FLTK The Fast Light Toolkit A C graphical
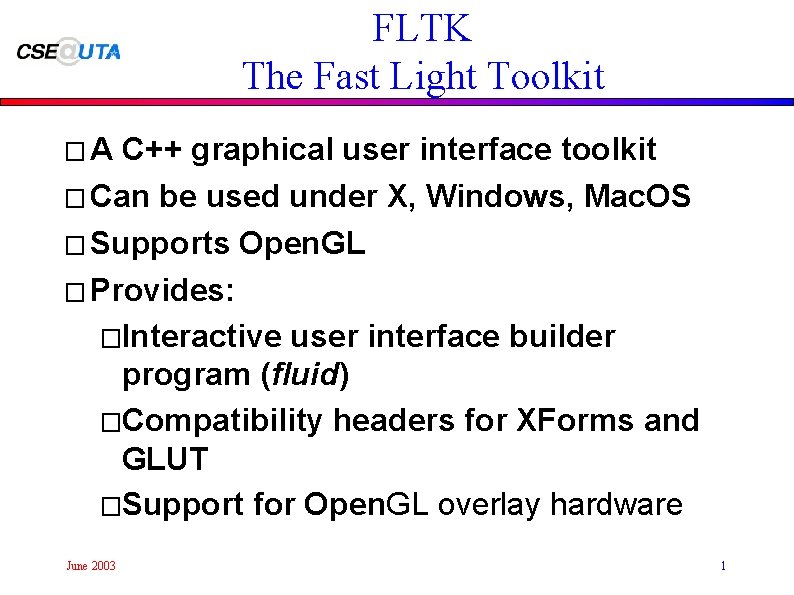
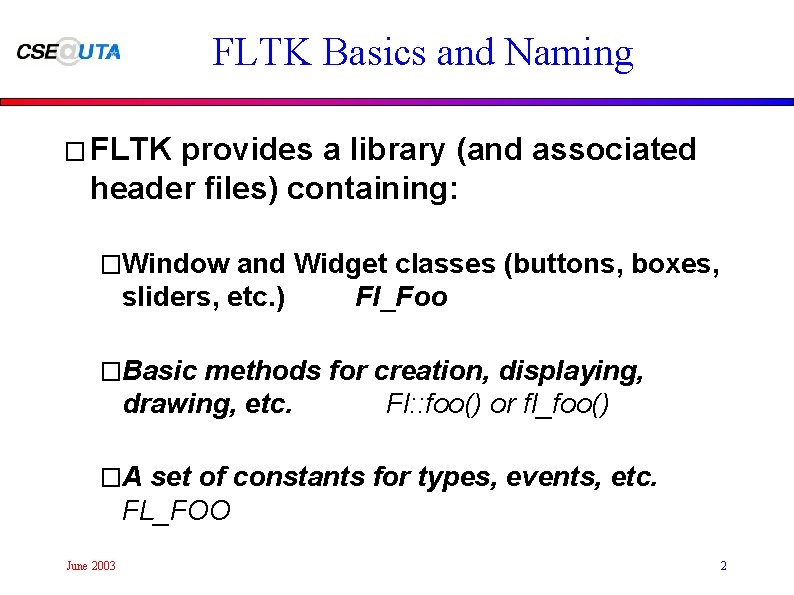
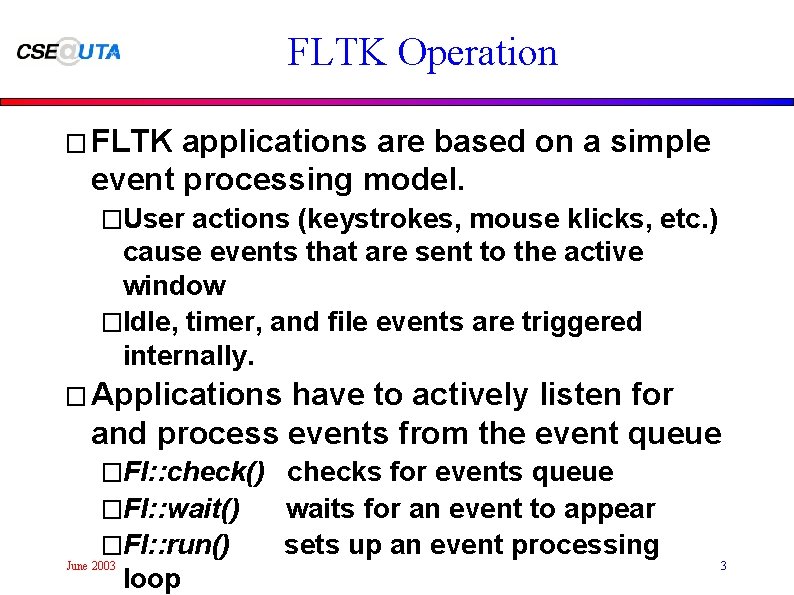
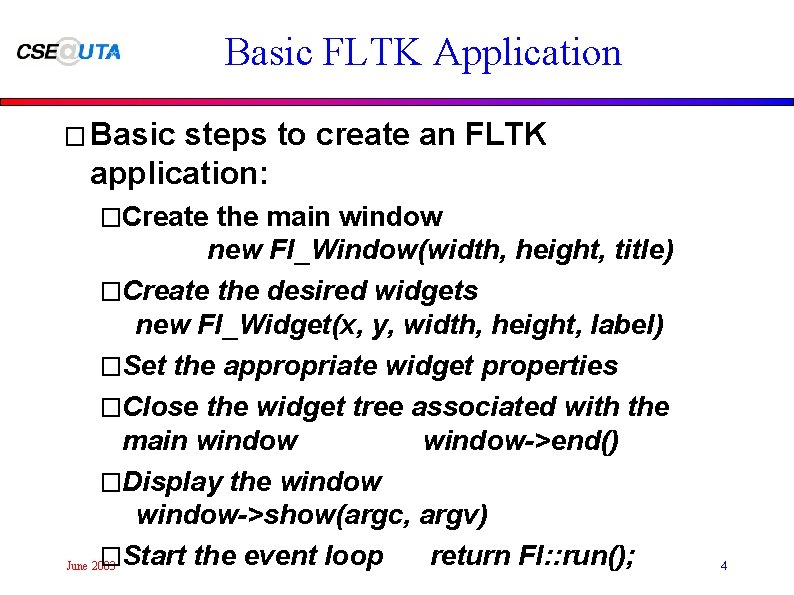
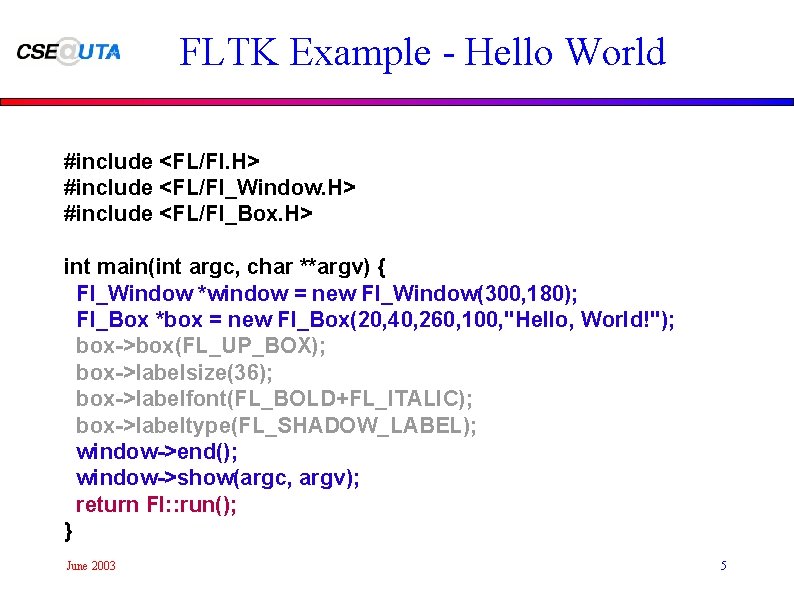
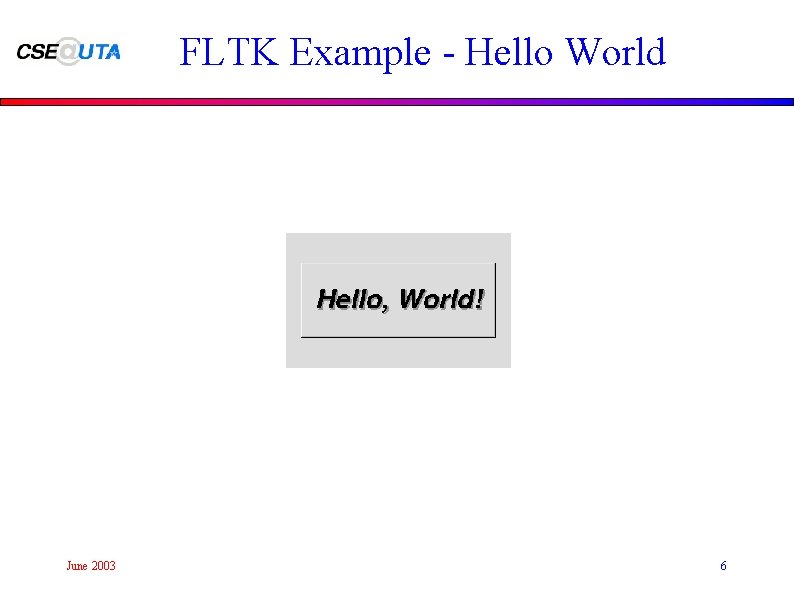
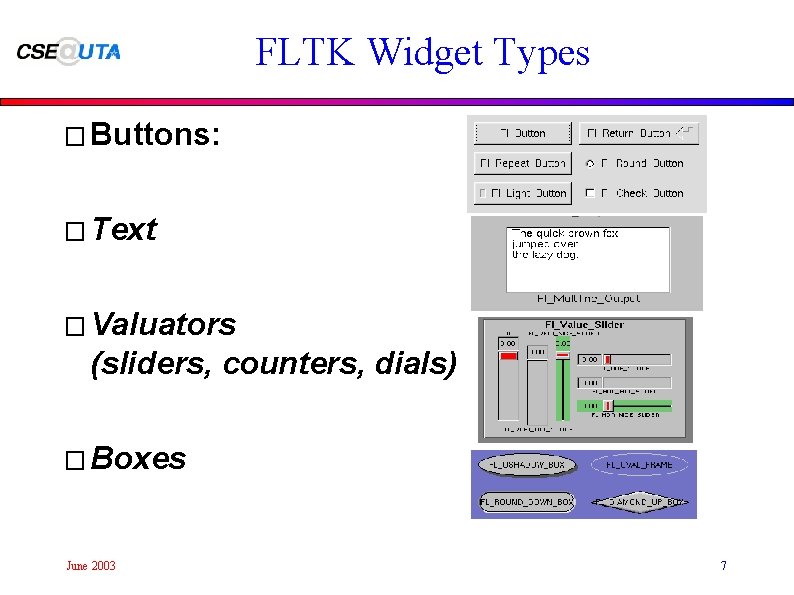
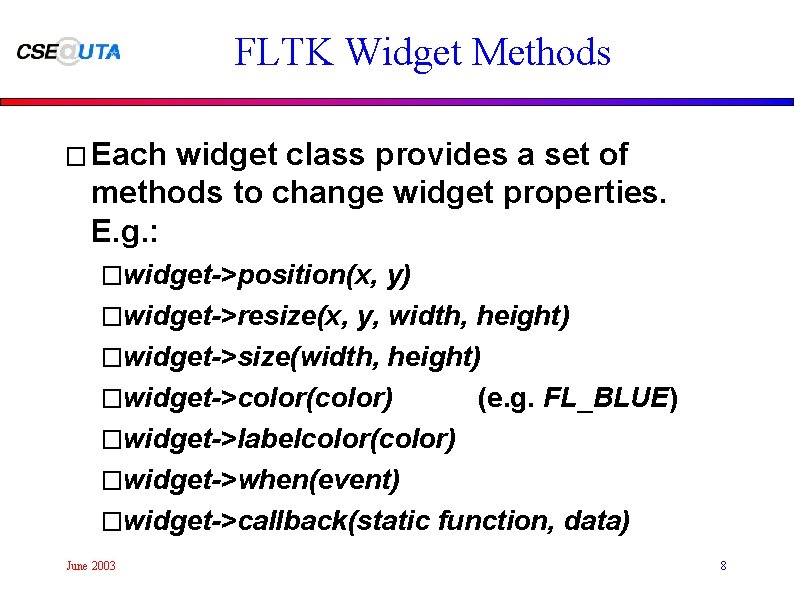
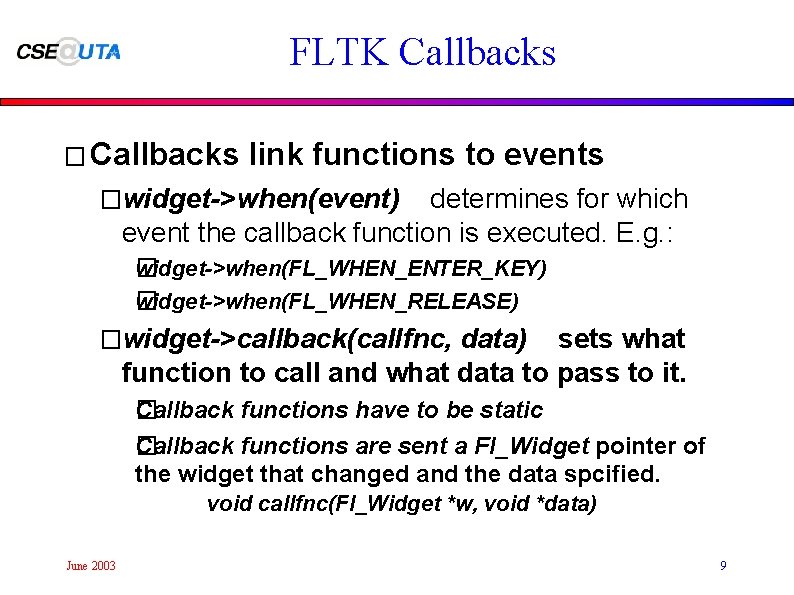
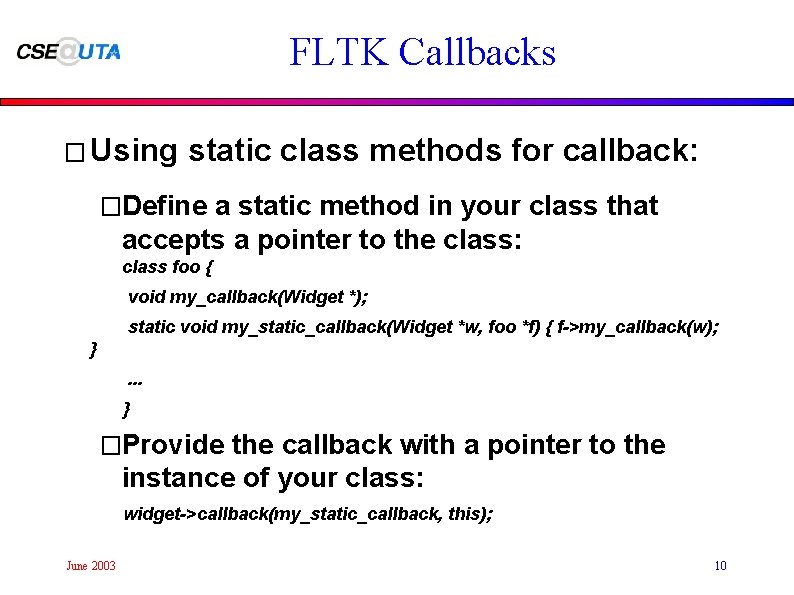
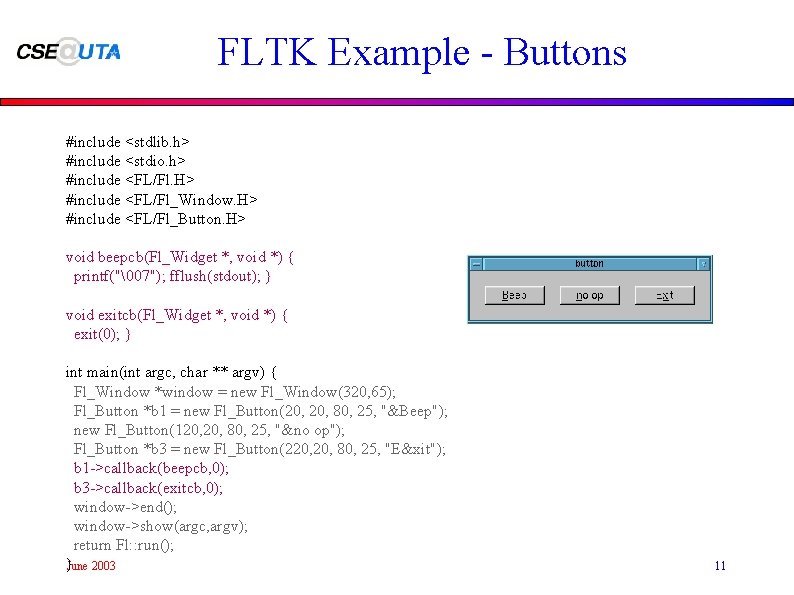
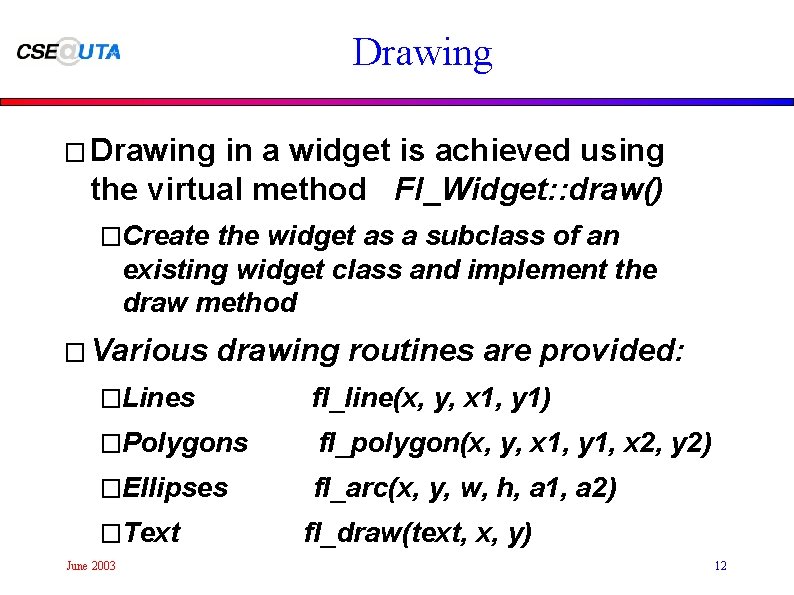
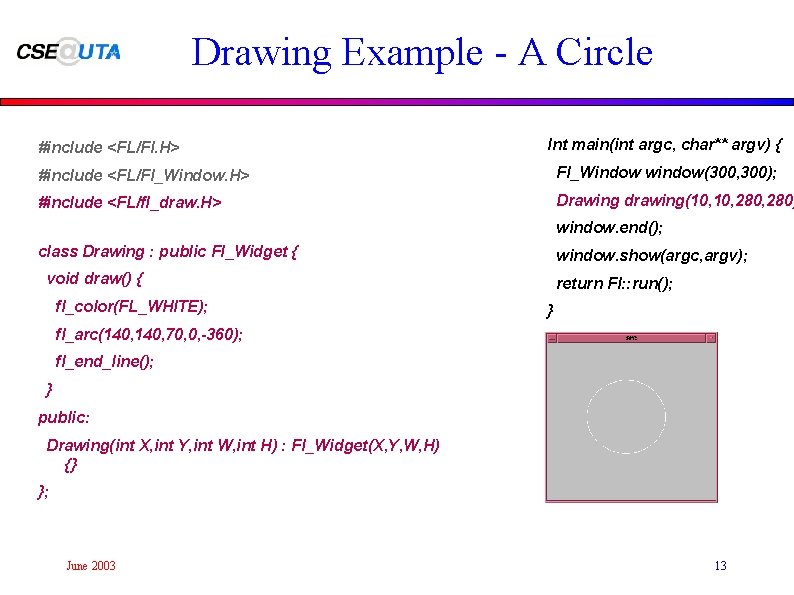
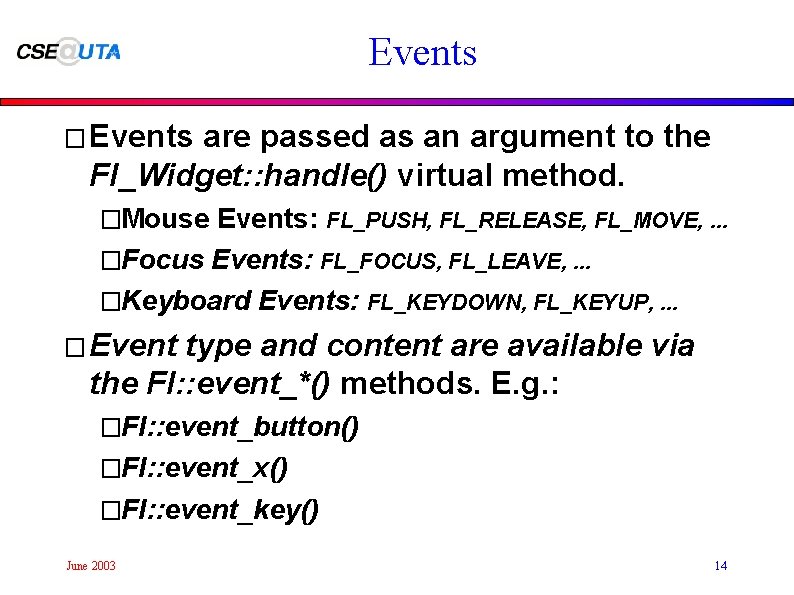
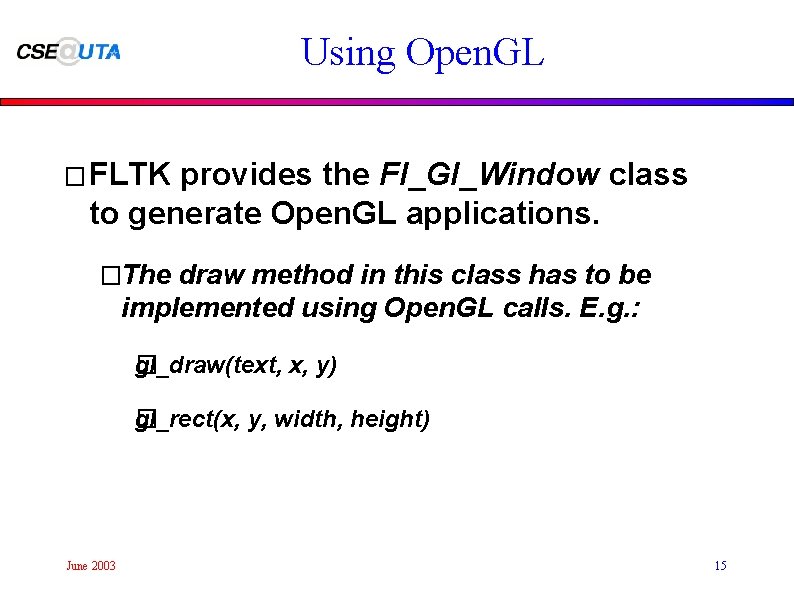
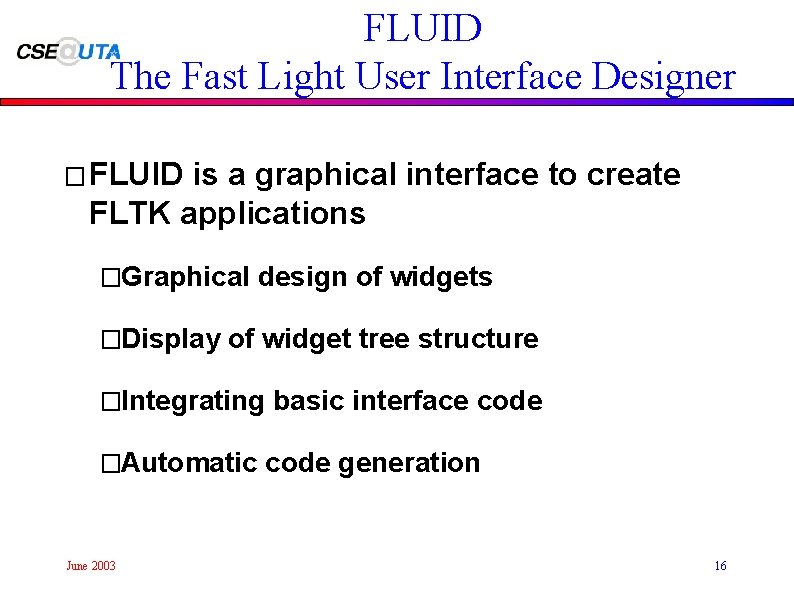
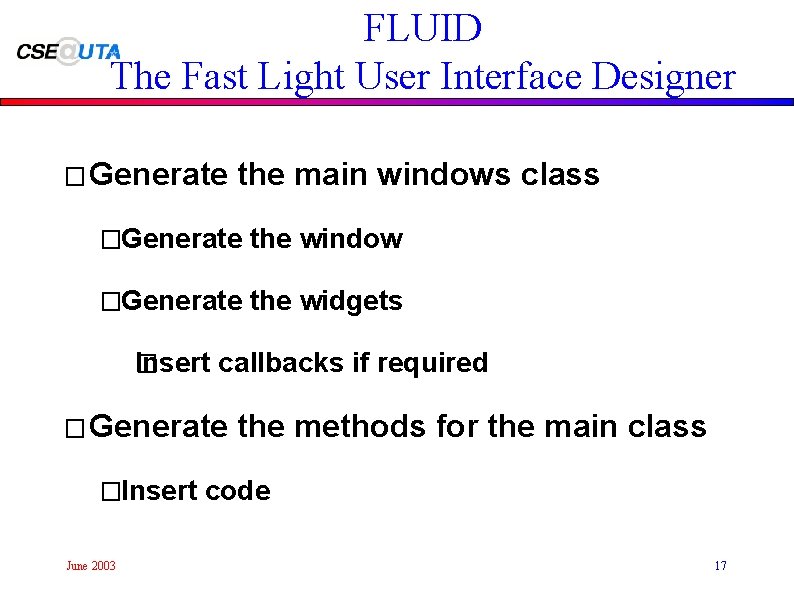
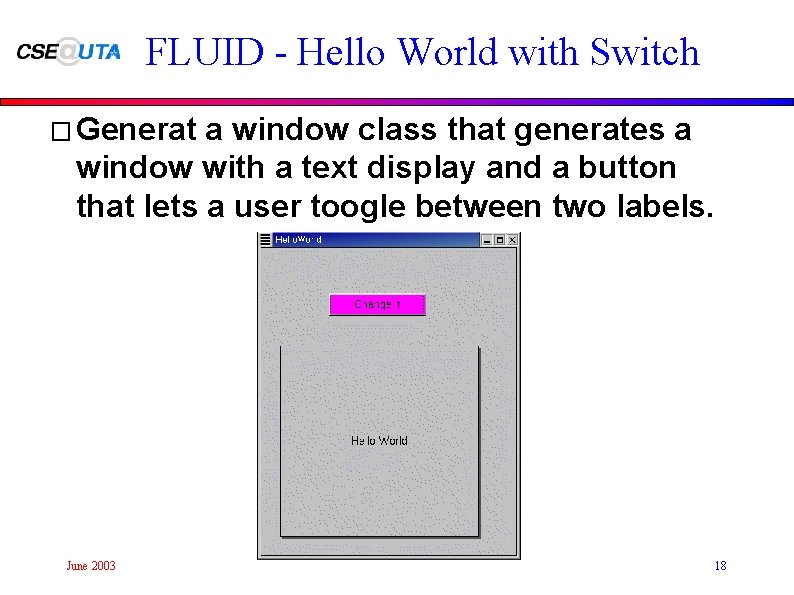
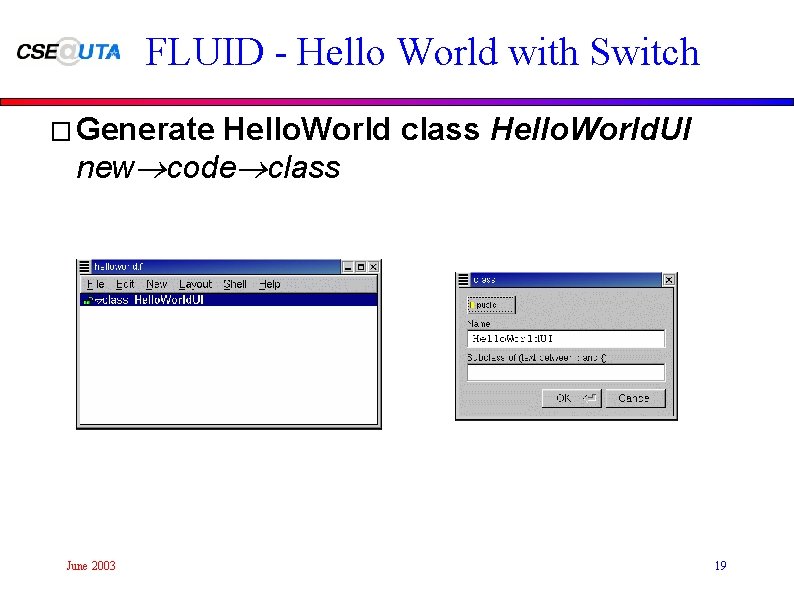
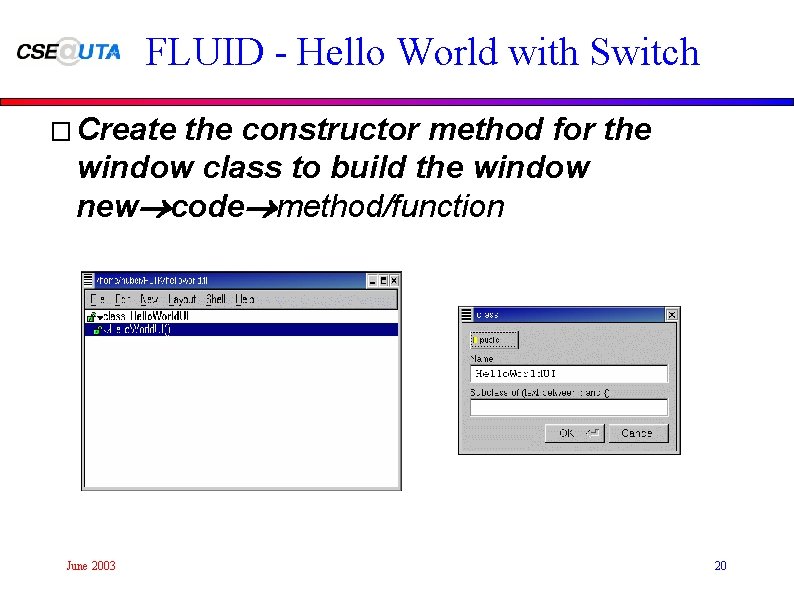
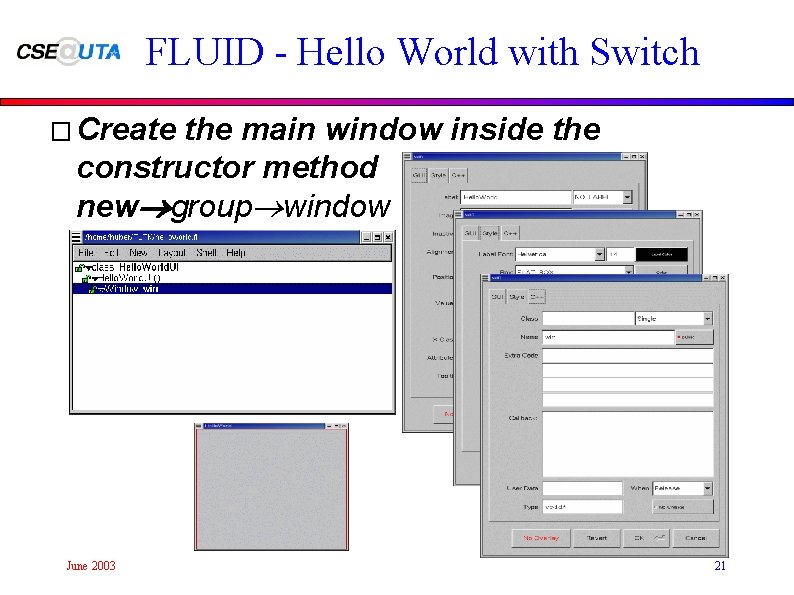
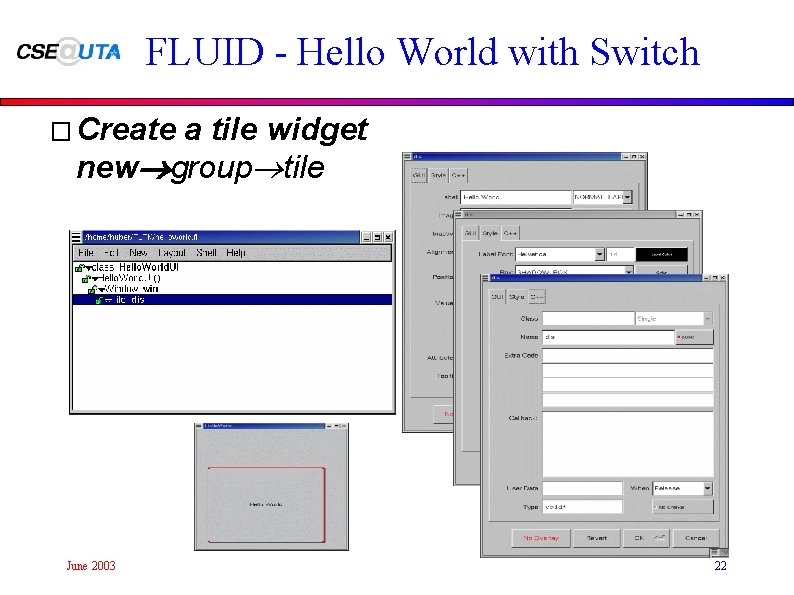
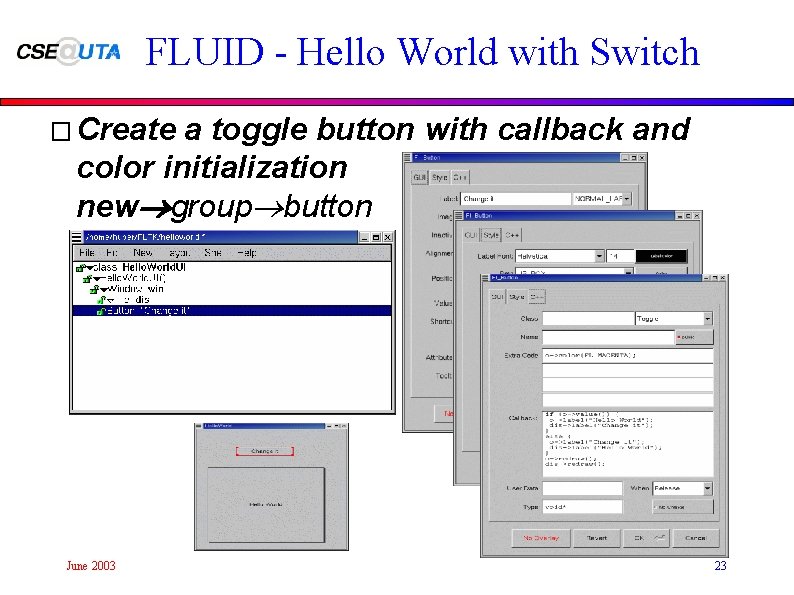
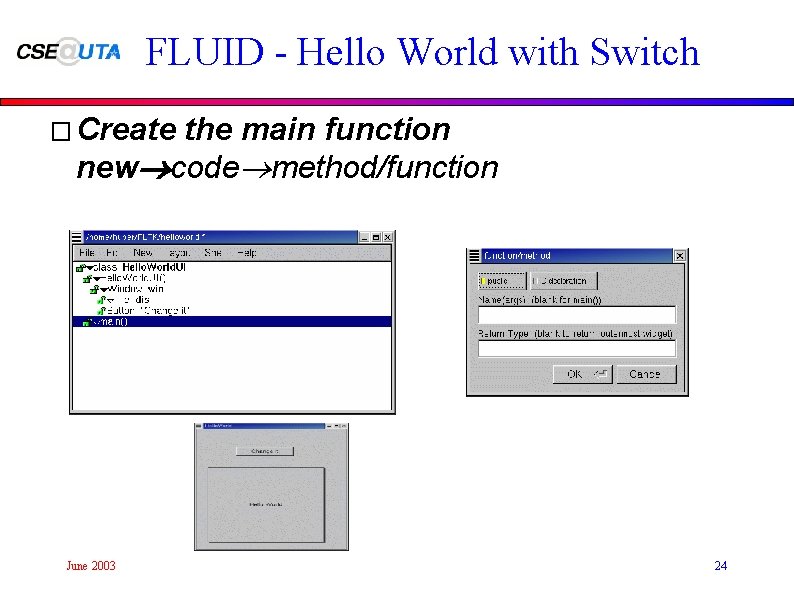
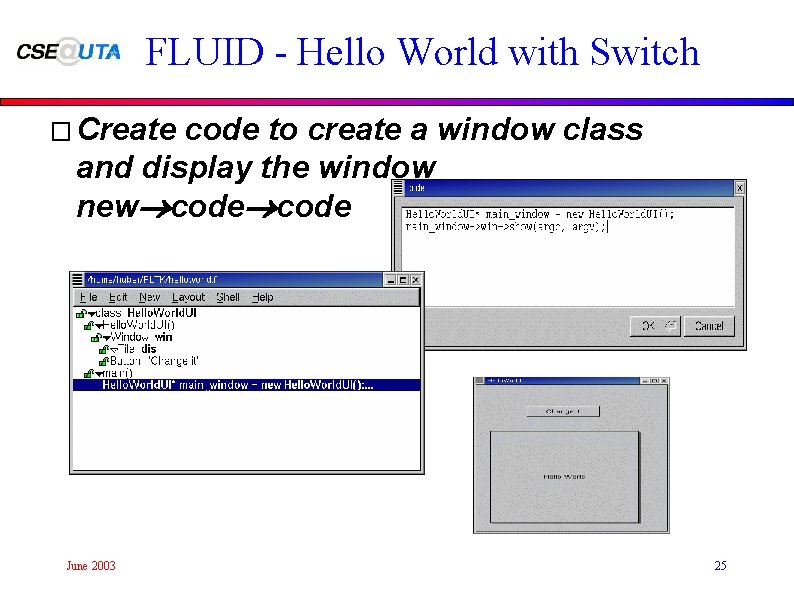
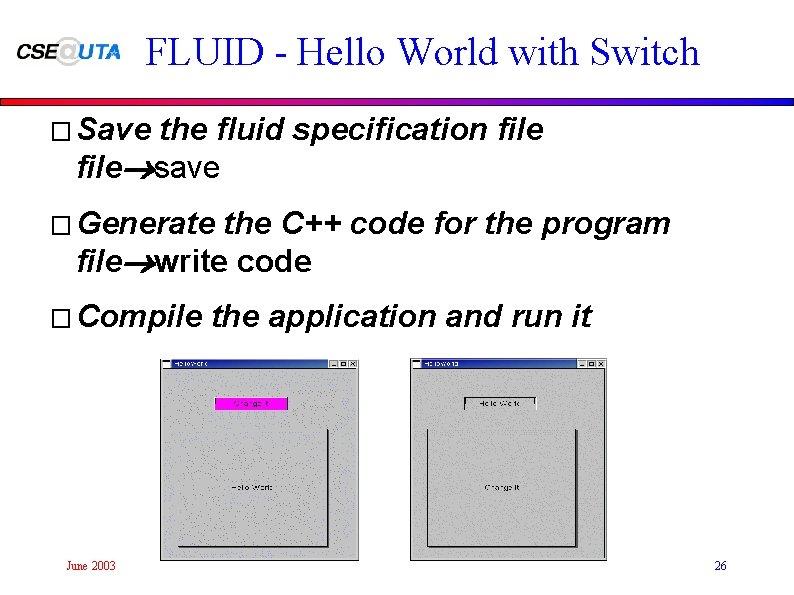
- Slides: 26
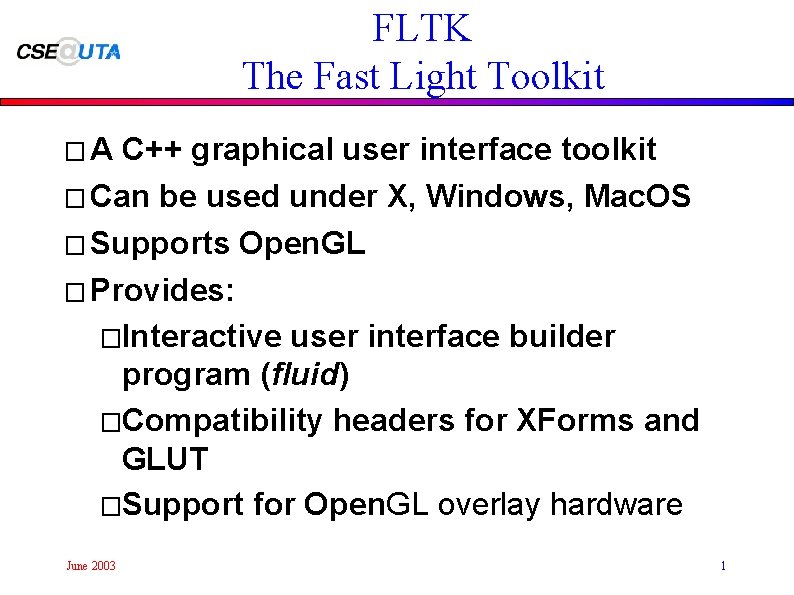
FLTK The Fast Light Toolkit �A C++ graphical user interface toolkit � Can be used under X, Windows, Mac. OS � Supports Open. GL � Provides: �Interactive user interface builder program (fluid) �Compatibility headers for XForms and GLUT �Support for Open. GL overlay hardware June 2003 1
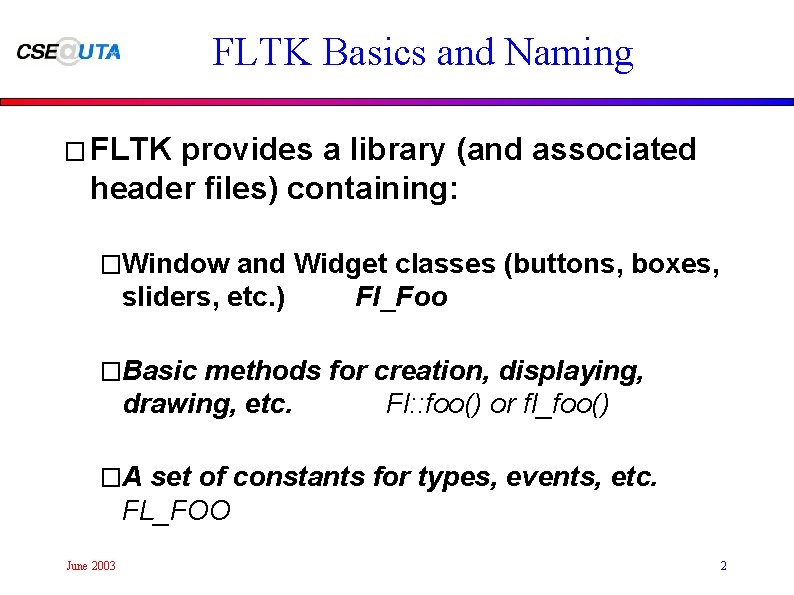
FLTK Basics and Naming � FLTK provides a library (and associated header files) containing: �Window and Widget classes (buttons, boxes, sliders, etc. ) Fl_Foo �Basic methods for creation, displaying, drawing, etc. Fl: : foo() or fl_foo() �A set of constants for types, events, etc. FL_FOO June 2003 2
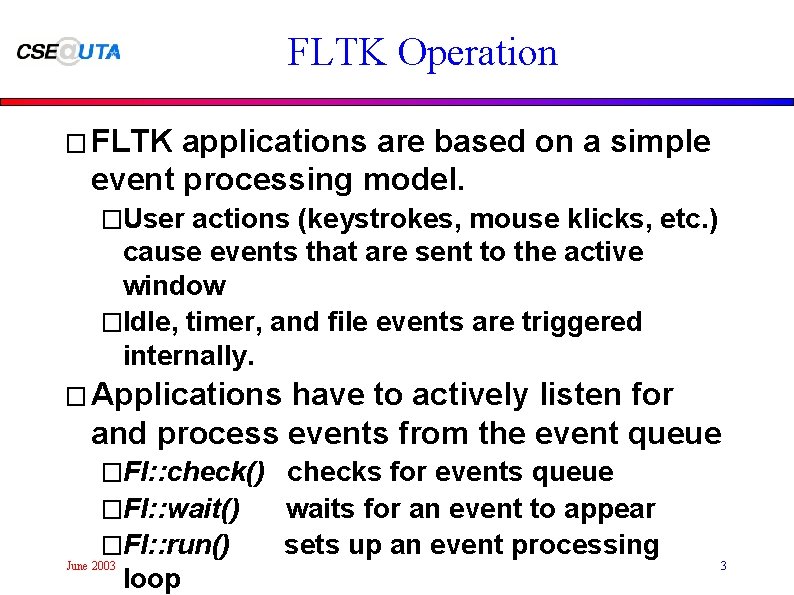
FLTK Operation � FLTK applications are based on a simple event processing model. �User actions (keystrokes, mouse klicks, etc. ) cause events that are sent to the active window �Idle, timer, and file events are triggered internally. � Applications have to actively listen for and process events from the event queue �Fl: : check() checks for events queue �Fl: : wait() �Fl: : run() June 2003 loop waits for an event to appear sets up an event processing 3
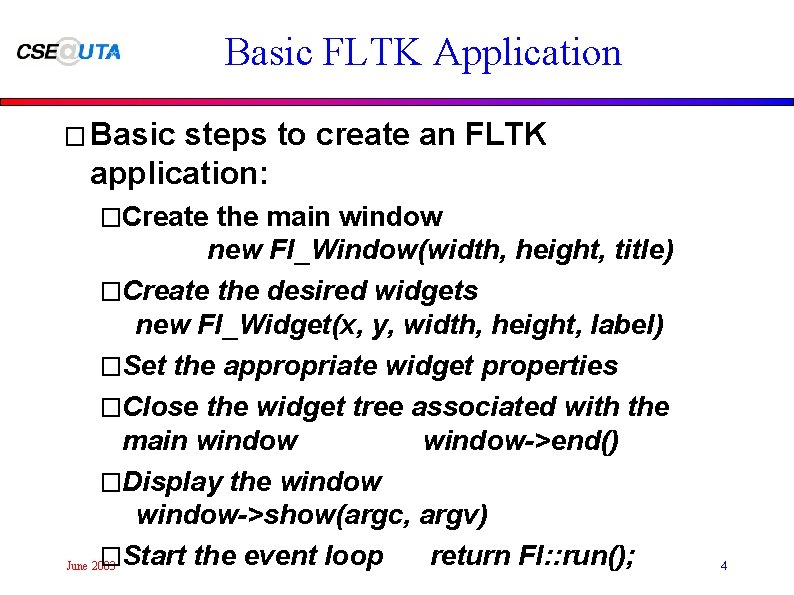
Basic FLTK Application � Basic steps to create an FLTK application: �Create the main window new Fl_Window(width, height, title) �Create the desired widgets new Fl_Widget(x, y, width, height, label) �Set the appropriate widget properties �Close the widget tree associated with the main window->end() �Display the window->show(argc, argv) �Start the event loop return Fl: : run(); June 2003 4
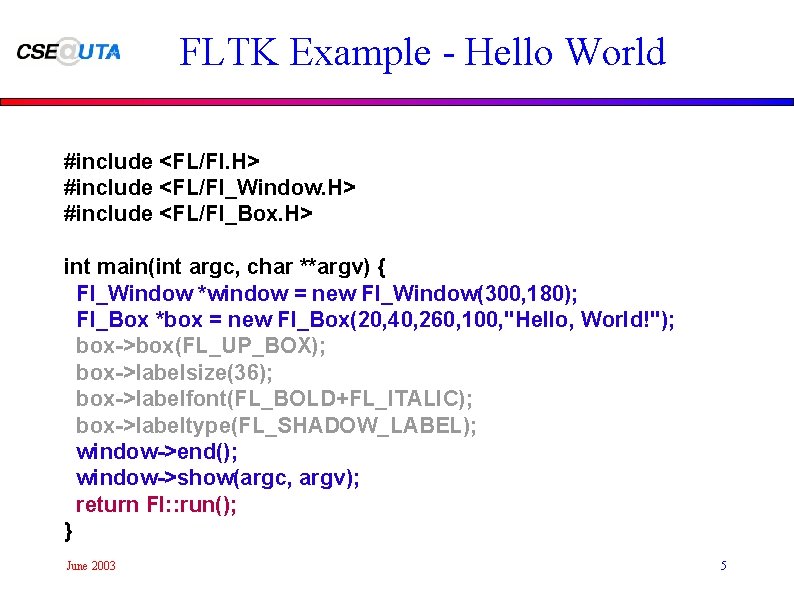
FLTK Example - Hello World #include <FL/Fl. H> #include <FL/Fl_Window. H> #include <FL/Fl_Box. H> int main(int argc, char **argv) { Fl_Window *window = new Fl_Window(300, 180); Fl_Box *box = new Fl_Box(20, 40, 260, 100, "Hello, World!"); box->box(FL_UP_BOX); box->labelsize(36); box->labelfont(FL_BOLD+FL_ITALIC); box->labeltype(FL_SHADOW_LABEL); window->end(); window->show(argc, argv); return Fl: : run(); } June 2003 5
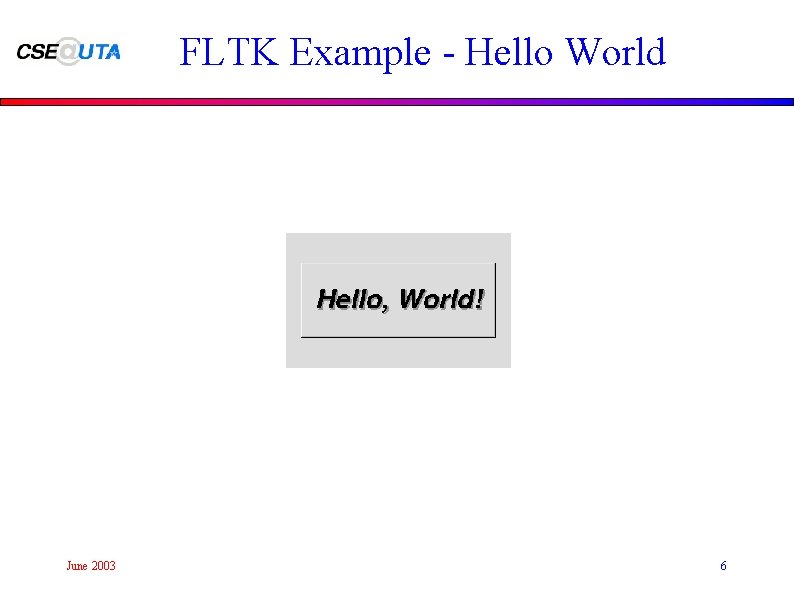
FLTK Example - Hello World June 2003 6
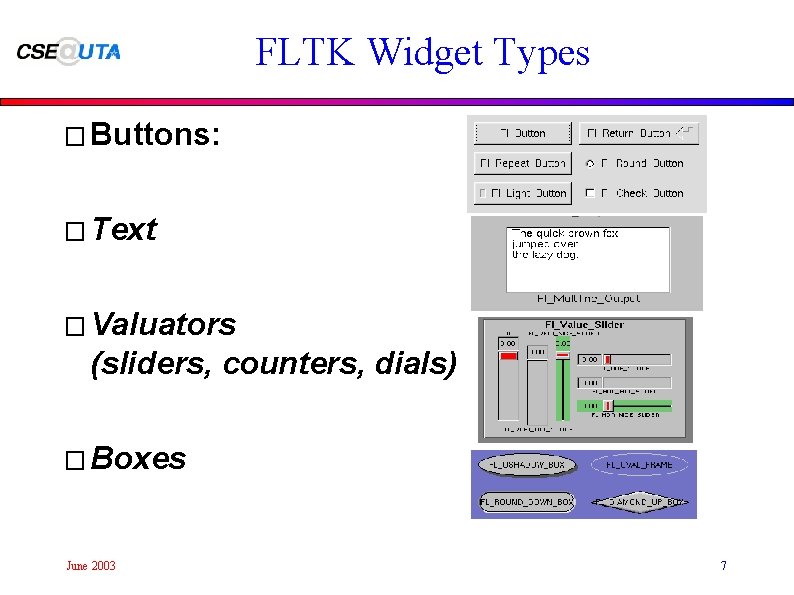
FLTK Widget Types � Buttons: � Text � Valuators (sliders, counters, dials) � Boxes June 2003 7
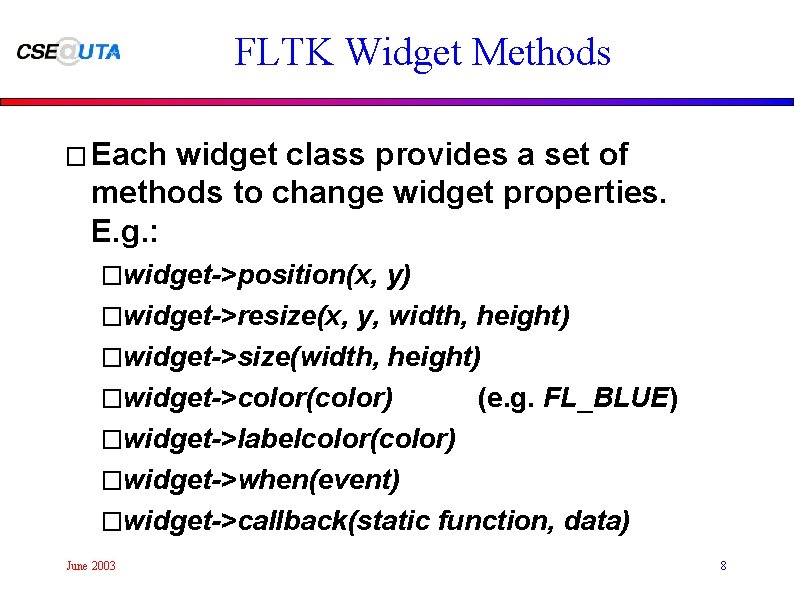
FLTK Widget Methods � Each widget class provides a set of methods to change widget properties. E. g. : �widget->position(x, y) �widget->resize(x, y, width, height) �widget->size(width, height) �widget->color(color) (e. g. FL_BLUE) �widget->labelcolor(color) �widget->when(event) �widget->callback(static function, data) June 2003 8
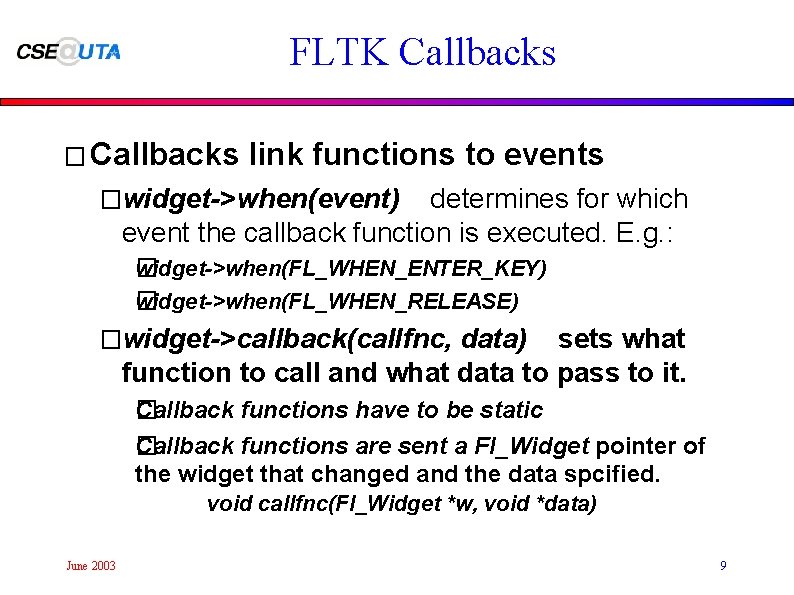
FLTK Callbacks � Callbacks link functions to events �widget->when(event) determines for which event the callback function is executed. E. g. : �idget->when(FL_WHEN_ENTER_KEY) w �idget->when(FL_WHEN_RELEASE) w �widget->callback(callfnc, data) sets what function to call and what data to pass to it. �allback functions have to be static C Callback functions are sent a Fl_Widget pointer of � the widget that changed and the data spcified. void callfnc(Fl_Widget *w, void *data) June 2003 9
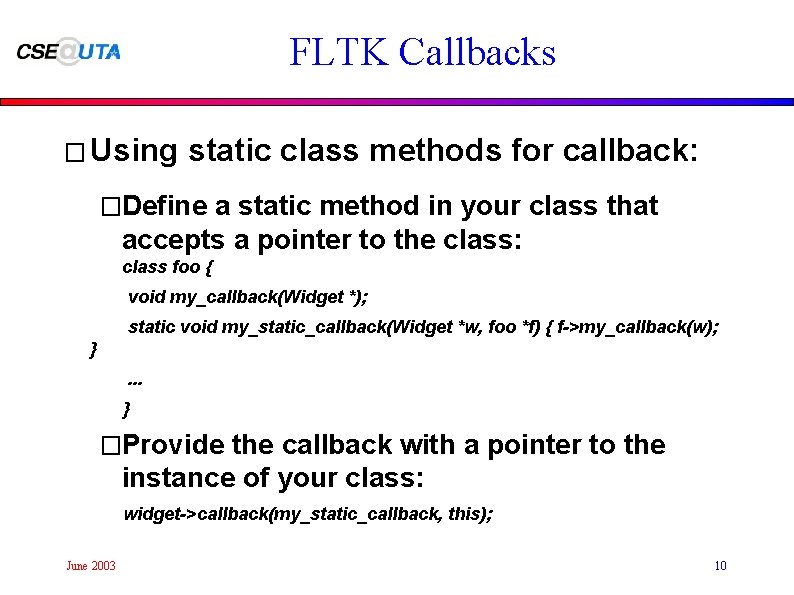
FLTK Callbacks � Using static class methods for callback: �Define a static method in your class that accepts a pointer to the class: class foo { void my_callback(Widget *); static void my_static_callback(Widget *w, foo *f) { f->my_callback(w); }. . . } �Provide the callback with a pointer to the instance of your class: widget->callback(my_static_callback, this); June 2003 10
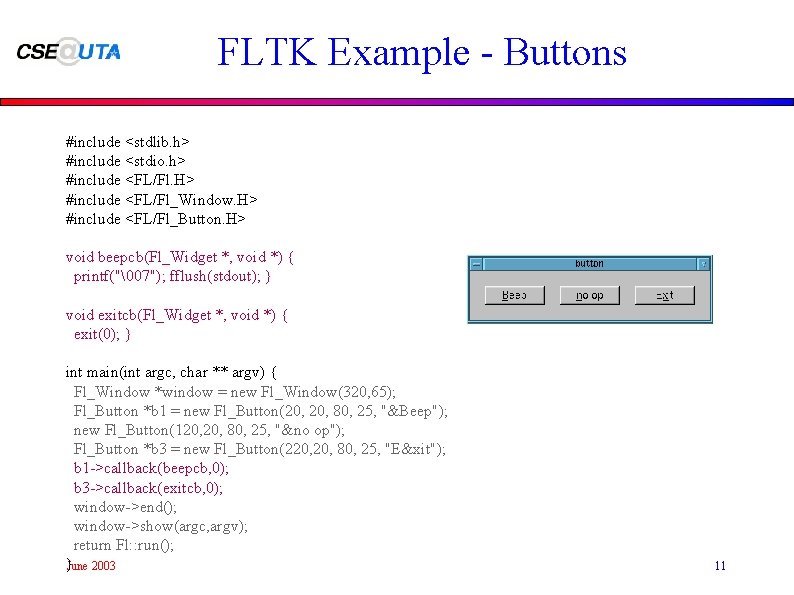
FLTK Example - Buttons #include <stdlib. h> #include <stdio. h> #include <FL/Fl. H> #include <FL/Fl_Window. H> #include <FL/Fl_Button. H> void beepcb(Fl_Widget *, void *) { printf("