Pseudocode and Flowcharts Expressing Algorithms to Solve Programming
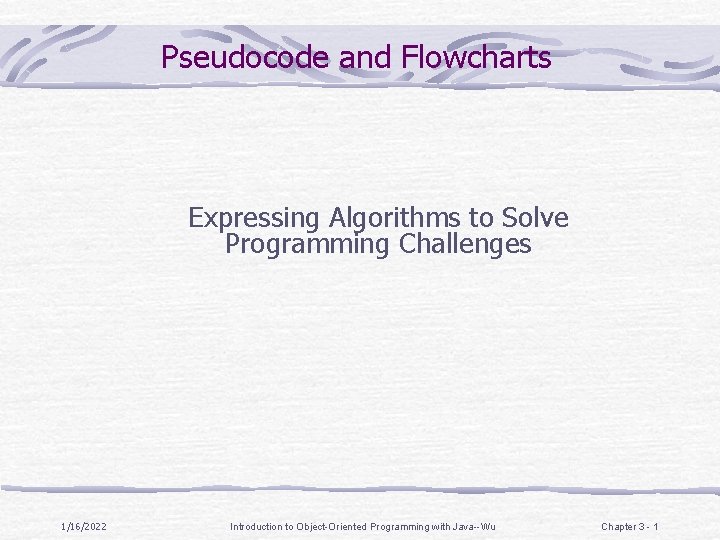
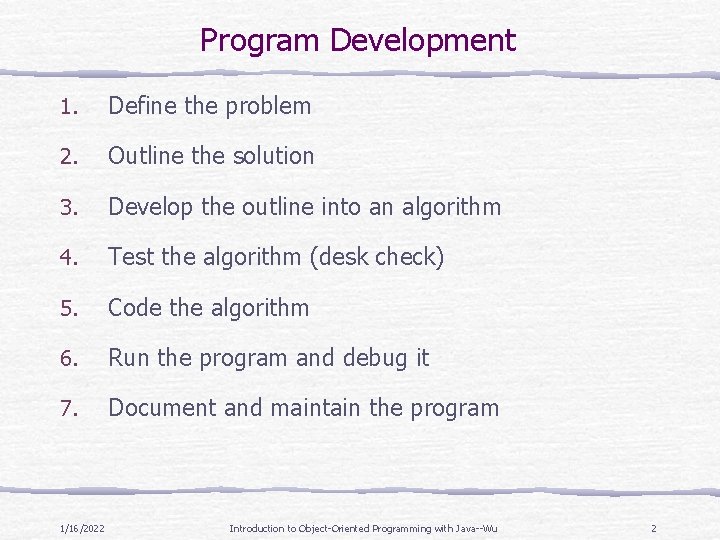
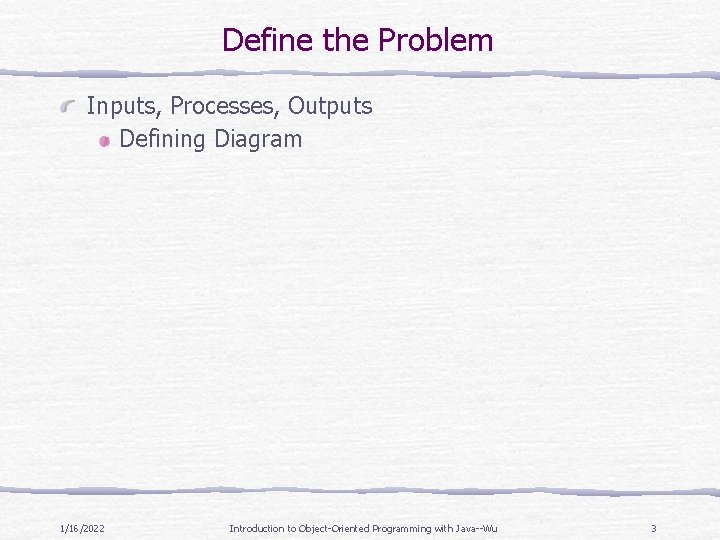
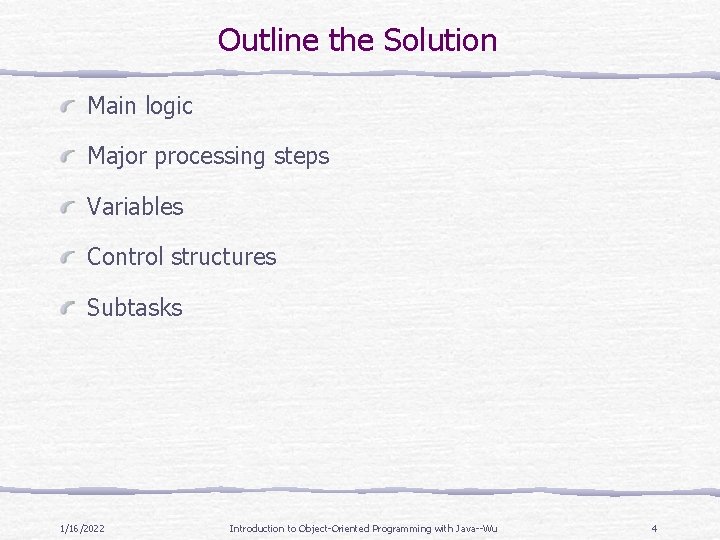
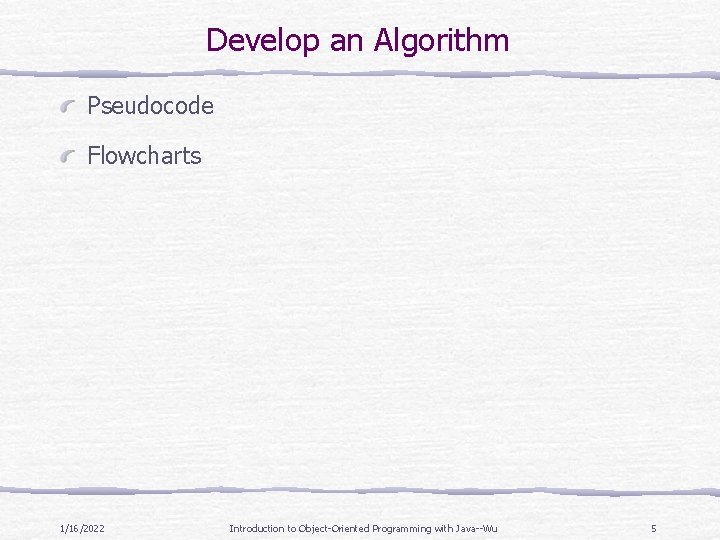
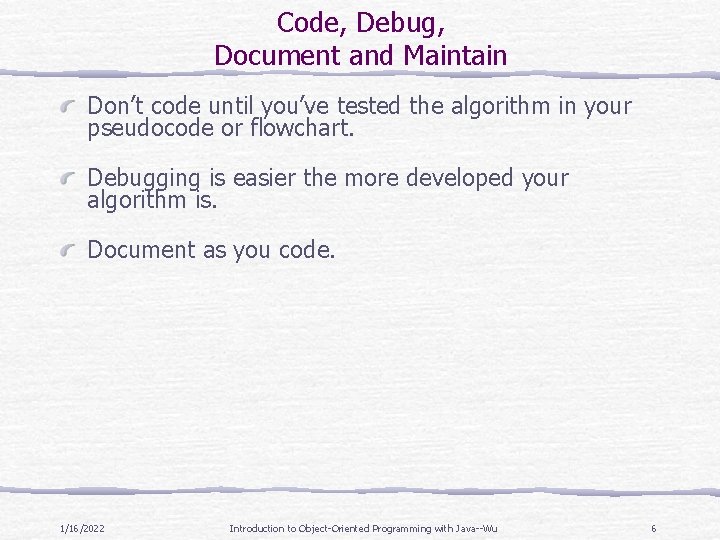
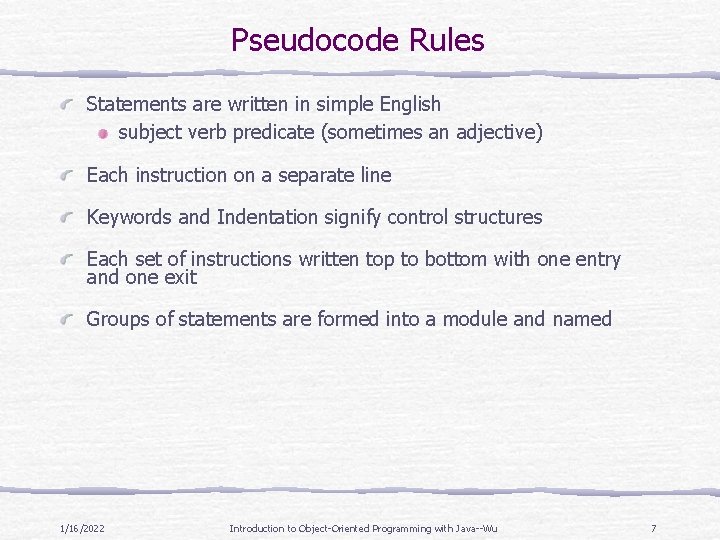
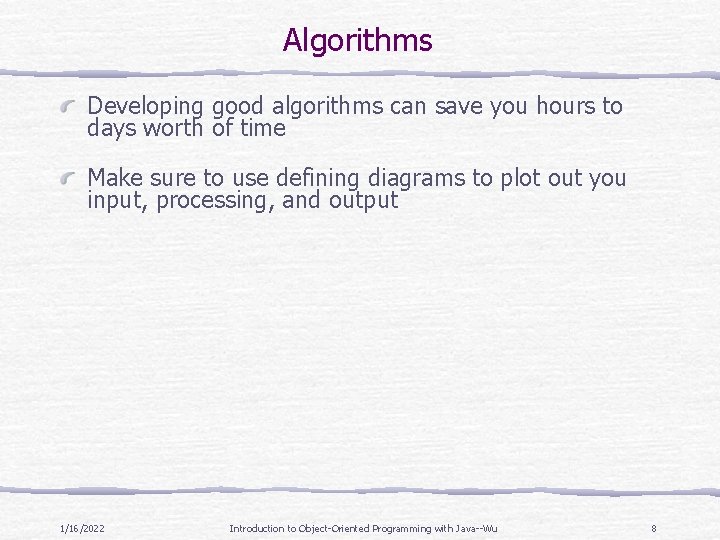
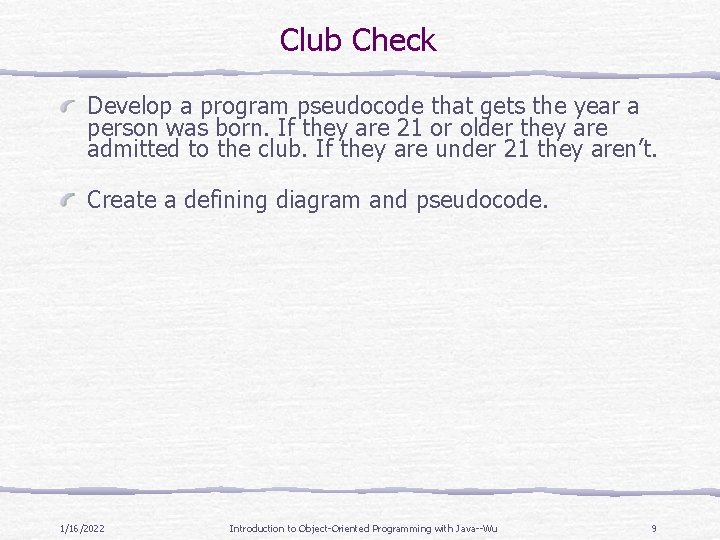
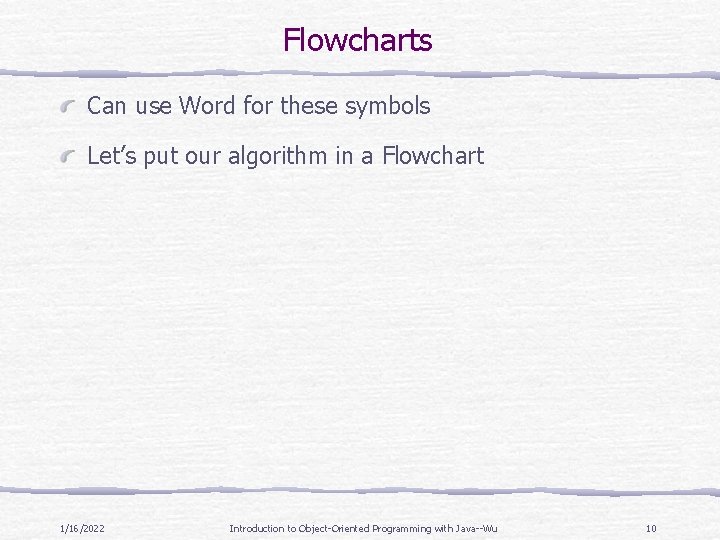
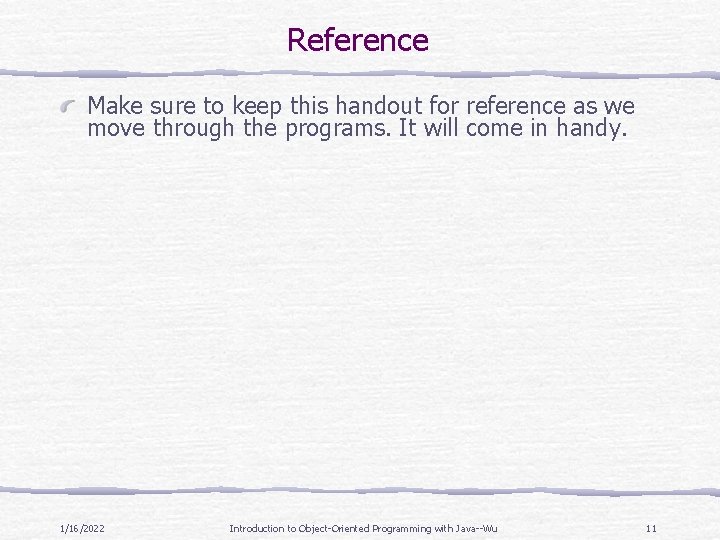
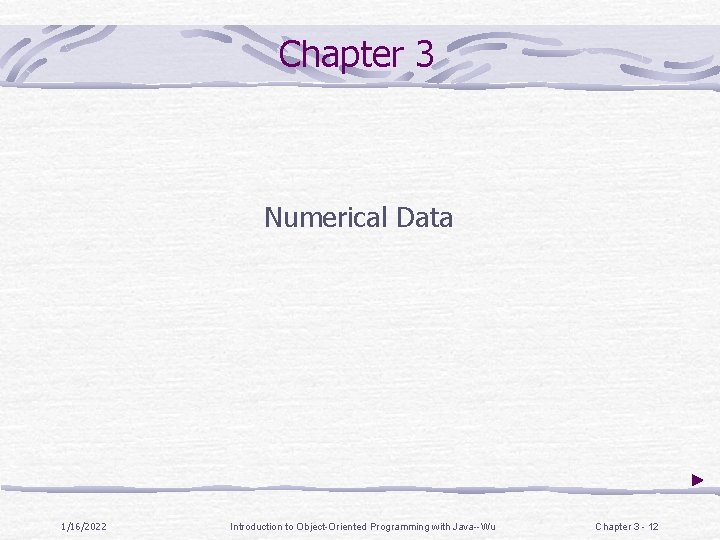
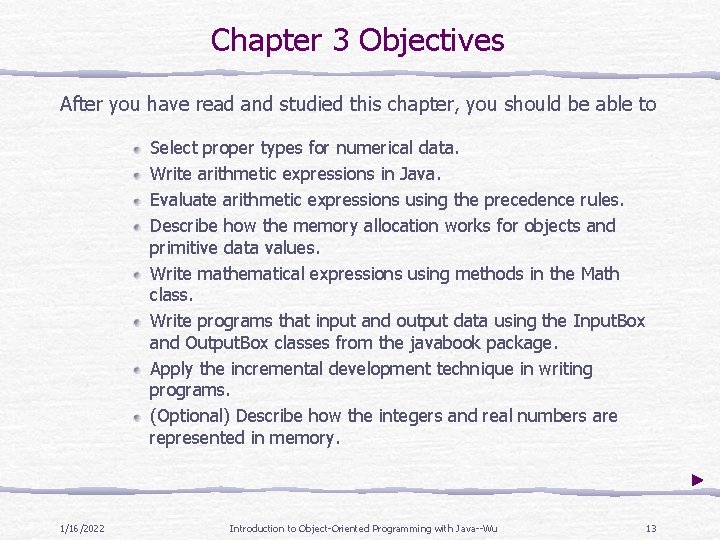
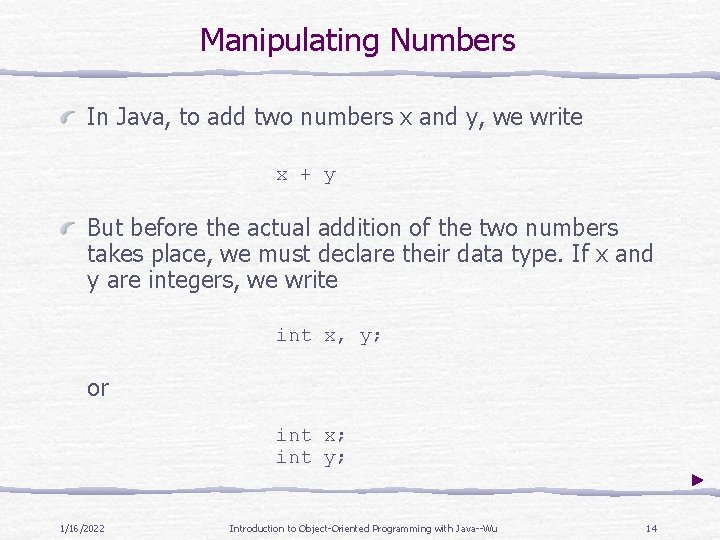
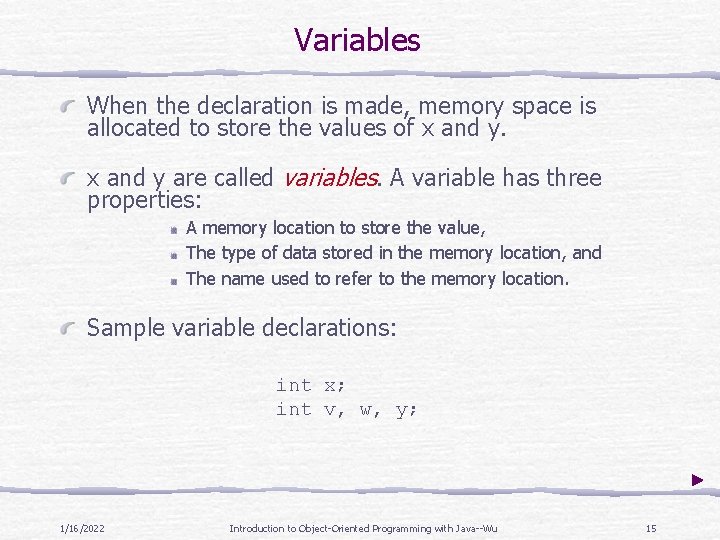
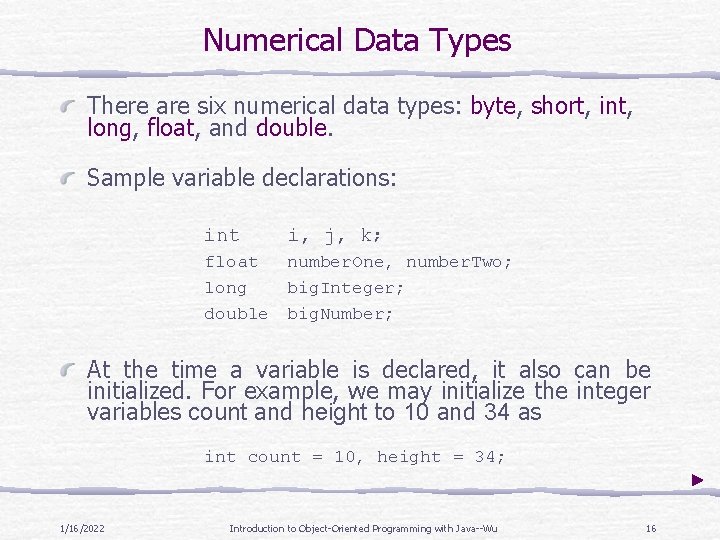
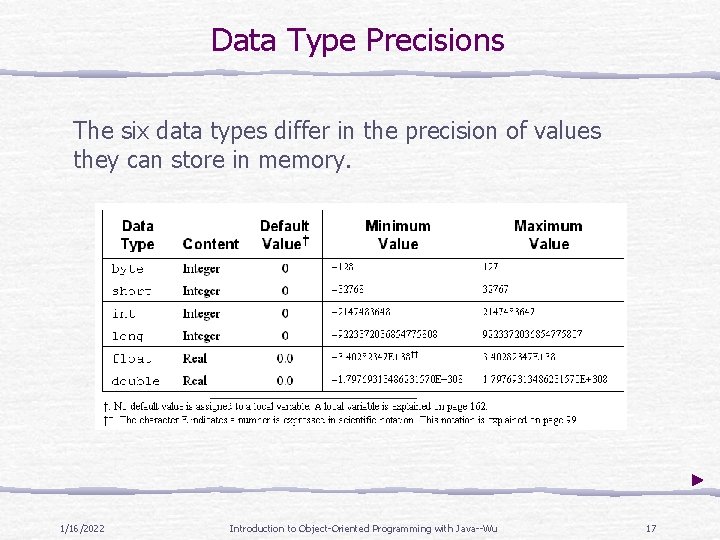
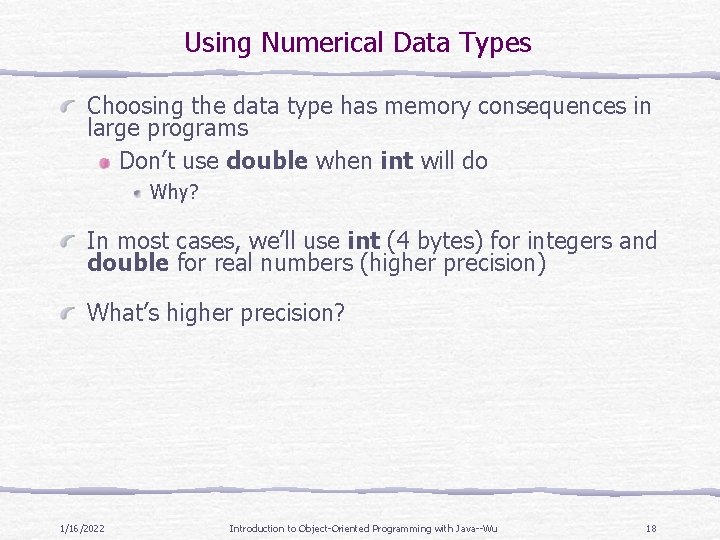
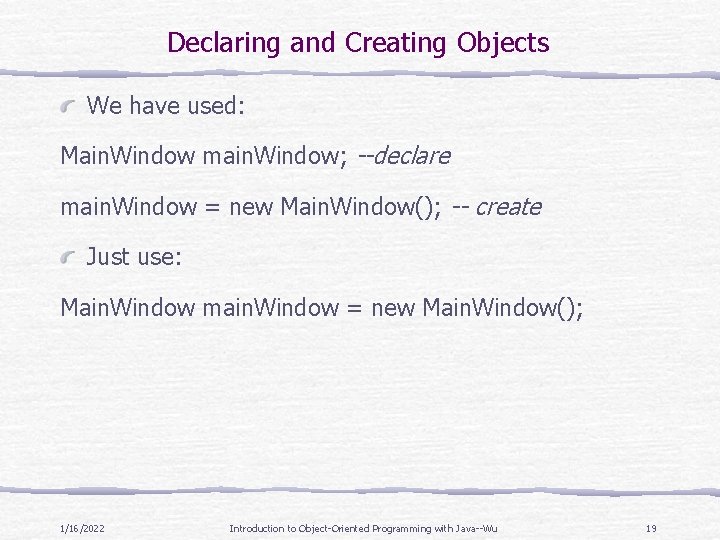
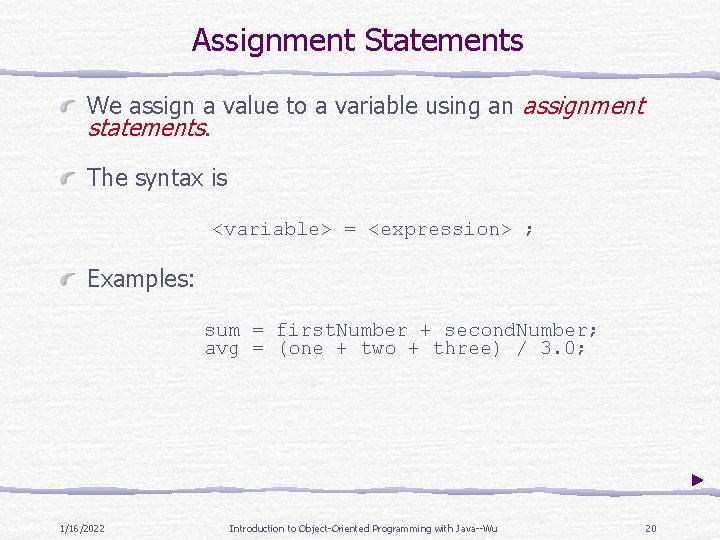
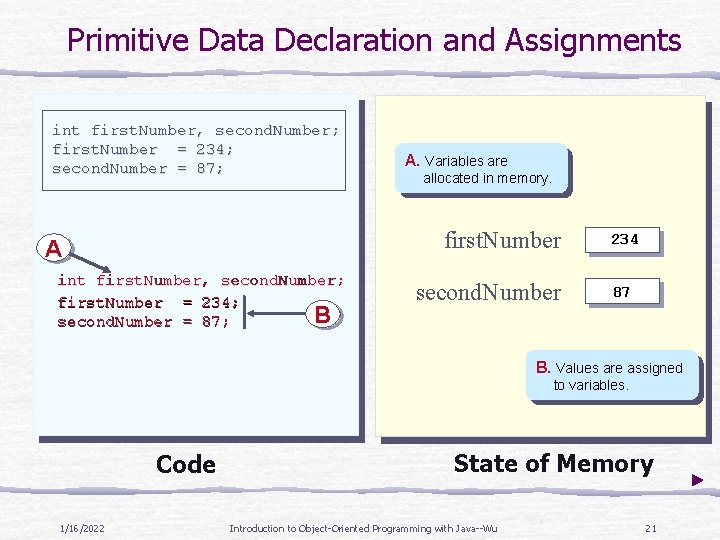
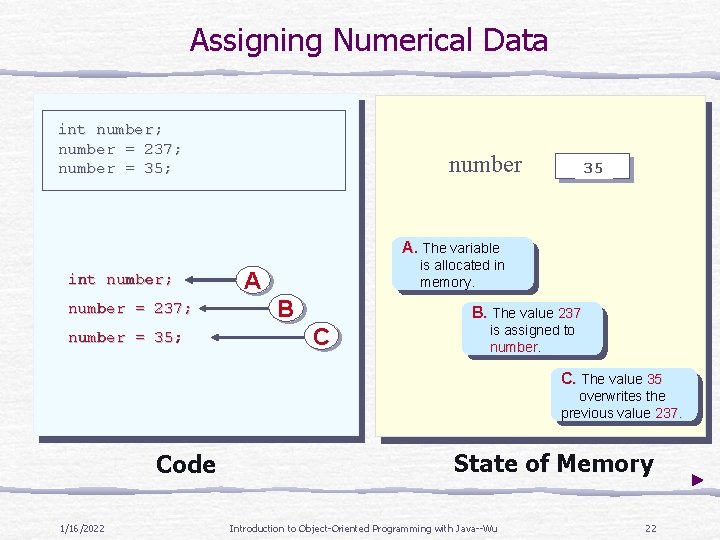
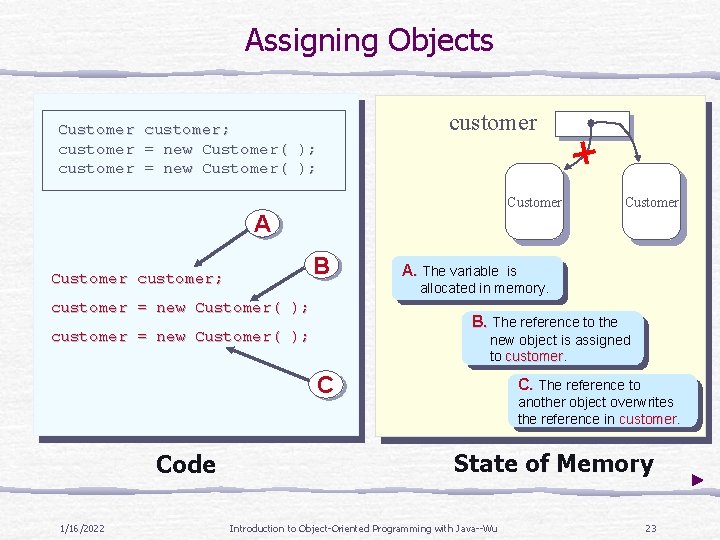
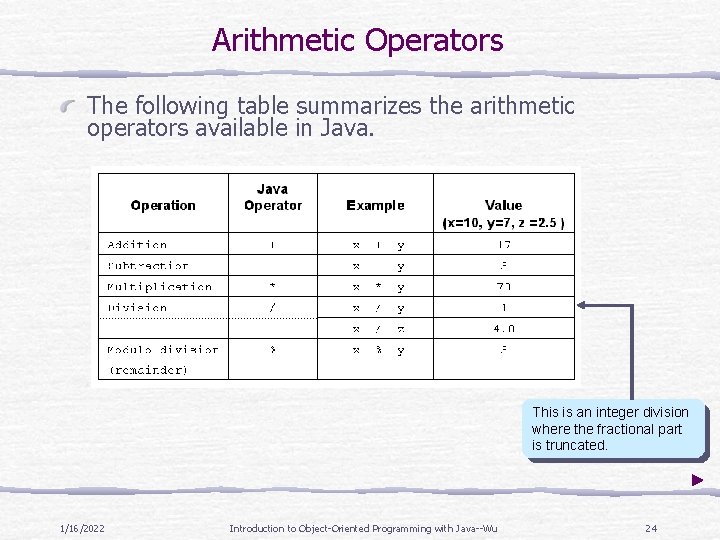
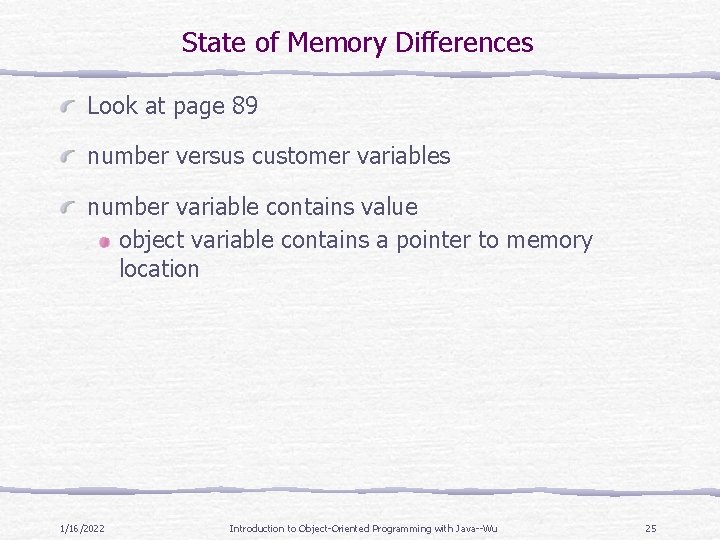
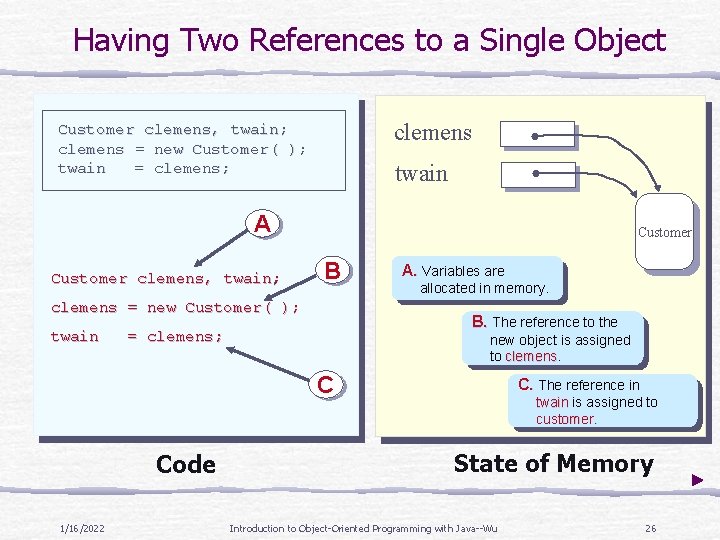
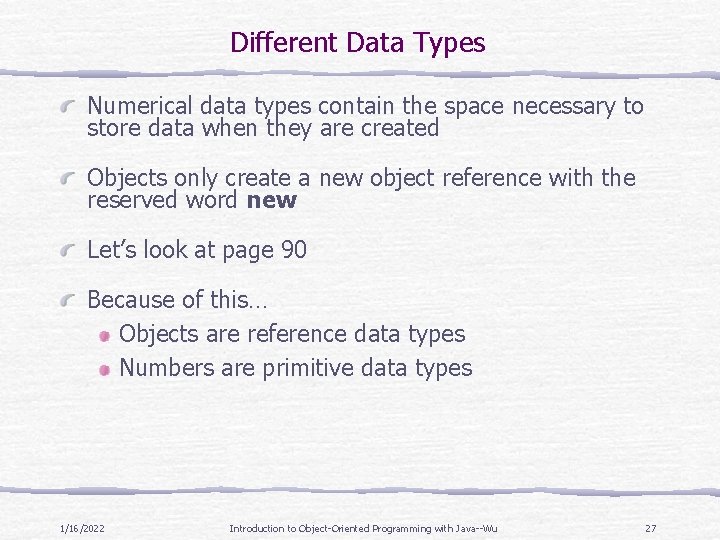
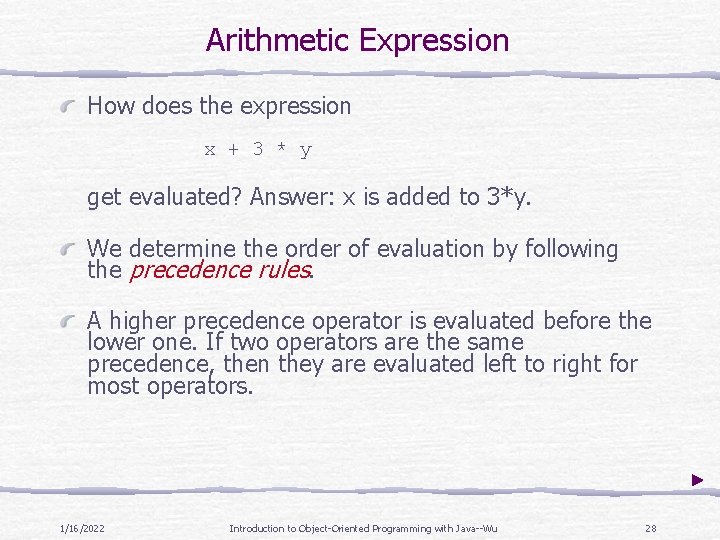
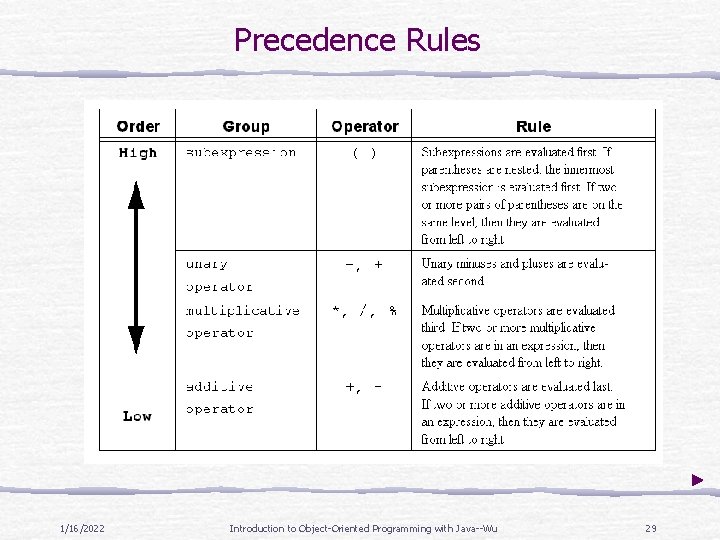
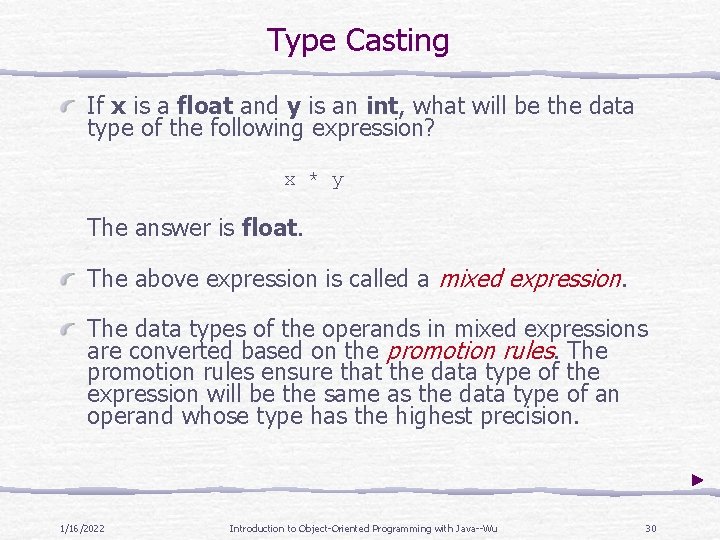
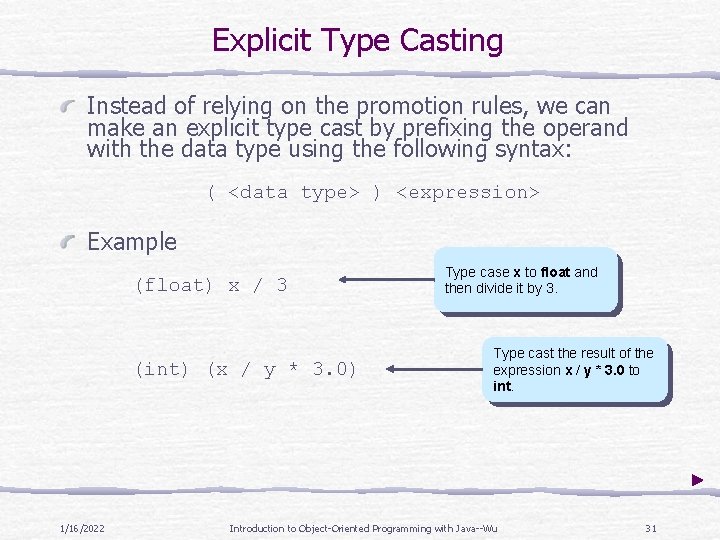
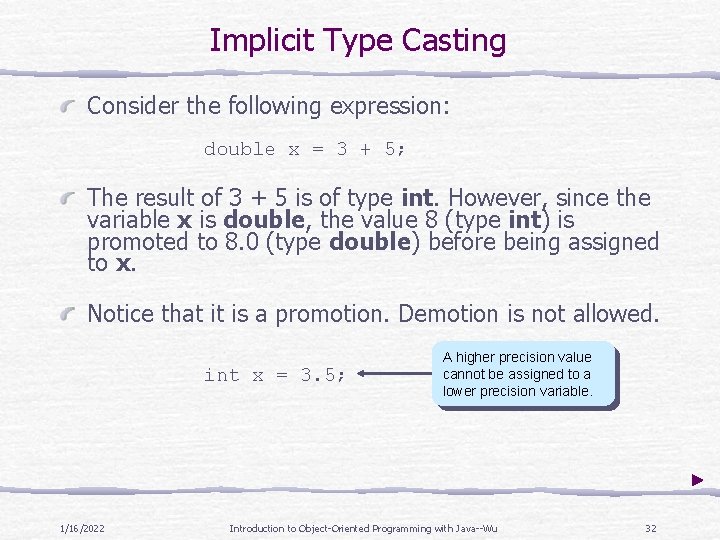
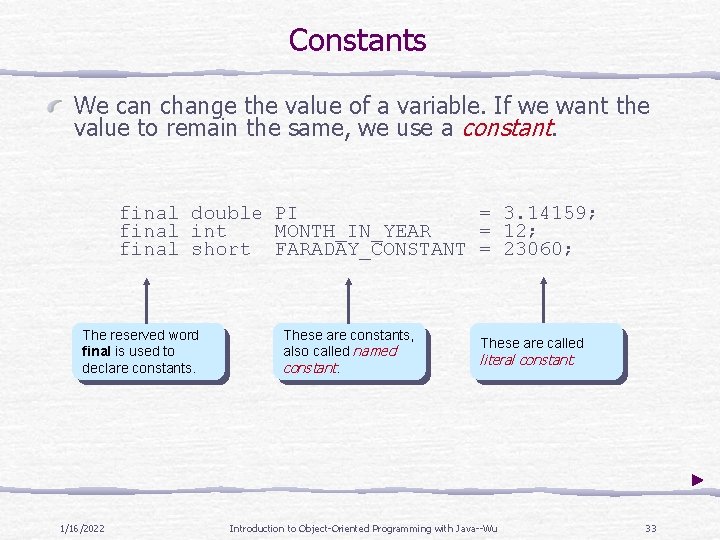
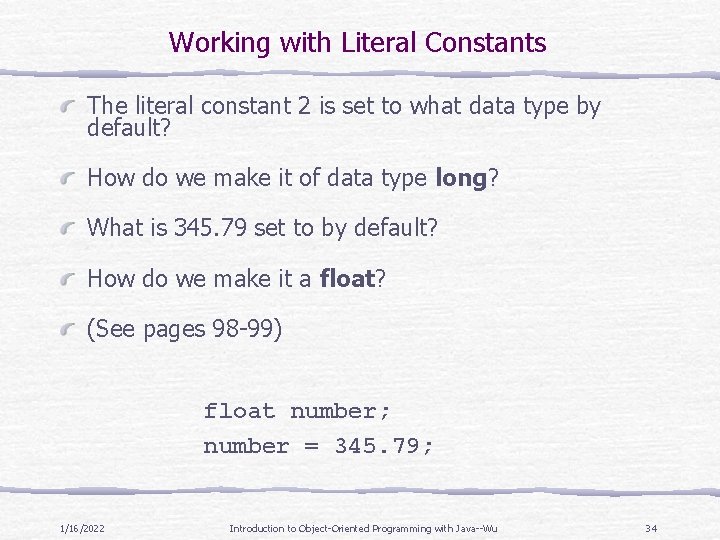
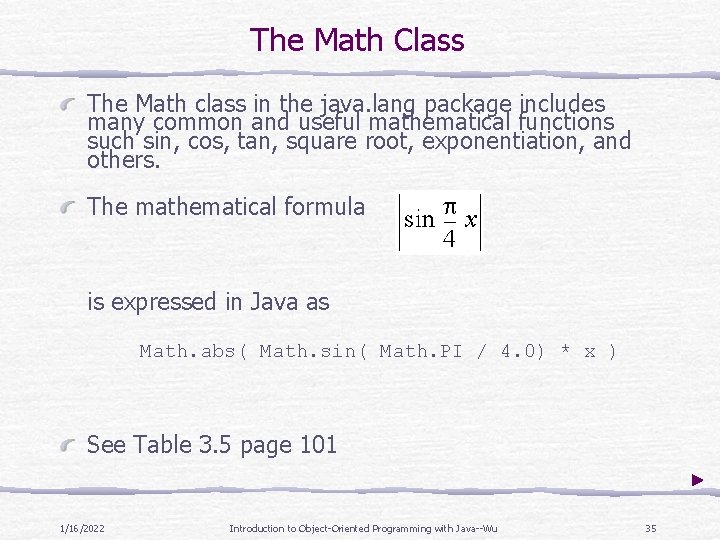
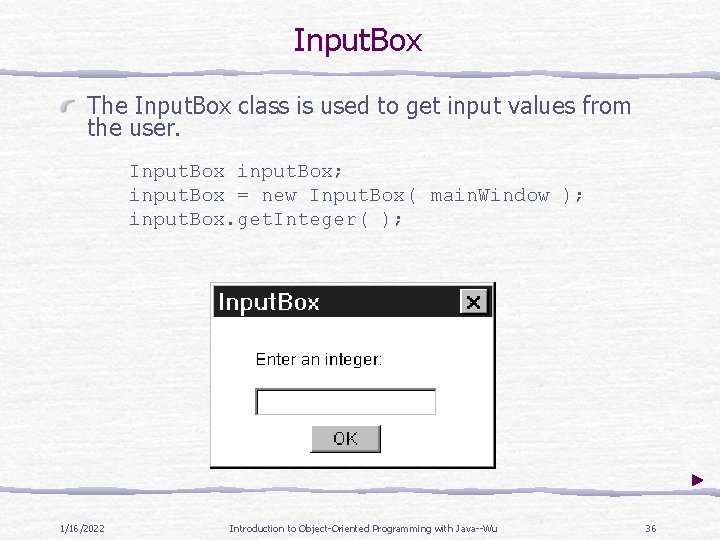
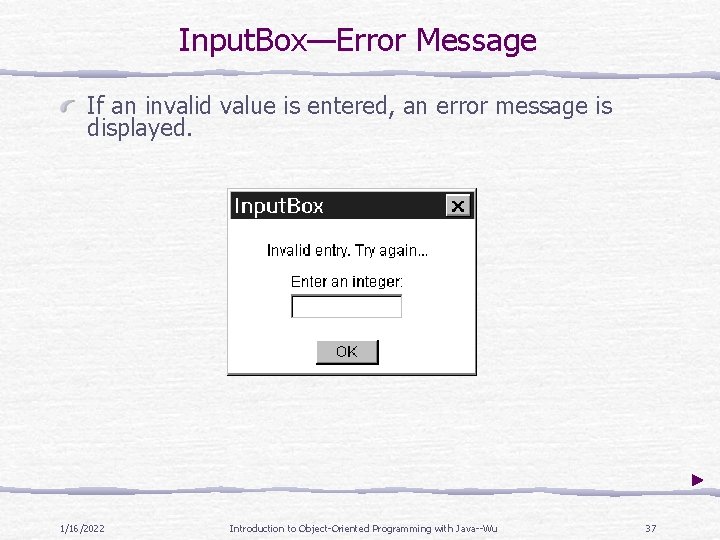
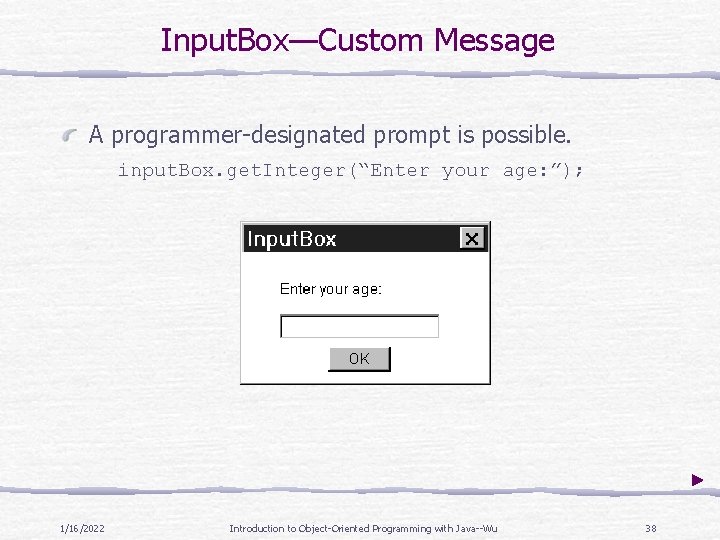
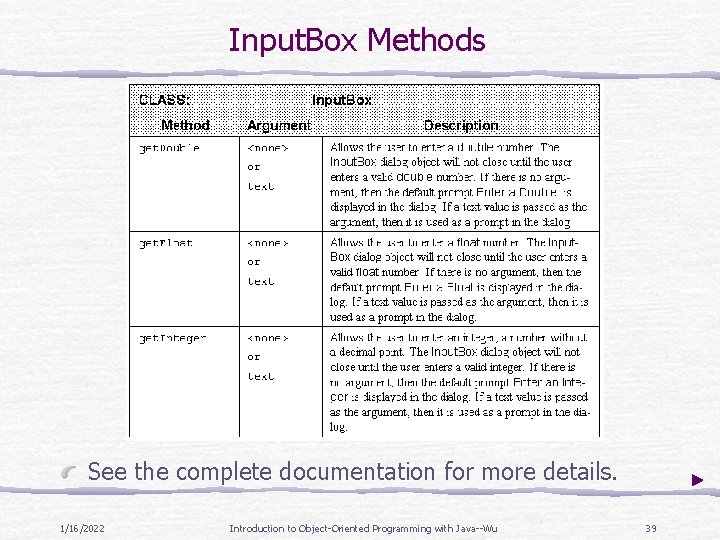
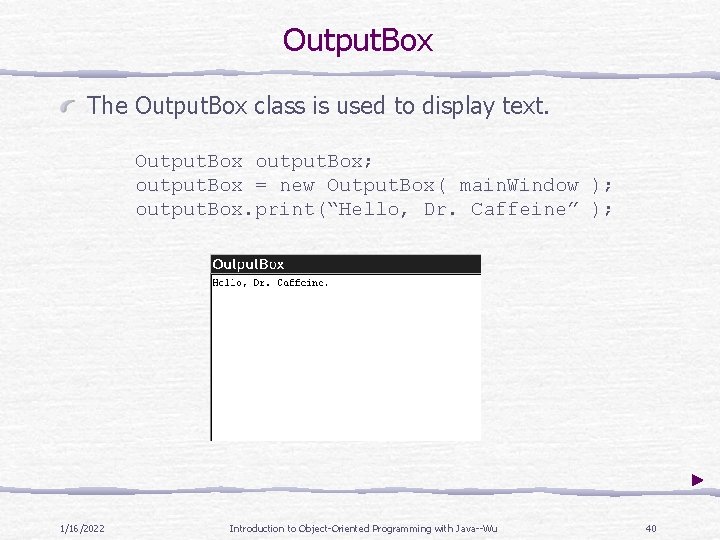
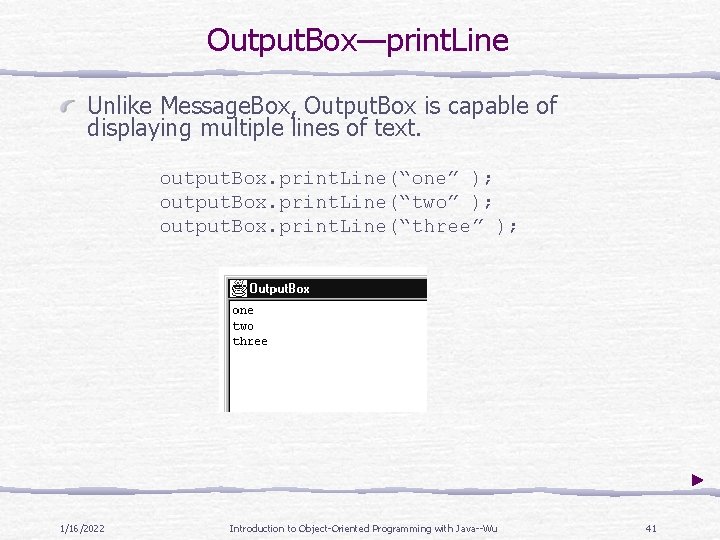
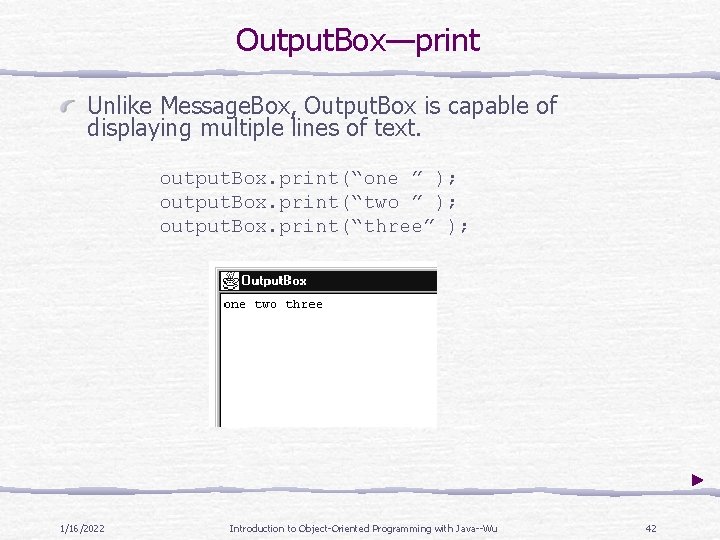
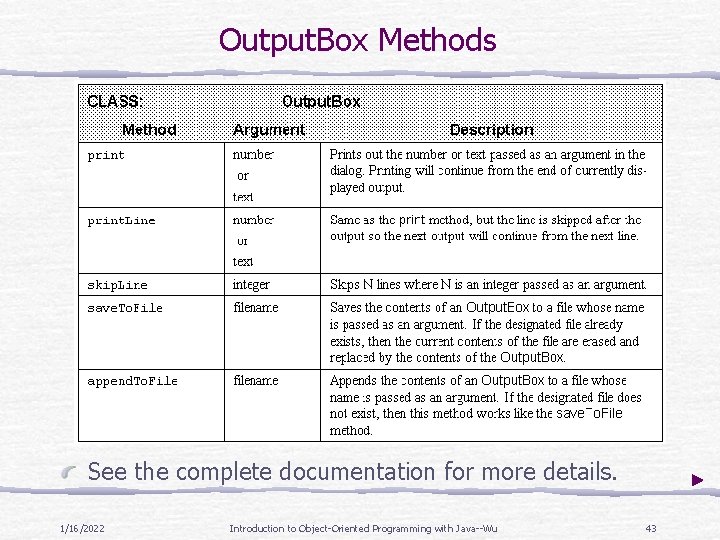
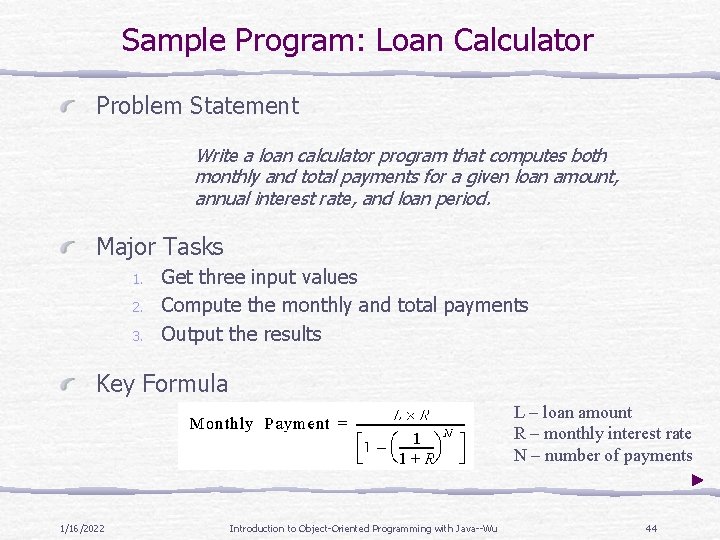
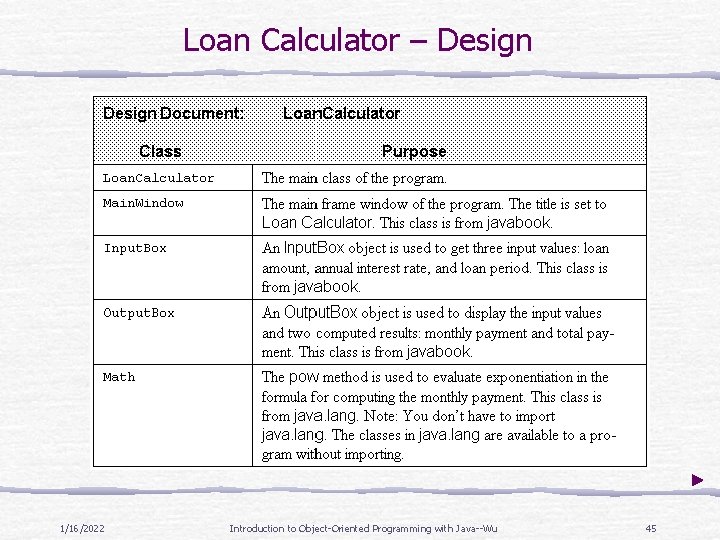
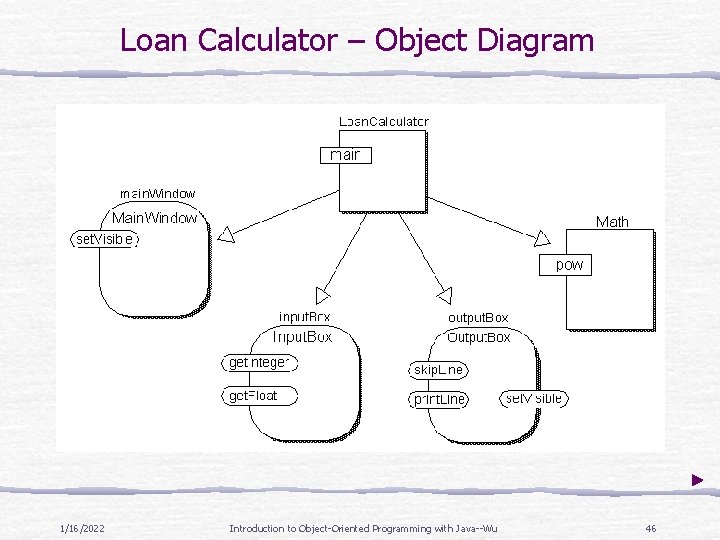
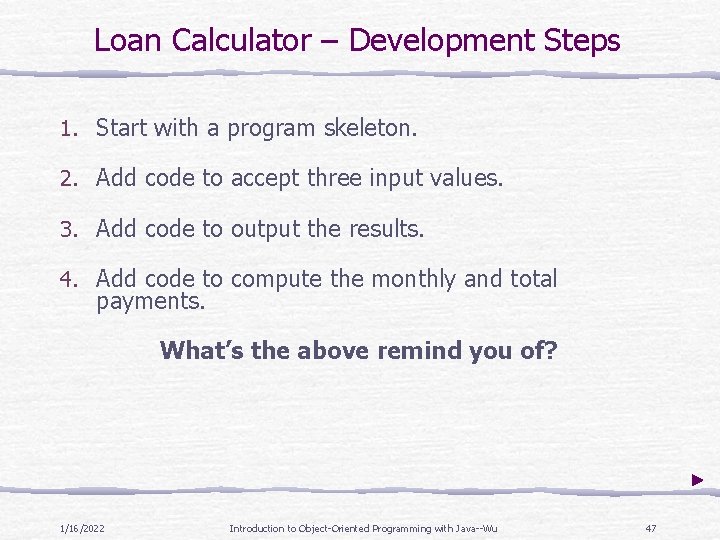
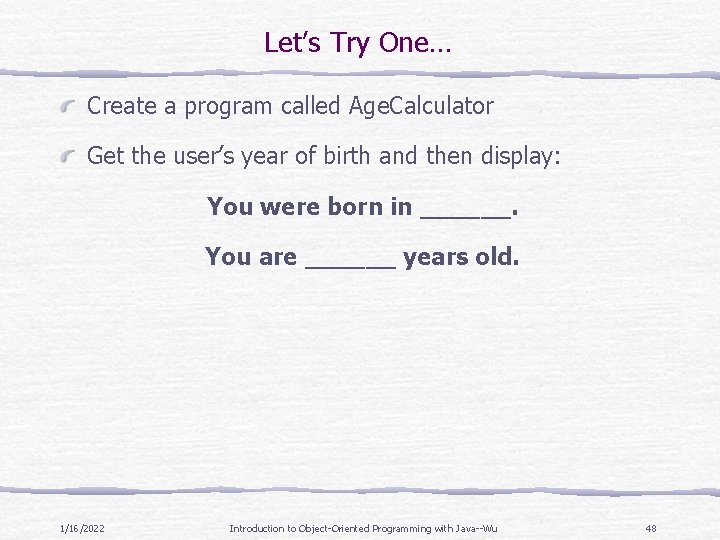
- Slides: 48
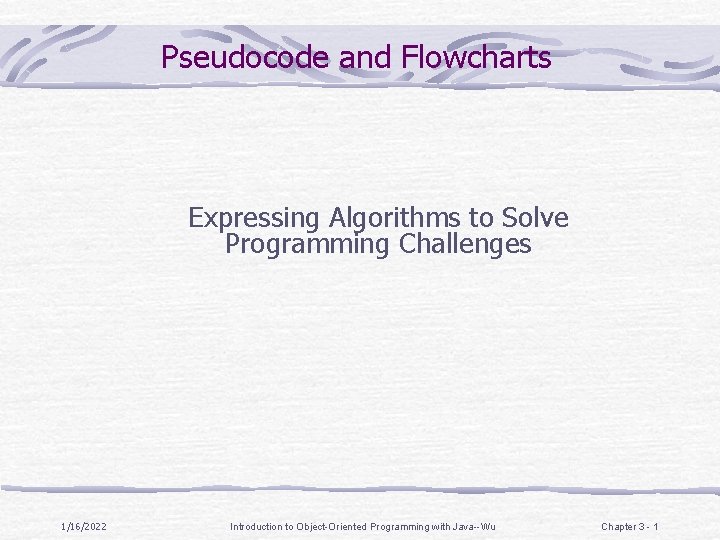
Pseudocode and Flowcharts Expressing Algorithms to Solve Programming Challenges 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu Chapter 3 - 1
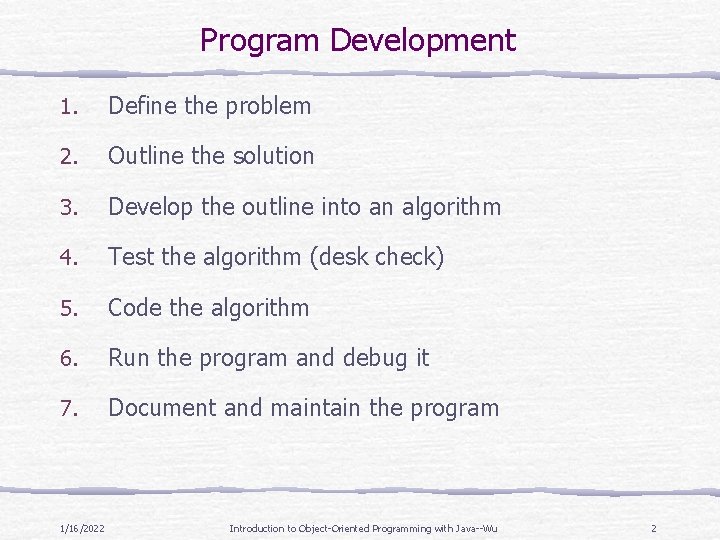
Program Development 1. Define the problem 2. Outline the solution 3. Develop the outline into an algorithm 4. Test the algorithm (desk check) 5. Code the algorithm 6. Run the program and debug it 7. Document and maintain the program 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 2
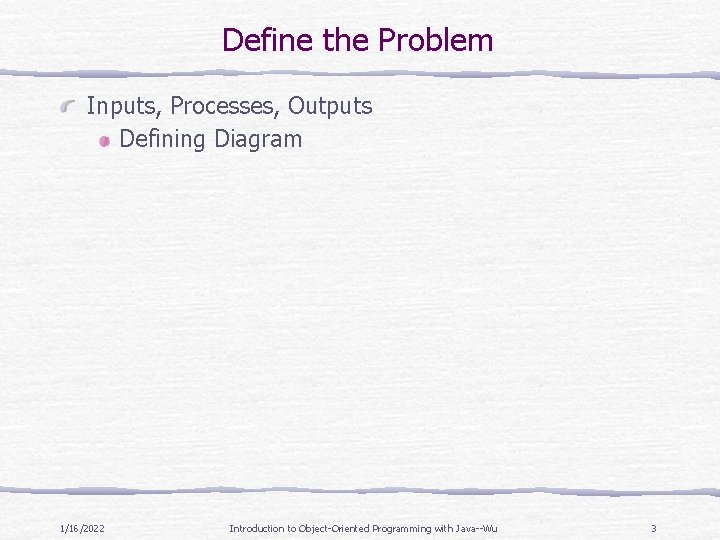
Define the Problem Inputs, Processes, Outputs Defining Diagram 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 3
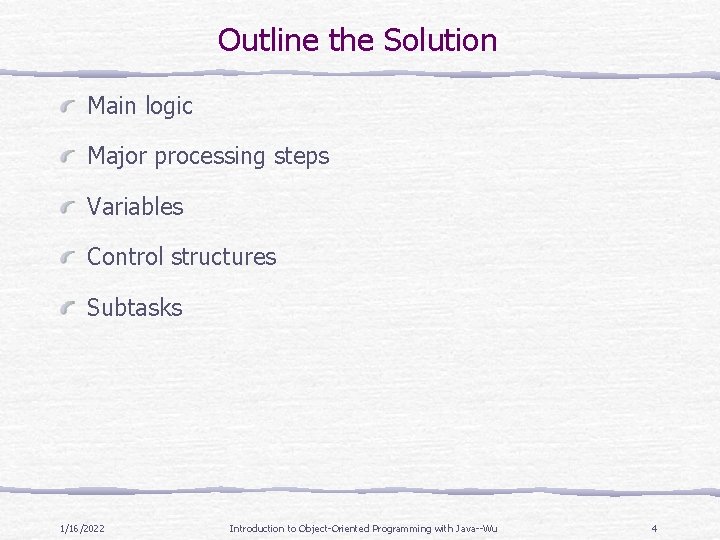
Outline the Solution Main logic Major processing steps Variables Control structures Subtasks 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 4
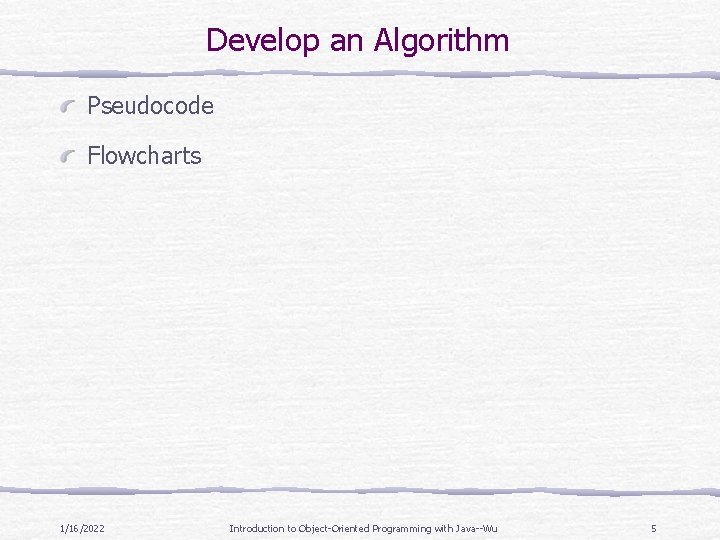
Develop an Algorithm Pseudocode Flowcharts 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 5
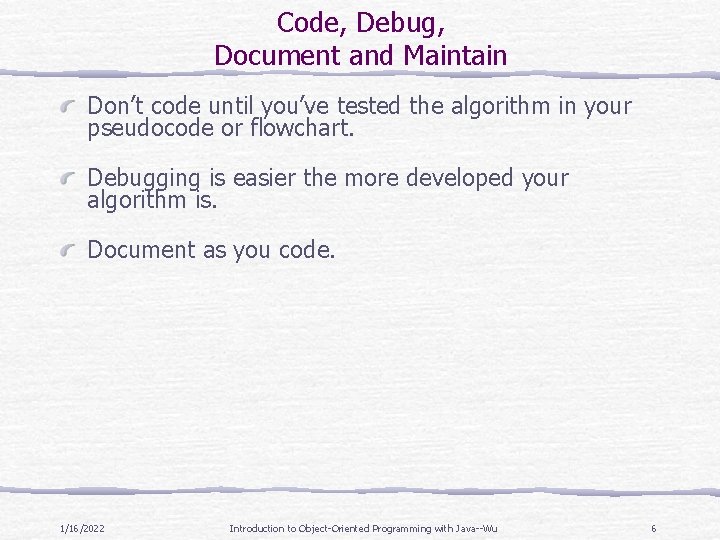
Code, Debug, Document and Maintain Don’t code until you’ve tested the algorithm in your pseudocode or flowchart. Debugging is easier the more developed your algorithm is. Document as you code. 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 6
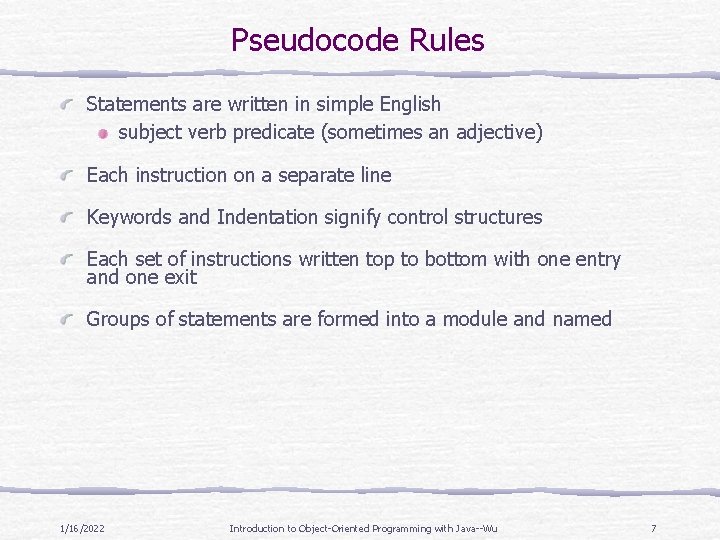
Pseudocode Rules Statements are written in simple English subject verb predicate (sometimes an adjective) Each instruction on a separate line Keywords and Indentation signify control structures Each set of instructions written top to bottom with one entry and one exit Groups of statements are formed into a module and named 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 7
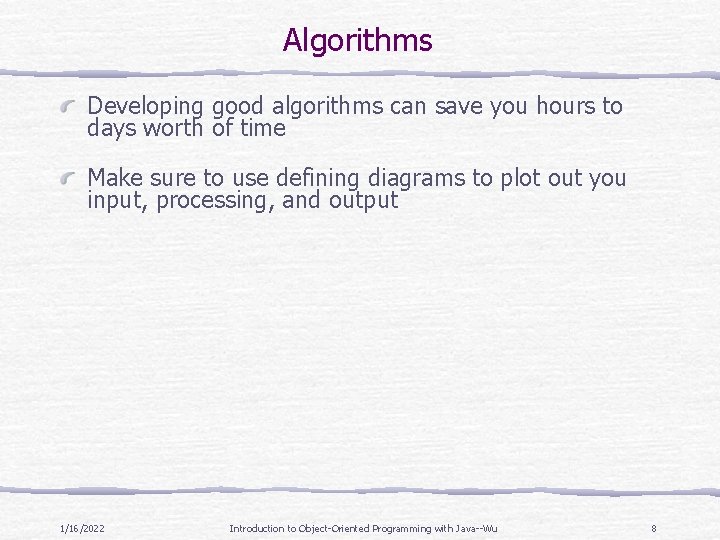
Algorithms Developing good algorithms can save you hours to days worth of time Make sure to use defining diagrams to plot out you input, processing, and output 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 8
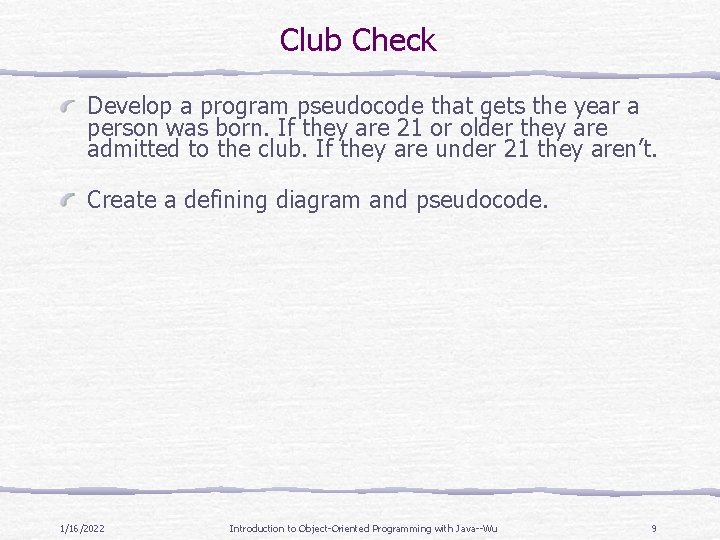
Club Check Develop a program pseudocode that gets the year a person was born. If they are 21 or older they are admitted to the club. If they are under 21 they aren’t. Create a defining diagram and pseudocode. 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 9
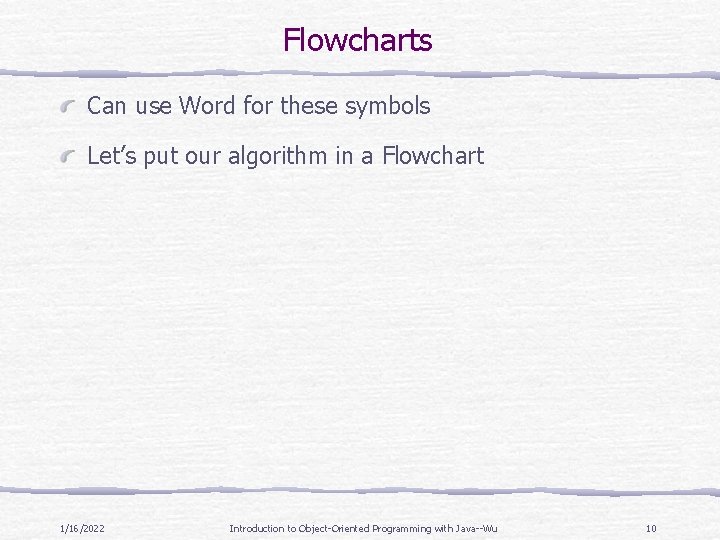
Flowcharts Can use Word for these symbols Let’s put our algorithm in a Flowchart 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 10
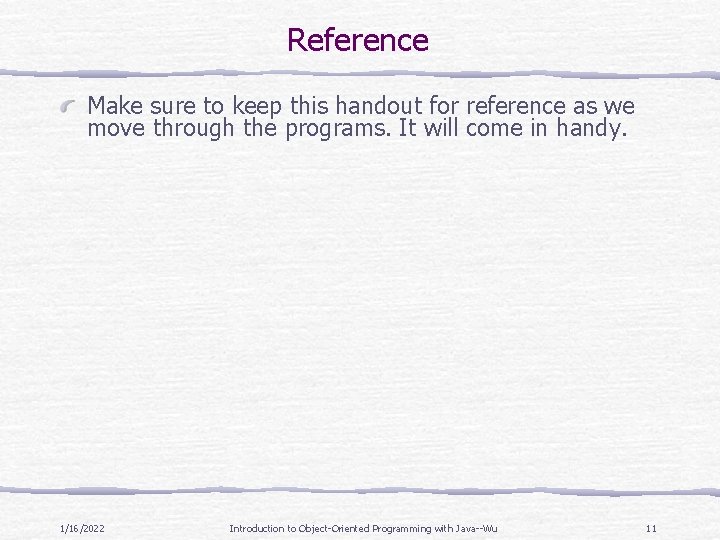
Reference Make sure to keep this handout for reference as we move through the programs. It will come in handy. 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 11
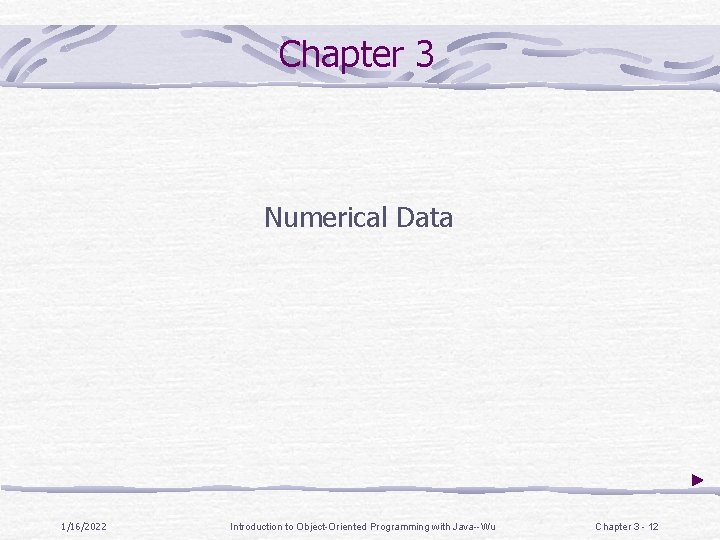
Chapter 3 Numerical Data 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu Chapter 3 - 12
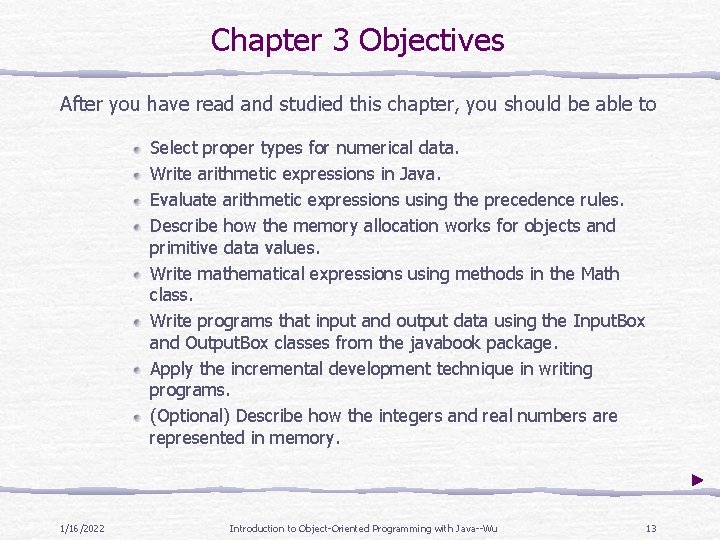
Chapter 3 Objectives After you have read and studied this chapter, you should be able to Select proper types for numerical data. Write arithmetic expressions in Java. Evaluate arithmetic expressions using the precedence rules. Describe how the memory allocation works for objects and primitive data values. Write mathematical expressions using methods in the Math class. Write programs that input and output data using the Input. Box and Output. Box classes from the javabook package. Apply the incremental development technique in writing programs. (Optional) Describe how the integers and real numbers are represented in memory. 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 13
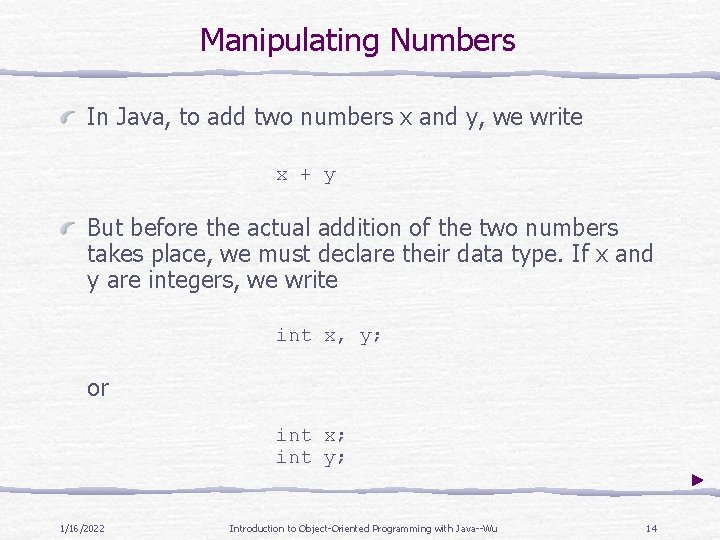
Manipulating Numbers In Java, to add two numbers x and y, we write x + y But before the actual addition of the two numbers takes place, we must declare their data type. If x and y are integers, we write int x, y; or int x; int y; 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 14
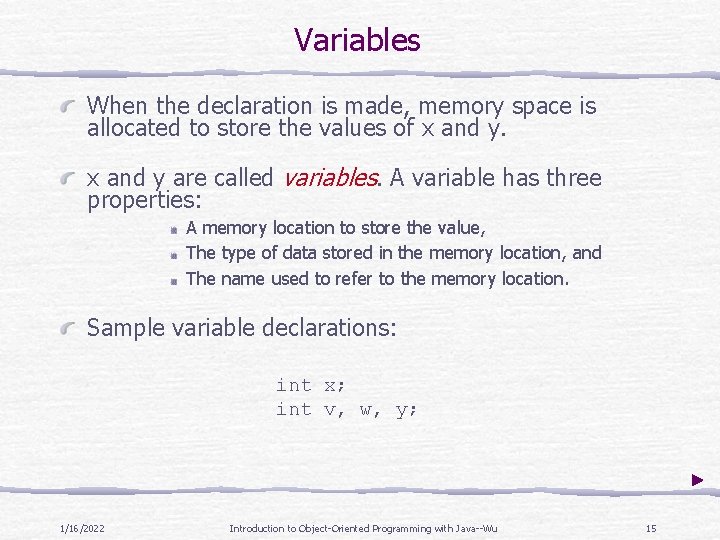
Variables When the declaration is made, memory space is allocated to store the values of x and y are called variables. A variable has three properties: A memory location to store the value, The type of data stored in the memory location, and The name used to refer to the memory location. Sample variable declarations: int x; int v, w, y; 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 15
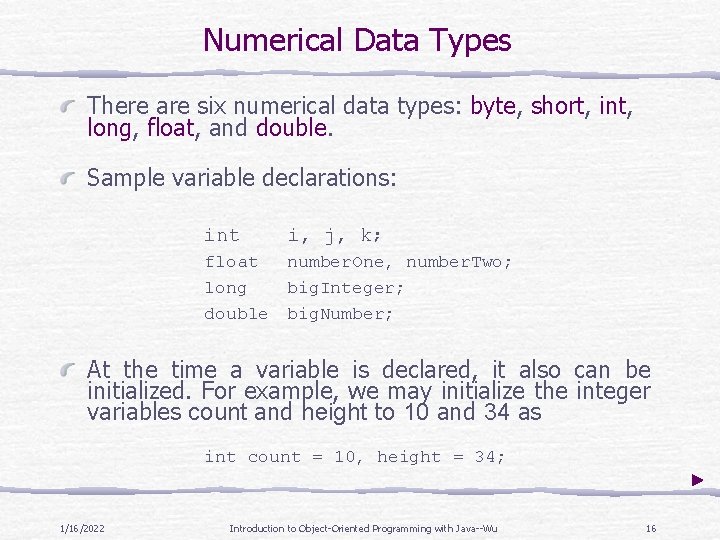
Numerical Data Types There are six numerical data types: byte, short, int, long, float, and double. Sample variable declarations: int i, j, k; float long double number. One, number. Two; big. Integer; big. Number; At the time a variable is declared, it also can be initialized. For example, we may initialize the integer variables count and height to 10 and 34 as int count = 10, height = 34; 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 16
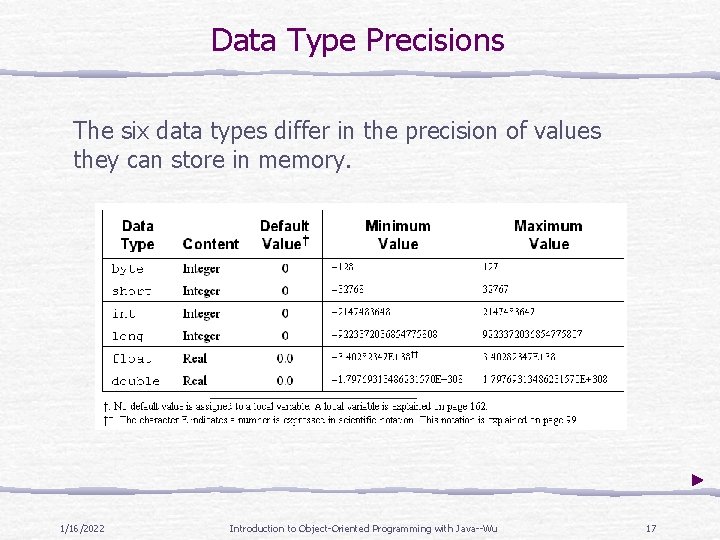
Data Type Precisions The six data types differ in the precision of values they can store in memory. 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 17
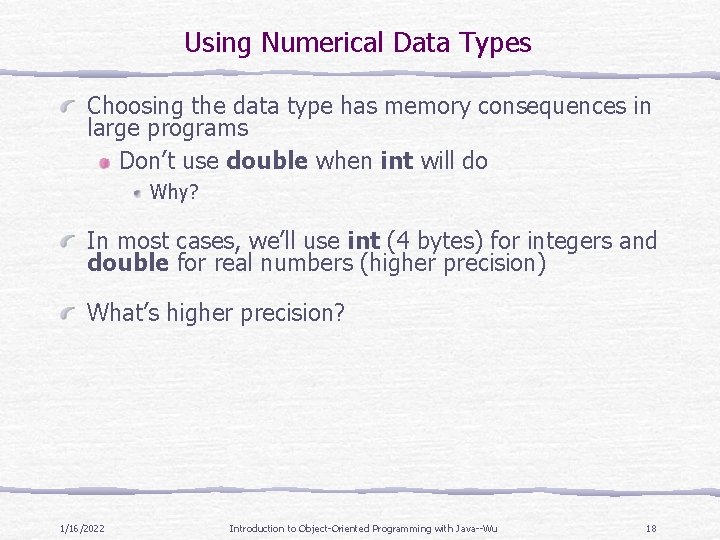
Using Numerical Data Types Choosing the data type has memory consequences in large programs Don’t use double when int will do Why? In most cases, we’ll use int (4 bytes) for integers and double for real numbers (higher precision) What’s higher precision? 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 18
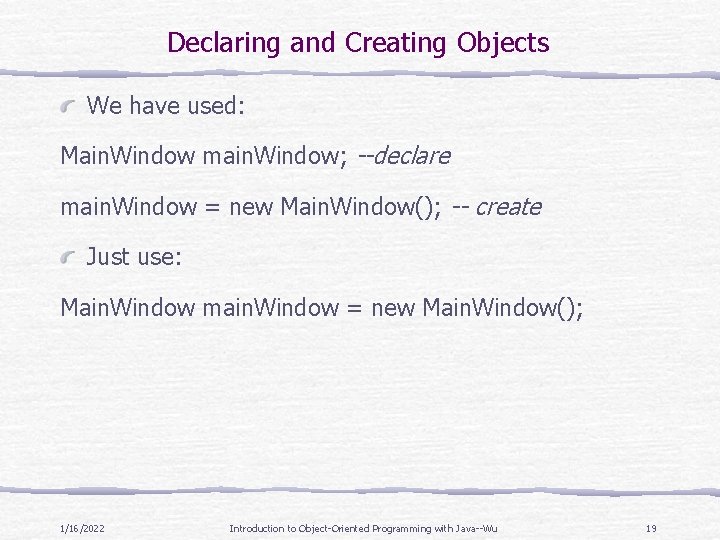
Declaring and Creating Objects We have used: Main. Window main. Window; --declare main. Window = new Main. Window(); -- create Just use: Main. Window main. Window = new Main. Window(); 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 19
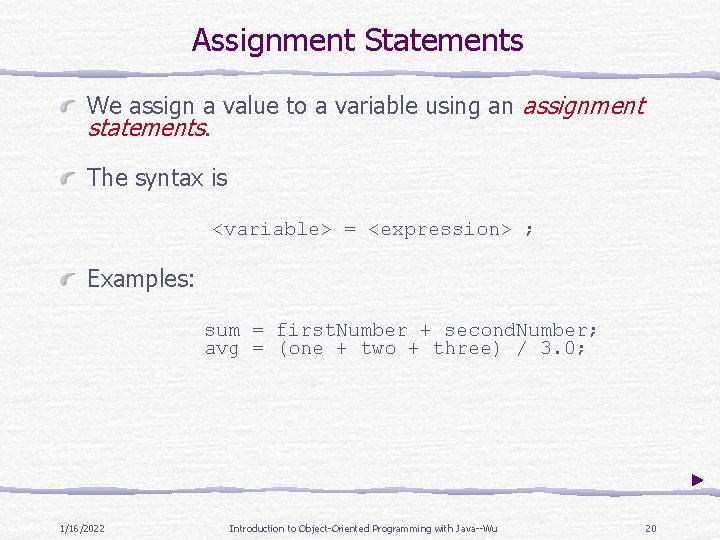
Assignment Statements We assign a value to a variable using an assignment statements. The syntax is <variable> = <expression> ; Examples: sum = first. Number + second. Number; avg = (one + two + three) / 3. 0; 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 20
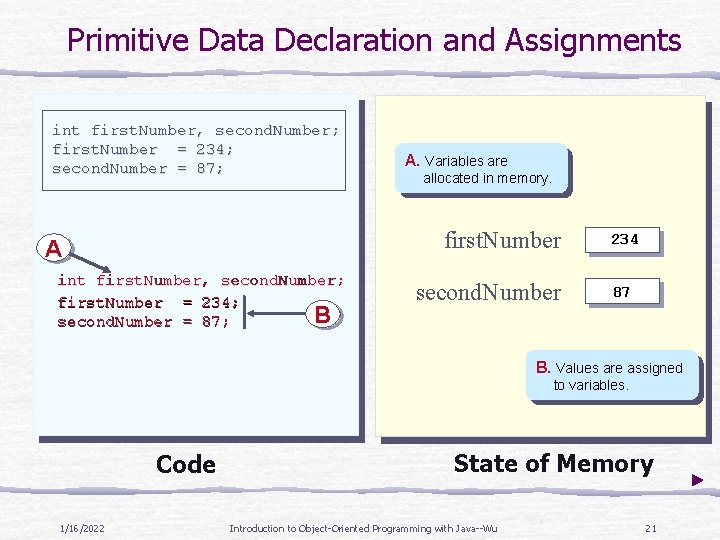
Primitive Data Declaration and Assignments int first. Number, second. Number; first. Number = 234; second. Number = 87; A. Variables are allocated in memory. first. Number A int first. Number, , second. Number; ; first. Number = 234; B second. Number = 87; second. Number 234 87 B. Values are assigned to variables. Code 1/16/2022 State of Memory Introduction to Object-Oriented Programming with Java--Wu 21
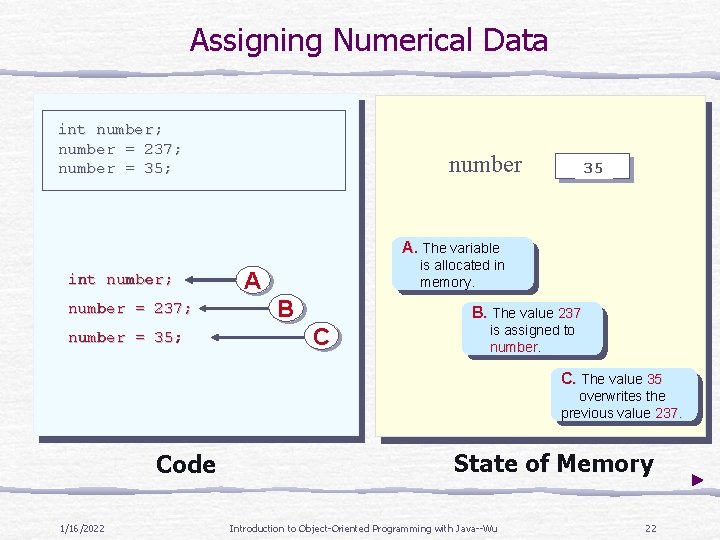
Assigning Numerical Data int number; number = 237; number = 35; number 35 237 A. The variable int number; number = 237; number = 35; is allocated in memory. A B B. The value 237 C is assigned to number C. The value 35 overwrites the previous value 237. Code 1/16/2022 State of Memory Introduction to Object-Oriented Programming with Java--Wu 22
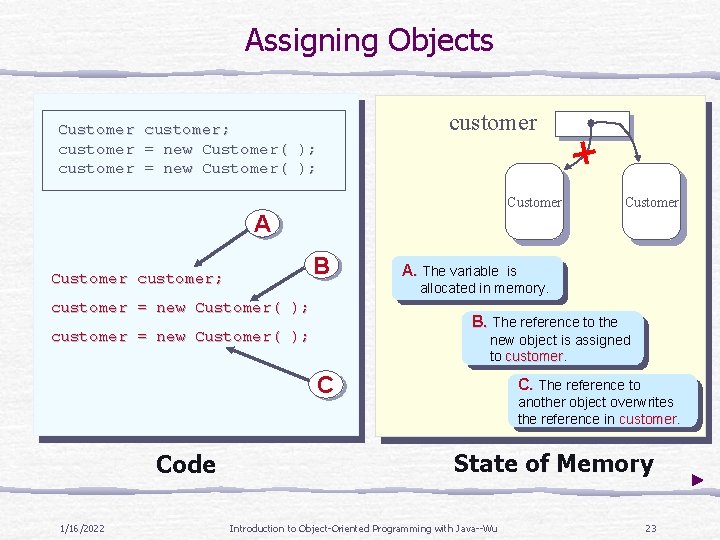
Assigning Objects Customer customer; customer = new Customer( ); customer Customer A B Customer customer; A. The variable is allocated in memory. customer = new Customer( ); B. The reference to the customer = new Customer( ); new object is assigned to customer C Code 1/16/2022 C. The reference to another object overwrites the reference in customer. State of Memory Introduction to Object-Oriented Programming with Java--Wu 23
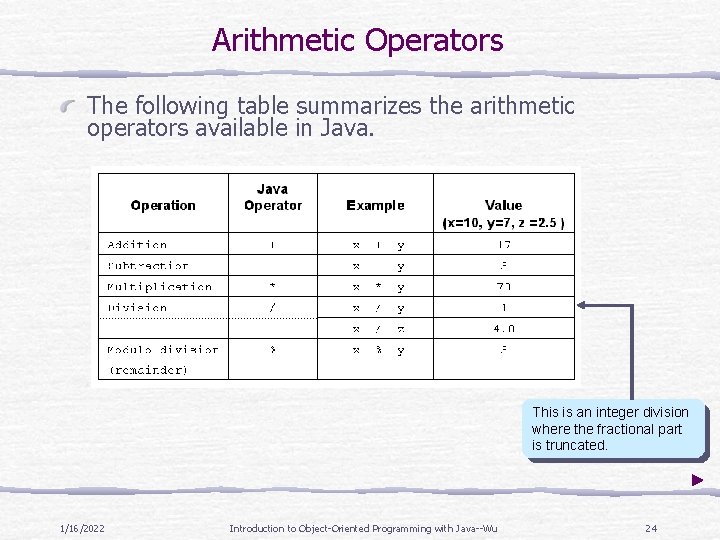
Arithmetic Operators The following table summarizes the arithmetic operators available in Java. This is an integer division where the fractional part is truncated. 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 24
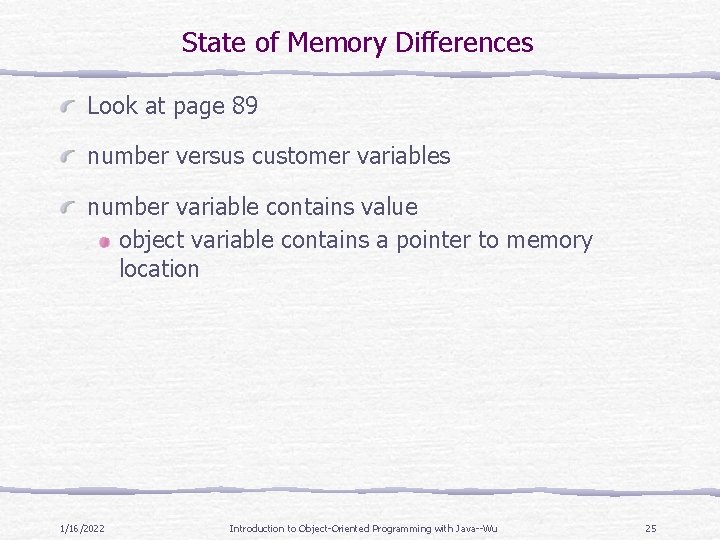
State of Memory Differences Look at page 89 number versus customer variables number variable contains value object variable contains a pointer to memory location 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 25
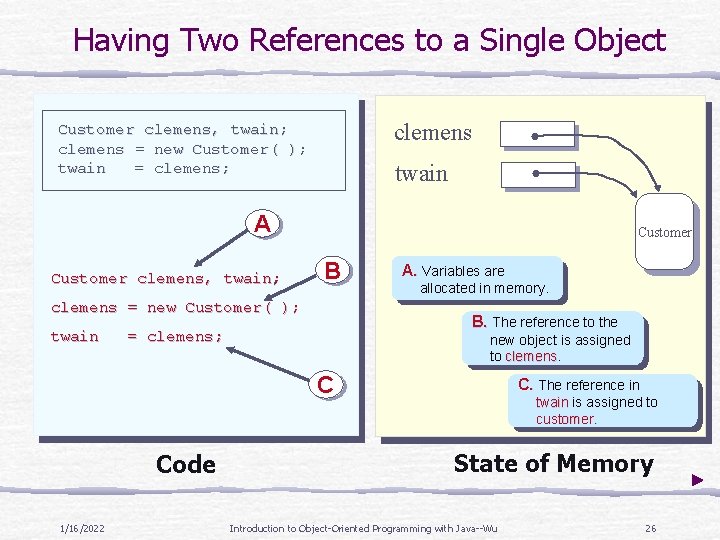
Having Two References to a Single Object clemens Customer clemens, twain; clemens = new Customer( ); twain = clemens; twain A Customer clemens, twain; Customer B clemens = new Customer( ); twain A. Variables are allocated in memory. B. The reference to the = clemens; new object is assigned to clemens C Code 1/16/2022 C. The reference in twain is assigned to customer. State of Memory Introduction to Object-Oriented Programming with Java--Wu 26
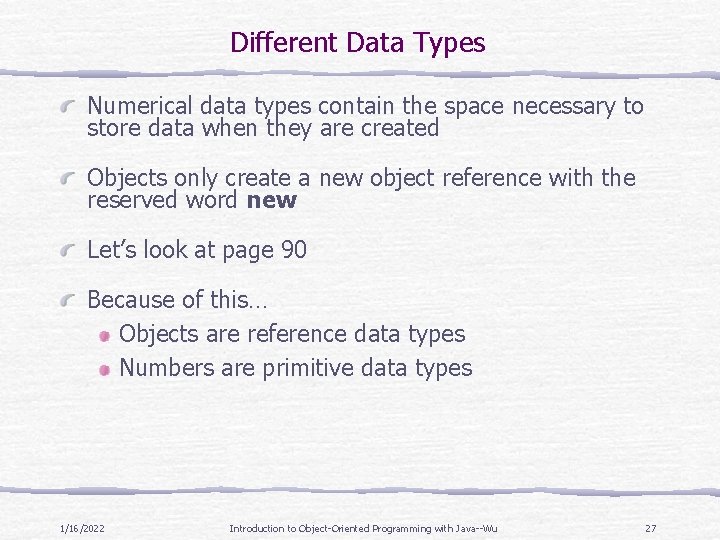
Different Data Types Numerical data types contain the space necessary to store data when they are created Objects only create a new object reference with the reserved word new Let’s look at page 90 Because of this… Objects are reference data types Numbers are primitive data types 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 27
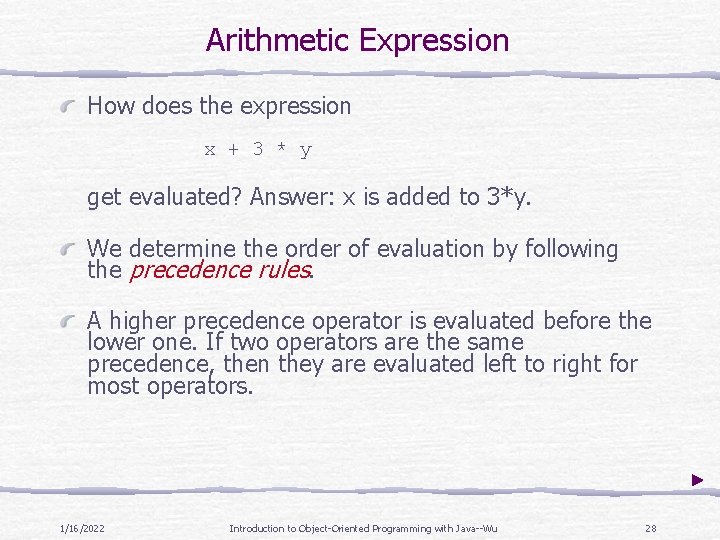
Arithmetic Expression How does the expression x + 3 * y get evaluated? Answer: x is added to 3*y. We determine the order of evaluation by following the precedence rules. A higher precedence operator is evaluated before the lower one. If two operators are the same precedence, then they are evaluated left to right for most operators. 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 28
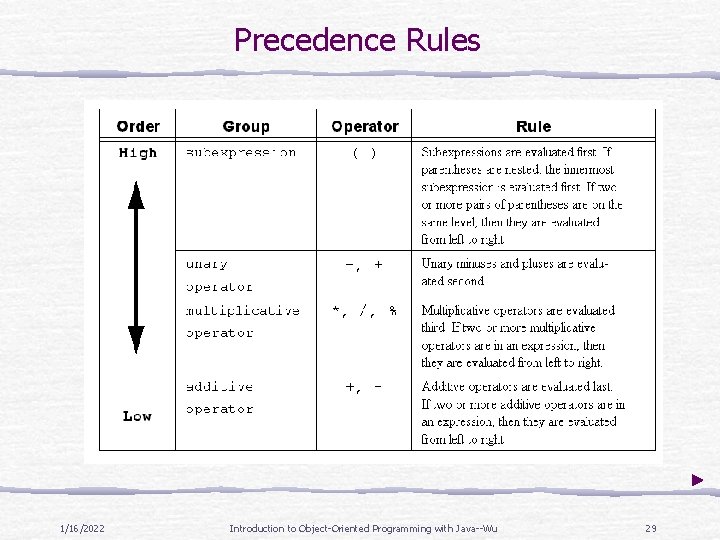
Precedence Rules 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 29
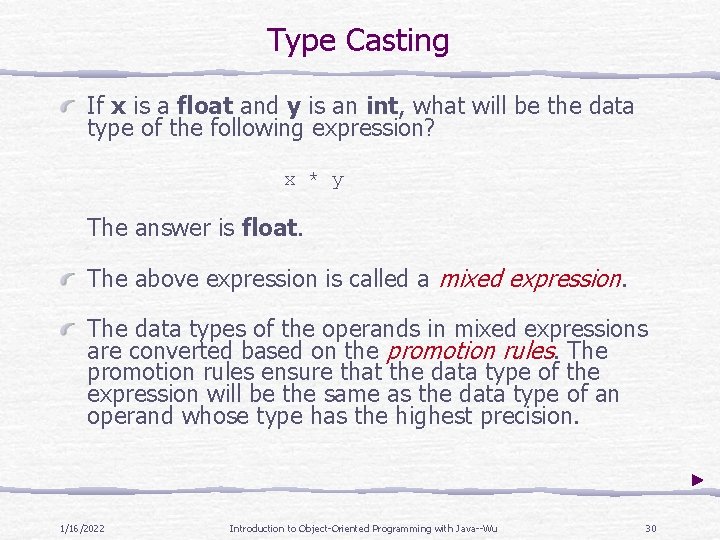
Type Casting If x is a float and y is an int, what will be the data type of the following expression? x * y The answer is float. The above expression is called a mixed expression. The data types of the operands in mixed expressions are converted based on the promotion rules. The promotion rules ensure that the data type of the expression will be the same as the data type of an operand whose type has the highest precision. 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 30
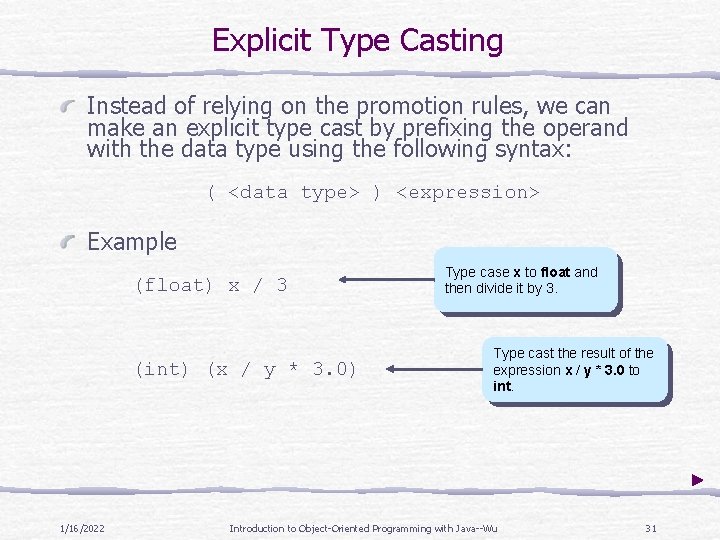
Explicit Type Casting Instead of relying on the promotion rules, we can make an explicit type cast by prefixing the operand with the data type using the following syntax: ( <data type> ) <expression> Example (float) x / 3 (int) (x / y * 3. 0) 1/16/2022 Type case x to float and then divide it by 3. Type cast the result of the expression x / y * 3. 0 to int. Introduction to Object-Oriented Programming with Java--Wu 31
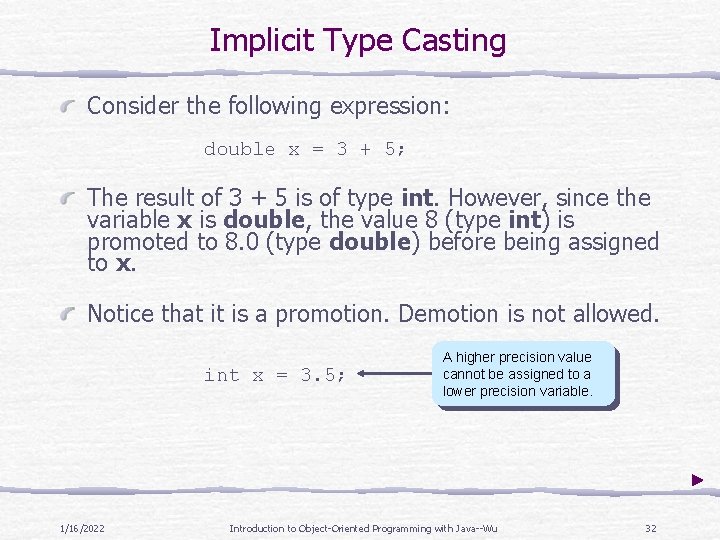
Implicit Type Casting Consider the following expression: double x = 3 + 5; The result of 3 + 5 is of type int. However, since the variable x is double, the value 8 (type int) is promoted to 8. 0 (type double) before being assigned to x. Notice that it is a promotion. Demotion is not allowed. int x = 3. 5; 1/16/2022 A higher precision value cannot be assigned to a lower precision variable. Introduction to Object-Oriented Programming with Java--Wu 32
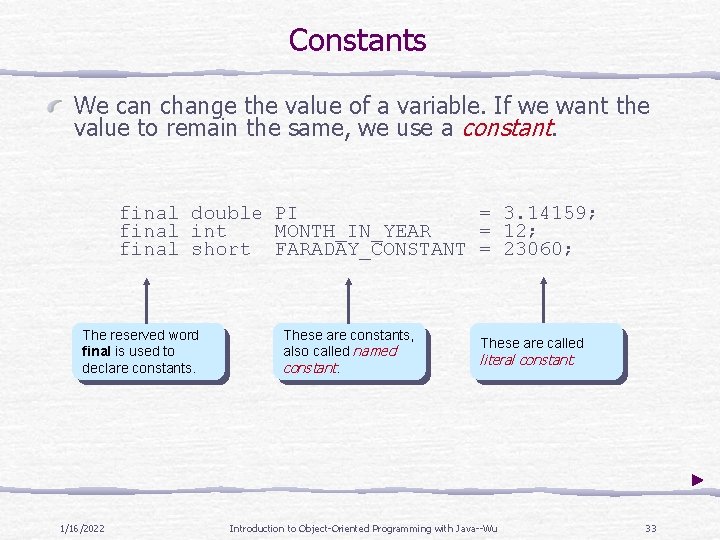
Constants We can change the value of a variable. If we want the value to remain the same, we use a constant. final double PI = 3. 14159; final int MONTH_IN_YEAR = 12; final short FARADAY_CONSTANT = 23060; The reserved word final is used to declare constants. 1/16/2022 These are constants, also called named constant. These are called literal constant. Introduction to Object-Oriented Programming with Java--Wu 33
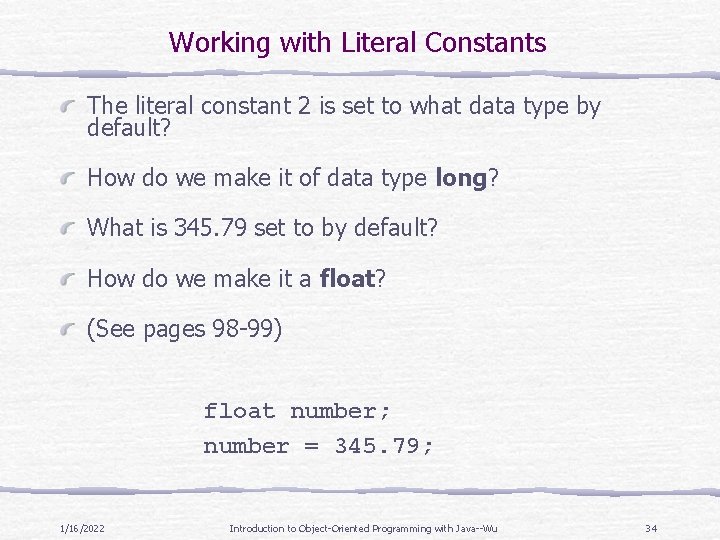
Working with Literal Constants The literal constant 2 is set to what data type by default? How do we make it of data type long? What is 345. 79 set to by default? How do we make it a float? (See pages 98 -99) float number; number = 345. 79; 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 34
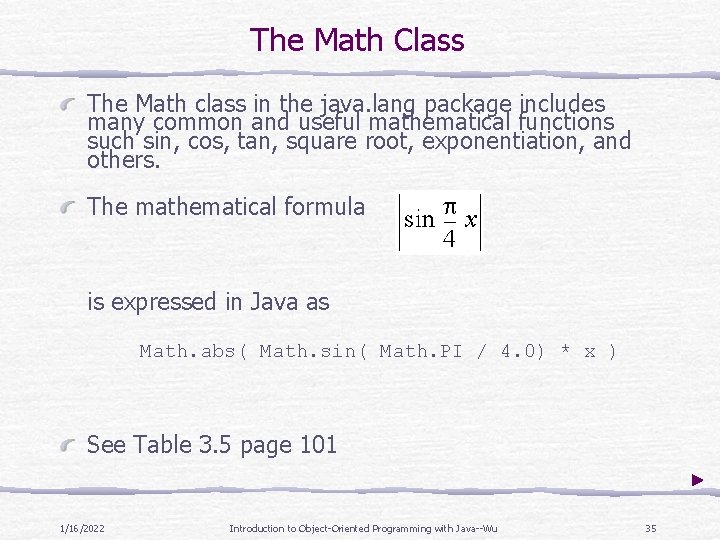
The Math Class The Math class in the java. lang package includes many common and useful mathematical functions such sin, cos, tan, square root, exponentiation, and others. The mathematical formula is expressed in Java as Math. abs( Math. sin( Math. PI / 4. 0) * x ) See Table 3. 5 page 101 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 35
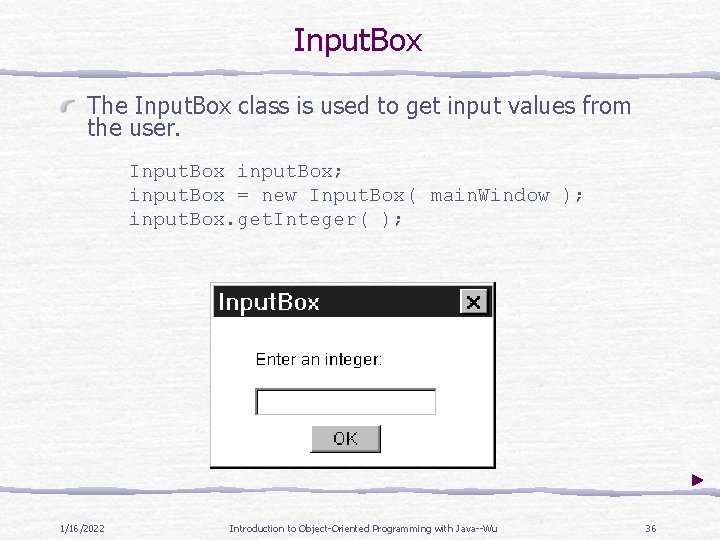
Input. Box The Input. Box class is used to get input values from the user. Input. Box input. Box; input. Box = new Input. Box( main. Window ); input. Box. get. Integer( ); 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 36
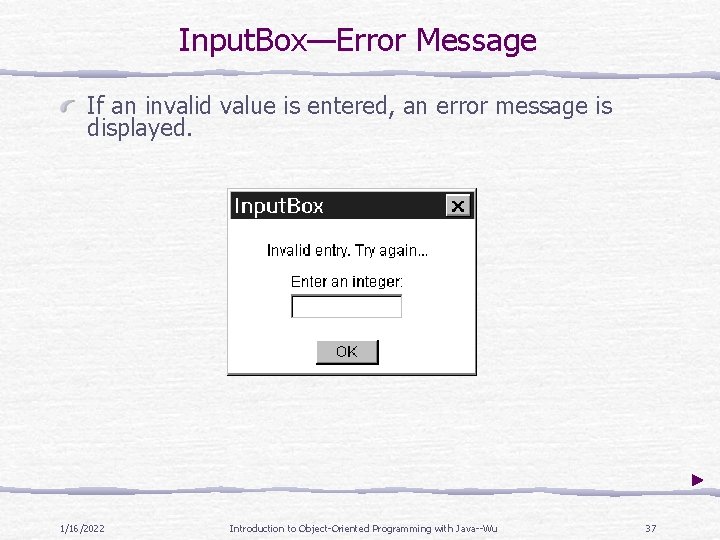
Input. Box—Error Message If an invalid value is entered, an error message is displayed. 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 37
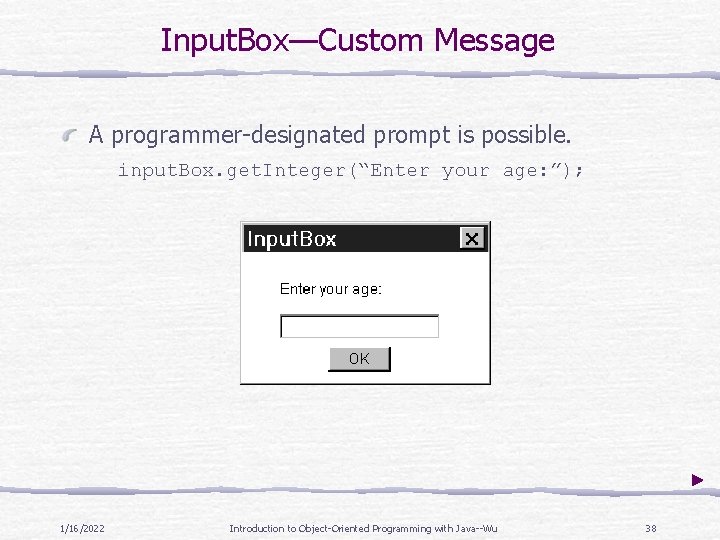
Input. Box—Custom Message A programmer-designated prompt is possible. input. Box. get. Integer(“Enter your age: ”); 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 38
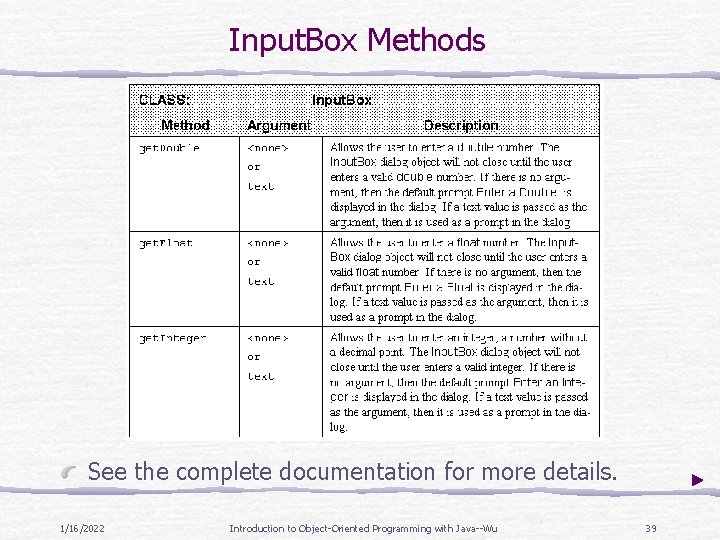
Input. Box Methods See the complete documentation for more details. 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 39
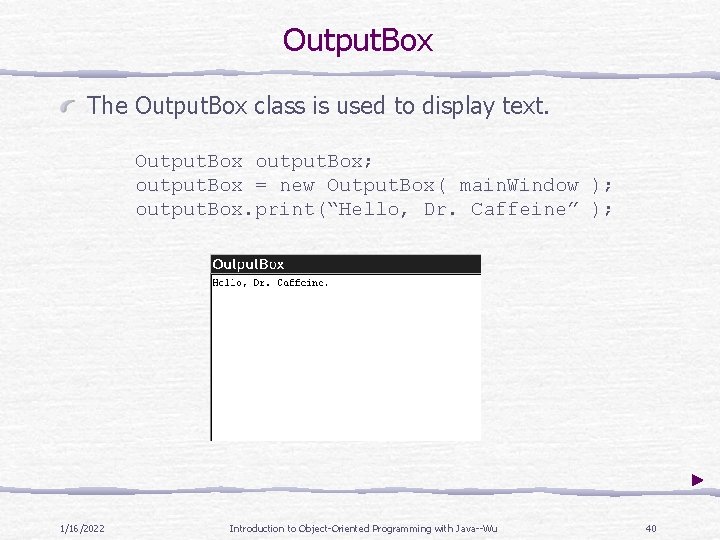
Output. Box The Output. Box class is used to display text. Output. Box output. Box; output. Box = new Output. Box( main. Window ); output. Box. print(“Hello, Dr. Caffeine” ); 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 40
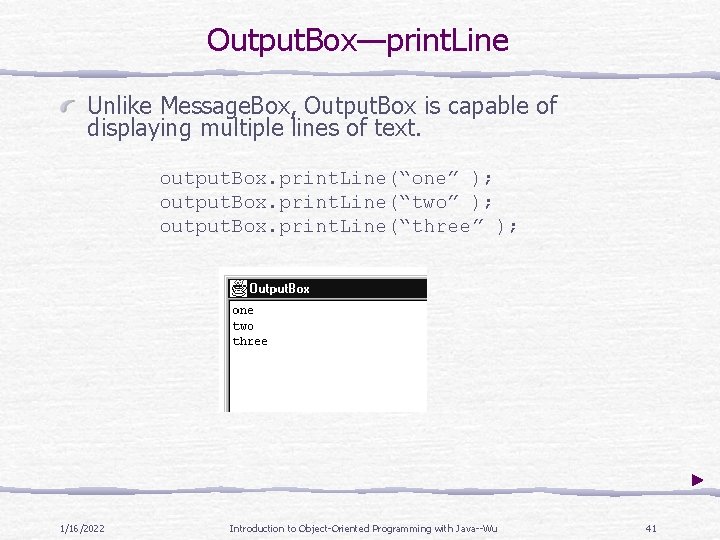
Output. Box—print. Line Unlike Message. Box, Output. Box is capable of displaying multiple lines of text. output. Box. print. Line(“one” ); output. Box. print. Line(“two” ); output. Box. print. Line(“three” ); 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 41
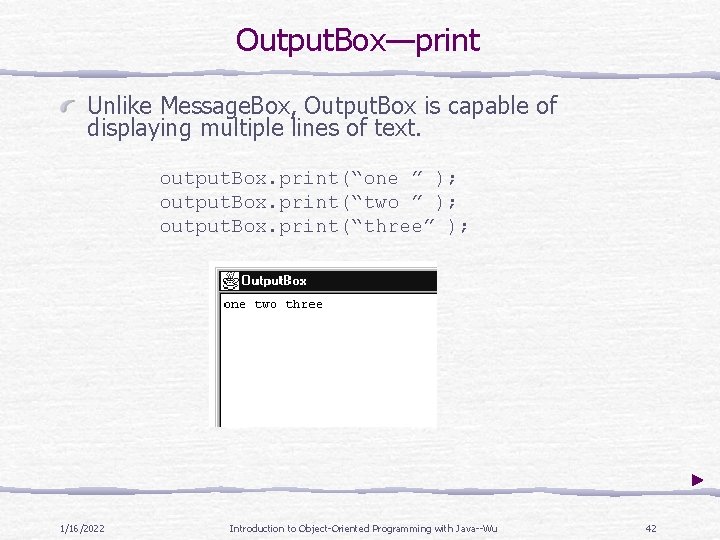
Output. Box—print Unlike Message. Box, Output. Box is capable of displaying multiple lines of text. output. Box. print(“one ” ); output. Box. print(“two ” ); output. Box. print(“three” ); 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 42
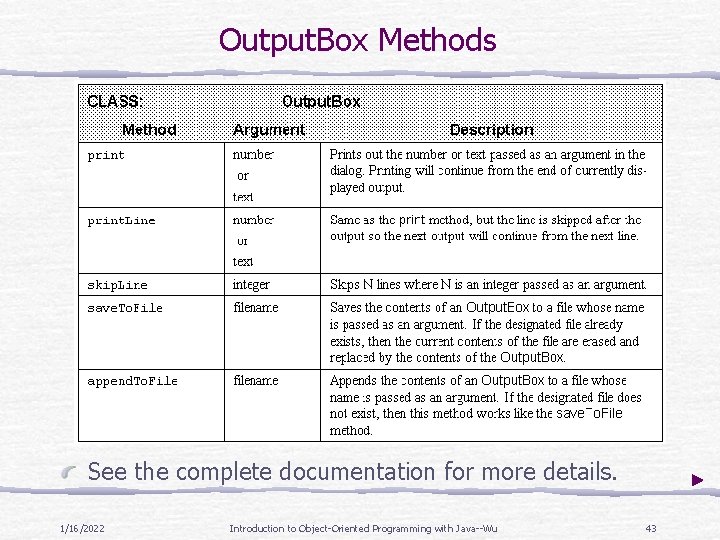
Output. Box Methods See the complete documentation for more details. 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 43
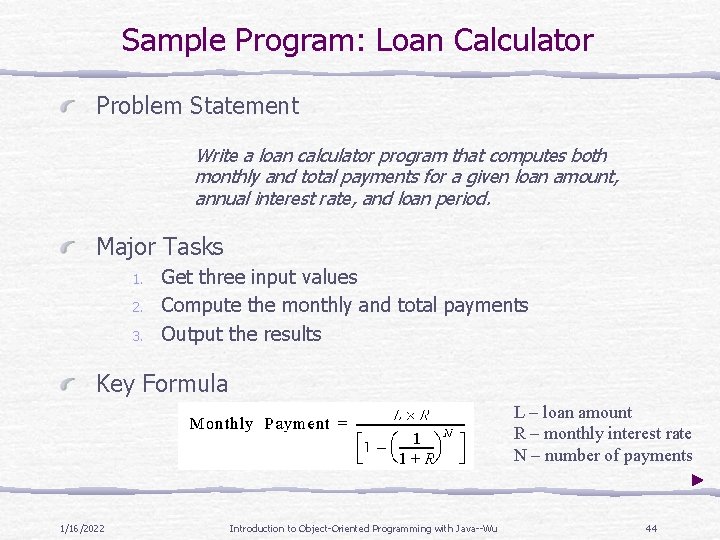
Sample Program: Loan Calculator Problem Statement Write a loan calculator program that computes both monthly and total payments for a given loan amount, annual interest rate, and loan period. Major Tasks 1. 2. 3. Get three input values Compute the monthly and total payments Output the results Key Formula L – loan amount R – monthly interest rate N – number of payments 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 44
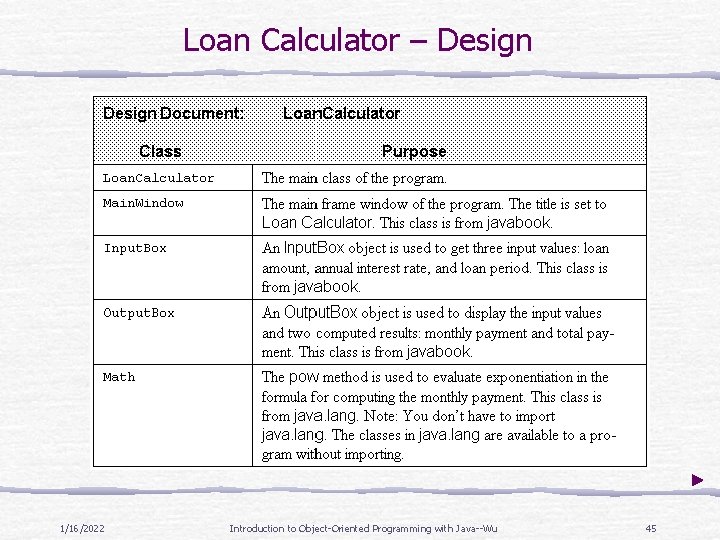
Loan Calculator – Design 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 45
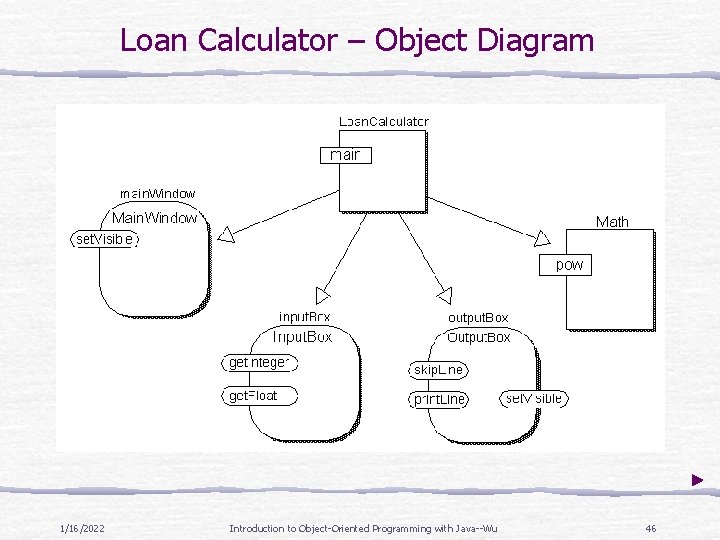
Loan Calculator – Object Diagram 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 46
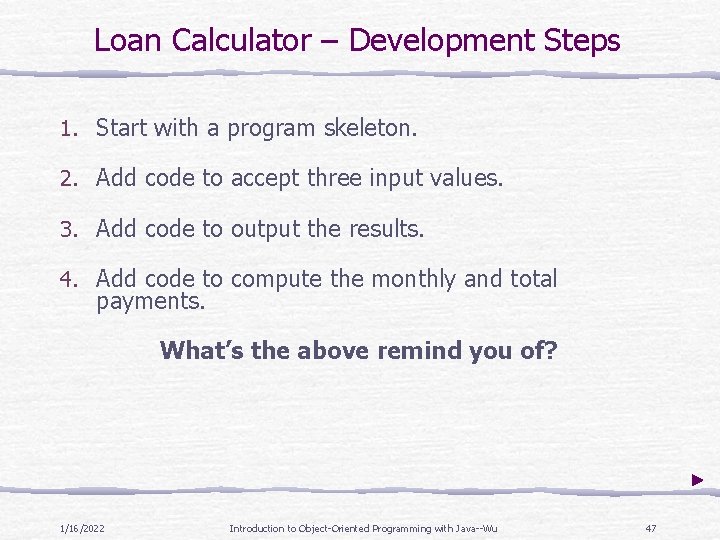
Loan Calculator – Development Steps 1. Start with a program skeleton. 2. Add code to accept three input values. 3. Add code to output the results. 4. Add code to compute the monthly and total payments. What’s the above remind you of? 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 47
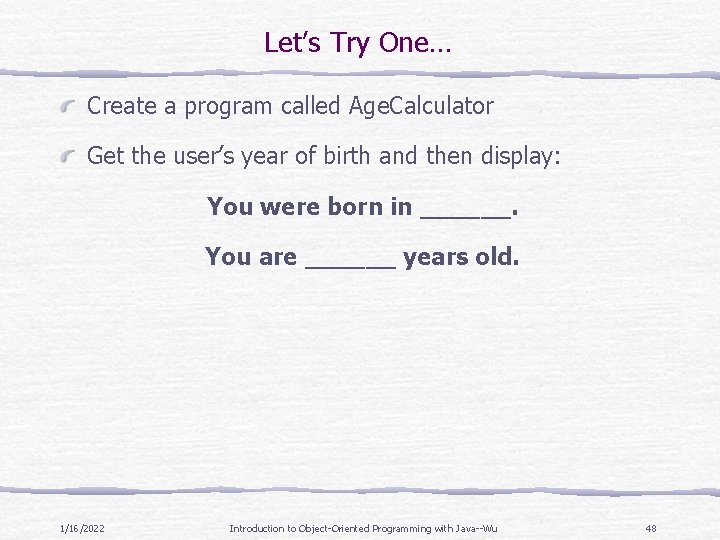
Let’s Try One… Create a program called Age. Calculator Get the user’s year of birth and then display: You were born in ______. You are ______ years old. 1/16/2022 Introduction to Object-Oriented Programming with Java--Wu 48
Algorithms and flowcharts
Flowchart symblos
Flowchart vs pseudocode
Algorithm vs flowchart
Hierarchy in pseudocode
Expressing algorithm
Xkcd time machine
Computational thinking algorithms and programming
Synchronization algorithms and concurrent programming
Dot product rules
A b a b c d e
How to solve problems programming
Perbedaan linear programming dan integer programming
Greedy algorithm vs dynamic programming
Runtime programming
Integer programming vs linear programming
Definisi linear
6-2 other ways of expressing the future
Measuring and expressing enthalpy changes
Likes and opinions
Expressing like and dislike dialogue
I wish past simple
Connectives contrast
17.2 measuring and expressing enthalpy changes
Expressing wishes and regrets
Expressing ability and inability ne demek
Reduction of adverbial clauses
Ability possibility obligation
Expressing opinions and feelings
Using and expressing measurements
In the help strategy, the h stands for healthful
Using and expressing measurements
Expressing interest and indifference
Design and analysis of algorithms syllabus
Ajit diwan iit bombay
Association analysis: basic concepts and algorithms
Computer arithmetic: algorithms and hardware designs
Kevin wayne princeton
Data structures and algorithms tutorial
Algorithms for select and join operations
Undecidable problems and unreasonable time algorithms.
Information retrieval data structures and algorithms
Data structures and algorithms bits pilani
Cluster analysis basic concepts and algorithms
Probabilistic analysis and randomized algorithms
Design and analysis of algorithms introduction
Algorithms for query processing and optimization
Parallel and distributed algorithms
Ajit diwan