Lecture 11 Code Generation Joey Paquet 2000 2014
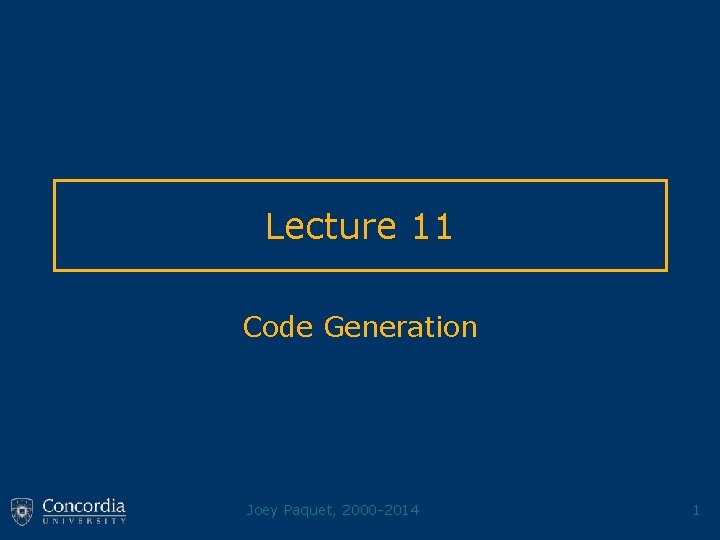
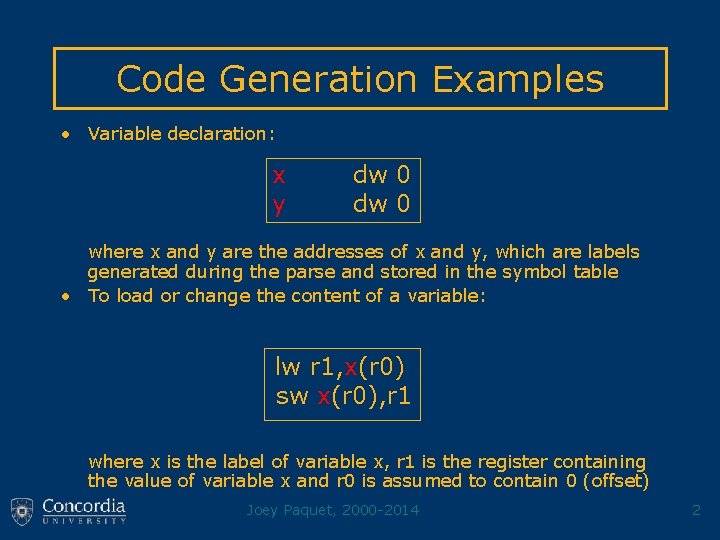
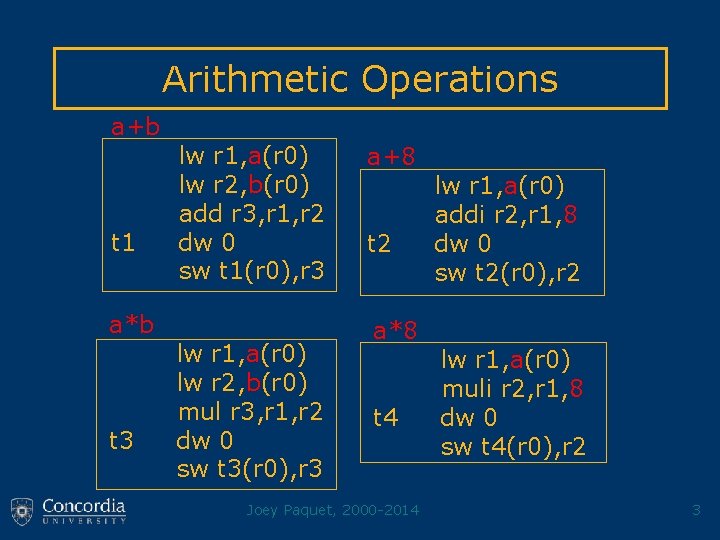
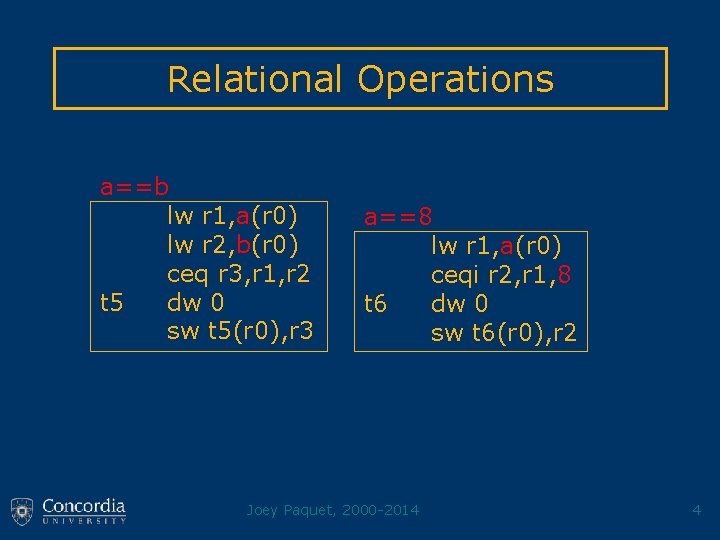
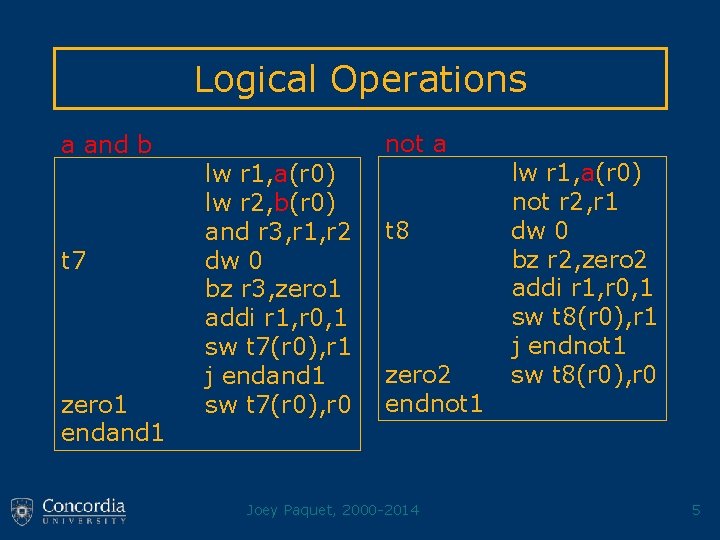
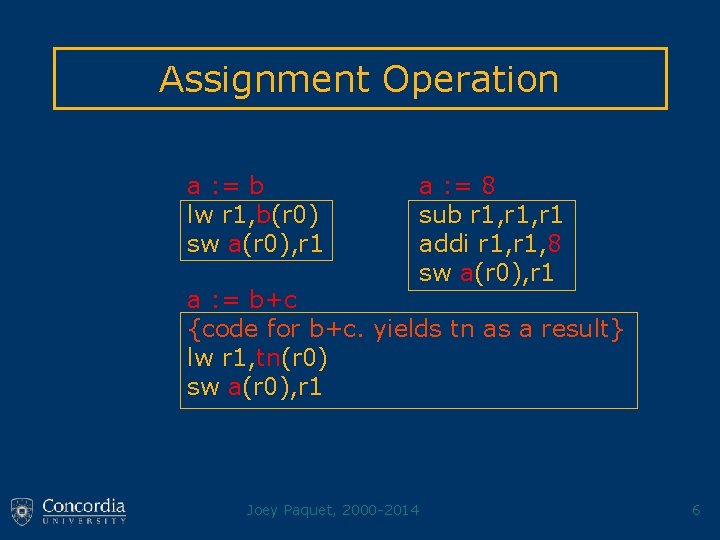
![Conditional Statements if a>b then a: =b else a: =0; [1] [2] [3] [4] Conditional Statements if a>b then a: =b else a: =0; [1] [2] [3] [4]](https://slidetodoc.com/presentation_image_h2/afa3d3f2521efa48382742ca2971b946/image-7.jpg)
![Loop Statements while a<b do a: =a+1; [1] [2] [3] [4] [5] gowhile 1 Loop Statements while a<b do a: =a+1; [1] [2] [3] [4] [5] gowhile 1](https://slidetodoc.com/presentation_image_h2/afa3d3f2521efa48382742ca2971b946/image-8.jpg)
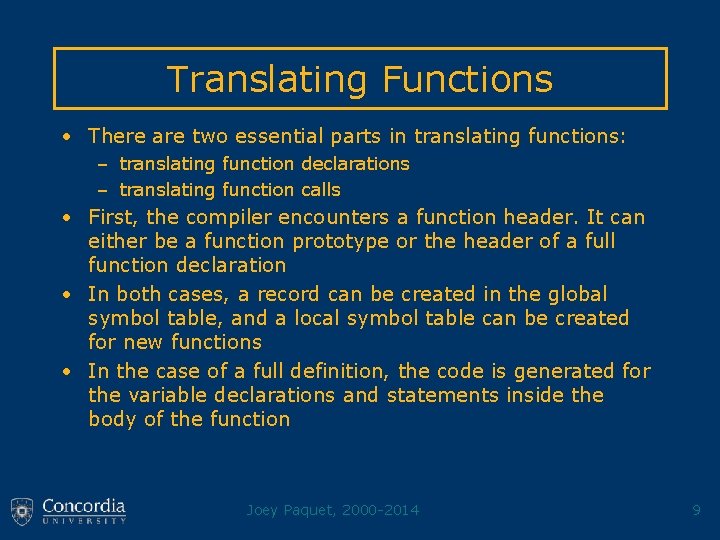
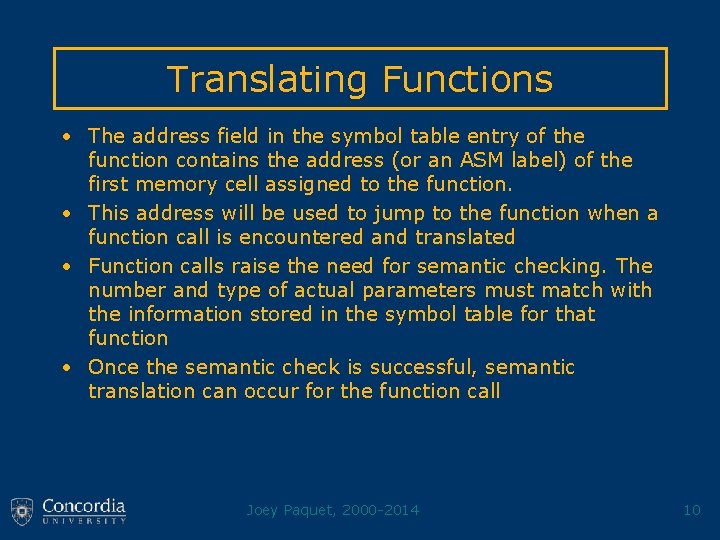
![Function Declarations int fn ( int a, int b ){ statlist }; [1] [2] Function Declarations int fn ( int a, int b ){ statlist }; [1] [2]](https://slidetodoc.com/presentation_image_h2/afa3d3f2521efa48382742ca2971b946/image-11.jpg)
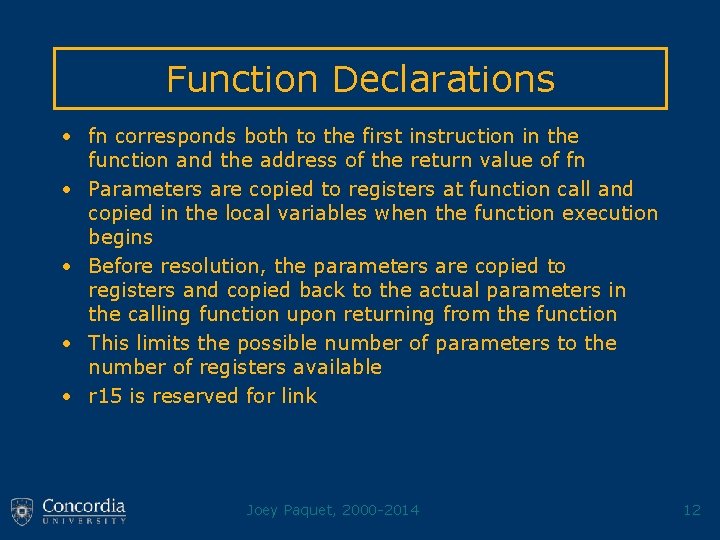
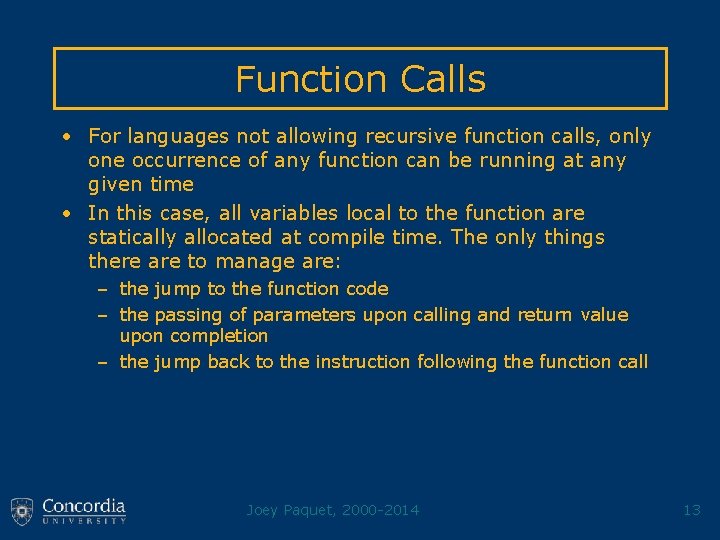
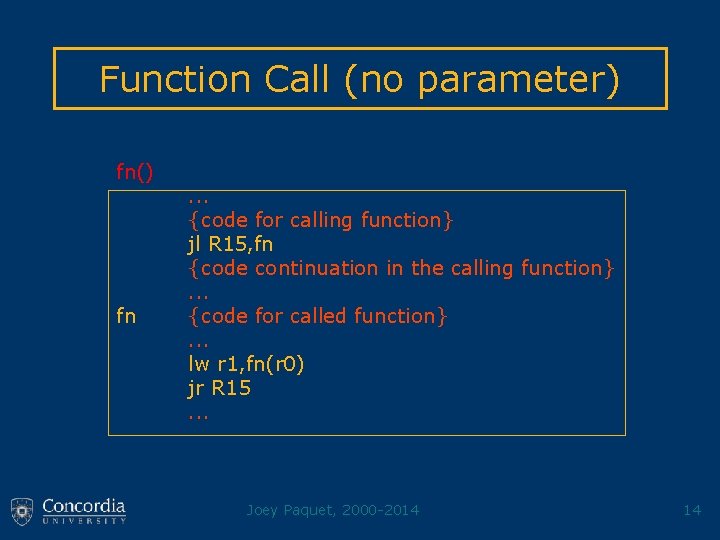
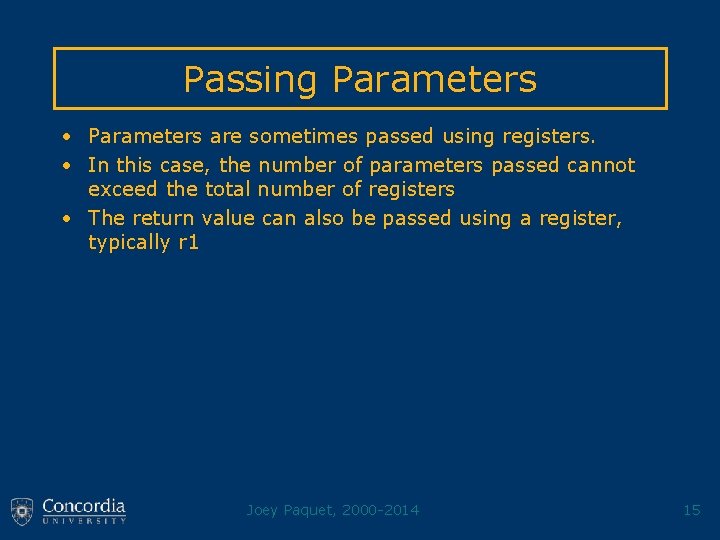
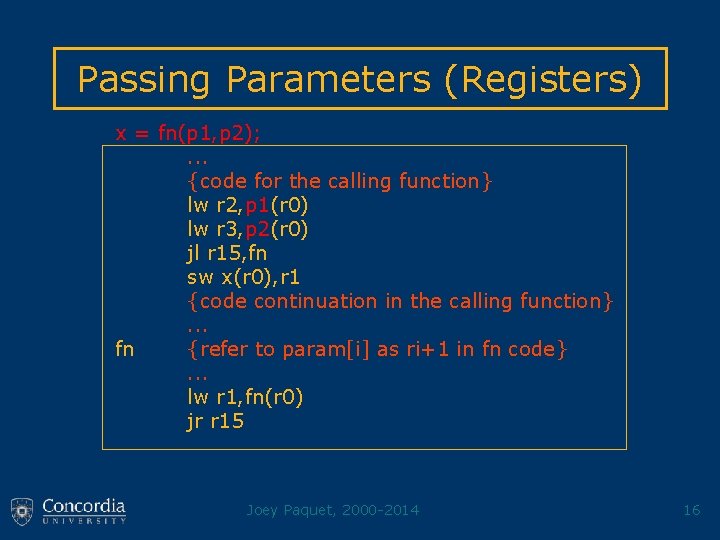
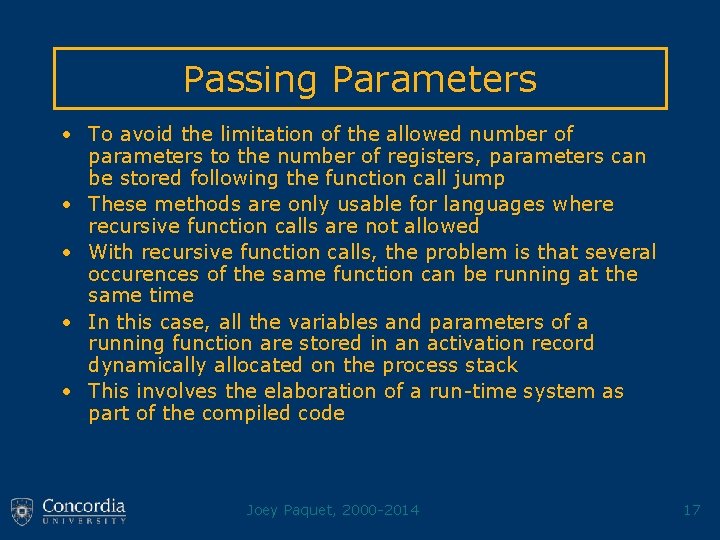
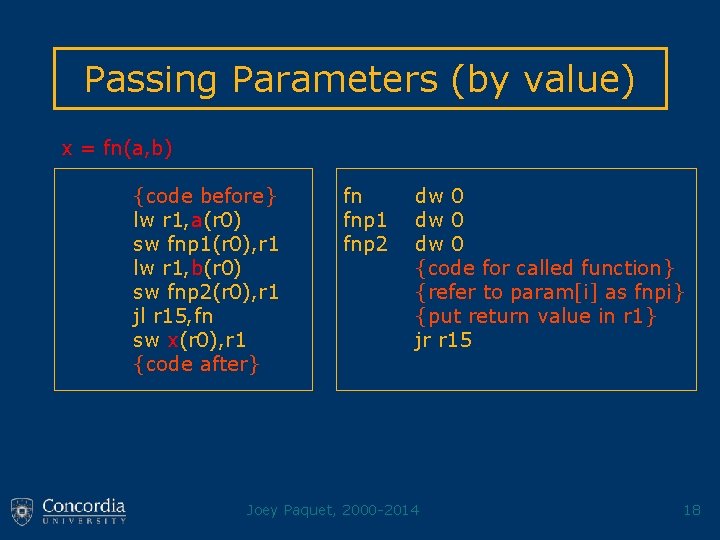
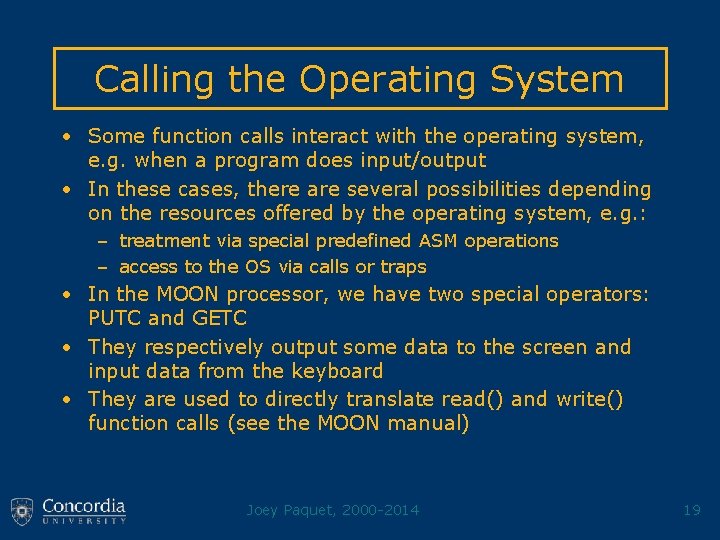
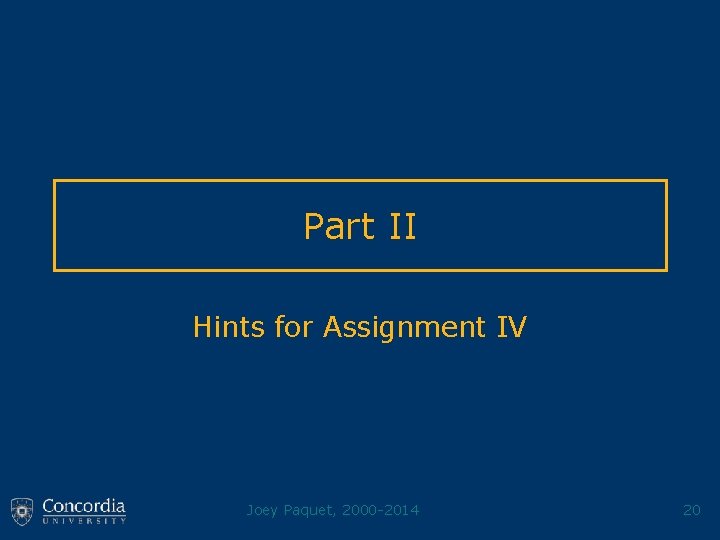
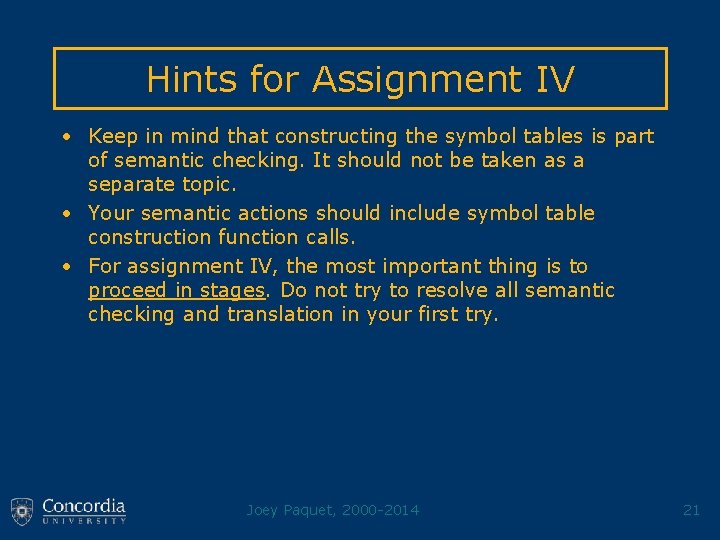
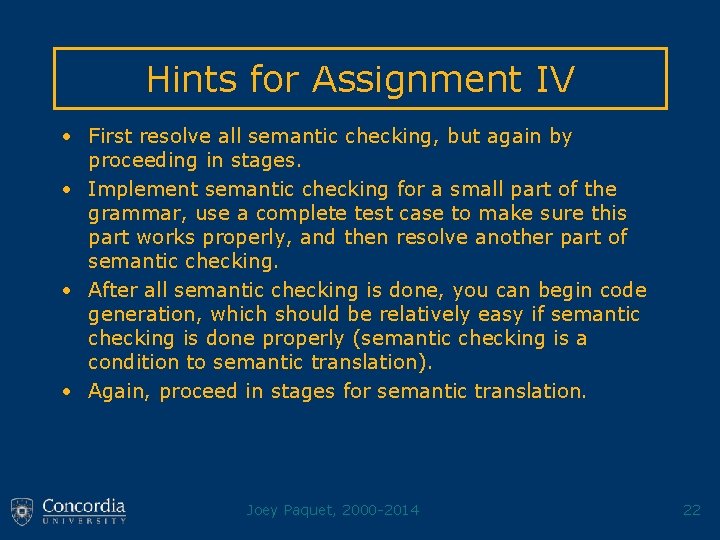
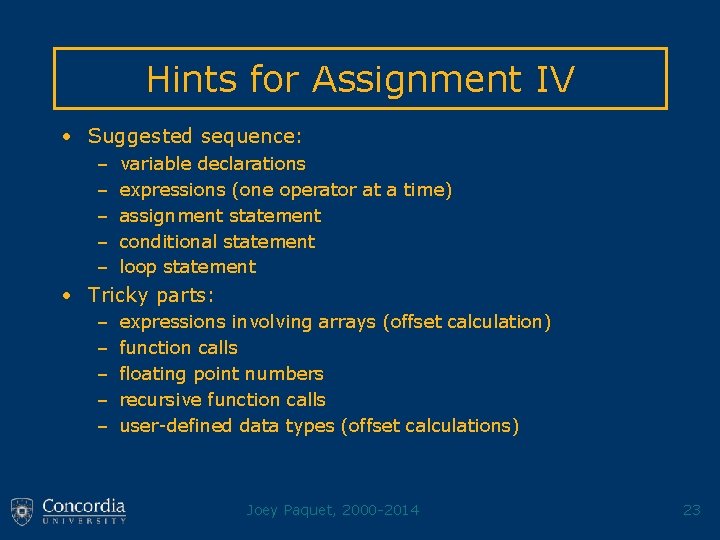
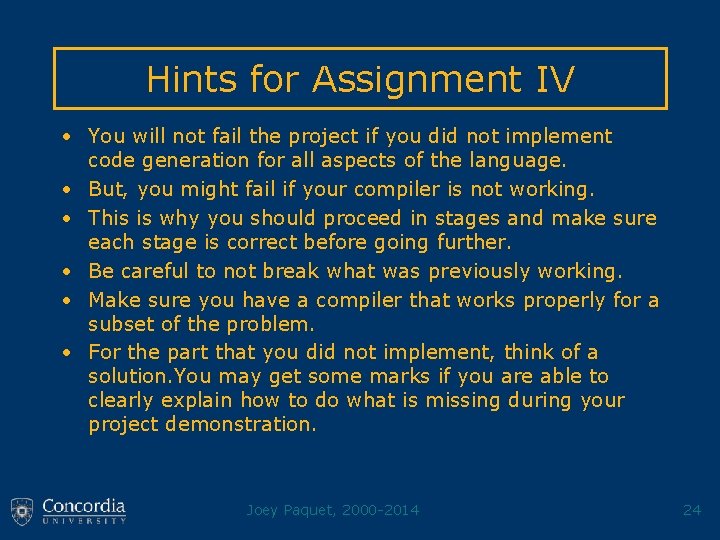
- Slides: 24
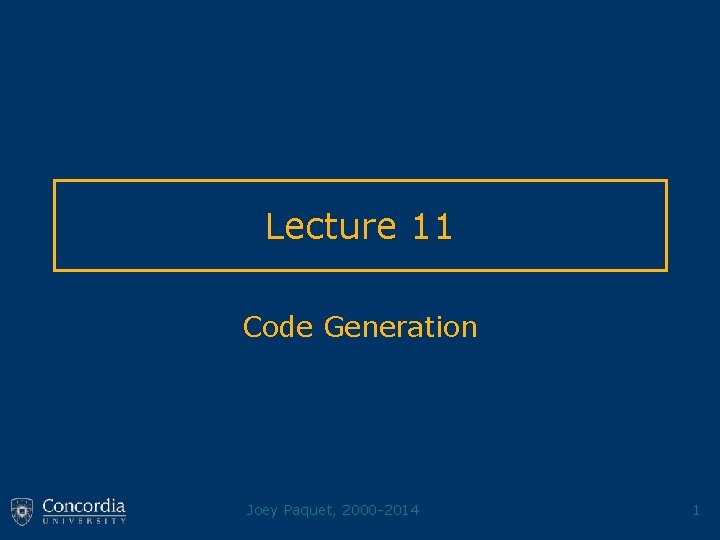
Lecture 11 Code Generation Joey Paquet, 2000 -2014 1
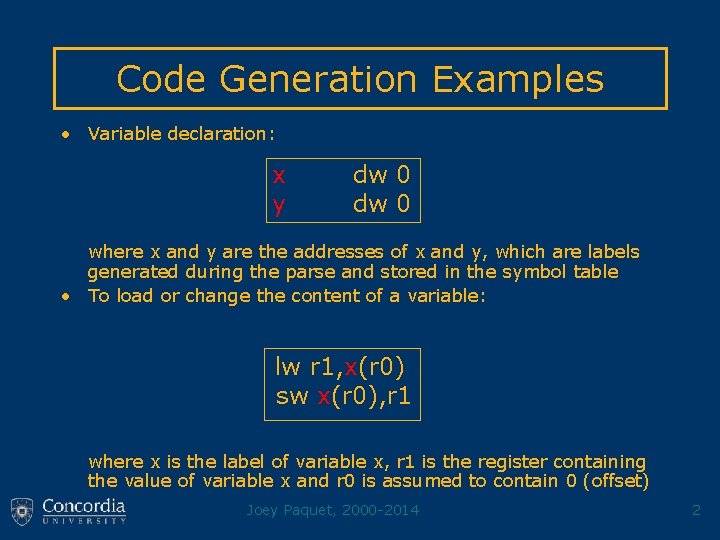
Code Generation Examples • Variable declaration: x y dw 0 where x and y are the addresses of x and y, which are labels generated during the parse and stored in the symbol table • To load or change the content of a variable: lw r 1, x(r 0) sw x(r 0), r 1 where x is the label of variable x, r 1 is the register containing the value of variable x and r 0 is assumed to contain 0 (offset) Joey Paquet, 2000 -2014 2
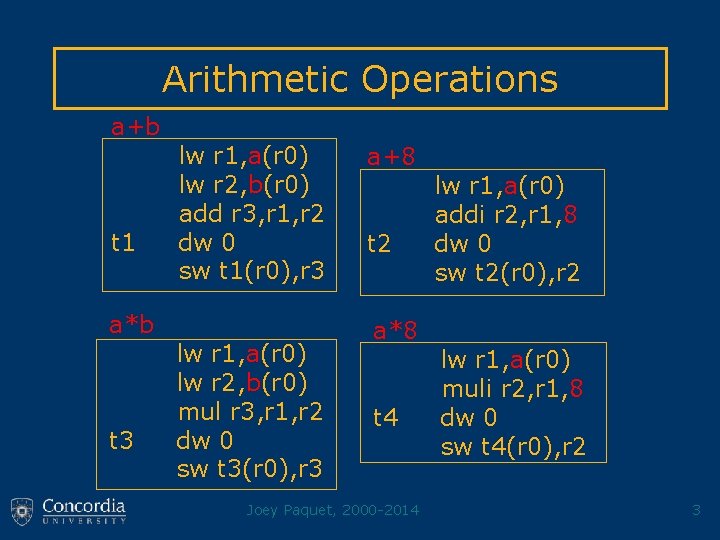
Arithmetic Operations a+b t 1 a*b t 3 lw r 1, a(r 0) lw r 2, b(r 0) add r 3, r 1, r 2 dw 0 sw t 1(r 0), r 3 lw r 1, a(r 0) lw r 2, b(r 0) mul r 3, r 1, r 2 dw 0 sw t 3(r 0), r 3 a+8 t 2 a*8 t 4 Joey Paquet, 2000 -2014 lw r 1, a(r 0) addi r 2, r 1, 8 dw 0 sw t 2(r 0), r 2 lw r 1, a(r 0) muli r 2, r 1, 8 dw 0 sw t 4(r 0), r 2 3
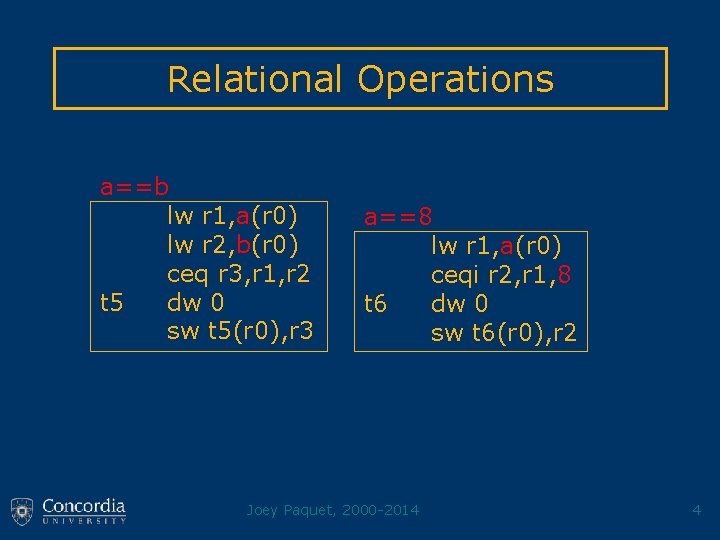
Relational Operations a==b lw r 1, a(r 0) lw r 2, b(r 0) ceq r 3, r 1, r 2 t 5 dw 0 sw t 5(r 0), r 3 a==8 lw r 1, a(r 0) ceqi r 2, r 1, 8 t 6 dw 0 sw t 6(r 0), r 2 Joey Paquet, 2000 -2014 4
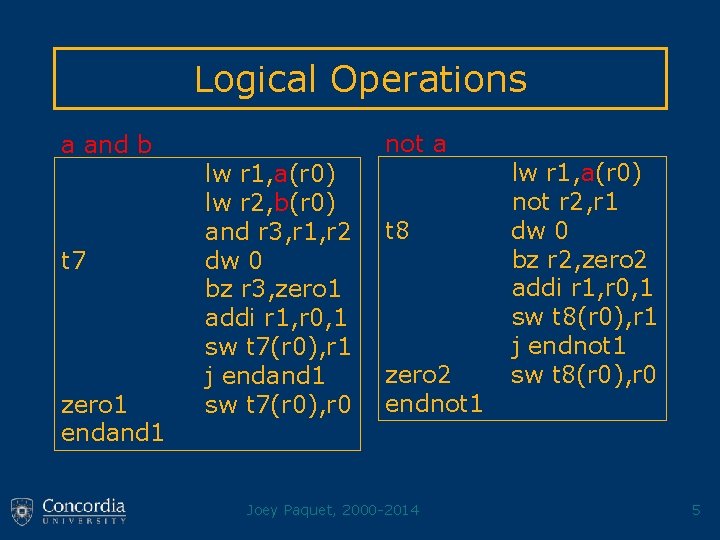
Logical Operations a and b t 7 zero 1 endand 1 not a lw r 1, a(r 0) lw r 2, b(r 0) and r 3, r 1, r 2 dw 0 bz r 3, zero 1 addi r 1, r 0, 1 sw t 7(r 0), r 1 j endand 1 sw t 7(r 0), r 0 t 8 zero 2 endnot 1 Joey Paquet, 2000 -2014 lw r 1, a(r 0) not r 2, r 1 dw 0 bz r 2, zero 2 addi r 1, r 0, 1 sw t 8(r 0), r 1 j endnot 1 sw t 8(r 0), r 0 5
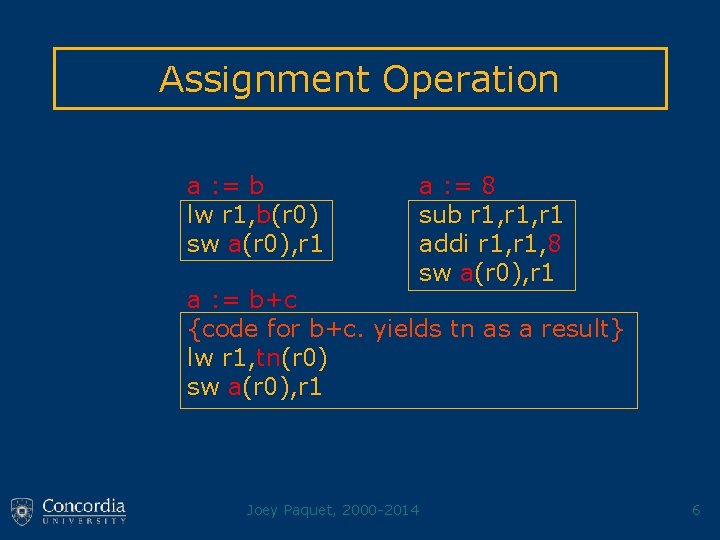
Assignment Operation a : = b lw r 1, b(r 0) sw a(r 0), r 1 a : = 8 sub r 1, r 1 addi r 1, 8 sw a(r 0), r 1 a : = b+c {code for b+c. yields tn as a result} lw r 1, tn(r 0) sw a(r 0), r 1 Joey Paquet, 2000 -2014 6
![Conditional Statements if ab then a b else a 0 1 2 3 4 Conditional Statements if a>b then a: =b else a: =0; [1] [2] [3] [4]](https://slidetodoc.com/presentation_image_h2/afa3d3f2521efa48382742ca2971b946/image-7.jpg)
Conditional Statements if a>b then a: =b else a: =0; [1] [2] [3] [4] [5] [6] else 1 [4] endif 1[6] {code for "a>b". yeilds tn as a result} lw r 1, tn(r 0) bz r 1, else 1 {code for "a: =b”} j endif 1 {code for "a: =0”} {code continuation} Joey Paquet, 2000 -2014 [1] [2] [3] [4] [5] 7
![Loop Statements while ab do a a1 1 2 3 4 5 gowhile 1 Loop Statements while a<b do a: =a+1; [1] [2] [3] [4] [5] gowhile 1](https://slidetodoc.com/presentation_image_h2/afa3d3f2521efa48382742ca2971b946/image-8.jpg)
Loop Statements while a<b do a: =a+1; [1] [2] [3] [4] [5] gowhile 1 [1] {code for "a<b". yeilds tn as a result} lw r 1, tn(r 0) bz r 1, endwhile 1 {code for statblock (a: =a+1)} j gowhile 1 endwhile 1[5] {code continuation} Joey Paquet, 2000 -2014 [2] [3] [4] [5] 8
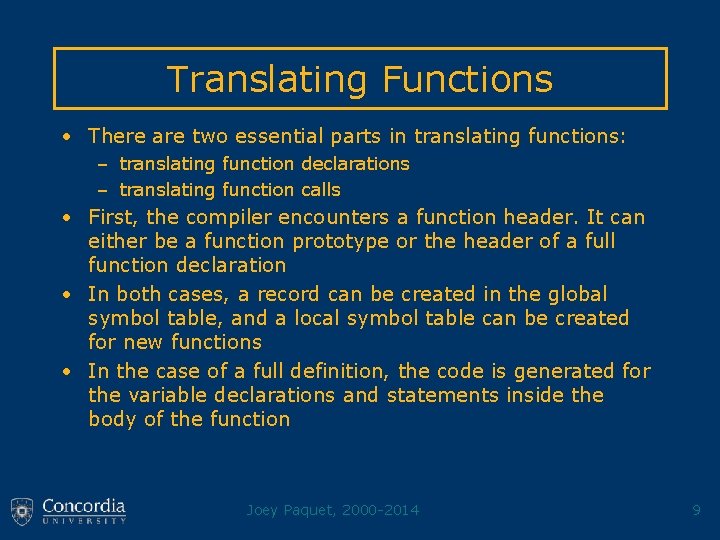
Translating Functions • There are two essential parts in translating functions: – translating function declarations – translating function calls • First, the compiler encounters a function header. It can either be a function prototype or the header of a full function declaration • In both cases, a record can be created in the global symbol table, and a local symbol table can be created for new functions • In the case of a full definition, the code is generated for the variable declarations and statements inside the body of the function Joey Paquet, 2000 -2014 9
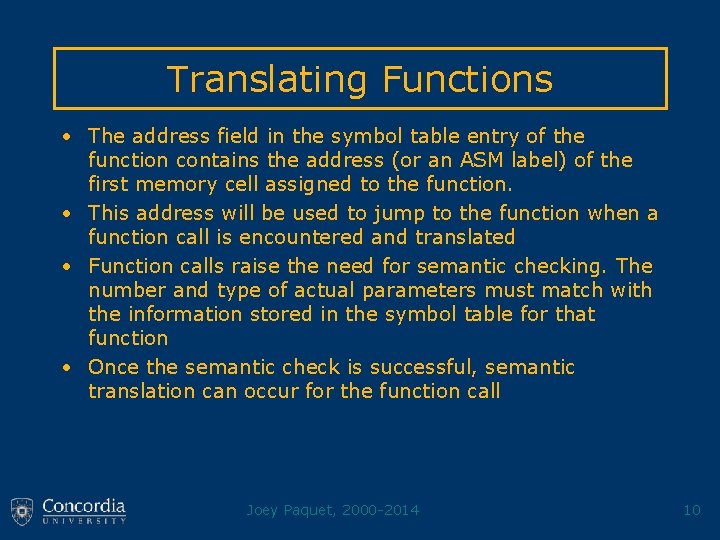
Translating Functions • The address field in the symbol table entry of the function contains the address (or an ASM label) of the first memory cell assigned to the function. • This address will be used to jump to the function when a function call is encountered and translated • Function calls raise the need for semantic checking. The number and type of actual parameters must match with the information stored in the symbol table for that function • Once the semantic check is successful, semantic translation can occur for the function call Joey Paquet, 2000 -2014 10
![Function Declarations int fn int a int b statlist 1 2 Function Declarations int fn ( int a, int b ){ statlist }; [1] [2]](https://slidetodoc.com/presentation_image_h2/afa3d3f2521efa48382742ca2971b946/image-11.jpg)
Function Declarations int fn ( int a, int b ){ statlist }; [1] [2] [3] [4] [5] fn fnp 1 fnp 2 dw 0 [1] dw 0 [2] sw fnp 1(r 0), r 2 [2] dw 0 [3] sw fnp 2(r 0), r 3 [3] {code for local var. decl. & statement list} [4] lw r 2, fnp 1(r 0) [5] lw r 3, fnp 2(r 0) [5] jr r 15 [5] Joey Paquet, 2000 -2014 11
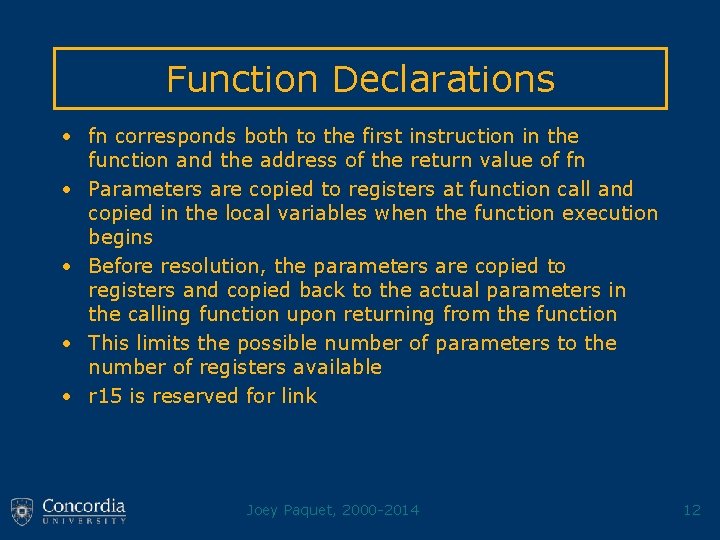
Function Declarations • fn corresponds both to the first instruction in the function and the address of the return value of fn • Parameters are copied to registers at function call and copied in the local variables when the function execution begins • Before resolution, the parameters are copied to registers and copied back to the actual parameters in the calling function upon returning from the function • This limits the possible number of parameters to the number of registers available • r 15 is reserved for link Joey Paquet, 2000 -2014 12
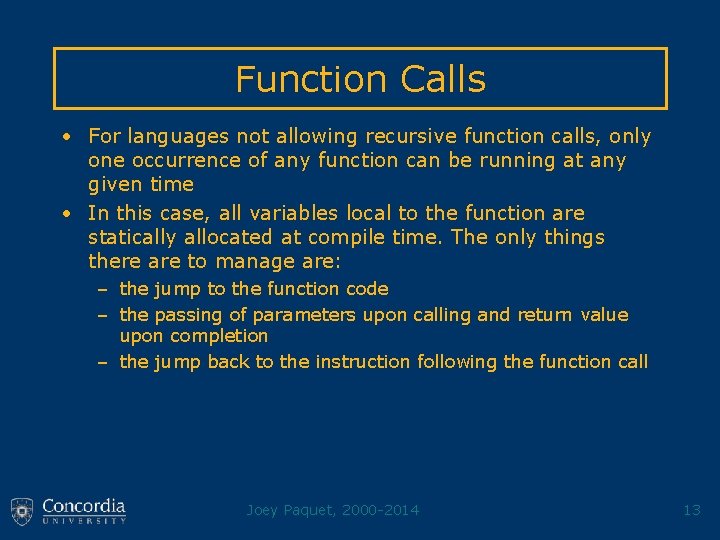
Function Calls • For languages not allowing recursive function calls, only one occurrence of any function can be running at any given time • In this case, all variables local to the function are statically allocated at compile time. The only things there are to manage are: – the jump to the function code – the passing of parameters upon calling and return value upon completion – the jump back to the instruction following the function call Joey Paquet, 2000 -2014 13
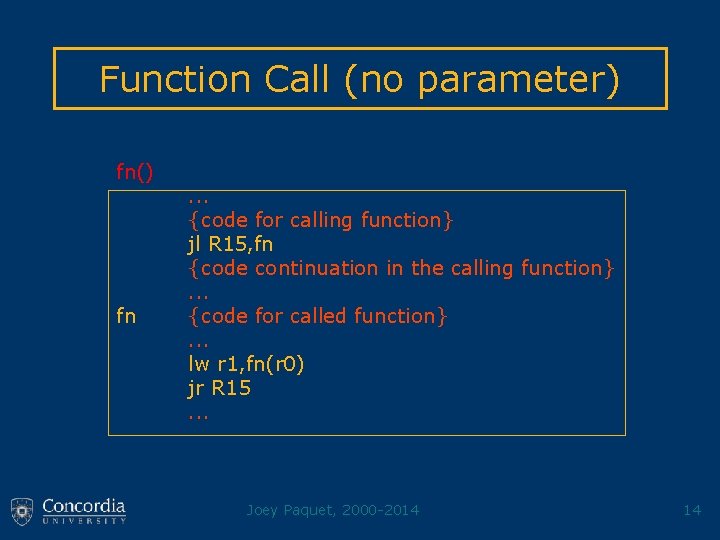
Function Call (no parameter) fn() fn . . . {code for calling function} jl R 15, fn {code continuation in the calling function}. . . {code for called function}. . . lw r 1, fn(r 0) jr R 15. . . Joey Paquet, 2000 -2014 14
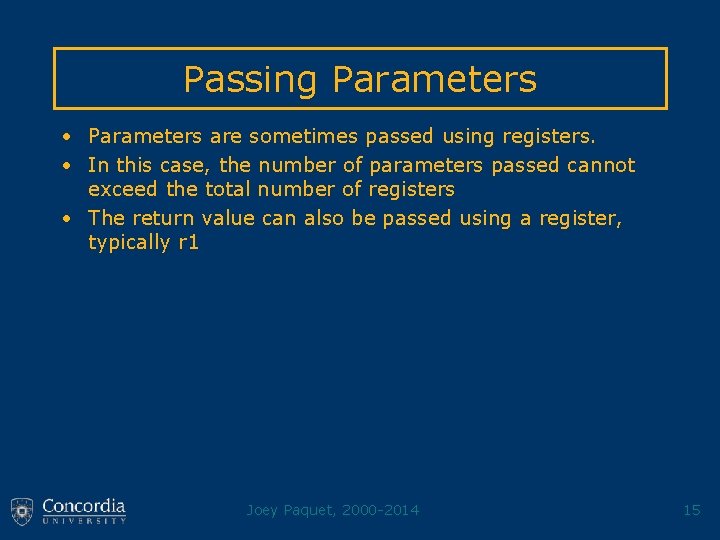
Passing Parameters • Parameters are sometimes passed using registers. • In this case, the number of parameters passed cannot exceed the total number of registers • The return value can also be passed using a register, typically r 1 Joey Paquet, 2000 -2014 15
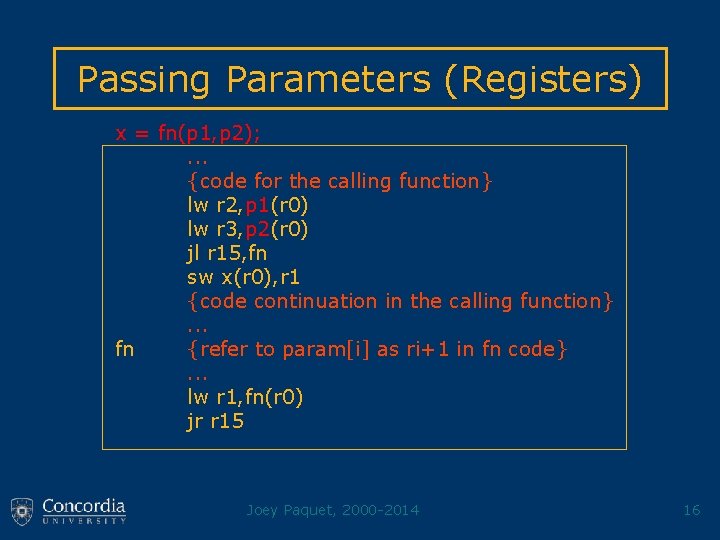
Passing Parameters (Registers) x = fn(p 1, p 2); . . . {code for the calling function} lw r 2, p 1(r 0) lw r 3, p 2(r 0) jl r 15, fn sw x(r 0), r 1 {code continuation in the calling function}. . . fn {refer to param[i] as ri+1 in fn code}. . . lw r 1, fn(r 0) jr r 15 Joey Paquet, 2000 -2014 16
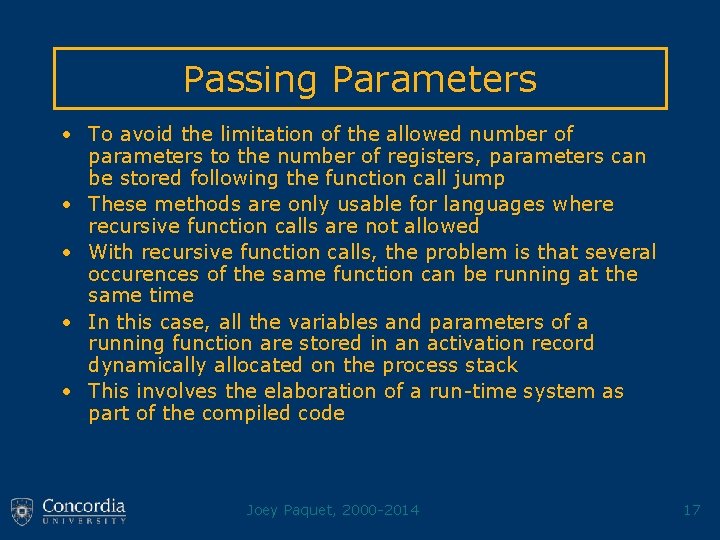
Passing Parameters • To avoid the limitation of the allowed number of parameters to the number of registers, parameters can be stored following the function call jump • These methods are only usable for languages where recursive function calls are not allowed • With recursive function calls, the problem is that several occurences of the same function can be running at the same time • In this case, all the variables and parameters of a running function are stored in an activation record dynamically allocated on the process stack • This involves the elaboration of a run-time system as part of the compiled code Joey Paquet, 2000 -2014 17
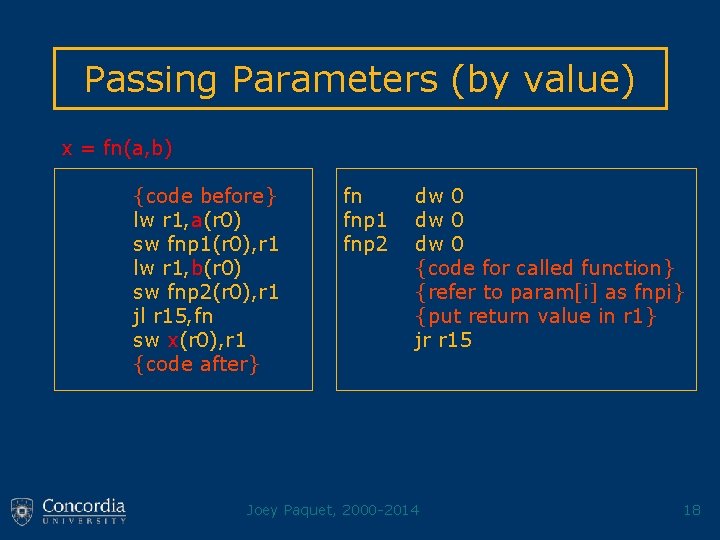
Passing Parameters (by value) x = fn(a, b) {code before} lw r 1, a(r 0) sw fnp 1(r 0), r 1 lw r 1, b(r 0) sw fnp 2(r 0), r 1 jl r 15, fn sw x(r 0), r 1 {code after} fn fnp 1 fnp 2 dw 0 {code for called function} {refer to param[i] as fnpi} {put return value in r 1} jr r 15 Joey Paquet, 2000 -2014 18
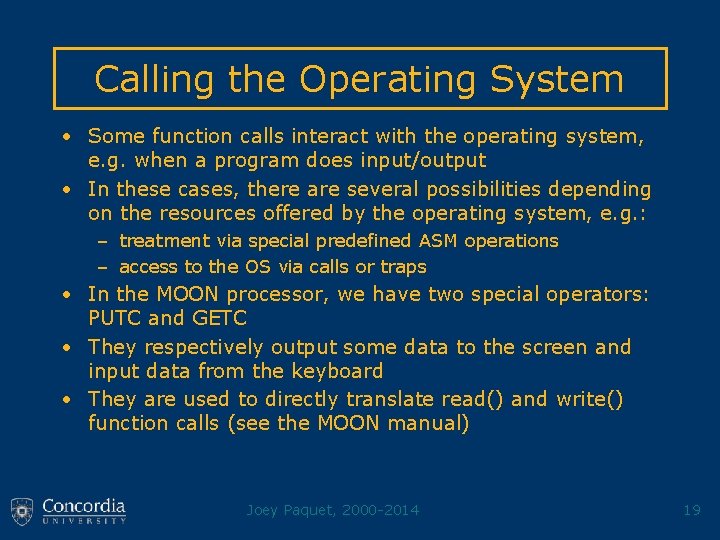
Calling the Operating System • Some function calls interact with the operating system, e. g. when a program does input/output • In these cases, there are several possibilities depending on the resources offered by the operating system, e. g. : – treatment via special predefined ASM operations – access to the OS via calls or traps • In the MOON processor, we have two special operators: PUTC and GETC • They respectively output some data to the screen and input data from the keyboard • They are used to directly translate read() and write() function calls (see the MOON manual) Joey Paquet, 2000 -2014 19
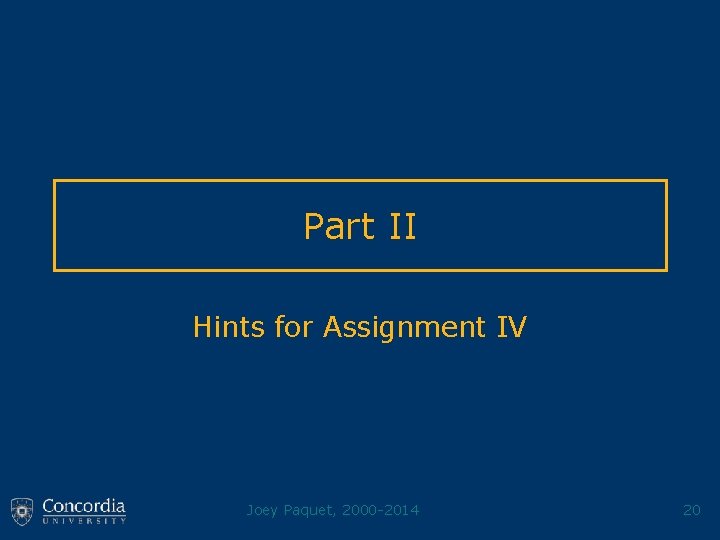
Part II Hints for Assignment IV Joey Paquet, 2000 -2014 20
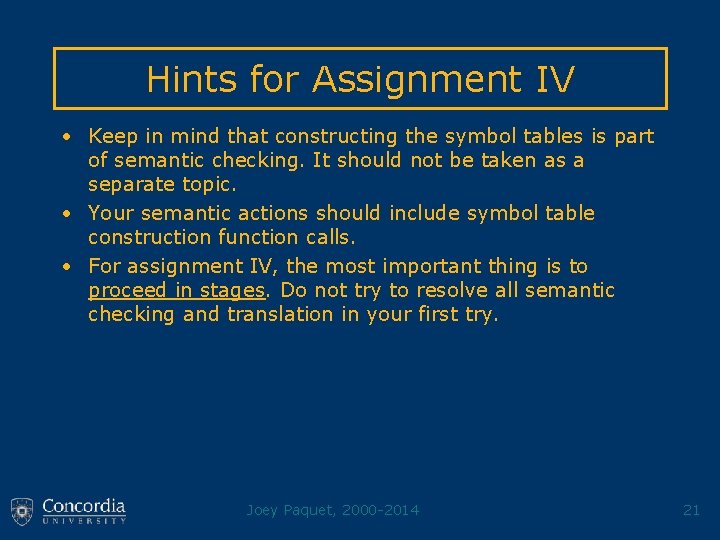
Hints for Assignment IV • Keep in mind that constructing the symbol tables is part of semantic checking. It should not be taken as a separate topic. • Your semantic actions should include symbol table construction function calls. • For assignment IV, the most important thing is to proceed in stages. Do not try to resolve all semantic checking and translation in your first try. Joey Paquet, 2000 -2014 21
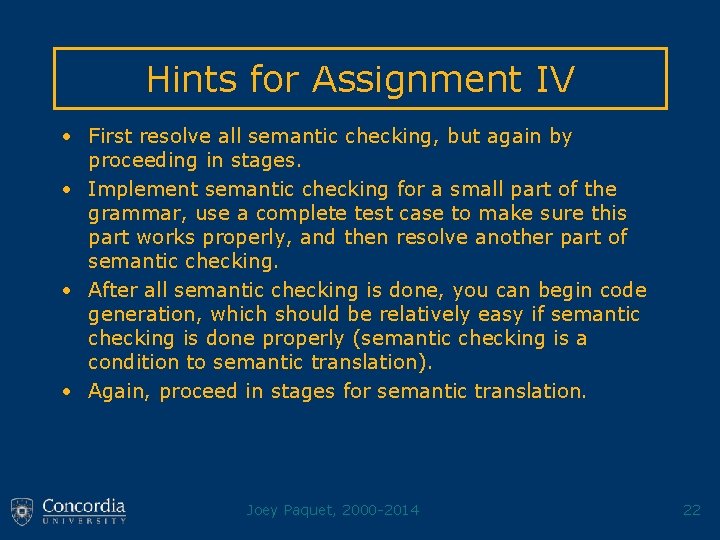
Hints for Assignment IV • First resolve all semantic checking, but again by proceeding in stages. • Implement semantic checking for a small part of the grammar, use a complete test case to make sure this part works properly, and then resolve another part of semantic checking. • After all semantic checking is done, you can begin code generation, which should be relatively easy if semantic checking is done properly (semantic checking is a condition to semantic translation). • Again, proceed in stages for semantic translation. Joey Paquet, 2000 -2014 22
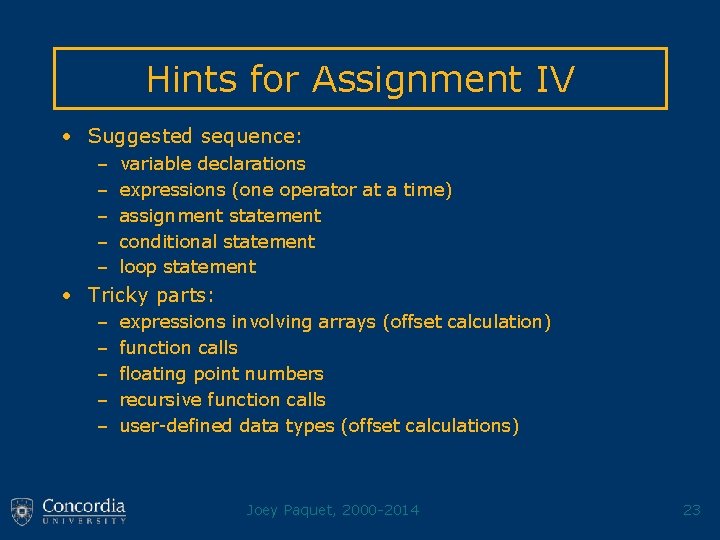
Hints for Assignment IV • Suggested sequence: – – – variable declarations expressions (one operator at a time) assignment statement conditional statement loop statement • Tricky parts: – – – expressions involving arrays (offset calculation) function calls floating point numbers recursive function calls user-defined data types (offset calculations) Joey Paquet, 2000 -2014 23
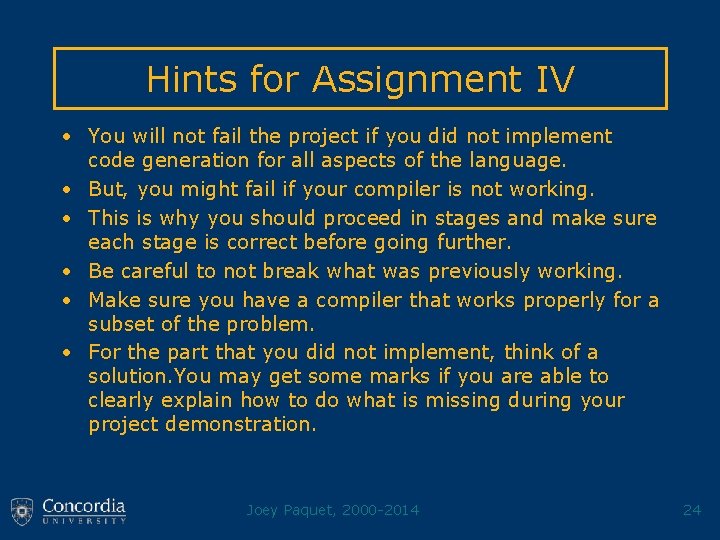
Hints for Assignment IV • You will not fail the project if you did not implement code generation for all aspects of the language. • But, you might fail if your compiler is not working. • This is why you should proceed in stages and make sure each stage is correct before going further. • Be careful to not break what was previously working. • Make sure you have a compiler that works properly for a subset of the problem. • For the part that you did not implement, think of a solution. You may get some marks if you are able to clearly explain how to do what is missing during your project demonstration. Joey Paquet, 2000 -2014 24
Joey paquet
Naomi rachel king
Joey paquet
Relevé de température haccp
Clasificacion de varices
John paquet
Vrices
Paquet tva
Parois de la fosse axillaire
Connexion au serveur dynamic link en cours
Second generation vs first generation antipsychotics
You're good and your mercy is forever
01:640:244 lecture notes - lecture 15: plat, idah, farad
Code commit code build code deploy
Joey azofeifa
Characters in tangerine by edward bloor
Joey movers
Joey salloway
Joey siu
Ermington scouts
Natalie rae miller
Joey stiles
Graphical display
Joey kaye
Joey sylvester