First Data Structure Definition A data structure is
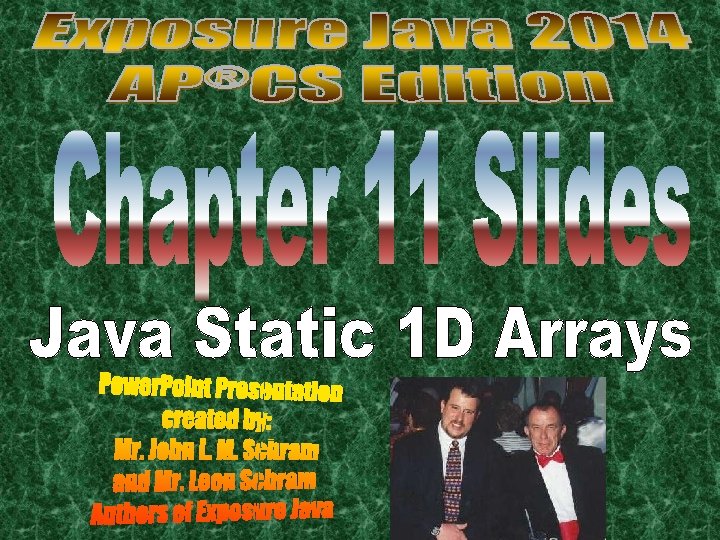
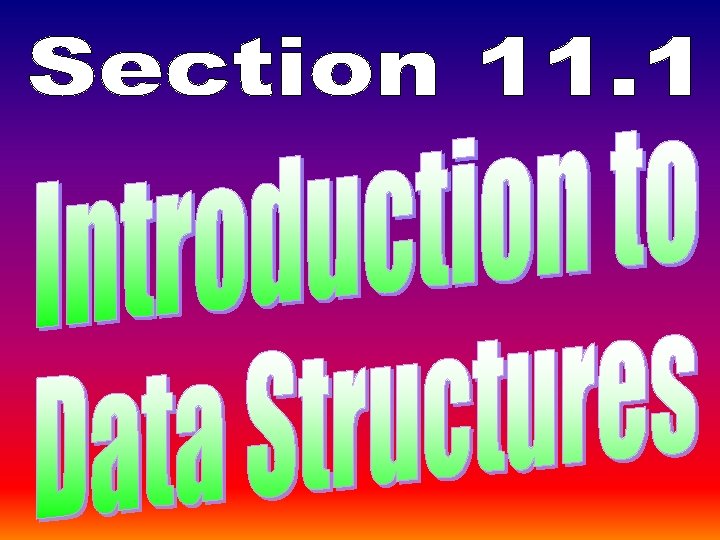
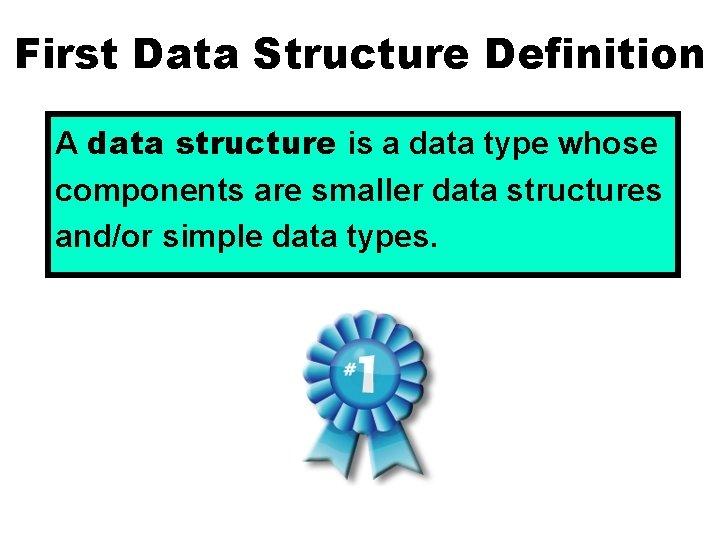
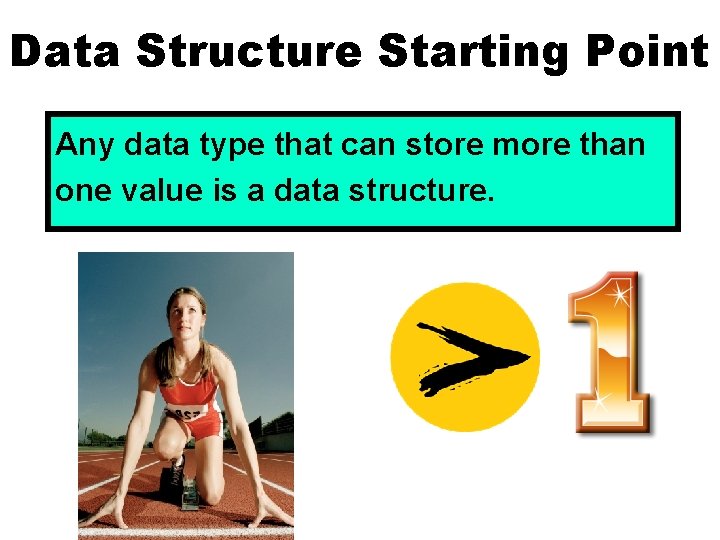
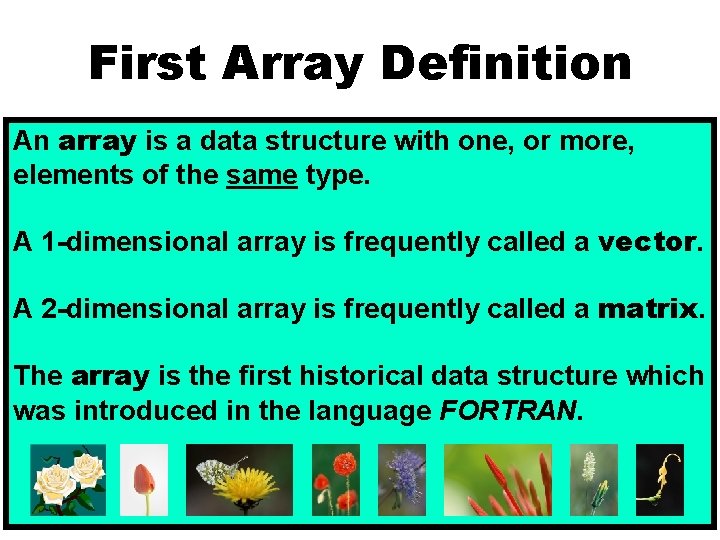
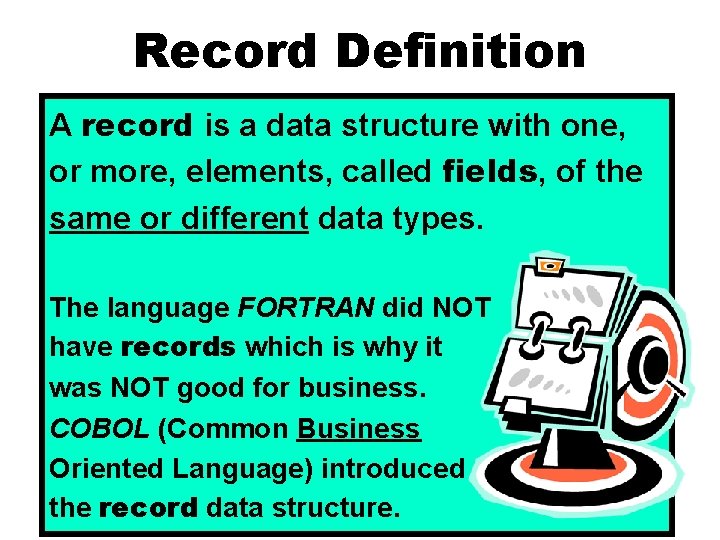
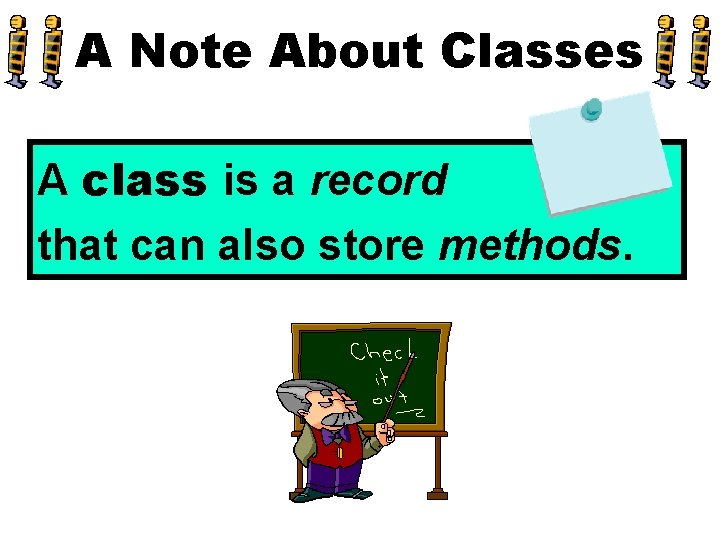
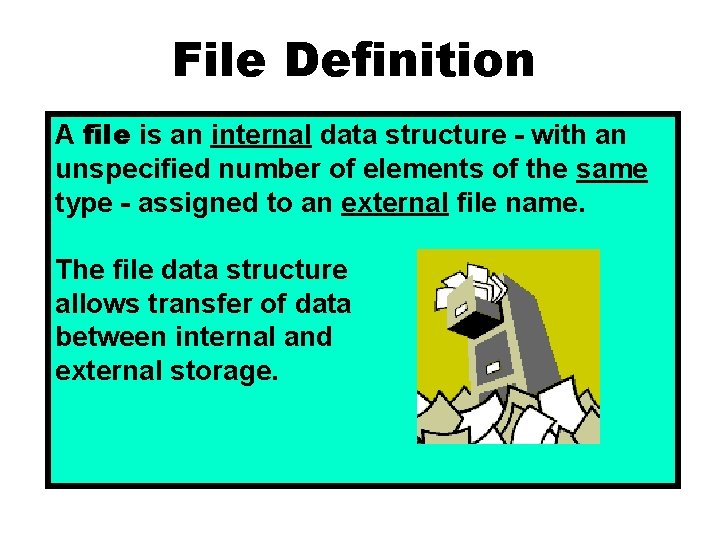
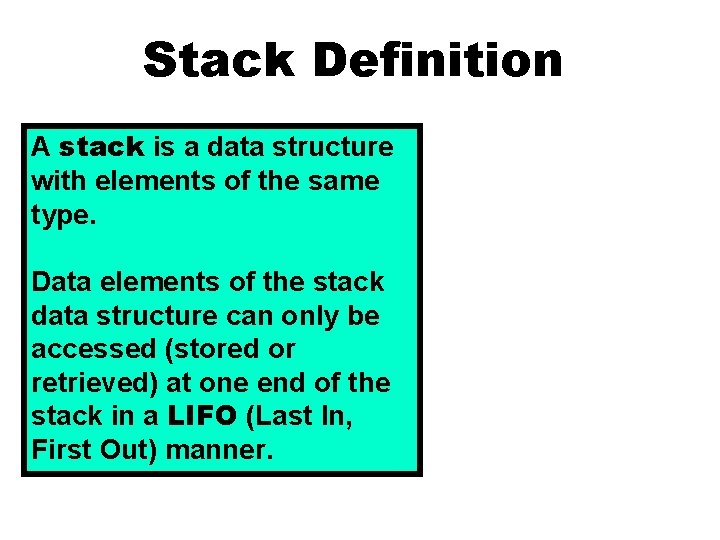
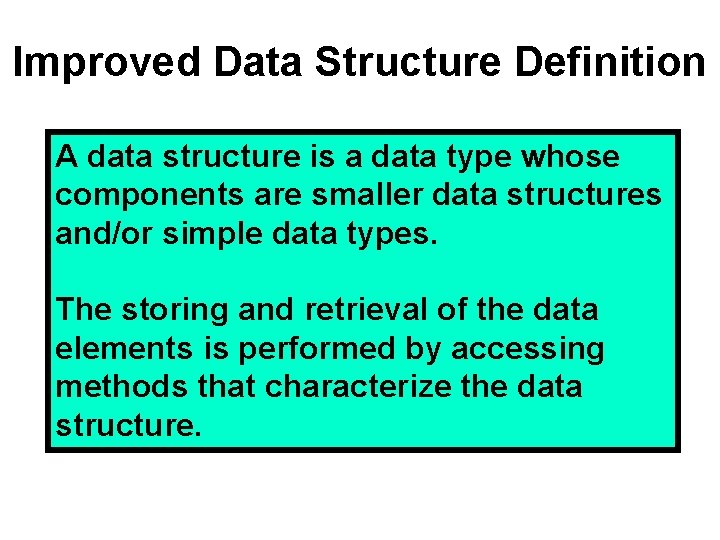
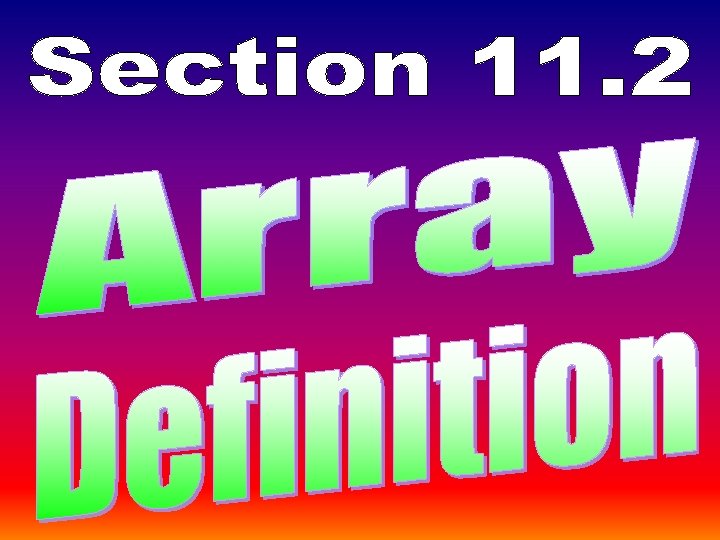
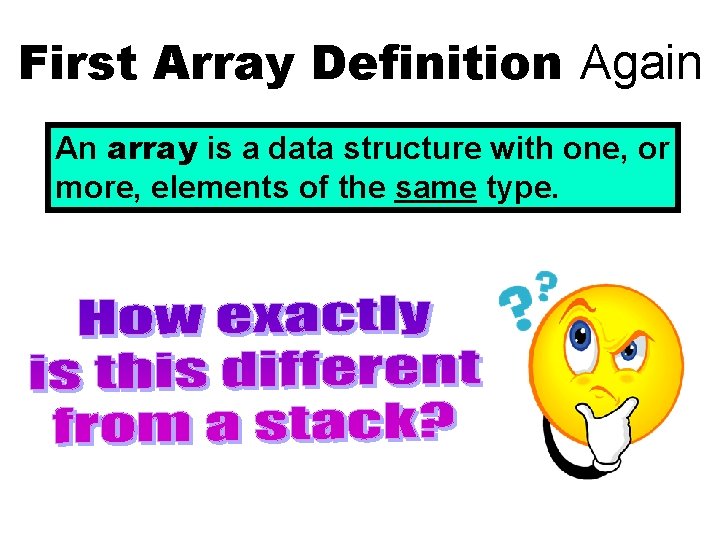
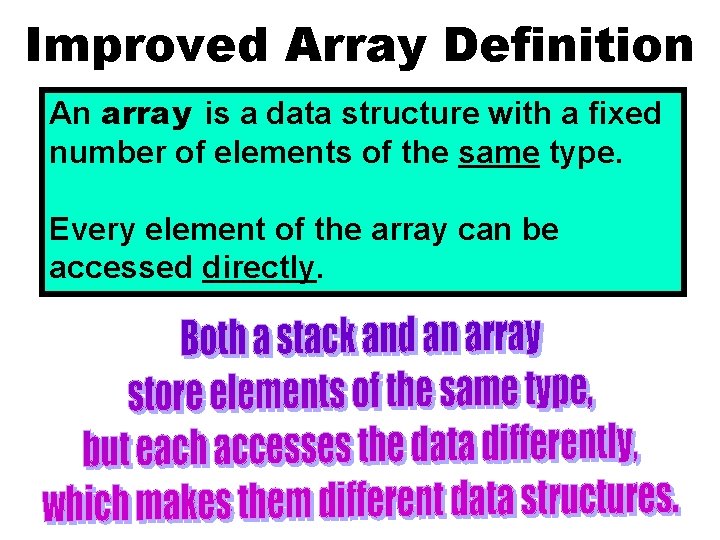
![Array Example [16] Ingrid [17] Darlene [18] Gene [19] Sean [20] Stephan ie [15] Array Example [16] Ingrid [17] Darlene [18] Gene [19] Sean [20] Stephan ie [15]](https://slidetodoc.com/presentation_image_h2/cdfc99b78f29645cb18e7408585d1819/image-14.jpg)
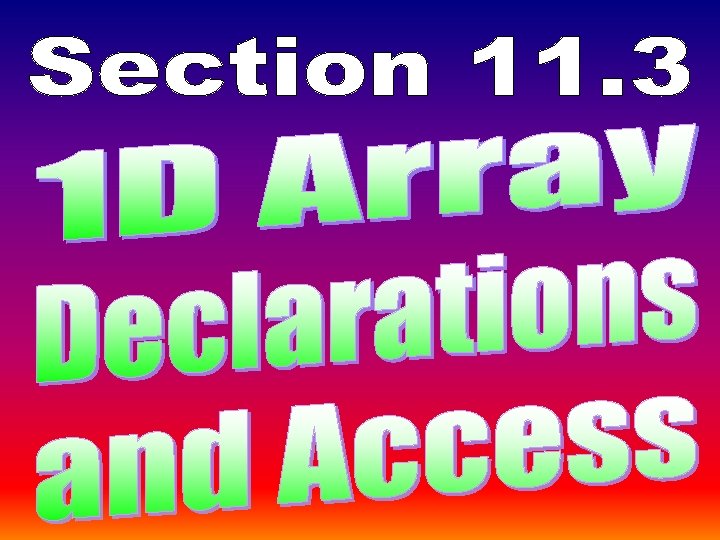
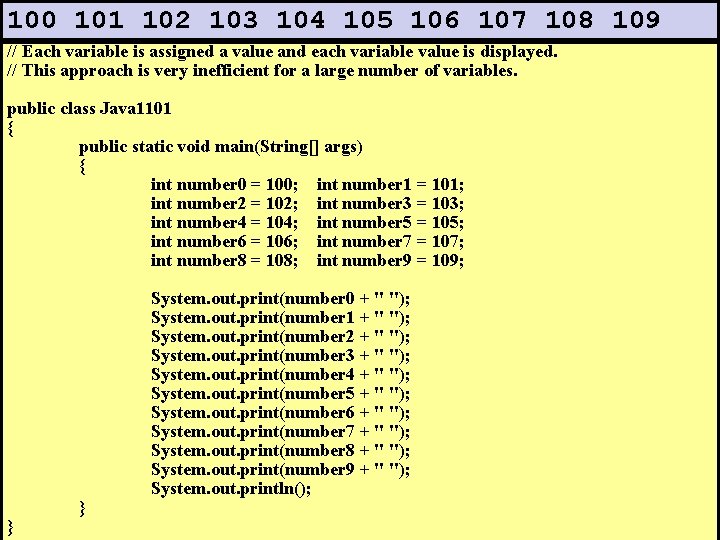
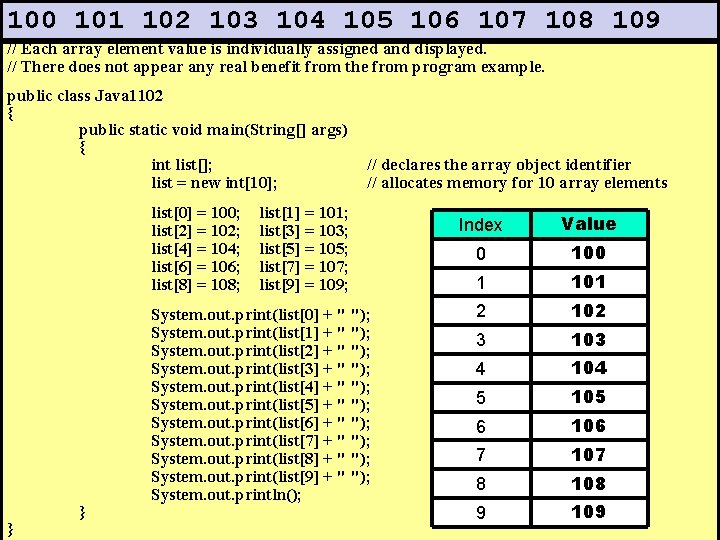
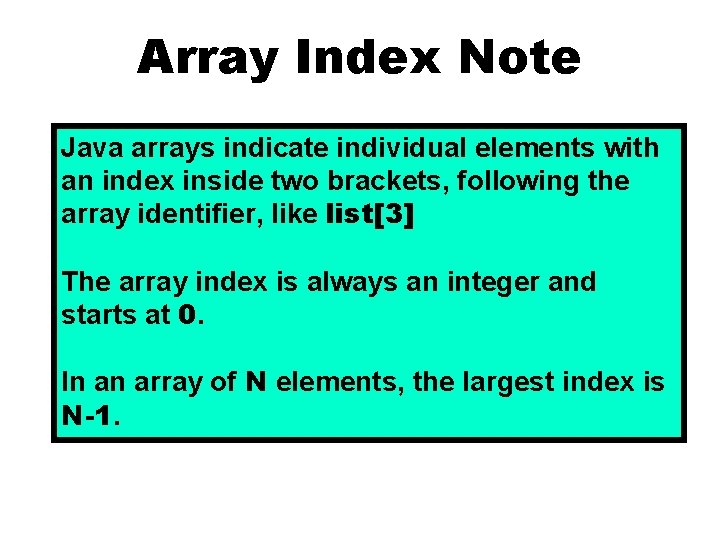
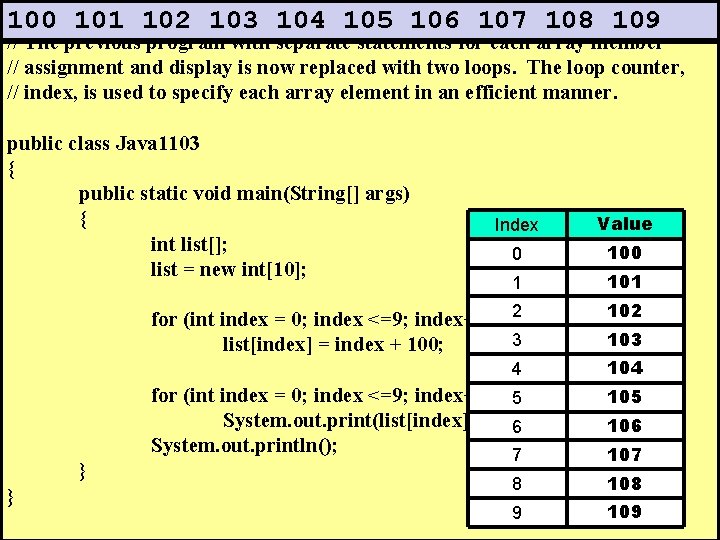
![Defining Static Arrays int list[]; // declares the array list identifier list = new Defining Static Arrays int list[]; // declares the array list identifier list = new](https://slidetodoc.com/presentation_image_h2/cdfc99b78f29645cb18e7408585d1819/image-20.jpg)
![Defining Static Arrays Preferred Method int list[ ] = new int[10]; char names[ ] Defining Static Arrays Preferred Method int list[ ] = new int[10]; char names[ ]](https://slidetodoc.com/presentation_image_h2/cdfc99b78f29645cb18e7408585d1819/image-21.jpg)
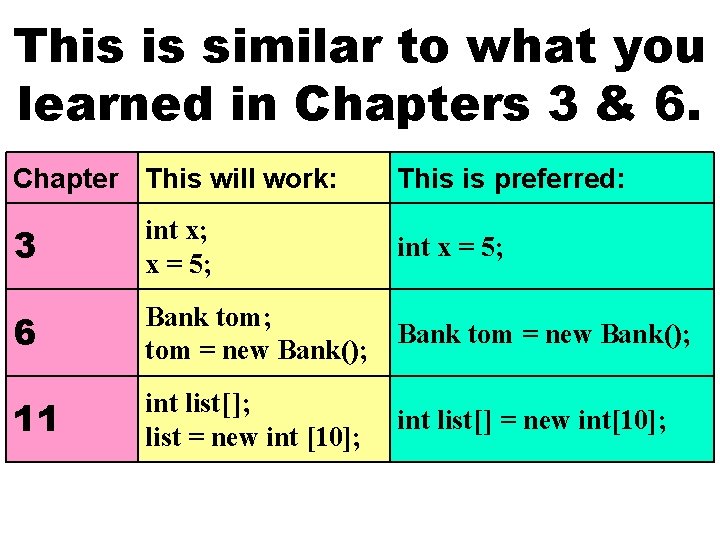
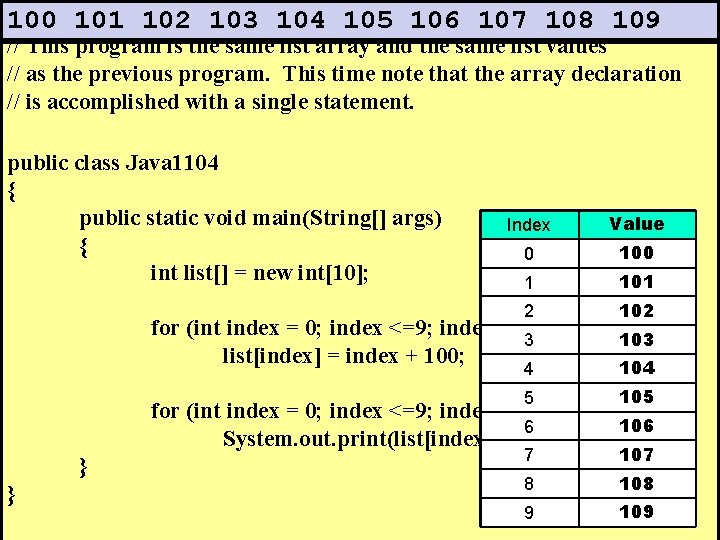
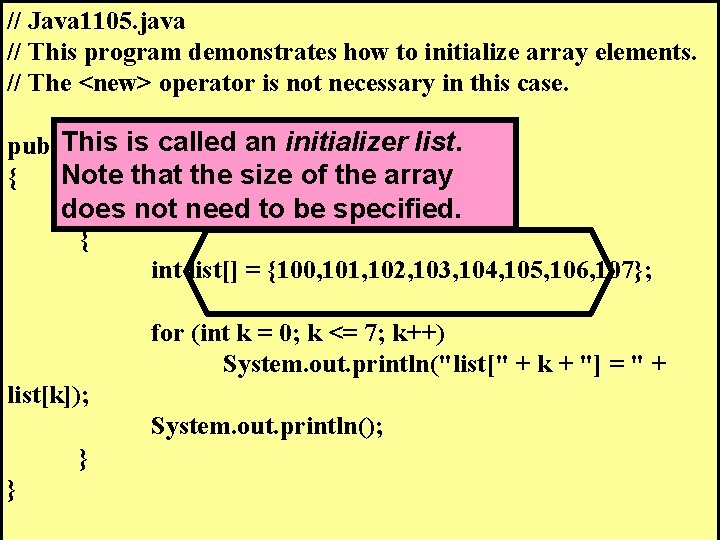
![list[0] = 100 // Java 1105. java list[1] = 101 // This program demonstrates list[0] = 100 // Java 1105. java list[1] = 101 // This program demonstrates](https://slidetodoc.com/presentation_image_h2/cdfc99b78f29645cb18e7408585d1819/image-25.jpg)
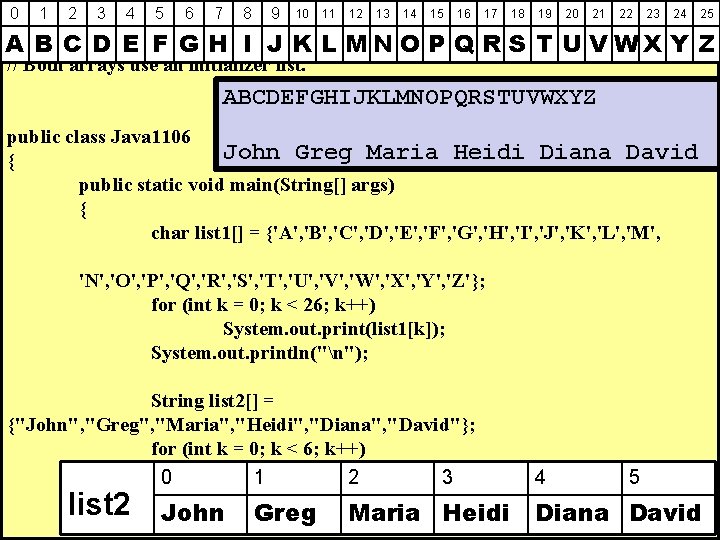
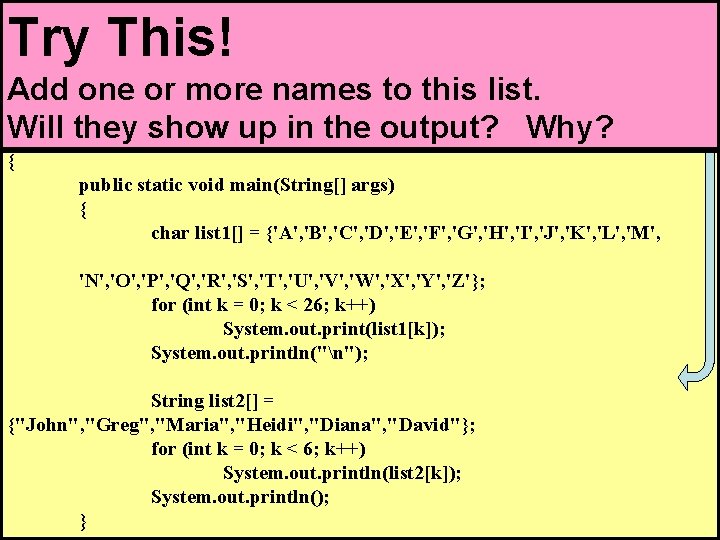
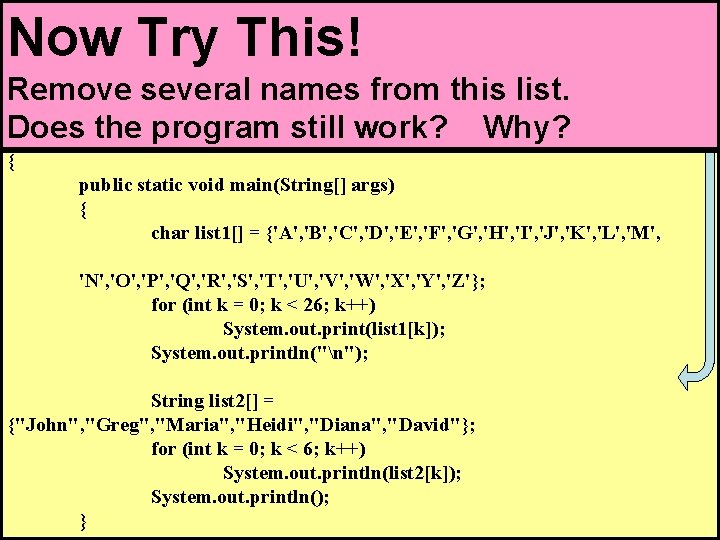
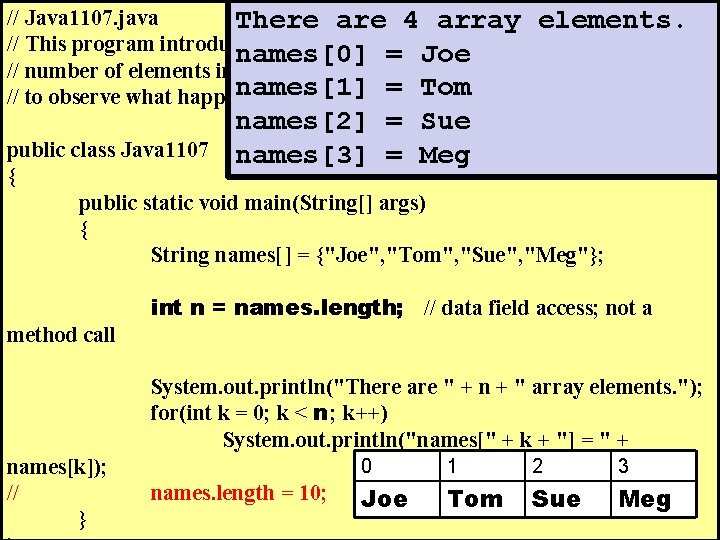
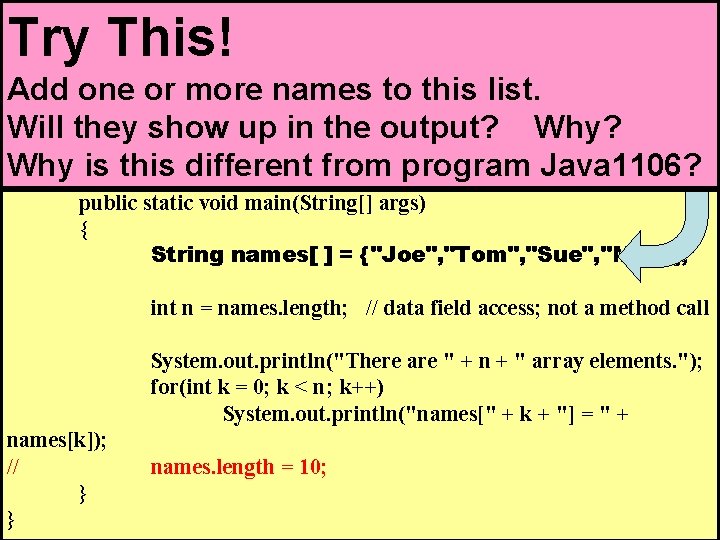
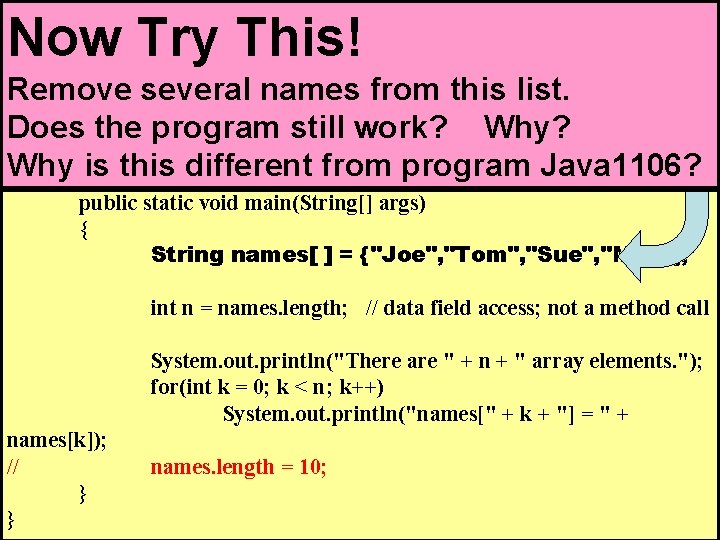
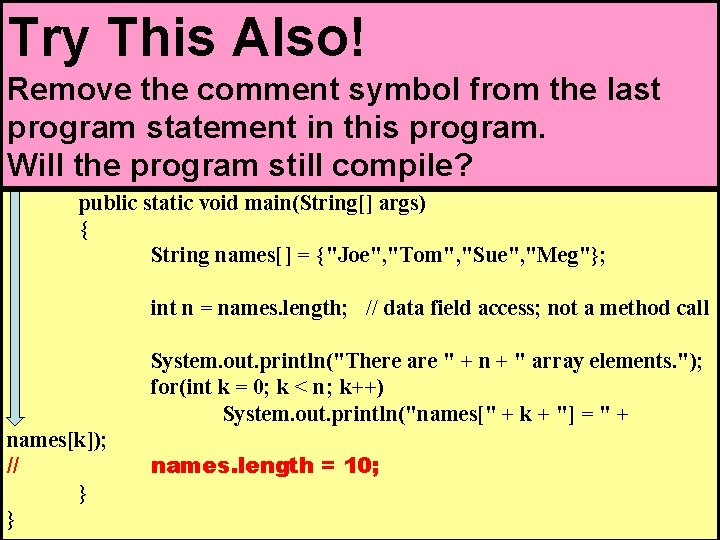
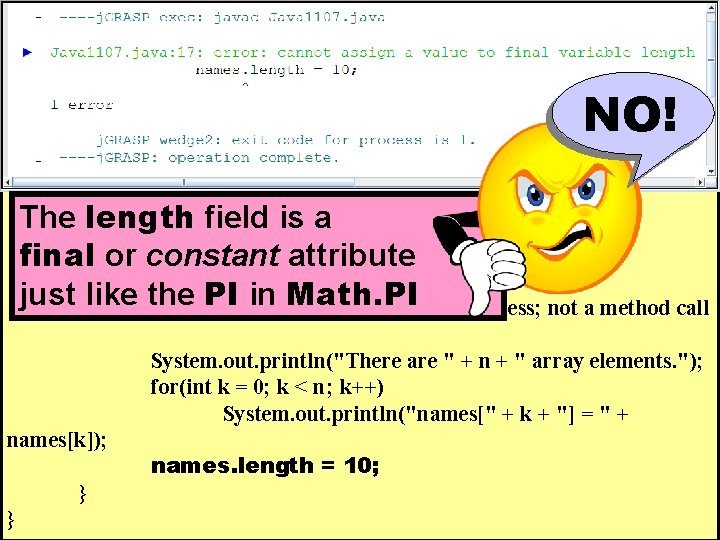
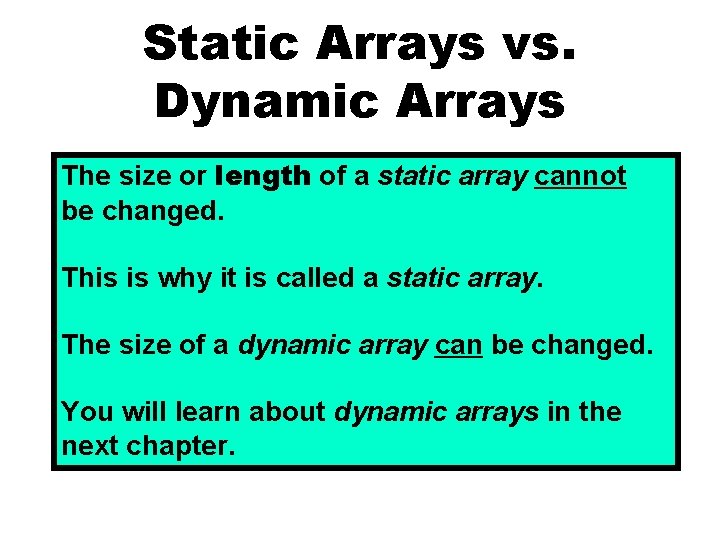
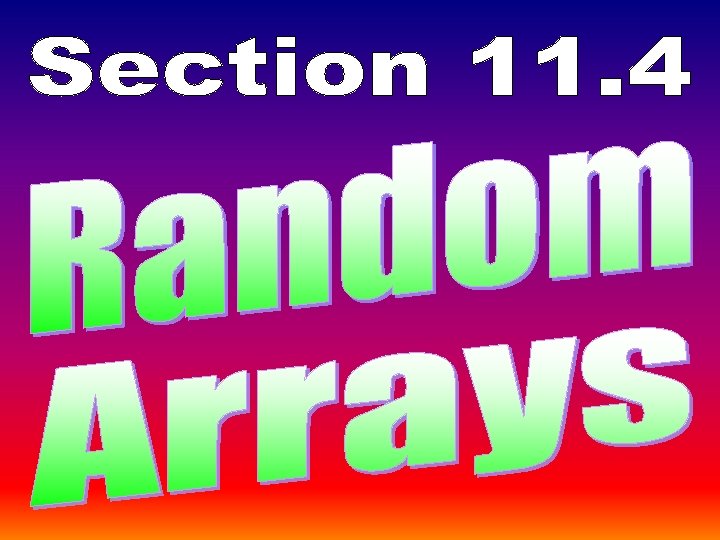
![list[0] = 244 // Java 1108. java list[1] = 594 // This program fills list[0] = 244 // Java 1108. java list[1] = 594 // This program fills](https://slidetodoc.com/presentation_image_h2/cdfc99b78f29645cb18e7408585d1819/image-36.jpg)
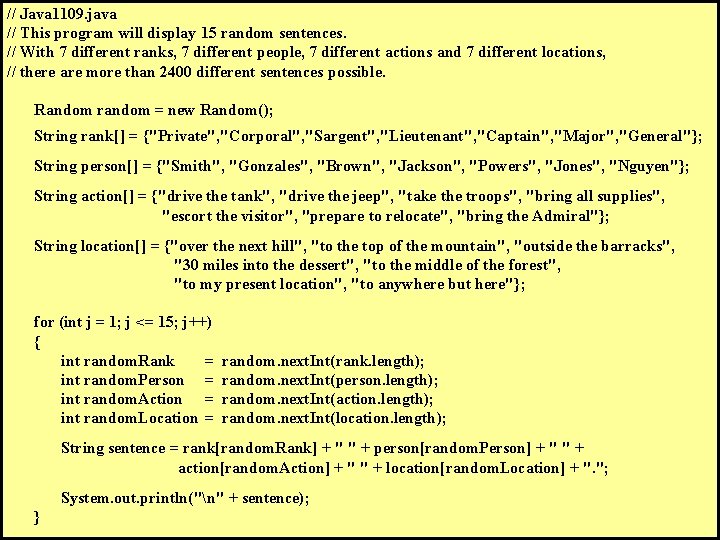
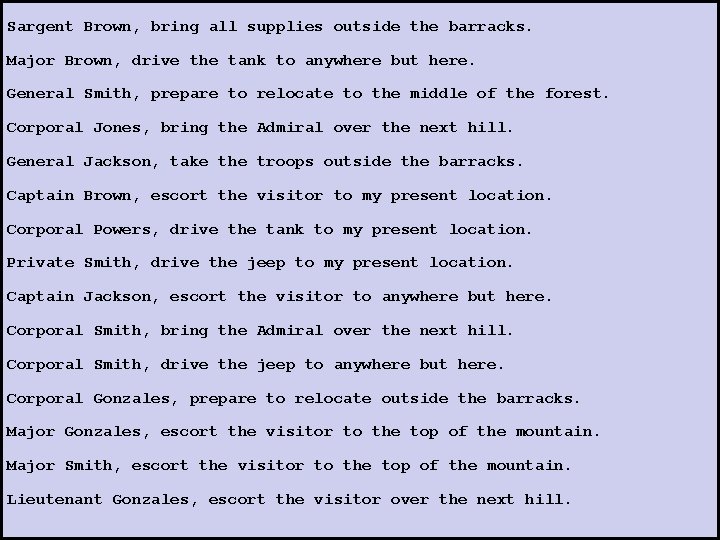
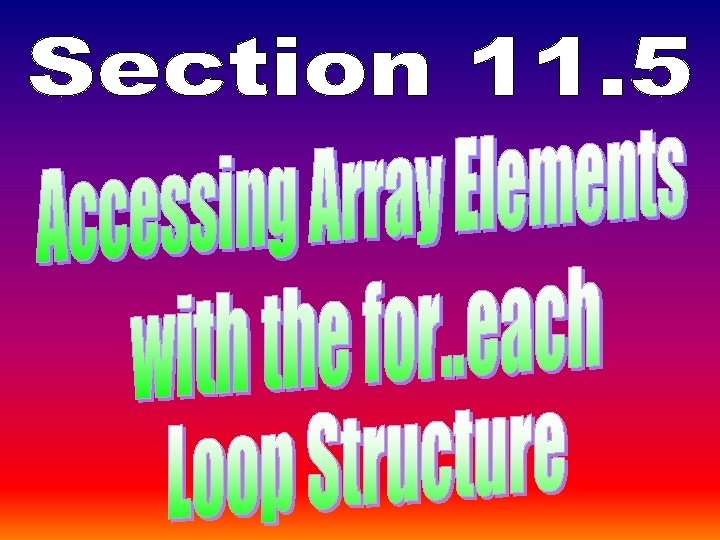
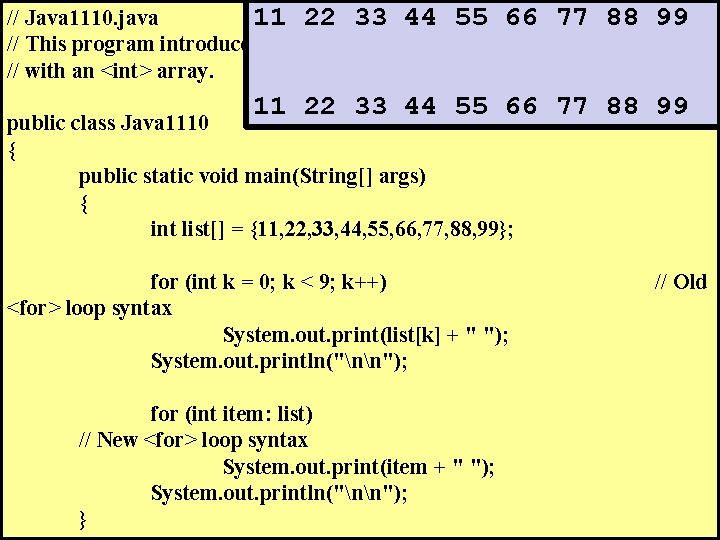
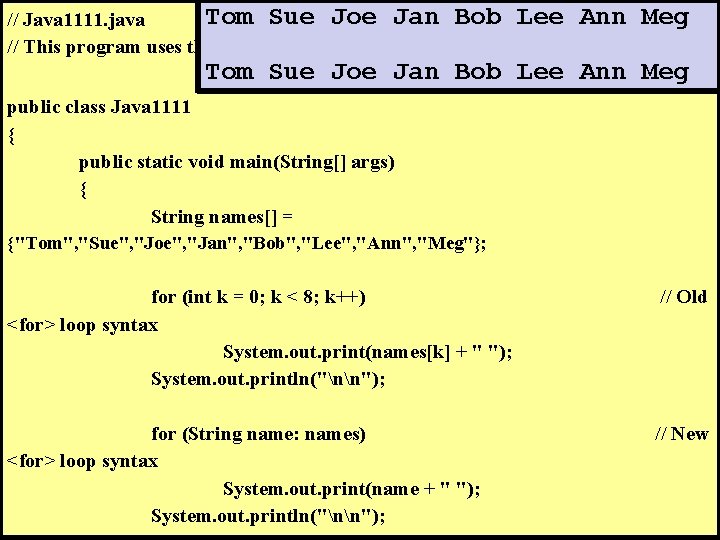
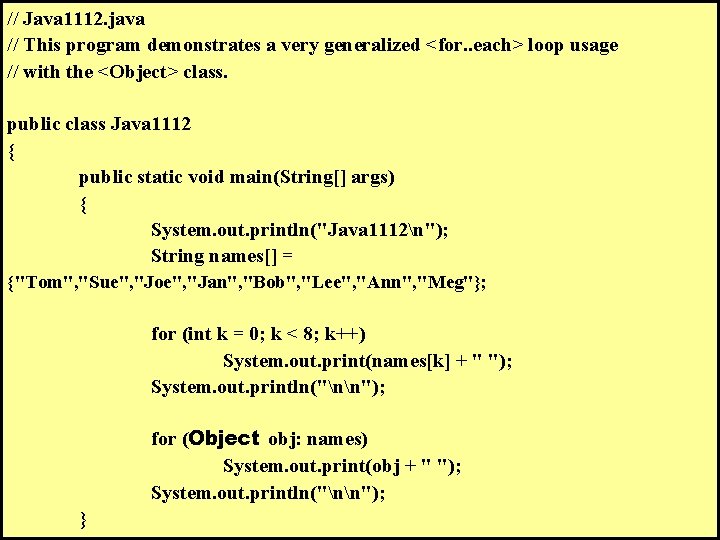
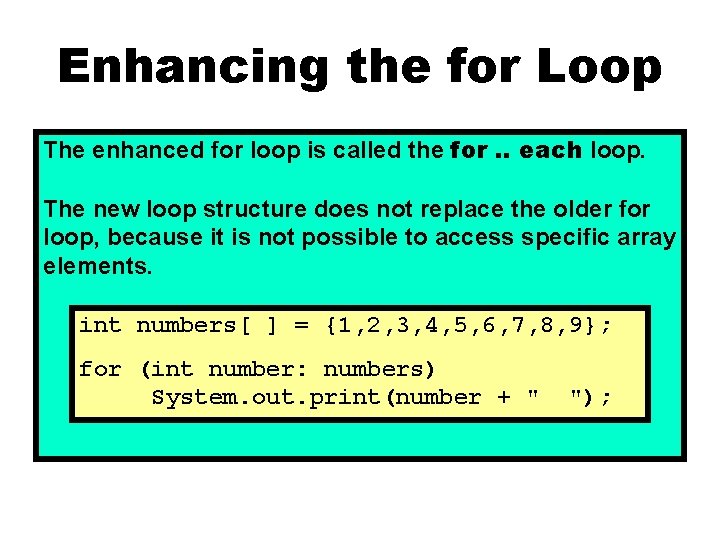
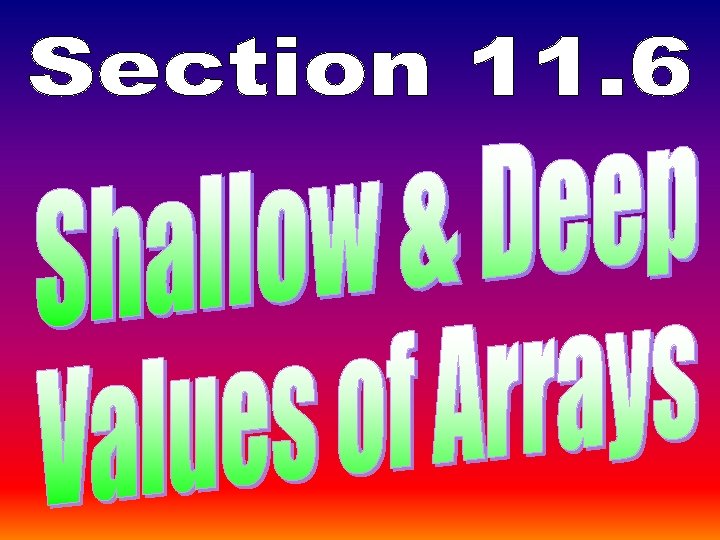
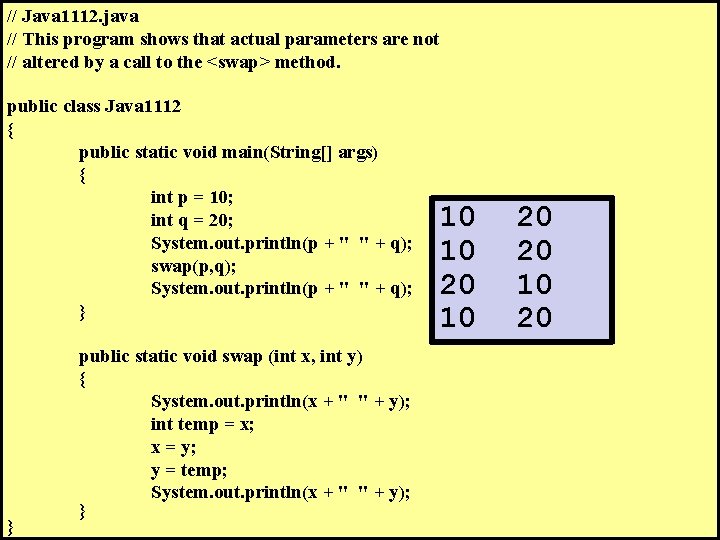
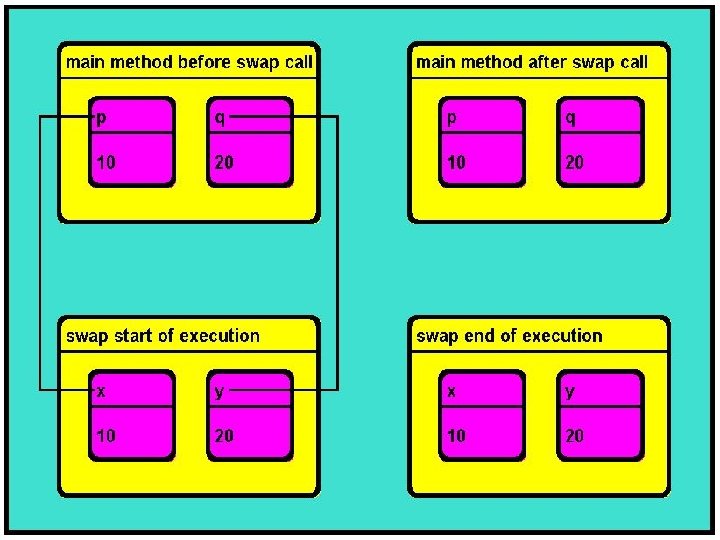
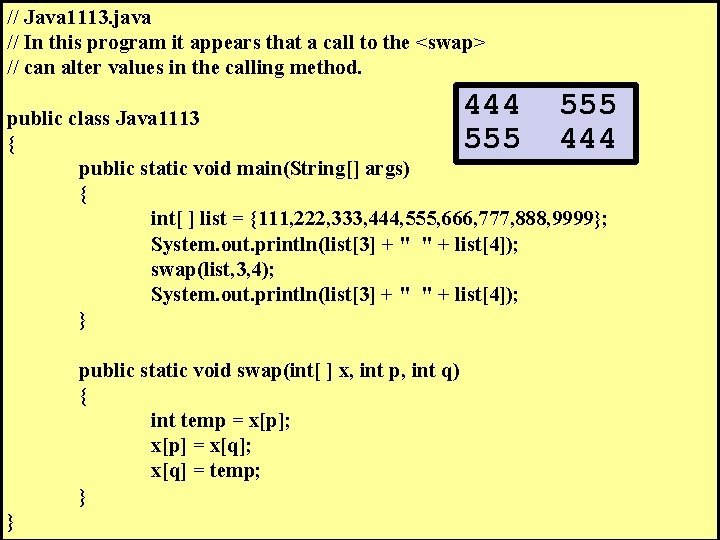
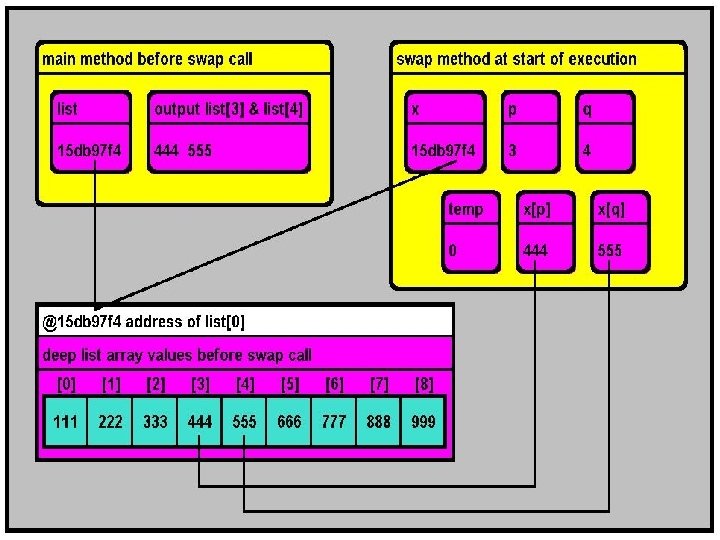
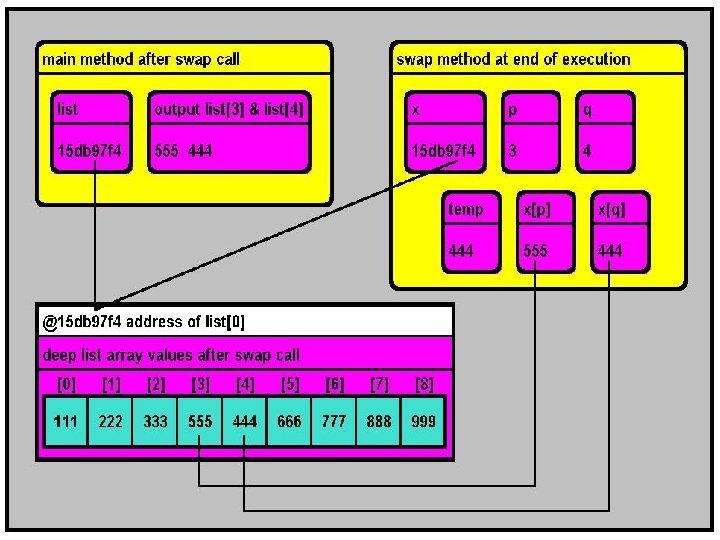
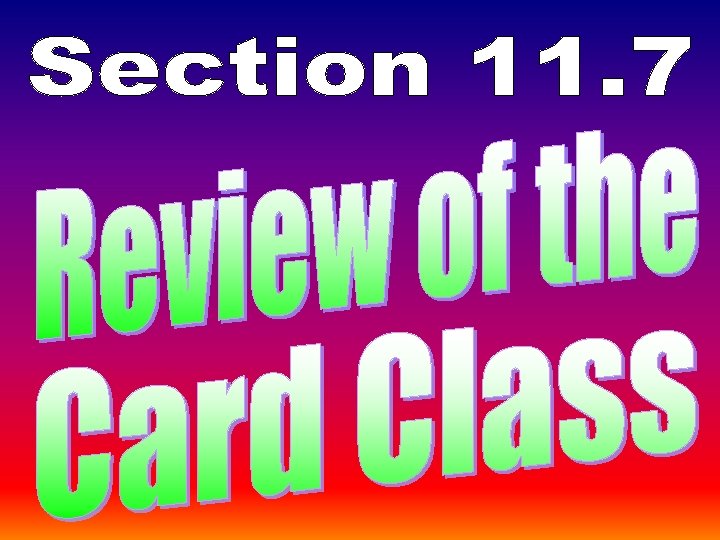
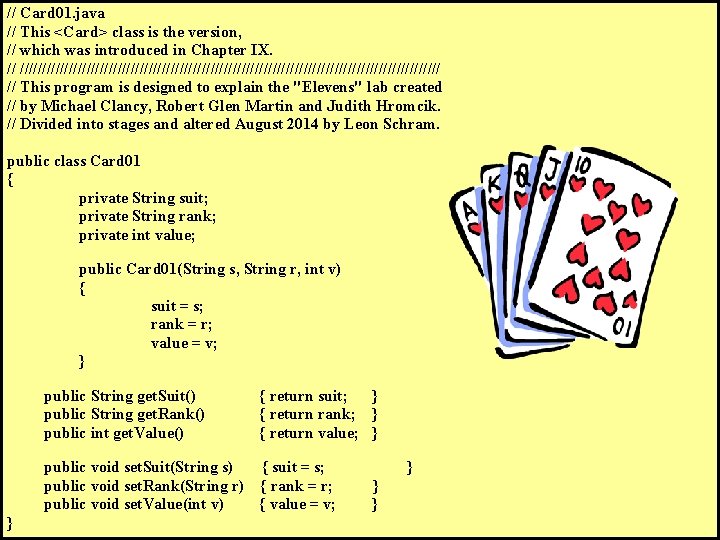
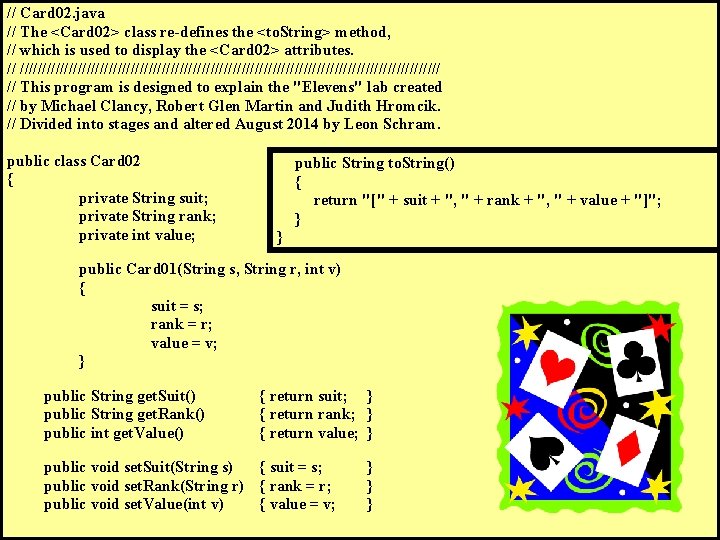
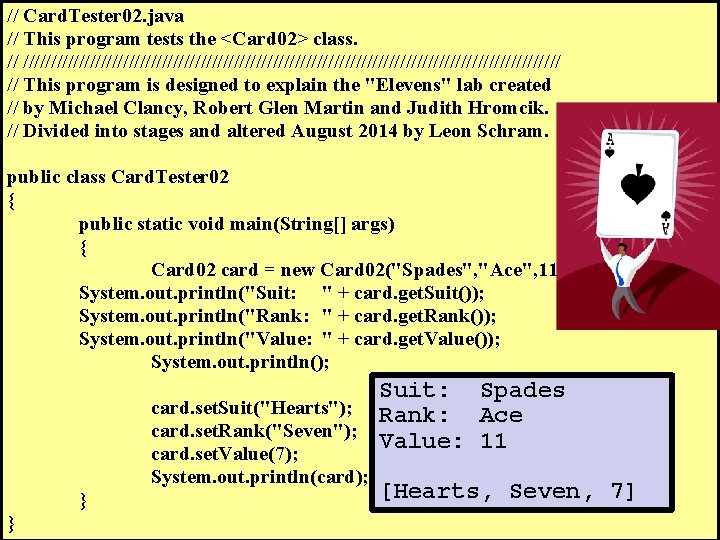
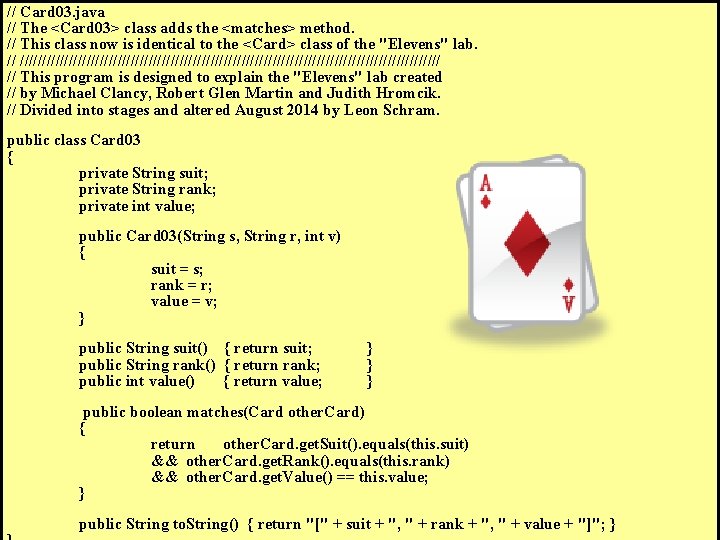
![// Card. Tester 03. java [Spades, Ace, 11] // This program tests the <Card // Card. Tester 03. java [Spades, Ace, 11] // This program tests the <Card](https://slidetodoc.com/presentation_image_h2/cdfc99b78f29645cb18e7408585d1819/image-55.jpg)
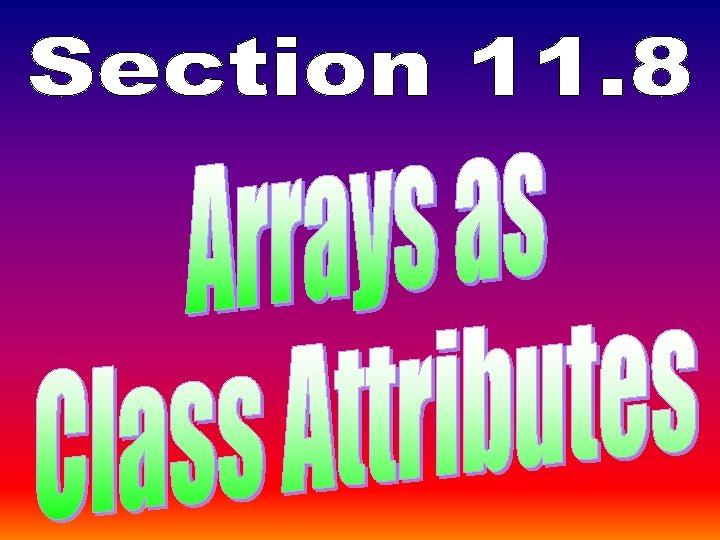
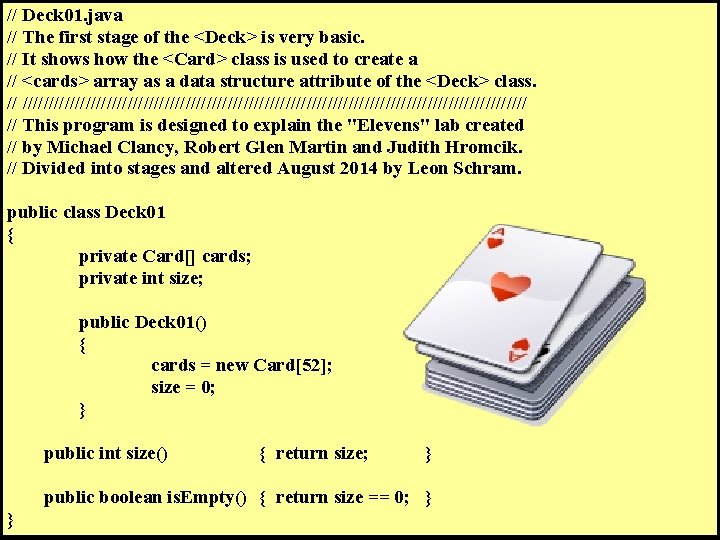
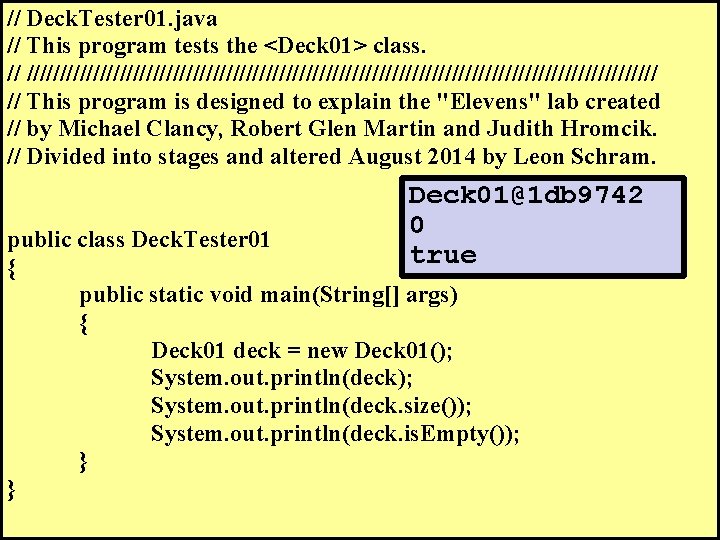
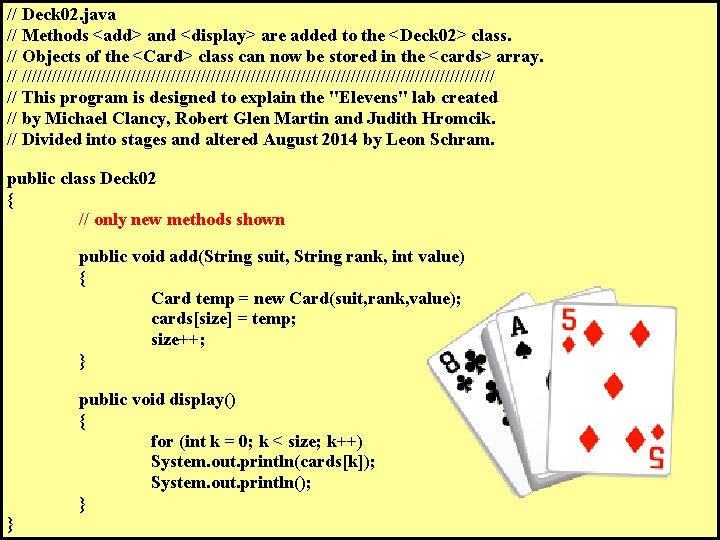
![// Deck. Tester 02. java [Clubs, Three, 3] // This program tests the <Deck // Deck. Tester 02. java [Clubs, Three, 3] // This program tests the <Deck](https://slidetodoc.com/presentation_image_h2/cdfc99b78f29645cb18e7408585d1819/image-60.jpg)
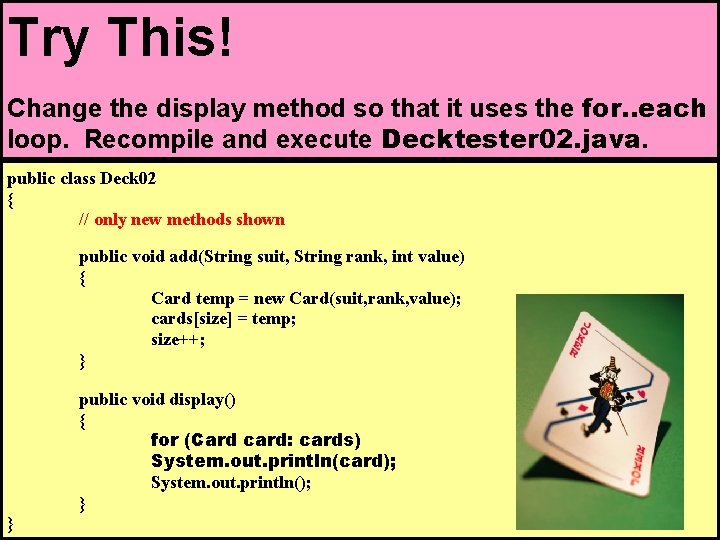
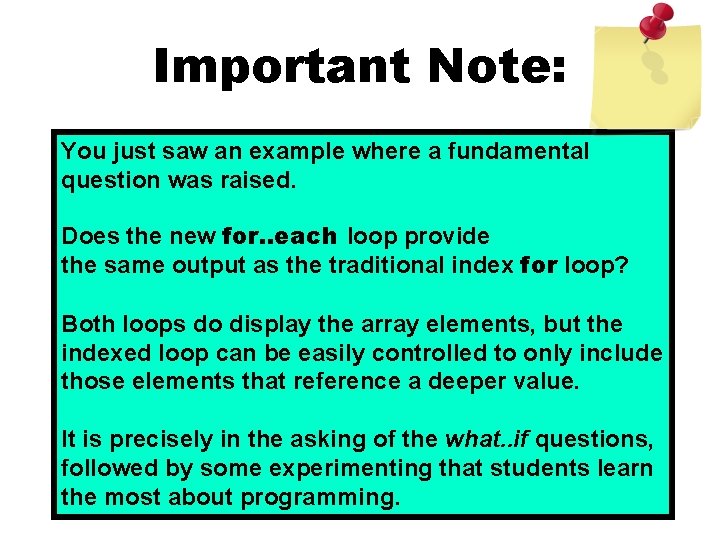
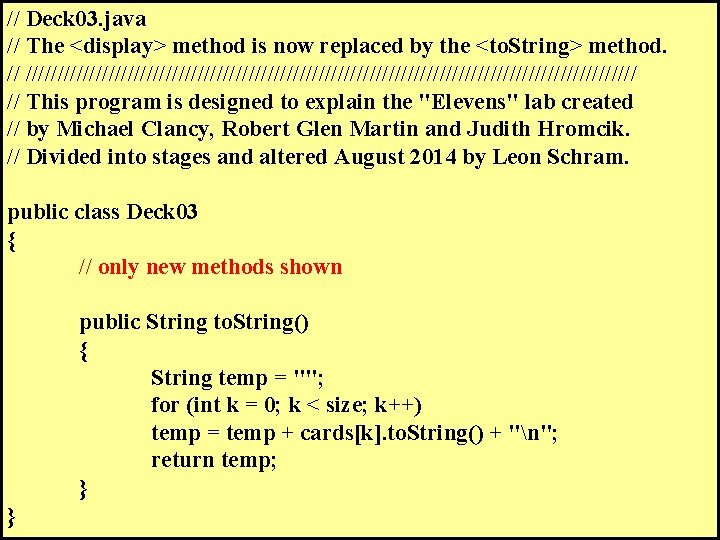
![// Deck. Tester 03. java [Clubs, Three, 3] // This program tests the <Deck // Deck. Tester 03. java [Clubs, Three, 3] // This program tests the <Deck](https://slidetodoc.com/presentation_image_h2/cdfc99b78f29645cb18e7408585d1819/image-64.jpg)
- Slides: 64
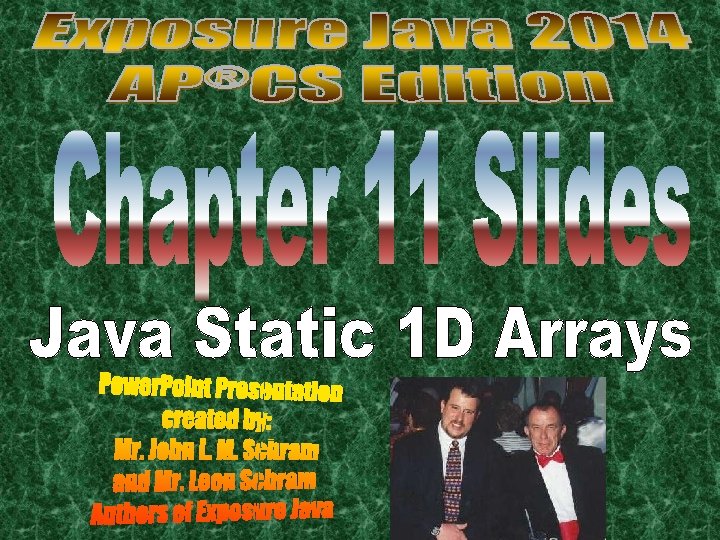
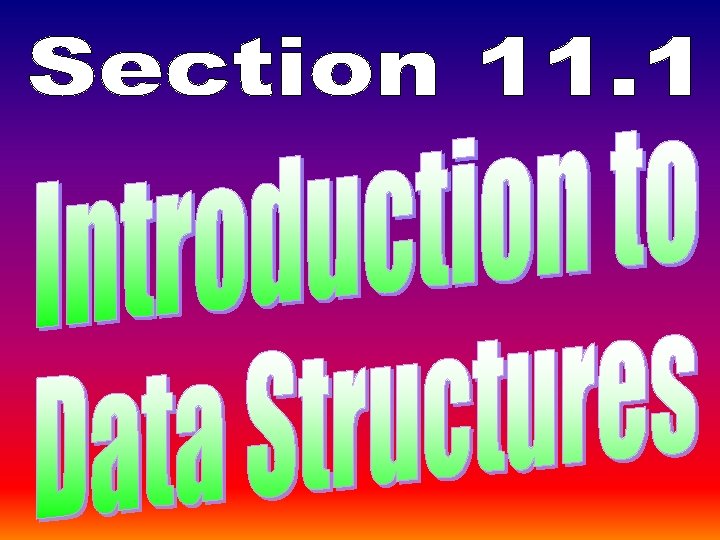
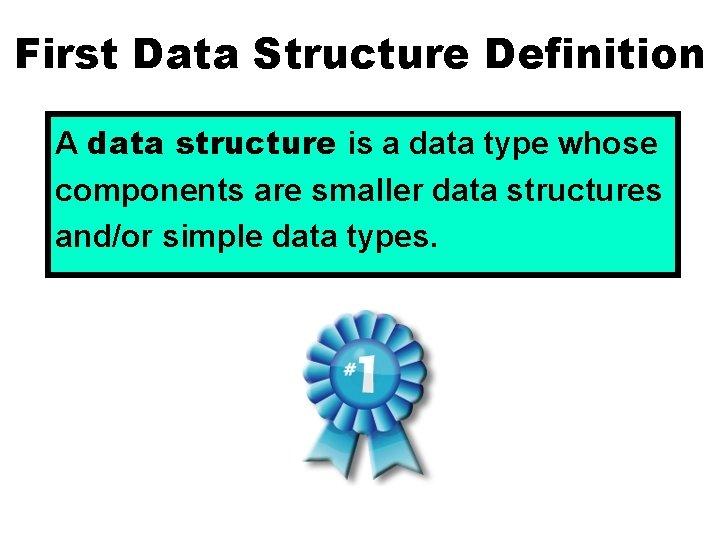
First Data Structure Definition A data structure is a data type whose components are smaller data structures and/or simple data types.
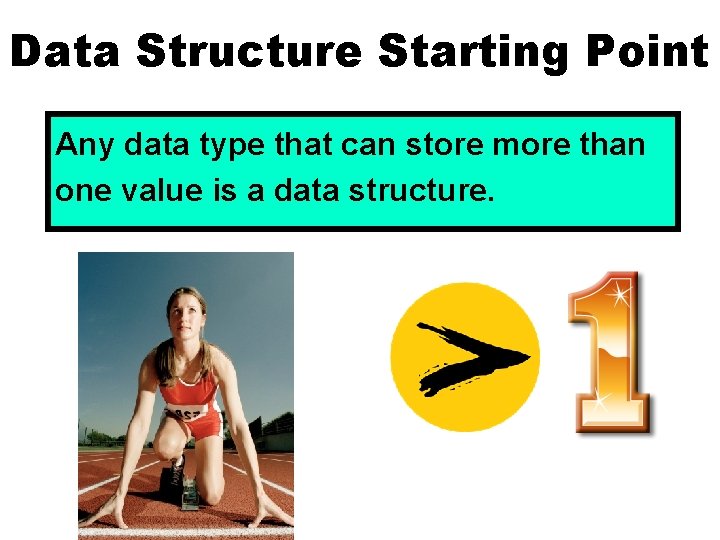
Data Structure Starting Point Any data type that can store more than one value is a data structure.
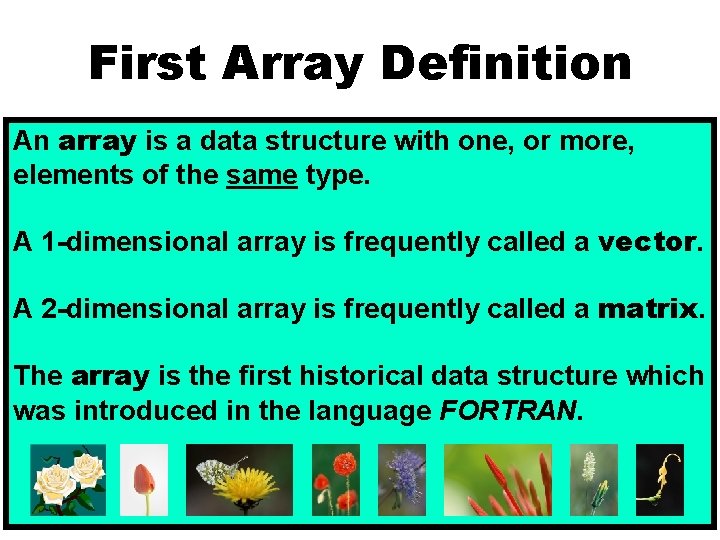
First Array Definition An array is a data structure with one, or more, elements of the same type. A 1 -dimensional array is frequently called a vector. A 2 -dimensional array is frequently called a matrix. The array is the first historical data structure which was introduced in the language FORTRAN.
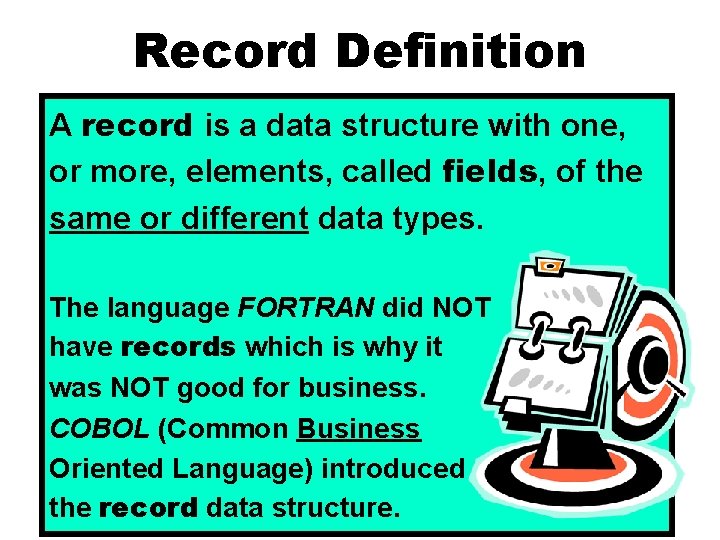
Record Definition A record is a data structure with one, or more, elements, called fields, of the same or different data types. The language FORTRAN did NOT have records which is why it was NOT good for business. COBOL (Common Business Oriented Language) introduced the record data structure.
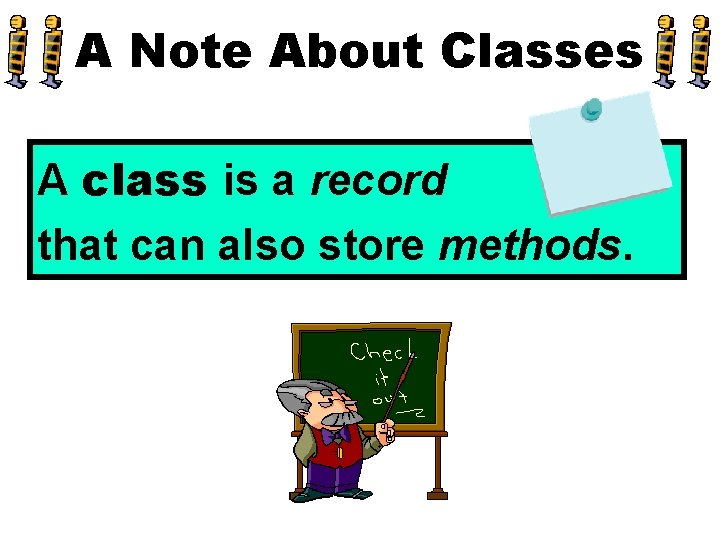
A Note About Classes A class is a record that can also store methods.
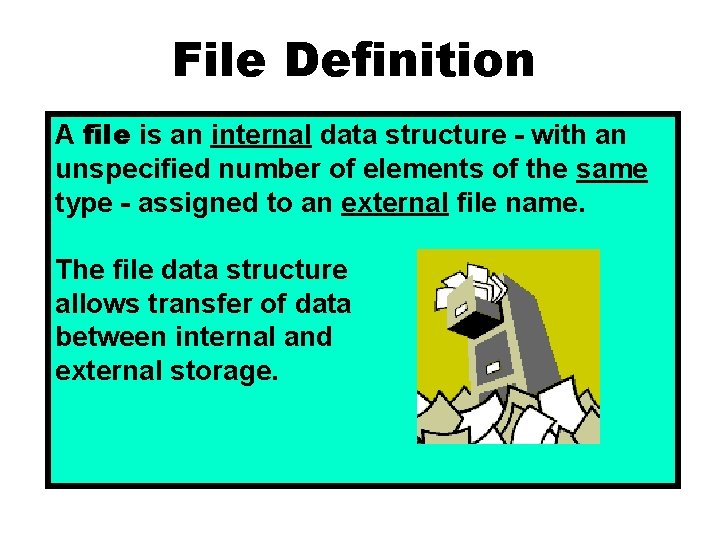
File Definition A file is an internal data structure - with an unspecified number of elements of the same type - assigned to an external file name. The file data structure allows transfer of data between internal and external storage.
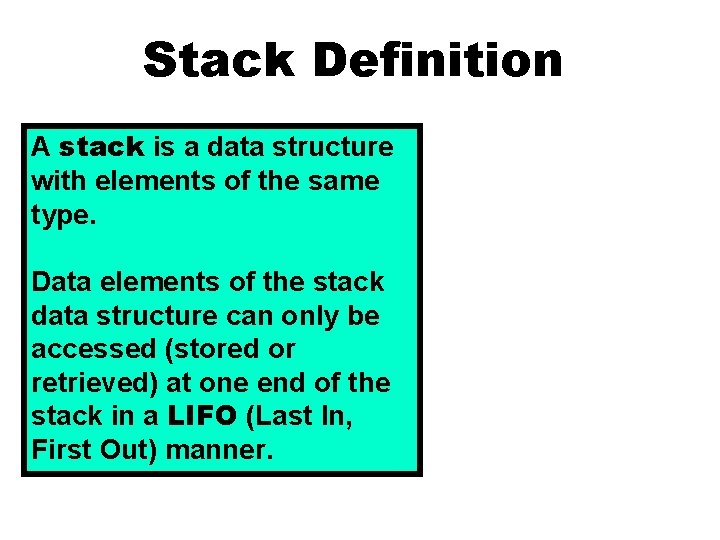
Stack Definition A stack is a data structure with elements of the same type. Data elements of the stack data structure can only be accessed (stored or retrieved) at one end of the stack in a LIFO (Last In, First Out) manner.
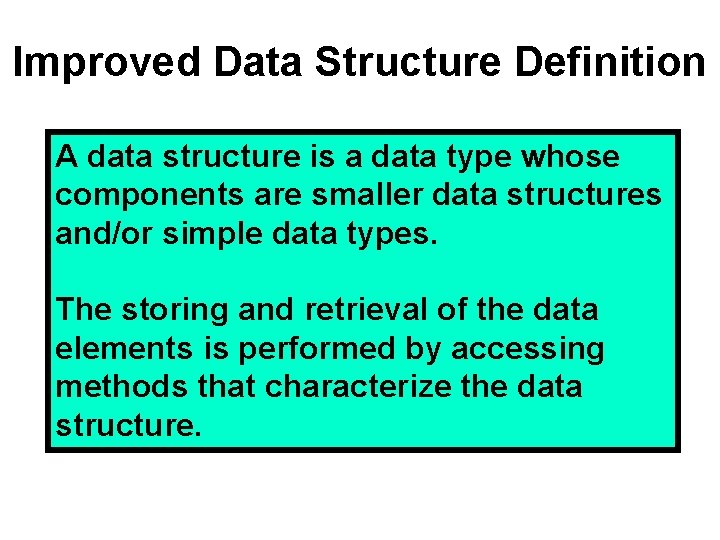
Improved Data Structure Definition A data structure is a data type whose components are smaller data structures and/or simple data types. The storing and retrieval of the data elements is performed by accessing methods that characterize the data structure.
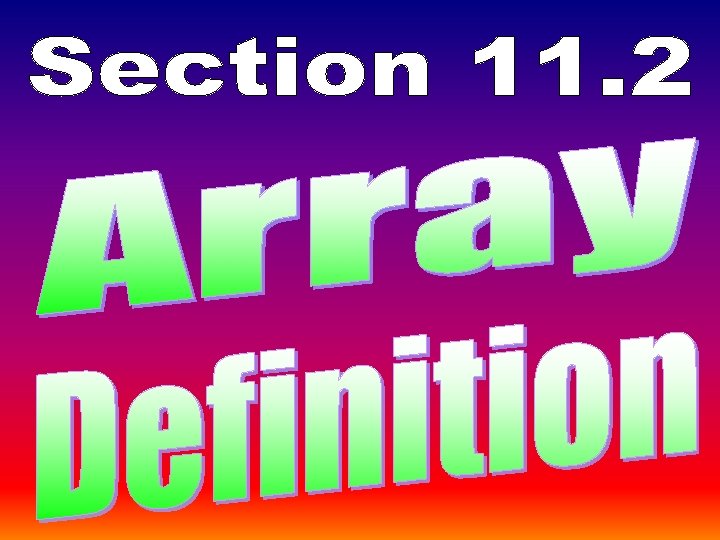
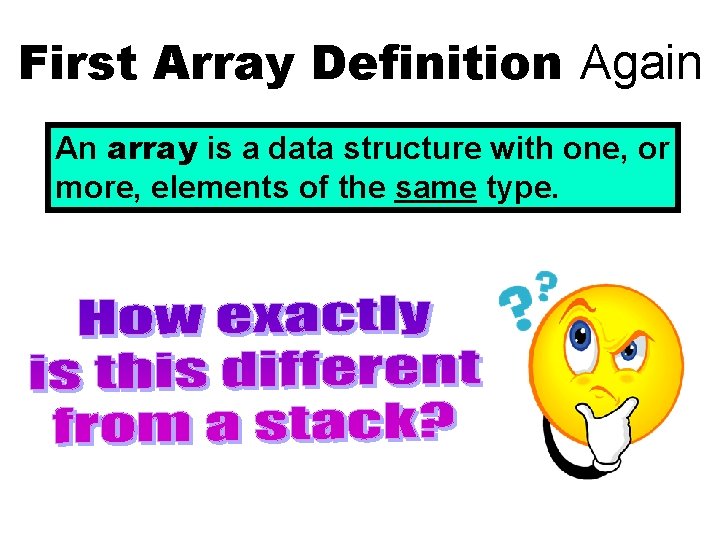
First Array Definition Again An array is a data structure with one, or more, elements of the same type.
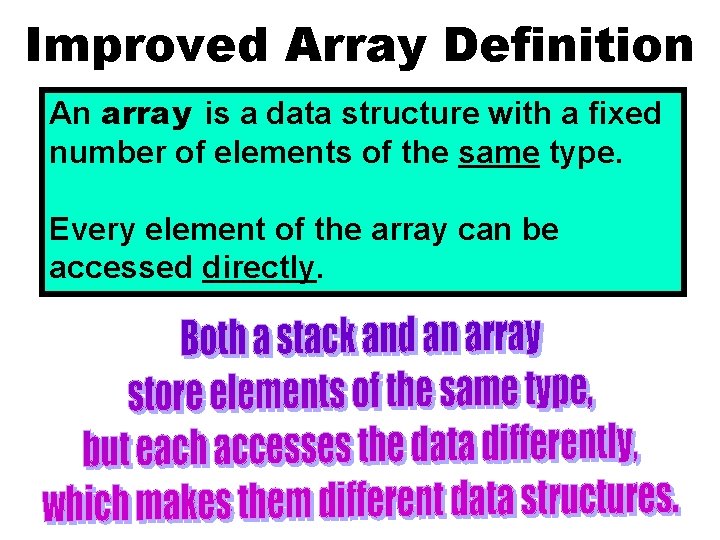
Improved Array Definition An array is a data structure with a fixed number of elements of the same type. Every element of the array can be accessed directly.
![Array Example 16 Ingrid 17 Darlene 18 Gene 19 Sean 20 Stephan ie 15 Array Example [16] Ingrid [17] Darlene [18] Gene [19] Sean [20] Stephan ie [15]](https://slidetodoc.com/presentation_image_h2/cdfc99b78f29645cb18e7408585d1819/image-14.jpg)
Array Example [16] Ingrid [17] Darlene [18] Gene [19] Sean [20] Stephan ie [15] Haley [11] Holly [12] Blake [13] [14] Michelle Remy [06] Diana [07] Jessica [08] David [09] [10] Anthony Alec [01] Isolde [02] John [03] Greg [04] Maria [05] Heidi
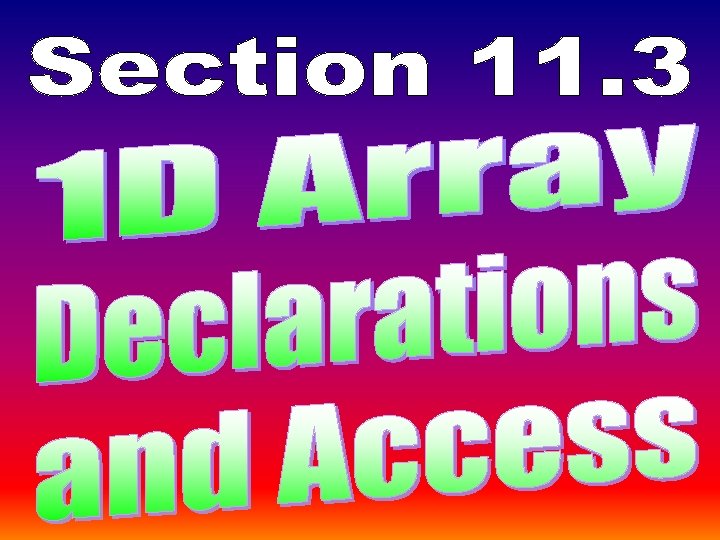
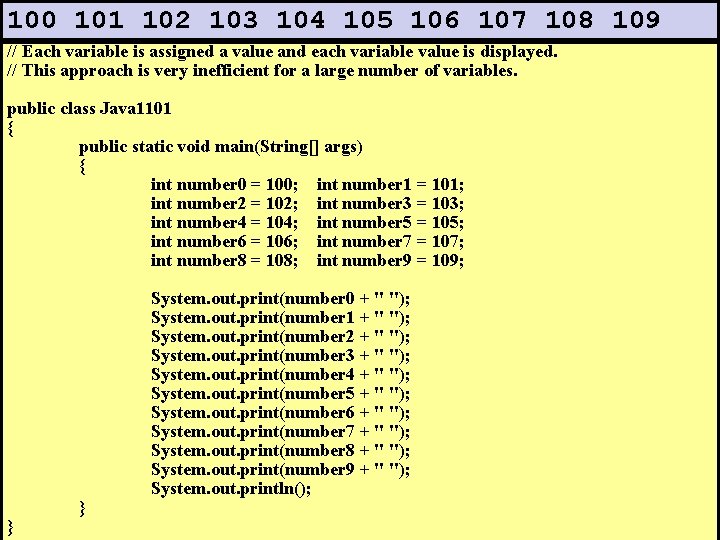
// Java 1101. java 100 101 102 103 104 105 106 107 108 // This program declares 10 different int variables. // Each variable is assigned a value and each variable value is displayed. // This approach is very inefficient for a large number of variables. public class Java 1101 { public static void main(String[] args) { int number 0 = 100; int number 1 = 101; int number 2 = 102; int number 3 = 103; int number 4 = 104; int number 5 = 105; int number 6 = 106; int number 7 = 107; int number 8 = 108; int number 9 = 109; } } System. out. print(number 0 + " "); System. out. print(number 1 + " "); System. out. print(number 2 + " "); System. out. print(number 3 + " "); System. out. print(number 4 + " "); System. out. print(number 5 + " "); System. out. print(number 6 + " "); System. out. print(number 7 + " "); System. out. print(number 8 + " "); System. out. print(number 9 + " "); System. out. println(); 109
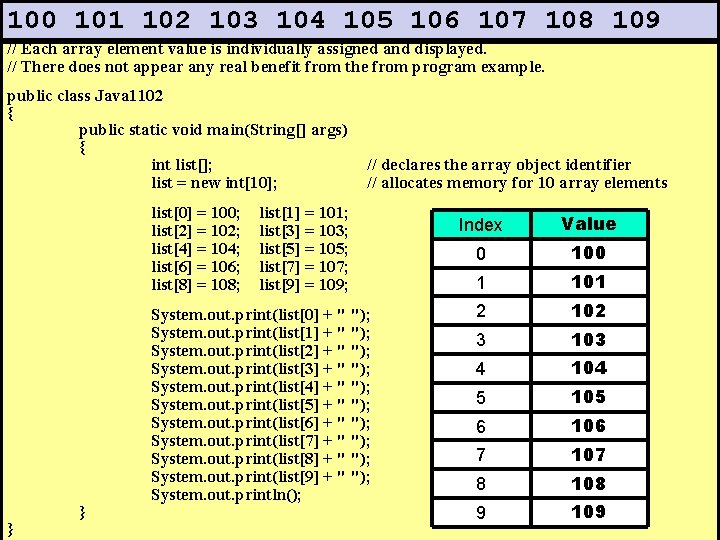
// Java 1102. java // This program declares an array of 10 <int> elements. // Each array element value is individually assigned and displayed. // There does not appear any real benefit from the from program example. 100 101 102 103 104 105 106 107 108 109 public class Java 1102 { public static void main(String[] args) { int list[]; list = new int[10]; list[0] = 100; list[2] = 102; list[4] = 104; list[6] = 106; list[8] = 108; } } // declares the array object identifier // allocates memory for 10 array elements list[1] = 101; list[3] = 103; list[5] = 105; list[7] = 107; list[9] = 109; System. out. print(list[0] + " "); System. out. print(list[1] + " "); System. out. print(list[2] + " "); System. out. print(list[3] + " "); System. out. print(list[4] + " "); System. out. print(list[5] + " "); System. out. print(list[6] + " "); System. out. print(list[7] + " "); System. out. print(list[8] + " "); System. out. print(list[9] + " "); System. out. println(); Index Value 0 100 1 101 2 102 3 103 4 104 5 105 6 106 7 107 8 108 9 109
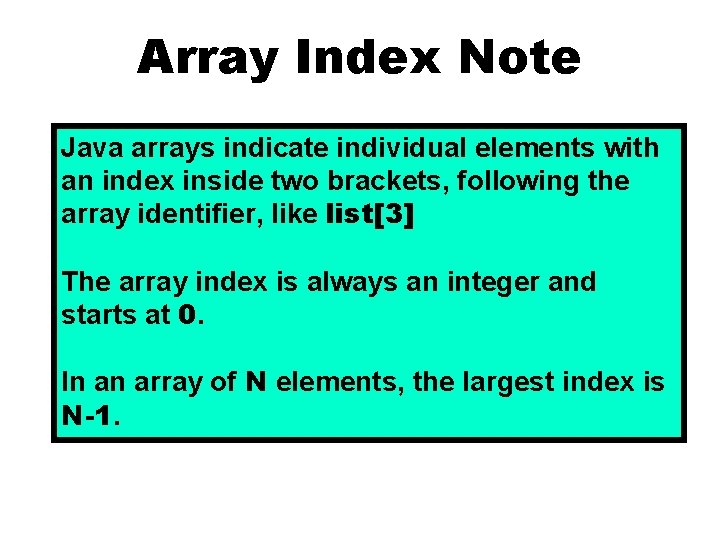
Array Index Note Java arrays indicate individual elements with an index inside two brackets, following the array identifier, like list[3] The array index is always an integer and starts at 0. In an array of N elements, the largest index is N-1.
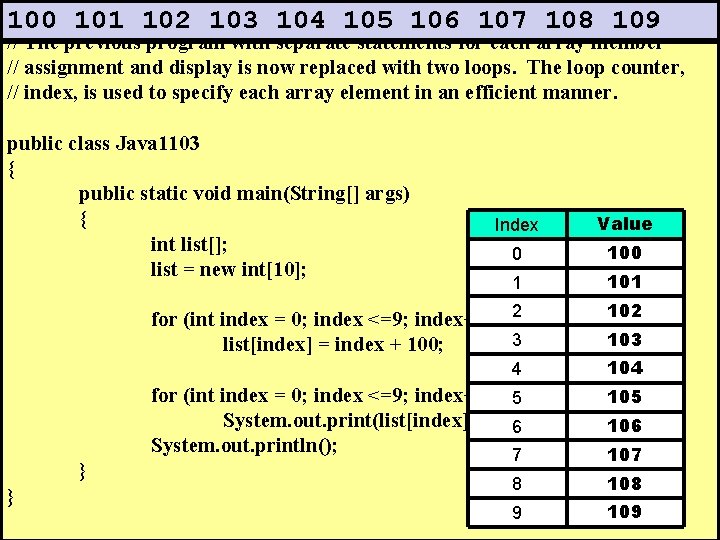
// Java 1103. java 100 101 102 103 104 105 106 107 108 109 // The previous program with separate statements for each array member // assignment and display is now replaced with two loops. The loop counter, // index, is used to specify each array element in an efficient manner. public class Java 1103 { public static void main(String[] args) { int list[]; list = new int[10]; Index Value 0 100 1 101 for (int index = 0; index <=9; index++) 2 3 list[index] = index + 100; 102 4 } } for (int index = 0; index <=9; index++) 5 System. out. print(list[index] + " "); 6 System. out. println(); 7 103 104 105 106 107 8 108 9 109
![Defining Static Arrays int list declares the array list identifier list new Defining Static Arrays int list[]; // declares the array list identifier list = new](https://slidetodoc.com/presentation_image_h2/cdfc99b78f29645cb18e7408585d1819/image-20.jpg)
Defining Static Arrays int list[]; // declares the array list identifier list = new int[10]; // allocates memory for 10 integers char names[]; // declares the names array identifier names = new char[25]; // allocates memory for 25 characters double grades[]; // declares the grades array identifier grades = new double[50]; // allocates memory for 50 doubles
![Defining Static Arrays Preferred Method int list new int10 char names Defining Static Arrays Preferred Method int list[ ] = new int[10]; char names[ ]](https://slidetodoc.com/presentation_image_h2/cdfc99b78f29645cb18e7408585d1819/image-21.jpg)
Defining Static Arrays Preferred Method int list[ ] = new int[10]; char names[ ] = new char[25]; double grades[ ] = new double[50];
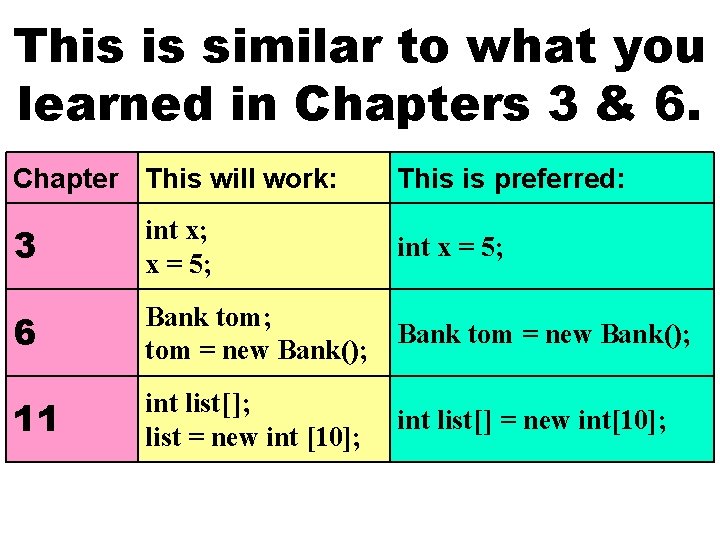
This is similar to what you learned in Chapters 3 & 6. Chapter This will work: This is preferred: 3 int x; x = 5; int x = 5; 6 Bank tom; tom = new Bank(); Bank tom = new Bank(); 11 int list[ ]; list = new int [10]; int list[ ] = new int[10];
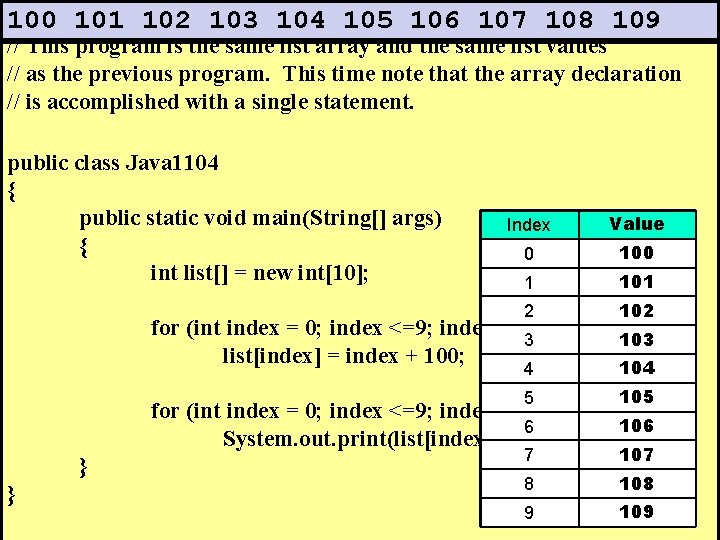
// Java 1104. java 100 101 102 103 104 105 106 107 108 109 // This program is the same list array and the same list values // as the previous program. This time note that the array declaration // is accomplished with a single statement. public class Java 1104 { public static void main(String[] args) { int list[] = new int[10]; Index Value 0 100 1 101 2 102 for (int index = 0; index <=9; index++)3 list[index] = index + 100; 4 104 5 105 for (int index = 0; index <=9; index++) 6 System. out. print(list[index] + " "); } } 103 106 7 107 8 108 9 109
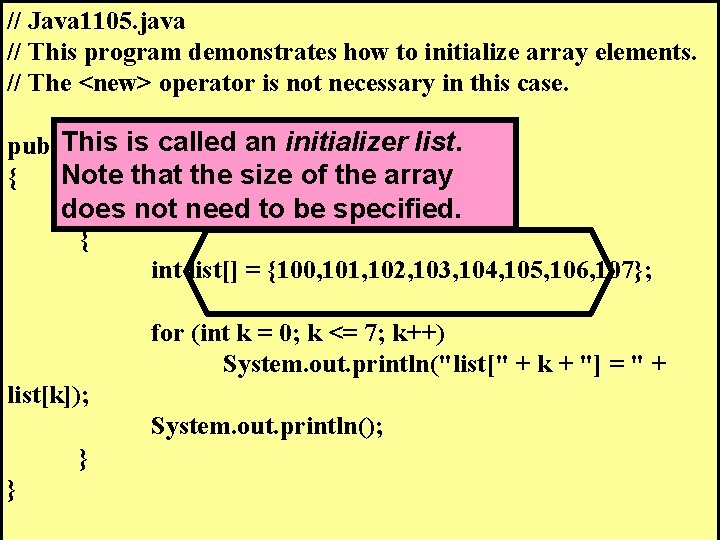
// Java 1105. java // This program demonstrates how to initialize array elements. // The <new> operator is not necessary in this case. This called an initializer list. public classis. Java 1105 Note that the size of the array { public static voidtomain(String[] args) does not need be specified. { int list[] = {100, 101, 102, 103, 104, 105, 106, 107}; for (int k = 0; k <= 7; k++) System. out. println("list[" + k + "] = " + list[k]); System. out. println(); } }
![list0 100 Java 1105 java list1 101 This program demonstrates list[0] = 100 // Java 1105. java list[1] = 101 // This program demonstrates](https://slidetodoc.com/presentation_image_h2/cdfc99b78f29645cb18e7408585d1819/image-25.jpg)
list[0] = 100 // Java 1105. java list[1] = 101 // This program demonstrates how to initialize array elements. list[2] = 102 // The <new> operator is not necessary in this case. list[3] list[4] public class Java 1105 list[5] { list[6] public static void main(String[] args) list[7] { = = = 103 104 105 106 107 int list[] = {100, 101, 102, 103, 104, 105, 106, 107}; for (int k = 0; k <= 7; k++) System. out. println("list[" + k + "] = " + list[k]); } } 0 System. out. println(); 1 2 3 4 5 6 7 100 101 102 103 104 105 106 107
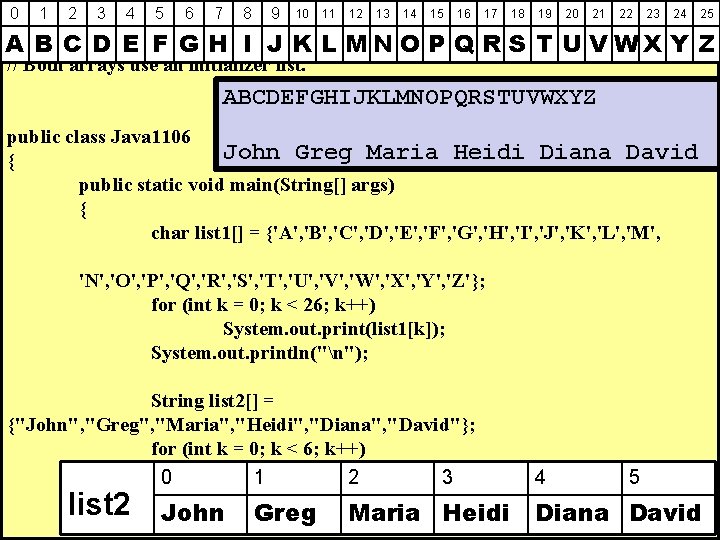
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 //0 Java 1106. java // This program a string A B C D E demonstrates F G H I a. Jcharacter K L Marray N Oand PQ R S array. TU // Both arrays use an initializer list. 21 22 23 24 25 VWX Y Z ABCDEFGHIJKLMNOPQRSTUVWXYZ public class Java 1106 John Greg Maria Heidi Diana David { public static void main(String[] args) { char list 1[] = {'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z'}; for (int k = 0; k < 26; k++) System. out. print(list 1[k]); System. out. println("n"); String list 2[] = {"John", "Greg", "Maria", "Heidi", "Diana", "David"}; for (int k = 0; k < 6; k++) System. out. println(list 2[k]); 0 1 2 3 System. out. println(); John Greg Maria Heidi } list 2 4 5 Diana David
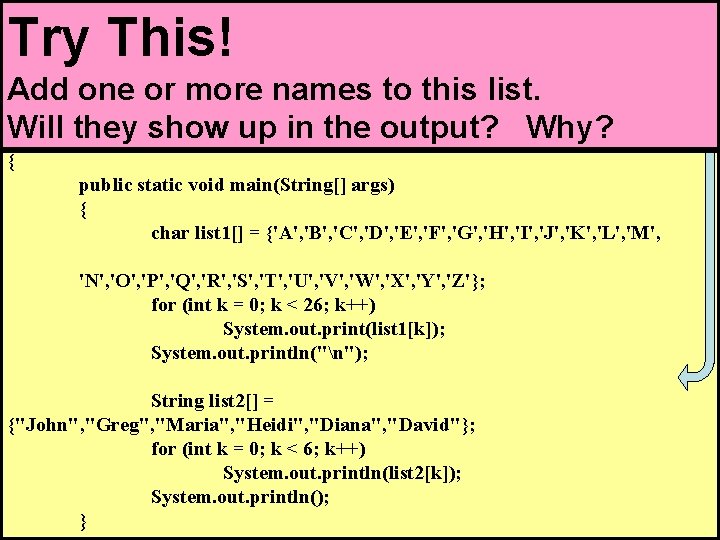
// Java 1106. java // This program demonstrates a character array and a string array. // Both arrays use an initializer list. Try This! Add one or more names to this list. Will they show up in the output? Why? public class Java 1106 { public static void main(String[] args) { char list 1[] = {'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z'}; for (int k = 0; k < 26; k++) System. out. print(list 1[k]); System. out. println("n"); String list 2[] = {"John", "Greg", "Maria", "Heidi", "Diana", "David"}; for (int k = 0; k < 6; k++) System. out. println(list 2[k]); System. out. println(); }
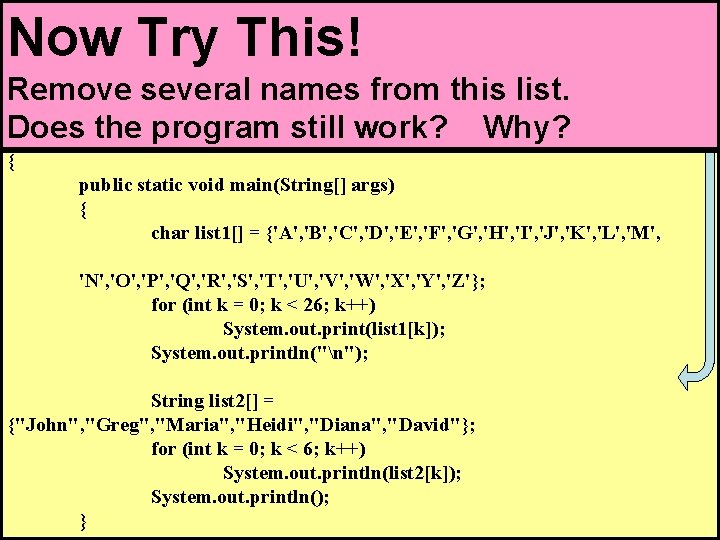
// Java 1106. java // This program demonstrates a character array and a string array. // Both arrays use an initializer list. Now Try This! Remove several names from this list. Does the program still work? Why? public class Java 1106 { public static void main(String[] args) { char list 1[] = {'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R', 'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z'}; for (int k = 0; k < 26; k++) System. out. print(list 1[k]); System. out. println("n"); String list 2[] = {"John", "Greg", "Maria", "Heidi", "Diana", "David"}; for (int k = 0; k < 6; k++) System. out. println(list 2[k]); System. out. println(); }
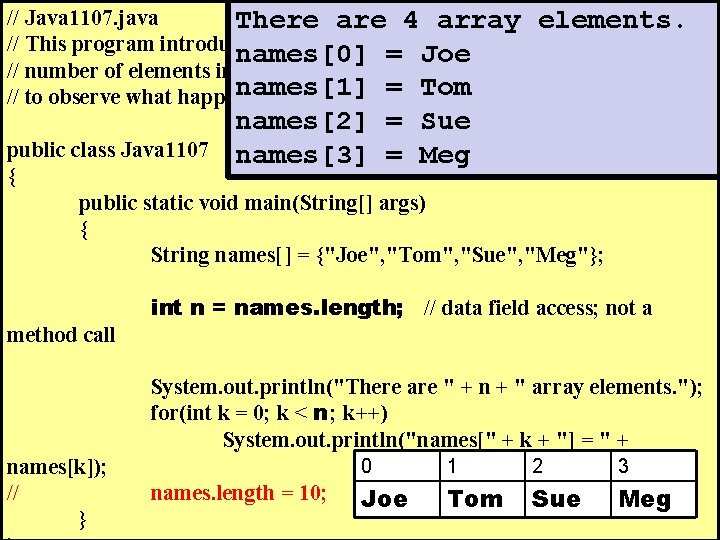
// Java 1107. java There are 4 array elements. // This program introduces the length field determine the names[0] = to. Joe // number of elements in the array. Remove the comments from line 17 names[1] = field Tomis altered. // to observe what happens when the length names[2] = Sue names[3] = Meg public class Java 1107 { public static void main(String[] args) { String names[ ] = {"Joe", "Tom", "Sue", "Meg"}; int n = names. length; // data field access; not a method call System. out. println("There are " + n + " array elements. "); for(int k = 0; k < n ; k++) System. out. println("names[" + k + "] = " + names[k]); // } names. length = 10; 0 1 2 3 Joe Tom Sue Meg
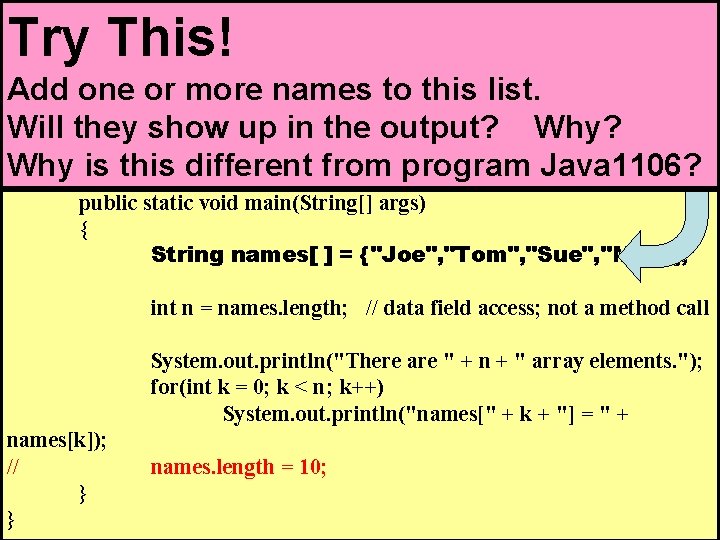
// Java 1107. java // This program introduces the length field to determine the // number of elements in the array. Remove the comments from line 16 Add onewhat or happens morewhen names to field thisis altered. list. // to observe the length Try This! Will they show up in the output? Why? public class Java 1107 Why is this different from program Java 1106? { public static void main(String[] args) { String names[ ] = { "Joe", "Tom", "Sue", "Meg"}; int n = names. length; // data field access; not a method call System. out. println("There are " + n + " array elements. "); for(int k = 0; k < n ; k++) System. out. println("names[" + k + "] = " + names[k]); // } } names. length = 10;
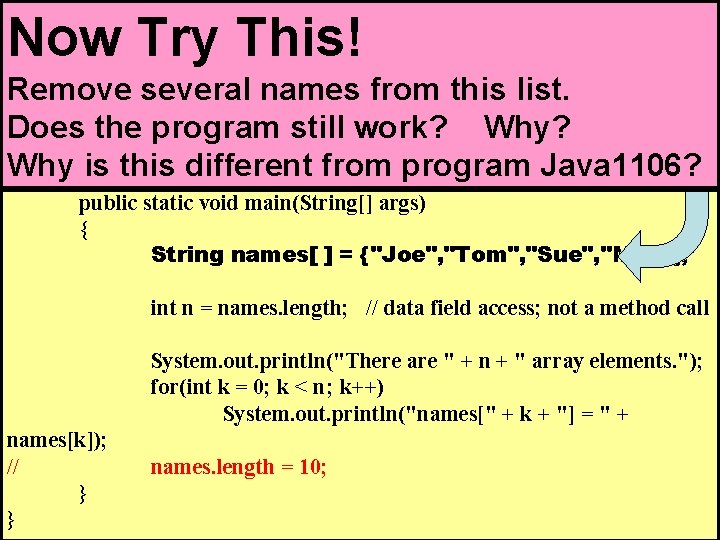
// Java 1107. java // This program introduces the length field to determine the // number of elements in the array. Remove the comments from line 16 Remove several from list. // to observe what happens names when the length fieldthis is altered. Now Try This! Does the program still work? Why? public class Java 1107 Why is this different from program Java 1106? { public static void main(String[] args) { String names[ ] = { "Joe", "Tom", "Sue", "Meg"}; int n = names. length; // data field access; not a method call System. out. println("There are " + n + " array elements. "); for(int k = 0; k < n ; k++) System. out. println("names[" + k + "] = " + names[k]); // } } names. length = 10;
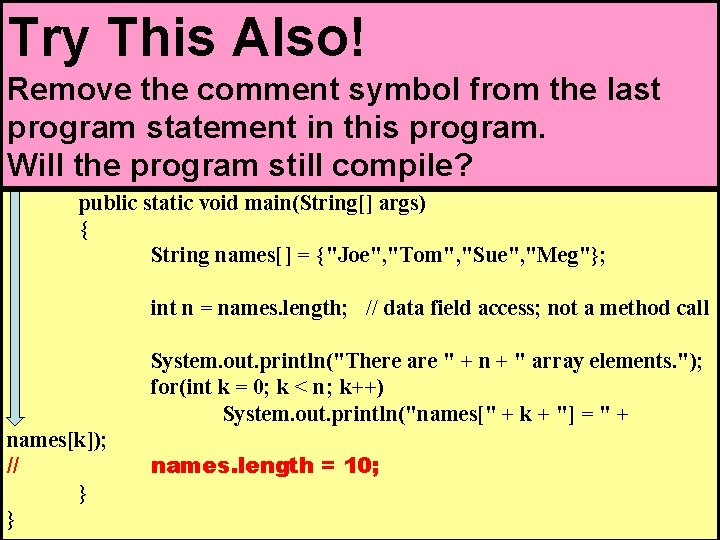
// Java 1107. java // This program introduces the length field to determine the // number of elements in the array. Remove the comments from line 16 Remove thehappens comment // to observe what when the symbol length field isfrom altered. the last Try This Also! program statement in this program. public class Java 1107 Will the program still compile? { public static void main(String[] args) { String names[ ] = { "Joe", "Tom", "Sue", "Meg"}; int n = names. length; // data field access; not a method call System. out. println("There are " + n + " array elements. "); for(int k = 0; k < n ; k++) System. out. println("names[" + k + "] = " + names[k]); // } } names. length = 10;
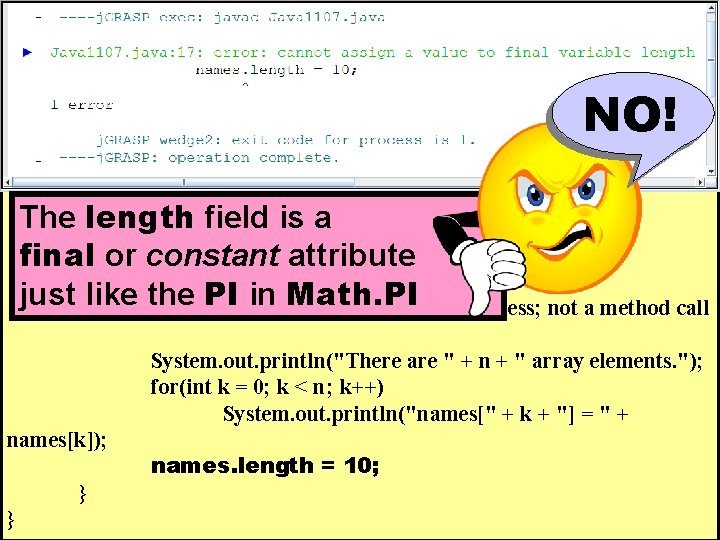
// Java 1107. java // This program introduces the length field to determine the // number of elements in the array. Remove the comments from line 16 // to observe what happens when the length field is altered. NO! public class Java 1107 { public static void main(String[] args) The {length field is a String names[ ]attribute = { "Joe", "Tom", "Sue", "Meg"}; final or constant just like the in Math. PI int n =PI names. length; // data field access; not a method call System. out. println("There are " + n + " array elements. "); for(int k = 0; k < n ; k++) System. out. println("names[" + k + "] = " + names[k]); } } names. length = 10;
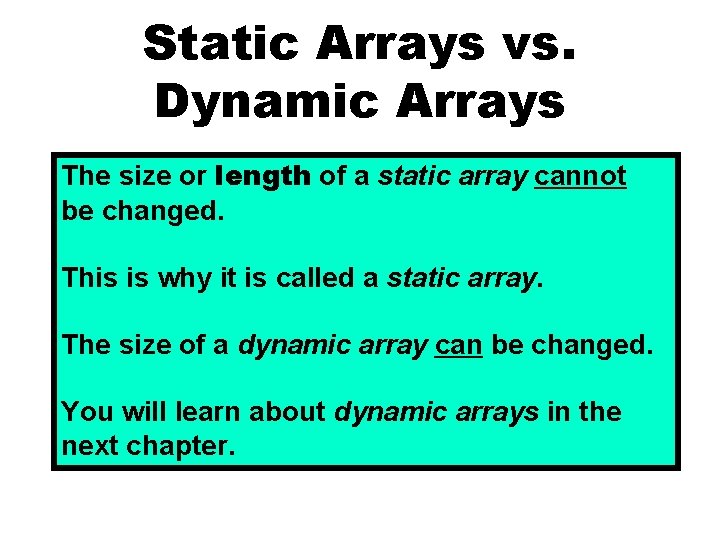
Static Arrays vs. Dynamic Arrays The size or length of a static array cannot be changed. This is why it is called a static array. The size of a dynamic array can be changed. You will learn about dynamic arrays in the next chapter.
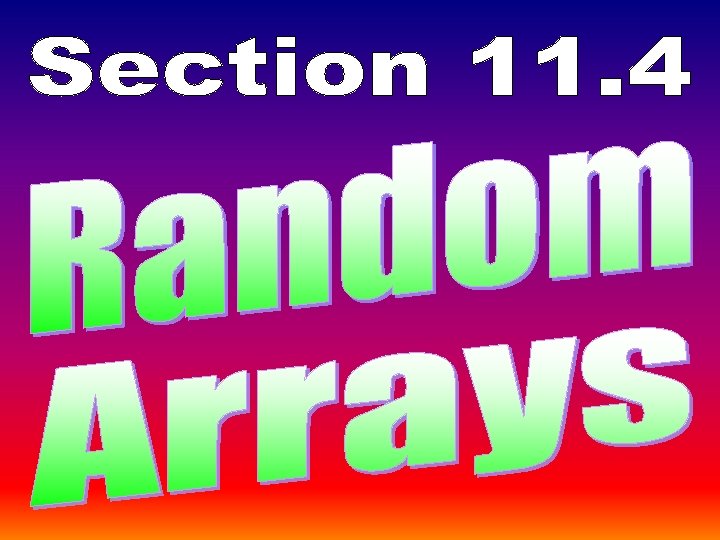
![list0 244 Java 1108 java list1 594 This program fills list[0] = 244 // Java 1108. java list[1] = 594 // This program fills](https://slidetodoc.com/presentation_image_h2/cdfc99b78f29645cb18e7408585d1819/image-36.jpg)
list[0] = 244 // Java 1108. java list[1] = 594 // This program fills an <int> array with a random set of numbers. list[2] = 902 list[3] = 385 list[4] = 949 list[5] = 792 list[6] = 636 list[7] = 894 list[8] = 126 list[9] = 103 list[10] = 938 list[11] = 592 list[12] = 584 list[13] = 112 900; list[14] = 766 list[15] = 791 list[16] = 447 list[17] = 298 list[18] = 596 list[19] = 243 public class Java 1108 { public static void main(String[] args) { int list[] = new int[20]; for (int k = 0; k < 20; k++) { double temp = Math. random() * int rand = (int) temp + 100; list[k] = rand; } for (int k = 0; k < 20; k++) System. out. println("list[" + k + "] = " + list[k]); }2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 0 1 } 24 59 90 38 94 79 63 89 12 10 93 59 58 11 76 79 44 29 59 24
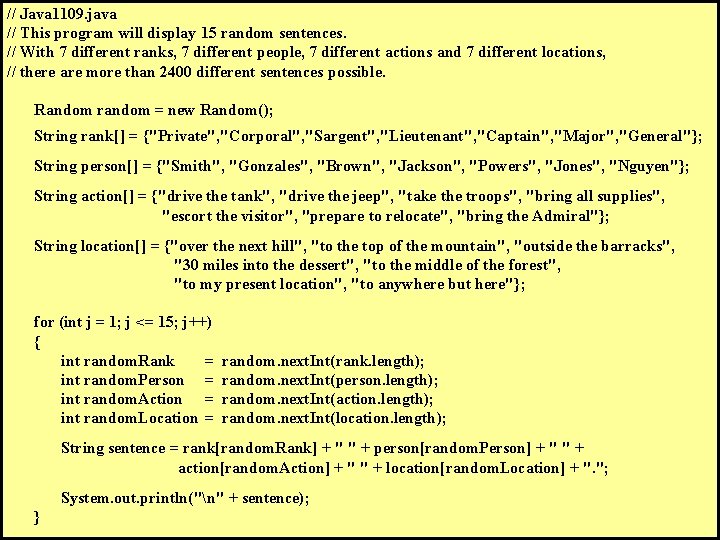
// Java 1109. java // This program will display 15 random sentences. // With 7 different ranks, 7 different people, 7 different actions and 7 different locations, // there are more than 2400 different sentences possible. Random random = new Random(); String rank[ ] = {"Private", "Corporal", "Sargent", "Lieutenant", "Captain", "Major", "General"}; String person[ ] = {"Smith", "Gonzales", "Brown", "Jackson", "Powers", "Jones", "Nguyen"}; String action[ ] = {"drive the tank", "drive the jeep", "take the troops", "bring all supplies", "escort the visitor", "prepare to relocate", "bring the Admiral"}; String location[ ] = {"over the next hill", "to the top of the mountain", "outside the barracks", "30 miles into the dessert", "to the middle of the forest", "to my present location", "to anywhere but here"}; for (int j = 1; j <= 15; j++) { int random. Rank = int random. Person = int random. Action = int random. Location = random. next. Int(rank. length); random. next. Int(person. length); random. next. Int(action. length); random. next. Int(location. length); String sentence = rank[random. Rank] + " " + person[random. Person] + " " + action[random. Action] + " " + location[random. Location] + ". "; System. out. println("n" + sentence); }
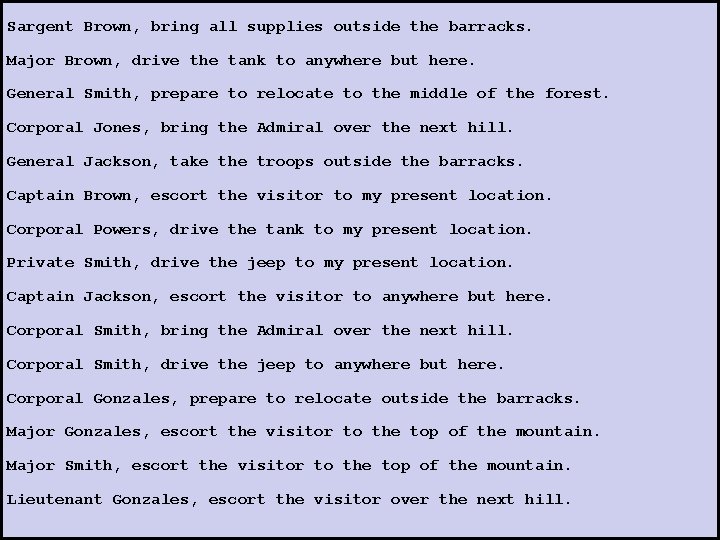
Sargent Brown, bring all supplies outside the barracks. Major Brown, drive the tank to anywhere but here. General Smith, prepare to relocate to the middle of the forest. Corporal Jones, bring the Admiral over the next hill. General Jackson, take the troops outside the barracks. Captain Brown, escort the visitor to my present location. Corporal Powers, drive the tank to my present location. Private Smith, drive the jeep to my present location. Captain Jackson, escort the visitor to anywhere but here. Corporal Smith, bring the Admiral over the next hill. Corporal Smith, drive the jeep to anywhere but here. Corporal Gonzales, prepare to relocate outside the barracks. Major Gonzales, escort the visitor to the top of the mountain. Major Smith, escort the visitor to the top of the mountain. Lieutenant Gonzales, escort the visitor over the next hill.
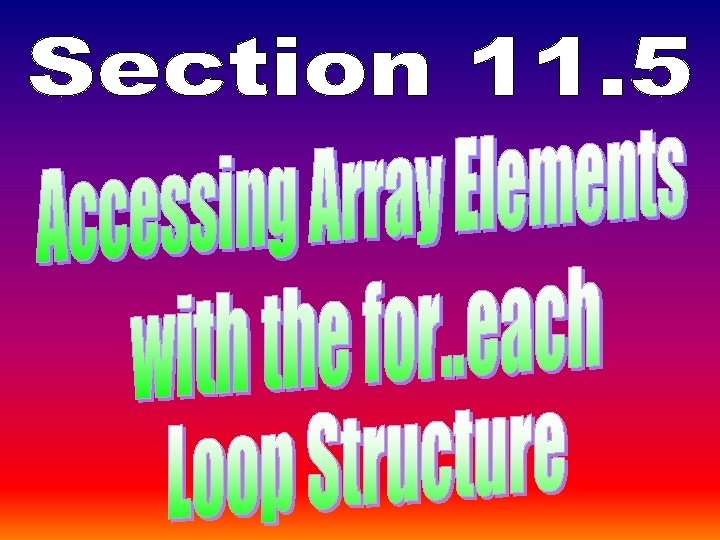
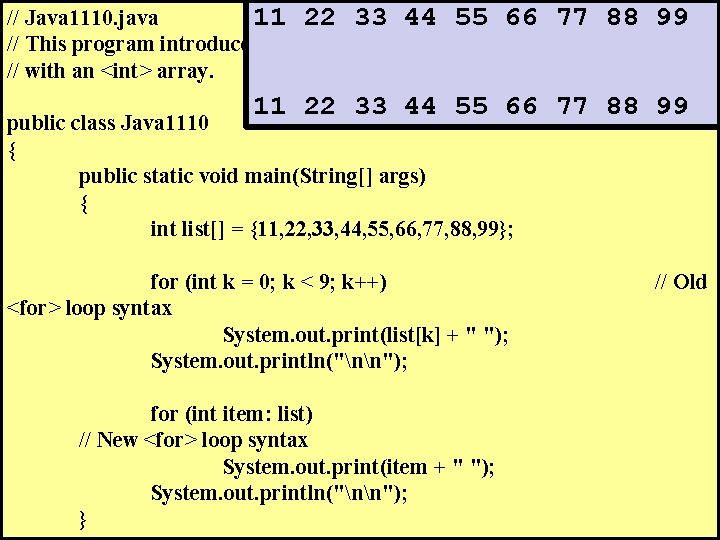
// Java 1110. java 11 22 33 44 55 66 77 88 99 // This program introduces the Java Version 5. 0 enhanced <for. . each> loop // with an <int> array. 11 22 33 44 55 66 77 88 99 public class Java 1110 { public static void main(String[] args) { int list[] = {11, 22, 33, 44, 55, 66, 77, 88, 99}; for (int k = 0; k < 9; k++) <for> loop syntax System. out. print(list[k] + " "); System. out. println("nn"); for (int item: list) // New <for> loop syntax System. out. print(item + " "); System. out. println("nn"); } // Old
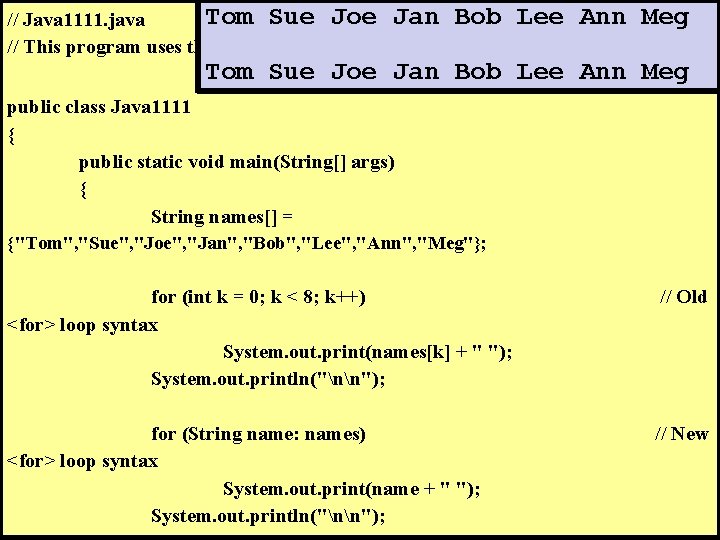
Tom Sue Joe Jan Bob Lee Ann Meg // Java 1111. java // This program uses the Java Version 5. 0 <for. . each> loop with a <String> array. Tom Sue Joe Jan Bob Lee Ann Meg public class Java 1111 { public static void main(String[] args) { String names[] = {"Tom", "Sue", "Joe", "Jan", "Bob", "Lee", "Ann", "Meg"}; for (int k = 0; k < 8; k++) <for> loop syntax System. out. print(names[k] + " "); System. out. println("nn"); // Old for (String name: names) <for> loop syntax System. out. print(name + " "); System. out. println("nn"); // New
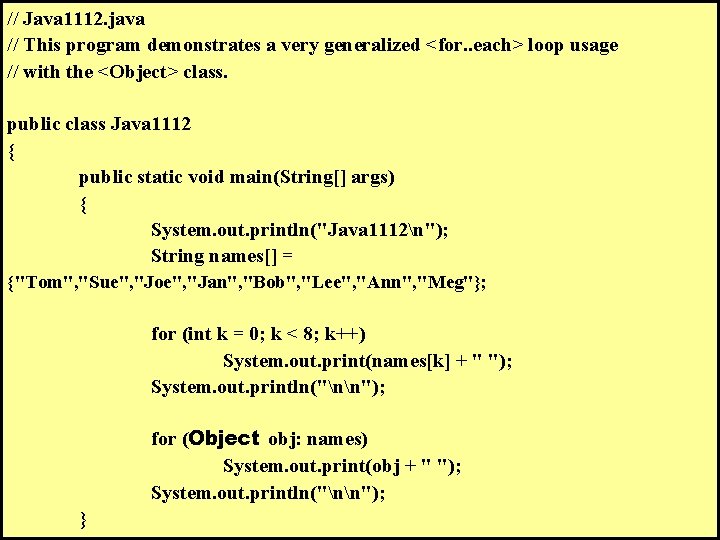
// Java 1112. java // This program demonstrates a very generalized <for. . each> loop usage // with the <Object> class. public class Java 1112 { public static void main(String[] args) { System. out. println("Java 1112n"); String names[] = {"Tom", "Sue", "Joe", "Jan", "Bob", "Lee", "Ann", "Meg"}; for (int k = 0; k < 8; k++) System. out. print(names[k] + " "); System. out. println("nn"); for (Object obj: names) System. out. print(obj + " "); System. out. println("nn"); }
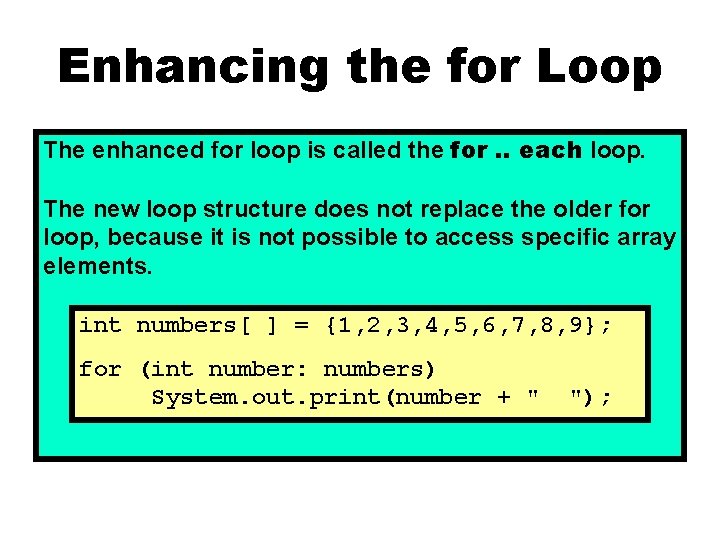
Enhancing the for Loop The enhanced for loop is called the for. . each loop. The new loop structure does not replace the older for loop, because it is not possible to access specific array elements. int numbers[ ] = {1, 2, 3, 4, 5, 6, 7, 8, 9}; for (int number: numbers) System. out. print(number + " ");
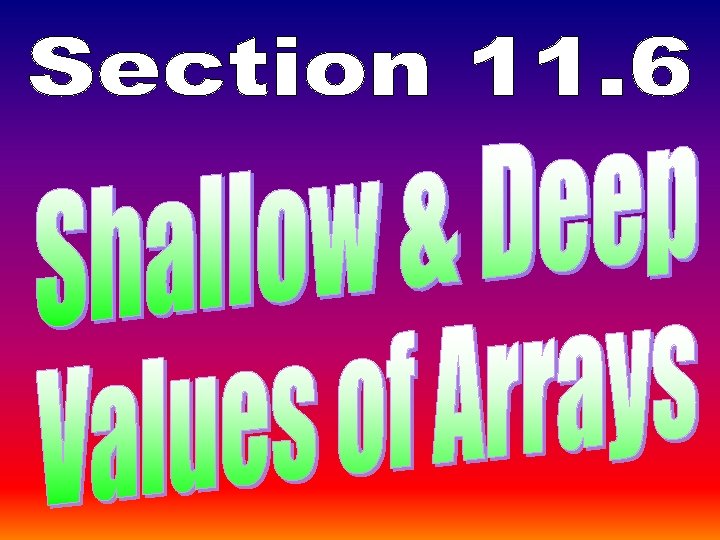
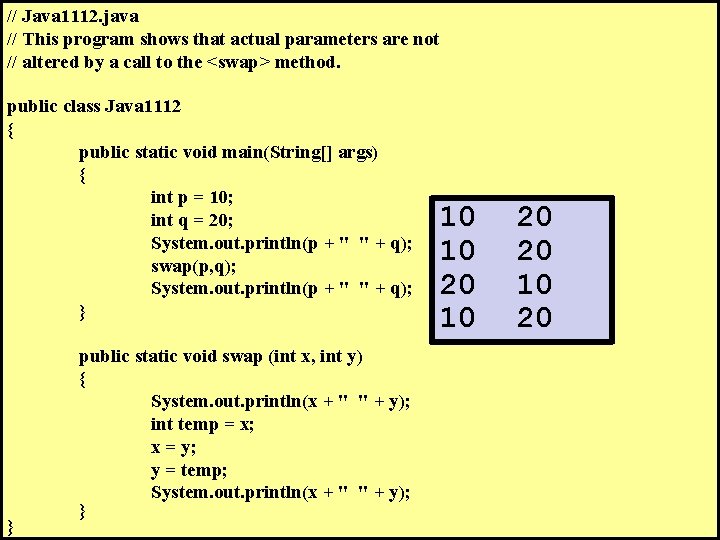
// Java 1112. java // This program shows that actual parameters are not // altered by a call to the <swap> method. public class Java 1112 { public static void main(String[] args) { int p = 10; int q = 20; System. out. println(p + " " + q); swap(p, q); System. out. println(p + " " + q); } } public static void swap (int x, int y) { System. out. println(x + " " + y); int temp = x; x = y; y = temp; System. out. println(x + " " + y); } 10 10 20 20 10 20
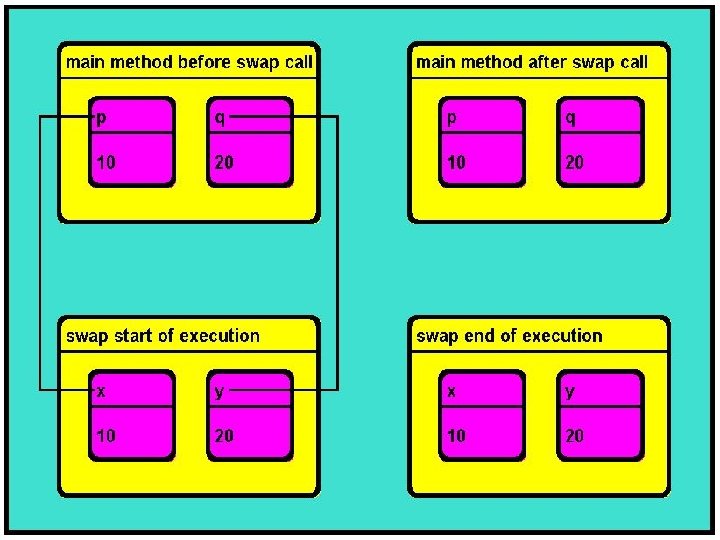
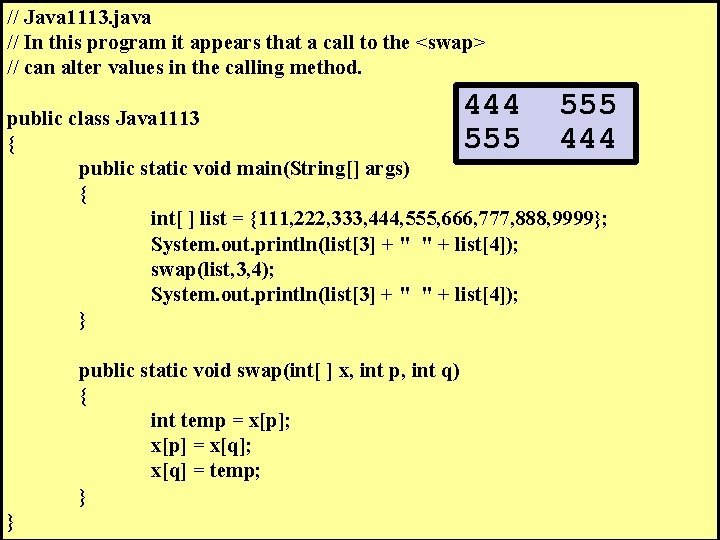
// Java 1113. java // In this program it appears that a call to the <swap> // can alter values in the calling method. 444 555 public class Java 1113 555 444 { public static void main(String[] args) { int[ ] list = {111, 222, 333, 444, 555, 666, 777, 888, 9999}; System. out. println(list[3] + " " + list[4]); swap(list, 3, 4); System. out. println(list[3] + " " + list[4]); } public static void swap(int[ ] x, int p, int q) { int temp = x[p]; x[p] = x[q]; x[q] = temp; } }
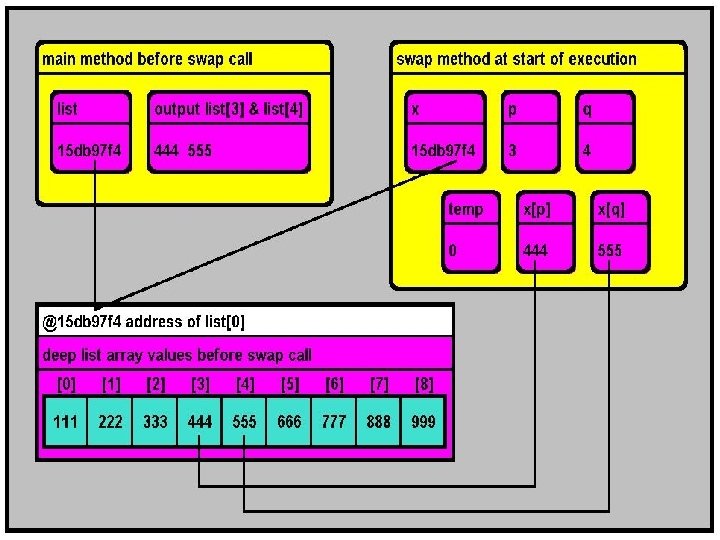
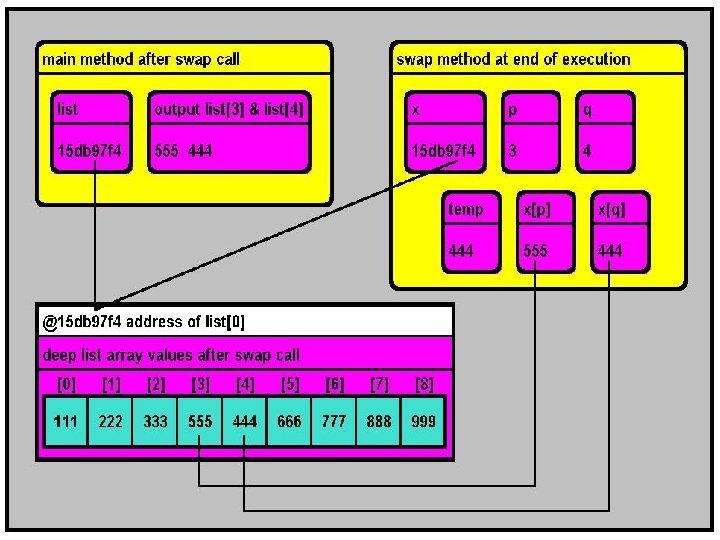
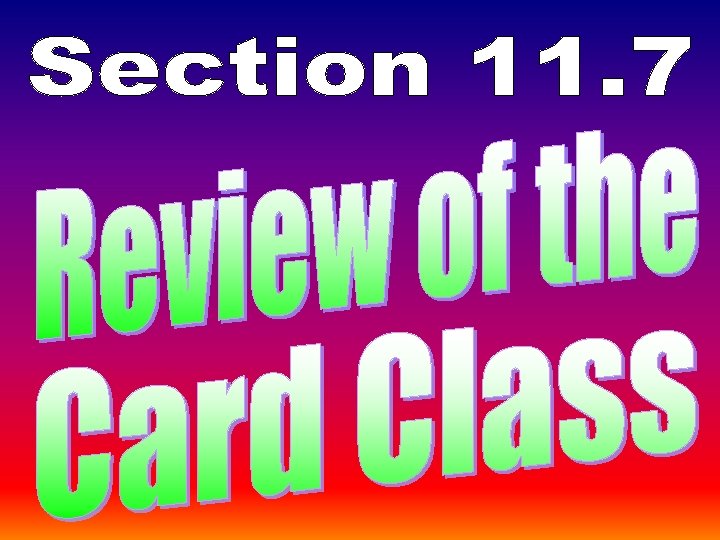
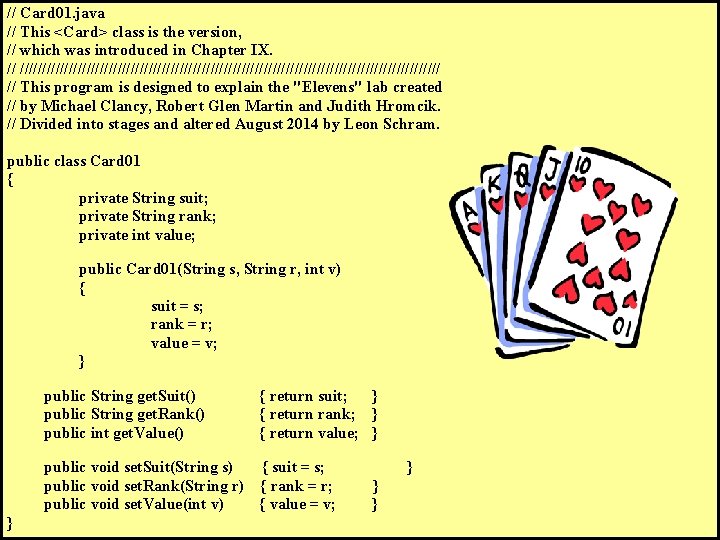
// Card 01. java // This <Card> class is the version, // which was introduced in Chapter IX. // //////////////////////////////////////////////// // This program is designed to explain the "Elevens" lab created // by Michael Clancy, Robert Glen Martin and Judith Hromcik. // Divided into stages and altered August 2014 by Leon Schram. public class Card 01 { private String suit; private String rank; private int value; public Card 01(String s, String r, int v) { suit = s; rank = r; value = v; } public String get. Suit() public String get. Rank() public int get. Value() { return suit; } { return rank; } { return value; } public void set. Suit(String s) { suit = s; public void set. Rank(String r) { rank = r; public void set. Value(int v) { value = v; } }
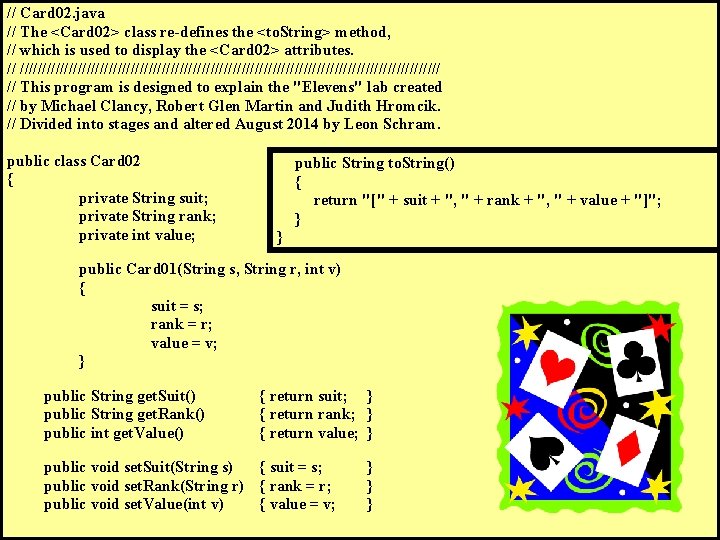
// Card 02. java // The <Card 02> class re-defines the <to. String> method, // which is used to display the <Card 02> attributes. // //////////////////////////////////////////////// // This program is designed to explain the "Elevens" lab created // by Michael Clancy, Robert Glen Martin and Judith Hromcik. // Divided into stages and altered August 2014 by Leon Schram. public class Card 02 { private String suit; private String rank; private int value; public String to. String() { return "[" + suit + ", " + rank + ", " + value + "]"; } } public Card 01(String s, String r, int v) { suit = s; rank = r; value = v; } public String get. Suit() public String get. Rank() public int get. Value() { return suit; } { return rank; } { return value; } public void set. Suit(String s) { suit = s; public void set. Rank(String r) { rank = r; public void set. Value(int v) { value = v; } } }
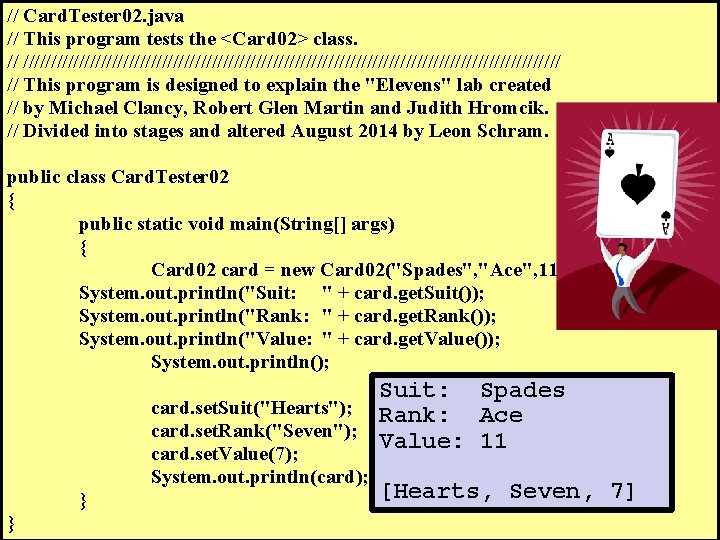
// Card. Tester 02. java // This program tests the <Card 02> class. // ///////////////////////////////////////////////// // This program is designed to explain the "Elevens" lab created // by Michael Clancy, Robert Glen Martin and Judith Hromcik. // Divided into stages and altered August 2014 by Leon Schram. public class Card. Tester 02 { public static void main(String[] args) { Card 02 card = new Card 02("Spades", "Ace", 11); System. out. println("Suit: " + card. get. Suit()); System. out. println("Rank: " + card. get. Rank()); System. out. println("Value: " + card. get. Value()); System. out. println(); Suit: Spades card. set. Suit("Hearts"); Rank: Ace card. set. Rank("Seven"); Value: 11 card. set. Value(7); System. out. println(card); } } [Hearts, Seven, 7]
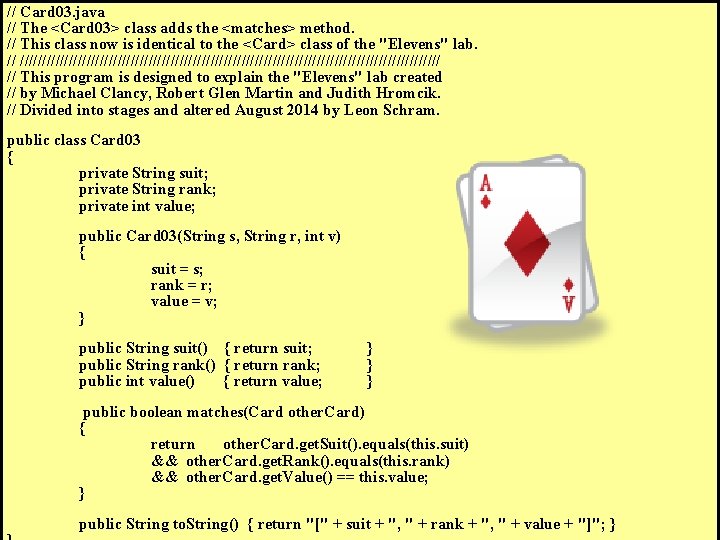
// Card 03. java // The <Card 03> class adds the <matches> method. // This class now is identical to the <Card> class of the "Elevens" lab. // //////////////////////////////////////////////// // This program is designed to explain the "Elevens" lab created // by Michael Clancy, Robert Glen Martin and Judith Hromcik. // Divided into stages and altered August 2014 by Leon Schram. public class Card 03 { private String suit; private String rank; private int value; public Card 03(String s, String r, int v) { suit = s; rank = r; value = v; } public String suit() { return suit; public String rank() { return rank; public int value() { return value; } } } public boolean matches(Card other. Card) { return other. Card. get. Suit(). equals(this. suit) && other. Card. get. Rank(). equals(this. rank) && other. Card. get. Value() == this. value; } public String to. String() { return "[" + suit + ", " + rank + ", " + value + "]"; }
![Card Tester 03 java Spades Ace 11 This program tests the Card // Card. Tester 03. java [Spades, Ace, 11] // This program tests the <Card](https://slidetodoc.com/presentation_image_h2/cdfc99b78f29645cb18e7408585d1819/image-55.jpg)
// Card. Tester 03. java [Spades, Ace, 11] // This program tests the <Card 03> class. [Clubs, Seven, 7] // ///////////////////////////// [Spades, Ace, 11] // This program is designed to explain the "Elevens" lab created // by Michael Clancy, Robert Glen Martin and Judith Hromcik. // Divided into stages and altered August 2014 byfalse Leon Schram. true false public class Card. Tester 03 { public static void main(String[] args) { Card 03 card 1 = new Card 03("Spades", "Ace", 11); Card 03 card 2 = new Card 03("Seven", "Clubs", 7); Card 03 card 3 = new Card 03("Spades", "Ace", 11); System. out. println(card 2); System. out. println(card 3); System. out. println(card 1. matches(card 2)); System. out. println(card 1. matches(card 3)); System. out. println(card 2. matches(card 3)); } }
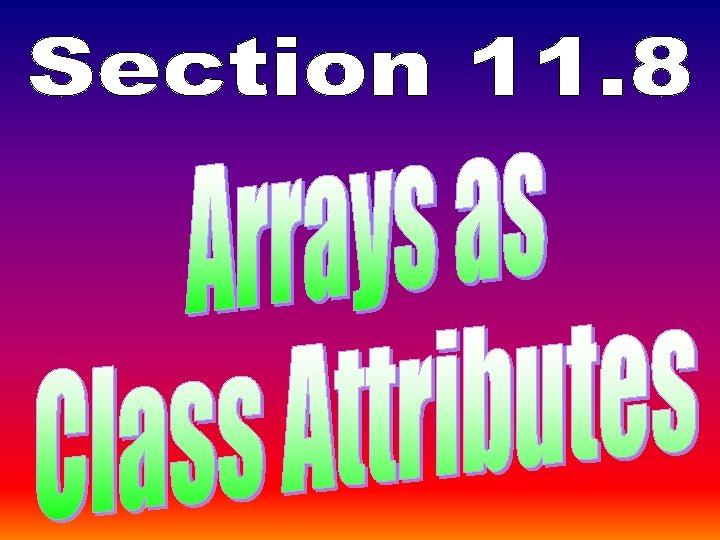
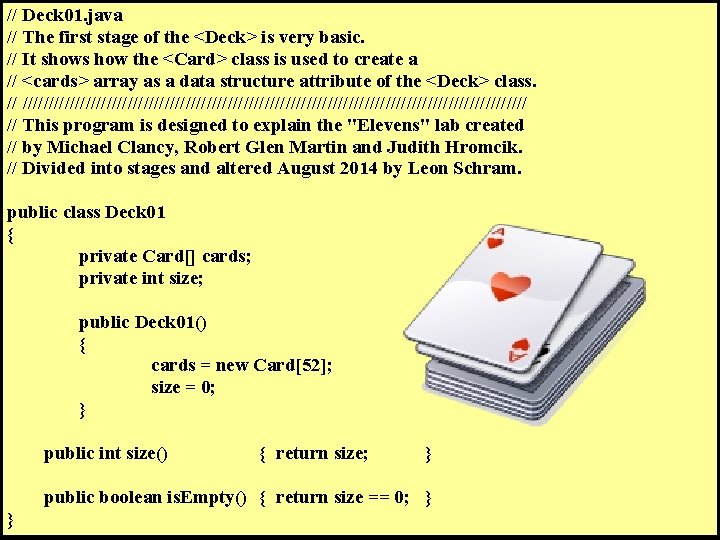
// Deck 01. java // The first stage of the <Deck> is very basic. // It shows how the <Card> class is used to create a // <cards> array as a data structure attribute of the <Deck> class. // //////////////////////////////////////////////// // This program is designed to explain the "Elevens" lab created // by Michael Clancy, Robert Glen Martin and Judith Hromcik. // Divided into stages and altered August 2014 by Leon Schram. public class Deck 01 { private Card[] cards; private int size; public Deck 01() { cards = new Card[52]; size = 0; } public int size() { return size; } public boolean is. Empty() { return size == 0; } }
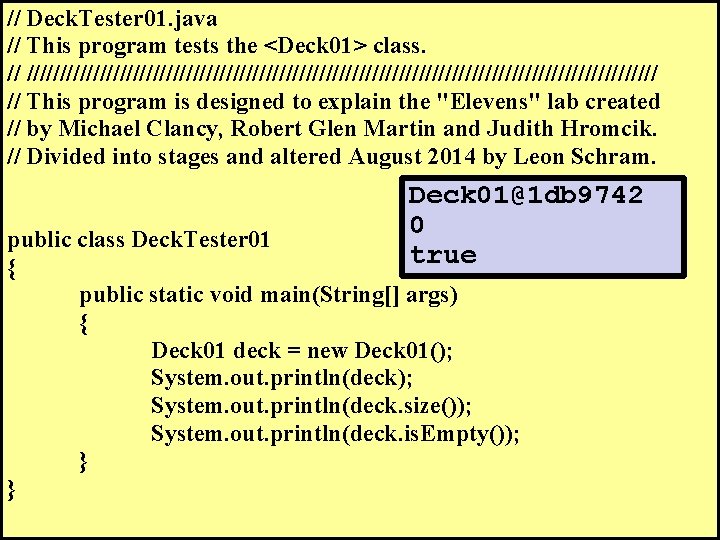
// Deck. Tester 01. java // This program tests the <Deck 01> class. // //////////////////////////////////////////////// // This program is designed to explain the "Elevens" lab created // by Michael Clancy, Robert Glen Martin and Judith Hromcik. // Divided into stages and altered August 2014 by Leon Schram. Deck 01@1 db 9742 0 true public class Deck. Tester 01 { public static void main(String[] args) { Deck 01 deck = new Deck 01(); System. out. println(deck. size()); System. out. println(deck. is. Empty()); } }
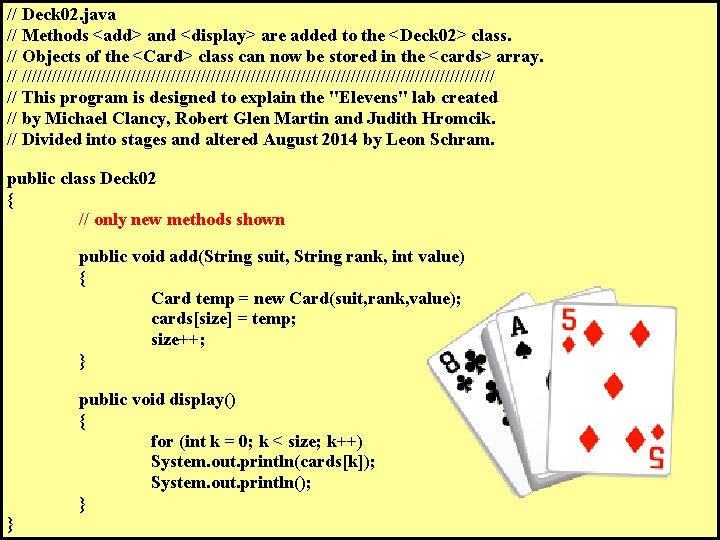
// Deck 02. java // Methods <add> and <display> are added to the <Deck 02> class. // Objects of the <Card> class can now be stored in the <cards> array. // //////////////////////////////////////////////// // This program is designed to explain the "Elevens" lab created // by Michael Clancy, Robert Glen Martin and Judith Hromcik. // Divided into stages and altered August 2014 by Leon Schram. public class Deck 02 { // only new methods shown public void add(String suit, String rank, int value) { Card temp = new Card(suit, rank, value); cards[size] = temp; size++; } public void display() { for (int k = 0; k < size; k++) System. out. println(cards[k]); System. out. println(); } }
![Deck Tester 02 java Clubs Three 3 This program tests the Deck // Deck. Tester 02. java [Clubs, Three, 3] // This program tests the <Deck](https://slidetodoc.com/presentation_image_h2/cdfc99b78f29645cb18e7408585d1819/image-60.jpg)
// Deck. Tester 02. java [Clubs, Three, 3] // This program tests the <Deck 02> class. [Diamonds, Four, 4] // ///////////////////////////// [Hearts, Five, 5] // This program is designed to explain the "Elevens" lab created Six, 6] // by Michael Clancy, Robert Glen Martin [Spades, and Judith Hromcik. // Divided into stages and altered August 2014 by Leon Schram. 4 false public class Deck. Tester 02 { public static void main(String[] args) { Deck 02 deck = new Deck 02(); deck. add("Clubs", "Three", 3); deck. add("Diamonds", "Four", 4); deck. add("Hearts", "Five", 5); deck. add("Spades", "Six", 6); deck. display(); System. out. println(deck. size()); System. out. println(deck. is. Empty()); } }
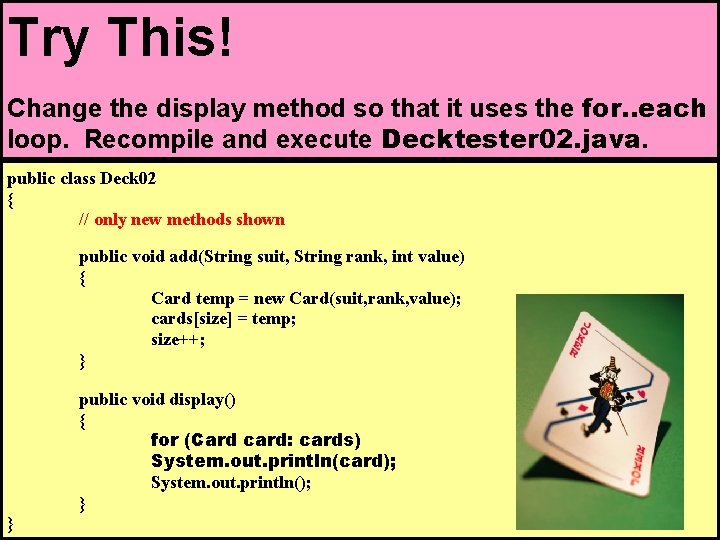
// Deck 02. java // Methods <add> and <display> are added to the <Deck 02> class. // Objects of the <Card> class can now be stored in the <cards> array. // ///////////////////////////// // This program is designed to explain the "Elevens" lab created Change display method so that it uses the for. . each // by Michael the Clancy, Robert Glen Martin and Judith Hromcik. // Divided Recompile into stages and altered 2014 by. Decktester 02. java. Leon Schram. loop. and. August execute Try This! public class Deck 02 { // only new methods shown public void add(String suit, String rank, int value) { Card temp = new Card(suit, rank, value); cards[size] = temp; size++; } public void display() { for (Card card: cards) System. out. println(card); System. out. println(); } }
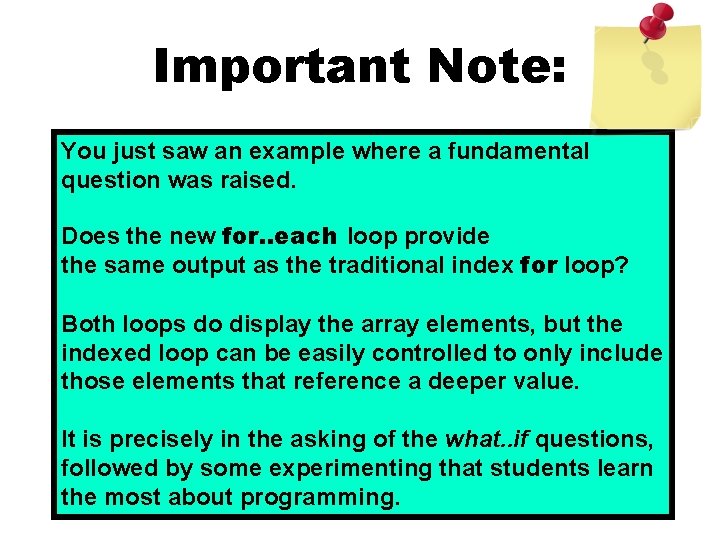
Important Note: You just saw an example where a fundamental question was raised. Does the new for. . each loop provide the same output as the traditional index for loop? Both loops do display the array elements, but the indexed loop can be easily controlled to only include those elements that reference a deeper value. It is precisely in the asking of the what. . if questions, followed by some experimenting that students learn the most about programming.
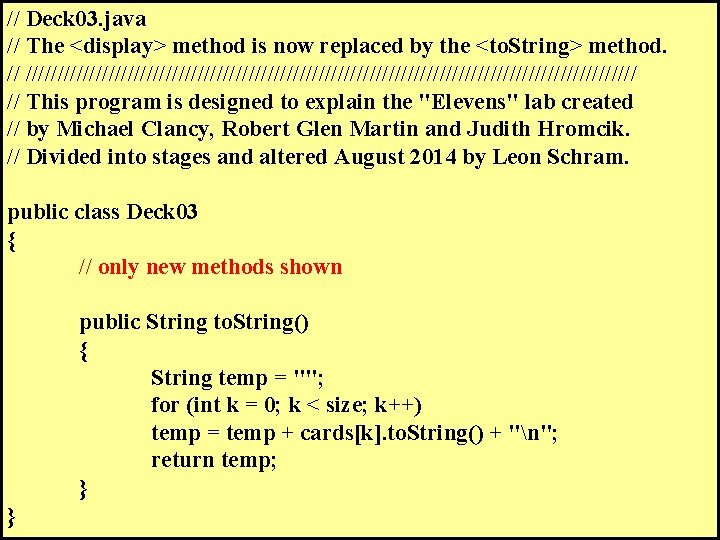
// Deck 03. java // The <display> method is now replaced by the <to. String> method. // //////////////////////////////////////////////// // This program is designed to explain the "Elevens" lab created // by Michael Clancy, Robert Glen Martin and Judith Hromcik. // Divided into stages and altered August 2014 by Leon Schram. public class Deck 03 { // only new methods shown public String to. String() { String temp = ""; for (int k = 0; k < size; k++) temp = temp + cards[k]. to. String() + "n"; return temp; } }
![Deck Tester 03 java Clubs Three 3 This program tests the Deck // Deck. Tester 03. java [Clubs, Three, 3] // This program tests the <Deck](https://slidetodoc.com/presentation_image_h2/cdfc99b78f29645cb18e7408585d1819/image-64.jpg)
// Deck. Tester 03. java [Clubs, Three, 3] // This program tests the <Deck 03> class. [Diamonds, Four, 4] // ///////////////////////////// [Hearts, Five, 5] // This program is designed to explain the "Elevens" lab created [Spades, Six, 6] // by Michael Clancy, Robert Glen Martin and Judith Hromcik. // Divided into stages and altered August 2014 by Leon Schram. 4 false public class Deck. Tester 03 { public static void main(String[] args) { Deck 03 deck = new Deck 03(); deck. add("Clubs", "Three", 3); deck. add("Diamonds", "Four", 4); deck. add("Hearts", "Five", 5); deck. add("Spades", "Six", 6); System. out. println(deck. size()); System. out. println(deck. is. Empty()); } }
Put first things first definition
What is static and dynamic data structure
The maturity continuum covey
State space search
Sdl first vs code first
Put first things first activities
Habit 3
Difference between code first and database first approach
First to invent or first to file
Data structure stack
Contoh soal stack dan jawabannya
First in first out
First come first serve
First come first serve gantt chart
Put first things first
Habit 3 put first things first
Put first things first video
Put first things first activities
First aid merit badge first aid kit
Objective of first aid
Put first things first
Record adalah
Data structure operations
Threaded binary tree
Explain classification of data structure with proper figure
Data structure classification
What is first conditional
First cbt session structure
If simple present
Braves
First continental congress definition
First call resolution presentation
First ionization energy definition
First ionisation energy definition
First aid definition
Limited third person
First pass effect in pharmacology
First pass metabolism definition pharmacology
Goals of first aid are
First generation of computer
Zero and first conditional exercises
Ionisation energy definition
What is successive ionisation energy
What is a covalent bond simple definition
Giant molecular structure vs simple molecular structure
@prof._jane_blendes:https://semawur.com/xujhhf16p
Deep structure in linguistics
Subject-dqrnghtp
Giant molecular structure vs simple molecular structure
Deep surface structure
Linear data structure
Linear data structure using sequential organization
Linear and nonlinear data structure
Diff between primitive and nonprimitive
Linear and nonlinear data structure
Bfs tree traversal
Topological sort definition
Contoh queue
Data structure metrics
Graph traversal in data structure
Sparse matrix in data structure
Polynomial addition using linked list in c
Sparse matrix in data structure
Josephus problem in c using array