SOEN 343 Software Design Computer Science and Software
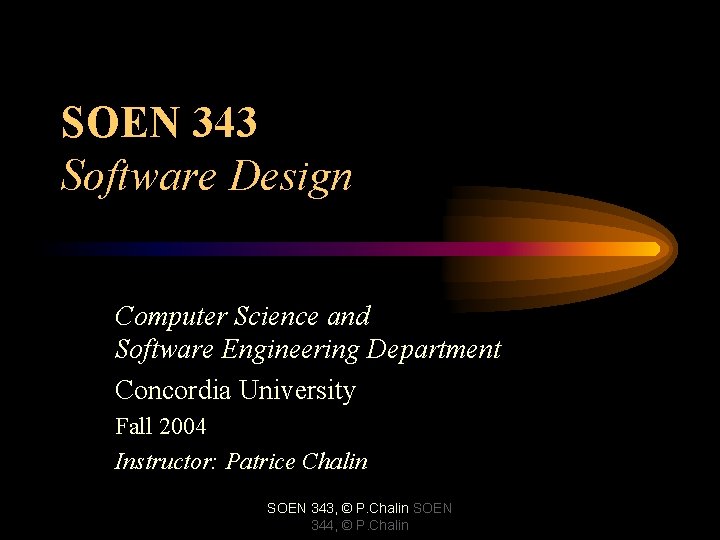
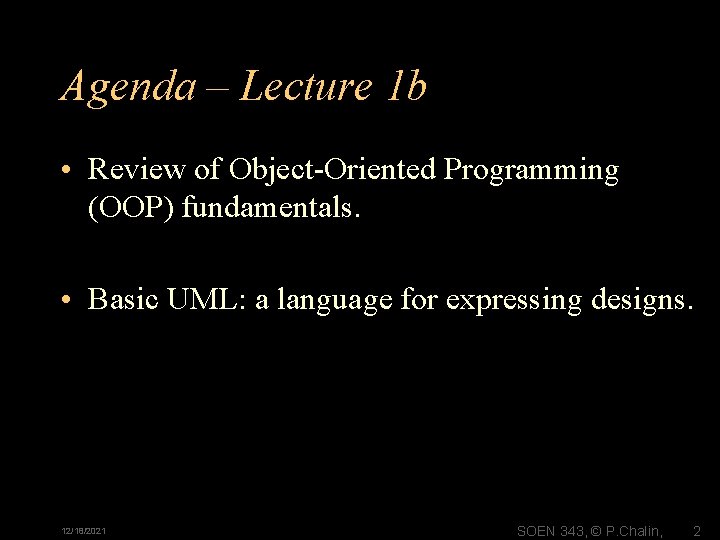
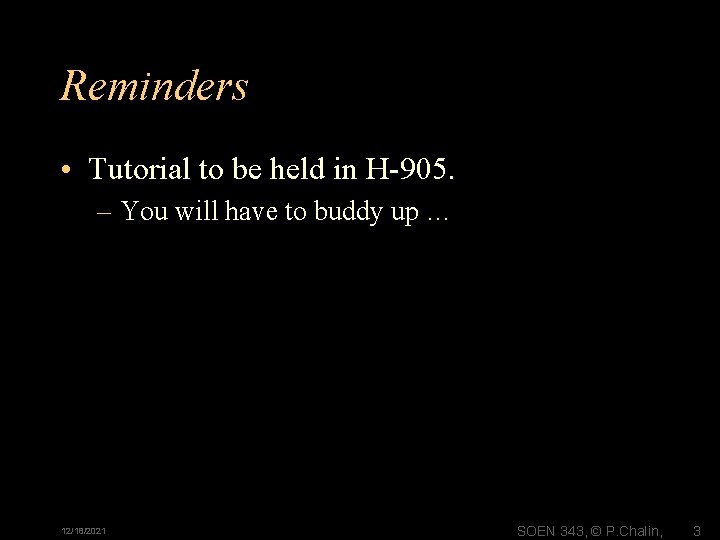
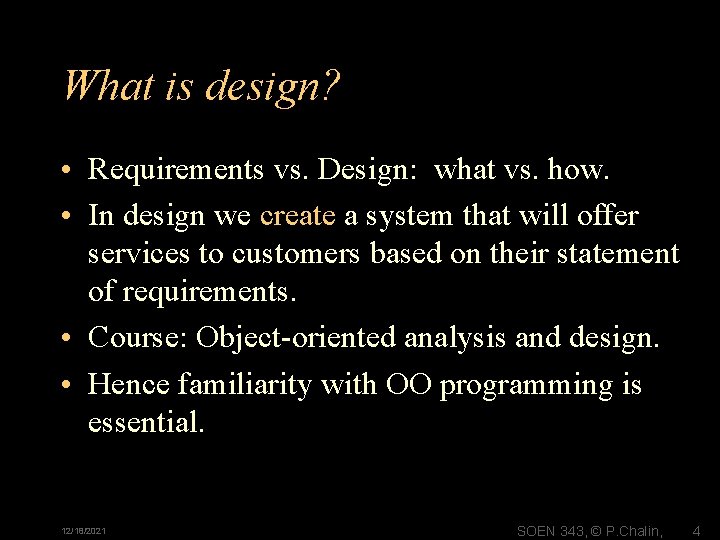
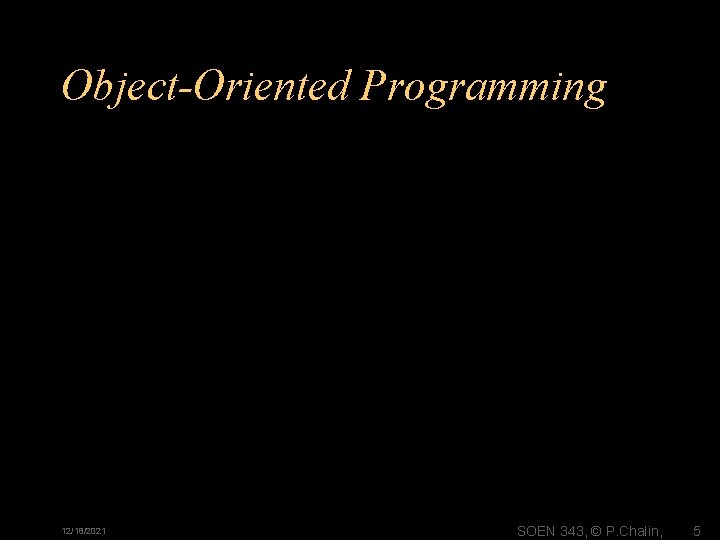
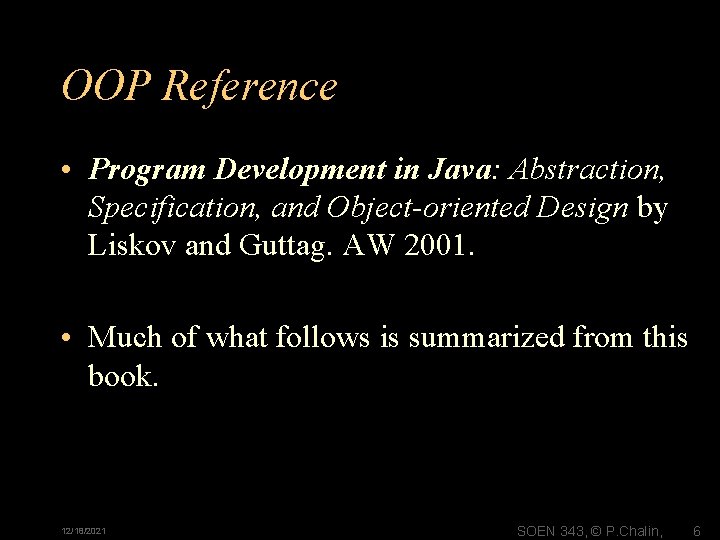
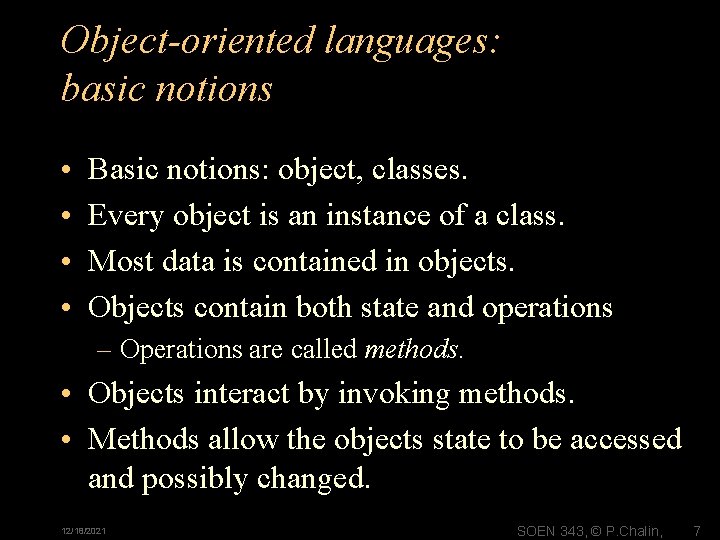
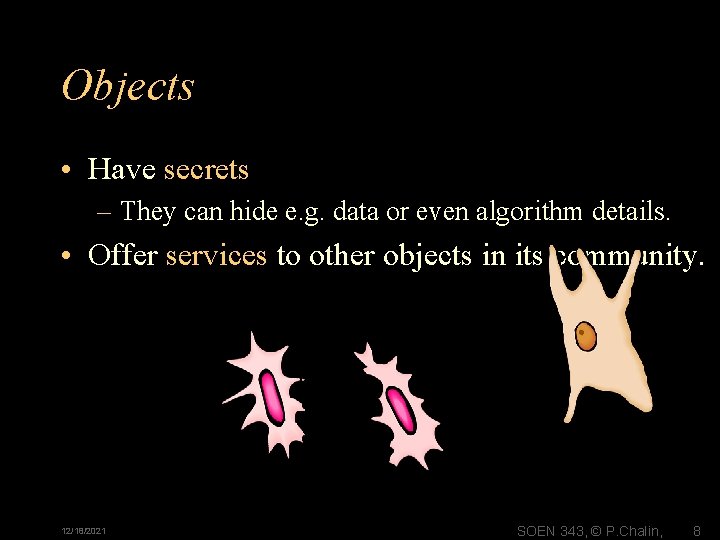
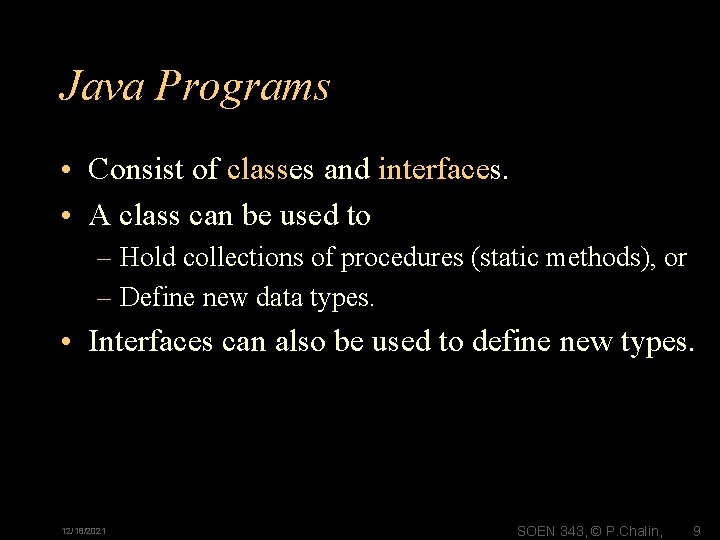
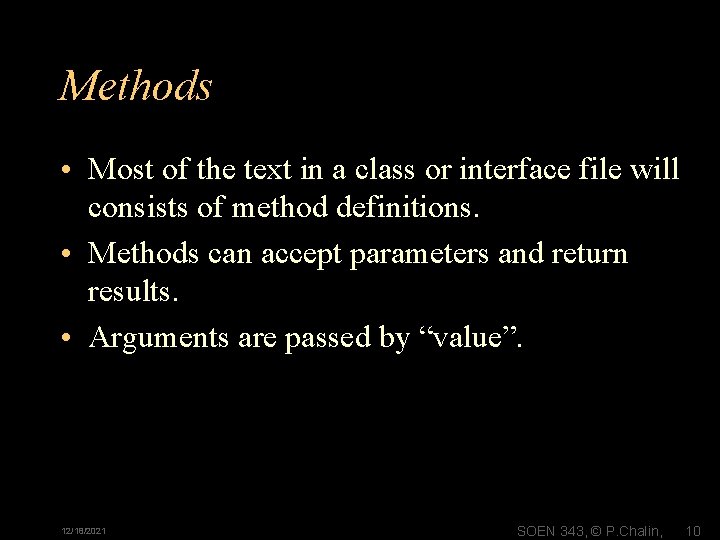
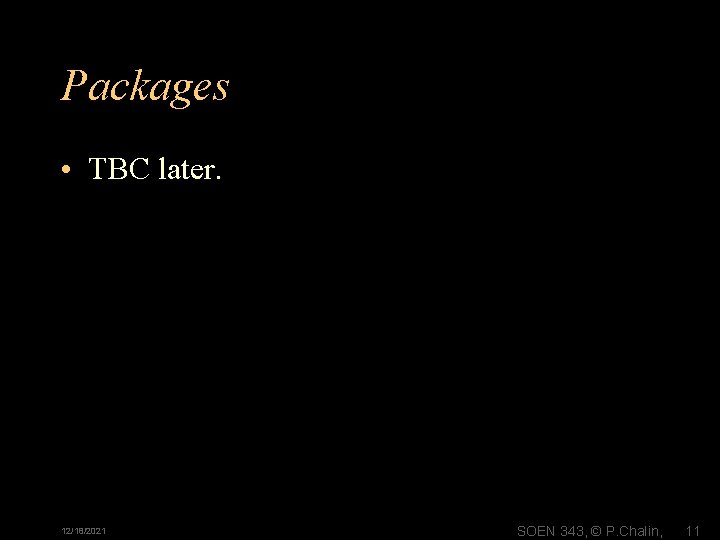
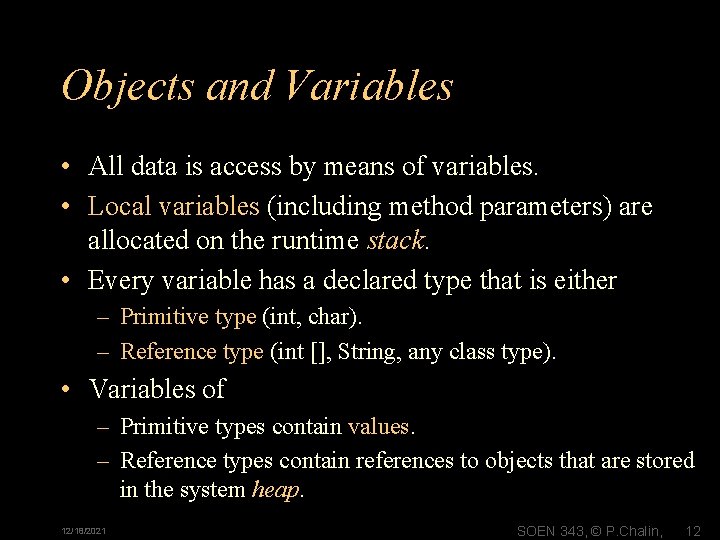
![Stack and Heap Stack Heap i j a b [2, 1, 0] 3 s Stack and Heap Stack Heap i j a b [2, 1, 0] 3 s](https://slidetodoc.com/presentation_image_h2/179b3b34d05f3585530f0925c6d5453c/image-13.jpg)
![Stack and Heap: Exercise • Given the following declarations int int[] String i = Stack and Heap: Exercise • Given the following declarations int int[] String i =](https://slidetodoc.com/presentation_image_h2/179b3b34d05f3585530f0925c6d5453c/image-14.jpg)
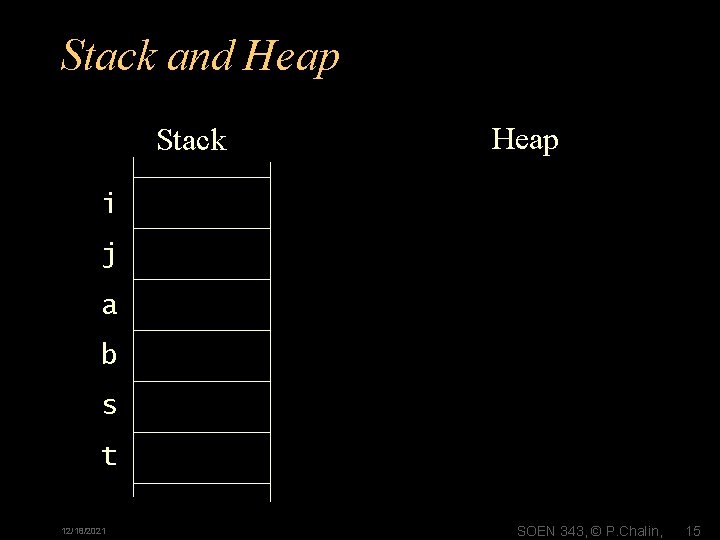
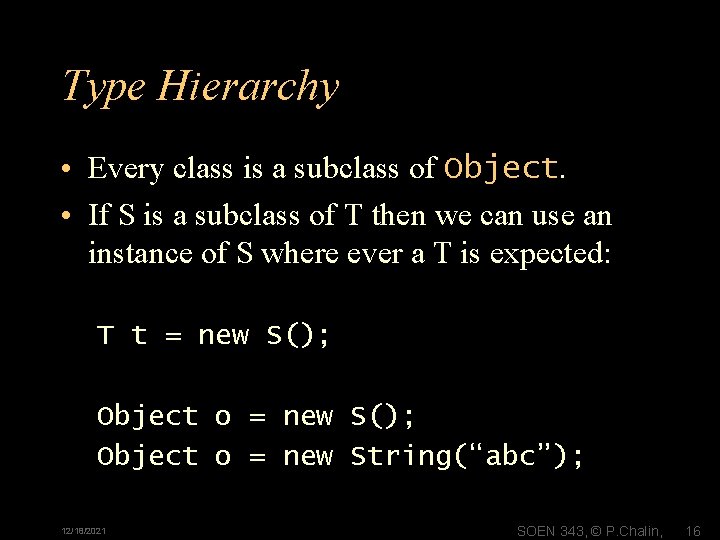
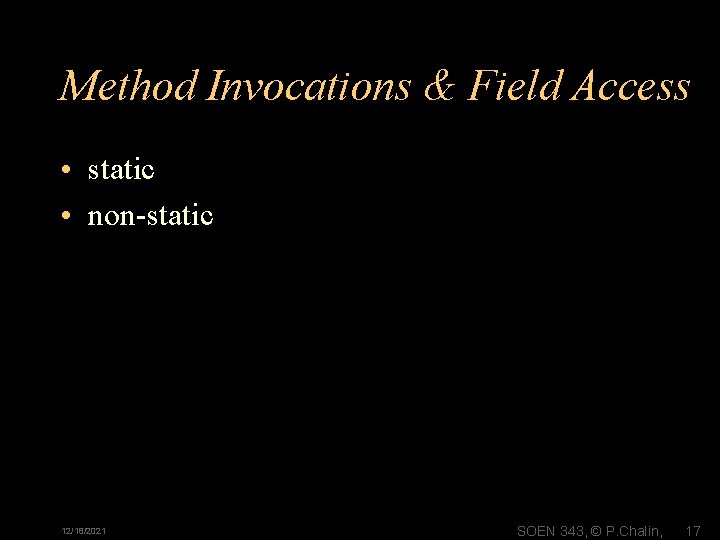
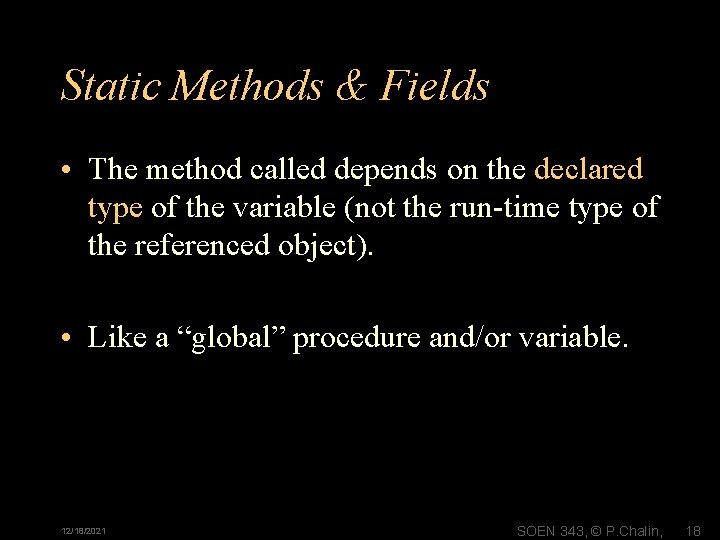
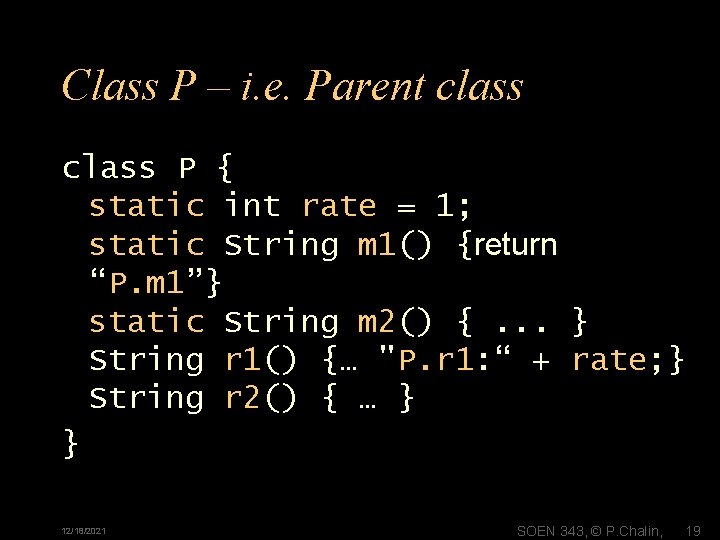
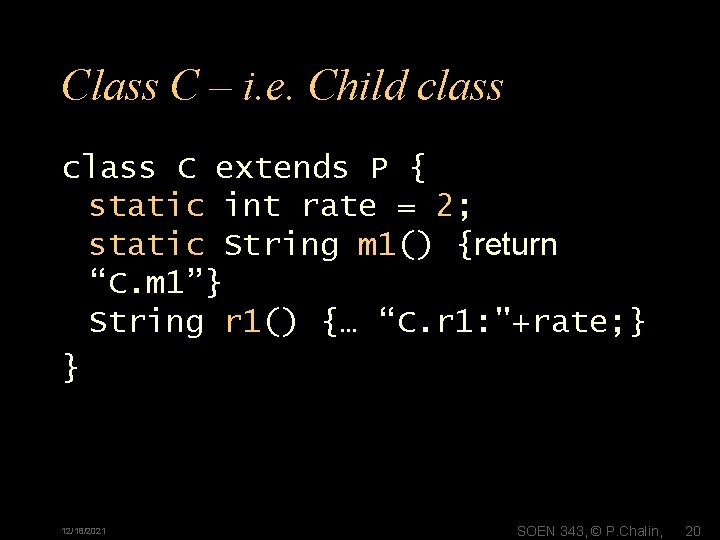
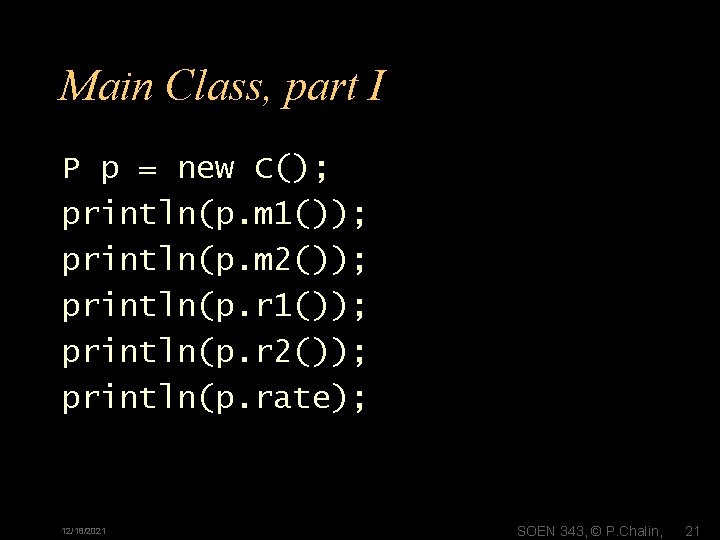
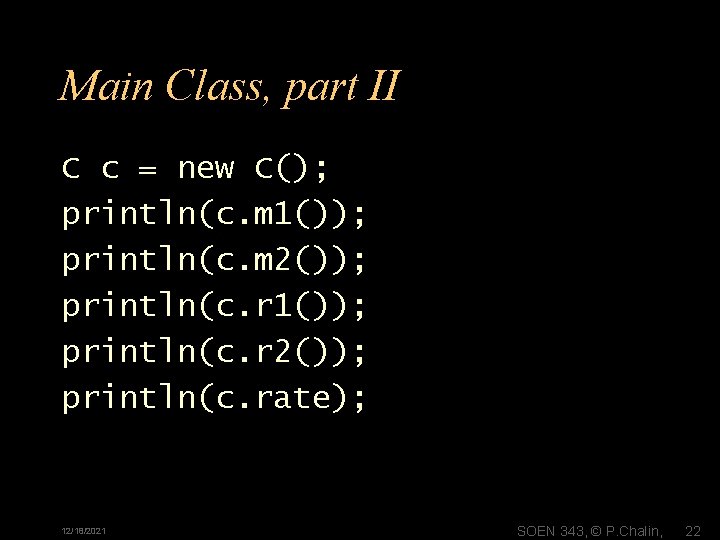
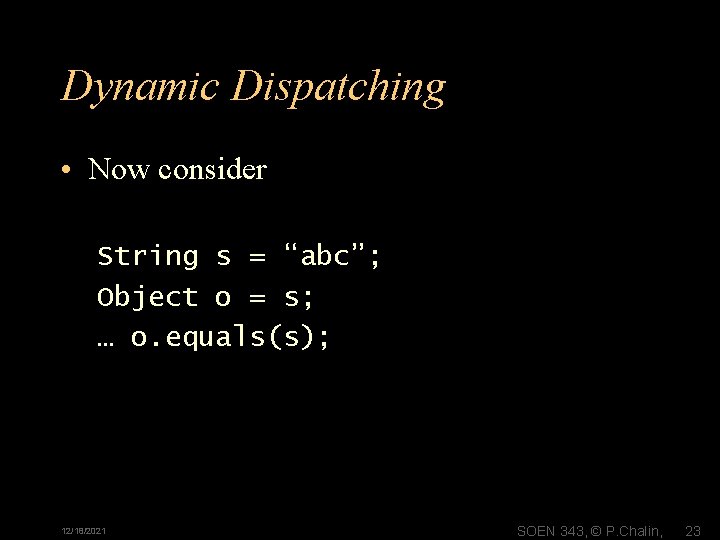
- Slides: 23
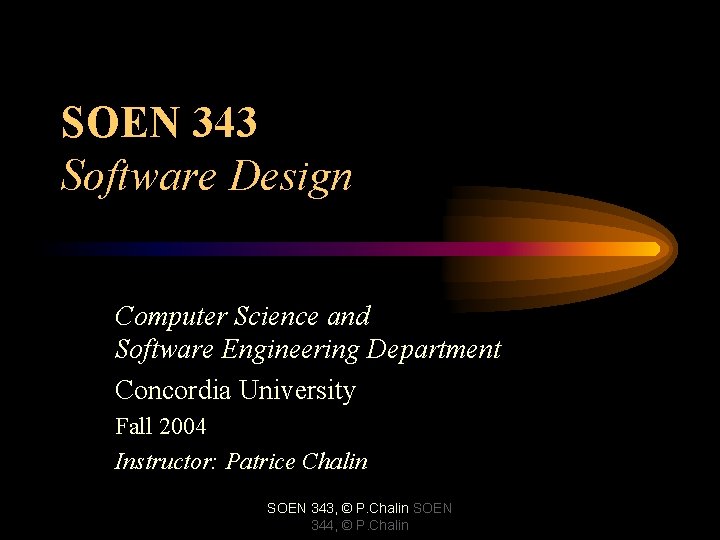
SOEN 343 Software Design Computer Science and Software Engineering Department Concordia University Fall 2004 Instructor: Patrice Chalin SOEN 343, © P. Chalin SOEN 344, © P. Chalin
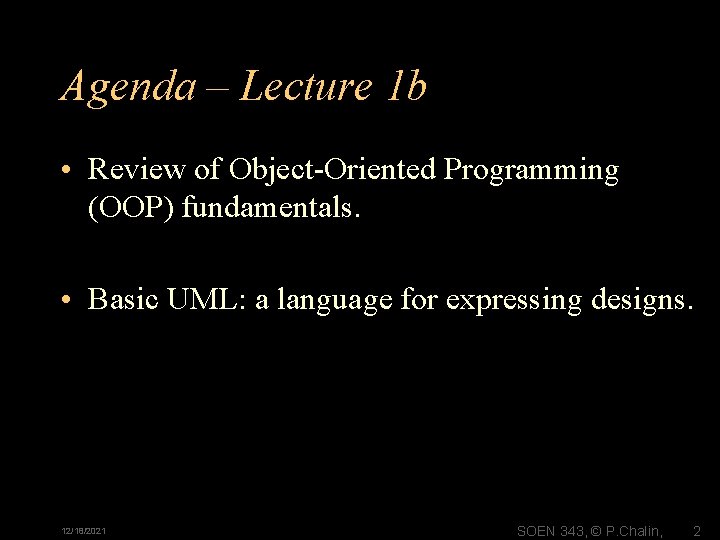
Agenda – Lecture 1 b • Review of Object-Oriented Programming (OOP) fundamentals. • Basic UML: a language for expressing designs. 12/18/2021 SOEN 343, © P. Chalin, 2
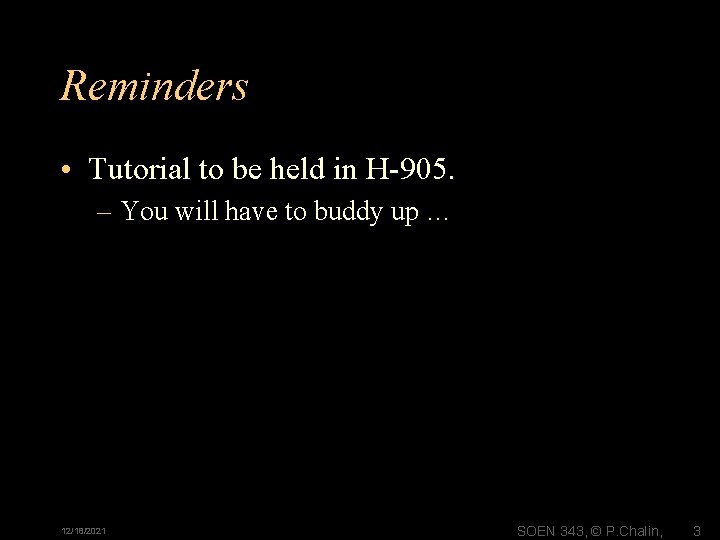
Reminders • Tutorial to be held in H-905. – You will have to buddy up … 12/18/2021 SOEN 343, © P. Chalin, 3
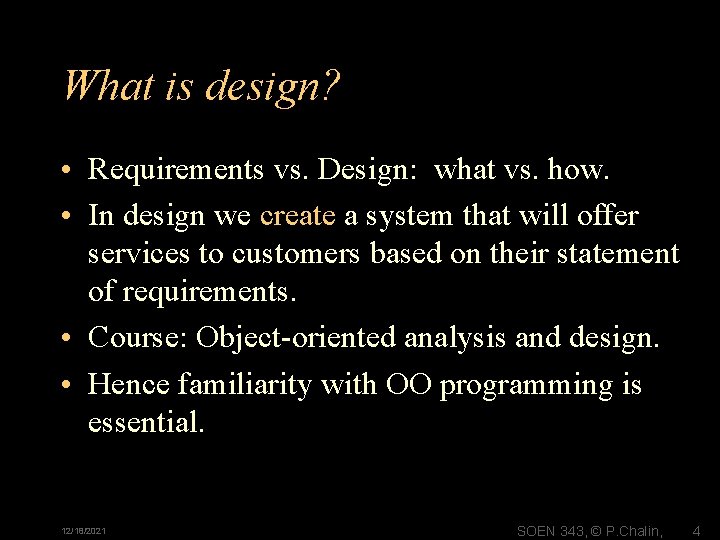
What is design? • Requirements vs. Design: what vs. how. • In design we create a system that will offer services to customers based on their statement of requirements. • Course: Object-oriented analysis and design. • Hence familiarity with OO programming is essential. 12/18/2021 SOEN 343, © P. Chalin, 4
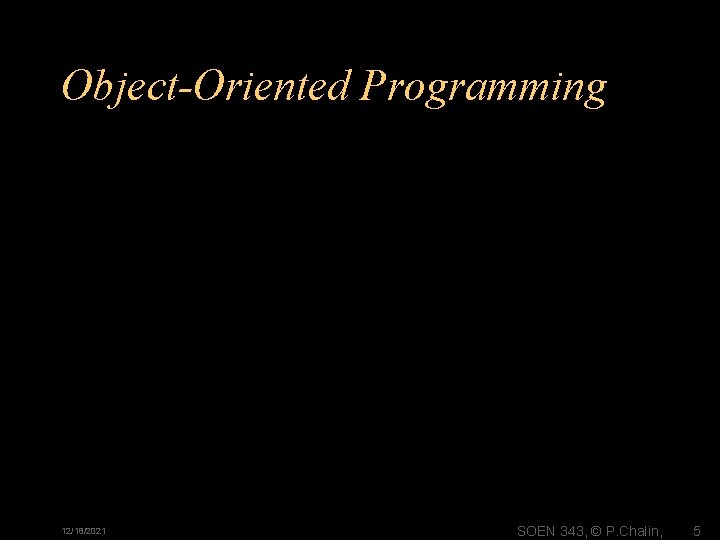
Object-Oriented Programming 12/18/2021 SOEN 343, © P. Chalin, 5
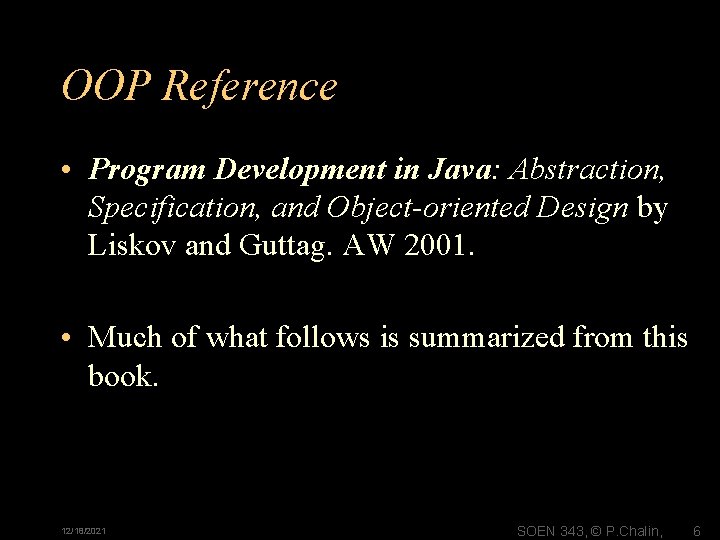
OOP Reference • Program Development in Java: Abstraction, Specification, and Object-oriented Design by Liskov and Guttag. AW 2001. • Much of what follows is summarized from this book. 12/18/2021 SOEN 343, © P. Chalin, 6
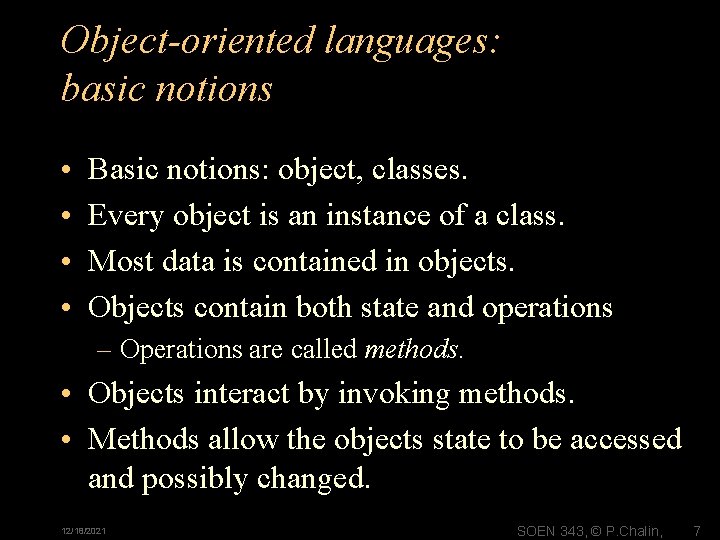
Object-oriented languages: basic notions • • Basic notions: object, classes. Every object is an instance of a class. Most data is contained in objects. Objects contain both state and operations – Operations are called methods. • Objects interact by invoking methods. • Methods allow the objects state to be accessed and possibly changed. 12/18/2021 SOEN 343, © P. Chalin, 7
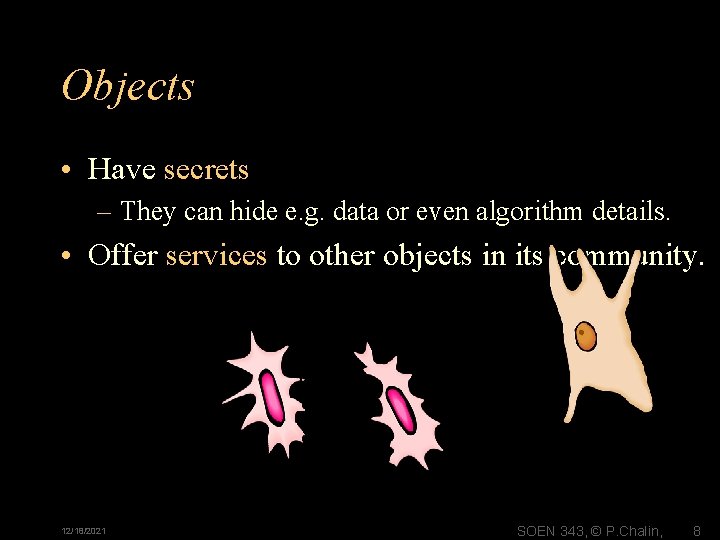
Objects • Have secrets – They can hide e. g. data or even algorithm details. • Offer services to other objects in its community. 12/18/2021 SOEN 343, © P. Chalin, 8
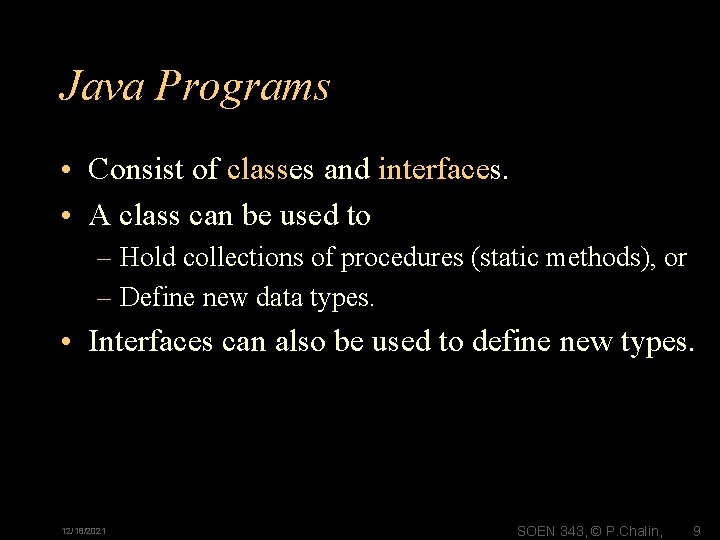
Java Programs • Consist of classes and interfaces. • A class can be used to – Hold collections of procedures (static methods), or – Define new data types. • Interfaces can also be used to define new types. 12/18/2021 SOEN 343, © P. Chalin, 9
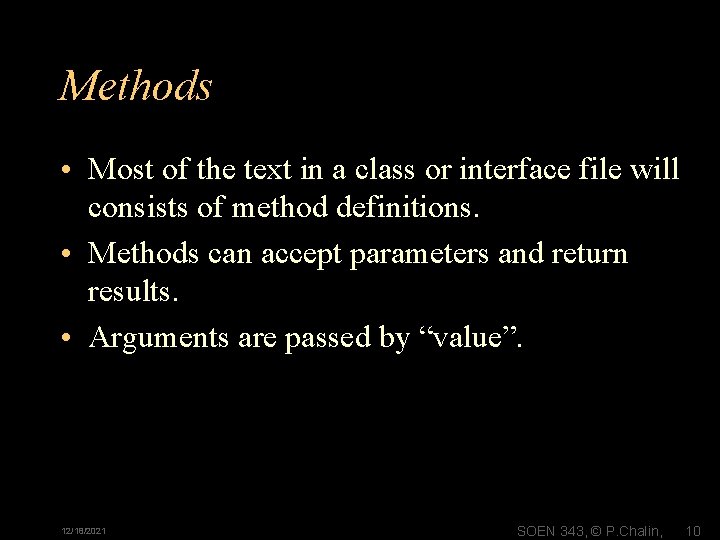
Methods • Most of the text in a class or interface file will consists of method definitions. • Methods can accept parameters and return results. • Arguments are passed by “value”. 12/18/2021 SOEN 343, © P. Chalin, 10
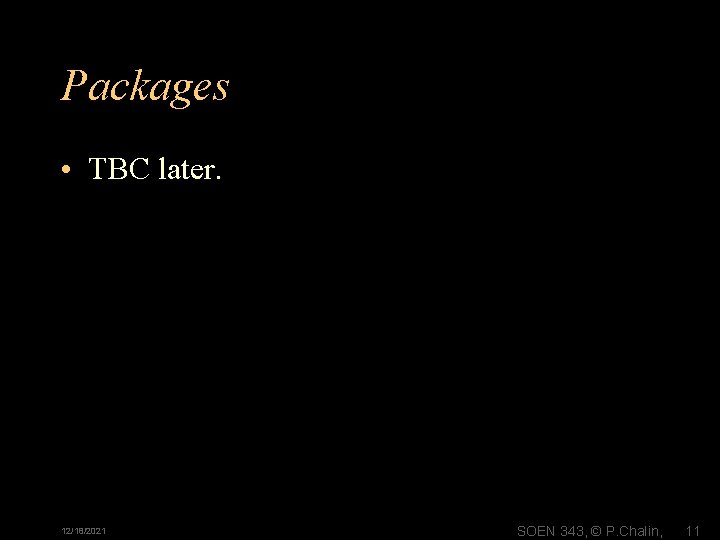
Packages • TBC later. 12/18/2021 SOEN 343, © P. Chalin, 11
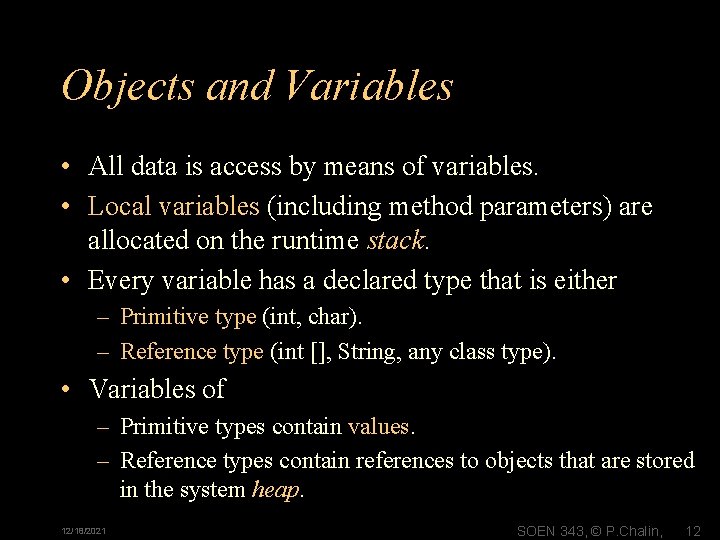
Objects and Variables • All data is access by means of variables. • Local variables (including method parameters) are allocated on the runtime stack. • Every variable has a declared type that is either – Primitive type (int, char). – Reference type (int [], String, any class type). • Variables of – Primitive types contain values. – Reference types contain references to objects that are stored in the system heap. 12/18/2021 SOEN 343, © P. Chalin, 12
![Stack and Heap Stack Heap i j a b 2 1 0 3 s Stack and Heap Stack Heap i j a b [2, 1, 0] 3 s](https://slidetodoc.com/presentation_image_h2/179b3b34d05f3585530f0925c6d5453c/image-13.jpg)
Stack and Heap Stack Heap i j a b [2, 1, 0] 3 s t 12/18/2021 SOEN 343, © P. Chalin, 13
![Stack and Heap Exercise Given the following declarations int int String i Stack and Heap: Exercise • Given the following declarations int int[] String i =](https://slidetodoc.com/presentation_image_h2/179b3b34d05f3585530f0925c6d5453c/image-14.jpg)
Stack and Heap: Exercise • Given the following declarations int int[] String i = j; a[] b = s = t = 6; = {1, 2, 3}; new int[2]; “abc”; null; • what will be the state of the stack and heap after they have all been processed? 12/18/2021 SOEN 343, © P. Chalin, 14
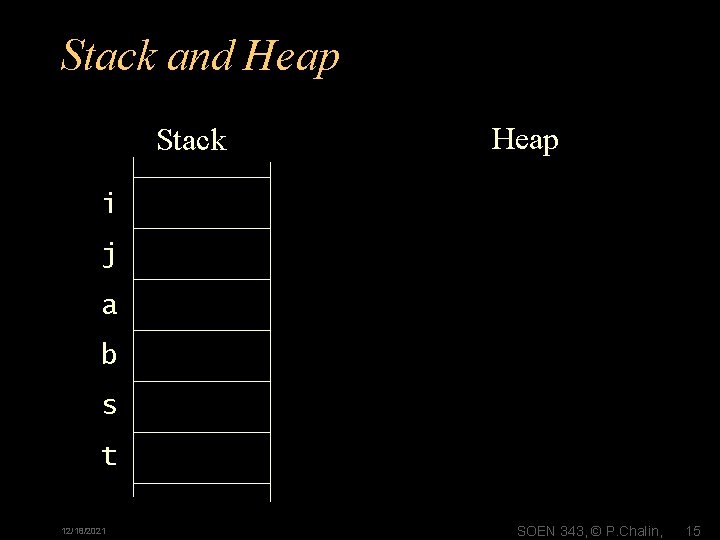
Stack and Heap Stack Heap i j a b s t 12/18/2021 SOEN 343, © P. Chalin, 15
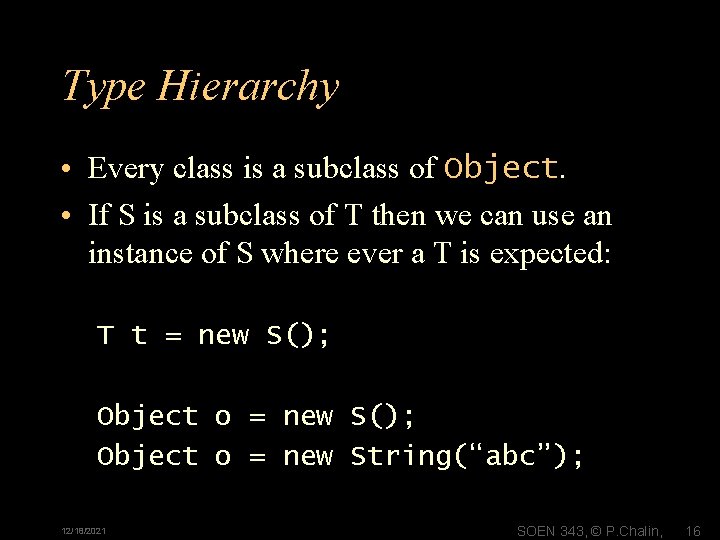
Type Hierarchy • Every class is a subclass of Object. • If S is a subclass of T then we can use an instance of S where ever a T is expected: T t = new S(); Object o = new String(“abc”); 12/18/2021 SOEN 343, © P. Chalin, 16
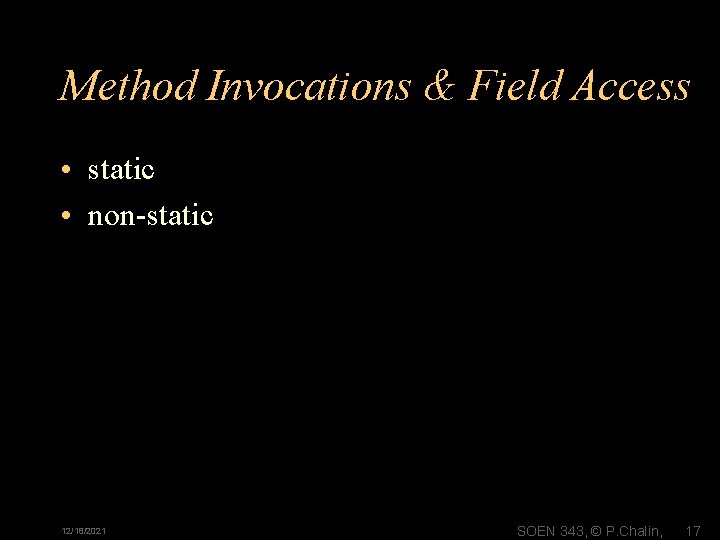
Method Invocations & Field Access • static • non-static 12/18/2021 SOEN 343, © P. Chalin, 17
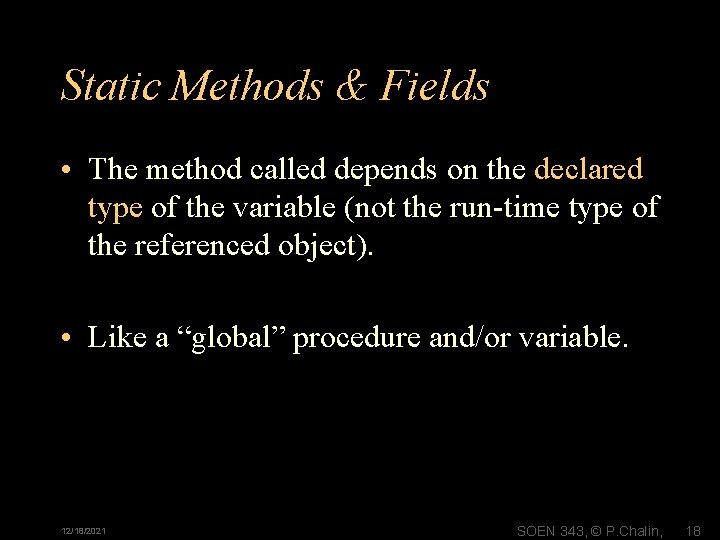
Static Methods & Fields • The method called depends on the declared type of the variable (not the run-time type of the referenced object). • Like a “global” procedure and/or variable. 12/18/2021 SOEN 343, © P. Chalin, 18
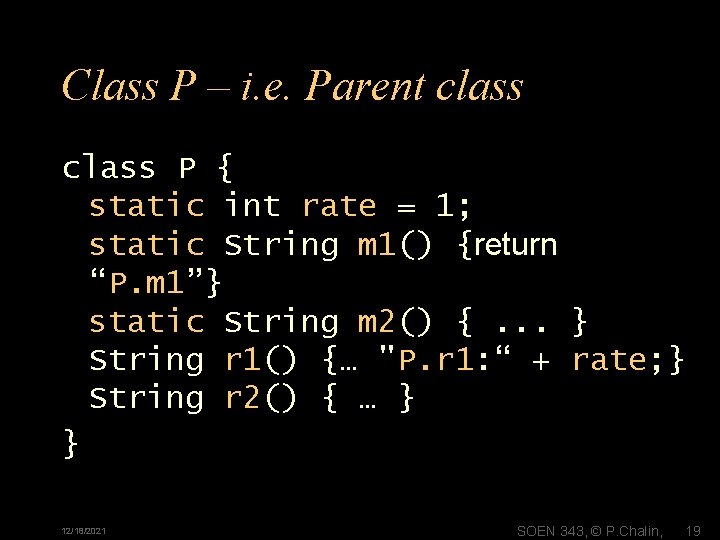
Class P – i. e. Parent class P { static int rate = 1; static String m 1() {return “P. m 1”} static String m 2() {. . . } String r 1() {… "P. r 1: “ + rate; } String r 2() { … } } 12/18/2021 SOEN 343, © P. Chalin, 19
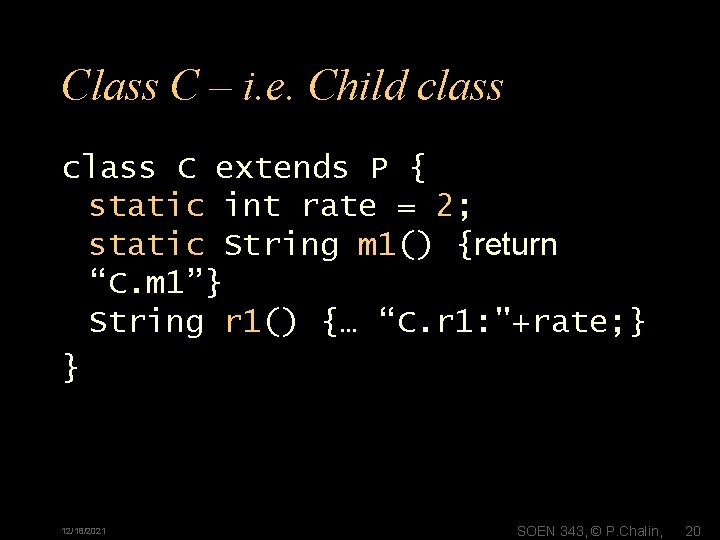
Class C – i. e. Child class C extends P { static int rate = 2; static String m 1() {return “C. m 1”} String r 1() {… “C. r 1: "+rate; } } 12/18/2021 SOEN 343, © P. Chalin, 20
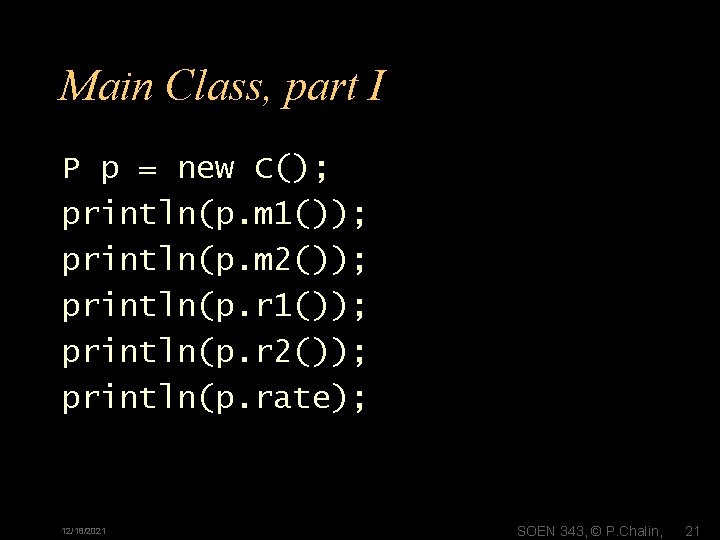
Main Class, part I P p = new C(); println(p. m 1()); println(p. m 2()); println(p. r 1()); println(p. r 2()); println(p. rate); 12/18/2021 SOEN 343, © P. Chalin, 21
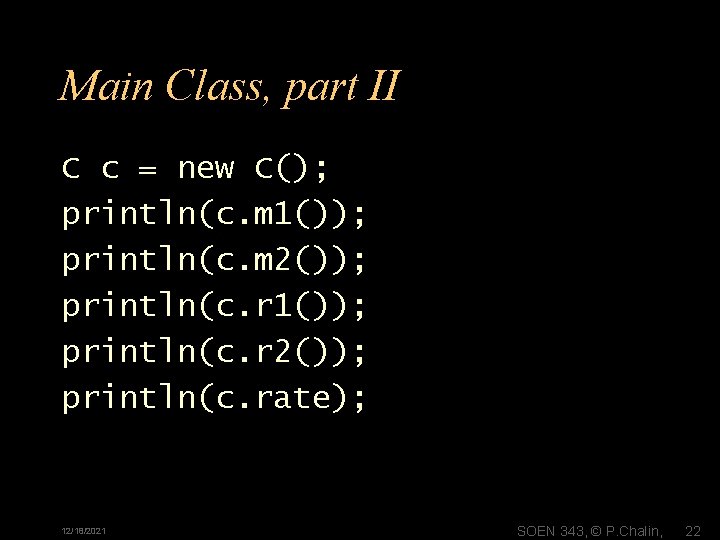
Main Class, part II C c = new C(); println(c. m 1()); println(c. m 2()); println(c. r 1()); println(c. r 2()); println(c. rate); 12/18/2021 SOEN 343, © P. Chalin, 22
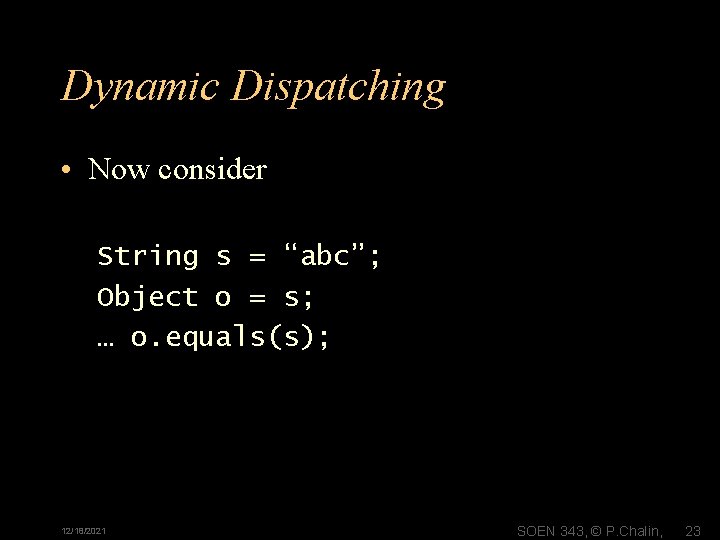
Dynamic Dispatching • Now consider String s = “abc”; Object o = s; … o. equals(s); 12/18/2021 SOEN 343, © P. Chalin, 23
Soen 385
Soen 343
Soen 343
Soen 343
Texas health and safety code 343
Special product formulas
Soen 6441
Soen 6441
Soen 6441
Soen 385
Soen 6441
Soen 342
As a child which subjects of science was your favourite
Csc 343
Log k = log a - ea/rt
Experiment 343
Cecs 343
Descompunerea lui 343
Cube root of 64/343
Cs 343
343 day
Log 343
343 pro session
343 jesus