Overview of the Composite Application Guidance for WPF
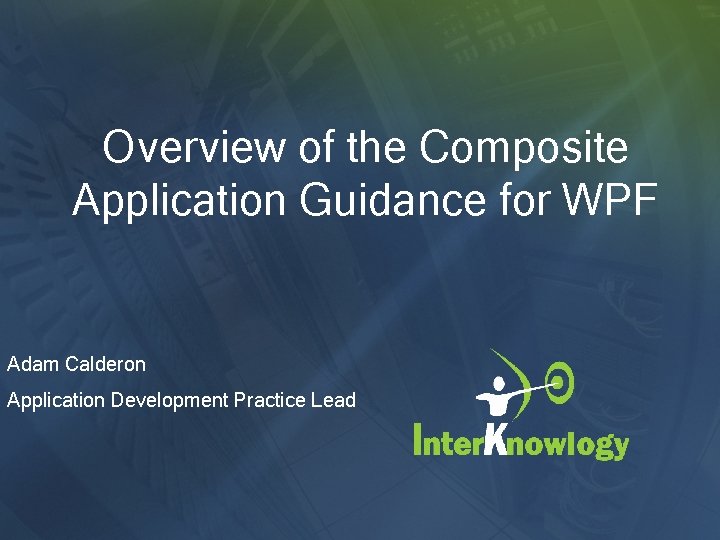
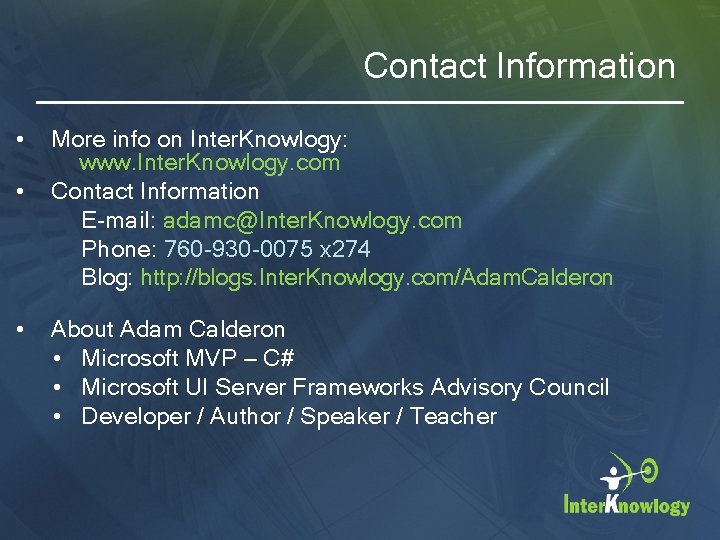
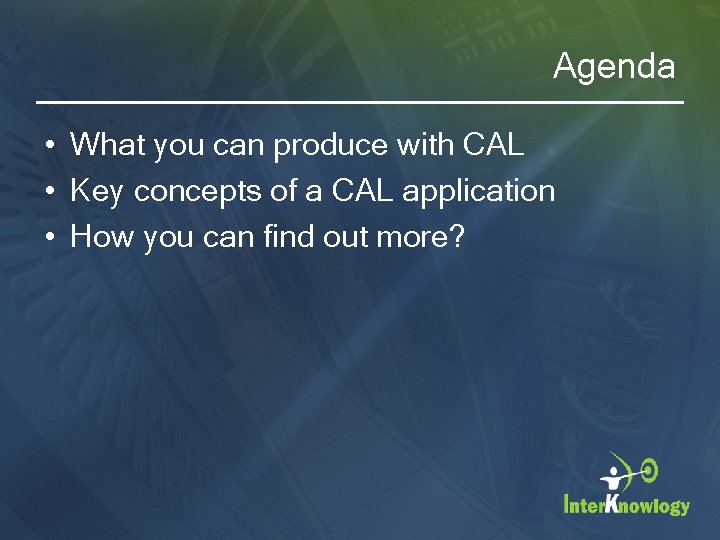
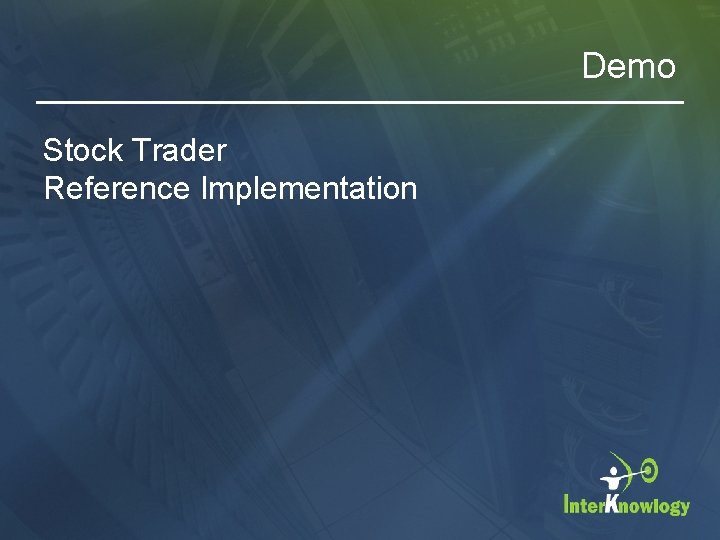
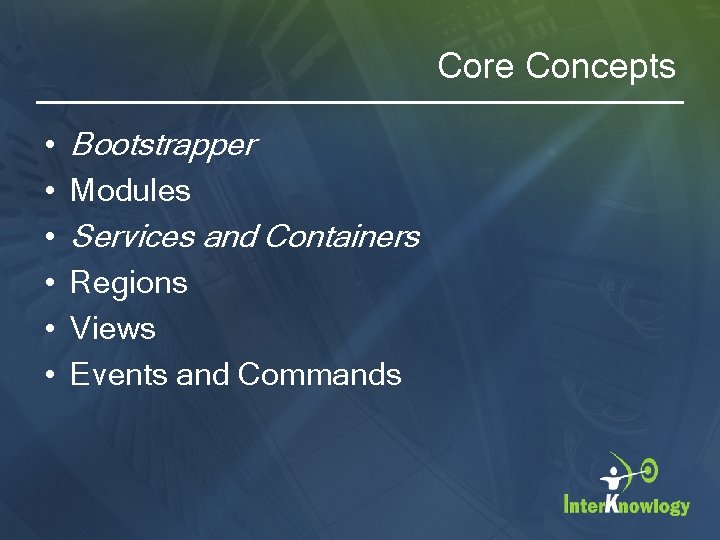
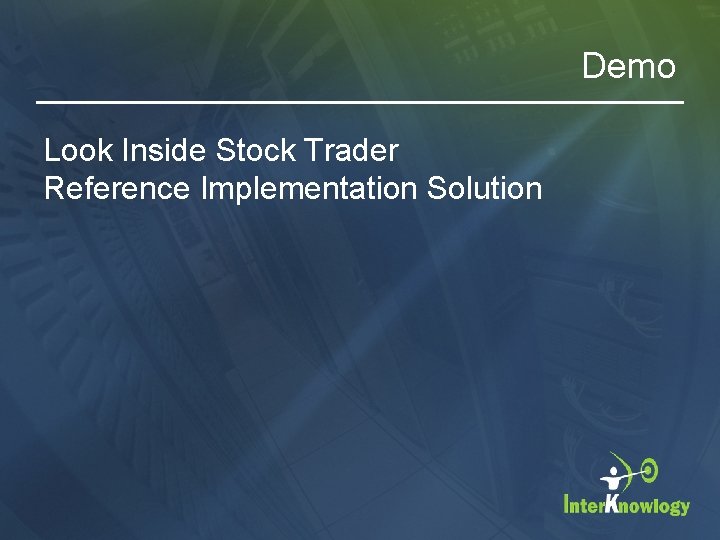
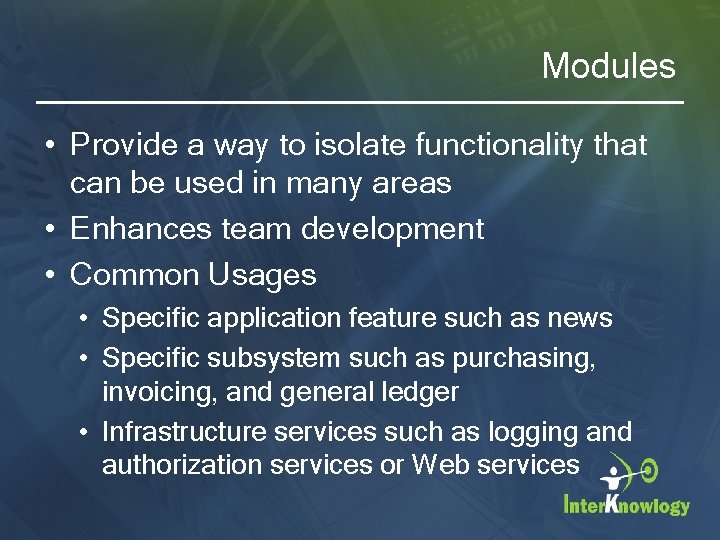
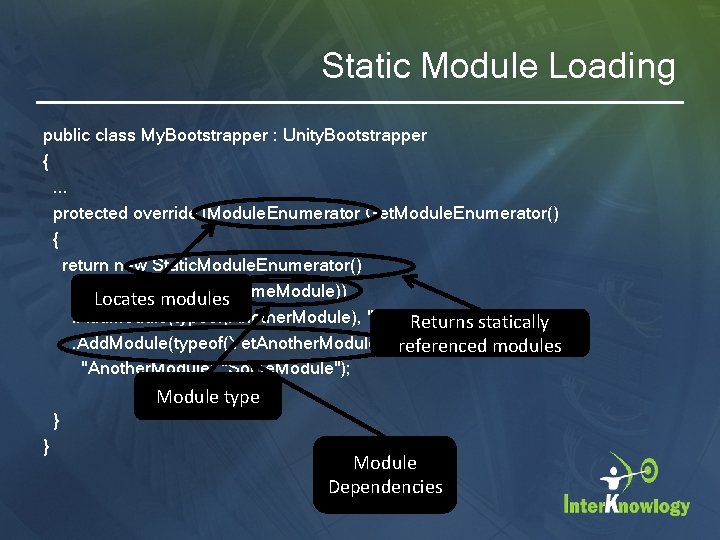
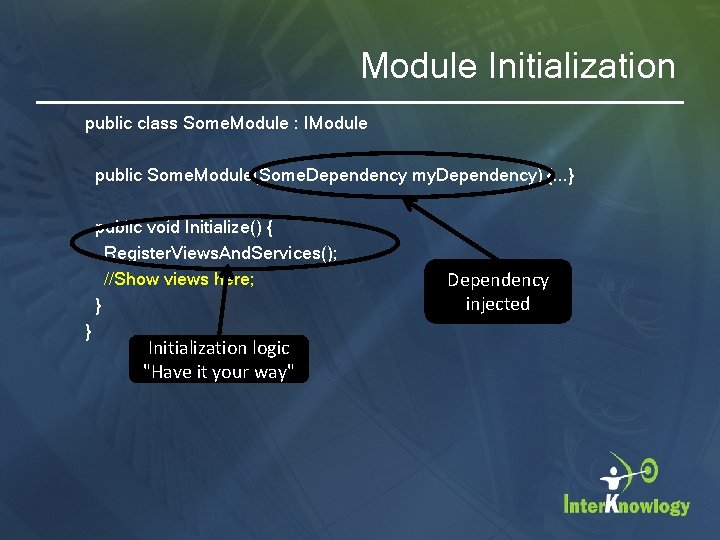
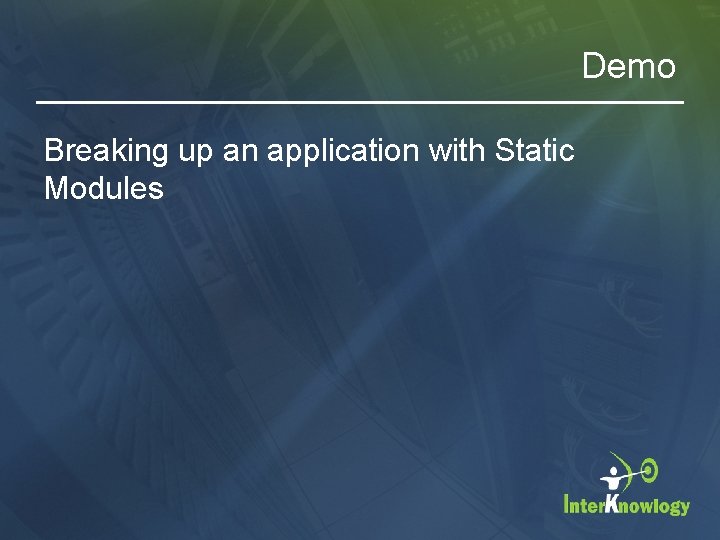
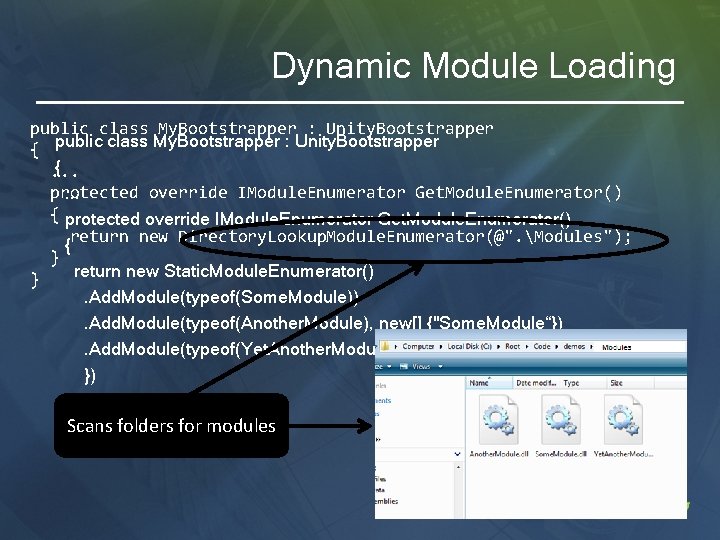
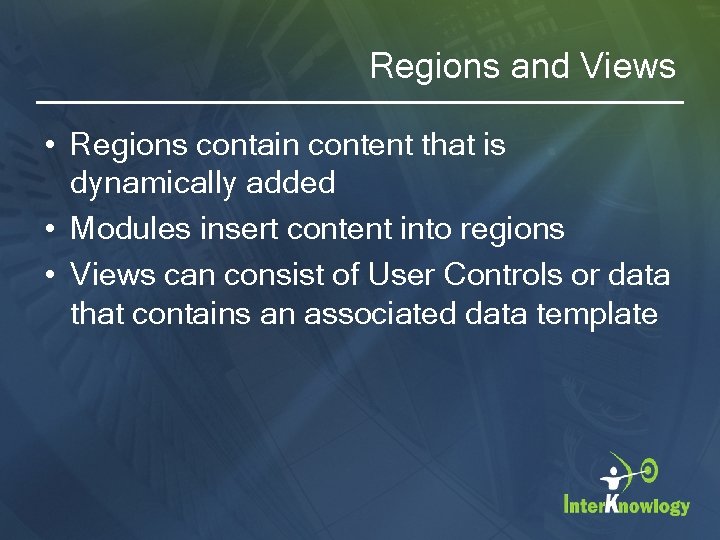
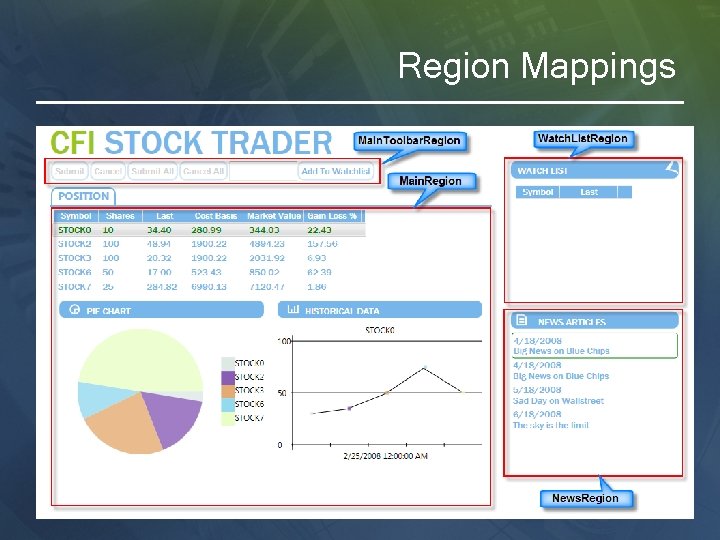
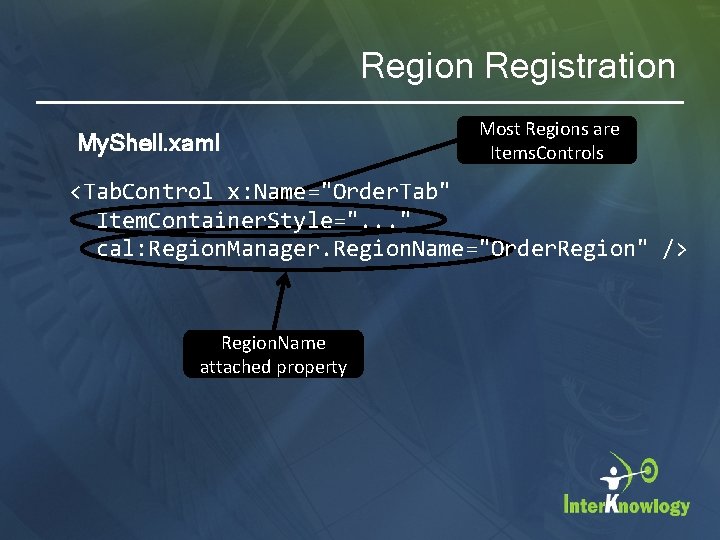
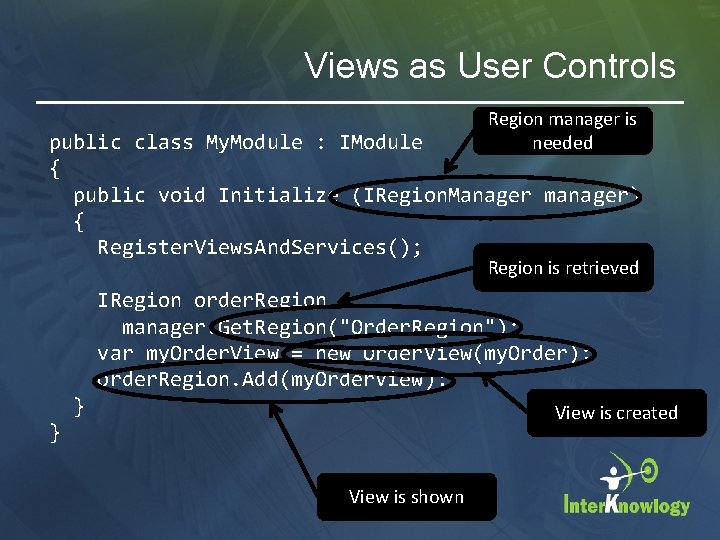
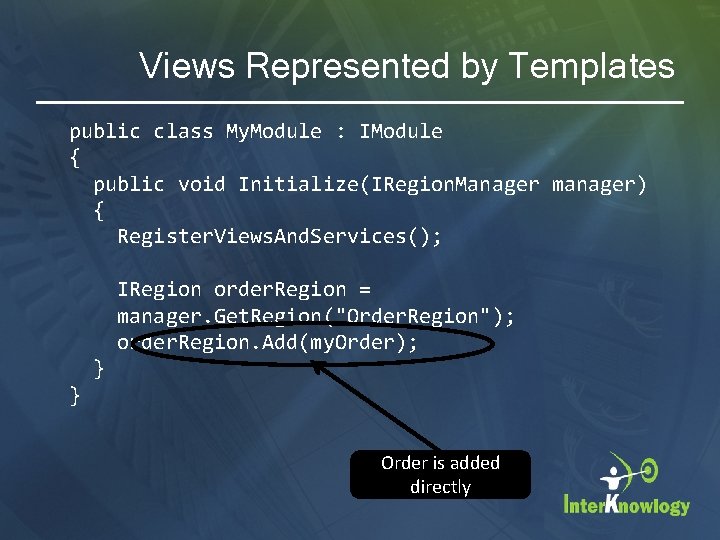
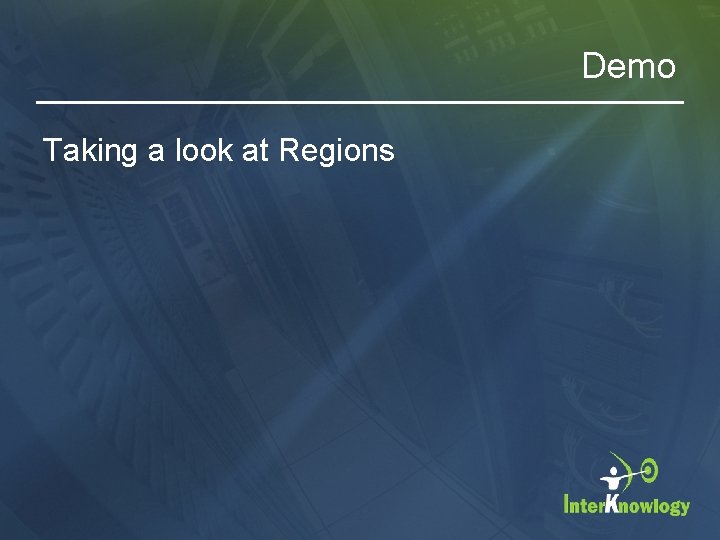
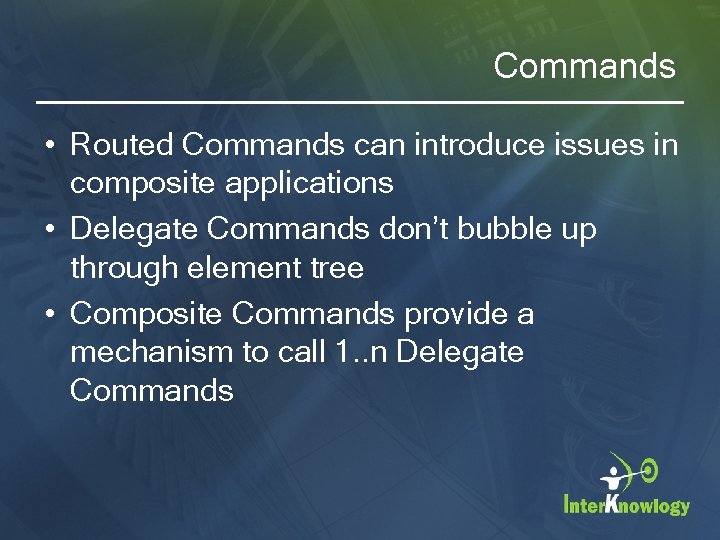
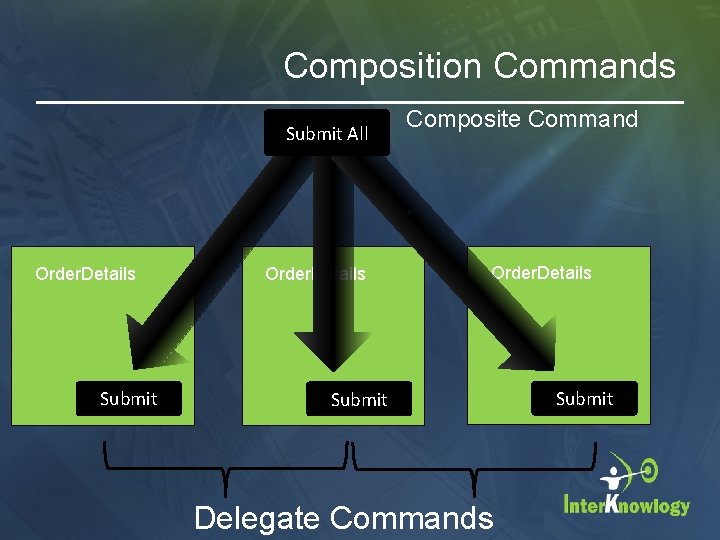
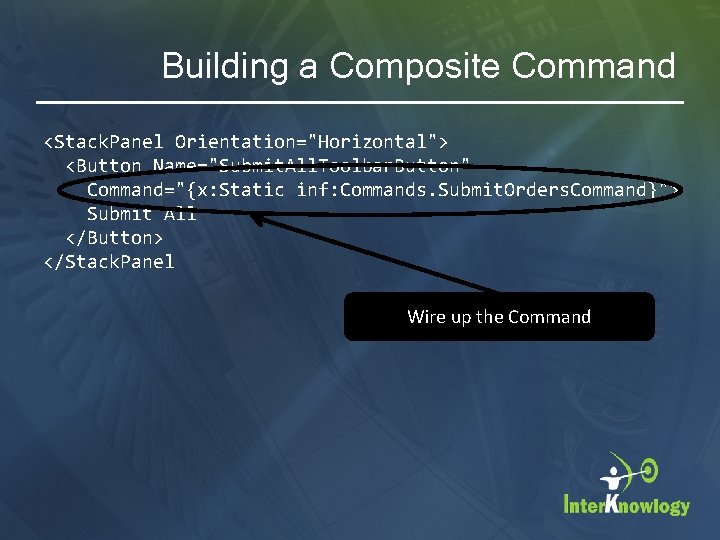
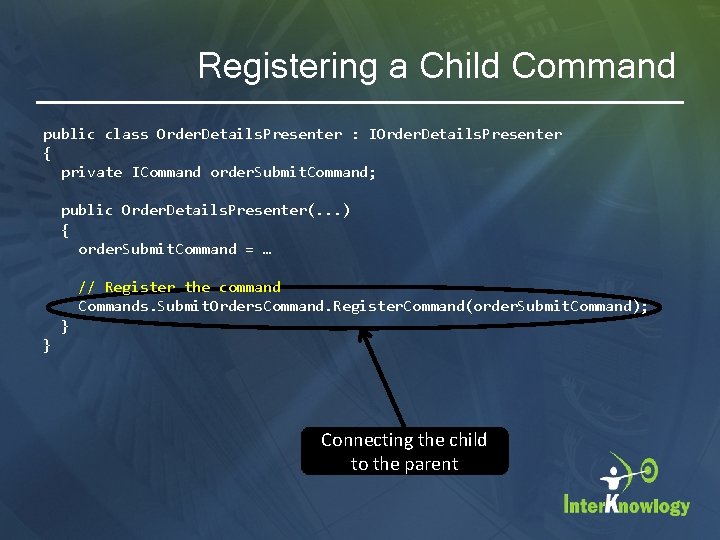
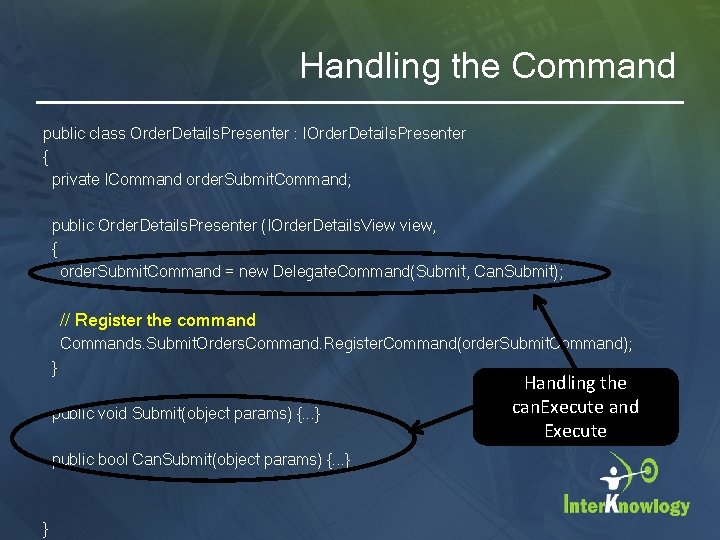
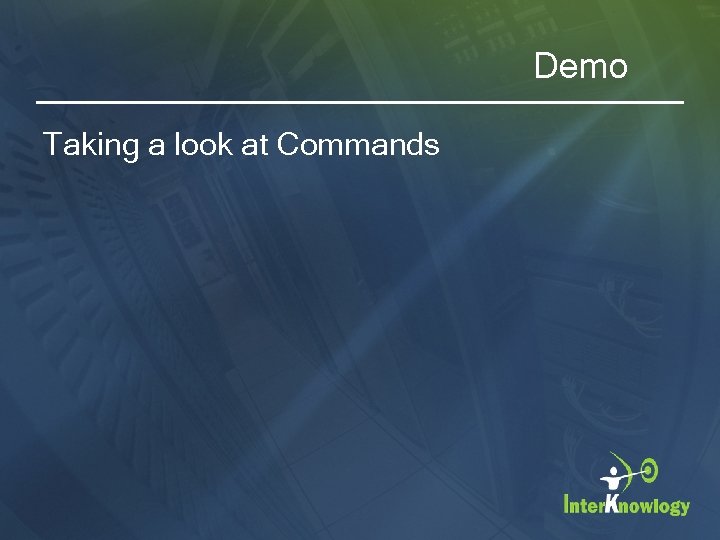
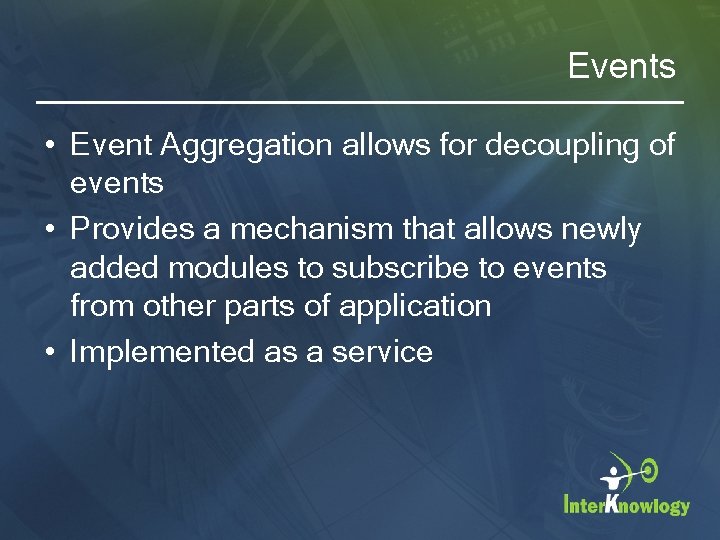
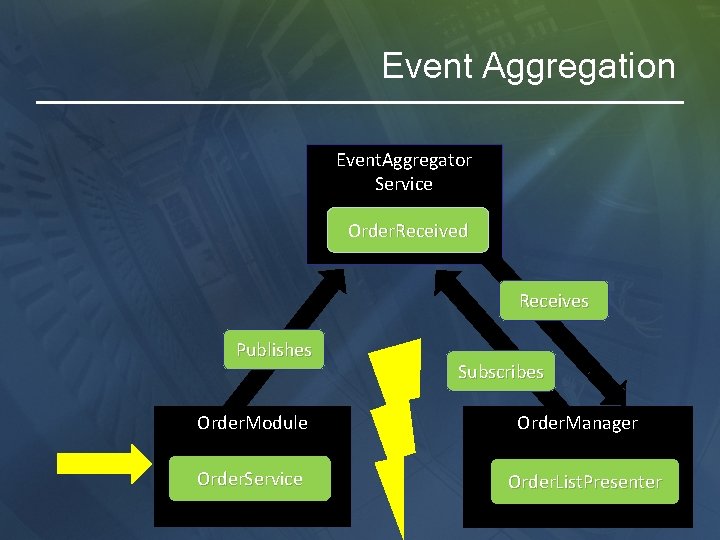
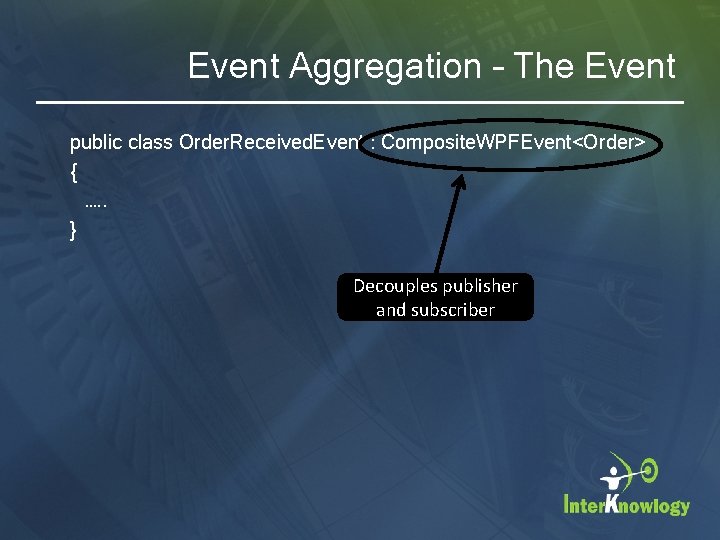
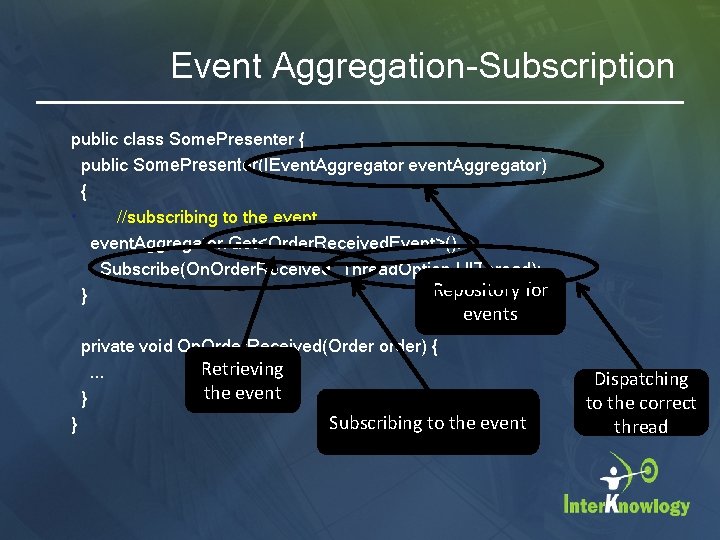
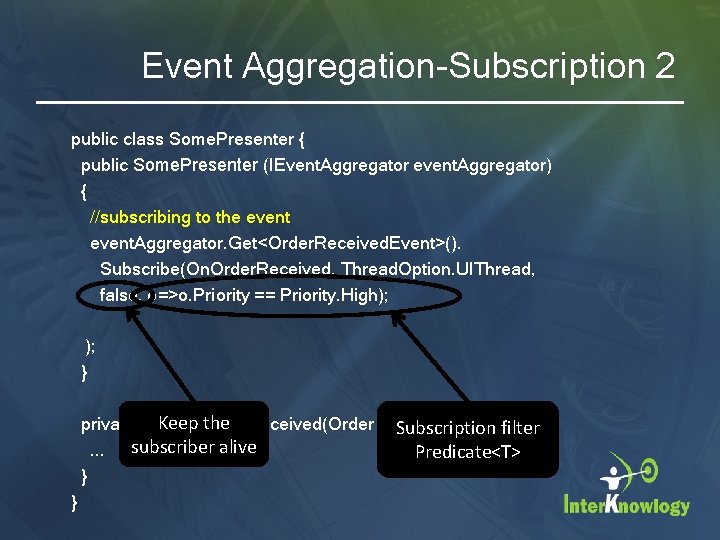
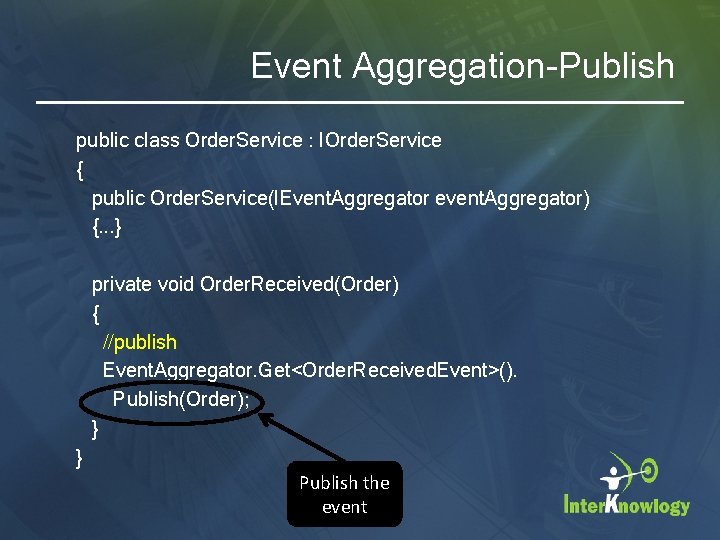
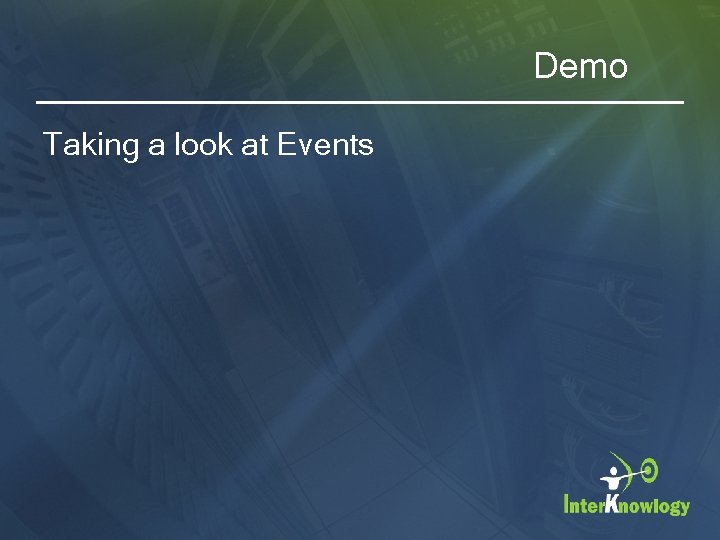
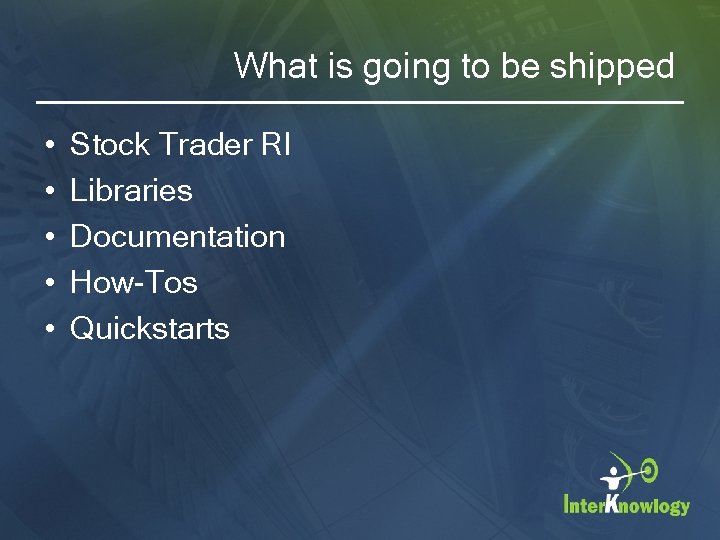
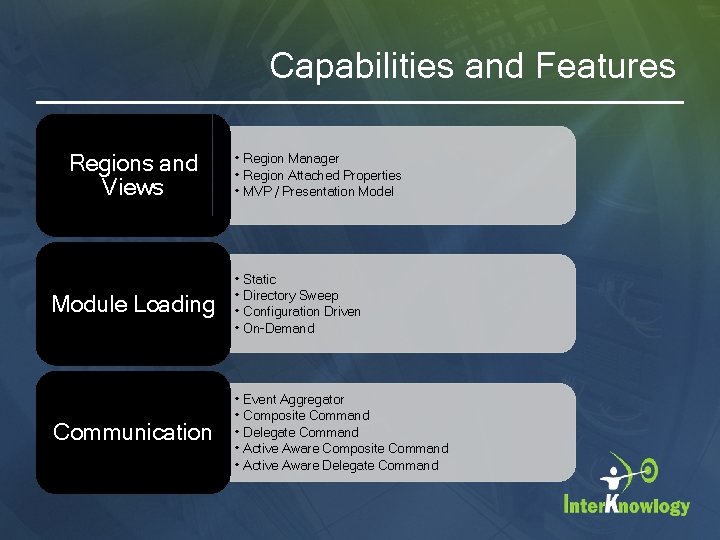
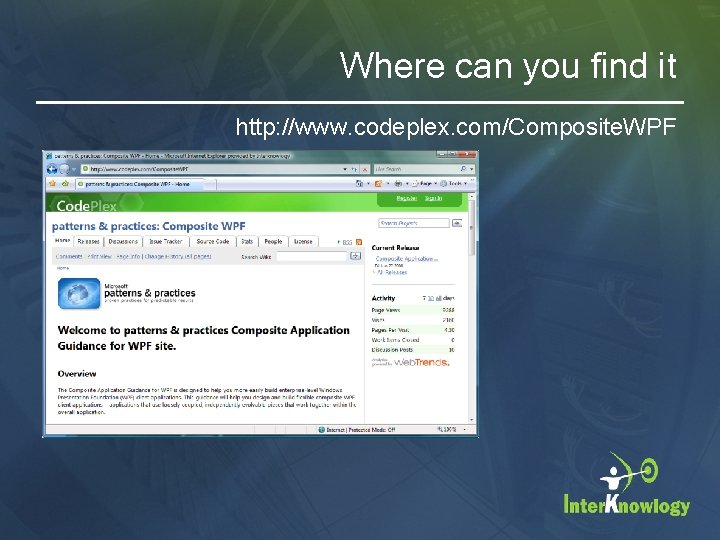
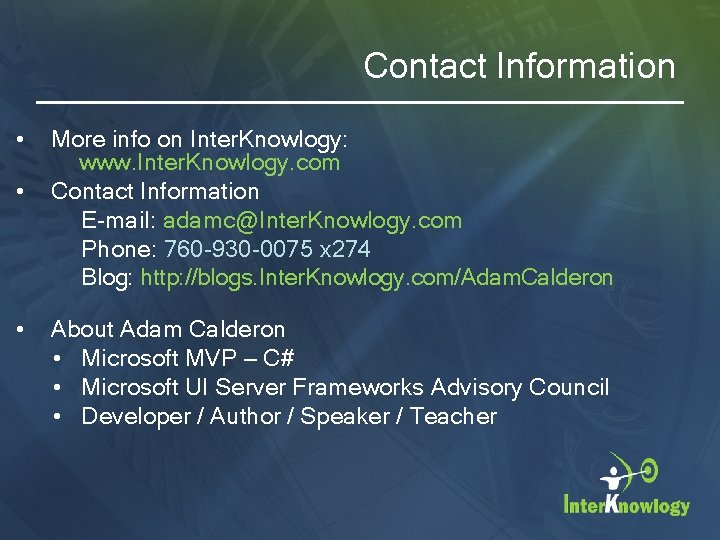
- Slides: 34
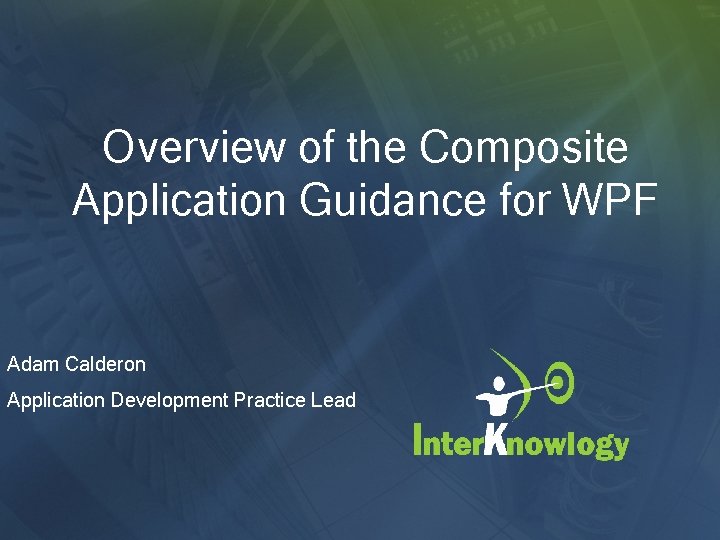
Overview of the Composite Application Guidance for WPF Adam Calderon Application Development Practice Lead
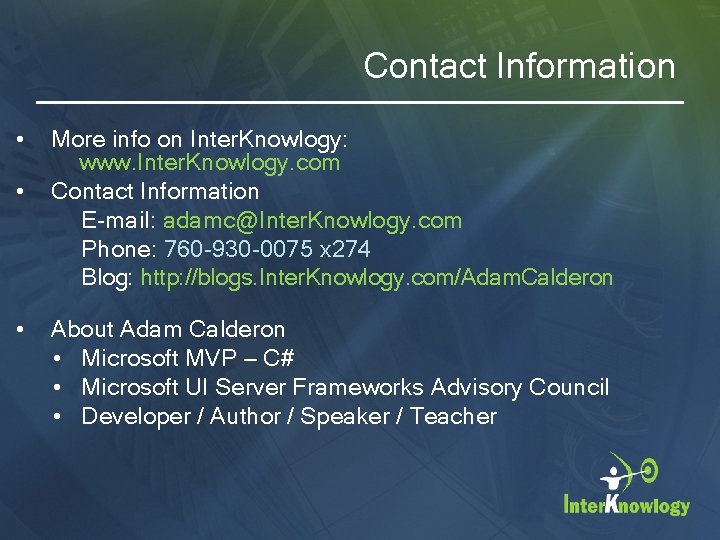
Contact Information • • • More info on Inter. Knowlogy: www. Inter. Knowlogy. com Contact Information E-mail: adamc@Inter. Knowlogy. com Phone: 760 -930 -0075 x 274 Blog: http: //blogs. Inter. Knowlogy. com/Adam. Calderon About Adam Calderon • Microsoft MVP – C# • Microsoft UI Server Frameworks Advisory Council • Developer / Author / Speaker / Teacher
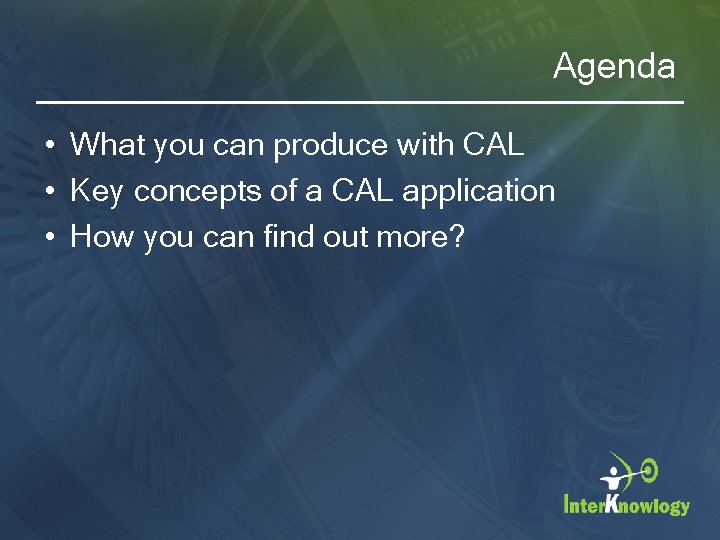
Agenda • What you can produce with CAL • Key concepts of a CAL application • How you can find out more?
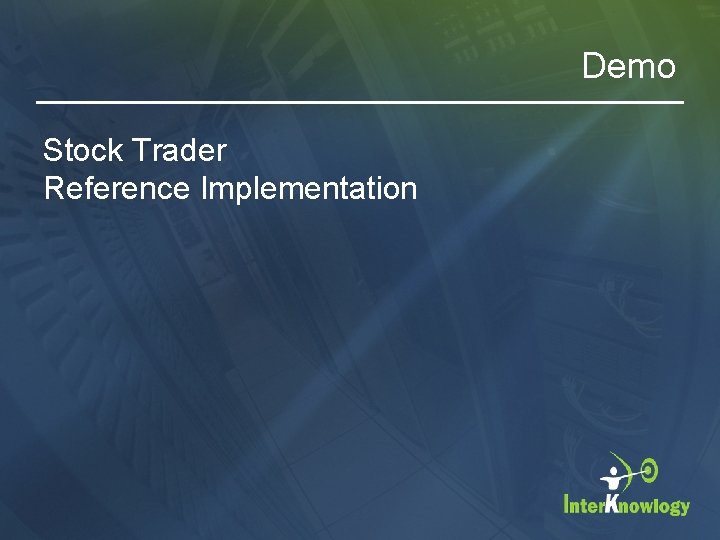
Demo Stock Trader Reference Implementation
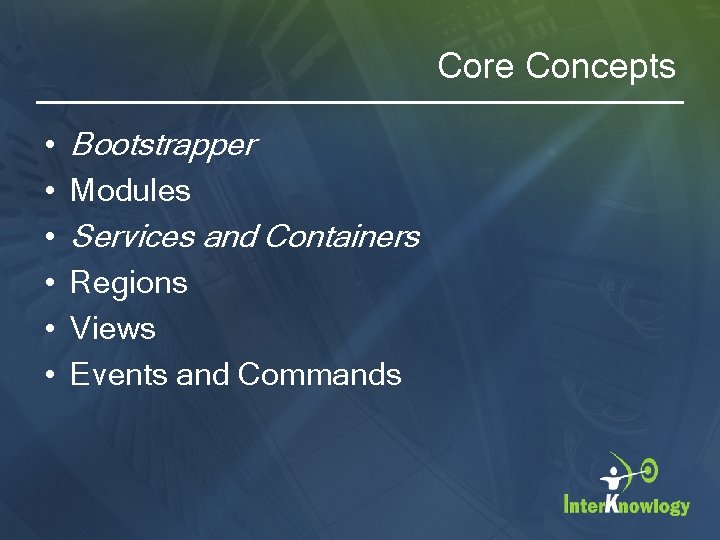
Core Concepts • • • Bootstrapper Modules Services and Containers Regions Views Events and Commands
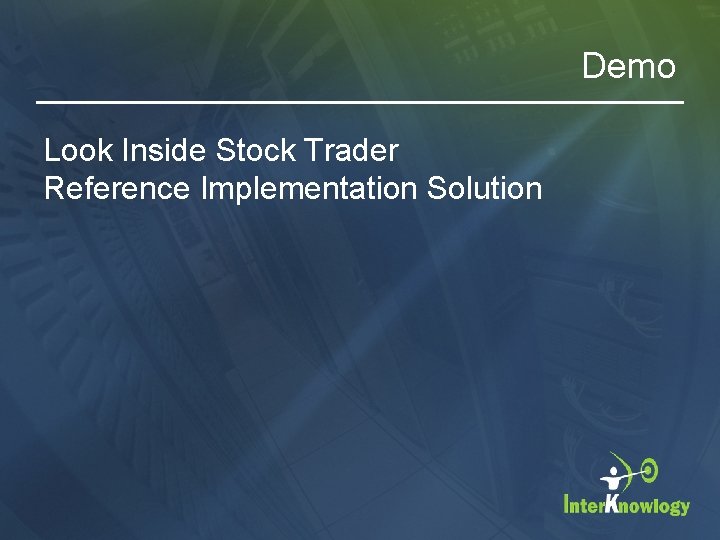
Demo Look Inside Stock Trader Reference Implementation Solution
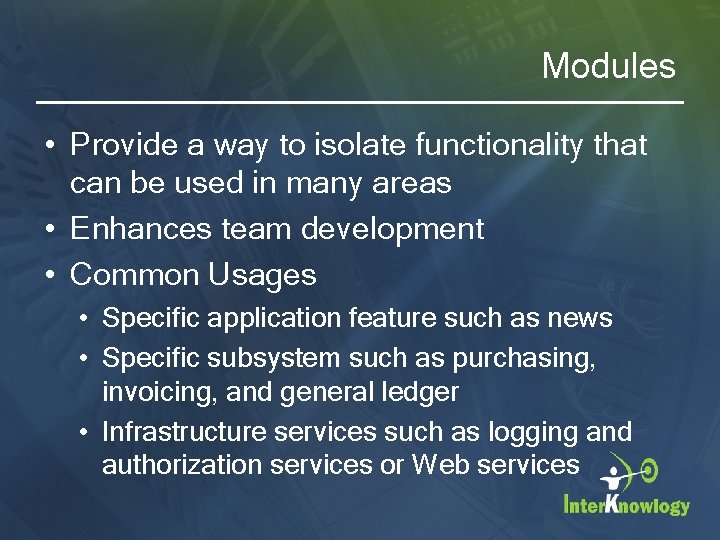
Modules • Provide a way to isolate functionality that can be used in many areas • Enhances team development • Common Usages • Specific application feature such as news • Specific subsystem such as purchasing, invoicing, and general ledger • Infrastructure services such as logging and authorization services or Web services
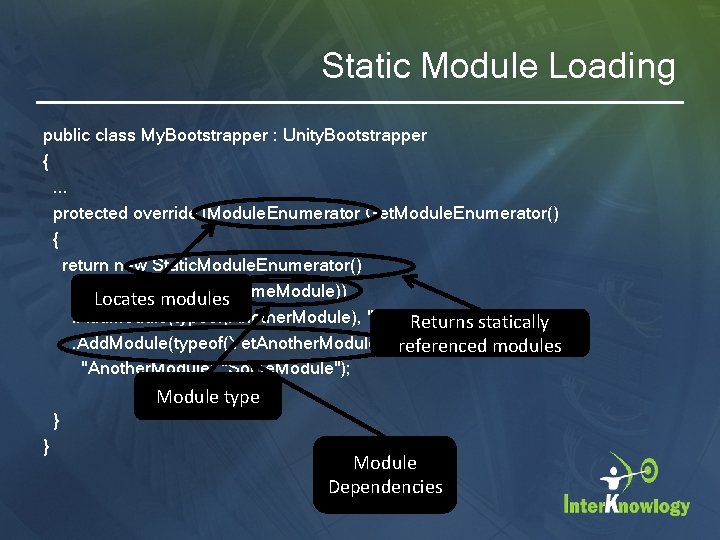
Static Module Loading public class My. Bootstrapper : Unity. Bootstrapper {. . . protected override IModule. Enumerator Get. Module. Enumerator() { return new Static. Module. Enumerator(). Add. Module(typeof(Some. Module)) Locates modules. Add. Module(typeof(Another. Module), "Some. Module") Returns statically. Add. Module(typeof(Yet. Another. Module), referenced modules "Another. Module", "Some. Module"); Module type } } Module Dependencies
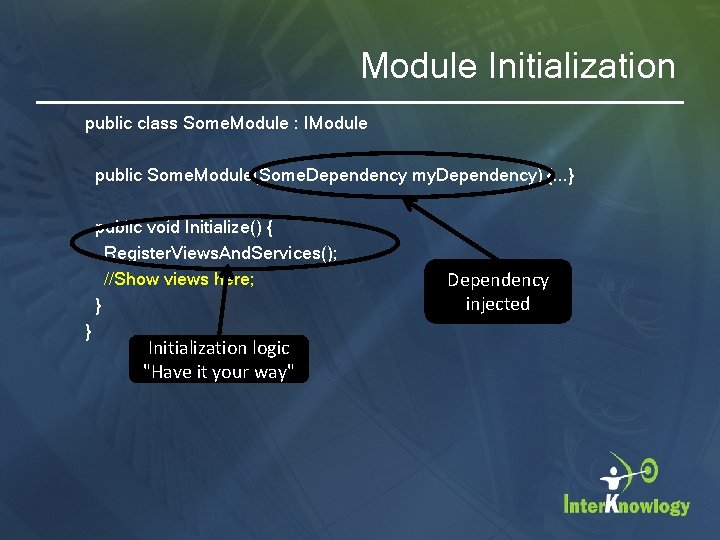
Module Initialization public class Some. Module : IModule public Some. Module(Some. Dependency my. Dependency) {. . . } public void Initialize() { Register. Views. And. Services(); //Show views here; } } Initialization logic "Have it your way" Dependency injected
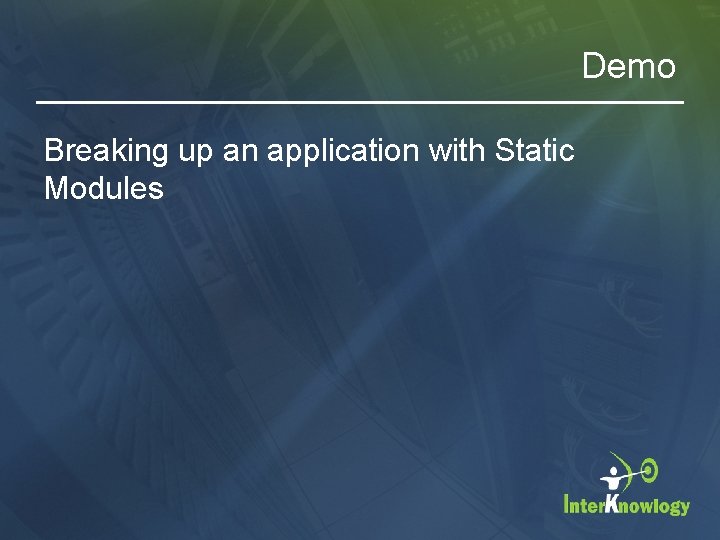
Demo Breaking up an application with Static Modules
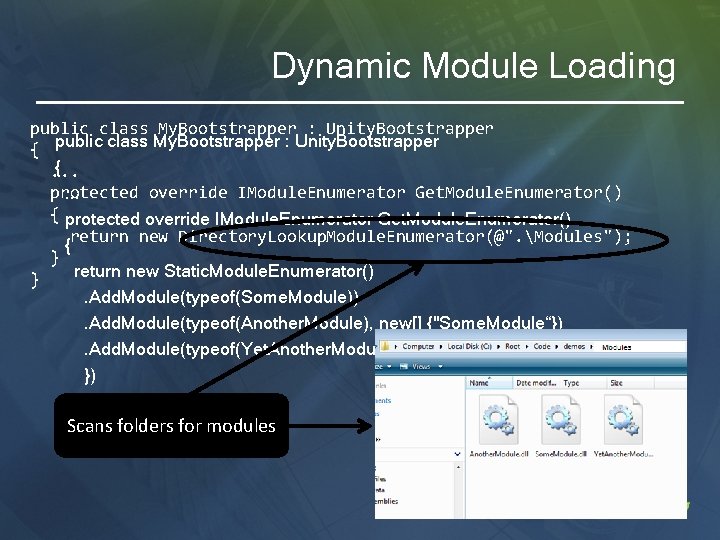
Dynamic Module Loading public class My. Bootstrapper : Unity. Bootstrapper {. . . protected override IModule. Enumerator Get. Module. Enumerator(). . . { protected override IModule. Enumerator Get. Module. Enumerator() return new Directory. Lookup. Module. Enumerator(@". Modules"); { } return new Static. Module. Enumerator() }. Add. Module(typeof(Some. Module)). Add. Module(typeof(Another. Module), new[] {"Some. Module“}). Add. Module(typeof(Yet. Another. Module), new[] { "Another. Module" }) } } Scans folders for modules
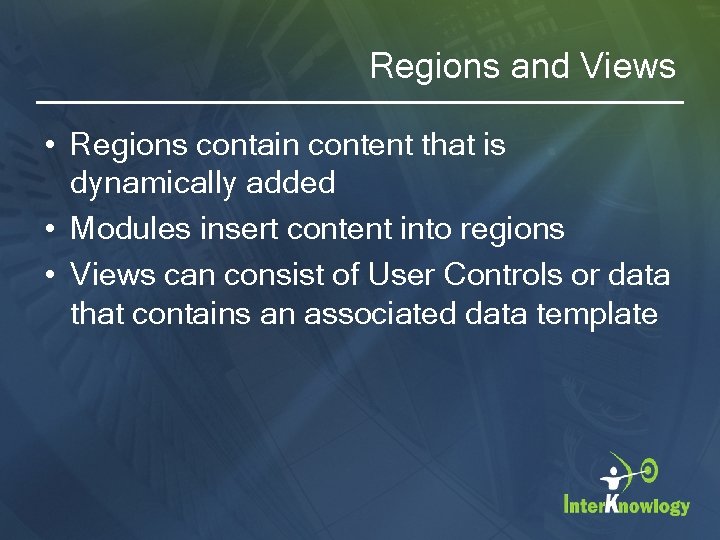
Regions and Views • Regions contain content that is dynamically added • Modules insert content into regions • Views can consist of User Controls or data that contains an associated data template
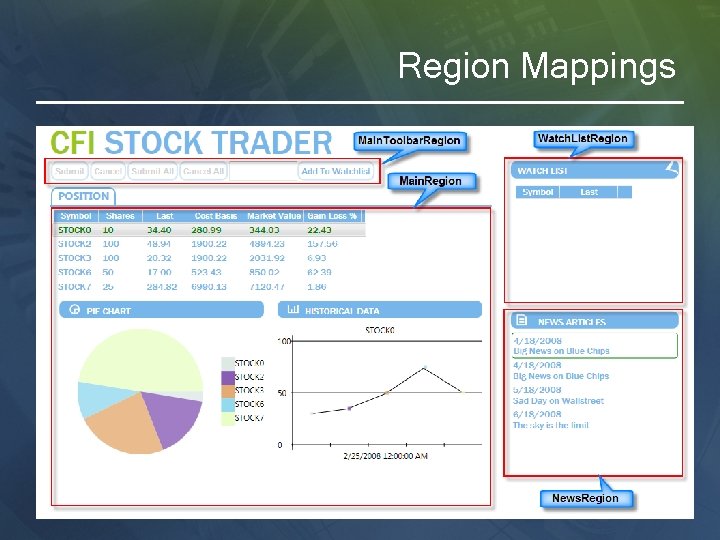
Region Mappings
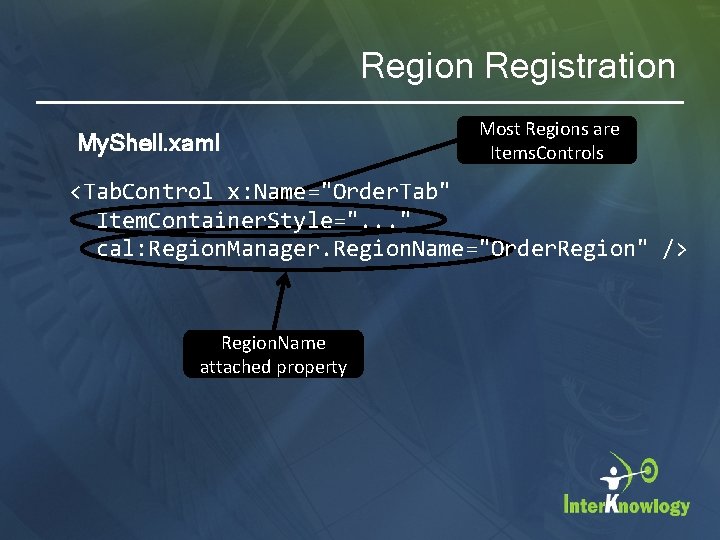
Region Registration My. Shell. xaml Most Regions are Items. Controls <Tab. Control x: Name="Order. Tab" Item. Container. Style=". . . " cal: Region. Manager. Region. Name="Order. Region" /> Region. Name attached property
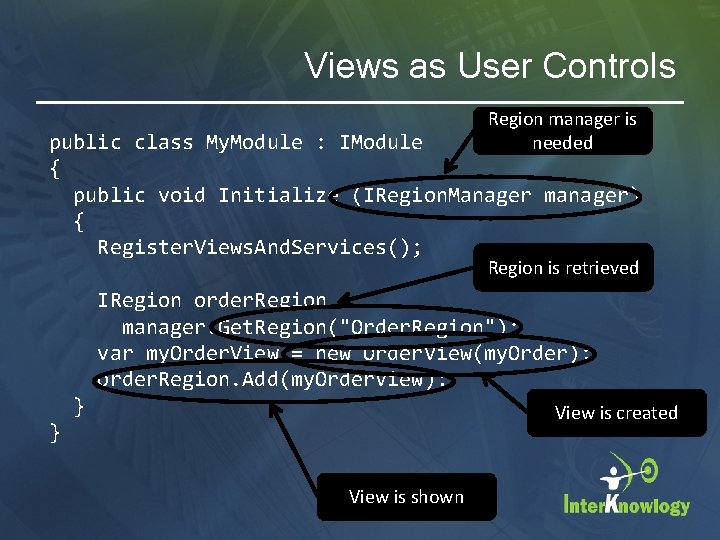
Views as User Controls Region manager is needed public class My. Module : IModule { public void Initialize (IRegion. Manager manager) { Register. Views. And. Services(); Region is retrieved IRegion order. Region = manager. Get. Region("Order. Region"); var my. Order. View = new Order. View(my. Order); order. Region. Add(my. Order. View); } View is created } View is shown
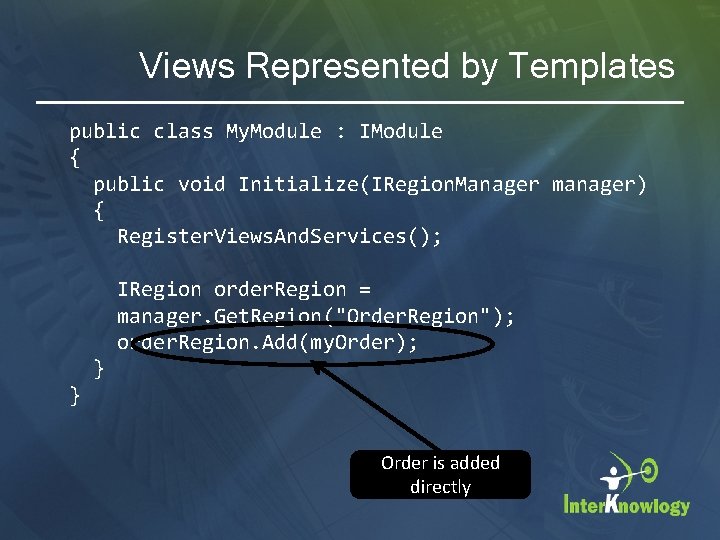
Views Represented by Templates public class My. Module : IModule { public void Initialize(IRegion. Manager manager) { Register. Views. And. Services(); IRegion order. Region = manager. Get. Region("Order. Region"); order. Region. Add(my. Order); } } Order is added directly
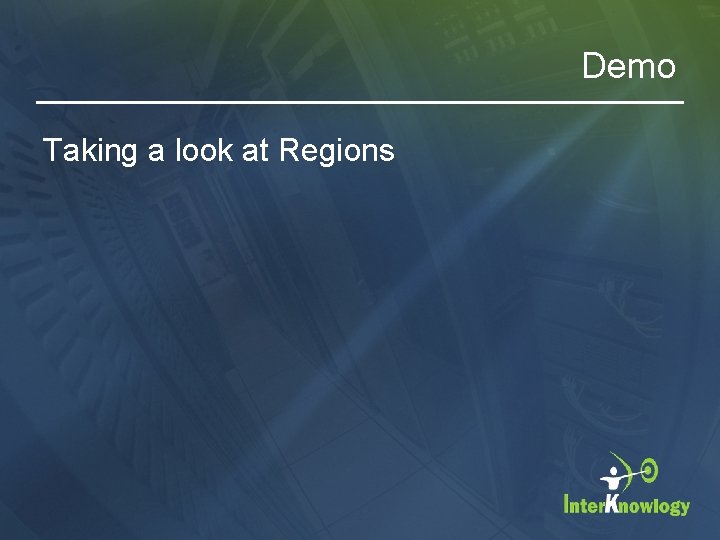
Demo Taking a look at Regions
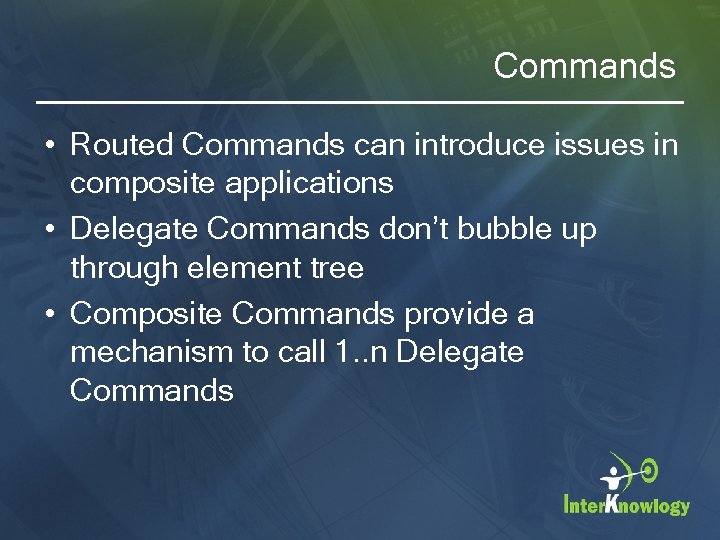
Commands • Routed Commands can introduce issues in composite applications • Delegate Commands don’t bubble up through element tree • Composite Commands provide a mechanism to call 1. . n Delegate Commands
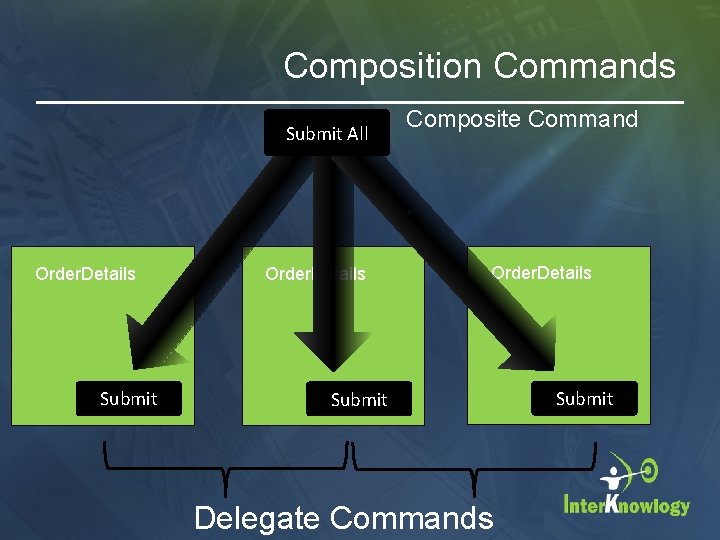
Composition Commands Submit All Order. Details Submit Order. Details Composite Command Order. Details Submit Delegate Commands Submit
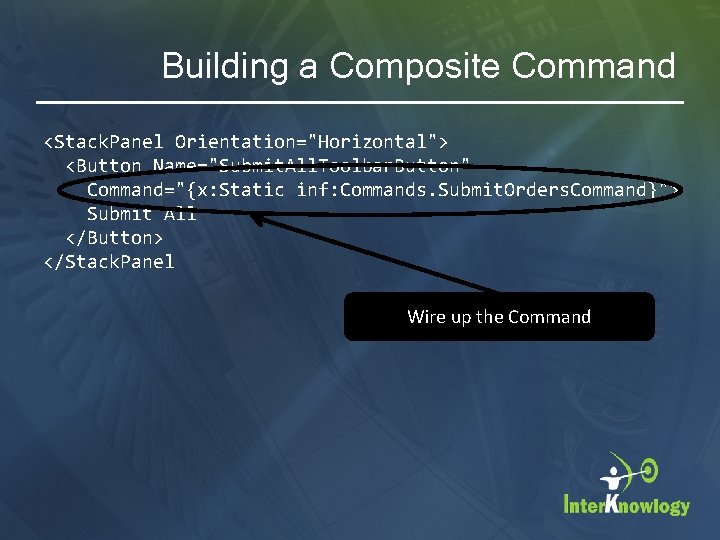
Building a Composite Command <Stack. Panel Orientation="Horizontal"> <Button Name="Submit. All. Toolbar. Button" Command="{x: Static inf: Commands. Submit. Orders. Command}"> Submit All </Button> </Stack. Panel Wire up the Command
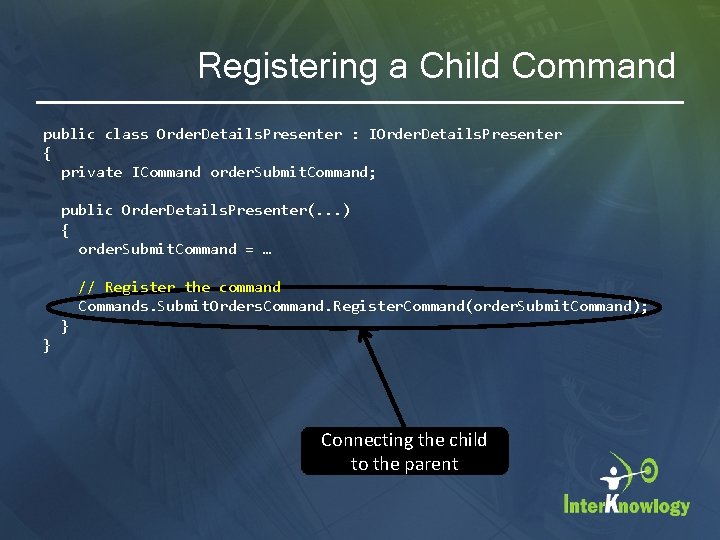
Registering a Child Command public class Order. Details. Presenter : IOrder. Details. Presenter { private ICommand order. Submit. Command; public Order. Details. Presenter(. . . ) { order. Submit. Command = … // Register the command Commands. Submit. Orders. Command. Register. Command(order. Submit. Command); } } Connecting the child to the parent
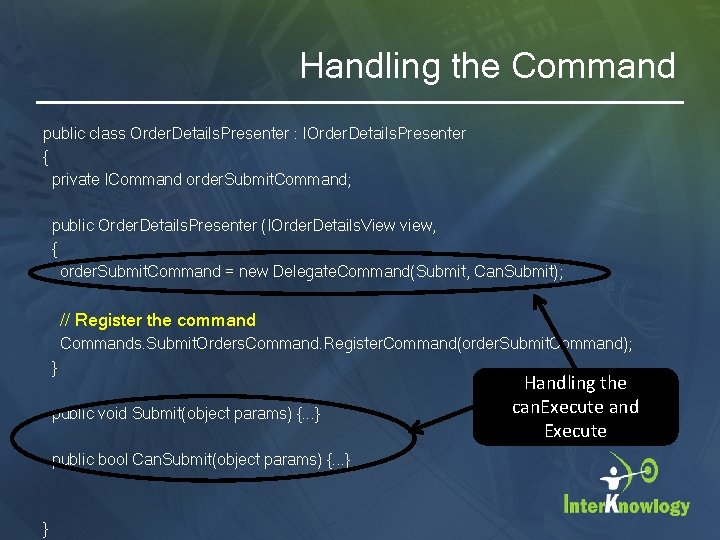
Handling the Command public class Order. Details. Presenter : IOrder. Details. Presenter { private ICommand order. Submit. Command; public Order. Details. Presenter (IOrder. Details. View view, { order. Submit. Command = new Delegate. Command(Submit, Can. Submit); // Register the command Commands. Submit. Orders. Command. Register. Command(order. Submit. Command); } public void Submit(object params) {. . . } public bool Can. Submit(object params) {. . . } } Handling the can. Execute and Execute
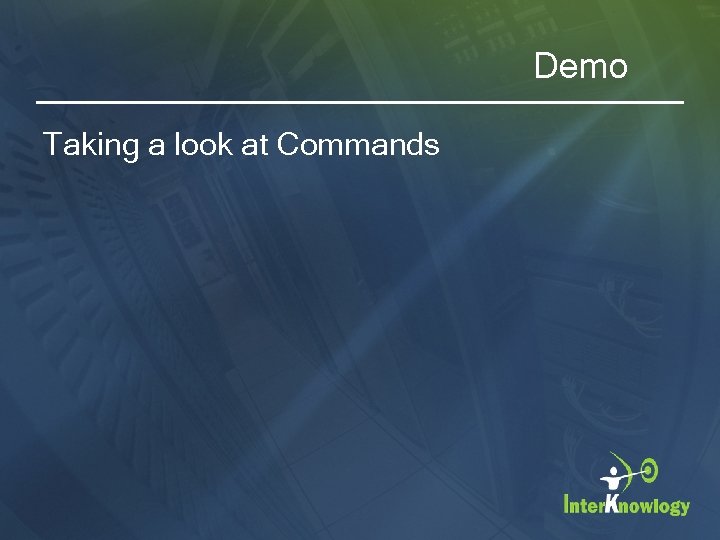
Demo Taking a look at Commands
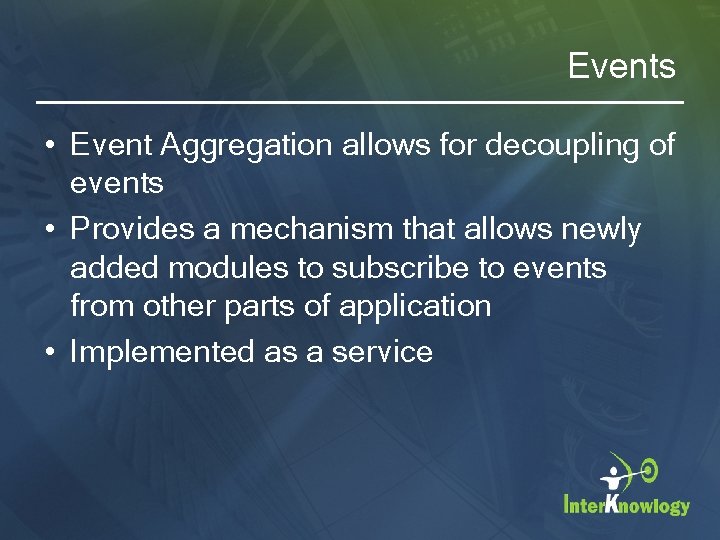
Events • Event Aggregation allows for decoupling of events • Provides a mechanism that allows newly added modules to subscribe to events from other parts of application • Implemented as a service
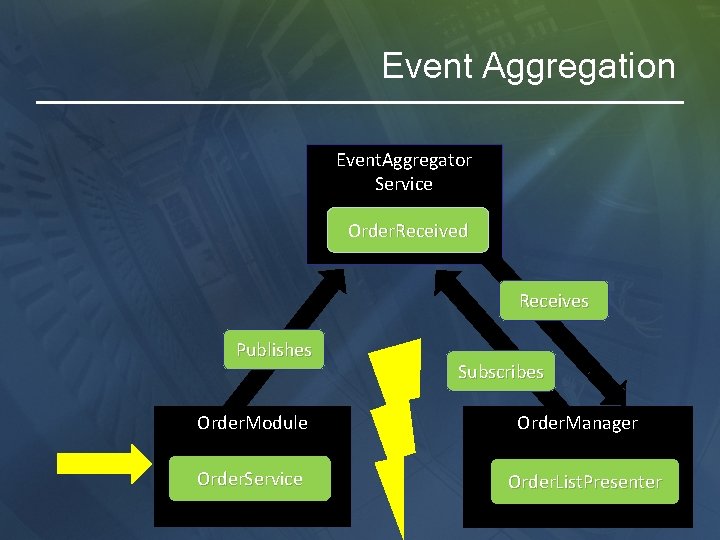
Event Aggregation Event. Aggregator Service Order. Received Receives Publishes Order. Module Order. Service Subscribes Order. Manager Order. List. Presenter
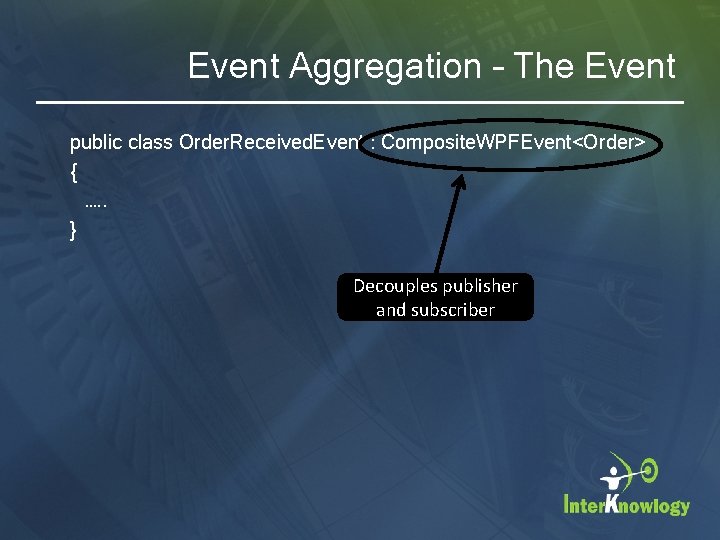
Event Aggregation – The Event public class Order. Received. Event : Composite. WPFEvent<Order> { …. . } Decouples publisher and subscriber
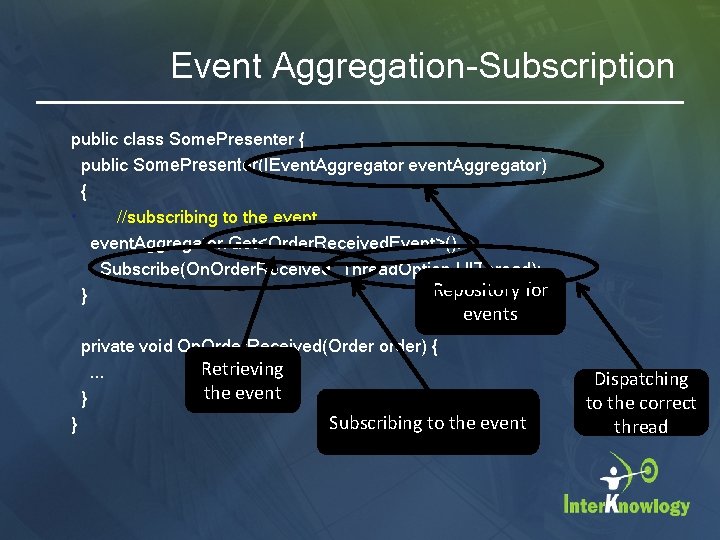
Event Aggregation-Subscription public class Some. Presenter { public Some. Presenter(IEvent. Aggregator event. Aggregator) { • //subscribing to the event. Aggregator. Get<Order. Received. Event>(). Subscribe(On. Order. Received, Thread. Option. UIThread); Repository for } events private void On. Order. Received(Order order) { Retrieving. . . the event } } Subscribing to the event Dispatching to the correct thread
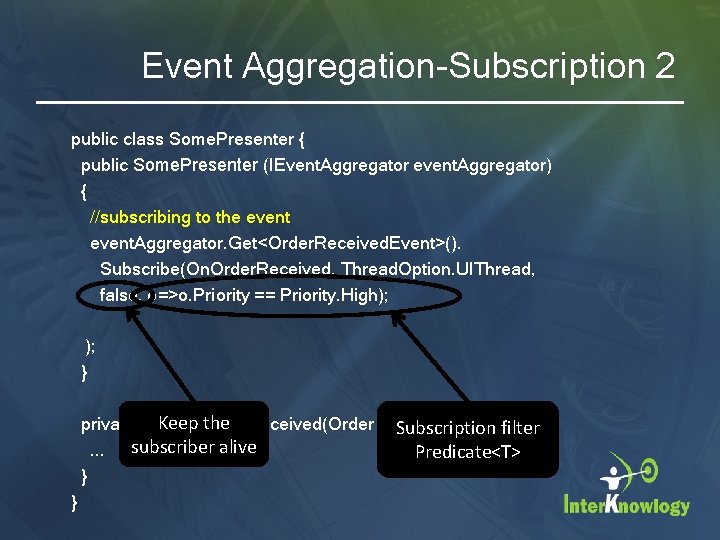
Event Aggregation-Subscription 2 public class Some. Presenter { public Some. Presenter (IEvent. Aggregator event. Aggregator) { //subscribing to the event. Aggregator. Get<Order. Received. Event>(). Subscribe(On. Order. Received, Thread. Option. UIThread, false, o=>o. Priority == Priority. High); ); } Keep the private void On. Order. Received(Order order) { Subscription filter. . . subscriber alive Predicate<T> } }
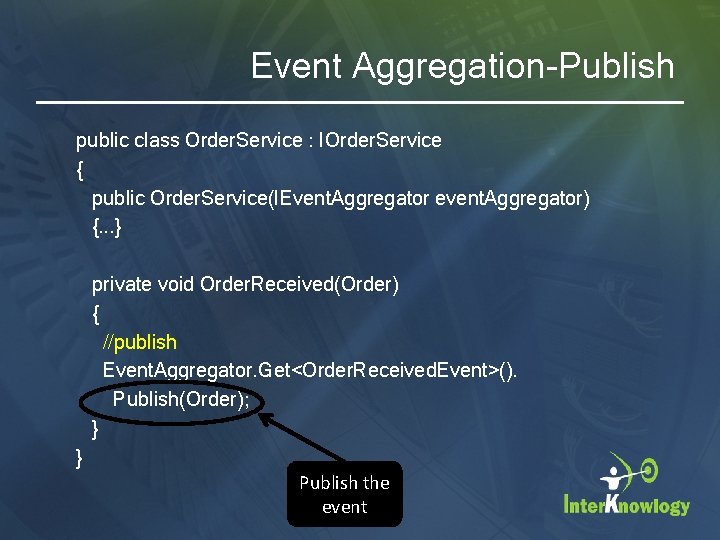
Event Aggregation-Publish public class Order. Service : IOrder. Service { public Order. Service(IEvent. Aggregator event. Aggregator) {. . . } private void Order. Received(Order) { //publish Event. Aggregator. Get<Order. Received. Event>(). Publish(Order); } } Publish the event
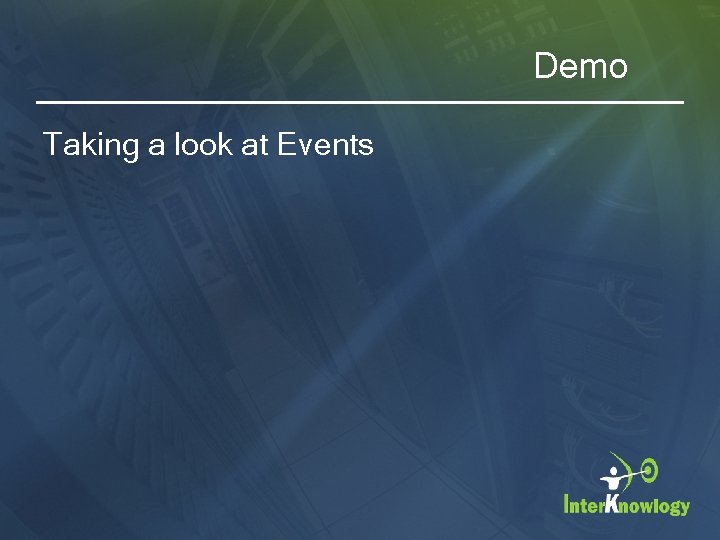
Demo Taking a look at Events
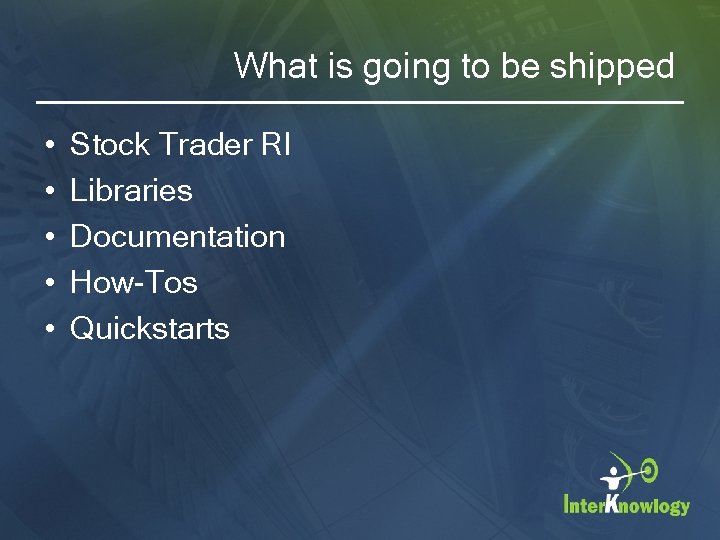
What is going to be shipped • • • Stock Trader RI Libraries Documentation How-Tos Quickstarts
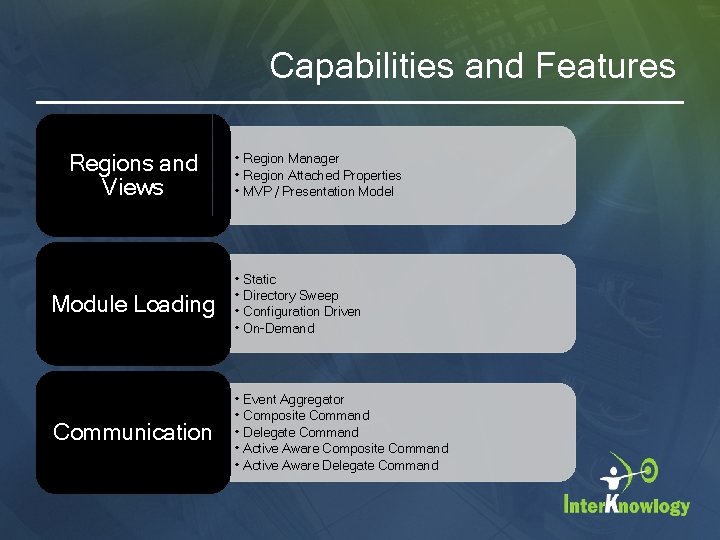
Capabilities and Features Regions and Views • Region Manager • Region Attached Properties • MVP / Presentation Model Module Loading • • Static Directory Sweep Configuration Driven On-Demand Communication • • • Event Aggregator Composite Command Delegate Command Active Aware Composite Command Active Aware Delegate Command
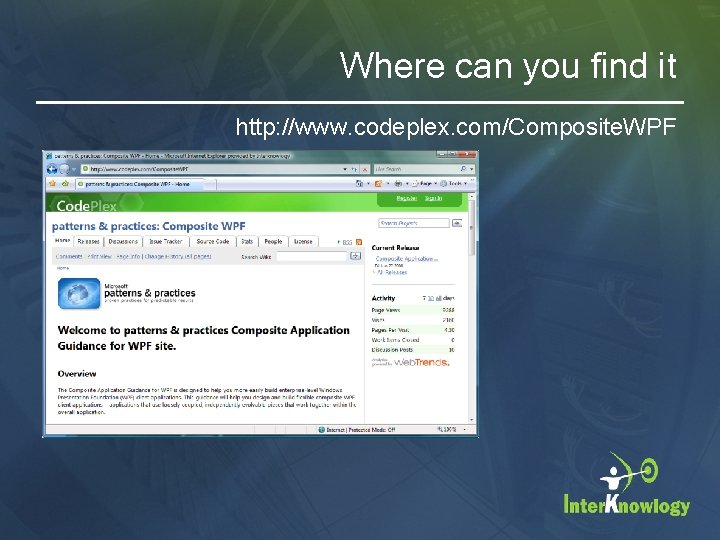
Where can you find it http: //www. codeplex. com/Composite. WPF
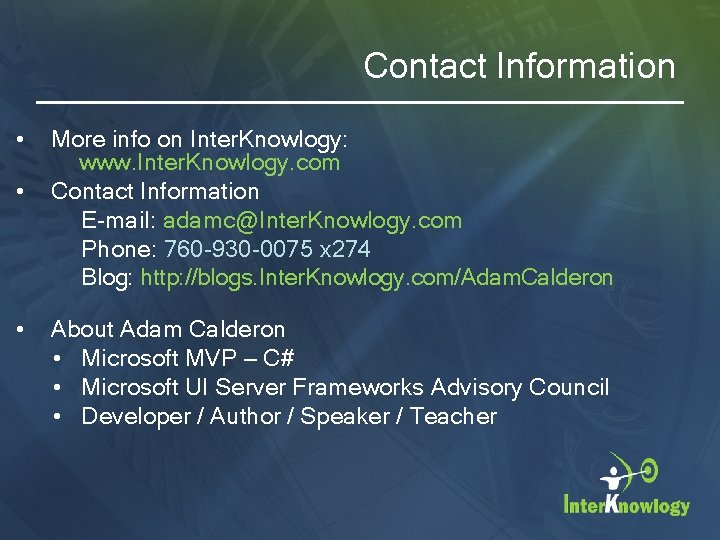
Contact Information • • • More info on Inter. Knowlogy: www. Inter. Knowlogy. com Contact Information E-mail: adamc@Inter. Knowlogy. com Phone: 760 -930 -0075 x 274 Blog: http: //blogs. Inter. Knowlogy. com/Adam. Calderon About Adam Calderon • Microsoft MVP – C# • Microsoft UI Server Frameworks Advisory Council • Developer / Author / Speaker / Teacher
Developing guidance skills
Hanau quint
Moment of inertia composite shapes
Owasp asvs
Composite application framework
Ibm tivoli composite application manager
Ibm tivoli monitoring
Tivoli composite application manager for applications
Wpf dersleri
Wpf windows form 混在
Wpf step by step
Mfc vs wpf
Wpf vs uwp
Windows platform foundation
Wpf dependency property
Wpf asystent
Window resources wpf
Wpf navigate to another page
Prism design pattern
Wpf d3dimage
Wpf touchdown
Wpf linear gradient brush
Wpf uniformgrid columnspan
Validationrule wpf
Wpf adaptive layout
Wpf design patterns
Wpf tutorial point
8wpf
Object routed
Wpf
Wpf asystent
Uwp prism
Wpf tips and tricks
Romarriket tidslinje
Boverket ka