MVC for Servlets Model View Controller Architecture MVC
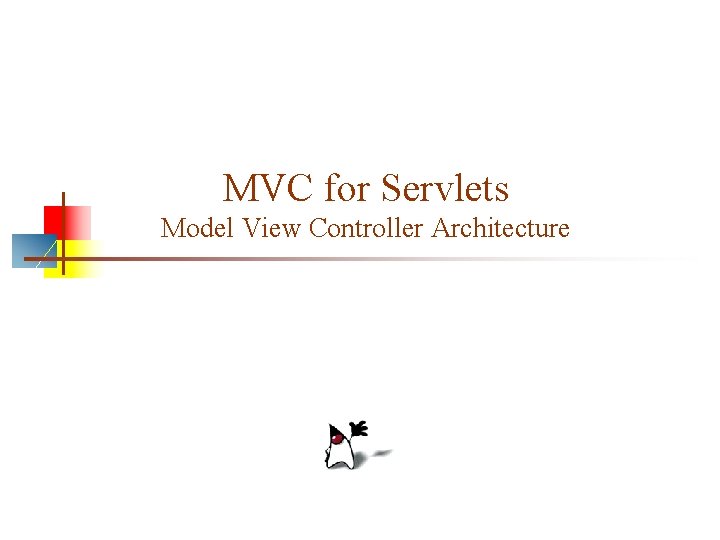
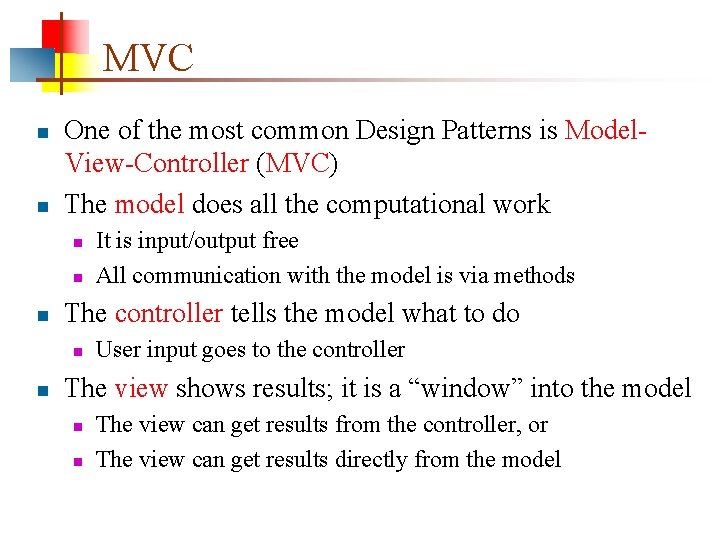
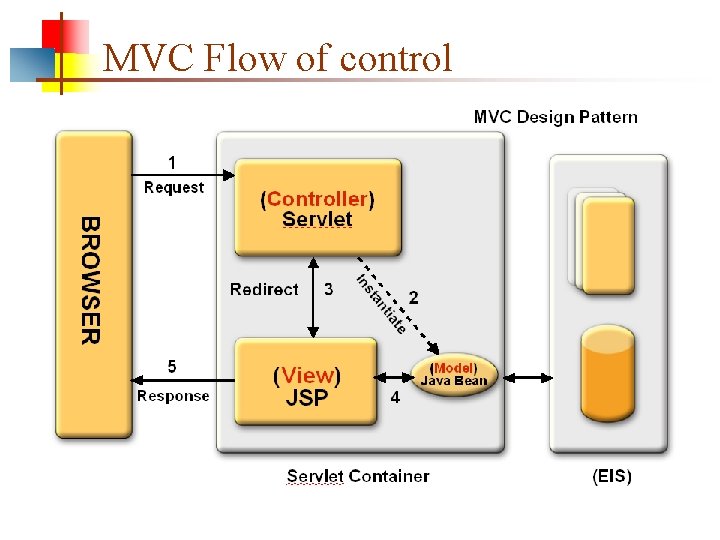
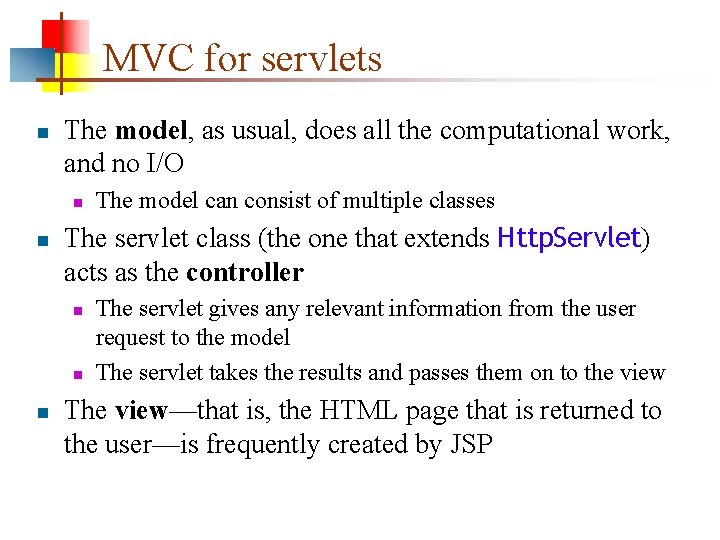
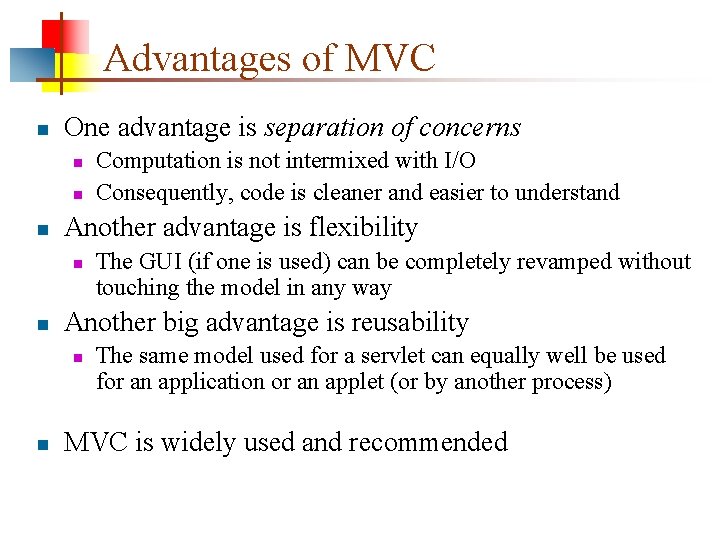
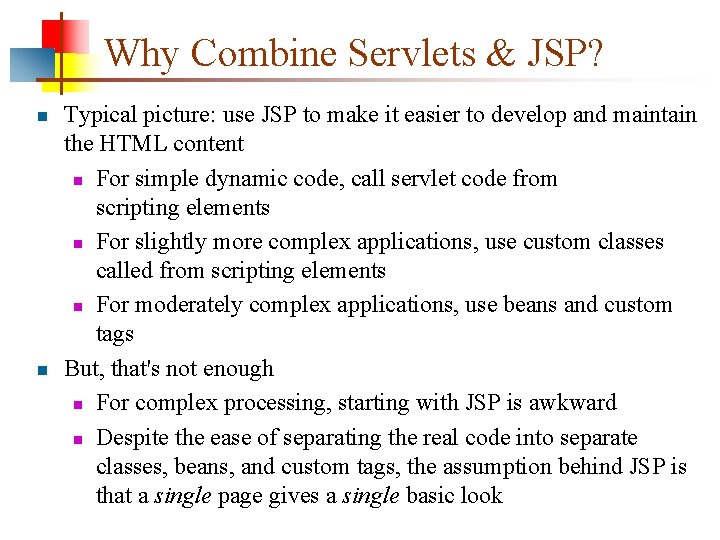
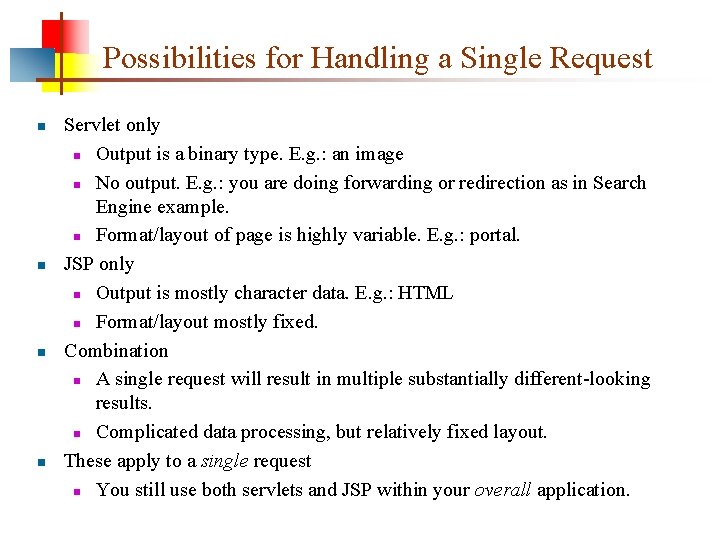
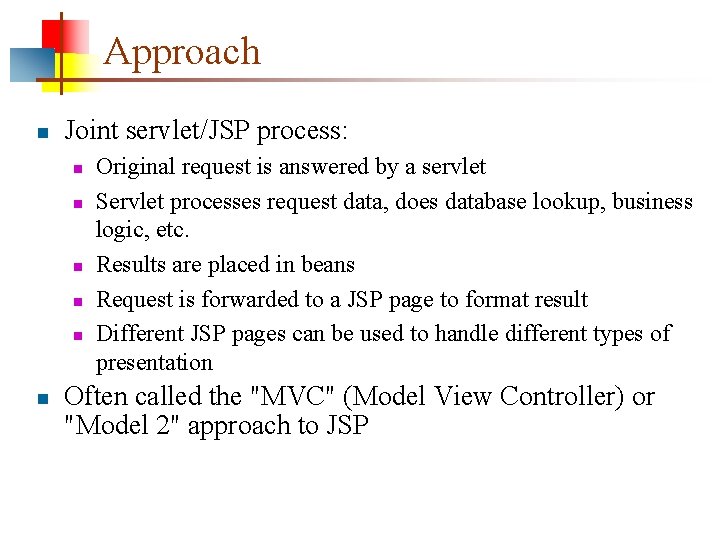
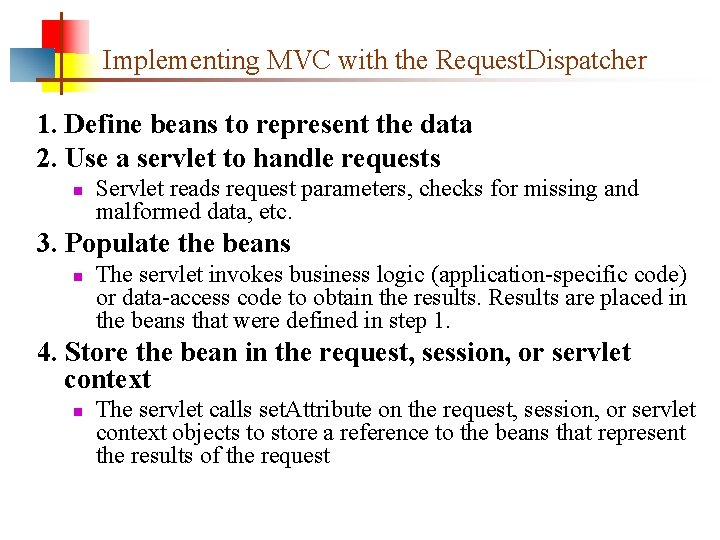
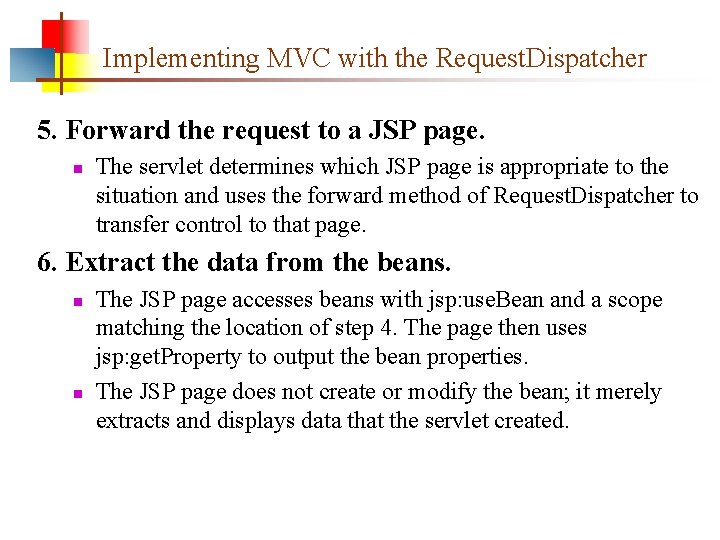
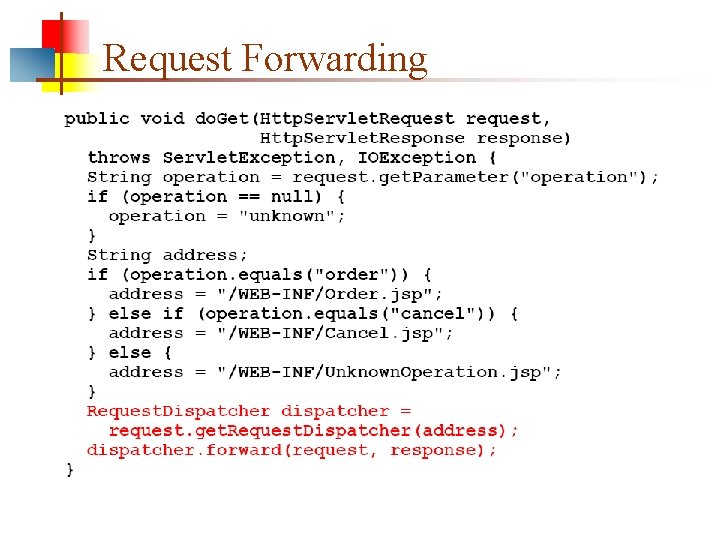
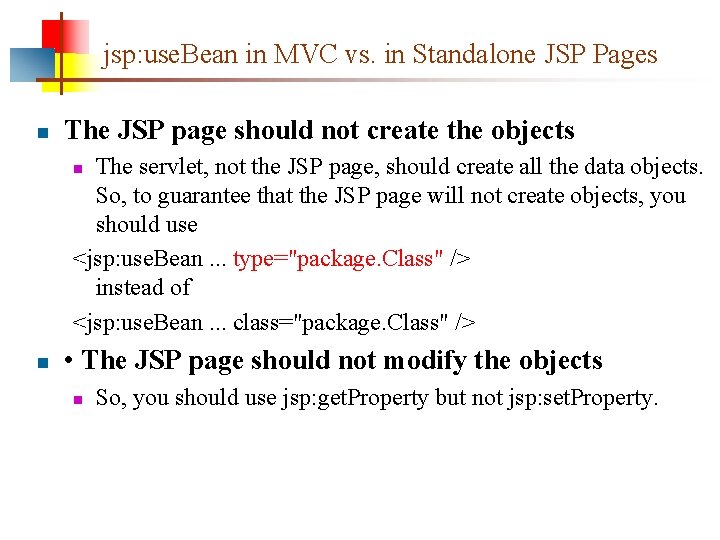
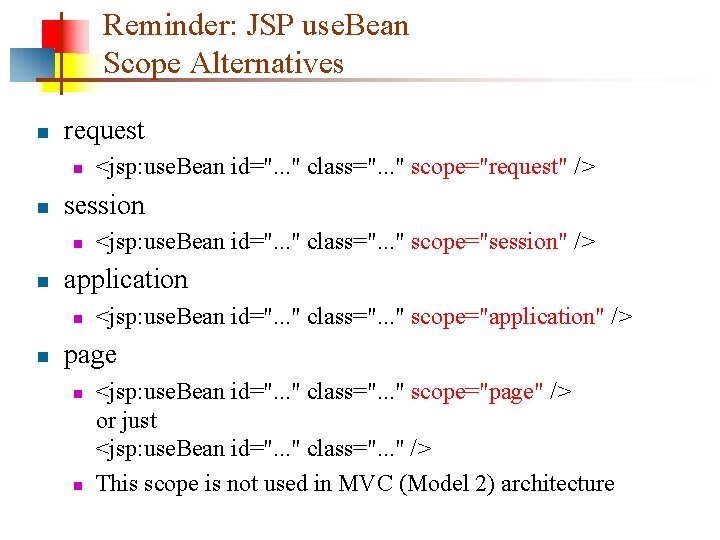
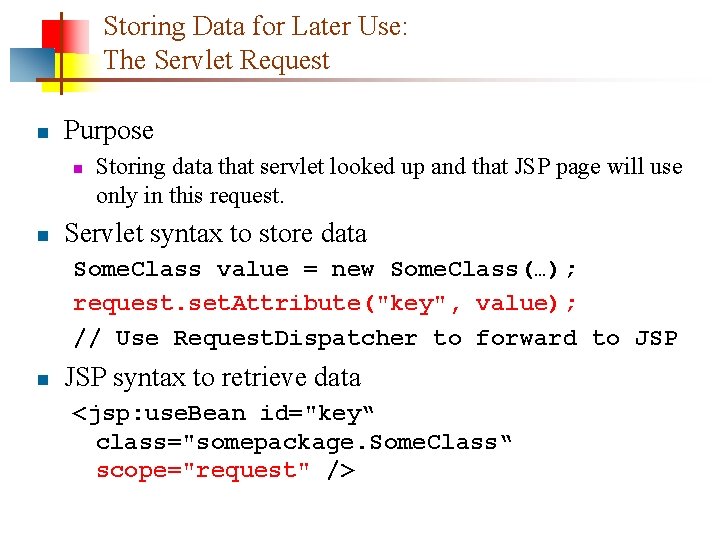
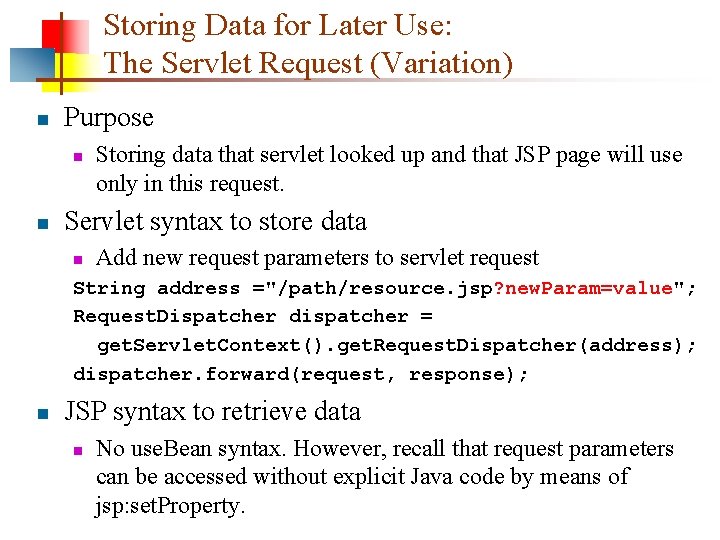
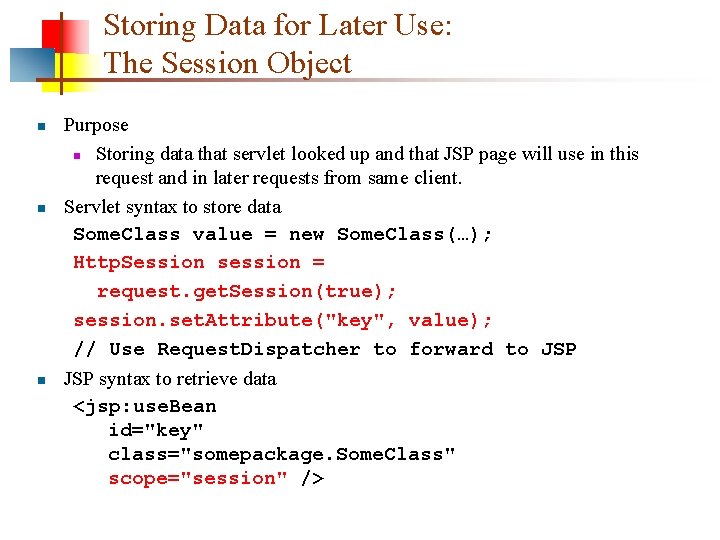
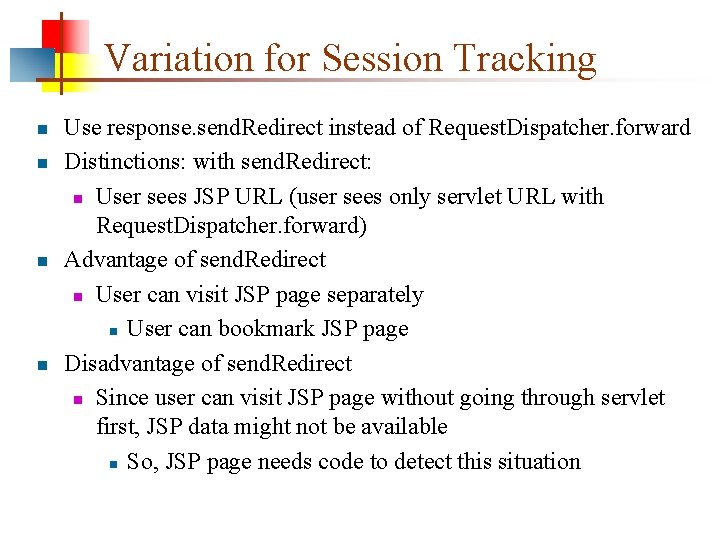
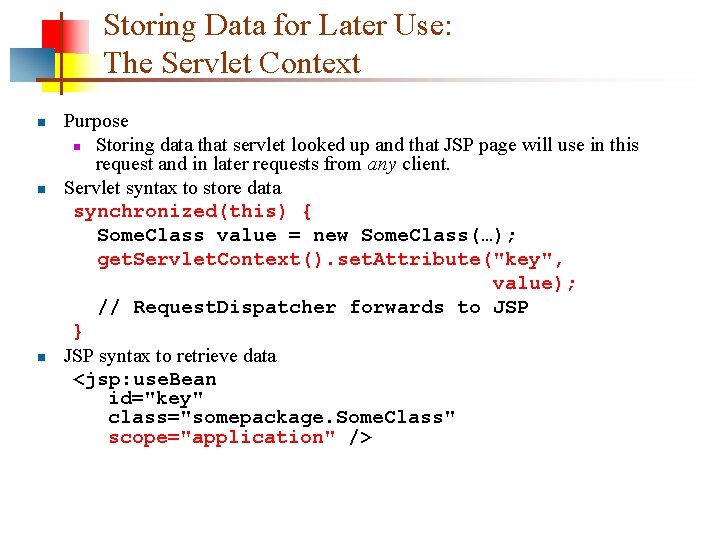
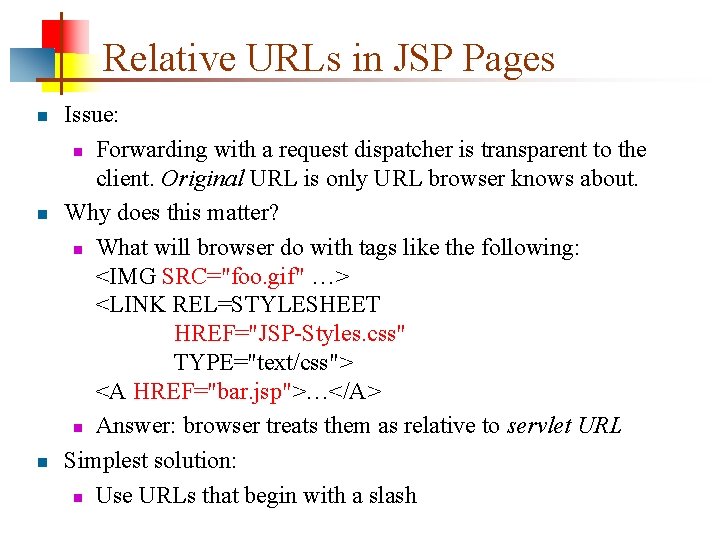
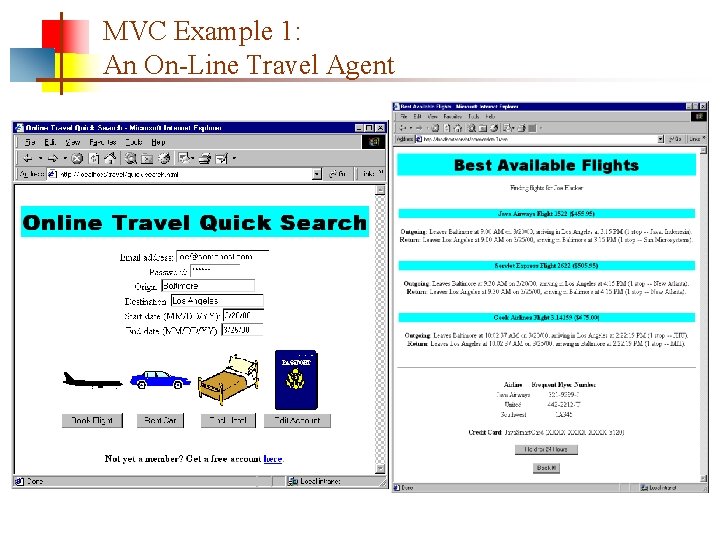
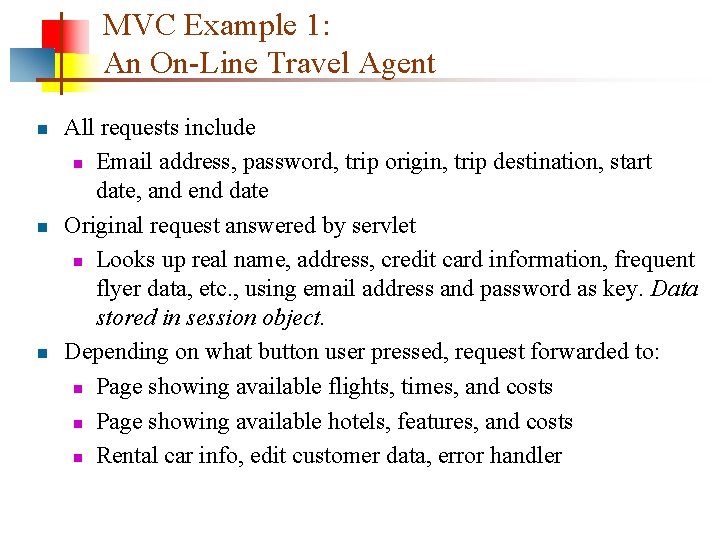
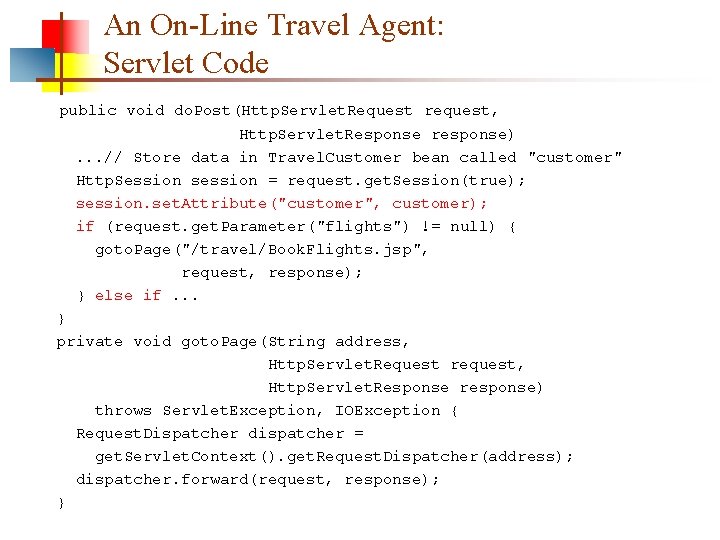
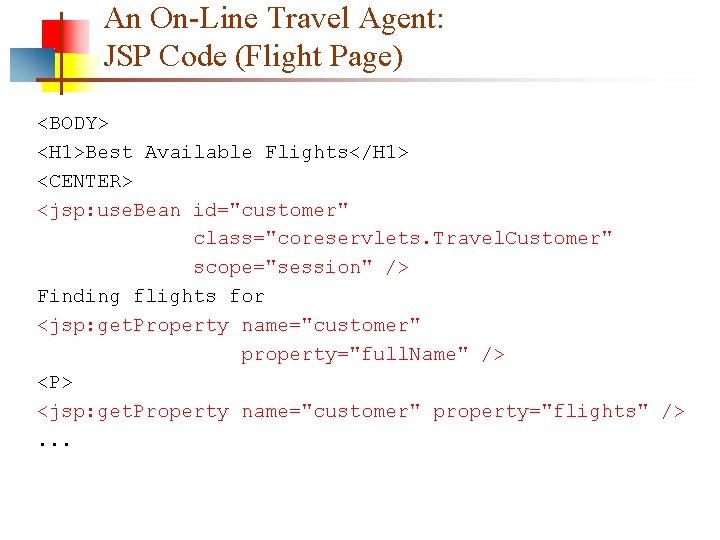
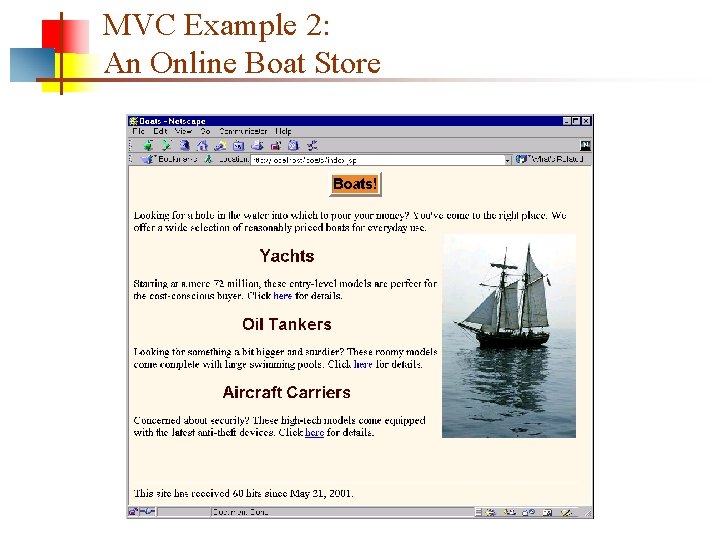
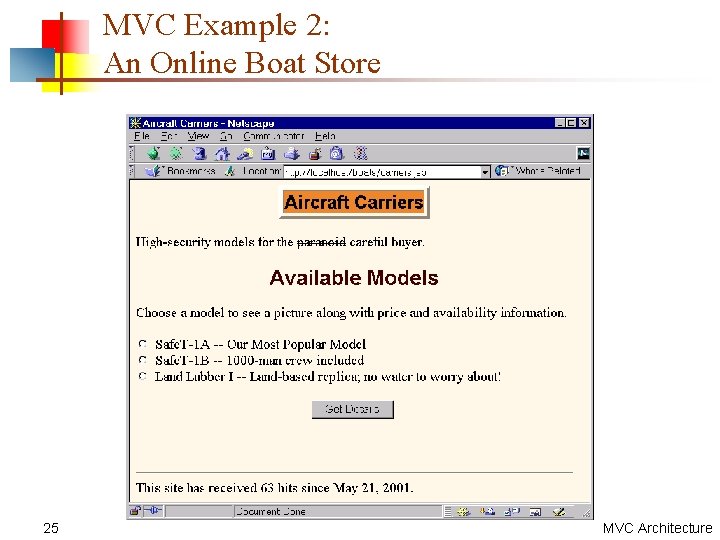
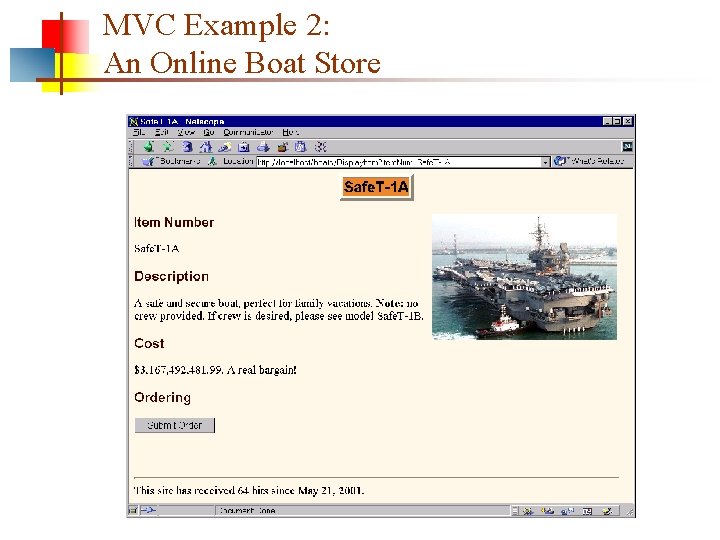
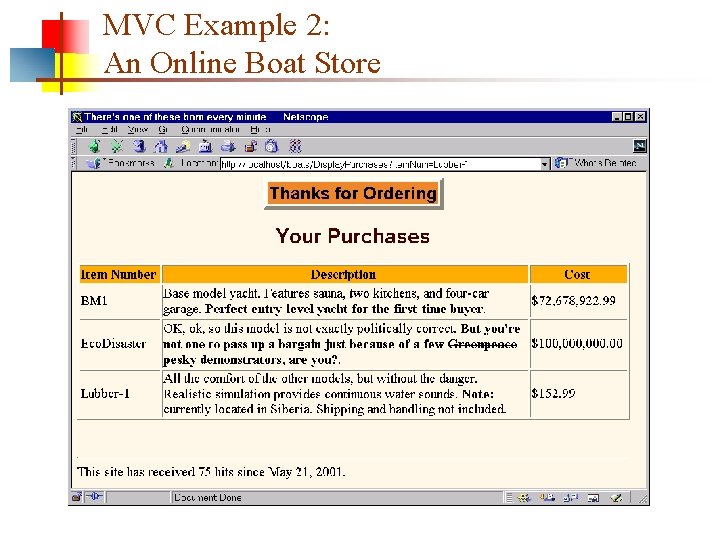
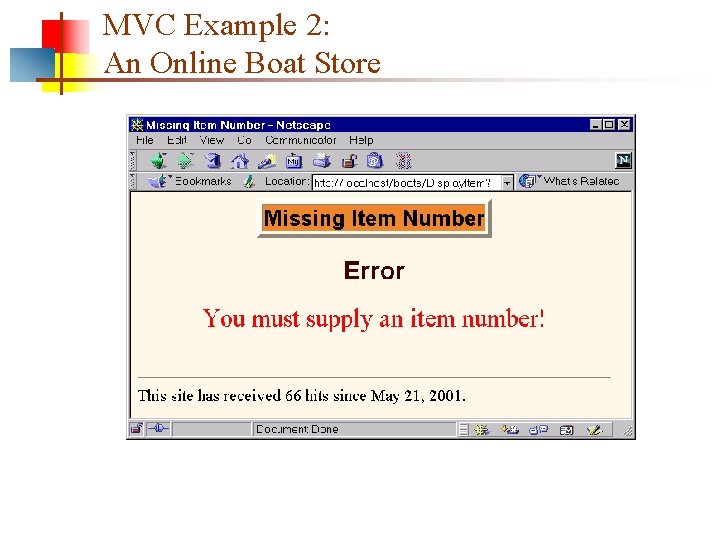
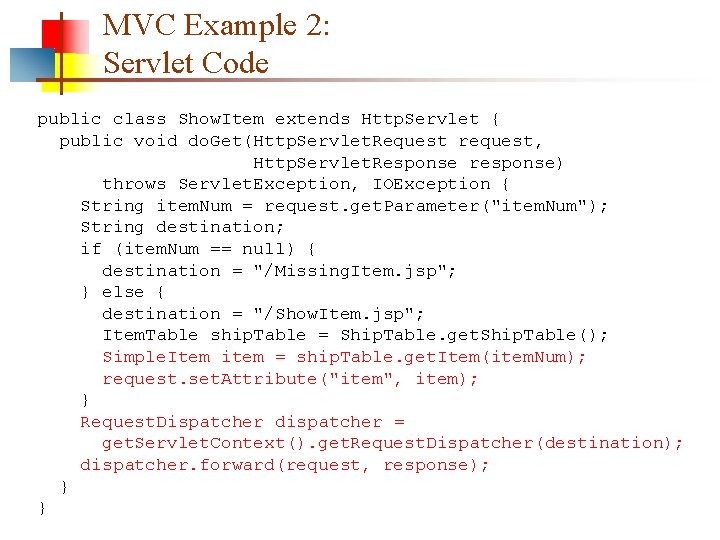
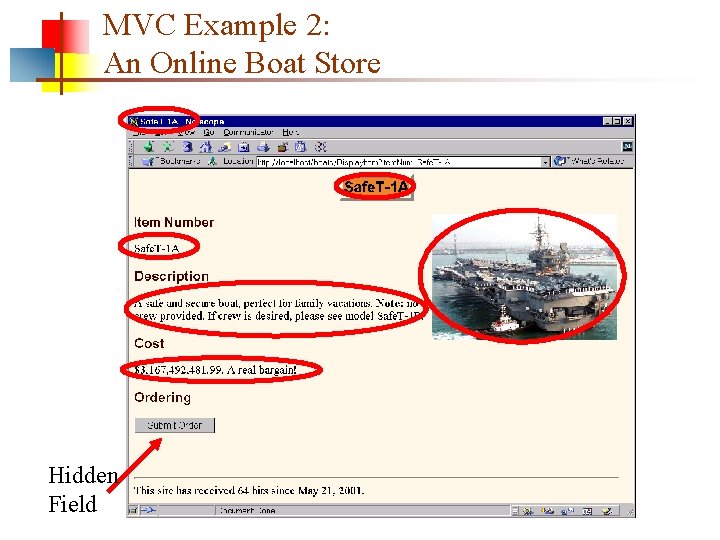
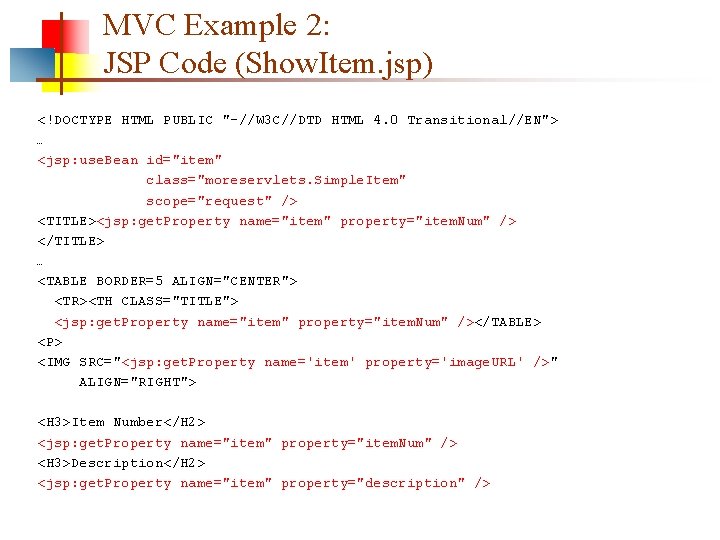
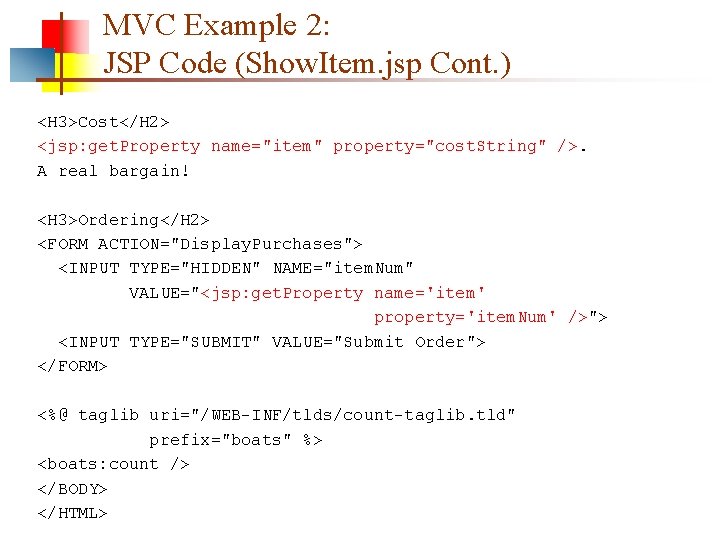
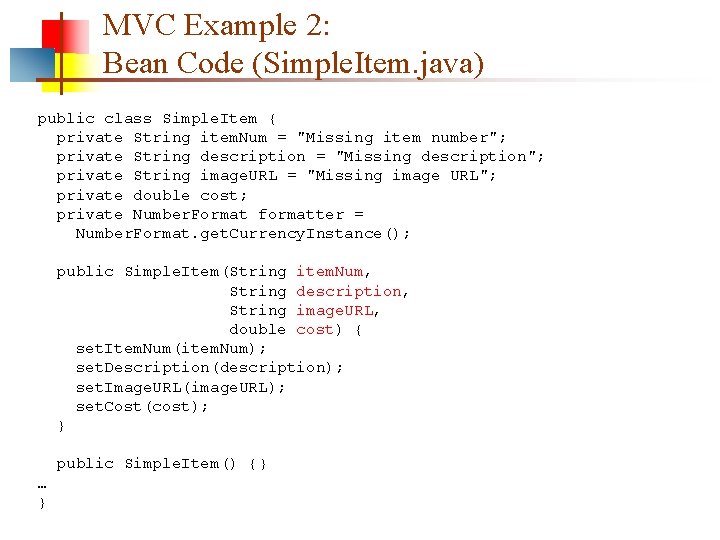
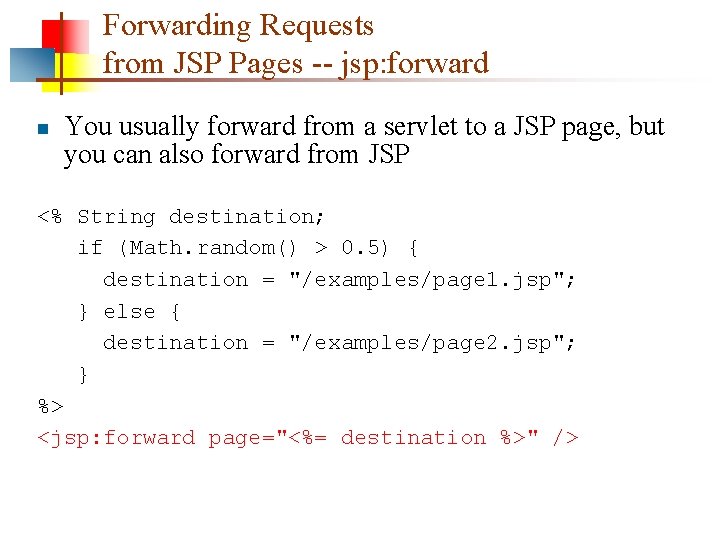
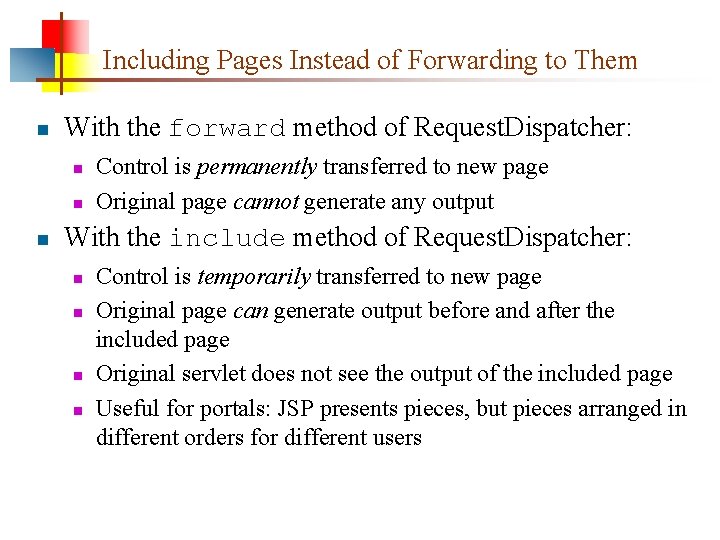
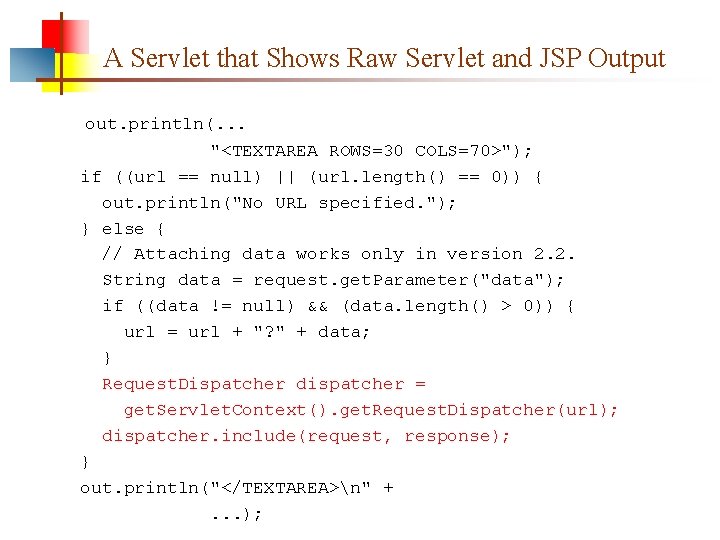
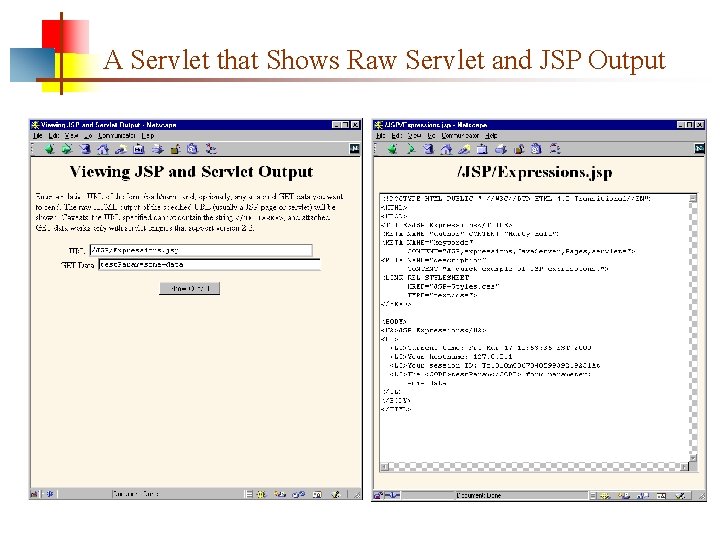
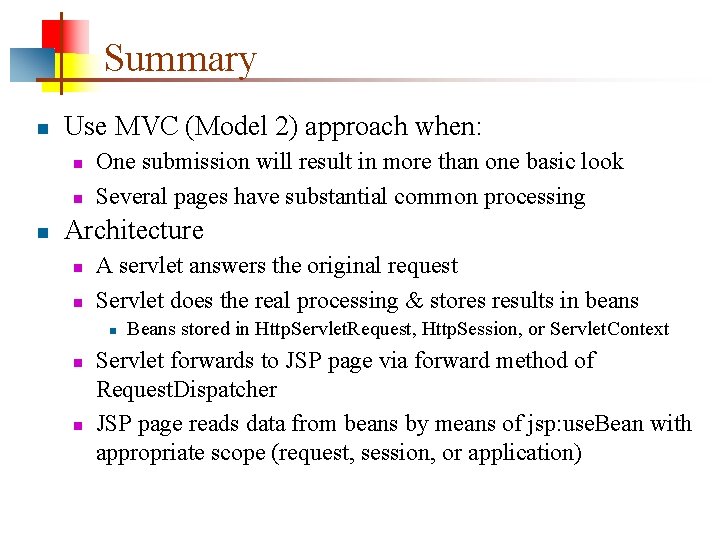
- Slides: 38
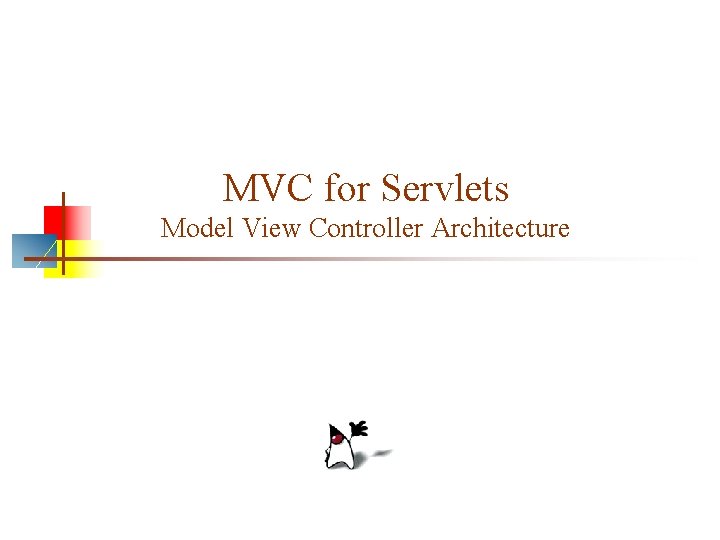
MVC for Servlets Model View Controller Architecture
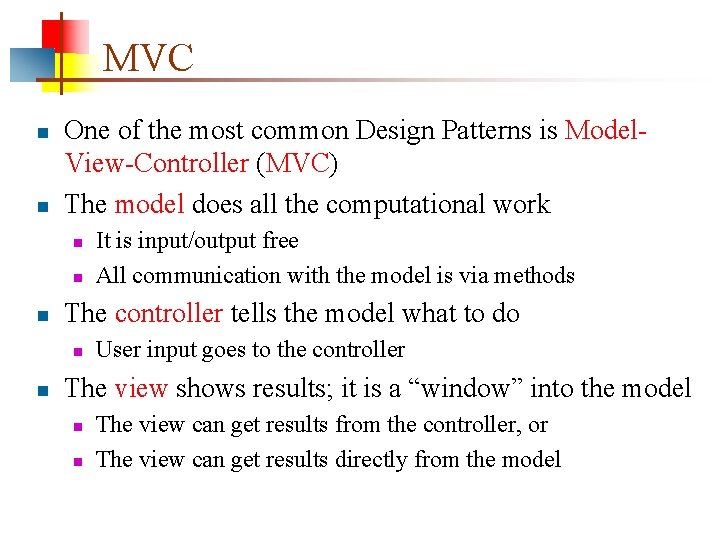
MVC n n One of the most common Design Patterns is Model. View-Controller (MVC) The model does all the computational work n n n The controller tells the model what to do n n It is input/output free All communication with the model is via methods User input goes to the controller The view shows results; it is a “window” into the model n n The view can get results from the controller, or The view can get results directly from the model
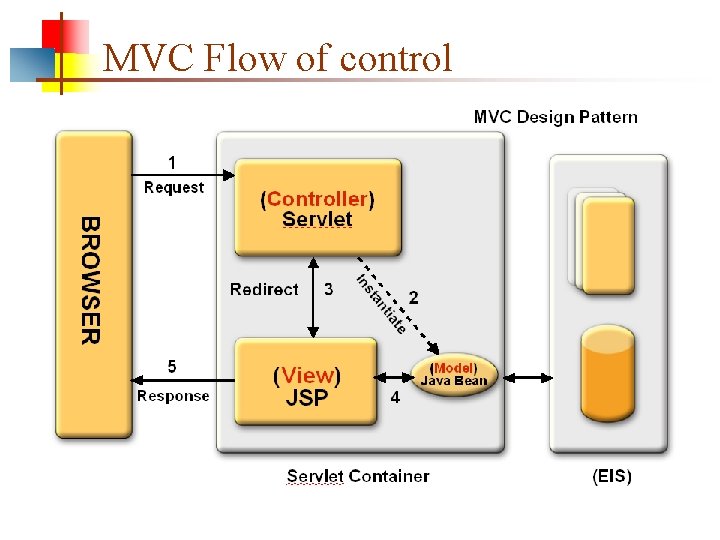
MVC Flow of control
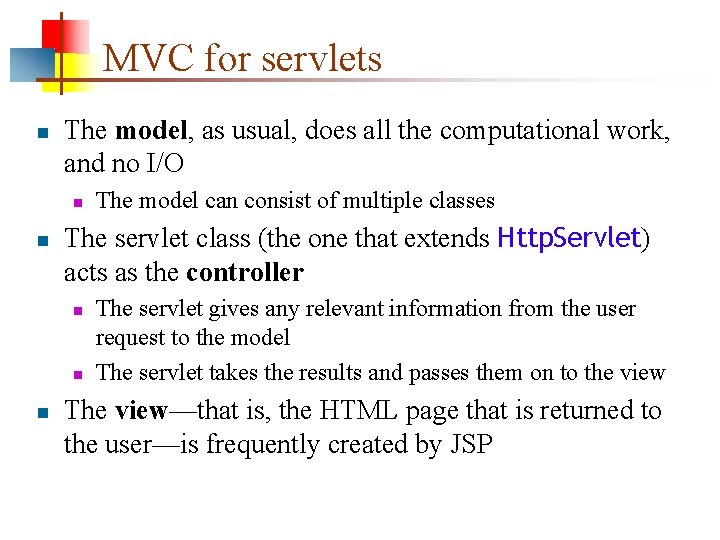
MVC for servlets n The model, as usual, does all the computational work, and no I/O n n The servlet class (the one that extends Http. Servlet) acts as the controller n n n The model can consist of multiple classes The servlet gives any relevant information from the user request to the model The servlet takes the results and passes them on to the view The view—that is, the HTML page that is returned to the user—is frequently created by JSP
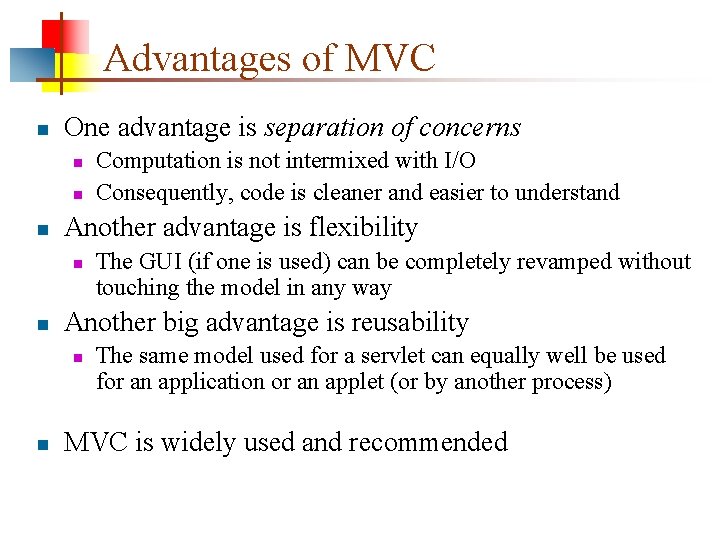
Advantages of MVC n One advantage is separation of concerns n n n Another advantage is flexibility n n The GUI (if one is used) can be completely revamped without touching the model in any way Another big advantage is reusability n n Computation is not intermixed with I/O Consequently, code is cleaner and easier to understand The same model used for a servlet can equally well be used for an application or an applet (or by another process) MVC is widely used and recommended
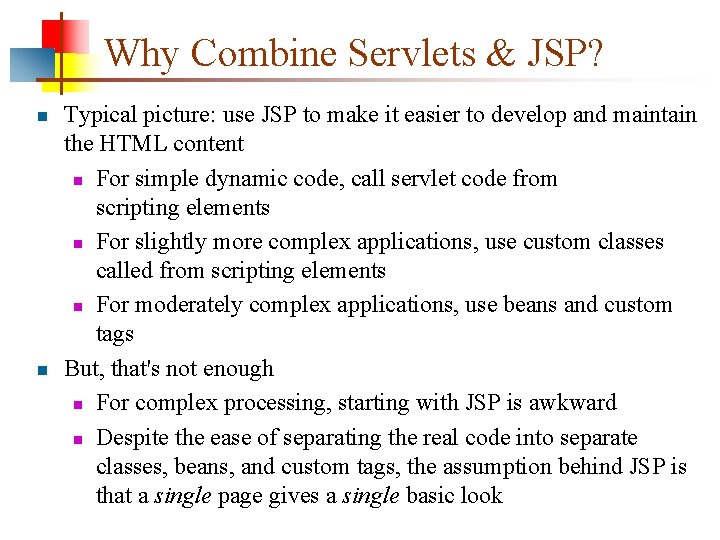
Why Combine Servlets & JSP? n n Typical picture: use JSP to make it easier to develop and maintain the HTML content n For simple dynamic code, call servlet code from scripting elements n For slightly more complex applications, use custom classes called from scripting elements n For moderately complex applications, use beans and custom tags But, that's not enough n For complex processing, starting with JSP is awkward n Despite the ease of separating the real code into separate classes, beans, and custom tags, the assumption behind JSP is that a single page gives a single basic look
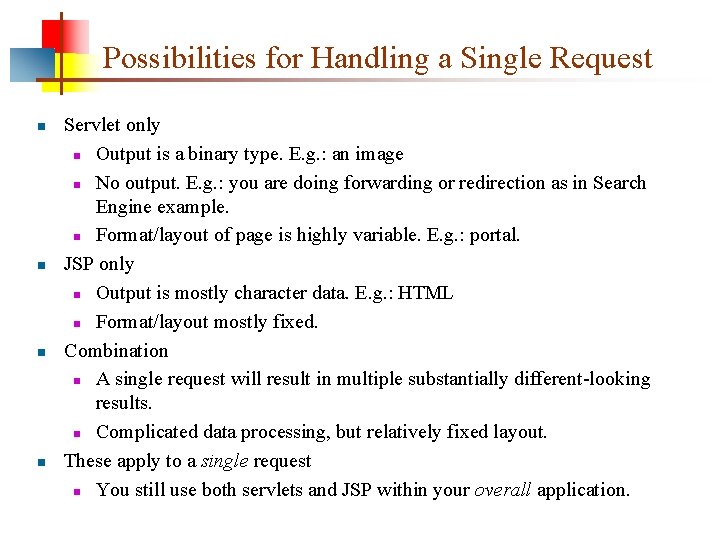
Possibilities for Handling a Single Request n n Servlet only n Output is a binary type. E. g. : an image n No output. E. g. : you are doing forwarding or redirection as in Search Engine example. n Format/layout of page is highly variable. E. g. : portal. JSP only n Output is mostly character data. E. g. : HTML n Format/layout mostly fixed. Combination n A single request will result in multiple substantially different-looking results. n Complicated data processing, but relatively fixed layout. These apply to a single request n You still use both servlets and JSP within your overall application.
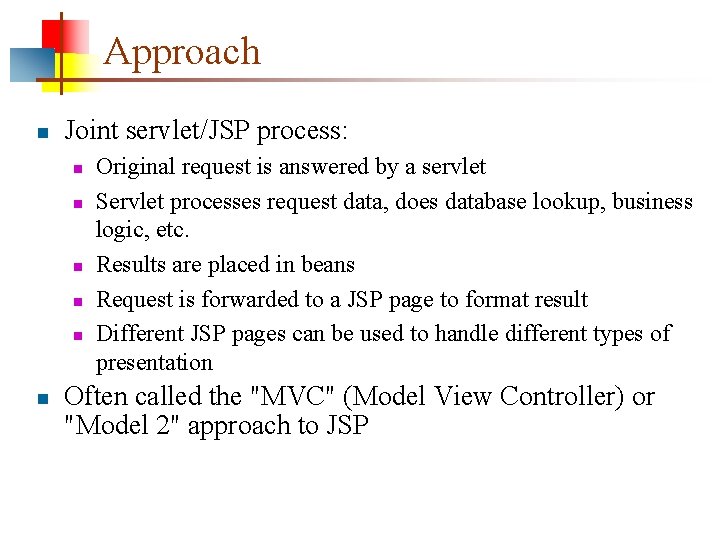
Approach n Joint servlet/JSP process: n n n Original request is answered by a servlet Servlet processes request data, does database lookup, business logic, etc. Results are placed in beans Request is forwarded to a JSP page to format result Different JSP pages can be used to handle different types of presentation Often called the "MVC" (Model View Controller) or "Model 2" approach to JSP
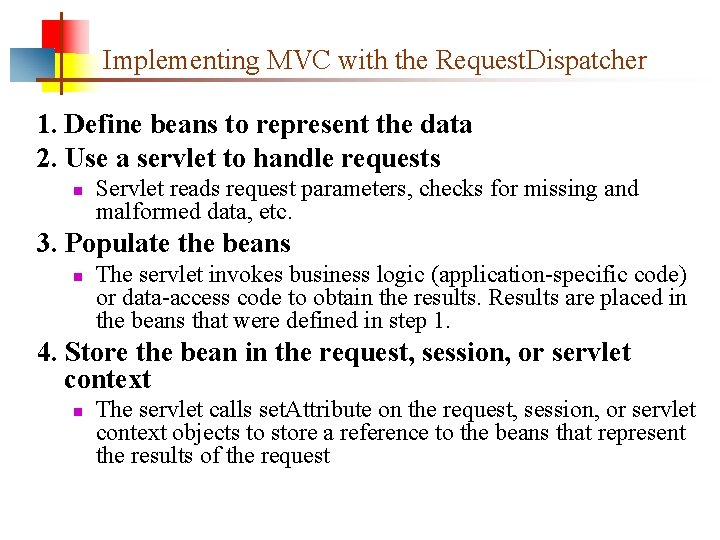
Implementing MVC with the Request. Dispatcher 1. Define beans to represent the data 2. Use a servlet to handle requests n Servlet reads request parameters, checks for missing and malformed data, etc. 3. Populate the beans n The servlet invokes business logic (application-specific code) or data-access code to obtain the results. Results are placed in the beans that were defined in step 1. 4. Store the bean in the request, session, or servlet context n The servlet calls set. Attribute on the request, session, or servlet context objects to store a reference to the beans that represent the results of the request
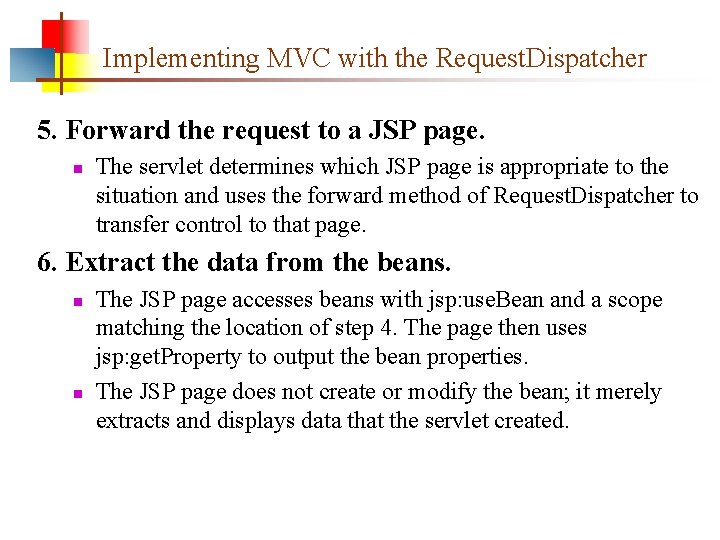
Implementing MVC with the Request. Dispatcher 5. Forward the request to a JSP page. n The servlet determines which JSP page is appropriate to the situation and uses the forward method of Request. Dispatcher to transfer control to that page. 6. Extract the data from the beans. n n The JSP page accesses beans with jsp: use. Bean and a scope matching the location of step 4. The page then uses jsp: get. Property to output the bean properties. The JSP page does not create or modify the bean; it merely extracts and displays data that the servlet created.
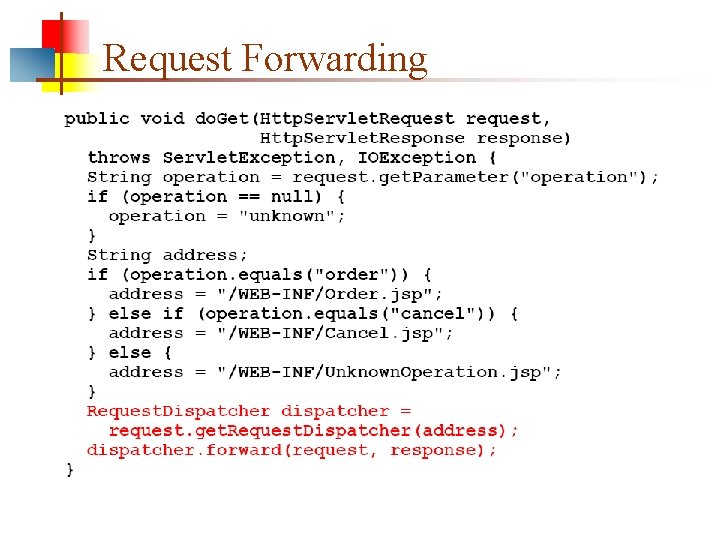
Request Forwarding
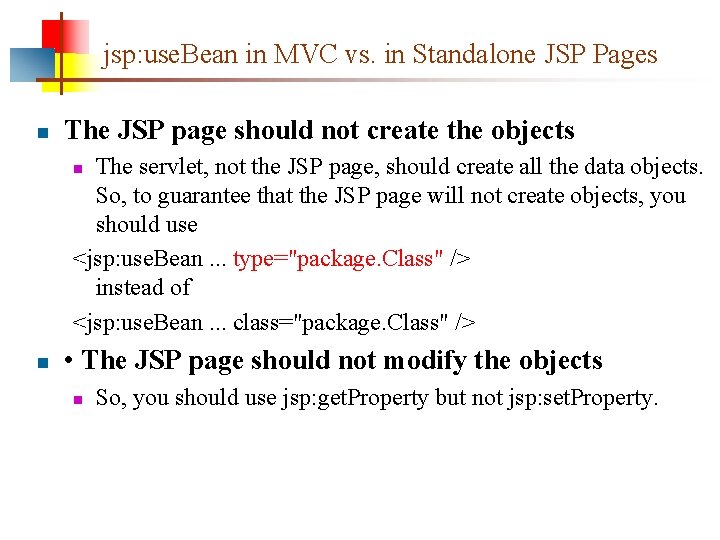
jsp: use. Bean in MVC vs. in Standalone JSP Pages n The JSP page should not create the objects The servlet, not the JSP page, should create all the data objects. So, to guarantee that the JSP page will not create objects, you should use <jsp: use. Bean. . . type="package. Class" /> instead of <jsp: use. Bean. . . class="package. Class" /> n n • The JSP page should not modify the objects n So, you should use jsp: get. Property but not jsp: set. Property.
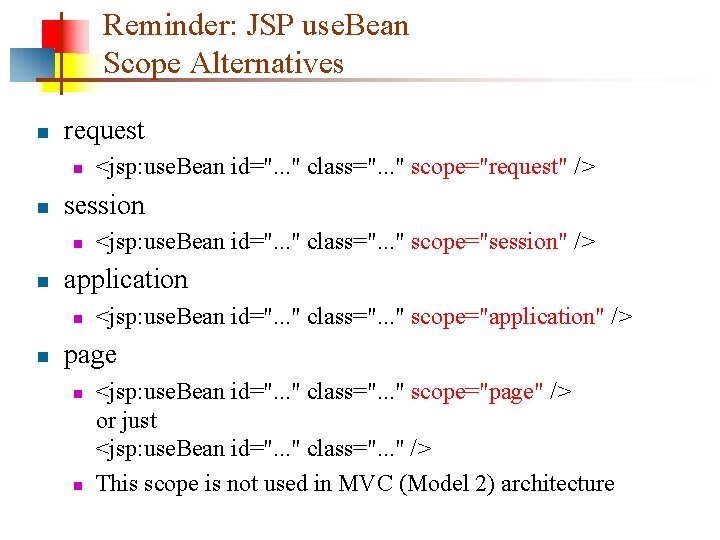
Reminder: JSP use. Bean Scope Alternatives n request n n session n n <jsp: use. Bean id=". . . " class=". . . " scope="session" /> application n n <jsp: use. Bean id=". . . " class=". . . " scope="request" /> <jsp: use. Bean id=". . . " class=". . . " scope="application" /> page n n <jsp: use. Bean id=". . . " class=". . . " scope="page" /> or just <jsp: use. Bean id=". . . " class=". . . " /> This scope is not used in MVC (Model 2) architecture
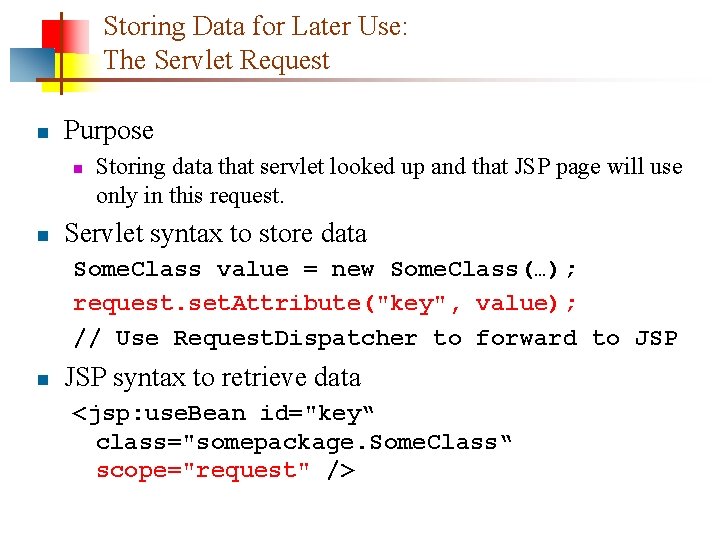
Storing Data for Later Use: The Servlet Request n Purpose n n Storing data that servlet looked up and that JSP page will use only in this request. Servlet syntax to store data Some. Class value = new Some. Class(…); request. set. Attribute("key", value); // Use Request. Dispatcher to forward to JSP n JSP syntax to retrieve data <jsp: use. Bean id="key“ class="somepackage. Some. Class“ scope="request" />
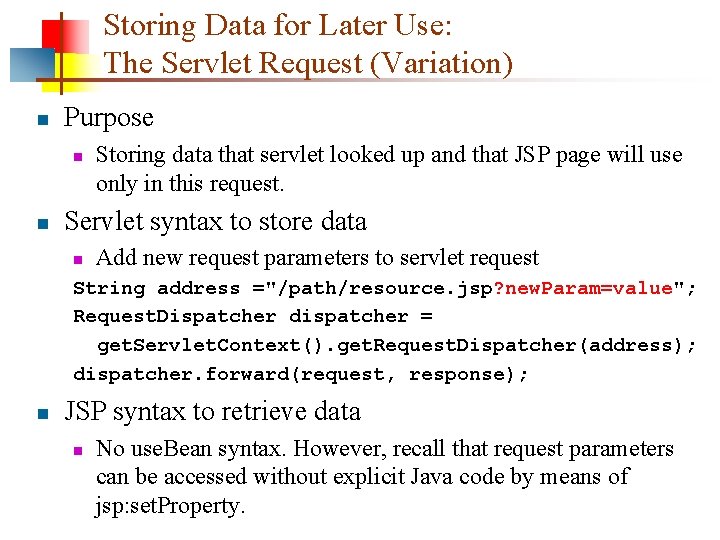
Storing Data for Later Use: The Servlet Request (Variation) n Purpose n n Storing data that servlet looked up and that JSP page will use only in this request. Servlet syntax to store data n Add new request parameters to servlet request String address ="/path/resource. jsp? new. Param=value"; Request. Dispatcher dispatcher = get. Servlet. Context(). get. Request. Dispatcher(address); dispatcher. forward(request, response); n JSP syntax to retrieve data n No use. Bean syntax. However, recall that request parameters can be accessed without explicit Java code by means of jsp: set. Property.
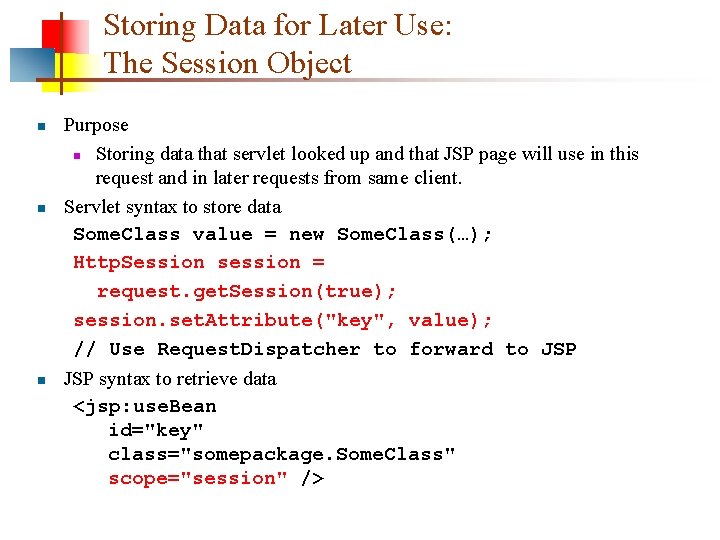
Storing Data for Later Use: The Session Object n n n Purpose n Storing data that servlet looked up and that JSP page will use in this request and in later requests from same client. Servlet syntax to store data Some. Class value = new Some. Class(…); Http. Session session = request. get. Session(true); session. set. Attribute("key", value); // Use Request. Dispatcher to forward to JSP syntax to retrieve data <jsp: use. Bean id="key" class="somepackage. Some. Class" scope="session" />
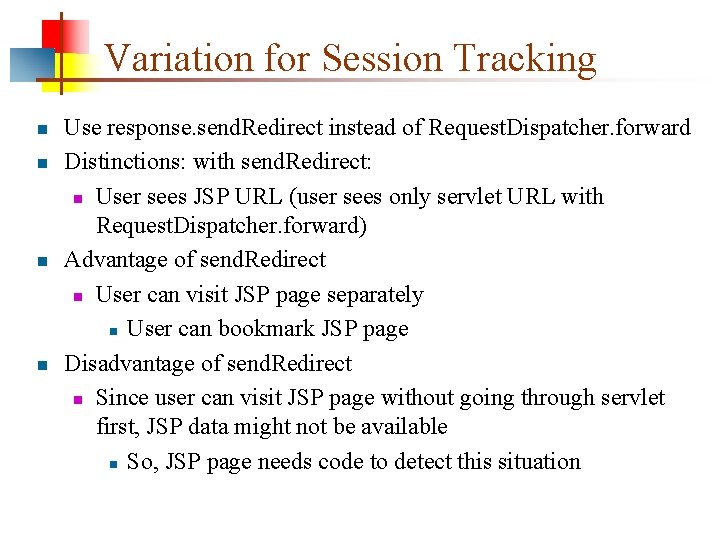
Variation for Session Tracking n n Use response. send. Redirect instead of Request. Dispatcher. forward Distinctions: with send. Redirect: n User sees JSP URL (user sees only servlet URL with Request. Dispatcher. forward) Advantage of send. Redirect n User can visit JSP page separately n User can bookmark JSP page Disadvantage of send. Redirect n Since user can visit JSP page without going through servlet first, JSP data might not be available n So, JSP page needs code to detect this situation
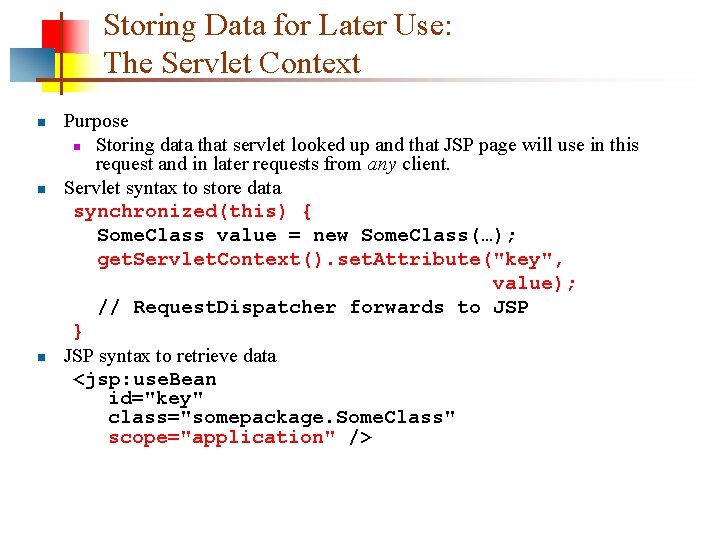
Storing Data for Later Use: The Servlet Context n n n Purpose n Storing data that servlet looked up and that JSP page will use in this request and in later requests from any client. Servlet syntax to store data synchronized(this) { Some. Class value = new Some. Class(…); get. Servlet. Context(). set. Attribute("key", value); // Request. Dispatcher forwards to JSP } JSP syntax to retrieve data <jsp: use. Bean id="key" class="somepackage. Some. Class" scope="application" />
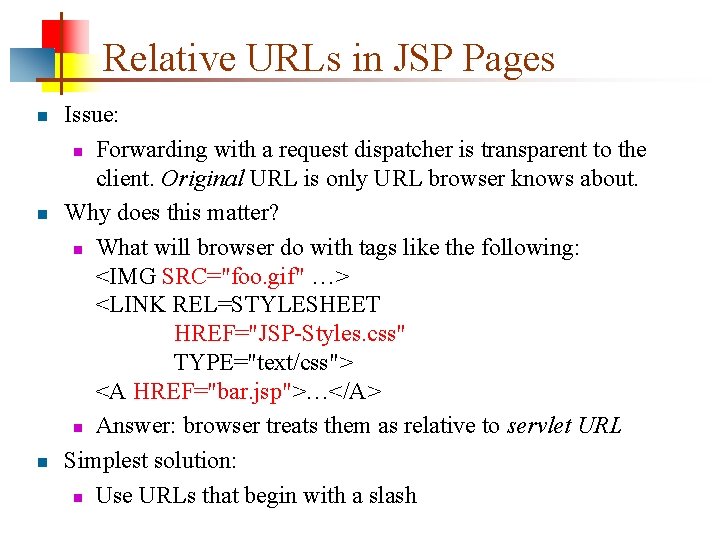
Relative URLs in JSP Pages n n n Issue: n Forwarding with a request dispatcher is transparent to the client. Original URL is only URL browser knows about. Why does this matter? n What will browser do with tags like the following: <IMG SRC="foo. gif" …> <LINK REL=STYLESHEET HREF="JSP-Styles. css" TYPE="text/css"> <A HREF="bar. jsp">…</A> n Answer: browser treats them as relative to servlet URL Simplest solution: n Use URLs that begin with a slash
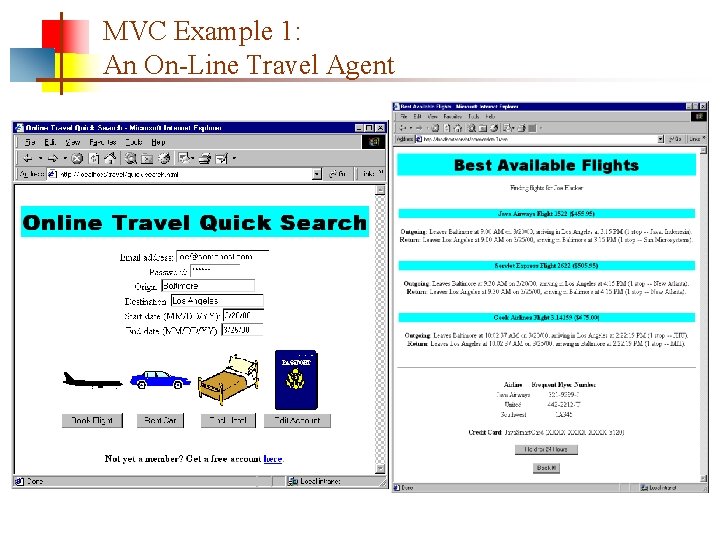
MVC Example 1: An On-Line Travel Agent
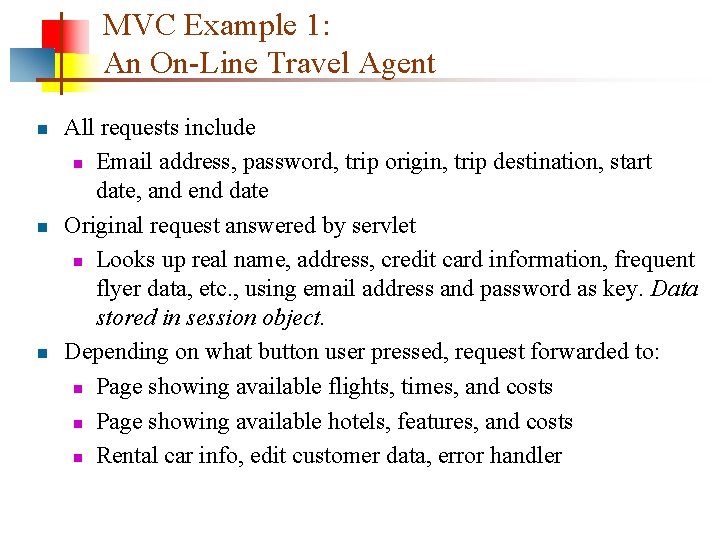
MVC Example 1: An On-Line Travel Agent n n n All requests include n Email address, password, trip origin, trip destination, start date, and end date Original request answered by servlet n Looks up real name, address, credit card information, frequent flyer data, etc. , using email address and password as key. Data stored in session object. Depending on what button user pressed, request forwarded to: n Page showing available flights, times, and costs n Page showing available hotels, features, and costs n Rental car info, edit customer data, error handler
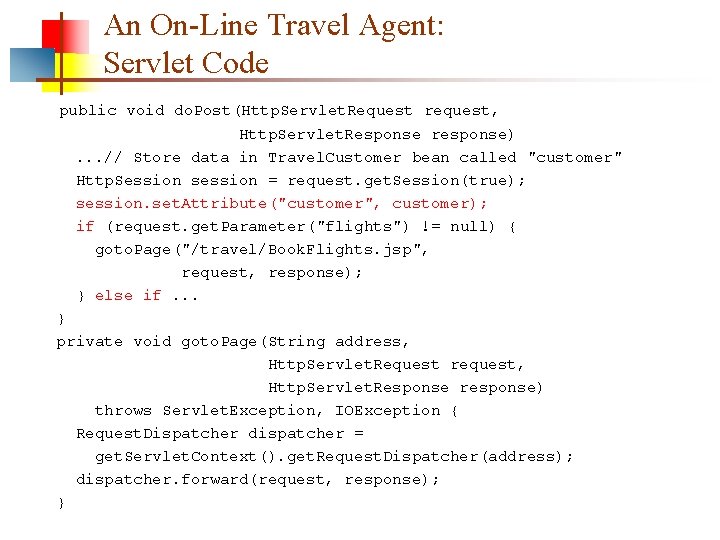
An On-Line Travel Agent: Servlet Code public void do. Post(Http. Servlet. Request request, Http. Servlet. Response response) . . . // Store data in Travel. Customer bean called "customer" Http. Session session = request. get. Session(true); session. set. Attribute("customer", customer); if (request. get. Parameter("flights") != null) { goto. Page("/travel/Book. Flights. jsp", request, response); } else if. . . } private void goto. Page(String address, Http. Servlet. Request request, Http. Servlet. Response response) throws Servlet. Exception, IOException { Request. Dispatcher dispatcher = get. Servlet. Context(). get. Request. Dispatcher(address); dispatcher. forward(request, response); }
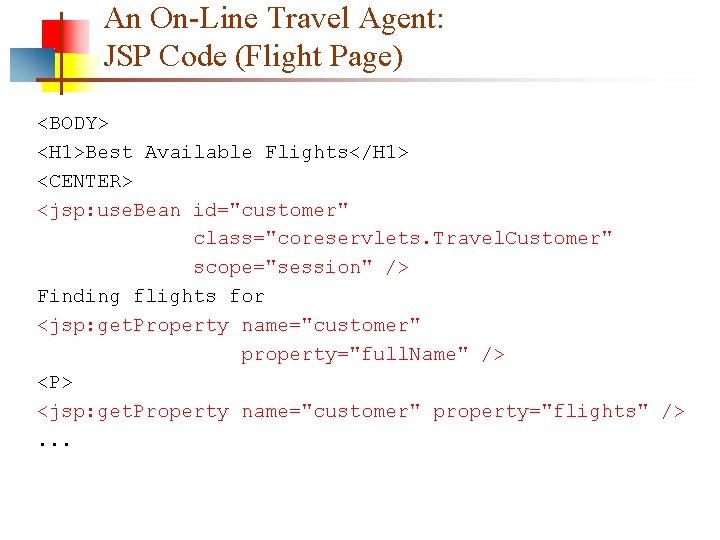
An On-Line Travel Agent: JSP Code (Flight Page) <BODY> <H 1>Best Available Flights</H 1> <CENTER> <jsp: use. Bean id="customer" class="coreservlets. Travel. Customer" scope="session" /> Finding flights for <jsp: get. Property name="customer" property="full. Name" /> <P> <jsp: get. Property name="customer" property="flights" />. . .
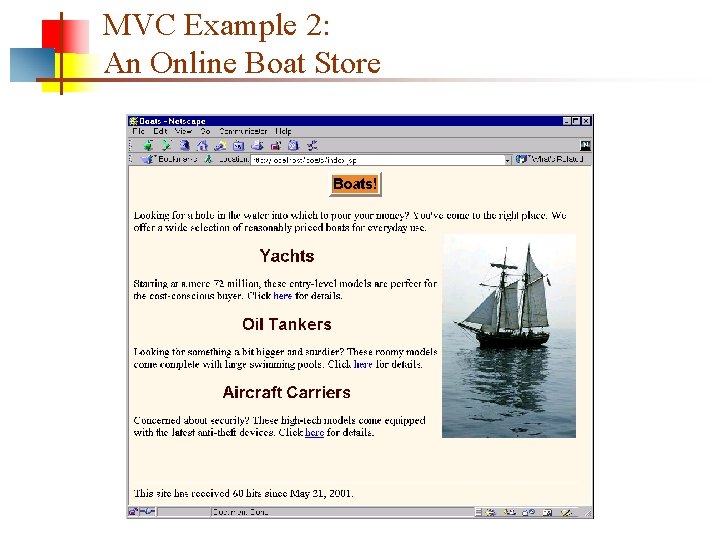
MVC Example 2: An Online Boat Store
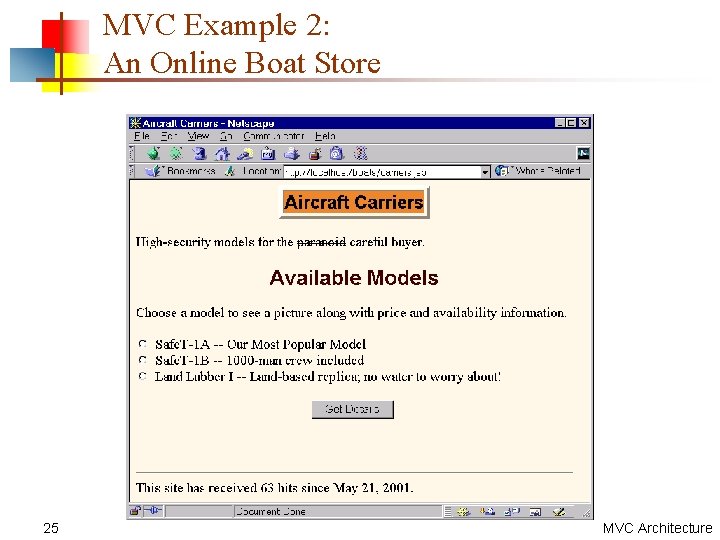
MVC Example 2: An Online Boat Store 25 MVC Architecture
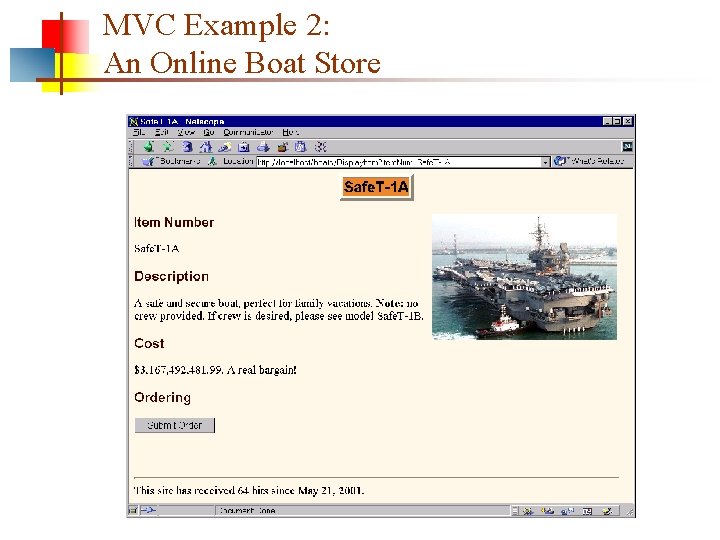
MVC Example 2: An Online Boat Store
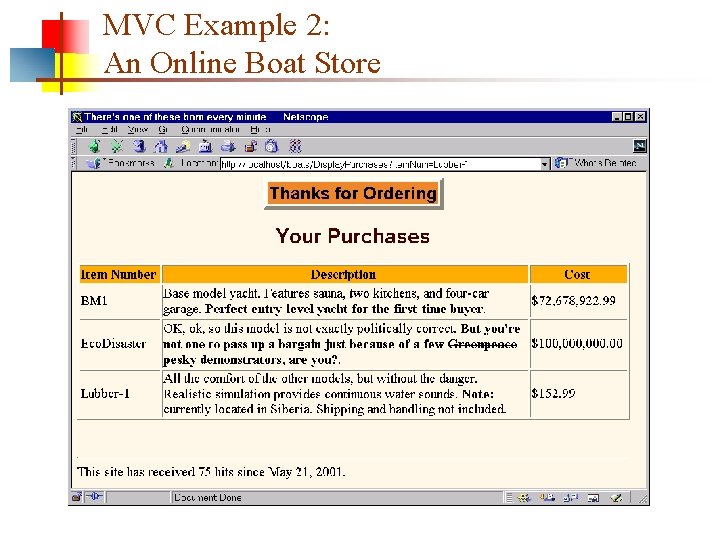
MVC Example 2: An Online Boat Store
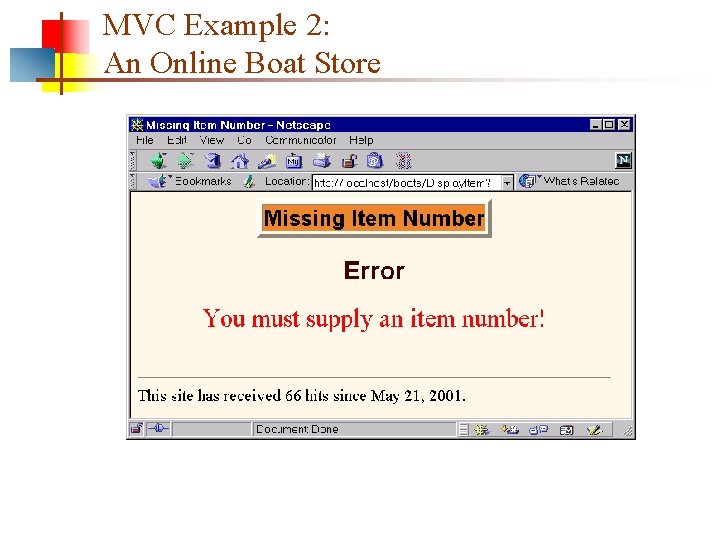
MVC Example 2: An Online Boat Store
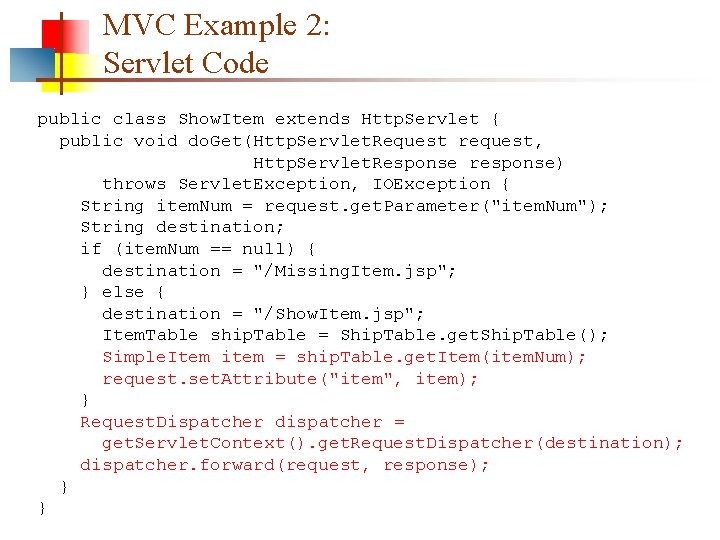
MVC Example 2: Servlet Code public class Show. Item extends Http. Servlet { public void do. Get(Http. Servlet. Request request, Http. Servlet. Response response) throws Servlet. Exception, IOException { String item. Num = request. get. Parameter("item. Num"); String destination; if (item. Num == null) { destination = "/Missing. Item. jsp"; } else { destination = "/Show. Item. jsp"; Item. Table ship. Table = Ship. Table. get. Ship. Table(); Simple. Item item = ship. Table. get. Item(item. Num); request. set. Attribute("item", item); } Request. Dispatcher dispatcher = get. Servlet. Context(). get. Request. Dispatcher(destination); dispatcher. forward(request, response); } }
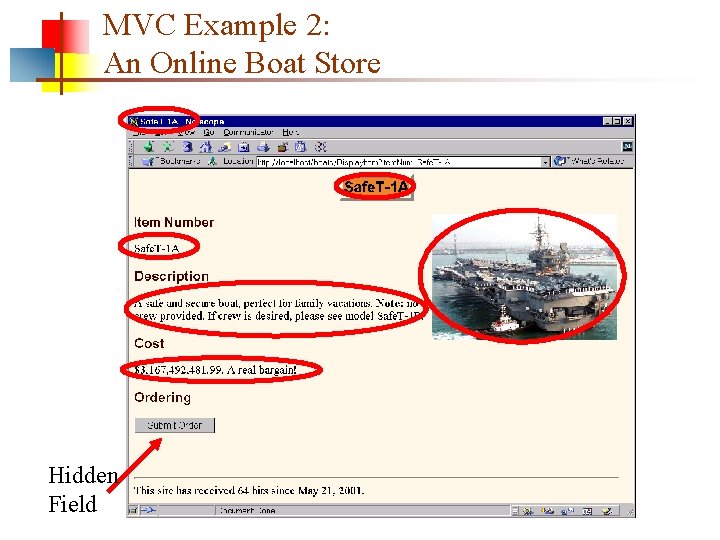
MVC Example 2: An Online Boat Store Hidden Field
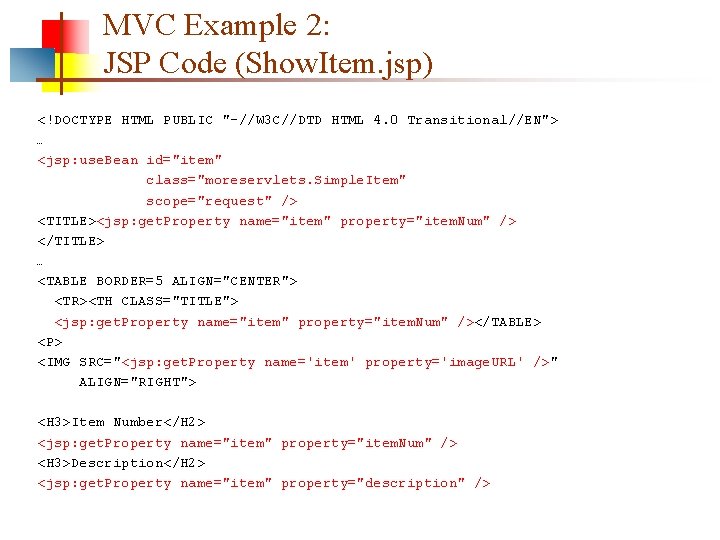
MVC Example 2: JSP Code (Show. Item. jsp) <!DOCTYPE HTML PUBLIC "-//W 3 C//DTD HTML 4. 0 Transitional//EN"> … <jsp: use. Bean id="item" class="moreservlets. Simple. Item" scope="request" /> <TITLE><jsp: get. Property name="item" property="item. Num" /> </TITLE> … <TABLE BORDER=5 ALIGN="CENTER"> <TR><TH CLASS="TITLE"> <jsp: get. Property name="item" property="item. Num" /></TABLE> <P> <IMG SRC="<jsp: get. Property name='item' property='image. URL' />" ALIGN="RIGHT"> <H 3>Item Number</H 2> <jsp: get. Property name="item" property="item. Num" /> <H 3>Description</H 2> <jsp: get. Property name="item" property="description" />
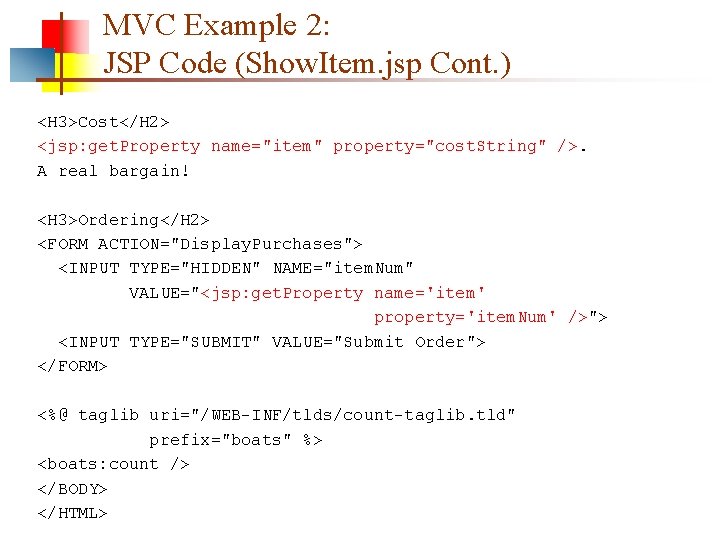
MVC Example 2: JSP Code (Show. Item. jsp Cont. ) <H 3>Cost</H 2> <jsp: get. Property name="item" property="cost. String" />. A real bargain! <H 3>Ordering</H 2> <FORM ACTION="Display. Purchases"> <INPUT TYPE="HIDDEN" NAME="item. Num" VALUE="<jsp: get. Property name='item' property='item. Num' />"> <INPUT TYPE="SUBMIT" VALUE="Submit Order"> </FORM> <%@ taglib uri="/WEB-INF/tlds/count-taglib. tld" prefix="boats" %> <boats: count /> </BODY> </HTML>
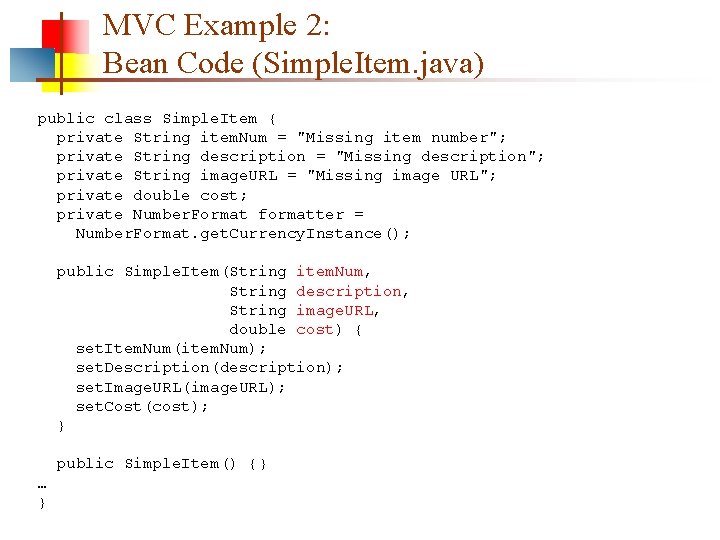
MVC Example 2: Bean Code (Simple. Item. java) public class Simple. Item { private String item. Num = "Missing item number"; private String description = "Missing description"; private String image. URL = "Missing image URL"; private double cost; private Number. Format formatter = Number. Format. get. Currency. Instance(); public Simple. Item(String item. Num, String description, String image. URL, double cost) { set. Item. Num(item. Num); set. Description(description); set. Image. URL(image. URL); set. Cost(cost); } public Simple. Item() {} … }
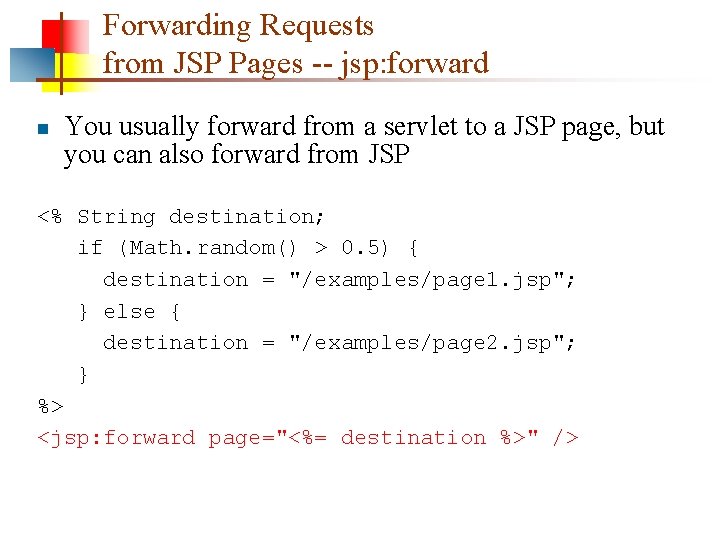
Forwarding Requests from JSP Pages -- jsp: forward n You usually forward from a servlet to a JSP page, but you can also forward from JSP <% String destination; if (Math. random() > 0. 5) { destination = "/examples/page 1. jsp"; } else { destination = "/examples/page 2. jsp"; } %> <jsp: forward page="<%= destination %>" />
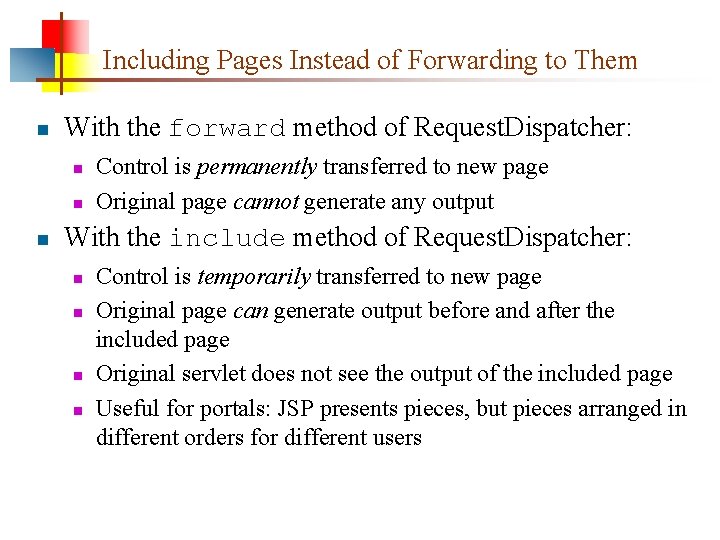
Including Pages Instead of Forwarding to Them n With the forward method of Request. Dispatcher: n n n Control is permanently transferred to new page Original page cannot generate any output With the include method of Request. Dispatcher: n n Control is temporarily transferred to new page Original page can generate output before and after the included page Original servlet does not see the output of the included page Useful for portals: JSP presents pieces, but pieces arranged in different orders for different users
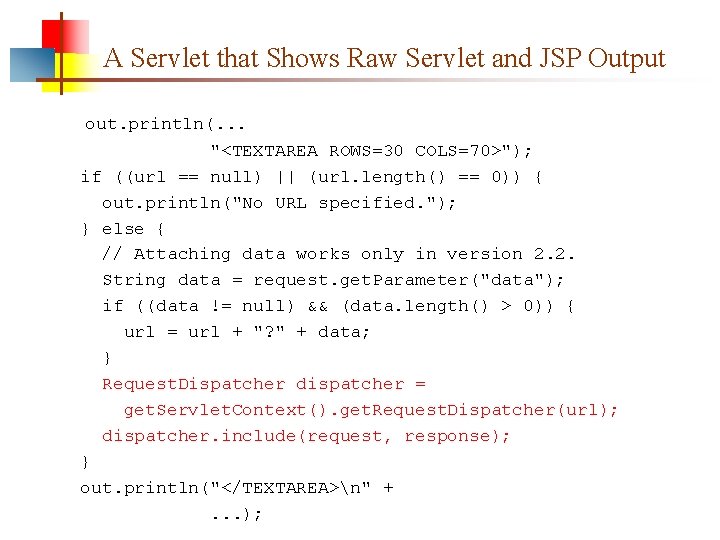
A Servlet that Shows Raw Servlet and JSP Output out. println(. . . "<TEXTAREA ROWS=30 COLS=70>"); if ((url == null) || (url. length() == 0)) { out. println("No URL specified. "); } else { // Attaching data works only in version 2. 2. String data = request. get. Parameter("data"); if ((data != null) && (data. length() > 0)) { url = url + "? " + data; } Request. Dispatcher dispatcher = get. Servlet. Context(). get. Request. Dispatcher(url); dispatcher. include(request, response); } out. println("</TEXTAREA>n" + . . . );
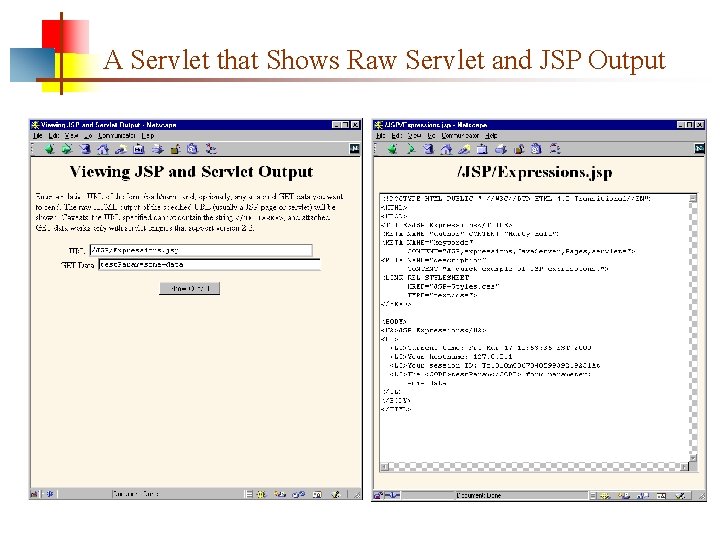
A Servlet that Shows Raw Servlet and JSP Output
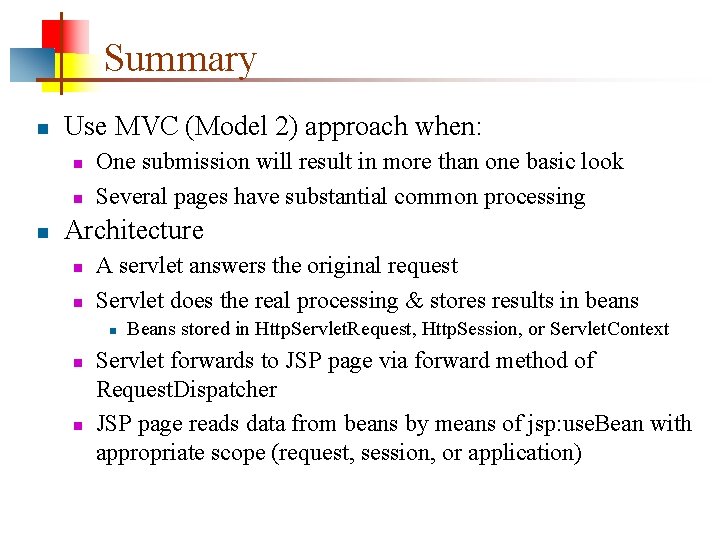
Summary n Use MVC (Model 2) approach when: n n n One submission will result in more than one basic look Several pages have substantial common processing Architecture n n A servlet answers the original request Servlet does the real processing & stores results in beans n n n Beans stored in Http. Servlet. Request, Http. Session, or Servlet. Context Servlet forwards to JSP page via forward method of Request. Dispatcher JSP page reads data from beans by means of jsp: use. Bean with appropriate scope (request, session, or application)
What is a partial view
Coreservlets
Servlets notes
Ruby paradigm
Model view controller
Pattern mvc java
Model view controller
Gridpane scene builder
Mvc architecture in jsp
Swing mvc
I/o modules in computer architecture
Ddr controller architecture
4+1 architectural view model
Model layer in mvc
Thin controller fat model
Formuö
Typiska drag för en novell
Tack för att ni lyssnade bild
Returpilarna
Shingelfrisyren
En lathund för arbete med kontinuitetshantering
Adressändring ideell förening
Tidbok för yrkesförare
A gastrica
Förklara densitet för barn
Datorkunskap för nybörjare
Stig kerman
Att skriva en debattartikel
Delegerande ledarskap
Nyckelkompetenser för livslångt lärande
Påbyggnader för flakfordon
Formel för lufttryck
Offentlig förvaltning
Jag har nigit för nymånens skära text
Presentera för publik crossboss
Vad är ett minoritetsspråk
Plats för toran ark
Treserva lathund
Mjälthilus