EECS 110 Lec 10 Definite Loops and User
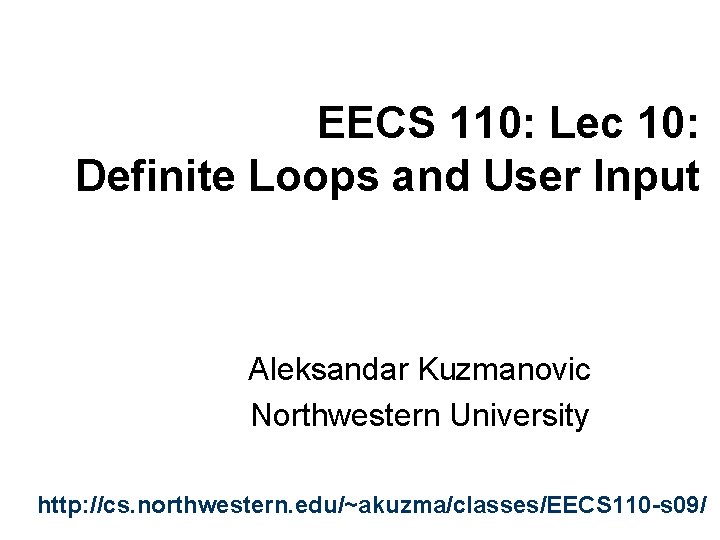
![Loops! We've seen variables change in-place before: [ x*6 for x in range(8) ] Loops! We've seen variables change in-place before: [ x*6 for x in range(8) ]](https://slidetodoc.com/presentation_image/e165a7de024e1f6aadca5d0080893cff/image-2.jpg)
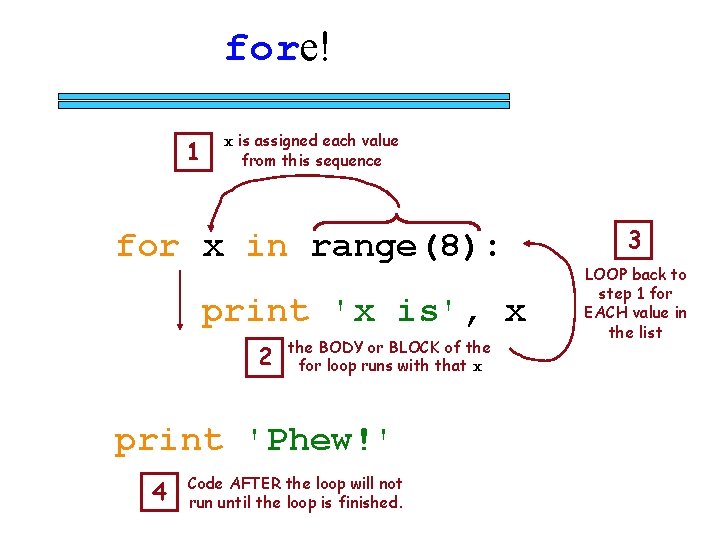
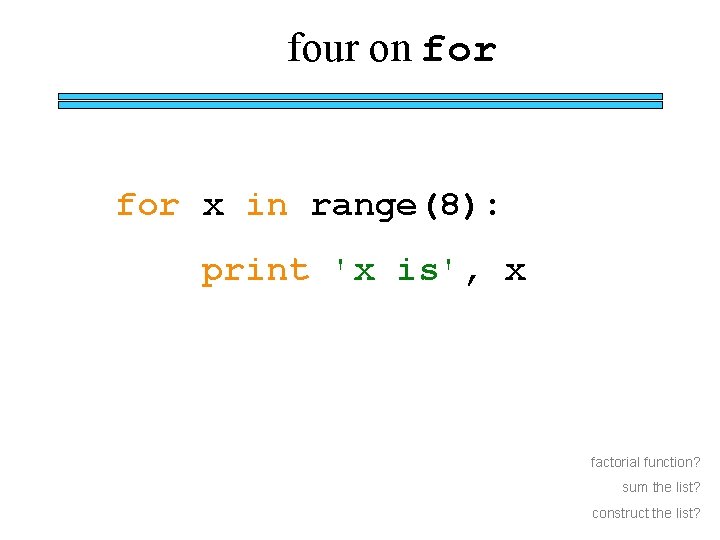
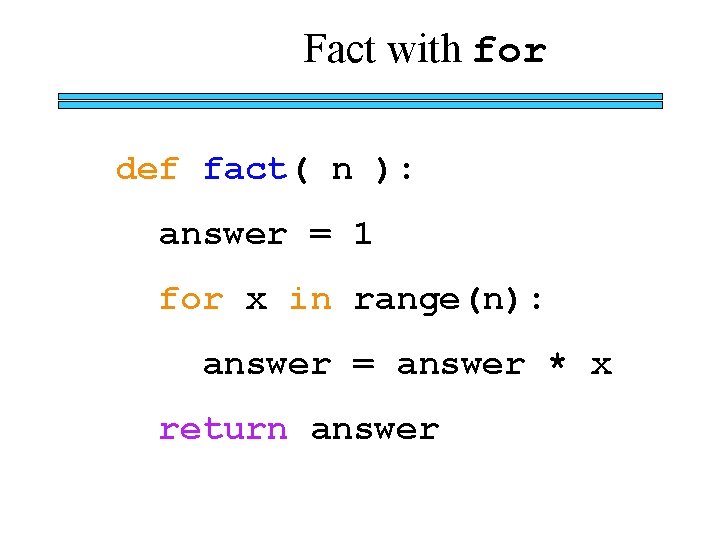
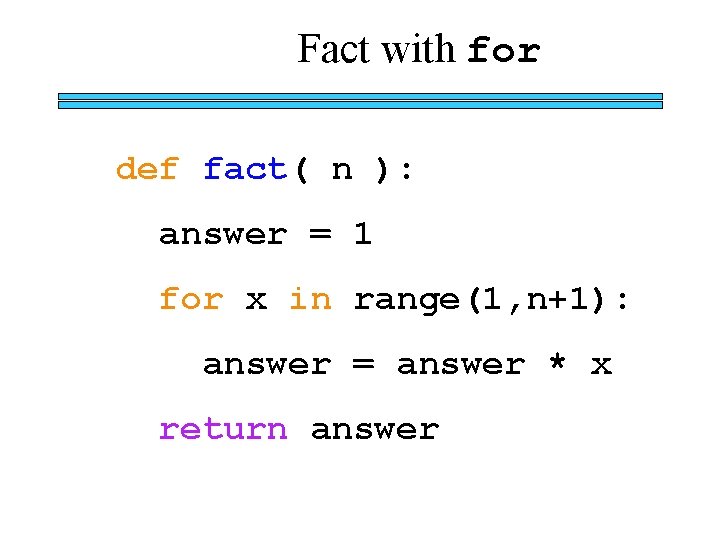
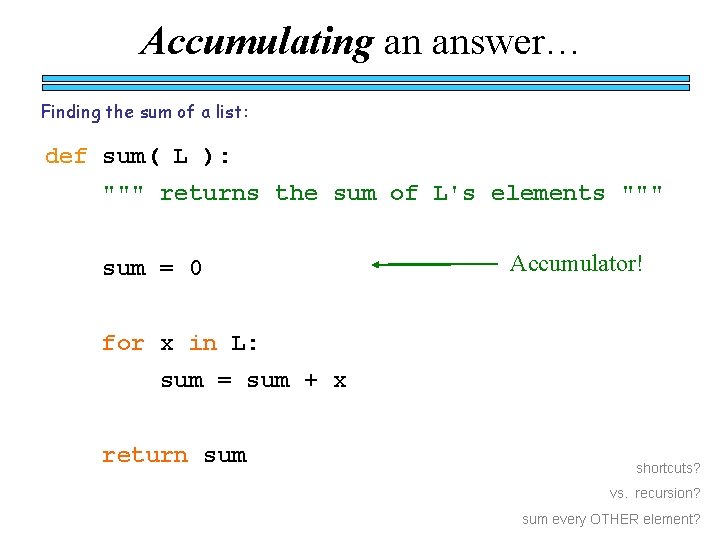
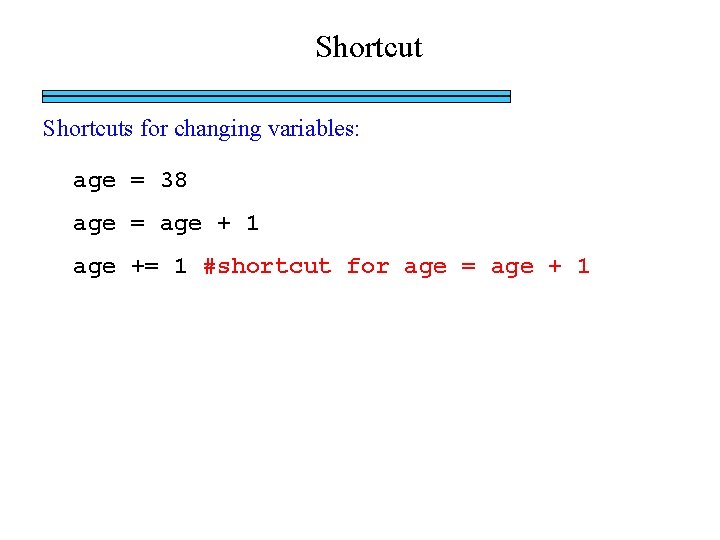
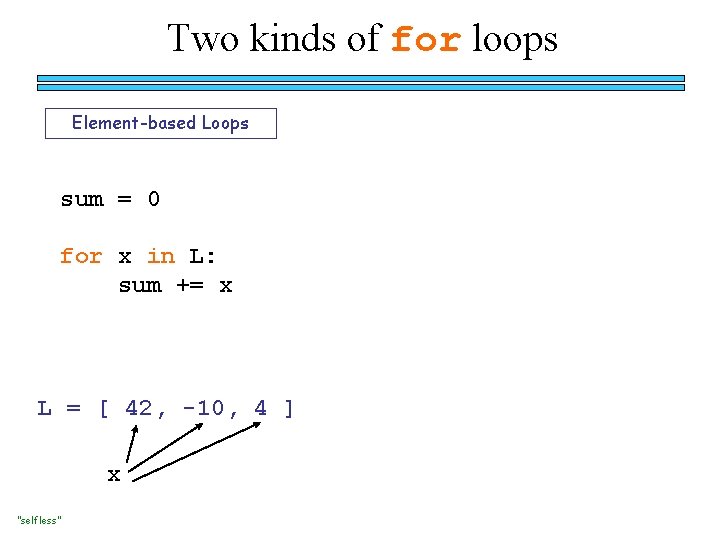
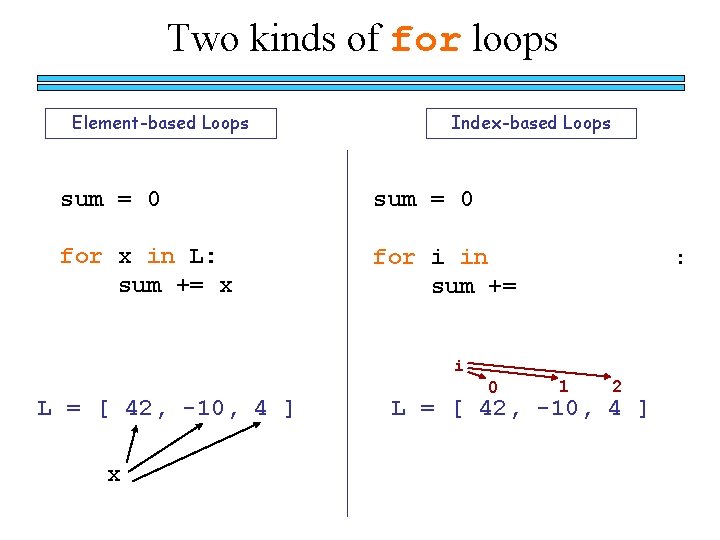
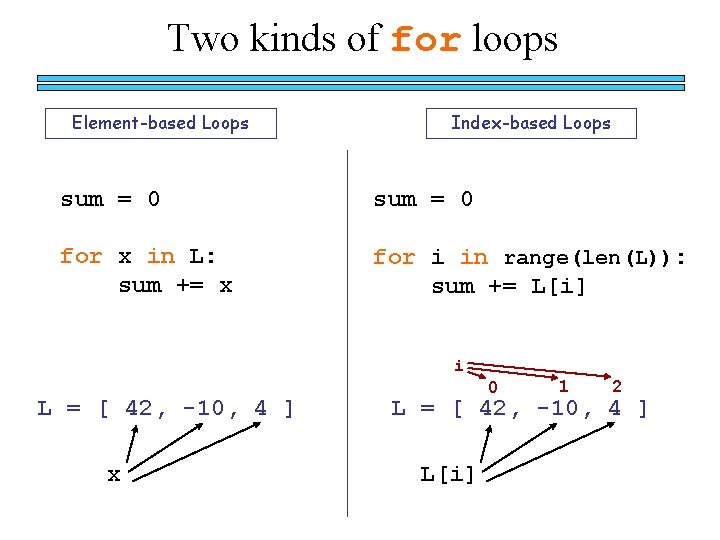
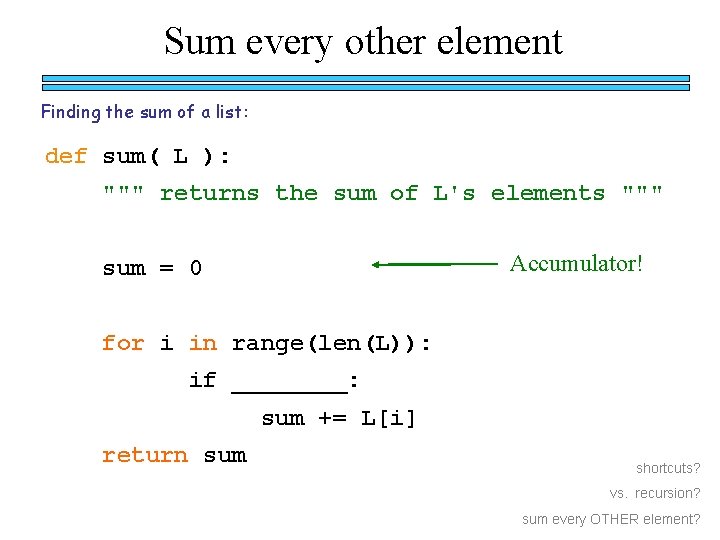
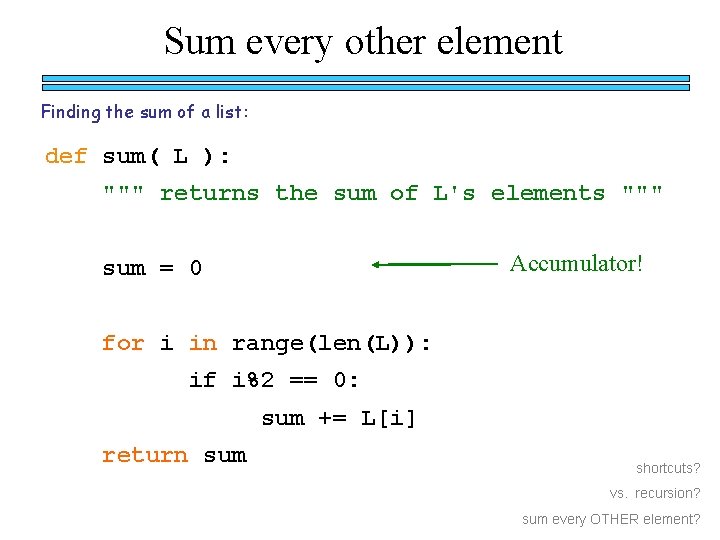
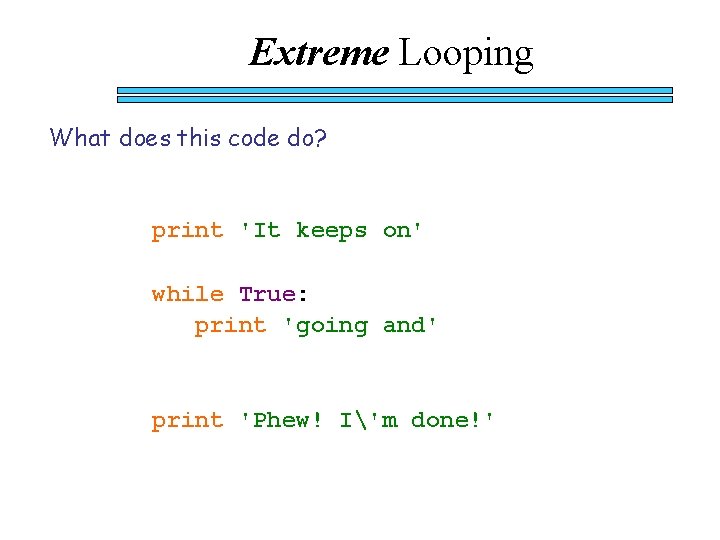
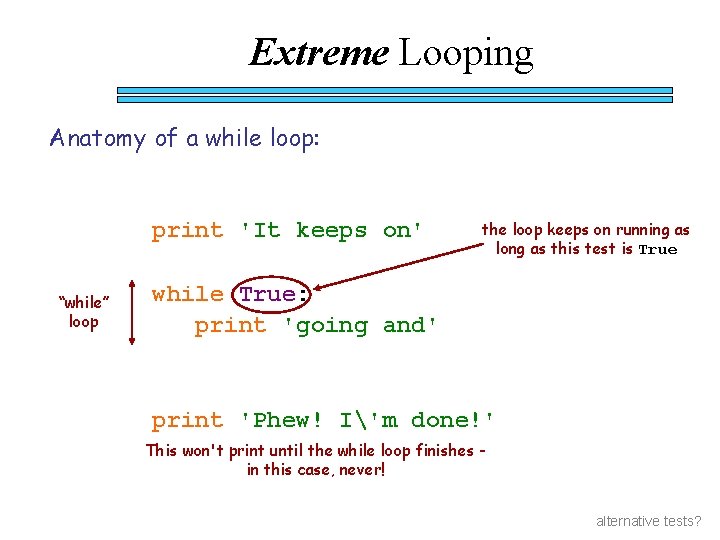
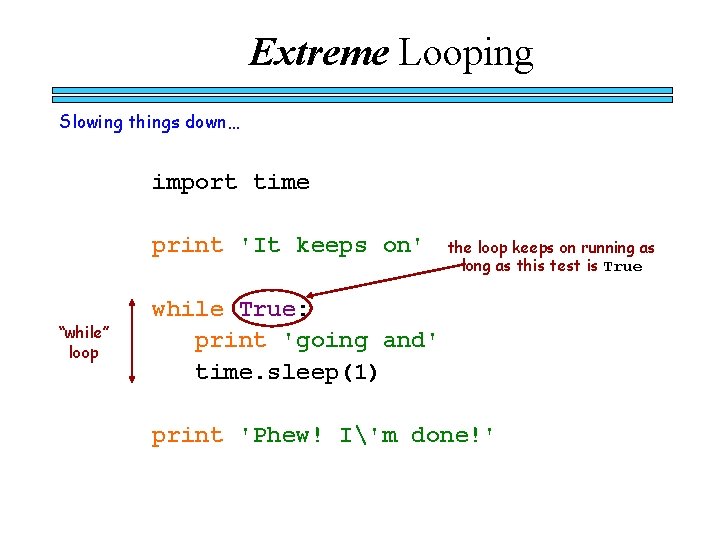
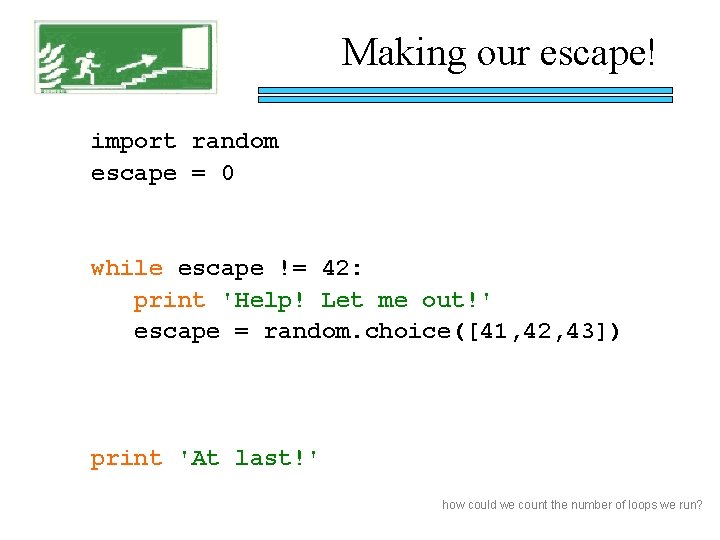
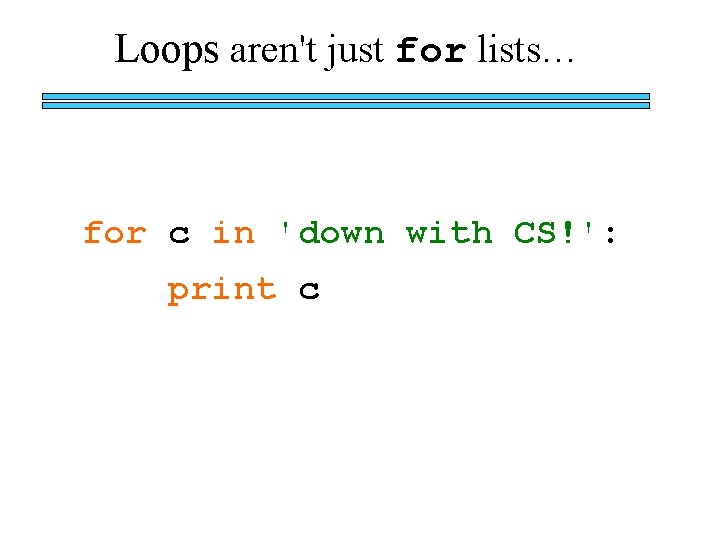
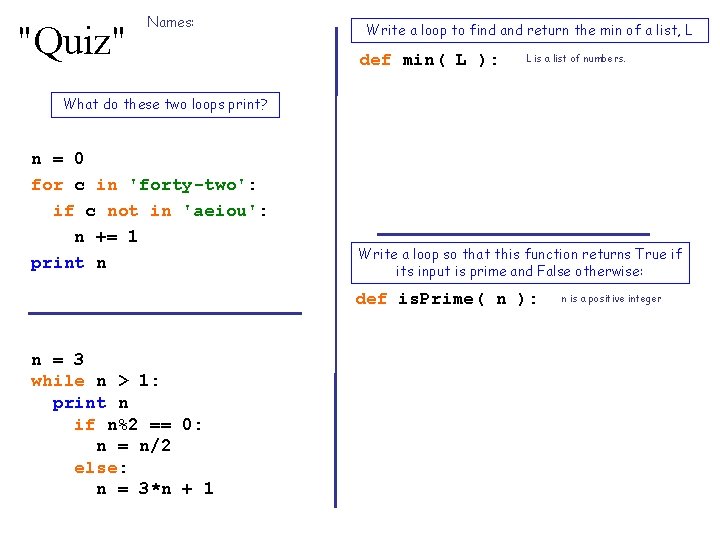
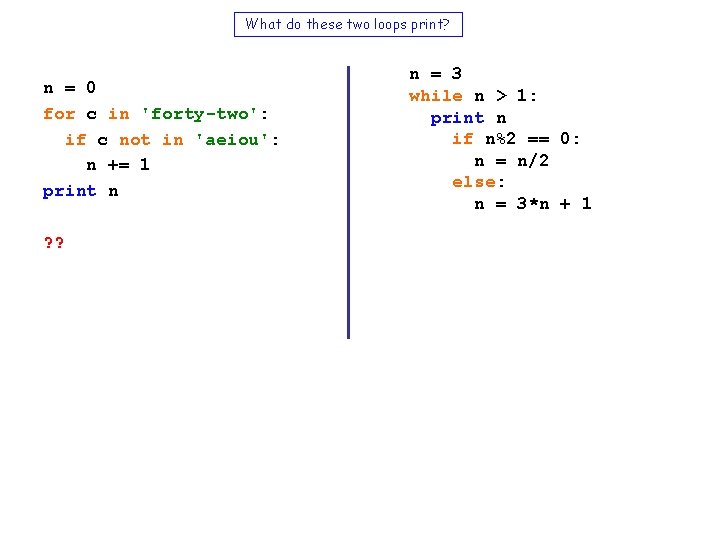
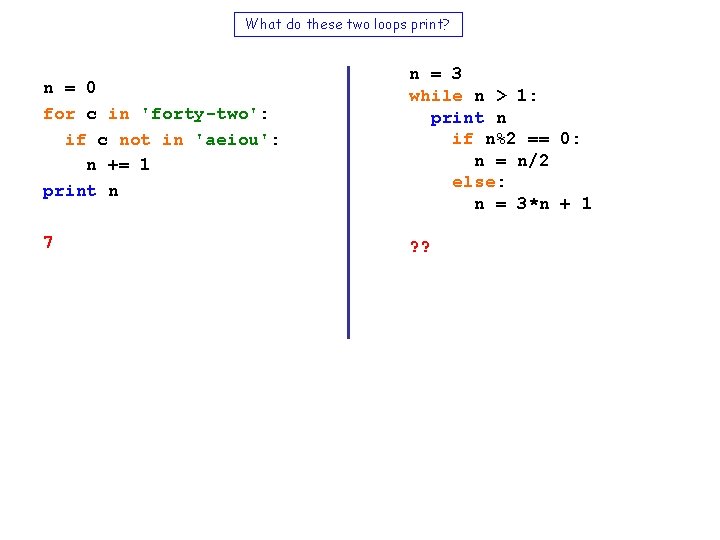
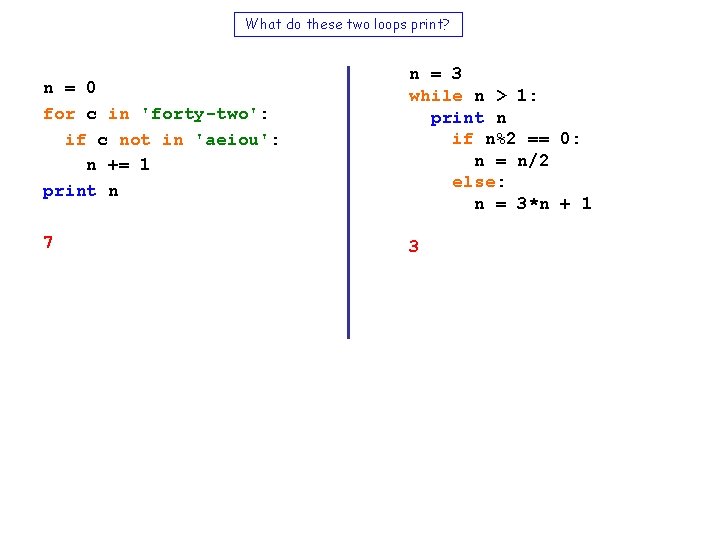
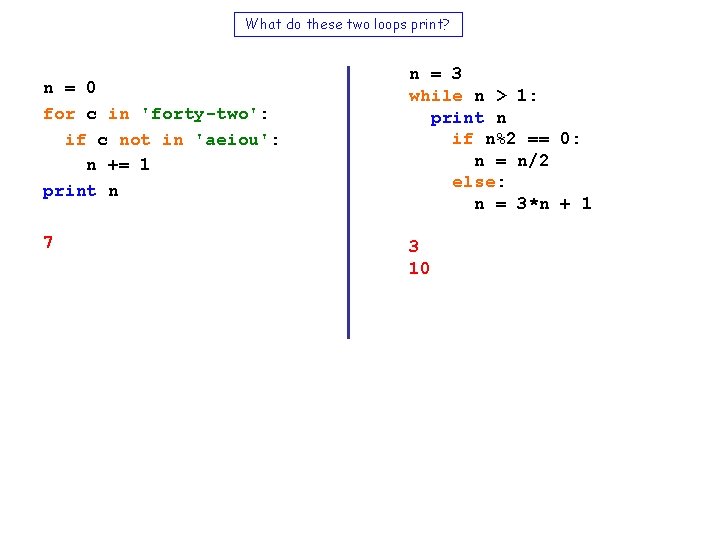
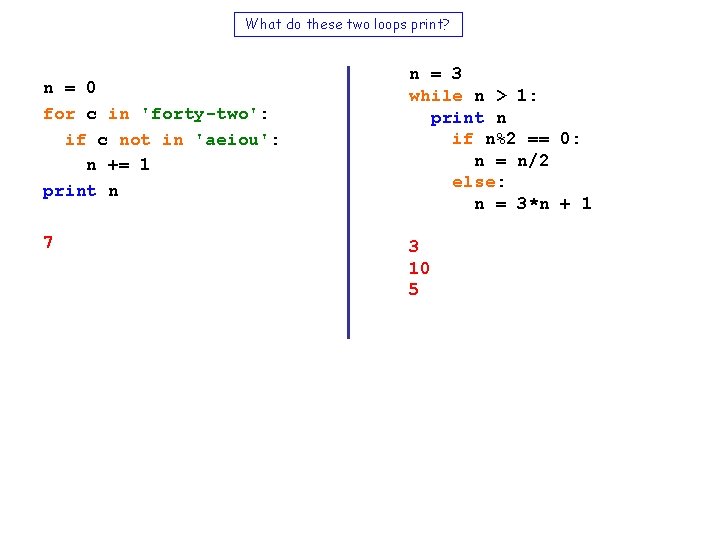
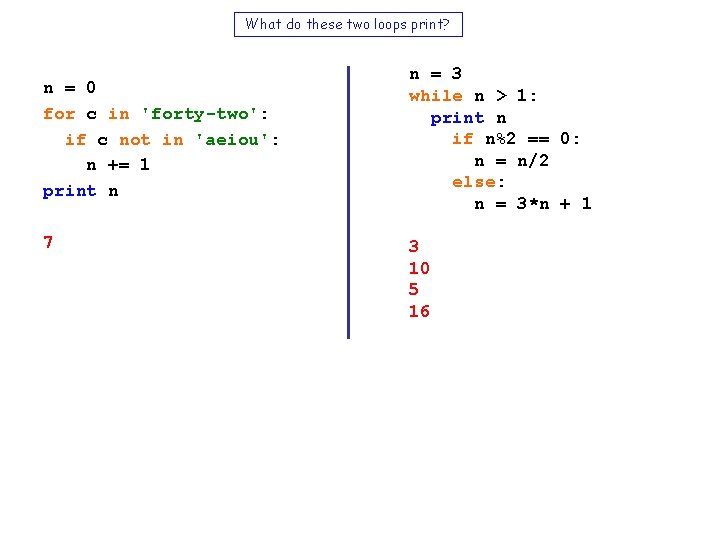
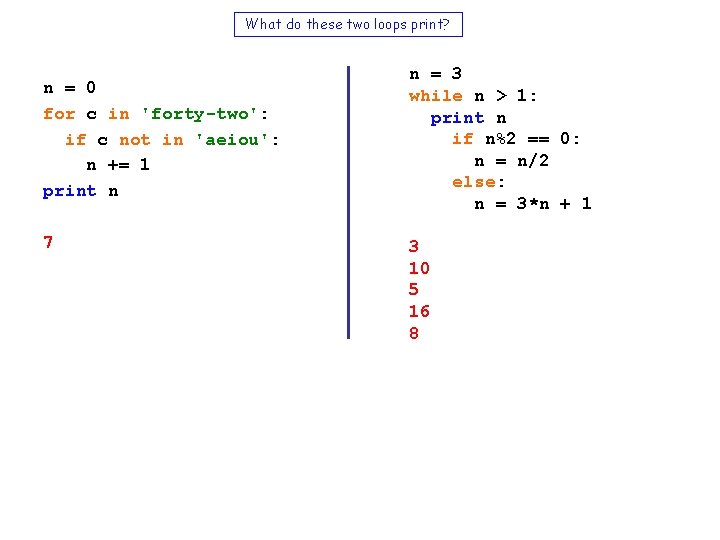
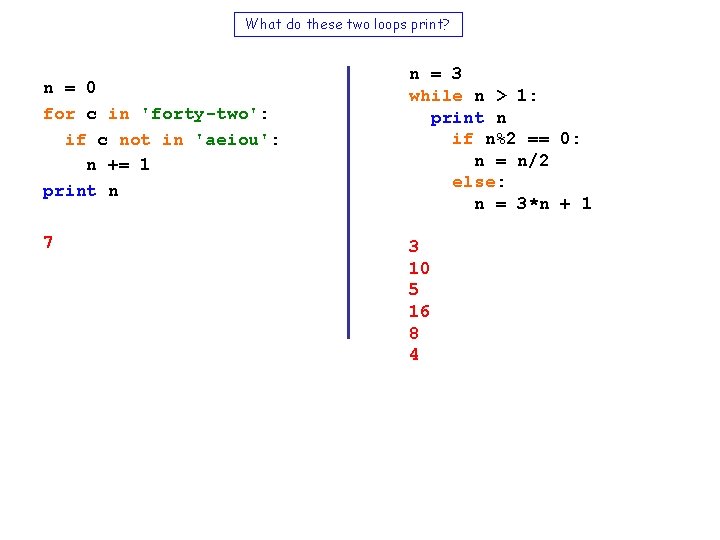
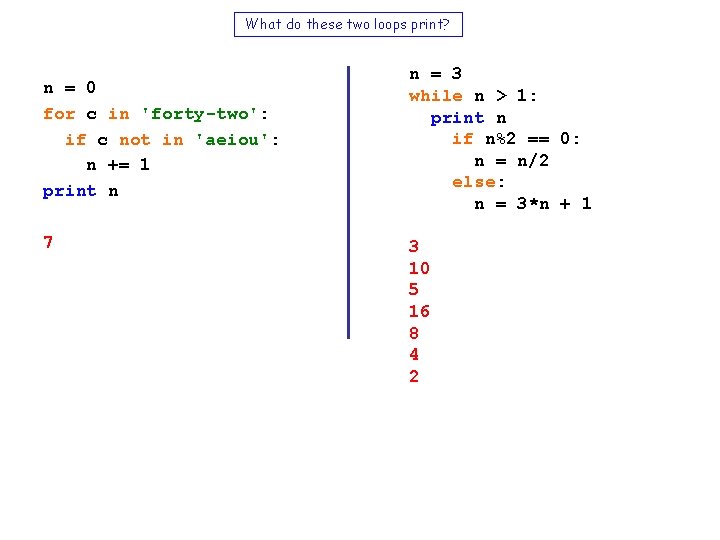
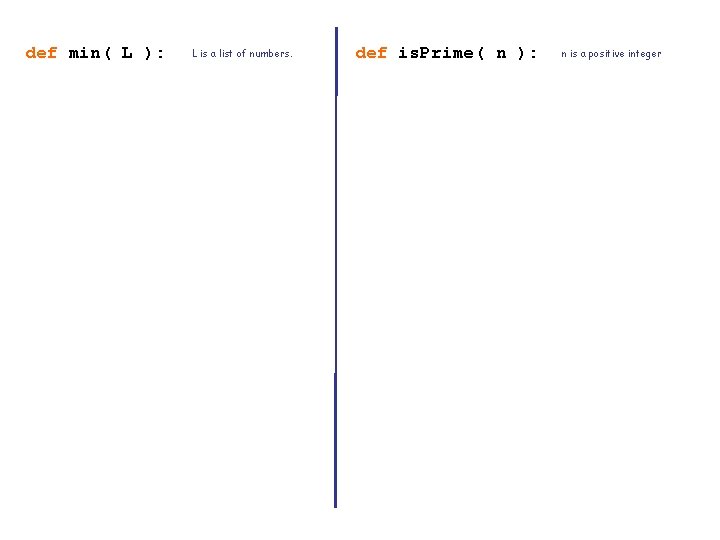
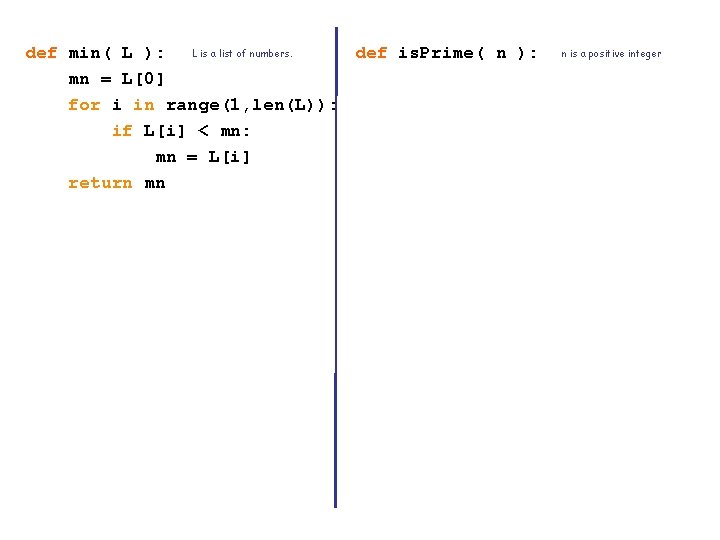
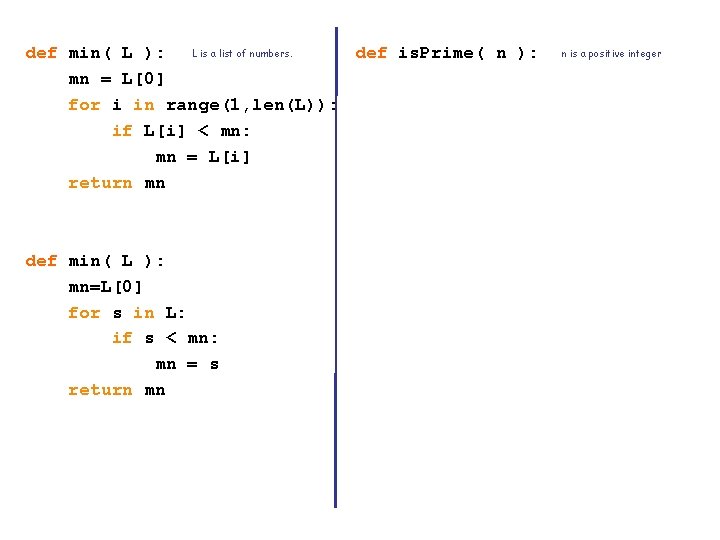
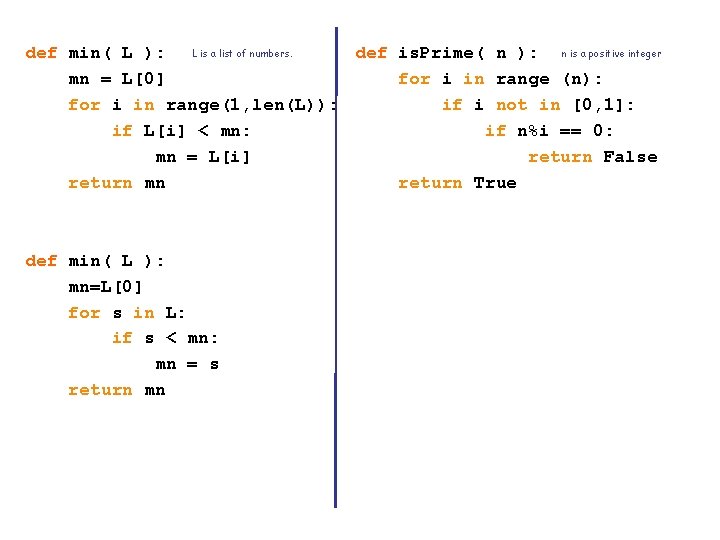
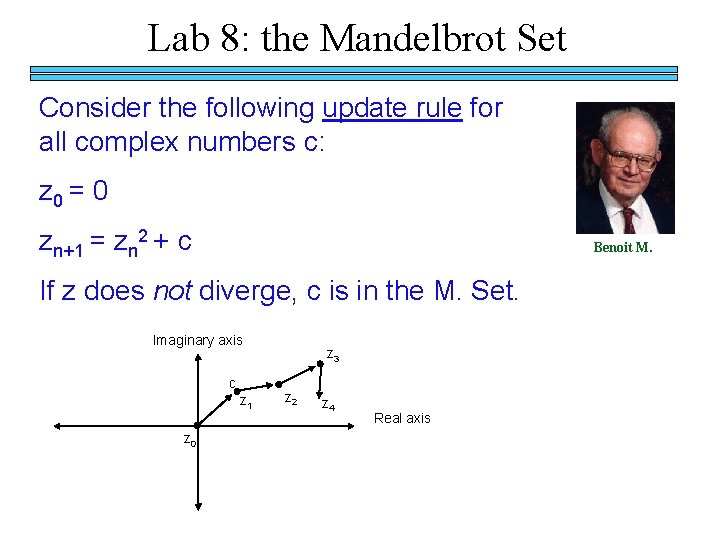
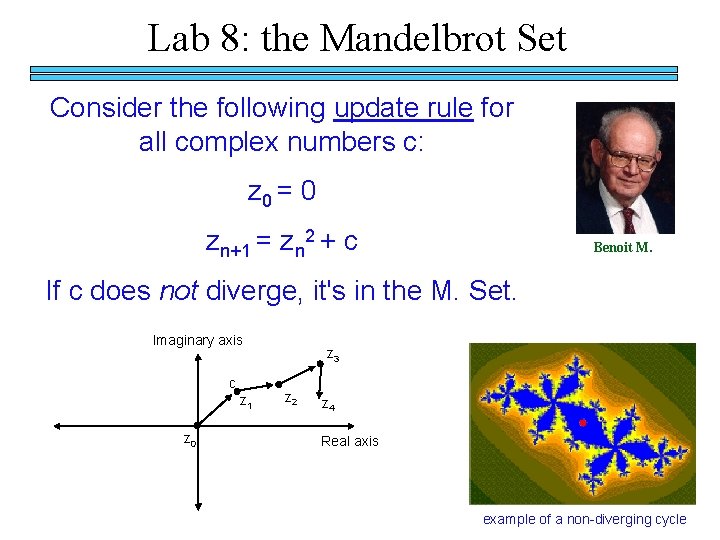
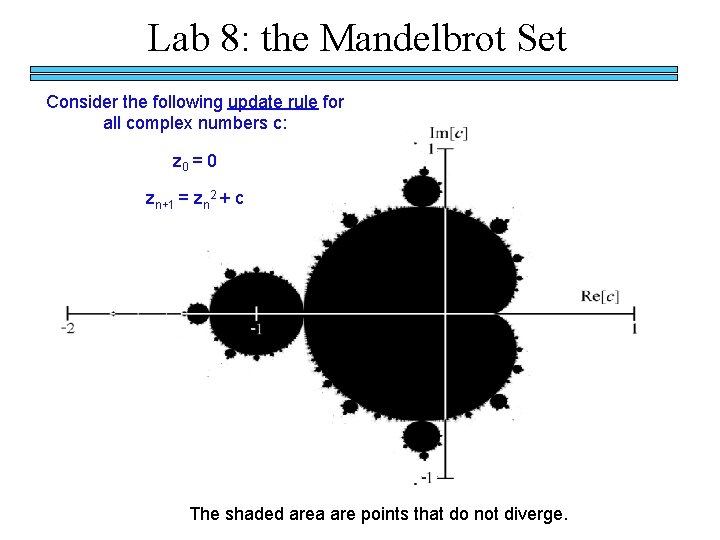
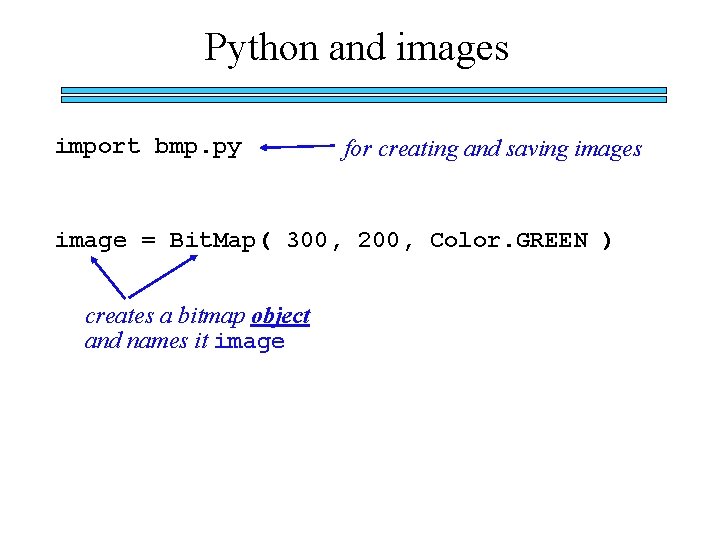
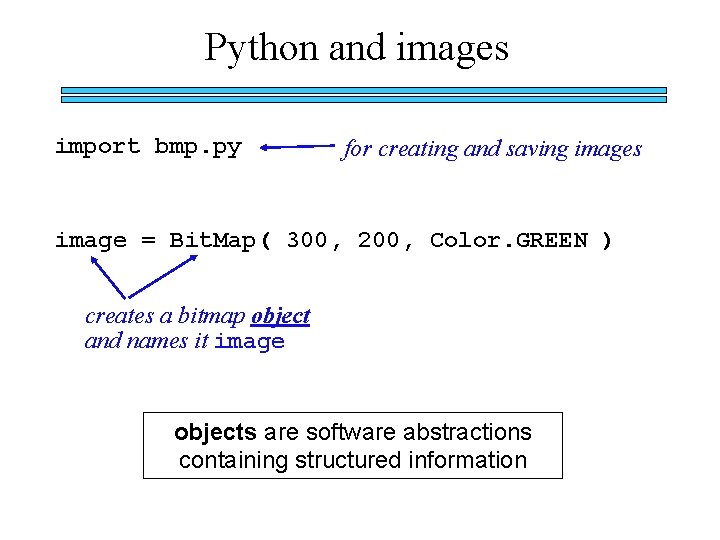
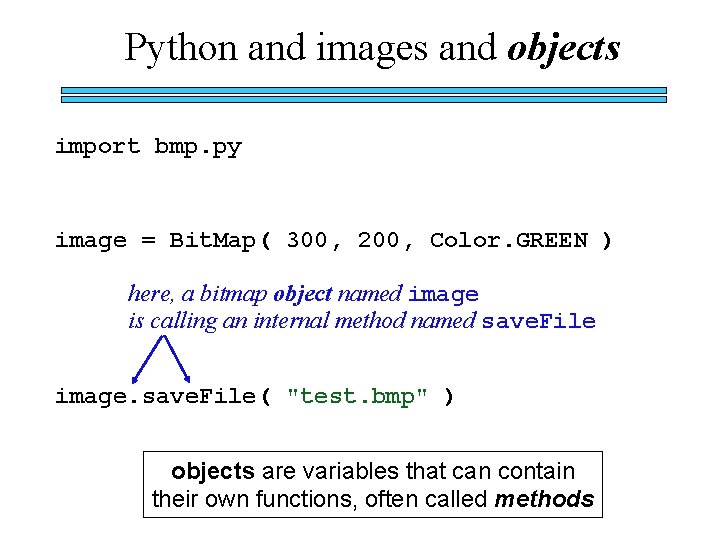
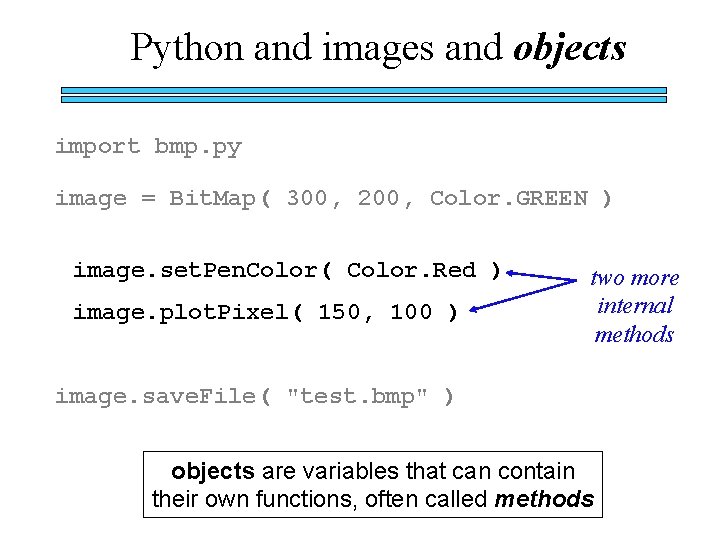
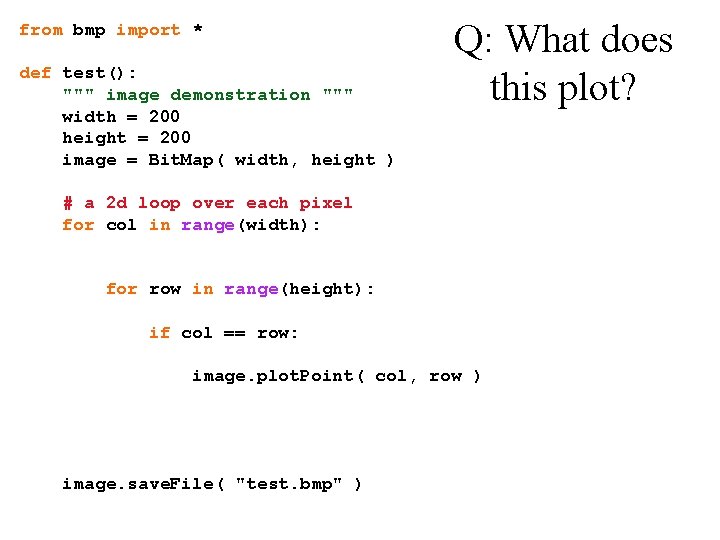
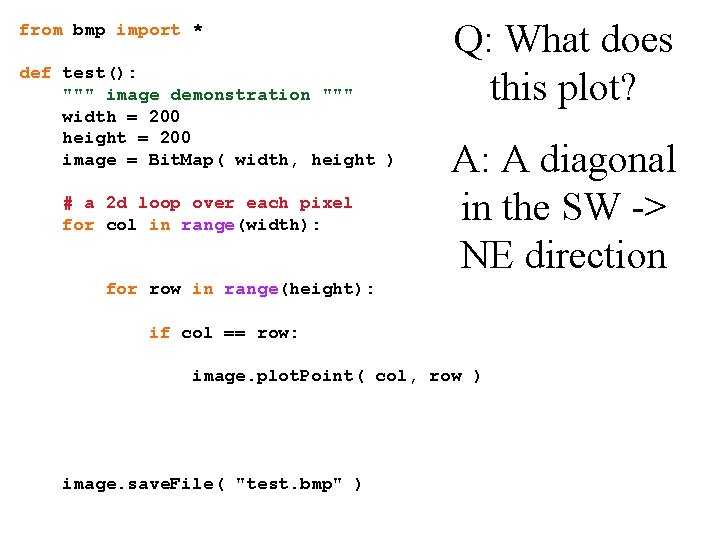
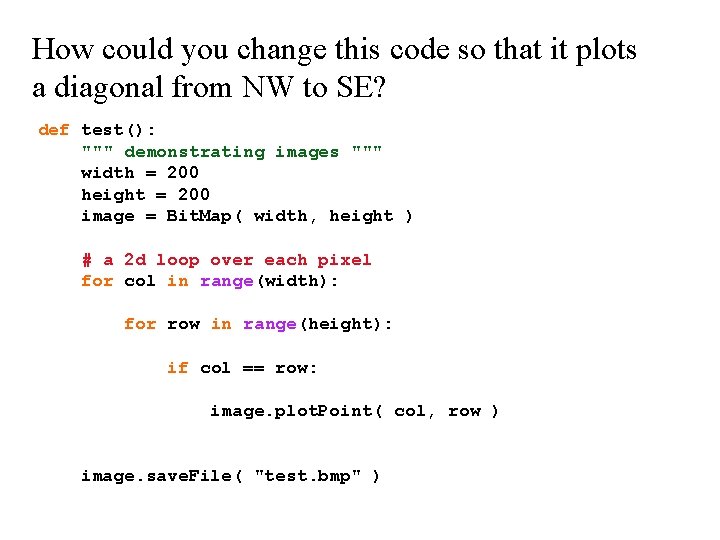
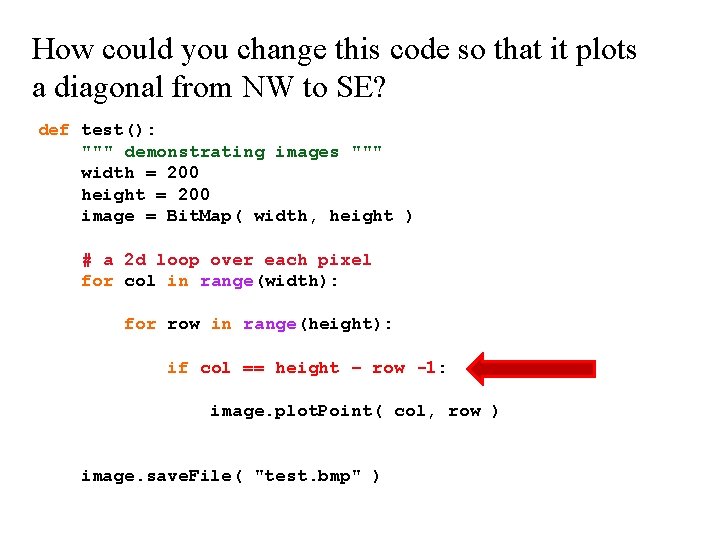
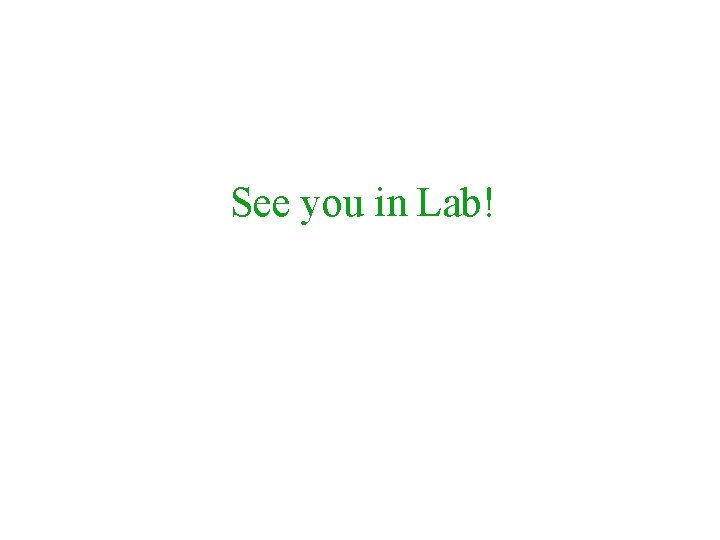
- Slides: 44
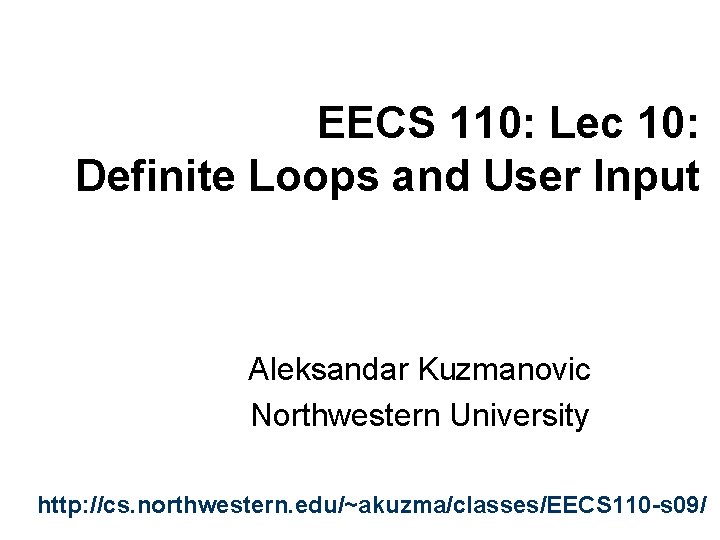
EECS 110: Lec 10: Definite Loops and User Input Aleksandar Kuzmanovic Northwestern University http: //cs. northwestern. edu/~akuzma/classes/EECS 110 -s 09/
![Loops Weve seen variables change inplace before x6 for x in range8 Loops! We've seen variables change in-place before: [ x*6 for x in range(8) ]](https://slidetodoc.com/presentation_image/e165a7de024e1f6aadca5d0080893cff/image-2.jpg)
Loops! We've seen variables change in-place before: [ x*6 for x in range(8) ] [ 0, 6, 12, 18, 24, 30, 36, 42 ] remember range?
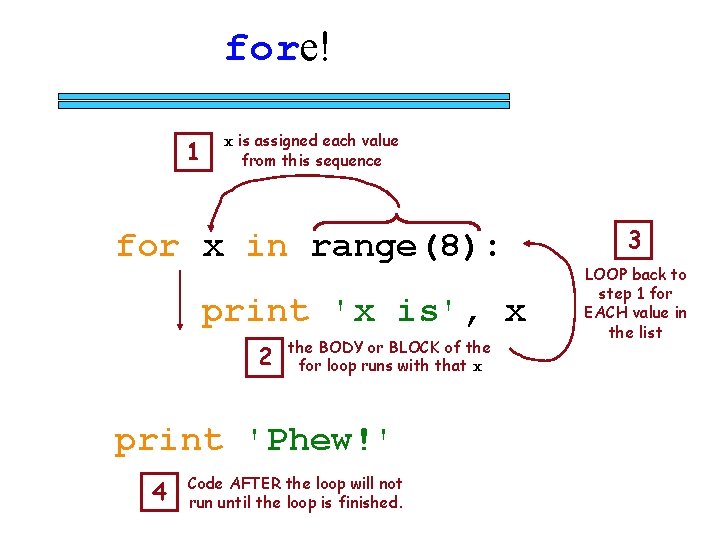
fore! 1 x is assigned each value from this sequence for x in range(8): print 'x is', x 2 the BODY or BLOCK of the for loop runs with that x print 'Phew!' 4 Code AFTER the loop will not run until the loop is finished. 3 LOOP back to step 1 for EACH value in the list
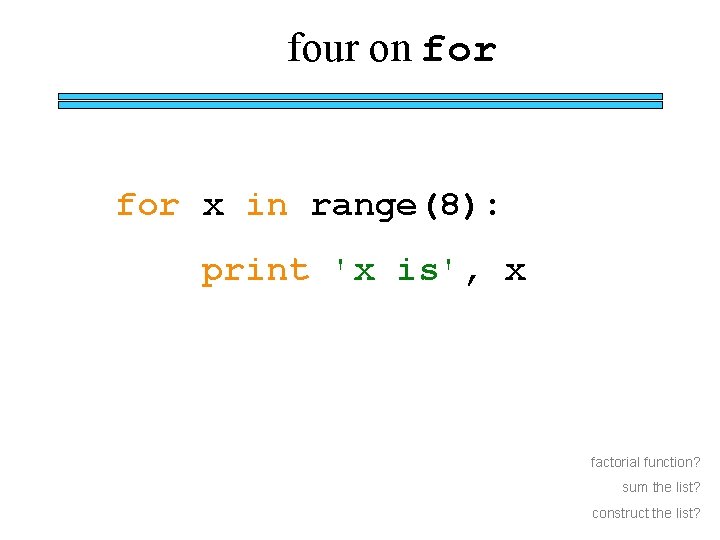
four on for x in range(8): print 'x is', x factorial function? sum the list? construct the list?
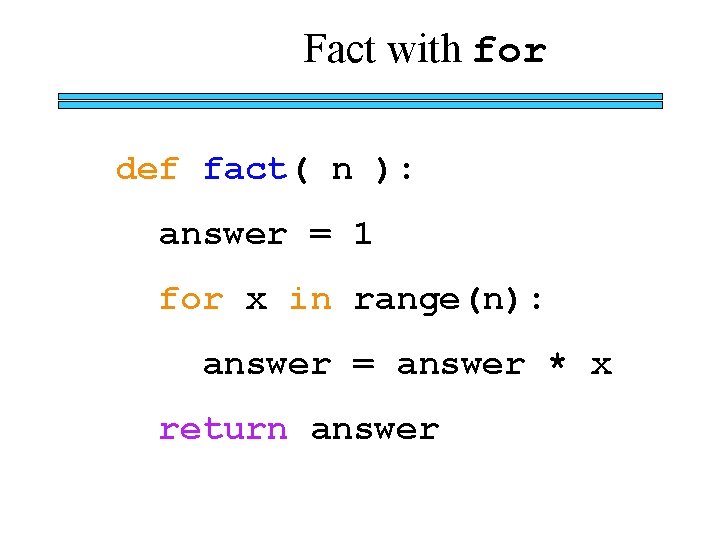
Fact with for def fact( n ): answer = 1 for x in range(n): answer = answer * x return answer
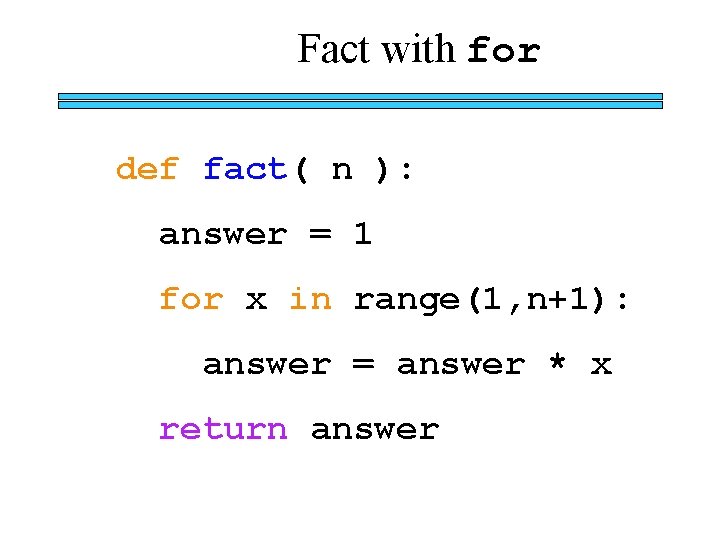
Fact with for def fact( n ): answer = 1 for x in range(1, n+1): answer = answer * x return answer
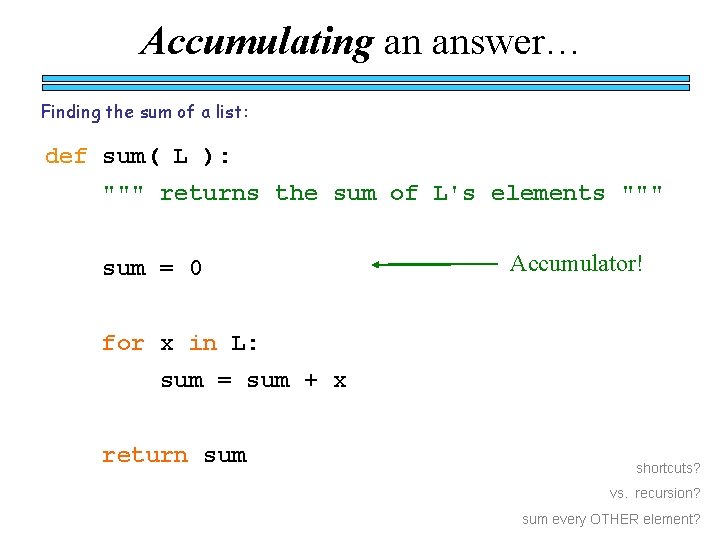
Accumulating an answer… Finding the sum of a list: def sum( L ): """ returns the sum of L's elements """ sum = 0 Accumulator! for x in L: sum = sum + x return sum shortcuts? vs. recursion? sum every OTHER element?
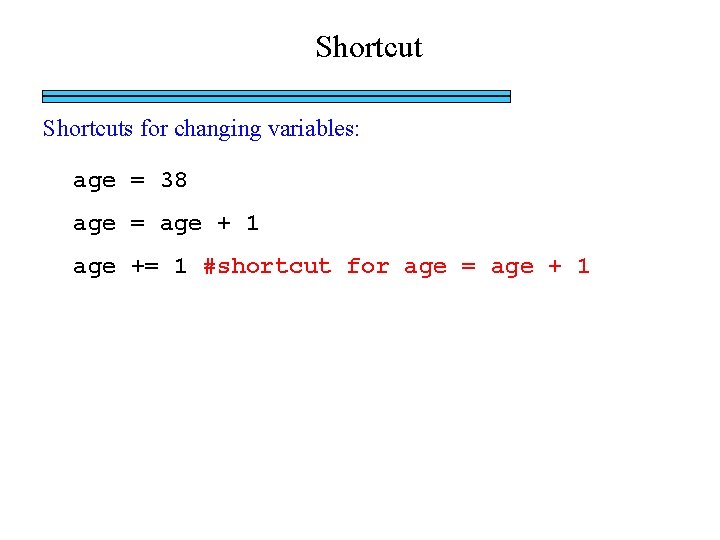
Shortcuts for changing variables: age = 38 age = age + 1 age += 1 #shortcut for age = age + 1
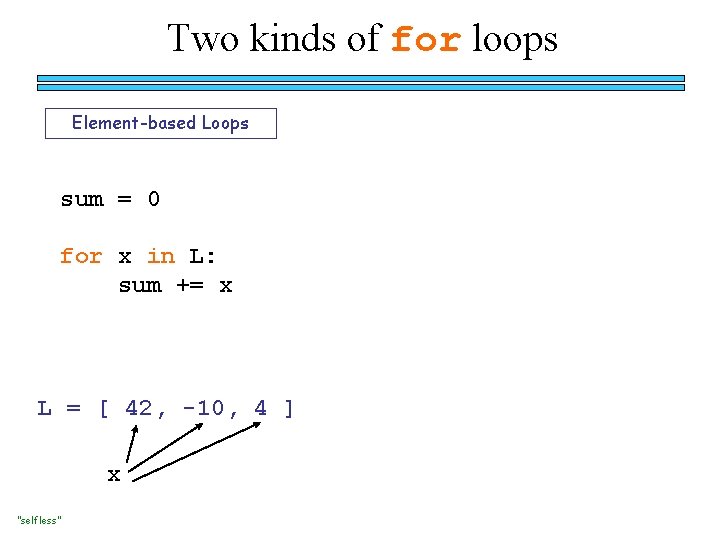
Two kinds of for loops Element-based Loops sum = 0 for x in L: sum += x L = [ 42, -10, 4 ] x "selfless"
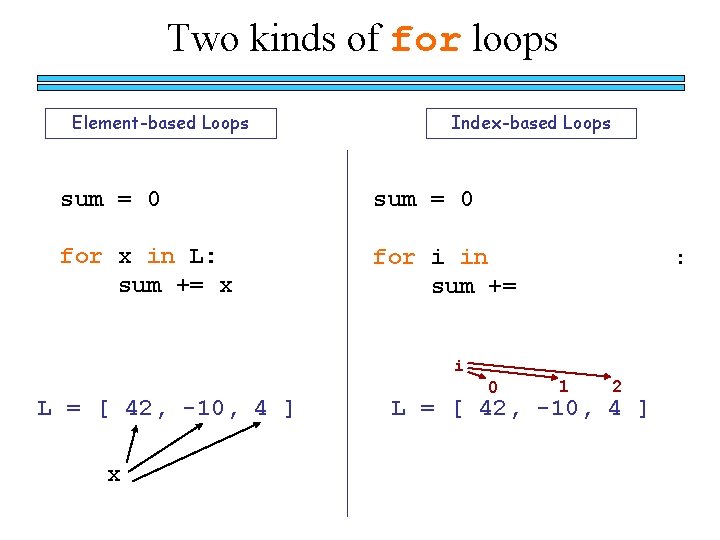
Two kinds of for loops Element-based Loops Index-based Loops sum = 0 for x in L: sum += x for i in sum += : i L = [ 42, -10, 4 ] x 0 1 2 L = [ 42, -10, 4 ]
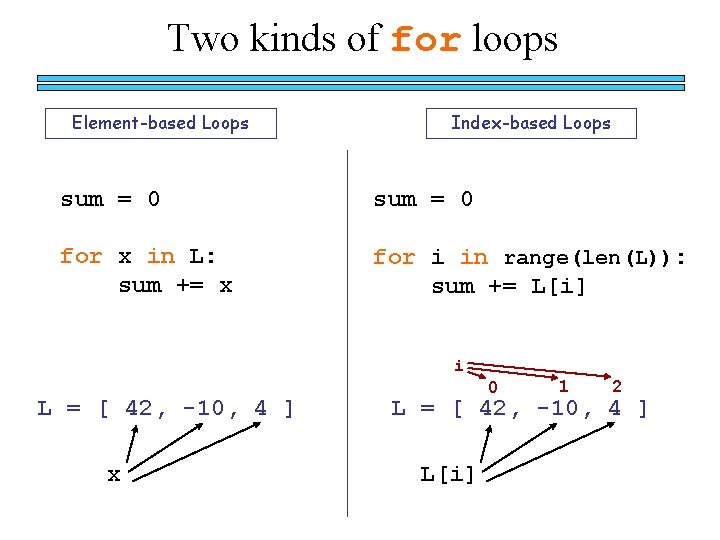
Two kinds of for loops Element-based Loops Index-based Loops sum = 0 for x in L: sum += x for i in range(len(L)): sum += L[i] i L = [ 42, -10, 4 ] x 0 1 2 L = [ 42, -10, 4 ] L[i]
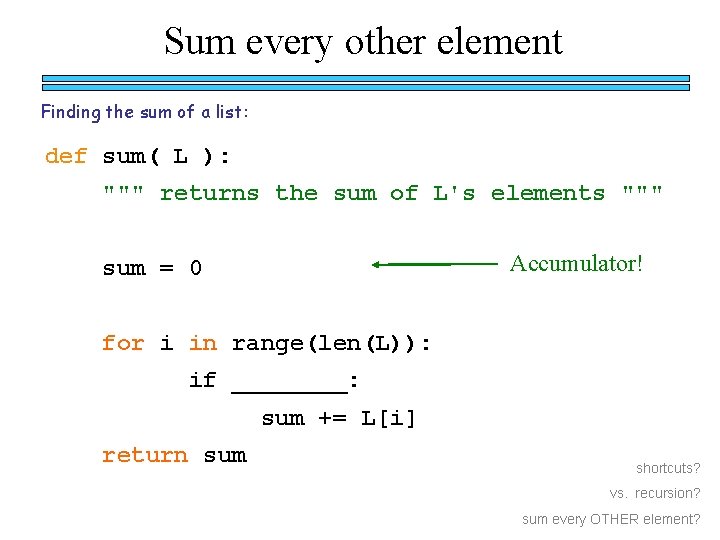
Sum every other element Finding the sum of a list: def sum( L ): """ returns the sum of L's elements """ Accumulator! sum = 0 for i in range(len(L)): if ____: sum += L[i] return sum shortcuts? vs. recursion? sum every OTHER element?
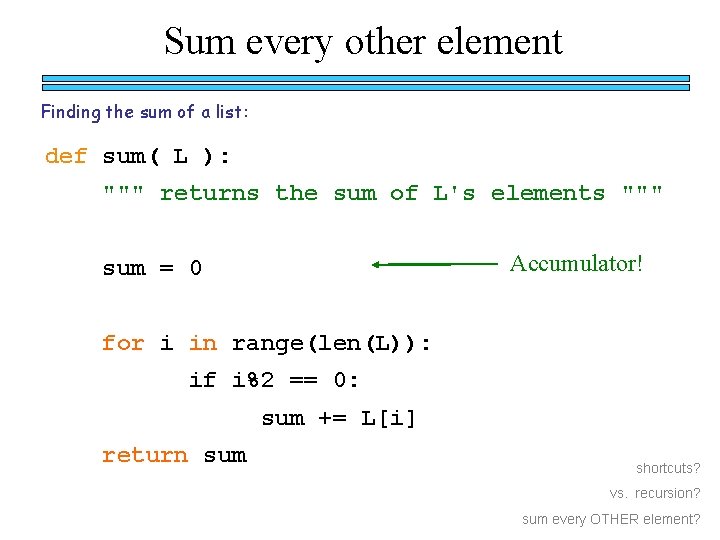
Sum every other element Finding the sum of a list: def sum( L ): """ returns the sum of L's elements """ Accumulator! sum = 0 for i in range(len(L)): if i%2 == 0: sum += L[i] return sum shortcuts? vs. recursion? sum every OTHER element?
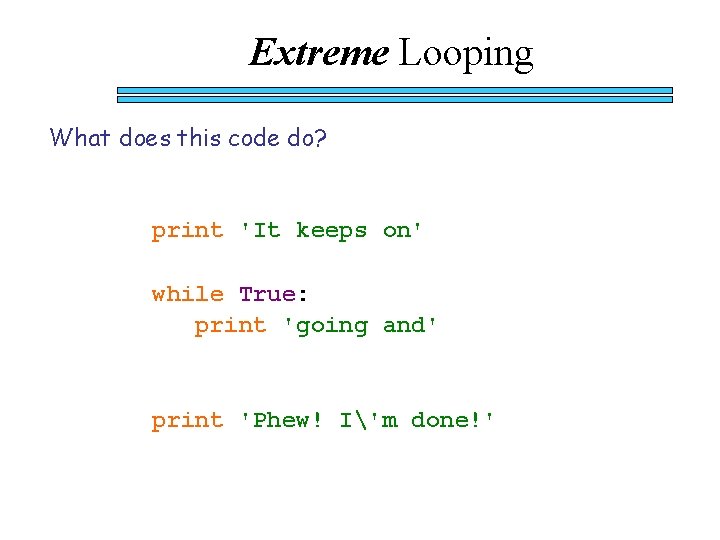
Extreme Looping What does this code do? print 'It keeps on' while True: print 'going and' print 'Phew! I'm done!'
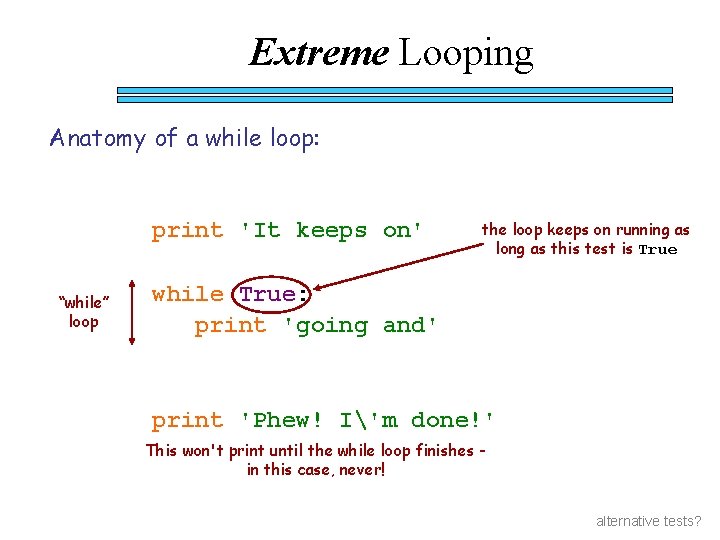
Extreme Looping Anatomy of a while loop: print 'It keeps on' “while” loop the loop keeps on running as long as this test is True while True: print 'going and' print 'Phew! I'm done!' This won't print until the while loop finishes in this case, never! alternative tests?
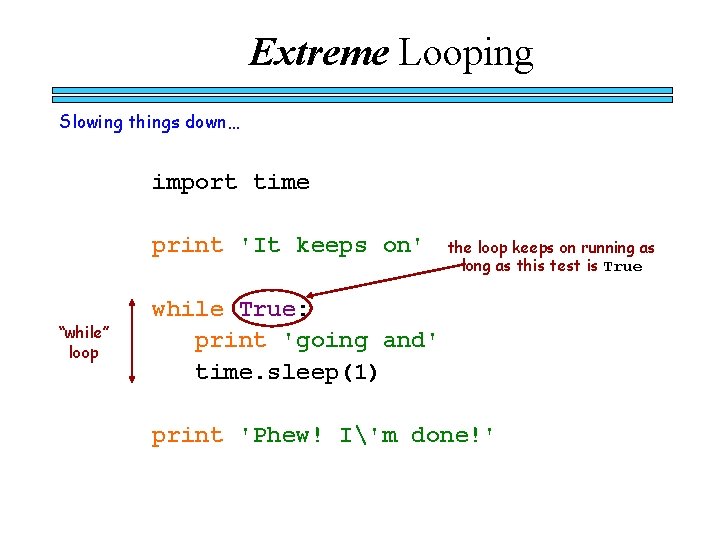
Extreme Looping Slowing things down… import time print 'It keeps on' “while” loop the loop keeps on running as long as this test is True while True: print 'going and' time. sleep(1) print 'Phew! I'm done!'
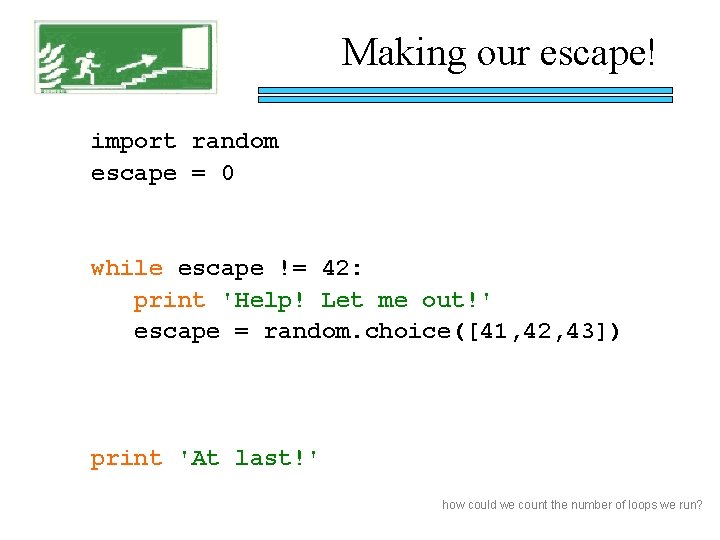
Making our escape! import random escape = 0 while escape != 42: print 'Help! Let me out!' escape = random. choice([41, 42, 43]) print 'At last!' how could we count the number of loops we run?
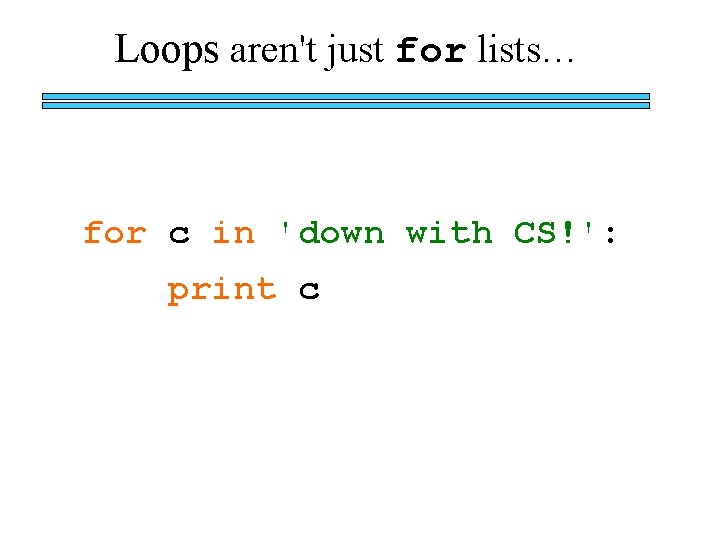
Loops aren't just for lists… for c in 'down with CS!': print c
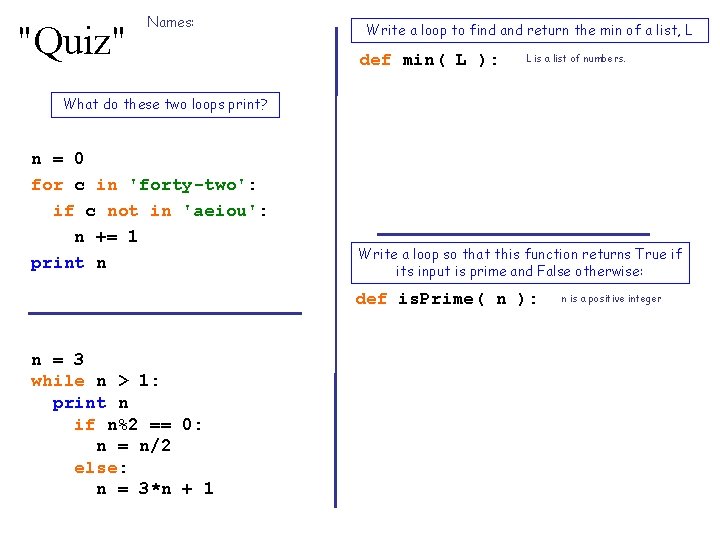
"Quiz" Names: Write a loop to find and return the min of a list, L def min( L ): L is a list of numbers. What do these two loops print? n = 0 for c in 'forty-two': if c not in 'aeiou': n += 1 print n Write a loop so that this function returns True if its input is prime and False otherwise: def is. Prime( n ): n = 3 while n > 1: print n if n%2 == 0: n = n/2 else: n = 3*n + 1 n is a positive integer
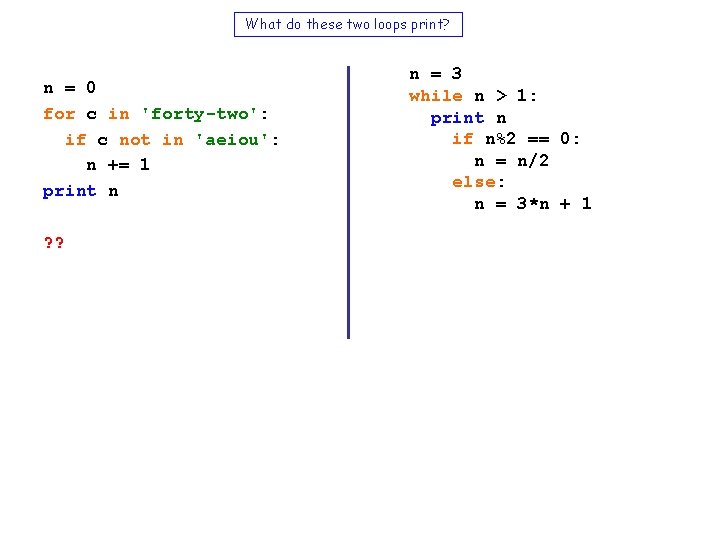
What do these two loops print? n = 0 for c in 'forty-two': if c not in 'aeiou': n += 1 print n ? ? n = 3 while n > 1: print n if n%2 == 0: n = n/2 else: n = 3*n + 1
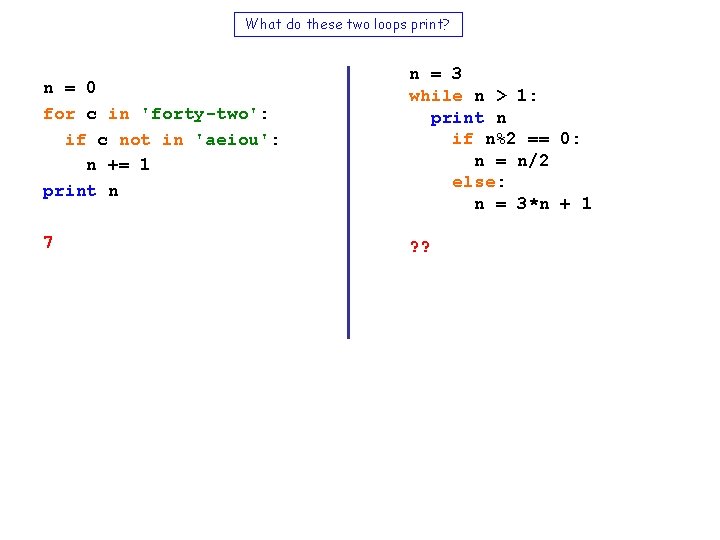
What do these two loops print? n = 0 for c in 'forty-two': if c not in 'aeiou': n += 1 print n n = 3 while n > 1: print n if n%2 == 0: n = n/2 else: n = 3*n + 1 7 ? ?
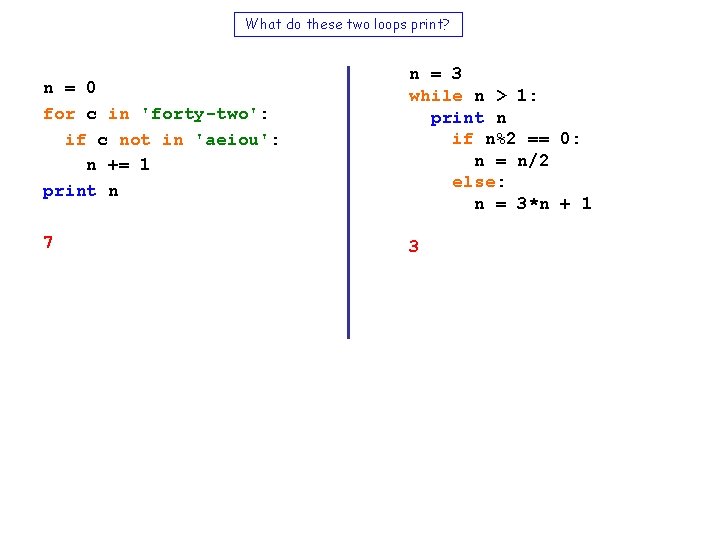
What do these two loops print? n = 0 for c in 'forty-two': if c not in 'aeiou': n += 1 print n n = 3 while n > 1: print n if n%2 == 0: n = n/2 else: n = 3*n + 1 7 3
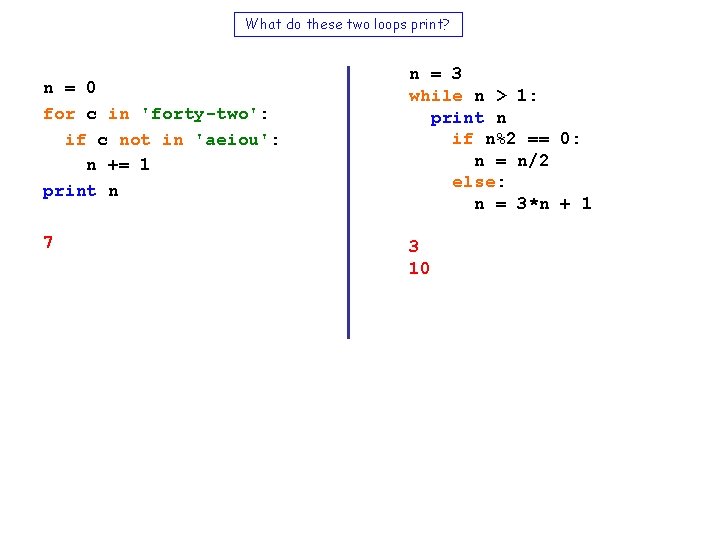
What do these two loops print? n = 0 for c in 'forty-two': if c not in 'aeiou': n += 1 print n 7 n = 3 while n > 1: print n if n%2 == 0: n = n/2 else: n = 3*n + 1 3 10
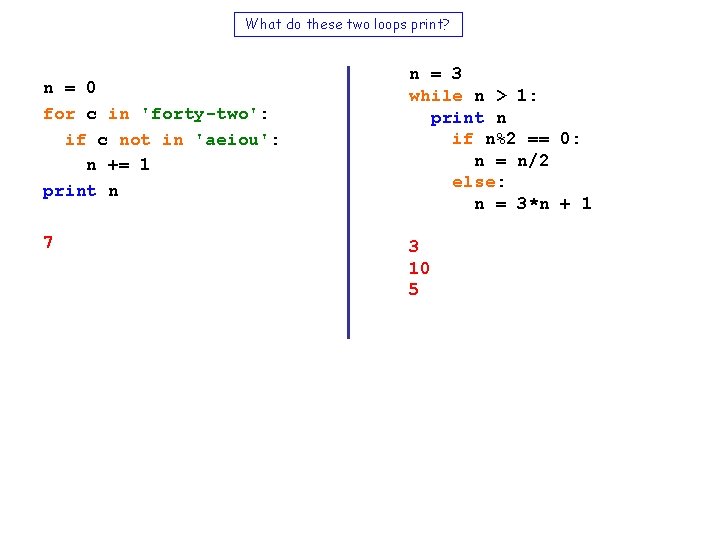
What do these two loops print? n = 0 for c in 'forty-two': if c not in 'aeiou': n += 1 print n 7 n = 3 while n > 1: print n if n%2 == 0: n = n/2 else: n = 3*n + 1 3 10 5
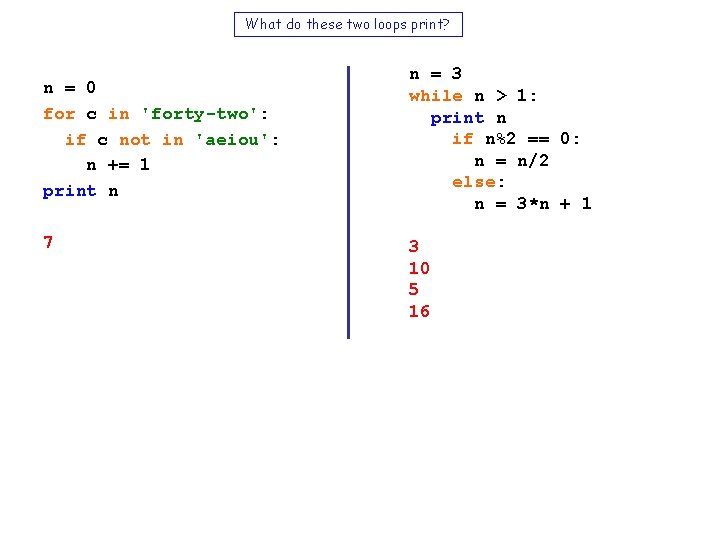
What do these two loops print? n = 0 for c in 'forty-two': if c not in 'aeiou': n += 1 print n 7 n = 3 while n > 1: print n if n%2 == 0: n = n/2 else: n = 3*n + 1 3 10 5 16
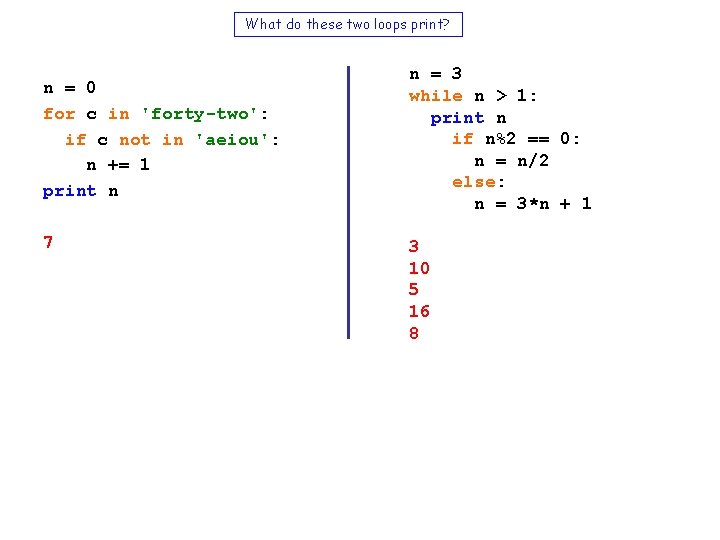
What do these two loops print? n = 0 for c in 'forty-two': if c not in 'aeiou': n += 1 print n 7 n = 3 while n > 1: print n if n%2 == 0: n = n/2 else: n = 3*n + 1 3 10 5 16 8
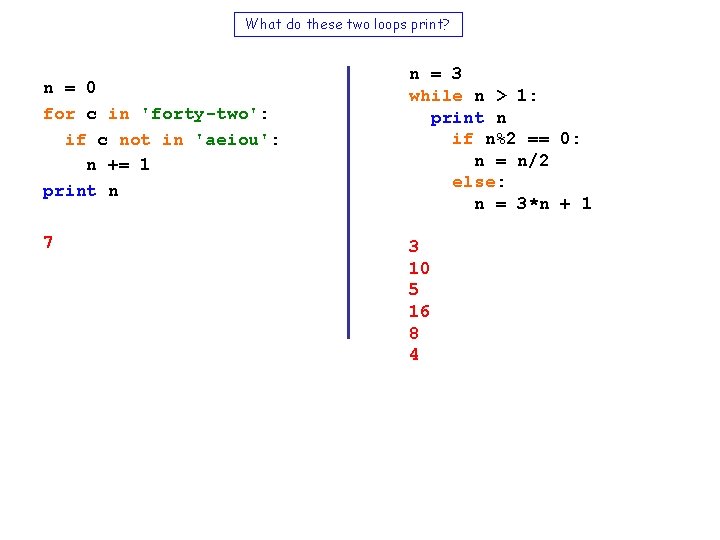
What do these two loops print? n = 0 for c in 'forty-two': if c not in 'aeiou': n += 1 print n 7 n = 3 while n > 1: print n if n%2 == 0: n = n/2 else: n = 3*n + 1 3 10 5 16 8 4
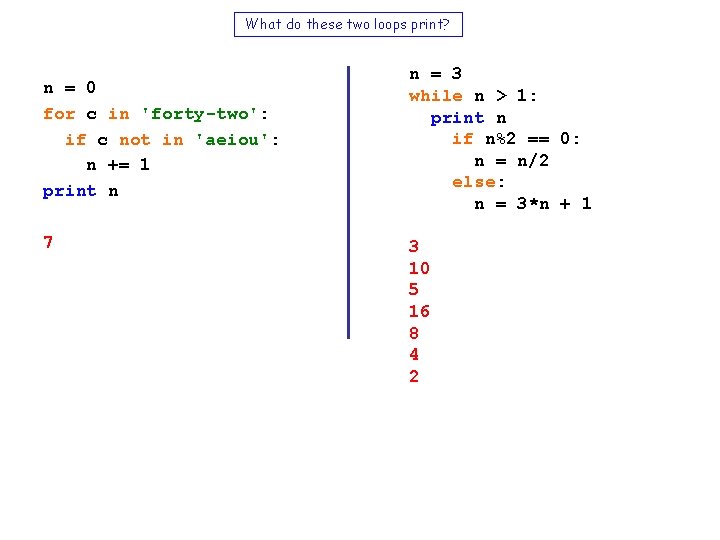
What do these two loops print? n = 0 for c in 'forty-two': if c not in 'aeiou': n += 1 print n 7 n = 3 while n > 1: print n if n%2 == 0: n = n/2 else: n = 3*n + 1 3 10 5 16 8 4 2
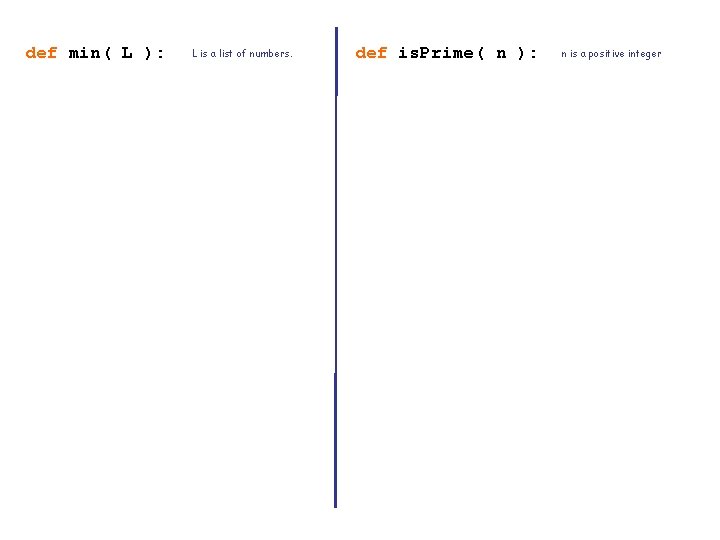
def min( L ): L is a list of numbers. def is. Prime( n ): n is a positive integer
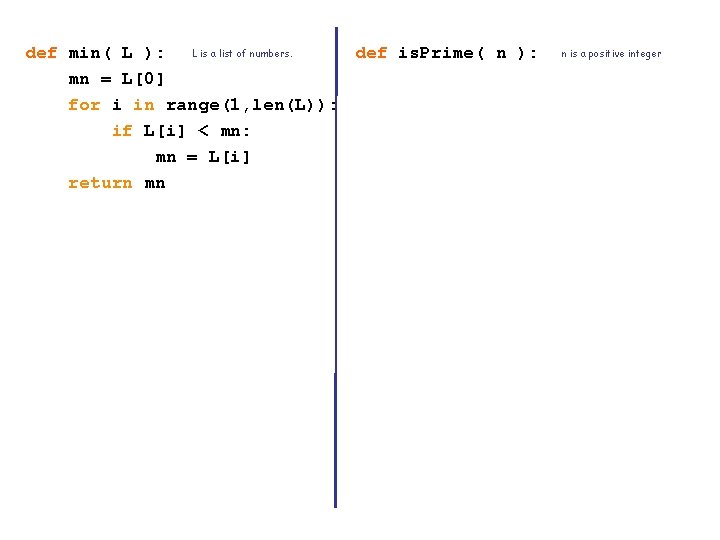
def min( L ): L is a list of numbers. def is. Prime( n ): mn = L[0] for i in range(1, len(L)): if L[i] < mn: mn = L[i] return mn n is a positive integer
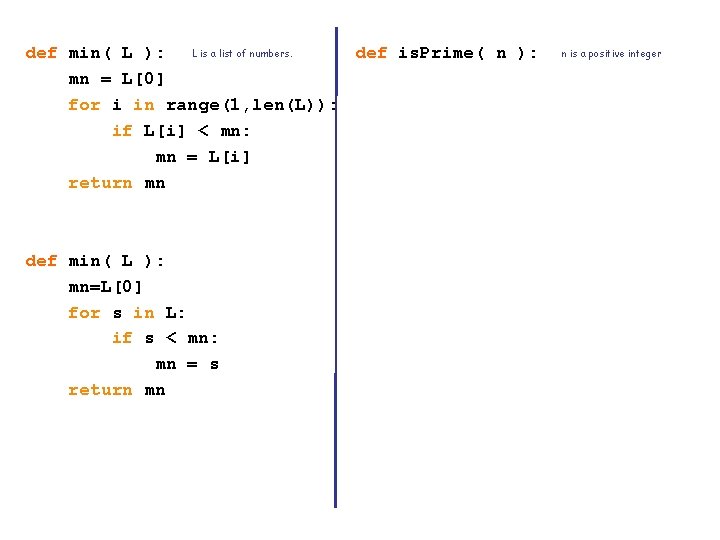
def is. Prime( n ): def min( L ): L is a list of numbers. mn = L[0] for i in range(1, len(L)): if L[i] < mn: mn = L[i] return mn def min( L ): mn=L[0] for s in L: if s < mn: mn = s return mn n is a positive integer
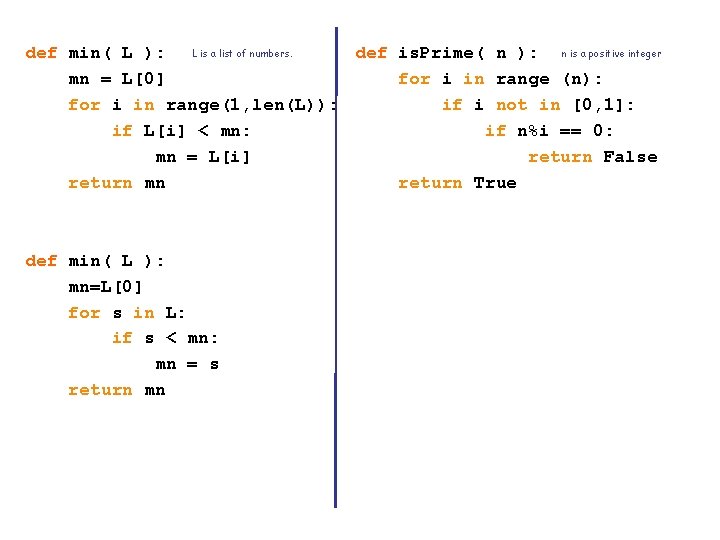
def is. Prime( n ): n is a positive integer def min( L ): L is a list of numbers. for i in range (n): mn = L[0] if i not in [0, 1]: for i in range(1, len(L)): if n%i == 0: if L[i] < mn: return False mn = L[i] return True return mn def min( L ): mn=L[0] for s in L: if s < mn: mn = s return mn
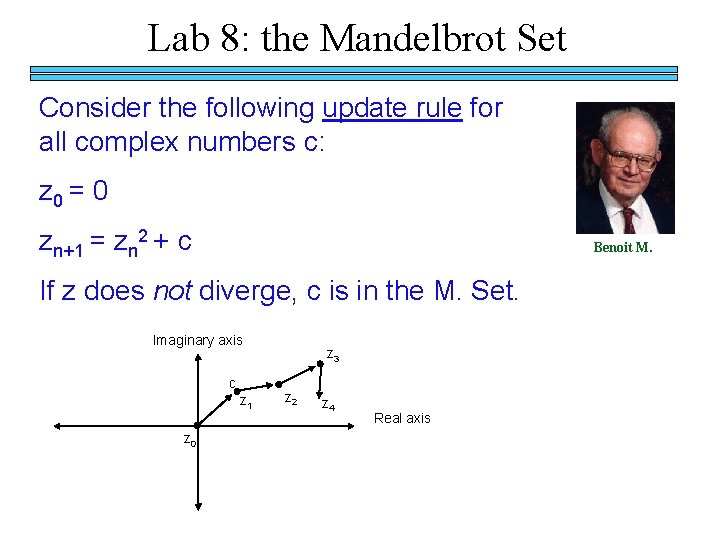
Lab 8: the Mandelbrot Set Consider the following update rule for all complex numbers c: z 0 = 0 zn+1 = zn 2 + c Benoit M. If z does not diverge, c is in the M. Set. Imaginary axis c z 1 z 0 z 3 z 2 z 4 Real axis
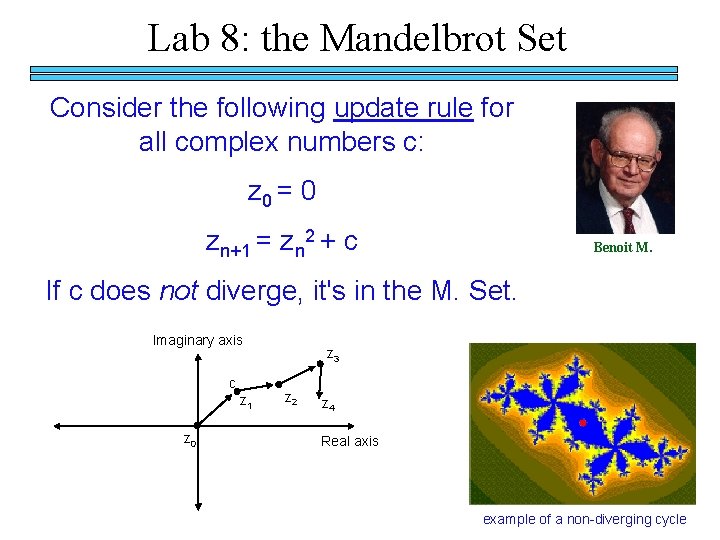
Lab 8: the Mandelbrot Set Consider the following update rule for all complex numbers c: z 0 = 0 zn+1 = zn 2 + c Benoit M. If c does not diverge, it's in the M. Set. Imaginary axis c z 1 z 0 z 3 z 2 z 4 Real axis example of a non-diverging cycle
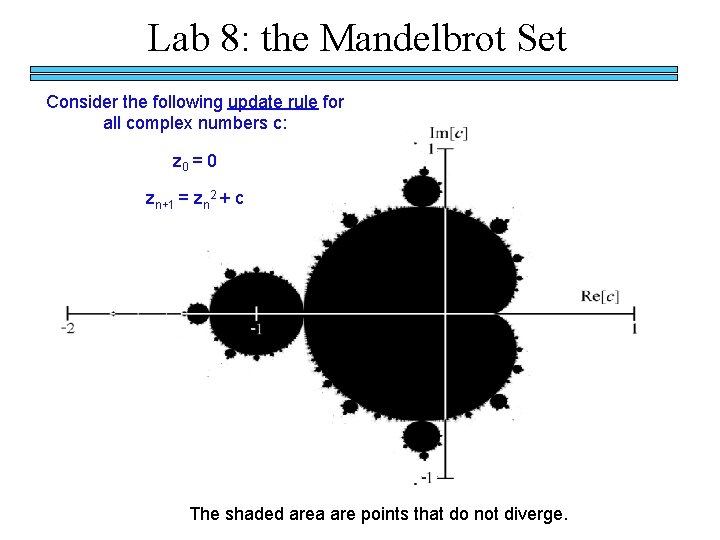
Lab 8: the Mandelbrot Set Consider the following update rule for all complex numbers c: z 0 = 0 zn+1 = zn 2 + c The shaded area are points that do not diverge.
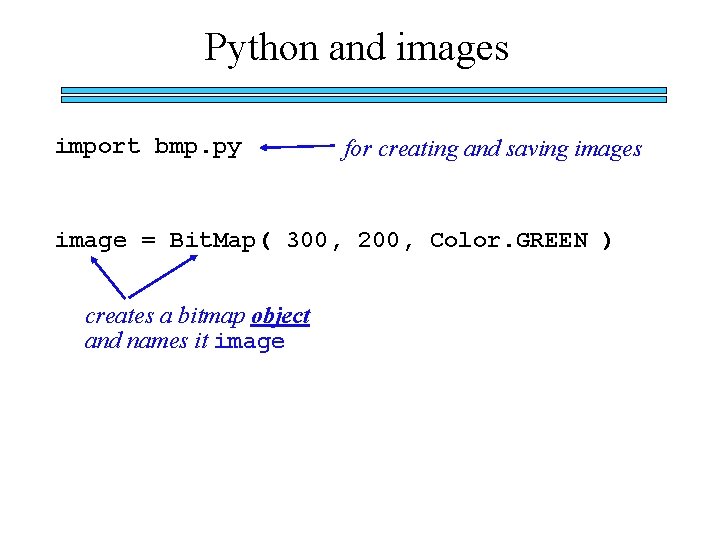
Python and images import bmp. py for creating and saving images image = Bit. Map( 300, 200, Color. GREEN ) creates a bitmap object and names it image
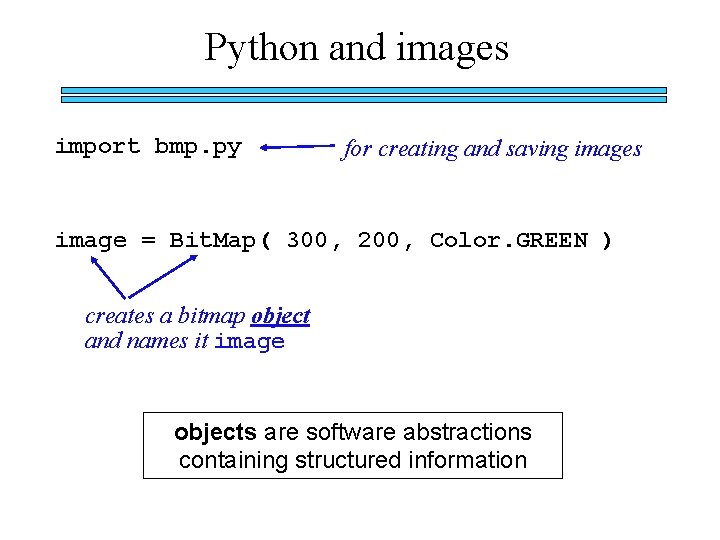
Python and images import bmp. py for creating and saving images image = Bit. Map( 300, 200, Color. GREEN ) creates a bitmap object and names it image objects are software abstractions containing structured information
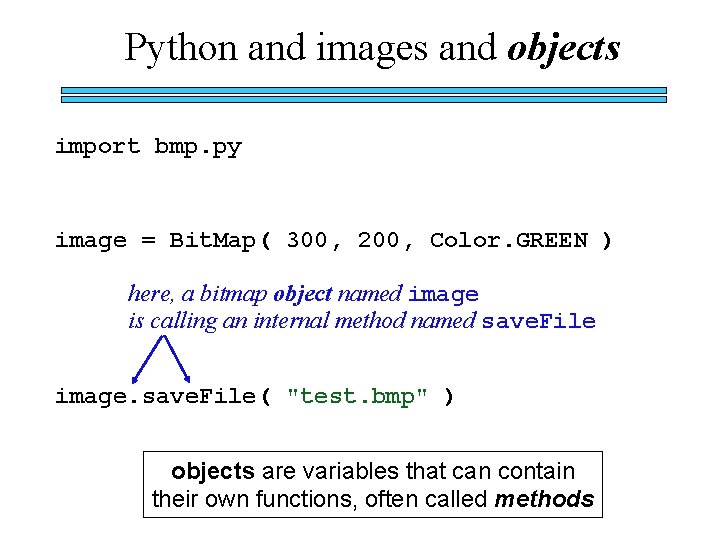
Python and images and objects import bmp. py image = Bit. Map( 300, 200, Color. GREEN ) here, a bitmap object named image is calling an internal method named save. File image. save. File( "test. bmp" ) objects are variables that can contain their own functions, often called methods
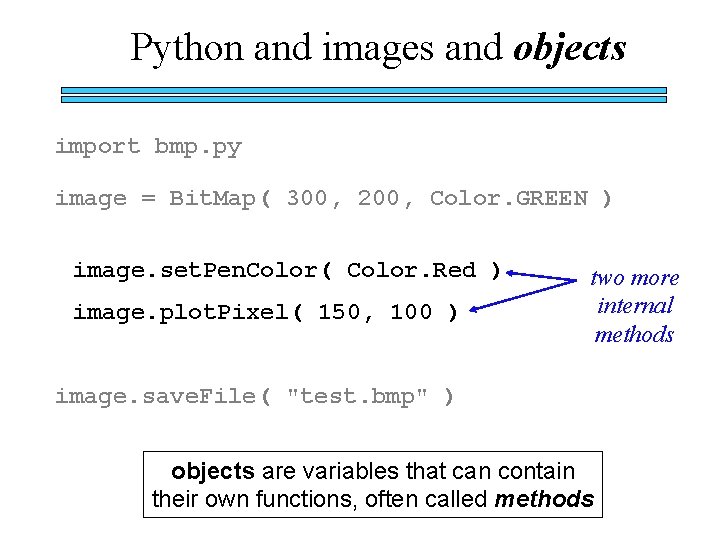
Python and images and objects import bmp. py image = Bit. Map( 300, 200, Color. GREEN ) image. set. Pen. Color( Color. Red ) image. plot. Pixel( 150, 100 ) two more internal methods image. save. File( "test. bmp" ) objects are variables that can contain their own functions, often called methods
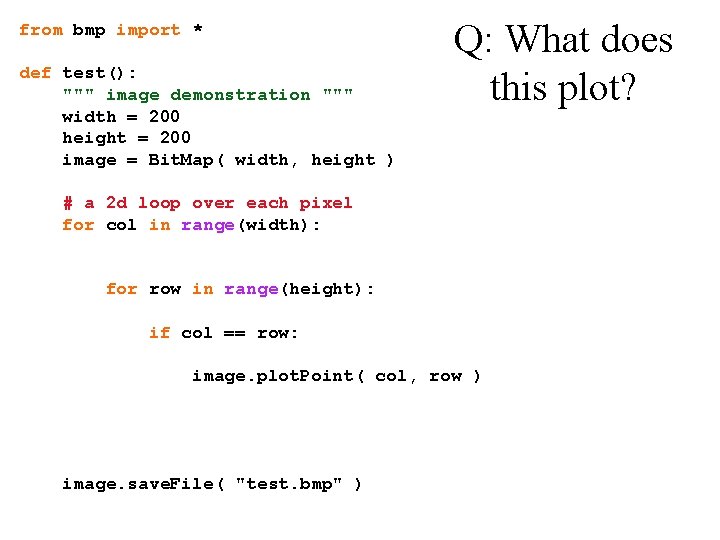
from bmp import * def test(): """ image demonstration """ width = 200 height = 200 image = Bit. Map( width, height ) Q: What does this plot? # a 2 d loop over each pixel for col in range(width): for row in range(height): if col == row: image. plot. Point( col, row ) image. save. File( "test. bmp" )
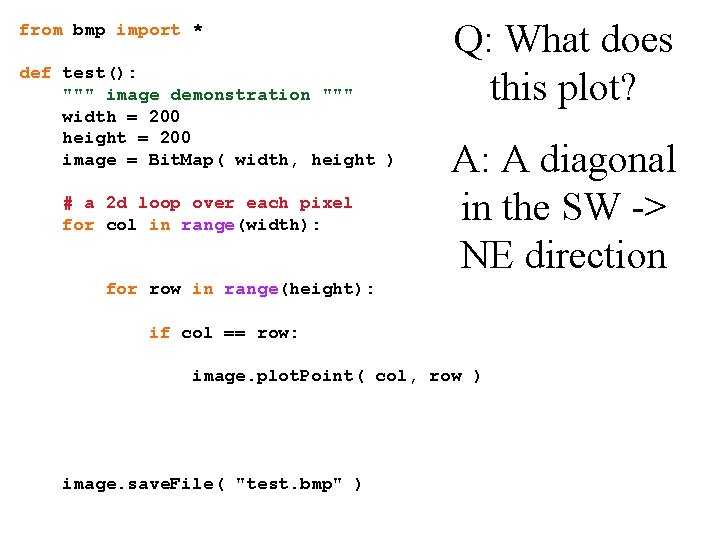
from bmp import * def test(): """ image demonstration """ width = 200 height = 200 image = Bit. Map( width, height ) # a 2 d loop over each pixel for col in range(width): Q: What does this plot? A: A diagonal in the SW -> NE direction for row in range(height): if col == row: image. plot. Point( col, row ) image. save. File( "test. bmp" )
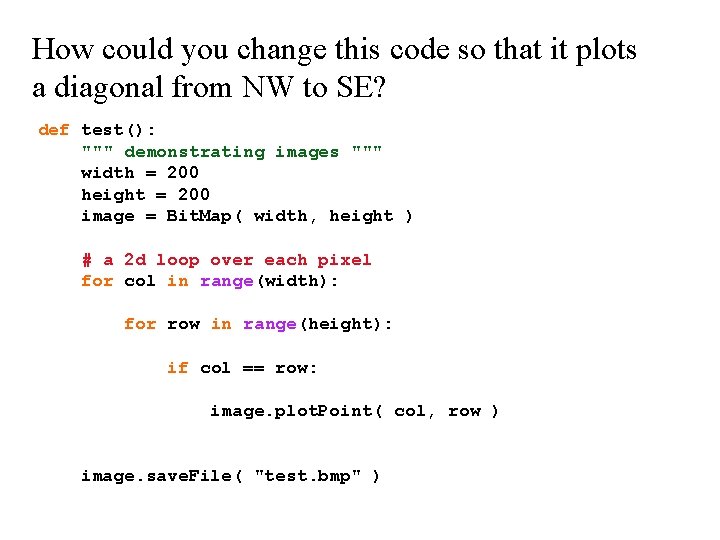
How could you change this code so that it plots a diagonal from NW to SE? def test(): """ demonstrating images """ width = 200 height = 200 image = Bit. Map( width, height ) # a 2 d loop over each pixel for col in range(width): for row in range(height): if col == row: image. plot. Point( col, row ) image. save. File( "test. bmp" )
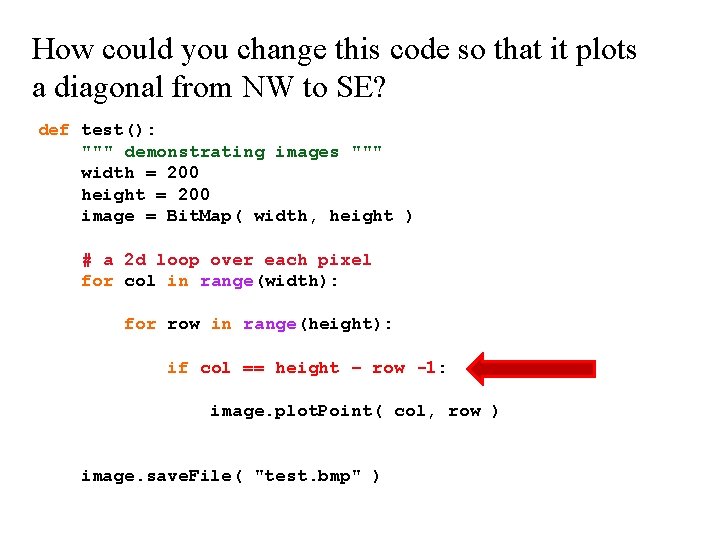
How could you change this code so that it plots a diagonal from NW to SE? def test(): """ demonstrating images """ width = 200 height = 200 image = Bit. Map( width, height ) # a 2 d loop over each pixel for col in range(width): for row in range(height): if col == height – row -1: image. plot. Point( col, row ) image. save. File( "test. bmp" )
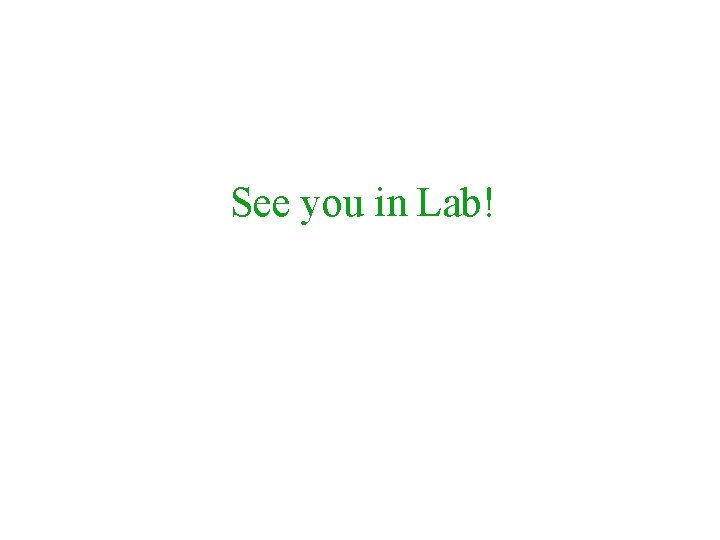
See you in Lab!
Vignette mutuelle 110/110
000 111 000
Eecs 110
Single user and multiple user operating system
Single user and multi user operating system
Scoreboard architecture
11th chemistry thermodynamics lec 13
Lec ditto
Lec scoreboard
Componentes del lec
11th chemistry thermodynamics lec 10
Biochemistry
August lec 250
Lec 16
Lec material
Art 455 lec
Lec
132000 lec
Xrl instruction in 8051
Tura analítica
Sekisui slec
416 lec
Lec
Brayton
Lec promotion
Lec anatomia
Lec hardver
Fenemates
Lec barbate
Lec hardver
Lec renal
252 lec
While loops and if-else structures
Branches circuit
For loop small basic
Pulmonary function test results
Reddish loops of gas
Nested while loops matlab
Types of loops in matlab
Programming puzzle
Perulangan looping
Geothermal loops design
Matlab for loop example
Cakewalk loops
Featureless loop of wangenstein