Intro Csp Tirgul 6 Command Line Arguments The
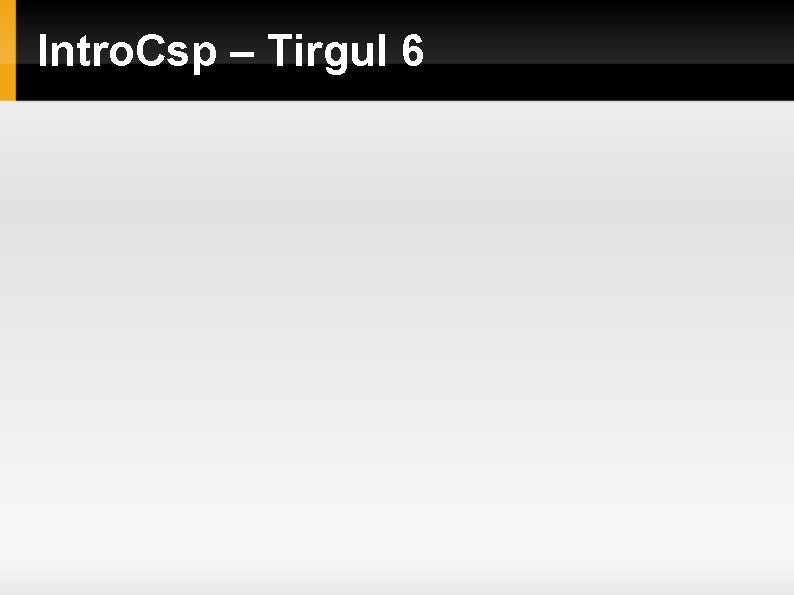
![Command Line Arguments The main method takes a String[] as a parameter. public static Command Line Arguments The main method takes a String[] as a parameter. public static](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-2.jpg)
![Example: public class Hello. Command. Line { public static void main(String[] args){ System. out. Example: public class Hello. Command. Line { public static void main(String[] args){ System. out.](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-3.jpg)
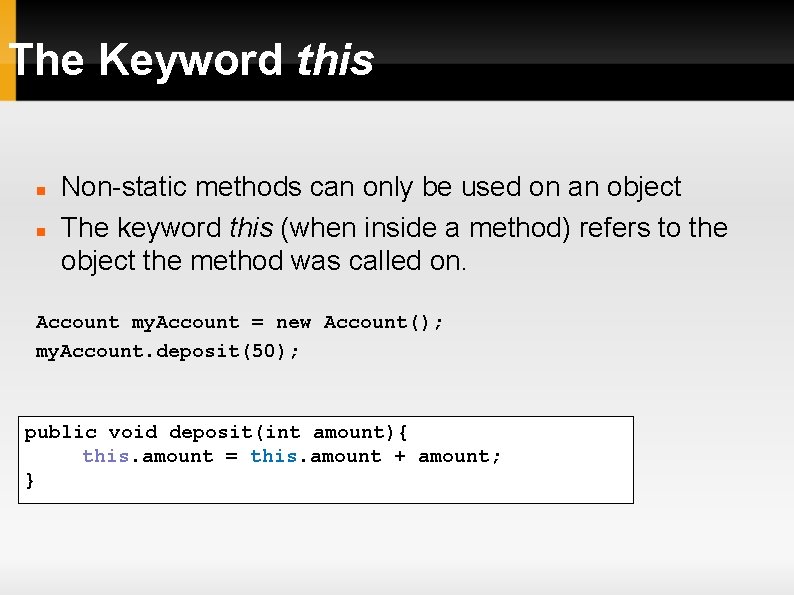
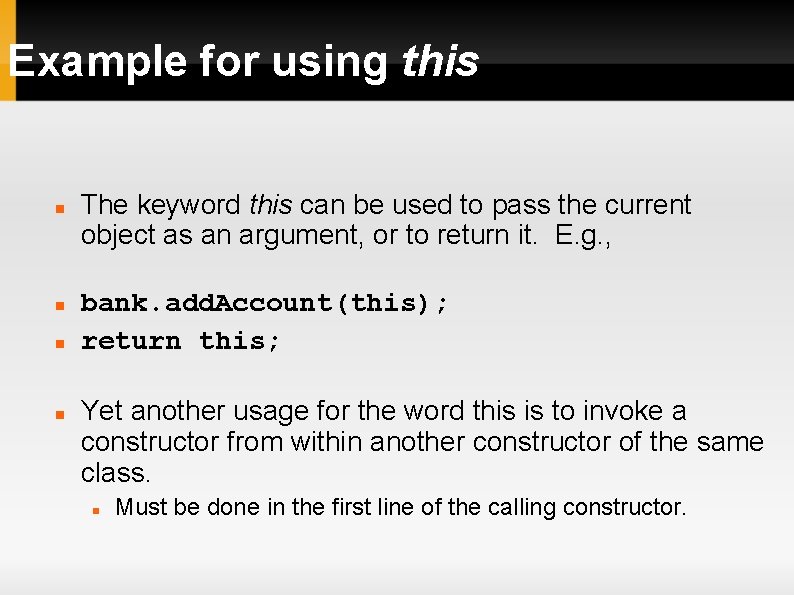
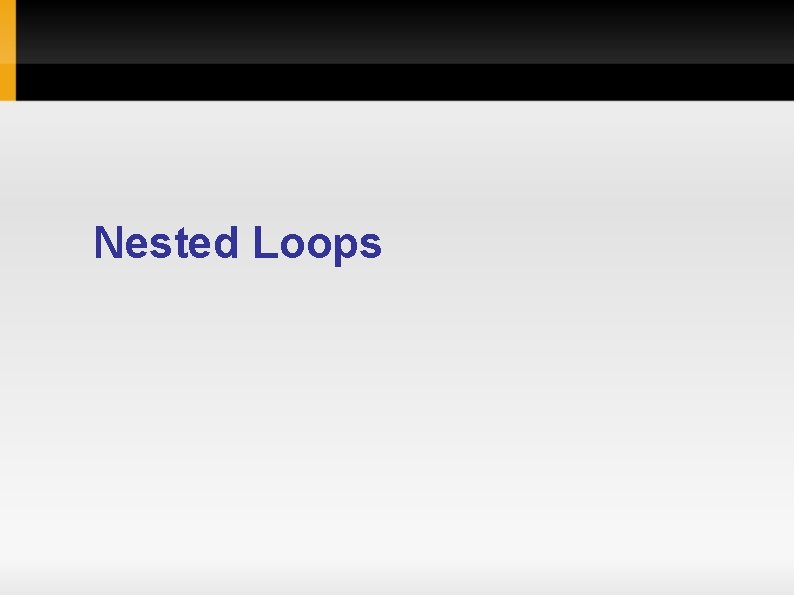
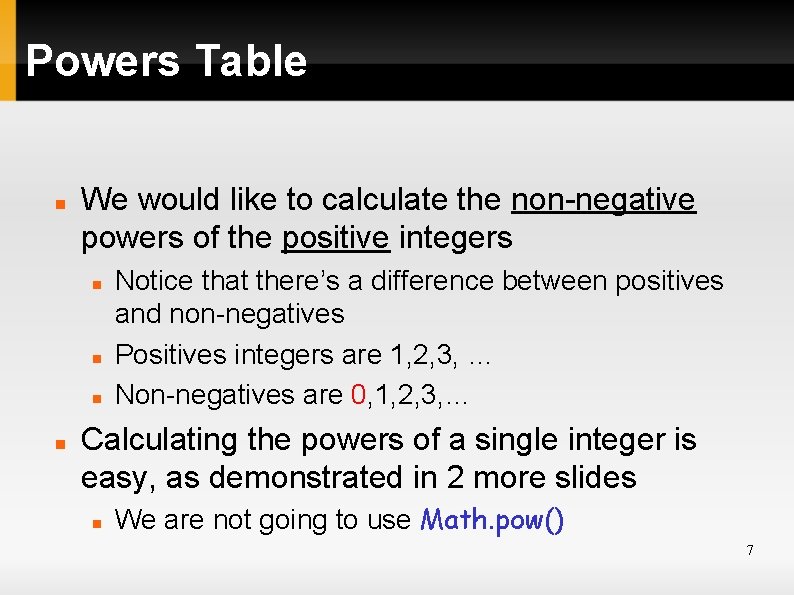
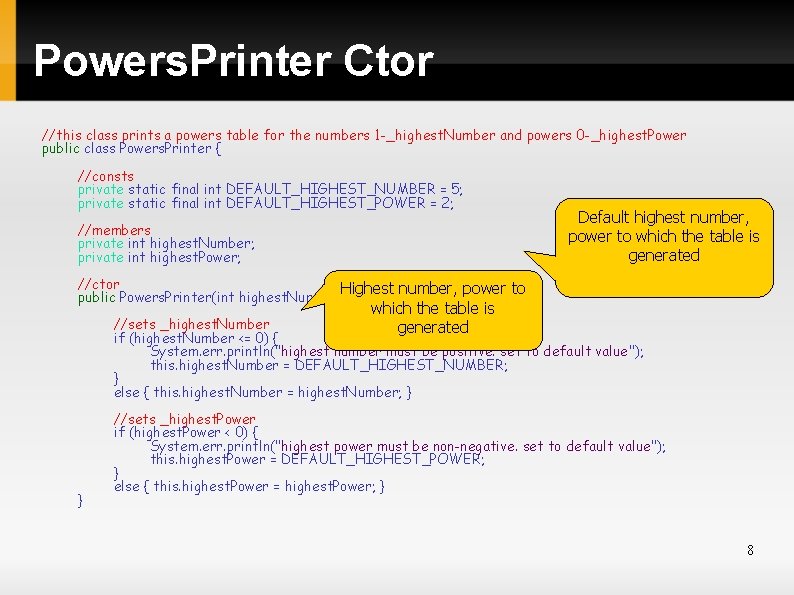
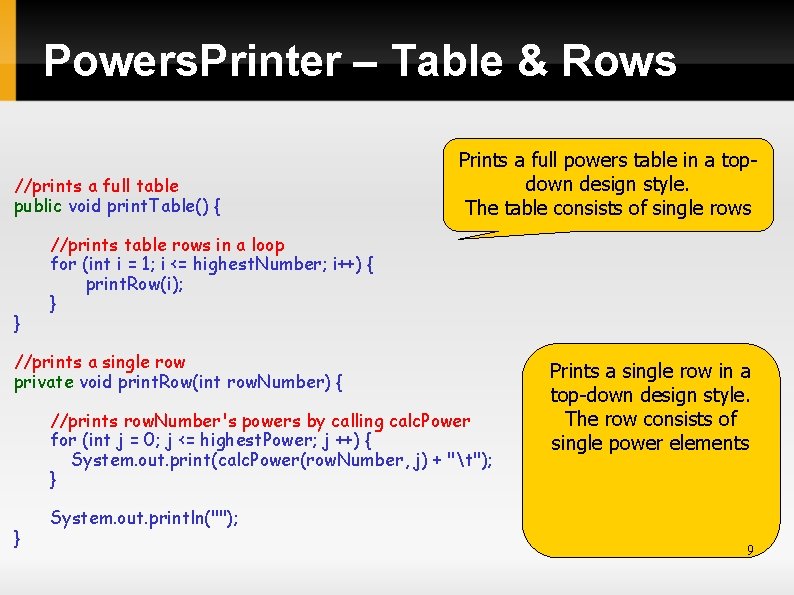
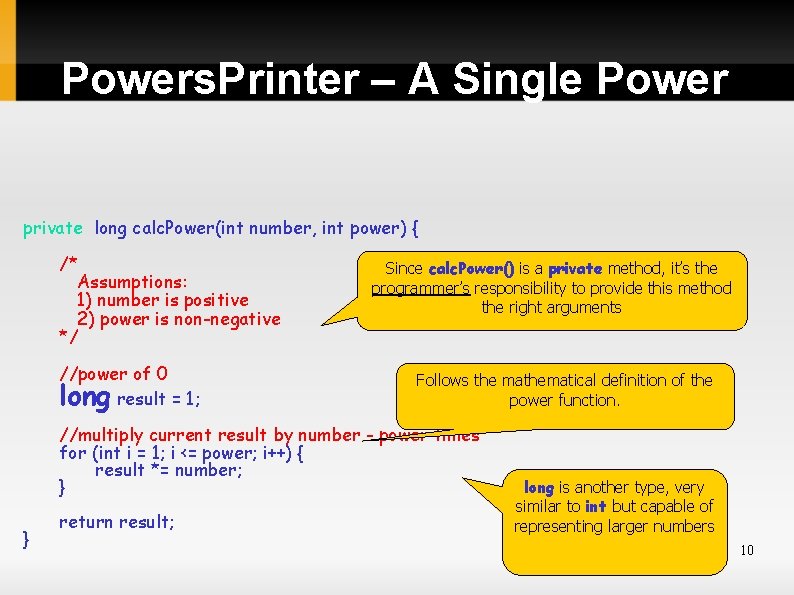
![Powers. Printer – Main public static void main(String[] argv) { argv is a Strings Powers. Printer – Main public static void main(String[] argv) { argv is a Strings](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-11.jpg)
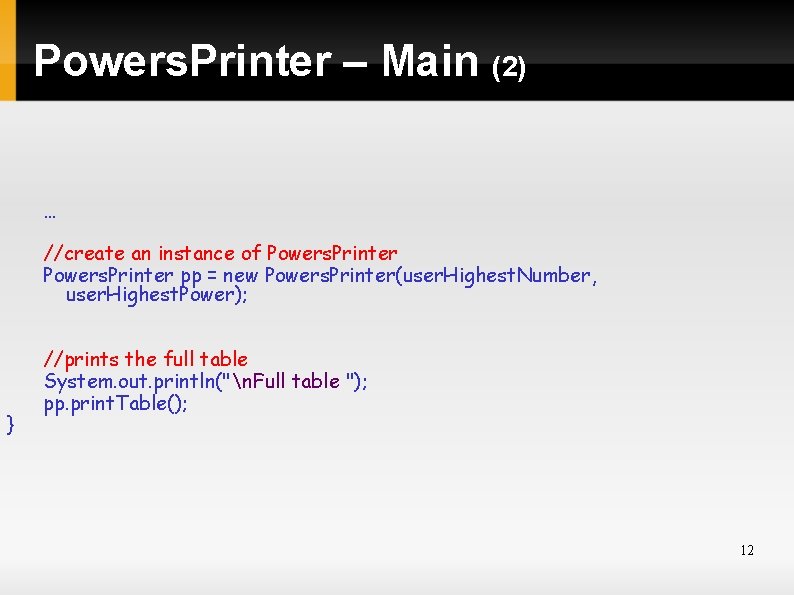
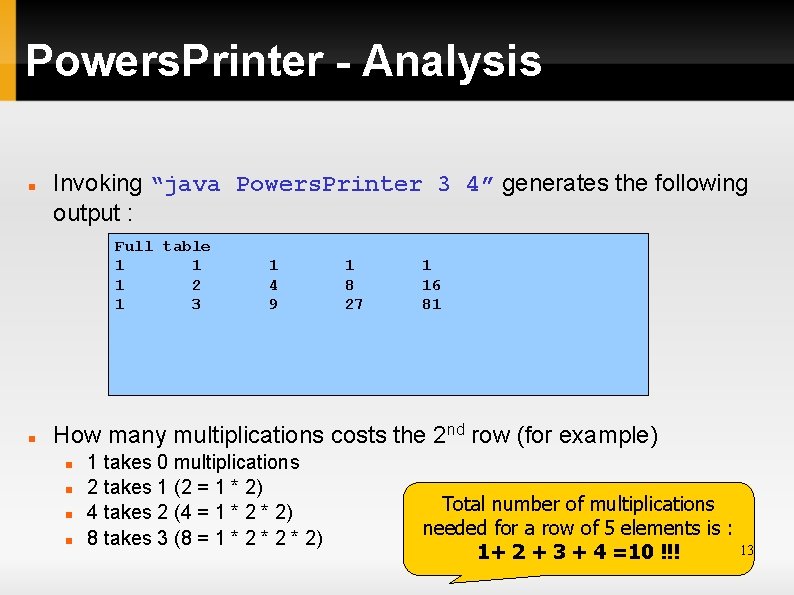
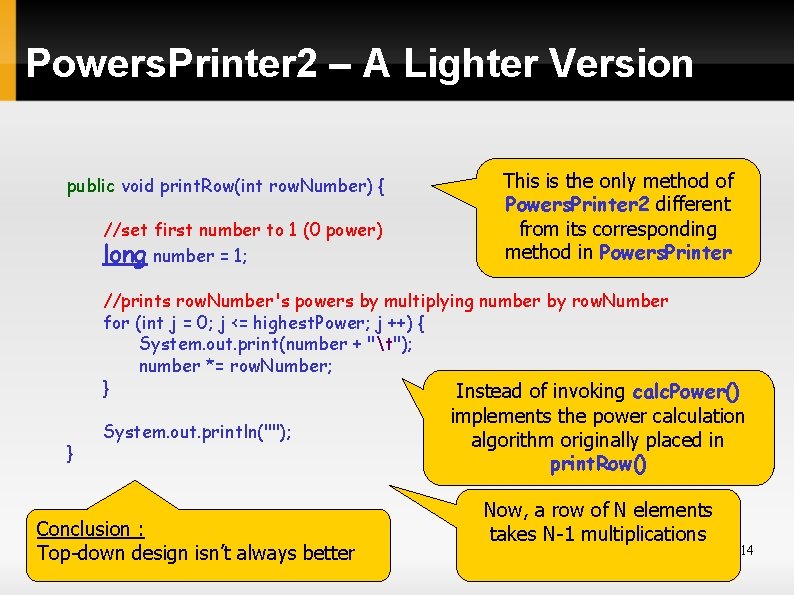
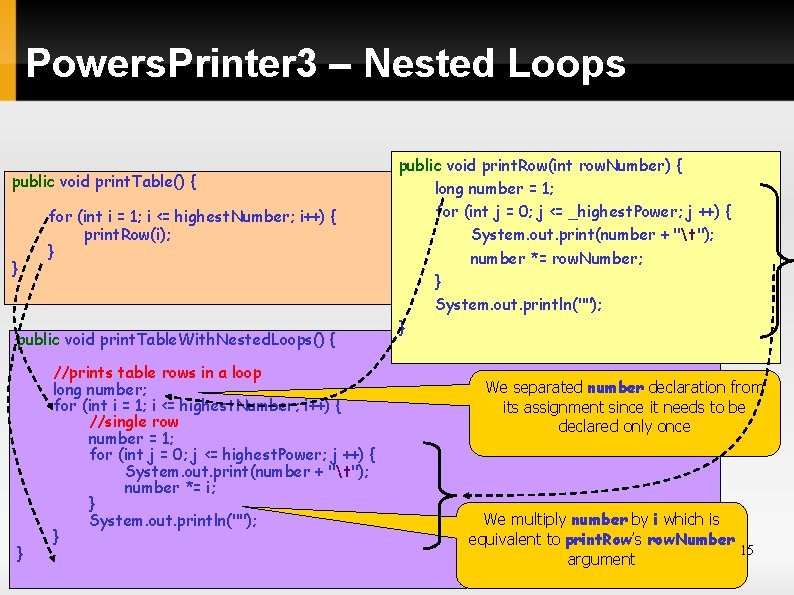
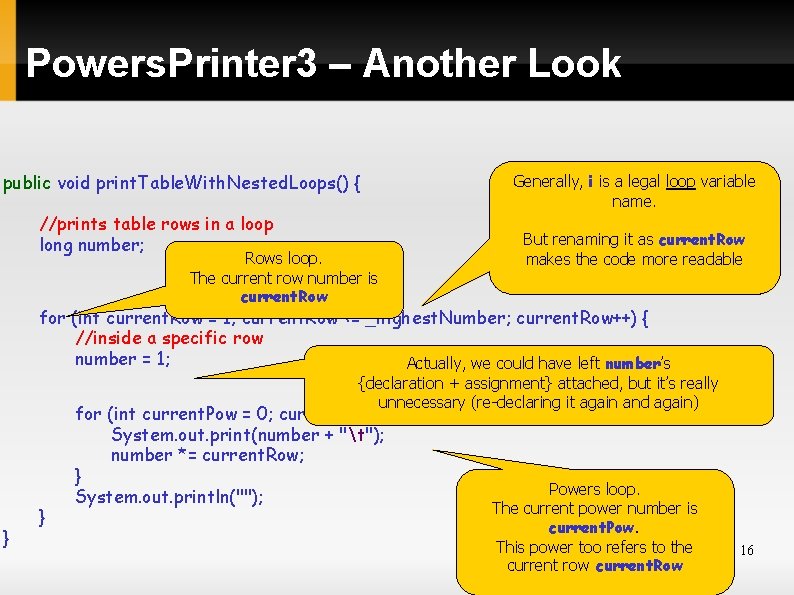
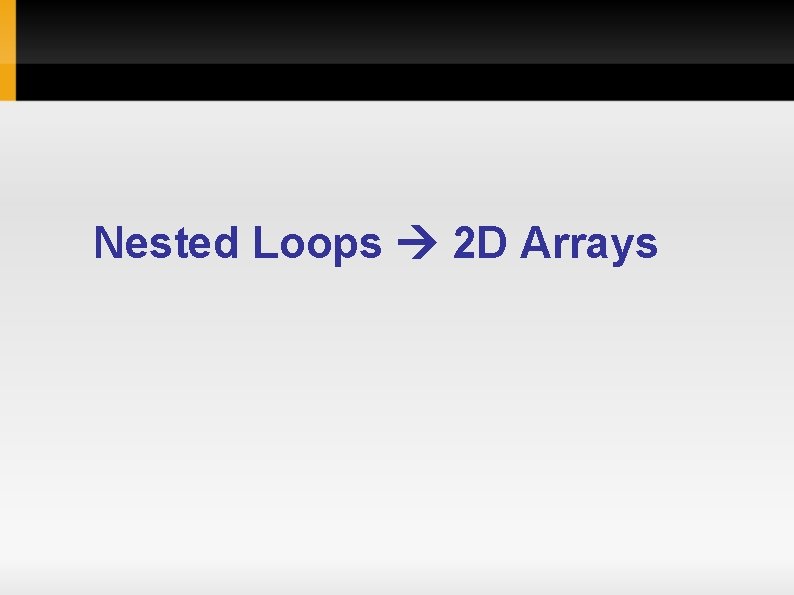
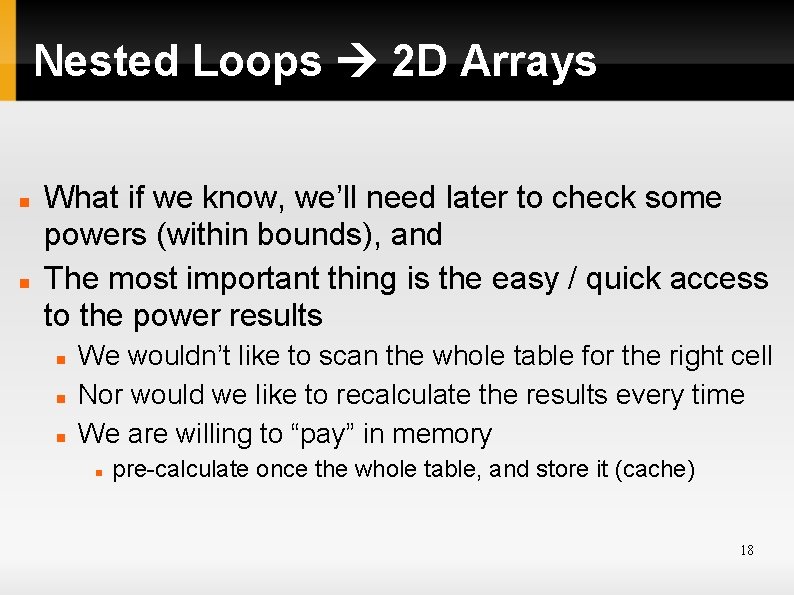
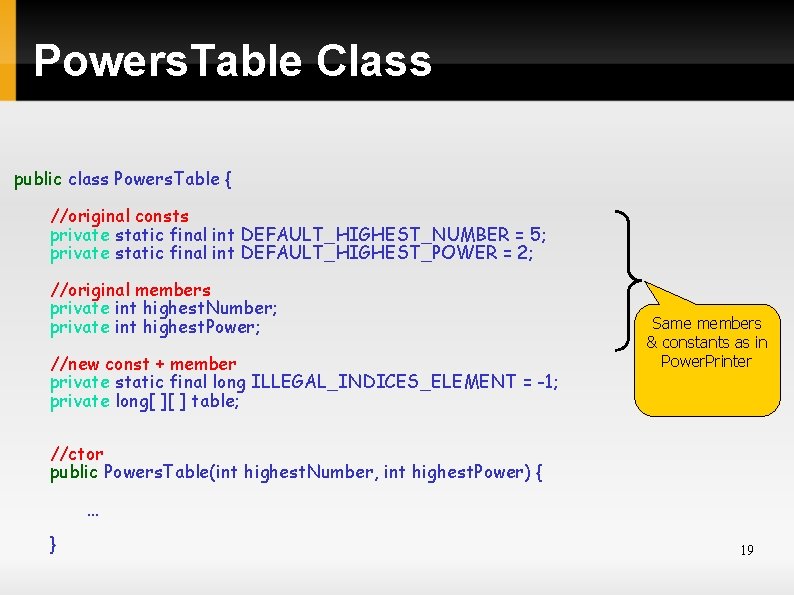
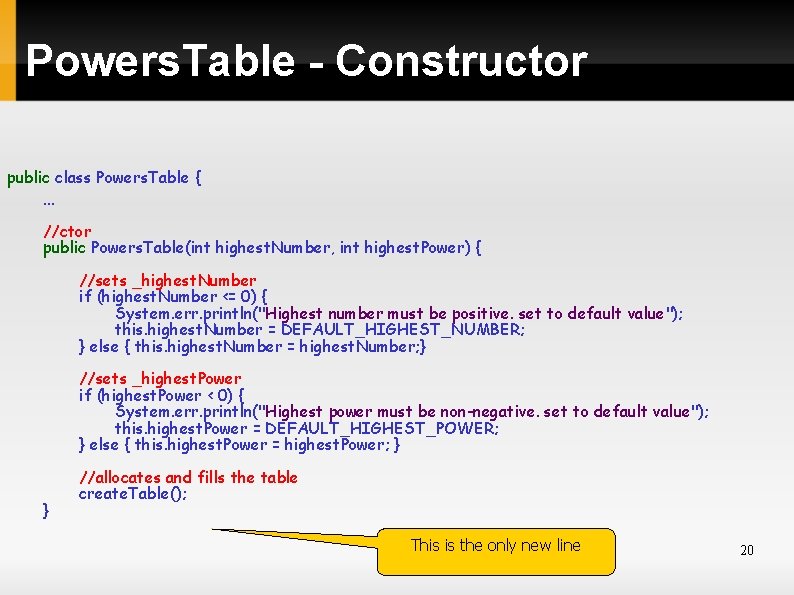
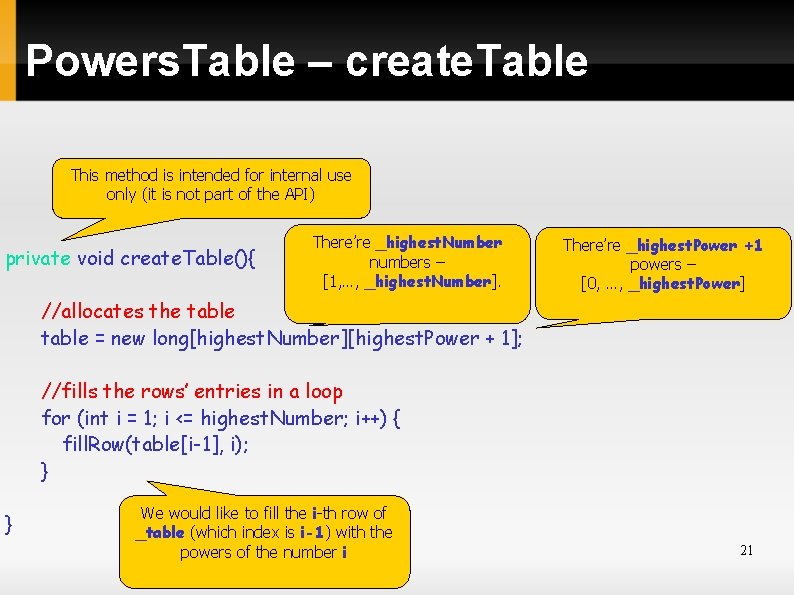
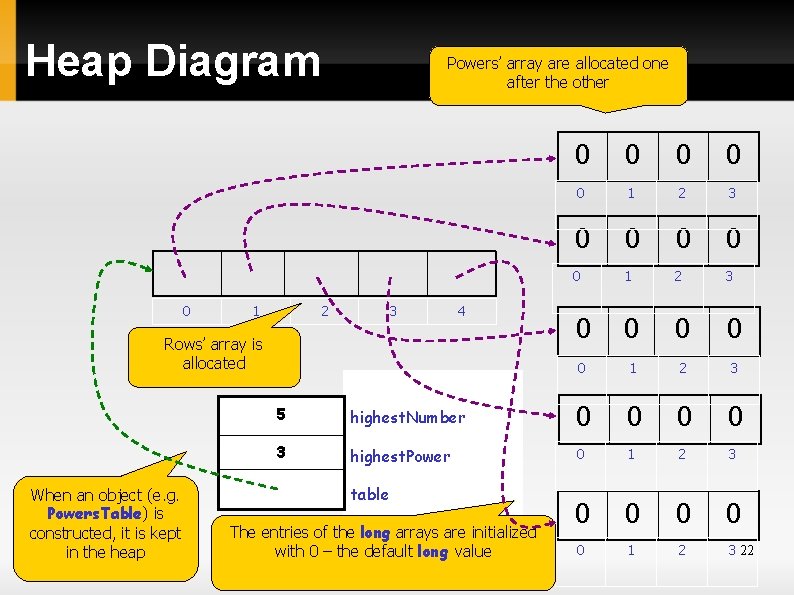
![Powers. Table - fill. Row private void fill. Row(long[] row, int row. Number) { Powers. Table - fill. Row private void fill. Row(long[] row, int row. Number) {](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-23.jpg)
![What Just Happened Here? table is created in create. Table table[i-1] is sent as What Just Happened Here? table is created in create. Table table[i-1] is sent as](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-24.jpg)
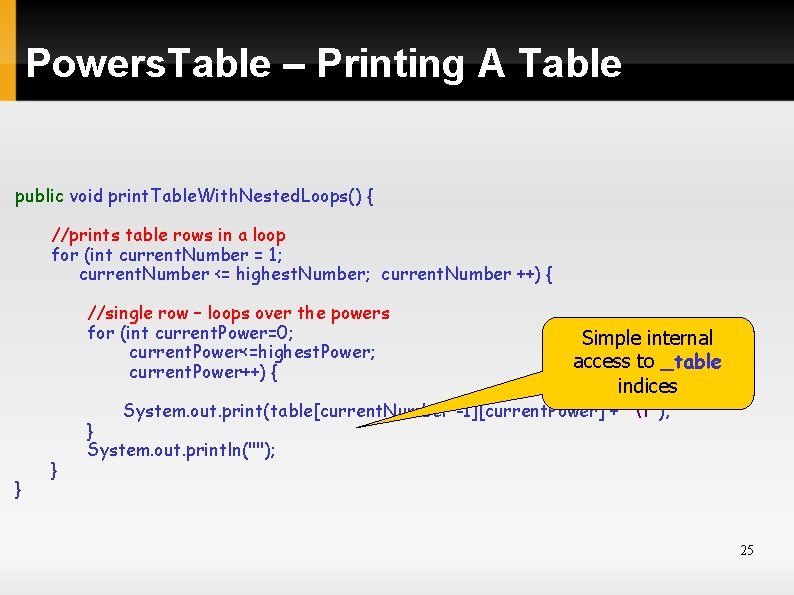
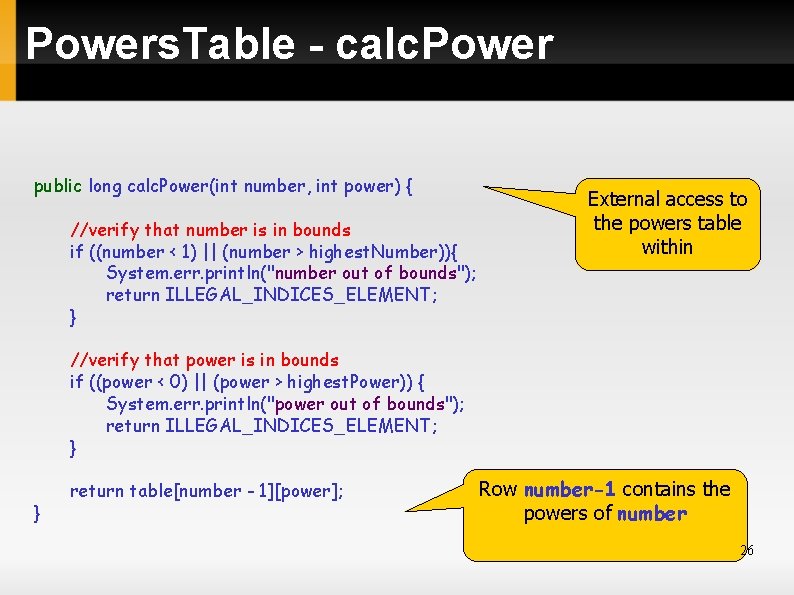
![Powers. Table - Main public static void main(String[] argv) { //verify that 2 arguments Powers. Table - Main public static void main(String[] argv) { //verify that 2 arguments](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-27.jpg)
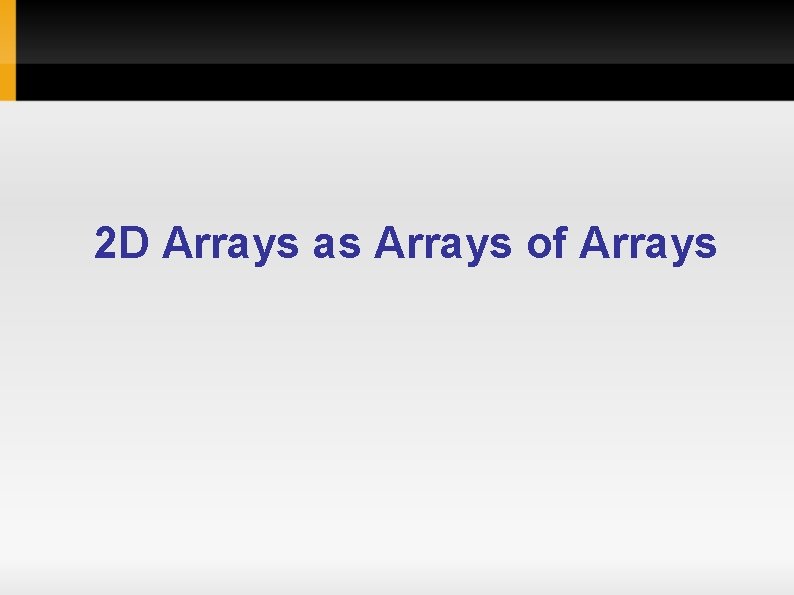
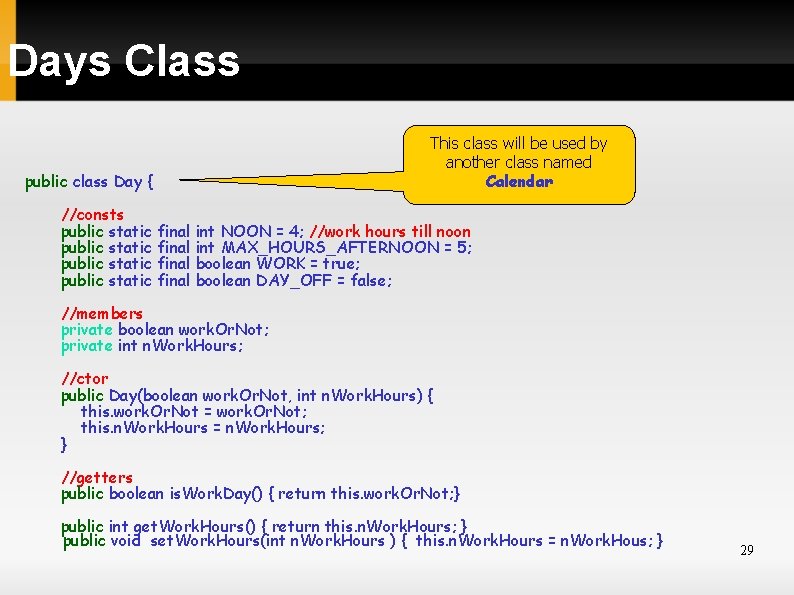
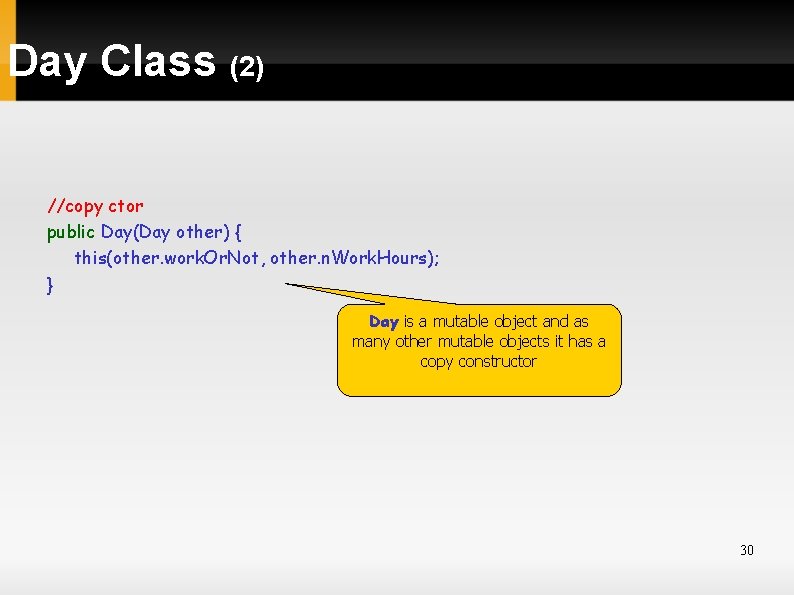
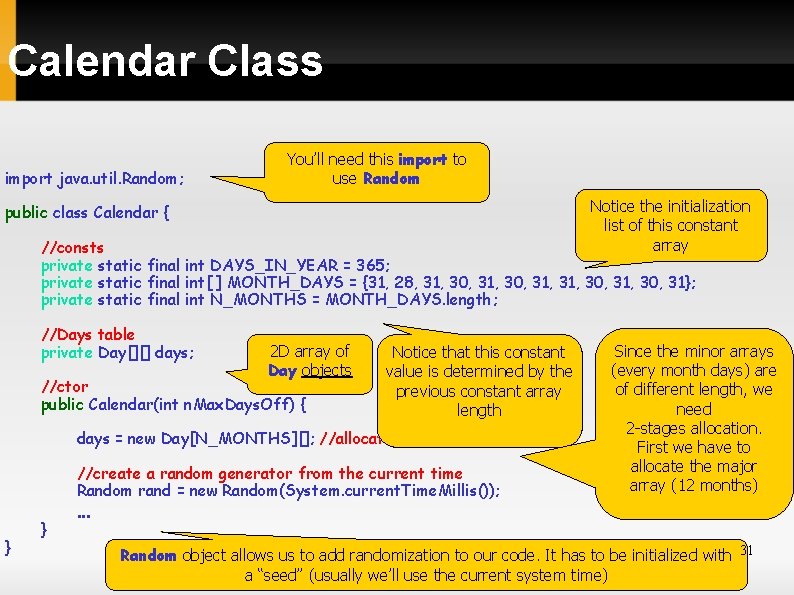
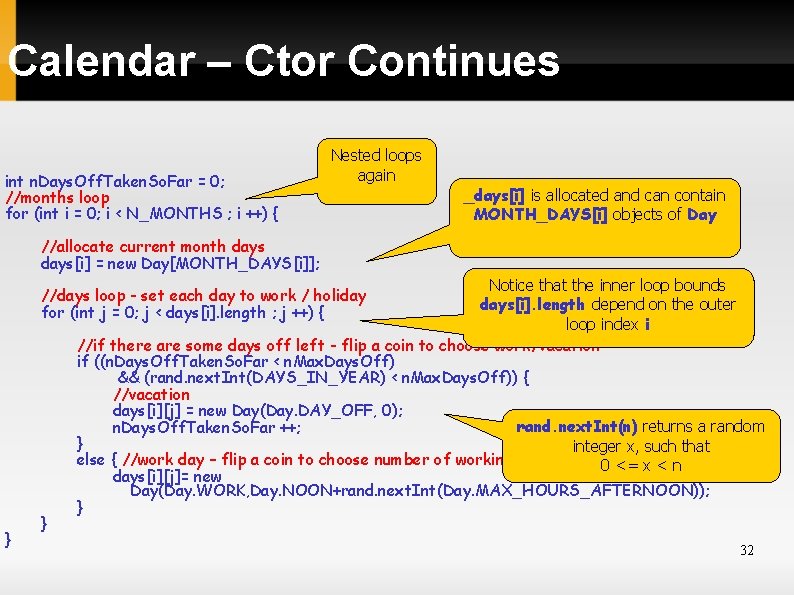
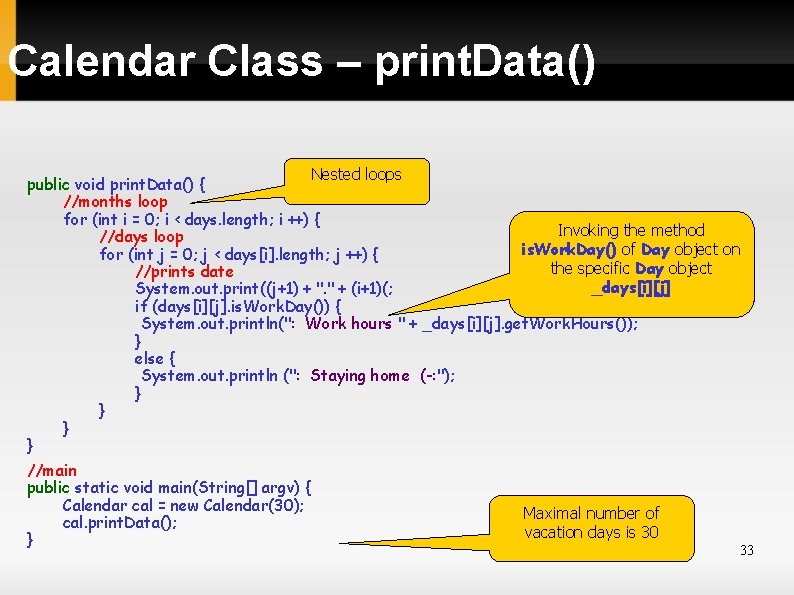
![Calendar - get. Calendar public Day[][] get. Calendar() { return days; } This method Calendar - get. Calendar public Day[][] get. Calendar() { return days; } This method](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-34.jpg)
![Calendar - get. Calendar public Day[][] get. Calendar() { //allocates major array (months) Day[][] Calendar - get. Calendar public Day[][] get. Calendar() { //allocates major array (months) Day[][]](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-35.jpg)
![Heap Diagram Arrays for days of each month are allocated days[1] 0 0 0 Heap Diagram Arrays for days of each month are allocated days[1] 0 0 0](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-36.jpg)
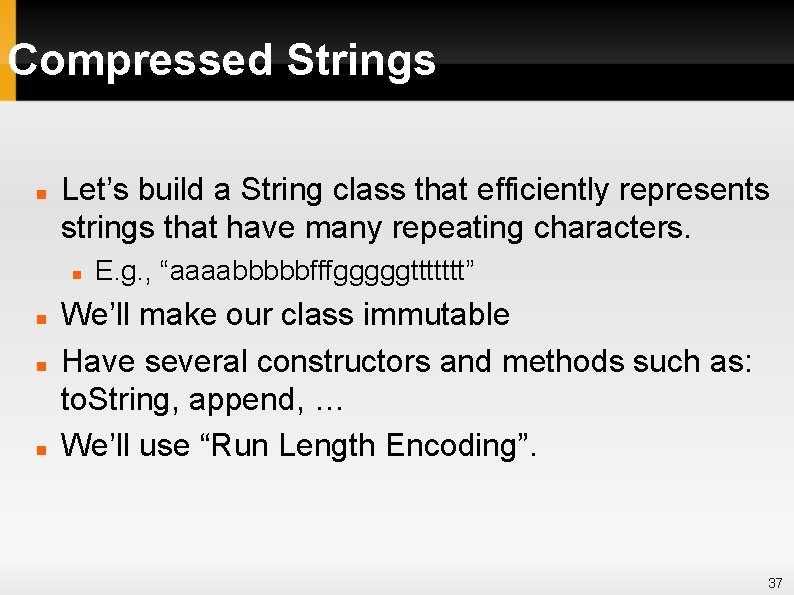
![Compressed Strings public class Compressed. String { private char[] chars; private int[] freq; Will Compressed Strings public class Compressed. String { private char[] chars; private int[] freq; Will](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-38.jpg)
![Compressed Strings – The First Constructor public Compressed. String(char[] string){ int compressed. Length=string. length; Compressed Strings – The First Constructor public Compressed. String(char[] string){ int compressed. Length=string. length;](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-39.jpg)
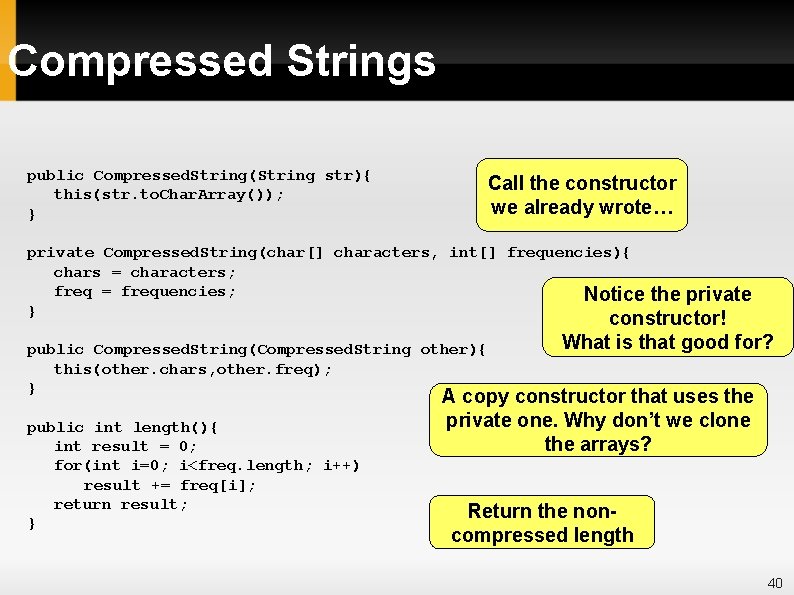
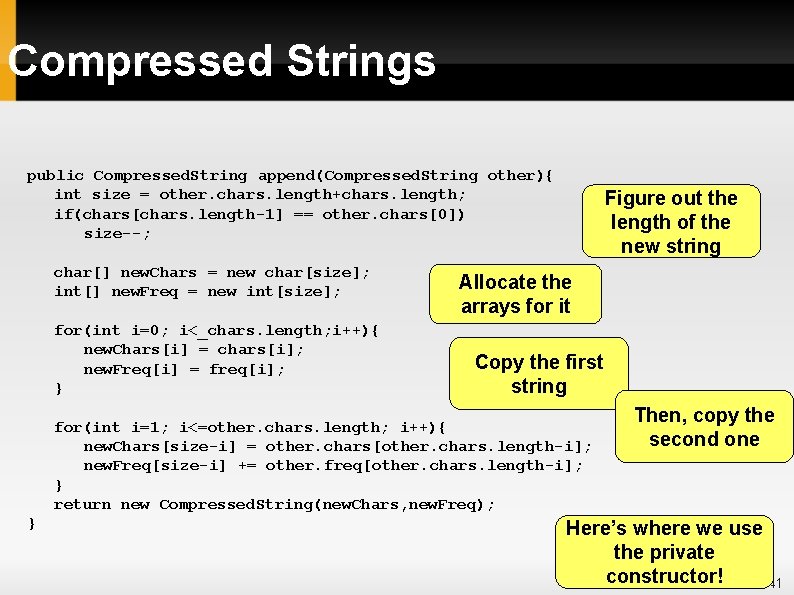
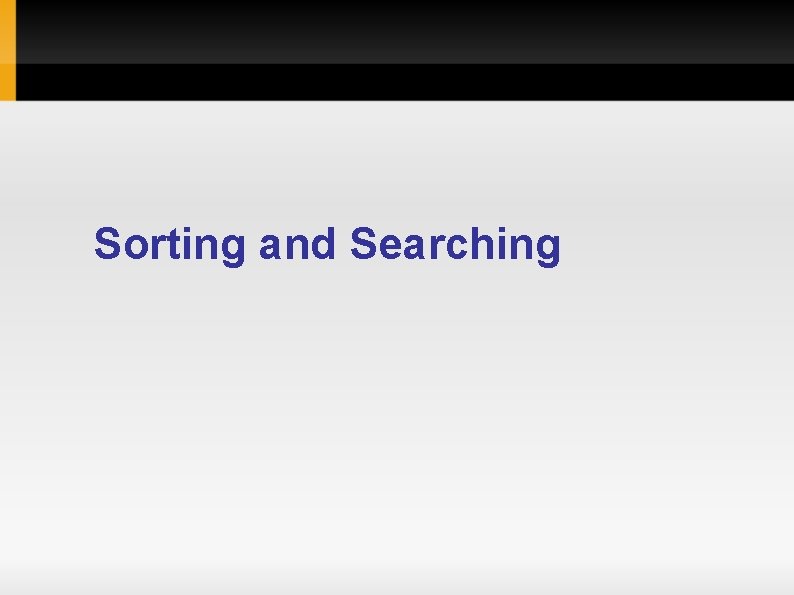
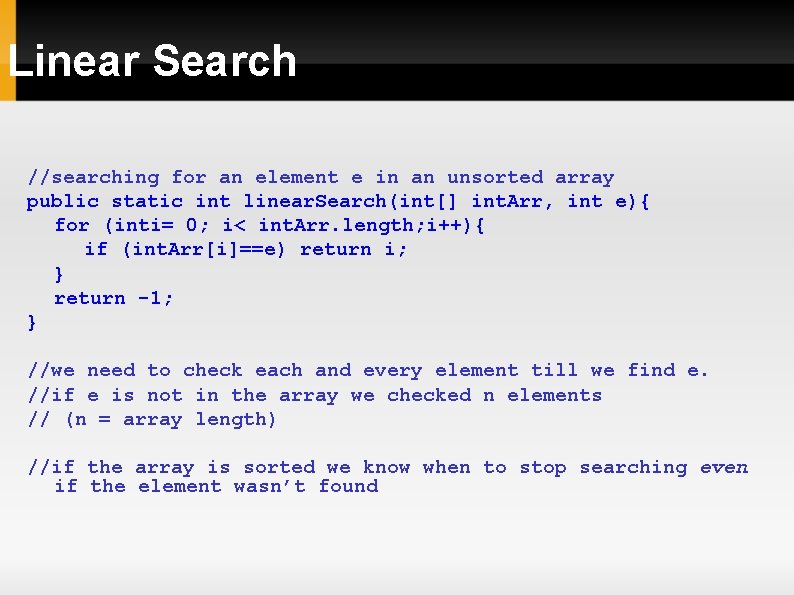
![Linear search in a sorted array public static int linear. Search(int[] int. Arr, int Linear search in a sorted array public static int linear. Search(int[] int. Arr, int](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-44.jpg)
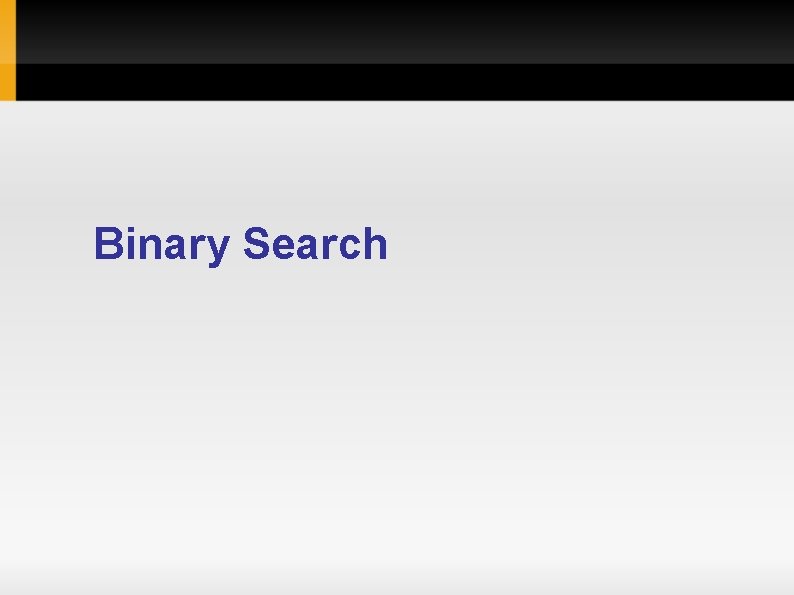
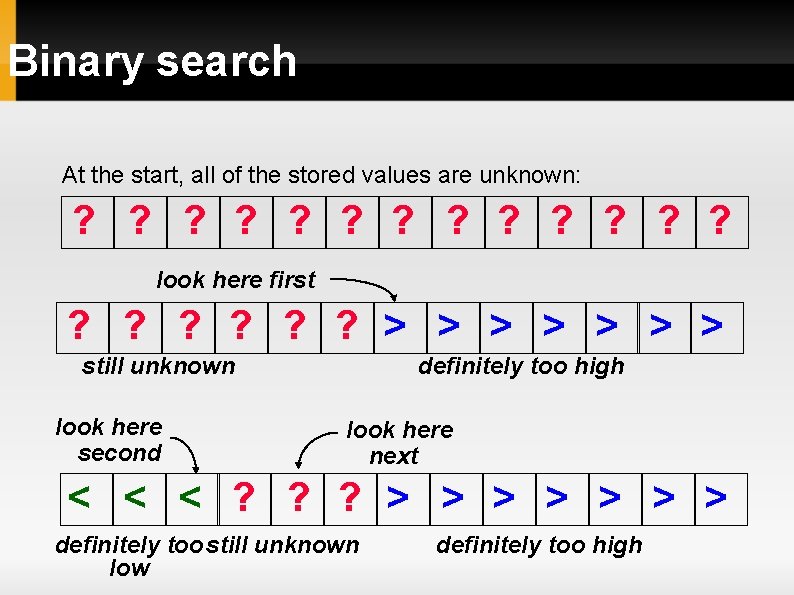
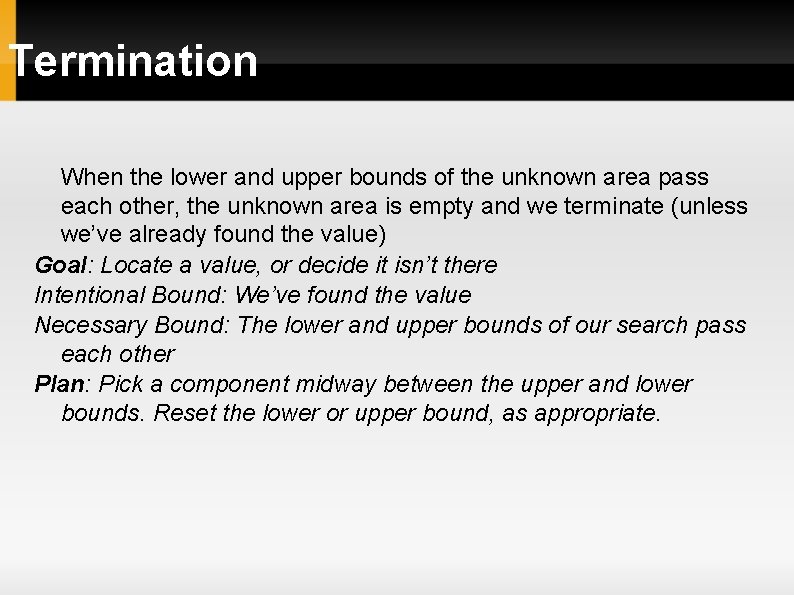
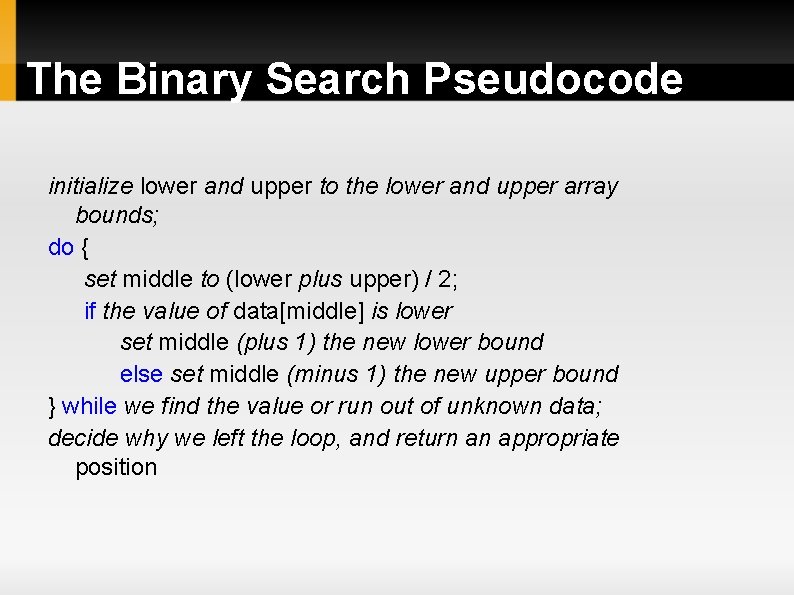
![The Binary Search Java Code int binary. Search (int[ ] data, int num) { The Binary Search Java Code int binary. Search (int[ ] data, int num) {](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-49.jpg)
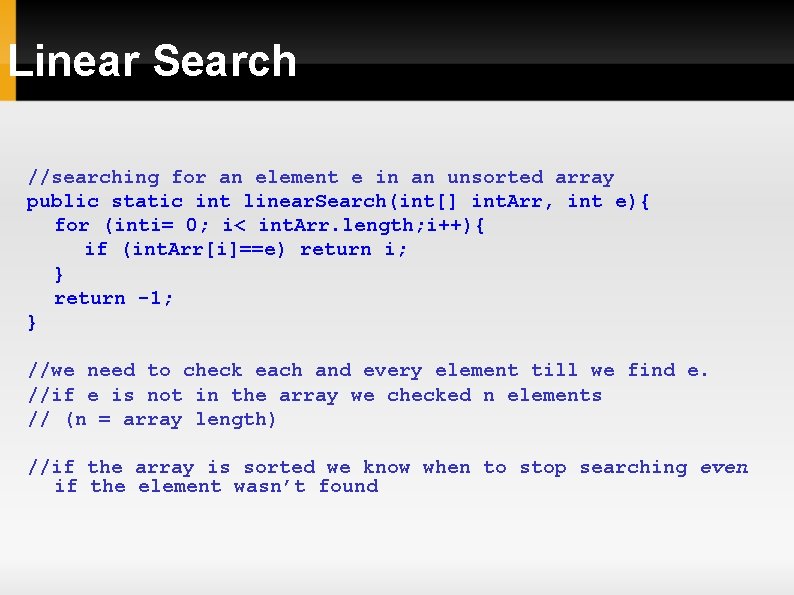
![Linear search in a sorted array public static int linear. Search(int[] int. Arr, int Linear search in a sorted array public static int linear. Search(int[] int. Arr, int](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-51.jpg)
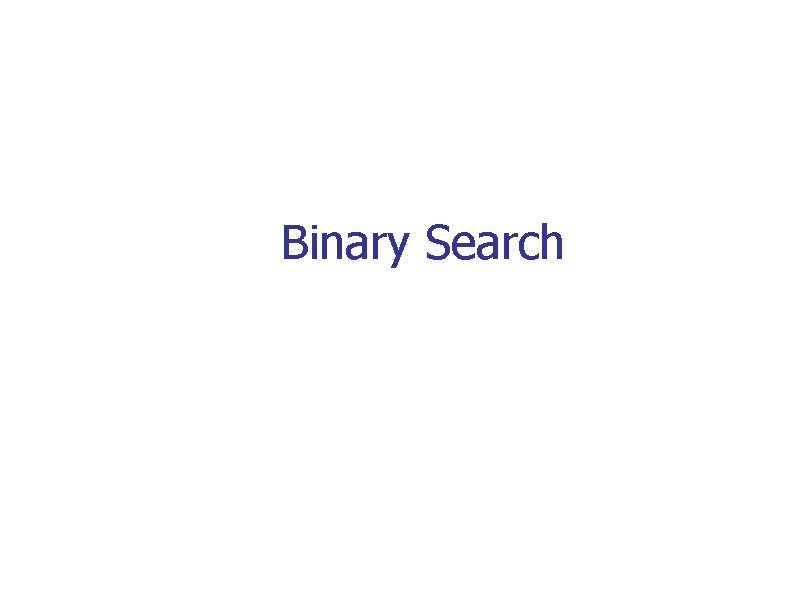
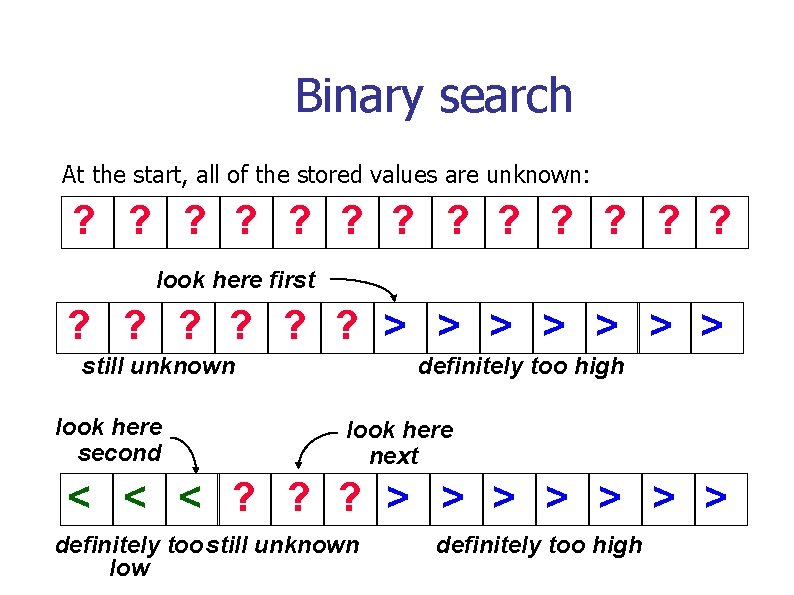
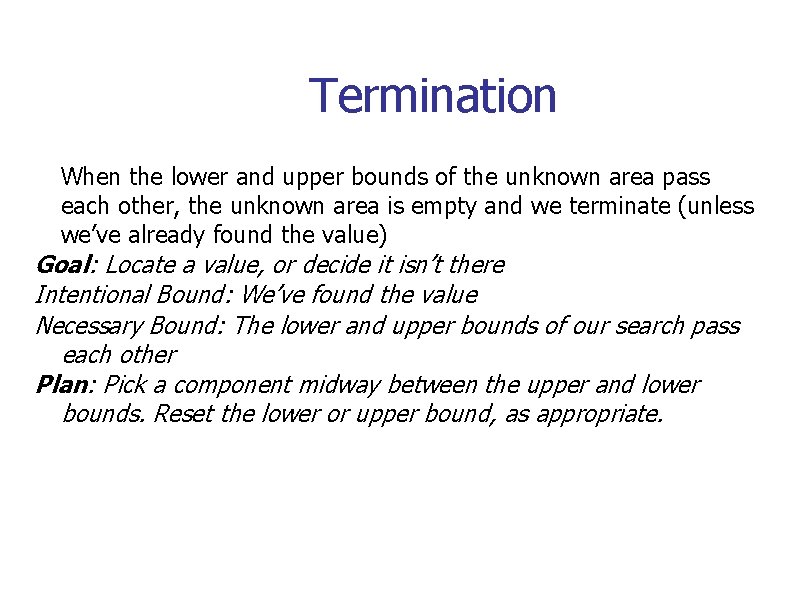
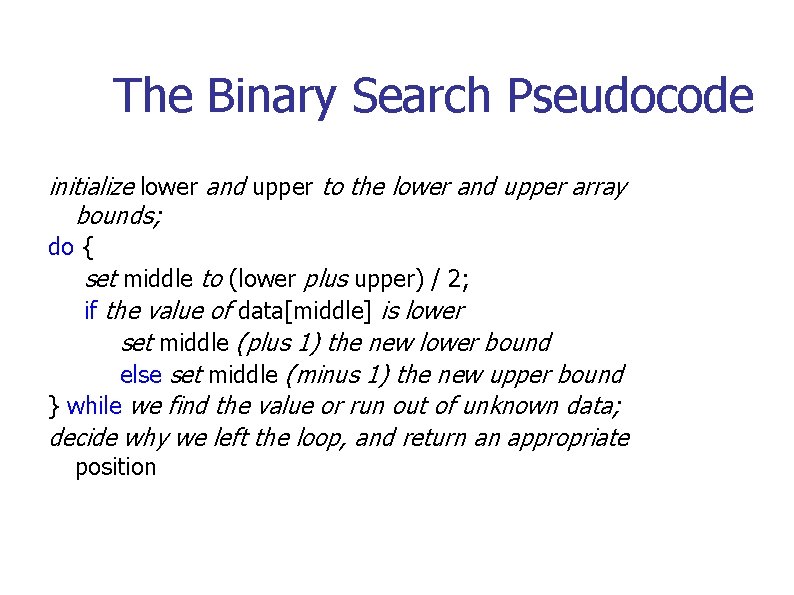
![The Binary Search Java Code int binary. Search (int[ ] data, int num) { The Binary Search Java Code int binary. Search (int[ ] data, int num) {](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-56.jpg)
- Slides: 56
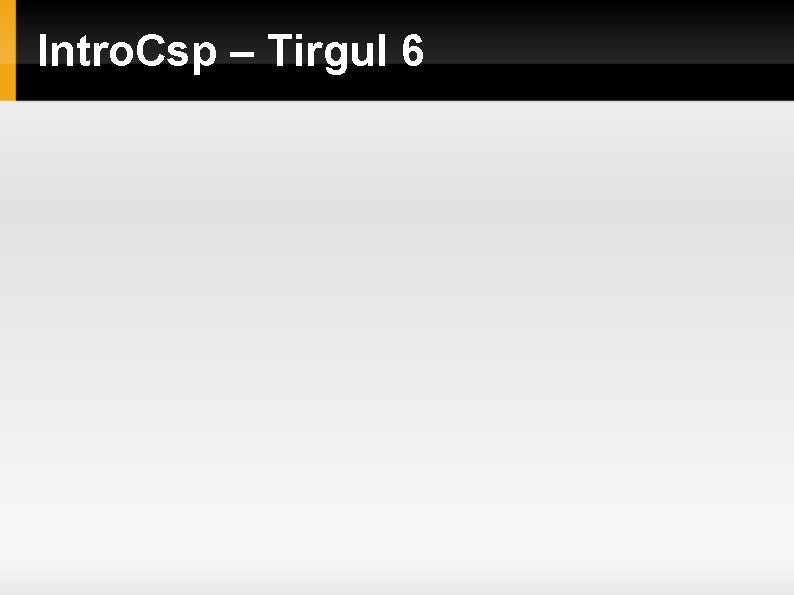
Intro. Csp – Tirgul 6
![Command Line Arguments The main method takes a String as a parameter public static Command Line Arguments The main method takes a String[] as a parameter. public static](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-2.jpg)
Command Line Arguments The main method takes a String[] as a parameter. public static void main(String[] args) The contents come from the command line that called the program: java Hello. Command. Line how are you 0 1 2 “how” “are” “you” args 2
![Example public class Hello Command Line public static void mainString args System out Example: public class Hello. Command. Line { public static void main(String[] args){ System. out.](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-3.jpg)
Example: public class Hello. Command. Line { public static void main(String[] args){ System. out. println("There are " + args. length + “ arguments"); System. out. println("They are: "); for(int i=0; i<args. length; i++){ System. out. println(args[i]); } } } 3
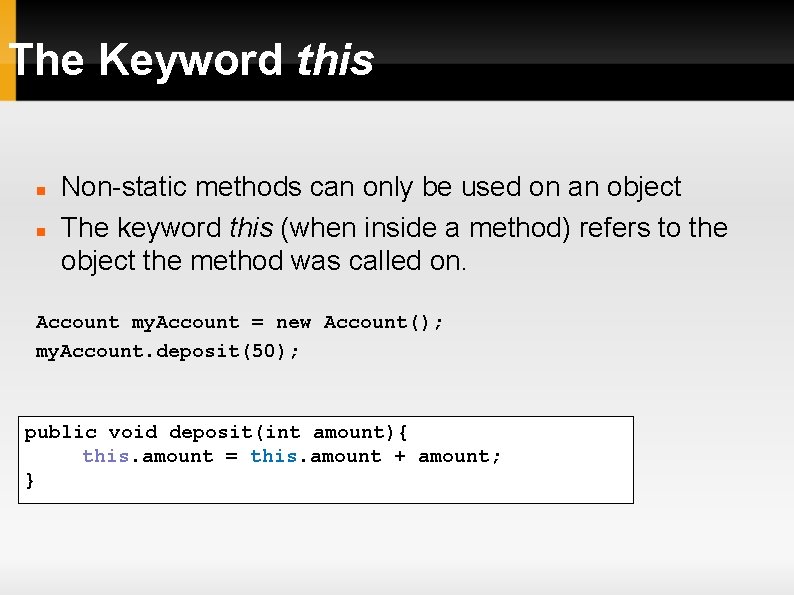
The Keyword this Non-static methods can only be used on an object The keyword this (when inside a method) refers to the object the method was called on. Account my. Account = new Account(); my. Account. deposit(50); public void deposit(int amount){ this. amount = this. amount + amount; }
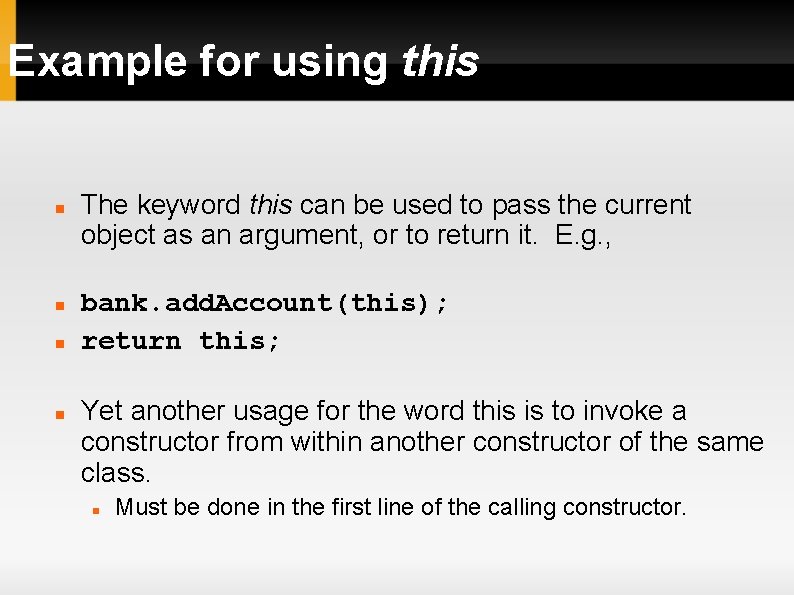
Example for using this The keyword this can be used to pass the current object as an argument, or to return it. E. g. , bank. add. Account(this); return this; Yet another usage for the word this is to invoke a constructor from within another constructor of the same class. Must be done in the first line of the calling constructor.
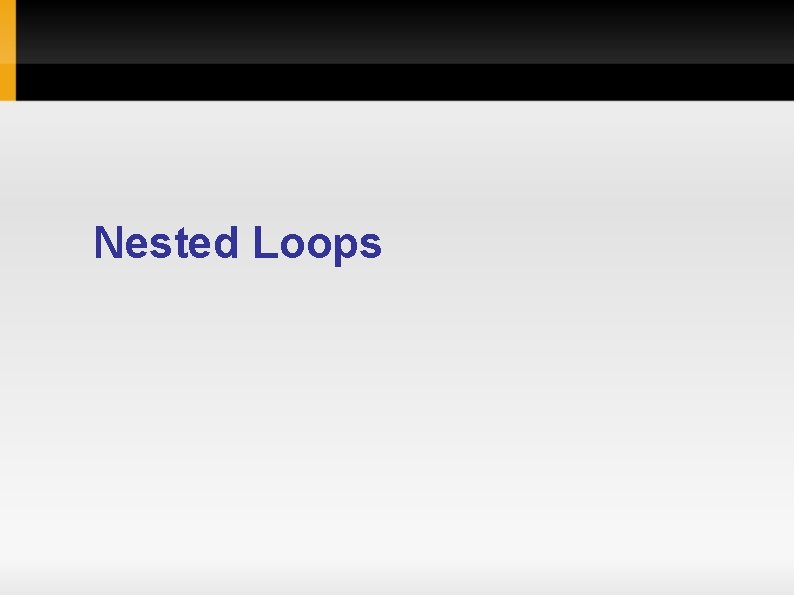
Nested Loops
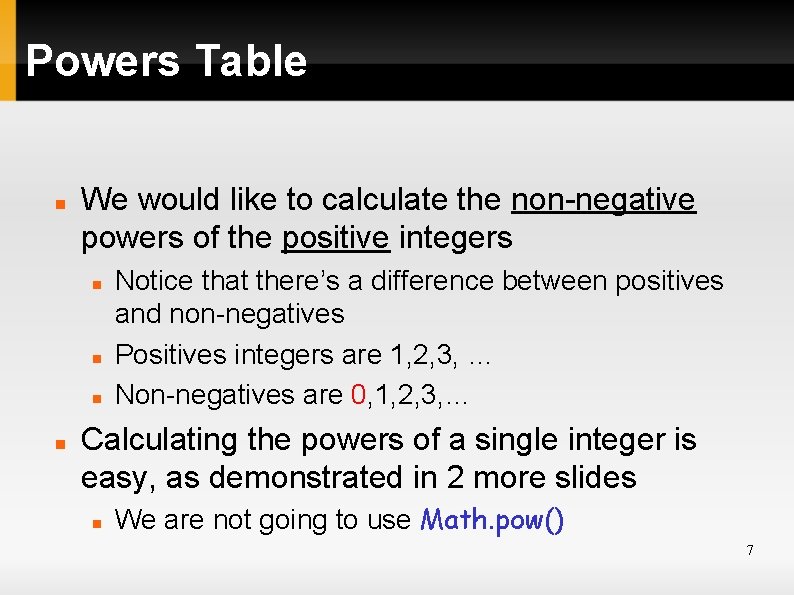
Powers Table We would like to calculate the non-negative powers of the positive integers Notice that there’s a difference between positives and non-negatives Positives integers are 1, 2, 3, … Non-negatives are 0, 1, 2, 3, … Calculating the powers of a single integer is easy, as demonstrated in 2 more slides We are not going to use Math. pow() 7
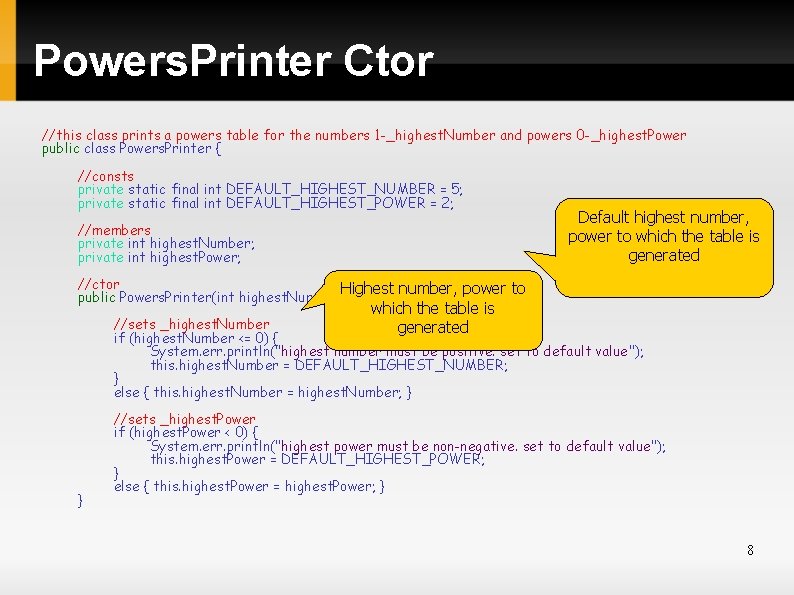
Powers. Printer Ctor //this class prints a powers table for the numbers 1 -_highest. Number and powers 0 -_highest. Power public class Powers. Printer { //consts private static final int DEFAULT_HIGHEST_NUMBER = 5; private static final int DEFAULT_HIGHEST_POWER = 2; //members private int highest. Number; private int highest. Power; Default highest number, power to which the table is generated //ctor Highest number, power to public Powers. Printer(int highest. Number, int highest. Power) { which the table is generated //sets _highest. Number if (highest. Number <= 0) { System. err. println("highest number must be positive. set to default value"); this. highest. Number = DEFAULT_HIGHEST_NUMBER; } else { this. highest. Number = highest. Number; } } //sets _highest. Power if (highest. Power < 0) { System. err. println("highest power must be non-negative. set to default value"); this. highest. Power = DEFAULT_HIGHEST_POWER; } else { this. highest. Power = highest. Power; } 8
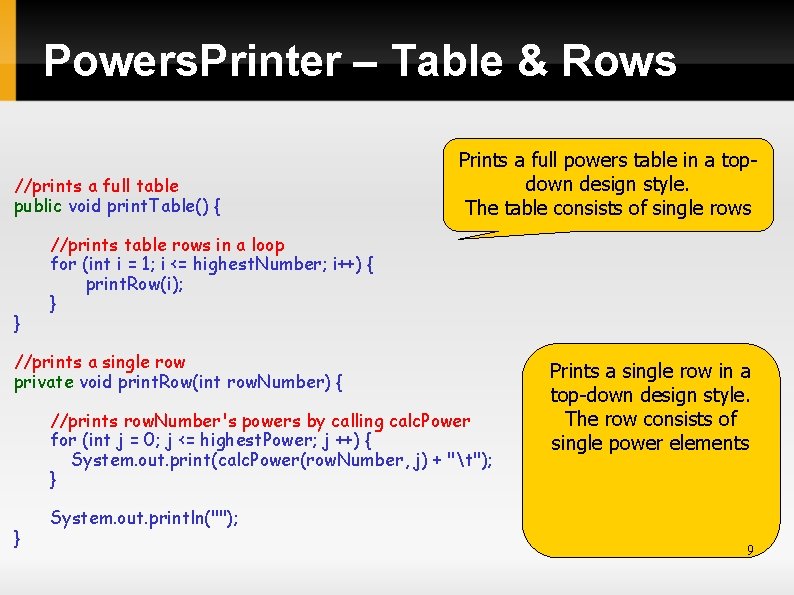
Powers. Printer – Table & Rows //prints a full table public void print. Table() { } Prints a full powers table in a topdown design style. The table consists of single rows //prints table rows in a loop for (int i = 1; i <= highest. Number; i++) { print. Row(i); } //prints a single row private void print. Row(int row. Number) { //prints row. Number's powers by calling calc. Power for (int j = 0; j <= highest. Power; j ++) { System. out. print(calc. Power(row. Number, j) + "t"); } } Prints a single row in a top-down design style. The row consists of single power elements System. out. println(""); 9
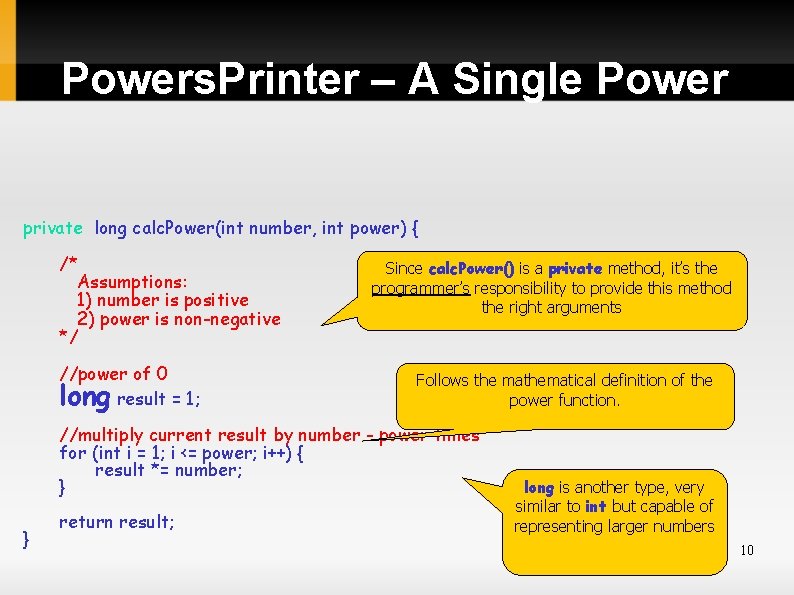
Powers. Printer – A Single Power private long calc. Power(int number, int power) { /* Assumptions: 1) number is positive 2) power is non-negative */ //power of 0 long result = 1; Since calc. Power() is a private method, it’s the programmer’s responsibility to provide this method the right arguments Follows the mathematical definition of the power function. //multiply current result by number - power times for (int i = 1; i <= power; i++) { result *= number; } } return result; long is another type, very similar to int but capable of representing larger numbers 10
![Powers Printer Main public static void mainString argv argv is a Strings Powers. Printer – Main public static void main(String[] argv) { argv is a Strings](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-11.jpg)
Powers. Printer – Main public static void main(String[] argv) { argv is a Strings array and has a length member //verify that 2 arguments were given if (argv. length < 2) { System. err. println("n. USAGE: java Powers. Printer <highest-number>" + " <highest-power>n"); System. exit(-1); If the user types The whole program terminates with } non-integer arguments, java exit code of -1 on System. exit(will throw 1) //get user arguments - assuming legal argument types Number. Format. Exception during runtime. int user. Highest. Number = Integer. parse. Int(argv[0]); int user. Highest. Power = Integer. parse. Int(argv[1]); … 11
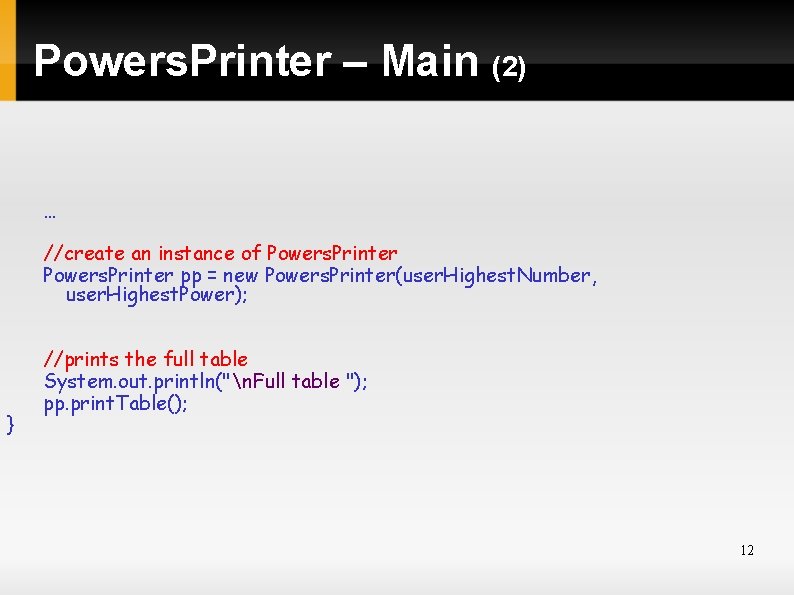
Powers. Printer – Main (2) … //create an instance of Powers. Printer pp = new Powers. Printer(user. Highest. Number, user. Highest. Power); } //prints the full table System. out. println("n. Full table "); pp. print. Table(); 12
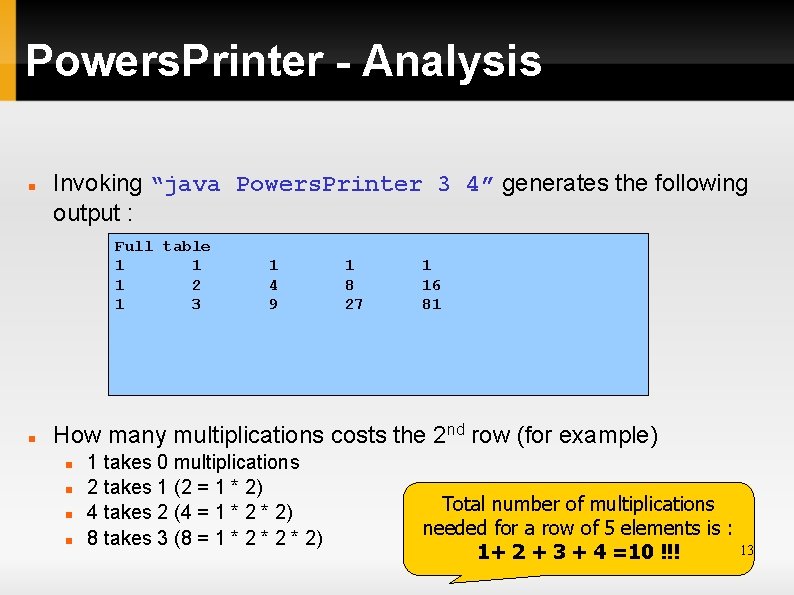
Powers. Printer - Analysis Invoking “java Powers. Printer 3 4” generates the following output : Full table 1 1 1 2 1 3 1 4 9 1 8 27 1 16 81 How many multiplications costs the 2 nd row (for example) 1 takes 0 multiplications 2 takes 1 (2 = 1 * 2) 4 takes 2 (4 = 1 * 2) 8 takes 3 (8 = 1 * 2 * 2) Total number of multiplications needed for a row of 5 elements is : 13 1+ 2 + 3 + 4 =10 !!!
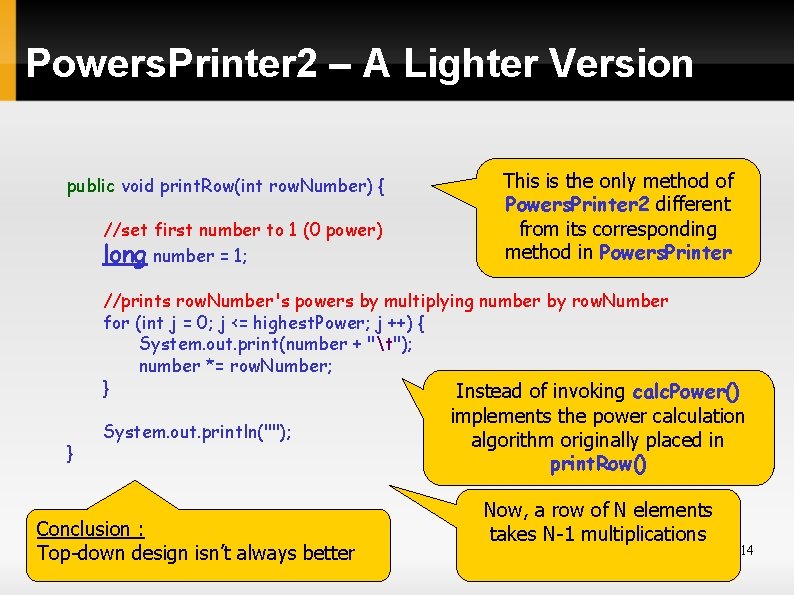
Powers. Printer 2 – A Lighter Version public void print. Row(int row. Number) { //set first number to 1 (0 power) long number = 1; This is the only method of Powers. Printer 2 different from its corresponding method in Powers. Printer //prints row. Number's powers by multiplying number by row. Number for (int j = 0; j <= highest. Power; j ++) { System. out. print(number + "t"); number *= row. Number; } Instead of invoking calc. Power() } System. out. println(""); Conclusion : Top-down design isn’t always better implements the power calculation algorithm originally placed in print. Row() Now, a row of N elements takes N-1 multiplications 14
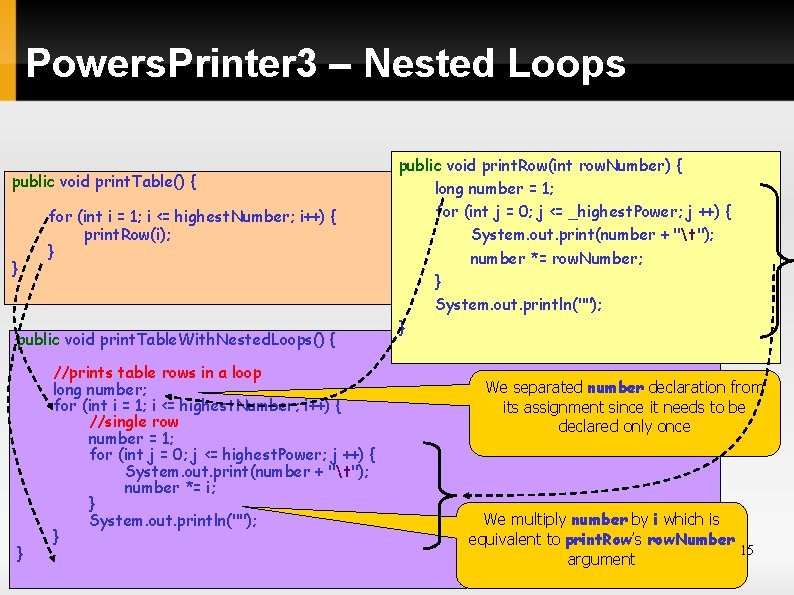
Powers. Printer 3 – Nested Loops public void print. Table() { } for (int i = 1; i <= highest. Number; i++) { print. Row(i); } public void print. Table. With. Nested. Loops() { } //prints table rows in a loop long number; for (int i = 1; i <= highest. Number; i++) { //single row number = 1; for (int j = 0; j <= highest. Power; j ++) { System. out. print(number + "t"); number *= i; } System. out. println(""); } public void print. Row(int row. Number) { long number = 1; for (int j = 0; j <= _highest. Power; j ++) { System. out. print(number + "t"); number *= row. Number; } System. out. println(""); } We separated number declaration from its assignment since it needs to be declared only once We multiply number by i which is equivalent to print. Row’s row. Number 15 argument
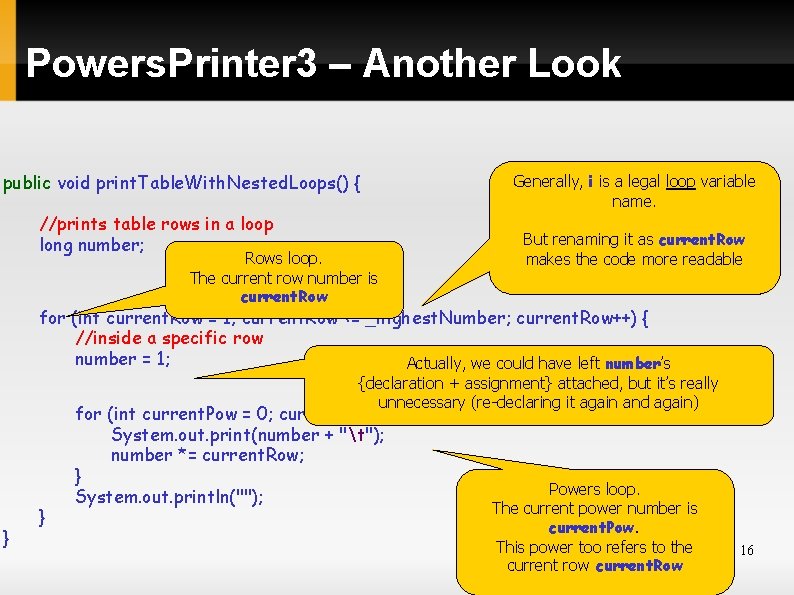
Powers. Printer 3 – Another Look public void print. Table. With. Nested. Loops() { //prints table rows in a loop long number; Rows loop. The current row number is current. Row Generally, i is a legal loop variable name. But renaming it as current. Row makes the code more readable for (int current. Row = 1; current. Row <= _highest. Number; current. Row++) { //inside a specific row number = 1; Actually, we could have left number’s {declaration + assignment} attached, but it’s really unnecessary (re-declaring it again and again) } } for (int current. Pow = 0; current. Pow <= _highest. Power; current. Pow ++) { System. out. print(number + "t"); number *= current. Row; } Powers loop. System. out. println(""); The current power number is current. Pow. This power too refers to the current row current. Row 16
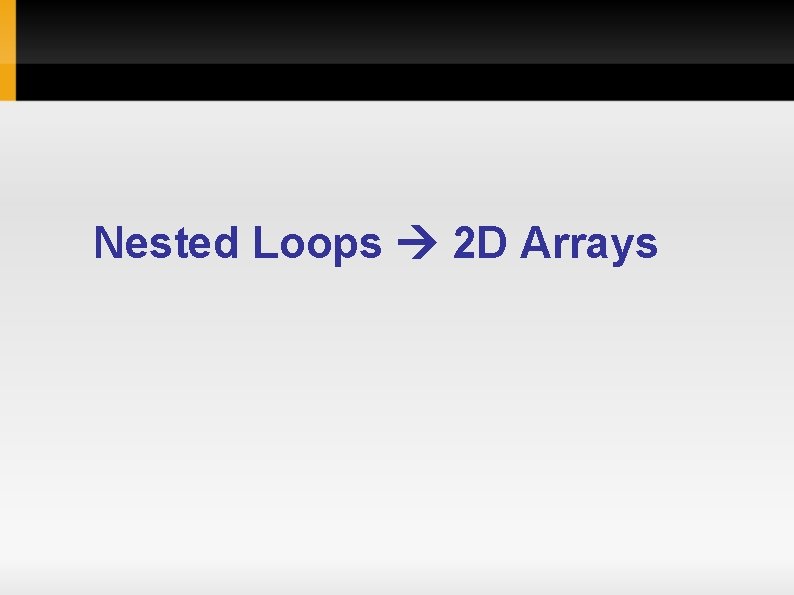
Nested Loops 2 D Arrays
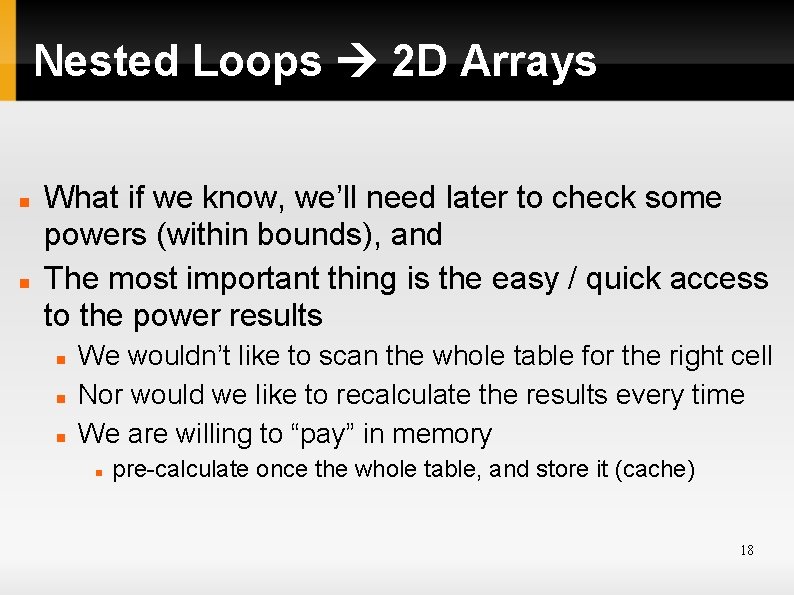
Nested Loops 2 D Arrays What if we know, we’ll need later to check some powers (within bounds), and The most important thing is the easy / quick access to the power results We wouldn’t like to scan the whole table for the right cell Nor would we like to recalculate the results every time We are willing to “pay” in memory pre-calculate once the whole table, and store it (cache) 18
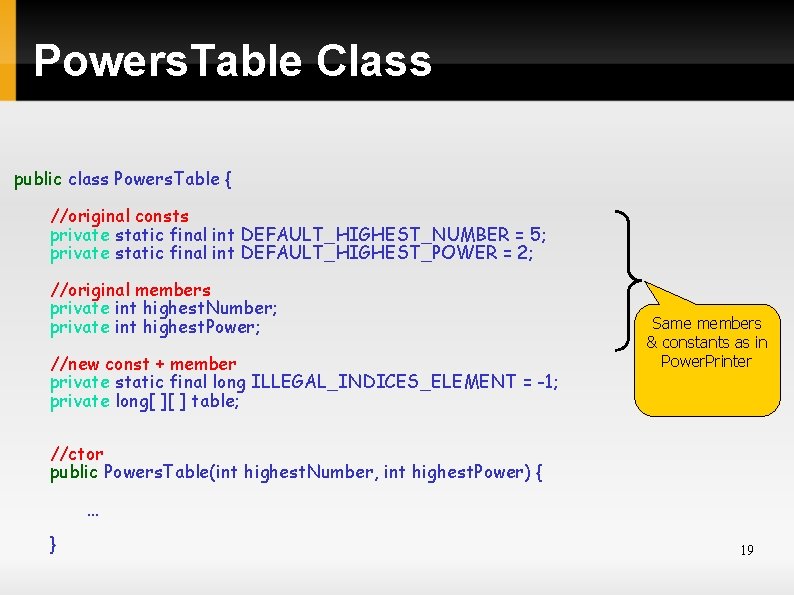
Powers. Table Class public class Powers. Table { //original consts private static final int DEFAULT_HIGHEST_NUMBER = 5; private static final int DEFAULT_HIGHEST_POWER = 2; //original members private int highest. Number; private int highest. Power; //new const + member private static final long ILLEGAL_INDICES_ELEMENT = -1; private long[ ][ ] table; Same members & constants as in Power. Printer //ctor public Powers. Table(int highest. Number, int highest. Power) { … } 19
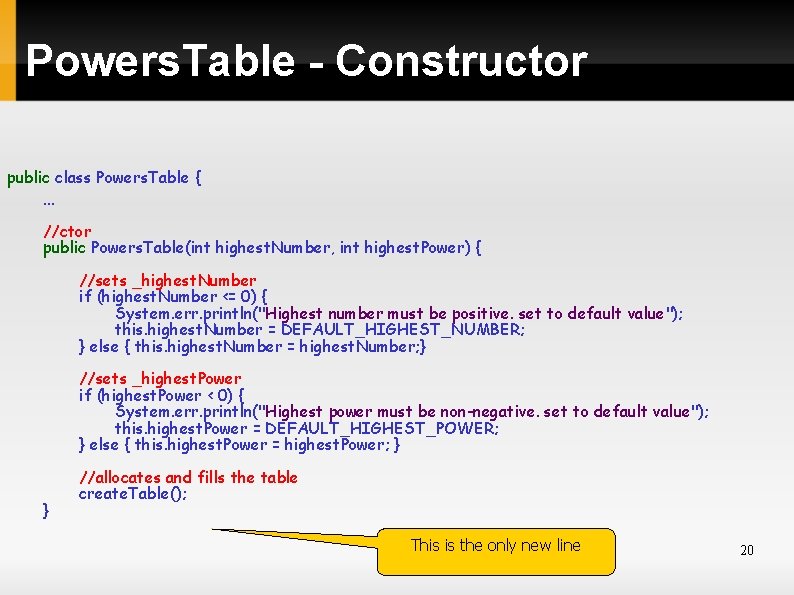
Powers. Table - Constructor public class Powers. Table { … //ctor public Powers. Table(int highest. Number, int highest. Power) { //sets _highest. Number if (highest. Number <= 0) { System. err. println("Highest number must be positive. set to default value"); this. highest. Number = DEFAULT_HIGHEST_NUMBER; } else { this. highest. Number = highest. Number; } //sets _highest. Power if (highest. Power < 0) { System. err. println("Highest power must be non-negative. set to default value"); this. highest. Power = DEFAULT_HIGHEST_POWER; } else { this. highest. Power = highest. Power; } } //allocates and fills the table create. Table(); This is the only new line 20
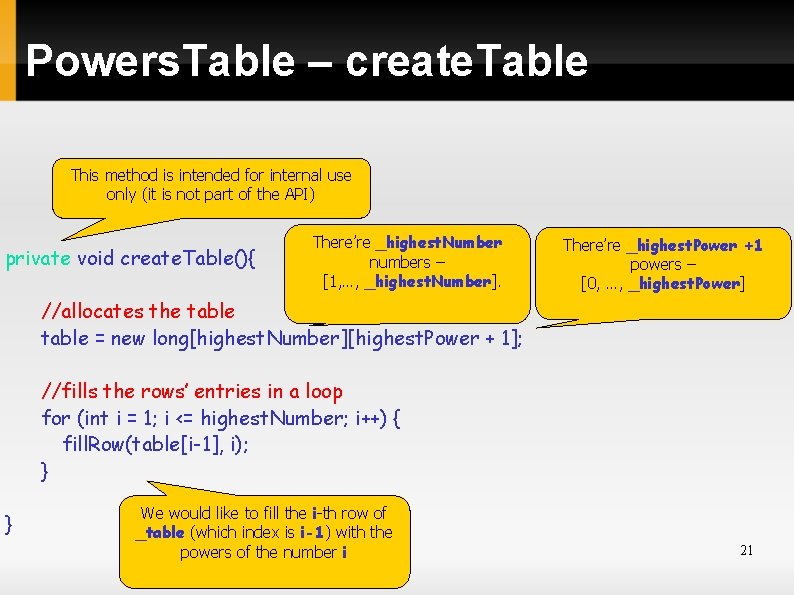
Powers. Table – create. Table This method is intended for internal use only (it is not part of the API) private void create. Table(){ There’re _highest. Number numbers – [1, …, _highest. Number]. There’re _highest. Power +1 powers – [0, …, _highest. Power] //allocates the table = new long[highest. Number][highest. Power + 1]; //fills the rows’ entries in a loop for (int i = 1; i <= highest. Number; i++) { fill. Row(table[i-1], i); } } We would like to fill the i-th row of _table (which index is i-1) with the powers of the number i 21
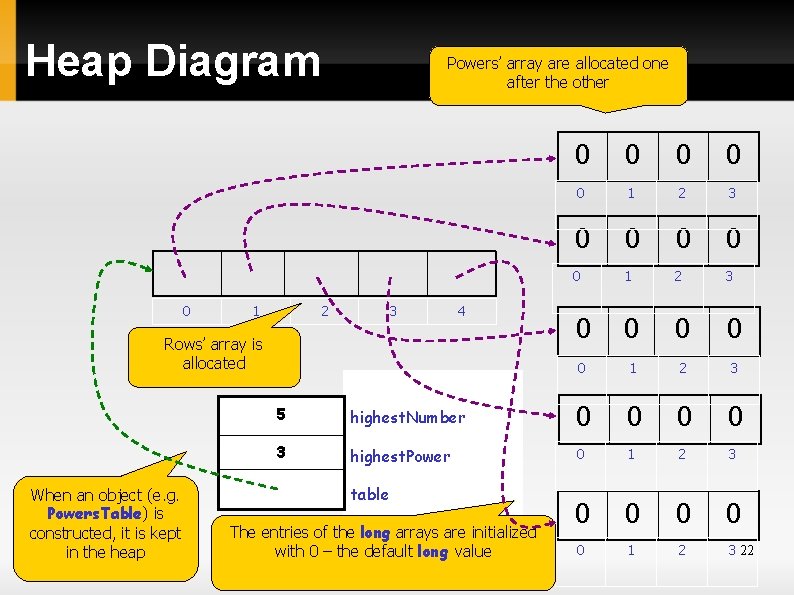
Heap Diagram 0 1 Powers’ array are allocated one after the other 2 3 4 Rows’ array is allocated When an object (e. g. Powers. Table) is constructed, it is kept in the heap 0 0 0 0 0 1 2 3 5 highest. Number 0 0 3 highest. Power 0 1 2 3 0 0 0 1 2 3 22 table The entries of the long arrays are initialized with 0 – the default long value
![Powers Table fill Row private void fill Rowlong row int row Number Powers. Table - fill. Row private void fill. Row(long[] row, int row. Number) {](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-23.jpg)
Powers. Table - fill. Row private void fill. Row(long[] row, int row. Number) { //power of 0 long number = 1; } fill. Row() doesn’t know and doesn’t care that the parameter row is only a single row belongs to a 2 D array //other powers - multiply number by row. Number for (int j = 0; j < row. length; j ++) { row[j] = number; number *= row. Number; We could have used } (_highest. Power + 1) instead of row. length, since there’re _highest. Power+1 powers in every row – 23 [0, …, _highest. Power]
![What Just Happened Here table is created in create Table tablei1 is sent as What Just Happened Here? table is created in create. Table table[i-1] is sent as](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-24.jpg)
What Just Happened Here? table is created in create. Table table[i-1] is sent as fill. Row’s argument create. Table() is about to invoke fille. Row(table[1], 2) row is created as a parameter of table[i-1] according to call by value concept Heap Stack (create. Table) Stack (fill. Row) table = 87 a 9 f 5 d i=2 row = 5 a 76 bfc table[i -1] = 5 a 76 bfc row. length = 4 number = 1 j=0 Power of 0 number = … Loop begins table[i-1] values were updated !!! 0 1 0 2 0 4 0 8 0 1 2 3 24
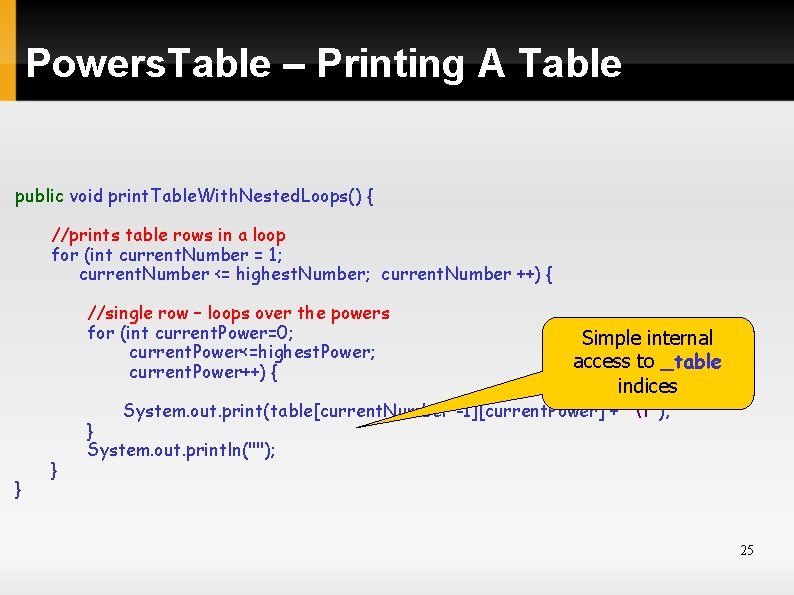
Powers. Table – Printing A Table public void print. Table. With. Nested. Loops() { //prints table rows in a loop for (int current. Number = 1; current. Number <= highest. Number; current. Number ++) { //single row – loops over the powers for (int current. Power=0; current. Power<=highest. Power; current. Power++) { Simple internal access to _table indices System. out. print(table[current. Number -1][current. Power] + "t"); } } } System. out. println(""); 25
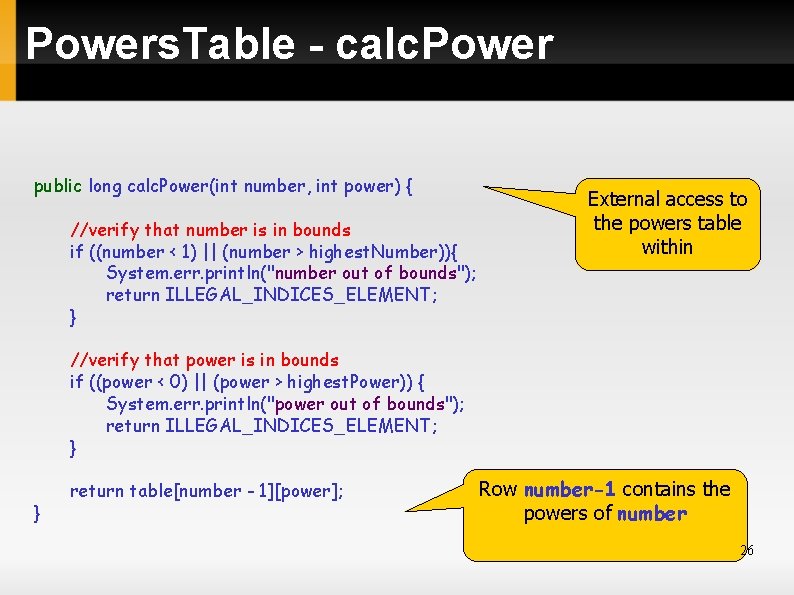
Powers. Table - calc. Power public long calc. Power(int number, int power) { //verify that number is in bounds if ((number < 1) || (number > highest. Number)){ System. err. println("number out of bounds"); return ILLEGAL_INDICES_ELEMENT; } External access to the powers table within //verify that power is in bounds if ((power < 0) || (power > highest. Power)) { System. err. println("power out of bounds"); return ILLEGAL_INDICES_ELEMENT; } } return table[number - 1][power]; Row number-1 contains the powers of number 26
![Powers Table Main public static void mainString argv verify that 2 arguments Powers. Table - Main public static void main(String[] argv) { //verify that 2 arguments](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-27.jpg)
Powers. Table - Main public static void main(String[] argv) { //verify that 2 arguments were given if (argv. length < 2) { System. err. println("n. USAGE: java Powers. Table <max-number> <max-power>n"); System. exit(-1); } Powers. Table has no static //get user arguments - assuming legal argument types methods and an instance must be int user. Highest. Number = Integer. parse. Int(argv[0]); constructed int user. Highest. Power = Integer. parse. Int(argv[1]); //create an instance of Powers. Table pp = new Powers. Table(user. Highest. Number, user. Highest. Power); //prints the full table System. out. println("n. Full table "); pp. print. Table. With. Nested. Loops(); } //prints a single power System. out. println("n. The " + user. Highest. Power + "-th power of “ + user. Highest. Number + " is : " + pp. calc. Power(user. Highest. Number, user. Highest. Power)); 27
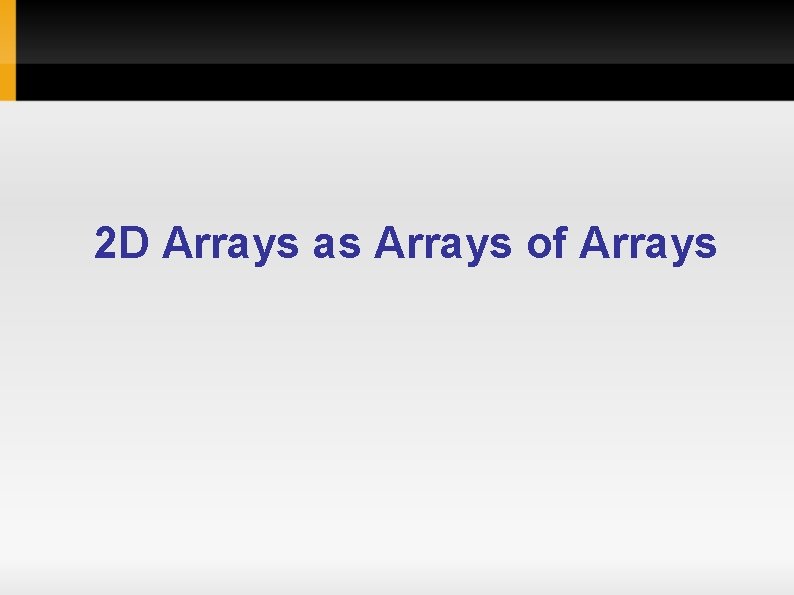
2 D Arrays as Arrays of Arrays
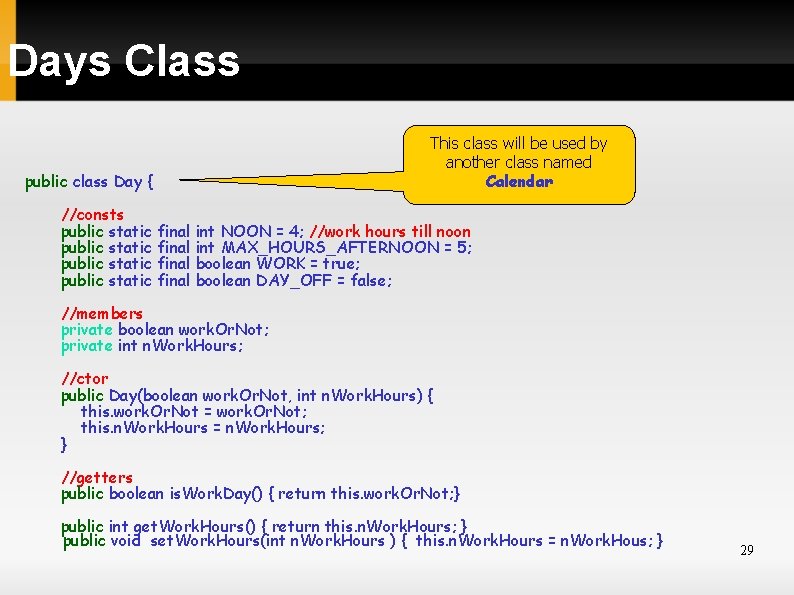
Days Class public class Day { This class will be used by another class named Calendar //consts public static final int NOON = 4; //work hours till noon public static final int MAX_HOURS_AFTERNOON = 5; public static final boolean WORK = true; public static final boolean DAY_OFF = false; //members private boolean work. Or. Not; private int n. Work. Hours; //ctor public Day(boolean work. Or. Not, int n. Work. Hours) { this. work. Or. Not = work. Or. Not; this. n. Work. Hours = n. Work. Hours; } //getters public boolean is. Work. Day() { return this. work. Or. Not; } public int get. Work. Hours() { return this. n. Work. Hours; } public void set. Work. Hours(int n. Work. Hours ) { this. n. Work. Hours = n. Work. Hous; } 29
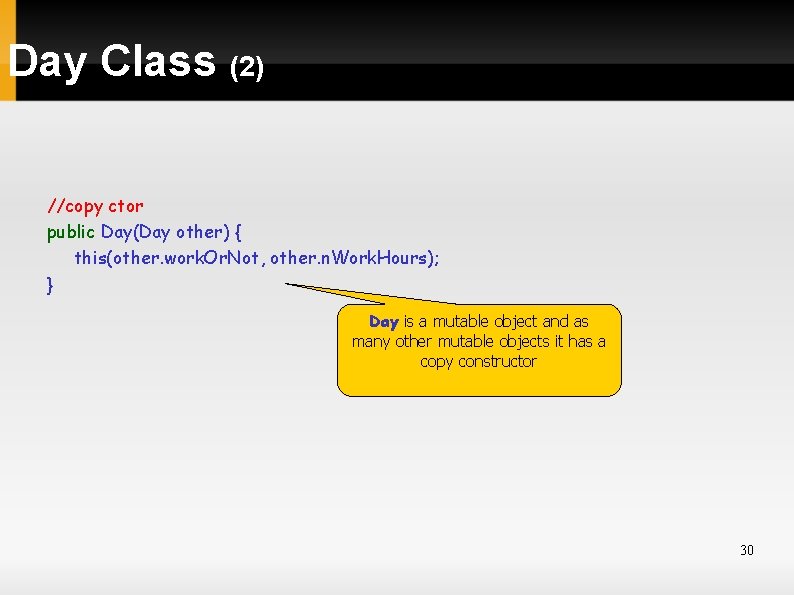
Day Class (2) //copy ctor public Day(Day other) { this(other. work. Or. Not, other. n. Work. Hours); } Day is a mutable object and as many other mutable objects it has a copy constructor 30
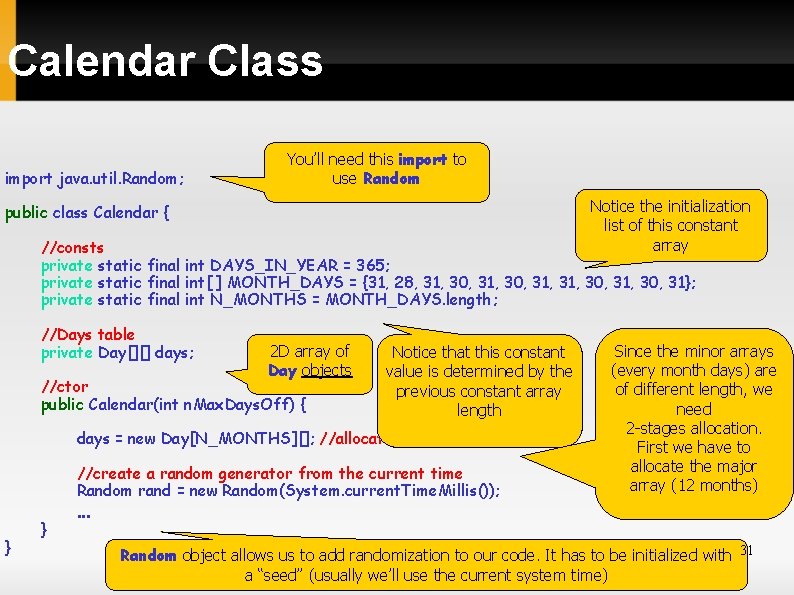
Calendar Class import java. util. Random; You’ll need this import to use Random Notice the initialization list of this constant array public class Calendar { //consts private static final int DAYS_IN_YEAR = 365; private static final int[ ] MONTH_DAYS = {31, 28, 31, 30, 31}; private static final int N_MONTHS = MONTH_DAYS. length; //Days table private Day[][] days; 2 D array of Day objects //ctor public Calendar(int n. Max. Days. Off) { Notice that this constant value is determined by the previous constant array length days = new Day[N_MONTHS][]; //allocates months //create a random generator from the current time Random rand = new Random(System. current. Time. Millis()); } } Since the minor arrays (every month days) are of different length, we need 2 -stages allocation. First we have to allocate the major array (12 months) … Random object allows us to add randomization to our code. It has to be initialized with 31 a “seed” (usually we’ll use the current system time)
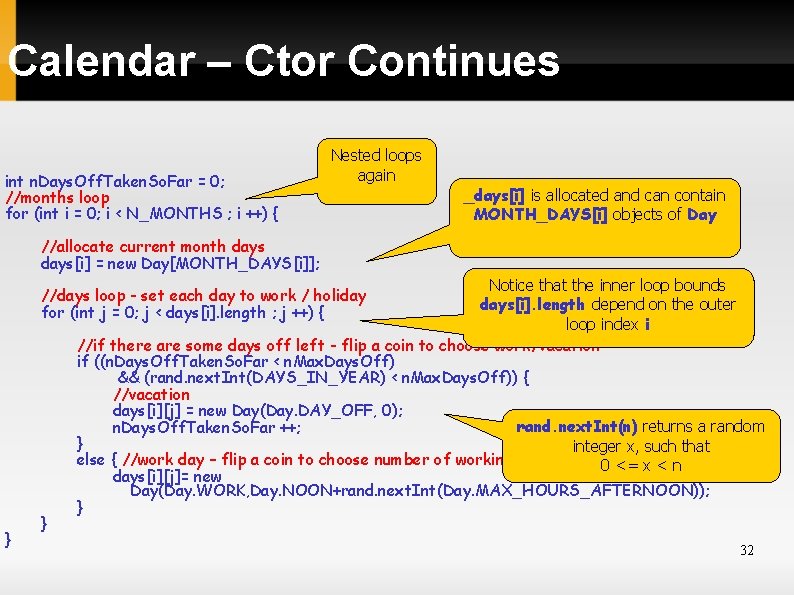
Calendar – Ctor Continues int n. Days. Off. Taken. So. Far = 0; //months loop for (int i = 0; i < N_MONTHS ; i ++) { Nested loops again _days[i] is allocated and can contain MONTH_DAYS[i] objects of Day //allocate current month days[i] = new Day[MONTH_DAYS[i]]; Notice that the inner loop bounds days[i]. length depend on the outer loop index i //if there are some days off left - flip a coin to choose work/vacation if ((n. Days. Off. Taken. So. Far < n. Max. Days. Off) && (rand. next. Int(DAYS_IN_YEAR) < n. Max. Days. Off)) { //vacation days[i][j] = new Day(Day. DAY_OFF, 0); rand. next. Int(n) returns a random n. Days. Off. Taken. So. Far ++; } integer x, such that else { //work day – flip a coin to choose number of working hours 0 <= x < n days[i][j]= new Day(Day. WORK, Day. NOON+rand. next. Int(Day. MAX_HOURS_AFTERNOON)); } //days loop - set each day to work / holiday for (int j = 0; j < days[i]. length ; j ++) { } } 32
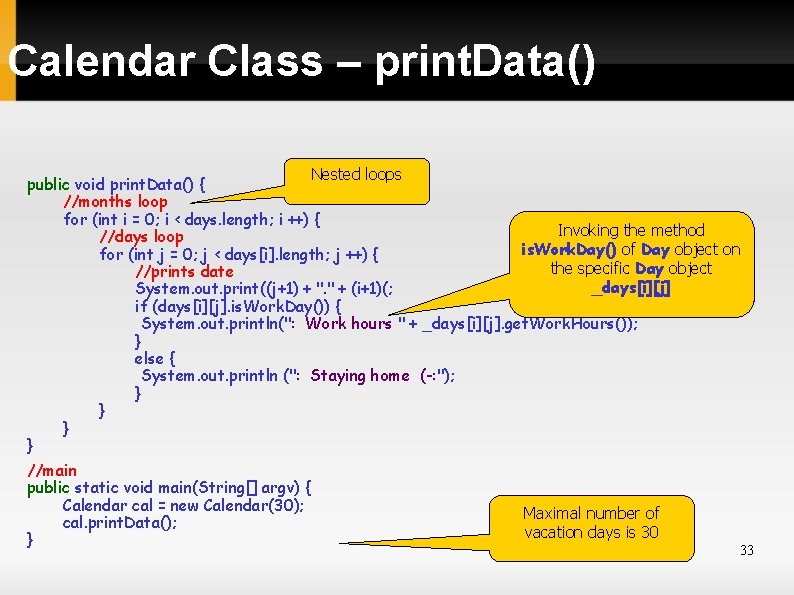
Calendar Class – print. Data() Nested loops public void print. Data() { //months loop for (int i = 0; i < days. length; i ++) { Invoking the method //days loop is. Work. Day() of Day object on for (int j = 0; j < days[i]. length; j ++) { the specific Day object //prints date _days[i][j] System. out. print((j+1) + ". " + (i+1)(; if (days[i][j]. is. Work. Day()) { System. out. println(": Work hours " + _days[i][j]. get. Work. Hours()); } else { System. out. println (": Staying home (-: "); } } //main public static void main(String[] argv) { Calendar cal = new Calendar(30); cal. print. Data(); } Maximal number of vacation days is 30 33
![Calendar get Calendar public Day get Calendar return days This method Calendar - get. Calendar public Day[][] get. Calendar() { return days; } This method](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-34.jpg)
Calendar - get. Calendar public Day[][] get. Calendar() { return days; } This method returns a 2 D-object array public Day[][] get. Calendar() { Day[][] copy = days. clone(); } Return copy; public Day[][] get. Calendar() { //allocates major array (months) Day[][] copy = new Day[days. length][]; //months loop for (int curr. Month = 0; curr. Month < copy. length; curr. Month ++) { //allocates inner array (days) copy[curr. Month] = days[curr. Month]. clone(); } return copy; } 34
![Calendar get Calendar public Day get Calendar allocates major array months Day Calendar - get. Calendar public Day[][] get. Calendar() { //allocates major array (months) Day[][]](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-35.jpg)
Calendar - get. Calendar public Day[][] get. Calendar() { //allocates major array (months) Day[][] copy = new Day[days. length][]; This method returns a 2 D-object array //months loop for (int curr. Month = 0; curr. Month < copy. length; curr. Month ++) { //allocates inner array (days) copy[curr. Month] = new Day[days[curr. Month]. length]; } } //days loop for (int curr. Day = 0; curr. Day < copy[curr. Month]. length; curr. Day++){ //copy the specific day copy[curr. Month][curr. Day] = new Day(days[curr. Month][curr. Day]); } return copy; 35
![Heap Diagram Arrays for days of each month are allocated days1 0 0 0 Heap Diagram Arrays for days of each month are allocated days[1] 0 0 0](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-36.jpg)
Heap Diagram Arrays for days of each month are allocated days[1] 0 0 0 …… 0 0 1 …. . . 11 0 30 0 27 days[1][27] Month’ array is allocated _days[] 0 0 1 2 3 0 0 0 …. . 3036
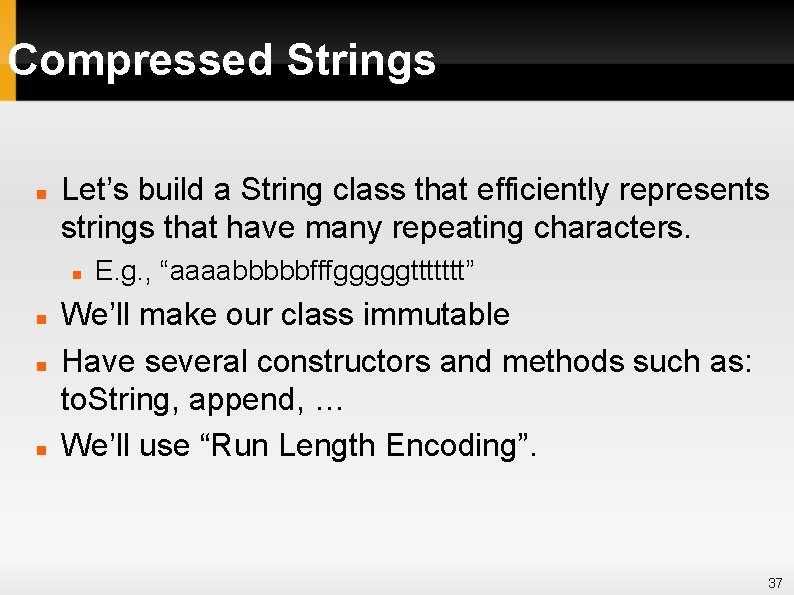
Compressed Strings Let’s build a String class that efficiently represents strings that have many repeating characters. E. g. , “aaaabbbbbfffgggggttttttt” We’ll make our class immutable Have several constructors and methods such as: to. String, append, … We’ll use “Run Length Encoding”. 37
![Compressed Strings public class Compressed String private char chars private int freq Will Compressed Strings public class Compressed. String { private char[] chars; private int[] freq; Will](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-38.jpg)
Compressed Strings public class Compressed. String { private char[] chars; private int[] freq; Will hold the characters And how many times they appear public String to. String(){ String result = ""; for(int i=0; i<chars. length; i++){ for(int j=0; j<freq[i]; j++){ result += chars[i]; } } return result; } … To decode the String: Add each character to the string several times } 38
![Compressed Strings The First Constructor public Compressed Stringchar string int compressed Lengthstring length Compressed Strings – The First Constructor public Compressed. String(char[] string){ int compressed. Length=string. length;](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-39.jpg)
Compressed Strings – The First Constructor public Compressed. String(char[] string){ int compressed. Length=string. length; for(int i=1; i<string. length; i++){ if(string[i]==string[i-1]) compressed. Length -= 1; } chars = new char[compressed. Length]; freq = new int[compressed. Length]; Find out the length of the String after it is compressed Allocate memory accordingly int index=0; for(int i=0; i<chars. length; i++){ chars[i] = string[index]; Compress int next. Index; String for(next. Index=index; next. Index<string. length && string[next. Index]==string[index]; next. Index++); freq[i] = next. Index-index; index = next. Index; } } the This method is quite long. It may be better to break it down to smaller pieces. 39
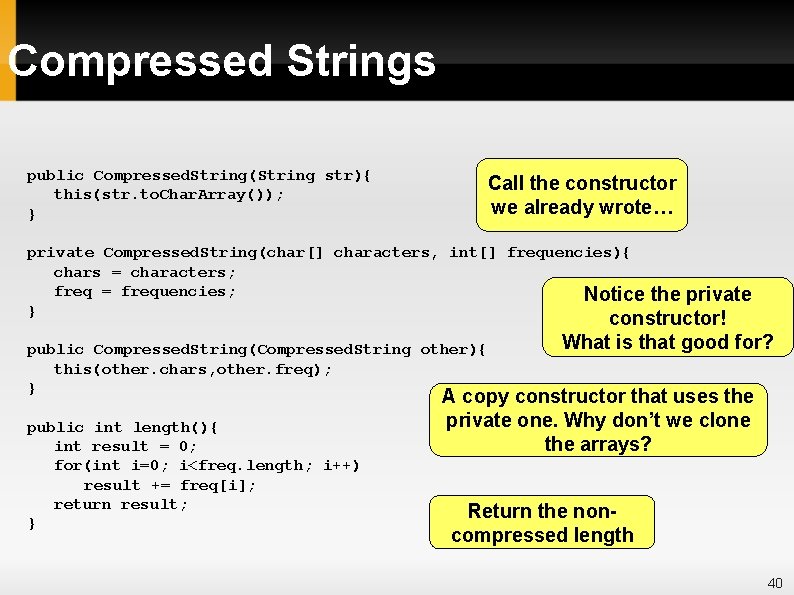
Compressed Strings public Compressed. String(String str){ this(str. to. Char. Array()); } Call the constructor we already wrote… private Compressed. String(char[] characters, int[] frequencies){ chars = characters; freq = frequencies; Notice } public Compressed. String(Compressed. String other){ this(other. chars, other. freq); } public int length(){ int result = 0; for(int i=0; i<freq. length; i++) result += freq[i]; return result; } the private constructor! What is that good for? A copy constructor that uses the private one. Why don’t we clone the arrays? Return the noncompressed length 40
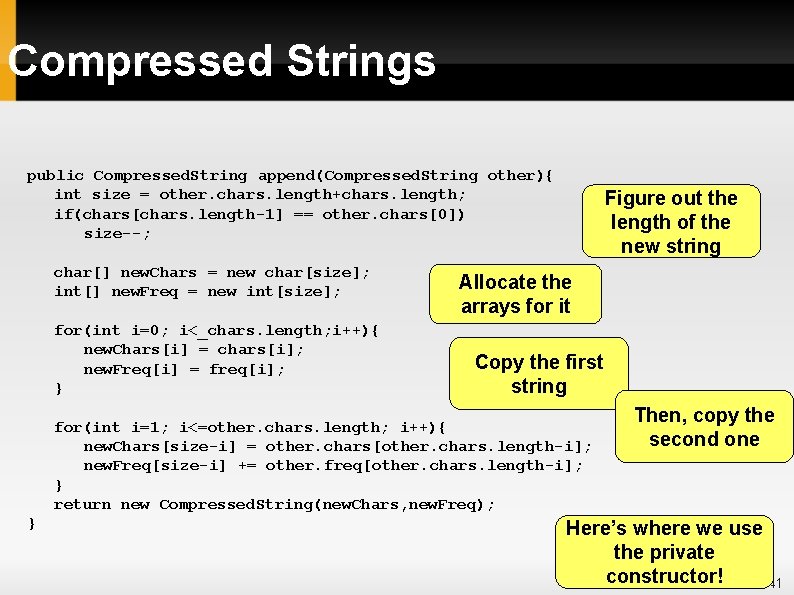
Compressed Strings public Compressed. String append(Compressed. String other){ int size = other. chars. length+chars. length; if(chars[chars. length-1] == other. chars[0]) size--; char[] new. Chars = new char[size]; int[] new. Freq = new int[size]; for(int i=0; i<_chars. length; i++){ new. Chars[i] = chars[i]; new. Freq[i] = freq[i]; } Figure out the length of the new string Allocate the arrays for it Copy the first string for(int i=1; i<=other. chars. length; i++){ new. Chars[size-i] = other. chars[other. chars. length-i]; new. Freq[size-i] += other. freq[other. chars. length-i]; } return new Compressed. String(new. Chars, new. Freq); } Then, copy the second one Here’s where we use the private constructor! 41
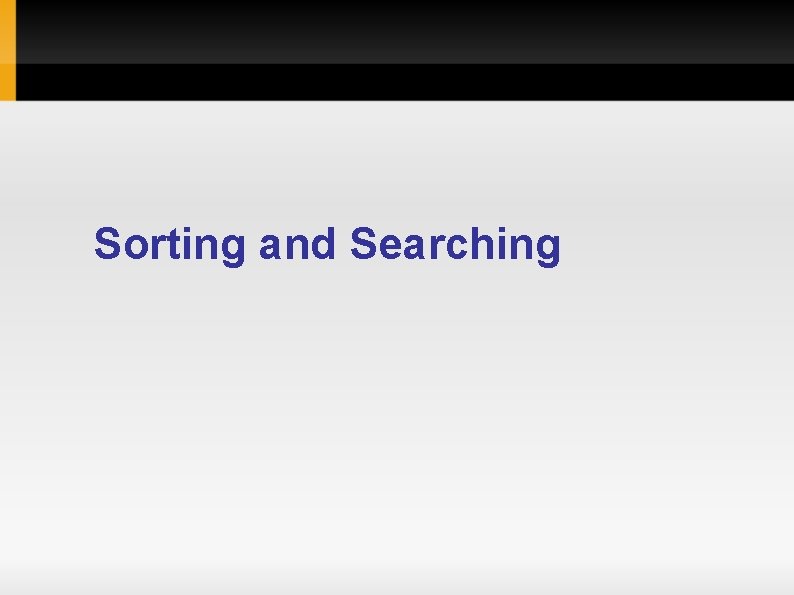
Sorting and Searching
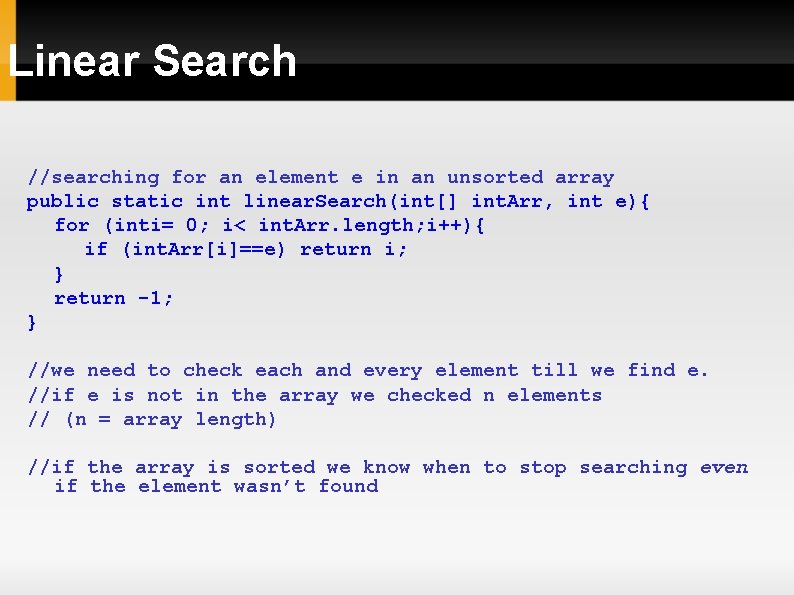
Linear Search //searching for an element e in an unsorted array public static int linear. Search(int[] int. Arr, int e){ for (inti= 0; i< int. Arr. length; i++){ if (int. Arr[i]==e) return i; } return -1; } //we need to check each and every element till we find e. //if e is not in the array we checked n elements // (n = array length) //if the array is sorted we know when to stop searching even if the element wasn’t found
![Linear search in a sorted array public static int linear Searchint int Arr int Linear search in a sorted array public static int linear. Search(int[] int. Arr, int](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-44.jpg)
Linear search in a sorted array public static int linear. Search(int[] int. Arr, int e){ for (inti= 0; i< int. Arr. length; i++){ if (int. Arr[i]==e) { return i; } if (int. Arr[i] > e){ return -1; } } return -1; }
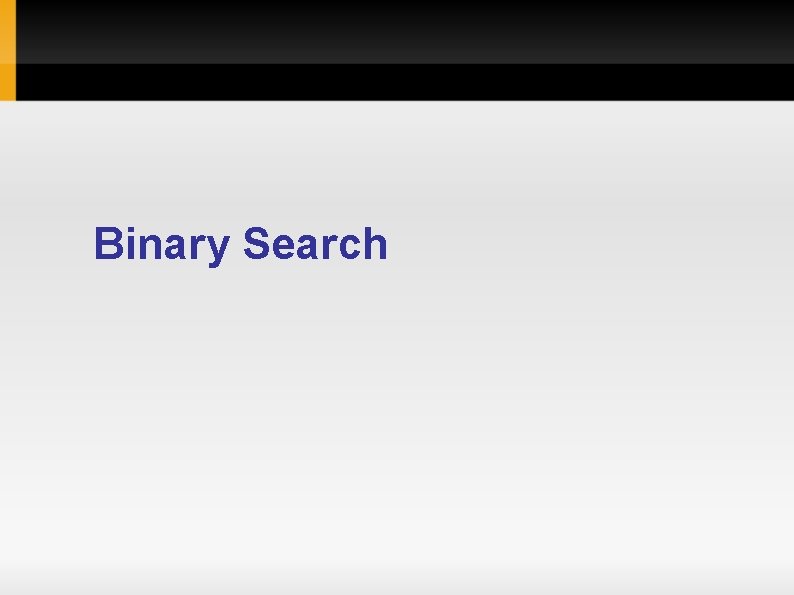
Binary Search
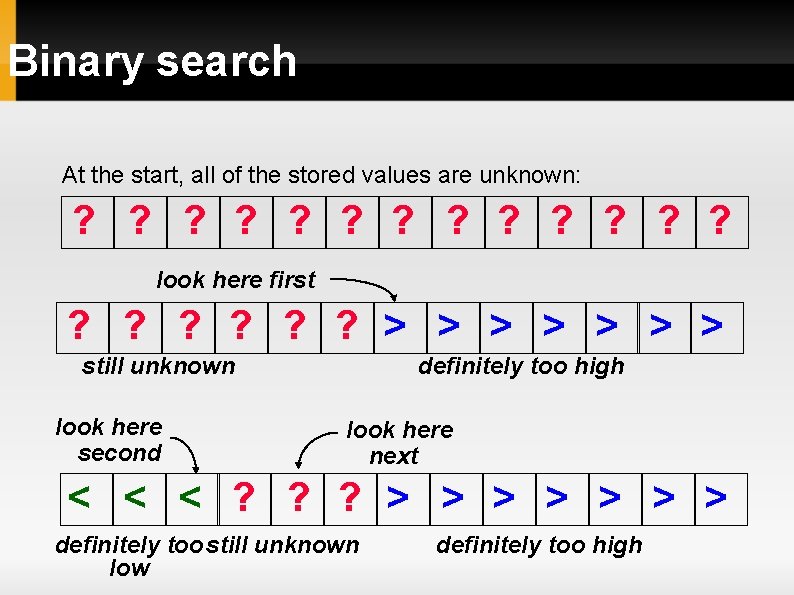
Binary search At the start, all of the stored values are unknown: ? ? ? ? look here first ? ? ? > > > > still unknown look here second definitely too high look here next < < < ? ? ? > > > > definitely too still unknown low definitely too high
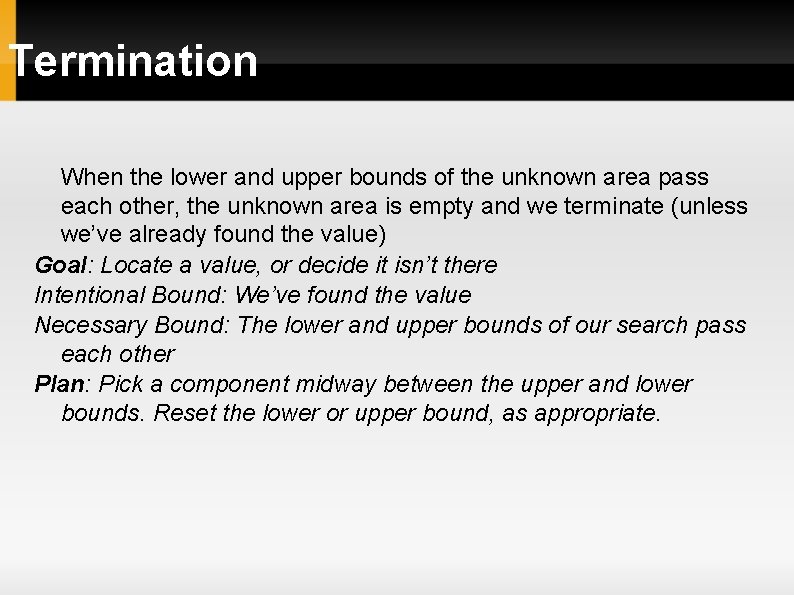
Termination When the lower and upper bounds of the unknown area pass each other, the unknown area is empty and we terminate (unless we’ve already found the value) Goal: Locate a value, or decide it isn’t there Intentional Bound: We’ve found the value Necessary Bound: The lower and upper bounds of our search pass each other Plan: Pick a component midway between the upper and lower bounds. Reset the lower or upper bound, as appropriate.
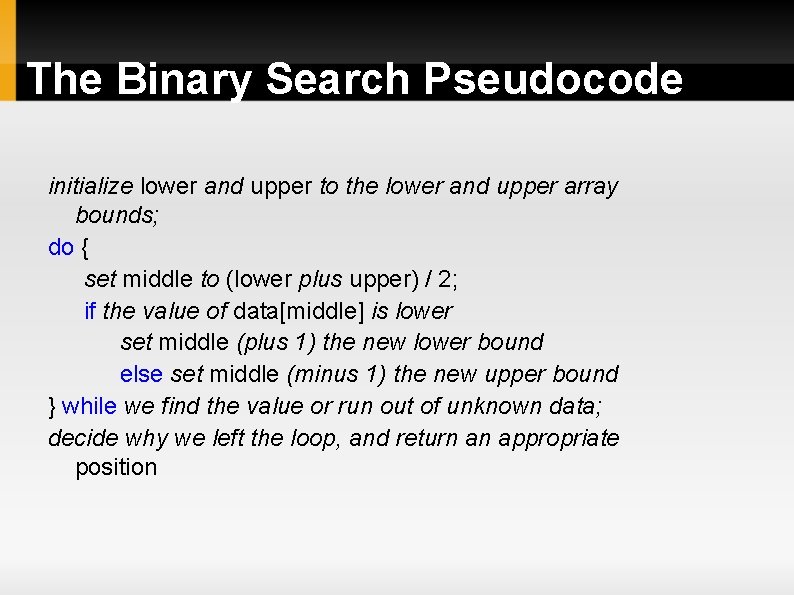
The Binary Search Pseudocode initialize lower and upper to the lower and upper array bounds; do { set middle to (lower plus upper) / 2; if the value of data[middle] is lower set middle (plus 1) the new lower bound else set middle (minus 1) the new upper bound } while we find the value or run out of unknown data; decide why we left the loop, and return an appropriate position
![The Binary Search Java Code int binary Search int data int num The Binary Search Java Code int binary. Search (int[ ] data, int num) {](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-49.jpg)
The Binary Search Java Code int binary. Search (int[ ] data, int num) { // Binary search for num in an ordered array int middle, lower = 0, upper = (data. length - 1); do { middle = ((lower + upper) / 2); if (num < data[middle]) upper = middle - 1; else lower = middle + 1; } while ( (data[middle] != num) && (lower <= upper) ); //Postcondition: if data[middle] isn't num, no // component is if (data[middle] == num) return middle; else return -1; }
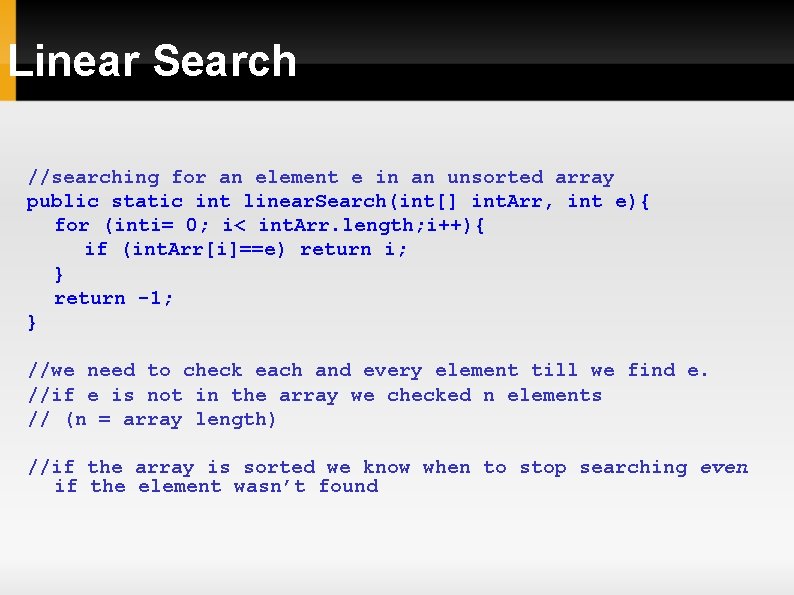
Linear Search //searching for an element e in an unsorted array public static int linear. Search(int[] int. Arr, int e){ for (inti= 0; i< int. Arr. length; i++){ if (int. Arr[i]==e) return i; } return -1; } //we need to check each and every element till we find e. //if e is not in the array we checked n elements // (n = array length) //if the array is sorted we know when to stop searching even if the element wasn’t found
![Linear search in a sorted array public static int linear Searchint int Arr int Linear search in a sorted array public static int linear. Search(int[] int. Arr, int](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-51.jpg)
Linear search in a sorted array public static int linear. Search(int[] int. Arr, int e){ for (inti= 0; i< int. Arr. length; i++){ if (int. Arr[i]==e) { return i; } if (int. Arr[i] > e){ return -1; } } return -1; }
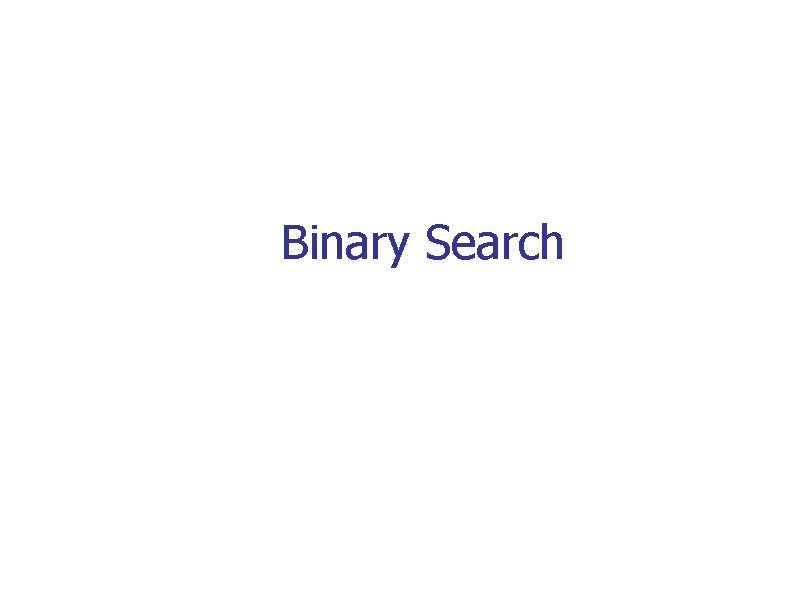
Binary Search
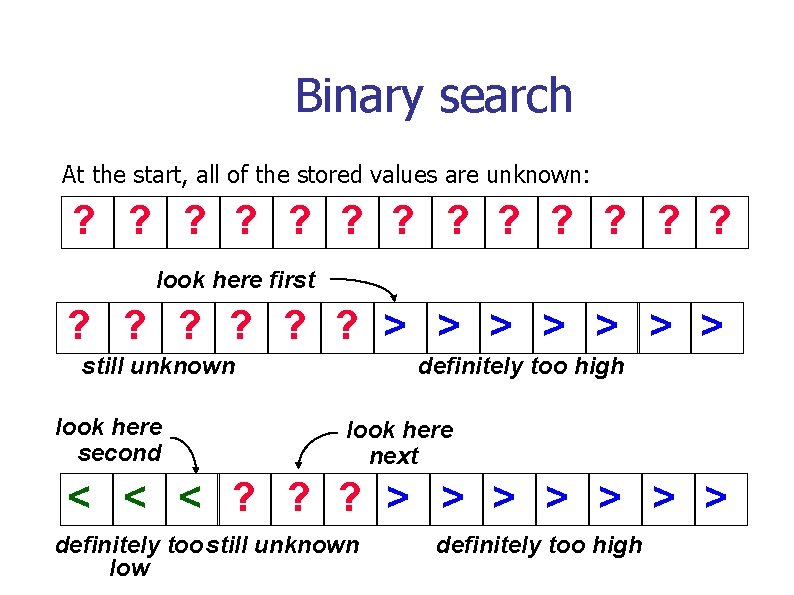
Binary search At the start, all of the stored values are unknown: ? ? ? ? look here first ? ? ? > > > > still unknown look here second definitely too high look here next < < < ? ? ? > > > > definitely too still unknown low definitely too high
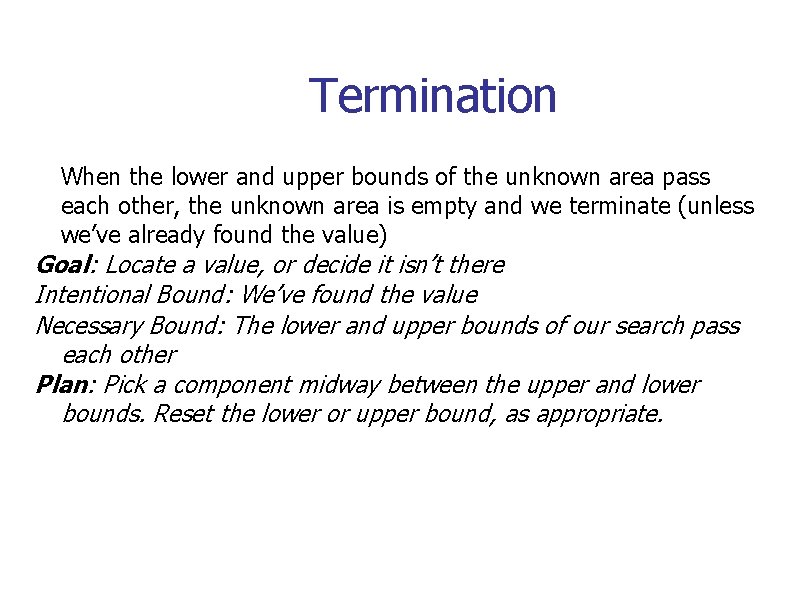
Termination When the lower and upper bounds of the unknown area pass each other, the unknown area is empty and we terminate (unless we’ve already found the value) Goal: Locate a value, or decide it isn’t there Intentional Bound: We’ve found the value Necessary Bound: The lower and upper bounds of our search pass each other Plan: Pick a component midway between the upper and lower bounds. Reset the lower or upper bound, as appropriate.
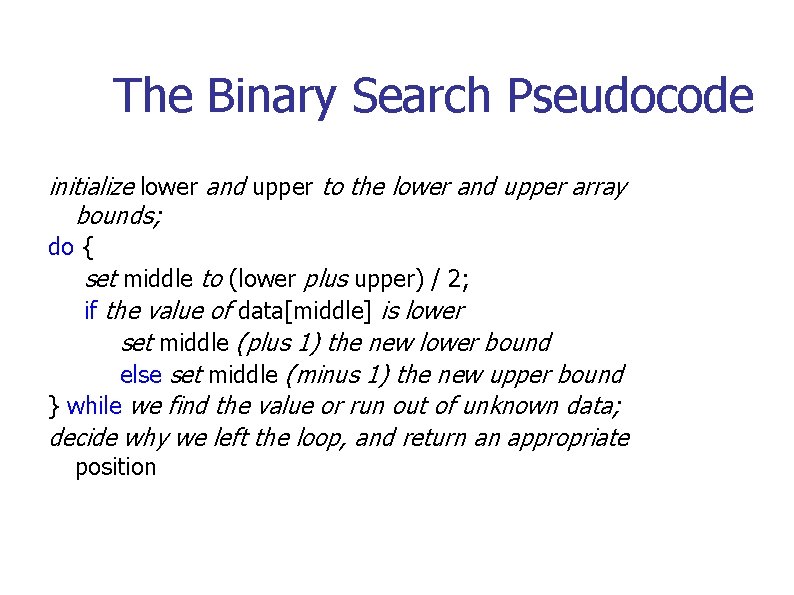
The Binary Search Pseudocode initialize lower and upper to the lower and upper array bounds; do { set middle to (lower plus upper) / 2; if the value of data[middle] is lower set middle (plus 1) the new lower bound else set middle (minus 1) the new upper bound } while we find the value or run out of unknown data; decide why we left the loop, and return an appropriate position
![The Binary Search Java Code int binary Search int data int num The Binary Search Java Code int binary. Search (int[ ] data, int num) {](https://slidetodoc.com/presentation_image_h/9b8e4e063c318891317783952ea3b0ce/image-56.jpg)
The Binary Search Java Code int binary. Search (int[ ] data, int num) { // Binary search for num in an ordered array int middle, lower = 0, upper = (data. length - 1); do { middle = ((lower + upper) / 2); if (num < data[middle]) upper = middle - 1; else lower = middle + 1; } while ( (data[middle] != num) && (lower <= upper) ); //Postcondition: if data[middle] isn't num, no // component is if (data[middle] == num) return middle; else return -1; }
Switch case in unix
A plain scale of 1cm=5m and show on it 37m
Hci adalah
Fortify sourceanalyzer command line
Command line sip client
Tfs command line tools
Command line logo
Export vmware to proxmox
Command line crash course
Rosrun tf tree
Ros publish static transform command line
Tabular editor advanced scripting
Svn command line tutorial
Oracle sql command line
Vertical line in r
Cygwin basic commands
Softwaredistribution download
Fastp command line
Module 5 - command line skills
Notmyfault command line
Jprofiler agent
Sqlcmd portable
Fortify scan results
Fortify sourceanalyzer command line options
Sequencing ap computer science principles
Csp mentoring
Constraint satisfaction problem in ai
Csp checker
Nihr csp
Azure migration to csp
Azure csp migration
Csp yammer
Surface hub proxy settings
Csp office 365 business
Python csp
Csp problem in ai
Csp problem in ai
Constraint satisfaction problem
Backtracking search algorithm for csp
Csp problem in ai
Microsoft csp program
Csp outline
Louise erdrich azure
Csp planning
Sms vs csp
Csp cpd portfolio
Csp sudoku
Cryptarithmetic problem
Csho vs csp
Csp
Csp indirect reseller incentives
Csp min
Csp
Csp
Csp rep
Csp
Isv cloud embed