L L Line CSE 420 Computer Games Lecture
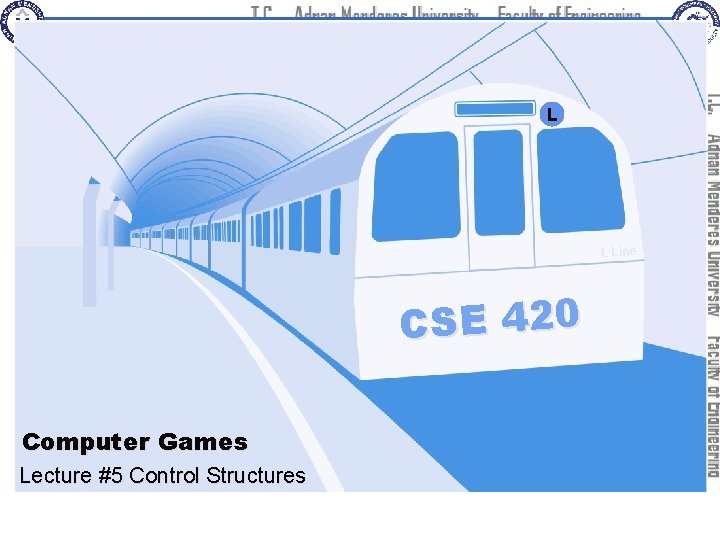
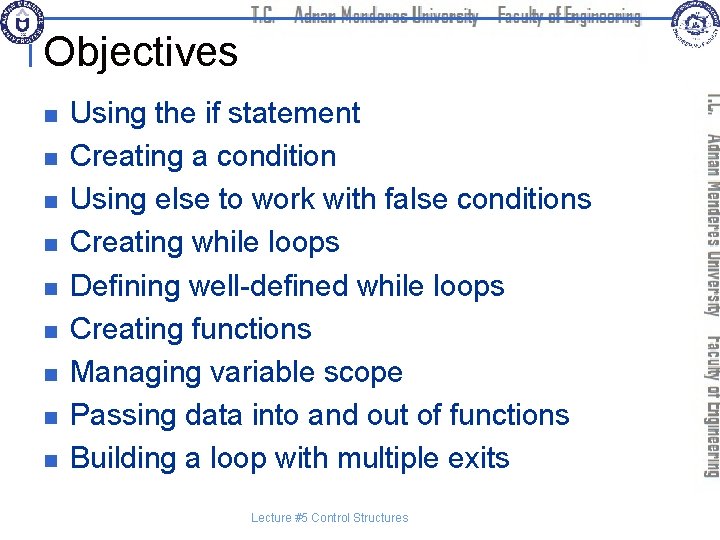
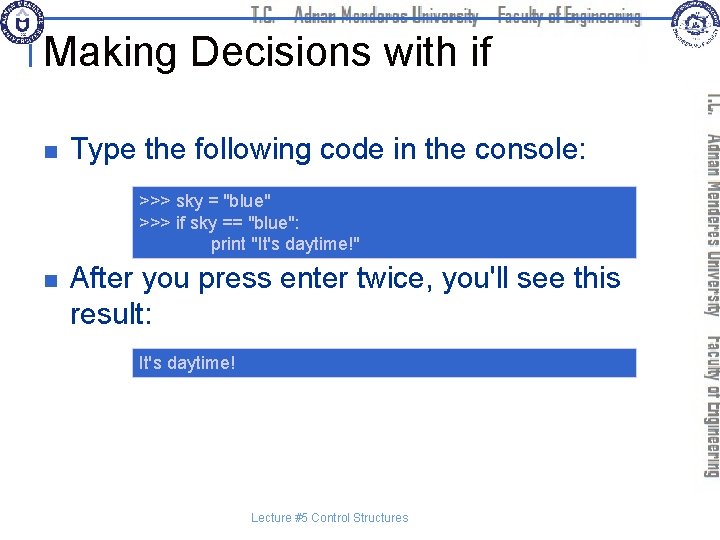
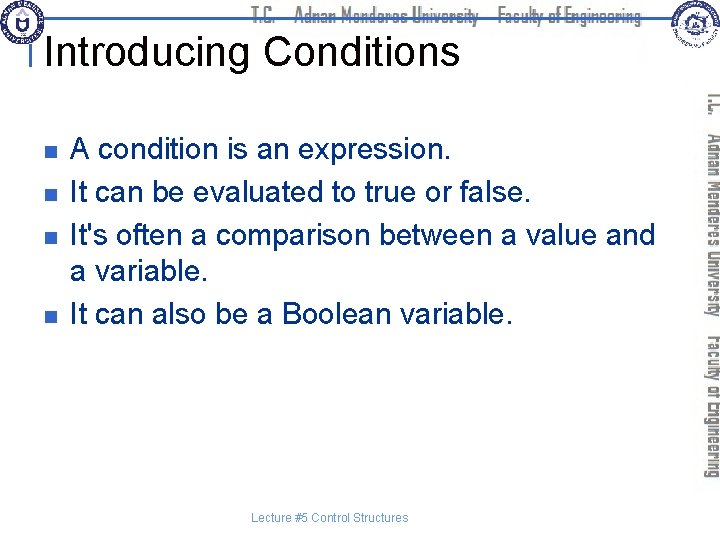
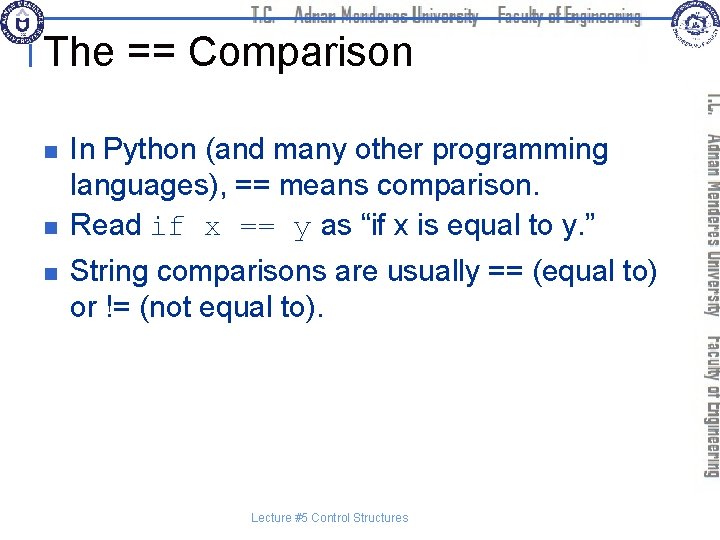
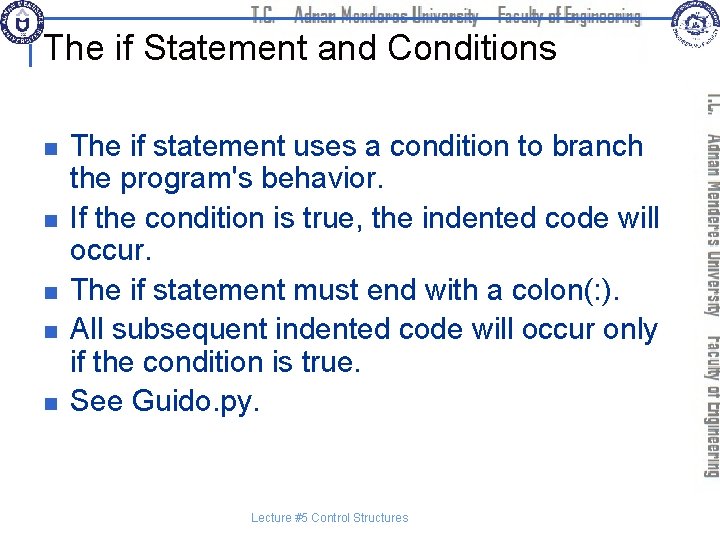
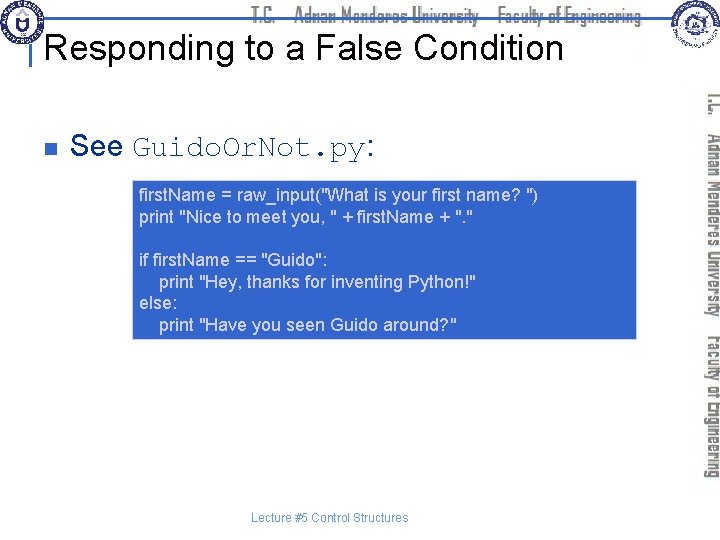
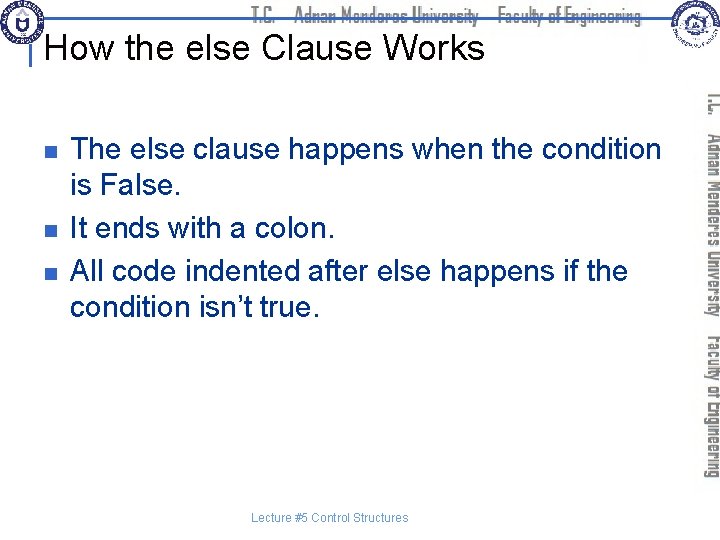
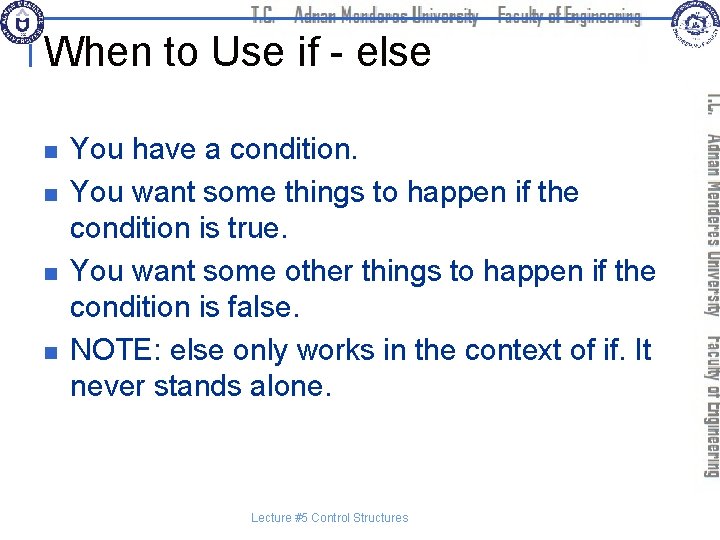
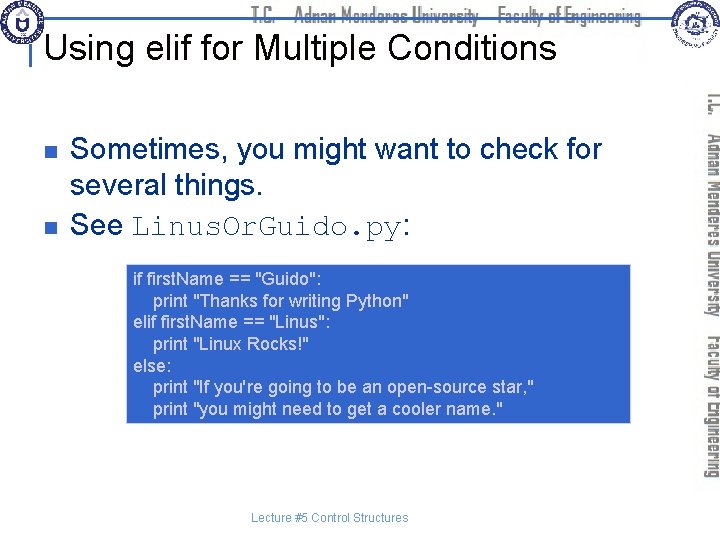
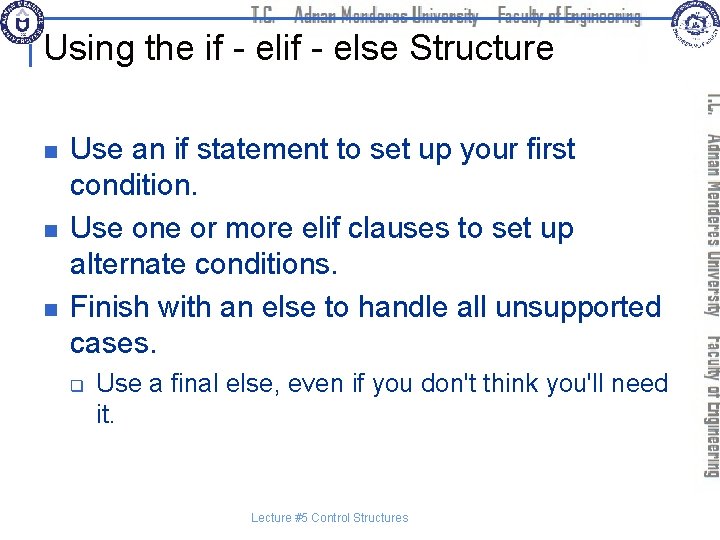
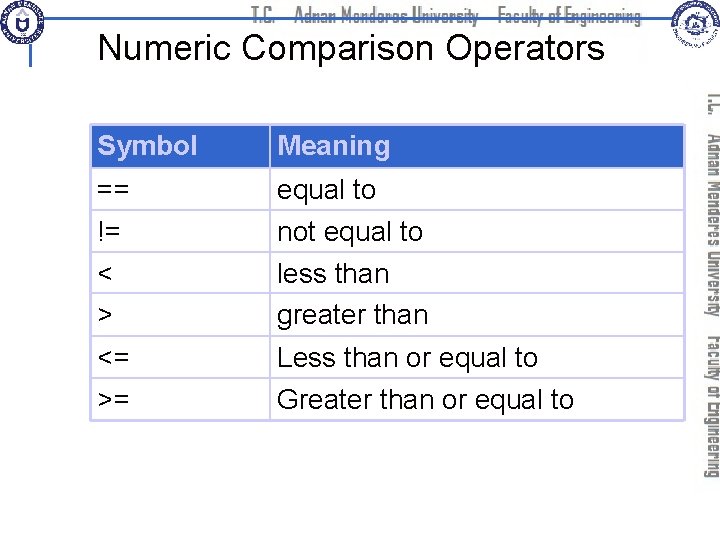
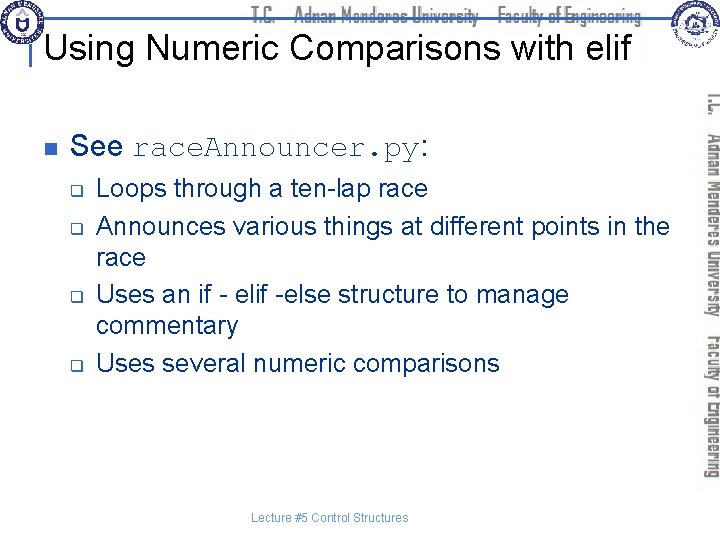
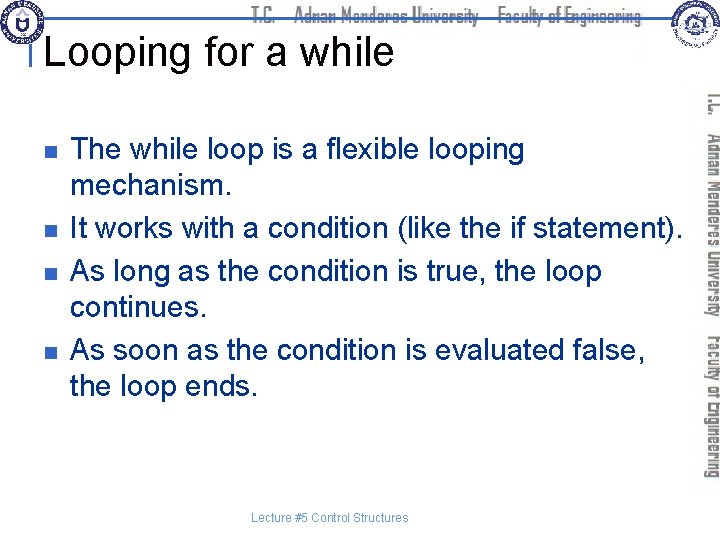
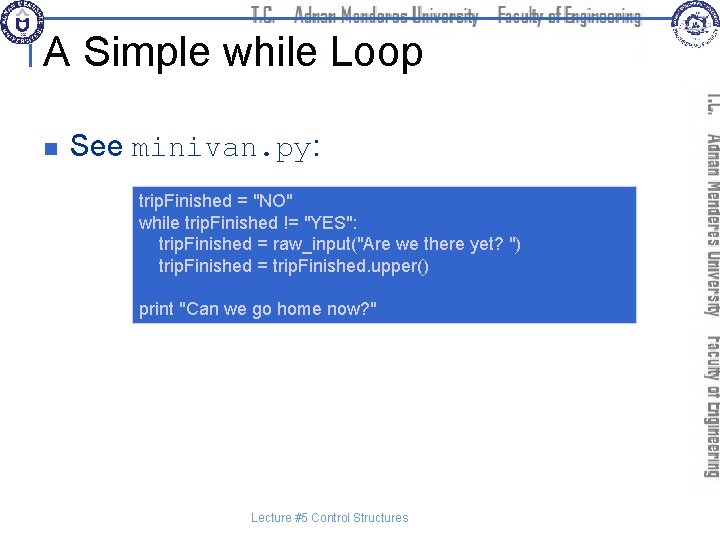
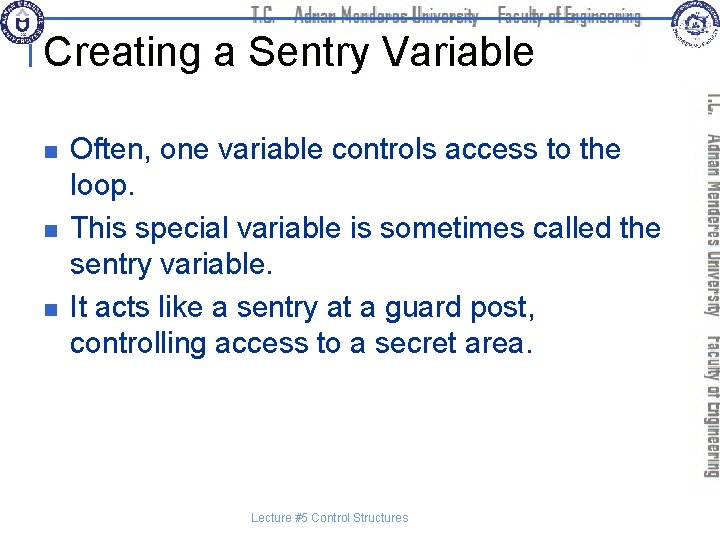
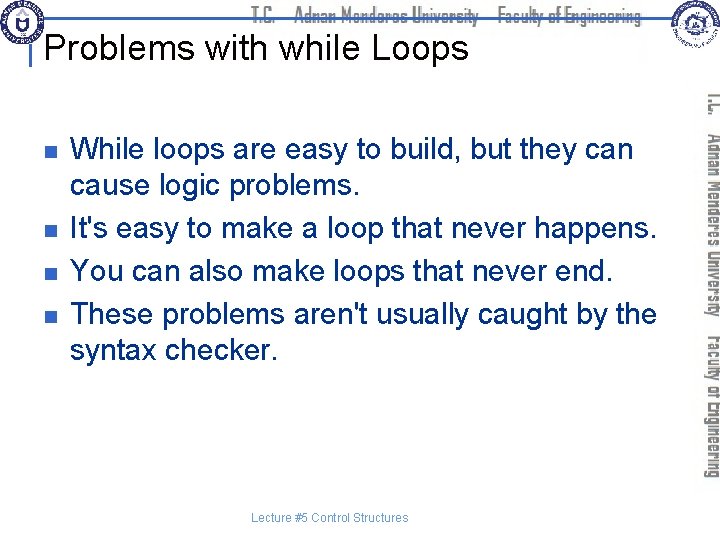
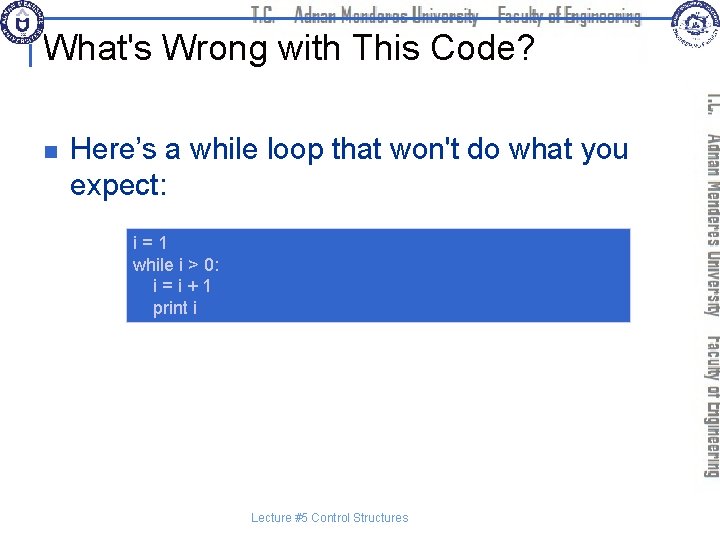
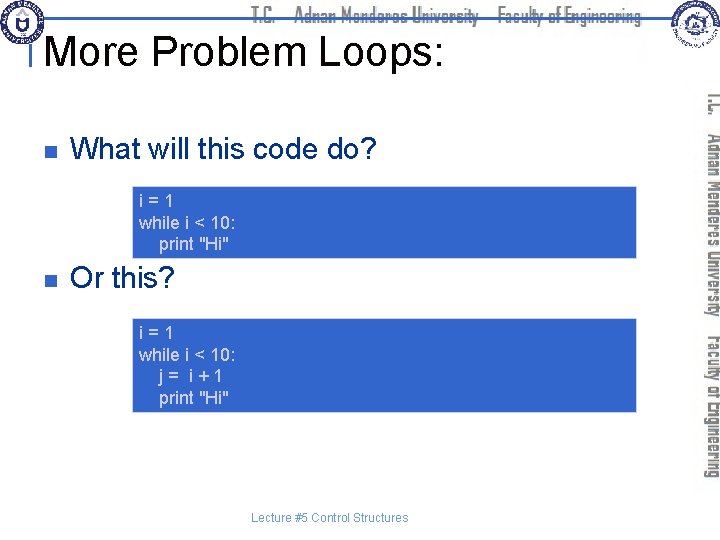
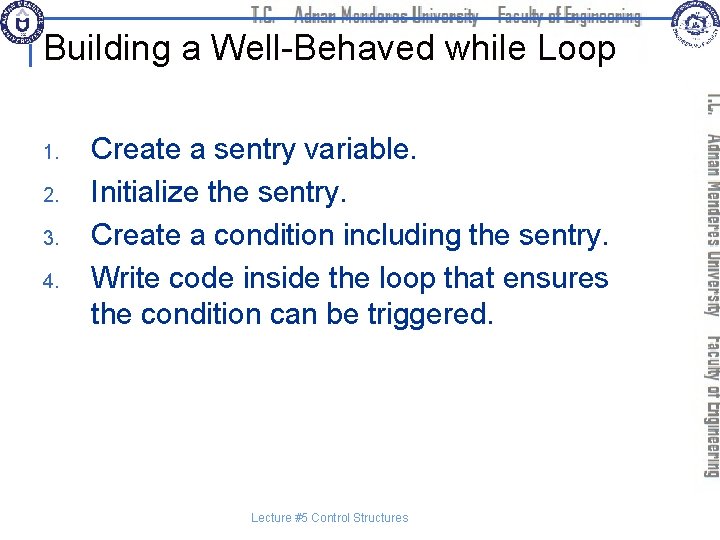
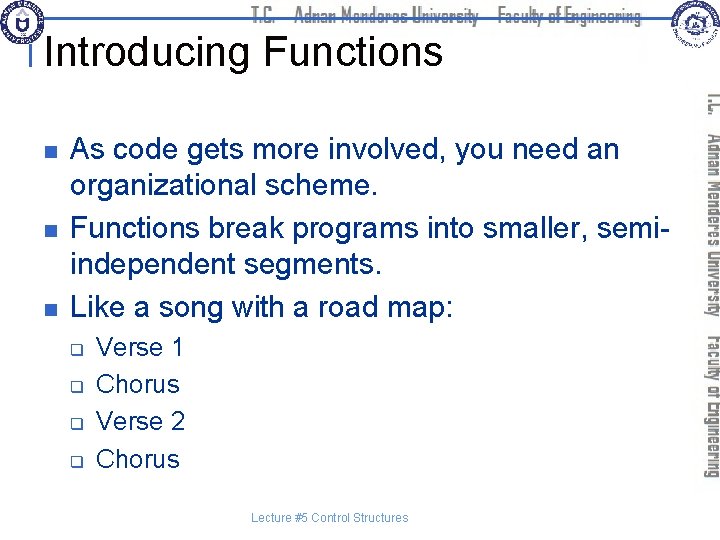
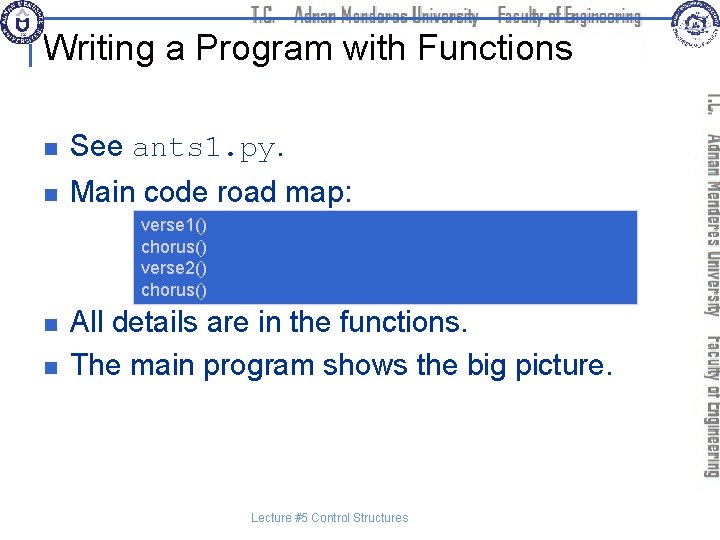
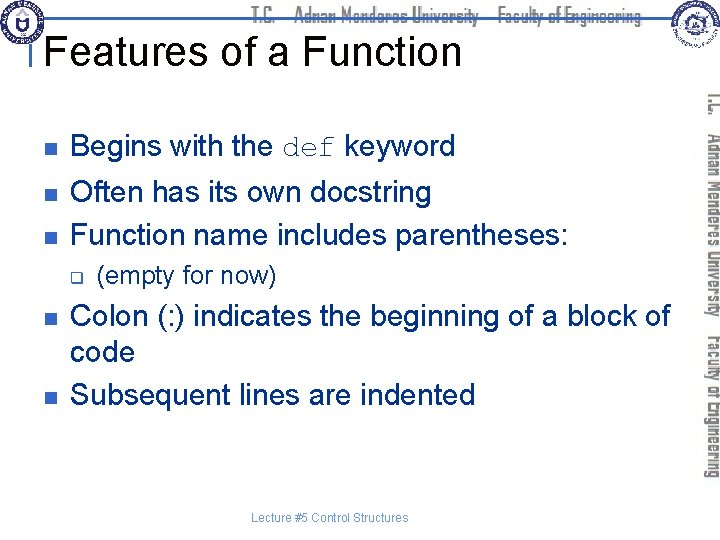
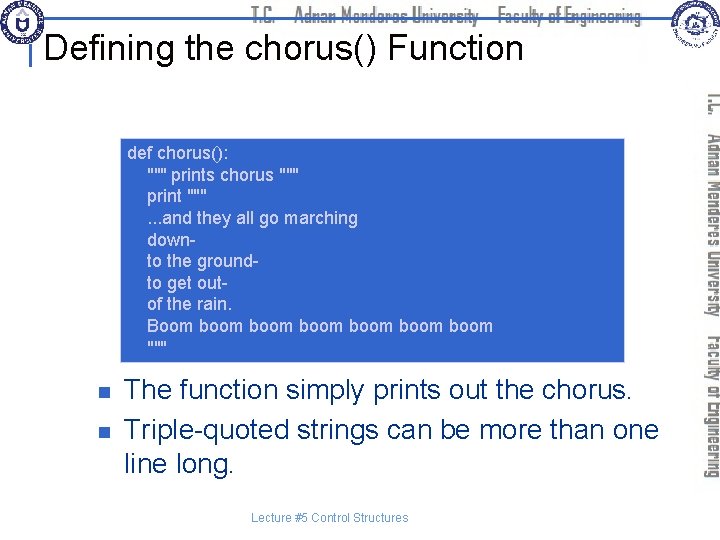
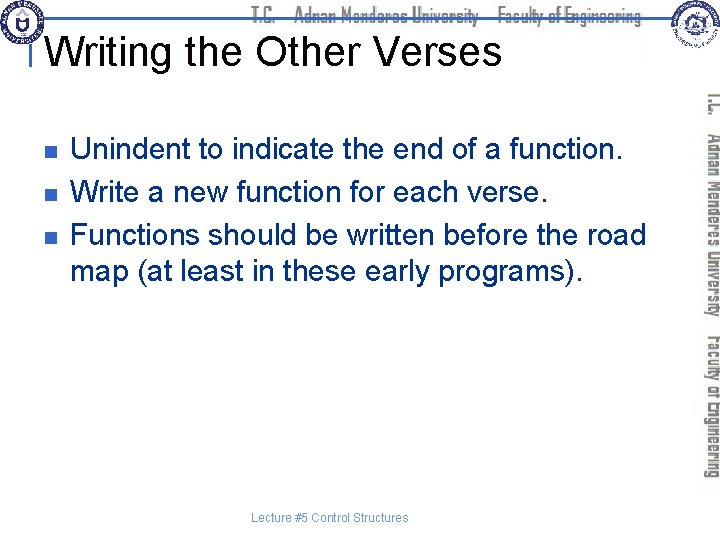
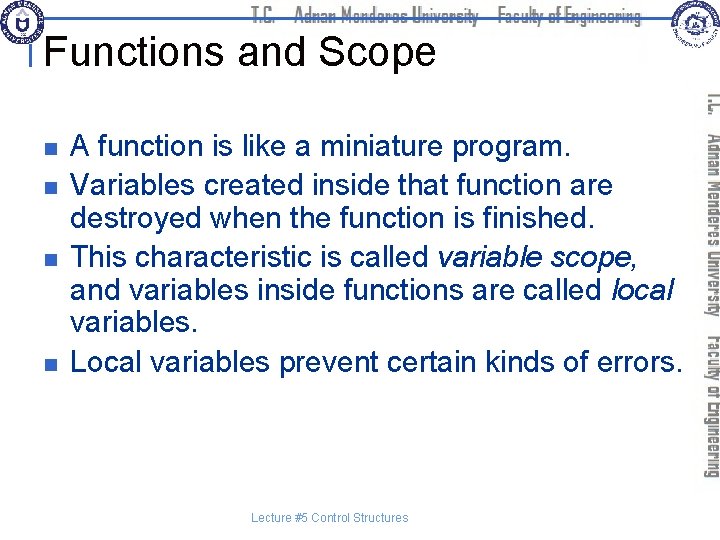
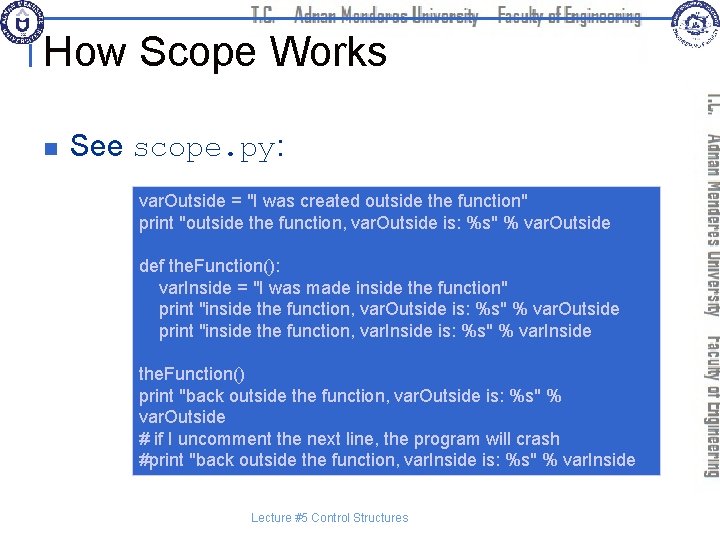
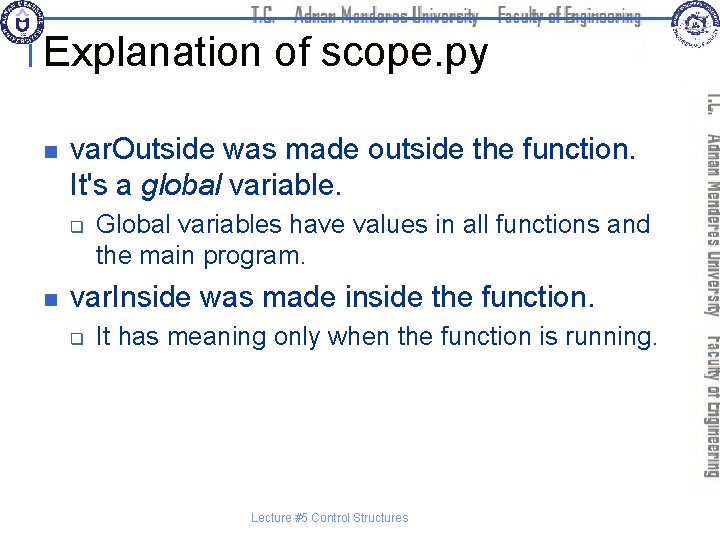
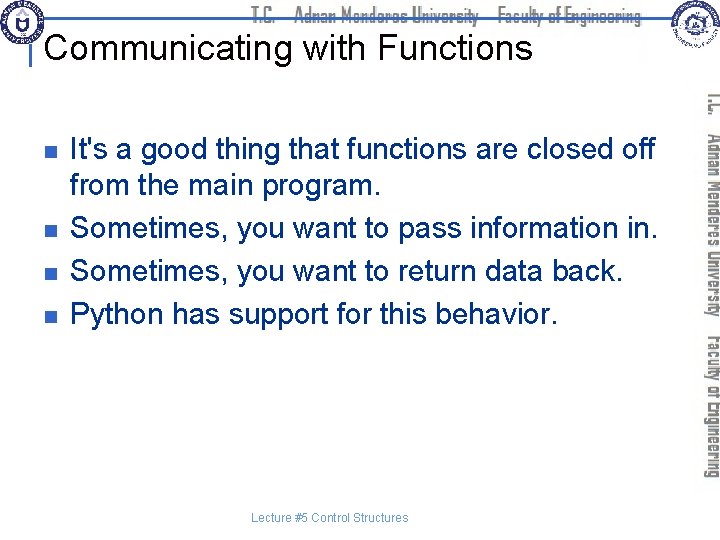
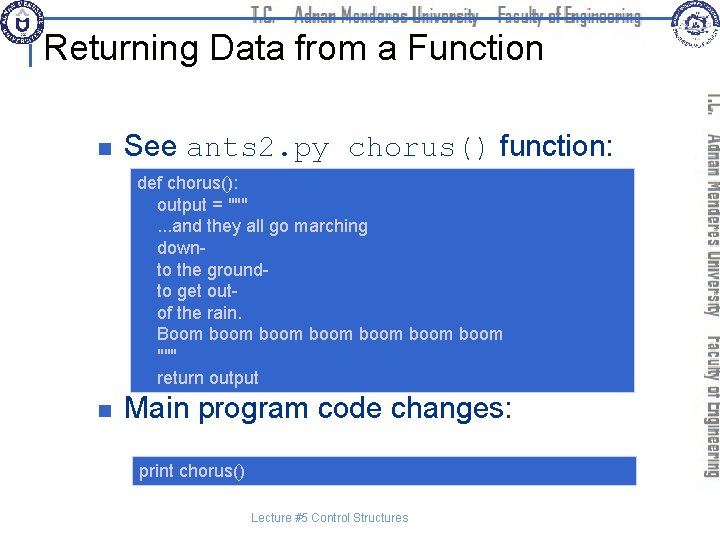
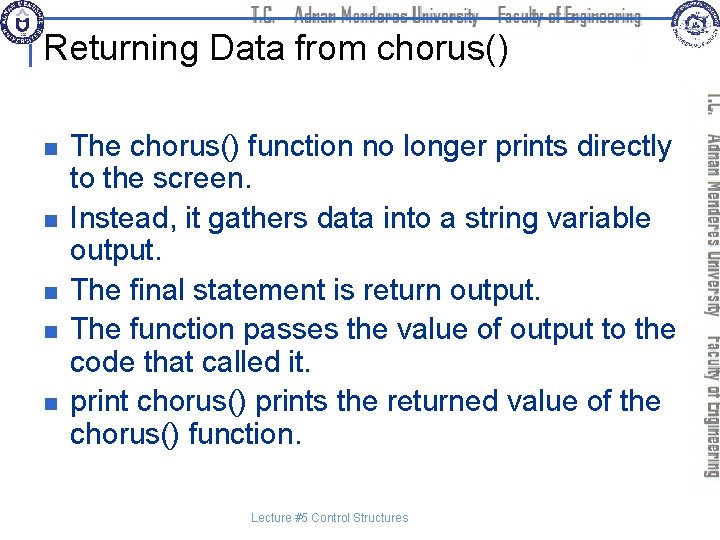
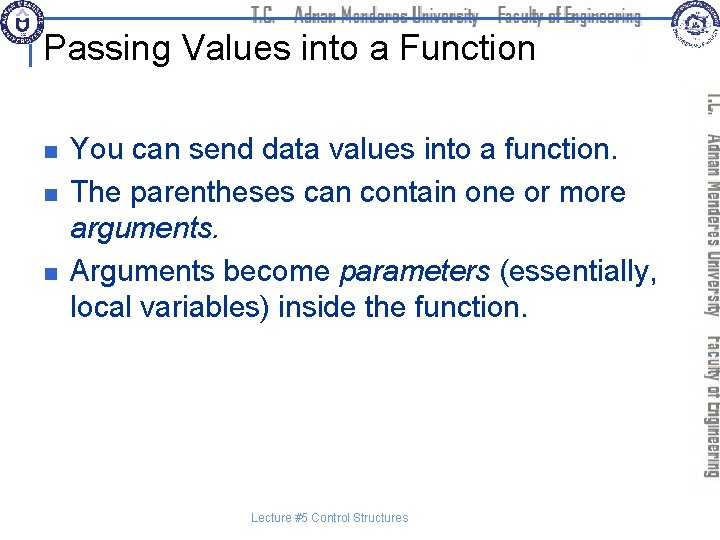
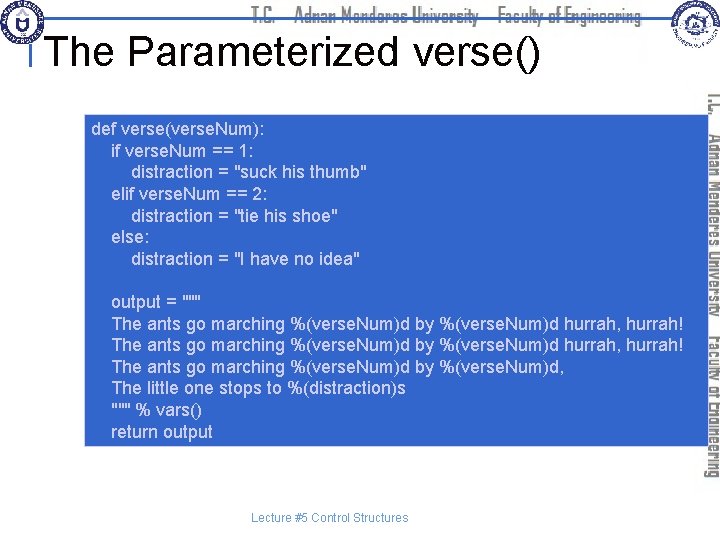
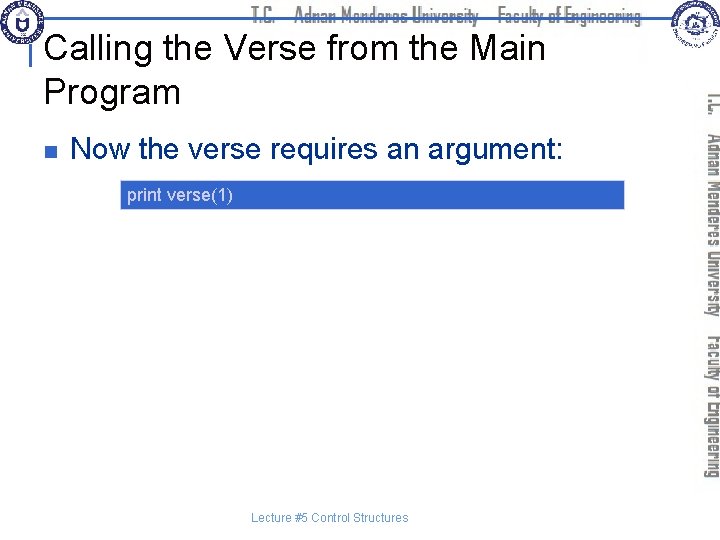
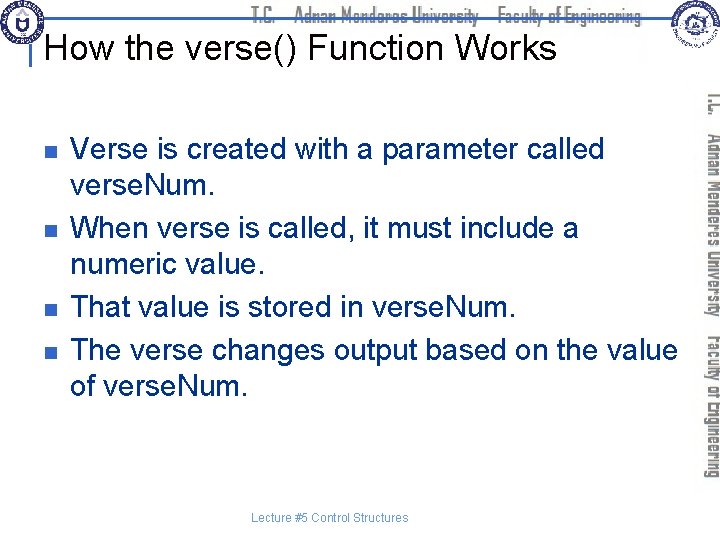
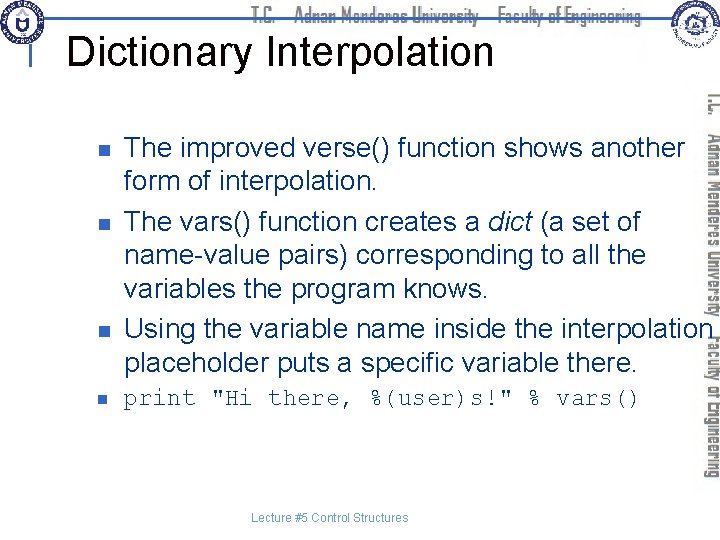
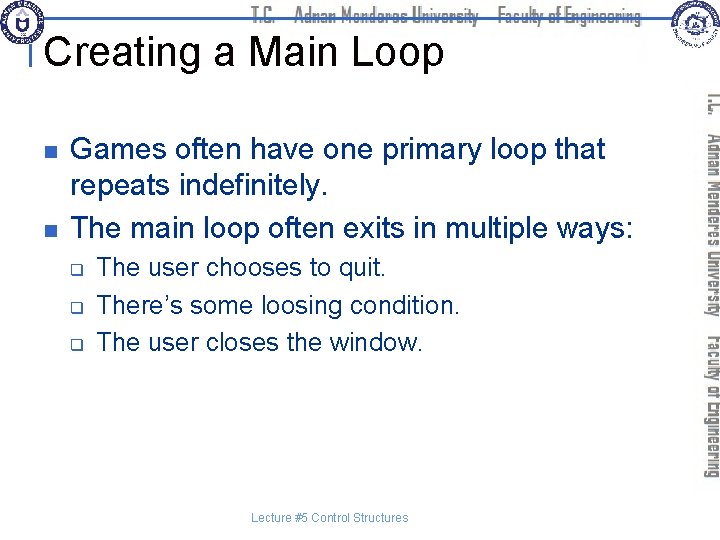
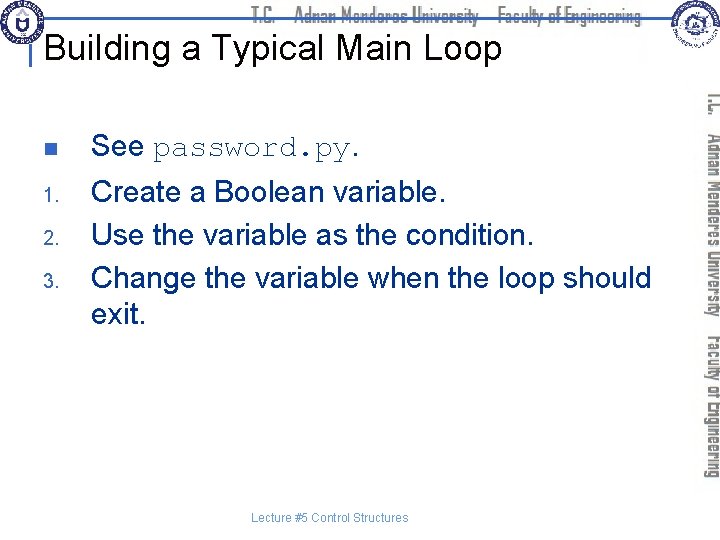
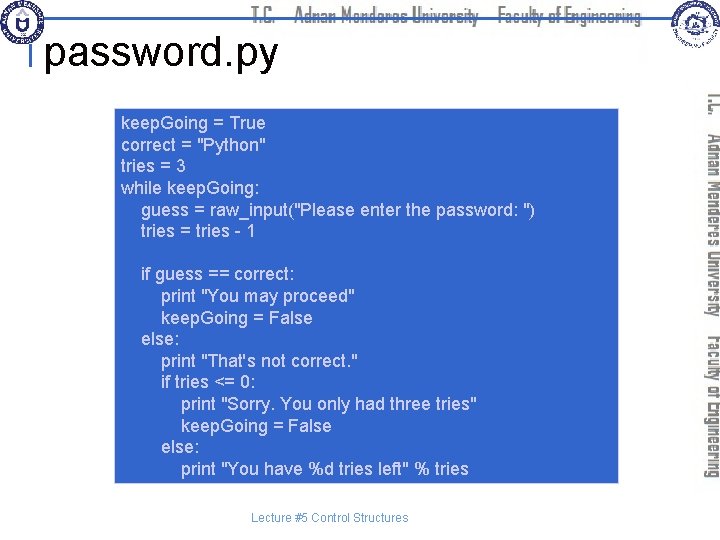
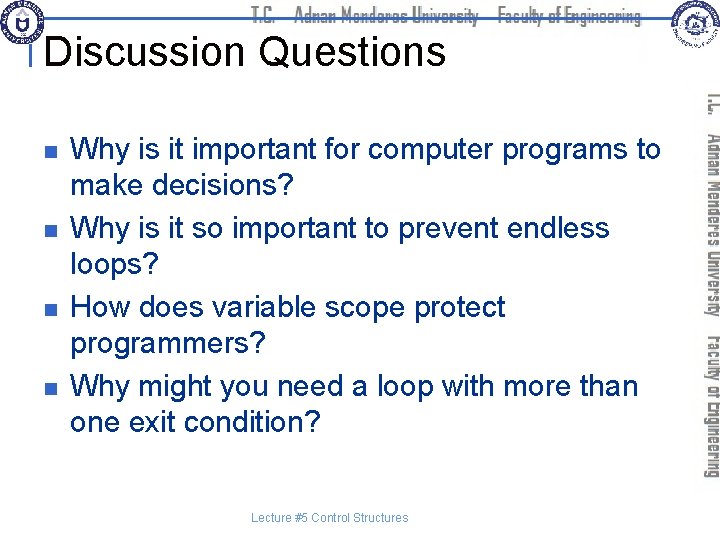
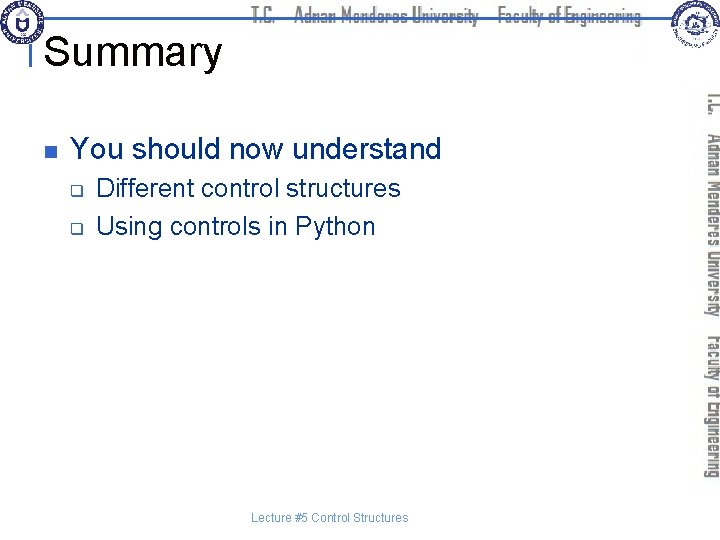
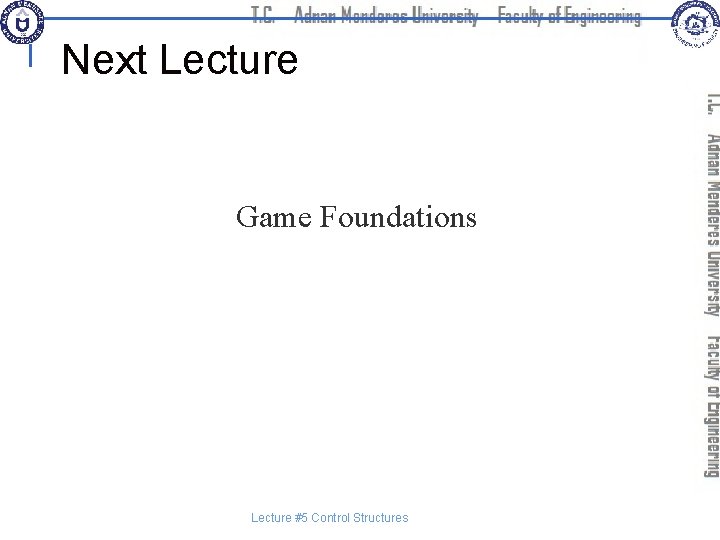
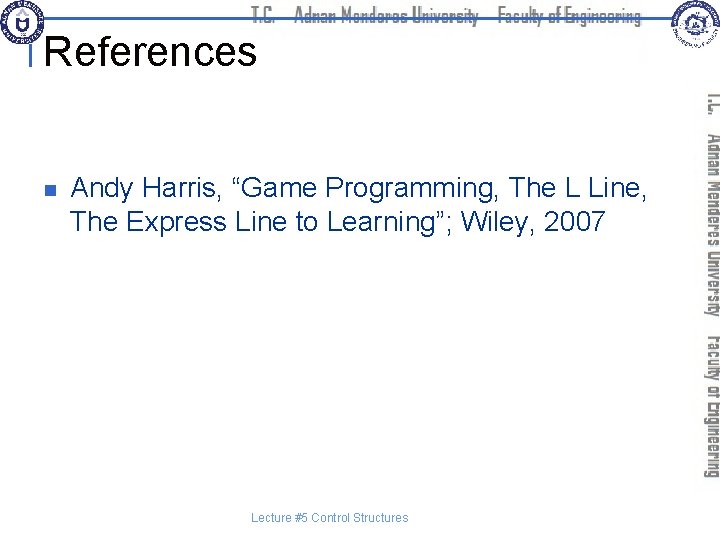
- Slides: 43
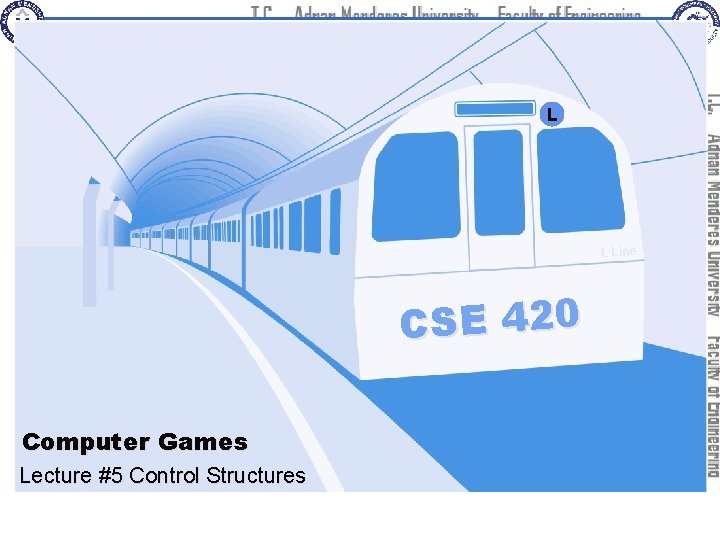
L L Line CSE 420 Computer Games Lecture #5 Control Structures
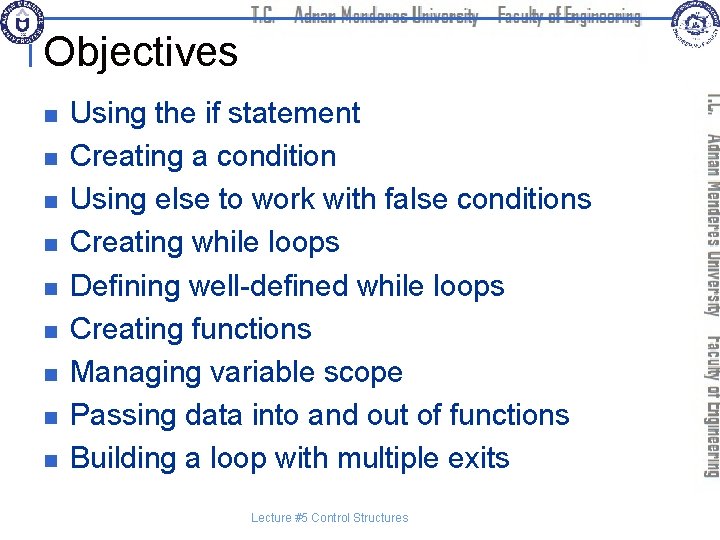
Objectives n n n n n Using the if statement Creating a condition Using else to work with false conditions Creating while loops Defining well-defined while loops Creating functions Managing variable scope Passing data into and out of functions Building a loop with multiple exits Lecture #5 Control Structures
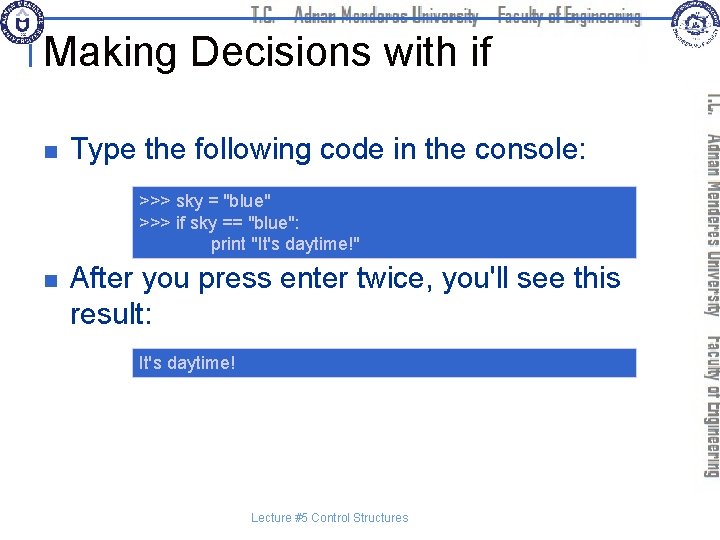
Making Decisions with if n Type the following code in the console: >>> sky = "blue" >>> if sky == "blue": print "It's daytime!" n After you press enter twice, you'll see this result: It's daytime! Lecture #5 Control Structures
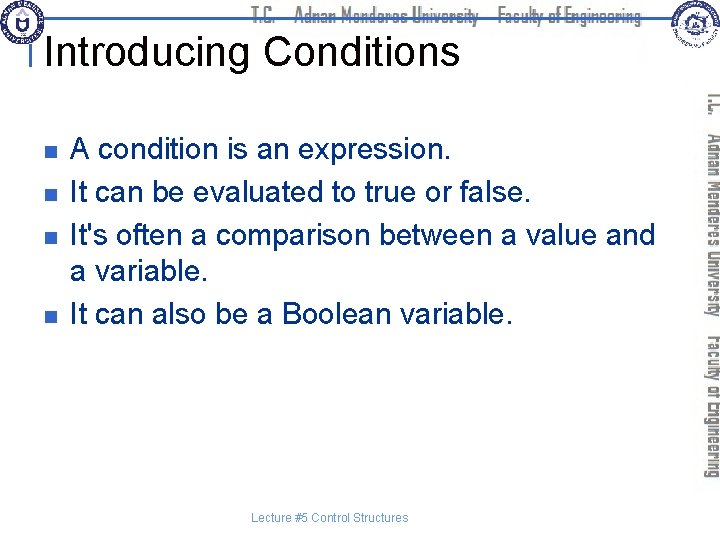
Introducing Conditions n n A condition is an expression. It can be evaluated to true or false. It's often a comparison between a value and a variable. It can also be a Boolean variable. Lecture #5 Control Structures
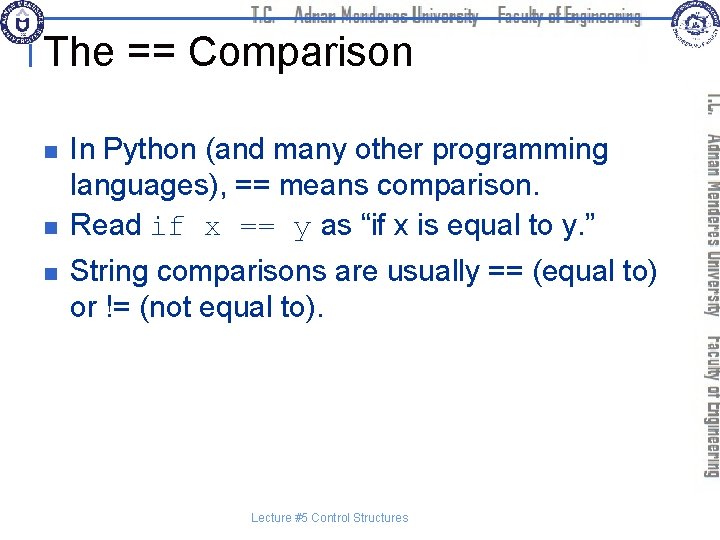
The == Comparison n In Python (and many other programming languages), == means comparison. Read if x == y as “if x is equal to y. ” String comparisons are usually == (equal to) or != (not equal to). Lecture #5 Control Structures
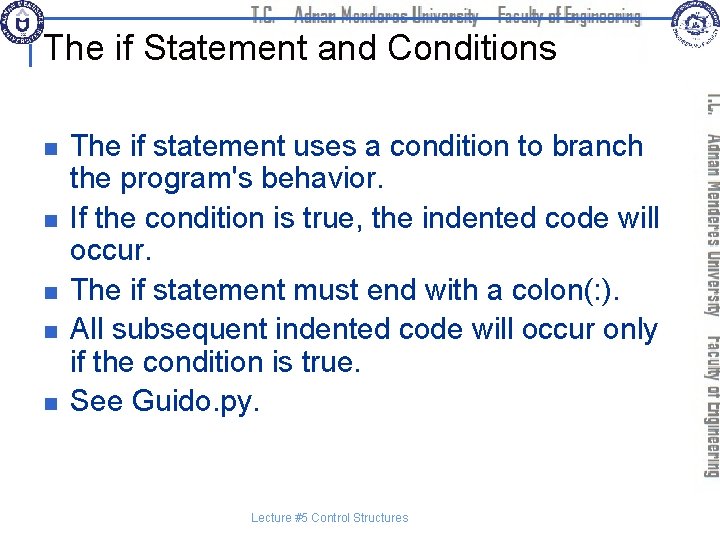
The if Statement and Conditions n n n The if statement uses a condition to branch the program's behavior. If the condition is true, the indented code will occur. The if statement must end with a colon(: ). All subsequent indented code will occur only if the condition is true. See Guido. py. Lecture #5 Control Structures
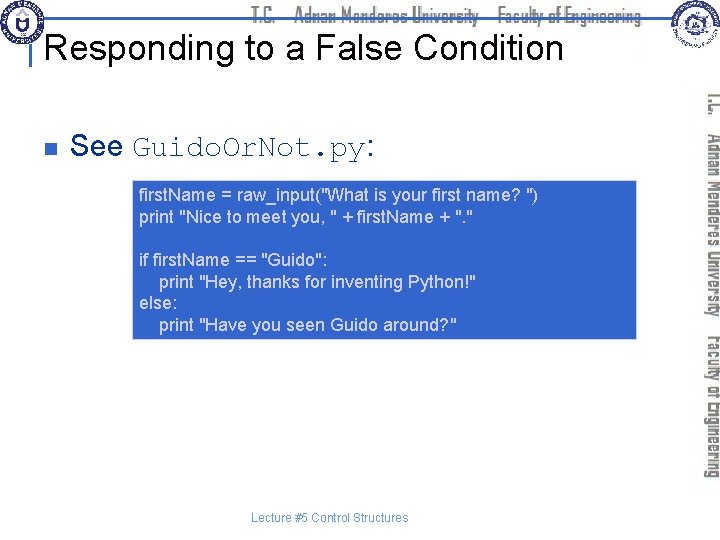
Responding to a False Condition n See Guido. Or. Not. py: first. Name = raw_input("What is your first name? ") print "Nice to meet you, " + first. Name + ". " if first. Name == "Guido": print "Hey, thanks for inventing Python!" else: print "Have you seen Guido around? " Lecture #5 Control Structures
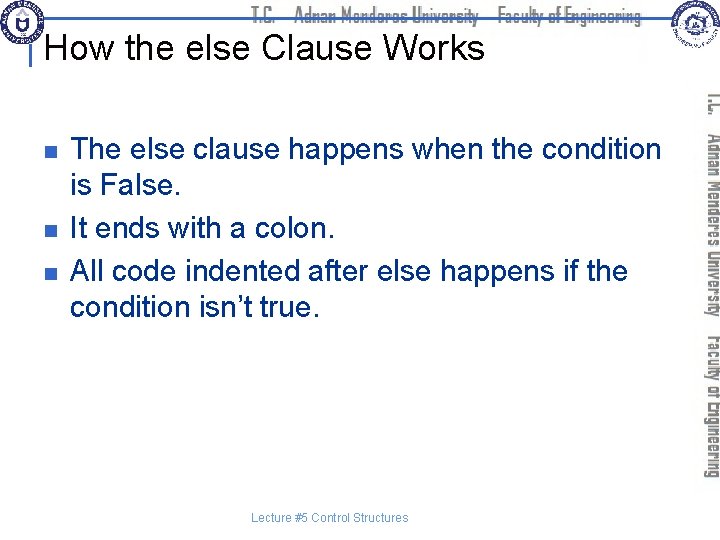
How the else Clause Works n n n The else clause happens when the condition is False. It ends with a colon. All code indented after else happens if the condition isn’t true. Lecture #5 Control Structures
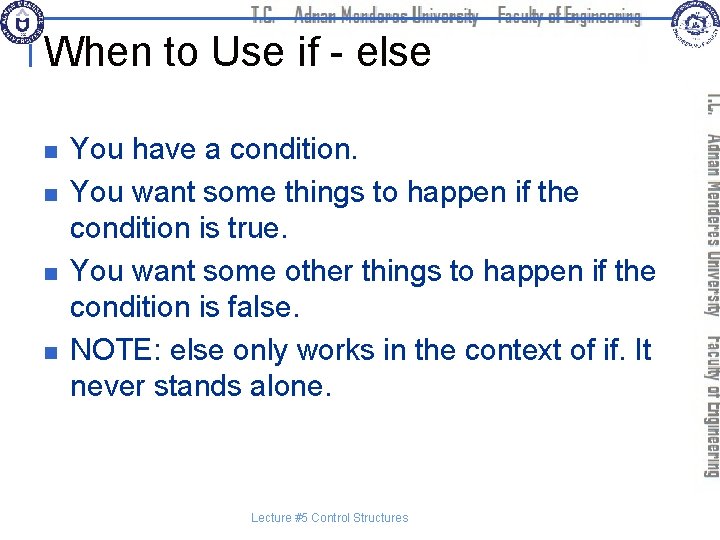
When to Use if - else n n You have a condition. You want some things to happen if the condition is true. You want some other things to happen if the condition is false. NOTE: else only works in the context of if. It never stands alone. Lecture #5 Control Structures
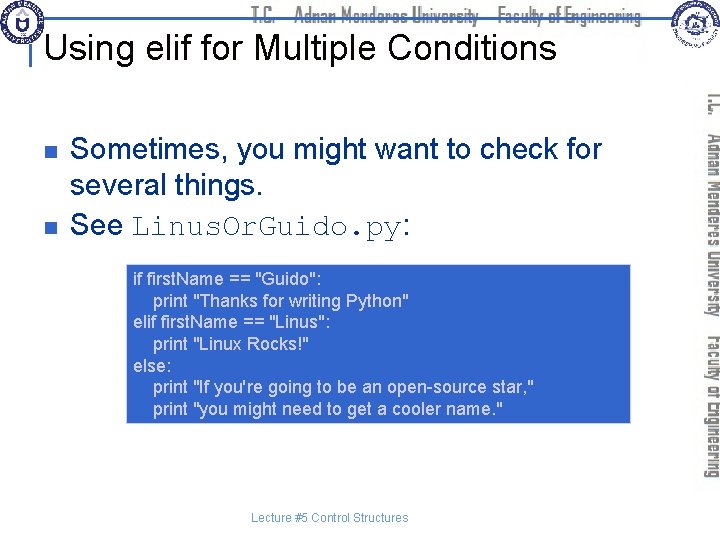
Using elif for Multiple Conditions n n Sometimes, you might want to check for several things. See Linus. Or. Guido. py: if first. Name == "Guido": print "Thanks for writing Python" elif first. Name == "Linus": print "Linux Rocks!" else: print "If you're going to be an open-source star, " print "you might need to get a cooler name. " Lecture #5 Control Structures
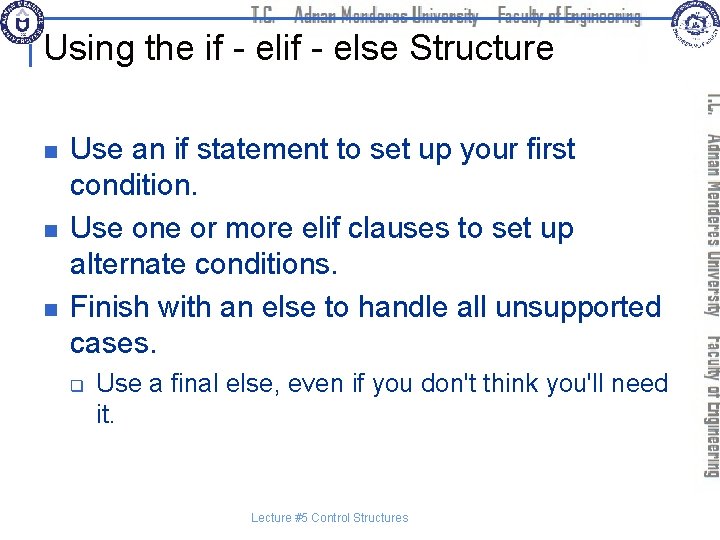
Using the if - else Structure n n n Use an if statement to set up your first condition. Use one or more elif clauses to set up alternate conditions. Finish with an else to handle all unsupported cases. q Use a final else, even if you don't think you'll need it. Lecture #5 Control Structures
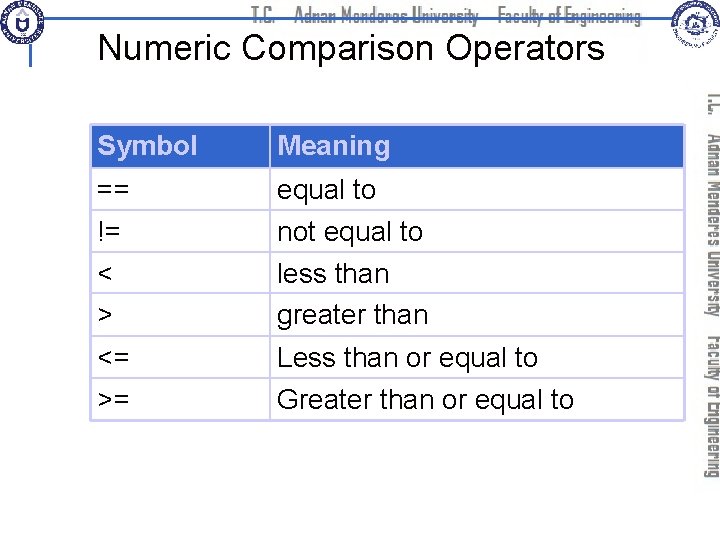
Numeric Comparison Operators Symbol Meaning == != < > equal to not equal to less than greater than <= >= Less than or equal to Greater than or equal to
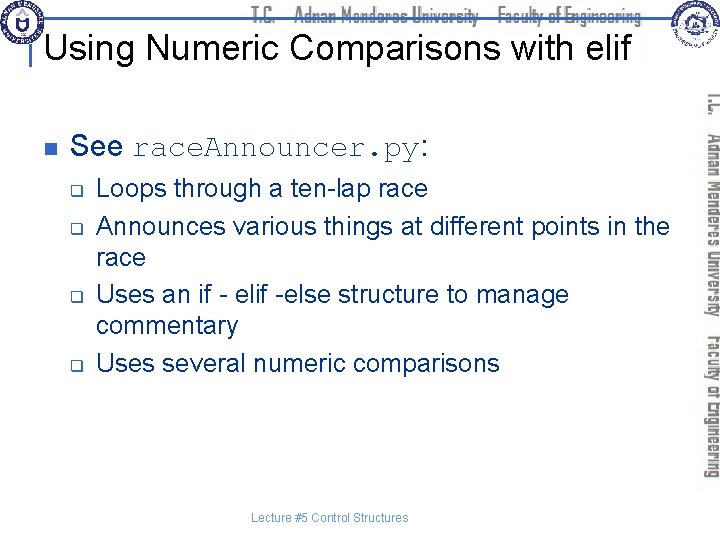
Using Numeric Comparisons with elif n See race. Announcer. py: q q Loops through a ten-lap race Announces various things at different points in the race Uses an if - elif -else structure to manage commentary Uses several numeric comparisons Lecture #5 Control Structures
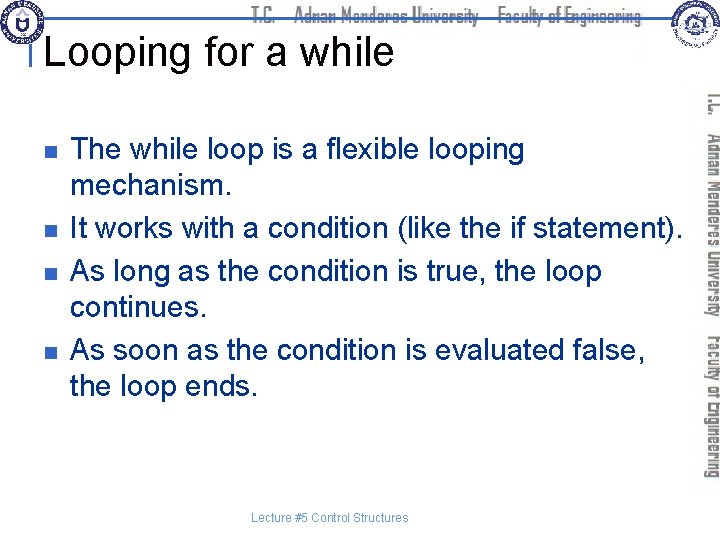
Looping for a while n n The while loop is a flexible looping mechanism. It works with a condition (like the if statement). As long as the condition is true, the loop continues. As soon as the condition is evaluated false, the loop ends. Lecture #5 Control Structures
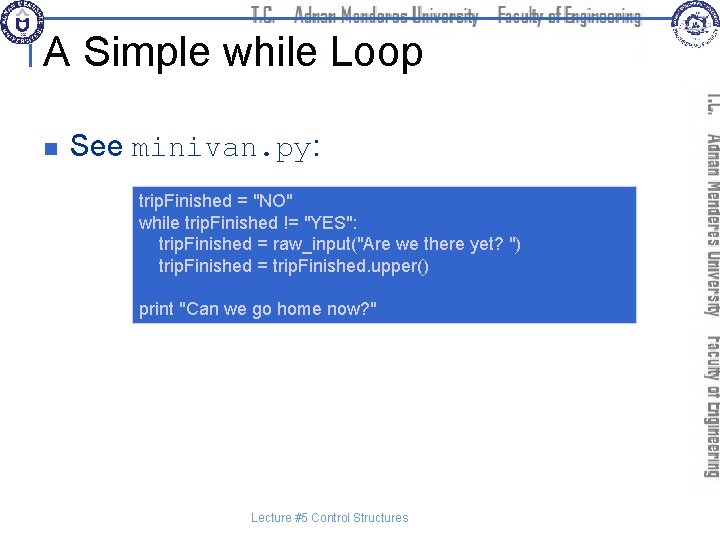
A Simple while Loop n See minivan. py: trip. Finished = "NO" while trip. Finished != "YES": trip. Finished = raw_input("Are we there yet? ") trip. Finished = trip. Finished. upper() print "Can we go home now? " Lecture #5 Control Structures
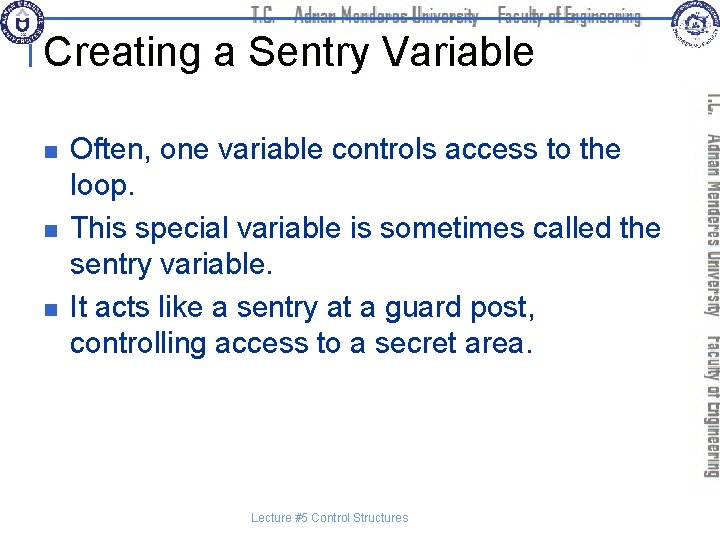
Creating a Sentry Variable n n n Often, one variable controls access to the loop. This special variable is sometimes called the sentry variable. It acts like a sentry at a guard post, controlling access to a secret area. Lecture #5 Control Structures
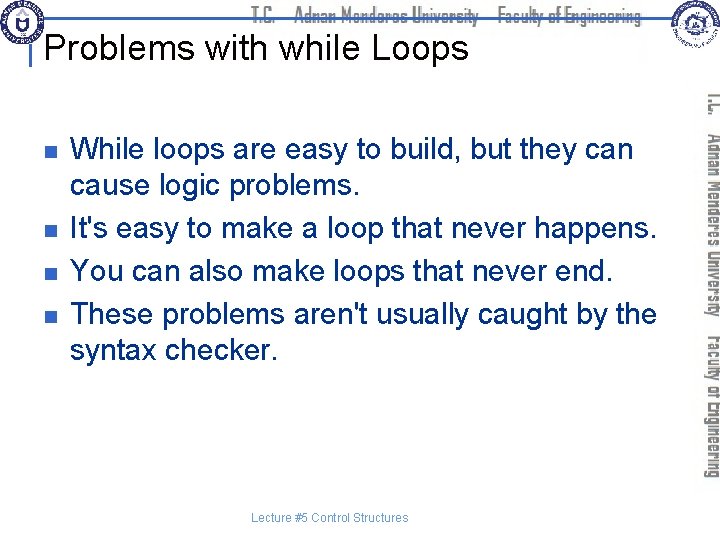
Problems with while Loops n n While loops are easy to build, but they can cause logic problems. It's easy to make a loop that never happens. You can also make loops that never end. These problems aren't usually caught by the syntax checker. Lecture #5 Control Structures
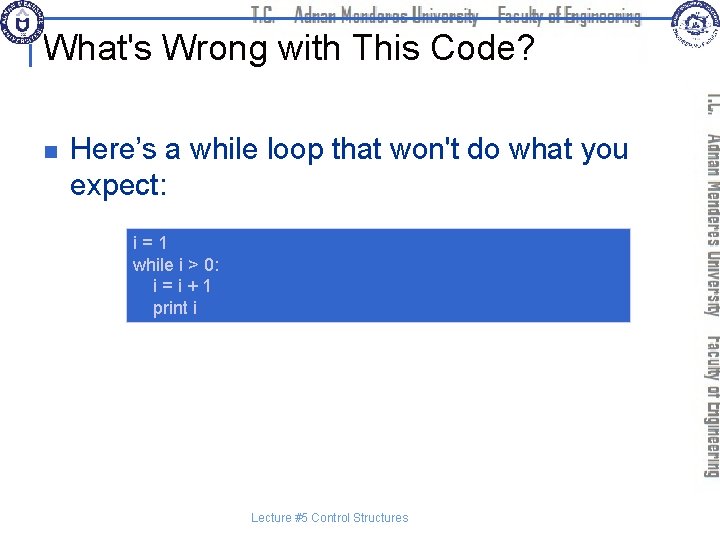
What's Wrong with This Code? n Here’s a while loop that won't do what you expect: i=1 while i > 0: i=i+1 print i Lecture #5 Control Structures
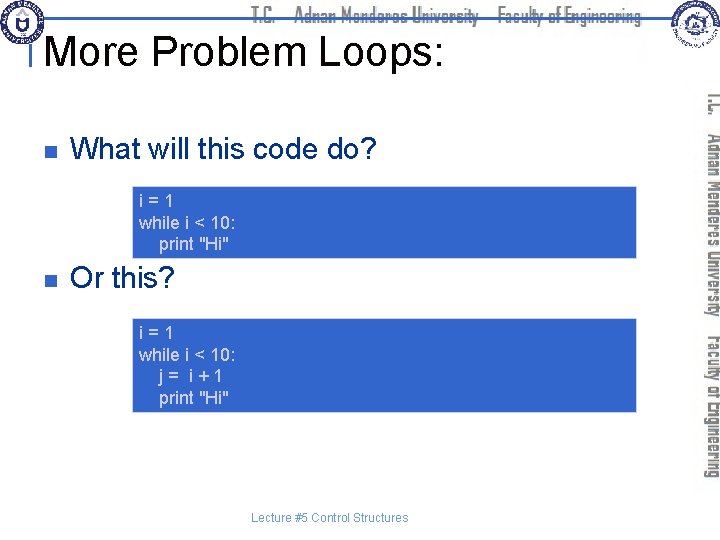
More Problem Loops: n What will this code do? i=1 while i < 10: print "Hi" n Or this? i=1 while i < 10: j= i+1 print "Hi" Lecture #5 Control Structures
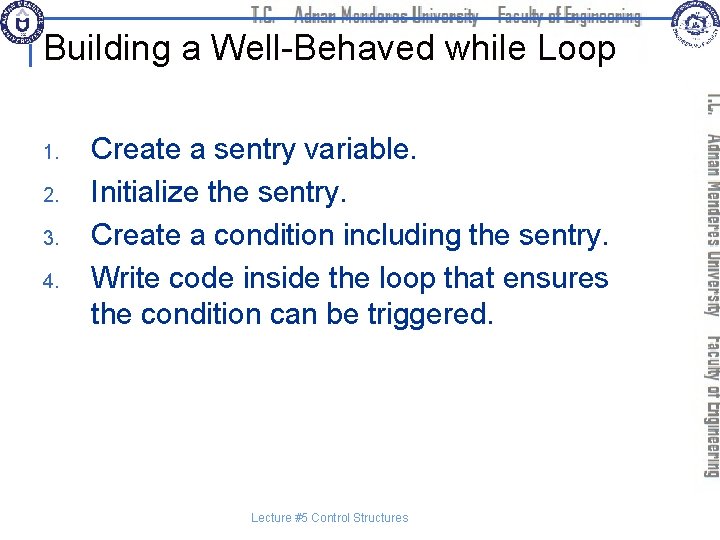
Building a Well-Behaved while Loop 1. 2. 3. 4. Create a sentry variable. Initialize the sentry. Create a condition including the sentry. Write code inside the loop that ensures the condition can be triggered. Lecture #5 Control Structures
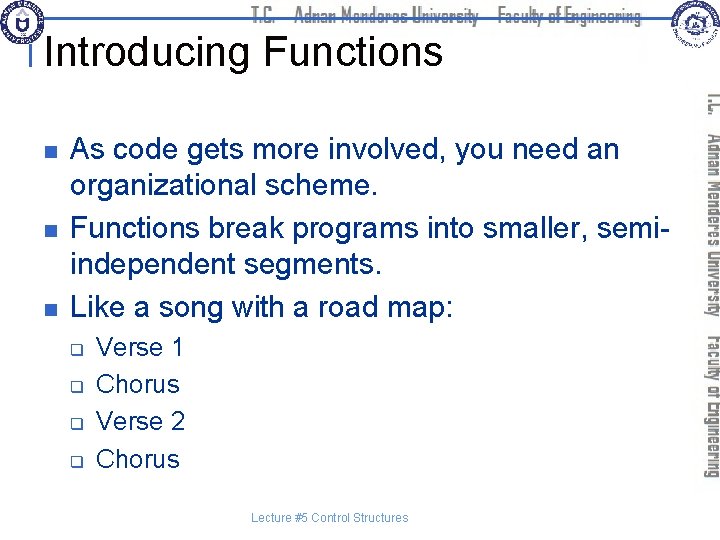
Introducing Functions n n n As code gets more involved, you need an organizational scheme. Functions break programs into smaller, semiindependent segments. Like a song with a road map: q q Verse 1 Chorus Verse 2 Chorus Lecture #5 Control Structures
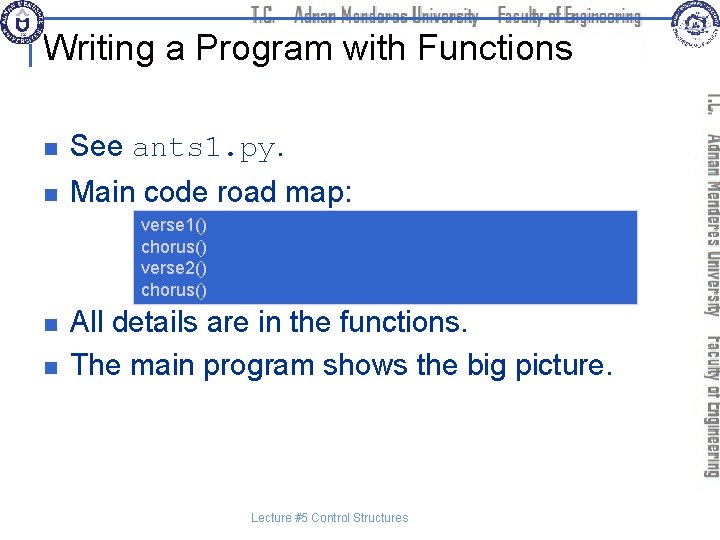
Writing a Program with Functions n See ants 1. py. n Main code road map: verse 1() chorus() verse 2() chorus() n n All details are in the functions. The main program shows the big picture. Lecture #5 Control Structures
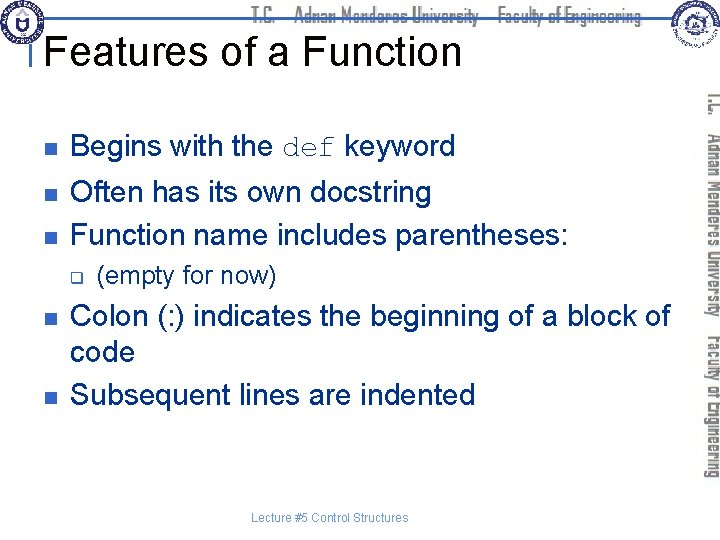
Features of a Function n Begins with the def keyword n Often has its own docstring Function name includes parentheses: n q n n (empty for now) Colon (: ) indicates the beginning of a block of code Subsequent lines are indented Lecture #5 Control Structures
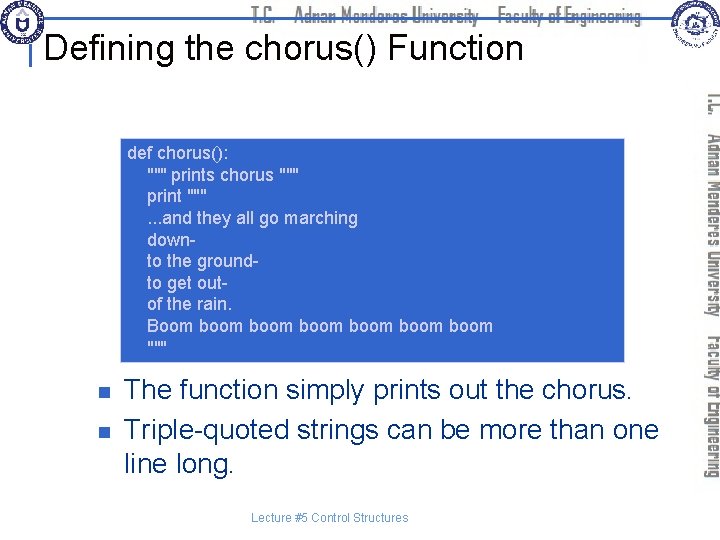
Defining the chorus() Function def chorus(): """ prints chorus """ print """. . . and they all go marching downto the groundto get outof the rain. Boom boom boom """ n n The function simply prints out the chorus. Triple-quoted strings can be more than one line long. Lecture #5 Control Structures
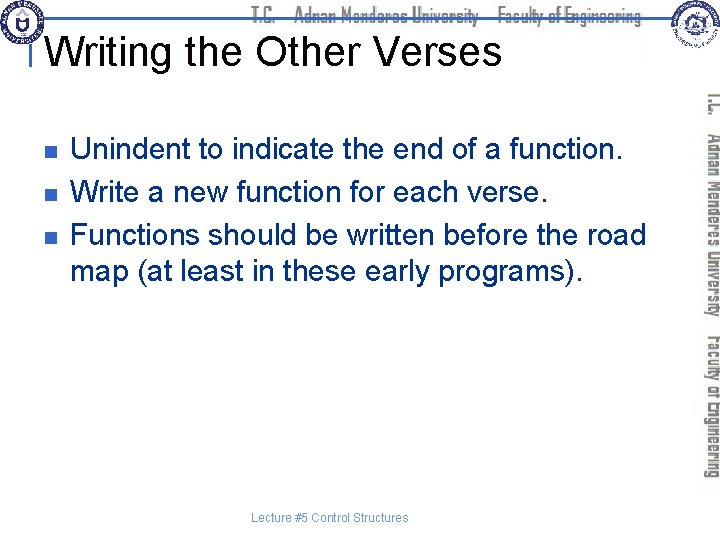
Writing the Other Verses n n n Unindent to indicate the end of a function. Write a new function for each verse. Functions should be written before the road map (at least in these early programs). Lecture #5 Control Structures
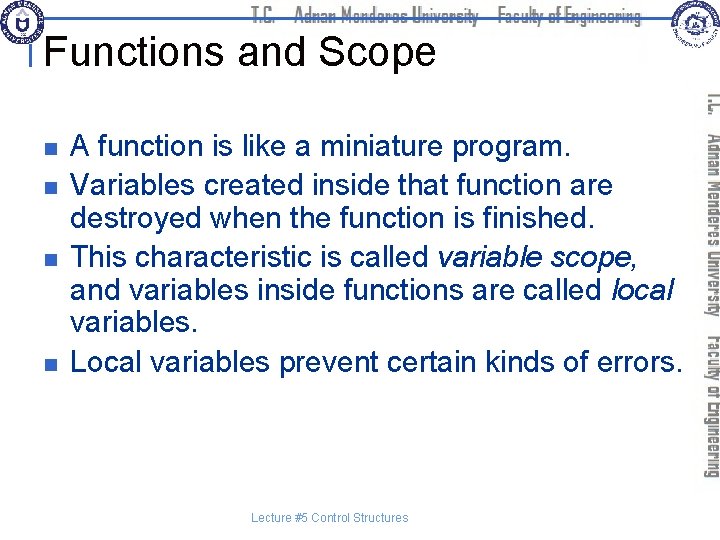
Functions and Scope n n A function is like a miniature program. Variables created inside that function are destroyed when the function is finished. This characteristic is called variable scope, and variables inside functions are called local variables. Local variables prevent certain kinds of errors. Lecture #5 Control Structures
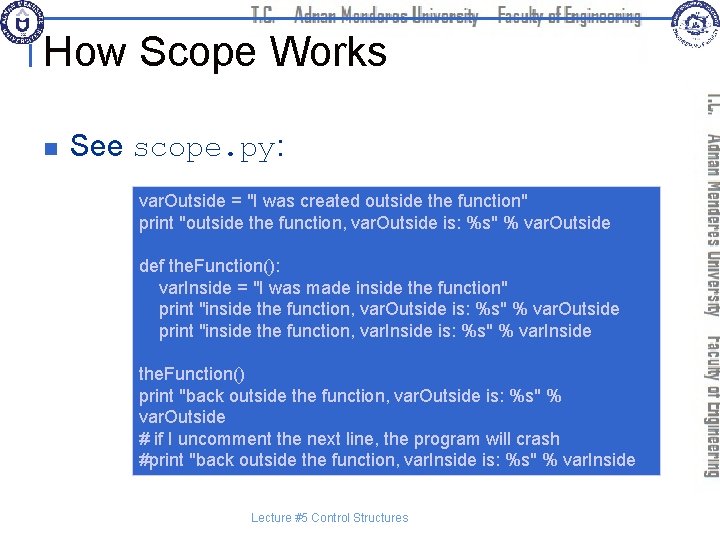
How Scope Works n See scope. py: var. Outside = "I was created outside the function" print "outside the function, var. Outside is: %s" % var. Outside def the. Function(): var. Inside = "I was made inside the function" print "inside the function, var. Outside is: %s" % var. Outside print "inside the function, var. Inside is: %s" % var. Inside the. Function() print "back outside the function, var. Outside is: %s" % var. Outside # if I uncomment the next line, the program will crash #print "back outside the function, var. Inside is: %s" % var. Inside Lecture #5 Control Structures
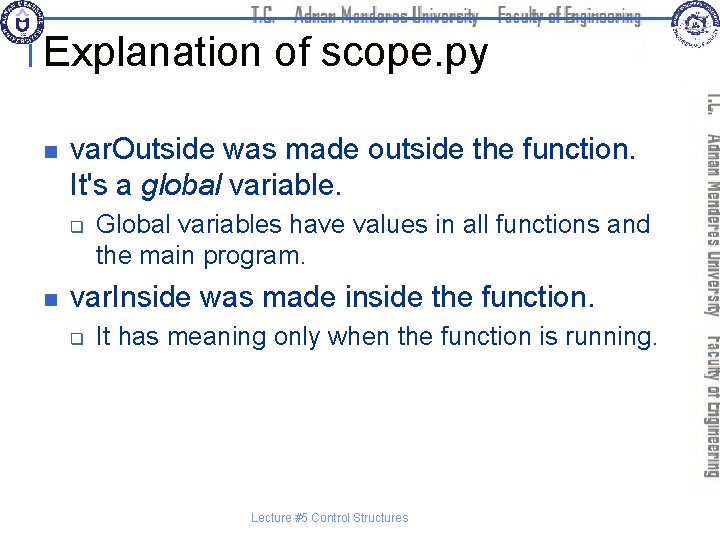
Explanation of scope. py n var. Outside was made outside the function. It's a global variable. q n Global variables have values in all functions and the main program. var. Inside was made inside the function. q It has meaning only when the function is running. Lecture #5 Control Structures
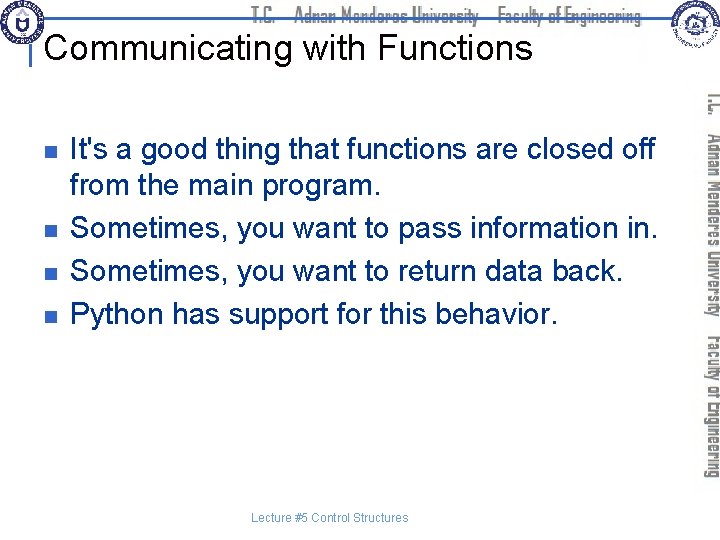
Communicating with Functions n n It's a good thing that functions are closed off from the main program. Sometimes, you want to pass information in. Sometimes, you want to return data back. Python has support for this behavior. Lecture #5 Control Structures
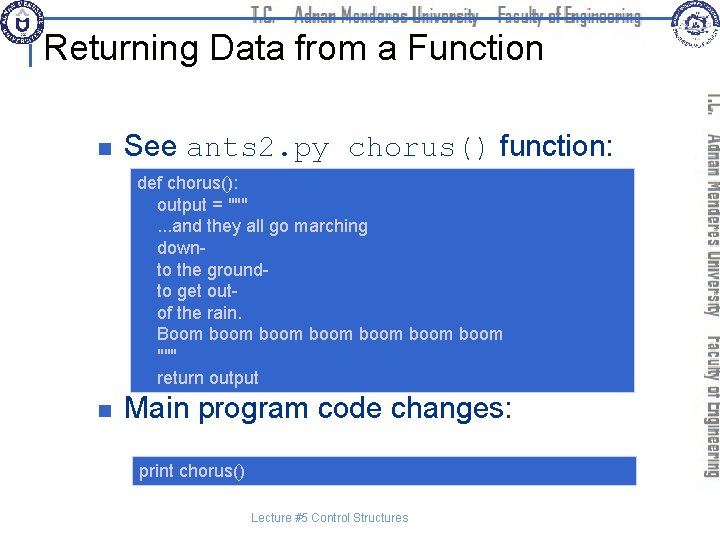
Returning Data from a Function n See ants 2. py chorus() function: def chorus(): output = """. . . and they all go marching downto the groundto get outof the rain. Boom boom boom """ return output n Main program code changes: print chorus() Lecture #5 Control Structures
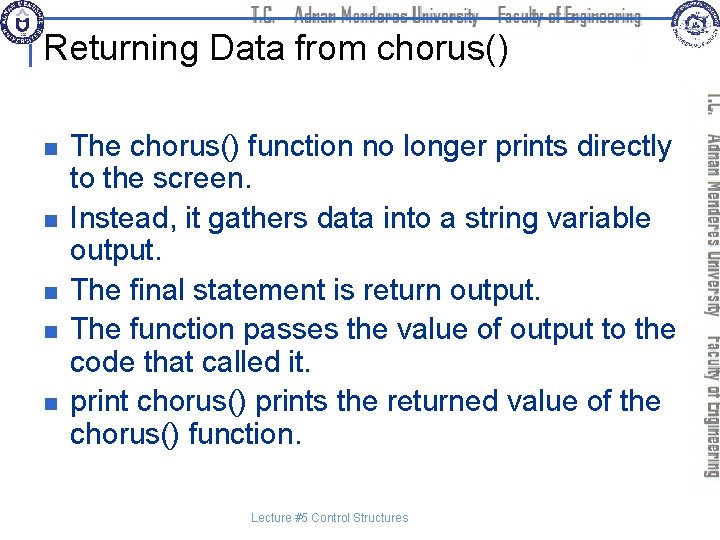
Returning Data from chorus() n n n The chorus() function no longer prints directly to the screen. Instead, it gathers data into a string variable output. The final statement is return output. The function passes the value of output to the code that called it. print chorus() prints the returned value of the chorus() function. Lecture #5 Control Structures
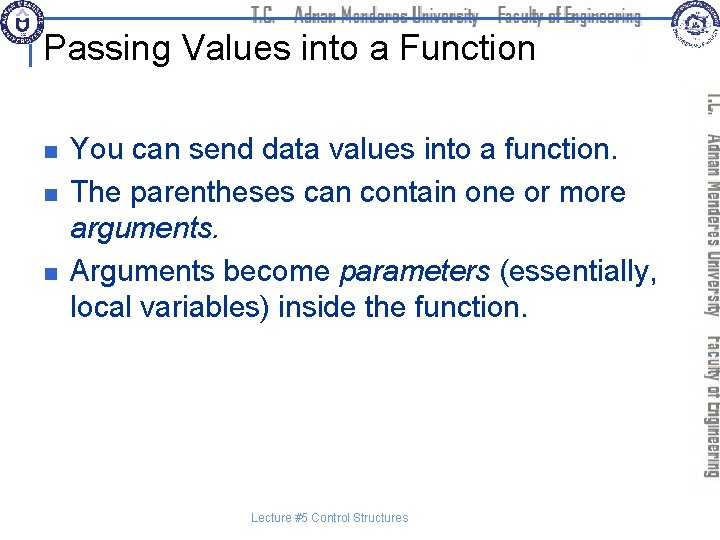
Passing Values into a Function n You can send data values into a function. The parentheses can contain one or more arguments. Arguments become parameters (essentially, local variables) inside the function. Lecture #5 Control Structures
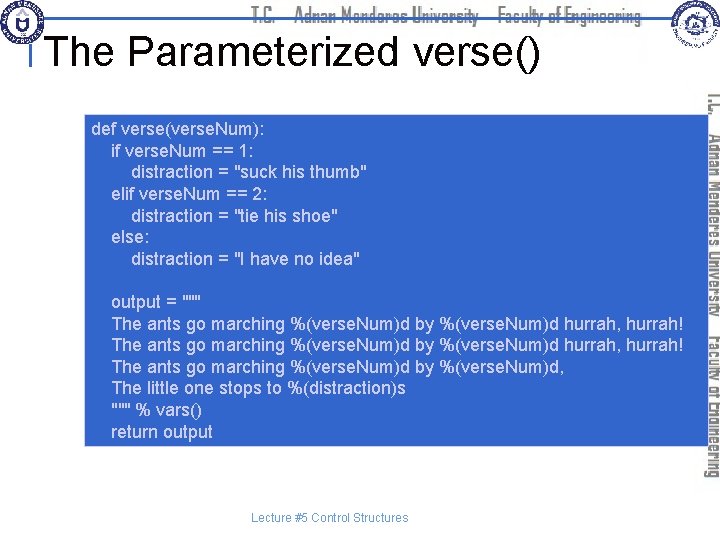
The Parameterized verse() def verse(verse. Num): if verse. Num == 1: distraction = "suck his thumb" elif verse. Num == 2: distraction = "tie his shoe" else: distraction = "I have no idea" output = """ The ants go marching %(verse. Num)d by %(verse. Num)d hurrah, hurrah! The ants go marching %(verse. Num)d by %(verse. Num)d, The little one stops to %(distraction)s """ % vars() return output Lecture #5 Control Structures
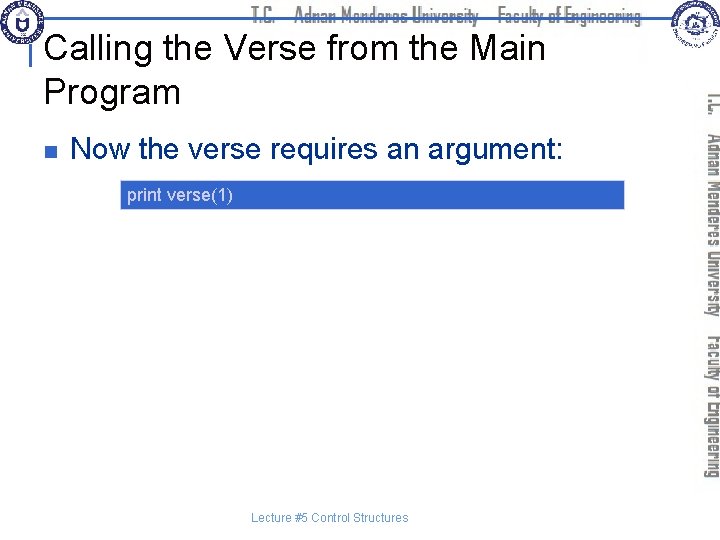
Calling the Verse from the Main Program n Now the verse requires an argument: print verse(1) Lecture #5 Control Structures
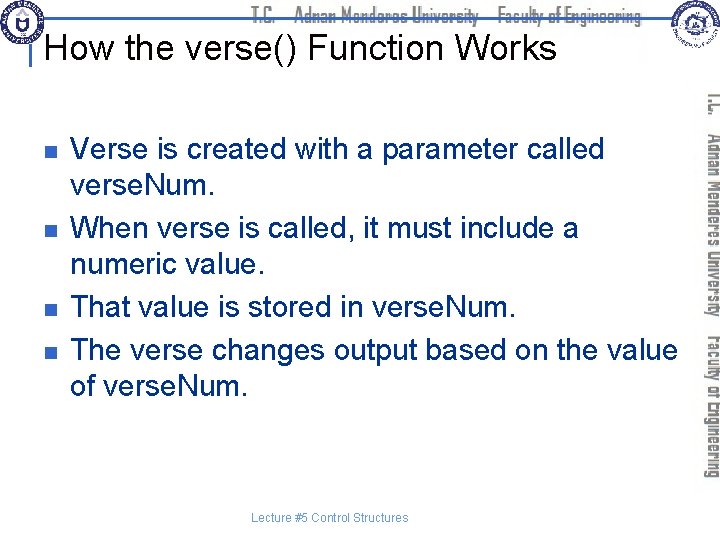
How the verse() Function Works n n Verse is created with a parameter called verse. Num. When verse is called, it must include a numeric value. That value is stored in verse. Num. The verse changes output based on the value of verse. Num. Lecture #5 Control Structures
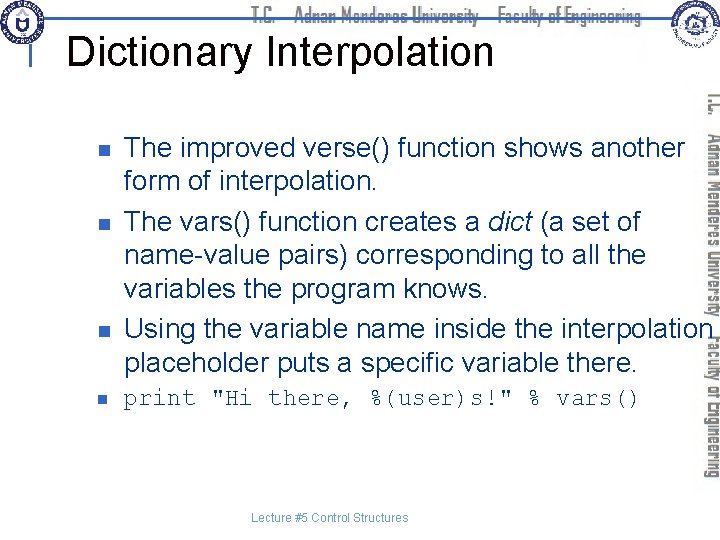
Dictionary Interpolation n n The improved verse() function shows another form of interpolation. The vars() function creates a dict (a set of name-value pairs) corresponding to all the variables the program knows. Using the variable name inside the interpolation placeholder puts a specific variable there. print "Hi there, %(user)s!" % vars() Lecture #5 Control Structures
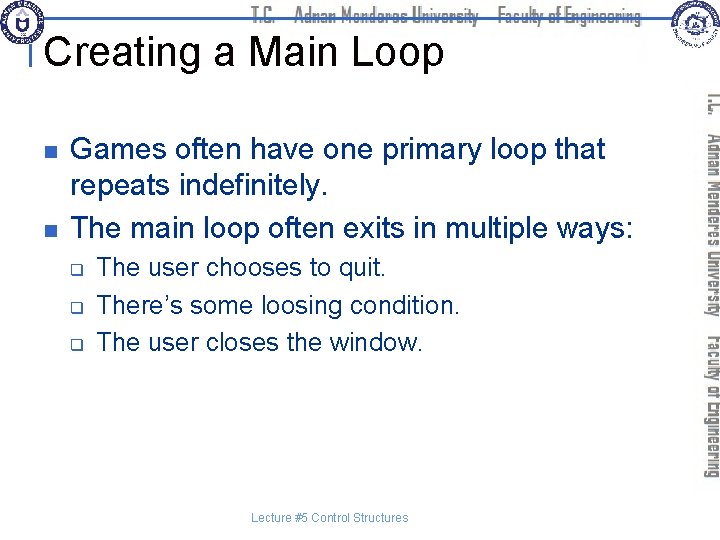
Creating a Main Loop n n Games often have one primary loop that repeats indefinitely. The main loop often exits in multiple ways: q q q The user chooses to quit. There’s some loosing condition. The user closes the window. Lecture #5 Control Structures
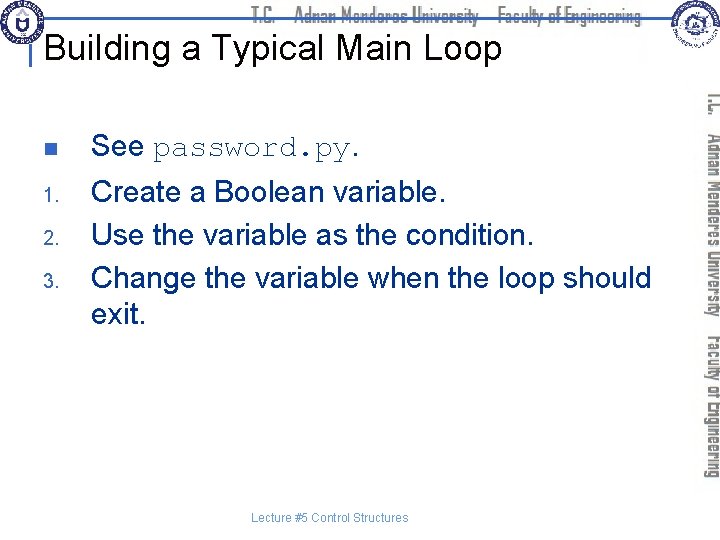
Building a Typical Main Loop n See password. py. 1. Create a Boolean variable. Use the variable as the condition. Change the variable when the loop should exit. 2. 3. Lecture #5 Control Structures
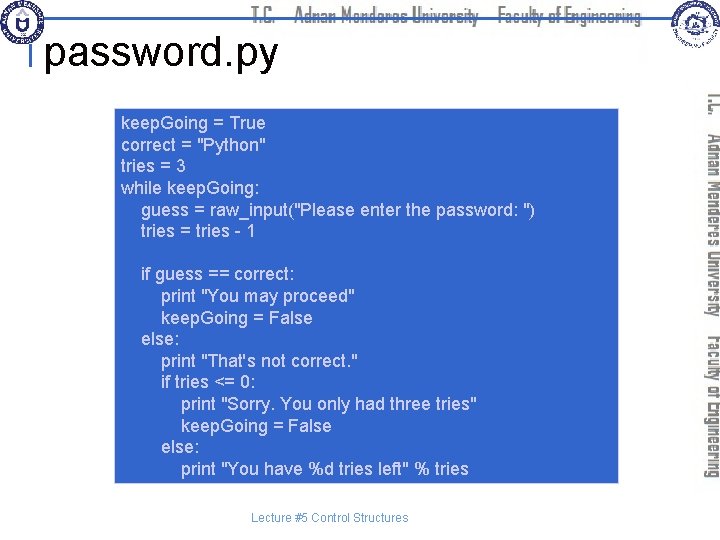
password. py keep. Going = True correct = "Python" tries = 3 while keep. Going: guess = raw_input("Please enter the password: ") tries = tries - 1 if guess == correct: print "You may proceed" keep. Going = False else: print "That's not correct. " if tries <= 0: print "Sorry. You only had three tries" keep. Going = False else: print "You have %d tries left" % tries Lecture #5 Control Structures
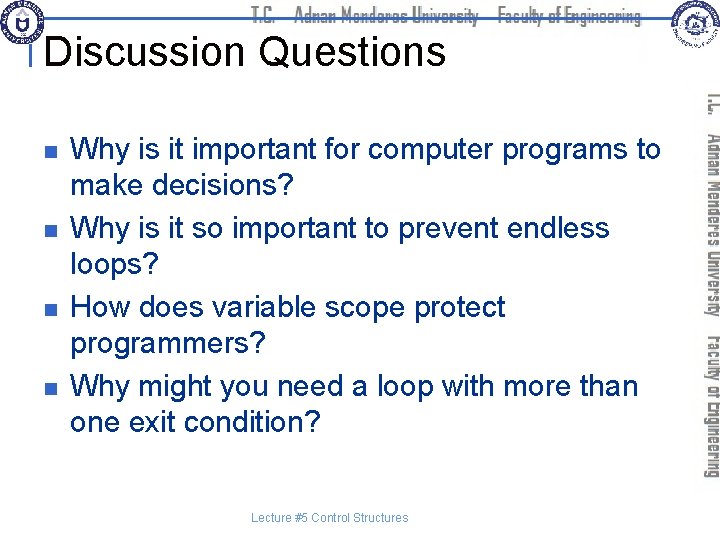
Discussion Questions n n Why is it important for computer programs to make decisions? Why is it so important to prevent endless loops? How does variable scope protect programmers? Why might you need a loop with more than one exit condition? Lecture #5 Control Structures
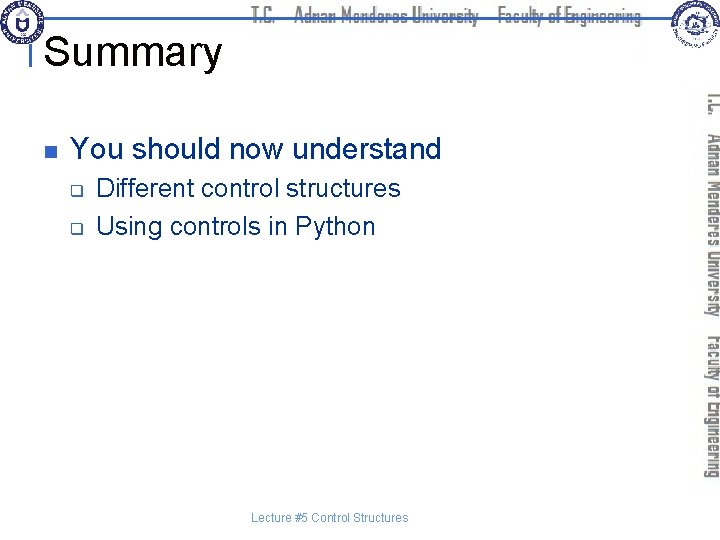
Summary n You should now understand q q Different control structures Using controls in Python Lecture #5 Control Structures
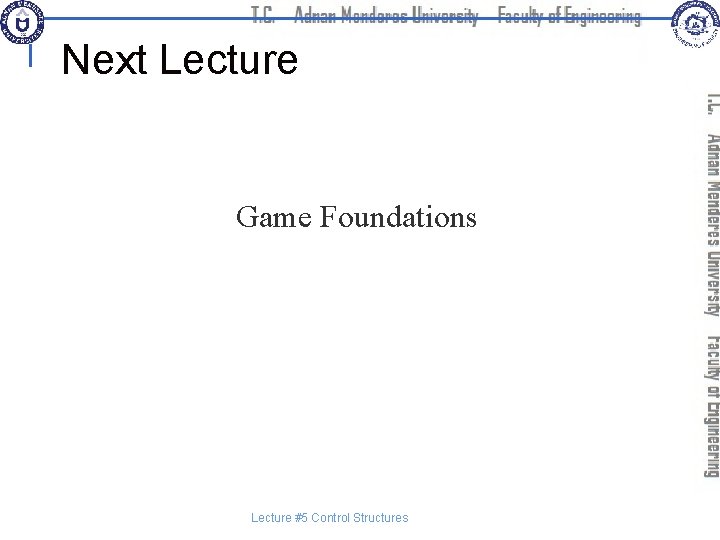
Next Lecture Game Foundations Lecture #5 Control Structures
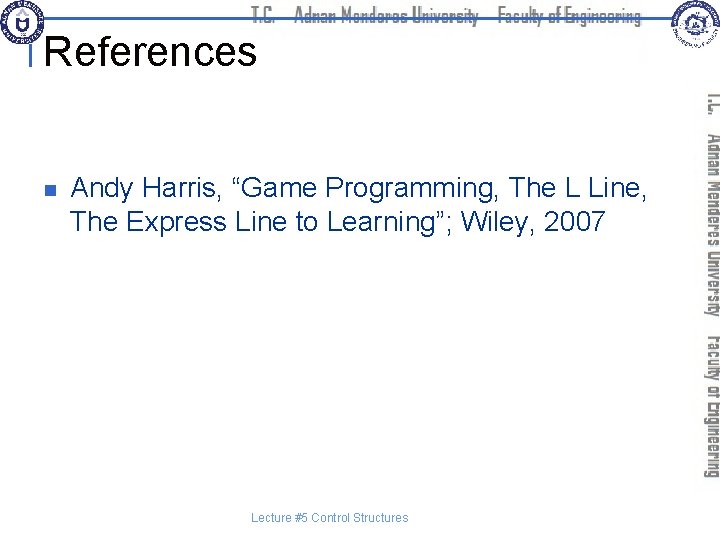
References n Andy Harris, “Game Programming, The L Line, The Express Line to Learning”; Wiley, 2007 Lecture #5 Control Structures
01:640:244 lecture notes - lecture 15: plat, idah, farad
Hunger games chapter 9 questions and answers
Outdoor games and indoor games
A plain scale of 1cm=5m and show on it 37m
Computer security 161 cryptocurrency lecture
Computer aided drug design lecture notes
Computer architecture lecture notes
Isa definition computer
Mini metric olympics
Artigo 420 do codigo civil
Major minor patch build
5x5^2 in index form
56 as a product of prime factors
Definition of
Doe order 420
Enve420
Rosenberger hvs420
Amg 420 discontinued
S420 class
Index currency option notes
Dme #7 steel
Thursday420
Med 420
Mis-420
420 internet marketing
Envee 420
Enve420
Enve420
Enve420
Notice of confidential info rule 2-420
Resolucion 420/2011
Drift time 420
420 marketing campaigns
Marginal distribution
Article 420
420 position
Zebra rw 420
Geo
Envee 420
Cpsc 420
420 ucas points
420 pst
260 in word form
Nasvac