is greater than that of child, this property generates Max Heap. • Based on this criteria a heap can be of two types − 1. Min-Heap 2. Max-Heap
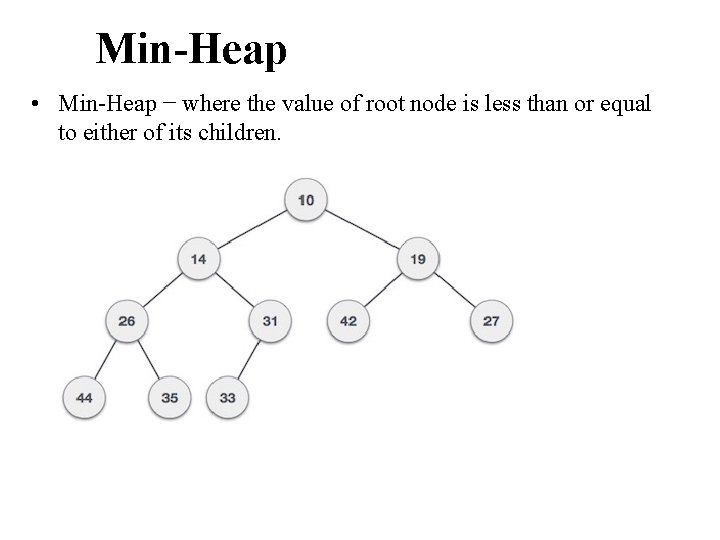
Min-Heap • Min-Heap − where the value of root node is less than or equal to either of its children.
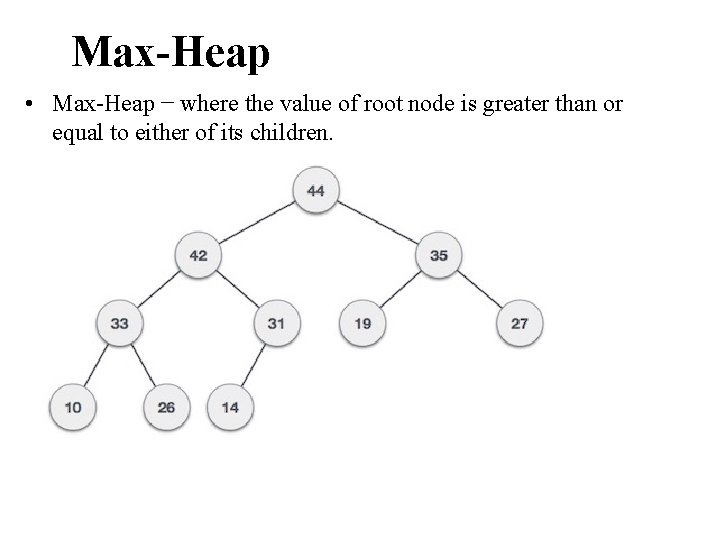
Max-Heap • Max-Heap − where the value of root node is greater than or equal to either of its children.
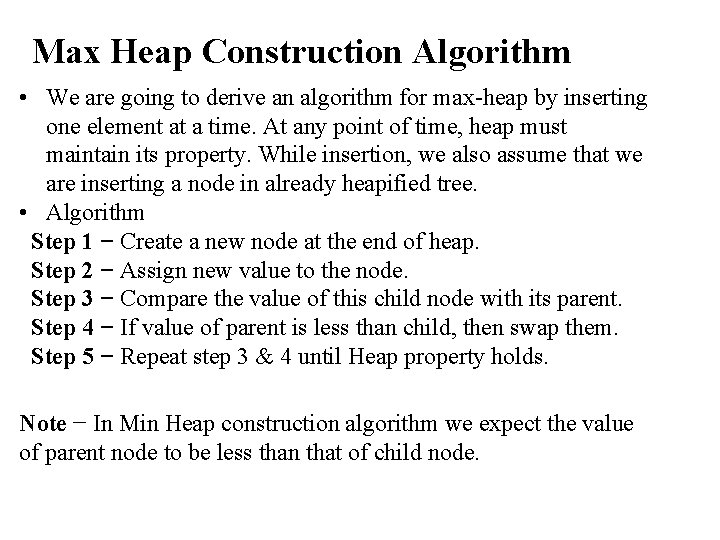
Max Heap Construction Algorithm • We are going to derive an algorithm for max-heap by inserting one element at a time. At any point of time, heap must maintain its property. While insertion, we also assume that we are inserting a node in already heapified tree. • Algorithm Step 1 − Create a new node at the end of heap. Step 2 − Assign new value to the node. Step 3 − Compare the value of this child node with its parent. Step 4 − If value of parent is less than child, then swap them. Step 5 − Repeat step 3 & 4 until Heap property holds. Note − In Min Heap construction algorithm we expect the value of parent node to be less than that of child node.
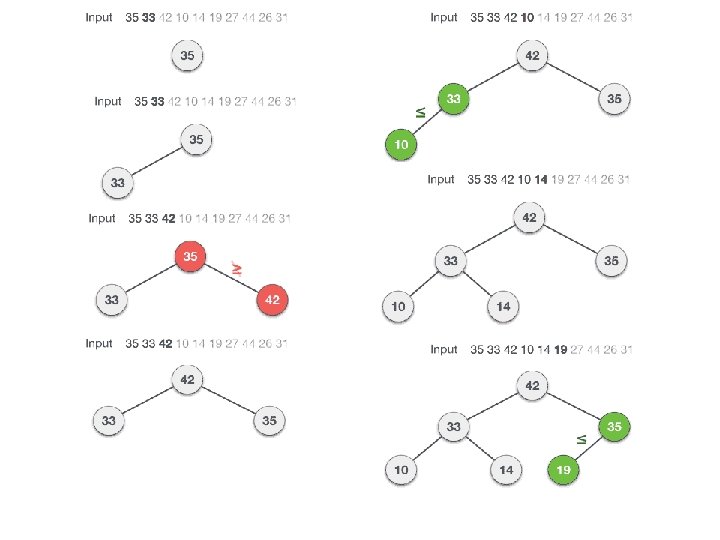
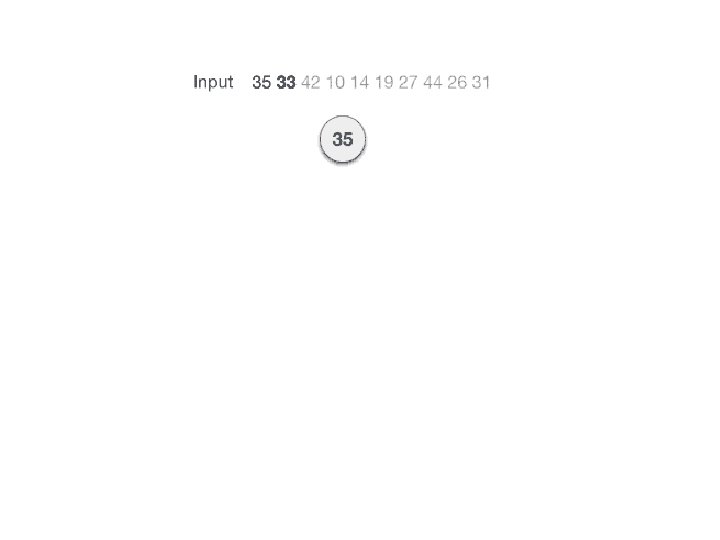
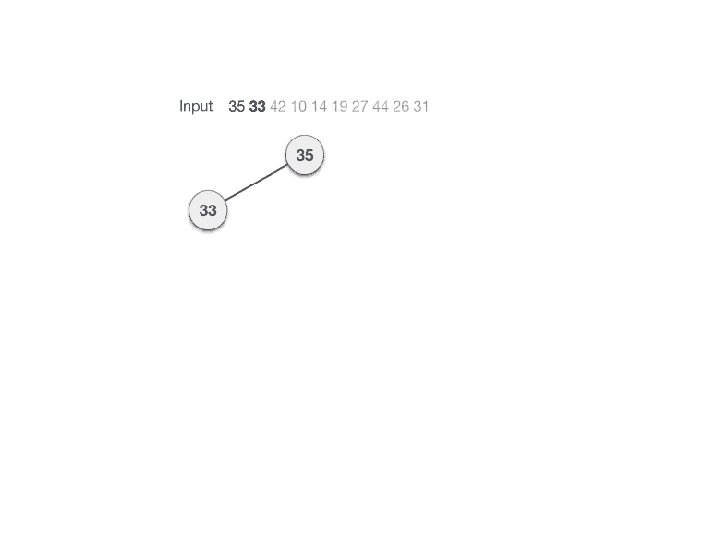
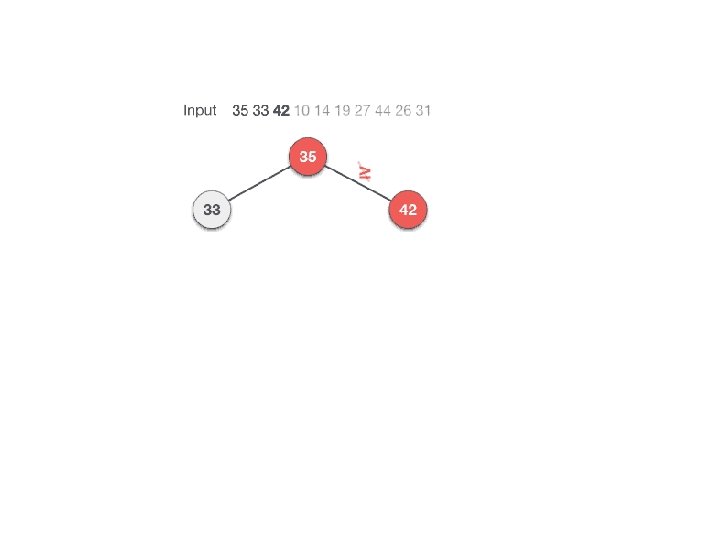
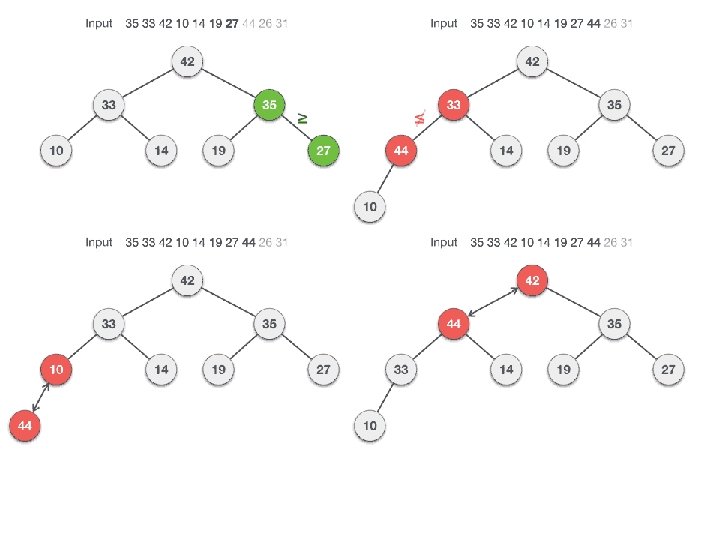
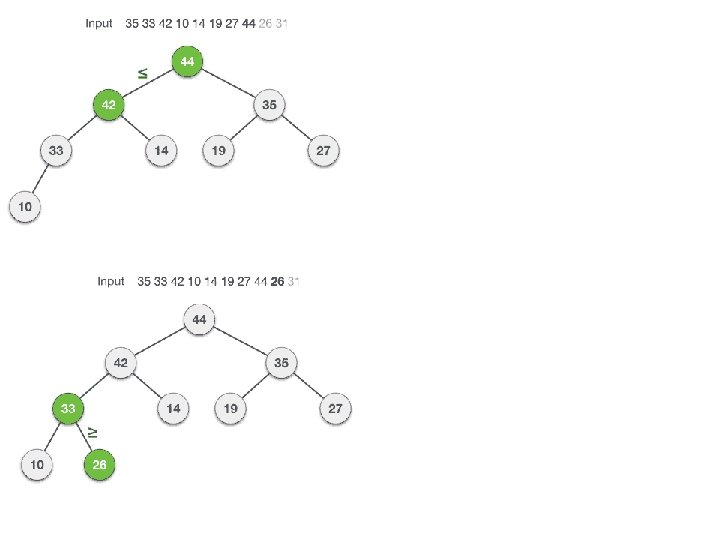