Designing Classes CSC 1051 Data Structures and Algorithms
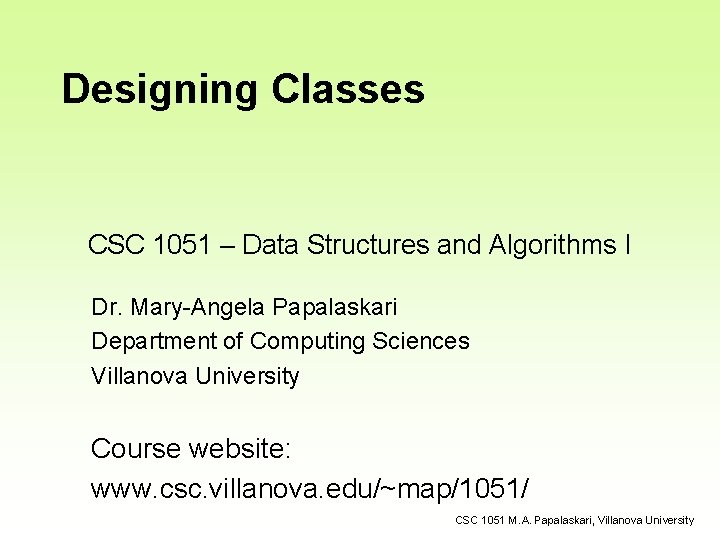
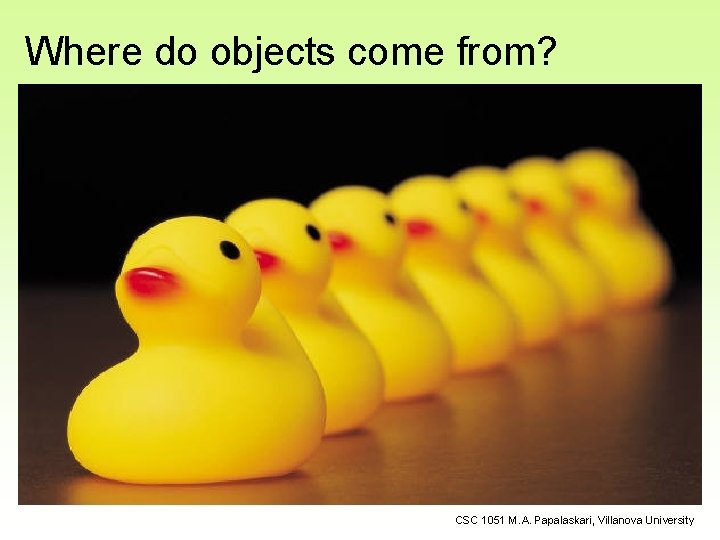
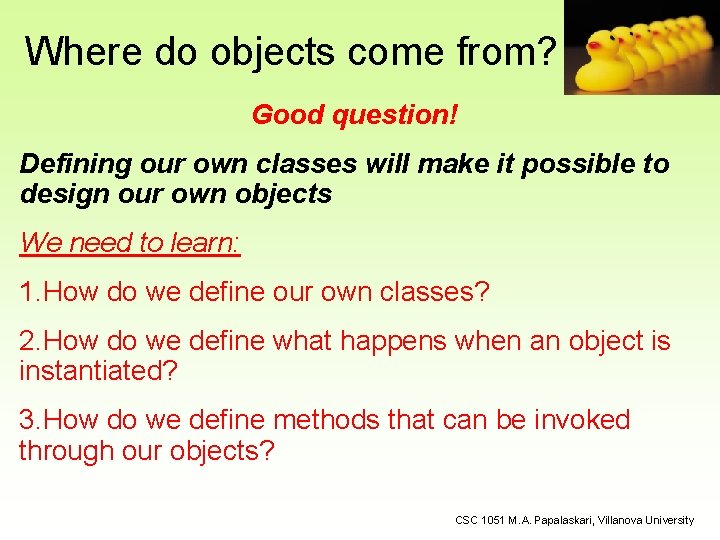
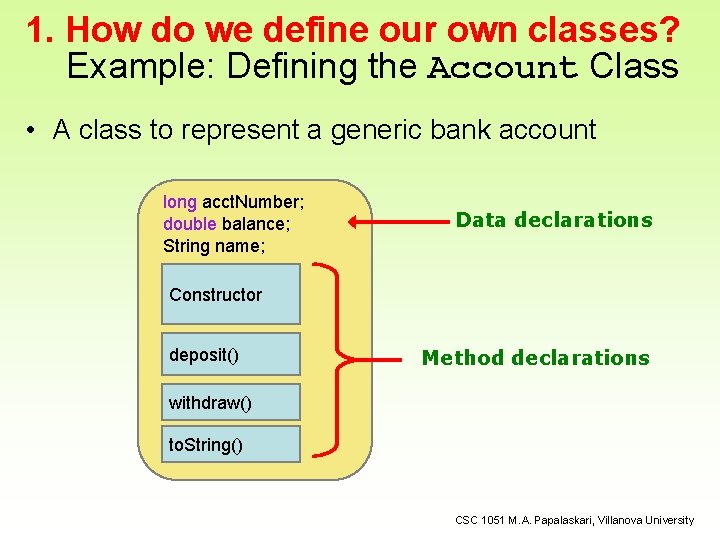
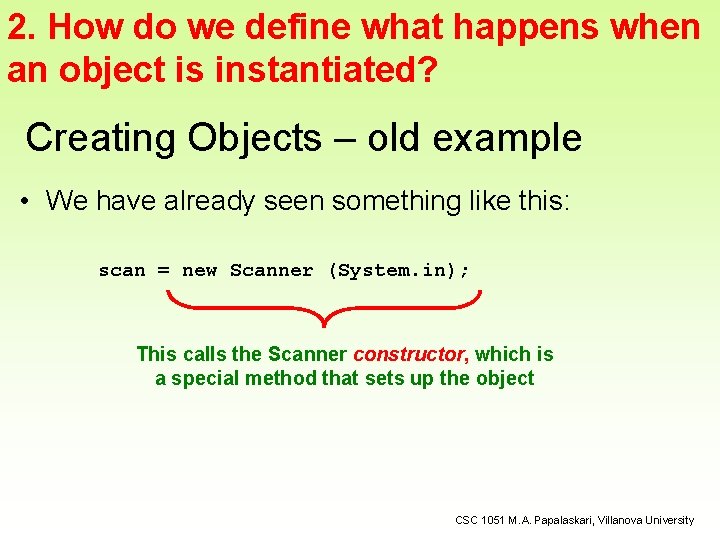
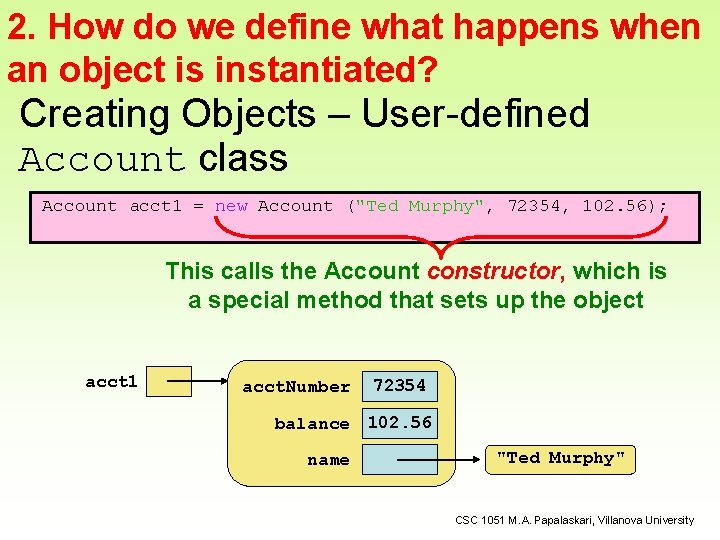
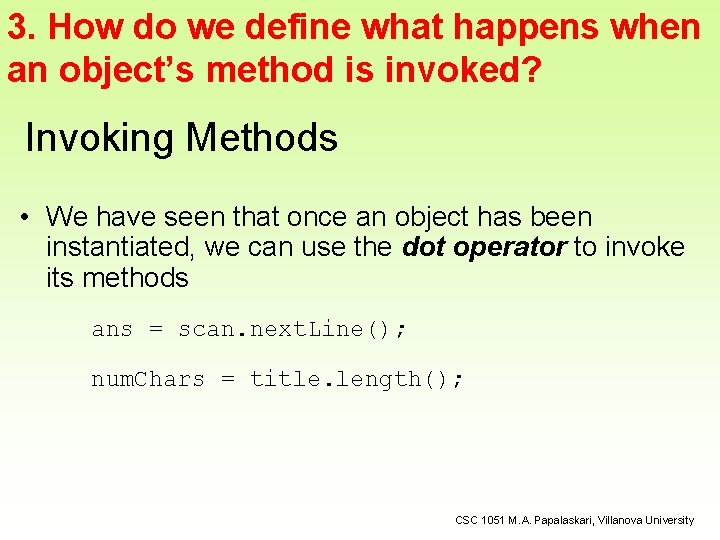
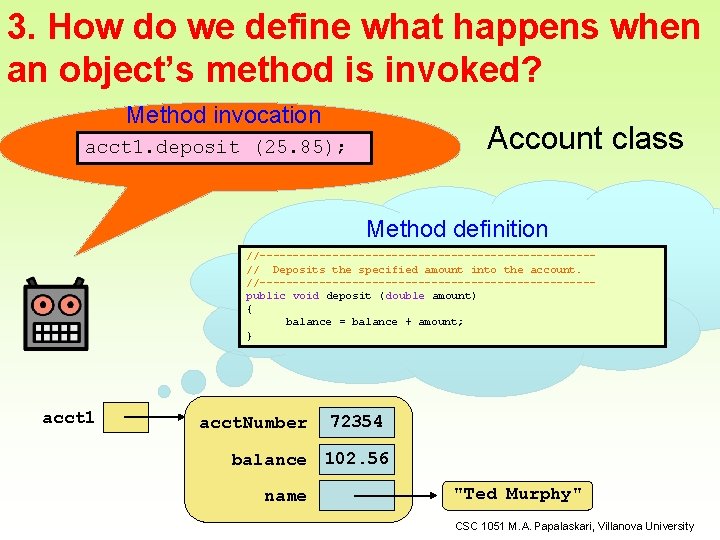
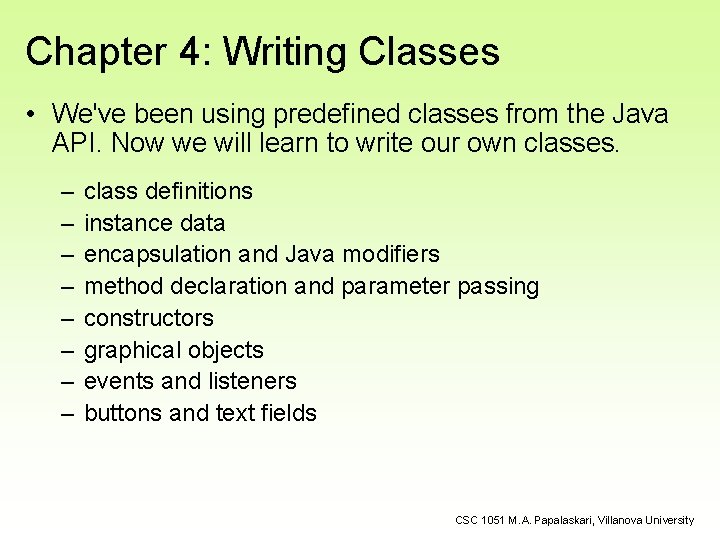
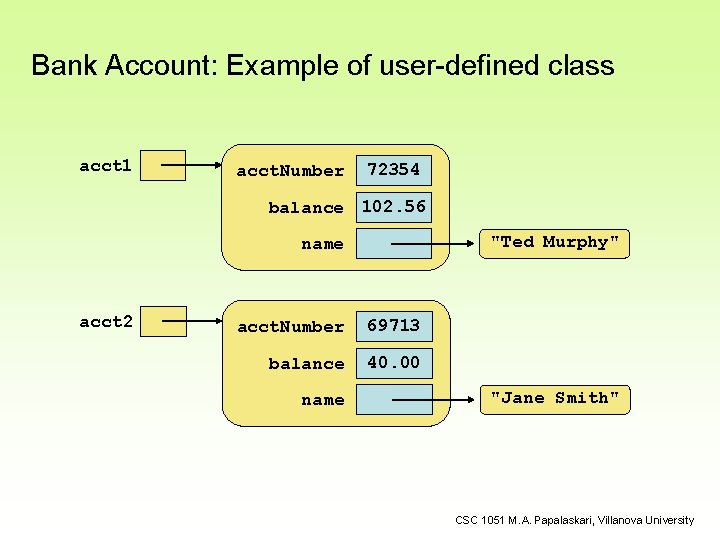
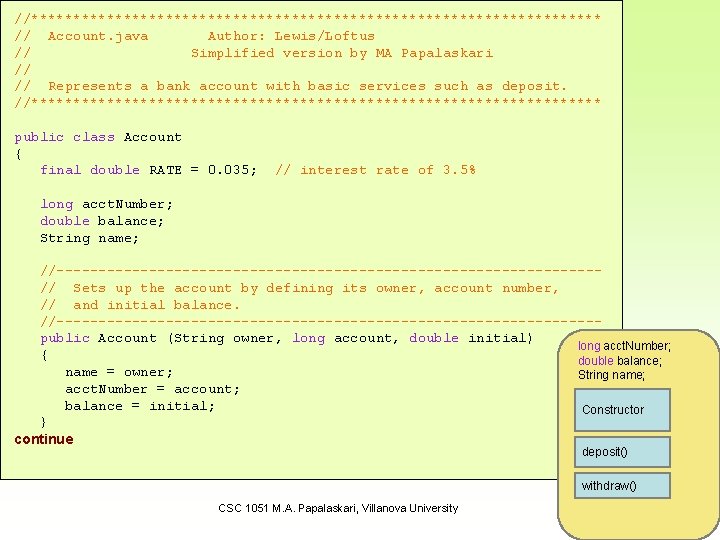
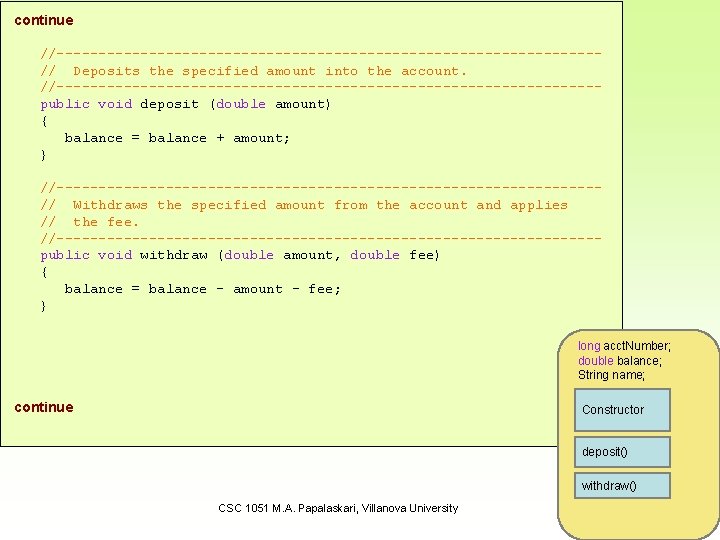
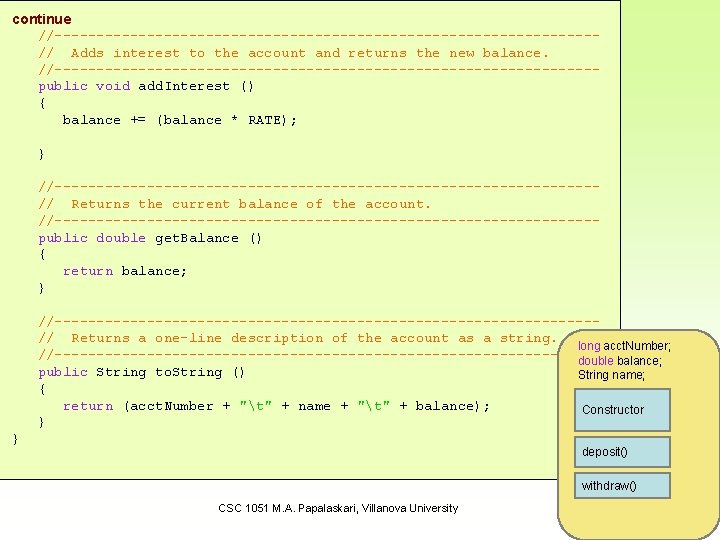
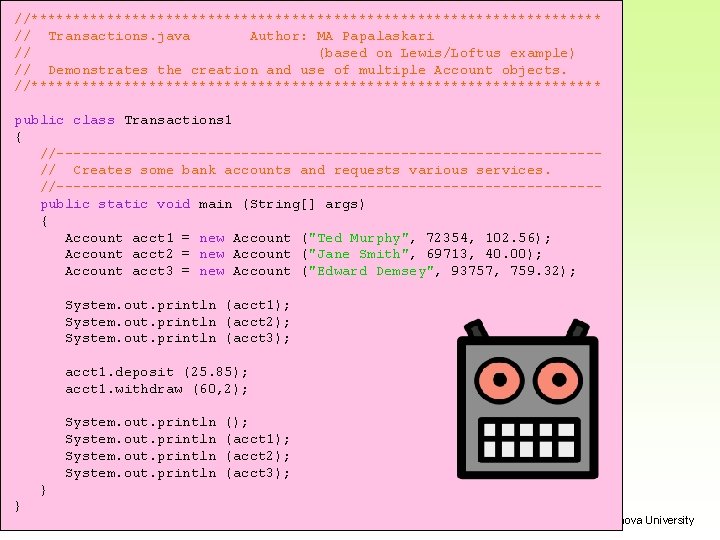
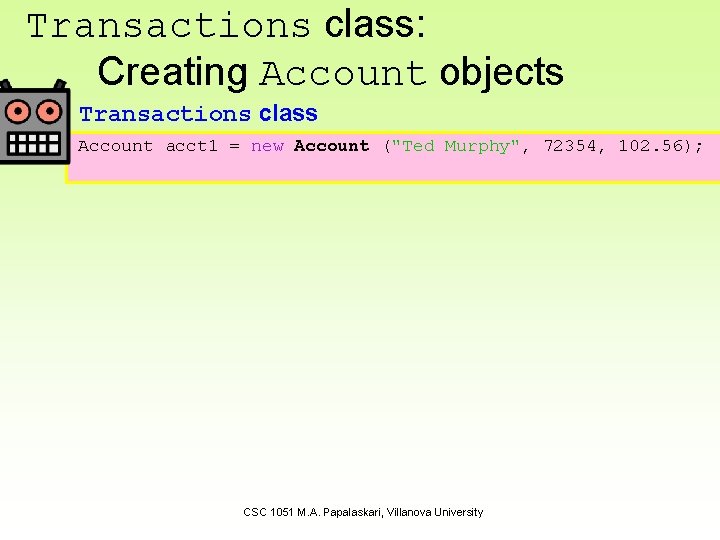
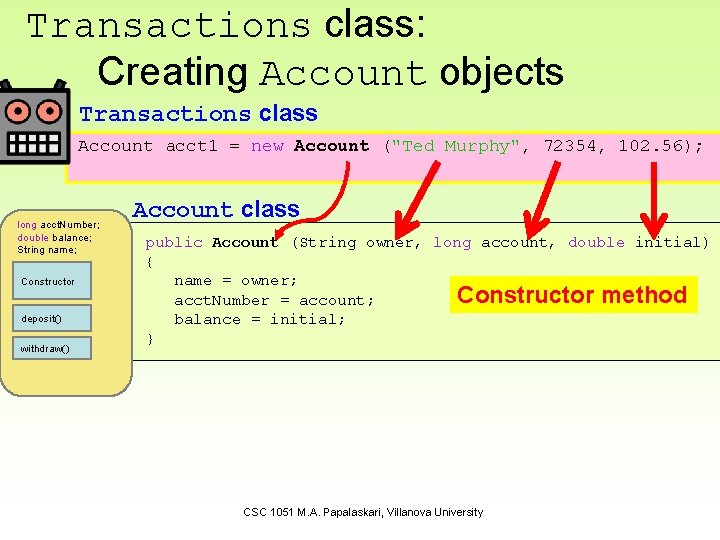
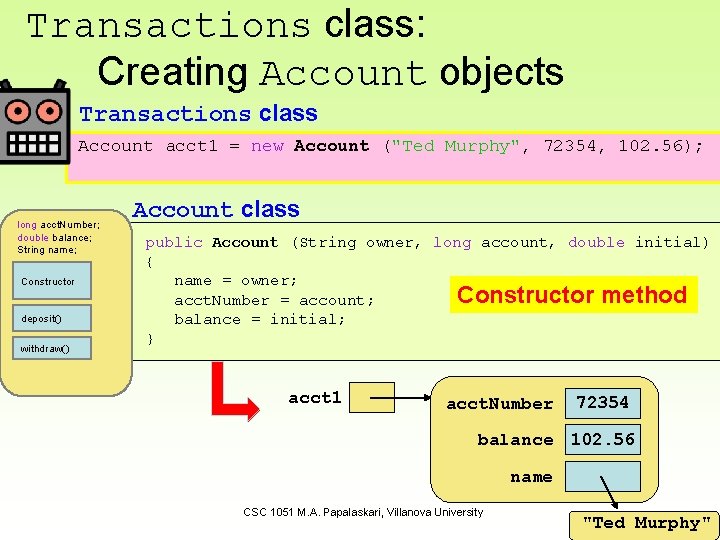
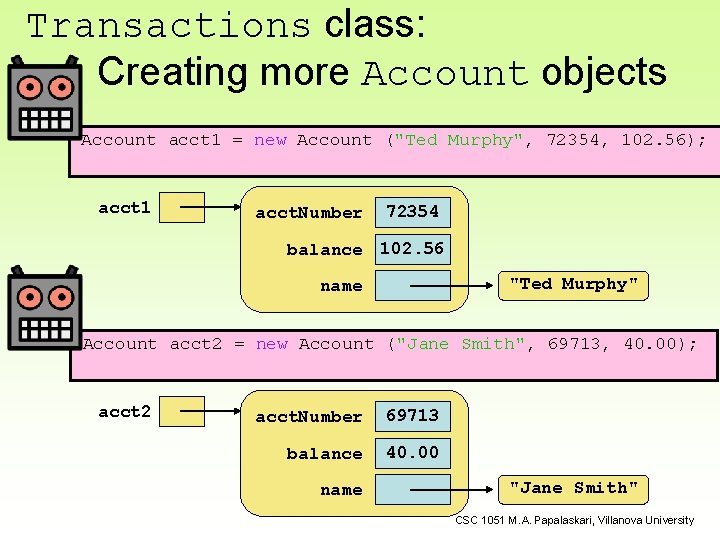
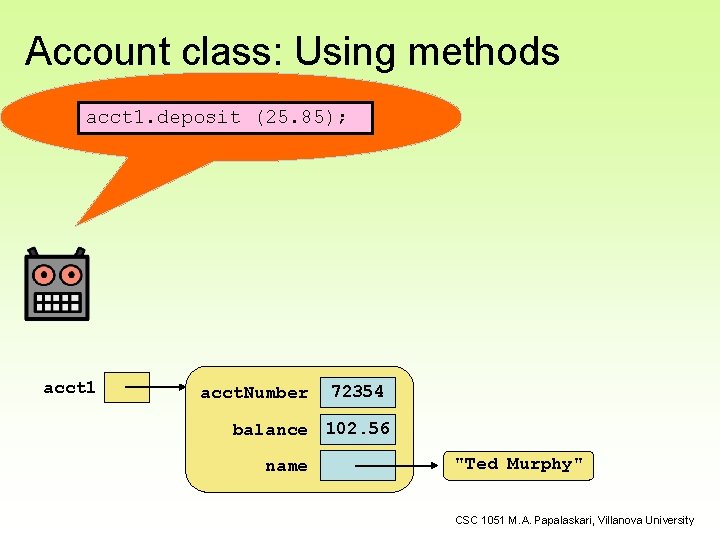
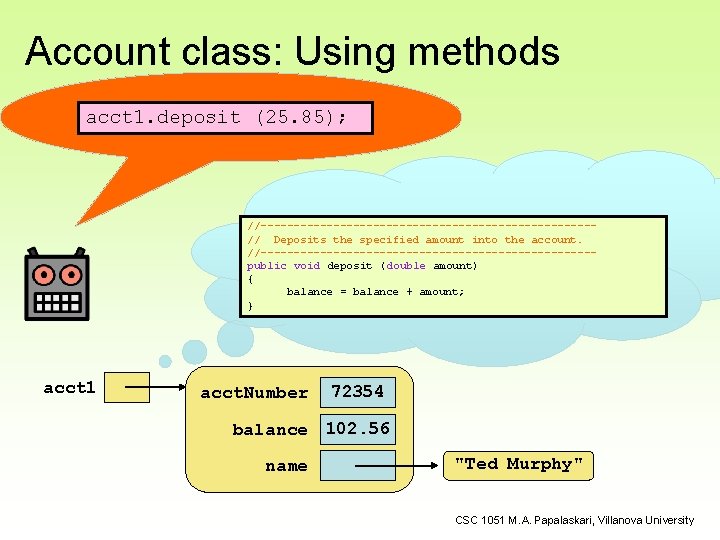
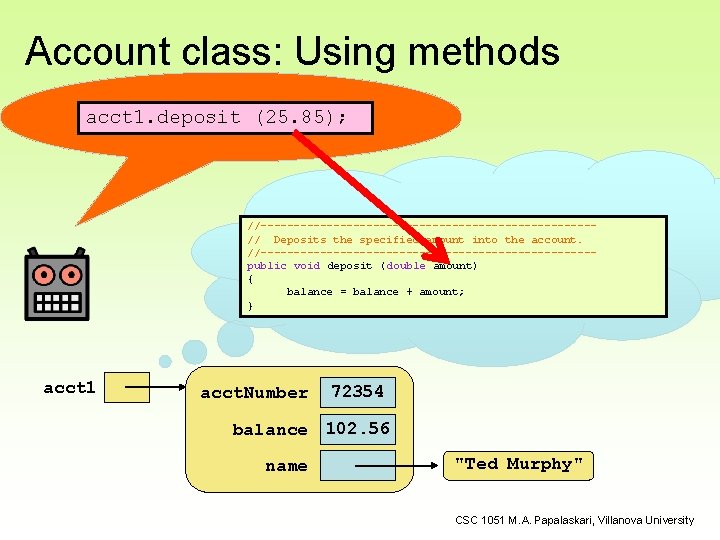
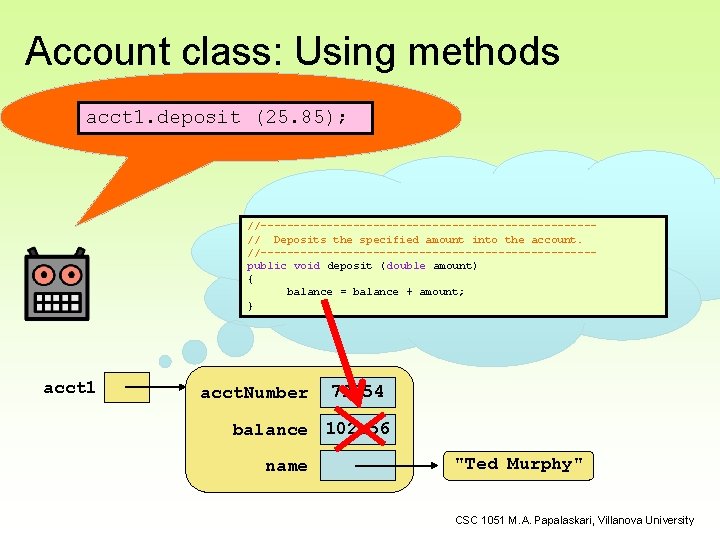
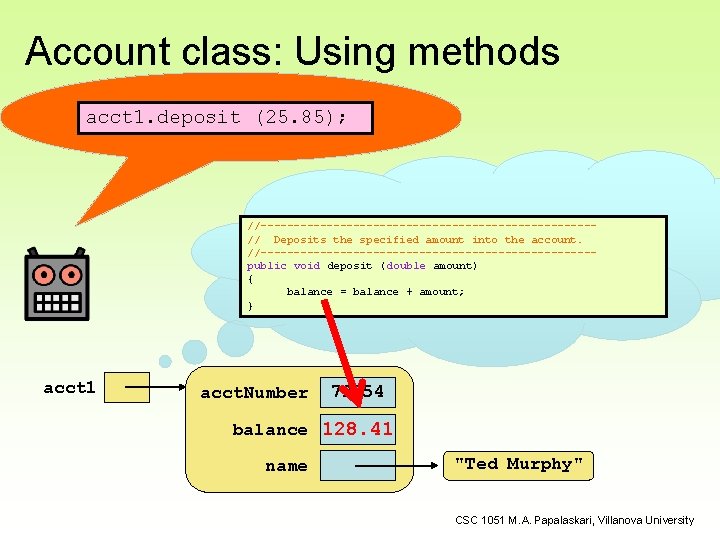
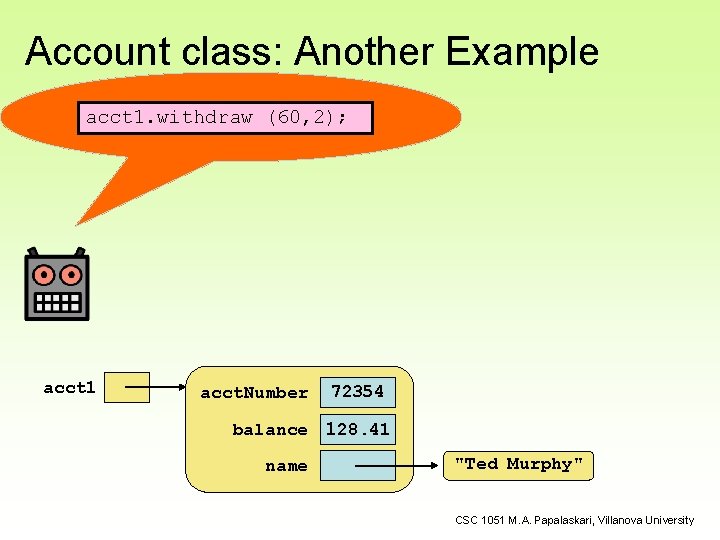
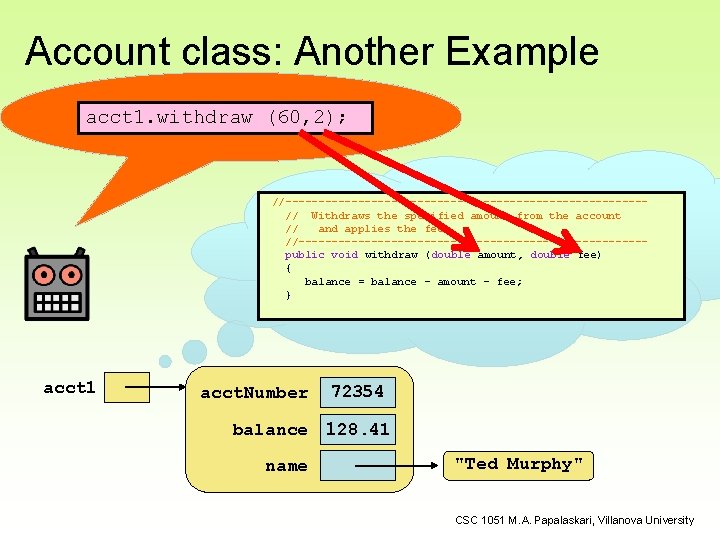
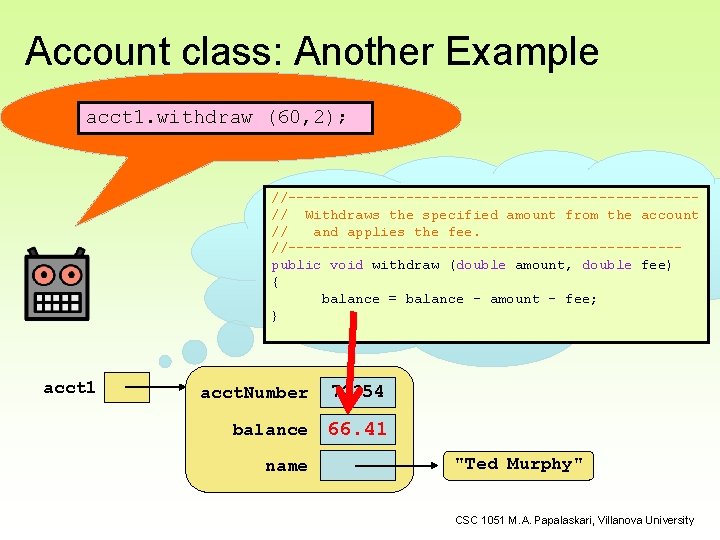
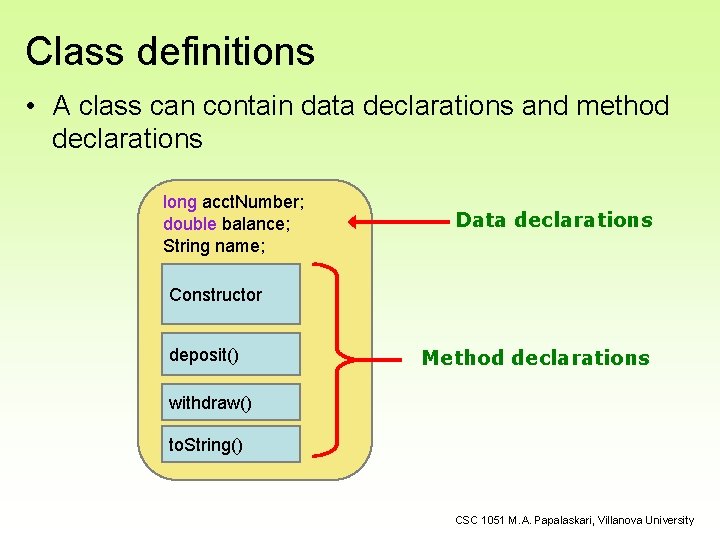
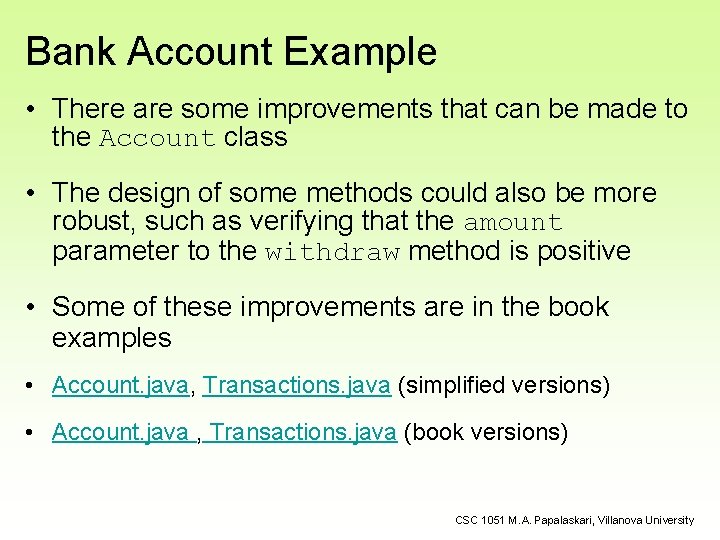
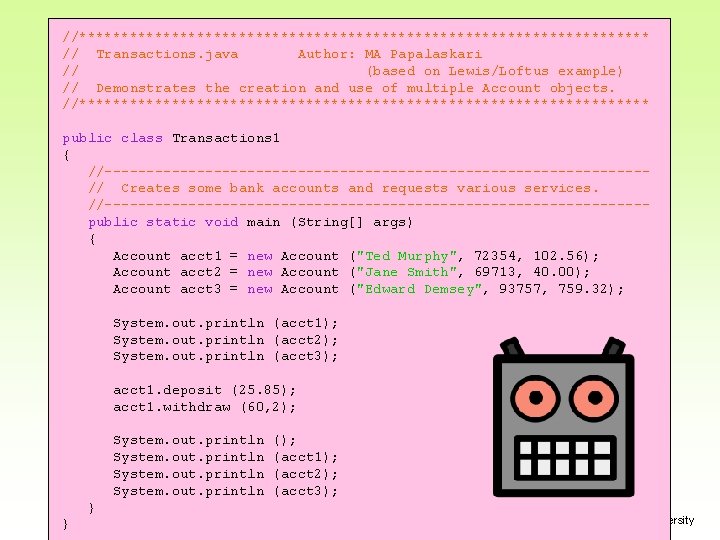
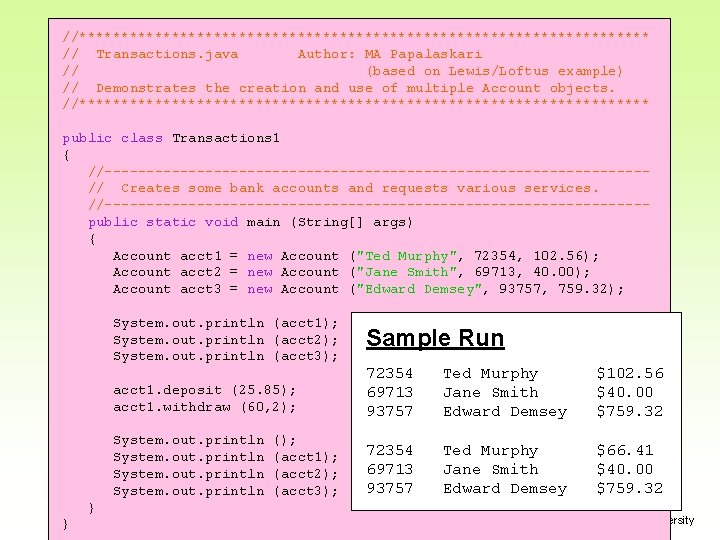
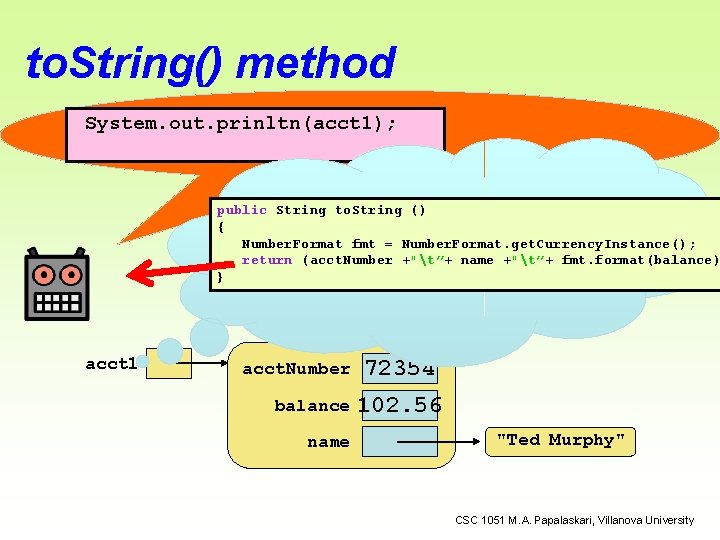
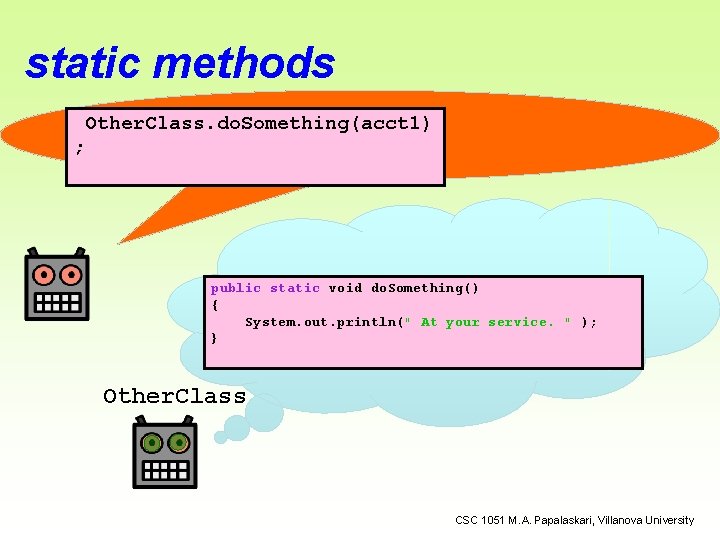
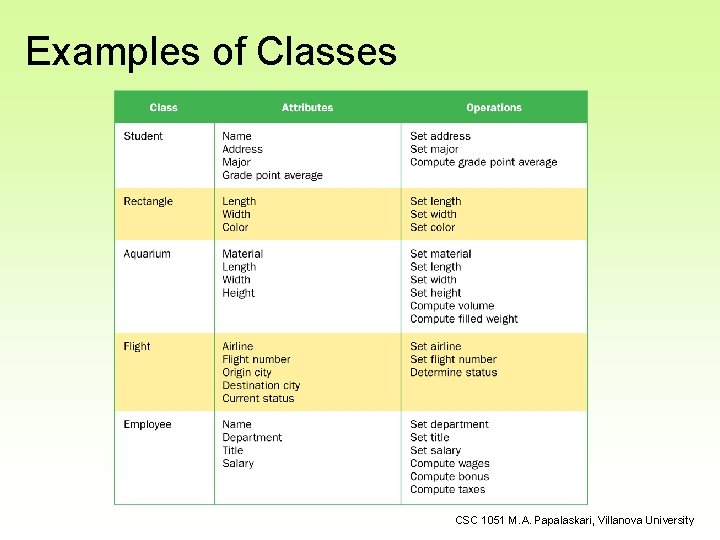
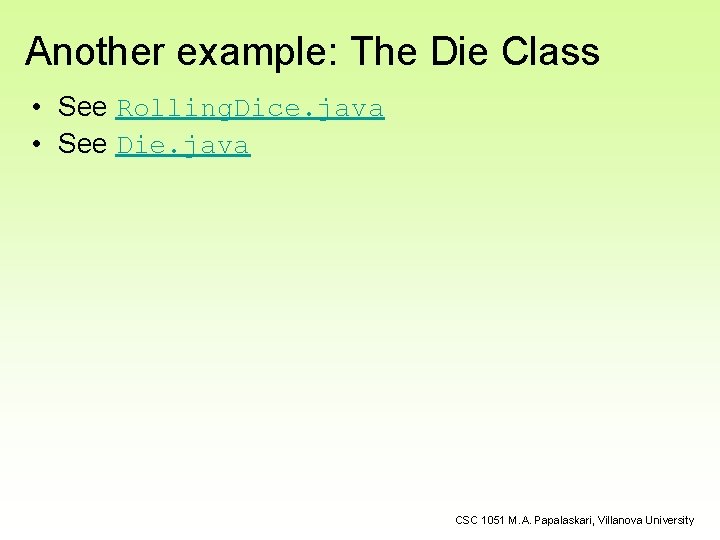
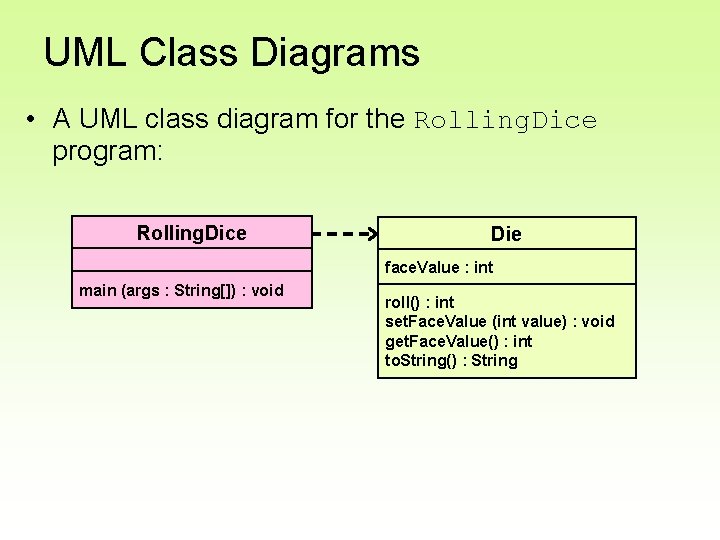
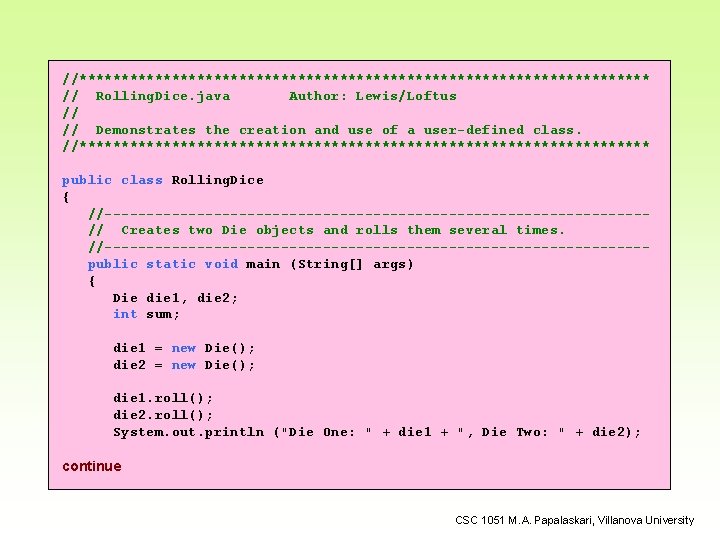
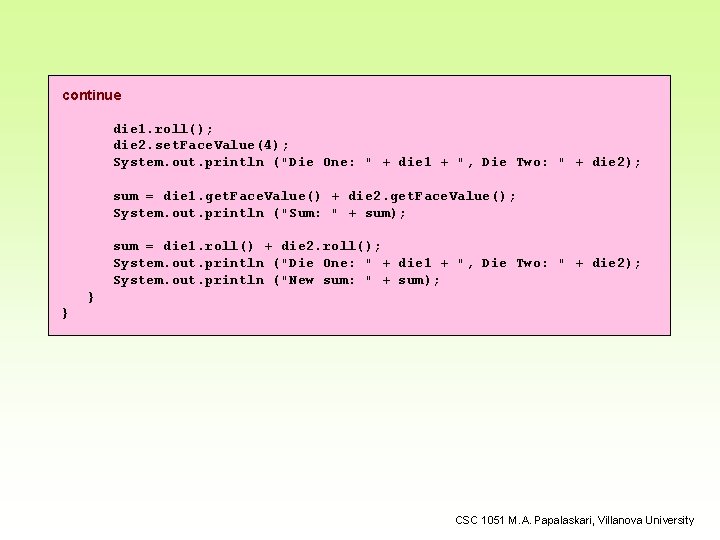
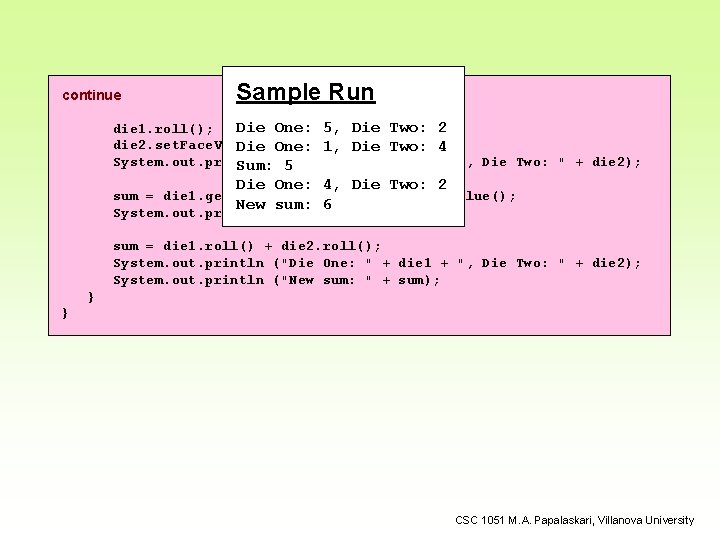
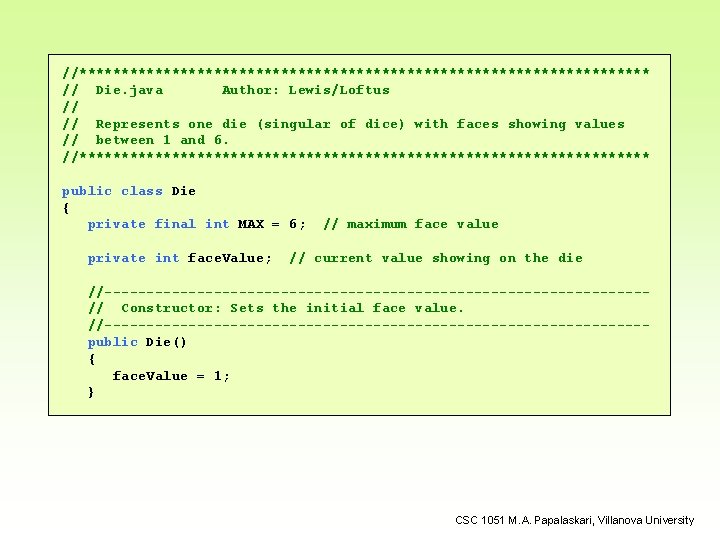
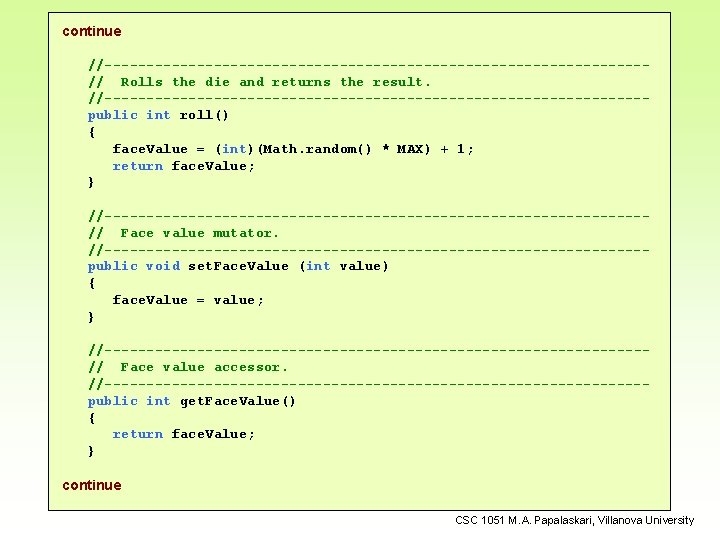
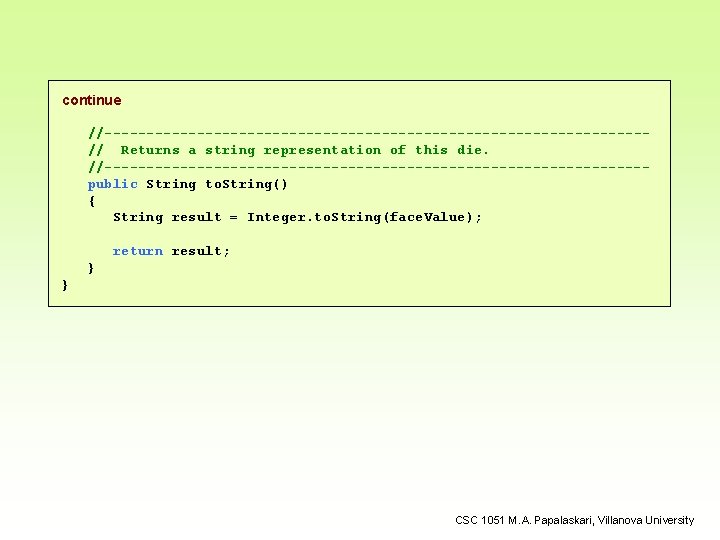
- Slides: 41
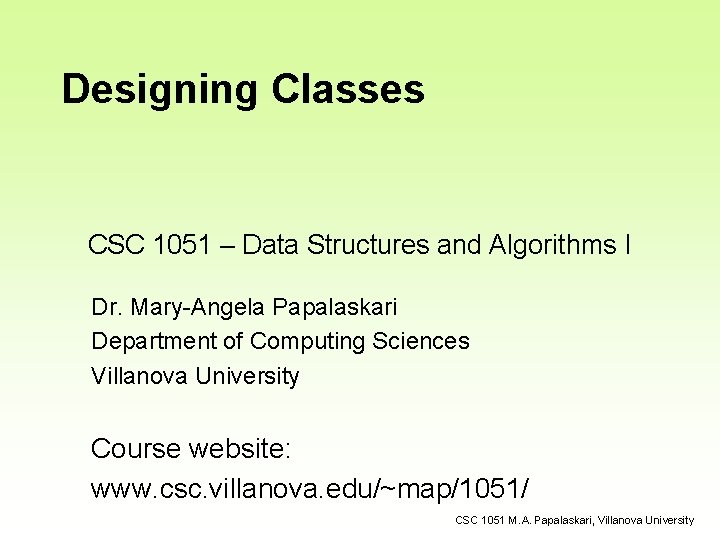
Designing Classes CSC 1051 – Data Structures and Algorithms I Dr. Mary-Angela Papalaskari Department of Computing Sciences Villanova University Course website: www. csc. villanova. edu/~map/1051/ CSC 1051 M. A. Papalaskari, Villanova University
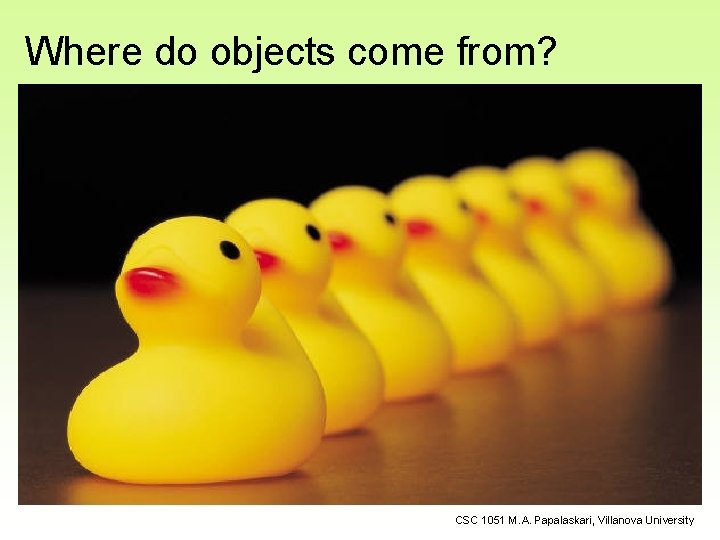
Where do objects come from? CSC 1051 M. A. Papalaskari, Villanova University
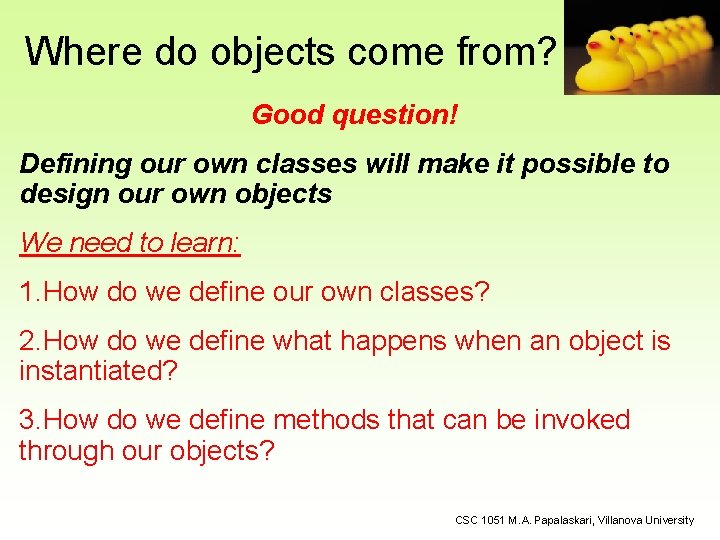
Where do objects come from? Good question! Defining our own classes will make it possible to design our own objects We need to learn: 1. How do we define our own classes? 2. How do we define what happens when an object is instantiated? 3. How do we define methods that can be invoked through our objects? CSC 1051 M. A. Papalaskari, Villanova University
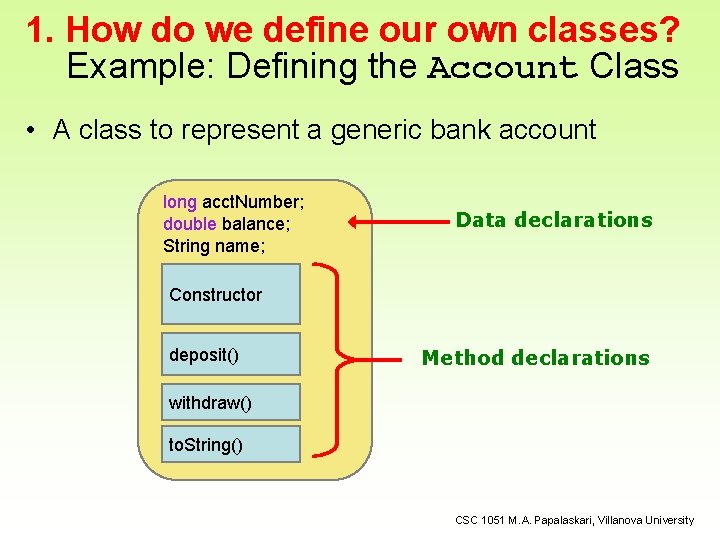
1. How do we define our own classes? Example: Defining the Account Class • A class to represent a generic bank account long acct. Number; double balance; String name; Data declarations Constructor deposit() Method declarations withdraw() to. String() CSC 1051 M. A. Papalaskari, Villanova University
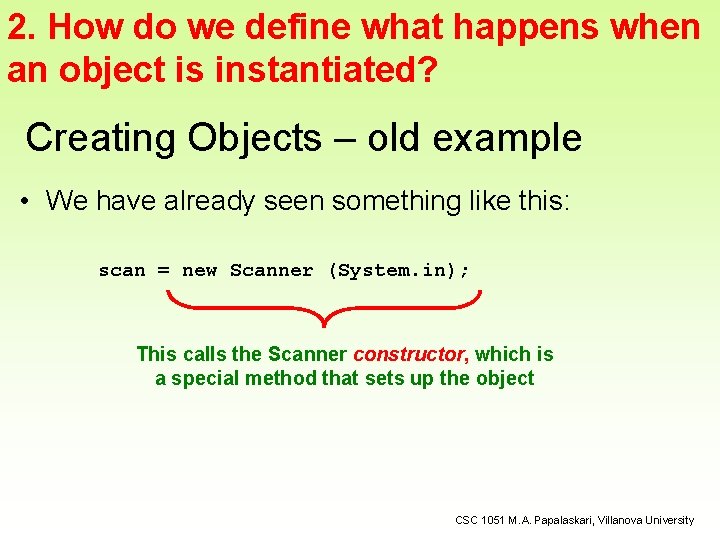
2. How do we define what happens when an object is instantiated? Creating Objects – old example • We have already seen something like this: scan = new Scanner (System. in); This calls the Scanner constructor, which is a special method that sets up the object CSC 1051 M. A. Papalaskari, Villanova University
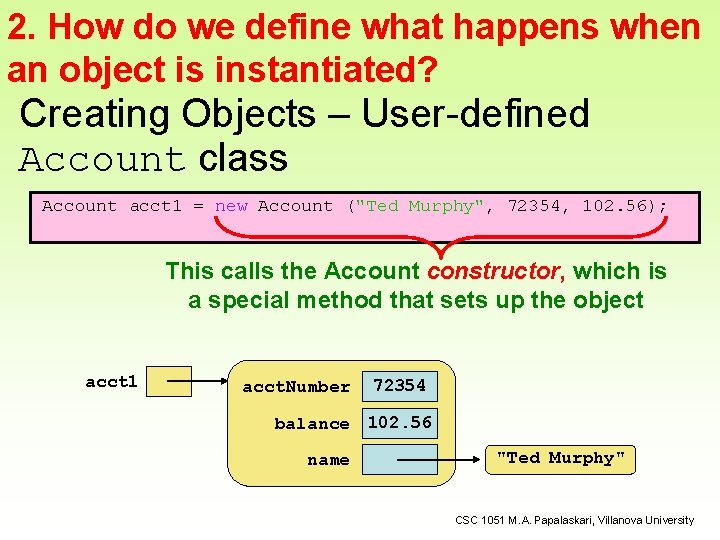
2. How do we define what happens when an object is instantiated? Creating Objects – User-defined Account class Account acct 1 = new Account ("Ted Murphy", 72354, 102. 56); This calls the Account constructor, which is a special method that sets up the object acct 1 acct. Number 72354 balance 102. 56 name "Ted Murphy" CSC 1051 M. A. Papalaskari, Villanova University
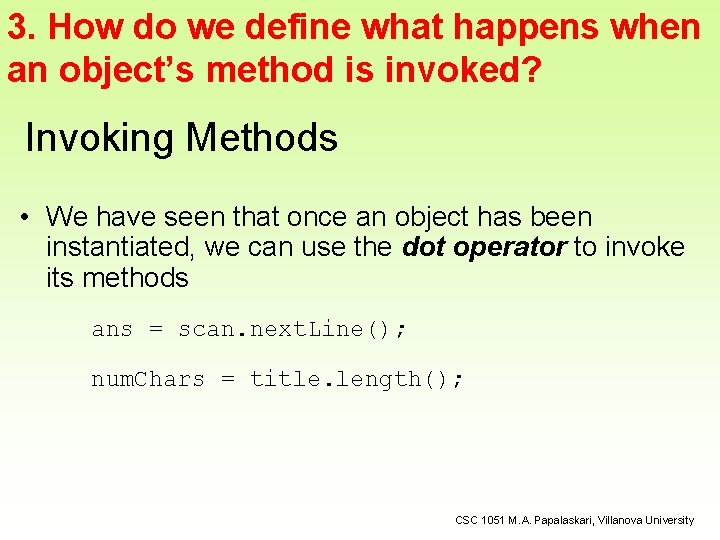
3. How do we define what happens when an object’s method is invoked? Invoking Methods • We have seen that once an object has been instantiated, we can use the dot operator to invoke its methods ans = scan. next. Line(); num. Chars = title. length(); CSC 1051 M. A. Papalaskari, Villanova University
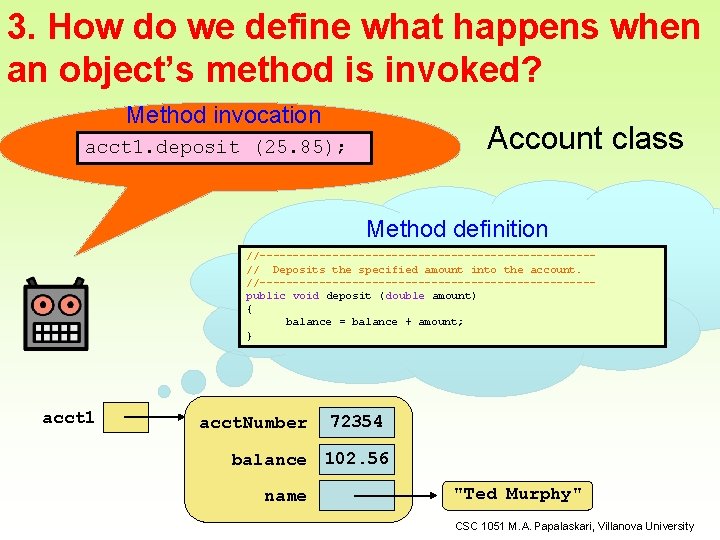
3. How do we define what happens when an object’s method is invoked? Method invocation Account class acct 1. deposit Me (25. 85); Method definition //-------------------------// Deposits the specified amount into the account. //-------------------------public void deposit (double amount) { balance = balance + amount; } acct 1 acct. Number 72354 balance 102. 56 name "Ted Murphy" CSC 1051 M. A. Papalaskari, Villanova University
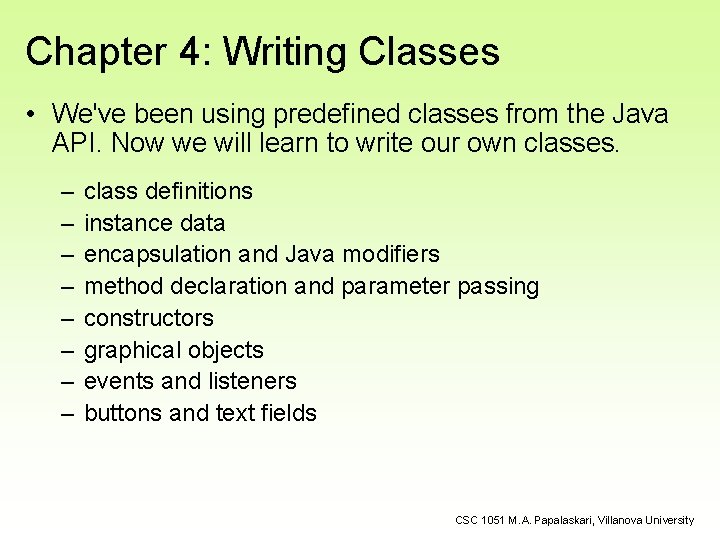
Chapter 4: Writing Classes • We've been using predefined classes from the Java API. Now we will learn to write our own classes. – – – – class definitions instance data encapsulation and Java modifiers method declaration and parameter passing constructors graphical objects events and listeners buttons and text fields CSC 1051 M. A. Papalaskari, Villanova University
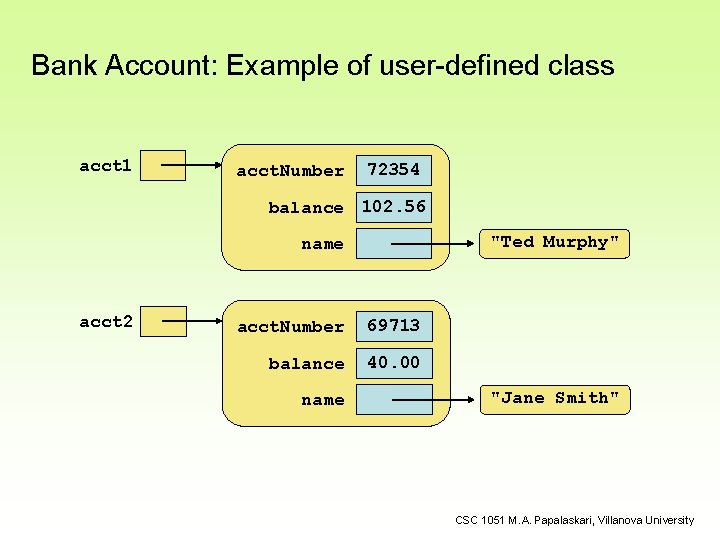
Bank Account: Example of user-defined class acct 1 acct. Number 72354 balance 102. 56 "Ted Murphy" name acct 2 acct. Number 69713 balance 40. 00 name "Jane Smith" CSC 1051 M. A. Papalaskari, Villanova University
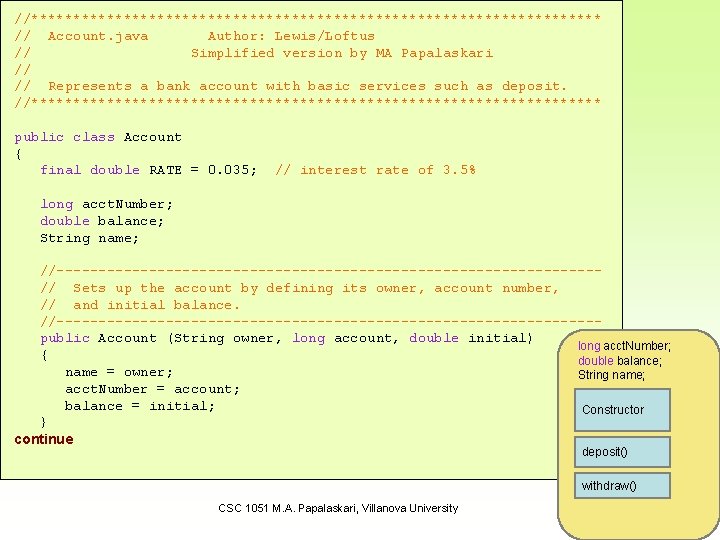
//********************************** // Account. java Author: Lewis/Loftus // Simplified version by MA Papalaskari // // Represents a bank account with basic services such as deposit. //********************************** public class Account { final double RATE = 0. 035; // interest rate of 3. 5% long acct. Number; double balance; String name; //--------------------------------// Sets up the account by defining its owner, account number, // and initial balance. //--------------------------------public Account (String owner, long account, double initial) long acct. Number; { double balance; name = owner; String name; acct. Number = account; balance = initial; Constructor } continue deposit() withdraw() CSC 1051 M. A. Papalaskari, Villanova University
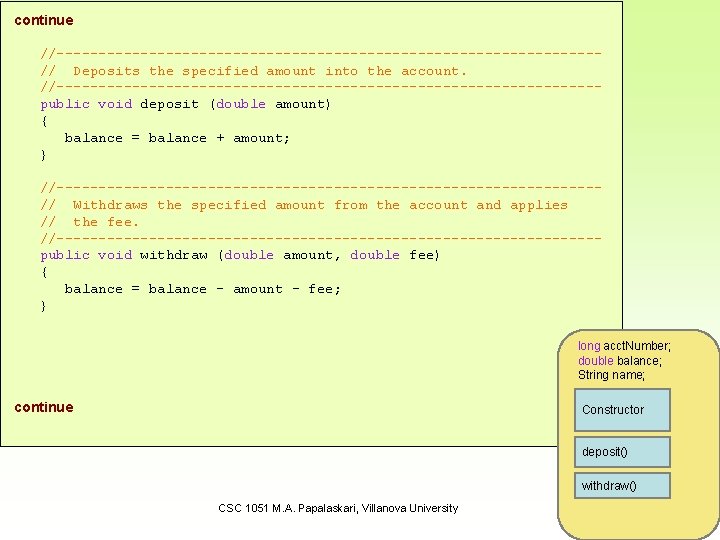
continue //--------------------------------// Deposits the specified amount into the account. //--------------------------------public void deposit (double amount) { balance = balance + amount; } //--------------------------------// Withdraws the specified amount from the account and applies // the fee. //--------------------------------public void withdraw (double amount, double fee) { balance = balance - amount - fee; } long acct. Number; double balance; String name; continue Constructor deposit() withdraw() CSC 1051 M. A. Papalaskari, Villanova University
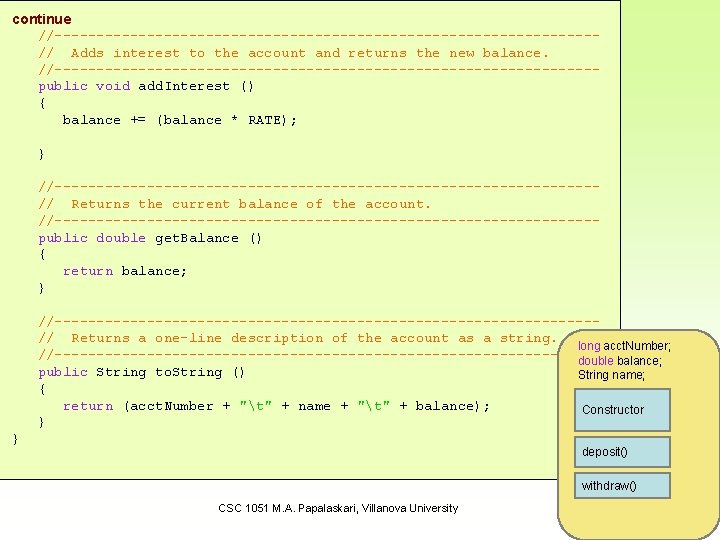
continue //--------------------------------// Adds interest to the account and returns the new balance. //--------------------------------public void add. Interest () { balance += (balance * RATE); } //--------------------------------// Returns the current balance of the account. //--------------------------------public double get. Balance () { return balance; } //--------------------------------// Returns a one-line description of the account as a string. long acct. Number; //--------------------------------double balance; public String to. String () String name; { return (acct. Number + "t" + name + "t" + balance); Constructor } } deposit() withdraw() CSC 1051 M. A. Papalaskari, Villanova University
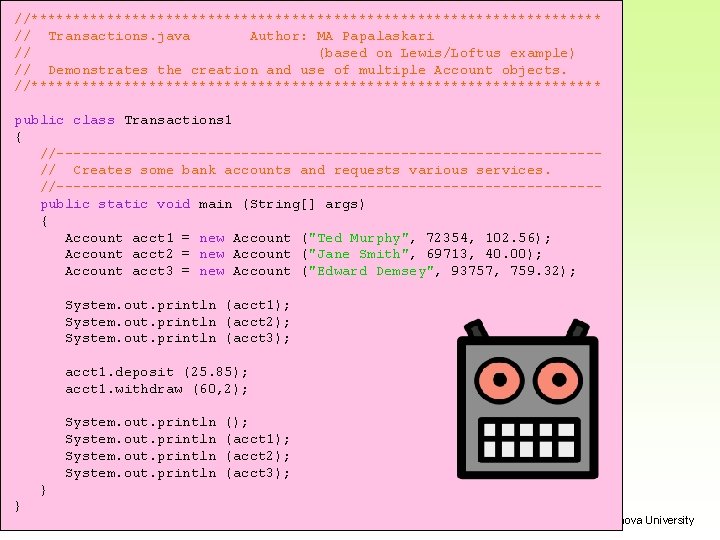
//********************************** // Transactions. java Author: MA Papalaskari // (based on Lewis/Loftus example) // Demonstrates the creation and use of multiple Account objects. //********************************** public class Transactions 1 { //--------------------------------// Creates some bank accounts and requests various services. //--------------------------------public static void main (String[] args) { Account acct 1 = new Account ("Ted Murphy", 72354, 102. 56); Account acct 2 = new Account ("Jane Smith", 69713, 40. 00); Account acct 3 = new Account ("Edward Demsey", 93757, 759. 32); System. out. println (acct 1); System. out. println (acct 2); System. out. println (acct 3); acct 1. deposit (25. 85); acct 1. withdraw (60, 2); System. out. println (); (acct 1); (acct 2); (acct 3); } } CSC 1051 M. A. Papalaskari, Villanova University
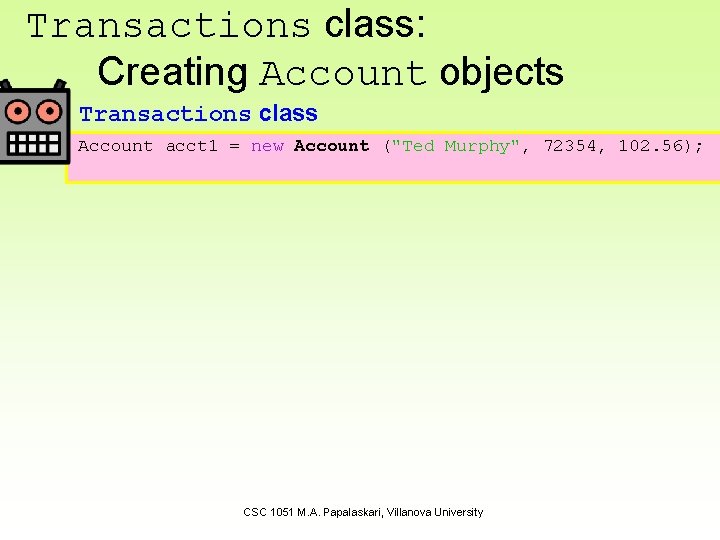
Transactions class: Creating Account objects Transactions class Account acct 1 = new Account ("Ted Murphy", 72354, 102. 56); CSC 1051 M. A. Papalaskari, Villanova University
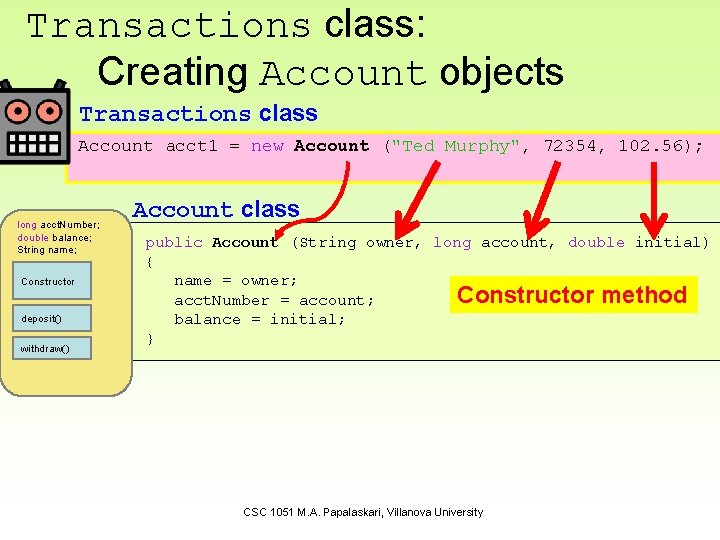
Transactions class: Creating Account objects Transactions class Account acct 1 = new Account ("Ted Murphy", 72354, 102. 56); long acct. Number; double balance; String name; Constructor deposit() withdraw() Account class public Account (String owner, long account, double initial) { name = owner; Constructor method acct. Number = account; balance = initial; } CSC 1051 M. A. Papalaskari, Villanova University
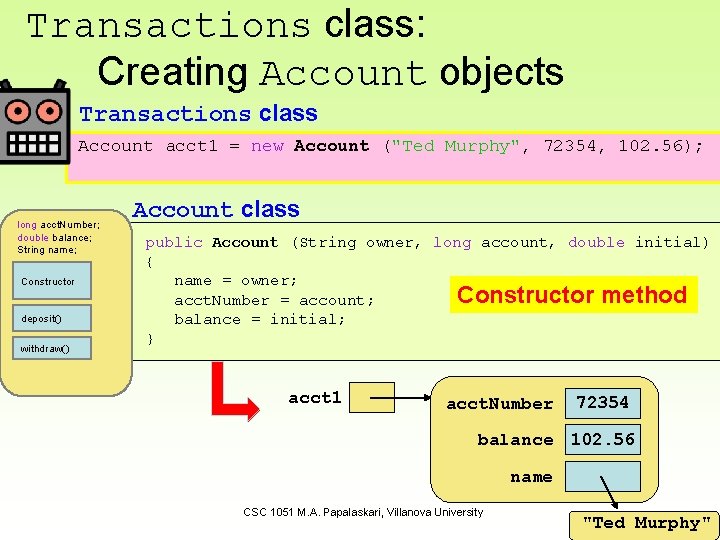
Transactions class: Creating Account objects Transactions class Account acct 1 = new Account ("Ted Murphy", 72354, 102. 56); long acct. Number; double balance; String name; Constructor deposit() withdraw() Account class public Account (String owner, long account, double initial) { name = owner; Constructor method acct. Number = account; balance = initial; } acct 1 acct. Number 72354 balance 102. 56 name CSC 1051 M. A. Papalaskari, Villanova University "Ted Murphy"
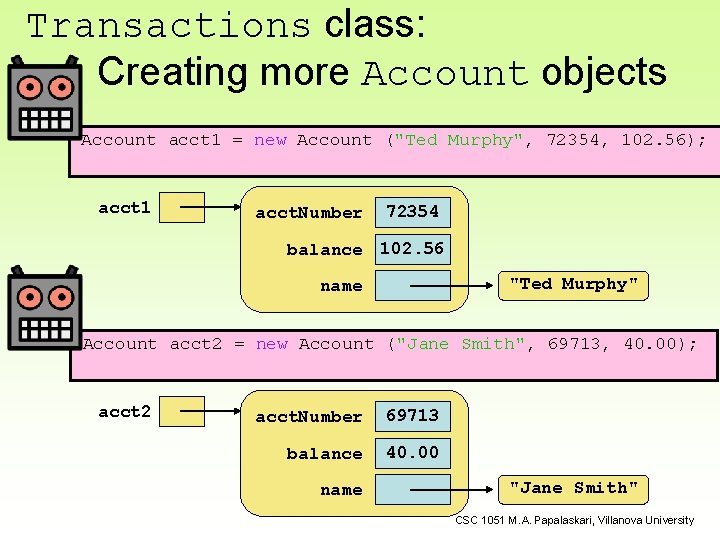
Transactions class: Creating more Account objects Account acct 1 = new Account ("Ted Murphy", 72354, 102. 56); acct 1 acct. Number 72354 balance 102. 56 "Ted Murphy" name Account acct 2 = new Account ("Jane Smith", 69713, 40. 00); acct 2 acct. Number 69713 balance 40. 00 name "Jane Smith" CSC 1051 M. A. Papalaskari, Villanova University
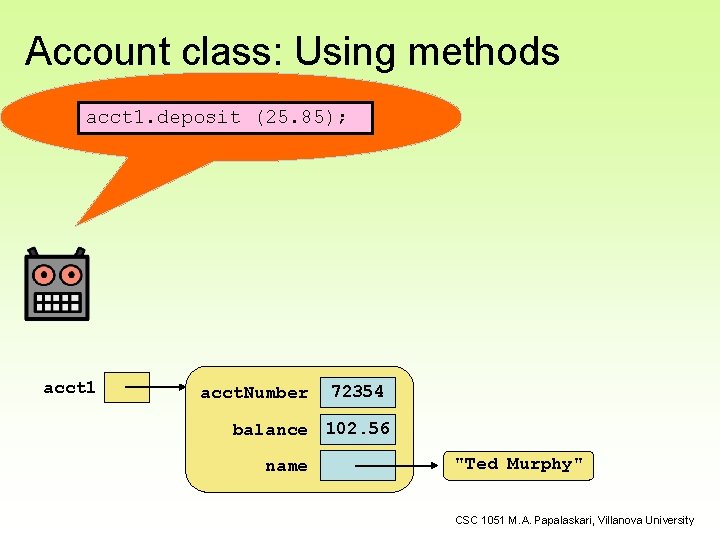
Account class: Using methods acct 1. deposit (25. 85); acct 1 acct. Number 72354 balance 102. 56 name "Ted Murphy" CSC 1051 M. A. Papalaskari, Villanova University
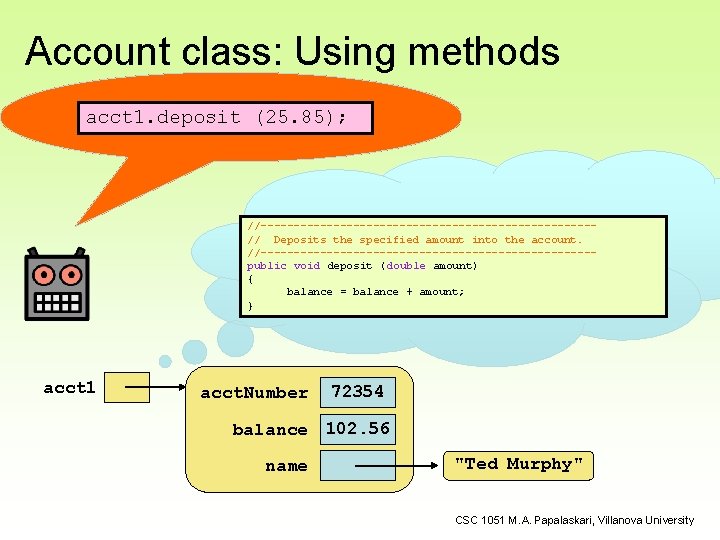
Account class: Using methods acct 1. deposit (25. 85); //-------------------------// Deposits the specified amount into the account. //-------------------------public void deposit (double amount) { balance = balance + amount; } acct 1 acct. Number 72354 balance 102. 56 name "Ted Murphy" CSC 1051 M. A. Papalaskari, Villanova University
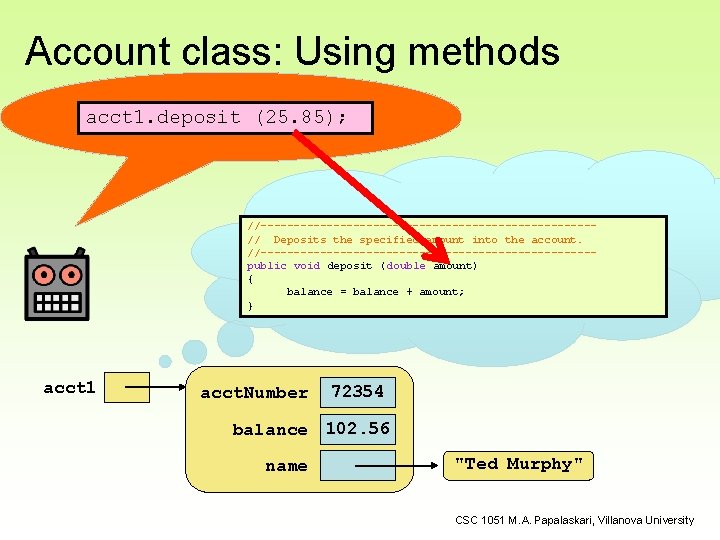
Account class: Using methods acct 1. deposit (25. 85); //-------------------------// Deposits the specified amount into the account. //-------------------------public void deposit (double amount) { balance = balance + amount; } acct 1 acct. Number 72354 balance 102. 56 name "Ted Murphy" CSC 1051 M. A. Papalaskari, Villanova University
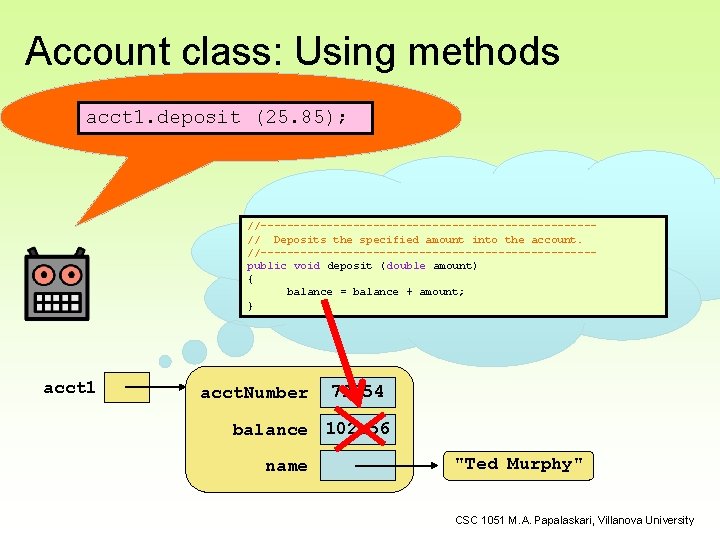
Account class: Using methods acct 1. deposit (25. 85); //-------------------------// Deposits the specified amount into the account. //-------------------------public void deposit (double amount) { balance = balance + amount; } acct 1 acct. Number 72354 balance 102. 56 name "Ted Murphy" CSC 1051 M. A. Papalaskari, Villanova University
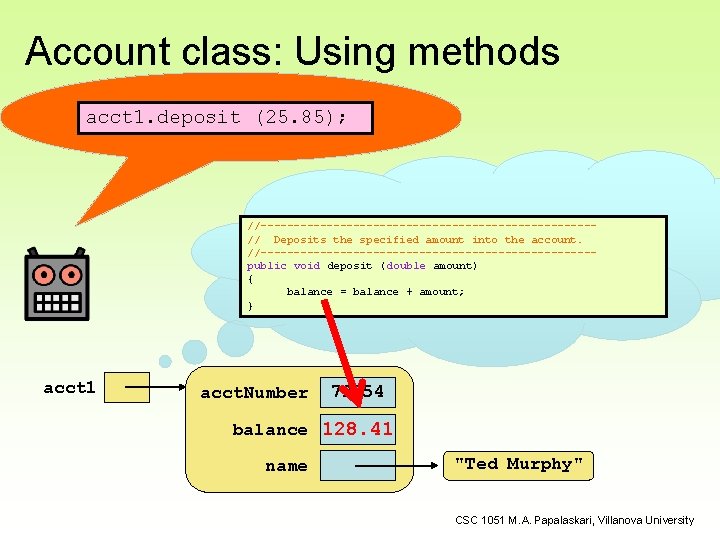
Account class: Using methods acct 1. deposit (25. 85); //-------------------------// Deposits the specified amount into the account. //-------------------------public void deposit (double amount) { balance = balance + amount; } acct 1 acct. Number 72354 balance 128. 41 name "Ted Murphy" CSC 1051 M. A. Papalaskari, Villanova University
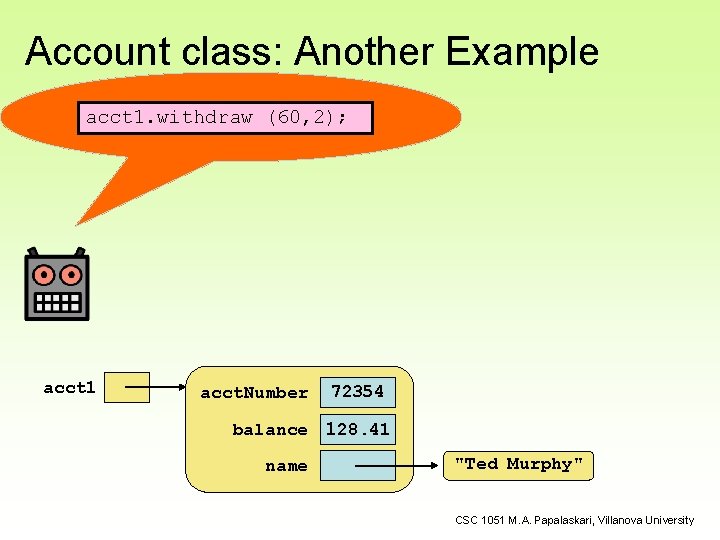
Account class: Another Example acct 1. withdraw (60, 2); acct 1 acct. Number 72354 balance 128. 41 name "Ted Murphy" CSC 1051 M. A. Papalaskari, Villanova University
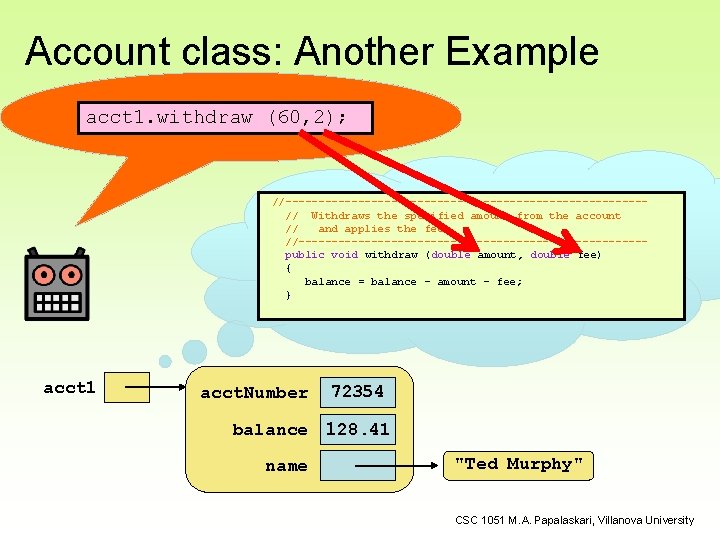
Account class: Another Example acct 1. withdraw (60, 2); //---------------------------// Withdraws the specified amount from the account // and applies the fee. //--------------------------public void withdraw (double amount, double fee) { balance = balance - amount - fee; } acct 1 acct. Number 72354 balance 128. 41 name "Ted Murphy" CSC 1051 M. A. Papalaskari, Villanova University
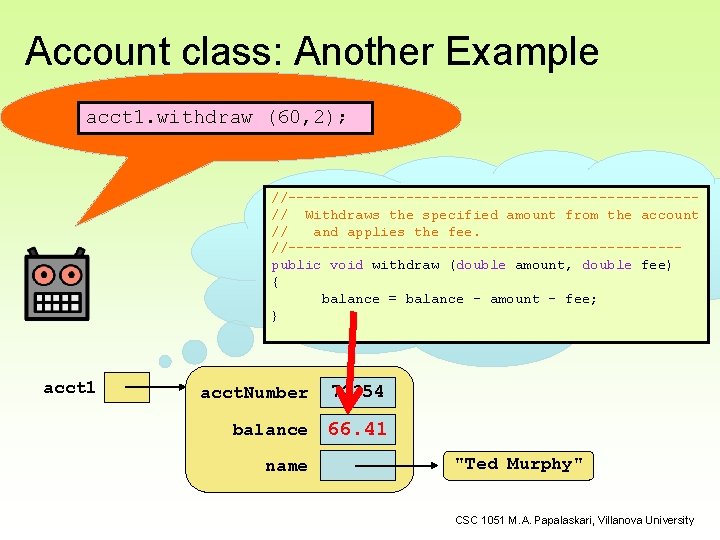
Account class: Another Example acct 1. withdraw (60, 2); //------------------------// Withdraws the specified amount from the account // and applies the fee. //-----------------------public void withdraw (double amount, double fee) { balance = balance - amount - fee; } acct 1 acct. Number 72354 balance 66. 41 name "Ted Murphy" CSC 1051 M. A. Papalaskari, Villanova University
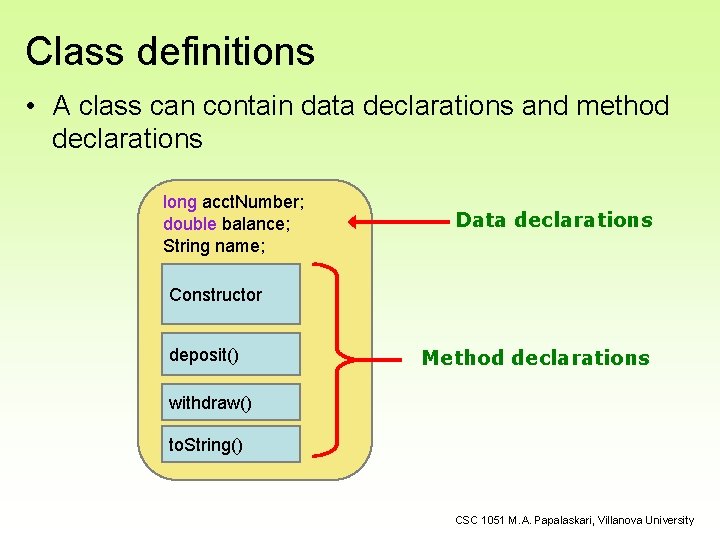
Class definitions • A class can contain data declarations and method declarations long acct. Number; double balance; String name; Data declarations Constructor deposit() Method declarations withdraw() to. String() CSC 1051 M. A. Papalaskari, Villanova University
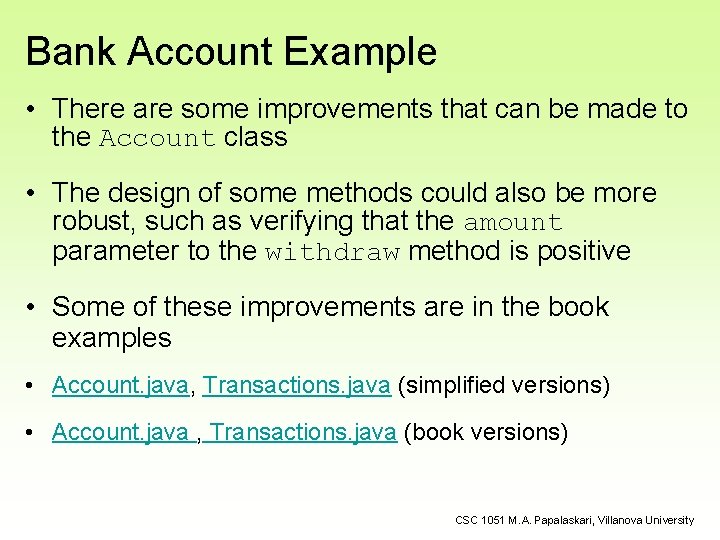
Bank Account Example • There are some improvements that can be made to the Account class • The design of some methods could also be more robust, such as verifying that the amount parameter to the withdraw method is positive • Some of these improvements are in the book examples • Account. java, Transactions. java (simplified versions) • Account. java , Transactions. java (book versions) CSC 1051 M. A. Papalaskari, Villanova University
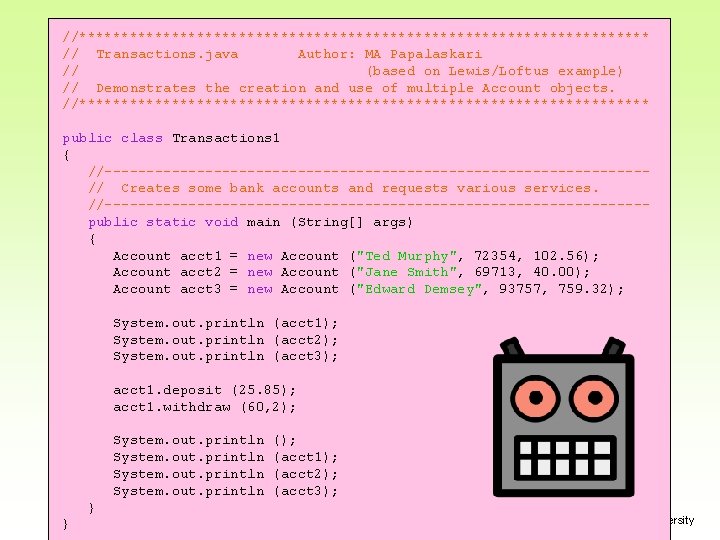
//********************************** // Transactions. java Author: MA Papalaskari // (based on Lewis/Loftus example) // Demonstrates the creation and use of multiple Account objects. //********************************** public class Transactions 1 { //--------------------------------// Creates some bank accounts and requests various services. //--------------------------------public static void main (String[] args) { Account acct 1 = new Account ("Ted Murphy", 72354, 102. 56); Account acct 2 = new Account ("Jane Smith", 69713, 40. 00); Account acct 3 = new Account ("Edward Demsey", 93757, 759. 32); System. out. println (acct 1); System. out. println (acct 2); System. out. println (acct 3); acct 1. deposit (25. 85); acct 1. withdraw (60, 2); System. out. println } } (); (acct 1); (acct 2); (acct 3); CSC 1051 M. A. Papalaskari, Villanova University
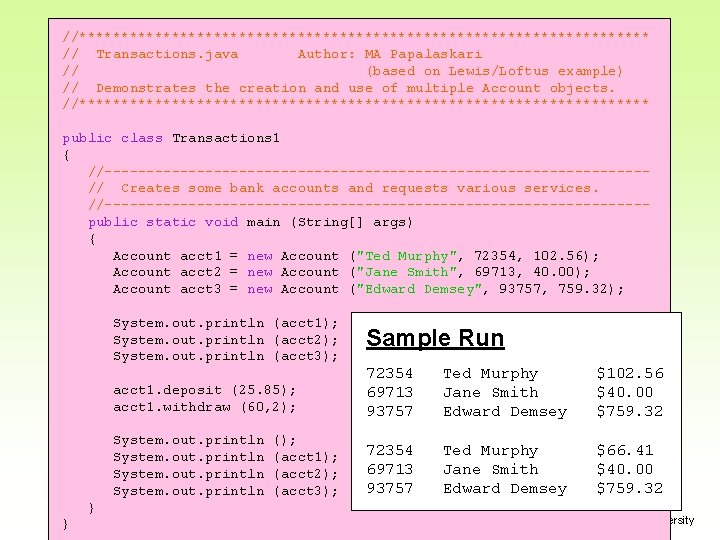
//********************************** // Transactions. java Author: MA Papalaskari // (based on Lewis/Loftus example) // Demonstrates the creation and use of multiple Account objects. //********************************** public class Transactions 1 { //--------------------------------// Creates some bank accounts and requests various services. //--------------------------------public static void main (String[] args) { Account acct 1 = new Account ("Ted Murphy", 72354, 102. 56); Account acct 2 = new Account ("Jane Smith", 69713, 40. 00); Account acct 3 = new Account ("Edward Demsey", 93757, 759. 32); System. out. println (acct 1); System. out. println (acct 2); System. out. println (acct 3); } } Sample Run acct 1. deposit (25. 85); acct 1. withdraw (60, 2); 72354 69713 93757 Ted Murphy Jane Smith Edward Demsey $102. 56 $40. 00 $759. 32 System. out. println 72354 69713 93757 Ted Murphy Jane Smith Edward Demsey $66. 41 $40. 00 $759. 32 (); (acct 1); (acct 2); (acct 3); CSC 1051 M. A. Papalaskari, Villanova University
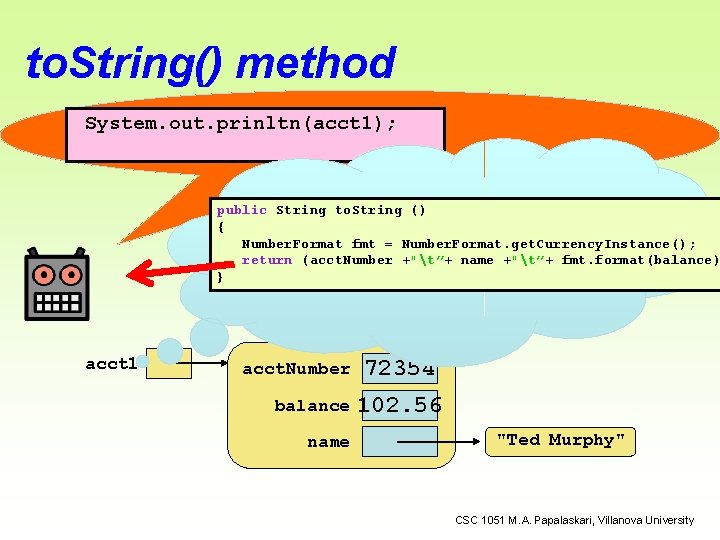
to. String() method System. out. prinltn(acct 1); public String to. String () { Number. Format fmt = Number. Format. get. Currency. Instance(); return (acct. Number +"t”+ name +"t”+ fmt. format(balance) } acct 1 acct. Number balance name 72354 102. 56 "Ted Murphy" CSC 1051 M. A. Papalaskari, Villanova University
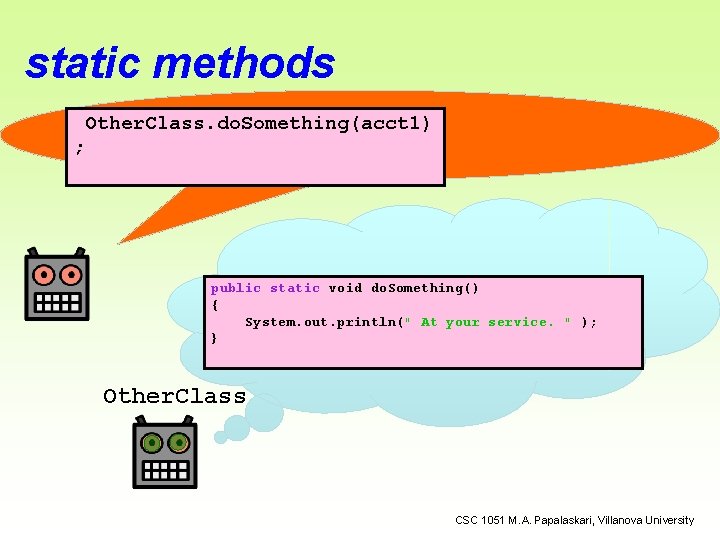
static methods Other. Class. do. Something(acct 1) ; public static void do. Something() { System. out. println(" At your service. " ); } Other. Class CSC 1051 M. A. Papalaskari, Villanova University
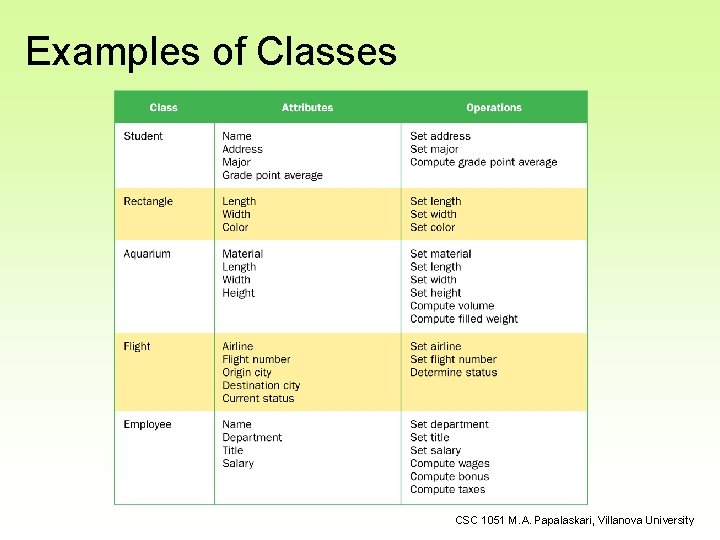
Examples of Classes CSC 1051 M. A. Papalaskari, Villanova University
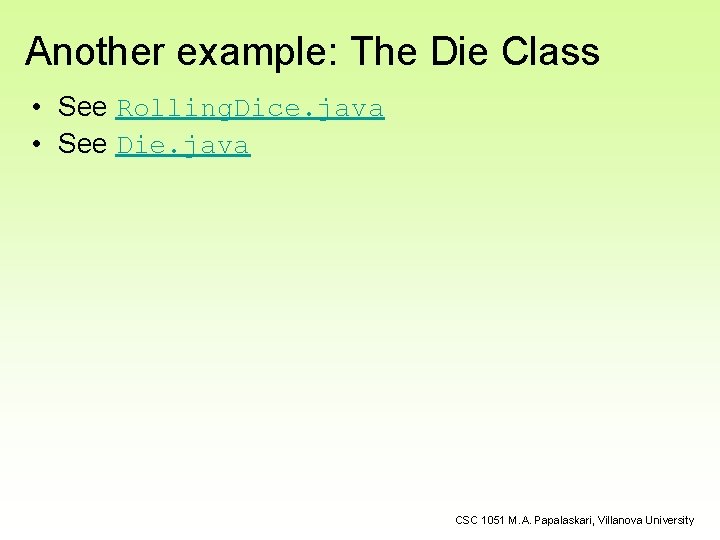
Another example: The Die Class • See Rolling. Dice. java • See Die. java CSC 1051 M. A. Papalaskari, Villanova University
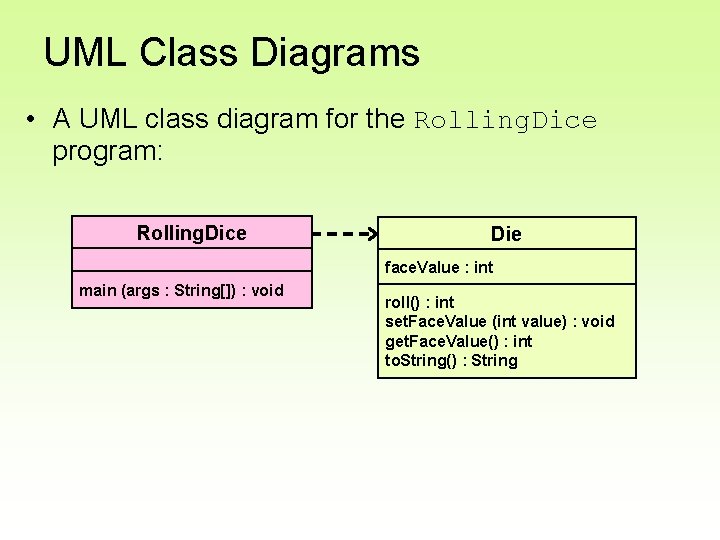
UML Class Diagrams • A UML class diagram for the Rolling. Dice program: Rolling. Dice Die face. Value : int main (args : String[]) : void roll() : int set. Face. Value (int value) : void get. Face. Value() : int to. String() : String
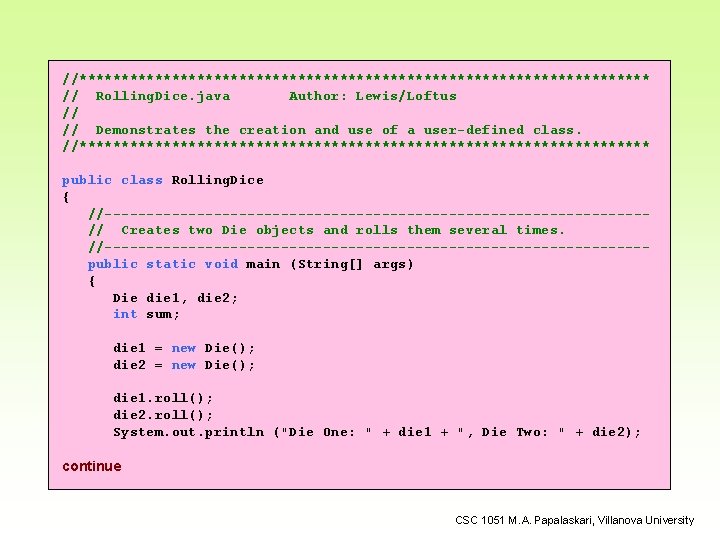
//********************************** // Rolling. Dice. java Author: Lewis/Loftus // // Demonstrates the creation and use of a user-defined class. //********************************** public class Rolling. Dice { //--------------------------------// Creates two Die objects and rolls them several times. //--------------------------------public static void main (String[] args) { Die die 1, die 2; int sum; die 1 = new Die(); die 2 = new Die(); die 1. roll(); die 2. roll(); System. out. println ("Die One: " + die 1 + ", Die Two: " + die 2); continue CSC 1051 M. A. Papalaskari, Villanova University
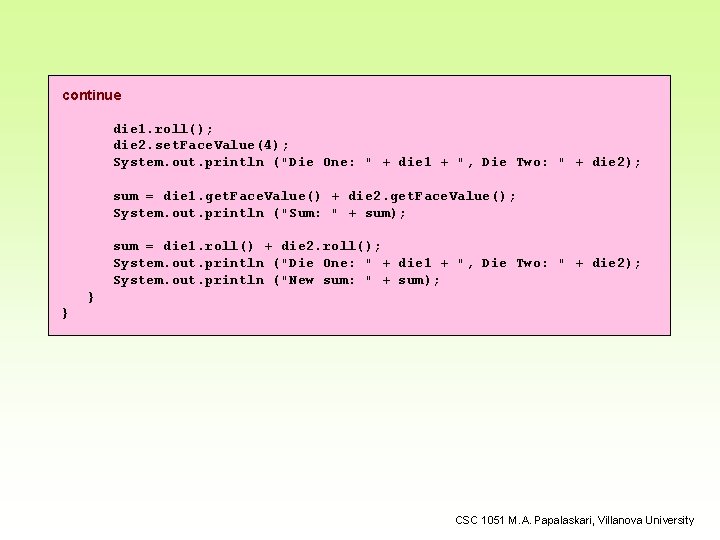
continue die 1. roll(); die 2. set. Face. Value(4); System. out. println ("Die One: " + die 1 + ", Die Two: " + die 2); sum = die 1. get. Face. Value() + die 2. get. Face. Value(); System. out. println ("Sum: " + sum); sum = die 1. roll() + die 2. roll(); System. out. println ("Die One: " + die 1 + ", Die Two: " + die 2); System. out. println ("New sum: " + sum); } } CSC 1051 M. A. Papalaskari, Villanova University
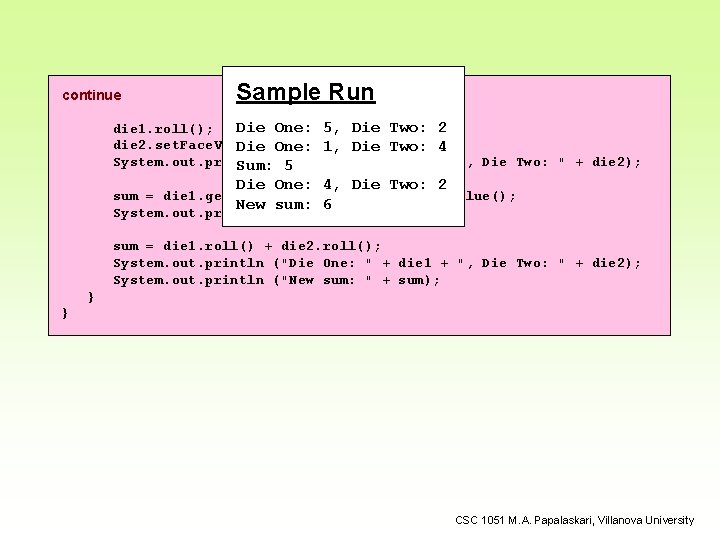
continue Sample Run Die One: 5, Die Two: 2 die 1. roll(); die 2. set. Face. Value(4); Die One: 1, Die Two: 4 System. out. println One: " + die 1 + ", Die Two: " + die 2); Sum: ("Die 5 Die One: 4, Die Two: 2 sum = die 1. get. Face. Value() + die 2. get. Face. Value(); New sum: 6 System. out. println ("Sum: " + sum); sum = die 1. roll() + die 2. roll(); System. out. println ("Die One: " + die 1 + ", Die Two: " + die 2); System. out. println ("New sum: " + sum); } } CSC 1051 M. A. Papalaskari, Villanova University
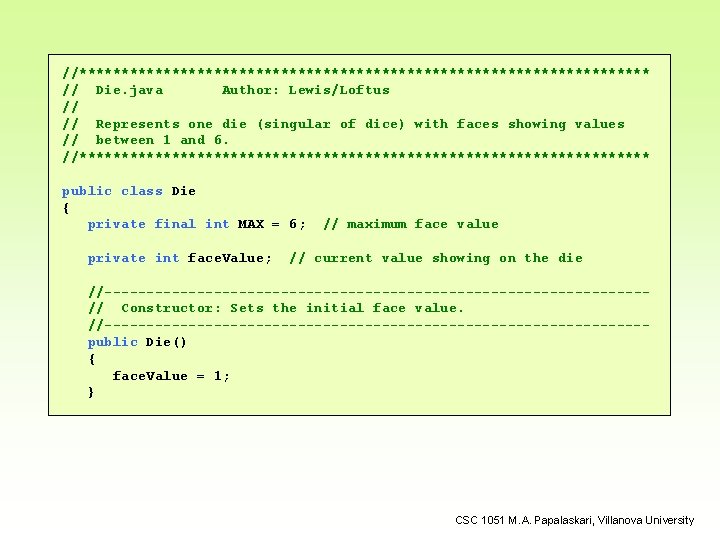
//********************************** // Die. java Author: Lewis/Loftus // // Represents one die (singular of dice) with faces showing values // between 1 and 6. //********************************** public class Die { private final int MAX = 6; private int face. Value; // maximum face value // current value showing on the die //--------------------------------// Constructor: Sets the initial face value. //--------------------------------public Die() { face. Value = 1; } CSC 1051 M. A. Papalaskari, Villanova University
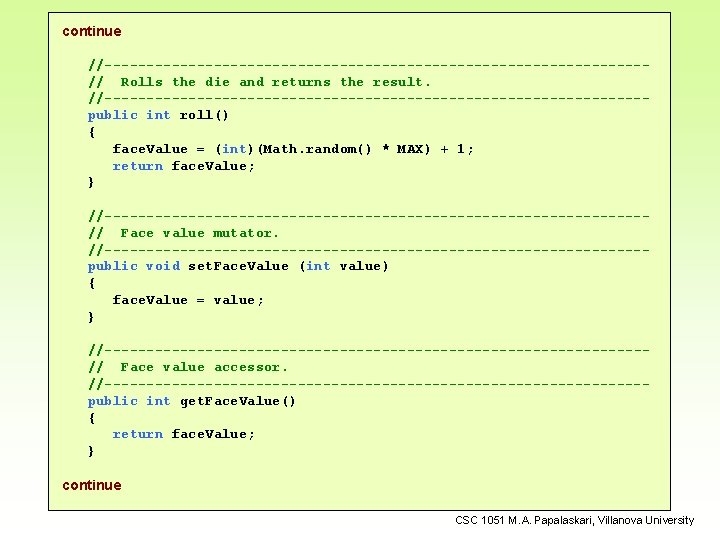
continue //--------------------------------// Rolls the die and returns the result. //--------------------------------public int roll() { face. Value = (int)(Math. random() * MAX) + 1; return face. Value; } //--------------------------------// Face value mutator. //--------------------------------public void set. Face. Value (int value) { face. Value = value; } //--------------------------------// Face value accessor. //--------------------------------public int get. Face. Value() { return face. Value; } continue CSC 1051 M. A. Papalaskari, Villanova University
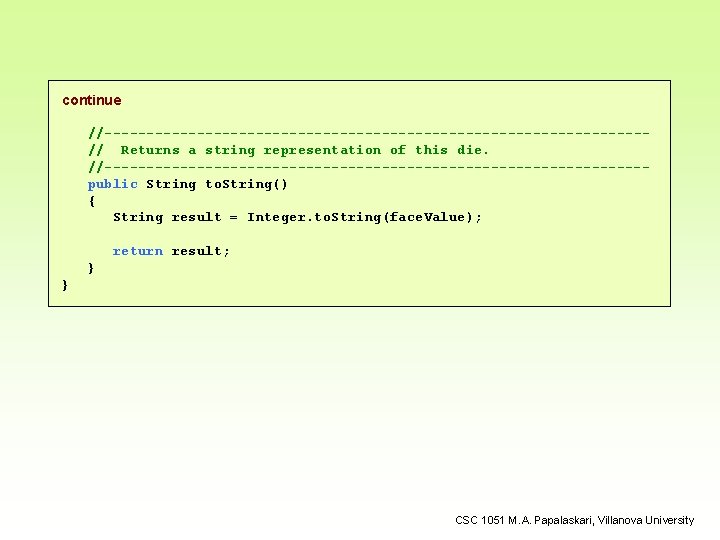
continue //--------------------------------// Returns a string representation of this die. //--------------------------------public String to. String() { String result = Integer. to. String(face. Value); return result; } } CSC 1051 M. A. Papalaskari, Villanova University
Manipularea manuala a maselor
Ajit diwan
Amit agarwal princeton
Data structures and algorithms tutorial
Information retrieval data structures and algorithms
Data structures and algorithms bits pilani
Ajit diwan iit bombay
Data structures and algorithms
Data structures and algorithms
Waterloo data structures and algorithms
Signature file structure in information retrieval system
Data structures and algorithms
Algorithms + data structures = programs
Designing a pay structure
Solidworks: designing bearings classes
Classes e sub classes de palavras
Pre ap classes vs regular classes
Homologous structures
Structures vs classes
Data stream
Csc data entry
Designing accurate data entry procedures
Explanation of code
Explain single pass macro processor
Assembler algorithm and data structures
Data structures and abstractions with java
Adts, data structures, and problem solving with c++
Data structures and algorithm
Persistent and ephemeral data structures
Designing and delivering oral and online presentation
Architecture is the art and science of designing buildings
Organizes data into categories called classes
Data science courses edison
Computational thinking algorithms and programming
1001 design
Association analysis: basic concepts and algorithms
Computer arithmetic: algorithms and hardware designs
Algorithms for select and join operations
Algorithms and flowcharts
Undecidable problems and unreasonable time algorithms.
Cluster analysis basic concepts and algorithms
Probabilistic analysis and randomized algorithms