SOEN 343 Software Design Computer Science and Software
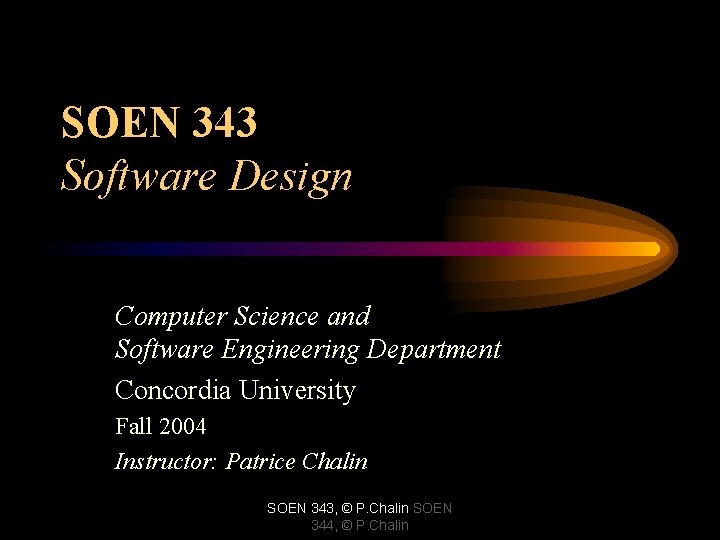
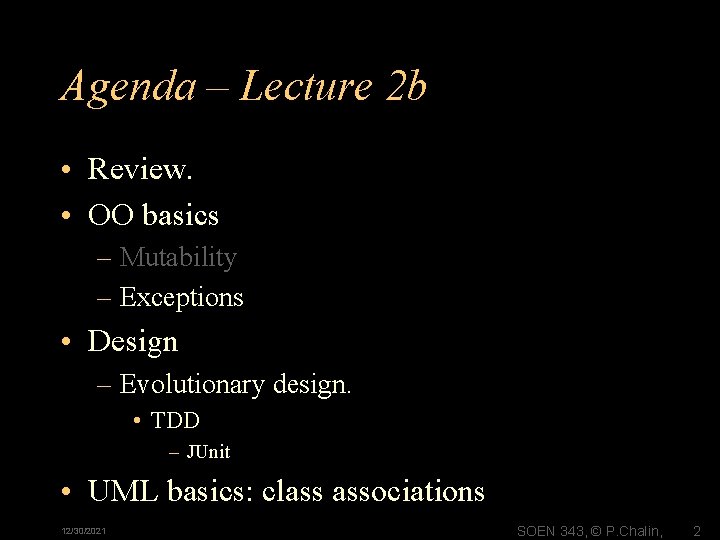
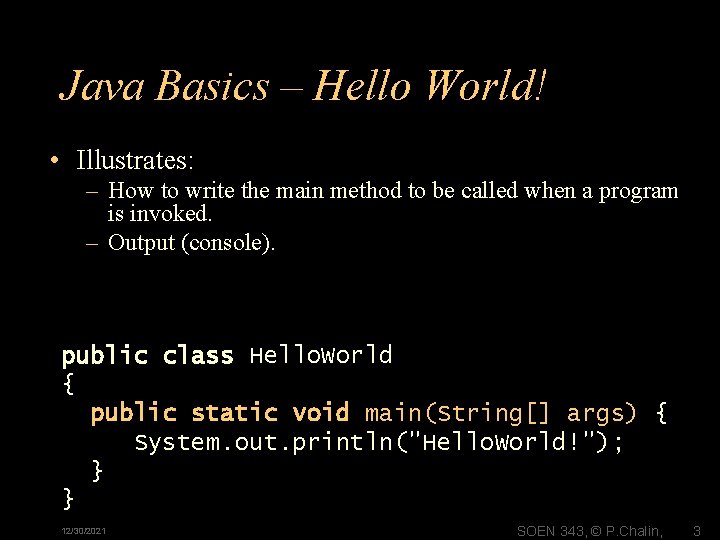
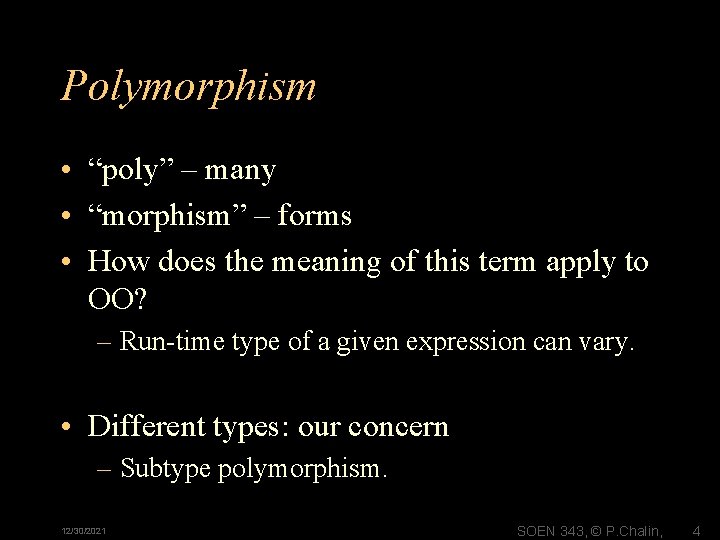
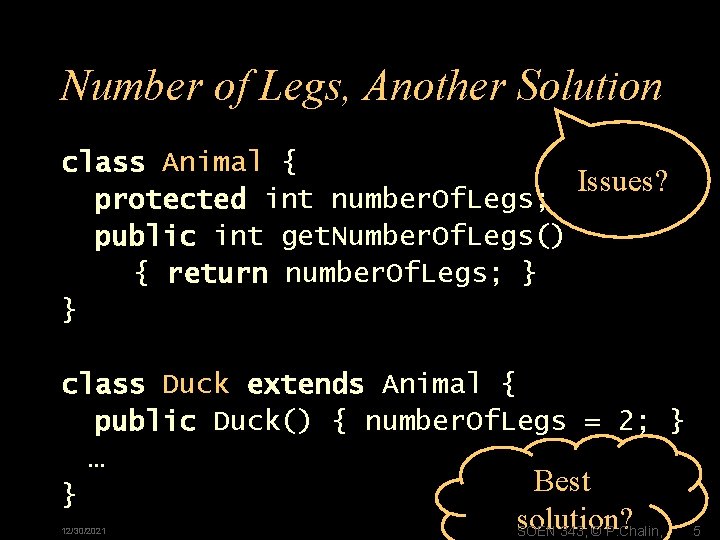
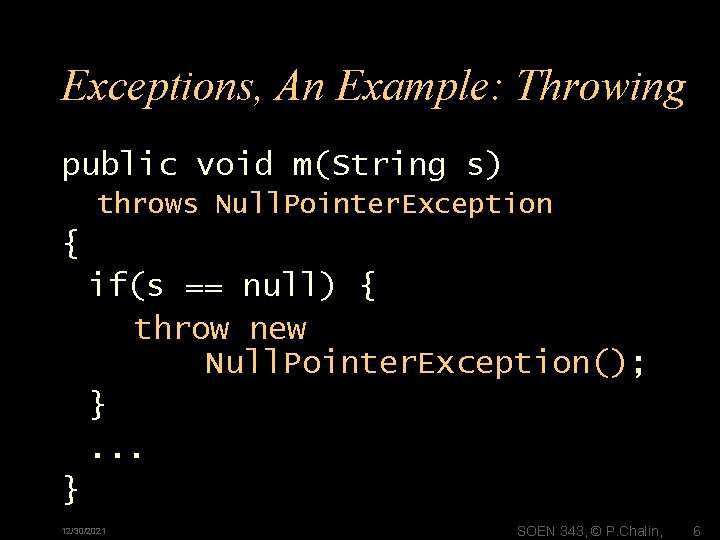
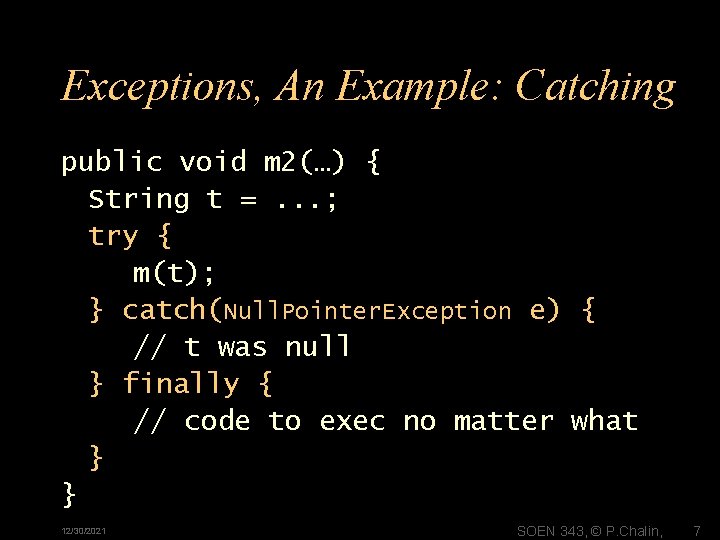
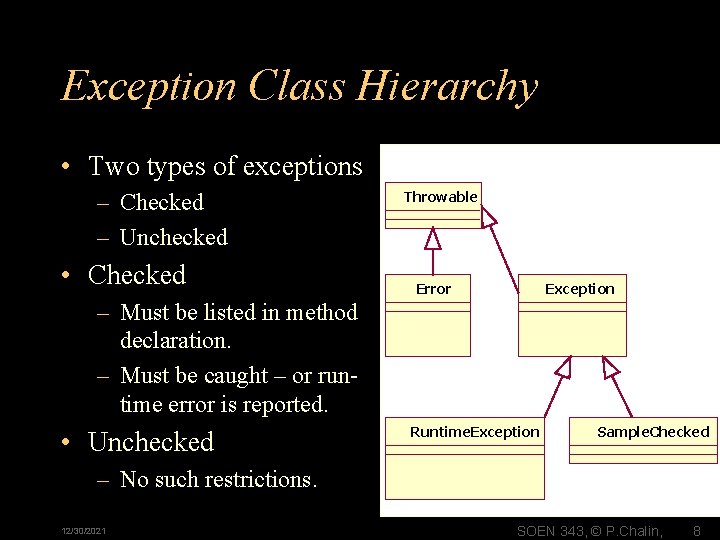
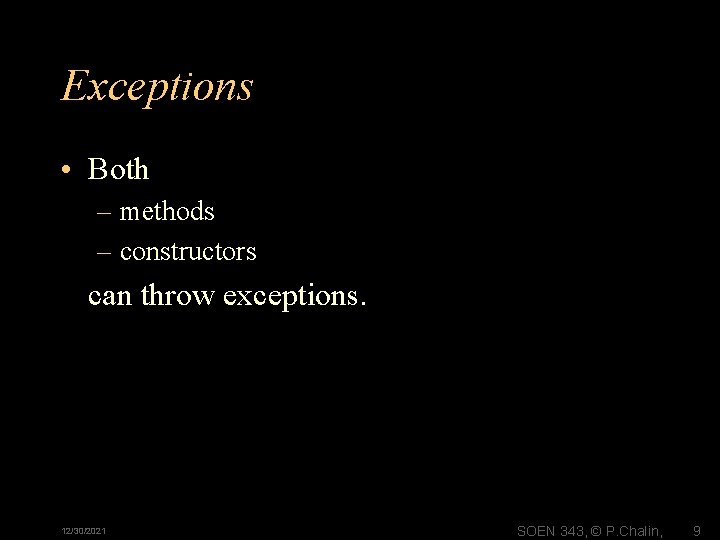
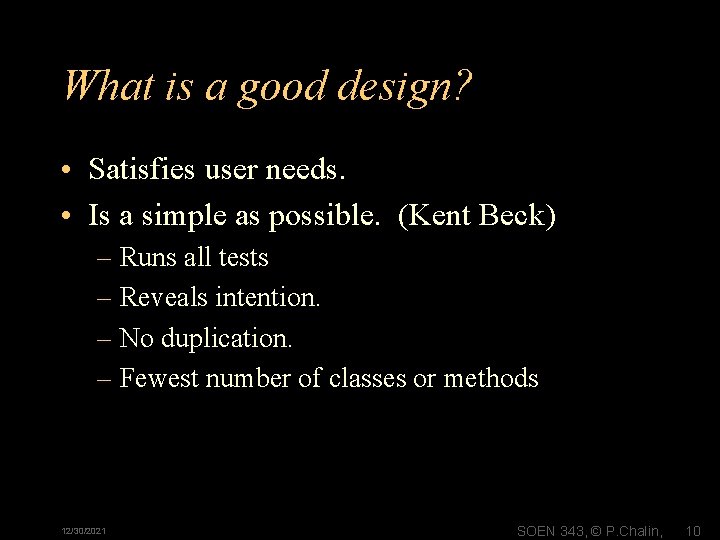
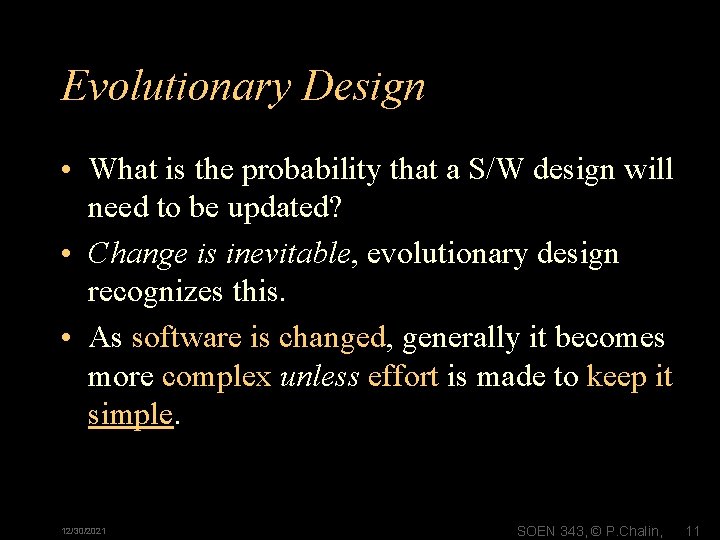
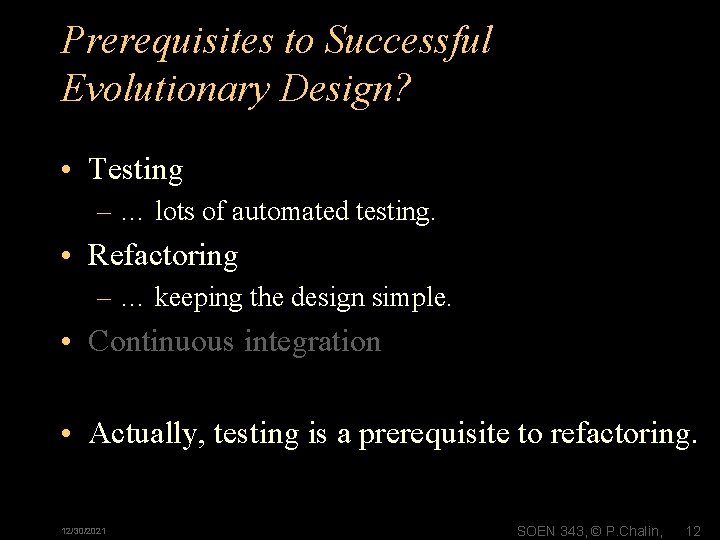
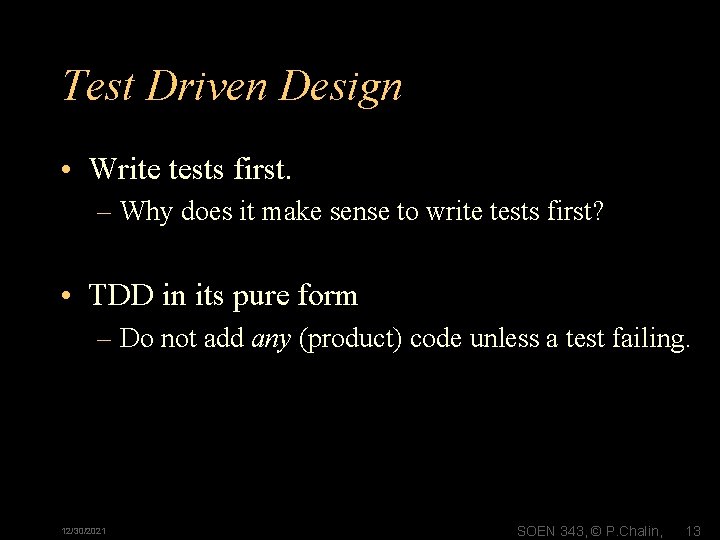
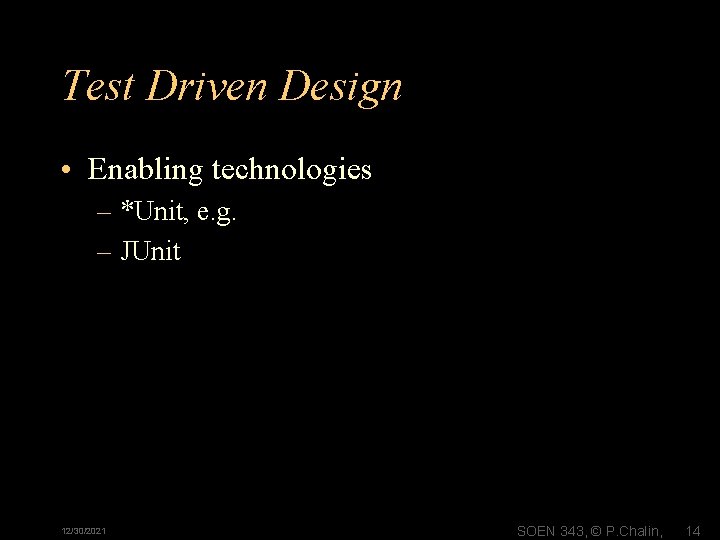
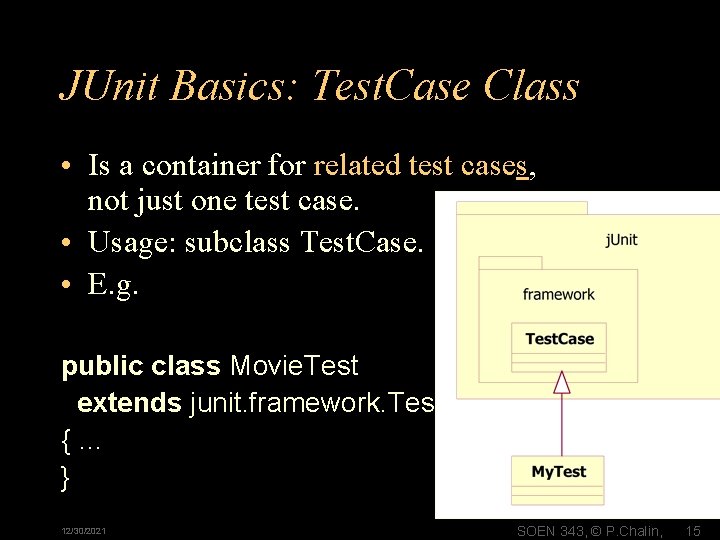
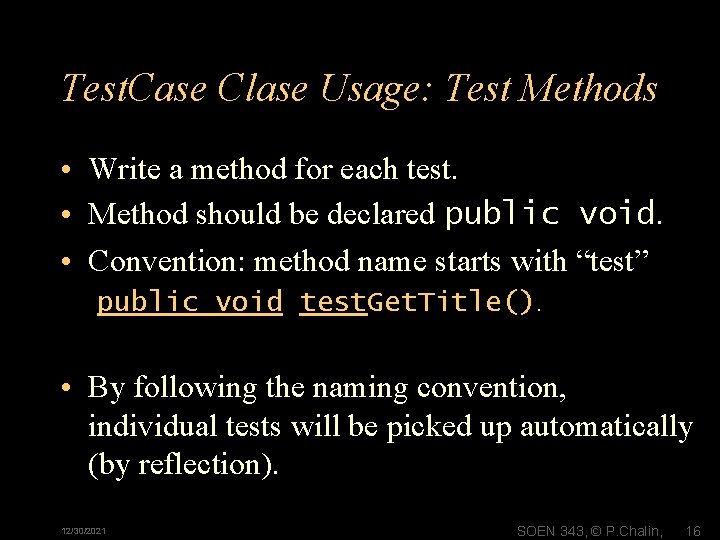
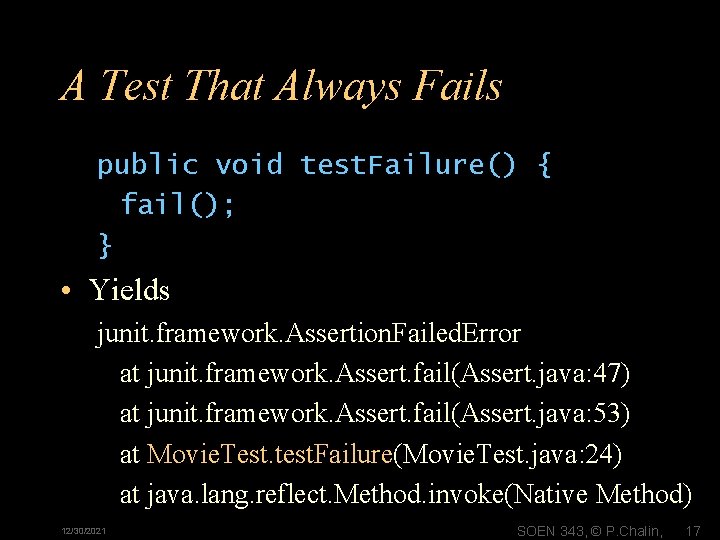
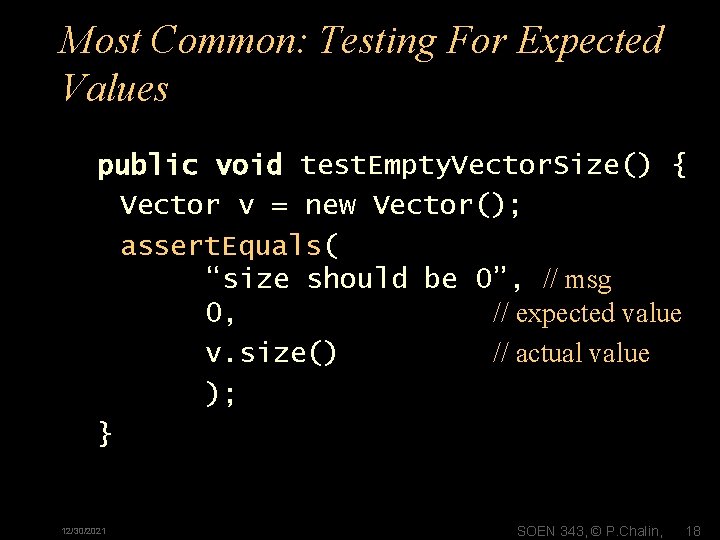
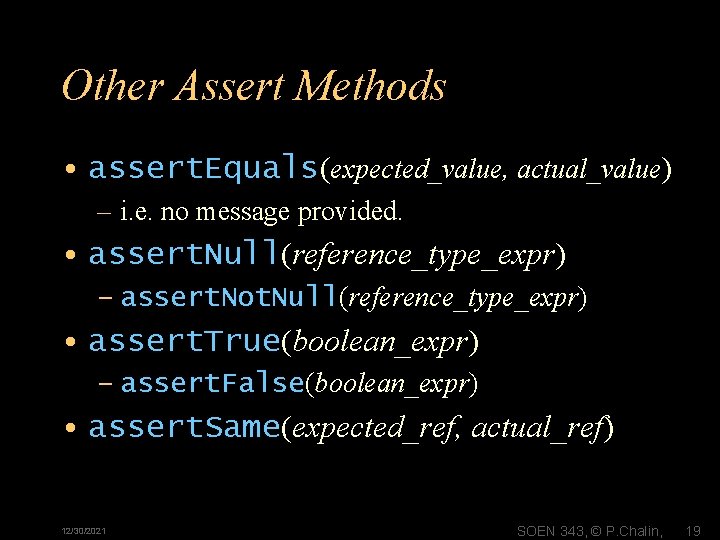
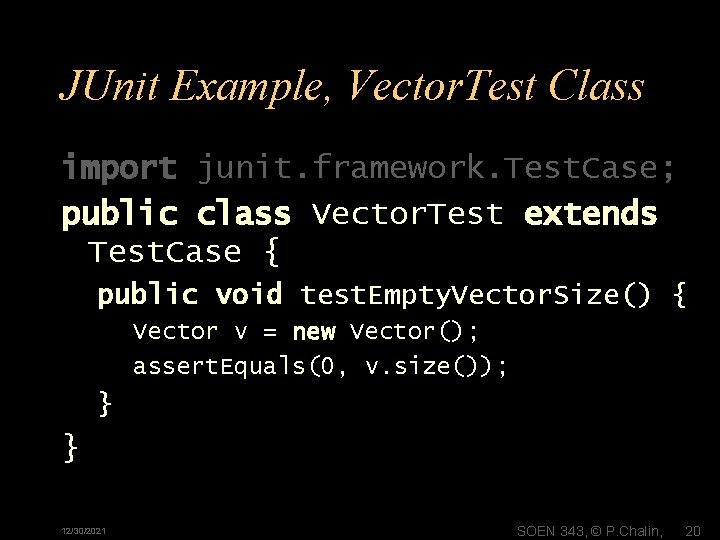
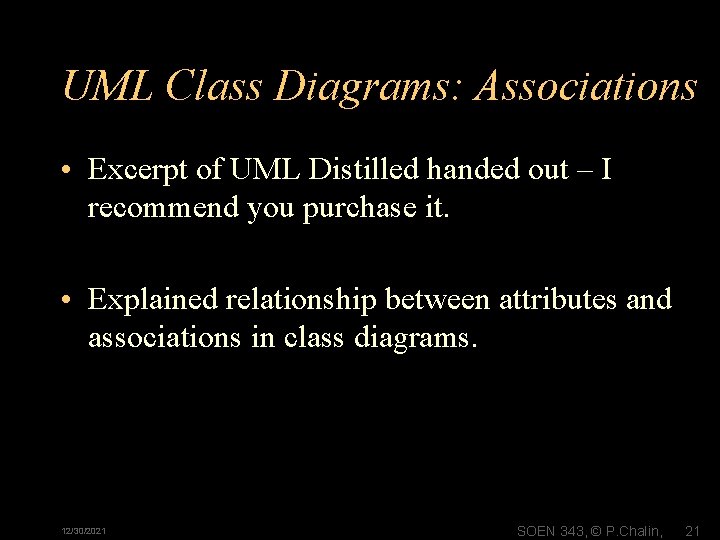
- Slides: 21
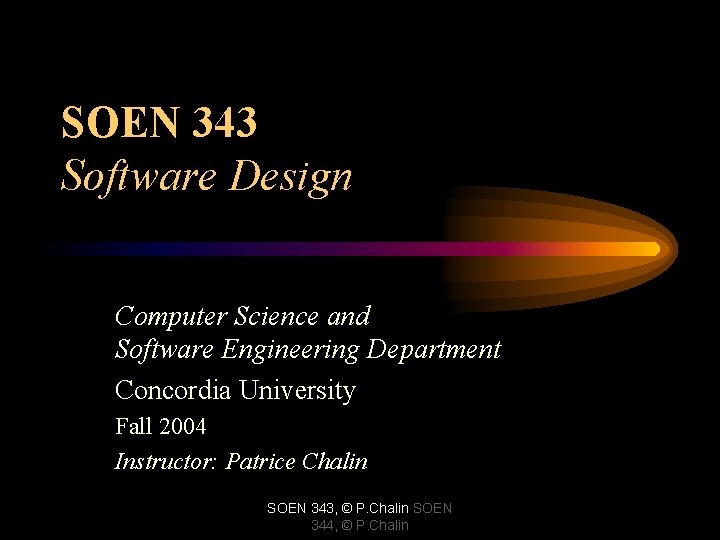
SOEN 343 Software Design Computer Science and Software Engineering Department Concordia University Fall 2004 Instructor: Patrice Chalin SOEN 343, © P. Chalin SOEN 344, © P. Chalin
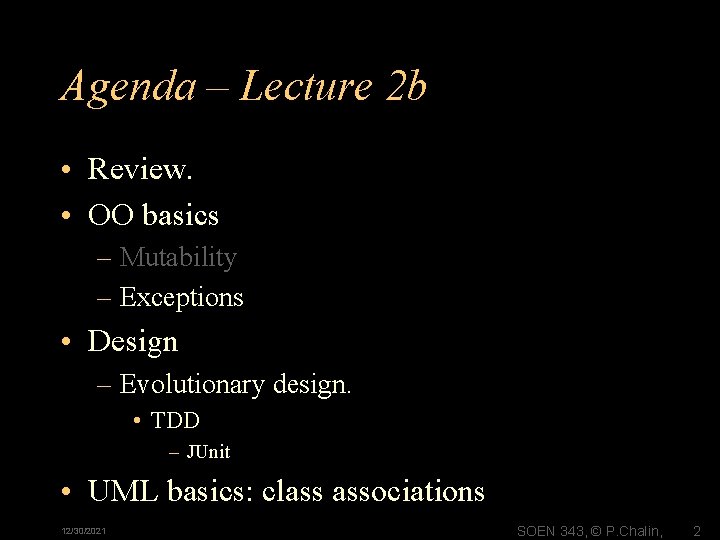
Agenda – Lecture 2 b • Review. • OO basics – Mutability – Exceptions • Design – Evolutionary design. • TDD – JUnit • UML basics: class associations 12/30/2021 SOEN 343, © P. Chalin, 2
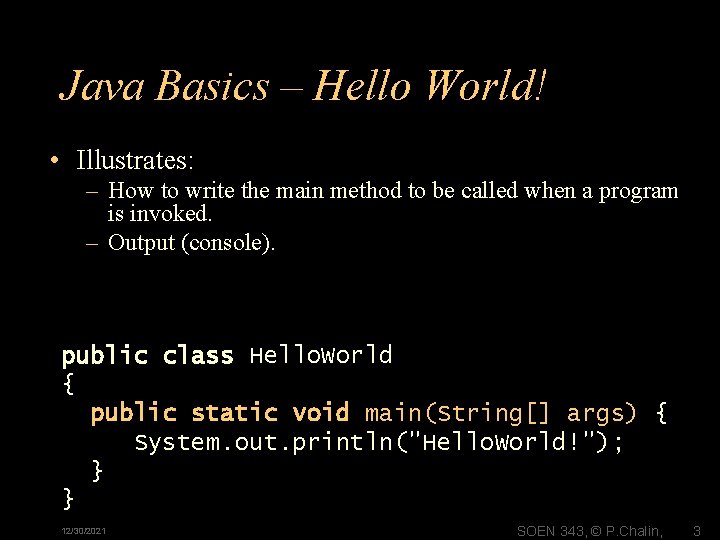
Java Basics – Hello World! • Illustrates: – How to write the main method to be called when a program is invoked. – Output (console). public class Hello. World { public static void main(String[] args) { System. out. println("Hello. World!"); } } 12/30/2021 SOEN 343, © P. Chalin, 3
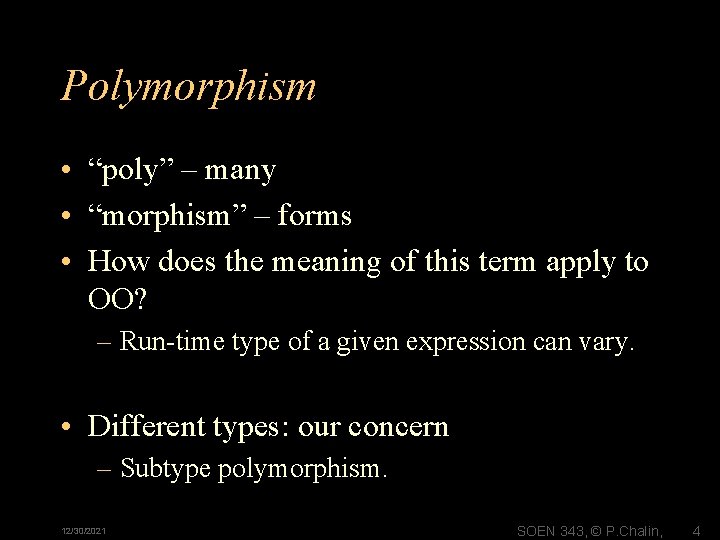
Polymorphism • “poly” – many • “morphism” – forms • How does the meaning of this term apply to OO? – Run-time type of a given expression can vary. • Different types: our concern – Subtype polymorphism. 12/30/2021 SOEN 343, © P. Chalin, 4
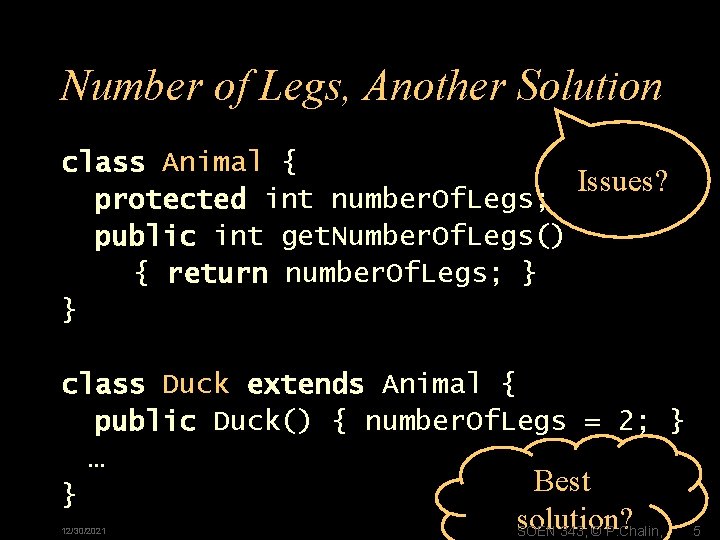
Number of Legs, Another Solution class Animal { Issues? protected int number. Of. Legs; public int get. Number. Of. Legs() { return number. Of. Legs; } } class Duck extends Animal { public Duck() { number. Of. Legs = 2; } … Best } 12/30/2021 solution? SOEN 343, © P. Chalin, 5
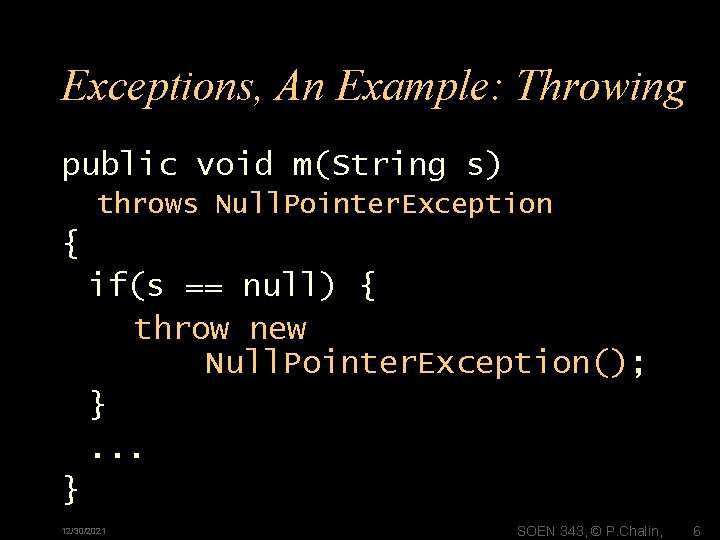
Exceptions, An Example: Throwing public void m(String s) throws Null. Pointer. Exception { if(s == null) { throw new Null. Pointer. Exception(); }. . . } 12/30/2021 SOEN 343, © P. Chalin, 6
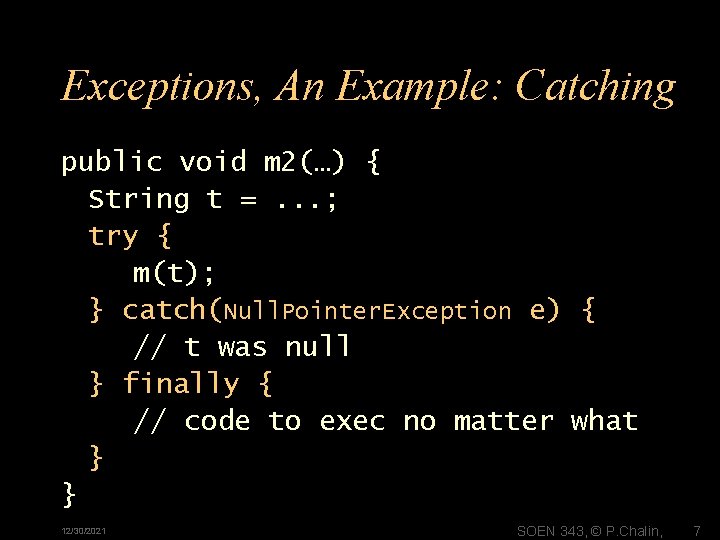
Exceptions, An Example: Catching public void m 2(…) { String t =. . . ; try { m(t); } catch(Null. Pointer. Exception e) { // t was null } finally { // code to exec no matter what } } 12/30/2021 SOEN 343, © P. Chalin, 7
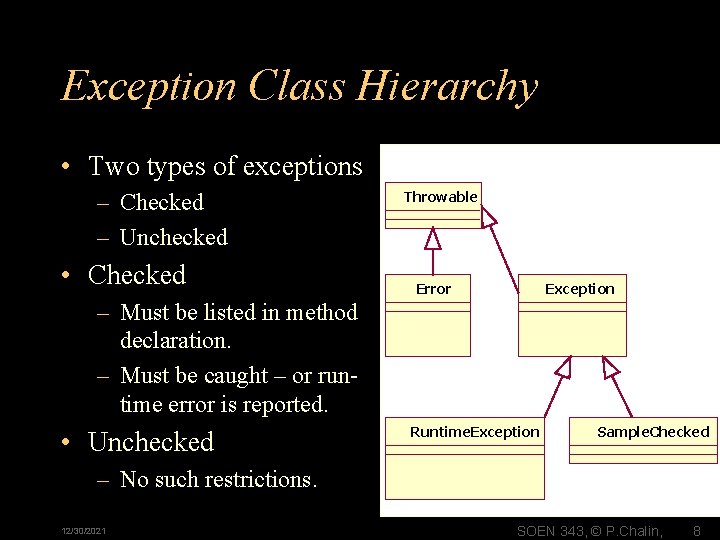
Exception Class Hierarchy • Two types of exceptions – Checked – Unchecked • Checked Throwable Error Exception – Must be listed in method declaration. – Must be caught – or runtime error is reported. • Unchecked Runtime. Exception Sample. Checked – No such restrictions. 12/30/2021 SOEN 343, © P. Chalin, 8
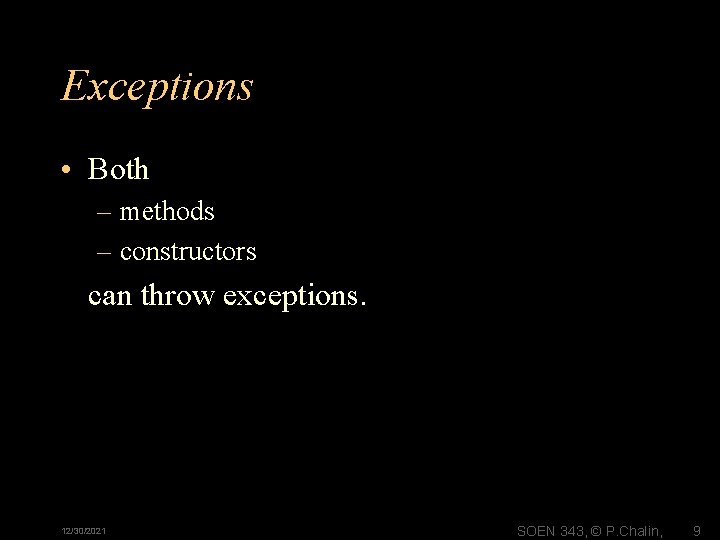
Exceptions • Both – methods – constructors can throw exceptions. 12/30/2021 SOEN 343, © P. Chalin, 9
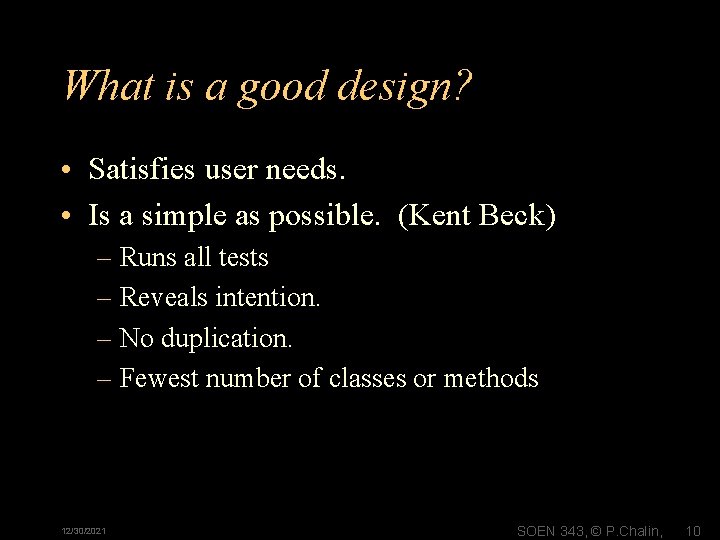
What is a good design? • Satisfies user needs. • Is a simple as possible. (Kent Beck) – Runs all tests – Reveals intention. – No duplication. – Fewest number of classes or methods 12/30/2021 SOEN 343, © P. Chalin, 10
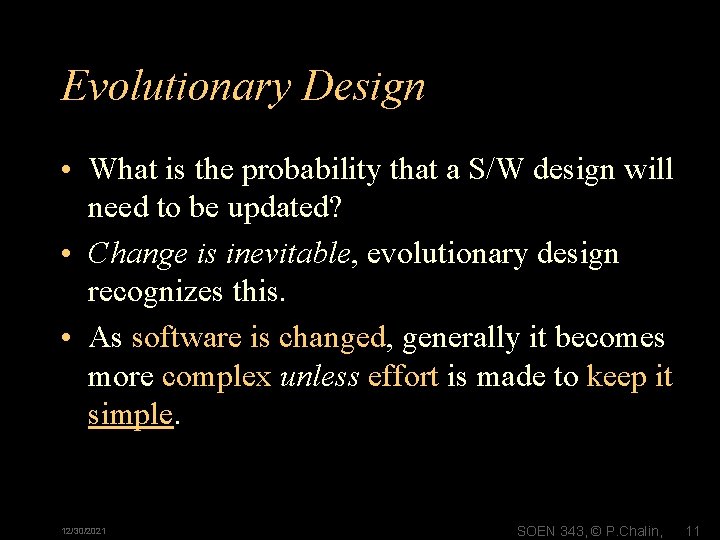
Evolutionary Design • What is the probability that a S/W design will need to be updated? • Change is inevitable, evolutionary design recognizes this. • As software is changed, generally it becomes more complex unless effort is made to keep it simple. 12/30/2021 SOEN 343, © P. Chalin, 11
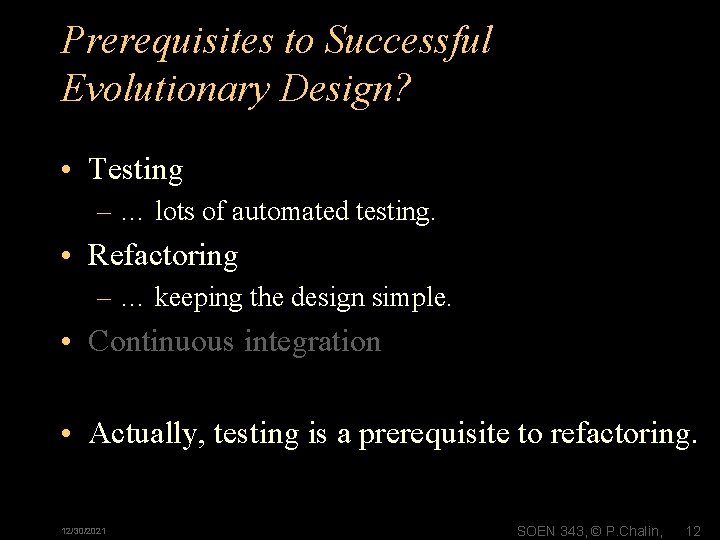
Prerequisites to Successful Evolutionary Design? • Testing – … lots of automated testing. • Refactoring – … keeping the design simple. • Continuous integration • Actually, testing is a prerequisite to refactoring. 12/30/2021 SOEN 343, © P. Chalin, 12
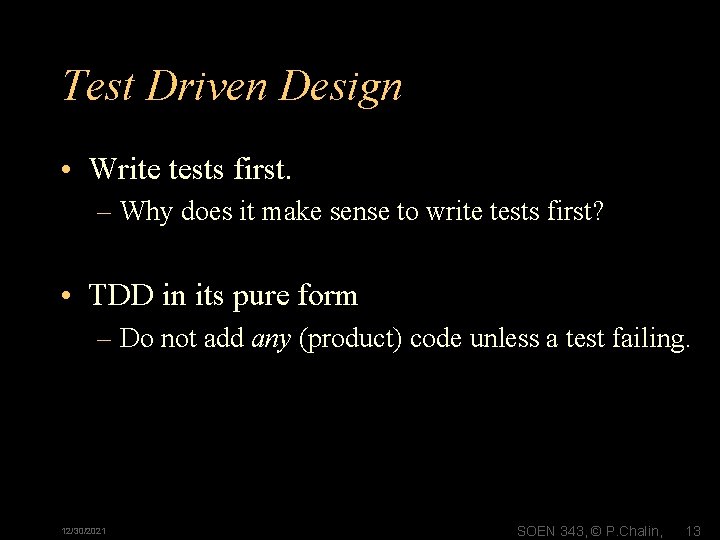
Test Driven Design • Write tests first. – Why does it make sense to write tests first? • TDD in its pure form – Do not add any (product) code unless a test failing. 12/30/2021 SOEN 343, © P. Chalin, 13
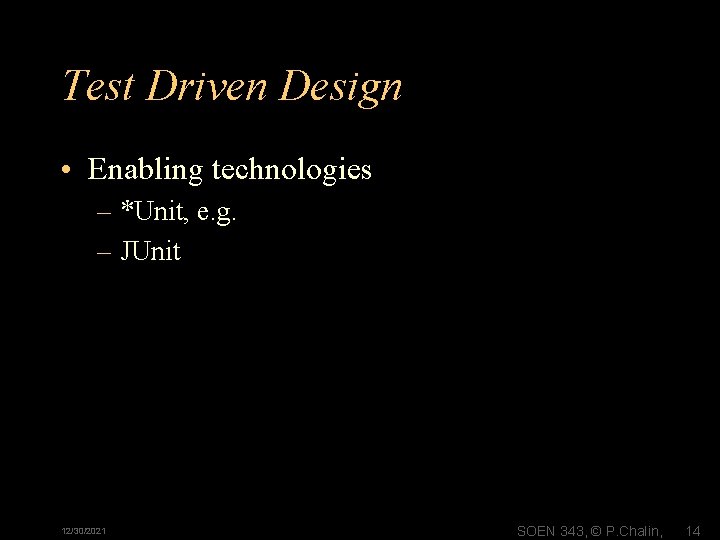
Test Driven Design • Enabling technologies – *Unit, e. g. – JUnit 12/30/2021 SOEN 343, © P. Chalin, 14
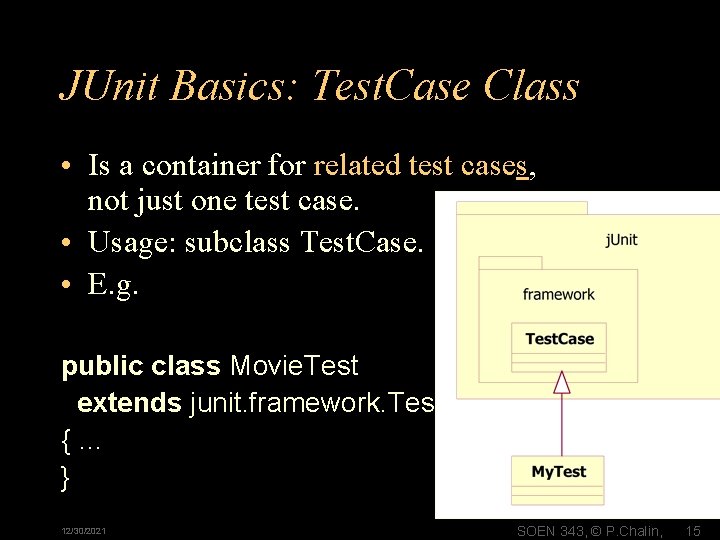
JUnit Basics: Test. Case Class • Is a container for related test cases, not just one test case. • Usage: subclass Test. Case. • E. g. public class Movie. Test extends junit. framework. Test. Case {… } 12/30/2021 SOEN 343, © P. Chalin, 15
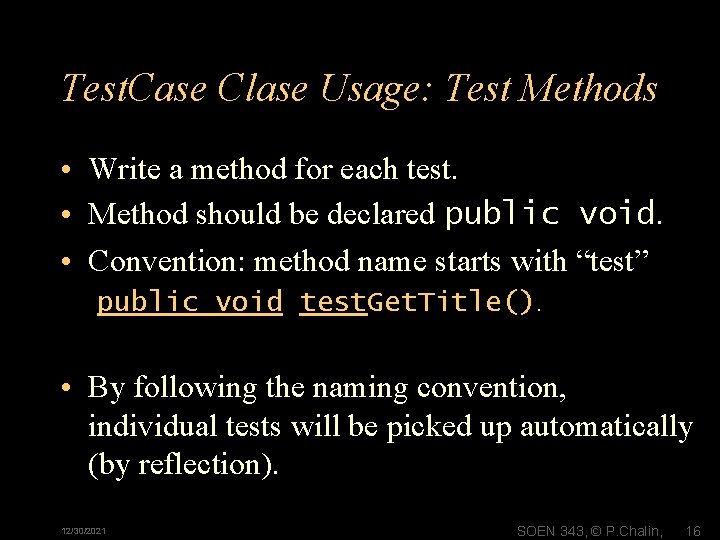
Test. Case Clase Usage: Test Methods • Write a method for each test. • Method should be declared public void. • Convention: method name starts with “test” public void test. Get. Title(). • By following the naming convention, individual tests will be picked up automatically (by reflection). 12/30/2021 SOEN 343, © P. Chalin, 16
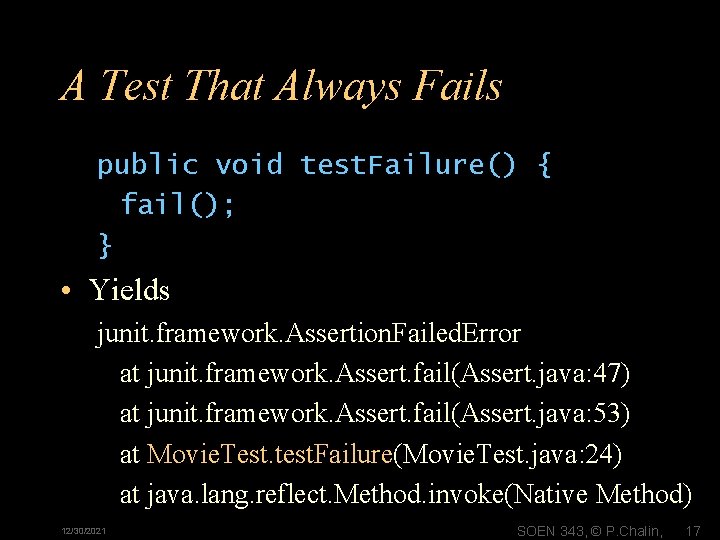
A Test That Always Fails public void test. Failure() { fail(); } • Yields junit. framework. Assertion. Failed. Error at junit. framework. Assert. fail(Assert. java: 47) at junit. framework. Assert. fail(Assert. java: 53) at Movie. Test. test. Failure(Movie. Test. java: 24) at java. lang. reflect. Method. invoke(Native Method) 12/30/2021 SOEN 343, © P. Chalin, 17
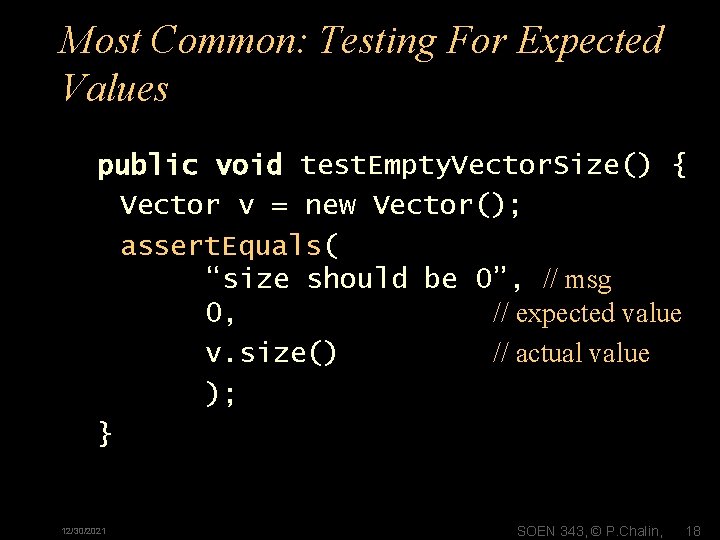
Most Common: Testing For Expected Values public void test. Empty. Vector. Size() { Vector v = new Vector(); assert. Equals( “size should be 0”, // msg 0, // expected value v. size() // actual value ); } 12/30/2021 SOEN 343, © P. Chalin, 18
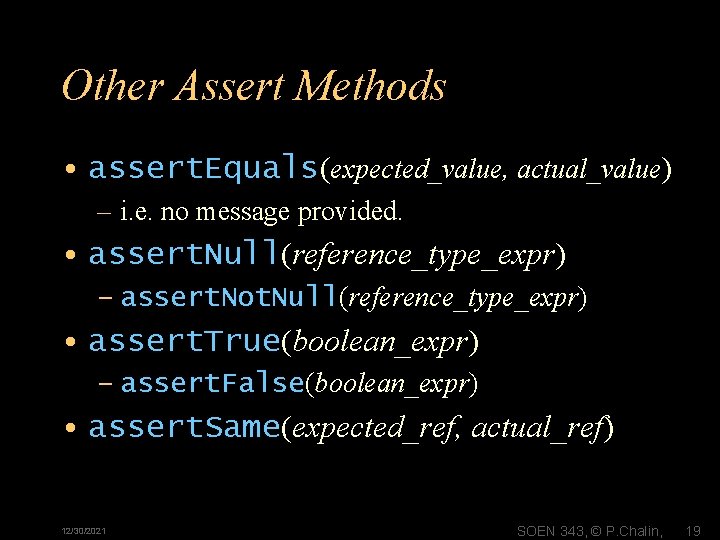
Other Assert Methods • assert. Equals(expected_value, actual_value) – i. e. no message provided. • assert. Null(reference_type_expr) – assert. Not. Null(reference_type_expr) • assert. True(boolean_expr) – assert. False(boolean_expr) • assert. Same(expected_ref, actual_ref) 12/30/2021 SOEN 343, © P. Chalin, 19
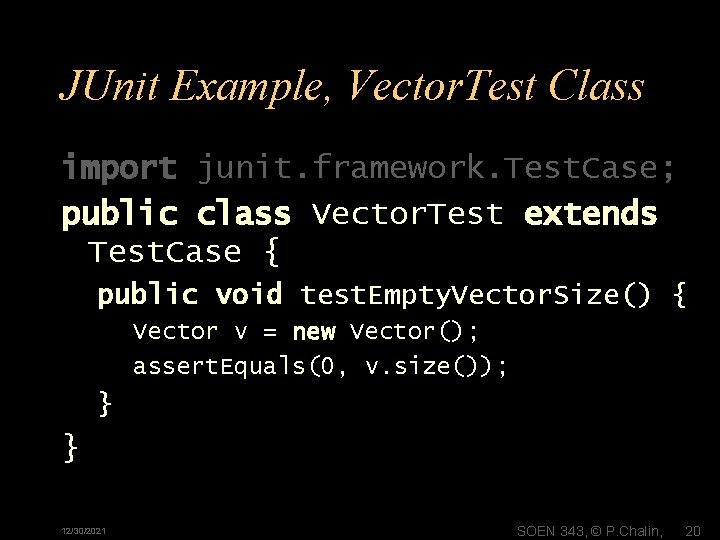
JUnit Example, Vector. Test Class import junit. framework. Test. Case; public class Vector. Test extends Test. Case { public void test. Empty. Vector. Size() { Vector v = new Vector(); assert. Equals(0, v. size()); } } 12/30/2021 SOEN 343, © P. Chalin, 20
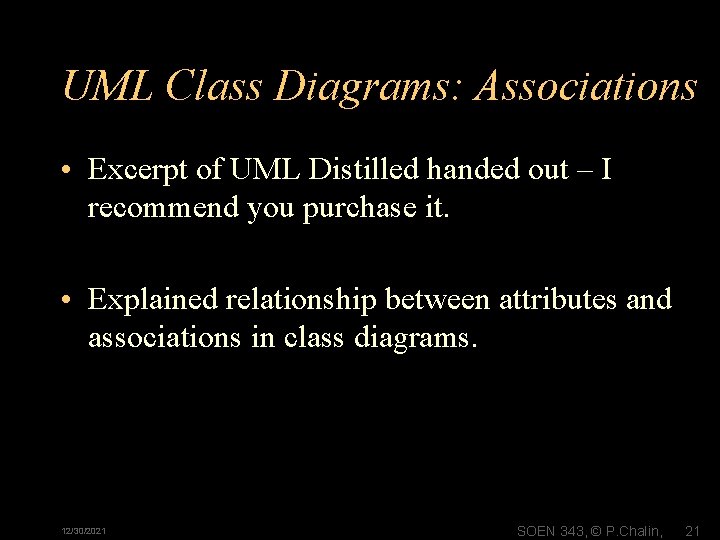
UML Class Diagrams: Associations • Excerpt of UML Distilled handed out – I recommend you purchase it. • Explained relationship between attributes and associations in class diagrams. 12/30/2021 SOEN 343, © P. Chalin, 21