SOEN 343 Software Design Section H Fall 2006
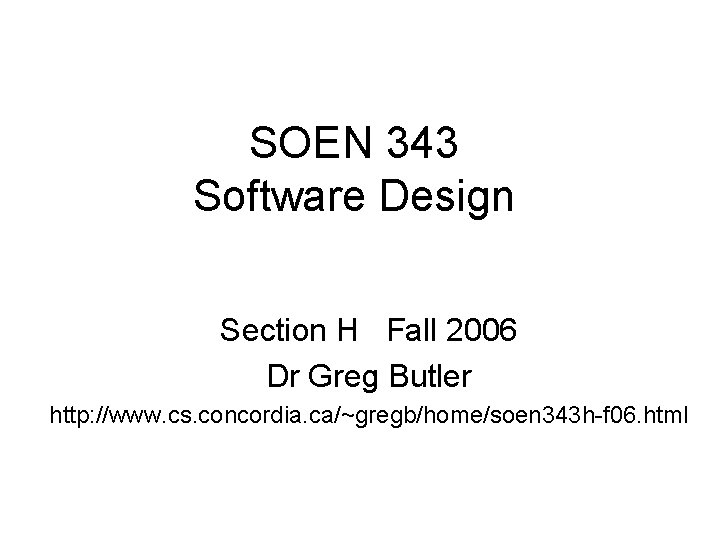
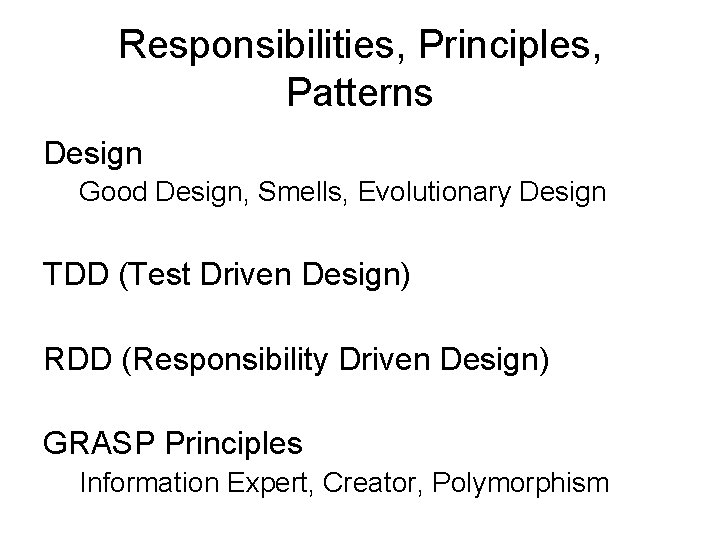
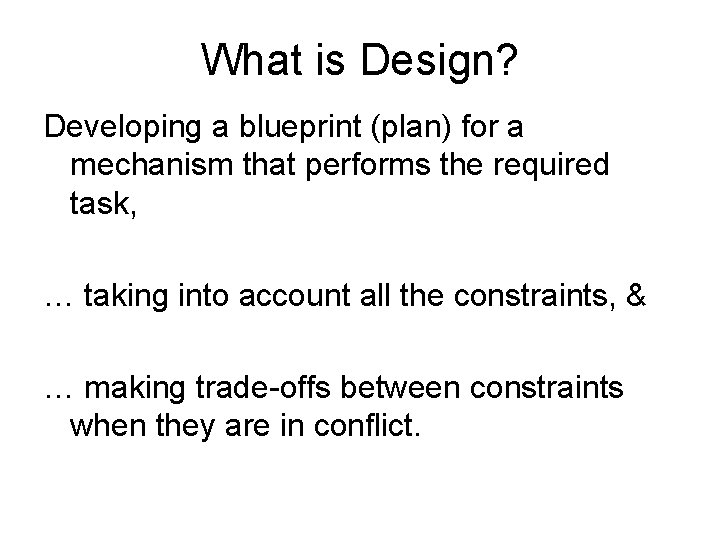
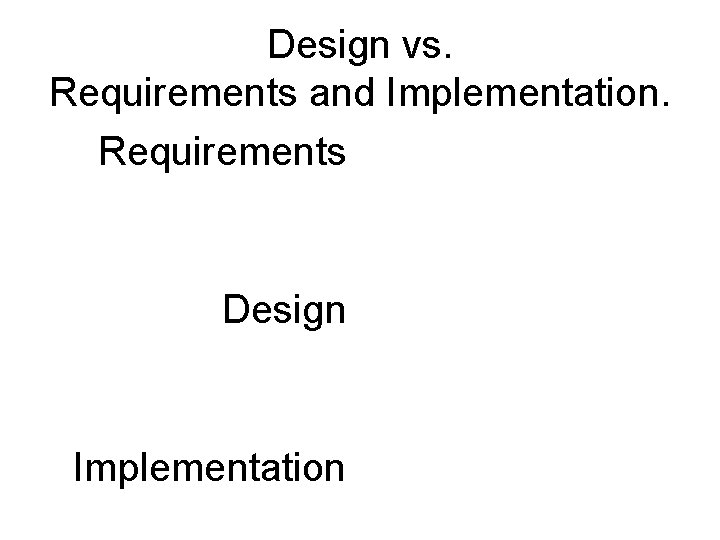
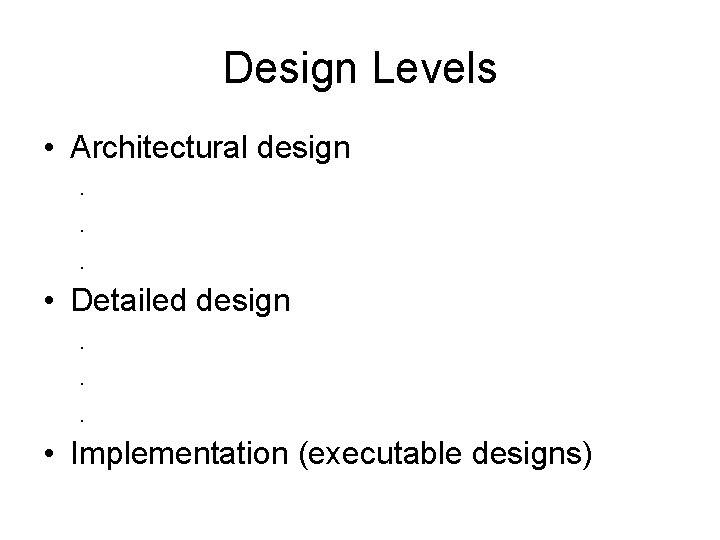
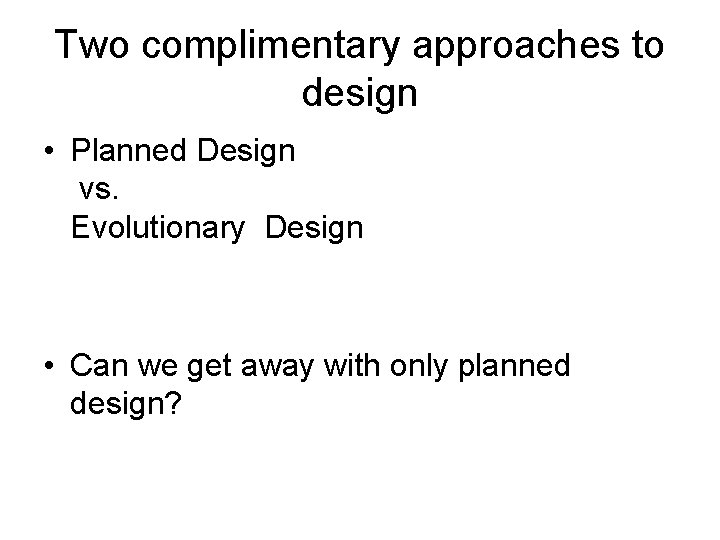
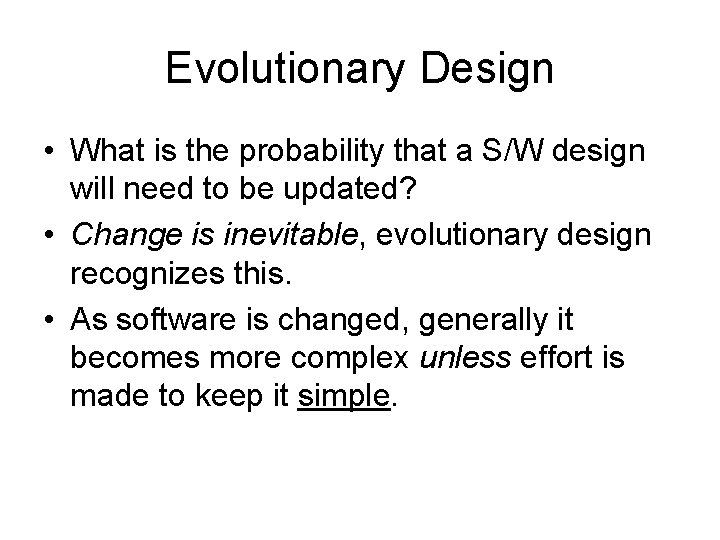
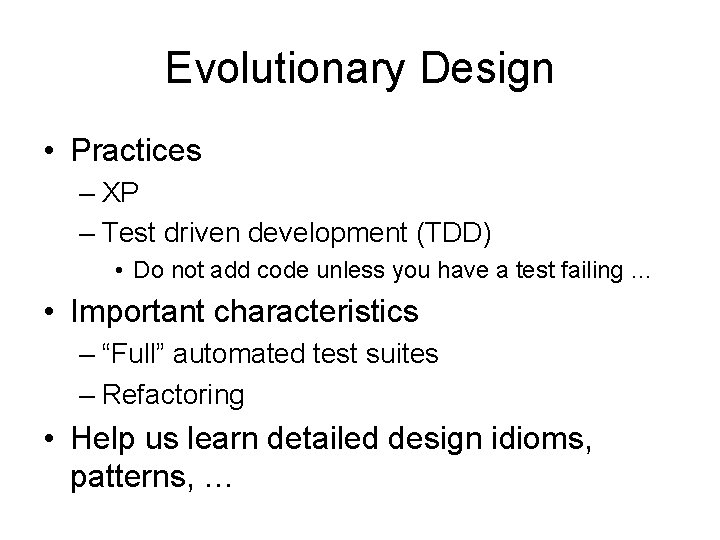
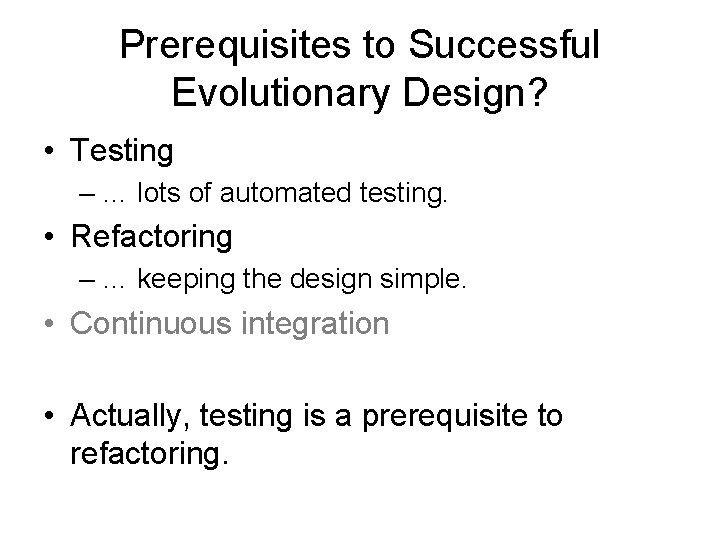
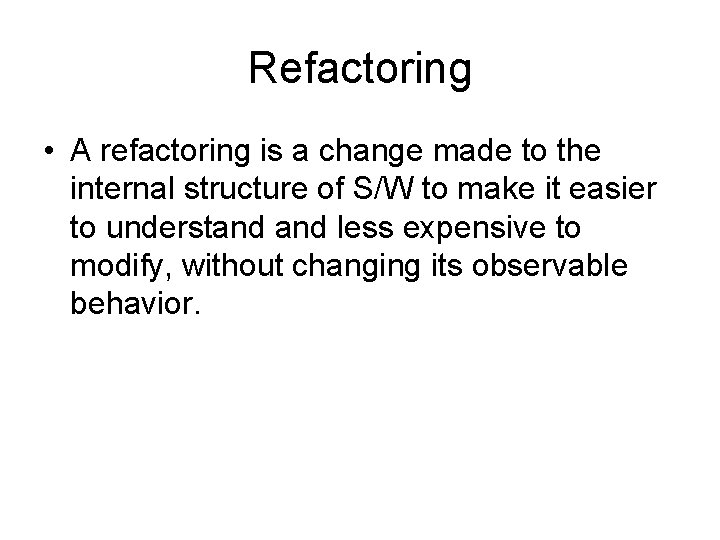
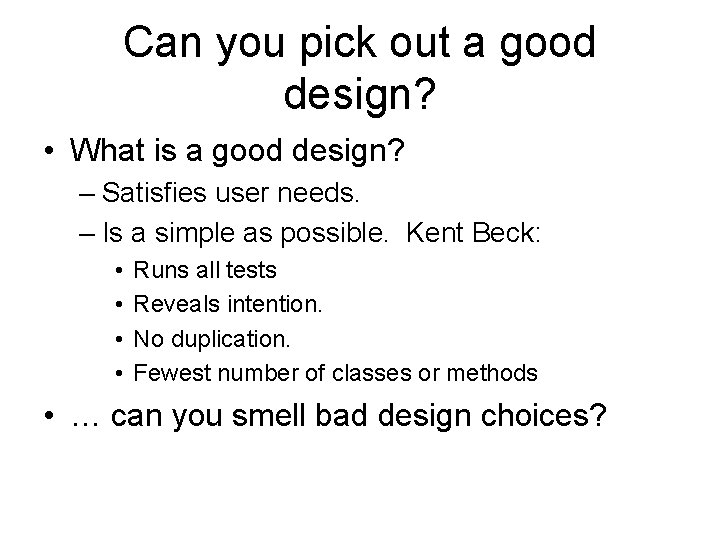
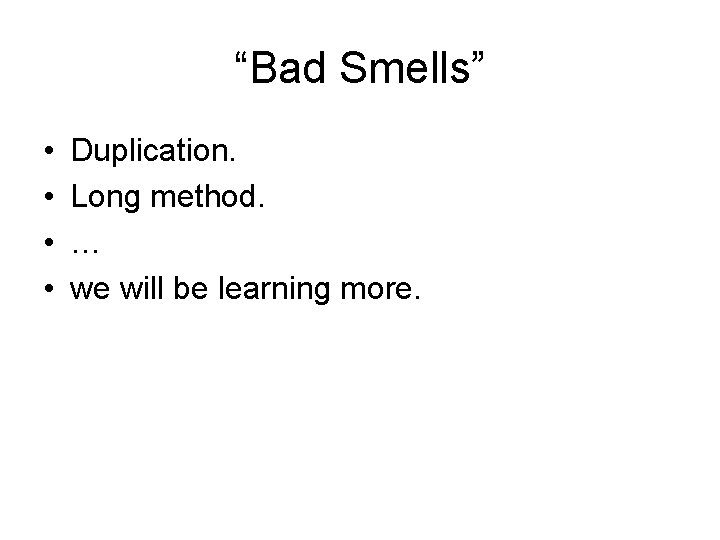
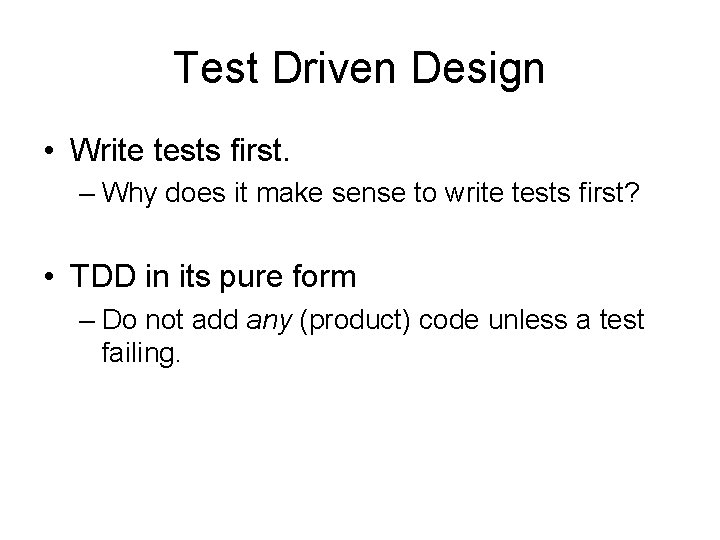
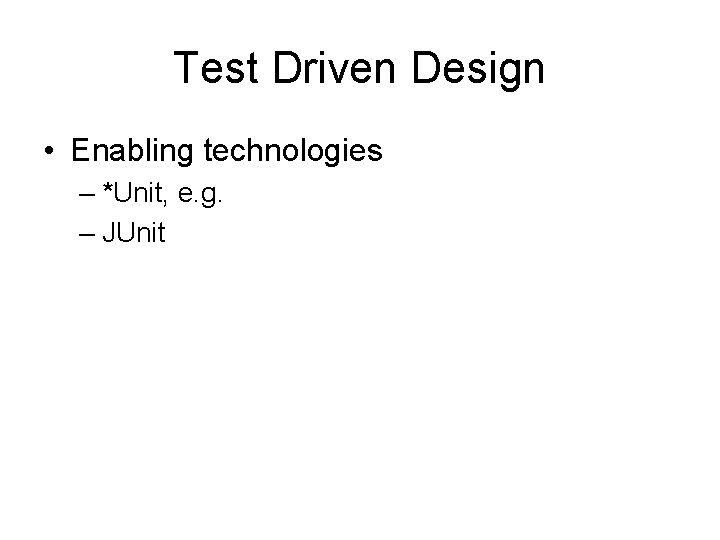
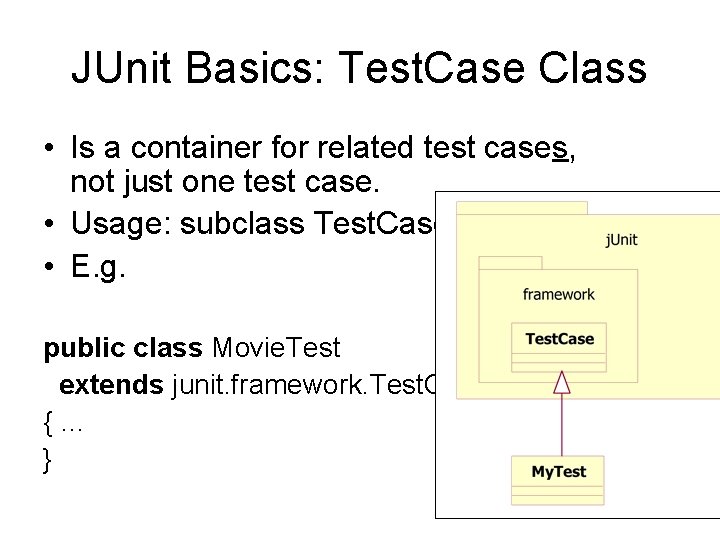
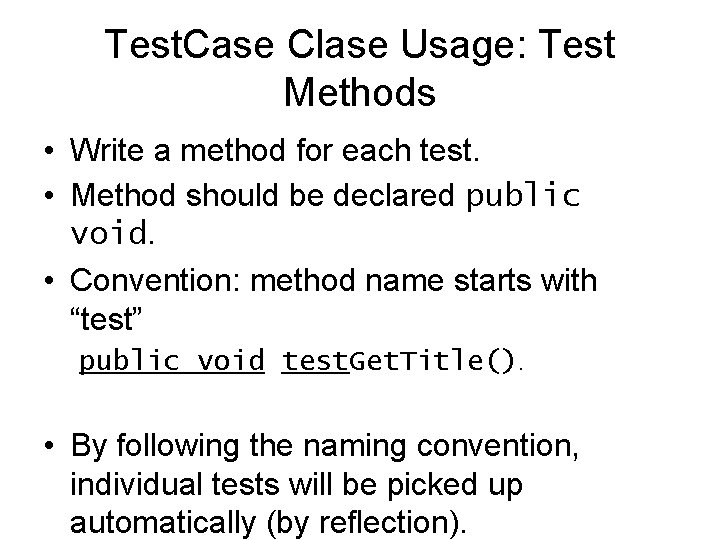
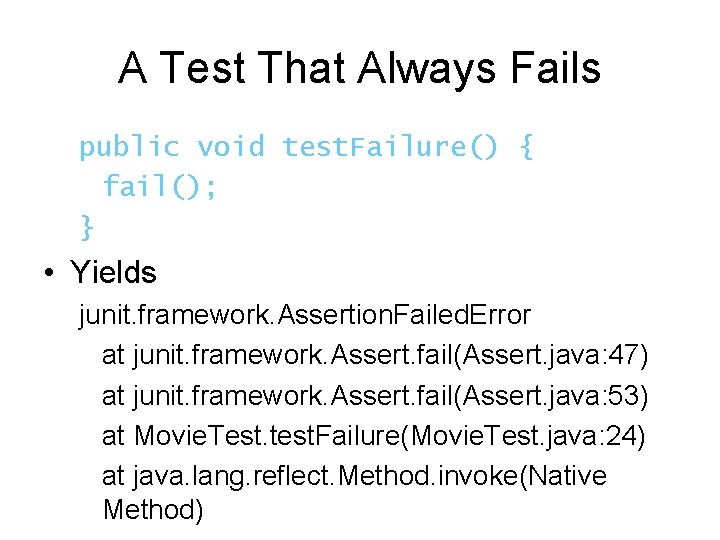
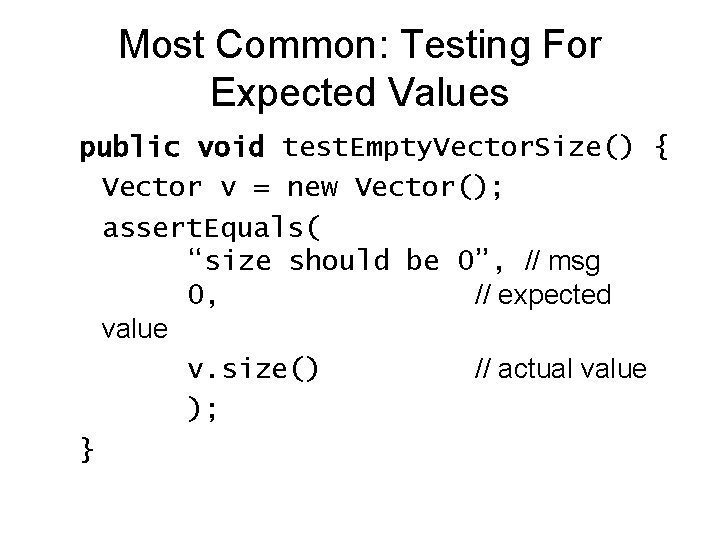
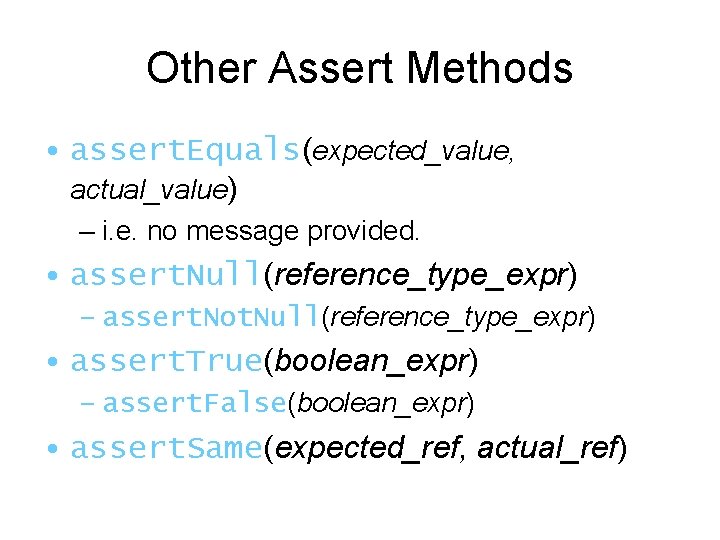
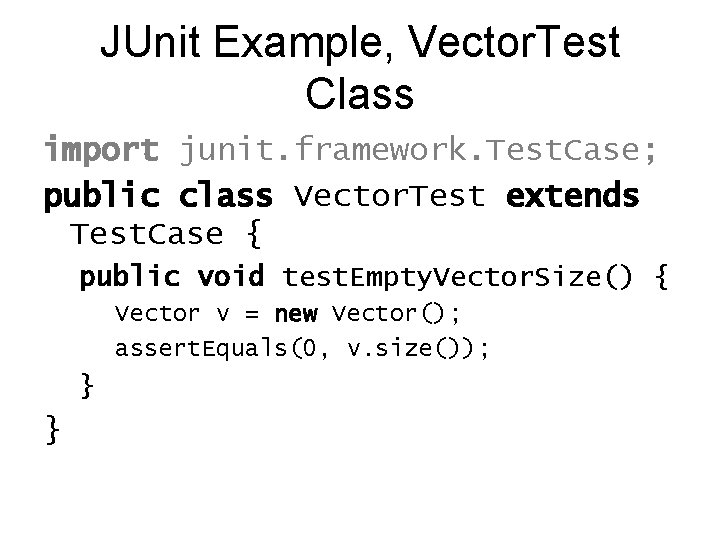
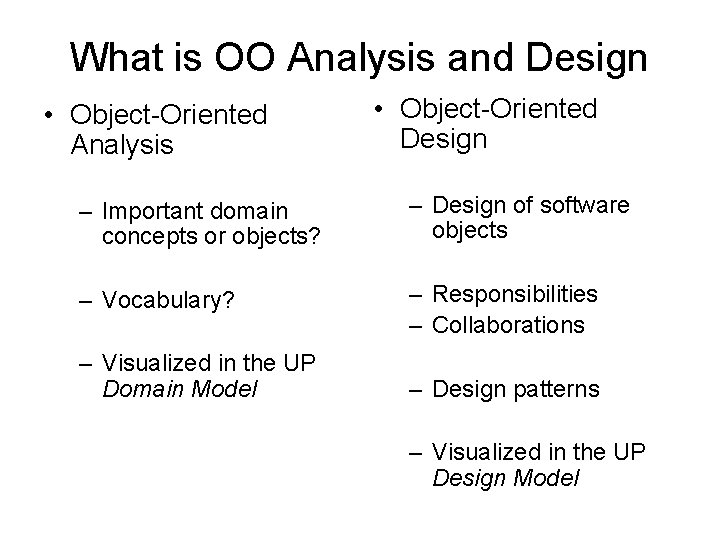
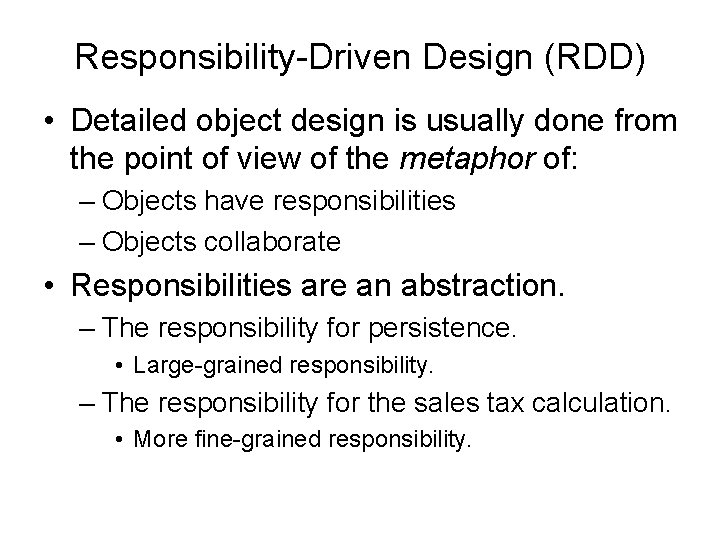
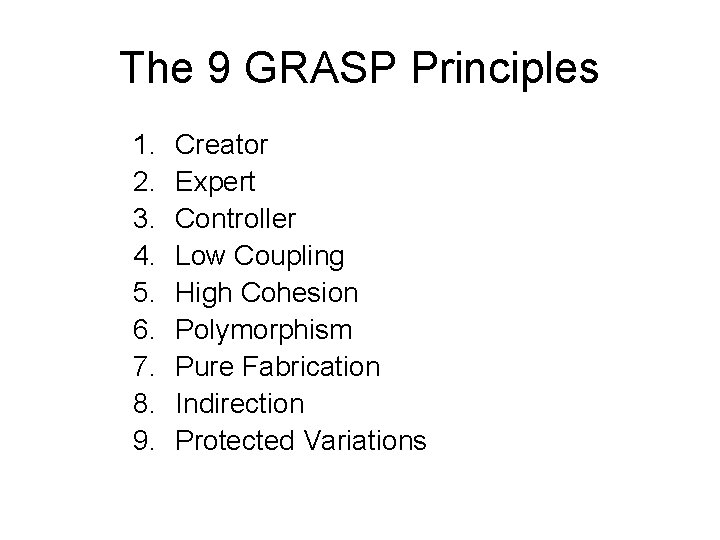
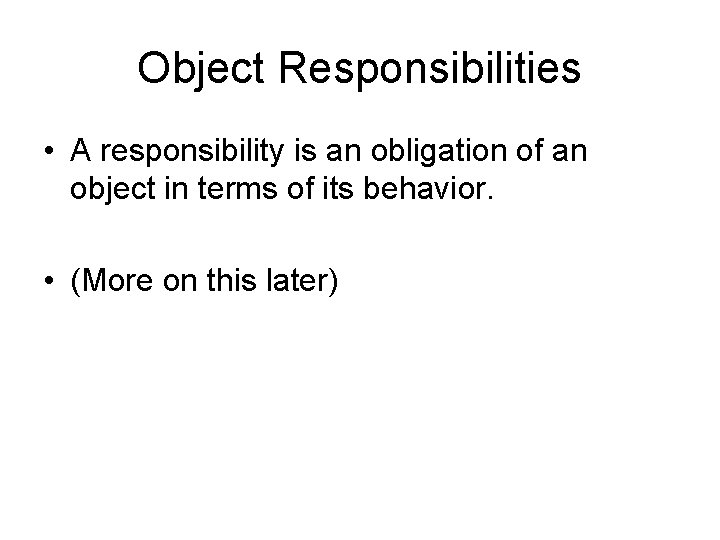
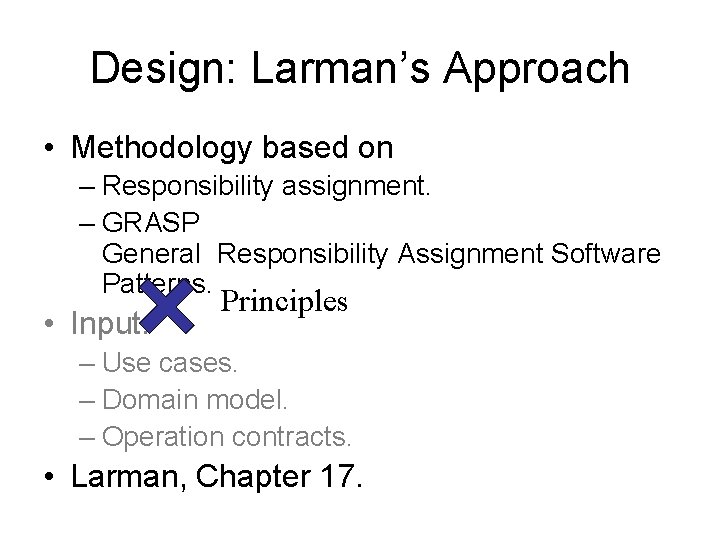
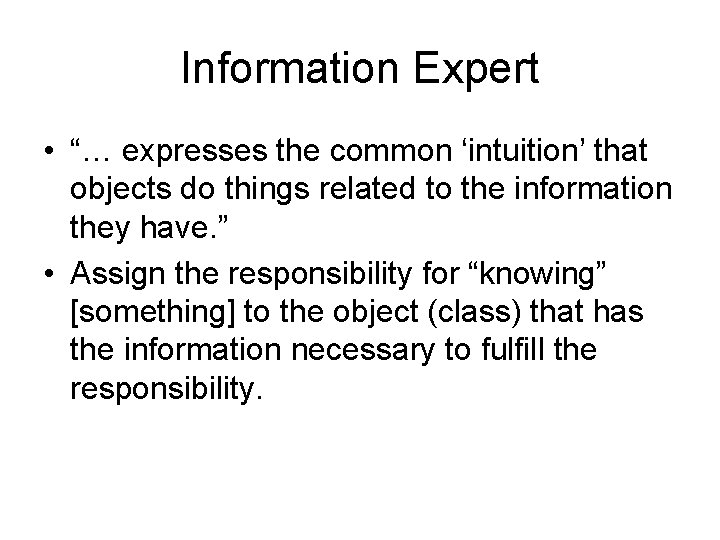
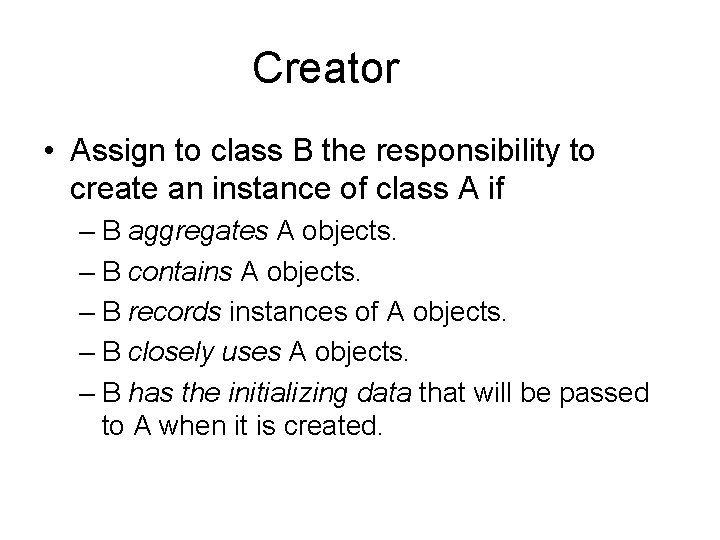
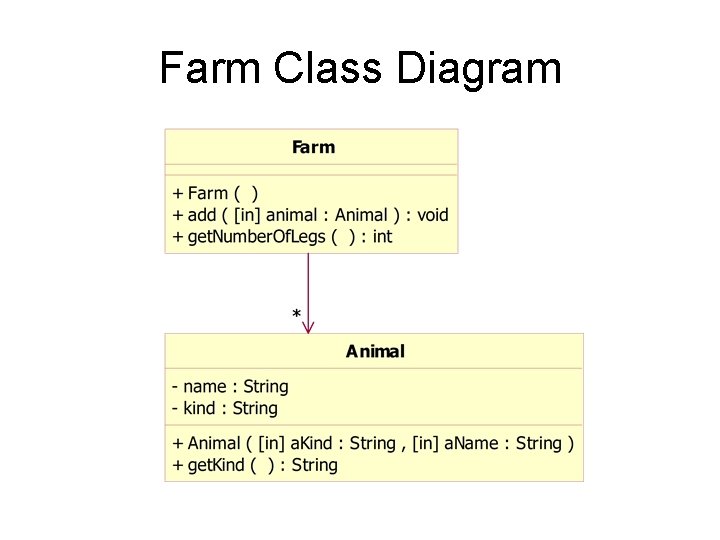
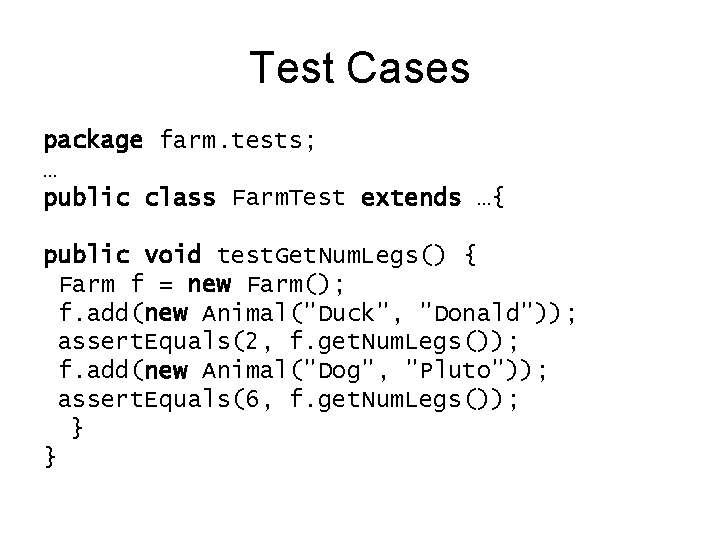
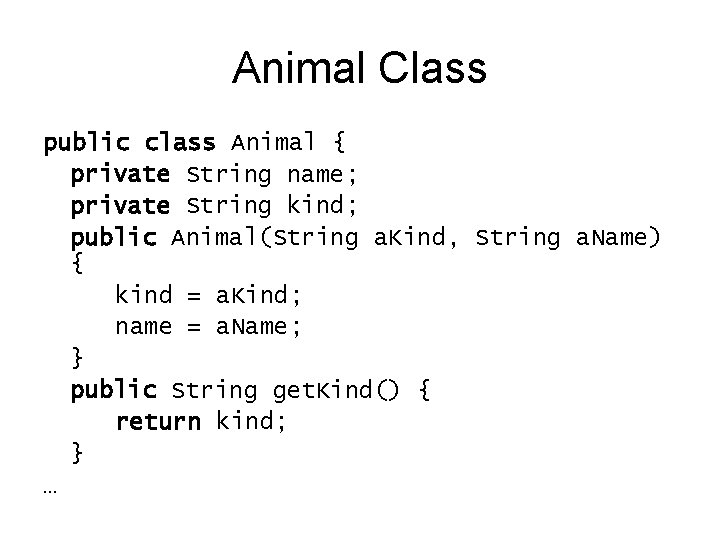
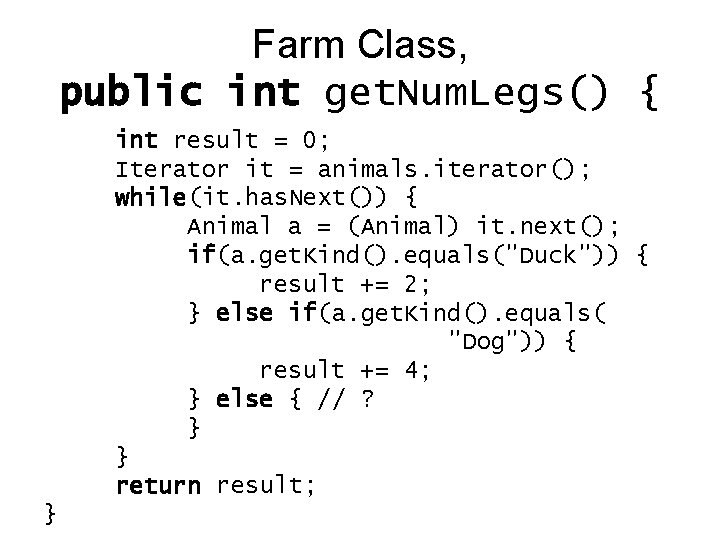
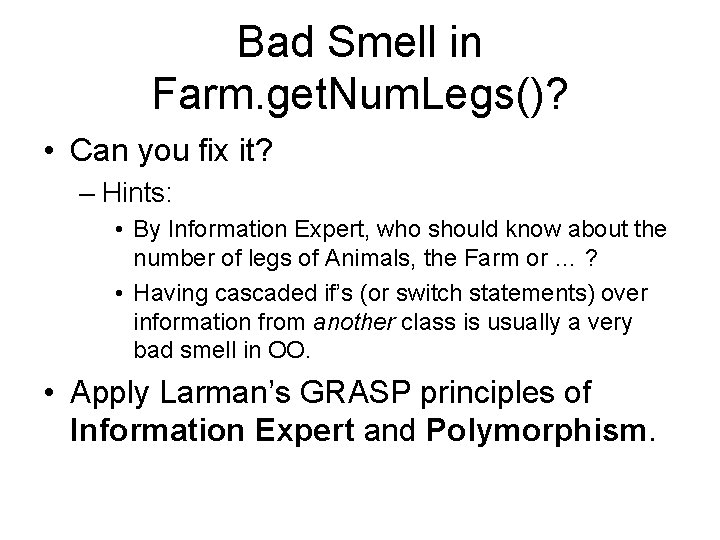
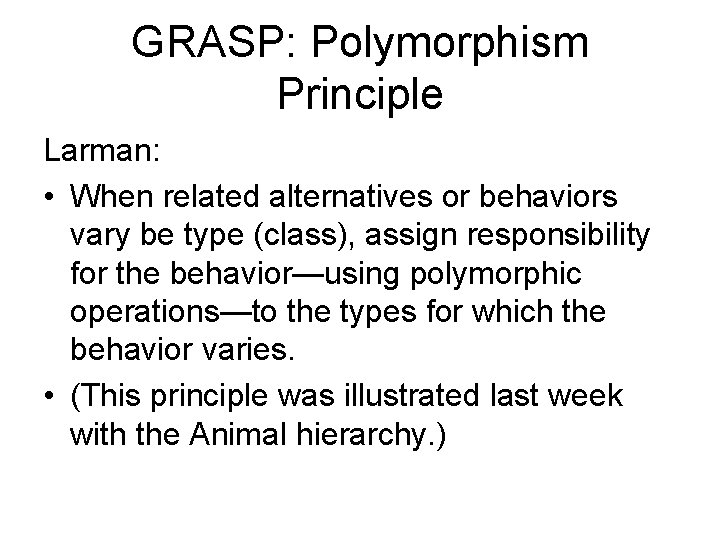
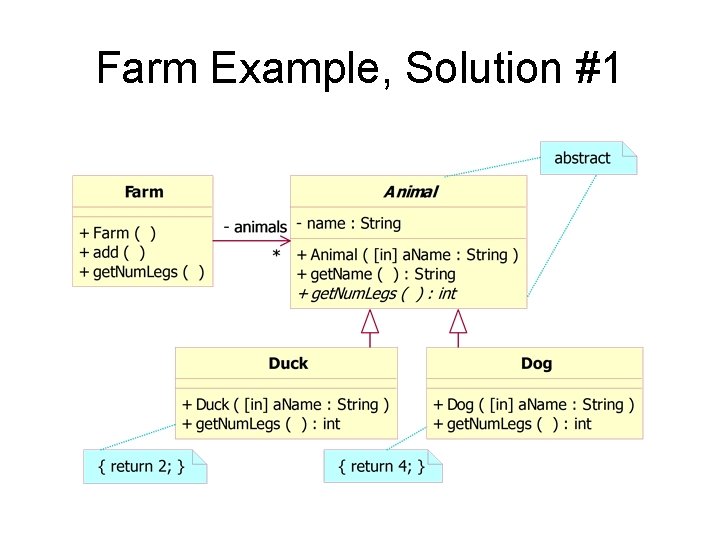
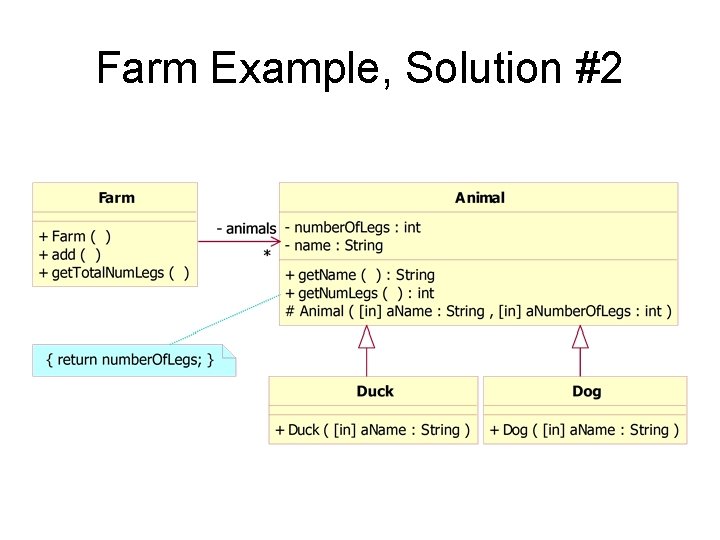
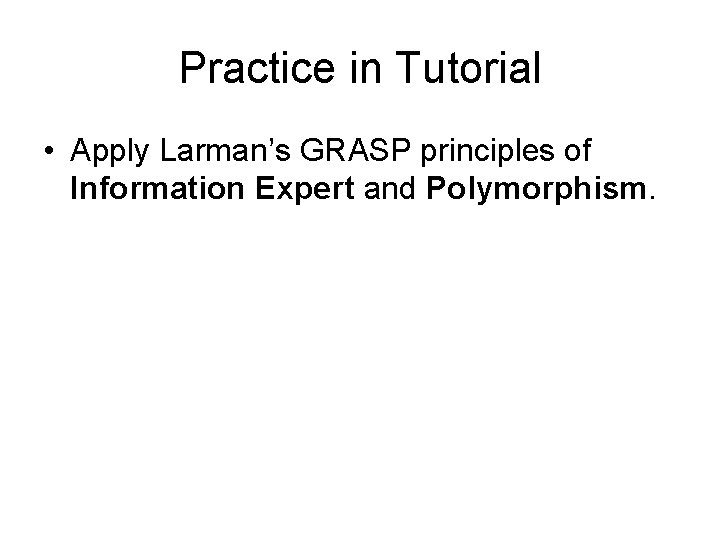
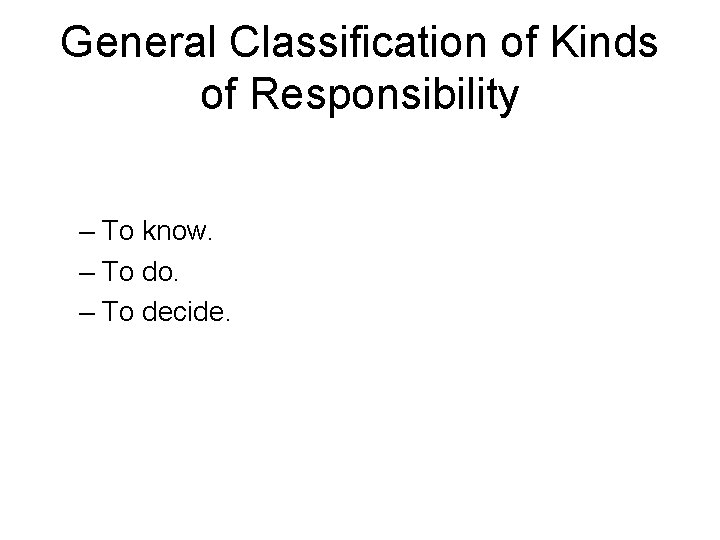
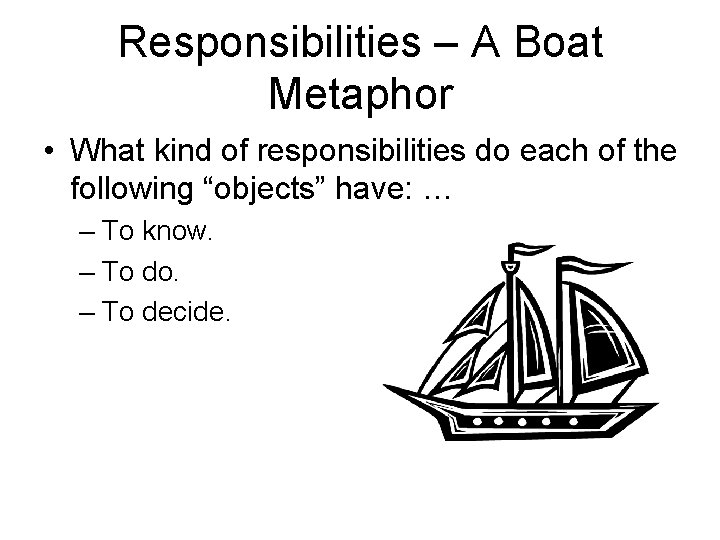
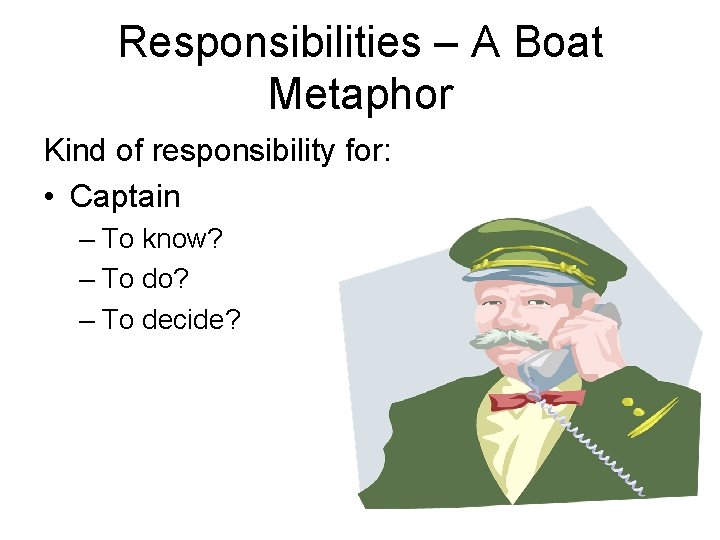
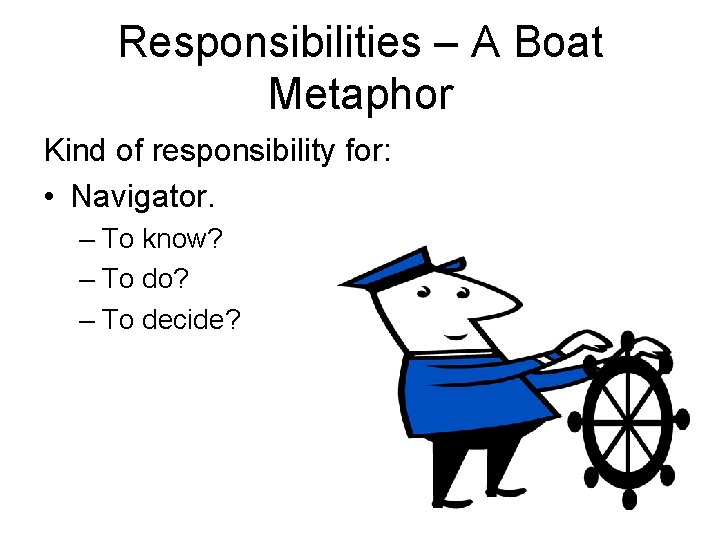
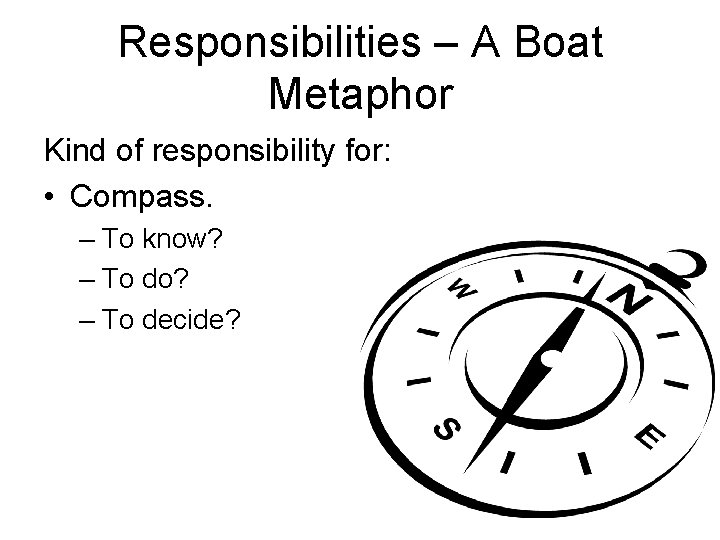
- Slides: 41
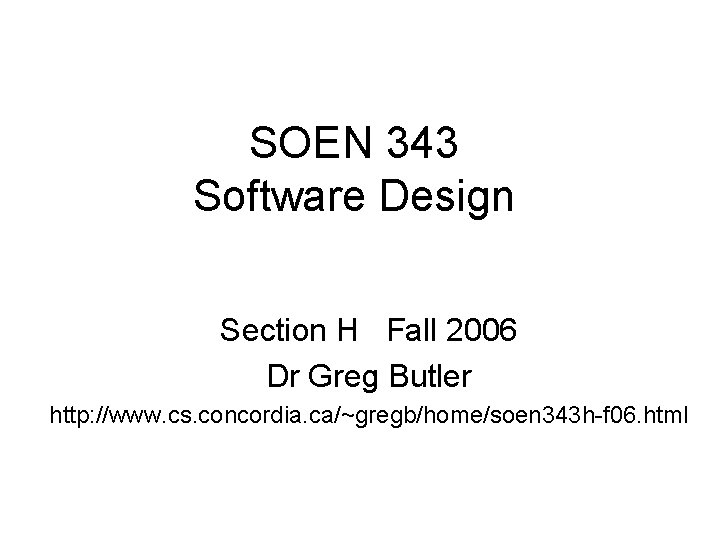
SOEN 343 Software Design Section H Fall 2006 Dr Greg Butler http: //www. cs. concordia. ca/~gregb/home/soen 343 h-f 06. html
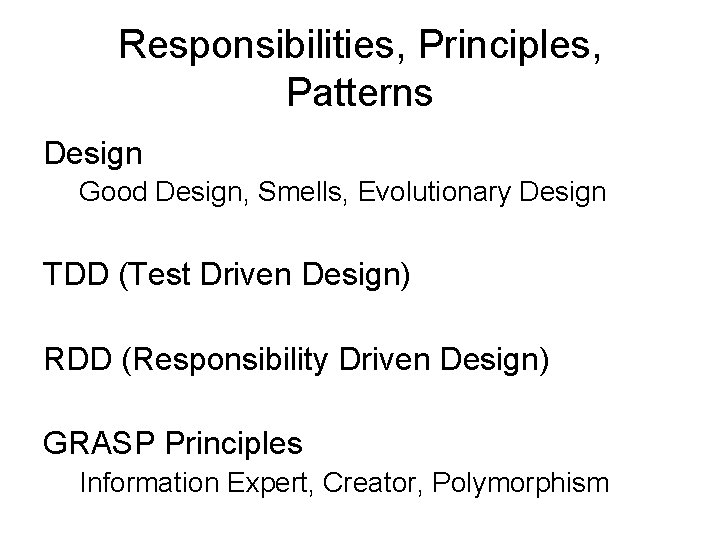
Responsibilities, Principles, Patterns Design Good Design, Smells, Evolutionary Design TDD (Test Driven Design) RDD (Responsibility Driven Design) GRASP Principles Information Expert, Creator, Polymorphism
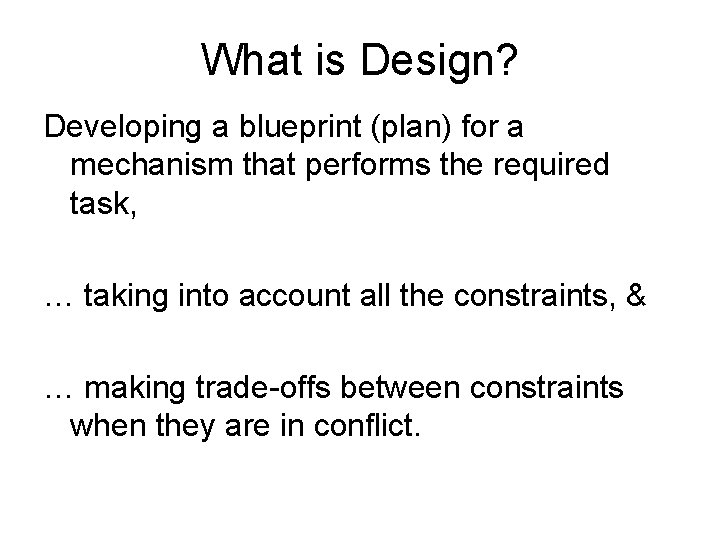
What is Design? Developing a blueprint (plan) for a mechanism that performs the required task, … taking into account all the constraints, & … making trade-offs between constraints when they are in conflict.
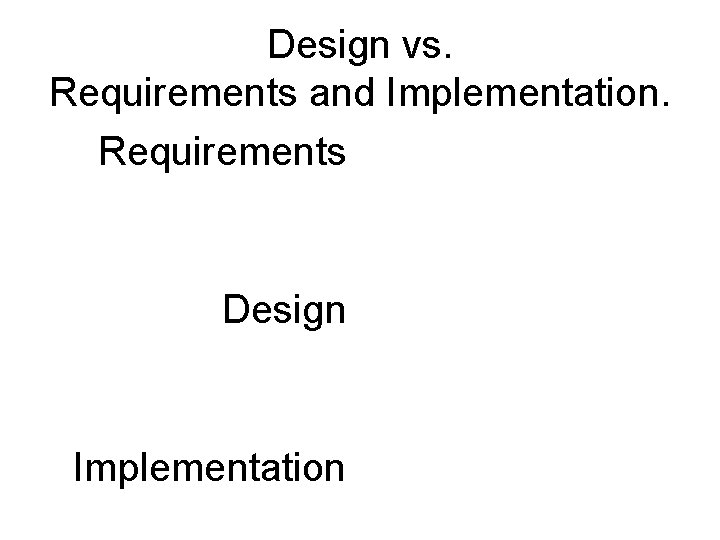
Design vs. Requirements and Implementation. Requirements Design Implementation
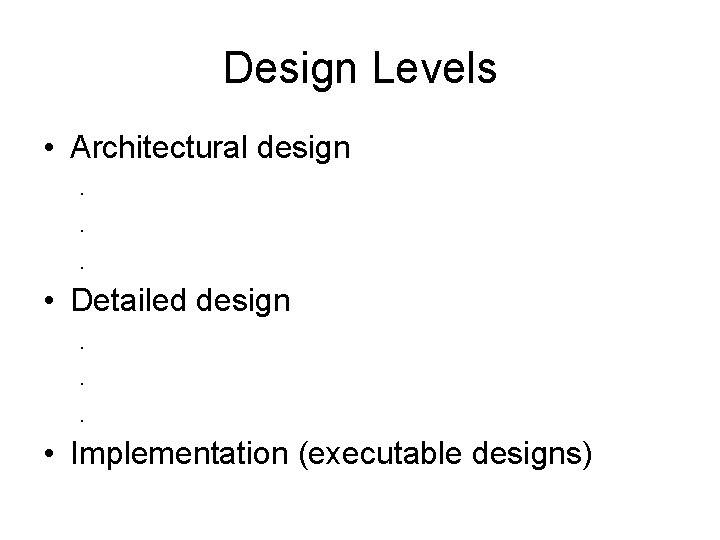
Design Levels • Architectural design. . . • Detailed design. . . • Implementation (executable designs)
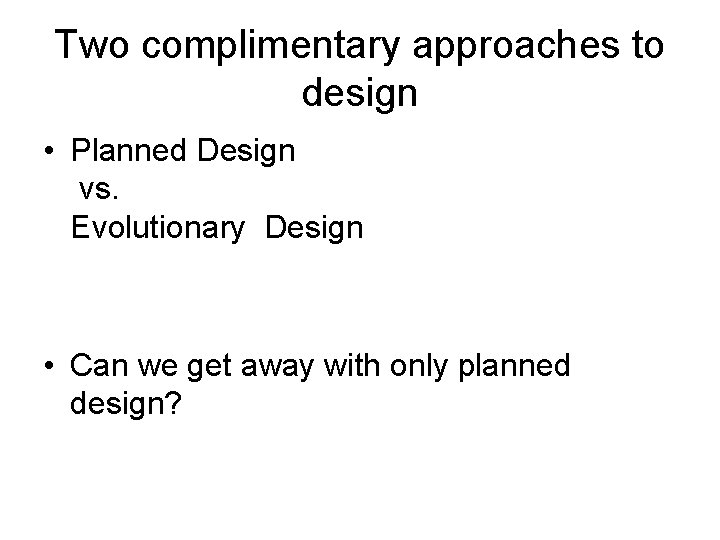
Two complimentary approaches to design • Planned Design vs. Evolutionary Design • Can we get away with only planned design?
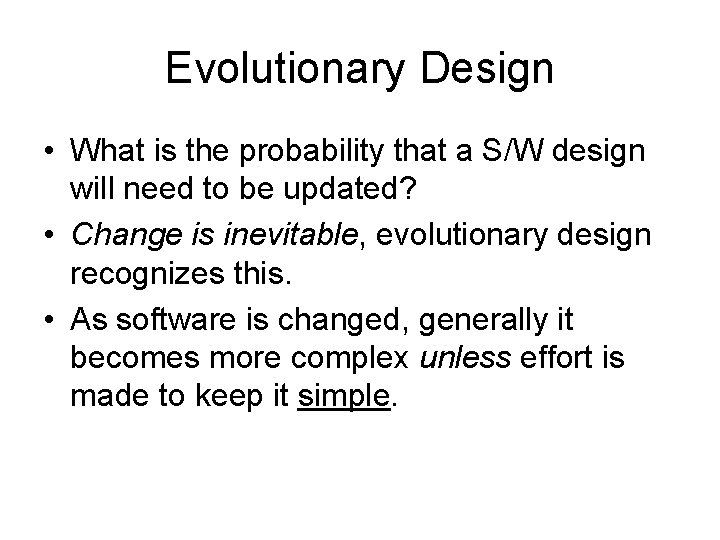
Evolutionary Design • What is the probability that a S/W design will need to be updated? • Change is inevitable, evolutionary design recognizes this. • As software is changed, generally it becomes more complex unless effort is made to keep it simple.
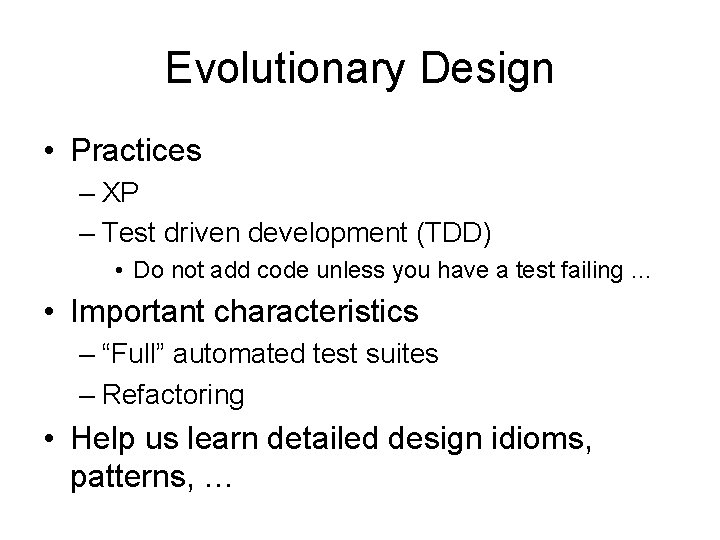
Evolutionary Design • Practices – XP – Test driven development (TDD) • Do not add code unless you have a test failing … • Important characteristics – “Full” automated test suites – Refactoring • Help us learn detailed design idioms, patterns, …
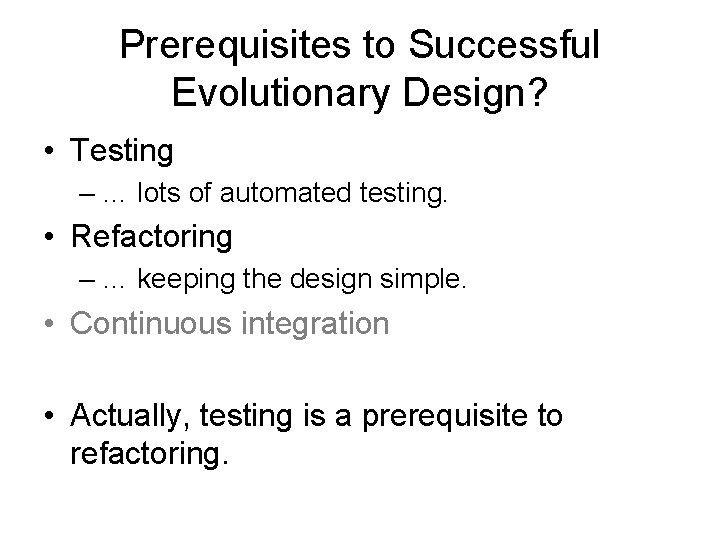
Prerequisites to Successful Evolutionary Design? • Testing – … lots of automated testing. • Refactoring – … keeping the design simple. • Continuous integration • Actually, testing is a prerequisite to refactoring.
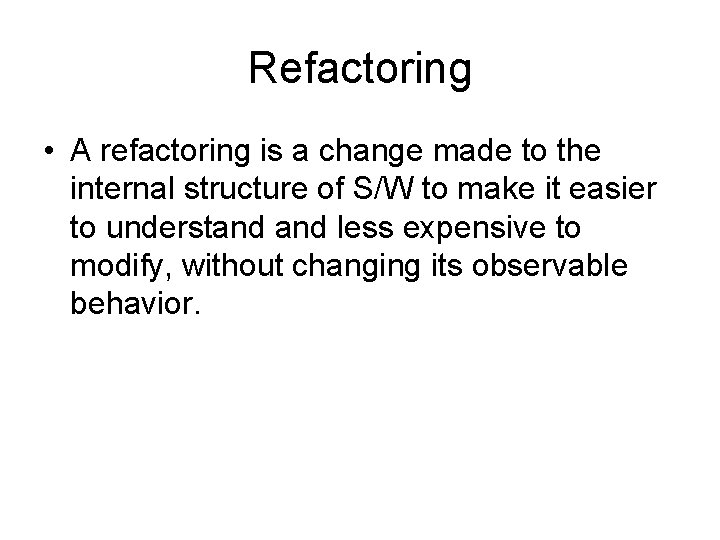
Refactoring • A refactoring is a change made to the internal structure of S/W to make it easier to understand less expensive to modify, without changing its observable behavior.
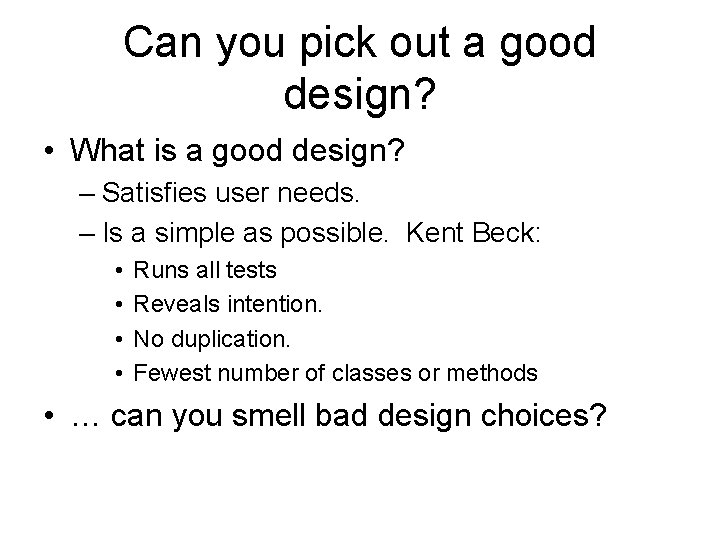
Can you pick out a good design? • What is a good design? – Satisfies user needs. – Is a simple as possible. Kent Beck: • • Runs all tests Reveals intention. No duplication. Fewest number of classes or methods • … can you smell bad design choices?
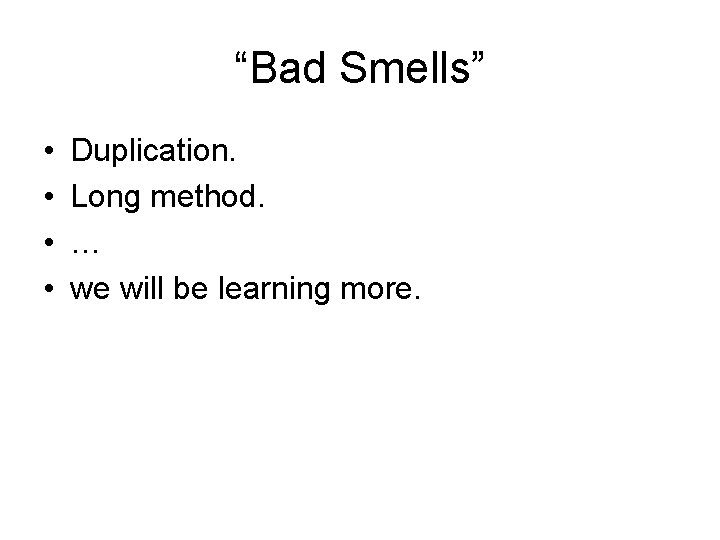
“Bad Smells” • • Duplication. Long method. … we will be learning more.
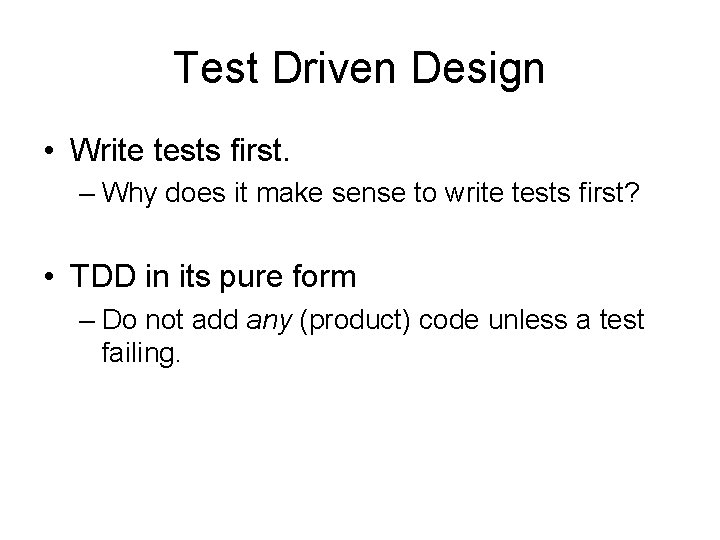
Test Driven Design • Write tests first. – Why does it make sense to write tests first? • TDD in its pure form – Do not add any (product) code unless a test failing.
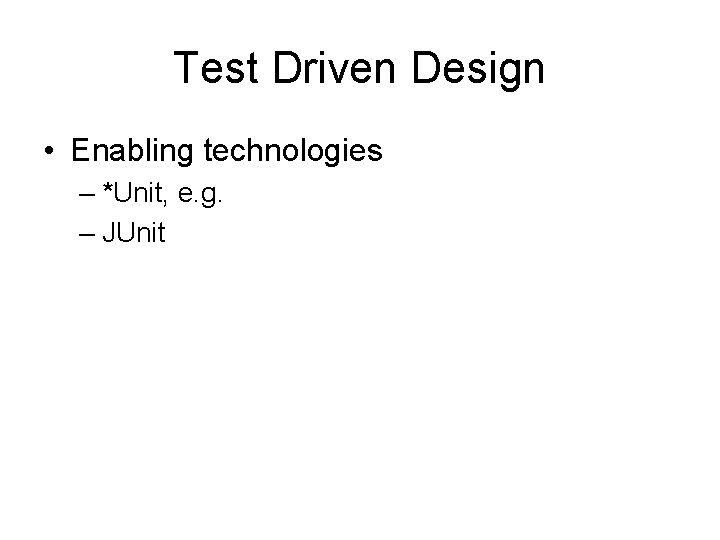
Test Driven Design • Enabling technologies – *Unit, e. g. – JUnit
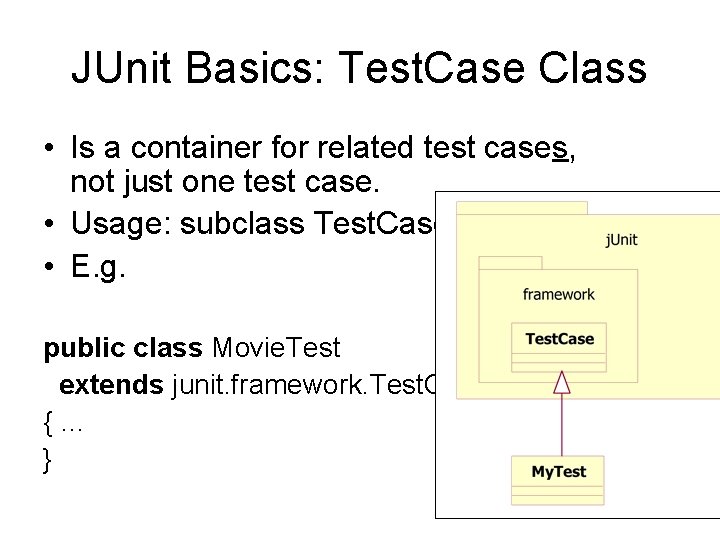
JUnit Basics: Test. Case Class • Is a container for related test cases, not just one test case. • Usage: subclass Test. Case. • E. g. public class Movie. Test extends junit. framework. Test. Case {… }
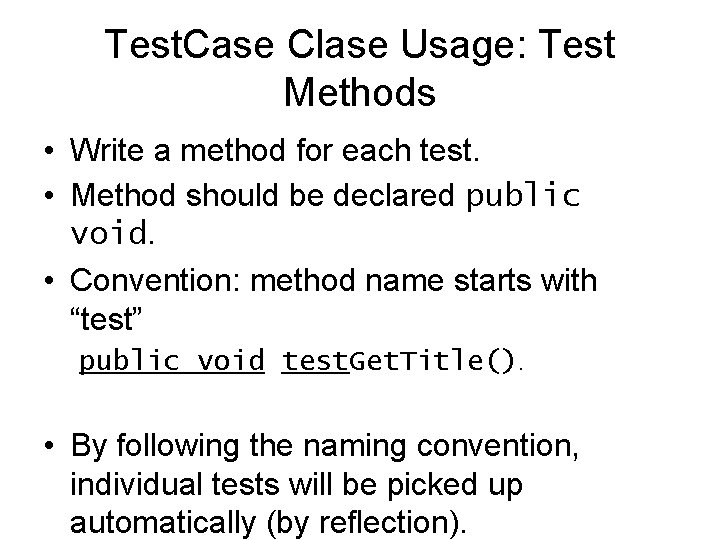
Test. Case Clase Usage: Test Methods • Write a method for each test. • Method should be declared public void. • Convention: method name starts with “test” public void test. Get. Title(). • By following the naming convention, individual tests will be picked up automatically (by reflection).
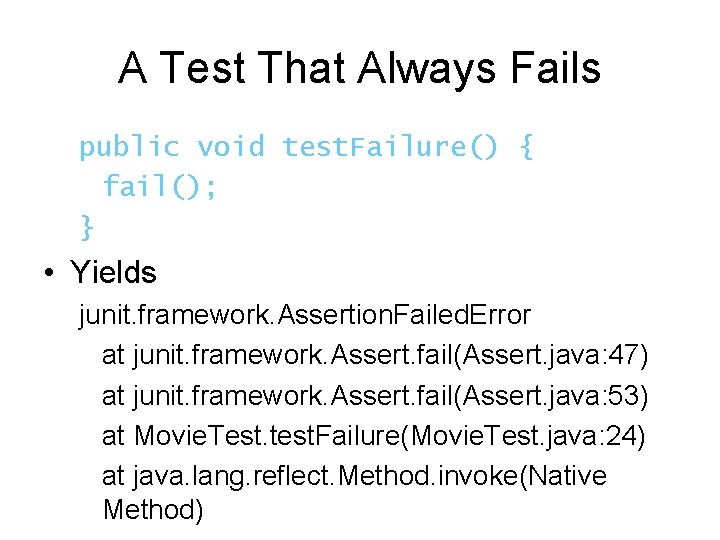
A Test That Always Fails public void test. Failure() { fail(); } • Yields junit. framework. Assertion. Failed. Error at junit. framework. Assert. fail(Assert. java: 47) at junit. framework. Assert. fail(Assert. java: 53) at Movie. Test. test. Failure(Movie. Test. java: 24) at java. lang. reflect. Method. invoke(Native Method)
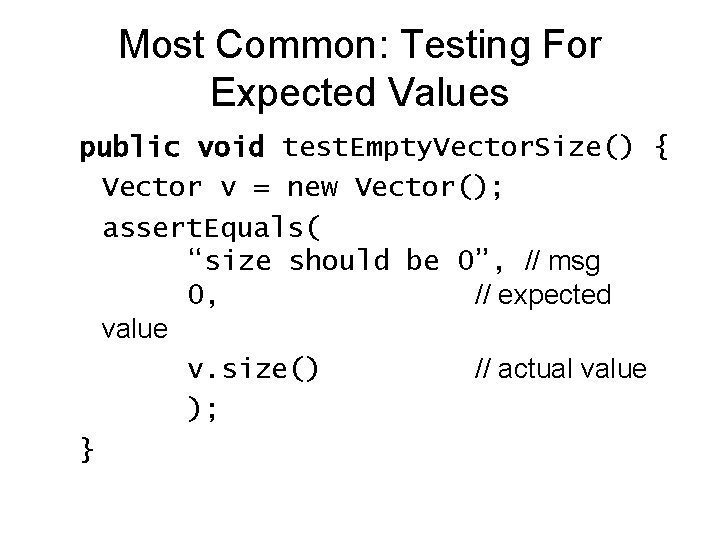
Most Common: Testing For Expected Values public void test. Empty. Vector. Size() { Vector v = new Vector(); assert. Equals( “size should be 0”, // msg 0, // expected value v. size() // actual value ); }
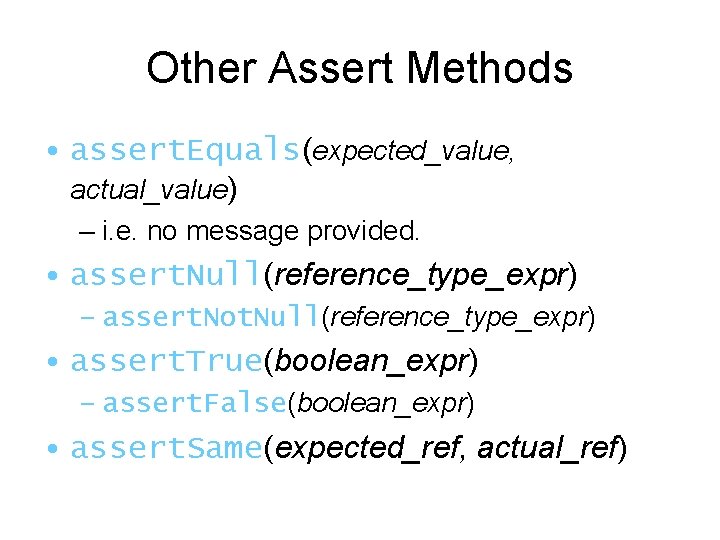
Other Assert Methods • assert. Equals(expected_value, actual_value) – i. e. no message provided. • assert. Null(reference_type_expr) – assert. Not. Null(reference_type_expr) • assert. True(boolean_expr) – assert. False(boolean_expr) • assert. Same(expected_ref, actual_ref)
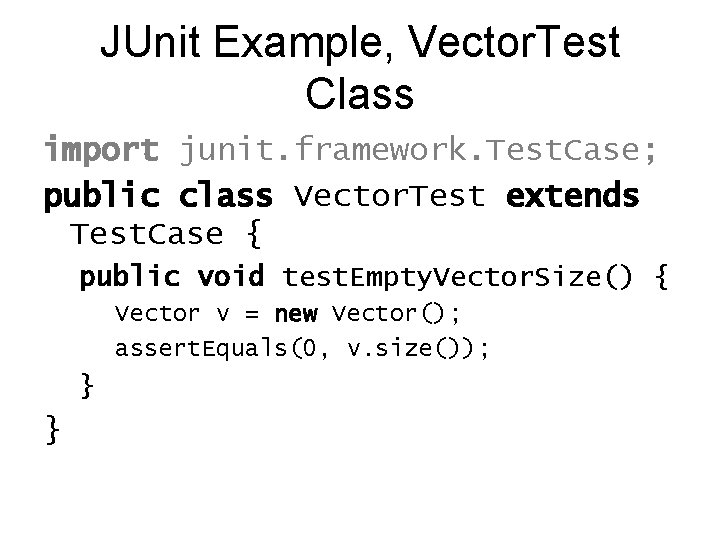
JUnit Example, Vector. Test Class import junit. framework. Test. Case; public class Vector. Test extends Test. Case { public void test. Empty. Vector. Size() { Vector v = new Vector(); assert. Equals(0, v. size()); } }
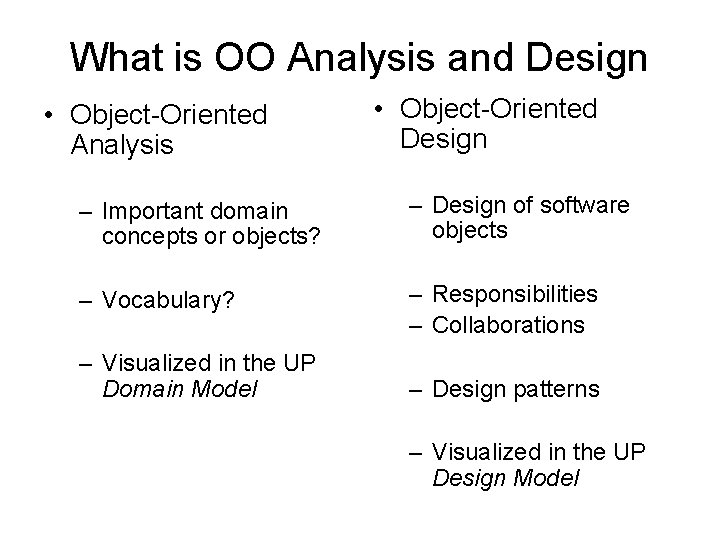
What is OO Analysis and Design • Object-Oriented Analysis • Object-Oriented Design – Important domain concepts or objects? – Design of software objects – Vocabulary? – Responsibilities – Collaborations – Visualized in the UP Domain Model – Design patterns – Visualized in the UP Design Model
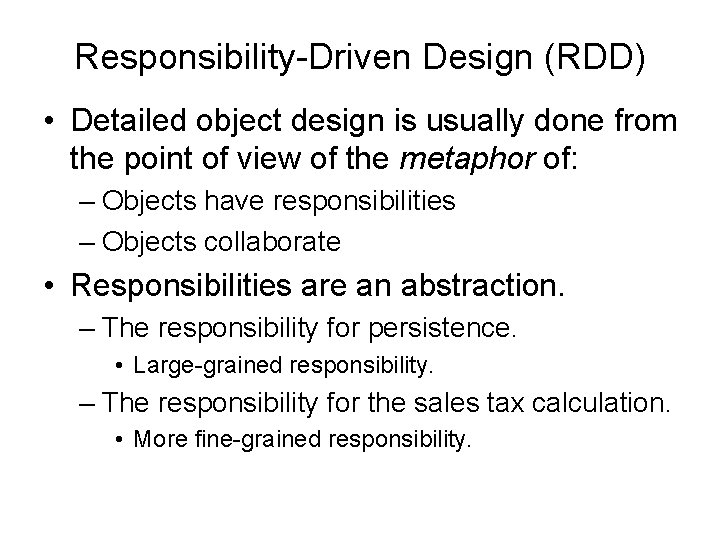
Responsibility-Driven Design (RDD) • Detailed object design is usually done from the point of view of the metaphor of: – Objects have responsibilities – Objects collaborate • Responsibilities are an abstraction. – The responsibility for persistence. • Large-grained responsibility. – The responsibility for the sales tax calculation. • More fine-grained responsibility.
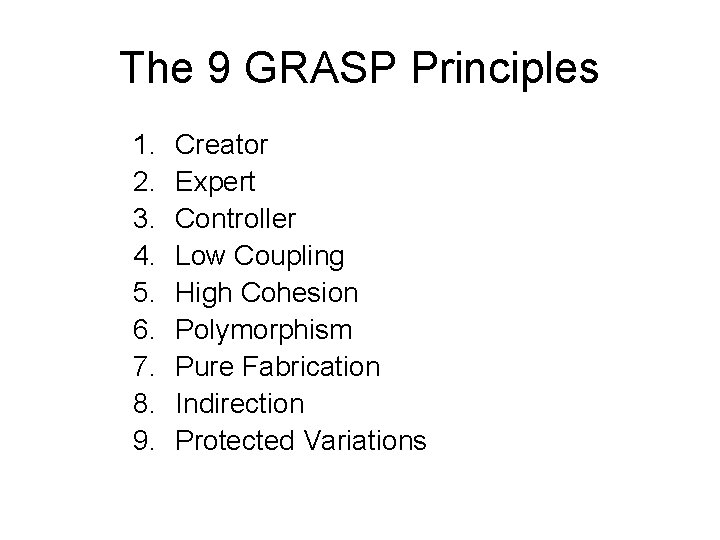
The 9 GRASP Principles 1. 2. 3. 4. 5. 6. 7. 8. 9. Creator Expert Controller Low Coupling High Cohesion Polymorphism Pure Fabrication Indirection Protected Variations
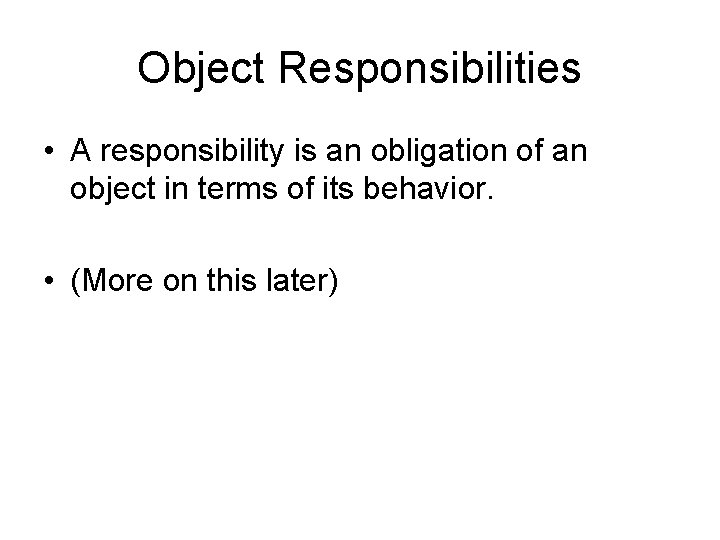
Object Responsibilities • A responsibility is an obligation of an object in terms of its behavior. • (More on this later)
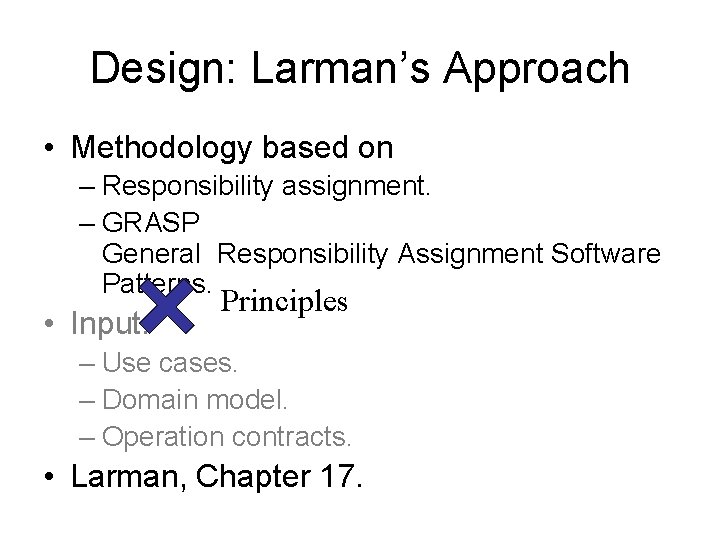
Design: Larman’s Approach • Methodology based on – Responsibility assignment. – GRASP General Responsibility Assignment Software Patterns. • Input: Principles – Use cases. – Domain model. – Operation contracts. • Larman, Chapter 17.
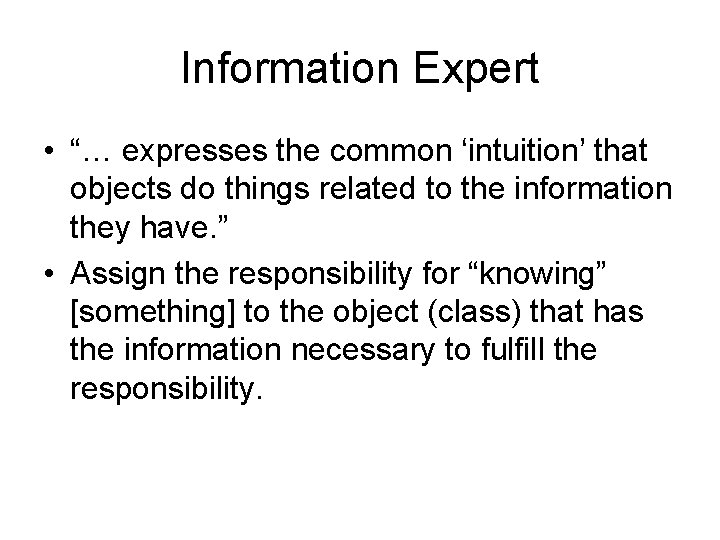
Information Expert • “… expresses the common ‘intuition’ that objects do things related to the information they have. ” • Assign the responsibility for “knowing” [something] to the object (class) that has the information necessary to fulfill the responsibility.
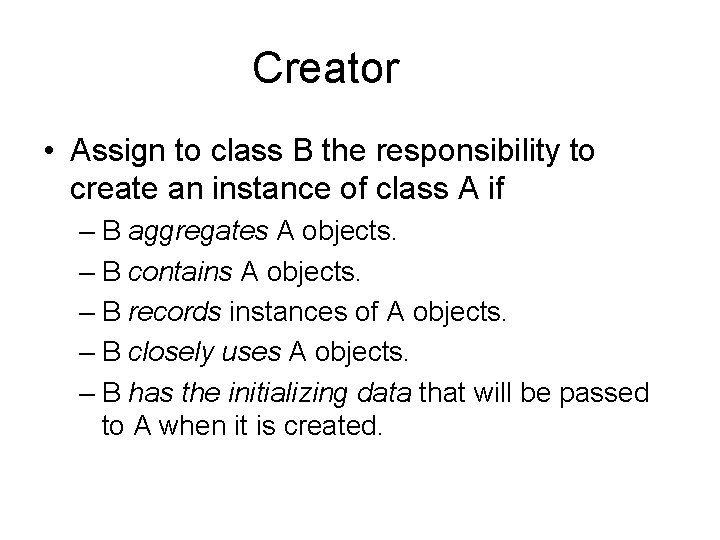
Creator • Assign to class B the responsibility to create an instance of class A if – B aggregates A objects. – B contains A objects. – B records instances of A objects. – B closely uses A objects. – B has the initializing data that will be passed to A when it is created.
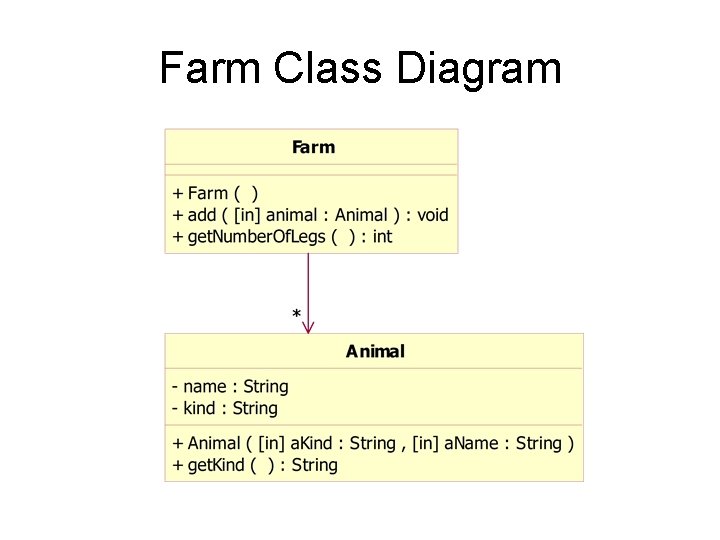
Farm Class Diagram - animals
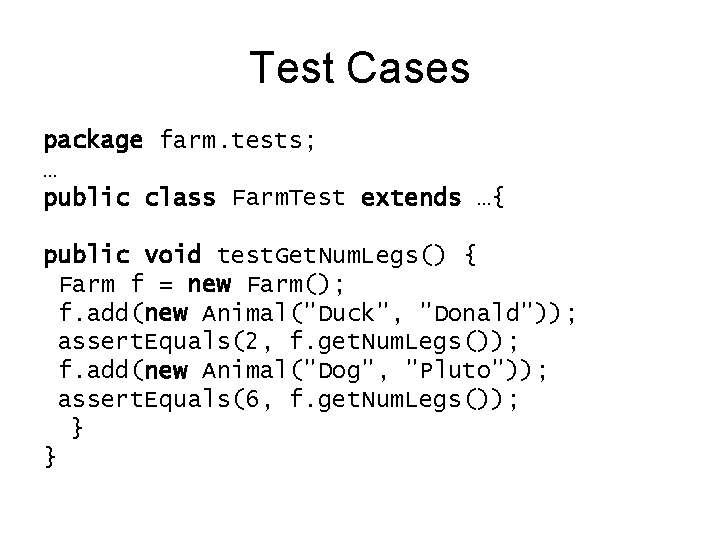
Test Cases package farm. tests; … public class Farm. Test extends …{ public void test. Get. Num. Legs() { Farm f = new Farm(); f. add(new Animal("Duck", "Donald")); assert. Equals(2, f. get. Num. Legs()); f. add(new Animal("Dog", "Pluto")); assert. Equals(6, f. get. Num. Legs()); } }
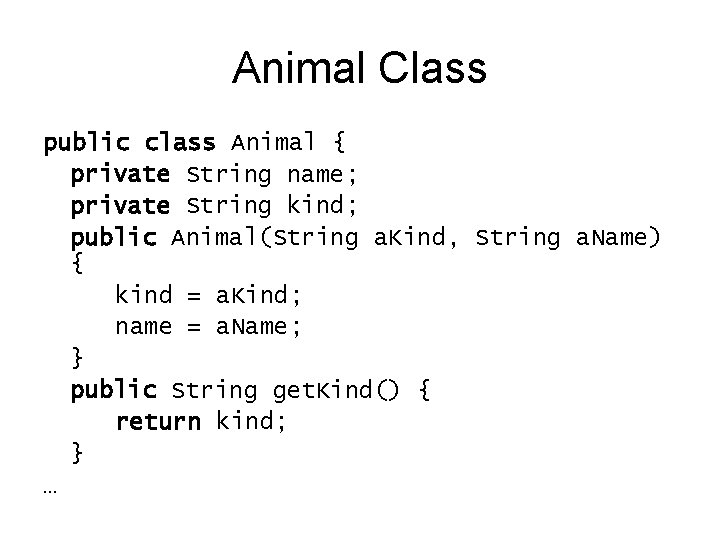
Animal Class public class Animal { private String name; private String kind; public Animal(String a. Kind, String a. Name) { kind = a. Kind; name = a. Name; } public String get. Kind() { return kind; } …
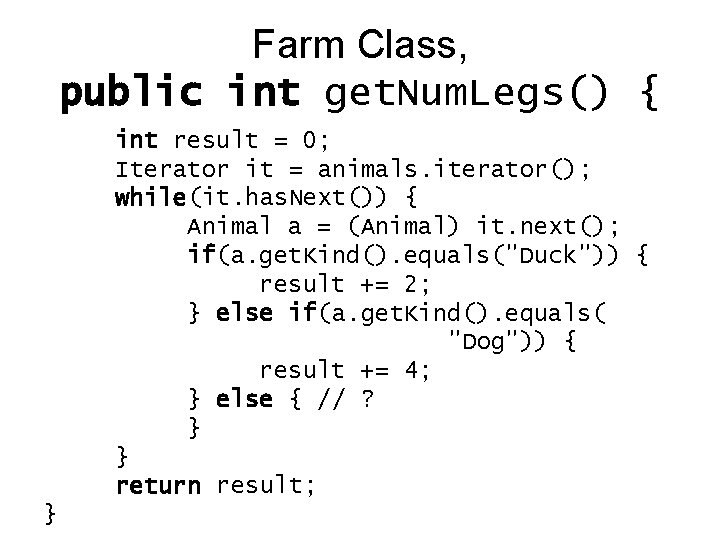
Farm Class, public int get. Num. Legs() { int result = 0; Iterator it = animals. iterator(); while(it. has. Next()) { Animal a = (Animal) it. next(); if(a. get. Kind(). equals("Duck")) { result += 2; } else if(a. get. Kind(). equals( "Dog")) { result += 4; } else { // ? } } return result; }
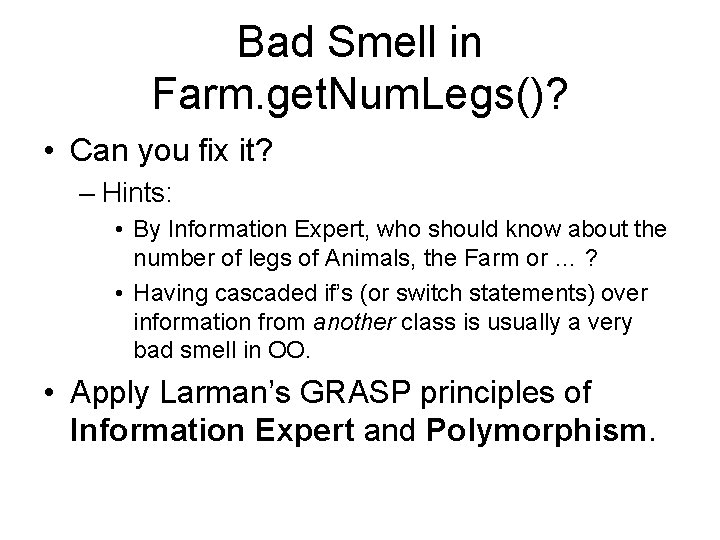
Bad Smell in Farm. get. Num. Legs()? • Can you fix it? – Hints: • By Information Expert, who should know about the number of legs of Animals, the Farm or … ? • Having cascaded if’s (or switch statements) over information from another class is usually a very bad smell in OO. • Apply Larman’s GRASP principles of Information Expert and Polymorphism.
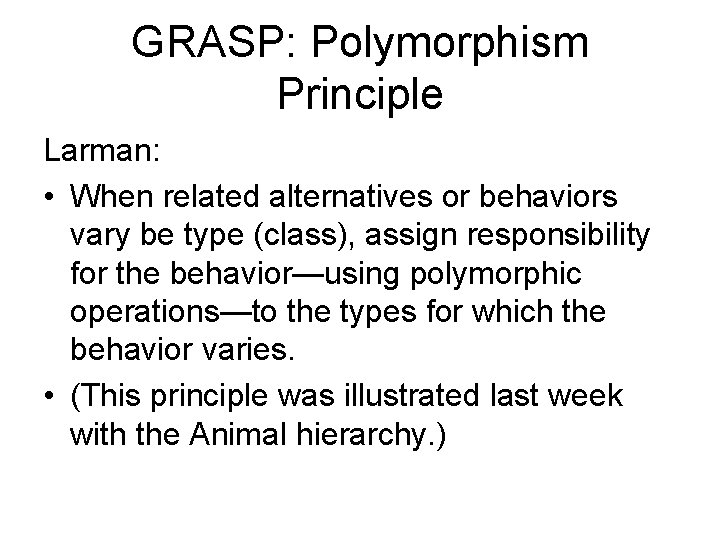
GRASP: Polymorphism Principle Larman: • When related alternatives or behaviors vary be type (class), assign responsibility for the behavior—using polymorphic operations—to the types for which the behavior varies. • (This principle was illustrated last week with the Animal hierarchy. )
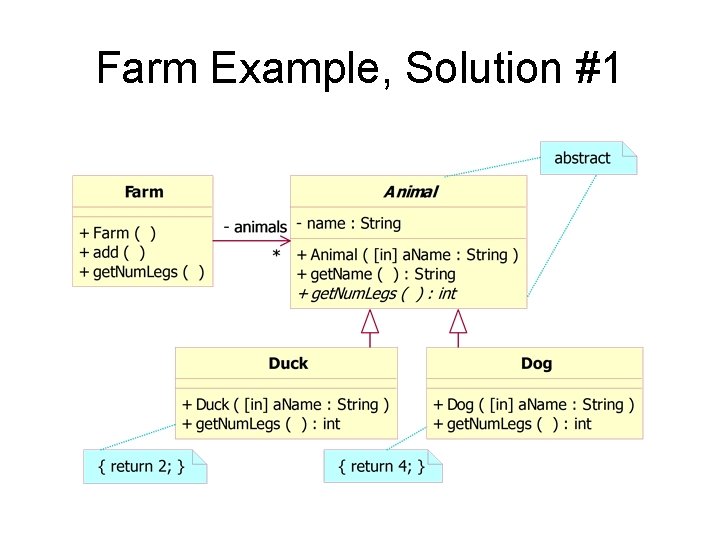
Farm Example, Solution #1
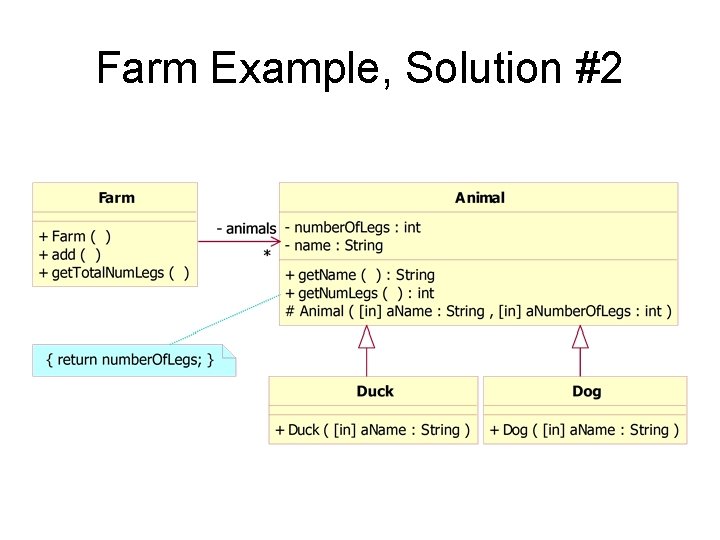
Farm Example, Solution #2
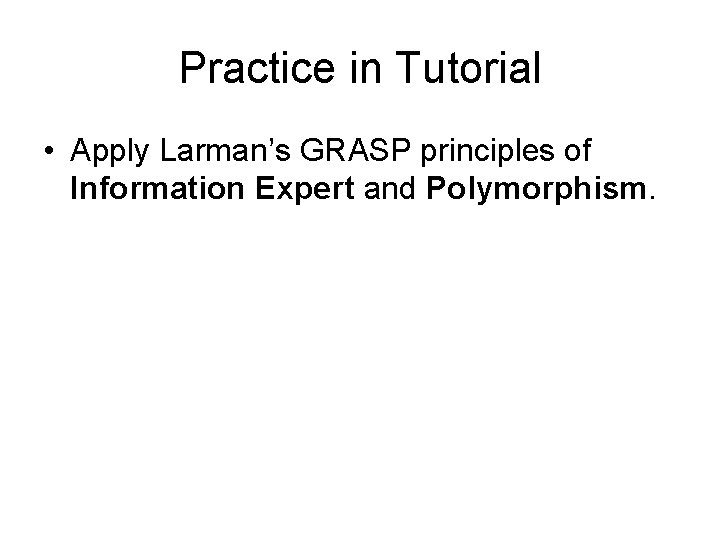
Practice in Tutorial • Apply Larman’s GRASP principles of Information Expert and Polymorphism.
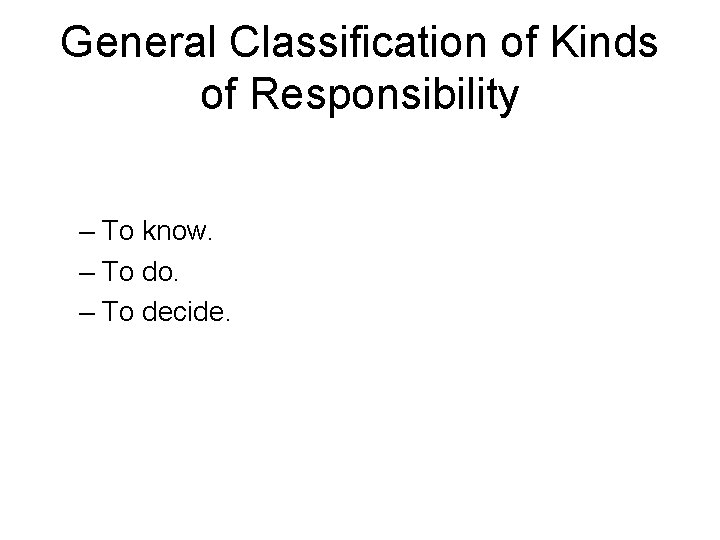
General Classification of Kinds of Responsibility – To know. – To do. – To decide.
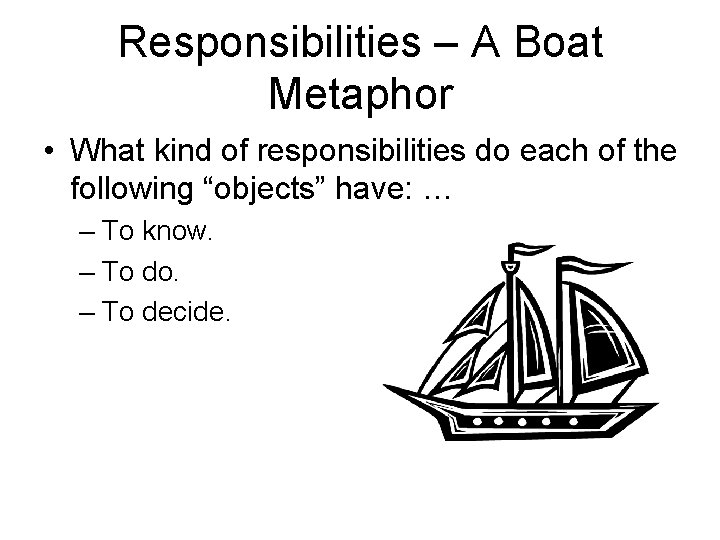
Responsibilities – A Boat Metaphor • What kind of responsibilities do each of the following “objects” have: … – To know. – To do. – To decide.
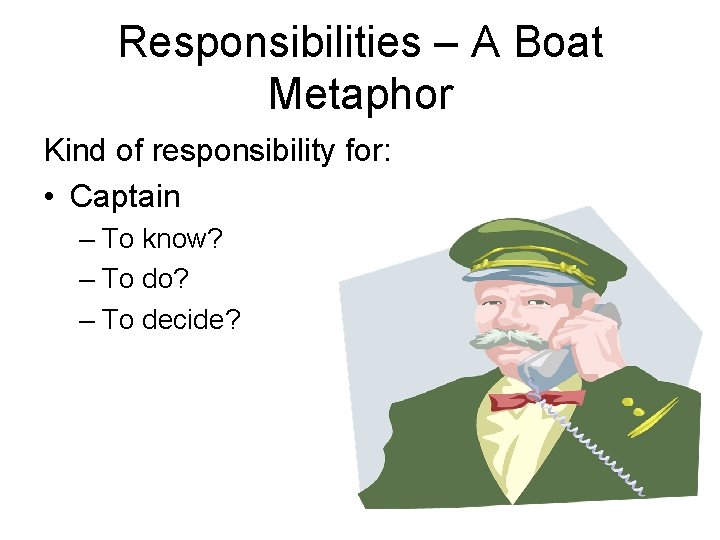
Responsibilities – A Boat Metaphor Kind of responsibility for: • Captain – To know? – To do? – To decide?
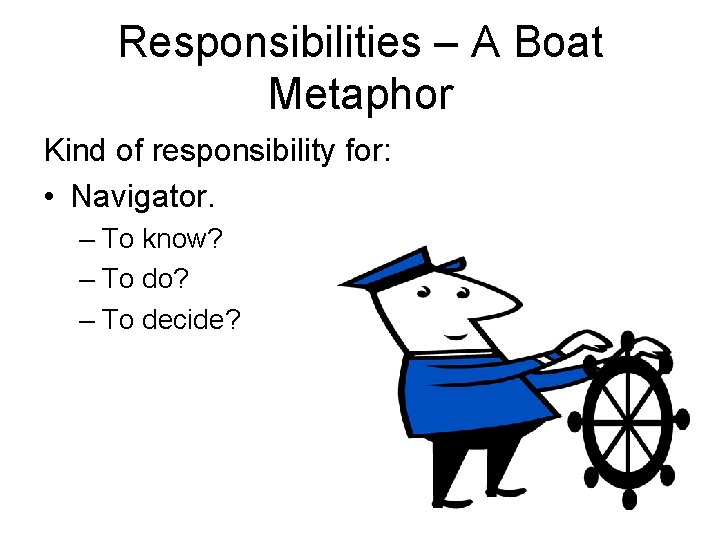
Responsibilities – A Boat Metaphor Kind of responsibility for: • Navigator. – To know? – To do? – To decide?
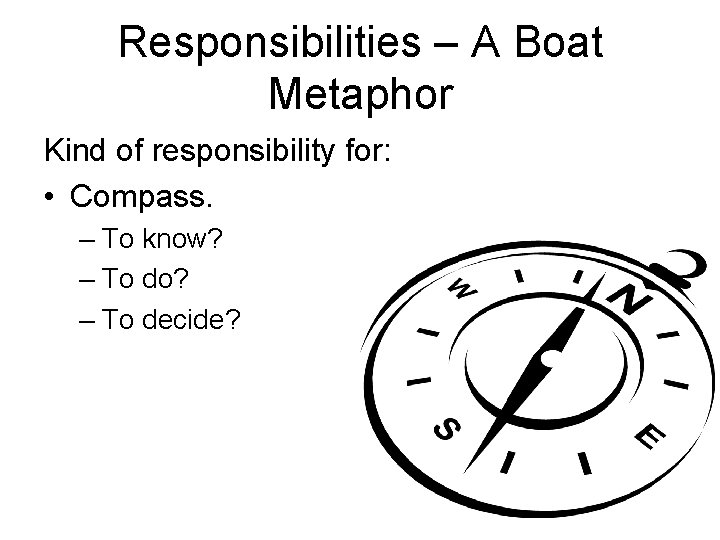
Responsibilities – A Boat Metaphor Kind of responsibility for: • Compass. – To know? – To do? – To decide?
Soen 385
Soen 343
Soen 343
Soen 343
Soen 6441 concordia
Soen 6441
Soen 6441
Soen 385
Soen 6441
Soen 342
Csc343
Texas health and safety code 343
Log k = log a - ea/rt
Experiment 343
Cecs 343
Descompunerea lui 343
X3 = 64/343
Cs 343
343 day
Log 343
343*15
343 jesus
Ds renkema
582 x 4
Cecs 343
Csc 343
Gcf of 64 and 343
Real time software design in software engineering
Software design fundamentals in software engineering
What line types are usually omitted from sectional views?
Removed section view drawing
Half section line
Chemical potential energy images
Chapter 10 section 1 meiosis worksheet answer key
A real friend 2006
Sentencia c-355 de 2006
Pengiktirafan ukm 2006
Monarch awards 2006
Syawal 2006
T. trimpe 2006 http //sciencespot.net/
Mercedes quesada etxaide
Sentencia c-355 de 2006 imagenes