Generic Functions A generic function is one that
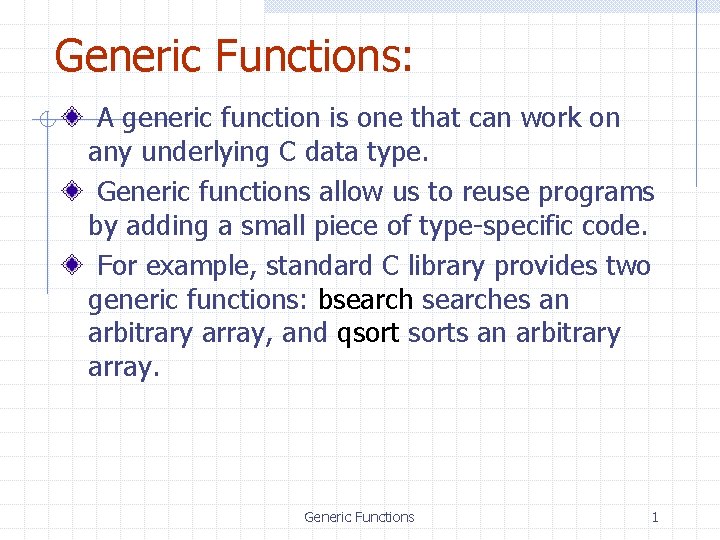
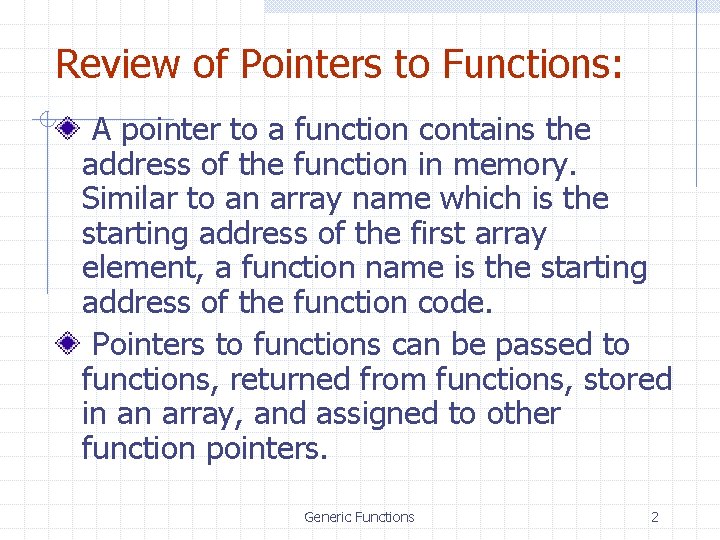
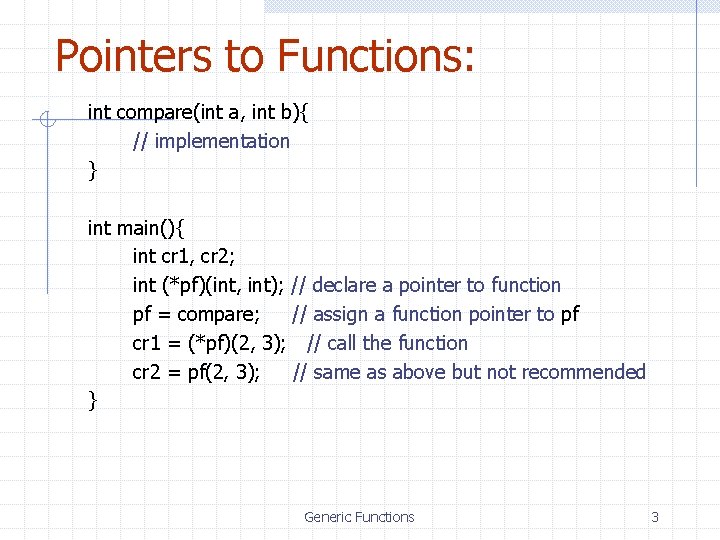
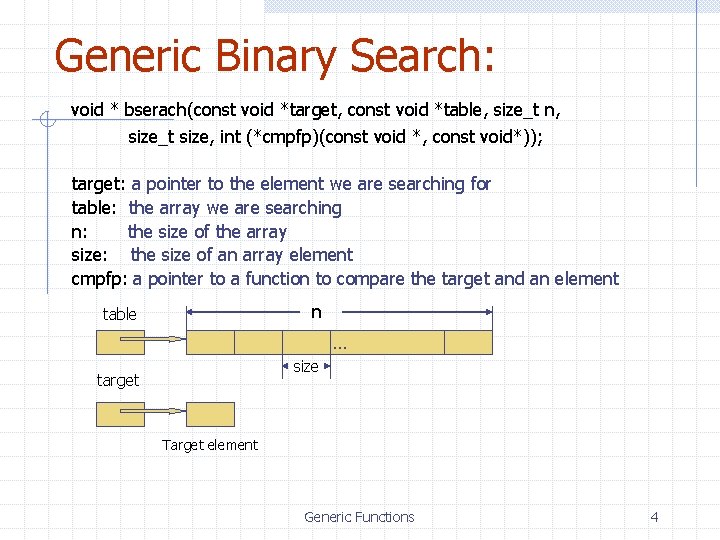
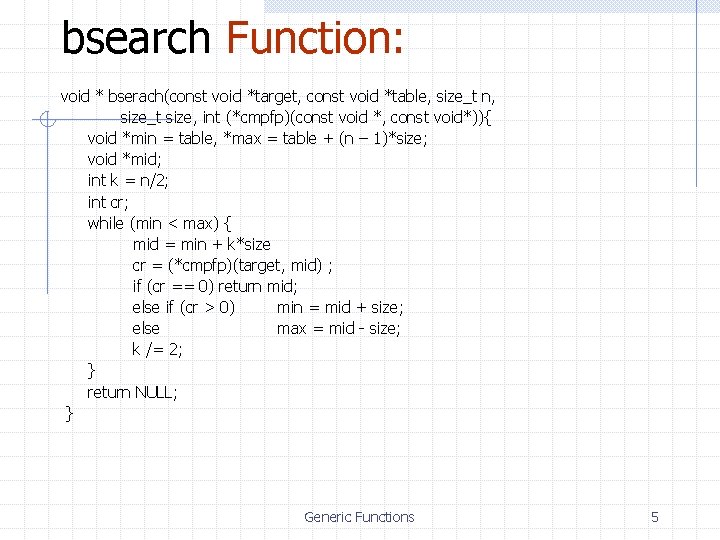
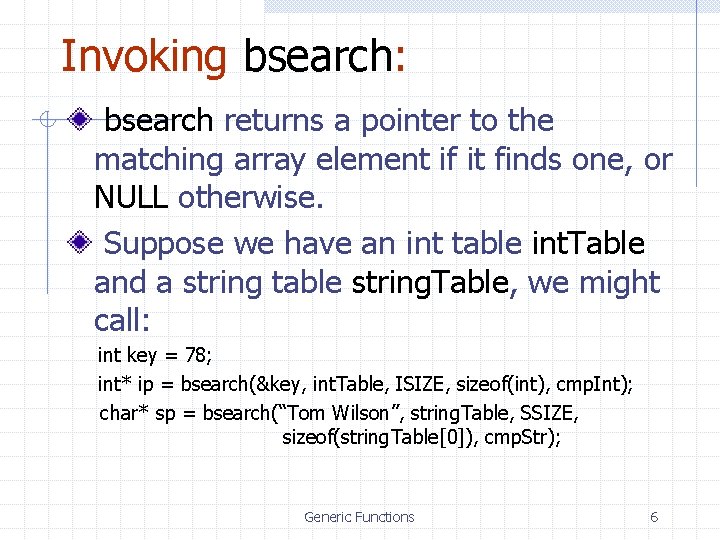
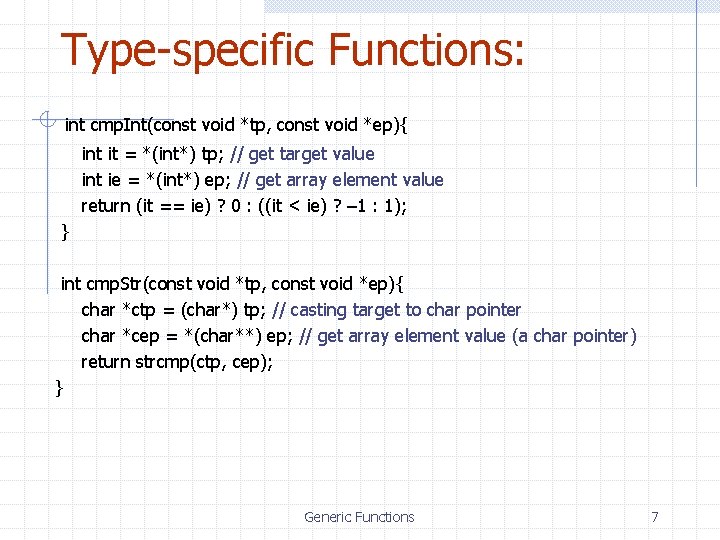
![Another Example: In-place insertion-sort an array of integers: void isort_int(int table[], int n){ int Another Example: In-place insertion-sort an array of integers: void isort_int(int table[], int n){ int](https://slidetodoc.com/presentation_image_h2/82201e4b6323afb4ff9b188af887b52d/image-8.jpg)
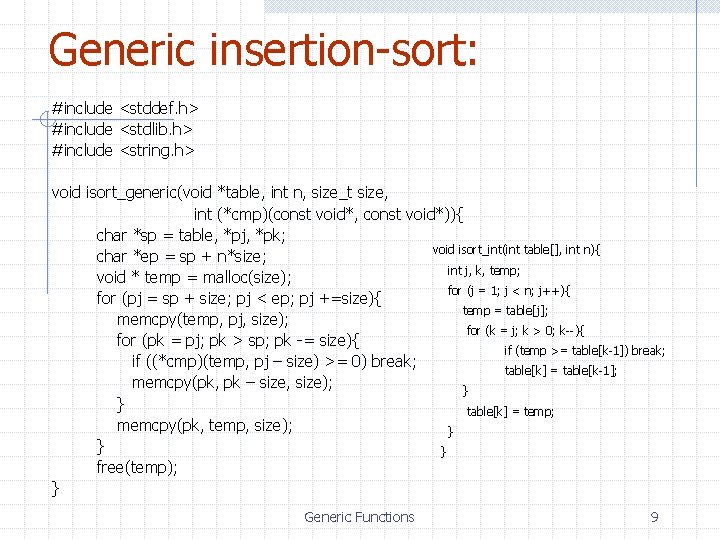
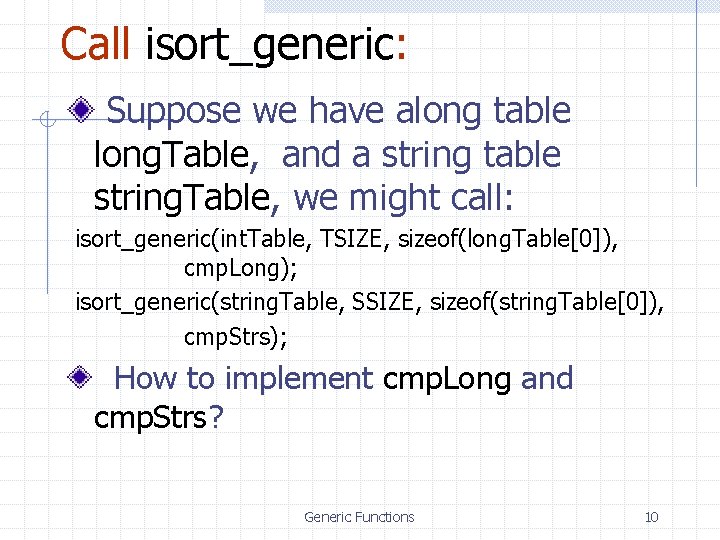
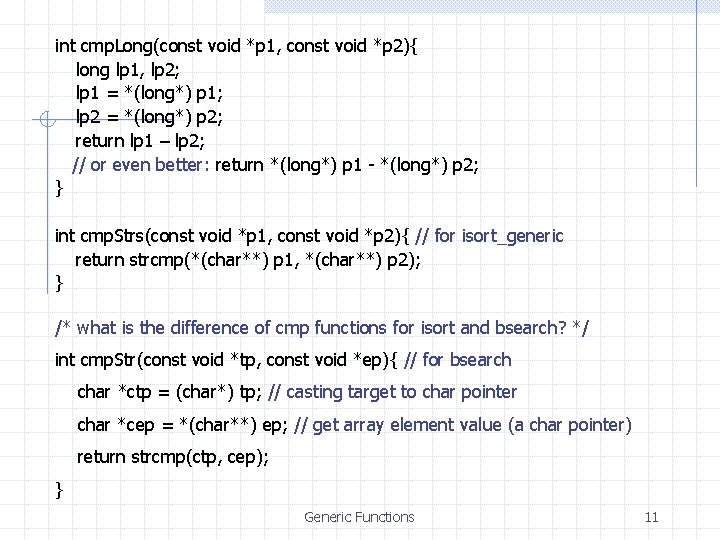
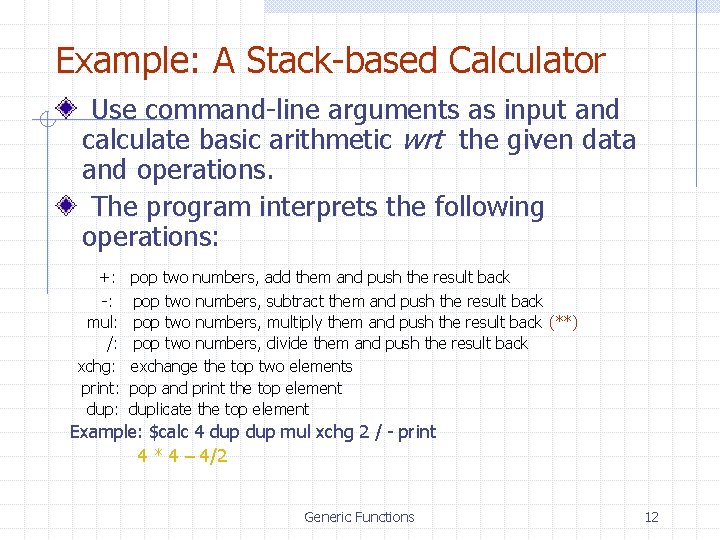
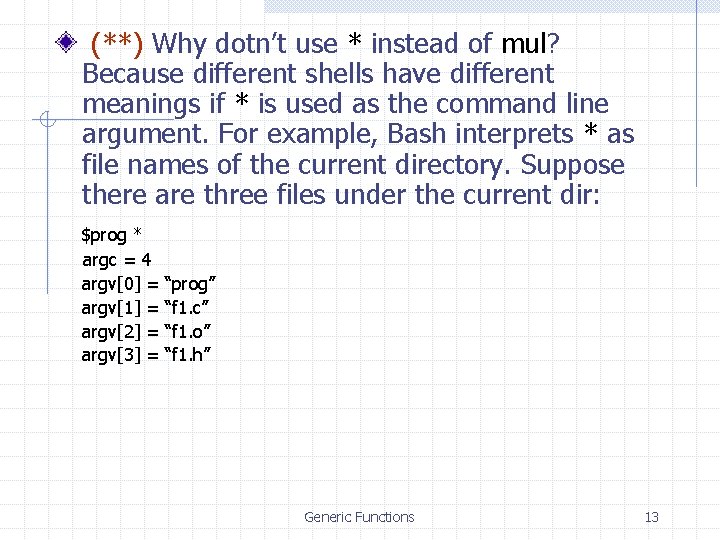
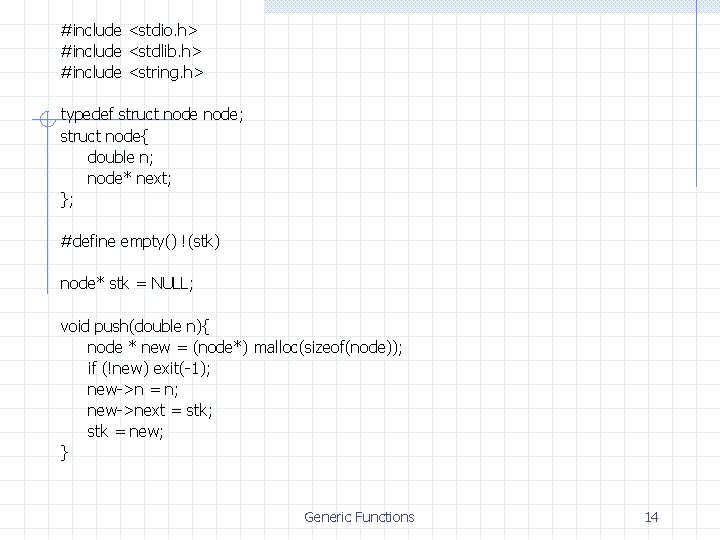
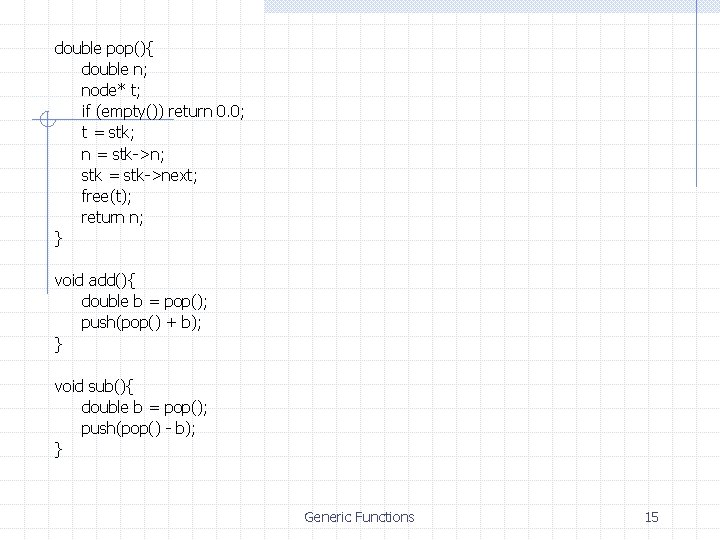
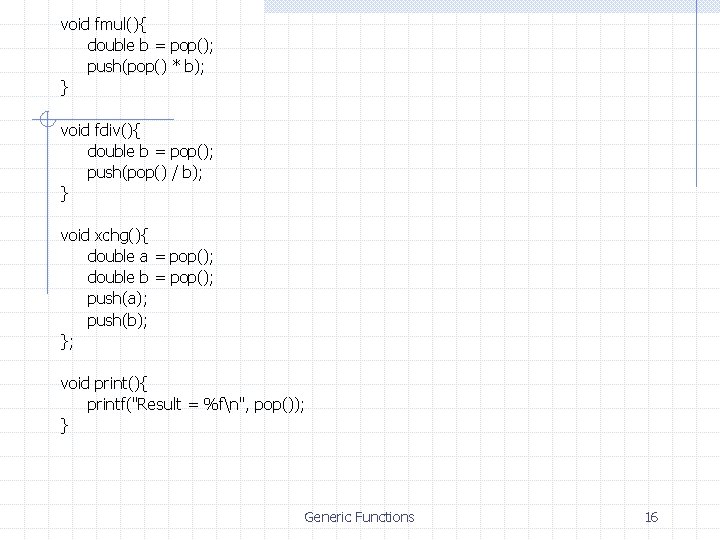
![void dup(){ double b = pop(); push(b); } char* operation[] ={ "+", "-", "mul", void dup(){ double b = pop(); push(b); } char* operation[] ={ "+", "-", "mul",](https://slidetodoc.com/presentation_image_h2/82201e4b6323afb4ff9b188af887b52d/image-17.jpg)
![int main(int argc, char* argv[]){ int i; for (i = 1; i < argc; int main(int argc, char* argv[]){ int i; for (i = 1; i < argc;](https://slidetodoc.com/presentation_image_h2/82201e4b6323afb4ff9b188af887b52d/image-18.jpg)
- Slides: 18
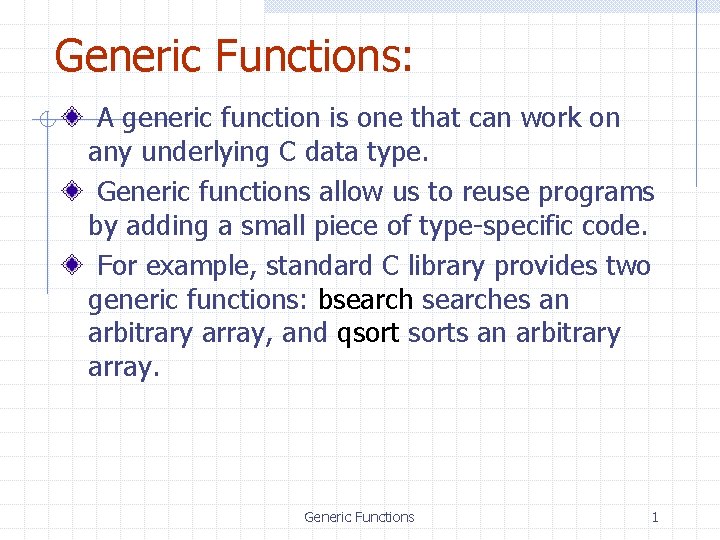
Generic Functions: A generic function is one that can work on any underlying C data type. Generic functions allow us to reuse programs by adding a small piece of type-specific code. For example, standard C library provides two generic functions: bsearches an arbitrary array, and qsorts an arbitrary array. Generic Functions 1
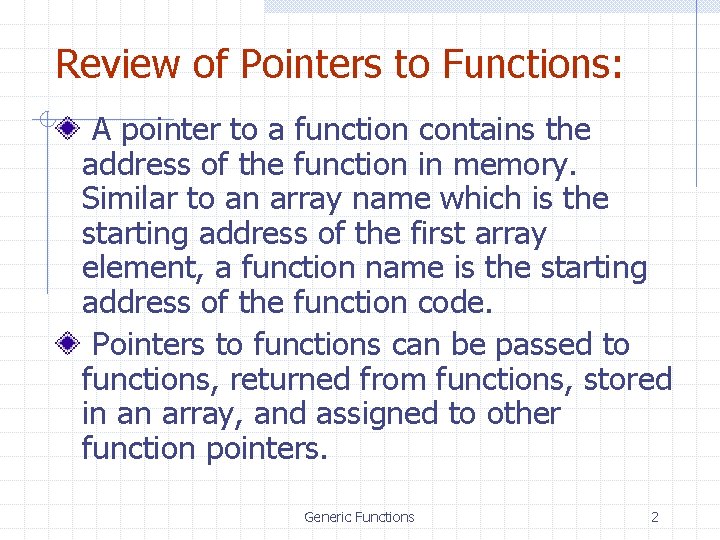
Review of Pointers to Functions: A pointer to a function contains the address of the function in memory. Similar to an array name which is the starting address of the first array element, a function name is the starting address of the function code. Pointers to functions can be passed to functions, returned from functions, stored in an array, and assigned to other function pointers. Generic Functions 2
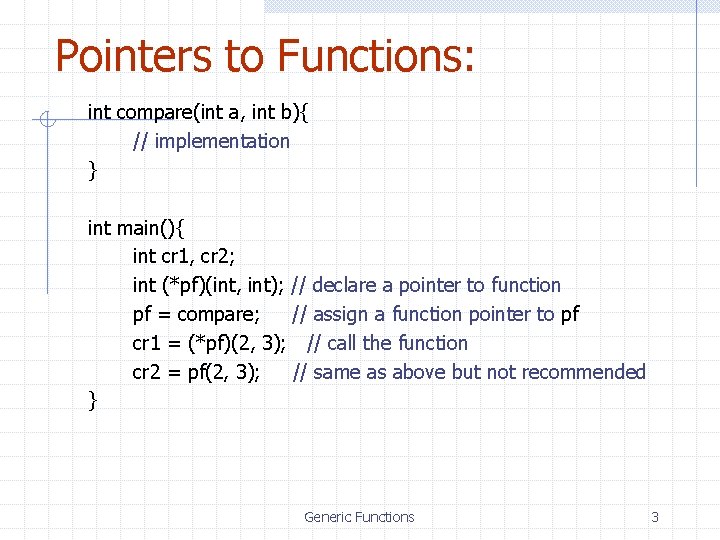
Pointers to Functions: int compare(int a, int b){ // implementation } int main(){ int cr 1, cr 2; int (*pf)(int, int); // declare a pointer to function pf = compare; // assign a function pointer to pf cr 1 = (*pf)(2, 3); // call the function cr 2 = pf(2, 3); // same as above but not recommended } Generic Functions 3
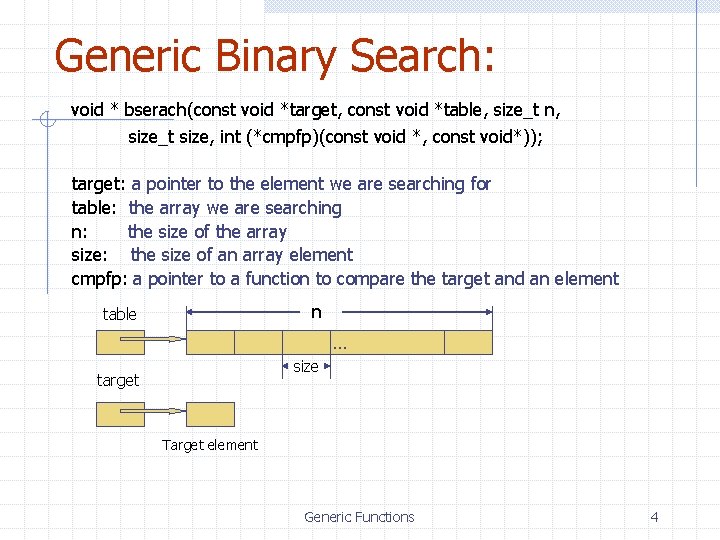
Generic Binary Search: void * bserach(const void *target, const void *table, size_t n, size_t size, int (*cmpfp)(const void *, const void*)); target: a pointer to the element we are searching for table: the array we are searching n: the size of the array size: the size of an array element cmpfp: a pointer to a function to compare the target and an element n table … size target Target element Generic Functions 4
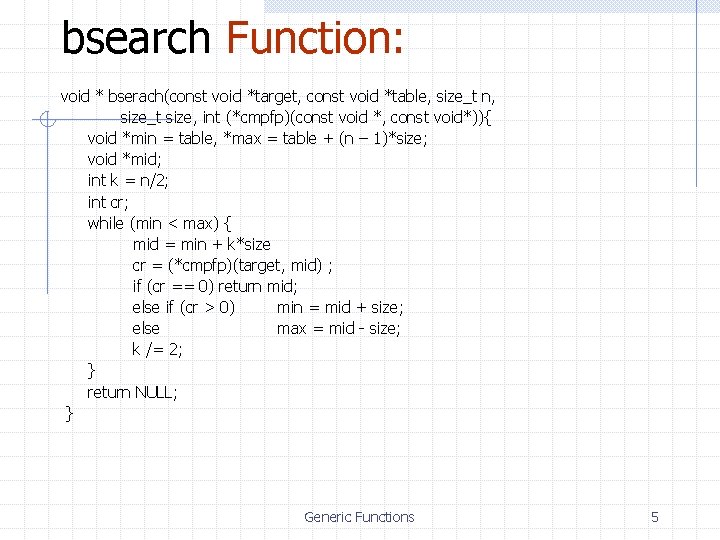
bsearch Function: void * bserach(const void *target, const void *table, size_t n, size_t size, int (*cmpfp)(const void *, const void*)){ void *min = table, *max = table + (n – 1)*size; void *mid; int k = n/2; int cr; while (min < max) { mid = min + k*size cr = (*cmpfp)(target, mid) ; if (cr == 0) return mid; else if (cr > 0) min = mid + size; else max = mid - size; k /= 2; } return NULL; } Generic Functions 5
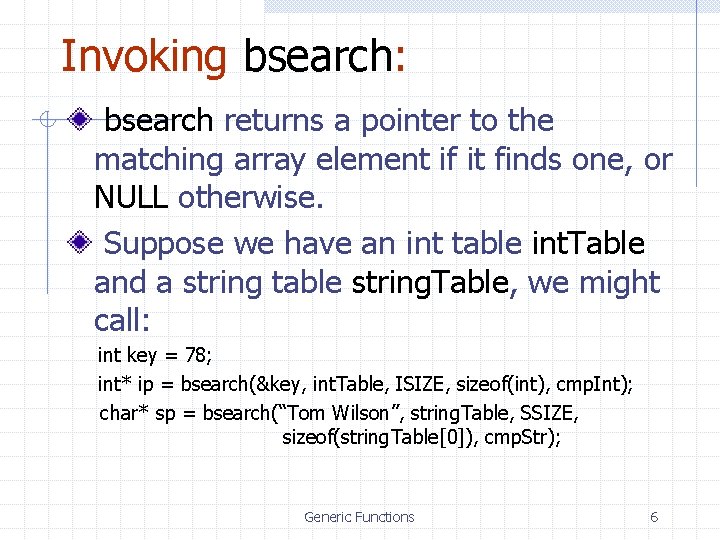
Invoking bsearch: bsearch returns a pointer to the matching array element if it finds one, or NULL otherwise. Suppose we have an int table int. Table and a string table string. Table, we might call: int key = 78; int* ip = bsearch(&key, int. Table, ISIZE, sizeof(int), cmp. Int); char* sp = bsearch(“Tom Wilson”, string. Table, SSIZE, sizeof(string. Table[0]), cmp. Str); Generic Functions 6
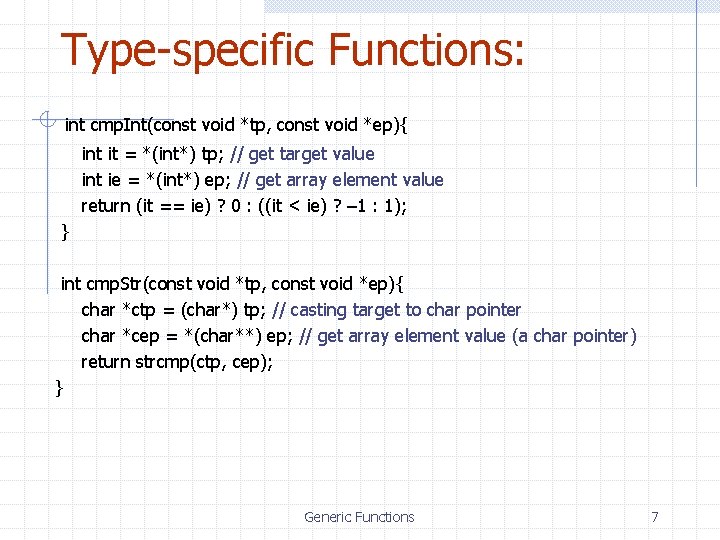
Type-specific Functions: int cmp. Int(const void *tp, const void *ep){ int it = *(int*) tp; // get target value int ie = *(int*) ep; // get array element value return (it == ie) ? 0 : ((it < ie) ? – 1 : 1); } int cmp. Str(const void *tp, const void *ep){ char *ctp = (char*) tp; // casting target to char pointer char *cep = *(char**) ep; // get array element value (a char pointer) return strcmp(ctp, cep); } Generic Functions 7
![Another Example Inplace insertionsort an array of integers void isortintint table int n int Another Example: In-place insertion-sort an array of integers: void isort_int(int table[], int n){ int](https://slidetodoc.com/presentation_image_h2/82201e4b6323afb4ff9b188af887b52d/image-8.jpg)
Another Example: In-place insertion-sort an array of integers: void isort_int(int table[], int n){ int j, k, temp; for (j = 1; j < n; j++){ temp = table[j]; for (k = j; k > 0; k--){ if (temp >= table[k-1]) break; table[k] = table[k-1]; } table[k] = temp; } } Generic Functions 8
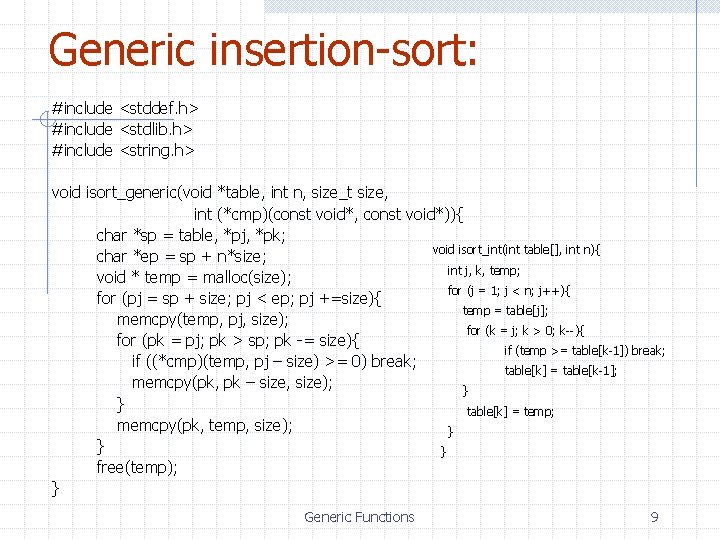
Generic insertion-sort: #include <stddef. h> #include <stdlib. h> #include <string. h> void isort_generic(void *table, int n, size_t size, int (*cmp)(const void*, const void*)){ char *sp = table, *pj, *pk; void isort_int(int table[], int n){ char *ep = sp + n*size; int j, k, temp; void * temp = malloc(size); for (j = 1; j < n; j++){ for (pj = sp + size; pj < ep; pj +=size){ temp = table[j]; memcpy(temp, pj, size); for (k = j; k > 0; k--){ for (pk = pj; pk > sp; pk -= size){ if (temp >= table[k-1]) break; if ((*cmp)(temp, pj – size) >= 0) break; table[k] = table[k-1]; memcpy(pk, pk – size, size); } } table[k] = temp; memcpy(pk, temp, size); } } } free(temp); } Generic Functions 9
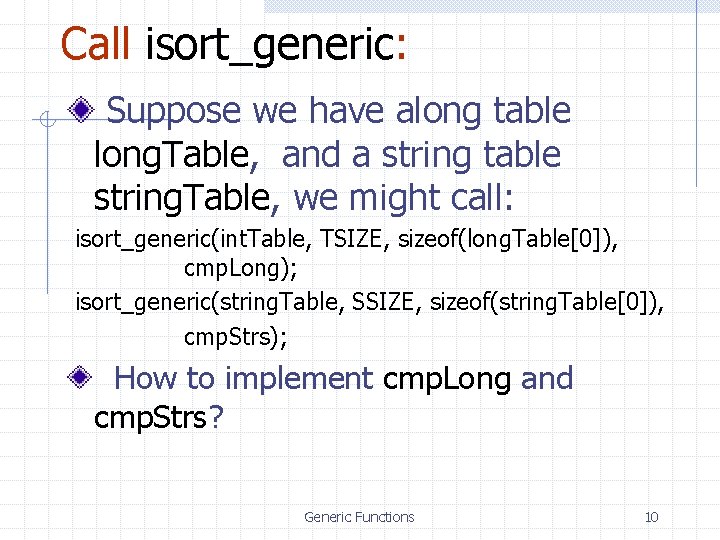
Call isort_generic: Suppose we have along table long. Table, and a string table string. Table, we might call: isort_generic(int. Table, TSIZE, sizeof(long. Table[0]), cmp. Long); isort_generic(string. Table, SSIZE, sizeof(string. Table[0]), cmp. Strs); How to implement cmp. Long and cmp. Strs? Generic Functions 10
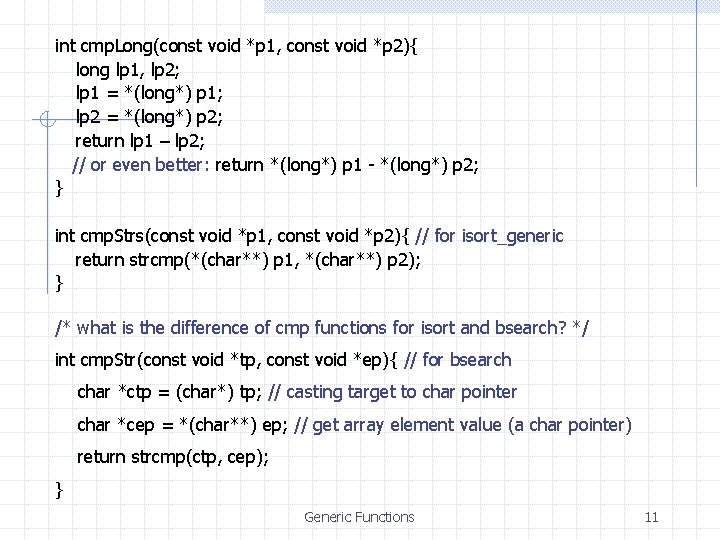
int cmp. Long(const void *p 1, const void *p 2){ long lp 1, lp 2; lp 1 = *(long*) p 1; lp 2 = *(long*) p 2; return lp 1 – lp 2; // or even better: return *(long*) p 1 - *(long*) p 2; } int cmp. Strs(const void *p 1, const void *p 2){ // for isort_generic return strcmp(*(char**) p 1, *(char**) p 2); } /* what is the difference of cmp functions for isort and bsearch? */ int cmp. Str(const void *tp, const void *ep){ // for bsearch char *ctp = (char*) tp; // casting target to char pointer char *cep = *(char**) ep; // get array element value (a char pointer) return strcmp(ctp, cep); } Generic Functions 11
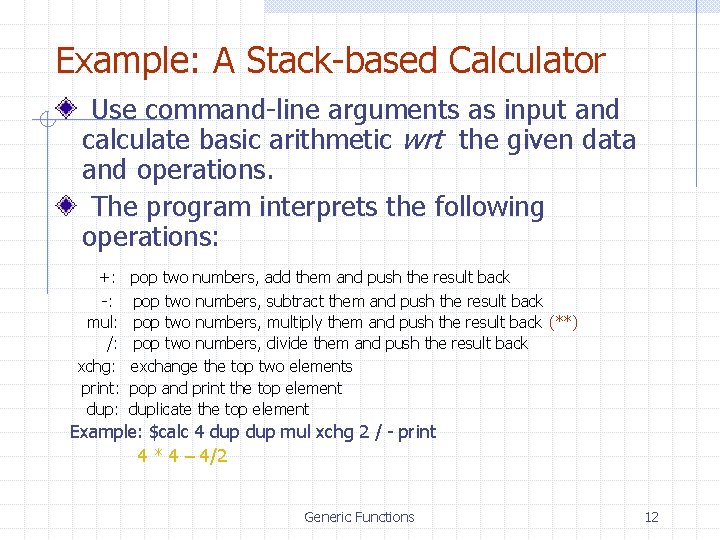
Example: A Stack-based Calculator Use command-line arguments as input and calculate basic arithmetic wrt the given data and operations. The program interprets the following operations: +: -: mul: /: xchg: print: dup: pop two numbers, add them and push the result back pop two numbers, subtract them and push the result back pop two numbers, multiply them and push the result back (**) pop two numbers, divide them and push the result back exchange the top two elements pop and print the top element duplicate the top element Example: $calc 4 dup mul xchg 2 / - print 4 * 4 – 4/2 Generic Functions 12
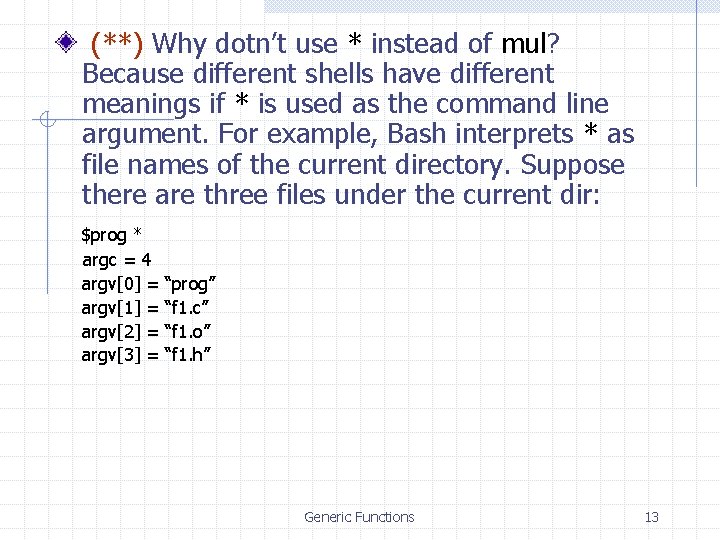
(**) Why dotn’t use * instead of mul? Because different shells have different meanings if * is used as the command line argument. For example, Bash interprets * as file names of the current directory. Suppose there are three files under the current dir: $prog * argc = 4 argv[0] = argv[1] = argv[2] = argv[3] = “prog” “f 1. c” “f 1. o” “f 1. h” Generic Functions 13
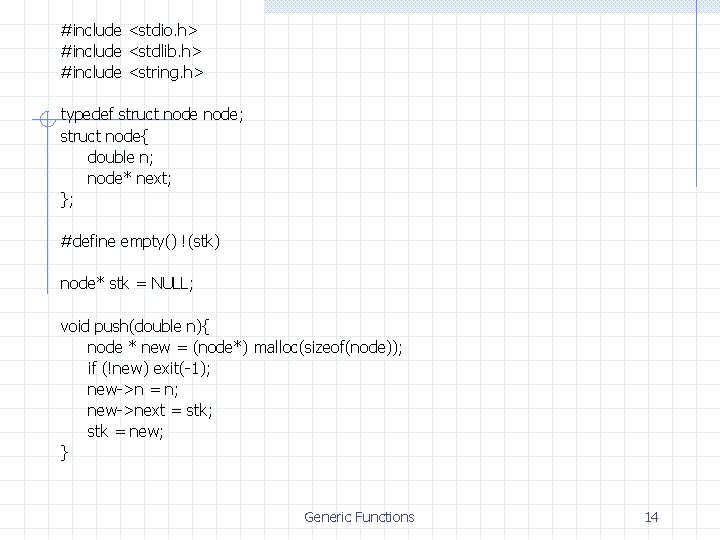
#include <stdio. h> #include <stdlib. h> #include <string. h> typedef struct node; struct node{ double n; node* next; }; #define empty() !(stk) node* stk = NULL; void push(double n){ node * new = (node*) malloc(sizeof(node)); if (!new) exit(-1); new->n = n; new->next = stk; stk = new; } Generic Functions 14
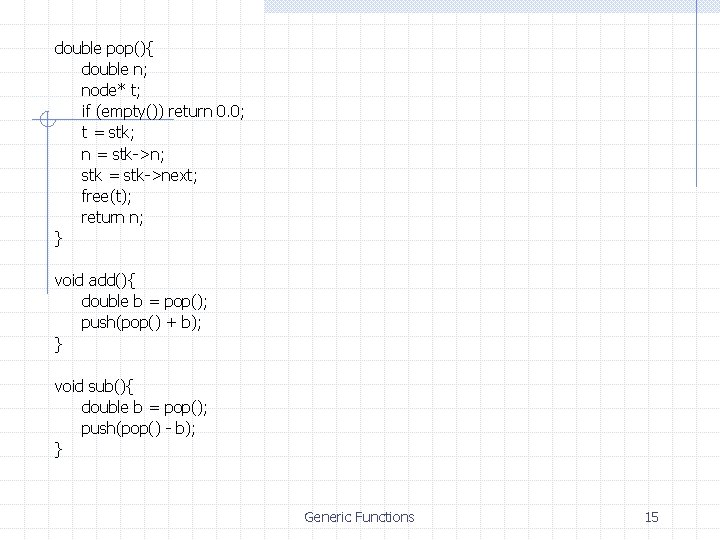
double pop(){ double n; node* t; if (empty()) return 0. 0; t = stk; n = stk->n; stk = stk->next; free(t); return n; } void add(){ double b = pop(); push(pop() + b); } void sub(){ double b = pop(); push(pop() - b); } Generic Functions 15
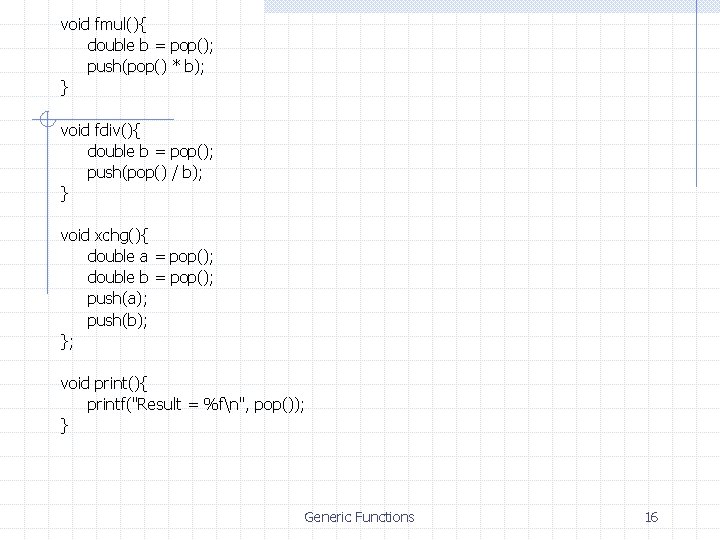
void fmul(){ double b = pop(); push(pop() * b); } void fdiv(){ double b = pop(); push(pop() / b); } void xchg(){ double a = pop(); double b = pop(); push(a); push(b); }; void print(){ printf("Result = %fn", pop()); } Generic Functions 16
![void dup double b pop pushb char operation mul void dup(){ double b = pop(); push(b); } char* operation[] ={ "+", "-", "mul",](https://slidetodoc.com/presentation_image_h2/82201e4b6323afb4ff9b188af887b52d/image-17.jpg)
void dup(){ double b = pop(); push(b); } char* operation[] ={ "+", "-", "mul", "/", "xchg", "print", "dup"}; void (*action[])() = {add, sub, fmul, fdiv, xchg, print, dup}; void exec(char * cmd){ int i; for (i = 0; i < sizeof(operation)/sizeof(char*); i++){ if (strcmp(cmd, operation[i]) == 0){ (*action[i])(); return; } } printf(“Illegal operation %sn”, cmd); } Generic Functions 17
![int mainint argc char argv int i for i 1 i argc int main(int argc, char* argv[]){ int i; for (i = 1; i < argc;](https://slidetodoc.com/presentation_image_h2/82201e4b6323afb4ff9b188af887b52d/image-18.jpg)
int main(int argc, char* argv[]){ int i; for (i = 1; i < argc; i++){ if (isdigit(argv[i][0])) push(atof(argv[i])); else exec(argv[i]); } exit(0); } Generic Functions 18
One to one and onto
Ngoại tâm thu thất chùm đôi
Block xoang nhĩ ecg
Thơ thất ngôn tứ tuyệt đường luật
Thơ thất ngôn tứ tuyệt đường luật
Walmart thất bại ở nhật
Tìm vết của mặt phẳng
Con hãy đưa tay khi thấy người vấp ngã
Tôn thất thuyết là ai
Gây tê cơ vuông thắt lưng
Sau thất bại ở hồ điển triệt
Are cubic functions one to one
One god one empire one religion
One one one little dog run
One king one law one faith
One empire one god one emperor
One team one plan one goal
See one do one teach one
See one, do one, teach one