Lecture 4 Syntactic Analysis Part II Joey Paquet
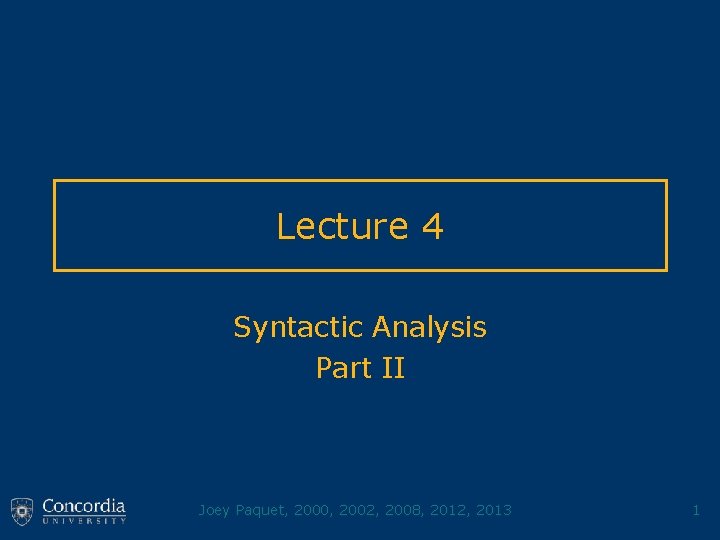
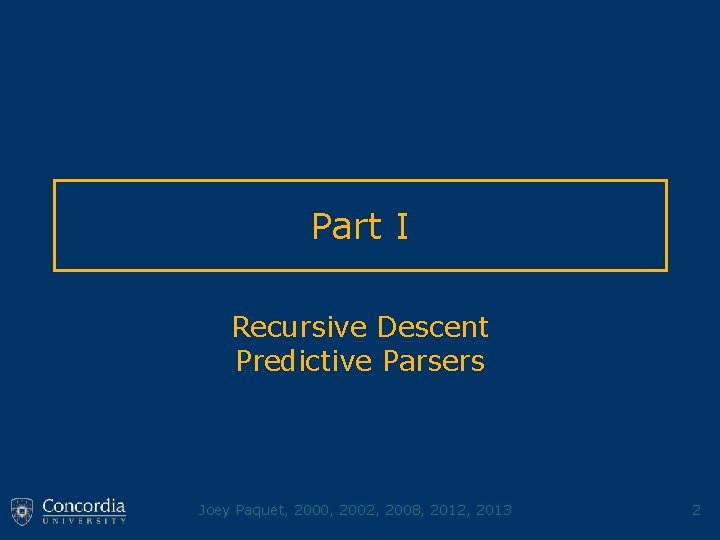
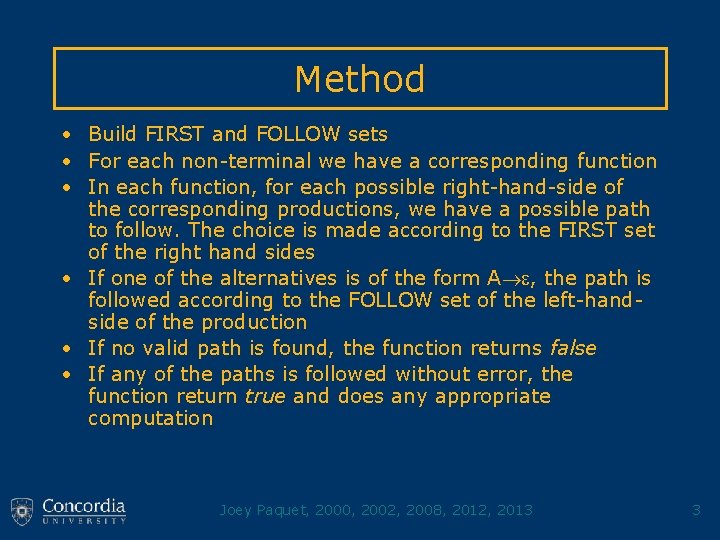
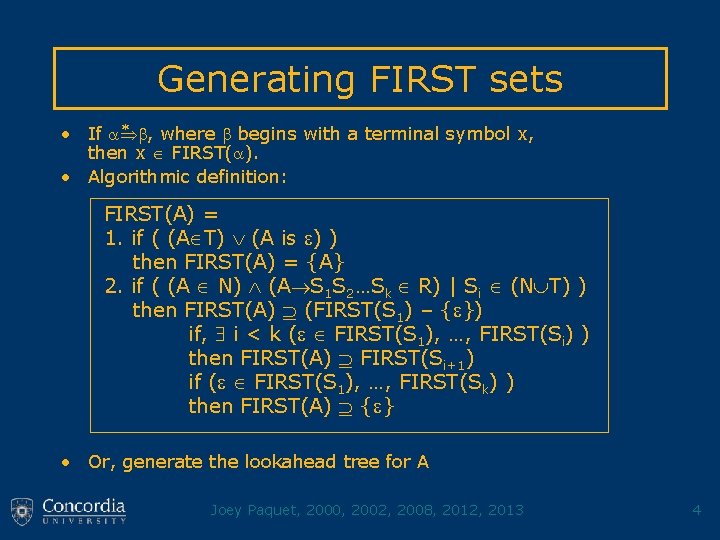
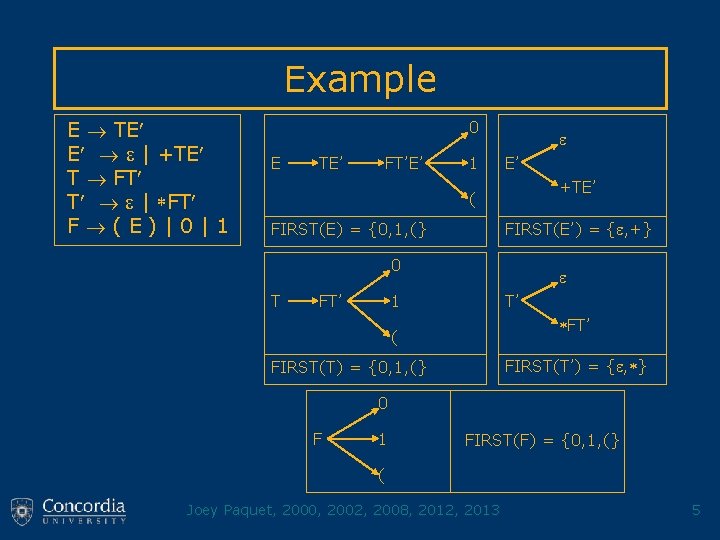
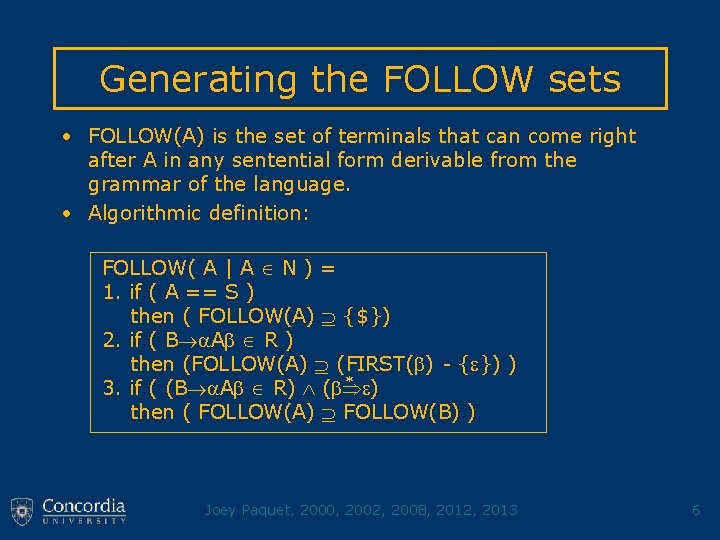
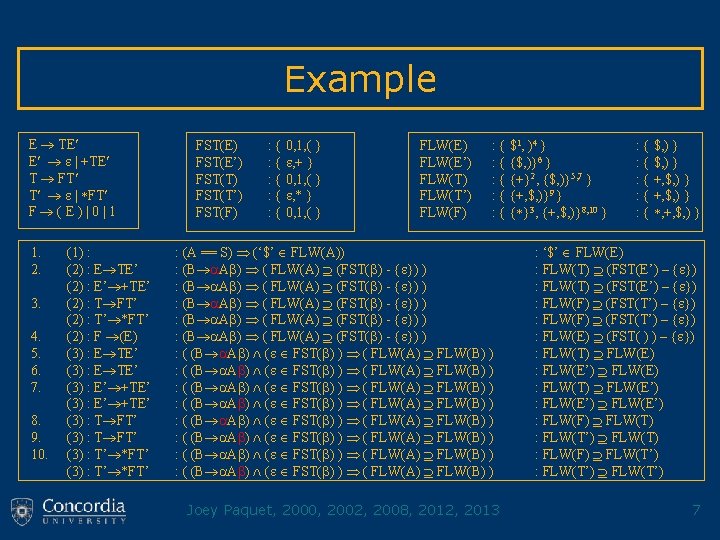
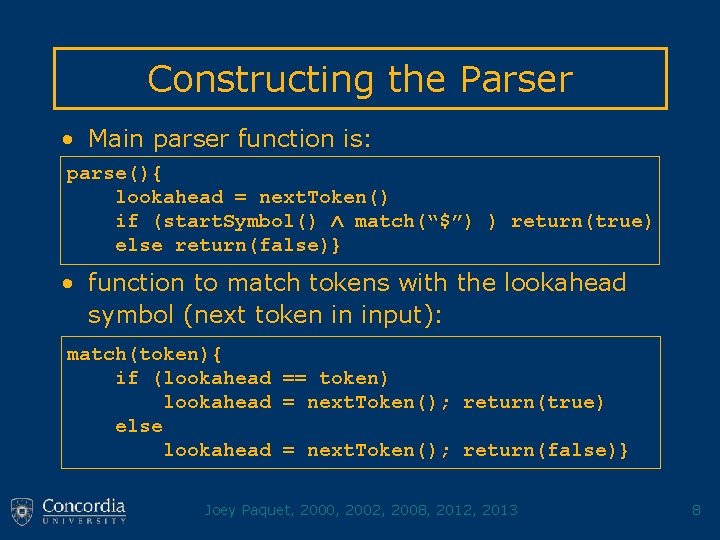
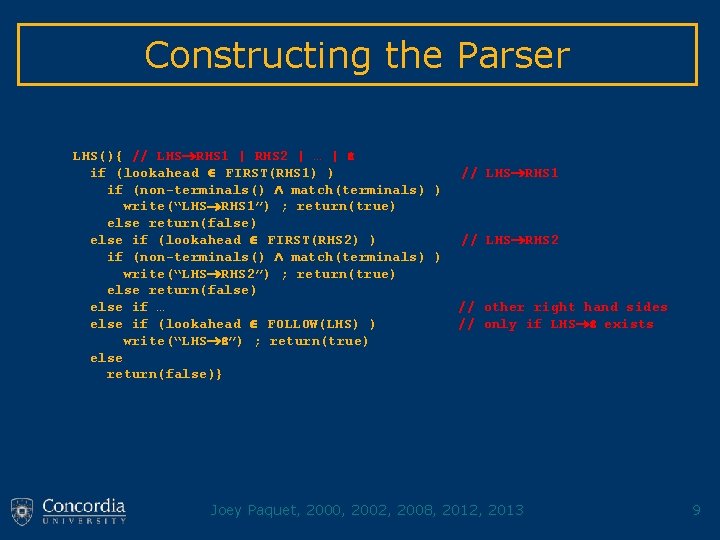
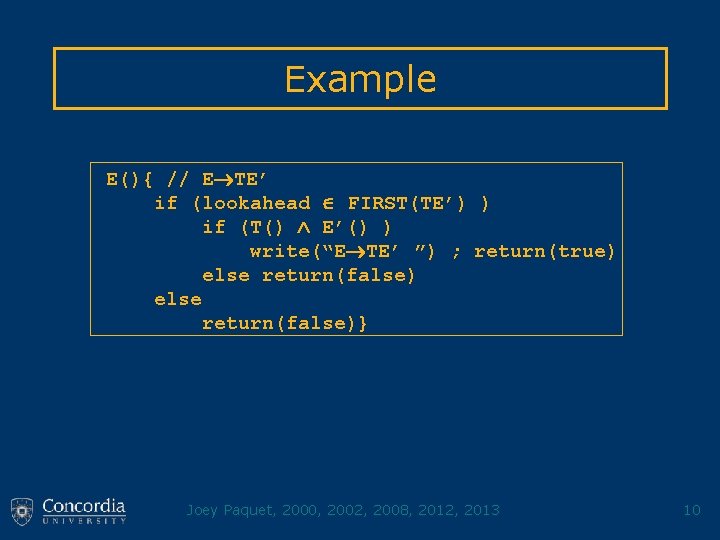
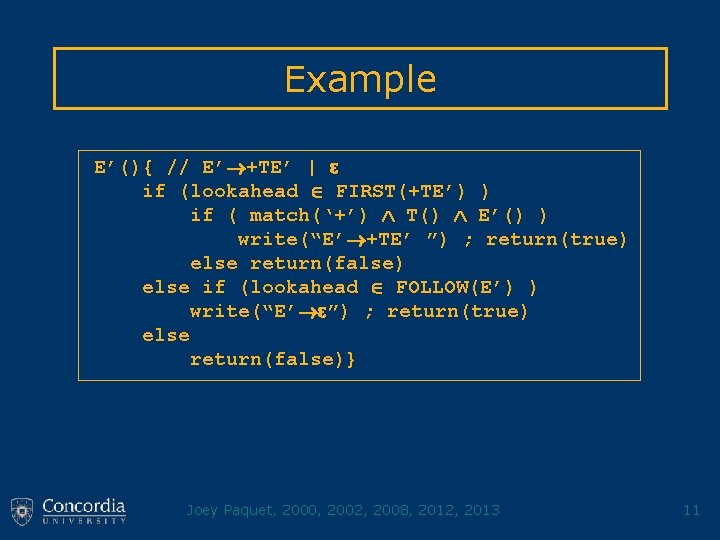
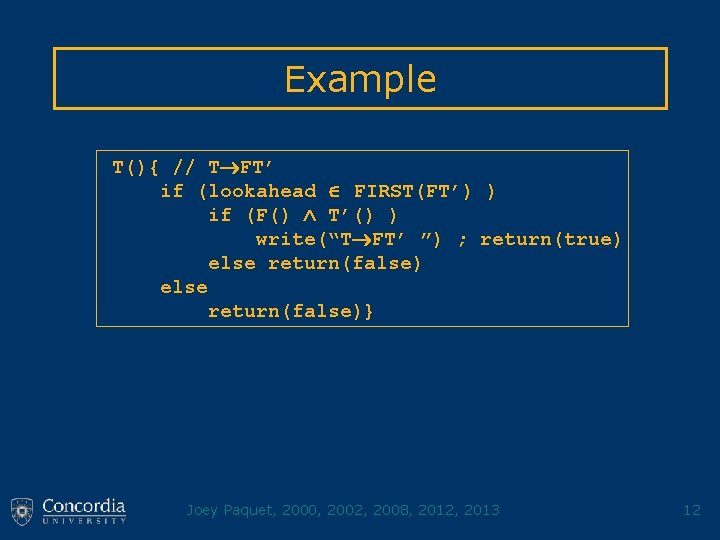
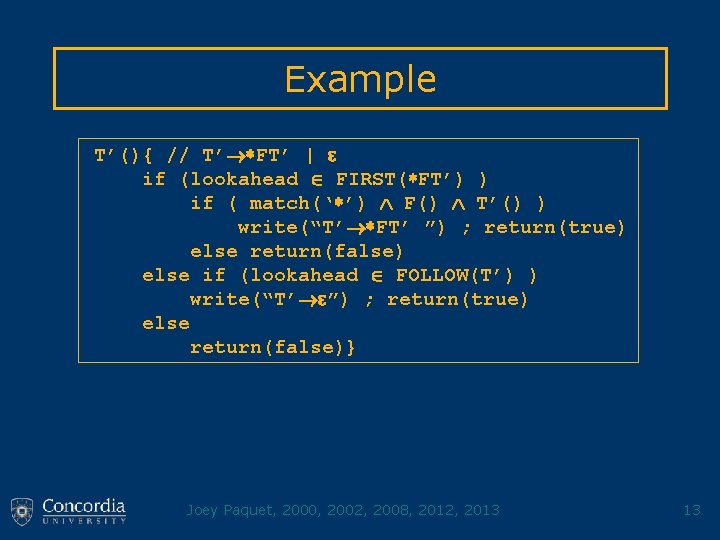
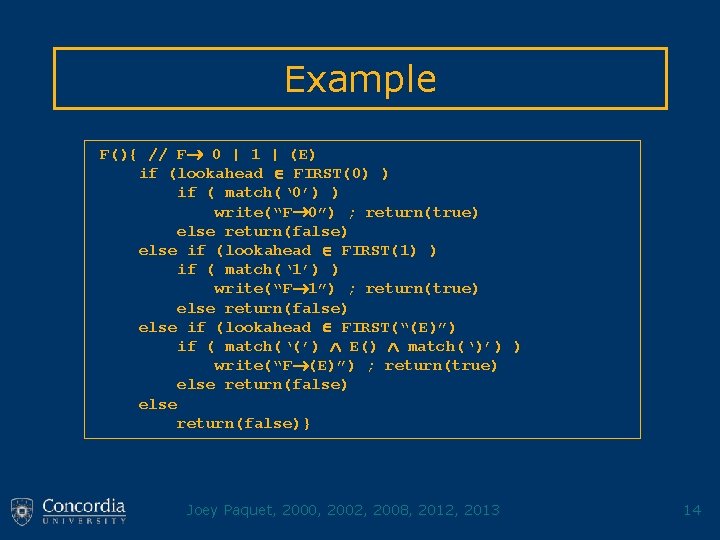
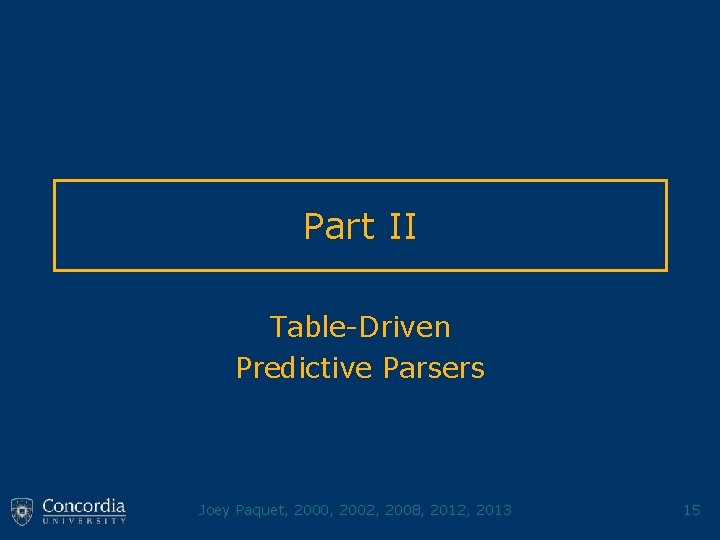
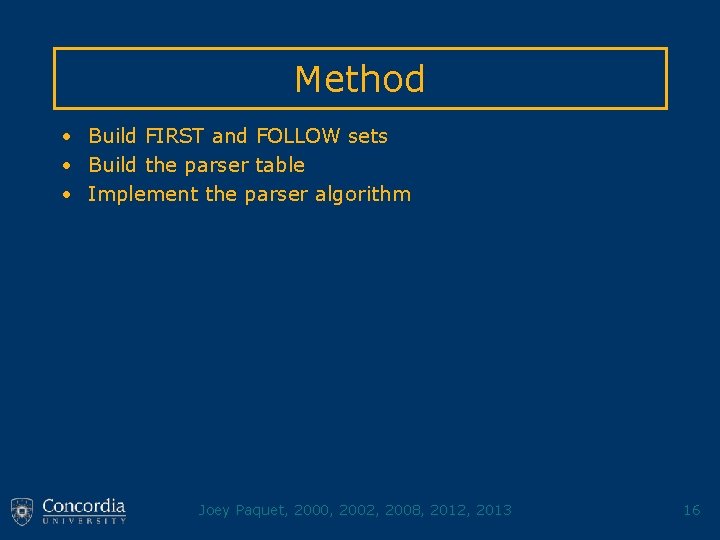
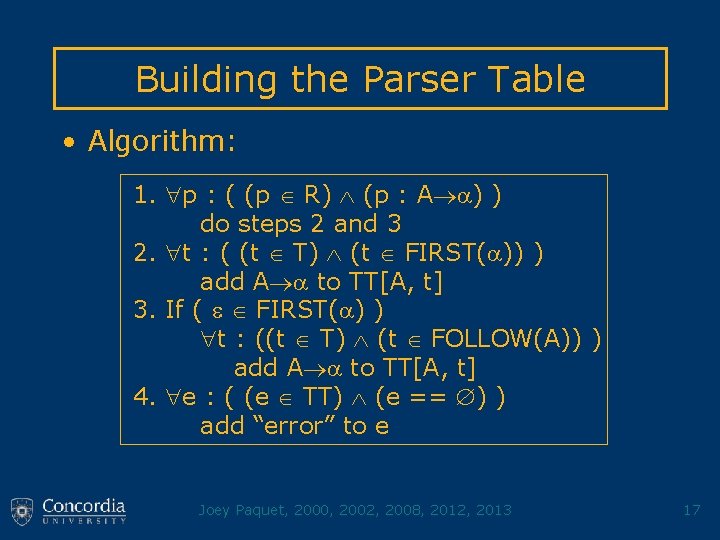
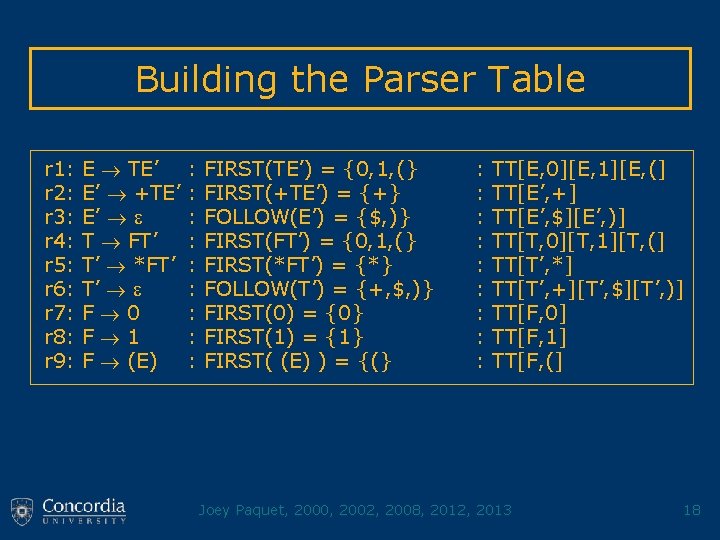
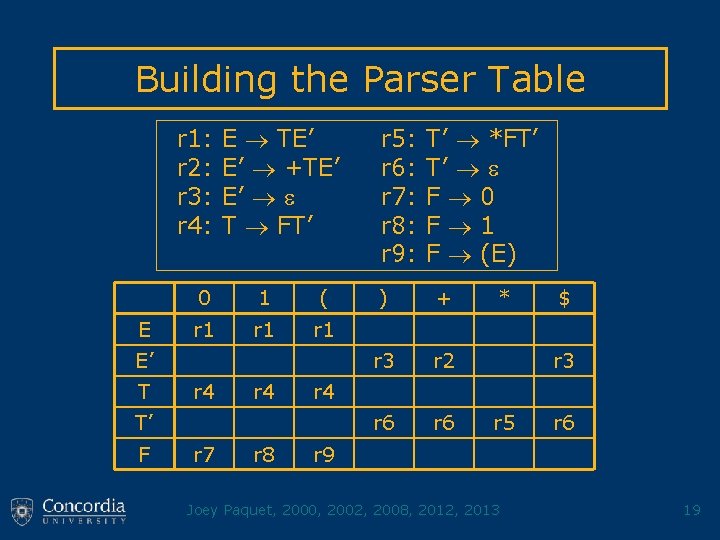
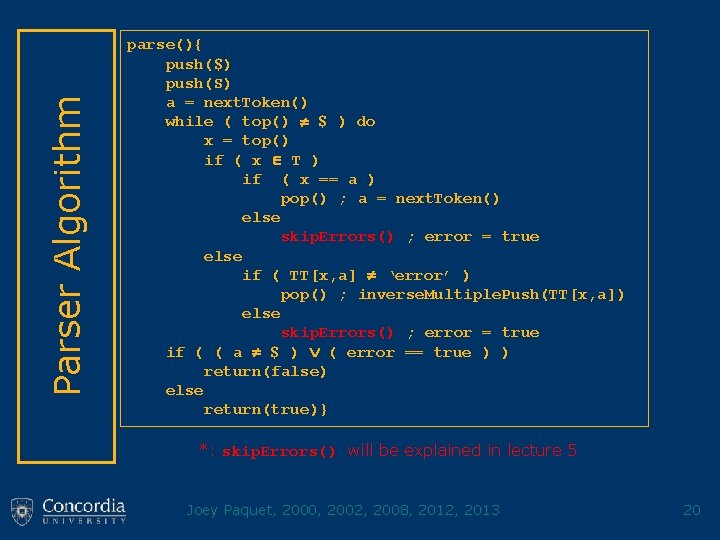
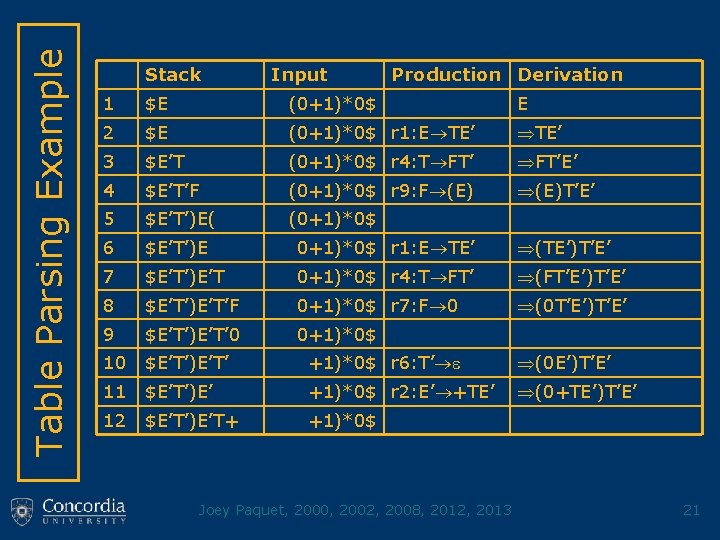
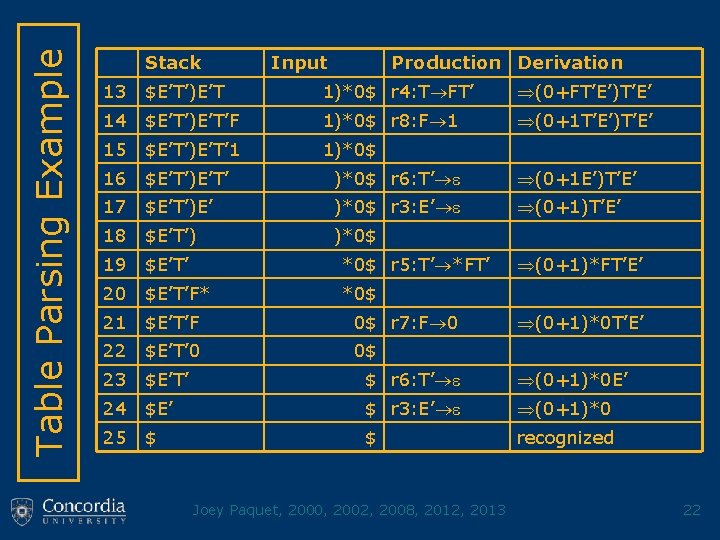
- Slides: 22
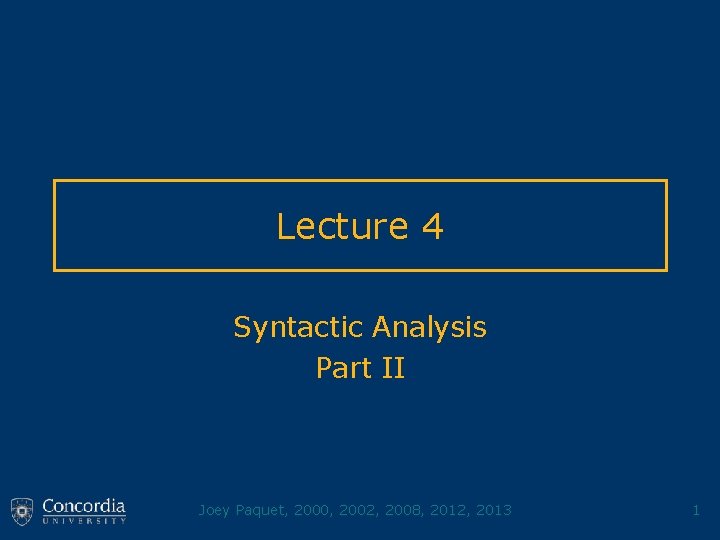
Lecture 4 Syntactic Analysis Part II Joey Paquet, 2000, 2002, 2008, 2012, 2013 1
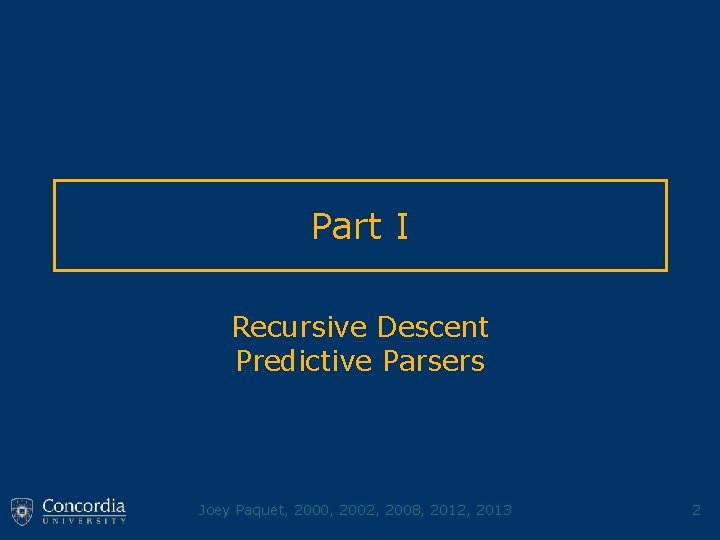
Part I Recursive Descent Predictive Parsers Joey Paquet, 2000, 2002, 2008, 2012, 2013 2
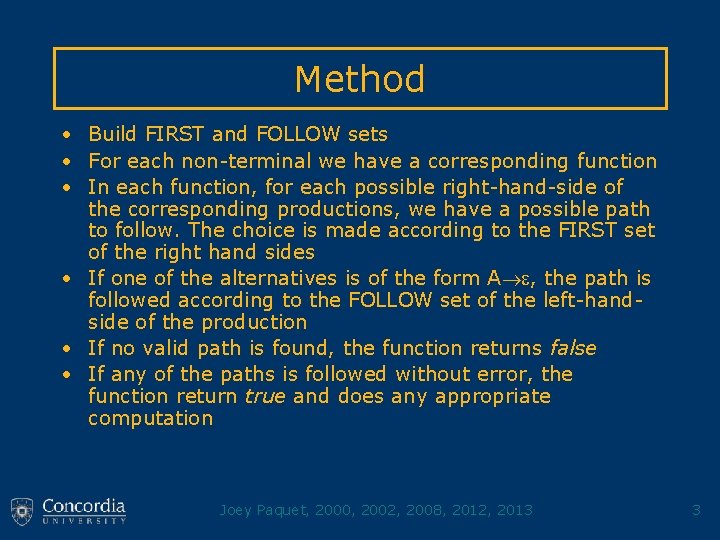
Method • Build FIRST and FOLLOW sets • For each non-terminal we have a corresponding function • In each function, for each possible right-hand-side of the corresponding productions, we have a possible path to follow. The choice is made according to the FIRST set of the right hand sides • If one of the alternatives is of the form A , the path is followed according to the FOLLOW set of the left-handside of the production • If no valid path is found, the function returns false • If any of the paths is followed without error, the function return true and does any appropriate computation Joey Paquet, 2000, 2002, 2008, 2012, 2013 3
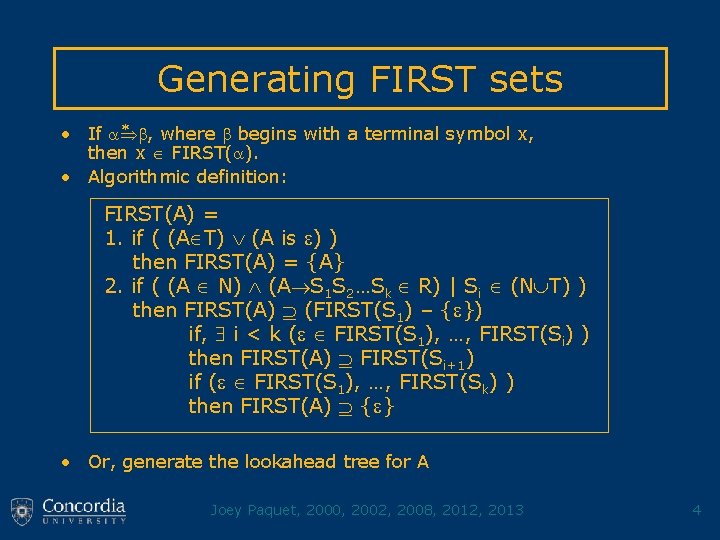
Generating FIRST sets • If , where begins with a terminal symbol x, then x FIRST( ). • Algorithmic definition: FIRST(A) = 1. if ( (A T) (A is ) ) then FIRST(A) = {A} 2. if ( (A N) (A S 1 S 2…Sk R) | Si (N T) ) then FIRST(A) (FIRST(S 1) – { }) if, i < k ( FIRST(S 1), …, FIRST(Si) ) then FIRST(A) FIRST(Si+1) if ( FIRST(S 1), …, FIRST(Sk) ) then FIRST(A) { } • Or, generate the lookahead tree for A Joey Paquet, 2000, 2002, 2008, 2012, 2013 4
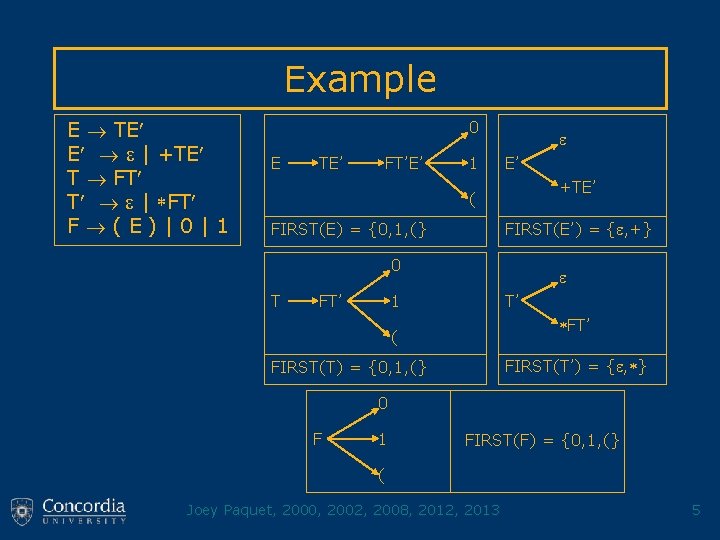
Example E TE E | +TE T FT T | FT F (E)|0|1 0 E TE’ FT’E’ 1 E’ +TE’ ( FIRST(E’) = { , +} FIRST(E) = {0, 1, (} 0 T FT’ T’ 1 FT’ ( FIRST(T’) = { , } FIRST(T) = {0, 1, (} 0 F 1 FIRST(F) = {0, 1, (} ( Joey Paquet, 2000, 2002, 2008, 2012, 2013 5
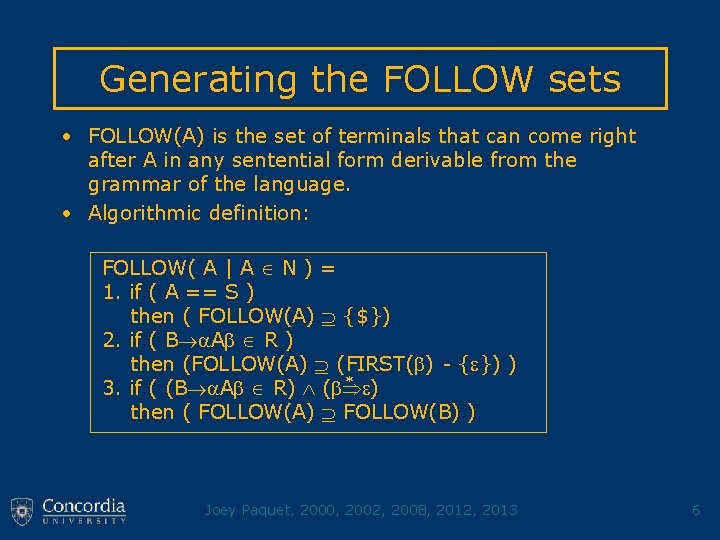
Generating the FOLLOW sets • FOLLOW(A) is the set of terminals that can come right after A in any sentential form derivable from the grammar of the language. • Algorithmic definition: FOLLOW( A | A N ) = 1. if ( A == S ) then ( FOLLOW(A) {$}) 2. if ( B A R ) then (FOLLOW(A) (FIRST( ) - { }) ) 3. if ( (B A R) ( ) then ( FOLLOW(A) FOLLOW(B) ) Joey Paquet, 2000, 2002, 2008, 2012, 2013 6
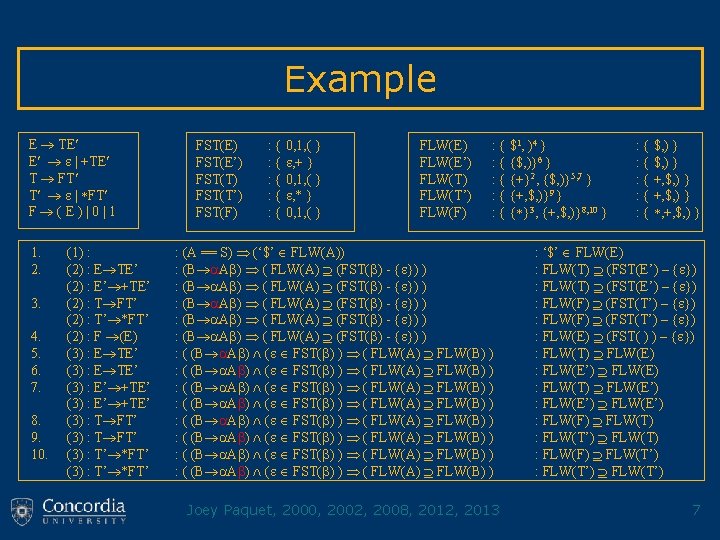
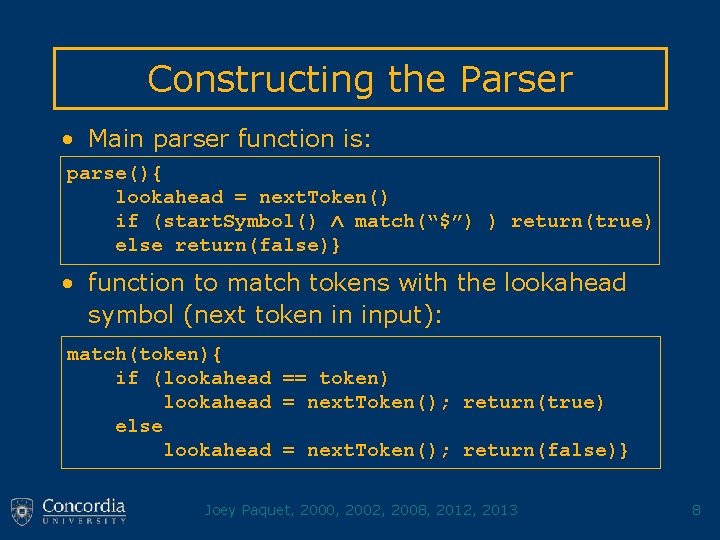
Constructing the Parser • Main parser function is: parse(){ lookahead = next. Token() if (start. Symbol() match(“$”) ) return(true) else return(false)} • function to match tokens with the lookahead symbol (next token in input): match(token){ if (lookahead == token) lookahead = next. Token(); return(true) else lookahead = next. Token(); return(false)} Joey Paquet, 2000, 2002, 2008, 2012, 2013 8
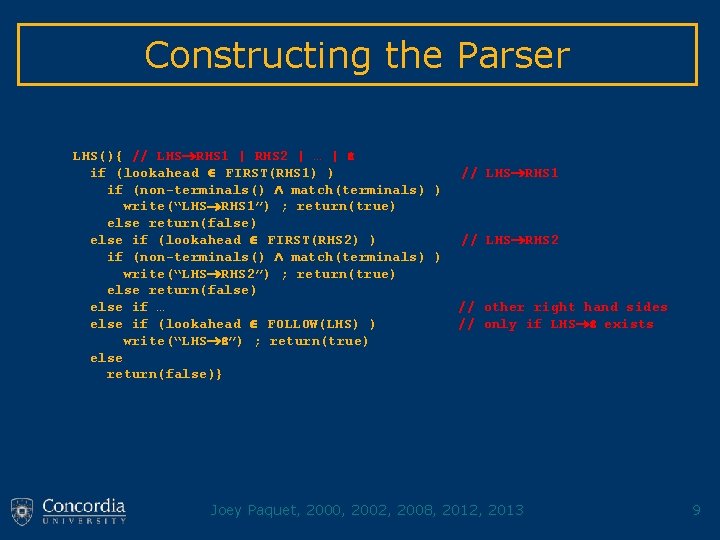
Constructing the Parser LHS(){ // LHS RHS 1 | RHS 2 | … | if (lookahead FIRST(RHS 1) ) if (non-terminals() match(terminals) ) write(“LHS RHS 1”) ; return(true) else return(false) else if (lookahead FIRST(RHS 2) ) if (non-terminals() match(terminals) ) write(“LHS RHS 2”) ; return(true) else return(false) else if … else if (lookahead FOLLOW(LHS) ) write(“LHS ”) ; return(true) else return(false)} // LHS RHS 1 // LHS RHS 2 // other right hand sides // only if LHS exists Joey Paquet, 2000, 2002, 2008, 2012, 2013 9
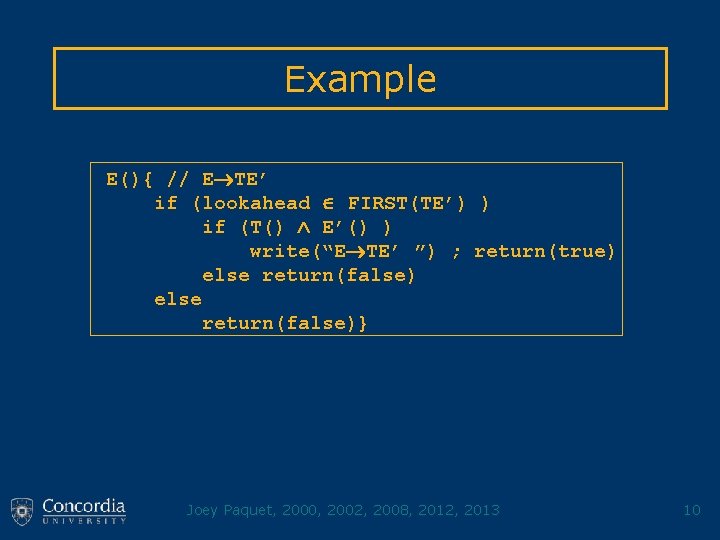
Example E(){ // E TE’ if (lookahead FIRST(TE’) ) if (T() E’() ) write(“E TE’ ”) ; return(true) else return(false)} Joey Paquet, 2000, 2002, 2008, 2012, 2013 10
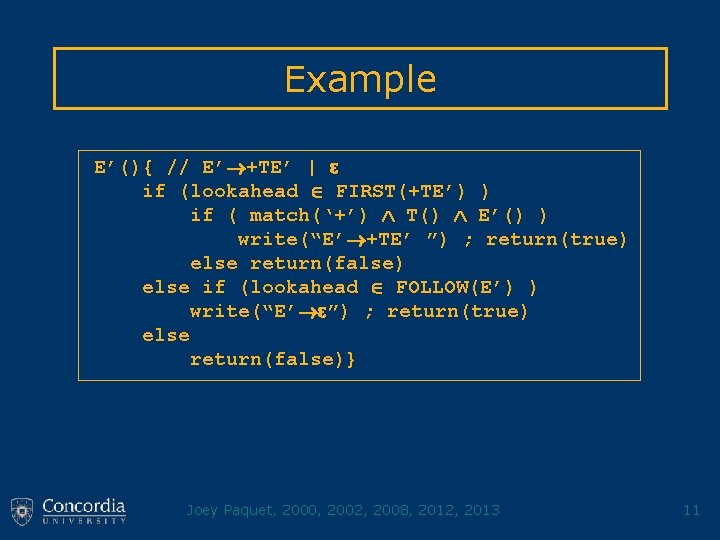
Example E’(){ // E’ +TE’ | if (lookahead FIRST(+TE’) ) if ( match(‘+’) T() E’() ) write(“E’ +TE’ ”) ; return(true) else return(false) else if (lookahead FOLLOW(E’) ) write(“E’ ”) ; return(true) else return(false)} Joey Paquet, 2000, 2002, 2008, 2012, 2013 11
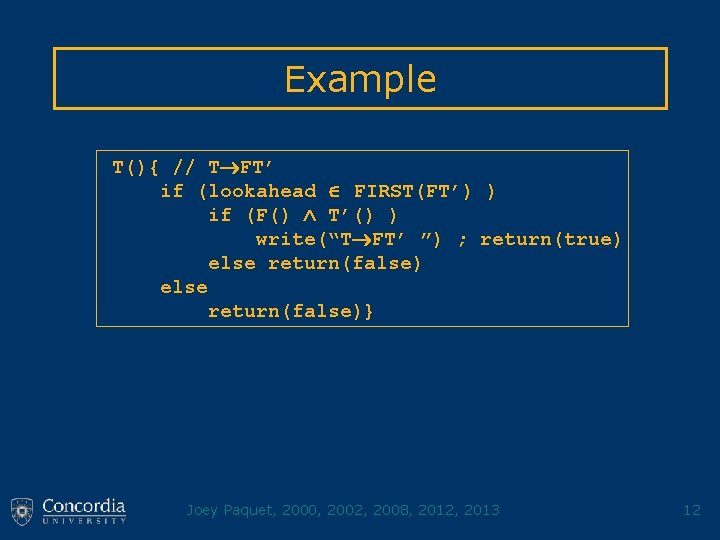
Example T(){ // T FT’ if (lookahead FIRST(FT’) ) if (F() T’() ) write(“T FT’ ”) ; return(true) else return(false)} Joey Paquet, 2000, 2002, 2008, 2012, 2013 12
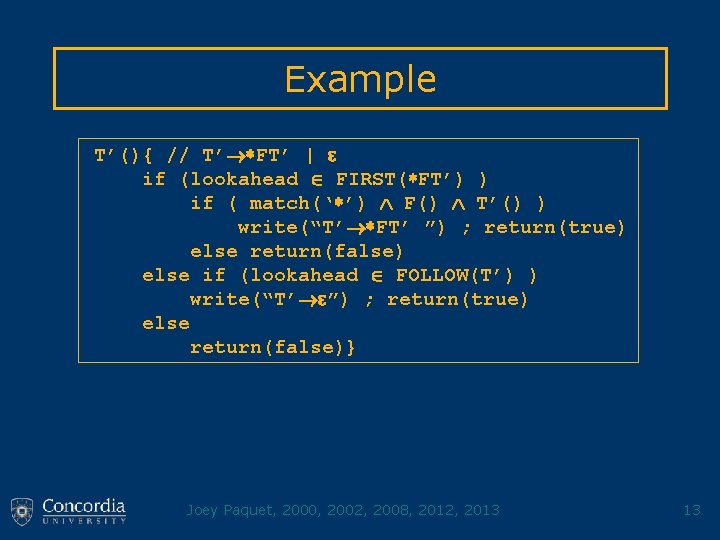
Example T’(){ // T’ FT’ | if (lookahead FIRST( FT’) ) if ( match(‘ ’) F() T’() ) write(“T’ FT’ ”) ; return(true) else return(false) else if (lookahead FOLLOW(T’) ) write(“T’ ”) ; return(true) else return(false)} Joey Paquet, 2000, 2002, 2008, 2012, 2013 13
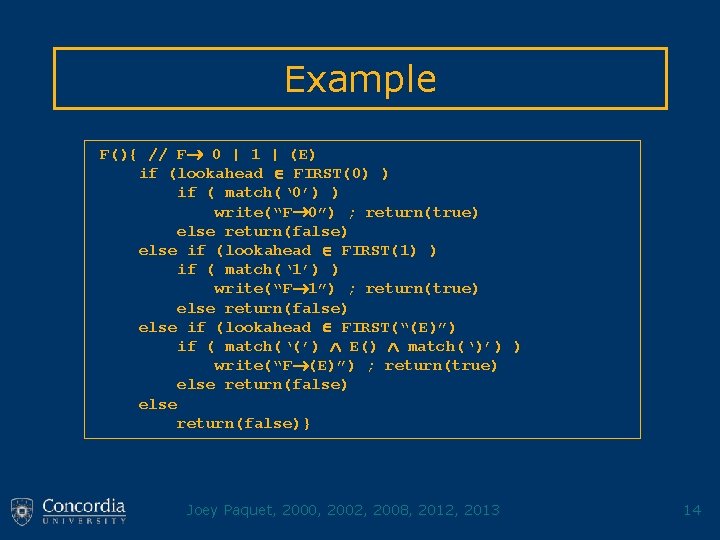
Example F(){ // F 0 | 1 | (E) if (lookahead FIRST(0) ) if ( match(‘ 0’) ) write(“F 0”) ; return(true) else return(false) else if (lookahead FIRST(1) ) if ( match(‘ 1’) ) write(“F 1”) ; return(true) else return(false) else if (lookahead FIRST(“(E)”) if ( match(‘(’) E() match(‘)’) ) write(“F (E)”) ; return(true) else return(false)} Joey Paquet, 2000, 2002, 2008, 2012, 2013 14
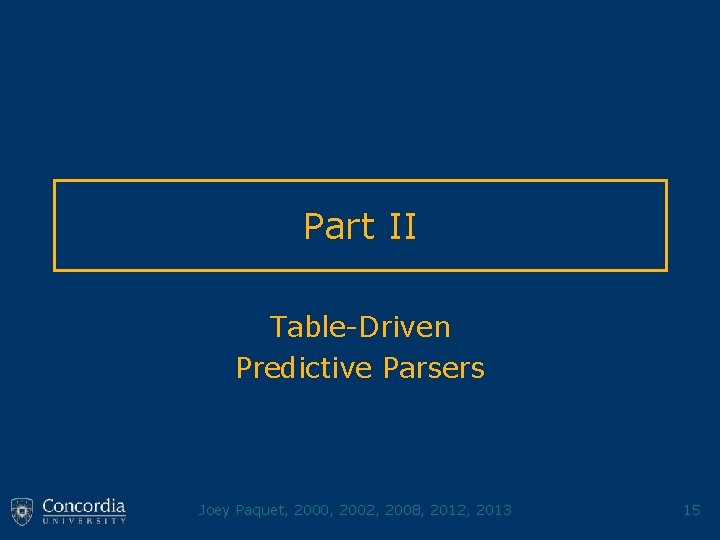
Part II Table-Driven Predictive Parsers Joey Paquet, 2000, 2002, 2008, 2012, 2013 15
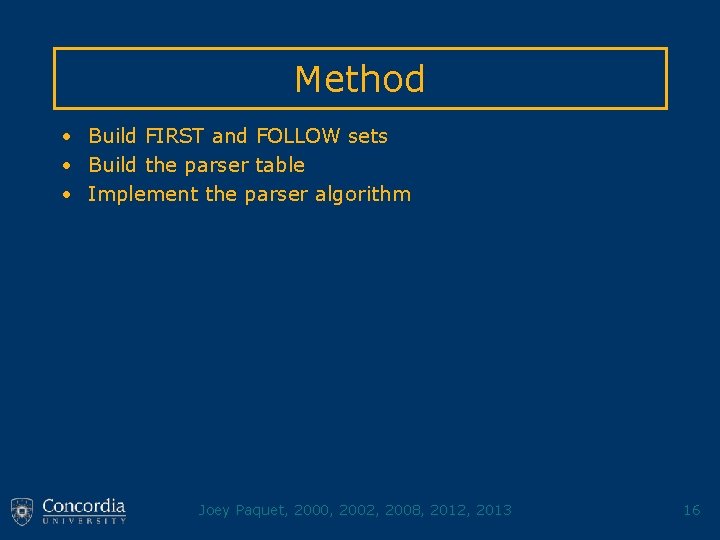
Method • Build FIRST and FOLLOW sets • Build the parser table • Implement the parser algorithm Joey Paquet, 2000, 2002, 2008, 2012, 2013 16
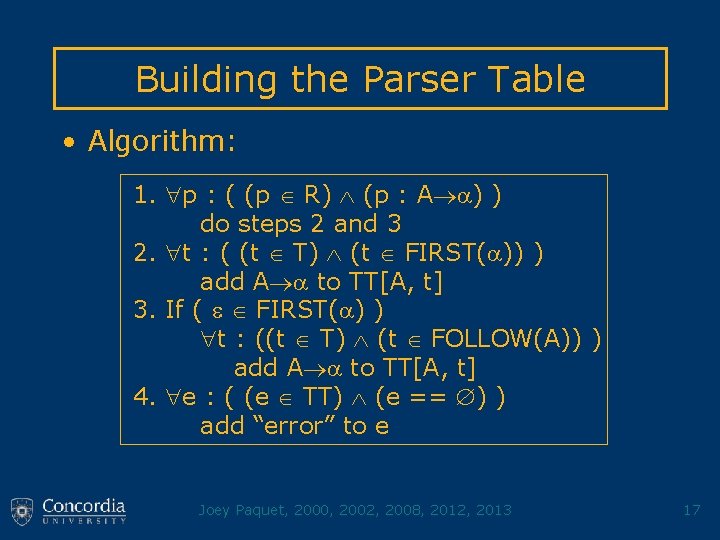
Building the Parser Table • Algorithm: 1. p : ( (p R) (p : A ) ) do steps 2 and 3 2. t : ( (t T) (t FIRST( )) ) add A to TT[A, t] 3. If ( FIRST( ) ) t : ((t T) (t FOLLOW(A)) ) add A to TT[A, t] 4. e : ( (e TT) (e == ) ) add “error” to e Joey Paquet, 2000, 2002, 2008, 2012, 2013 17
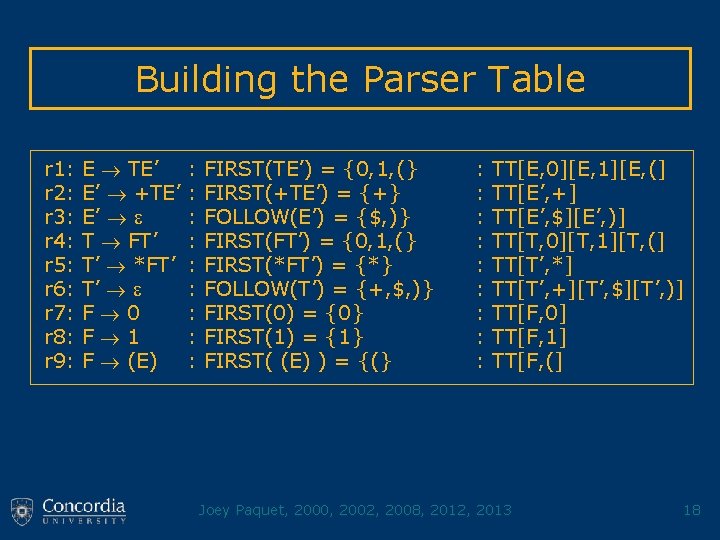
Building the Parser Table r 1: r 2: r 3: r 4: r 5: r 6: r 7: r 8: r 9: E TE’ E’ +TE’ E’ T FT’ T’ *FT’ T’ F 0 F 1 F (E) : : : : : FIRST(TE’) = {0, 1, (} FIRST(+TE’) = {+} FOLLOW(E’) = {$, )} FIRST(FT’) = {0, 1, (} FIRST(*FT’) = {*} FOLLOW(T’) = {+, $, )} FIRST(0) = {0} FIRST(1) = {1} FIRST( (E) ) = {(} : : : : : TT[E, 0][E, 1][E, (] TT[E’, +] TT[E’, $][E’, )] TT[T, 0][T, 1][T, (] TT[T’, *] TT[T’, +][T’, $][T’, )] TT[F, 0] TT[F, 1] TT[F, (] Joey Paquet, 2000, 2002, 2008, 2012, 2013 18
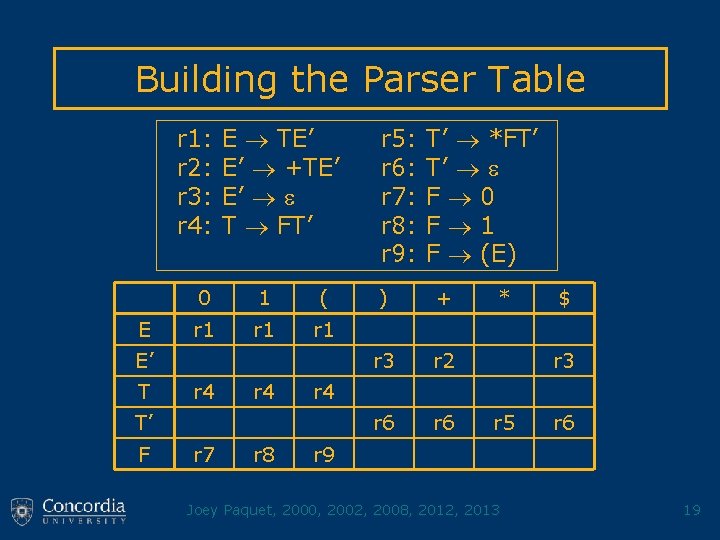
Building the Parser Table r 1: r 2: r 3: r 4: E E TE’ E’ +TE’ E’ T FT’ 0 1 ( r 1 r 1 E’ T r 4 r 7 r 8 T’ *FT’ T’ F 0 F 1 F (E) ) + r 3 r 2 r 6 * $ r 3 r 4 T’ F r 5: r 6: r 7: r 8: r 9: r 5 r 6 r 9 Joey Paquet, 2000, 2002, 2008, 2012, 2013 19
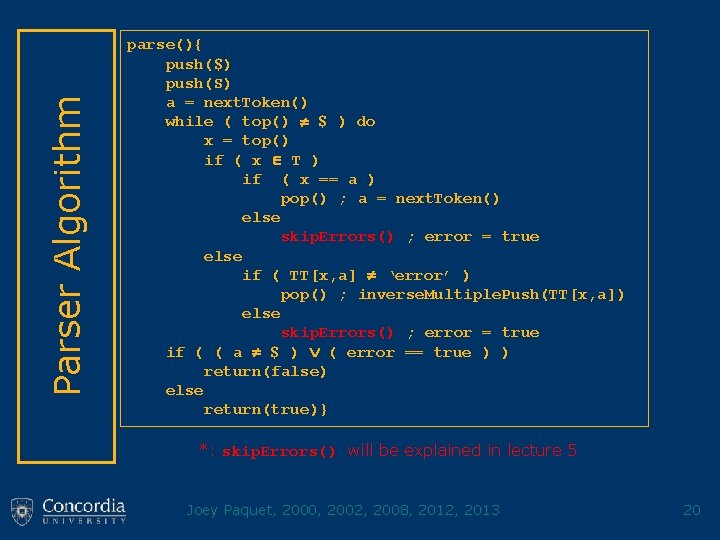
Parser Algorithm parse(){ push($) push(S) a = next. Token() while ( top() $ ) do x = top() if ( x T ) if ( x == a ) pop() ; a = next. Token() else skip. Errors() ; error = true else if ( TT[x, a] ‘error’ ) pop() ; inverse. Multiple. Push(TT[x, a]) else skip. Errors() ; error = true if ( ( a $ ) ( error == true ) ) return(false) else return(true)} *: skip. Errors() will be explained in lecture 5 Joey Paquet, 2000, 2002, 2008, 2012, 2013 20
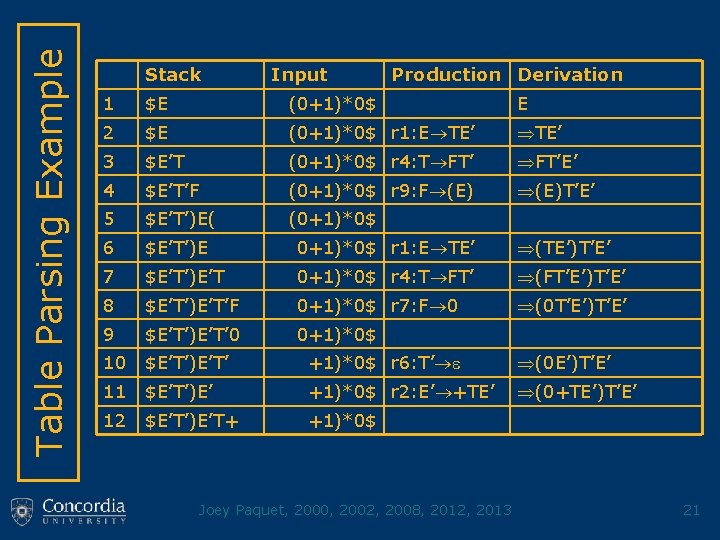
Table Parsing Example Stack Input Production Derivation 1 $E (0+1)*0$ E 2 $E (0+1)*0$ r 1: E TE’ 3 $E’T (0+1)*0$ r 4: T FT’E’ 4 $E’T’F (0+1)*0$ r 9: F (E)T’E’ 5 $E’T’)E( (0+1)*0$ 6 $E’T’)E 0+1)*0$ r 1: E TE’ (TE’)T’E’ 7 $E’T’)E’T 0+1)*0$ r 4: T FT’ (FT’E’)T’E’ 8 $E’T’)E’T’F 0+1)*0$ r 7: F 0 (0 T’E’)T’E’ 9 $E’T’)E’T’ 0 0+1)*0$ 10 $E’T’)E’T’ +1)*0$ r 6: T’ (0 E’)T’E’ 11 $E’T’)E’ +1)*0$ r 2: E’ +TE’ (0+TE’)T’E’ 12 $E’T’)E’T+ +1)*0$ Joey Paquet, 2000, 2002, 2008, 2012, 2013 21
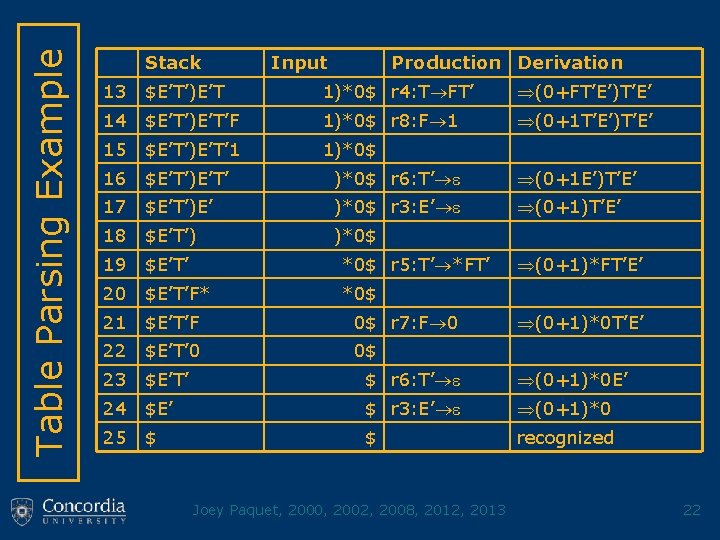
Table Parsing Example Stack Input Production Derivation 13 $E’T’)E’T 1)*0$ r 4: T FT’ (0+FT’E’)T’E’ 14 $E’T’)E’T’F 1)*0$ r 8: F 1 (0+1 T’E’)T’E’ 15 $E’T’)E’T’ 1 1)*0$ 16 $E’T’)E’T’ )*0$ r 6: T’ (0+1 E’)T’E’ 17 $E’T’)E’ )*0$ r 3: E’ (0+1)T’E’ 18 $E’T’) )*0$ 19 $E’T’ *0$ r 5: T’ *FT’ 20 $E’T’F* *0$ 21 $E’T’F 0$ r 7: F 0 22 $E’T’ 0 0$ 23 $E’T’ $ r 6: T’ (0+1)*0 E’ 24 $E’ $ r 3: E’ (0+1)*0 25 $ $ recognized Joey Paquet, 2000, 2002, 2008, 2012, 2013 (0+1)*FT’E’ (0+1)*0 T’E’ 22
Joey paquet
Naomi rachel king
Procedural programming languages
Prévention des tiac en restauration collective
Varices esofagicas clasificacion endoscopica
John paquet
Vrices
Paquet tva
La fosse axillaire
Larp logiciel
01:640:244 lecture notes - lecture 15: plat, idah, farad
The boy saw the man with the telescope tree diagram
812007
Syntactic analysis exercises
Symbols used in syntactic analysis
Joey azofeifa
Tangerine paul fisher
Joey movers
Joey salloway
Free entry and exit
Joey scout promise
Joey daun
Joey stiles