Overview History Hardware Software Development Life Cycle Programming
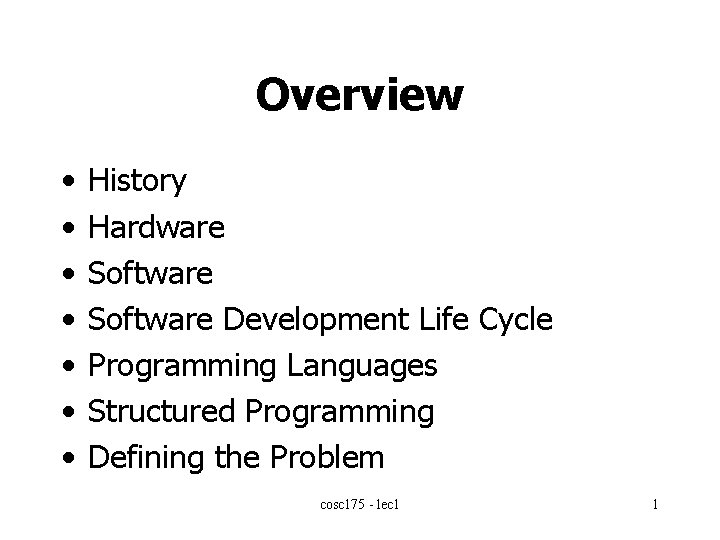
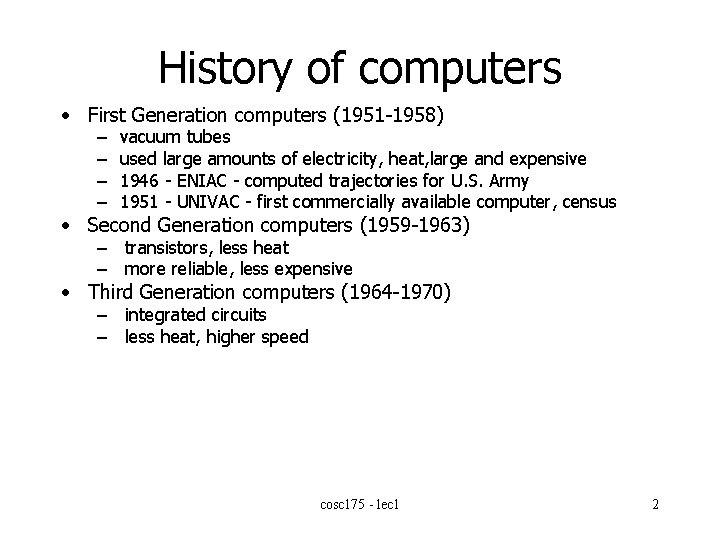
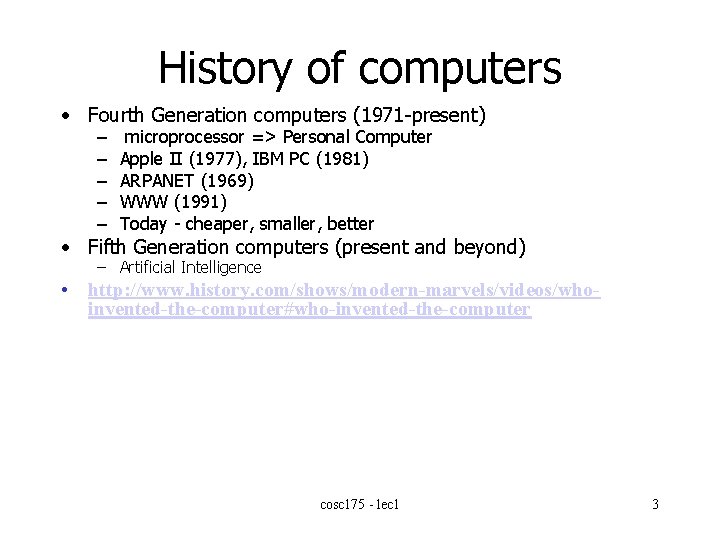
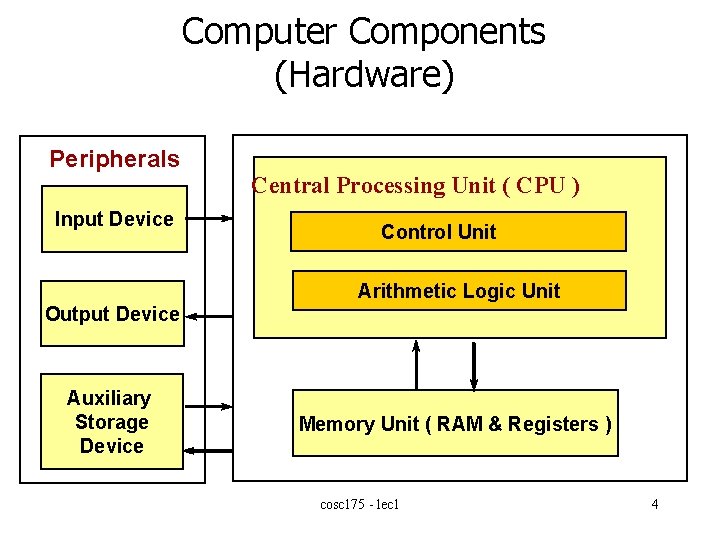
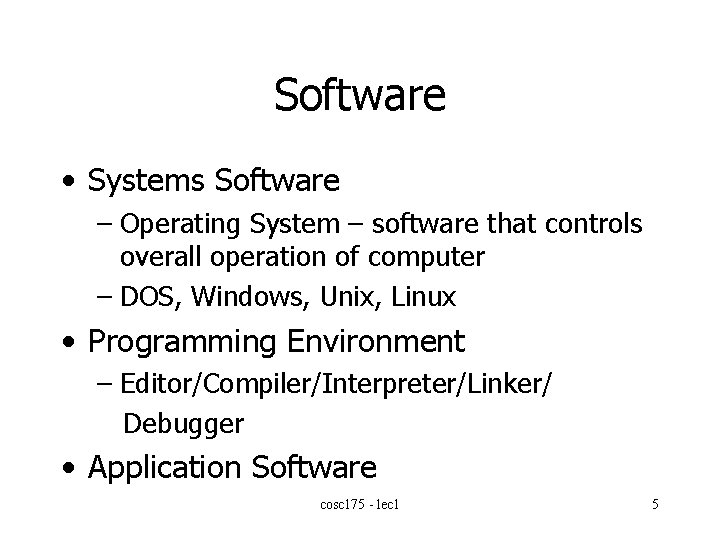
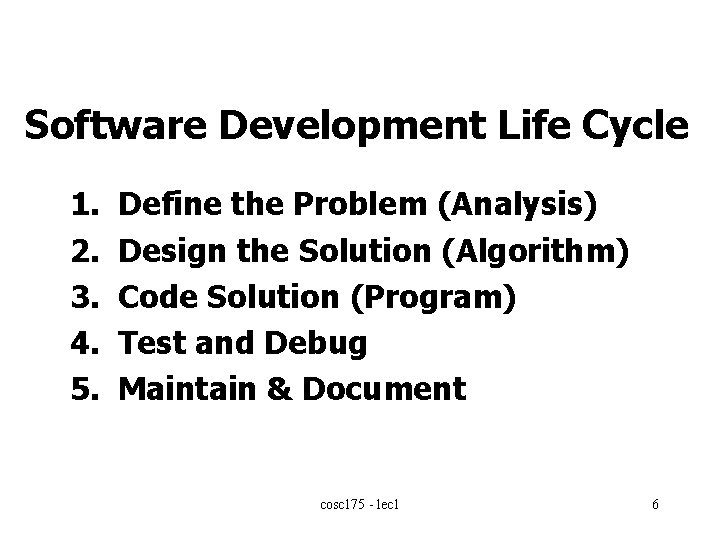
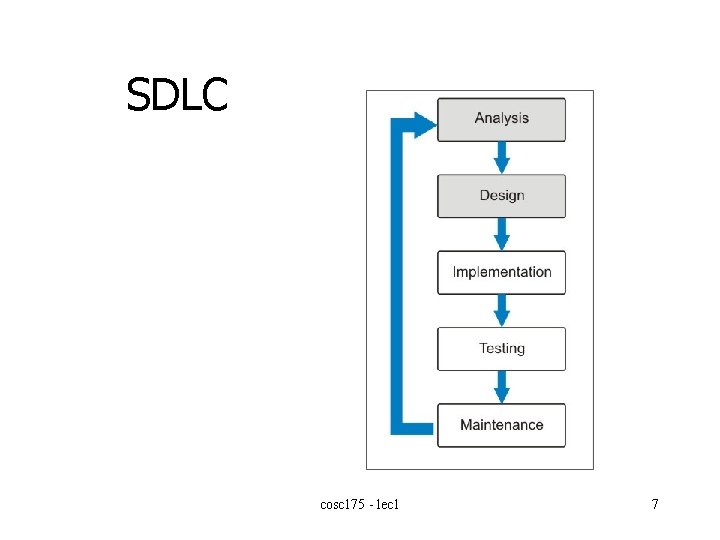
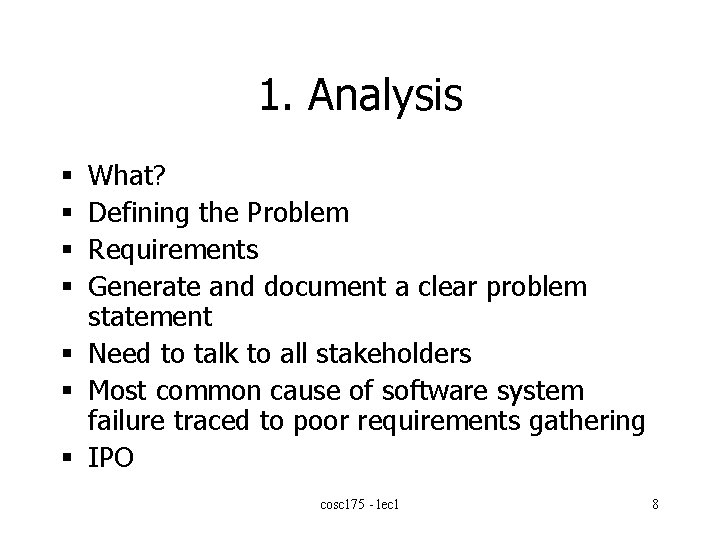
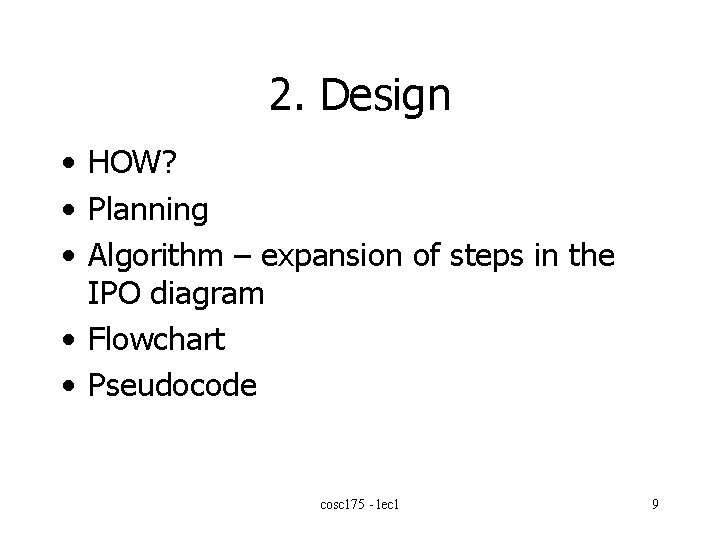
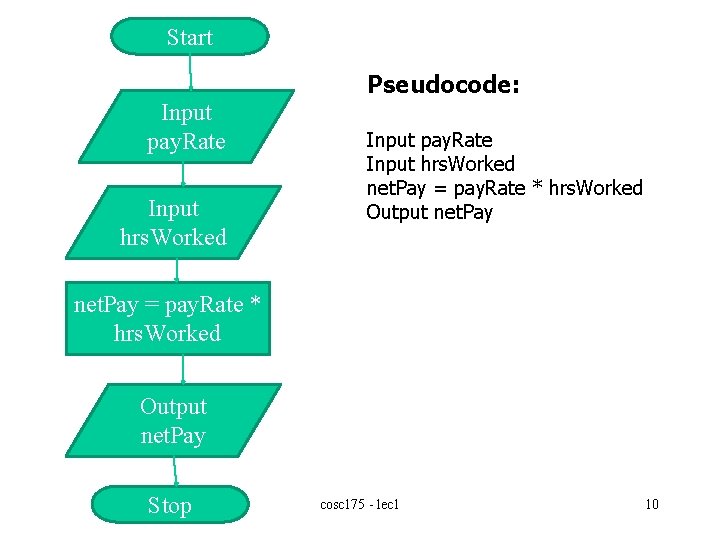
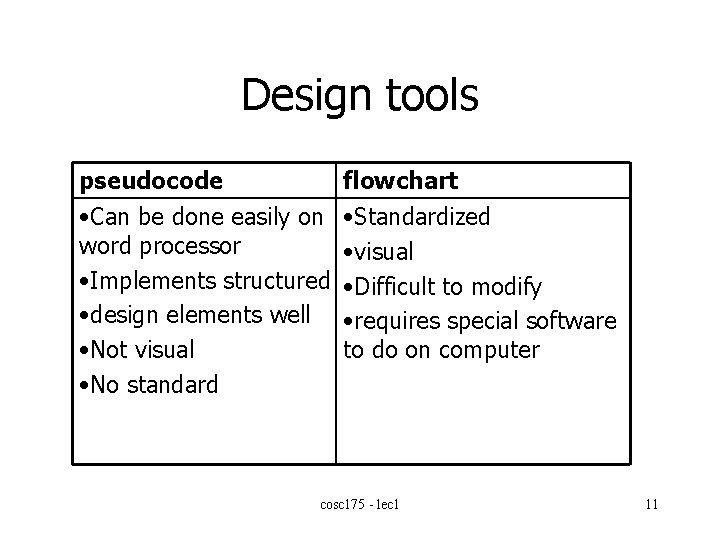
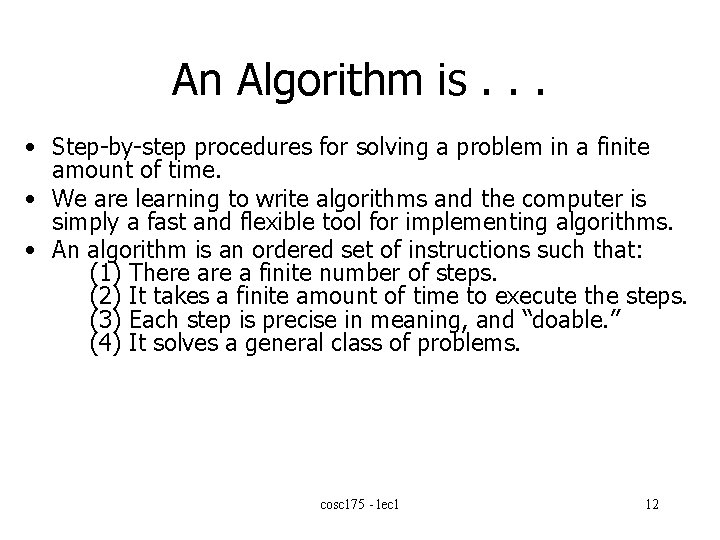
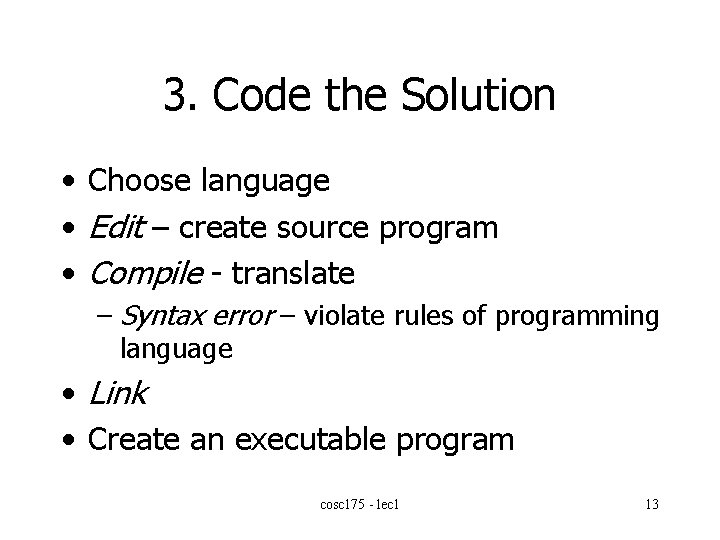
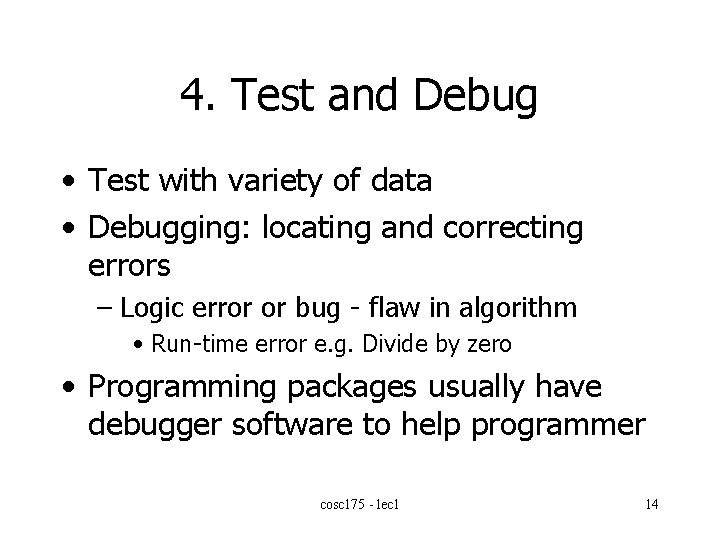
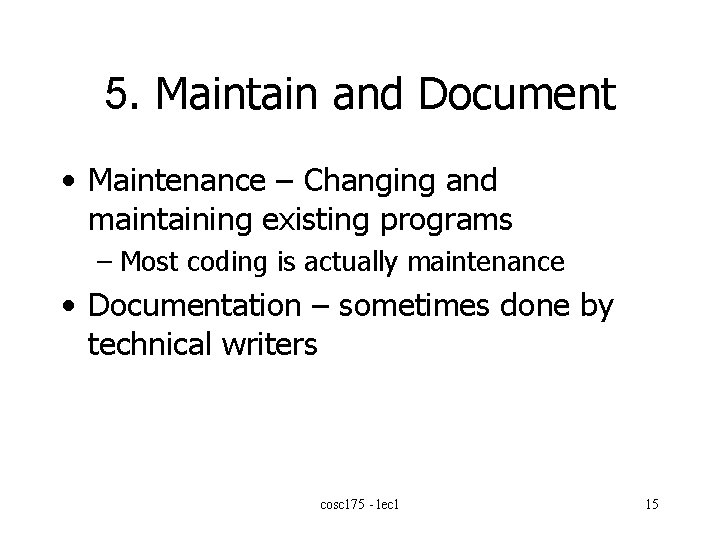
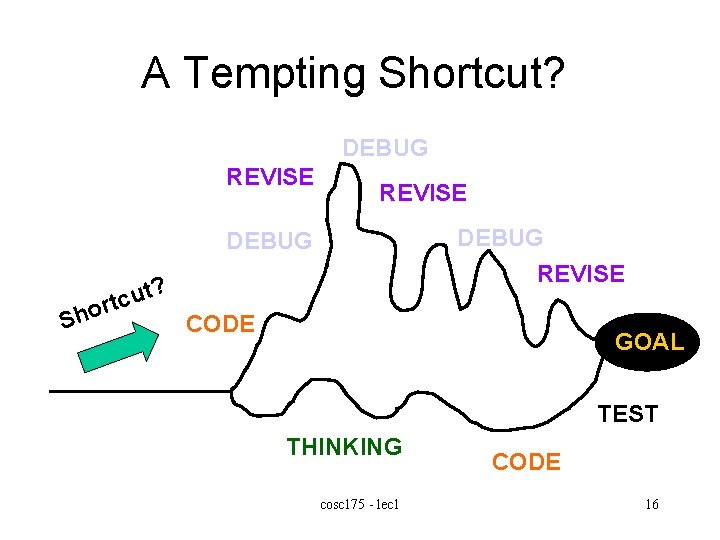
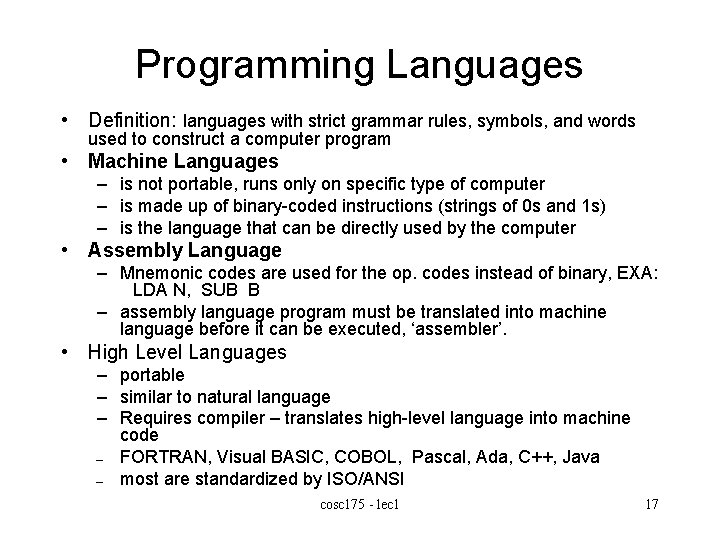
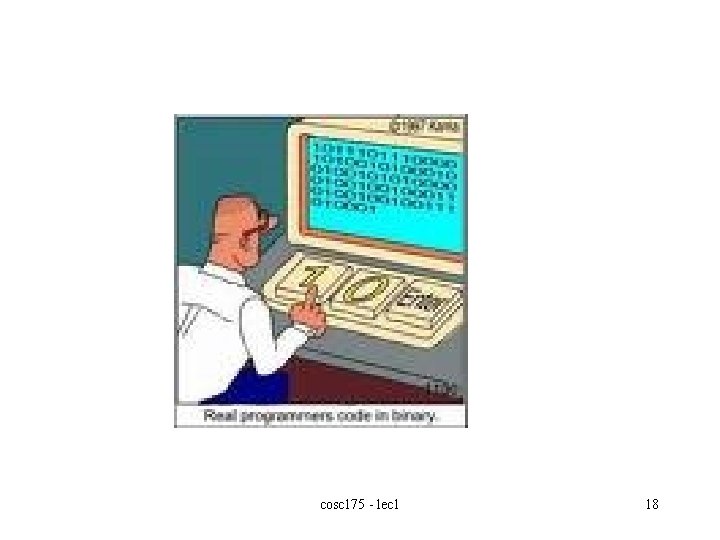
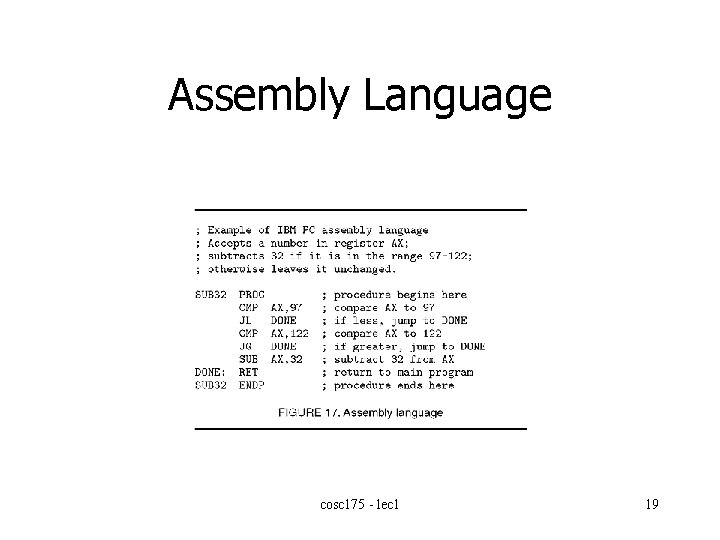
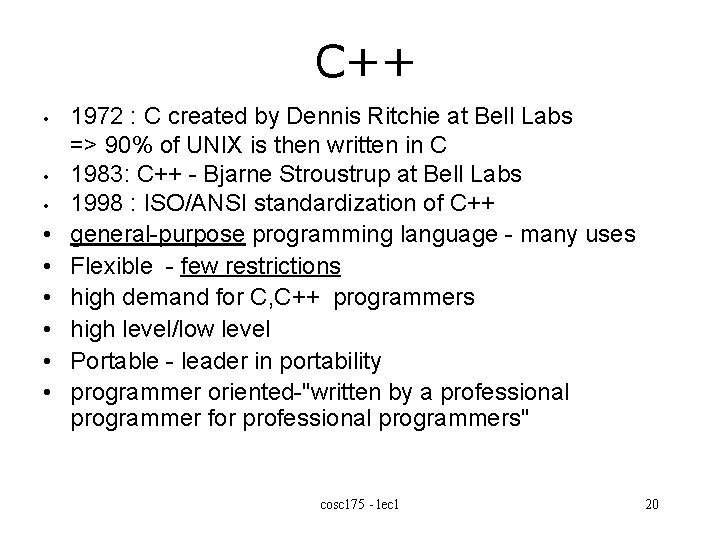
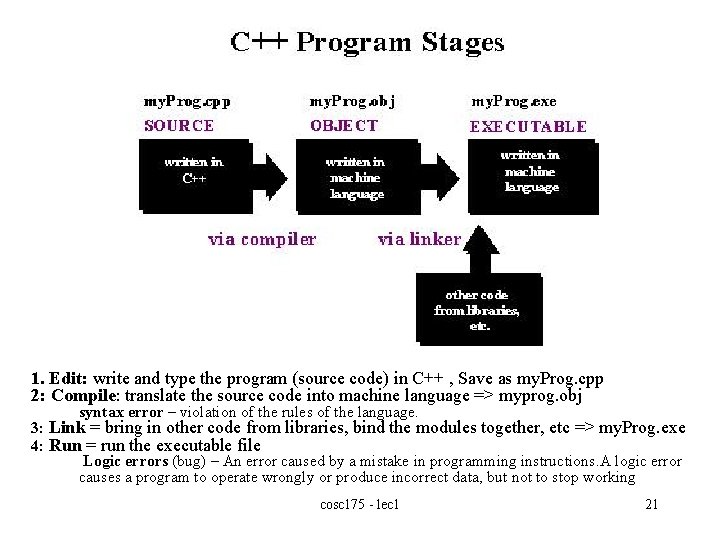
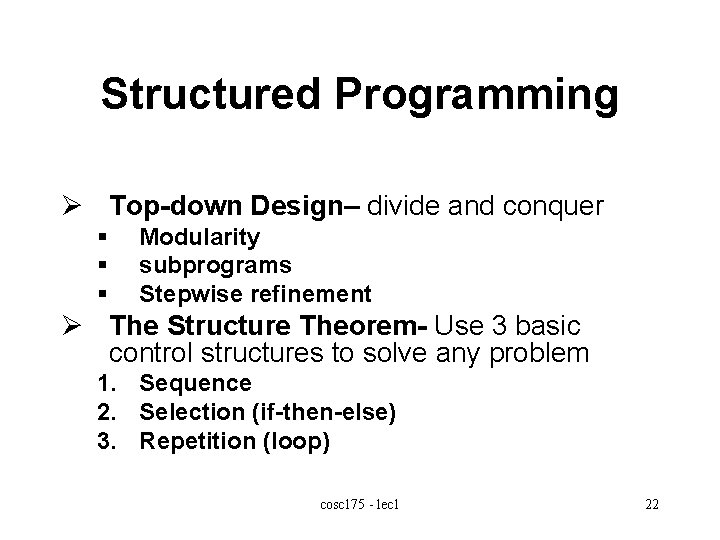
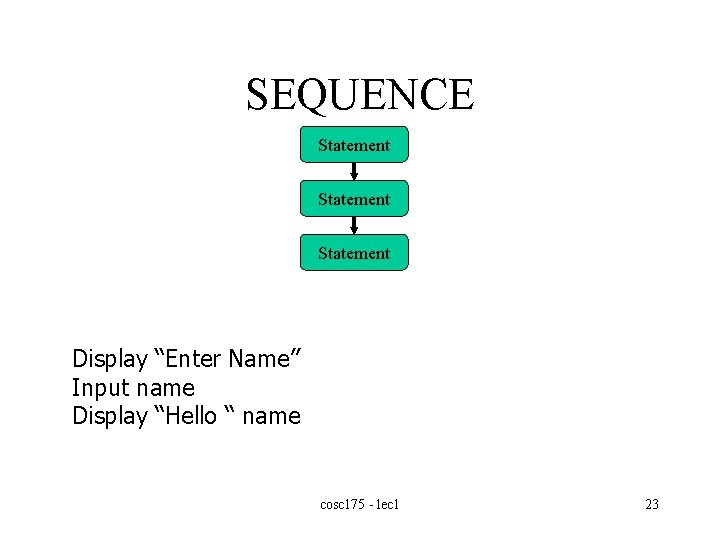
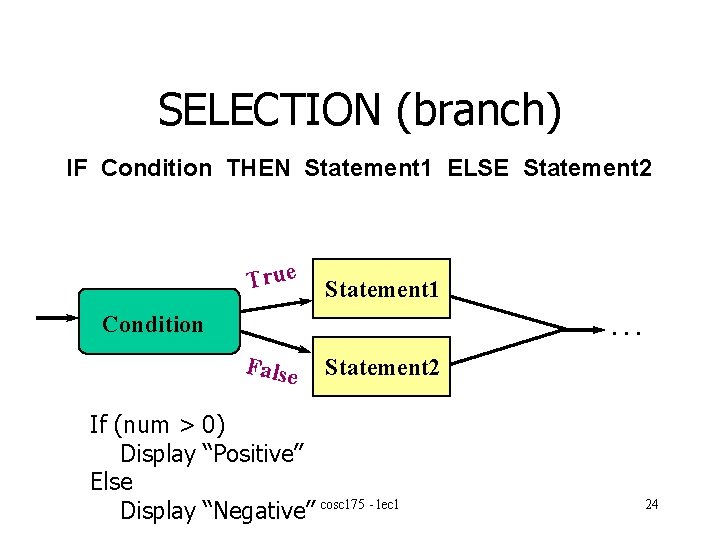
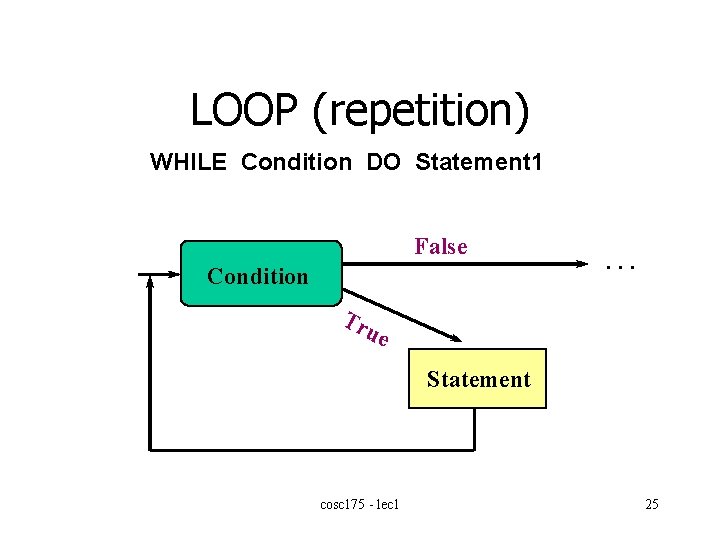
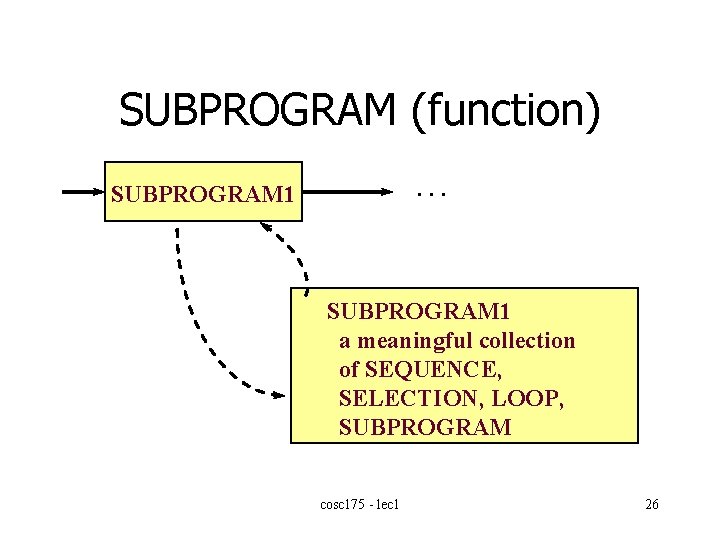
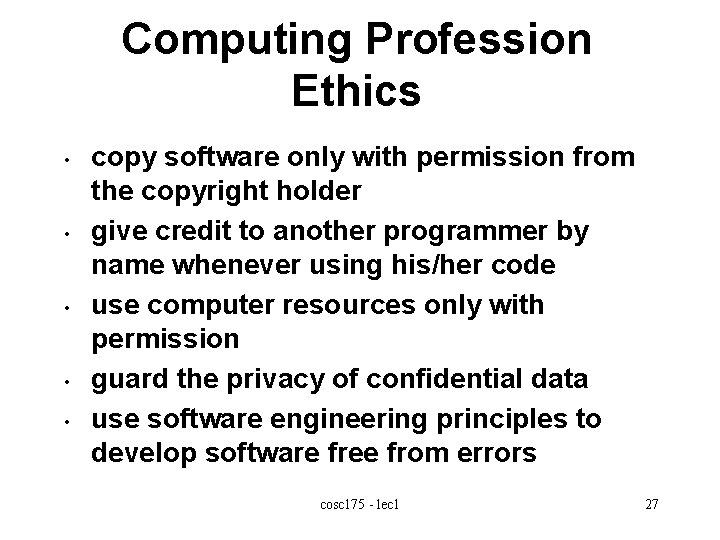
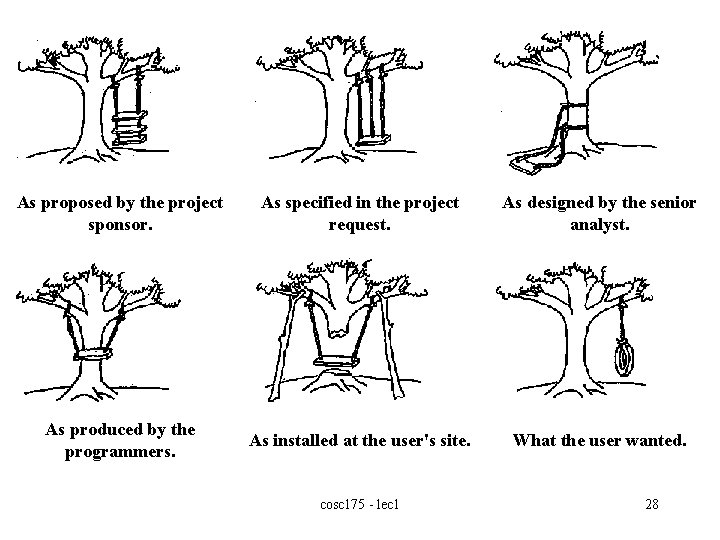
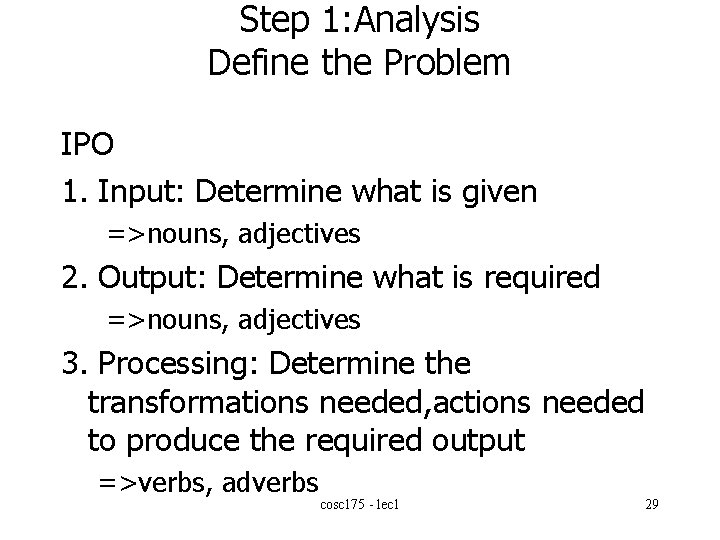
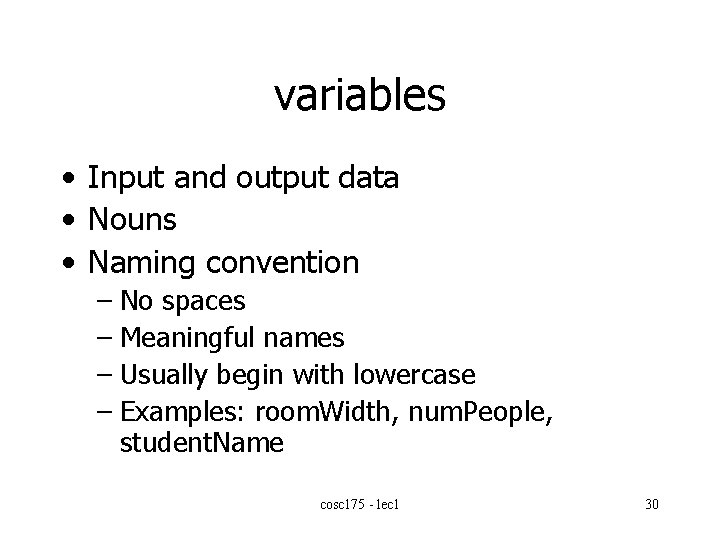
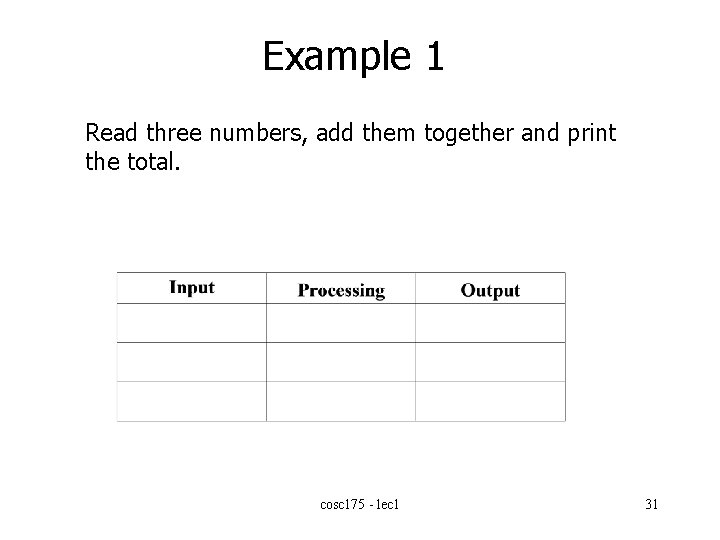
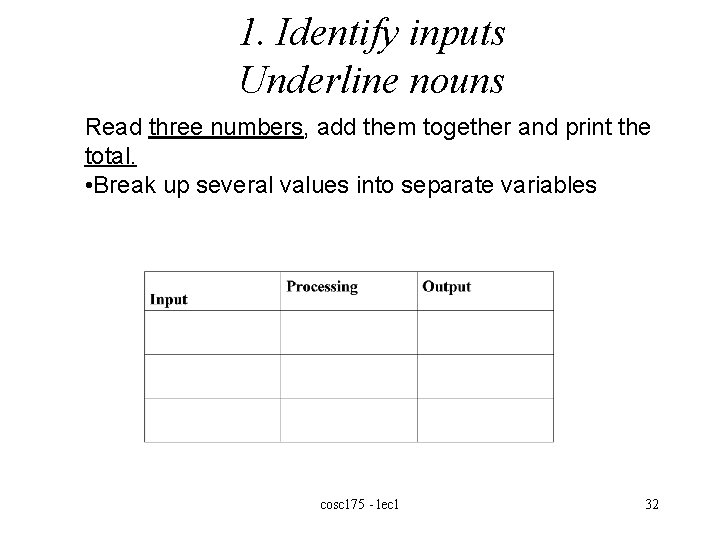
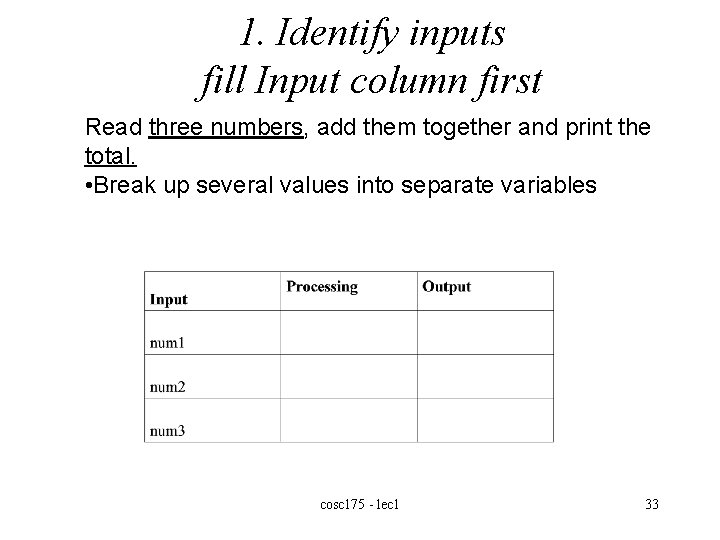
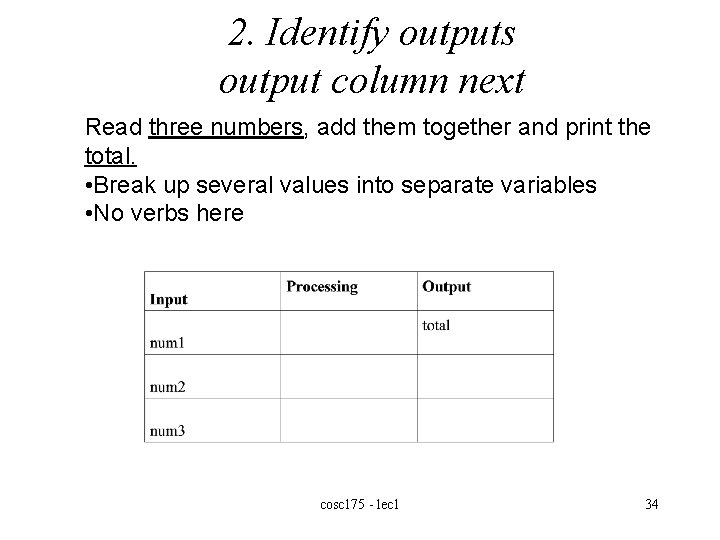
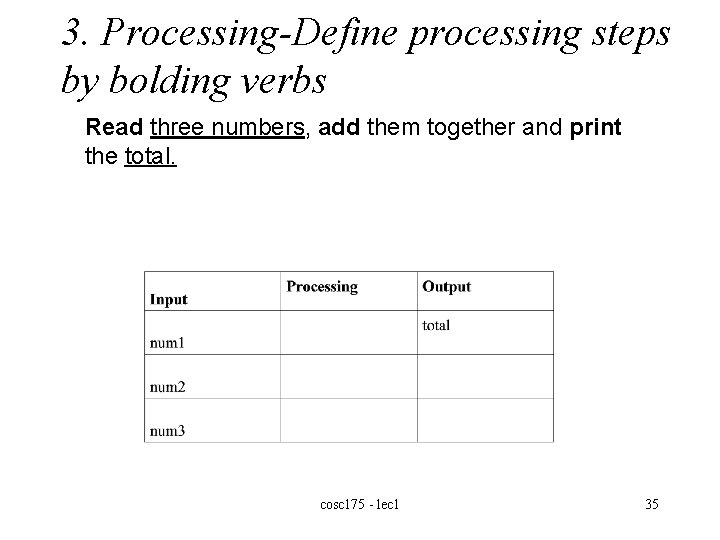
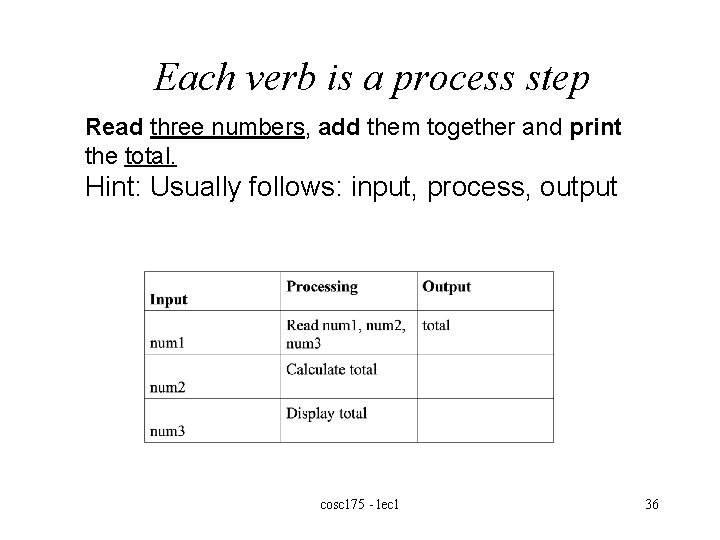
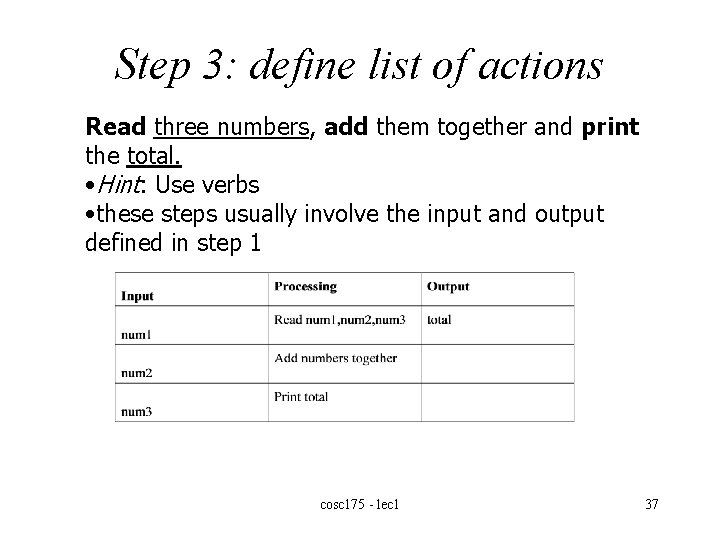
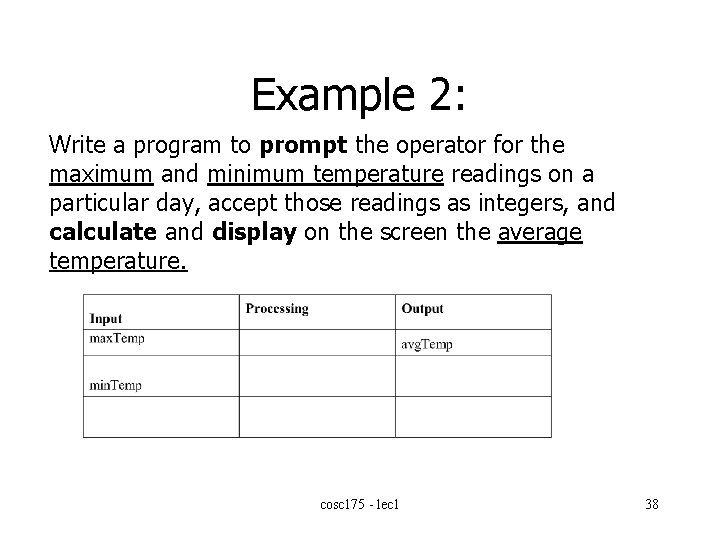
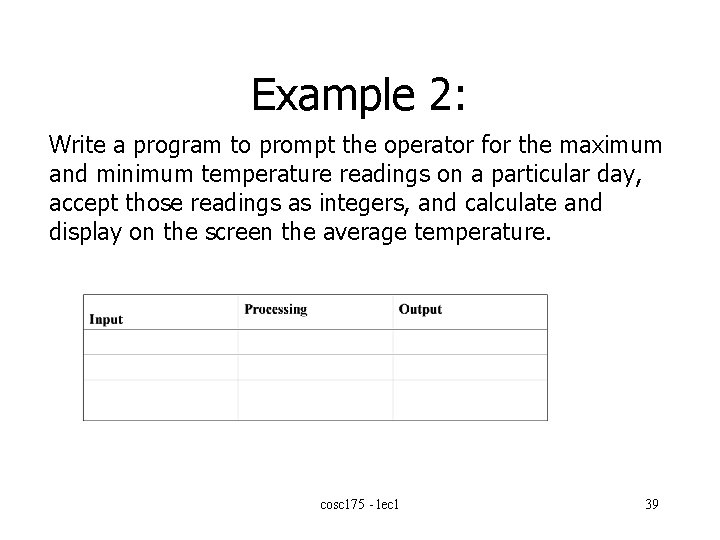
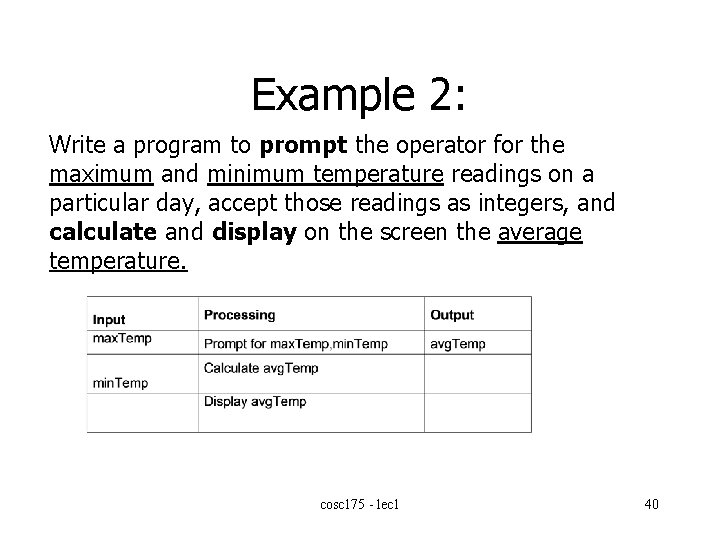
- Slides: 40
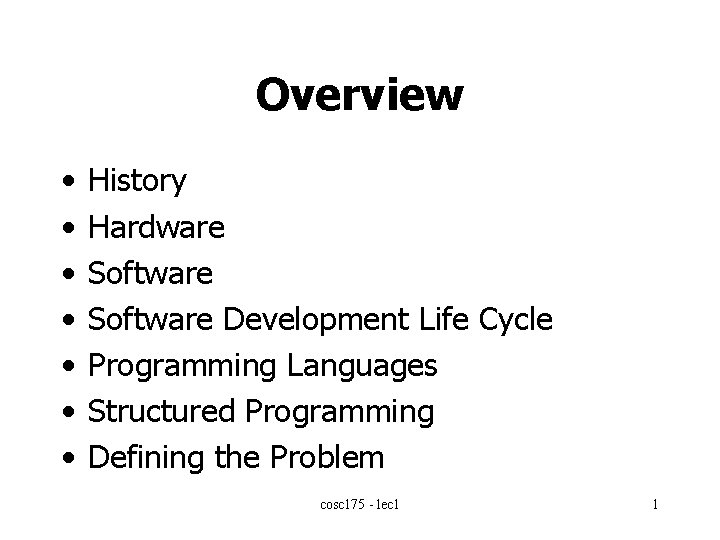
Overview • • History Hardware Software Development Life Cycle Programming Languages Structured Programming Defining the Problem cosc 175 - lec 1 1
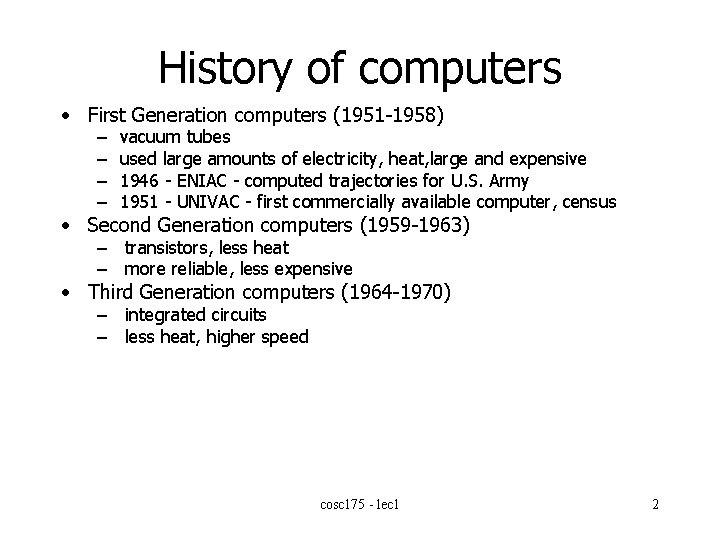
History of computers • First Generation computers (1951 -1958) – – vacuum tubes used large amounts of electricity, heat, large and expensive 1946 - ENIAC - computed trajectories for U. S. Army 1951 - UNIVAC - first commercially available computer, census • Second Generation computers (1959 -1963) – transistors, less heat – more reliable, less expensive • Third Generation computers (1964 -1970) – integrated circuits – less heat, higher speed cosc 175 - lec 1 2
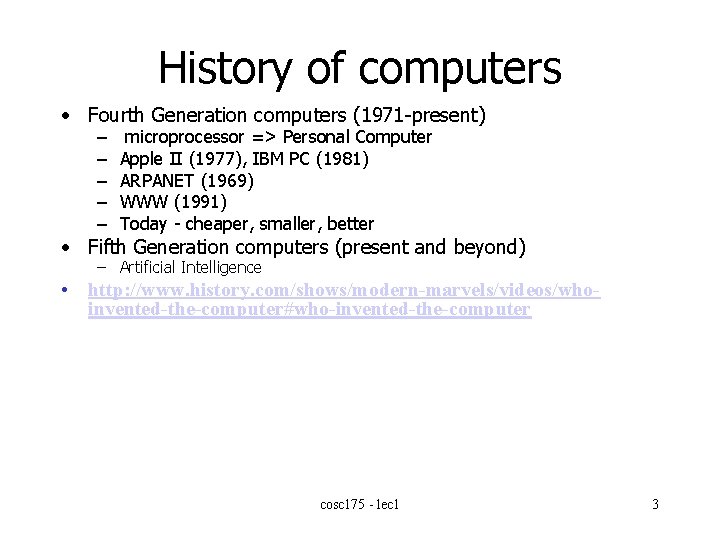
History of computers • Fourth Generation computers (1971 -present) – – – microprocessor => Personal Computer Apple II (1977), IBM PC (1981) ARPANET (1969) WWW (1991) Today - cheaper, smaller, better • Fifth Generation computers (present and beyond) – Artificial Intelligence • http: //www. history. com/shows/modern-marvels/videos/whoinvented-the-computer#who-invented-the-computer cosc 175 - lec 1 3
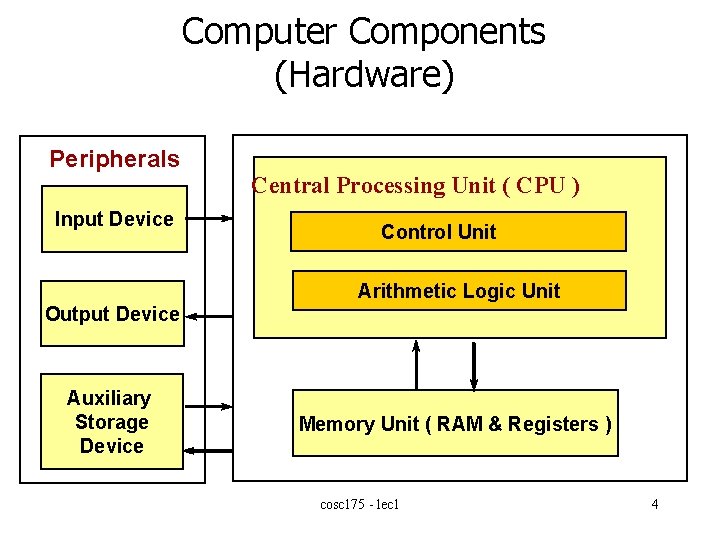
Computer Components (Hardware) Peripherals Central Processing Unit ( CPU ) Input Device Control Unit Arithmetic Logic Unit Output Device Auxiliary Storage Device Memory Unit ( RAM & Registers ) cosc 175 - lec 1 4
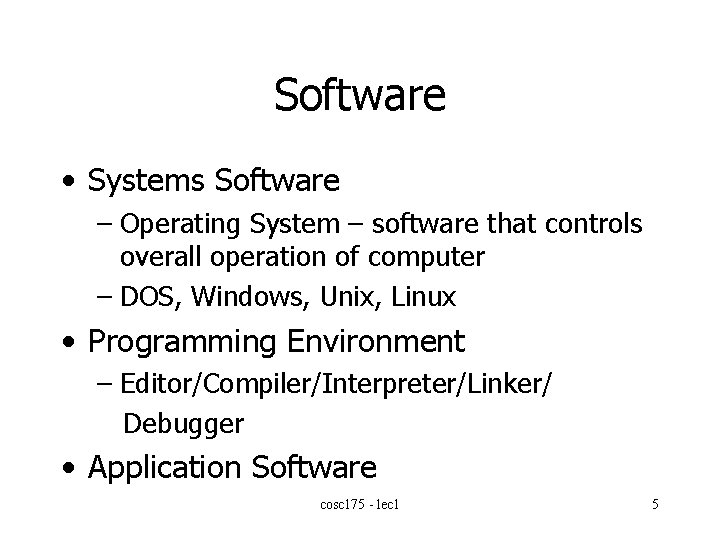
Software • Systems Software – Operating System – software that controls overall operation of computer – DOS, Windows, Unix, Linux • Programming Environment – Editor/Compiler/Interpreter/Linker/ Debugger • Application Software cosc 175 - lec 1 5
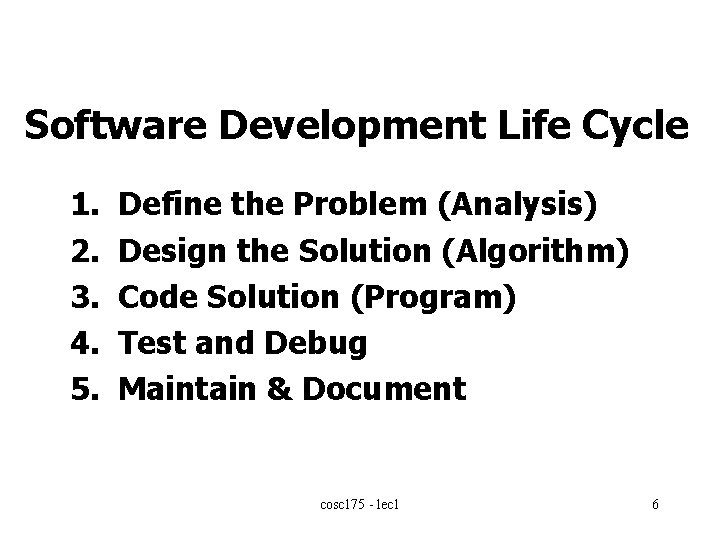
Software Development Life Cycle 1. 2. 3. 4. 5. Define the Problem (Analysis) Design the Solution (Algorithm) Code Solution (Program) Test and Debug Maintain & Document cosc 175 - lec 1 6
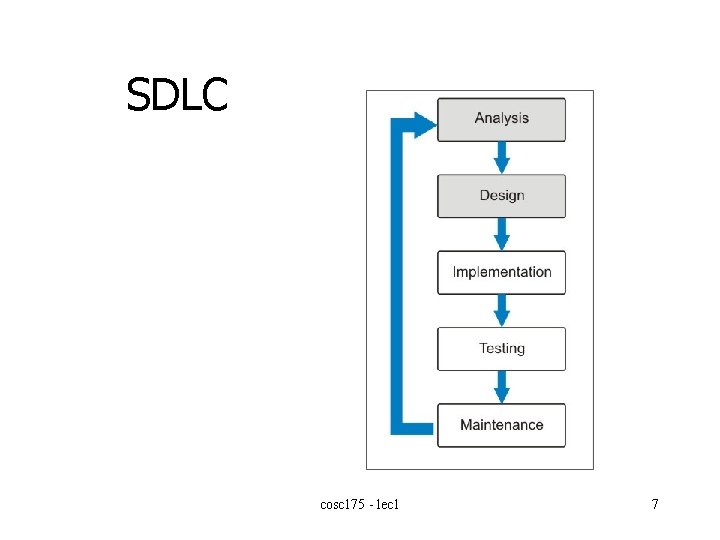
SDLC cosc 175 - lec 1 7
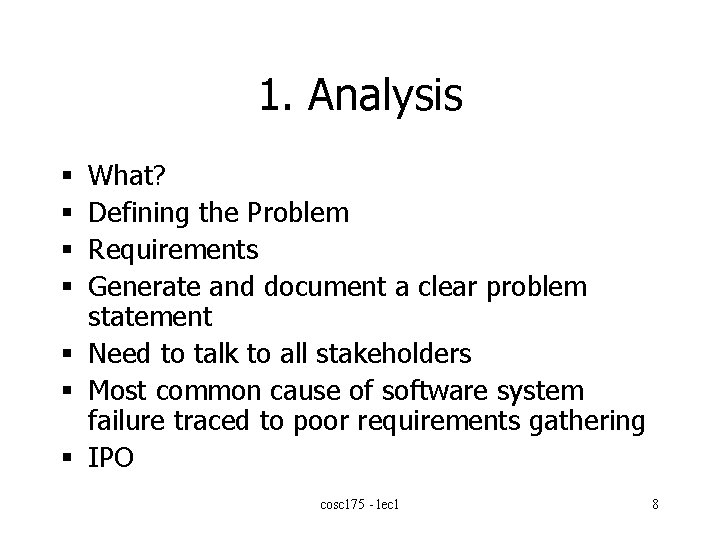
1. Analysis What? Defining the Problem Requirements Generate and document a clear problem statement § Need to talk to all stakeholders § Most common cause of software system failure traced to poor requirements gathering § IPO § § cosc 175 - lec 1 8
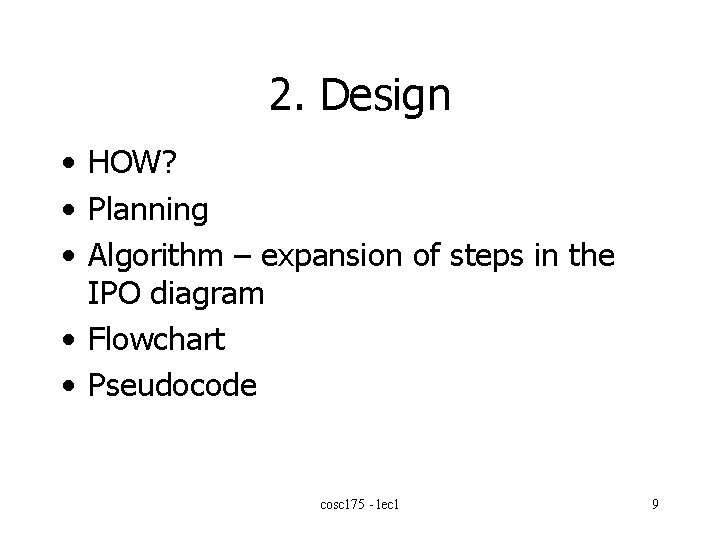
2. Design • HOW? • Planning • Algorithm – expansion of steps in the IPO diagram • Flowchart • Pseudocode cosc 175 - lec 1 9
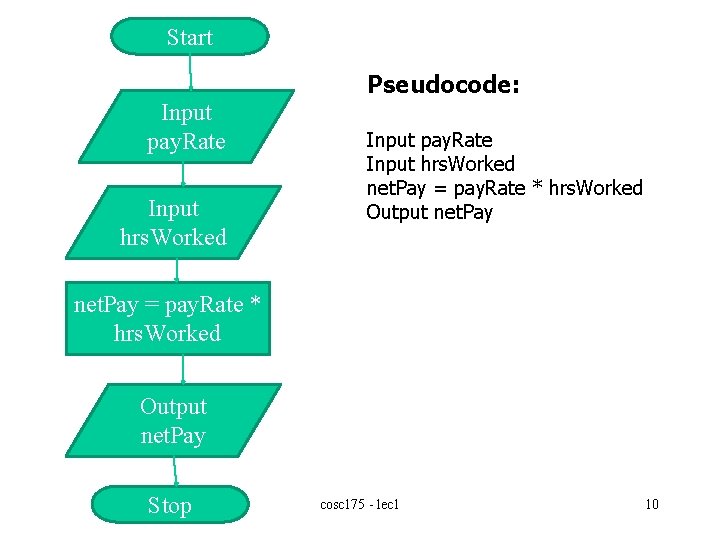
Start Pseudocode: Input pay. Rate Input hrs. Worked net. Pay = pay. Rate * hrs. Worked Output net. Pay Stop cosc 175 - lec 1 10
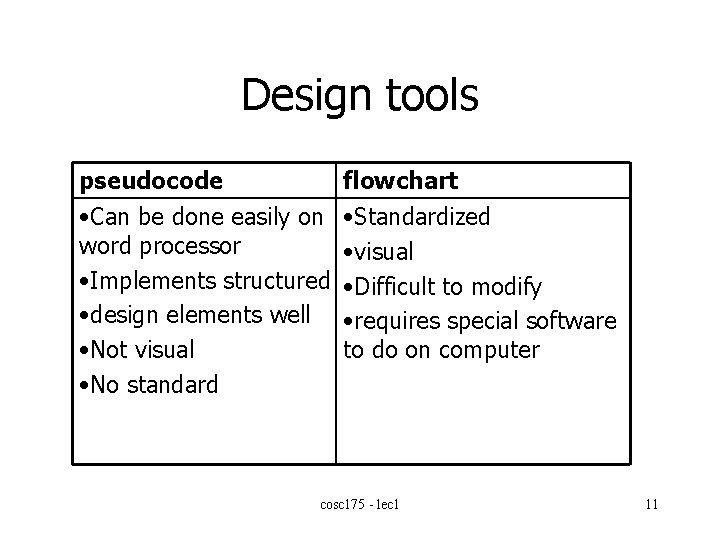
Design tools pseudocode • Can be done easily on word processor • Implements structured • design elements well • Not visual • No standard flowchart • Standardized • visual • Difficult to modify • requires special software to do on computer cosc 175 - lec 1 11
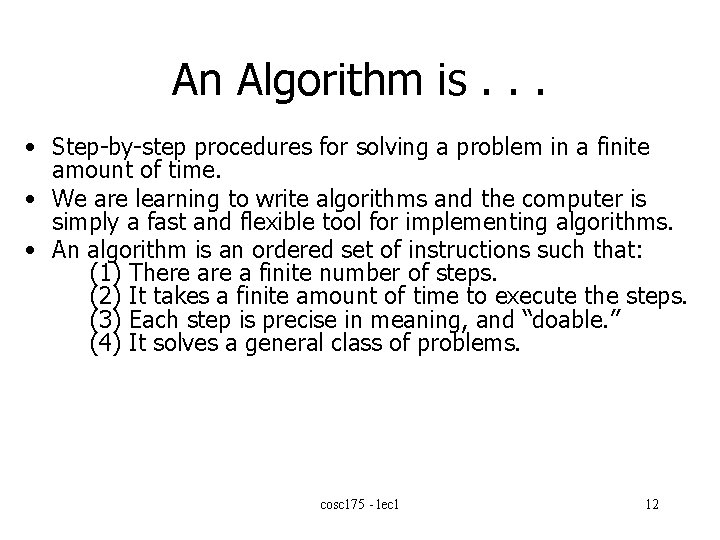
An Algorithm is. . . • Step-by-step procedures for solving a problem in a finite amount of time. • We are learning to write algorithms and the computer is simply a fast and flexible tool for implementing algorithms. • An algorithm is an ordered set of instructions such that: (1) There a finite number of steps. (2) It takes a finite amount of time to execute the steps. (3) Each step is precise in meaning, and “doable. ” (4) It solves a general class of problems. cosc 175 - lec 1 12
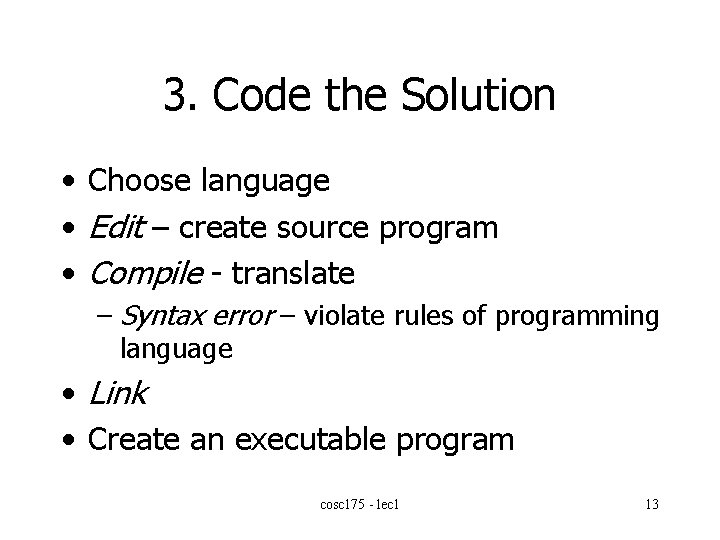
3. Code the Solution • Choose language • Edit – create source program • Compile - translate – Syntax error – violate rules of programming language • Link • Create an executable program cosc 175 - lec 1 13
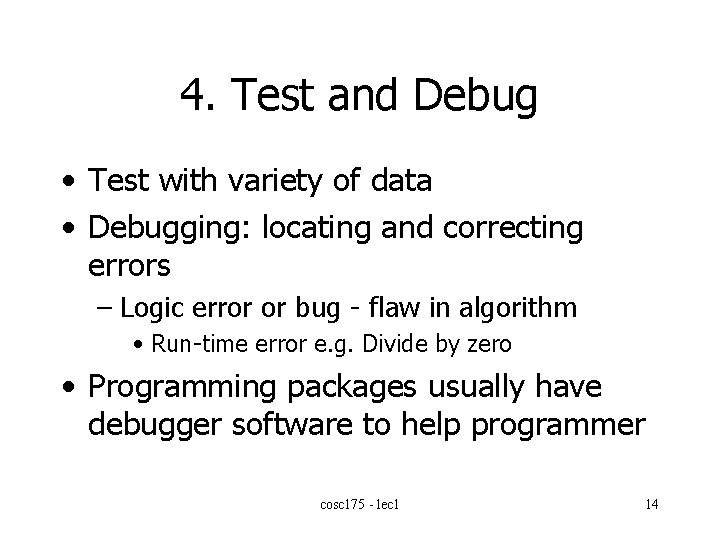
4. Test and Debug • Test with variety of data • Debugging: locating and correcting errors – Logic error or bug - flaw in algorithm • Run-time error e. g. Divide by zero • Programming packages usually have debugger software to help programmer cosc 175 - lec 1 14
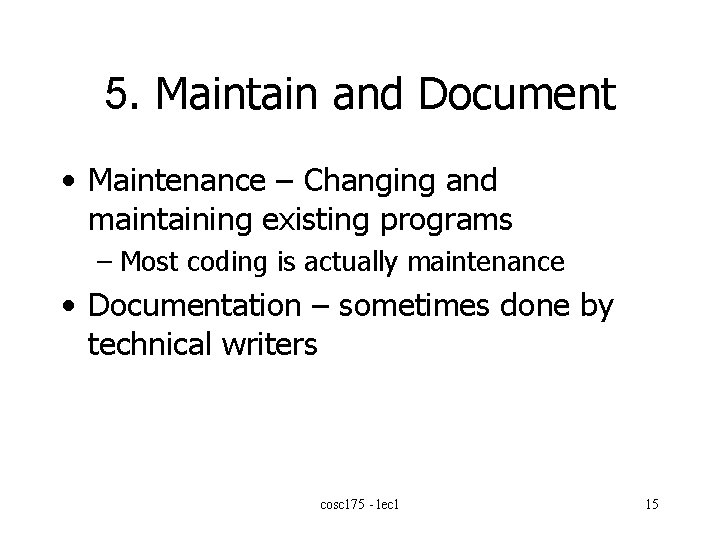
5. Maintain and Document • Maintenance – Changing and maintaining existing programs – Most coding is actually maintenance • Documentation – sometimes done by technical writers cosc 175 - lec 1 15
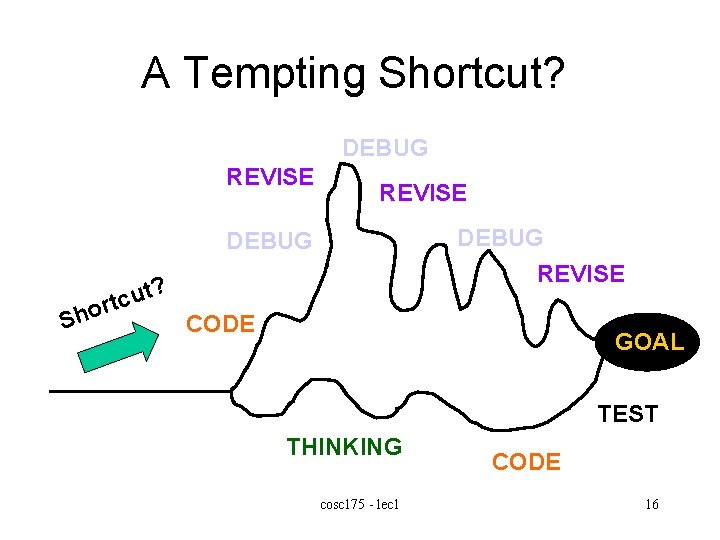
A Tempting Shortcut? DEBUG REVISE DEBUG t? u c t r Sho CODE GOAL TEST THINKING cosc 175 - lec 1 CODE 16
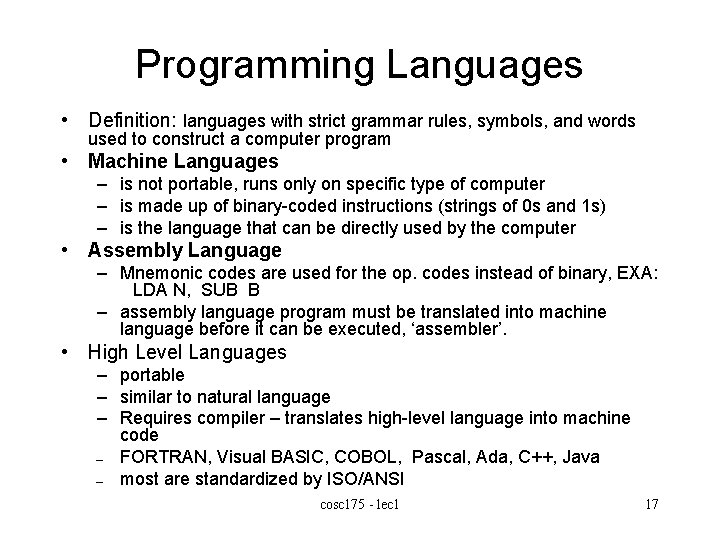
Programming Languages • Definition: languages with strict grammar rules, symbols, and words used to construct a computer program • Machine Languages – is not portable, runs only on specific type of computer – is made up of binary-coded instructions (strings of 0 s and 1 s) – is the language that can be directly used by the computer • Assembly Language – Mnemonic codes are used for the op. codes instead of binary, EXA: LDA N, SUB B – assembly language program must be translated into machine language before it can be executed, ‘assembler’. • High Level Languages – portable – similar to natural language – Requires compiler – translates high-level language into machine code – FORTRAN, Visual BASIC, COBOL, Pascal, Ada, C++, Java – most are standardized by ISO/ANSI cosc 175 - lec 1 17
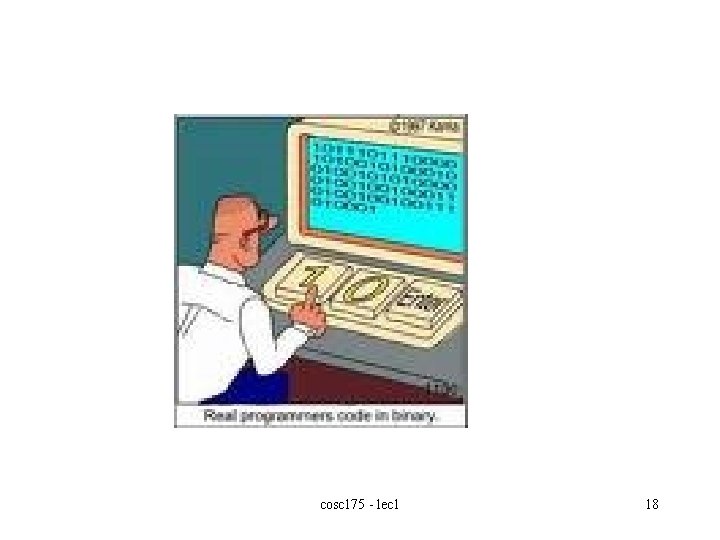
cosc 175 - lec 1 18
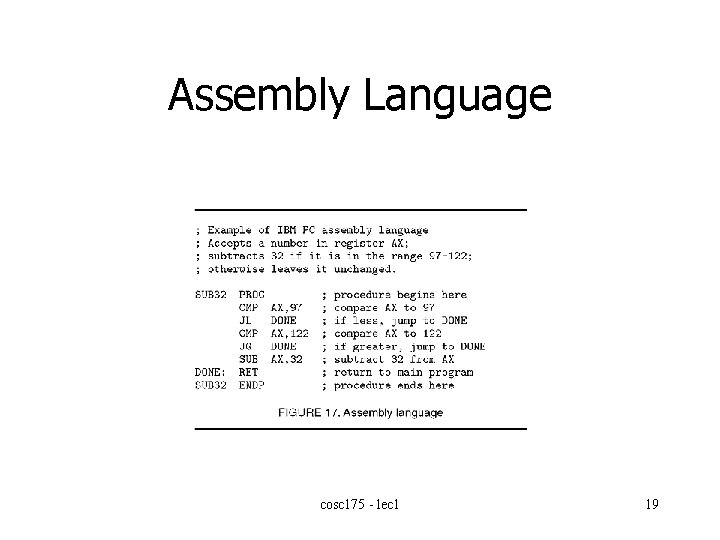
Assembly Language cosc 175 - lec 1 19
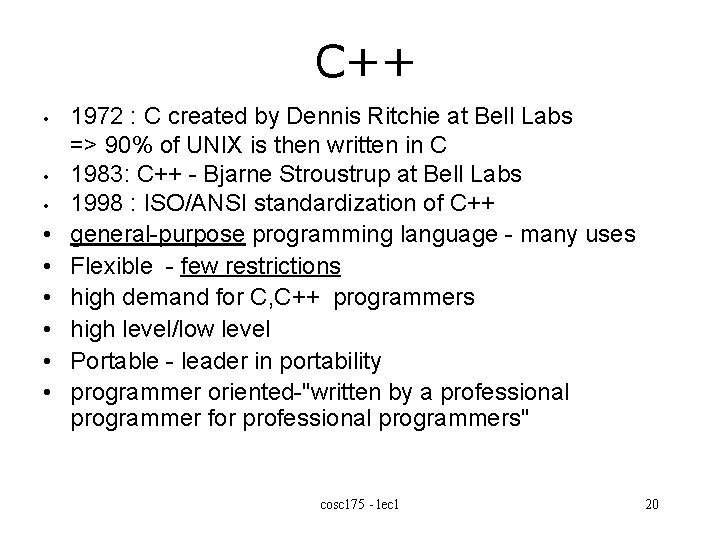
C++ • • • 1972 : C created by Dennis Ritchie at Bell Labs => 90% of UNIX is then written in C 1983: C++ - Bjarne Stroustrup at Bell Labs 1998 : ISO/ANSI standardization of C++ general-purpose programming language - many uses Flexible - few restrictions high demand for C, C++ programmers high level/low level Portable - leader in portability programmer oriented-"written by a professional programmer for professional programmers" cosc 175 - lec 1 20
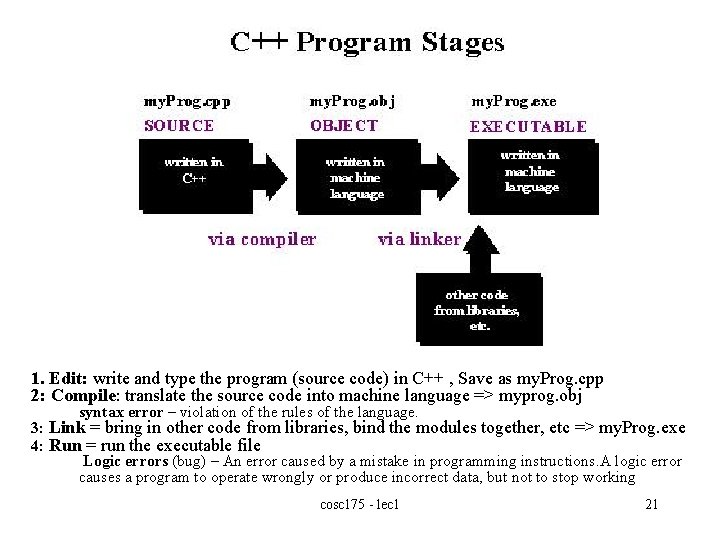
1. Edit: write and type the program (source code) in C++ , Save as my. Prog. cpp 2: Compile: translate the source code into machine language => myprog. obj syntax error – violation of the rules of the language. 3: Link = bring in other code from libraries, bind the modules together, etc => my. Prog. exe 4: Run = run the executable file Logic errors (bug) – An error caused by a mistake in programming instructions. A logic error causes a program to operate wrongly or produce incorrect data, but not to stop working cosc 175 - lec 1 21
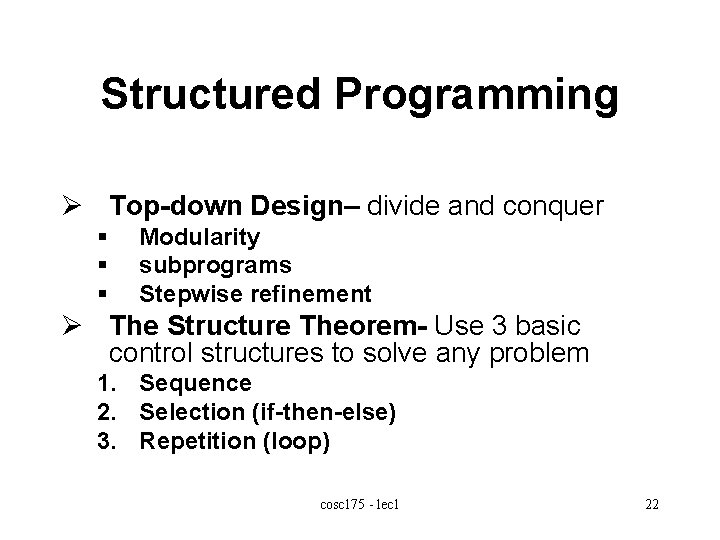
Structured Programming Ø Top-down Design– divide and conquer § § § Modularity subprograms Stepwise refinement Ø The Structure Theorem- Use 3 basic control structures to solve any problem 1. Sequence 2. Selection (if-then-else) 3. Repetition (loop) cosc 175 - lec 1 22
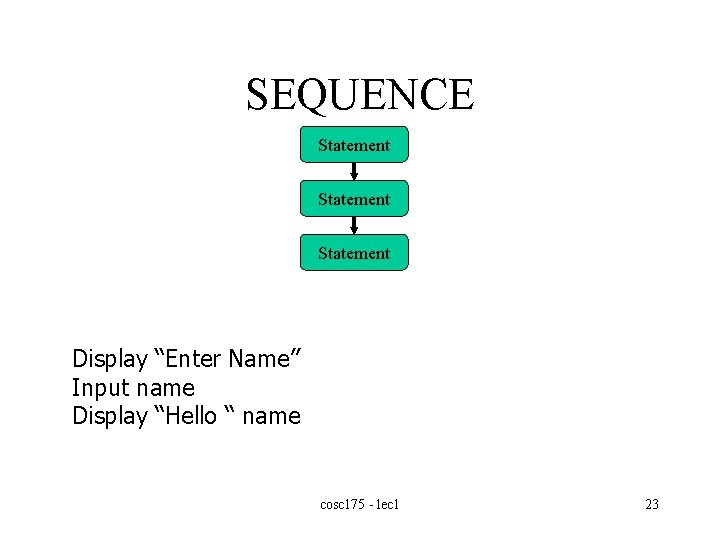
SEQUENCE Statement Display “Enter Name” Input name Display “Hello “ name cosc 175 - lec 1 23
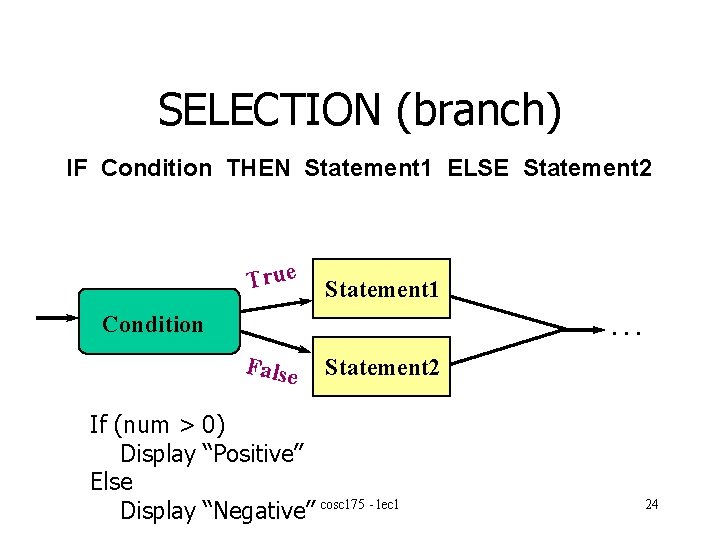
SELECTION (branch) IF Condition THEN Statement 1 ELSE Statement 2 True Statement 1 Statement Condition . . . False Statement 2 If (num > 0) Display “Positive” Else Display “Negative” cosc 175 - lec 1 24
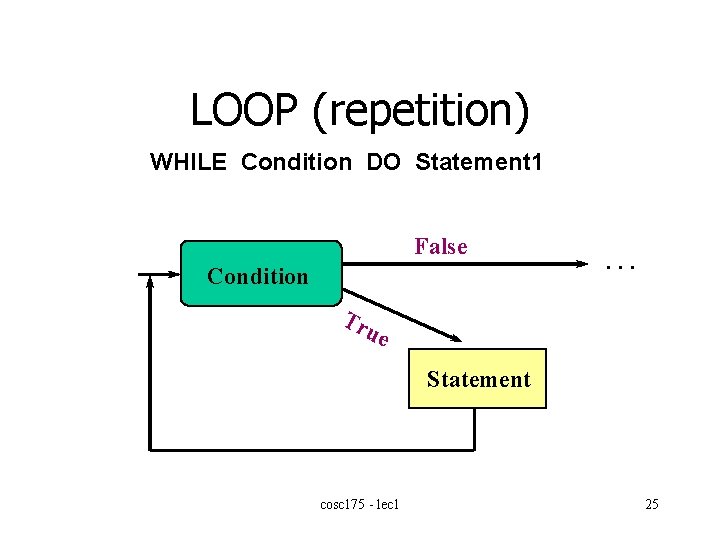
LOOP (repetition) WHILE Condition DO Statement 1 False Condition . . . Tr ue Statement cosc 175 - lec 1 25
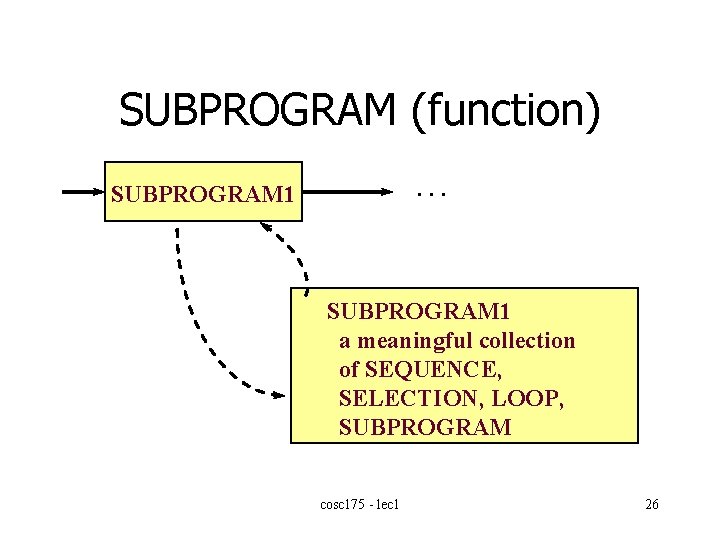
SUBPROGRAM (function). . . SUBPROGRAM 1 a meaningful collection of SEQUENCE, SELECTION, LOOP, SUBPROGRAM cosc 175 - lec 1 26
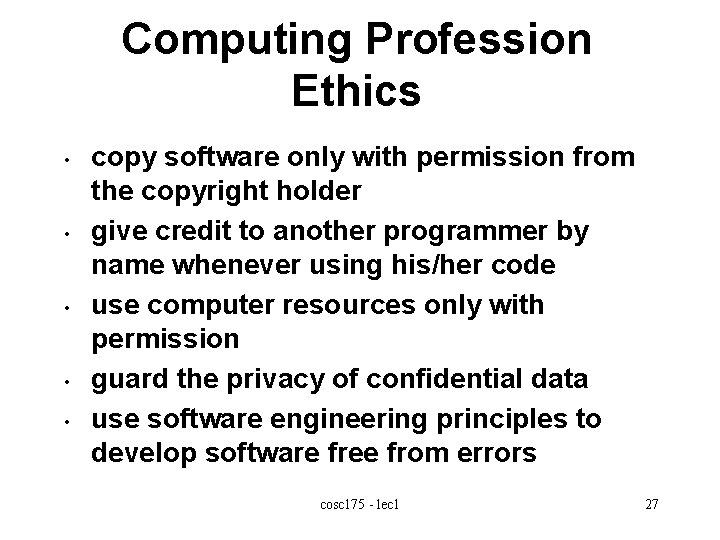
Computing Profession Ethics • • • copy software only with permission from the copyright holder give credit to another programmer by name whenever using his/her code use computer resources only with permission guard the privacy of confidential data use software engineering principles to develop software free from errors cosc 175 - lec 1 27
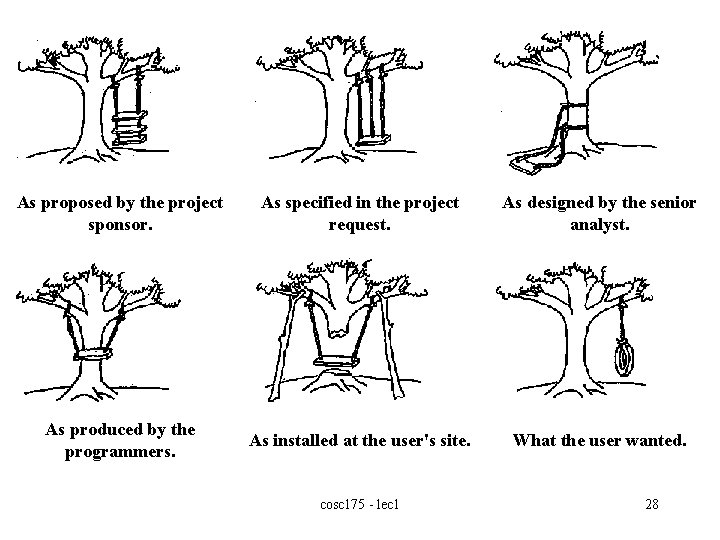
As proposed by the project sponsor. As specified in the project request. As produced by the programmers. As installed at the user's site. cosc 175 - lec 1 As designed by the senior analyst. What the user wanted. 28
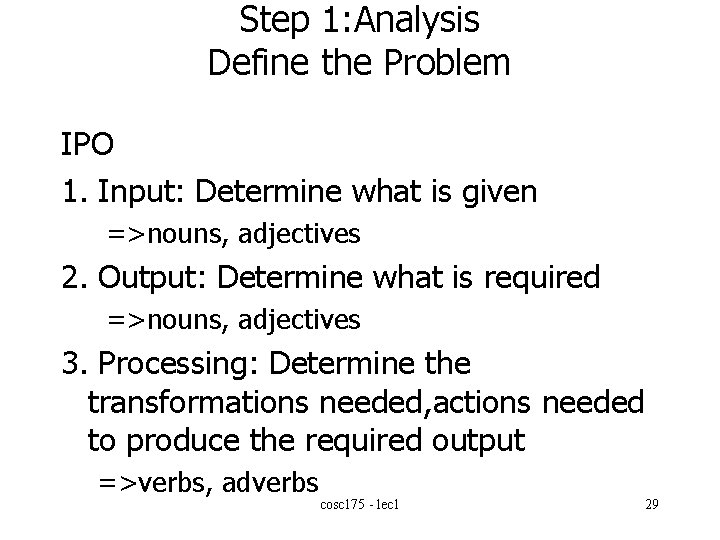
Step 1: Analysis Define the Problem IPO 1. Input: Determine what is given =>nouns, adjectives 2. Output: Determine what is required =>nouns, adjectives 3. Processing: Determine the transformations needed, actions needed to produce the required output =>verbs, adverbs cosc 175 - lec 1 29
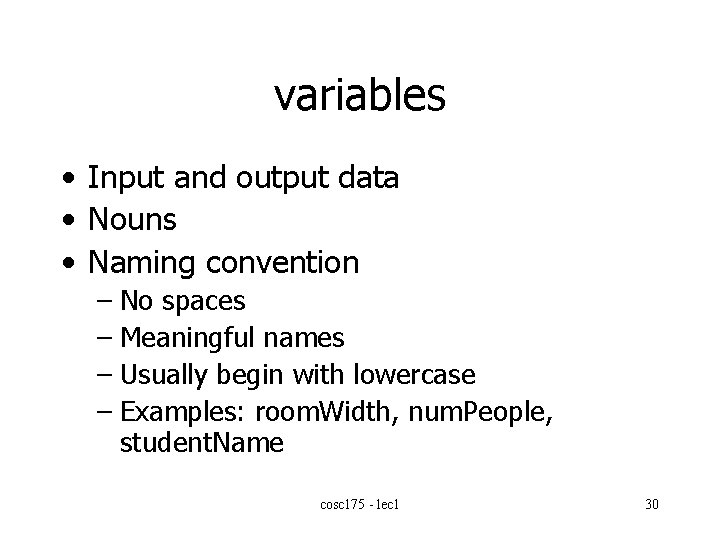
variables • Input and output data • Nouns • Naming convention – No spaces – Meaningful names – Usually begin with lowercase – Examples: room. Width, num. People, student. Name cosc 175 - lec 1 30
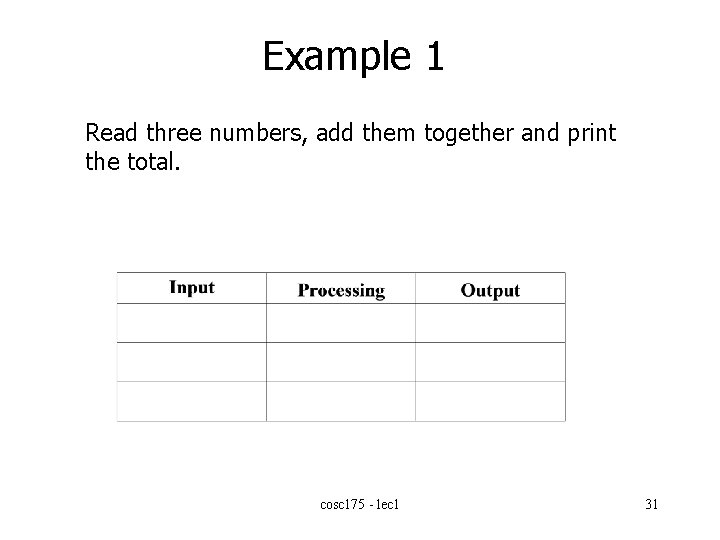
Example 1 Read three numbers, add them together and print the total. cosc 175 - lec 1 31
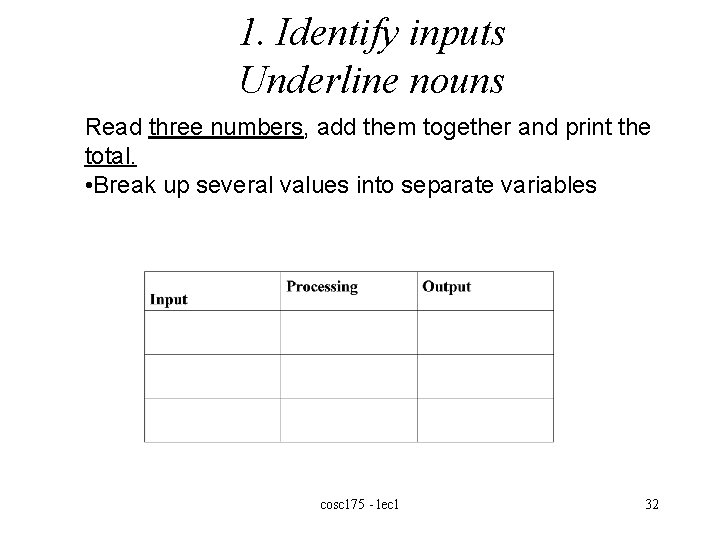
1. Identify inputs Underline nouns Read three numbers, add them together and print the total. • Break up several values into separate variables cosc 175 - lec 1 32
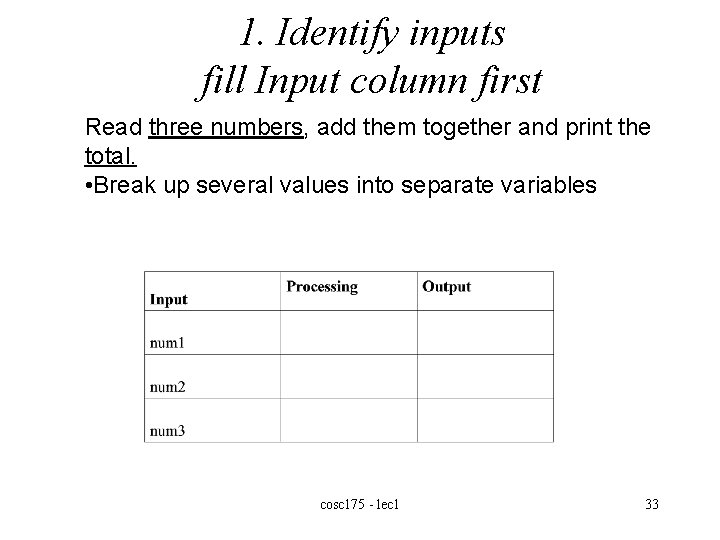
1. Identify inputs fill Input column first Read three numbers, add them together and print the total. • Break up several values into separate variables cosc 175 - lec 1 33
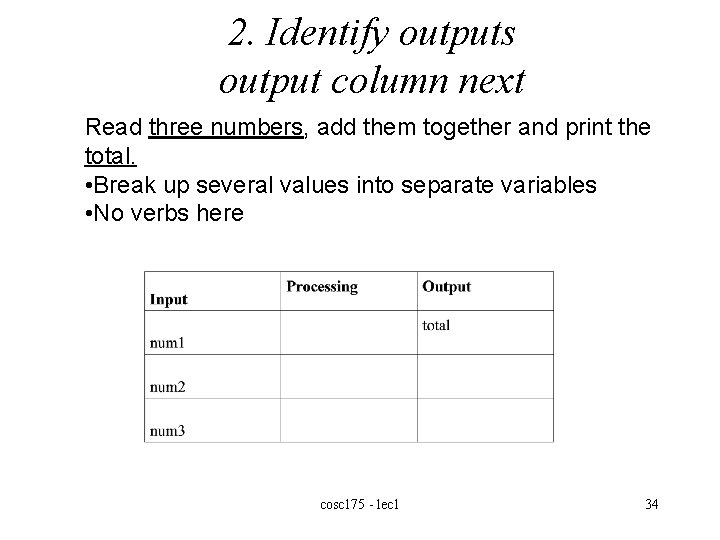
2. Identify outputs output column next Read three numbers, add them together and print the total. • Break up several values into separate variables • No verbs here cosc 175 - lec 1 34
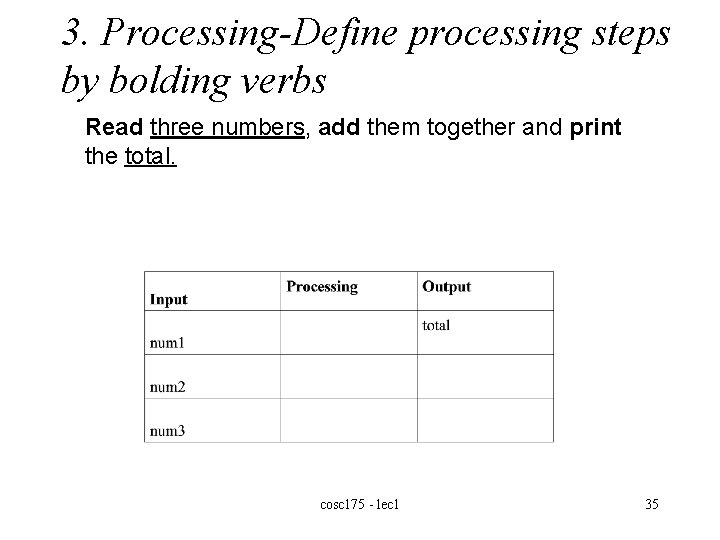
3. Processing-Define processing steps by bolding verbs Read three numbers, add them together and print the total. cosc 175 - lec 1 35
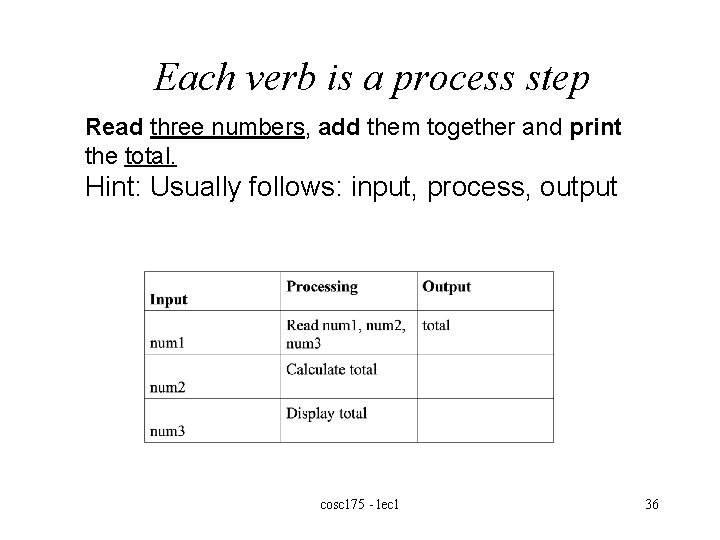
Each verb is a process step Read three numbers, add them together and print the total. Hint: Usually follows: input, process, output cosc 175 - lec 1 36
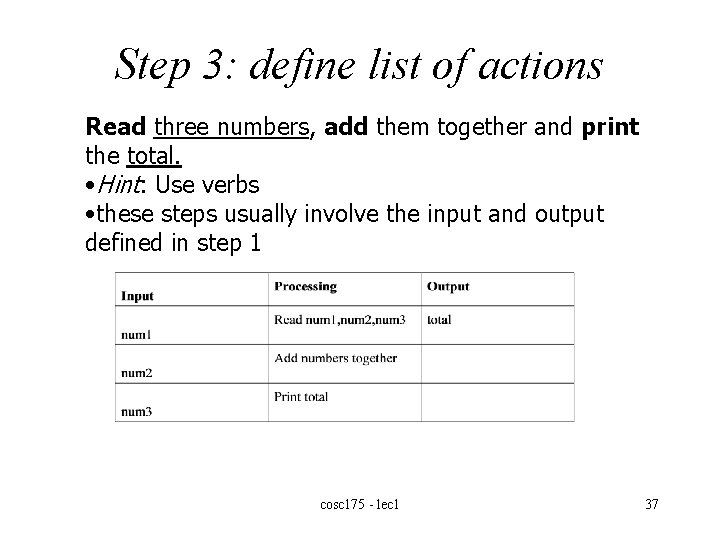
Step 3: define list of actions Read three numbers, add them together and print the total. • Hint: Use verbs • these steps usually involve the input and output defined in step 1 cosc 175 - lec 1 37
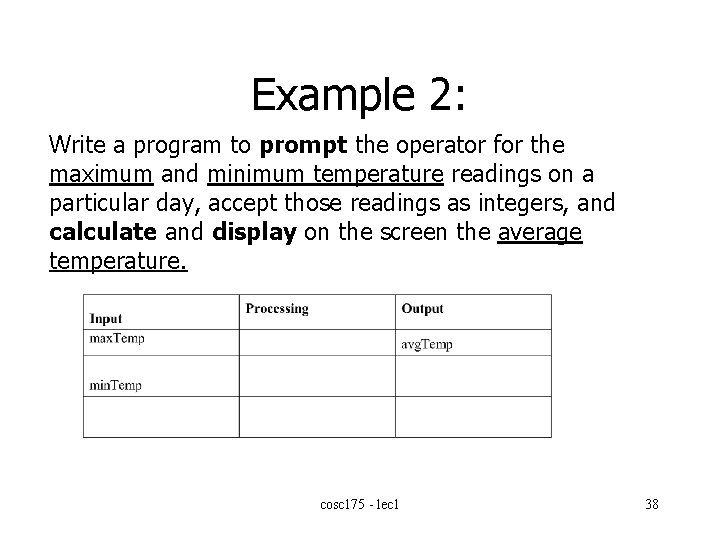
Example 2: Write a program to prompt the operator for the maximum and minimum temperature readings on a particular day, accept those readings as integers, and calculate and display on the screen the average temperature. cosc 175 - lec 1 38
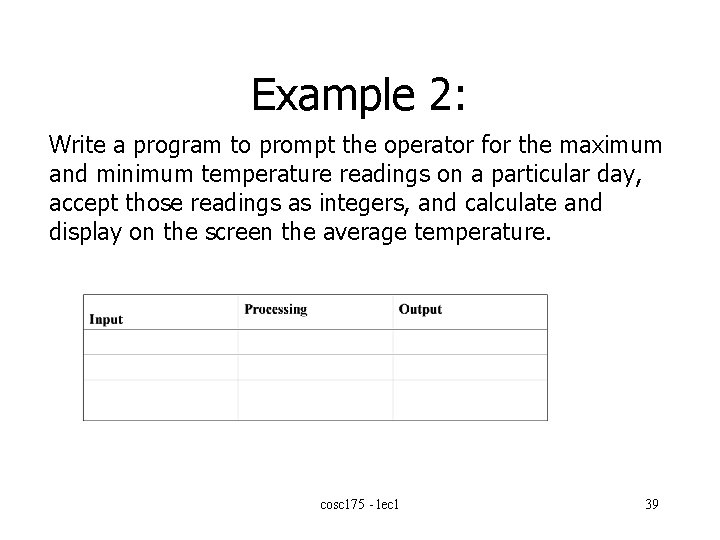
Example 2: Write a program to prompt the operator for the maximum and minimum temperature readings on a particular day, accept those readings as integers, and calculate and display on the screen the average temperature. cosc 175 - lec 1 39
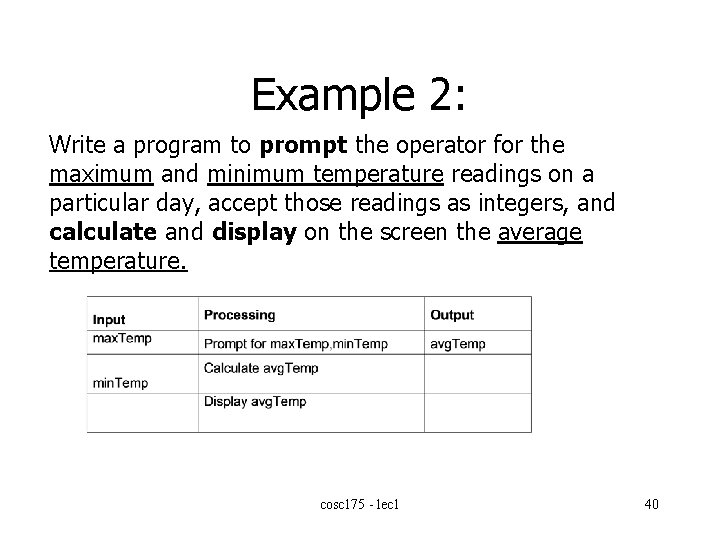
Example 2: Write a program to prompt the operator for the maximum and minimum temperature readings on a particular day, accept those readings as integers, and calculate and display on the screen the average temperature. cosc 175 - lec 1 40
History of software development life cycle
Software development life cycle models ppt
Object oriented system development life cycle
Odw 5e ch05 arrange the software development life cycle
Construction phase in software engineering
Sdlc maturity model
What is edlc
Application lifecycle management hp
Software brings computer hardware life how
Internal hardware components of a computer
Programming life cycle (plc)
Slidetodoc.com
Be next frontier software development
Android programming overview
Hardware description language vs programming language
Hardware programming language
Traditional systems development life cycle
Sdlc
New product development and product life cycle strategies
Database system development life cycle adalah
Multimedia development life cycle
System development life cycle
Expert system development life cycle
Dbms life cycle
Expert system development life cycle
Document development life cycle
System development life cycle of electronic health records
Sdlc technical writing
Network development life cycle
Object oriented development life cycle
System development life cycle
System development life cycle
System development life cycle ppt
Pengembangan produk baru dan strategi siklus hidup produk
System development of cross life cycle is
When to use sdlc
Traditional systems development life cycle
Object oriented development life cycle
6 stages of system life cycle
Ibm software development process
Ibm software life cycle