Getting started in Perl Intro to Perl for
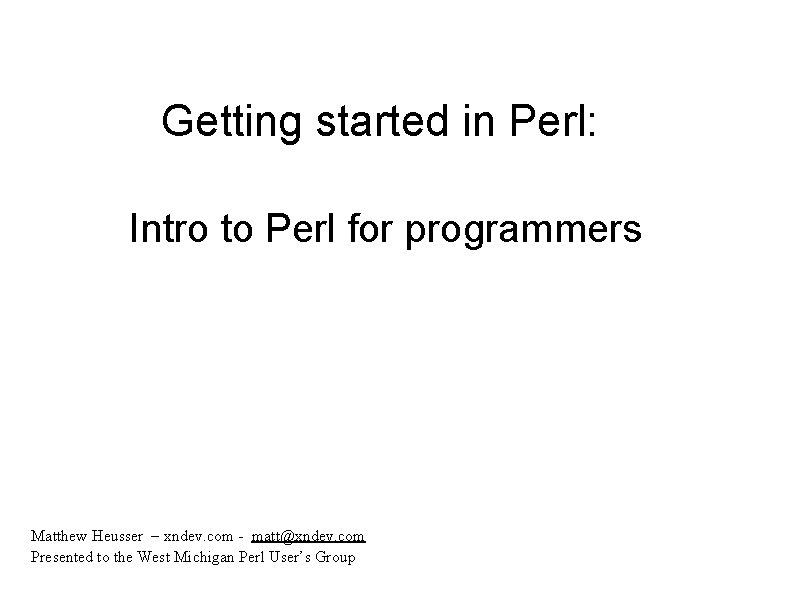
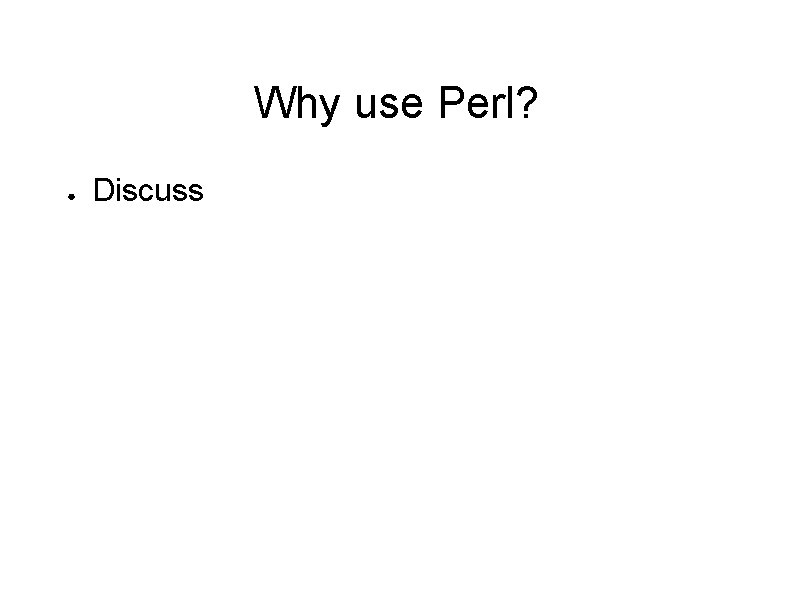
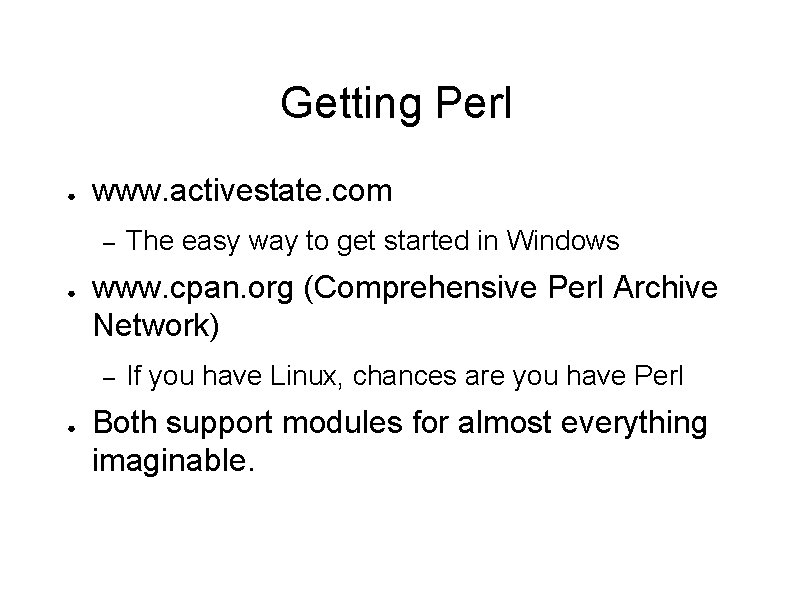
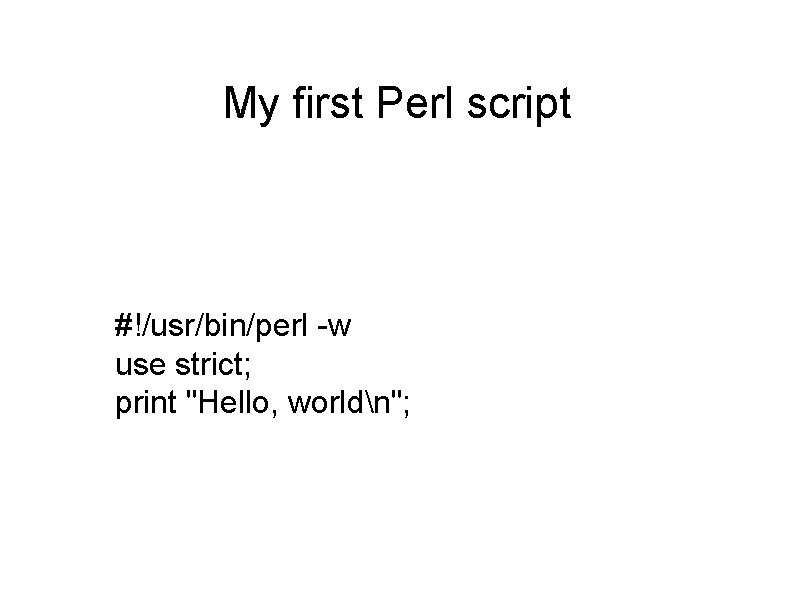
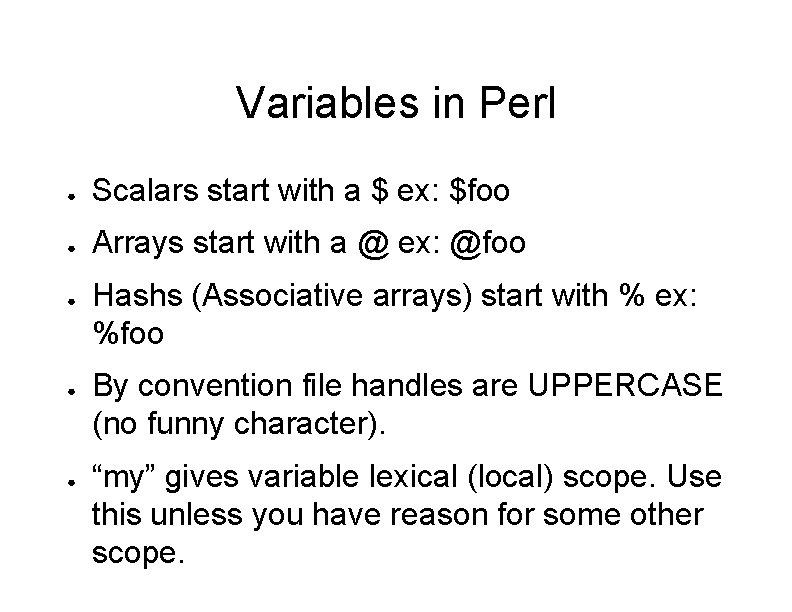
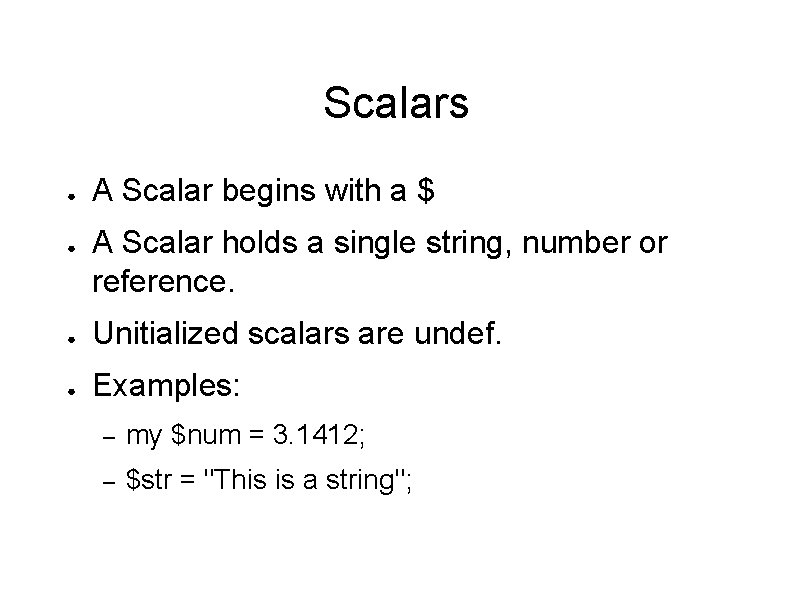
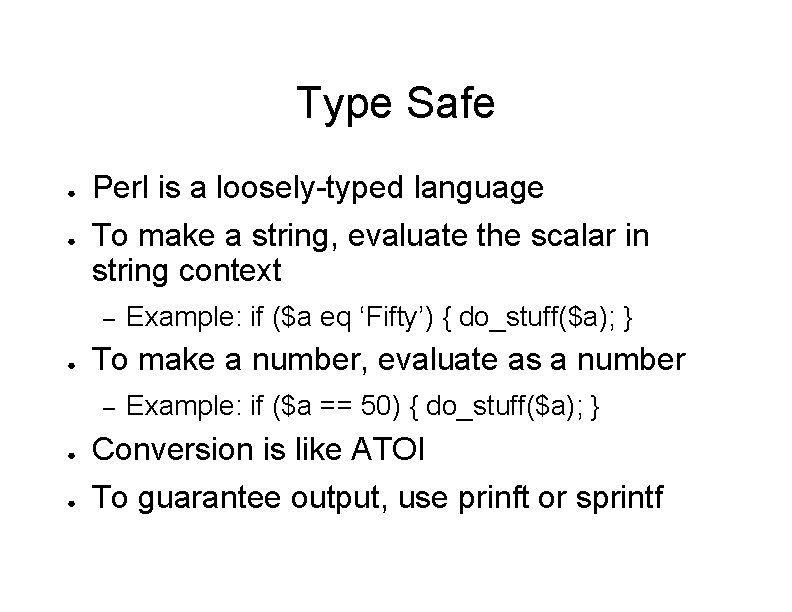
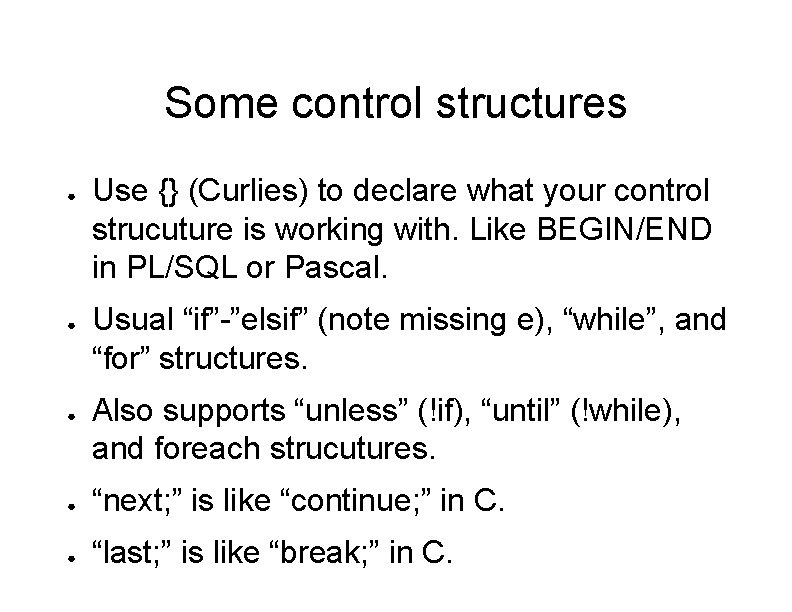
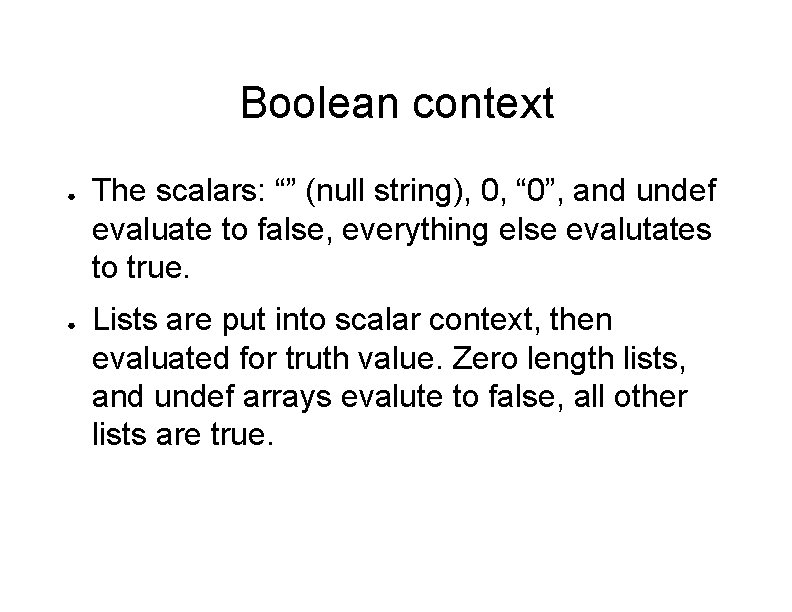
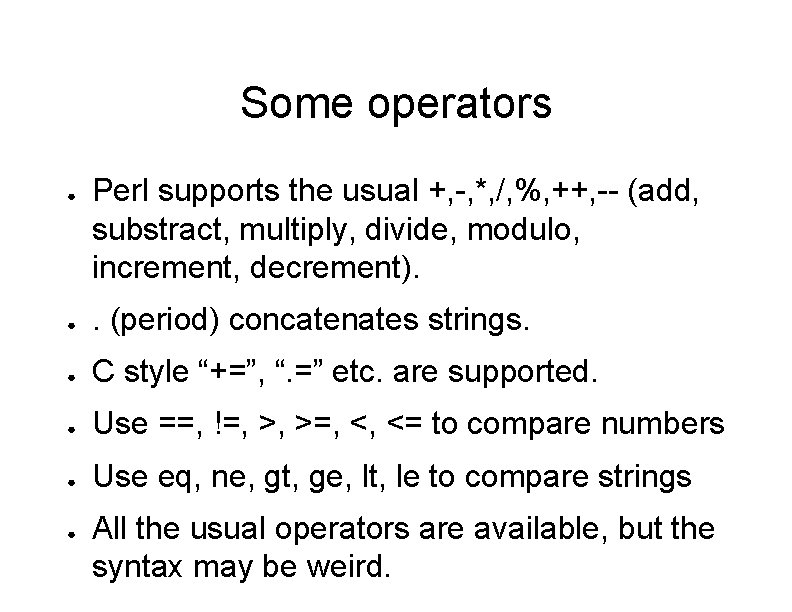
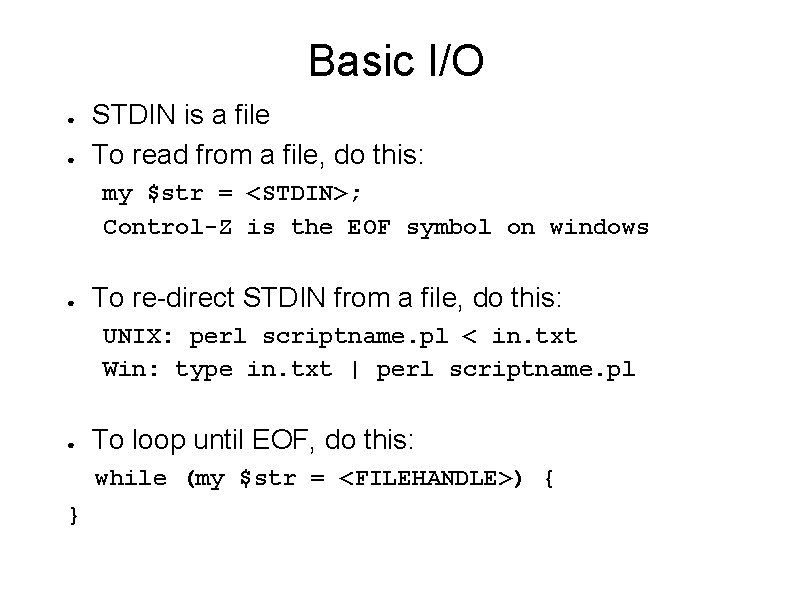
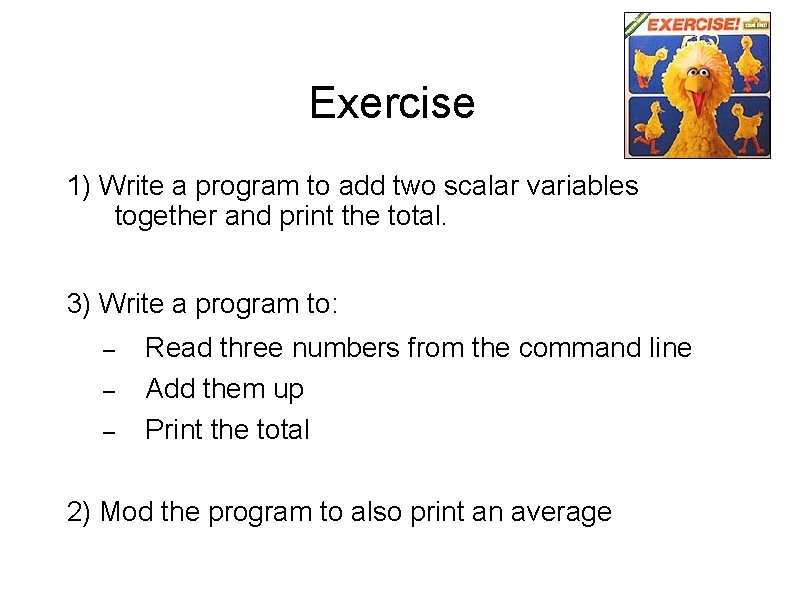
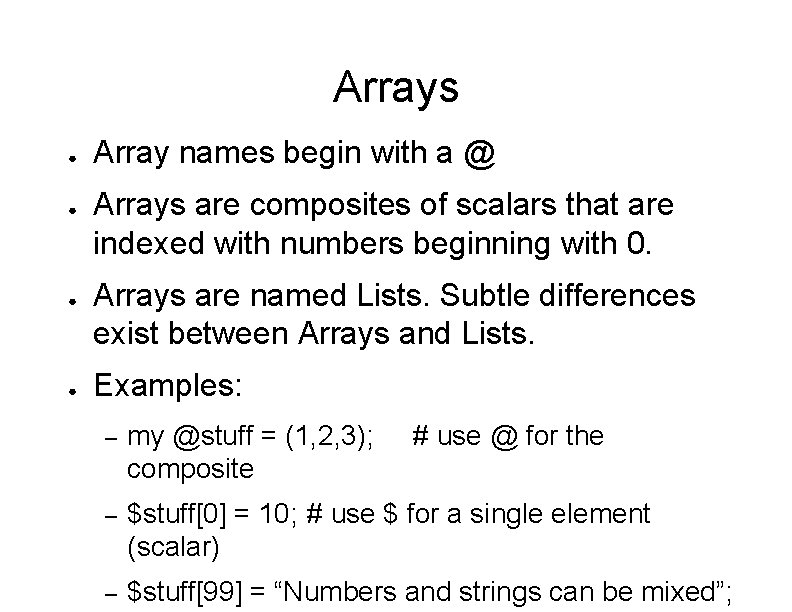
![Iterating over a list for (my $i=0; $i< scalar(@a); $i++) do_something($a[$i]); } for my Iterating over a list for (my $i=0; $i< scalar(@a); $i++) do_something($a[$i]); } for my](https://slidetodoc.com/presentation_image_h2/a0b54ce867e551c83350ec2c11e93e70/image-14.jpg)
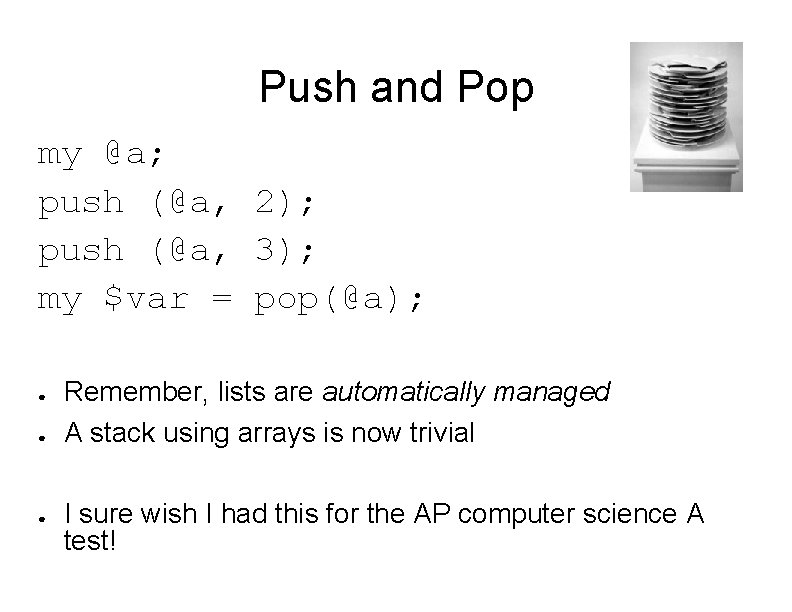
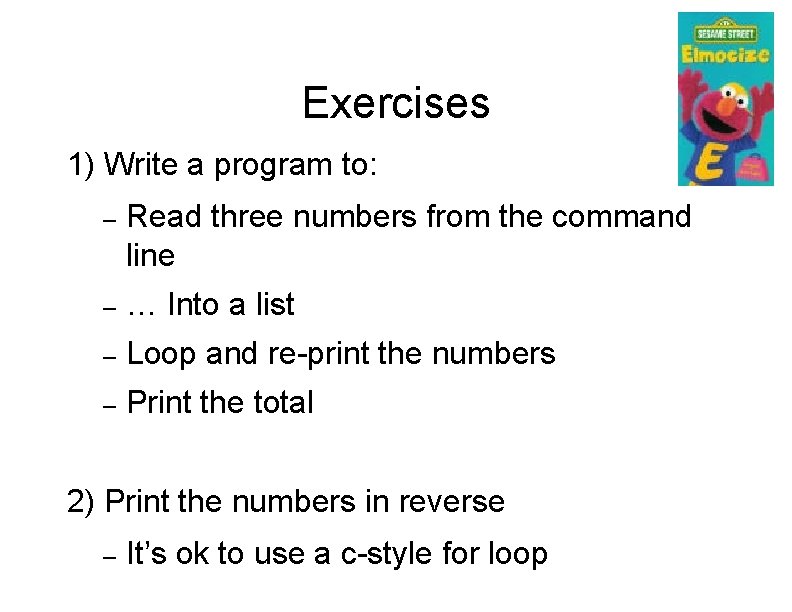
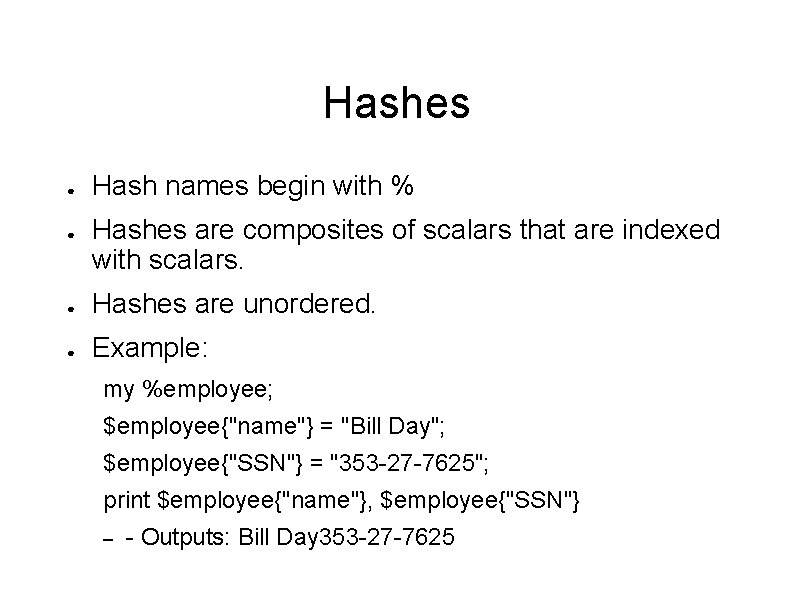
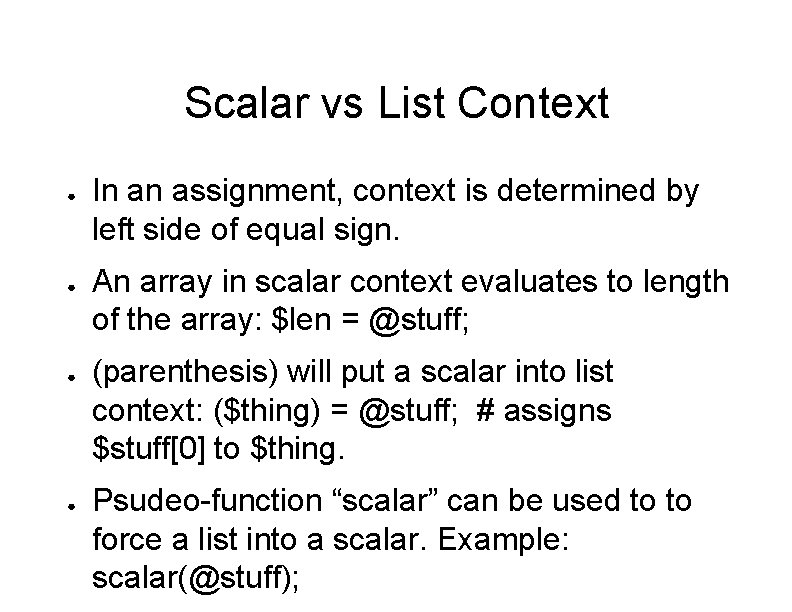
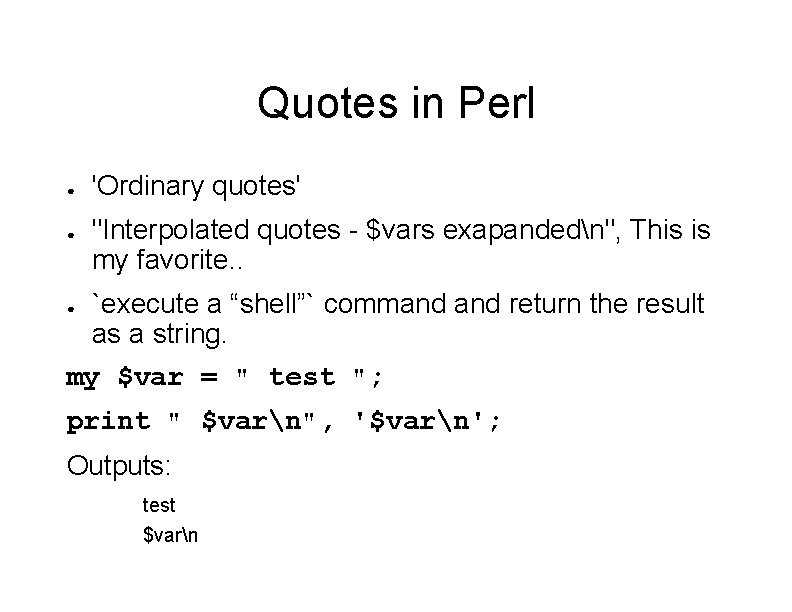
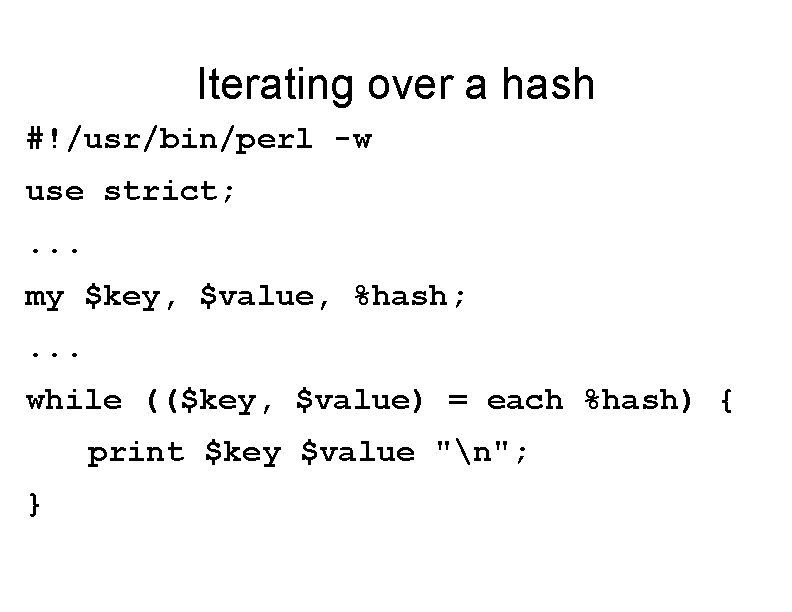
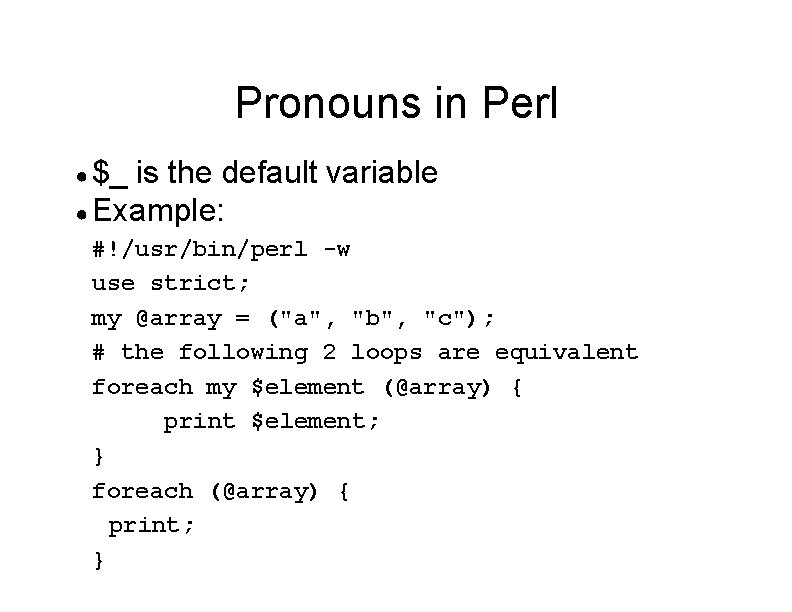
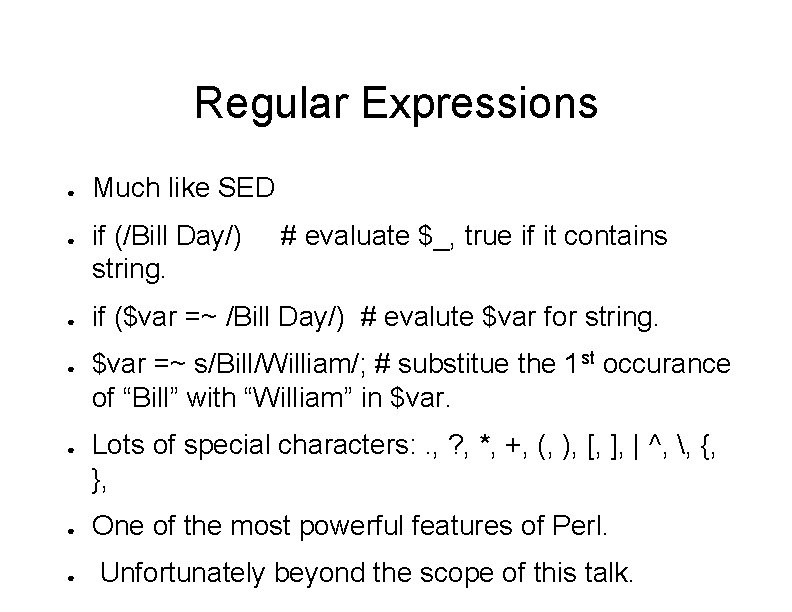
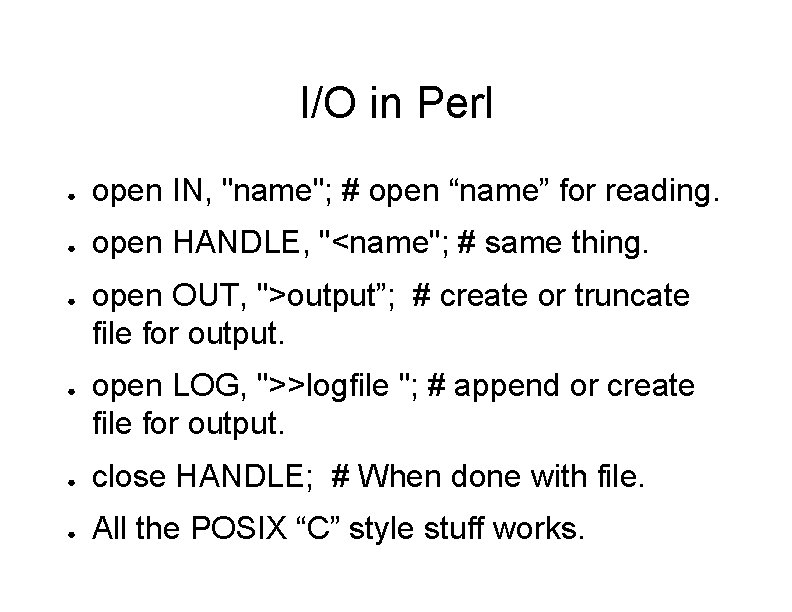
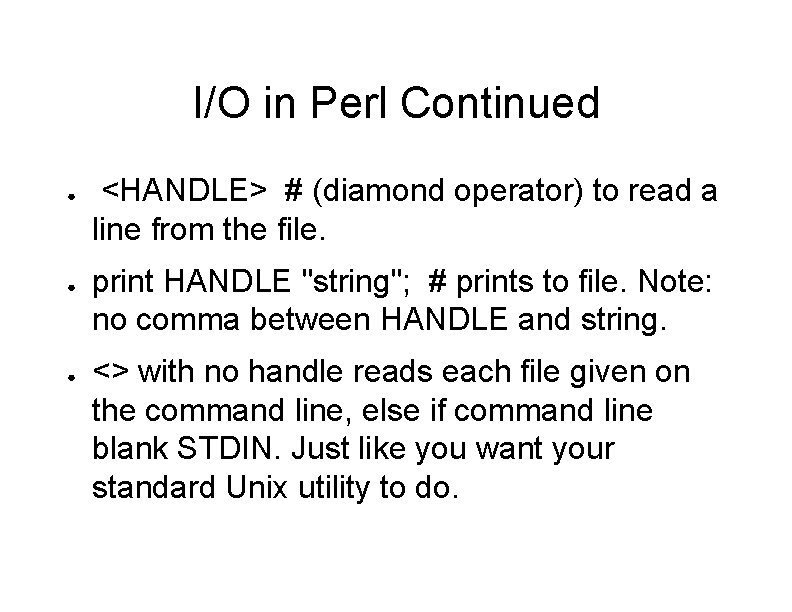
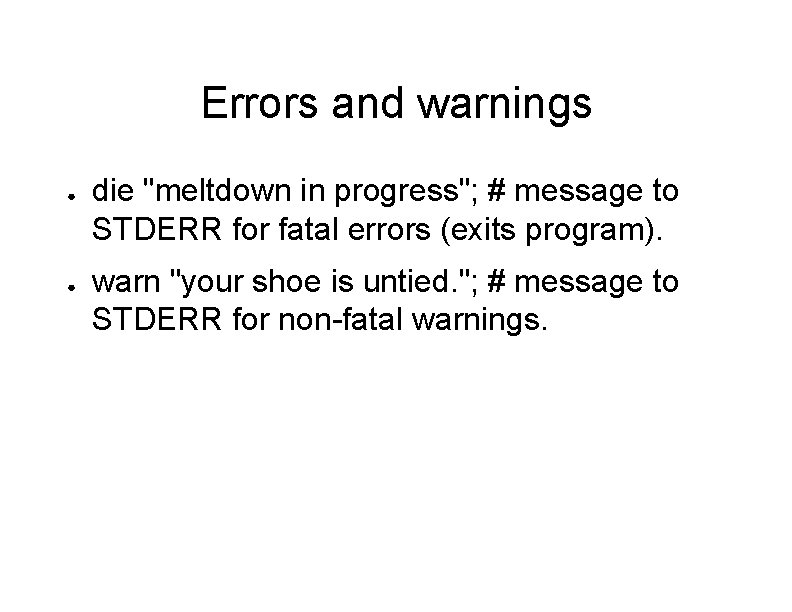
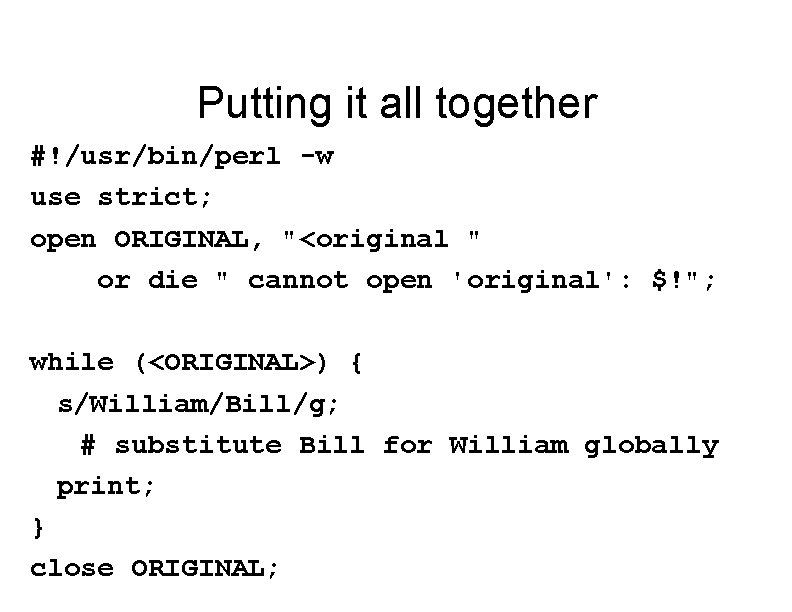
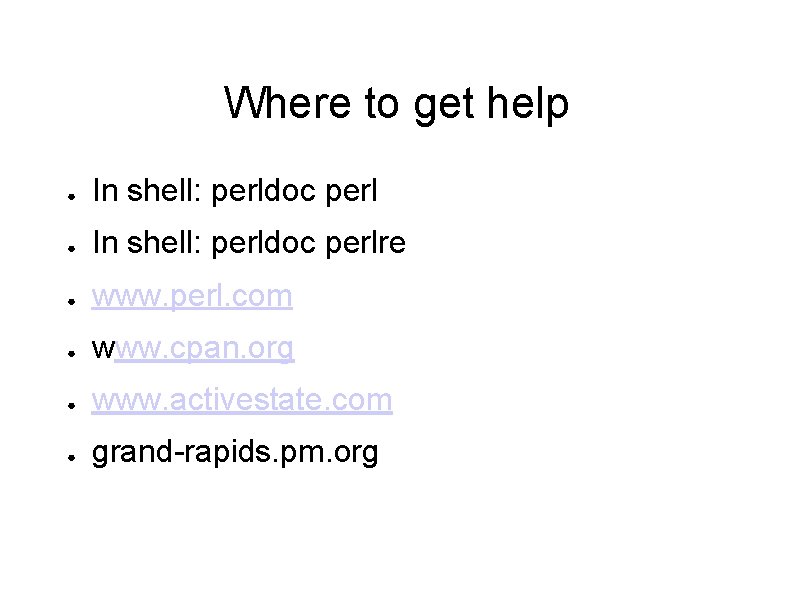
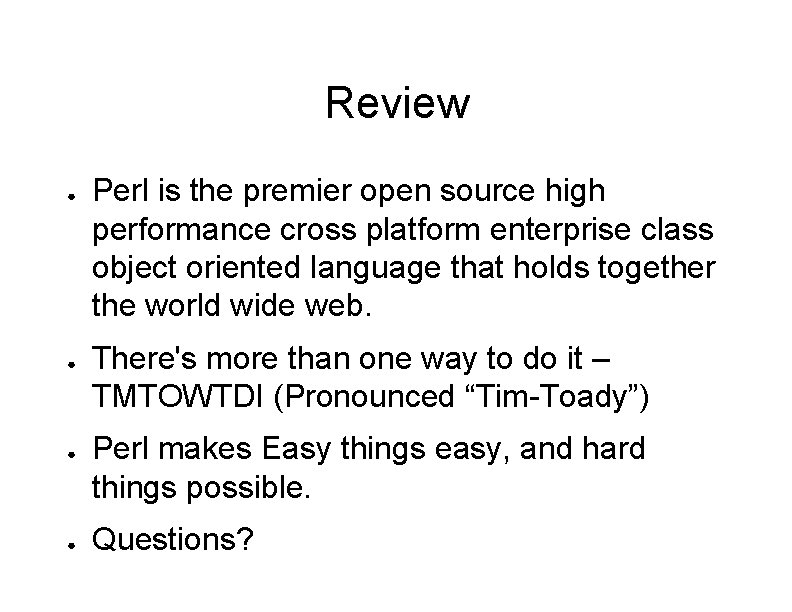
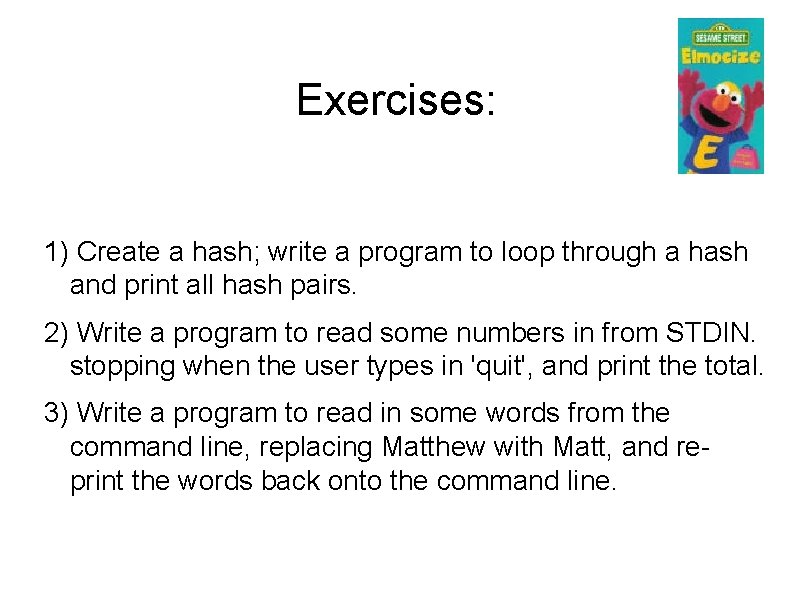
- Slides: 29
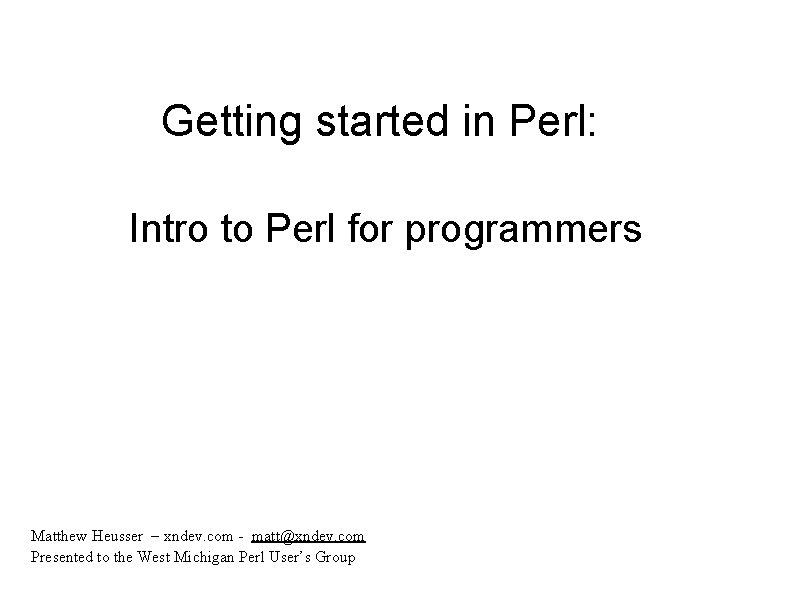
Getting started in Perl: Intro to Perl for programmers Matthew Heusser – xndev. com - matt@xndev. com Presented to the West Michigan Perl User’s Group
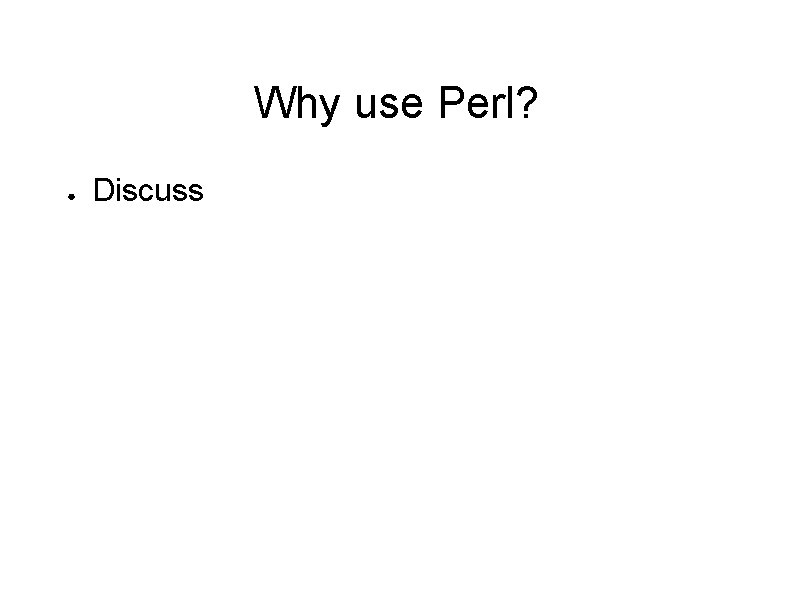
Why use Perl? ● Discuss
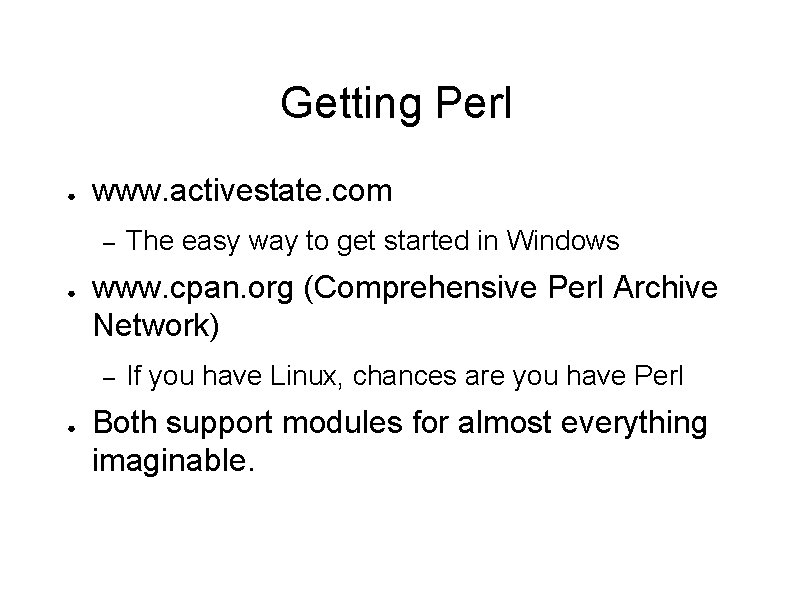
Getting Perl ● www. activestate. com – ● www. cpan. org (Comprehensive Perl Archive Network) – ● The easy way to get started in Windows If you have Linux, chances are you have Perl Both support modules for almost everything imaginable.
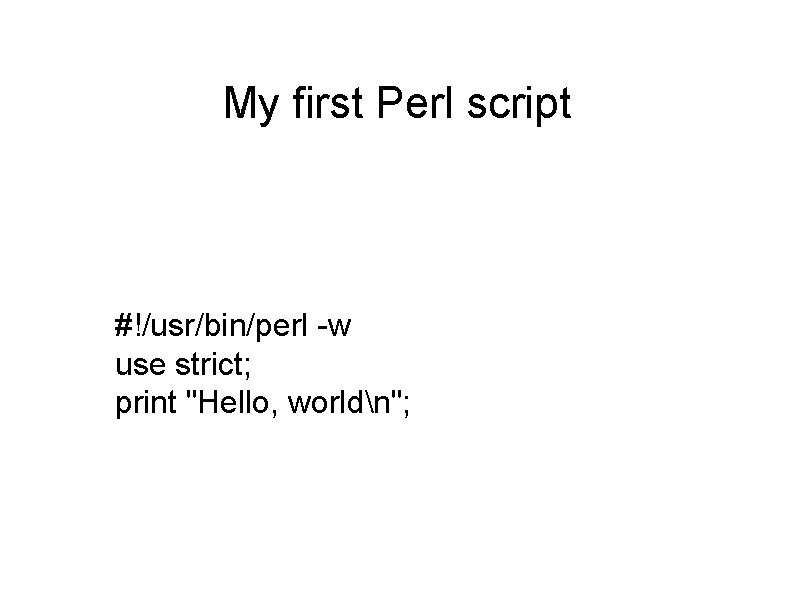
My first Perl script #!/usr/bin/perl -w use strict; print "Hello, worldn";
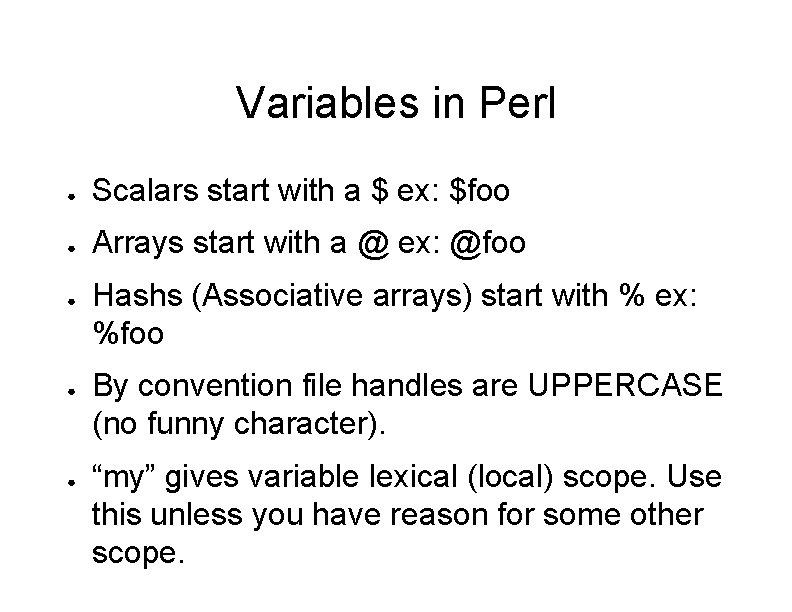
Variables in Perl ● Scalars start with a $ ex: $foo ● Arrays start with a @ ex: @foo ● ● ● Hashs (Associative arrays) start with % ex: %foo By convention file handles are UPPERCASE (no funny character). “my” gives variable lexical (local) scope. Use this unless you have reason for some other scope.
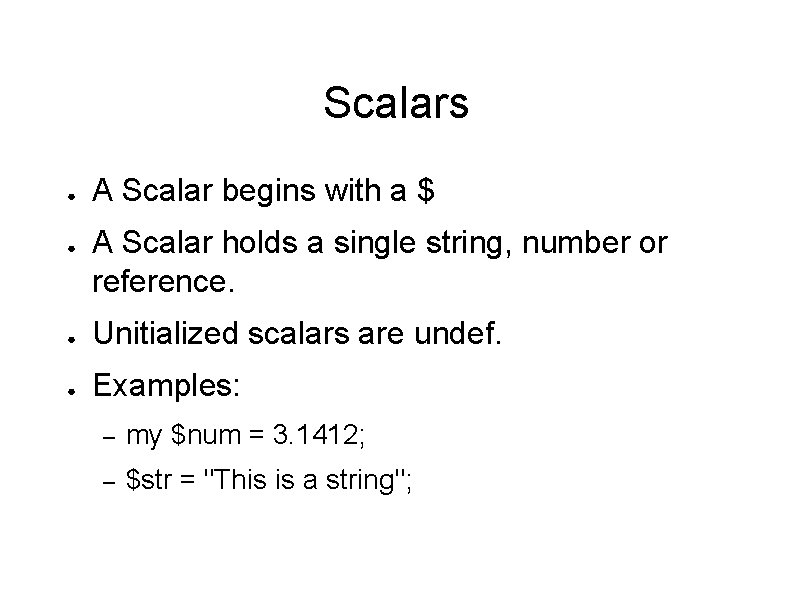
Scalars ● ● A Scalar begins with a $ A Scalar holds a single string, number or reference. ● Unitialized scalars are undef. ● Examples: – my $num = 3. 1412; – $str = "This is a string";
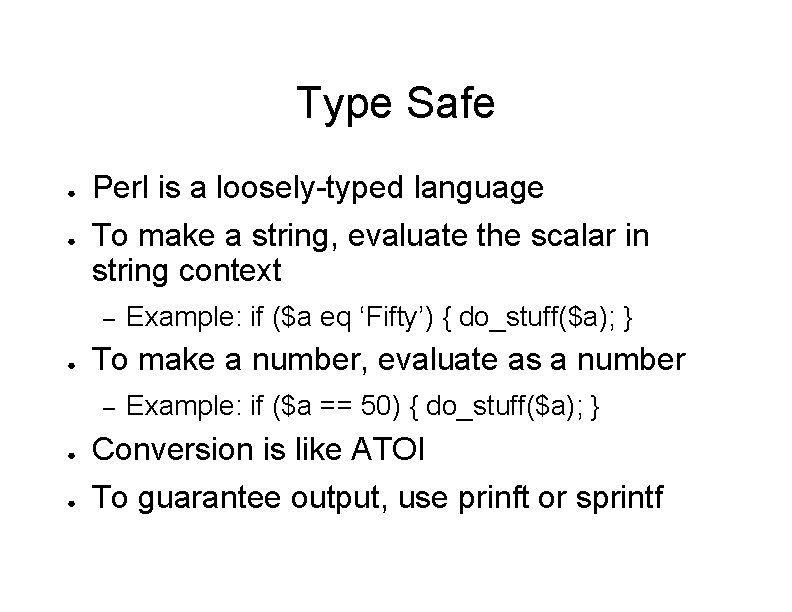
Type Safe ● ● Perl is a loosely-typed language To make a string, evaluate the scalar in string context – ● Example: if ($a eq ‘Fifty’) { do_stuff($a); } To make a number, evaluate as a number – Example: if ($a == 50) { do_stuff($a); } ● Conversion is like ATOI ● To guarantee output, use prinft or sprintf
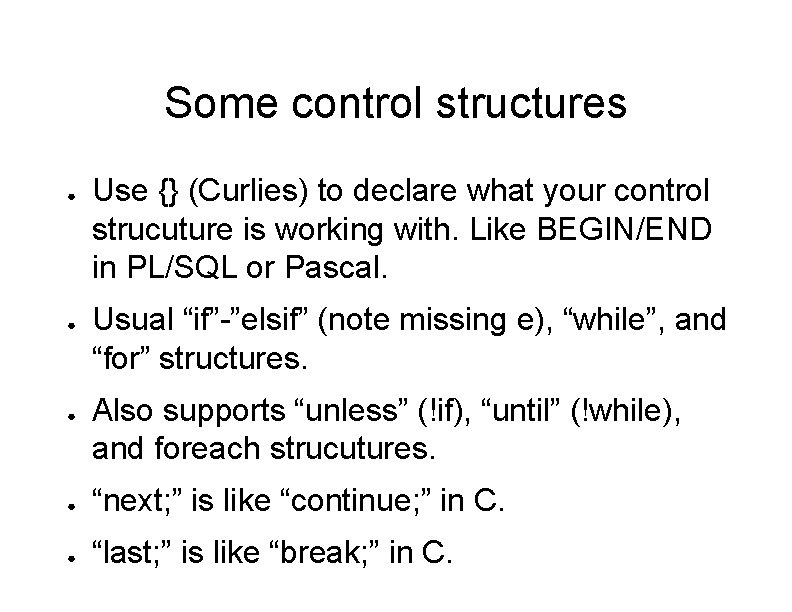
Some control structures ● ● ● Use {} (Curlies) to declare what your control strucuture is working with. Like BEGIN/END in PL/SQL or Pascal. Usual “if”-”elsif” (note missing e), “while”, and “for” structures. Also supports “unless” (!if), “until” (!while), and foreach strucutures. ● “next; ” is like “continue; ” in C. ● “last; ” is like “break; ” in C.
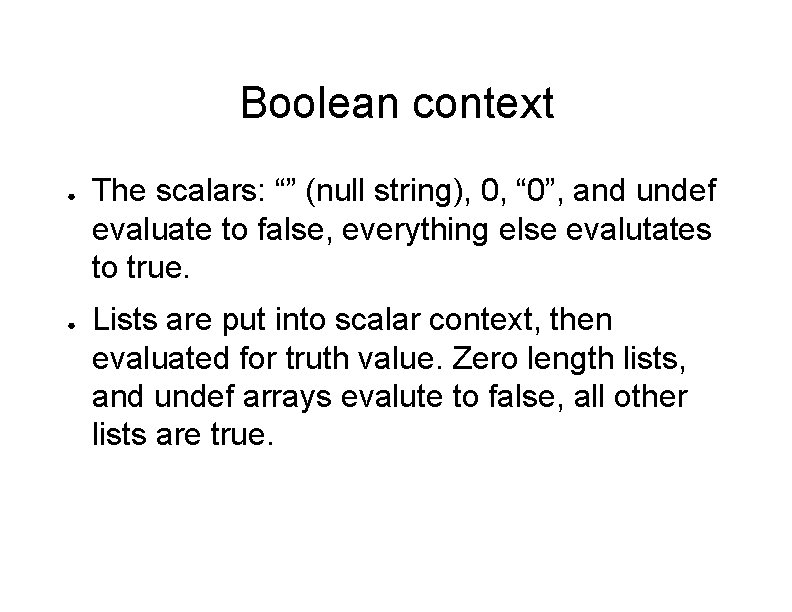
Boolean context ● ● The scalars: “” (null string), 0, “ 0”, and undef evaluate to false, everything else evalutates to true. Lists are put into scalar context, then evaluated for truth value. Zero length lists, and undef arrays evalute to false, all other lists are true.
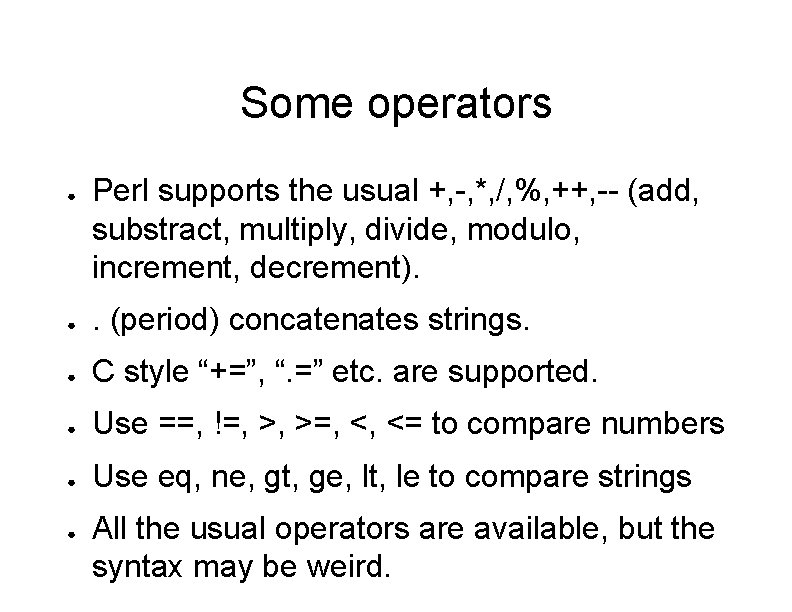
Some operators ● Perl supports the usual +, -, *, /, %, ++, -- (add, substract, multiply, divide, modulo, increment, decrement). ● . (period) concatenates strings. ● C style “+=”, “. =” etc. are supported. ● Use ==, !=, >, >=, <, <= to compare numbers ● Use eq, ne, gt, ge, lt, le to compare strings ● All the usual operators are available, but the syntax may be weird.
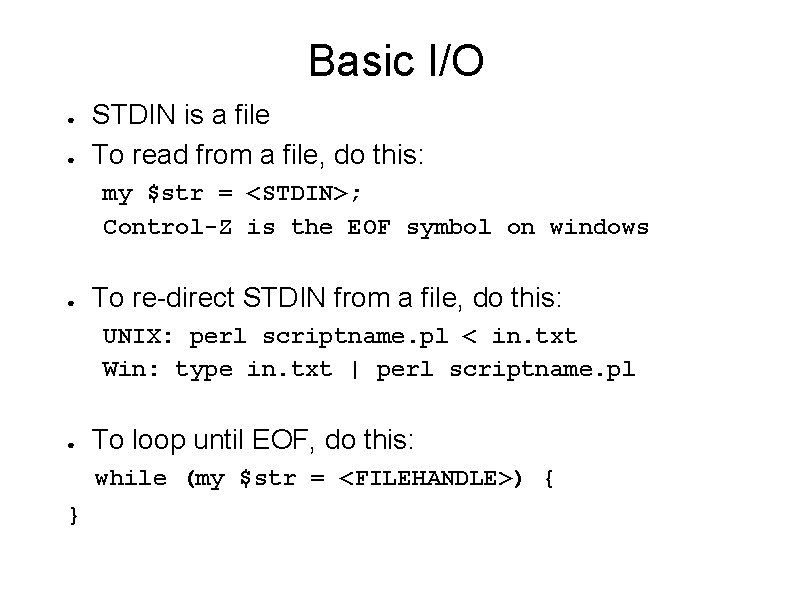
Basic I/O ● ● STDIN is a file To read from a file, do this: my $str = <STDIN>; Control-Z is the EOF symbol on windows ● To re-direct STDIN from a file, do this: UNIX: perl scriptname. pl < in. txt Win: type in. txt | perl scriptname. pl ● To loop until EOF, do this: while (my $str = <FILEHANDLE>) { }
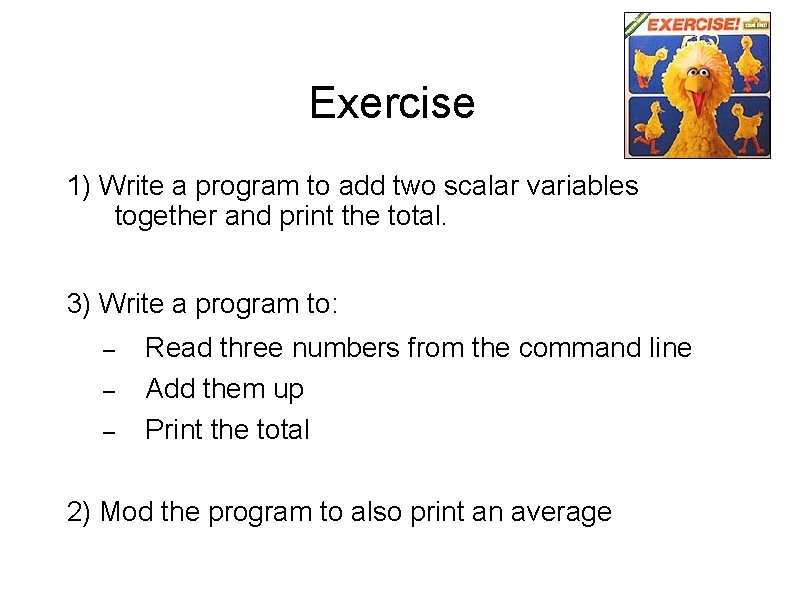
Exercise 1) Write a program to add two scalar variables together and print the total. 3) Write a program to: – – – Read three numbers from the command line Add them up Print the total 2) Mod the program to also print an average
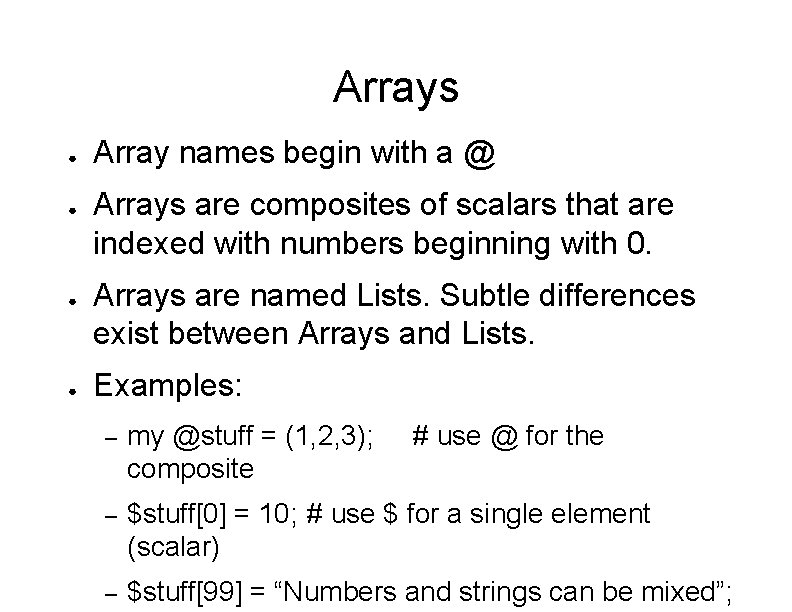
Arrays ● ● Array names begin with a @ Arrays are composites of scalars that are indexed with numbers beginning with 0. Arrays are named Lists. Subtle differences exist between Arrays and Lists. Examples: – my @stuff = (1, 2, 3); composite # use @ for the – $stuff[0] = 10; # use $ for a single element (scalar) – $stuff[99] = “Numbers and strings can be mixed”;
![Iterating over a list for my i0 i scalara i dosomethingai for my Iterating over a list for (my $i=0; $i< scalar(@a); $i++) do_something($a[$i]); } for my](https://slidetodoc.com/presentation_image_h2/a0b54ce867e551c83350ec2c11e93e70/image-14.jpg)
Iterating over a list for (my $i=0; $i< scalar(@a); $i++) do_something($a[$i]); } for my $val (@a) { do_something($val); } {
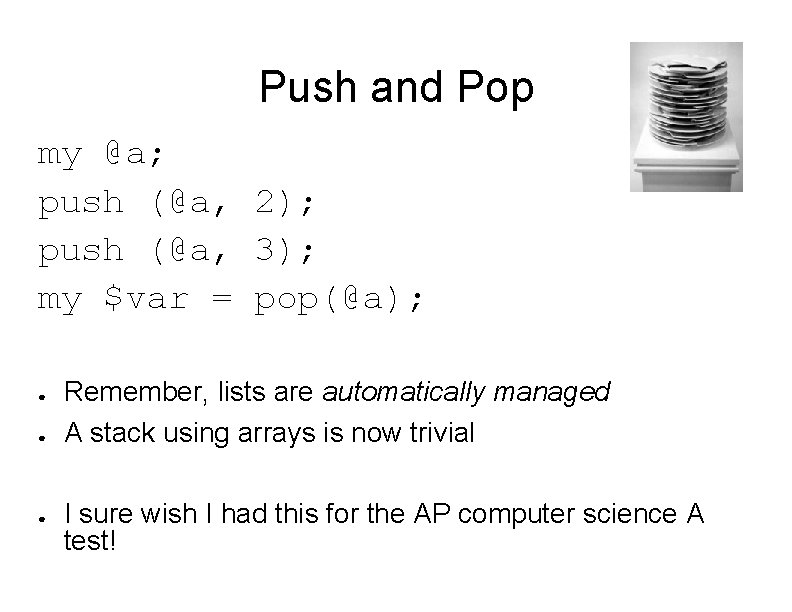
Push and Pop my @a; push (@a, 2); push (@a, 3); my $var = pop(@a); ● ● ● Remember, lists are automatically managed A stack using arrays is now trivial I sure wish I had this for the AP computer science A test!
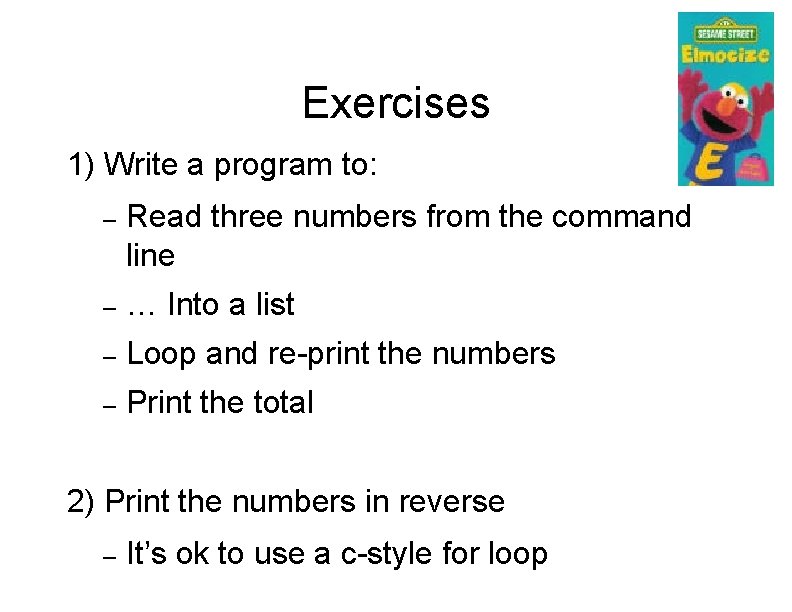
Exercises 1) Write a program to: – Read three numbers from the command line – … Into a list – Loop and re-print the numbers – Print the total 2) Print the numbers in reverse – It’s ok to use a c-style for loop
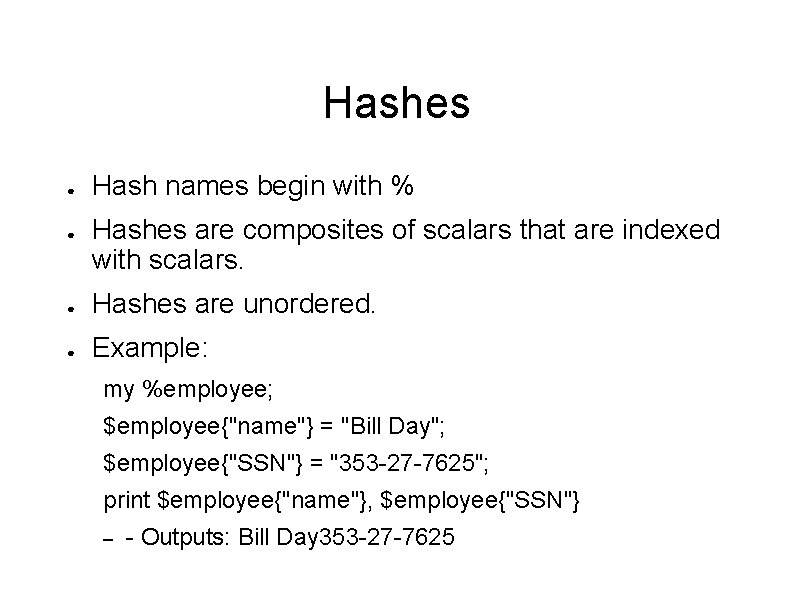
Hashes ● ● Hash names begin with % Hashes are composites of scalars that are indexed with scalars. ● Hashes are unordered. ● Example: my %employee; $employee{"name"} = "Bill Day"; $employee{"SSN"} = "353 -27 -7625"; print $employee{"name"}, $employee{"SSN"} – - Outputs: Bill Day 353 -27 -7625
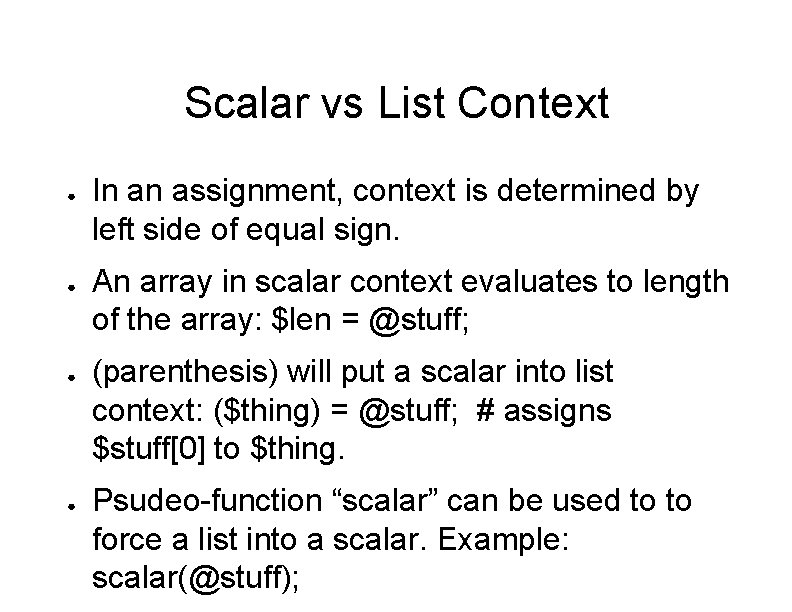
Scalar vs List Context ● ● In an assignment, context is determined by left side of equal sign. An array in scalar context evaluates to length of the array: $len = @stuff; (parenthesis) will put a scalar into list context: ($thing) = @stuff; # assigns $stuff[0] to $thing. Psudeo-function “scalar” can be used to to force a list into a scalar. Example: scalar(@stuff);
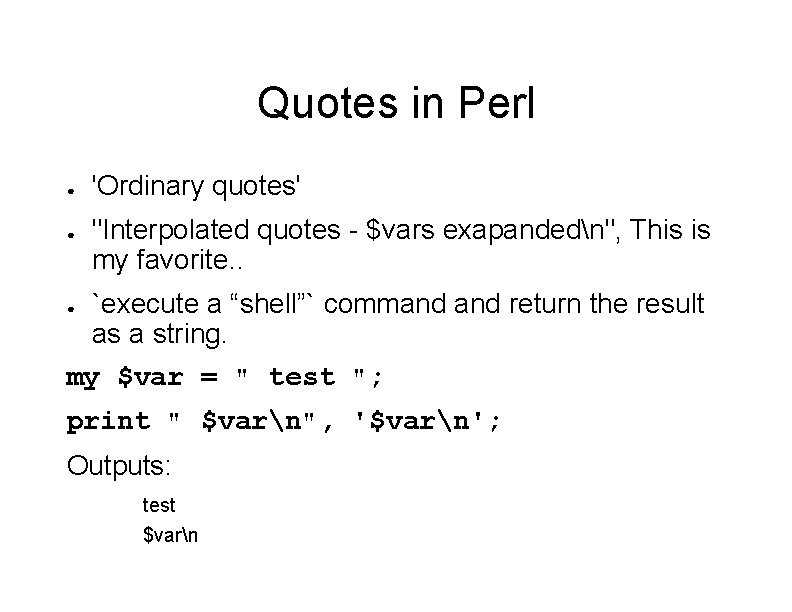
Quotes in Perl ● ● 'Ordinary quotes' "Interpolated quotes - $vars exapandedn", This is my favorite. . `execute a “shell”` command return the result as a string. my $var = " test "; ● print " $varn", '$varn'; Outputs: test $varn
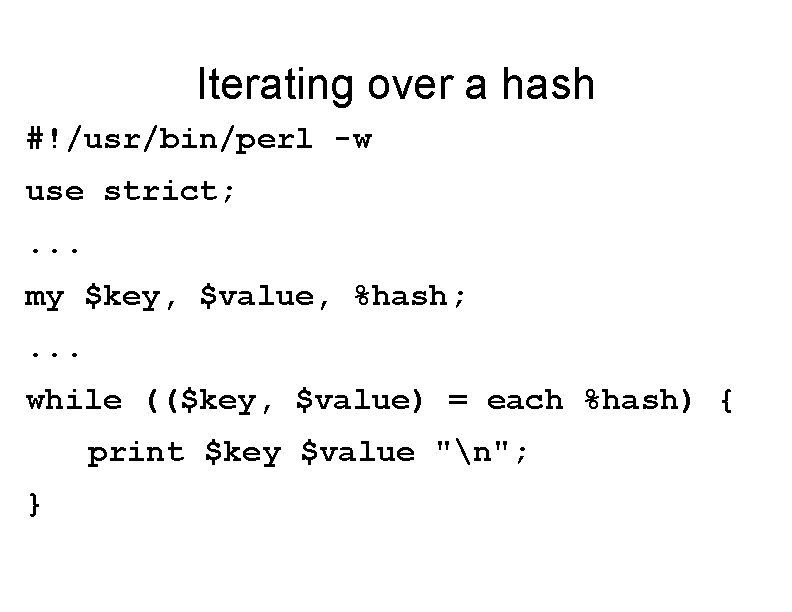
Iterating over a hash #!/usr/bin/perl -w use strict; . . . my $key, $value, %hash; . . . while (($key, $value) = each %hash) { print $key $value "n"; }
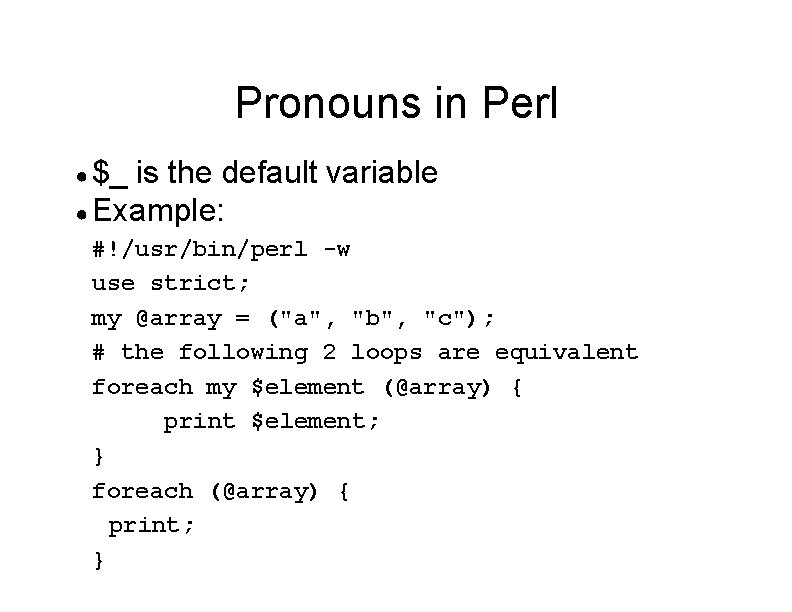
Pronouns in Perl ● $_ is the default variable ● Example: #!/usr/bin/perl -w use strict; my @array = ("a", "b", "c"); # the following 2 loops are equivalent foreach my $element (@array) { print $element; } foreach (@array) { print; }
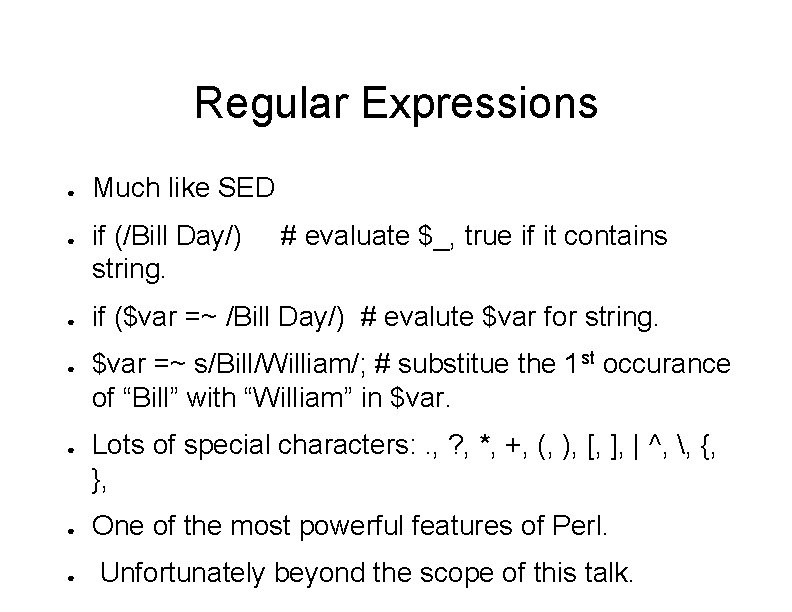
Regular Expressions ● ● ● ● Much like SED if (/Bill Day/) string. # evaluate $_, true if it contains if ($var =~ /Bill Day/) # evalute $var for string. $var =~ s/Bill/William/; # substitue the 1 st occurance of “Bill” with “William” in $var. Lots of special characters: . , ? , *, +, (, ), [, ], | ^, , {, }, One of the most powerful features of Perl. Unfortunately beyond the scope of this talk.
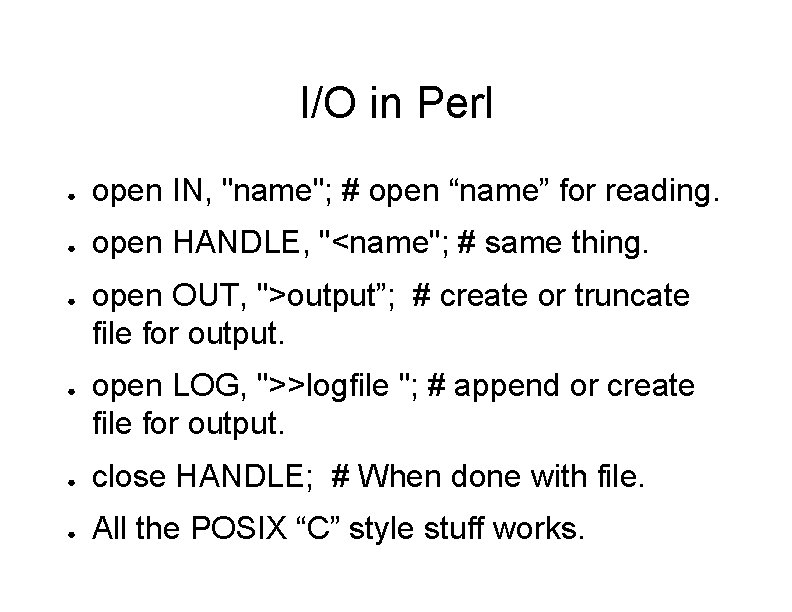
I/O in Perl ● open IN, "name"; # open “name” for reading. ● open HANDLE, "<name"; # same thing. ● ● open OUT, ">output”; # create or truncate file for output. open LOG, ">>logfile "; # append or create file for output. ● close HANDLE; # When done with file. ● All the POSIX “C” style stuff works.
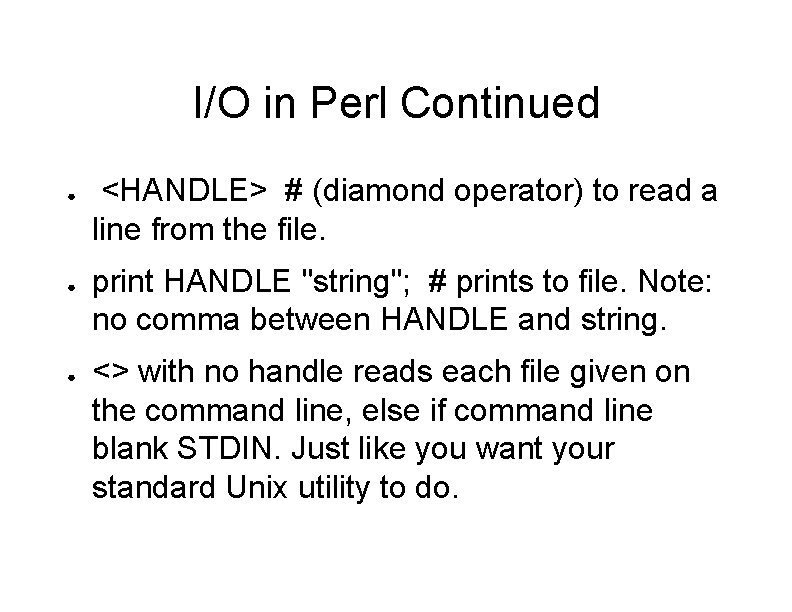
I/O in Perl Continued ● ● ● <HANDLE> # (diamond operator) to read a line from the file. print HANDLE "string"; # prints to file. Note: no comma between HANDLE and string. <> with no handle reads each file given on the command line, else if command line blank STDIN. Just like you want your standard Unix utility to do.
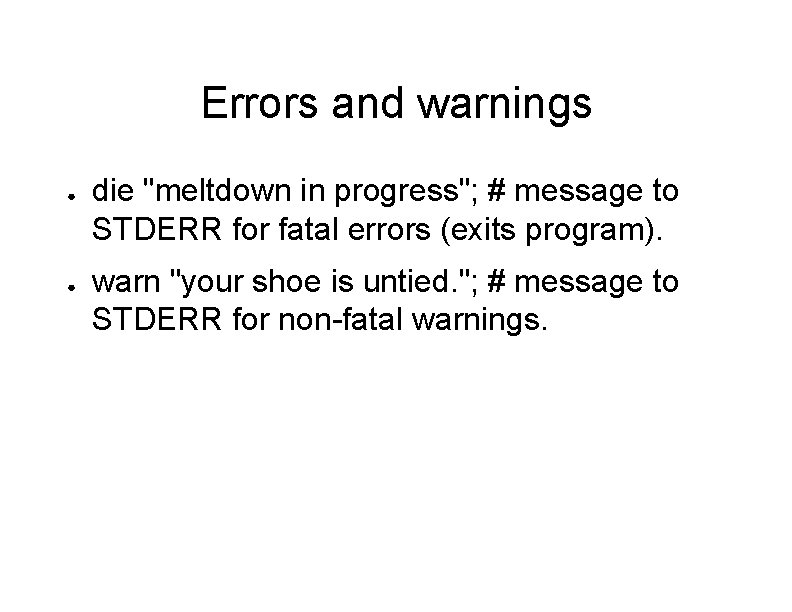
Errors and warnings ● ● die "meltdown in progress"; # message to STDERR for fatal errors (exits program). warn "your shoe is untied. "; # message to STDERR for non-fatal warnings.
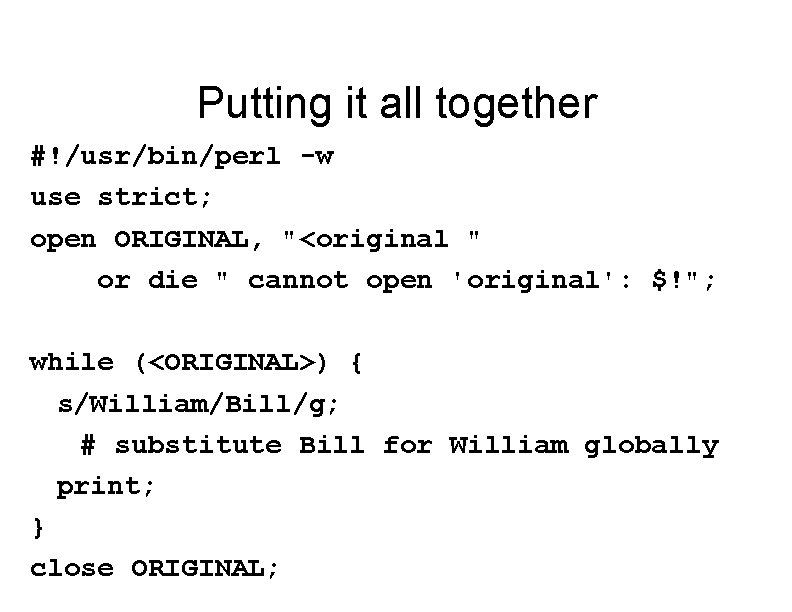
Putting it all together #!/usr/bin/perl -w use strict; open ORIGINAL, "<original " or die " cannot open 'original': $!"; while (<ORIGINAL>) { s/William/Bill/g; # substitute Bill for William globally print; } close ORIGINAL;
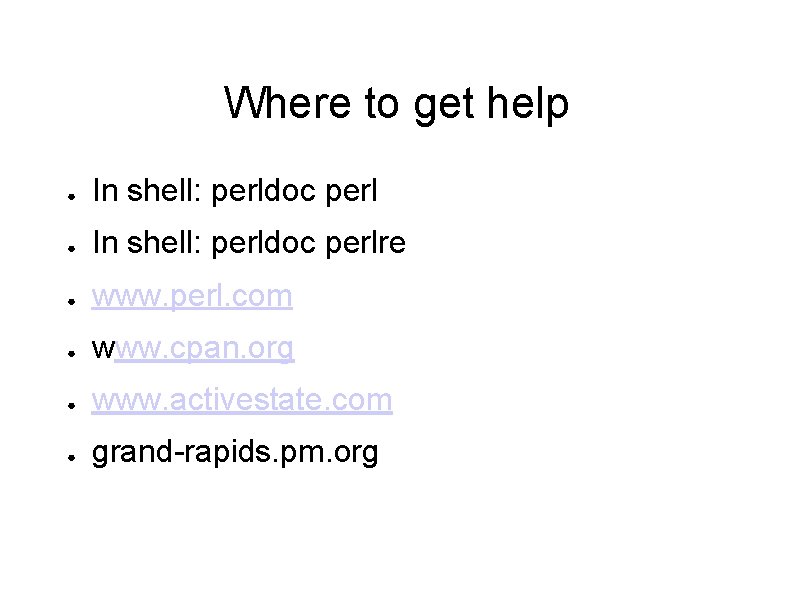
Where to get help ● In shell: perldoc perlre ● www. perl. com ● www. cpan. org ● www. activestate. com ● grand-rapids. pm. org
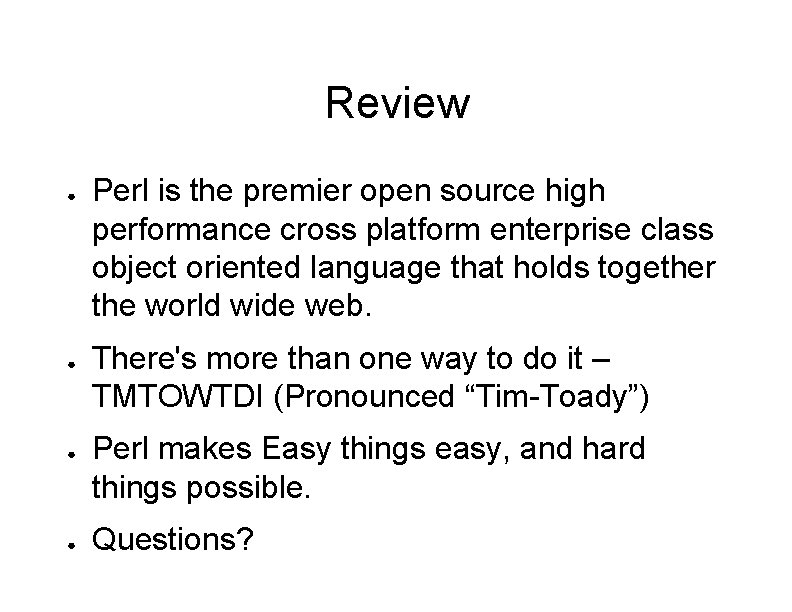
Review ● ● Perl is the premier open source high performance cross platform enterprise class object oriented language that holds together the world wide web. There's more than one way to do it – TMTOWTDI (Pronounced “Tim-Toady”) Perl makes Easy things easy, and hard things possible. Questions?
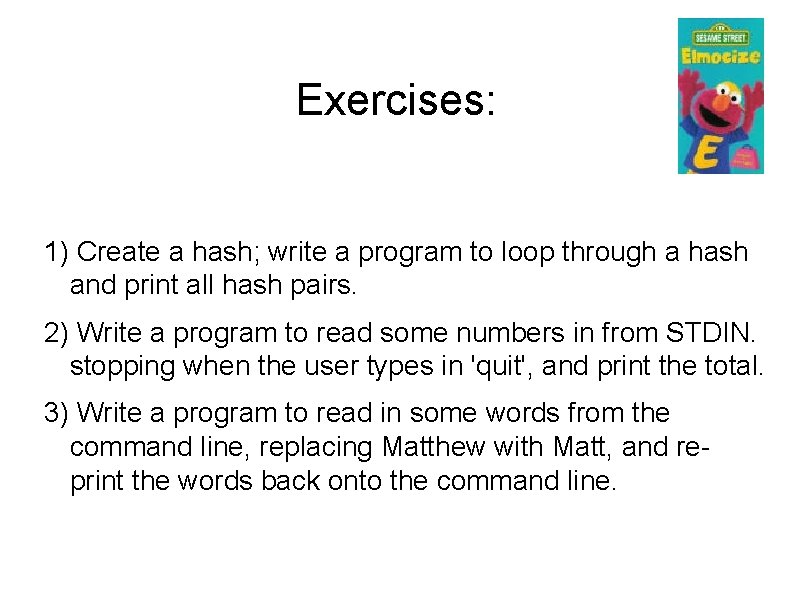
Exercises: 1) Create a hash; write a program to loop through a hash and print all hash pairs. 2) Write a program to read some numbers in from STDIN. stopping when the user types in 'quit', and print the total. 3) Write a program to read in some words from the command line, replacing Matthew with Matt, and reprint the words back onto the command line.
Perl getting started
The secret of getting ahead is getting started
Splunk getting started
Getting started with poll everywhere
Getting started with xilinx fpga
Mathematica getting started
Getting started with ft8
Find these things in unit 1
Rancher getting started
Android development getting started
Lua getting started
Getting started with vivado ip integrator
Unit 2 listen and read
Infuecers gone wild
Getting started with excel
Getting started with access
When does elena receive dolls from her family members
Unix for bioinformatics
Unit 1 getting started
Hi3ms
Getting started with microsoft outlook learning
Getting started with eclipse
Unit 1 local environment getting started
Intro to perl
Kanaans land
Cellorov
Lyckans minut erik lindorm analys
Strategi för svensk viltförvaltning
Ro i rom pax
Tack för att ni lyssnade bild