2 Perl What is Perl http www perl
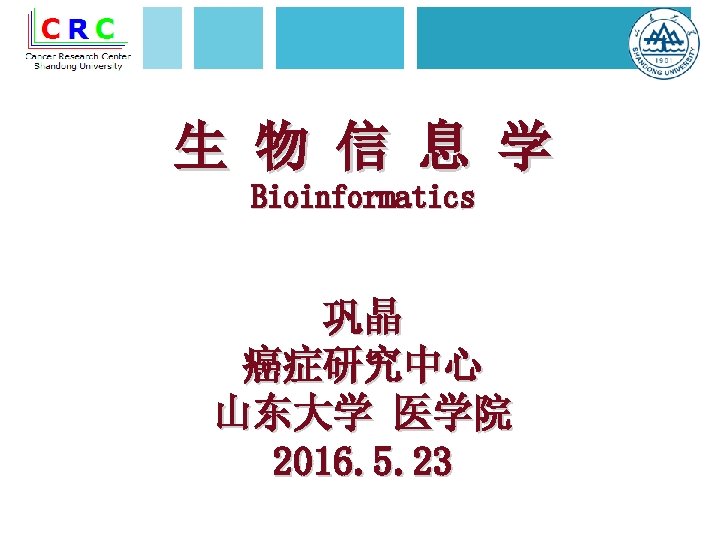
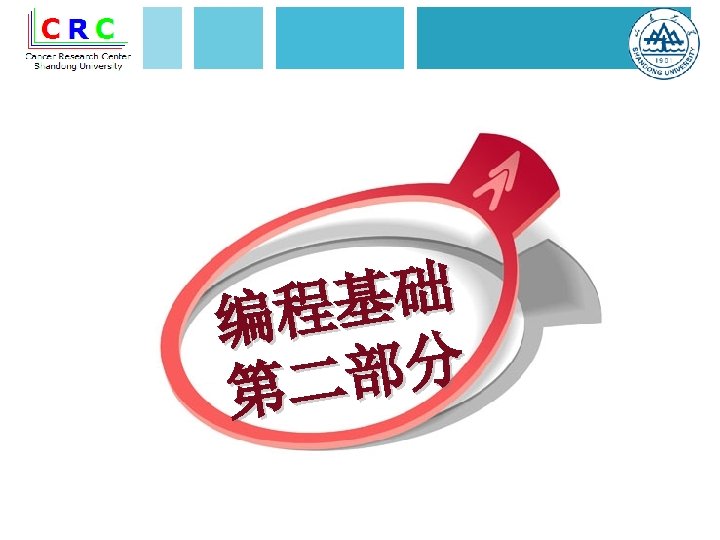
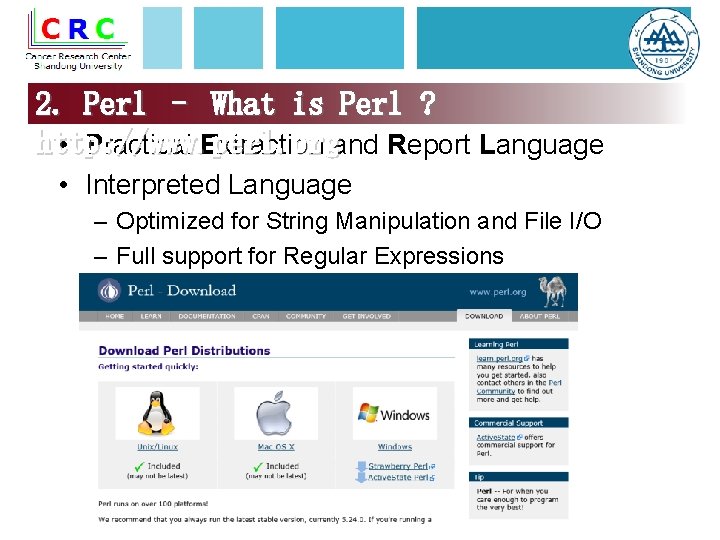
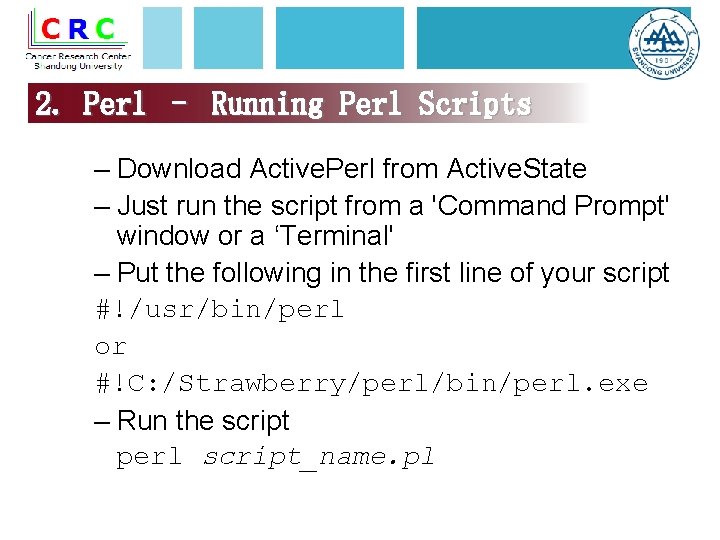
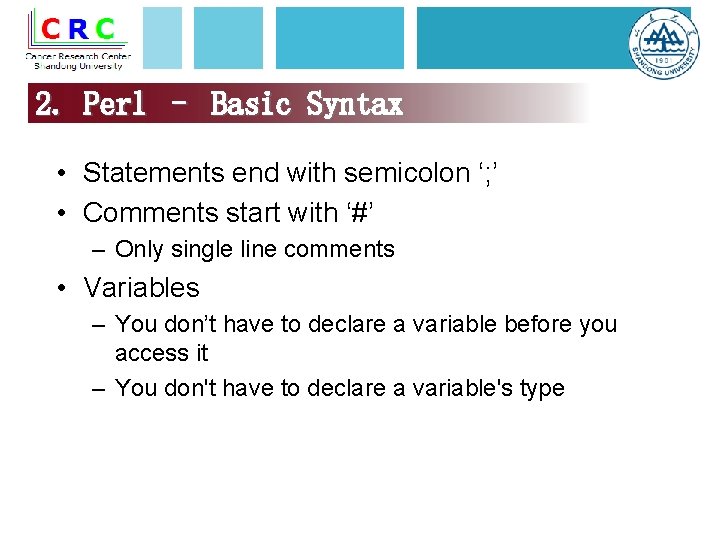
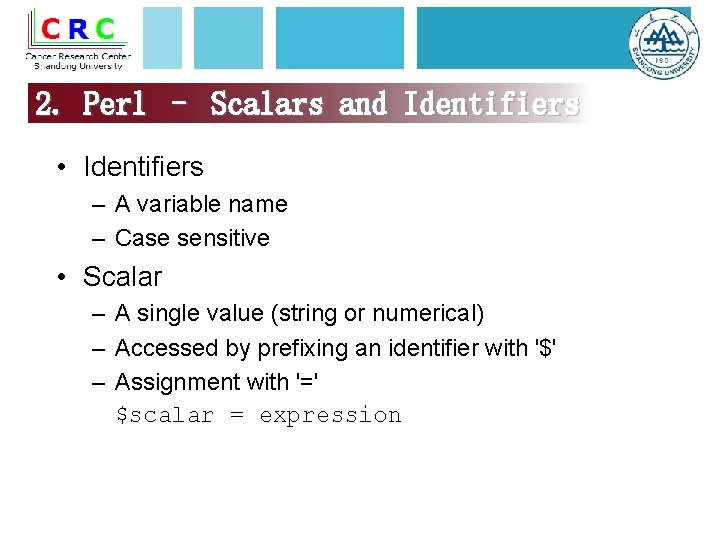
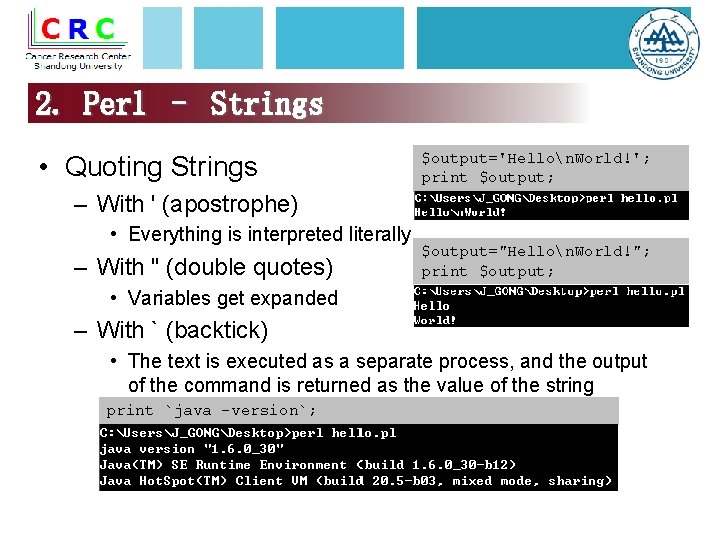
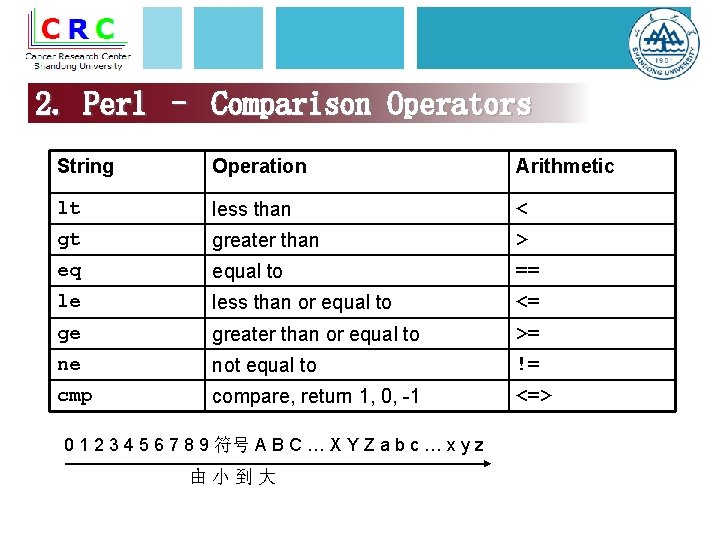
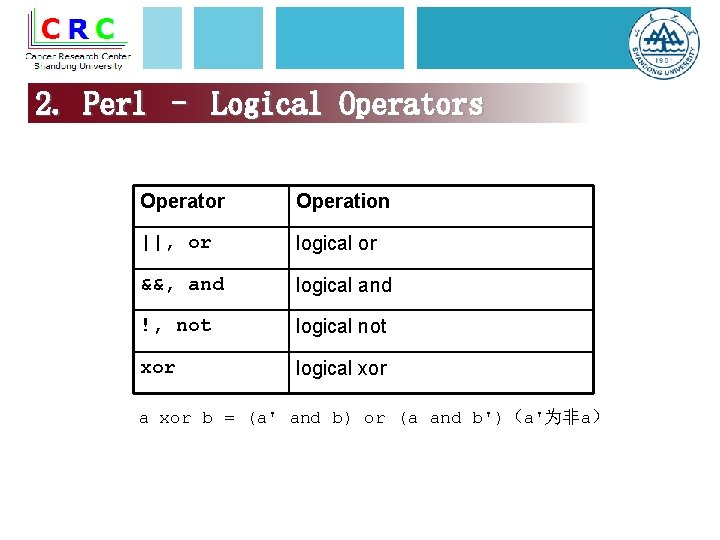
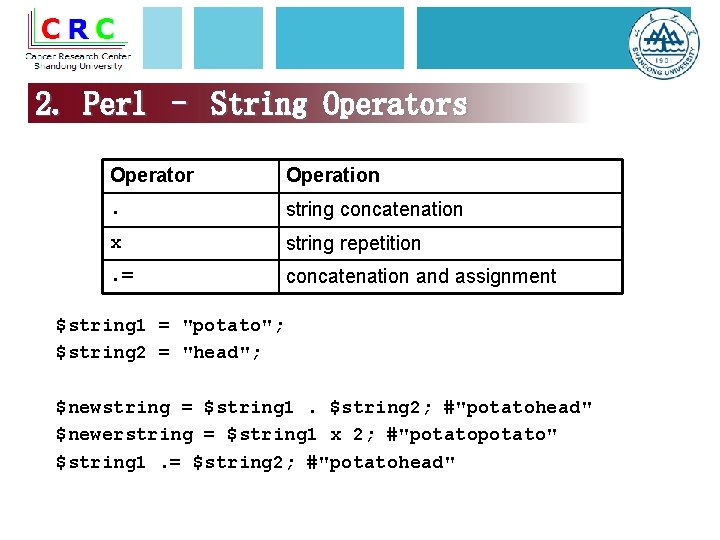
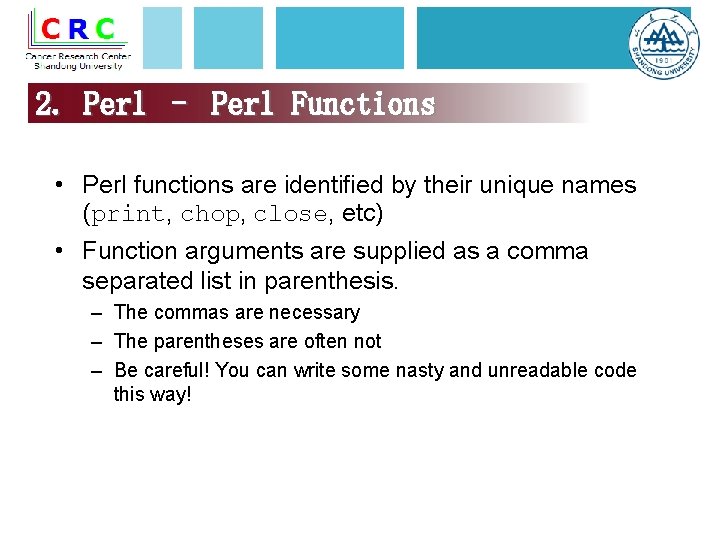
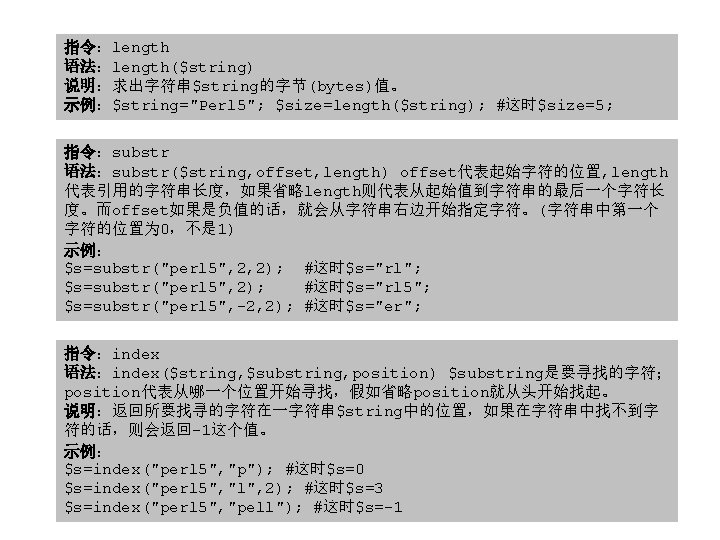
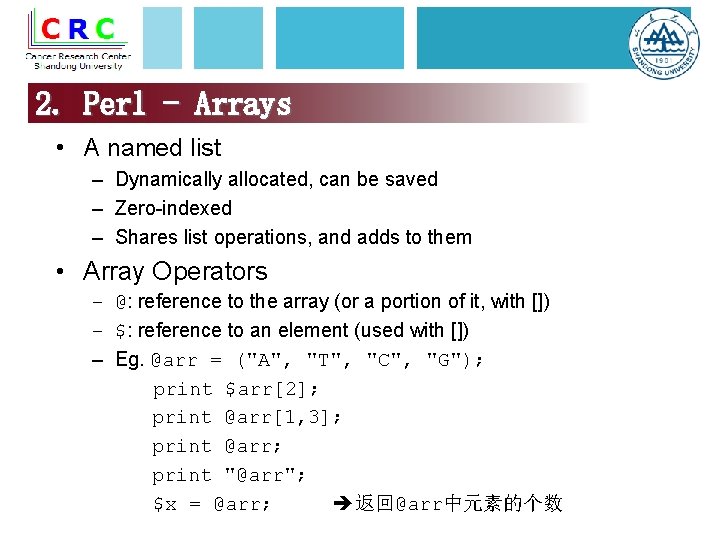
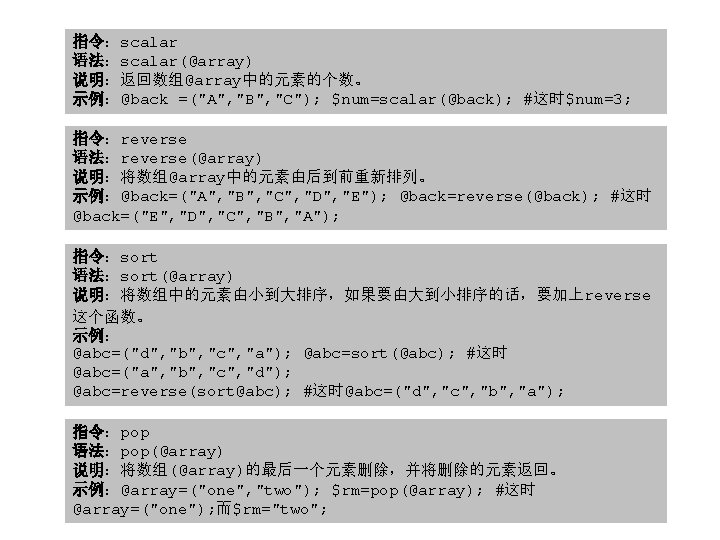
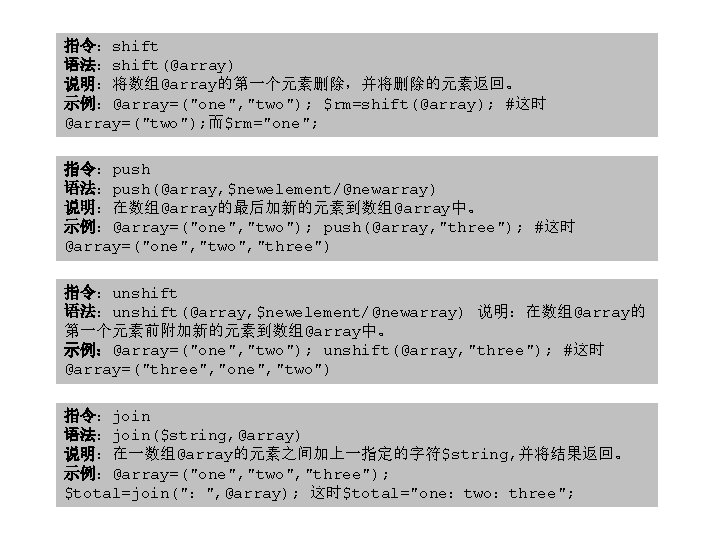
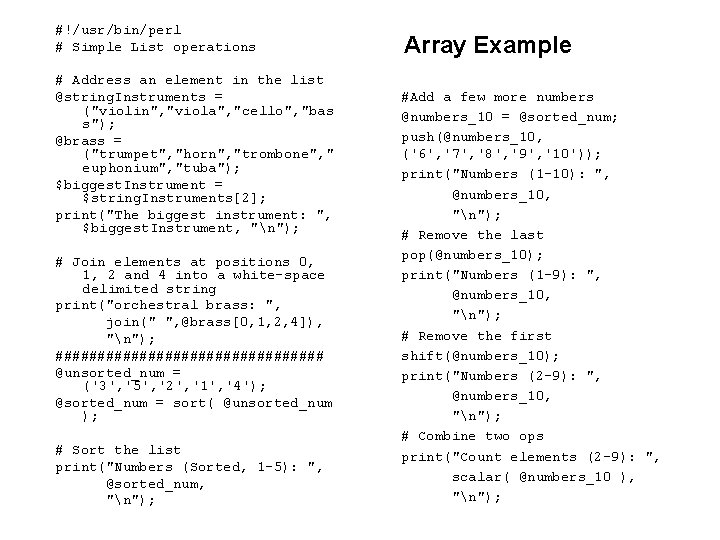
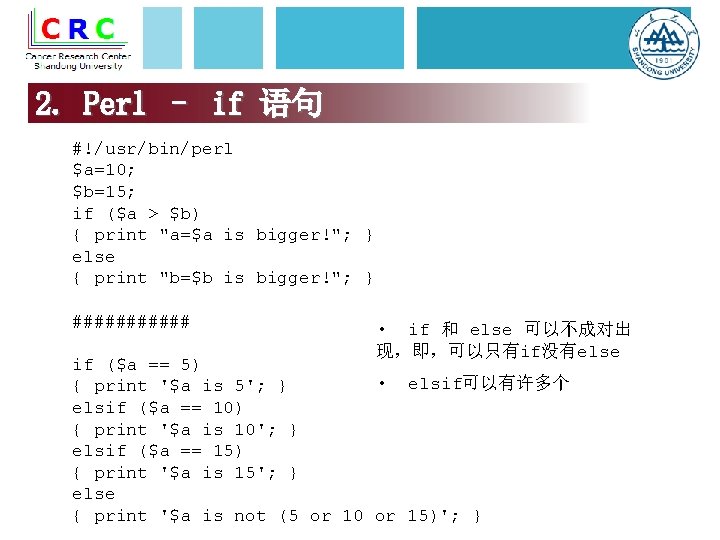
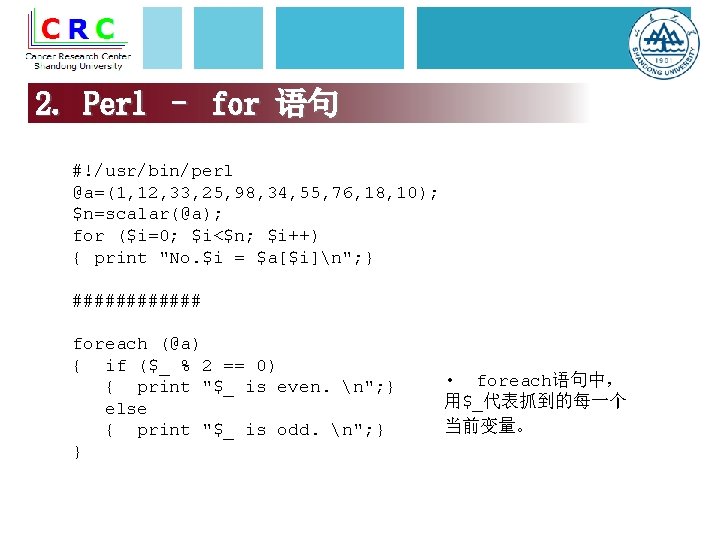
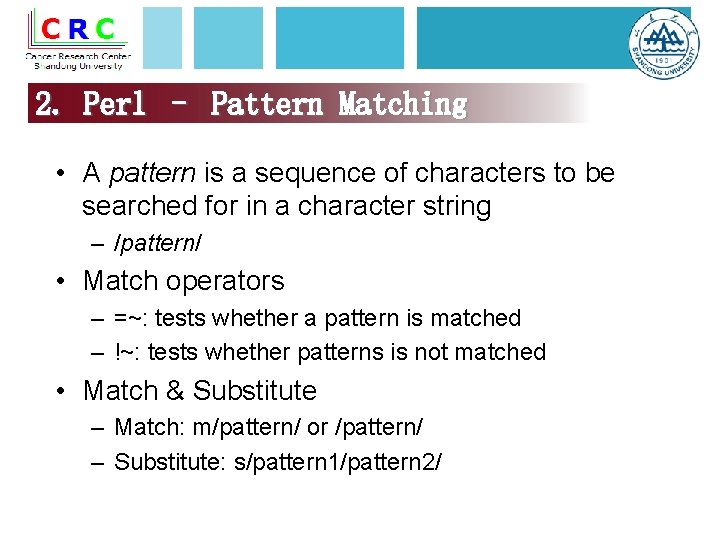
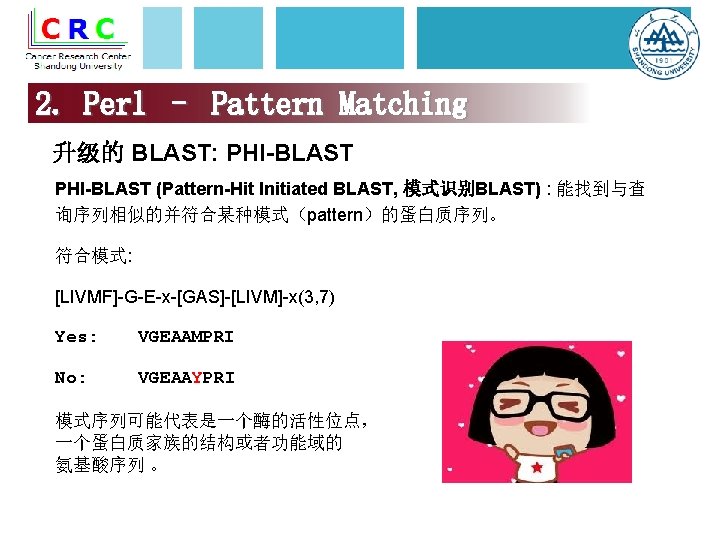
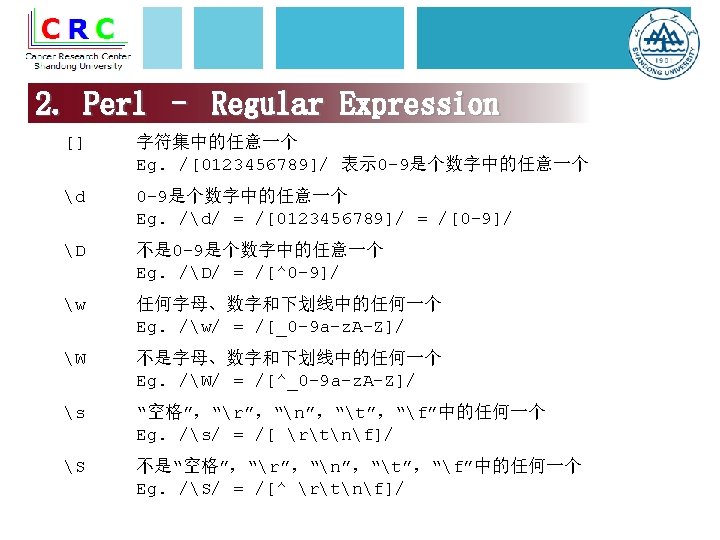
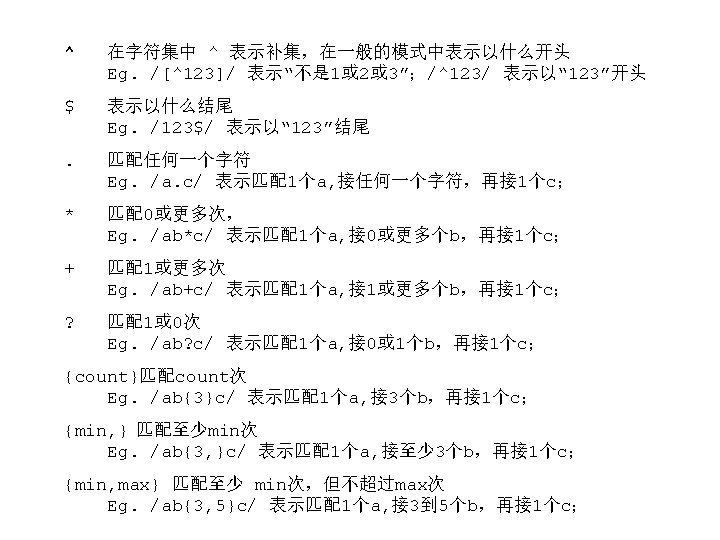
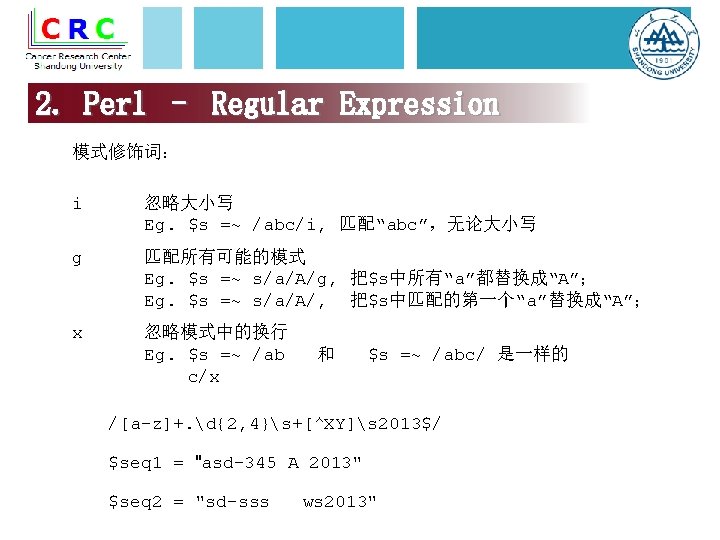
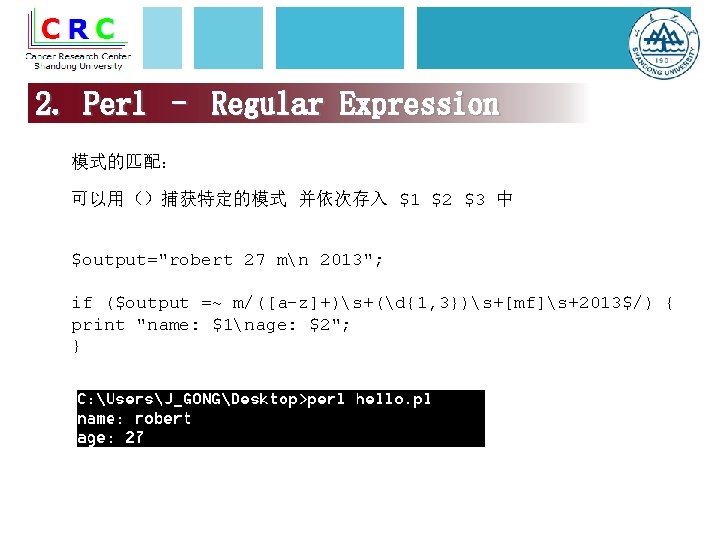
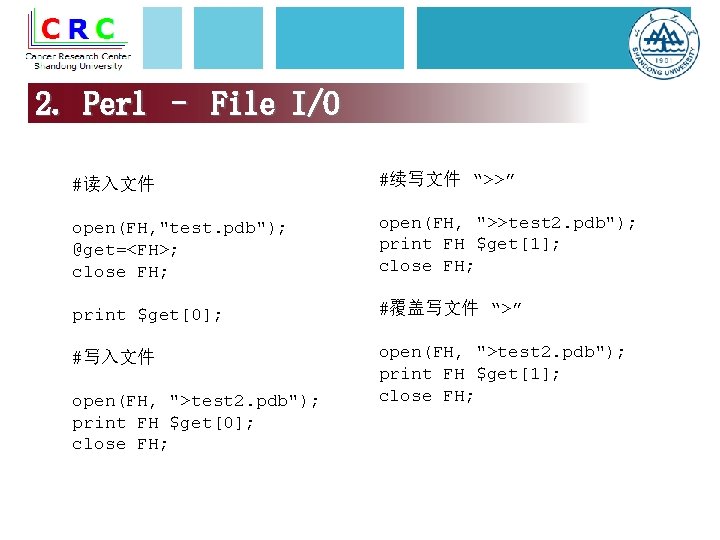
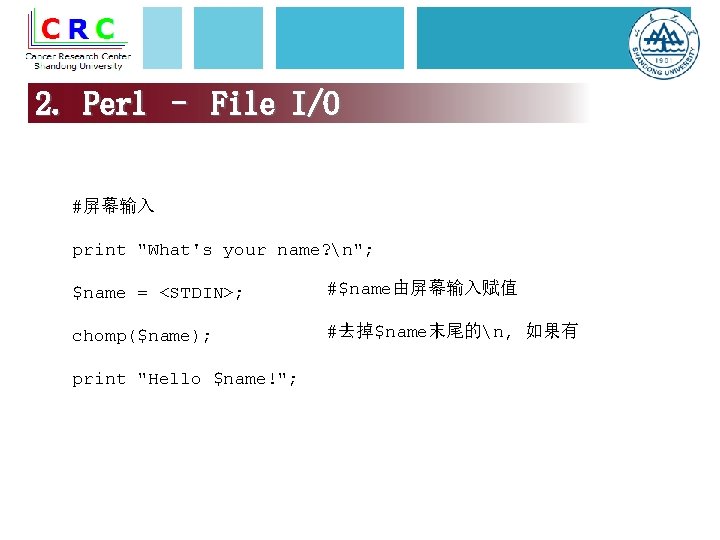
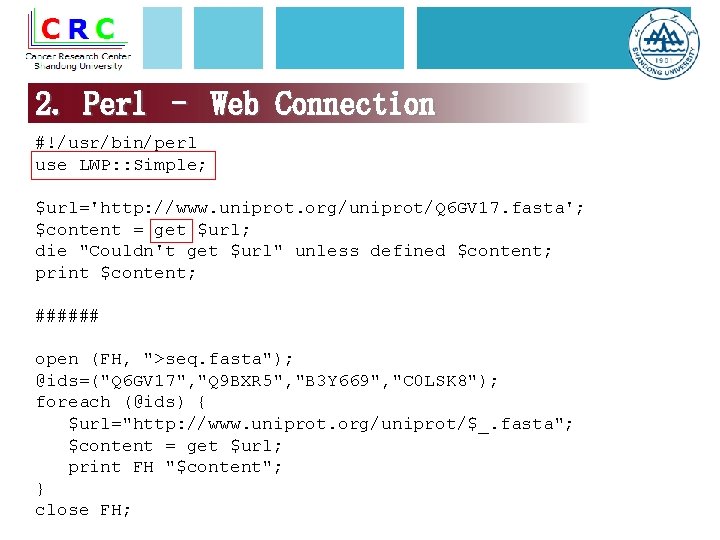
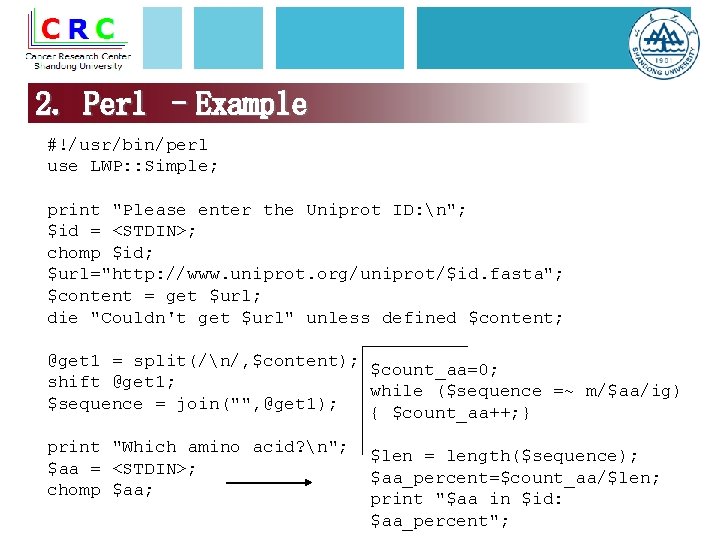
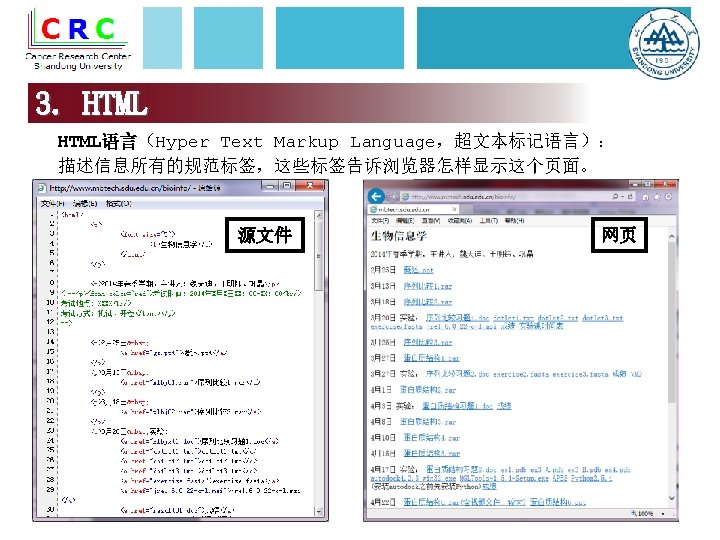
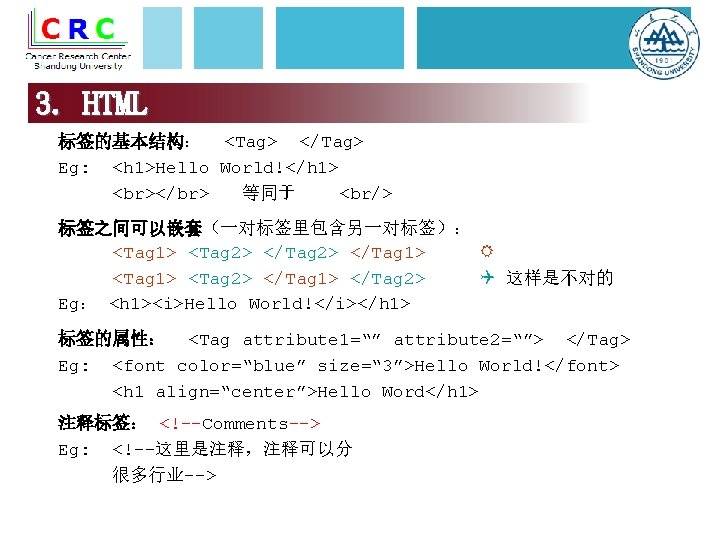
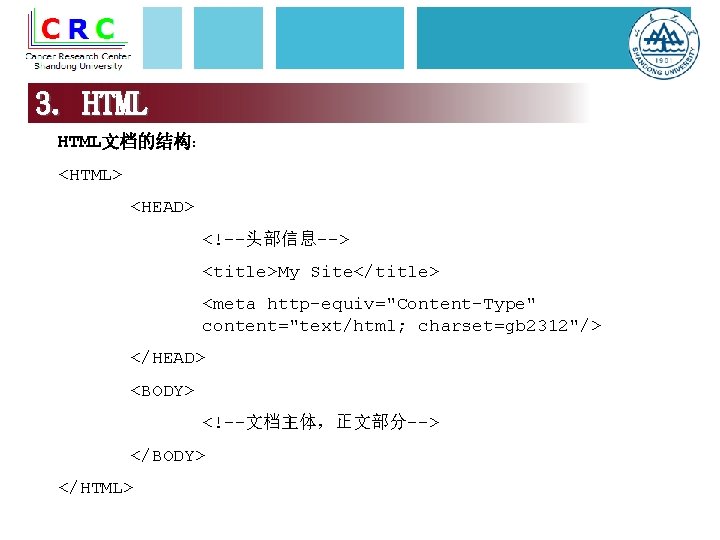
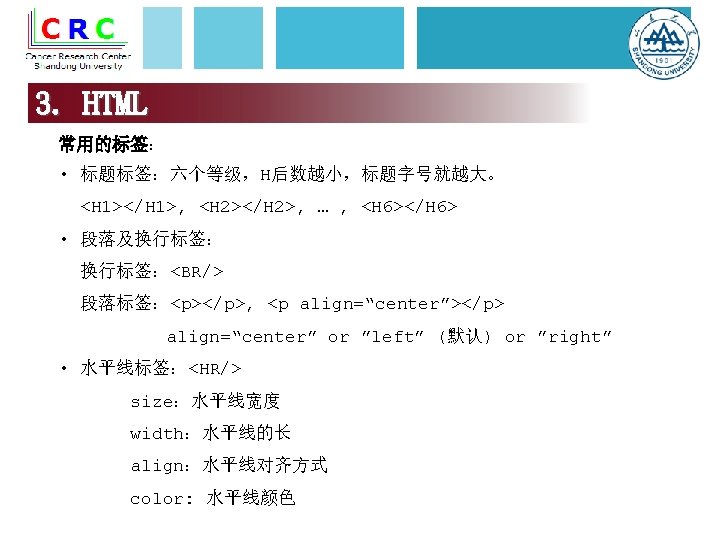
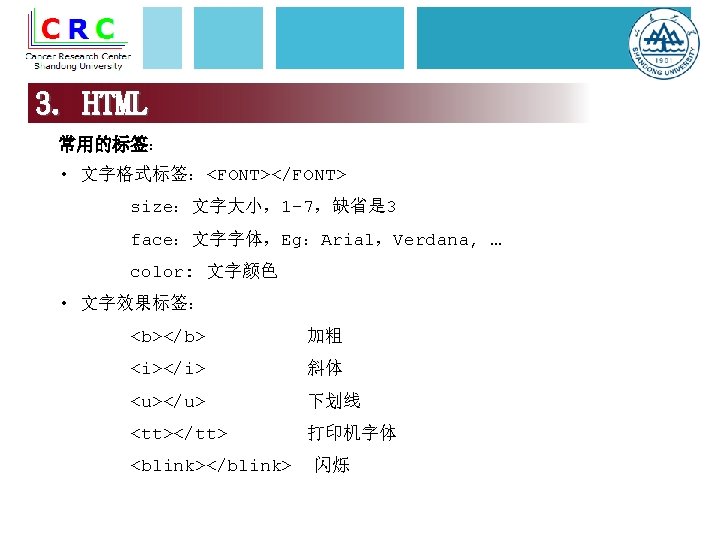
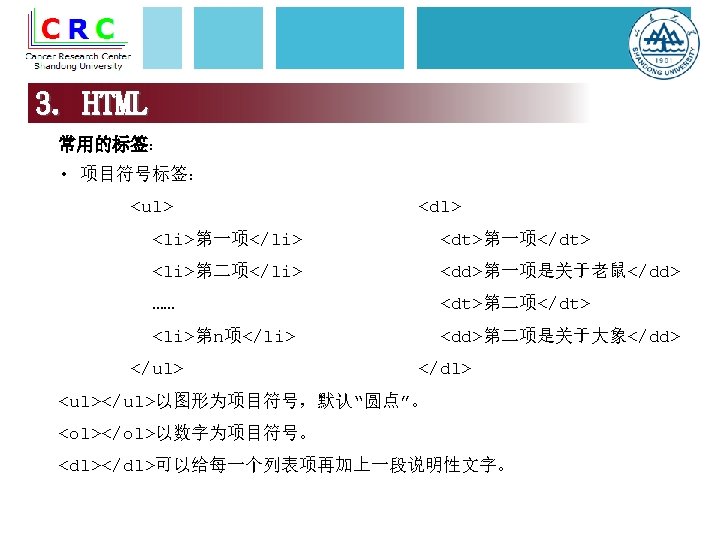
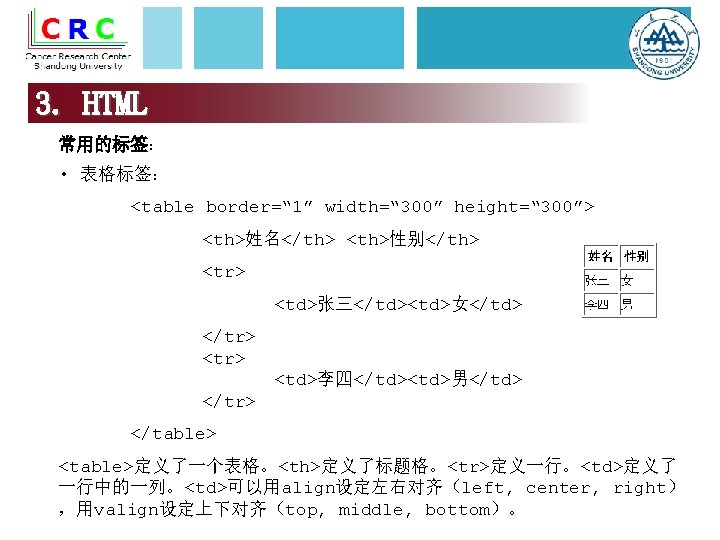
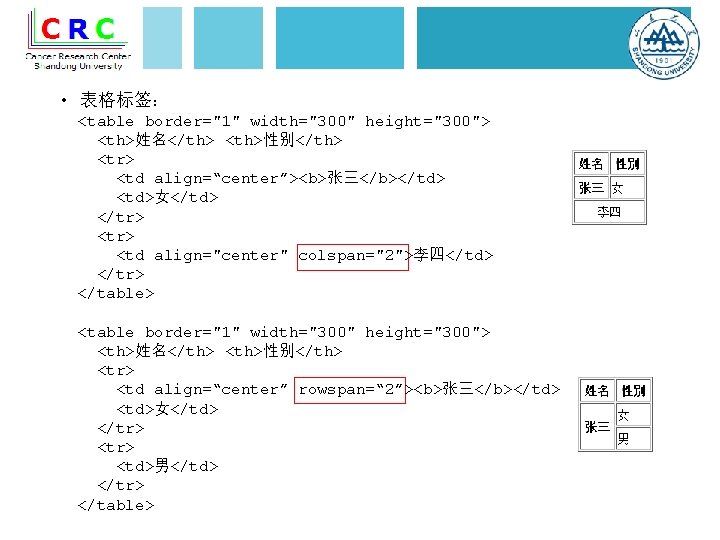
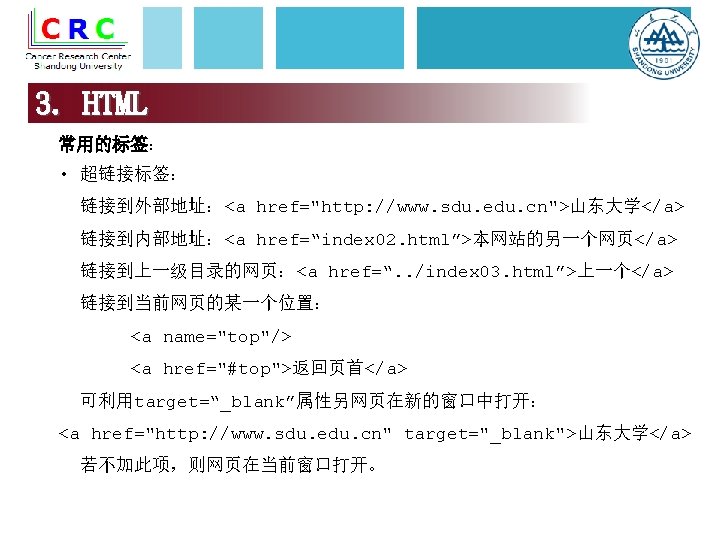
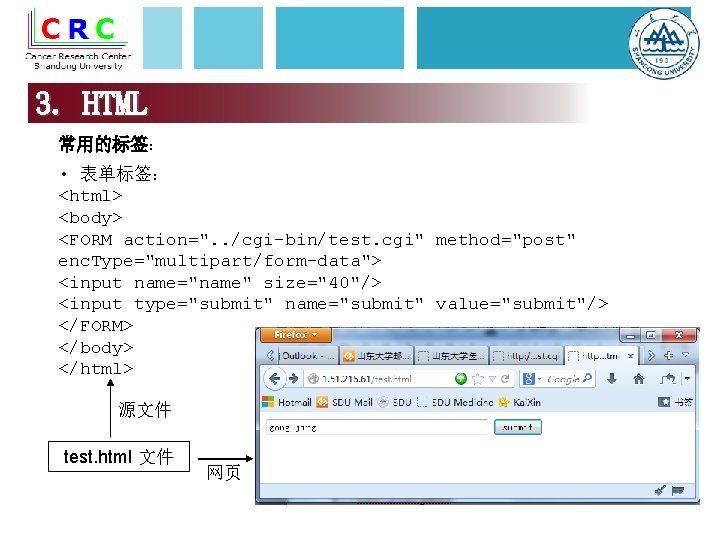
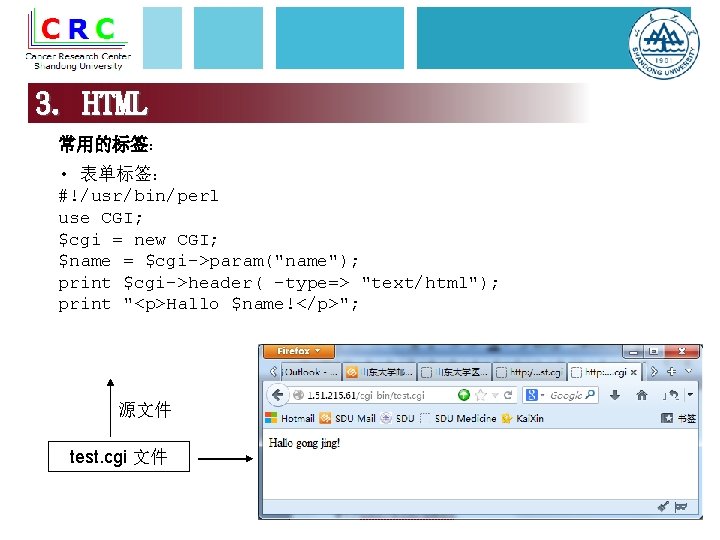
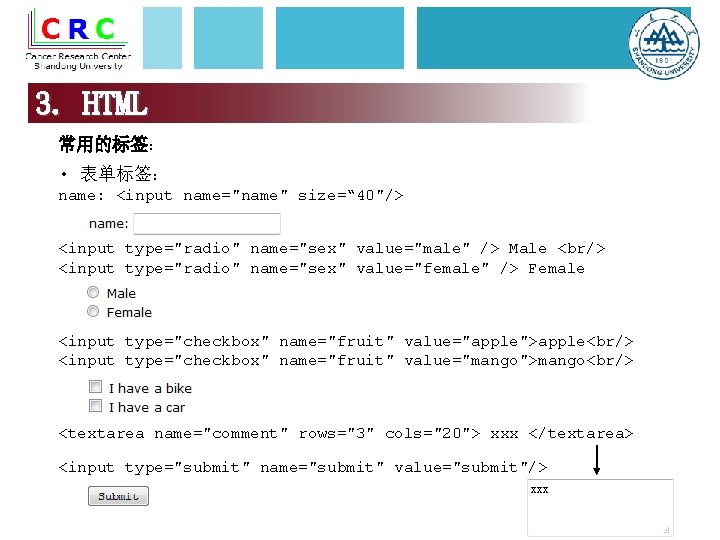
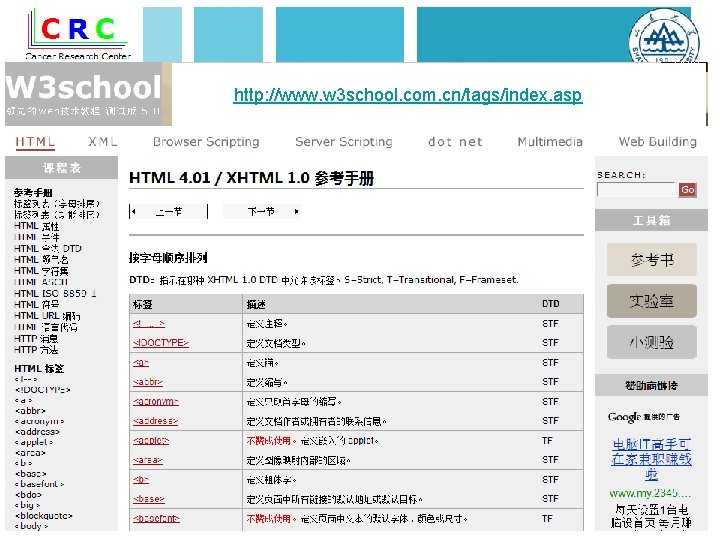
- Slides: 41
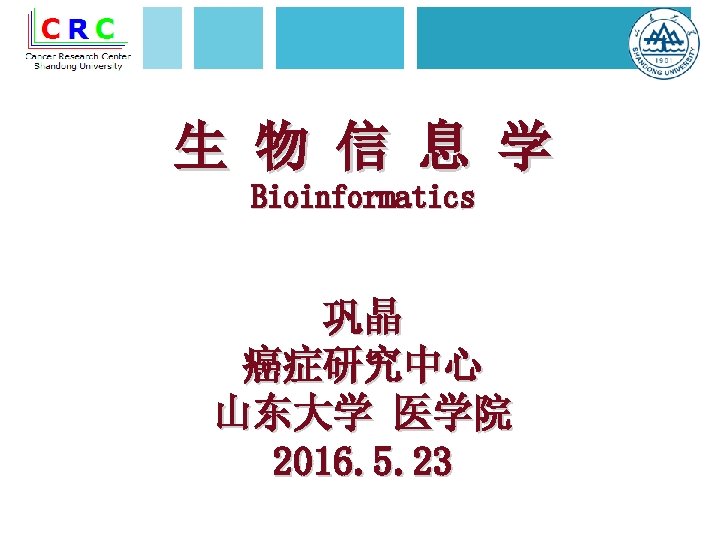
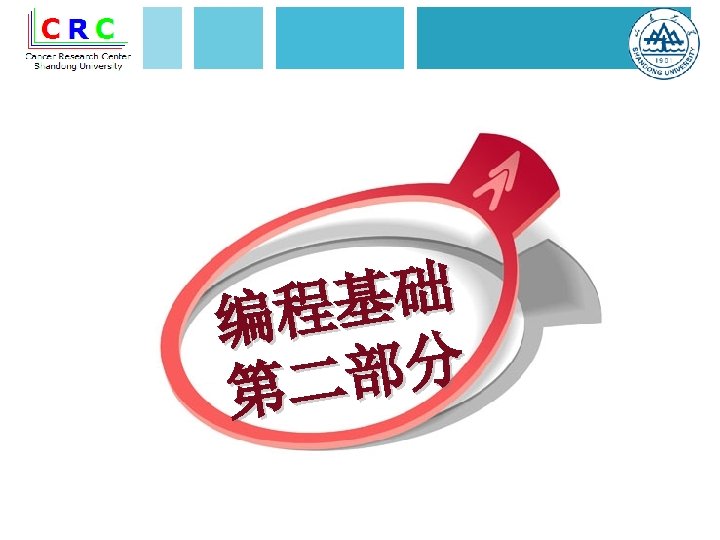
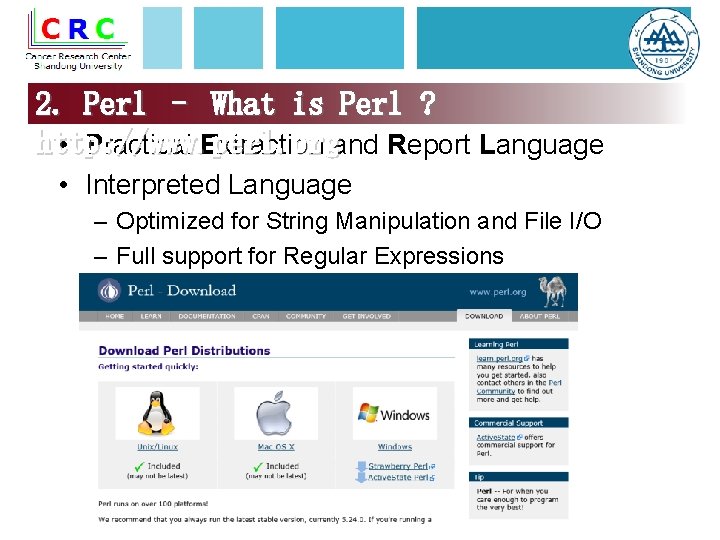
2. Perl – What is Perl ? http: //www. perl. org • Practical Extraction and Report Language • Interpreted Language – Optimized for String Manipulation and File I/O – Full support for Regular Expressions
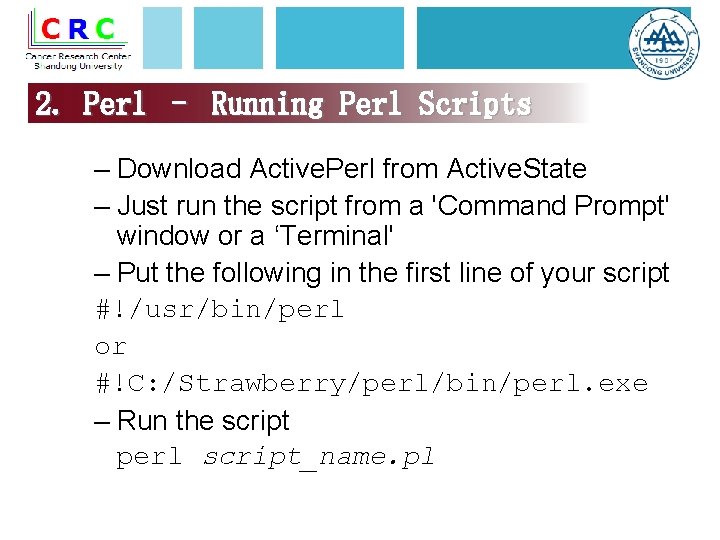
2. Perl – Running Perl Scripts – Download Active. Perl from Active. State – Just run the script from a 'Command Prompt' window or a ‘Terminal' – Put the following in the first line of your script #!/usr/bin/perl or #!C: /Strawberry/perl/bin/perl. exe – Run the script perl script_name. pl
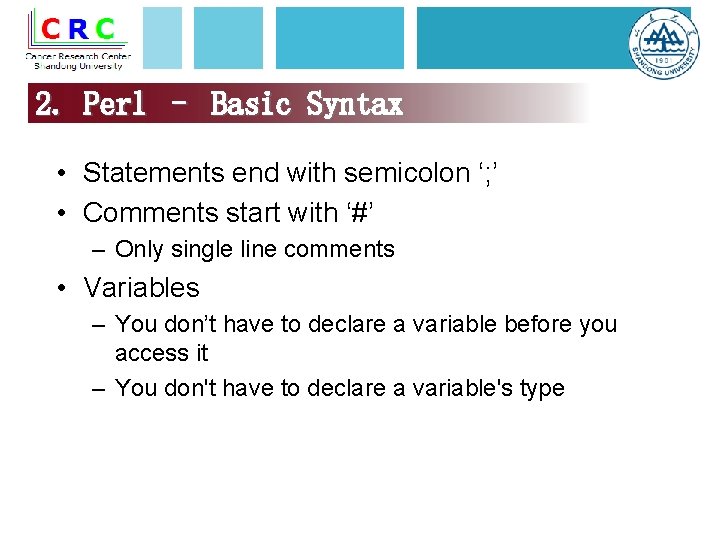
2. Perl – Basic Syntax • Statements end with semicolon ‘; ’ • Comments start with ‘#’ – Only single line comments • Variables – You don’t have to declare a variable before you access it – You don't have to declare a variable's type
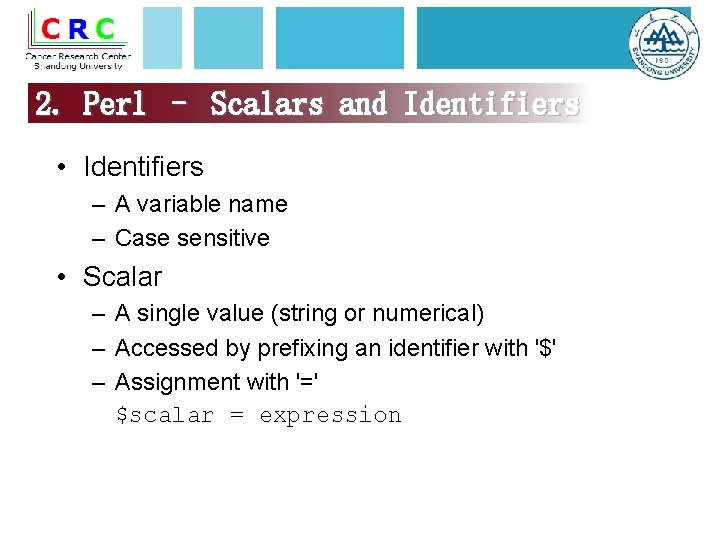
2. Perl – Scalars and Identifiers • Identifiers – A variable name – Case sensitive • Scalar – A single value (string or numerical) – Accessed by prefixing an identifier with '$' – Assignment with '=' $scalar = expression
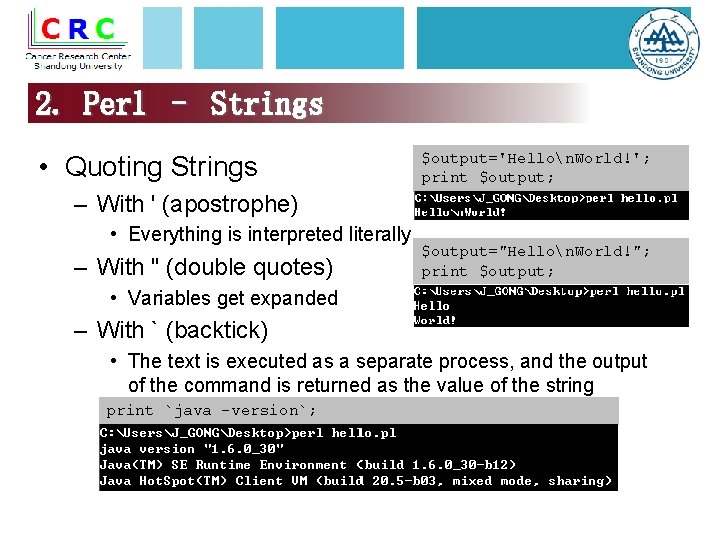
2. Perl – Strings • Quoting Strings $output='Hellon. World!'; print $output; – With ' (apostrophe) • Everything is interpreted literally – With " (double quotes) $output="Hellon. World!"; print $output; • Variables get expanded – With ` (backtick) • The text is executed as a separate process, and the output of the command is returned as the value of the string print `java -version`;
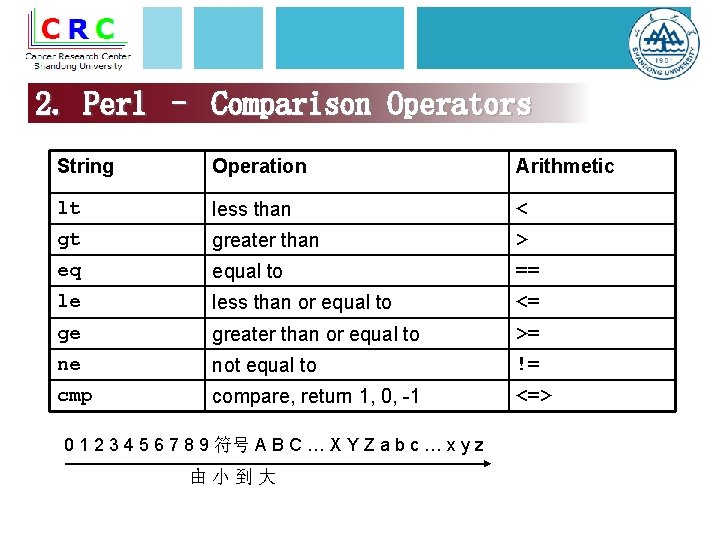
2. Perl – Comparison Operators String Operation Arithmetic lt less than < gt greater than > eq equal to == le less than or equal to <= ge greater than or equal to >= ne not equal to != cmp compare, return 1, 0, -1 <=> 0 1 2 3 4 5 6 7 8 9 符号 A B C … X Y Z a b c … x y z 由小到大
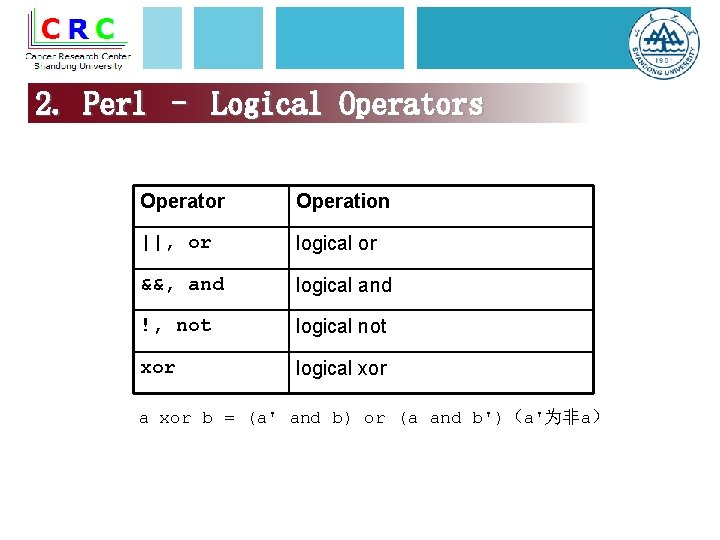
2. Perl – Logical Operators Operator Operation ||, or logical or &&, and logical and !, not logical not xor logical xor a xor b = (a' and b) or (a and b')(a'为非a)
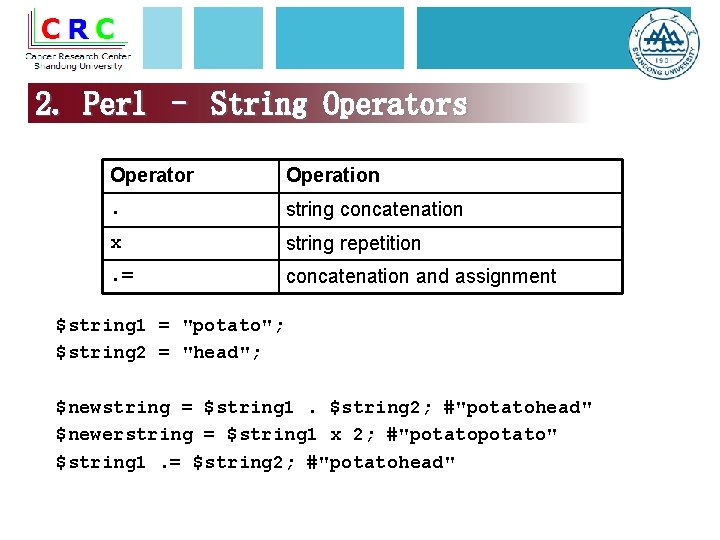
2. Perl – String Operators Operator Operation . string concatenation x string repetition . = concatenation and assignment $string 1 = "potato"; $string 2 = "head"; $newstring = $string 1. $string 2; #"potatohead" $newerstring = $string 1 x 2; #"potato" $string 1. = $string 2; #"potatohead"
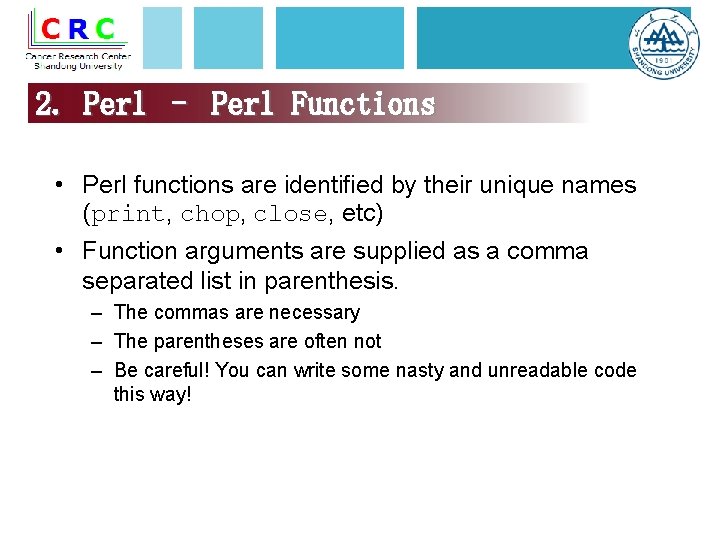
2. Perl – Perl Functions • Perl functions are identified by their unique names (print, chop, close, etc) • Function arguments are supplied as a comma separated list in parenthesis. – The commas are necessary – The parentheses are often not – Be careful! You can write some nasty and unreadable code this way!
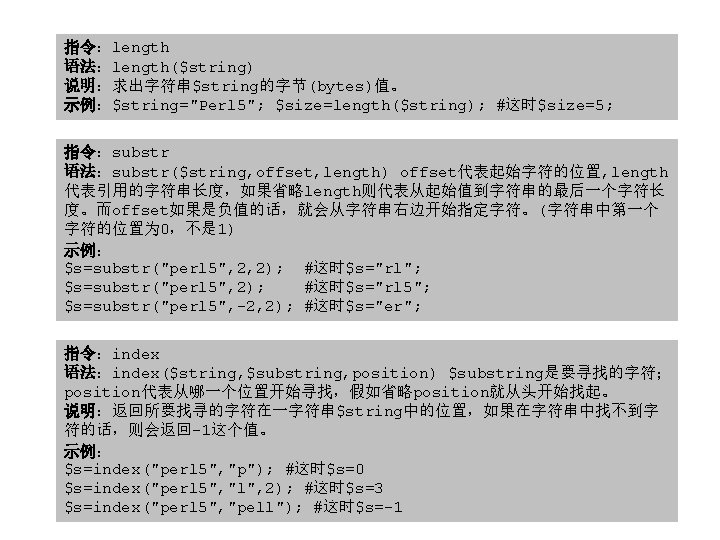
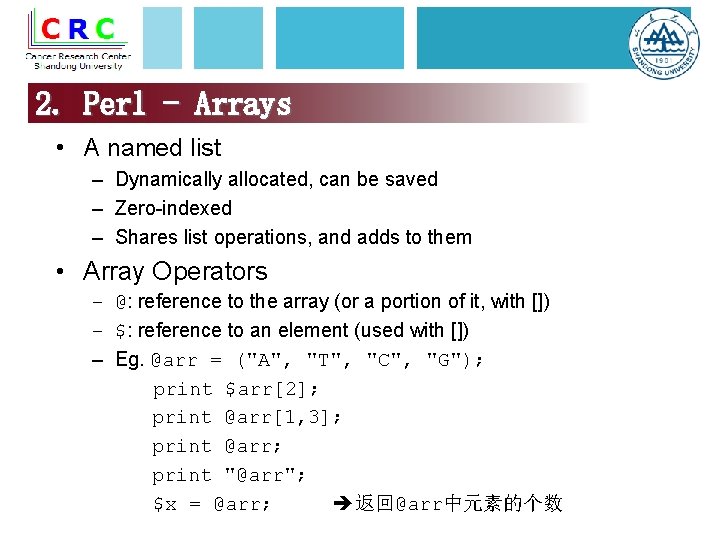
2. Perl - Arrays • A named list – Dynamically allocated, can be saved – Zero-indexed – Shares list operations, and adds to them • Array Operators – @: reference to the array (or a portion of it, with []) – $: reference to an element (used with []) – Eg. @arr = ("A", "T", "C", "G"); print $arr[2]; print @arr[1, 3]; print @arr; print "@arr"; $x = @arr; 返回@arr中元素的个数
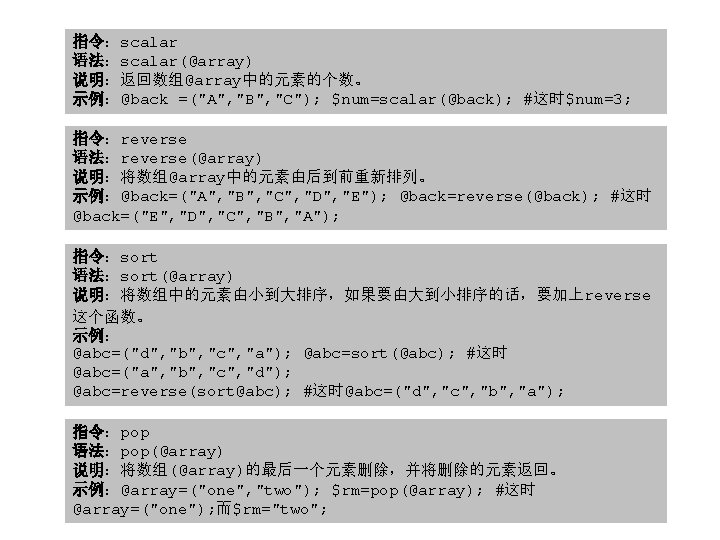
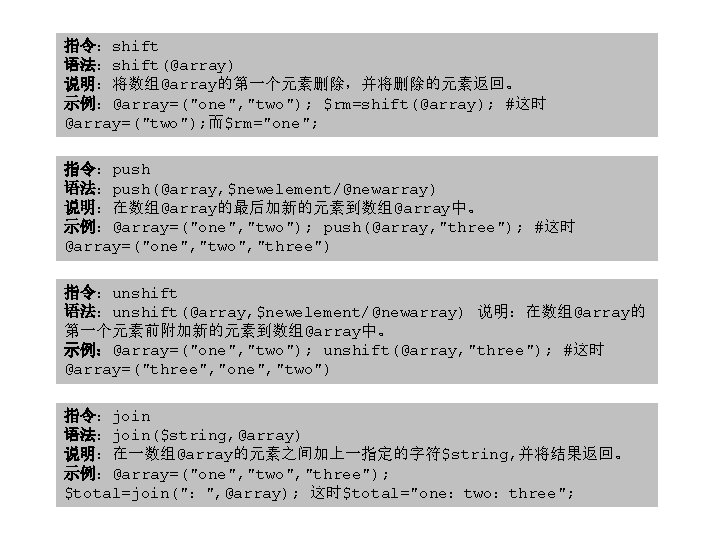
指令:shift 语法:shift(@array) 说明:将数组@array的第一个元素删除,并将删除的元素返回。 示例:@array=("one", "two"); $rm=shift(@array); #这时 @array=("two"); 而$rm="one"; 指令:push 语法:push(@array, $newelement/@newarray) 说明:在数组@array的最后加新的元素到数组@array中。 示例:@array=("one", "two"); push(@array, "three"); #这时 @array=("one", "two", "three") 指令:unshift 语法:unshift(@array, $newelement/@newarray) 说明:在数组@array的 第一个元素前附加新的元素到数组@array中。 示例:@array=("one", "two"); unshift(@array, "three"); #这时 @array=("three", "one", "two") 指令:join 语法:join($string, @array) 说明:在一数组@array的元素之间加上一指定的字符$string, 并将结果返回。 示例:@array=("one", "two", "three"); $total=join(":", @array); 这时$total="one:two:three";
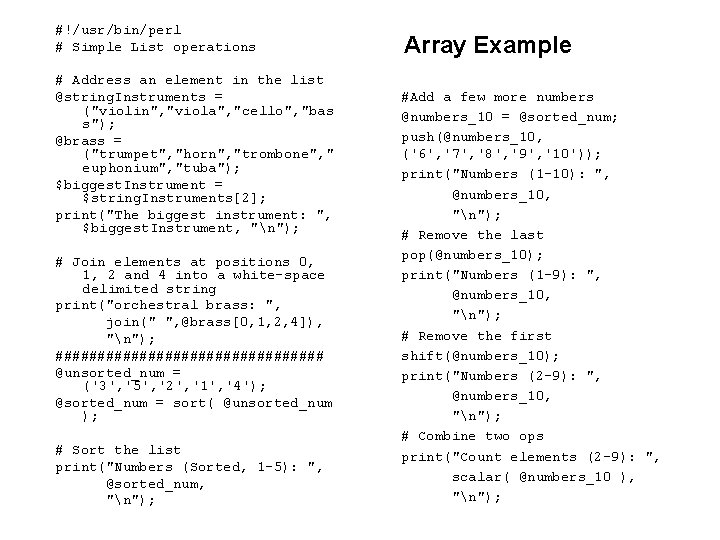
#!/usr/bin/perl # Simple List operations # Address an element in the list @string. Instruments = ("violin", "viola", "cello", "bas s"); @brass = ("trumpet", "horn", "trombone", " euphonium", "tuba"); $biggest. Instrument = $string. Instruments[2]; print("The biggest instrument: ", $biggest. Instrument, "n"); # Join elements at positions 0, 1, 2 and 4 into a white-space delimited string print("orchestral brass: ", join(" ", @brass[0, 1, 2, 4]), "n"); ################ @unsorted_num = ('3', '5', '2', '1', '4'); @sorted_num = sort( @unsorted_num ); # Sort the list print("Numbers (Sorted, 1 -5): ", @sorted_num, "n"); Array Example #Add a few more numbers @numbers_10 = @sorted_num; push(@numbers_10, ('6', '7', '8', '9', '10')); print("Numbers (1 -10): ", @numbers_10, "n"); # Remove the last pop(@numbers_10); print("Numbers (1 -9): ", @numbers_10, "n"); # Remove the first shift(@numbers_10); print("Numbers (2 -9): ", @numbers_10, "n"); # Combine two ops print("Count elements (2 -9): ", scalar( @numbers_10 ), "n");
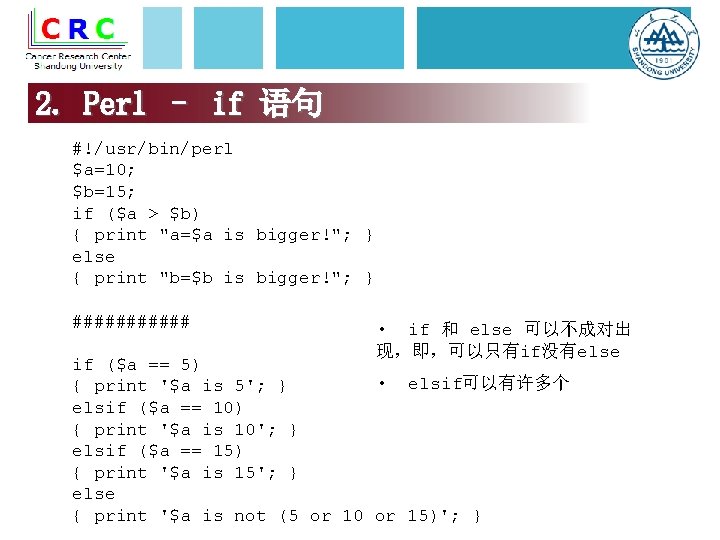
2. Perl – if 语句 #!/usr/bin/perl $a=10; $b=15; if ($a > $b) { print "a=$a is bigger!"; } else { print "b=$b is bigger!"; } ###### • if 和 else 可以不成对出 现,即,可以只有if没有else if ($a == 5) • elsif可以有许多个 { print '$a is 5'; } elsif ($a == 10) { print '$a is 10'; } elsif ($a == 15) { print '$a is 15'; } else { print '$a is not (5 or 10 or 15)'; }
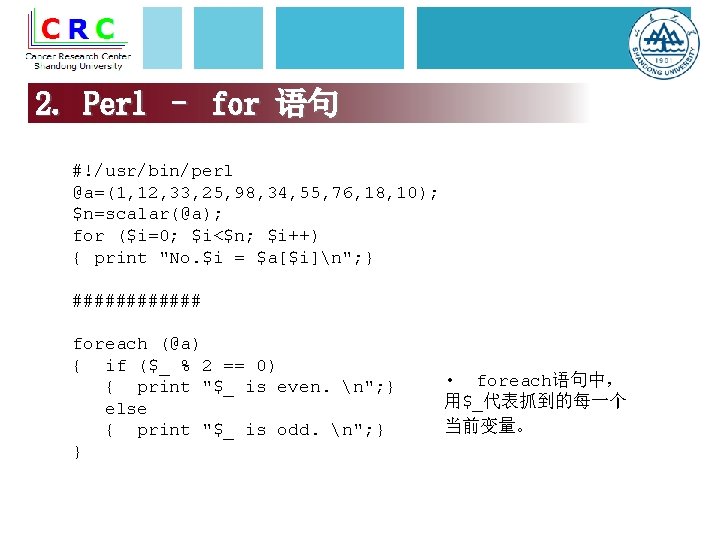
2. Perl – for 语句 #!/usr/bin/perl @a=(1, 12, 33, 25, 98, 34, 55, 76, 18, 10); $n=scalar(@a); for ($i=0; $i<$n; $i++) { print "No. $i = $a[$i]n"; } ###### foreach (@a) { if ($_ % 2 == 0) { print "$_ is even. n"; } else { print "$_ is odd. n"; } } • foreach语句中, 用$_代表抓到的每一个 当前变量。
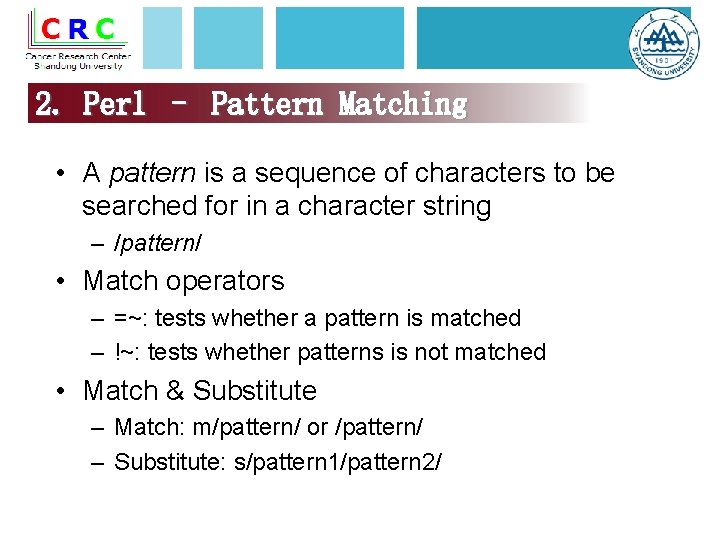
2. Perl – Pattern Matching • A pattern is a sequence of characters to be searched for in a character string – /pattern/ • Match operators – =~: tests whether a pattern is matched – !~: tests whether patterns is not matched • Match & Substitute – Match: m/pattern/ or /pattern/ – Substitute: s/pattern 1/pattern 2/
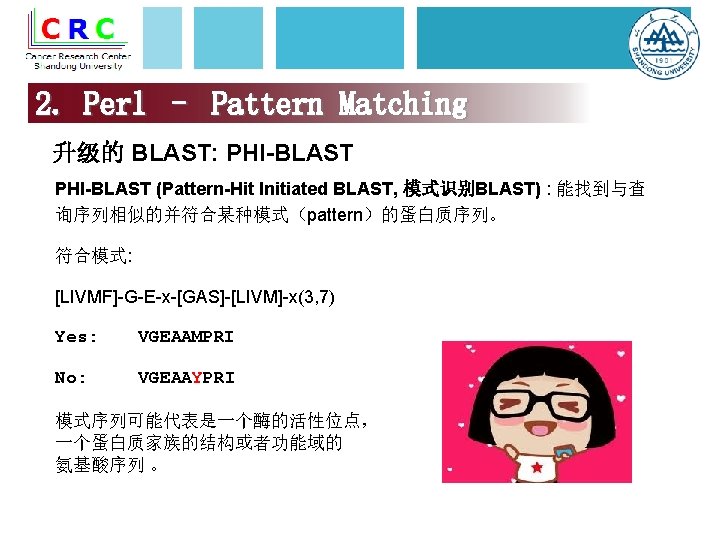
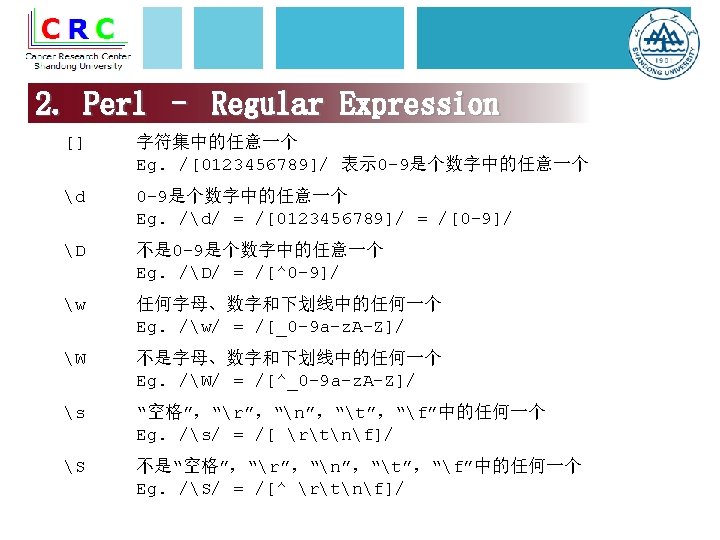
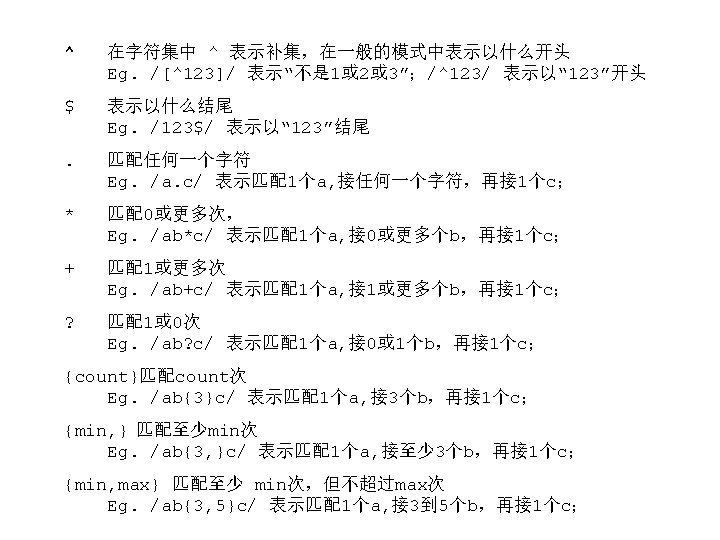
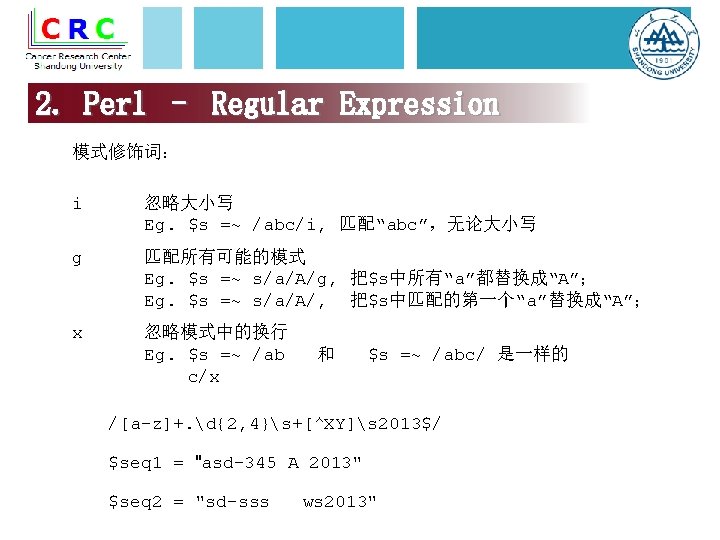
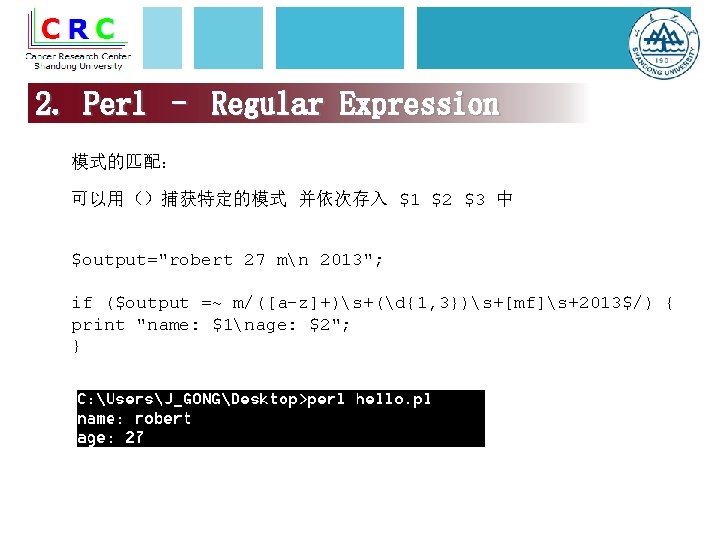
2. Perl – Regular Expression 模式的匹配: 可以用()捕获特定的模式 并依次存入 $1 $2 $3 中 $output="robert 27 mn 2013"; if ($output =~ m/([a-z]+)s+(d{1, 3})s+[mf]s+2013$/) { print "name: $1nage: $2"; }
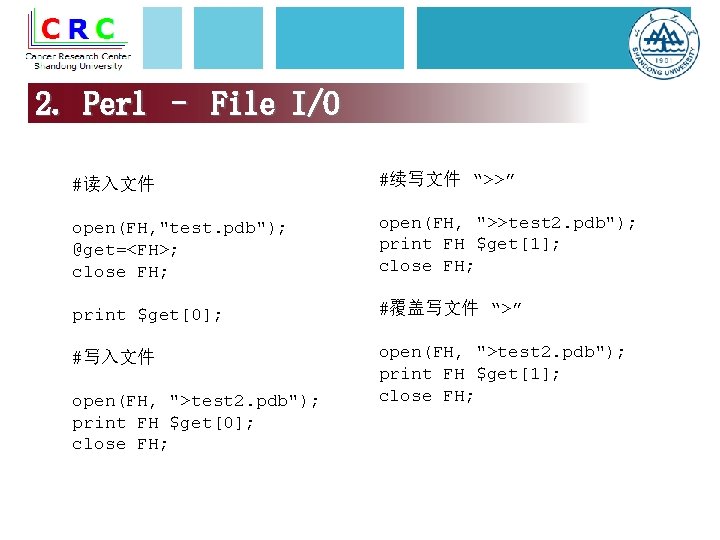
2. Perl – File I/O #读入文件 #续写文件 “>>” open(FH, "test. pdb"); @get=<FH>; close FH; open(FH, ">>test 2. pdb"); print FH $get[1]; close FH; print $get[0]; #覆盖写文件 “>” #写入文件 open(FH, ">test 2. pdb"); print FH $get[1]; close FH; open(FH, ">test 2. pdb"); print FH $get[0]; close FH;
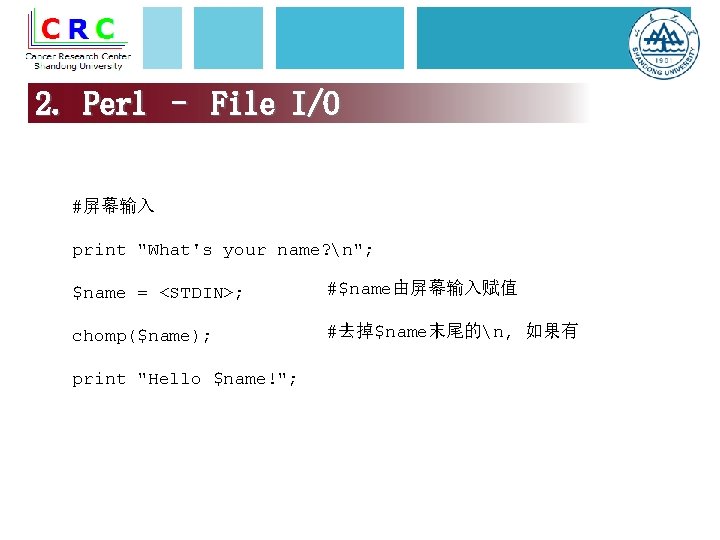
2. Perl – File I/O #屏幕输入 print "What's your name? n"; $name = <STDIN>; #$name由屏幕输入赋值 chomp($name); #去掉$name末尾的n, 如果有 print "Hello $name!";
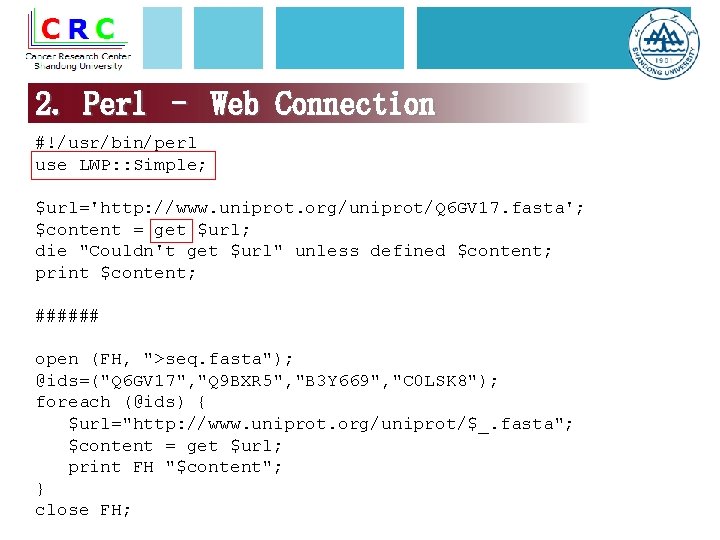
2. Perl – Web Connection #!/usr/bin/perl use LWP: : Simple; $url='http: //www. uniprot. org/uniprot/Q 6 GV 17. fasta'; $content = get $url; die "Couldn't get $url" unless defined $content; print $content; ###### open (FH, ">seq. fasta"); @ids=("Q 6 GV 17", "Q 9 BXR 5", "B 3 Y 669", "C 0 LSK 8"); foreach (@ids) { $url="http: //www. uniprot. org/uniprot/$_. fasta"; $content = get $url; print FH "$content"; } close FH;
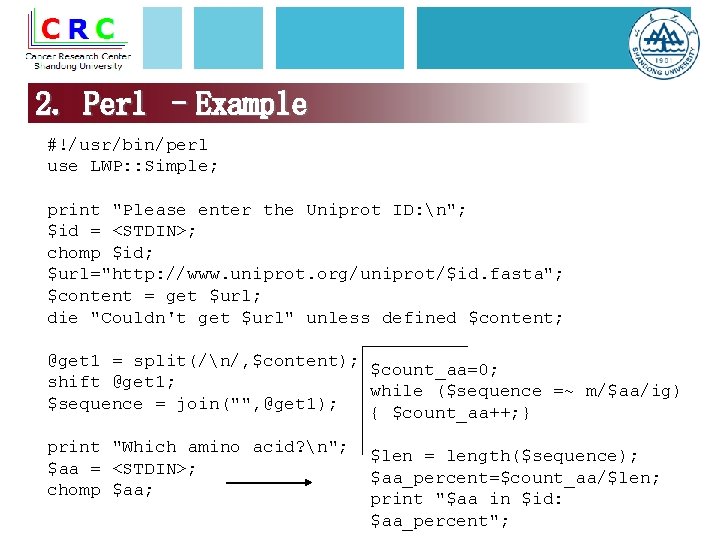
2. Perl –Example #!/usr/bin/perl use LWP: : Simple; print "Please enter the Uniprot ID: n"; $id = <STDIN>; chomp $id; $url="http: //www. uniprot. org/uniprot/$id. fasta"; $content = get $url; die "Couldn't get $url" unless defined $content; @get 1 = split(/n/, $content); $count_aa=0; shift @get 1; while ($sequence =~ m/$aa/ig) $sequence = join("", @get 1); { $count_aa++; } print "Which amino acid? n"; $aa = <STDIN>; chomp $aa; $len = length($sequence); $aa_percent=$count_aa/$len; print "$aa in $id: $aa_percent";
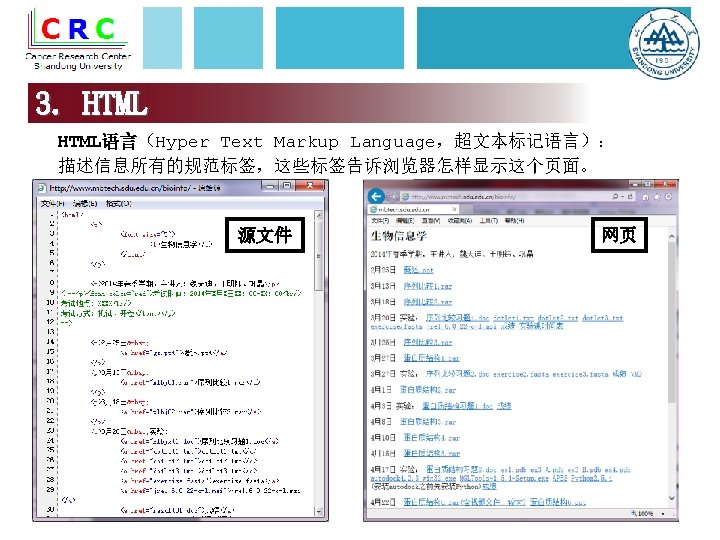
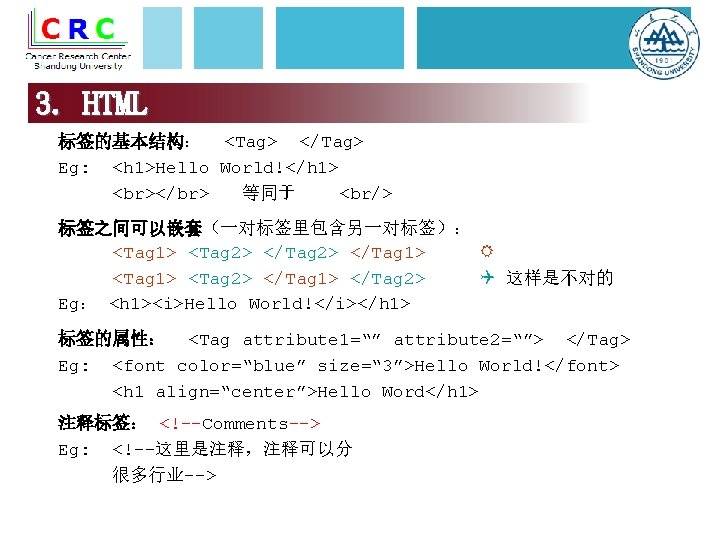
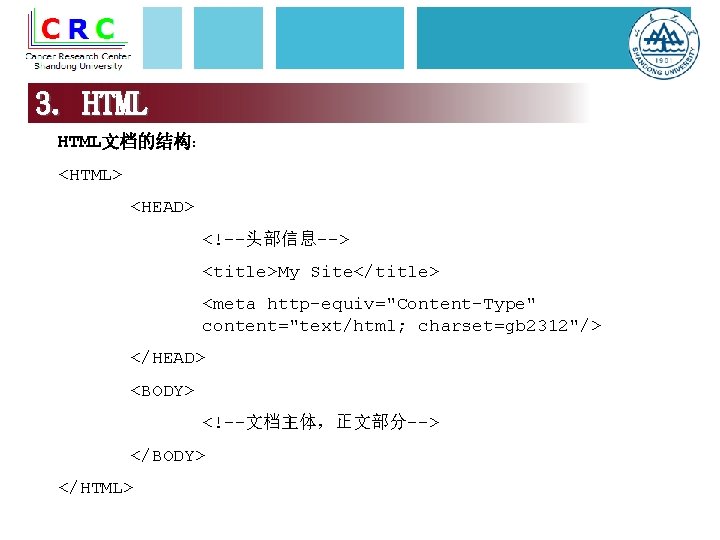
3. HTML文档的结构: <HTML> <HEAD> <!--头部信息--> <title>My Site</title> <meta http-equiv="Content-Type" content="text/html; charset=gb 2312"/> </HEAD> <BODY> <!--文档主体,正文部分--> </BODY> </HTML>
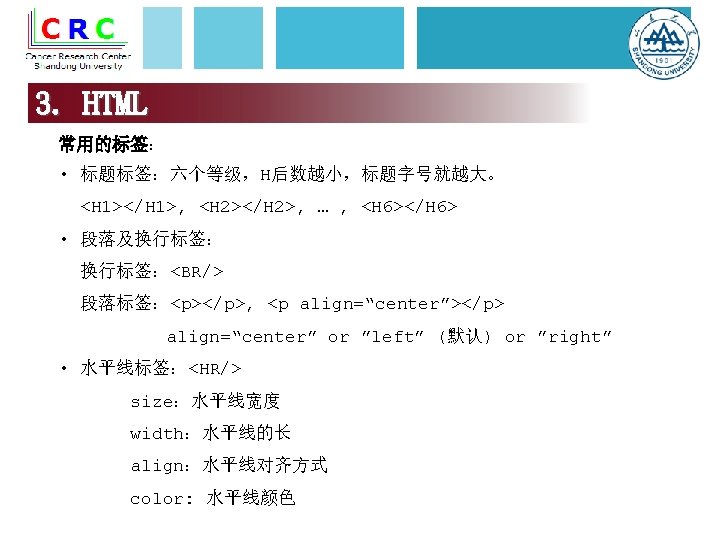
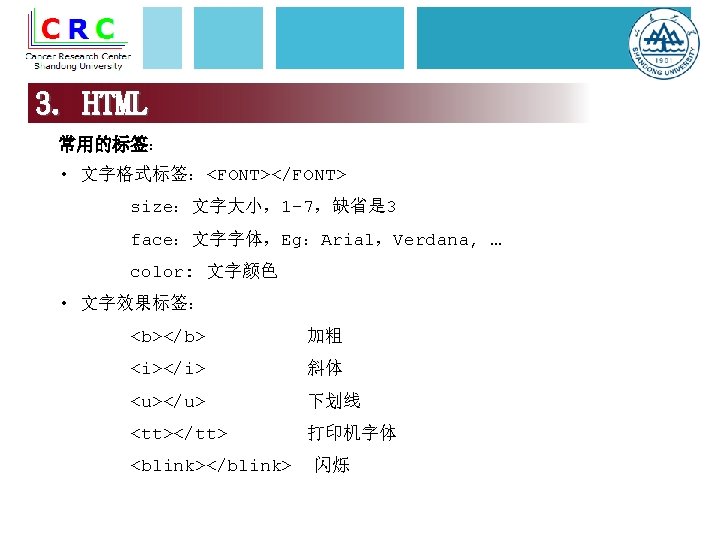
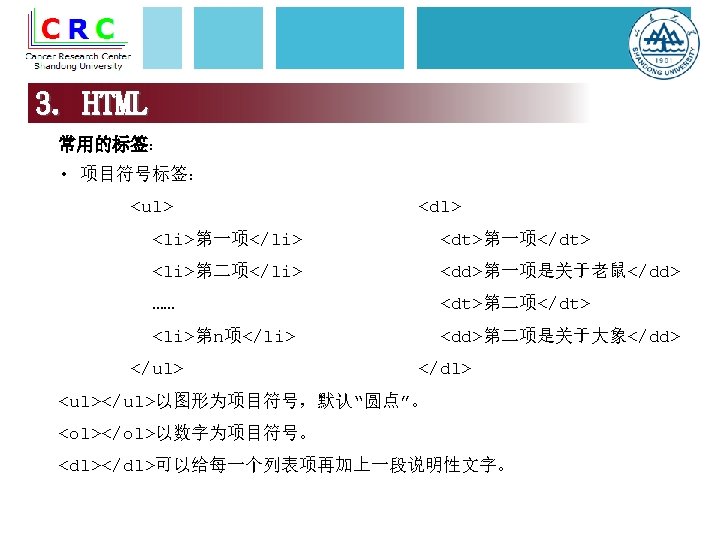
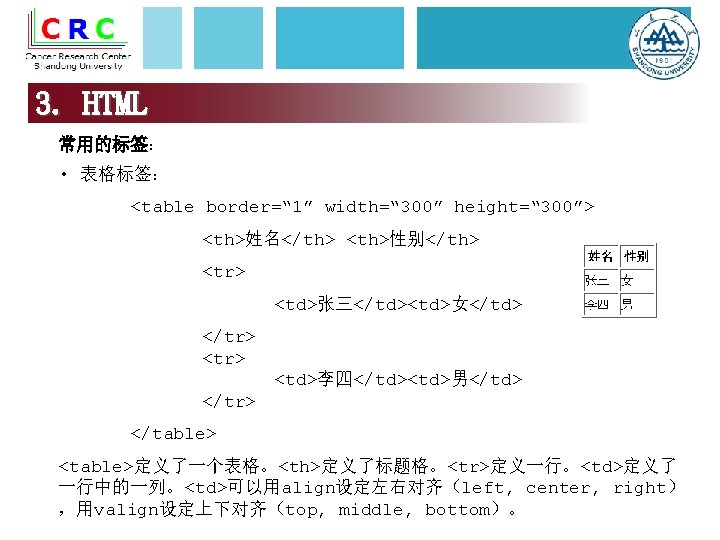
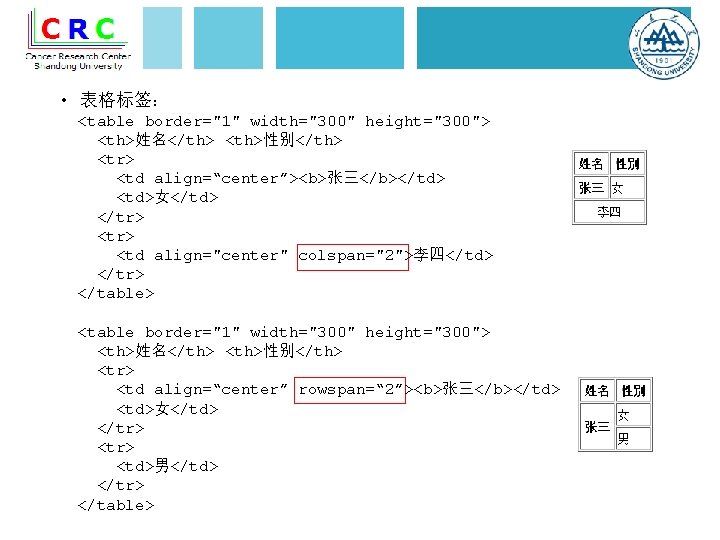
• 表格标签: <table border="1" width="300" height="300"> <th>姓名</th> <th>性别</th> <tr> <td align=“center”><b>张三</b></td> <td>女</td> </tr> <td align="center" colspan="2">李四</td> </tr> </table> <table border="1" width="300" height="300"> <th>姓名</th> <th>性别</th> <tr> <td align=“center” rowspan=“ 2”><b>张三</b></td> <td>女</td> </tr> <td>男</td> </tr> </table>
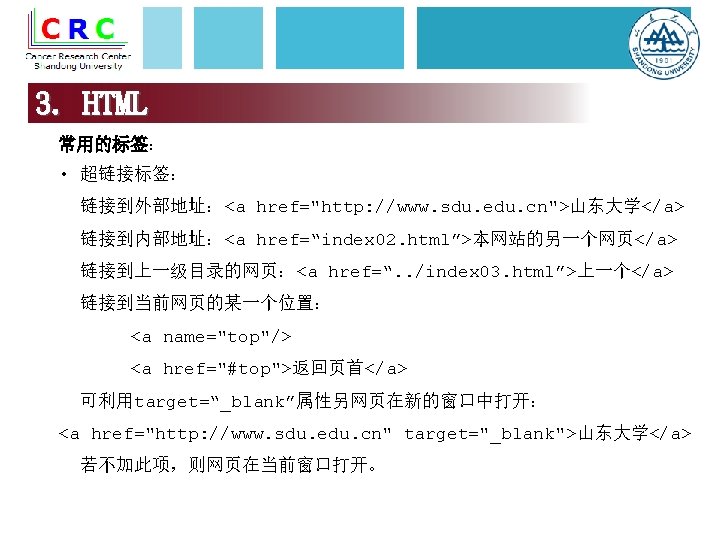
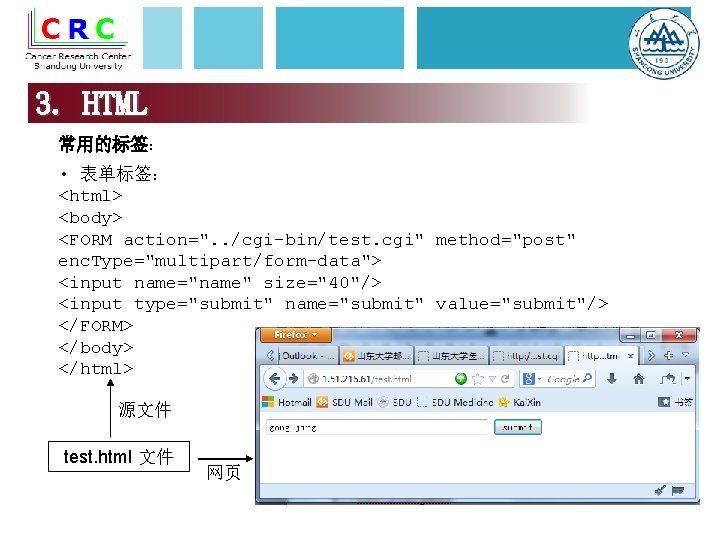
3. HTML 常用的标签: • 表单标签: <html> <body> <FORM action=". . /cgi-bin/test. cgi" method="post" enc. Type="multipart/form-data"> <input name="name" size="40"/> <input type="submit" name="submit" value="submit"/> </FORM> </body> </html> 源文件 test. html 文件 网页
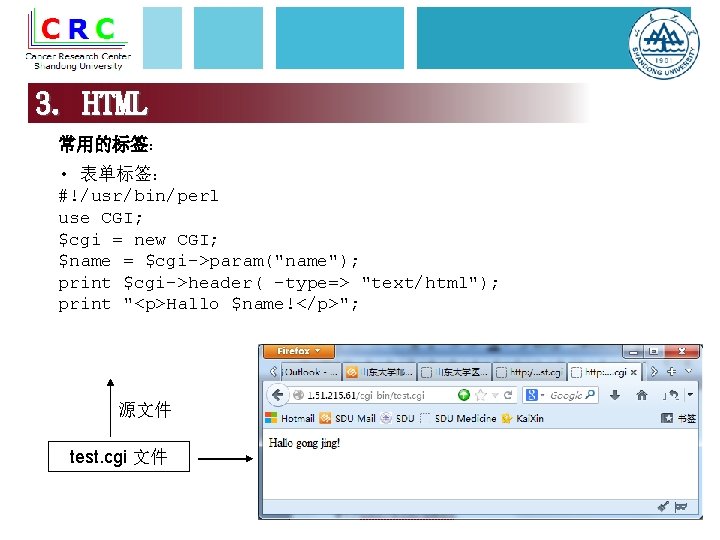
3. HTML 常用的标签: • 表单标签: #!/usr/bin/perl use CGI; $cgi = new CGI; $name = $cgi->param("name"); print $cgi->header( -type=> "text/html"); print "<p>Hallo $name!</p>"; 源文件 test. cgi 文件
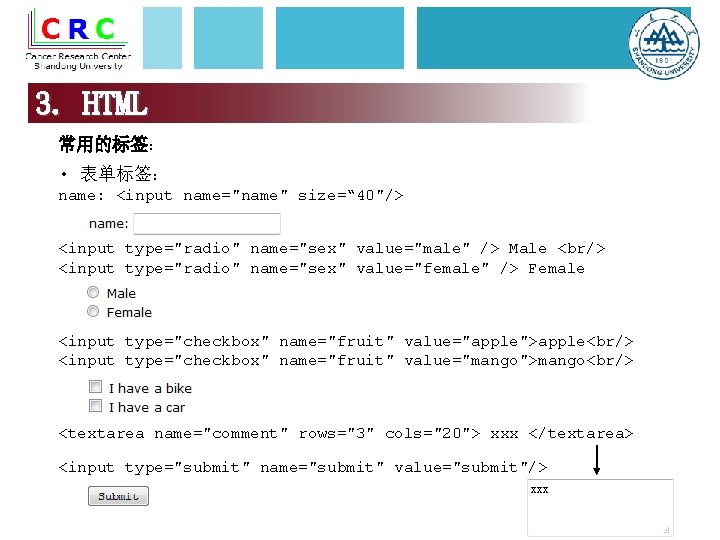
3. HTML 常用的标签: • 表单标签: name: <input name="name" size=“ 40"/> <input type="radio" name="sex" value="male" /> Male <br/> <input type="radio" name="sex" value="female" /> Female <input type="checkbox" name="fruit" value="apple">apple<br/> <input type="checkbox" name="fruit" value="mango">mango<br/> <textarea name="comment" rows="3" cols="20"> xxx </textarea> <input type="submit" name="submit" value="submit"/>
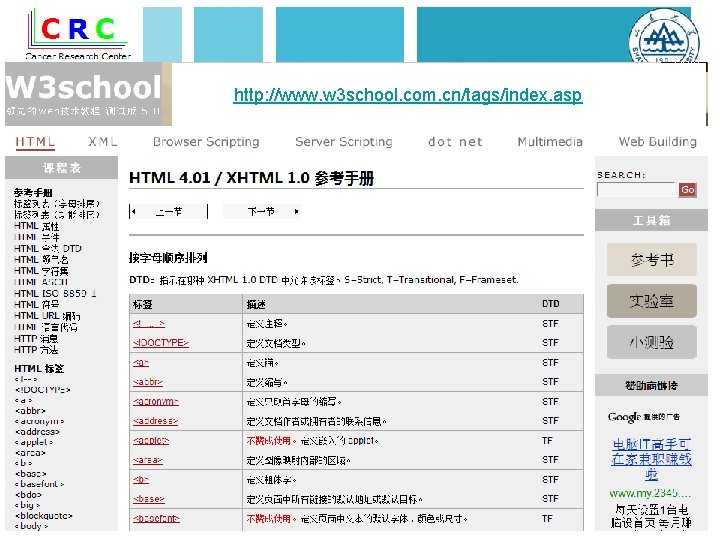
http: //www. w 3 school. com. cn/tags/index. asp
Http //mbs.meb.gov.tr/ http //www.alantercihleri.com
Http //siat.ung.ac.id atau http //pmb.ung.ac.id
Dan perl
Perl conditionals
Perl framework web
Perl round
Perl random number generator
Perl logger
Perl println
Introduction to cgi
Beginning perl for bioinformatics
Php custom exception
Soap lite
Dr jeffrey roach
Perl bioinformatics
Esb9999
Man perl
Cgi linkage in perl
Cgi linkage in perl
Perl log analysis
Regex
Perl data types
Perl bioinformatics
"commercial" perl or tcl or python
Nassim zellal
Practical extraction and reporting language
Obfuscated perl
Chromosomes examples in real life
Perl yaml example
Types of values
Perl cgi tutorial
Perl swig
Introduction to perl
Perl diamond
Perl hash table
Schengenlyzeum perl
Piper perl
Perl text manipulation
Perl linked list
Perl boolean operators
Perl paradigma
Talend