Data Structures LECTURE 7 Heapsort and priority queues
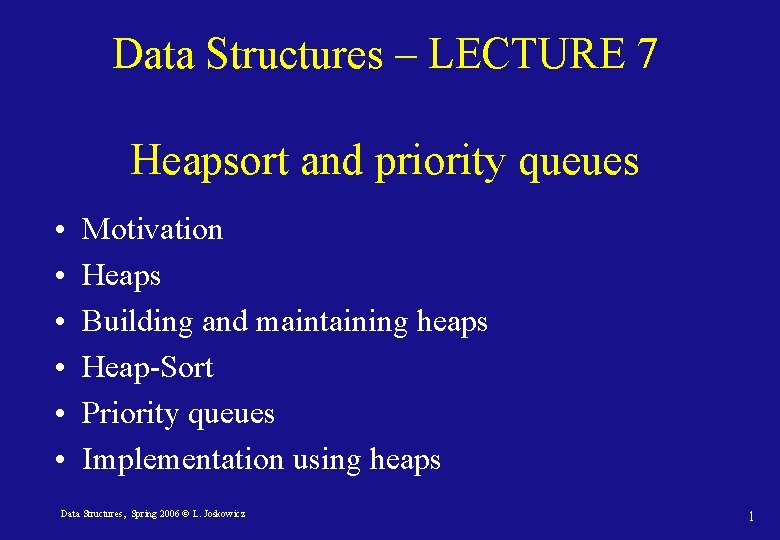
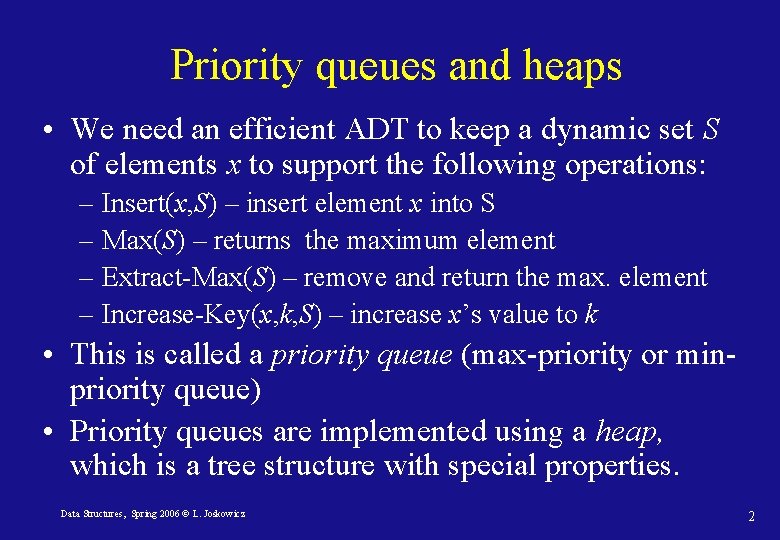
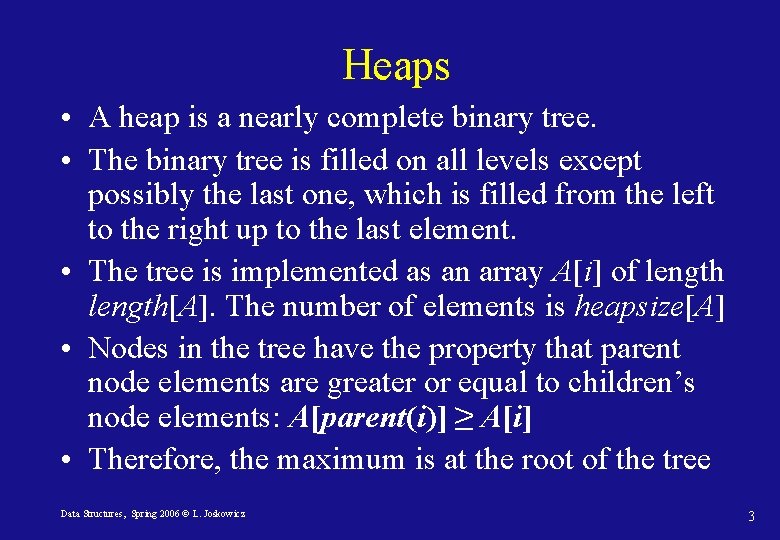
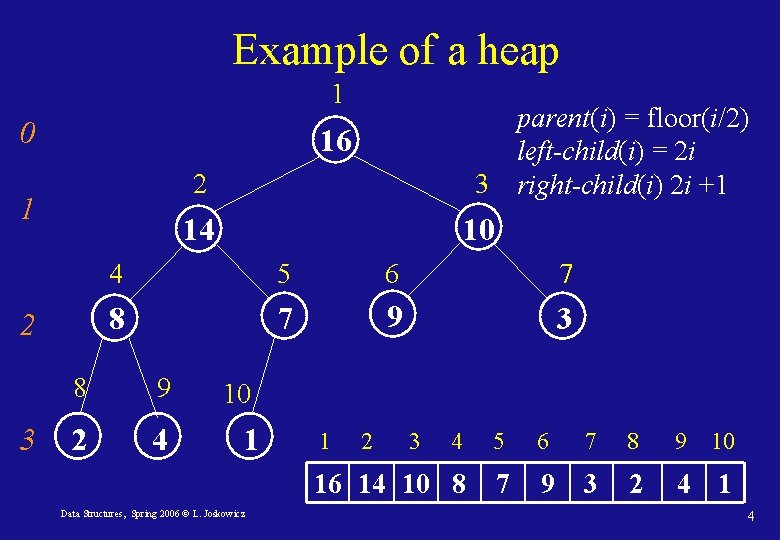
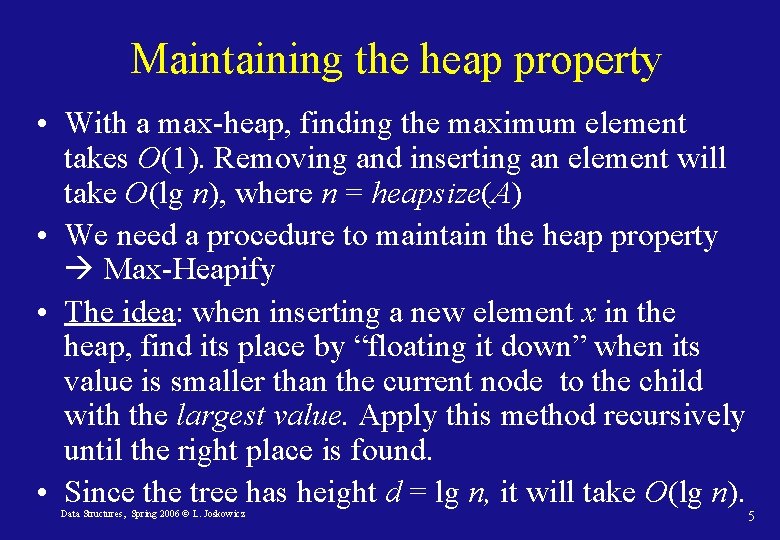
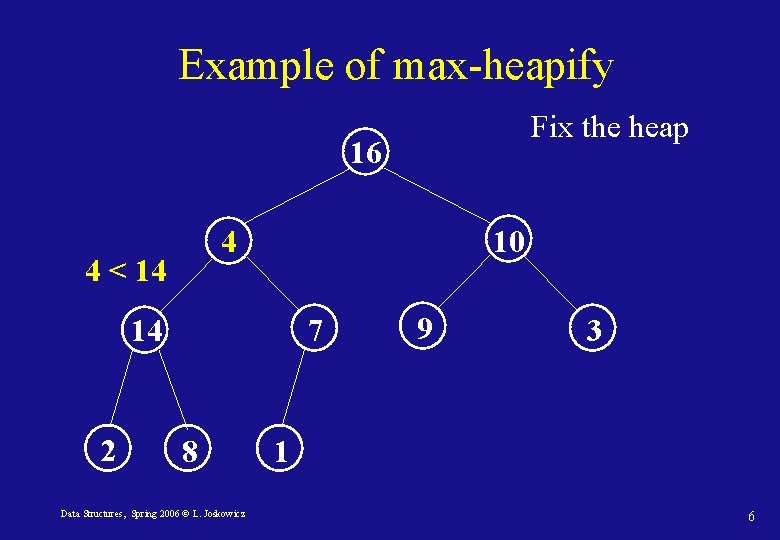
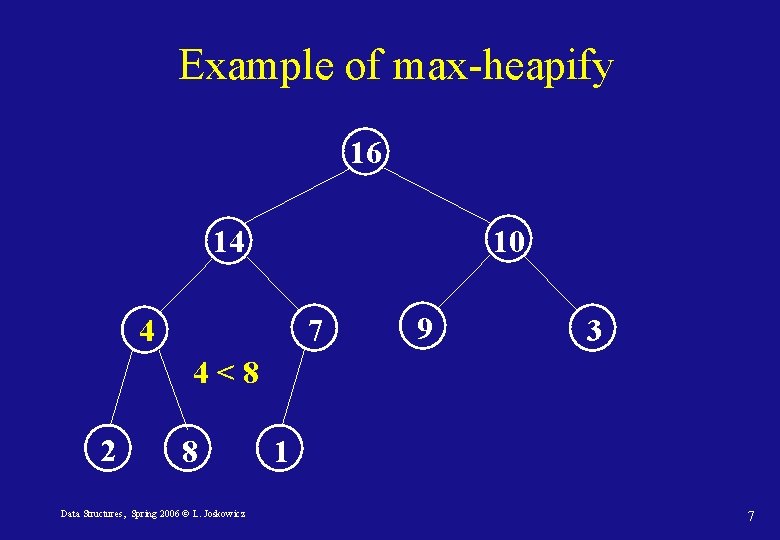
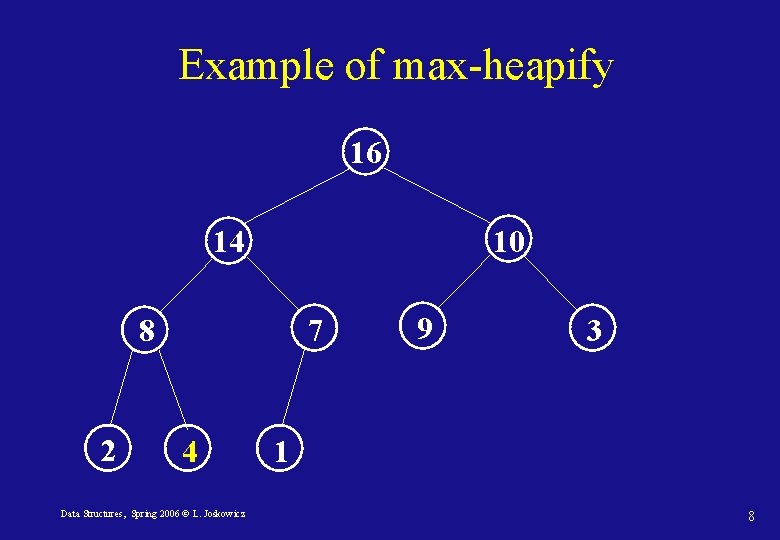
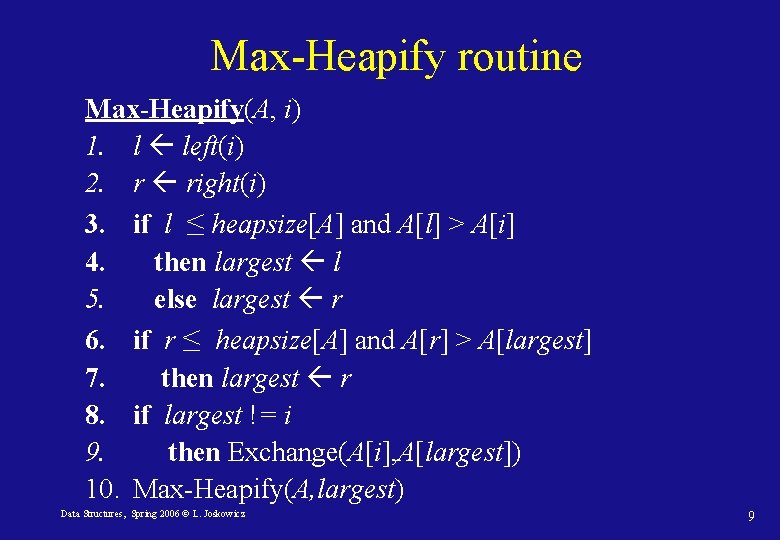
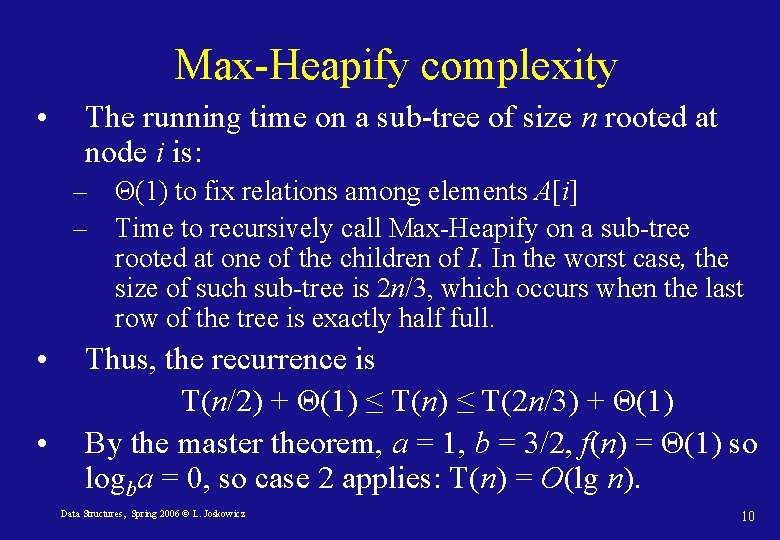
![Building a heap • Use Max-Heapify to recursively convert the array A[i] into a Building a heap • Use Max-Heapify to recursively convert the array A[i] into a](https://slidetodoc.com/presentation_image_h/35b388dd831010ed01b00b482cfb9e6b/image-11.jpg)
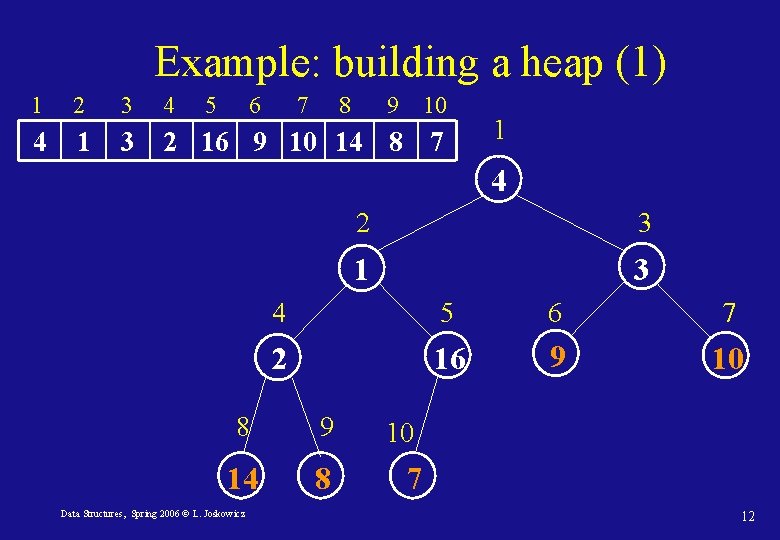
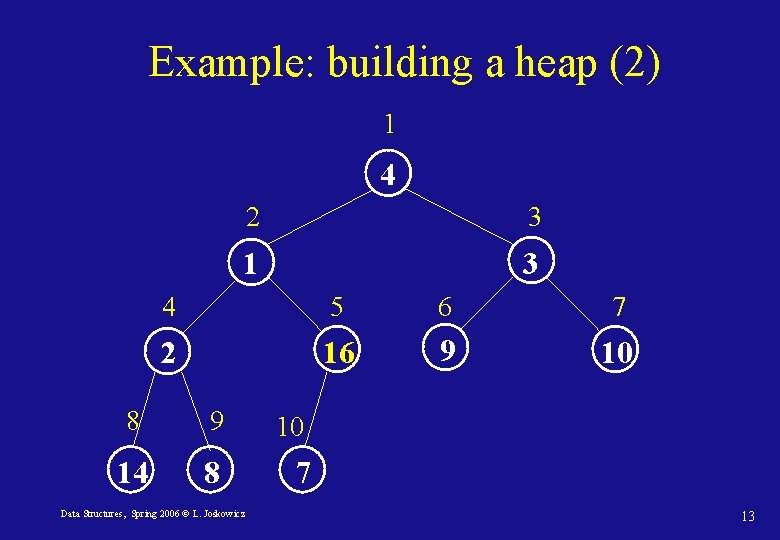
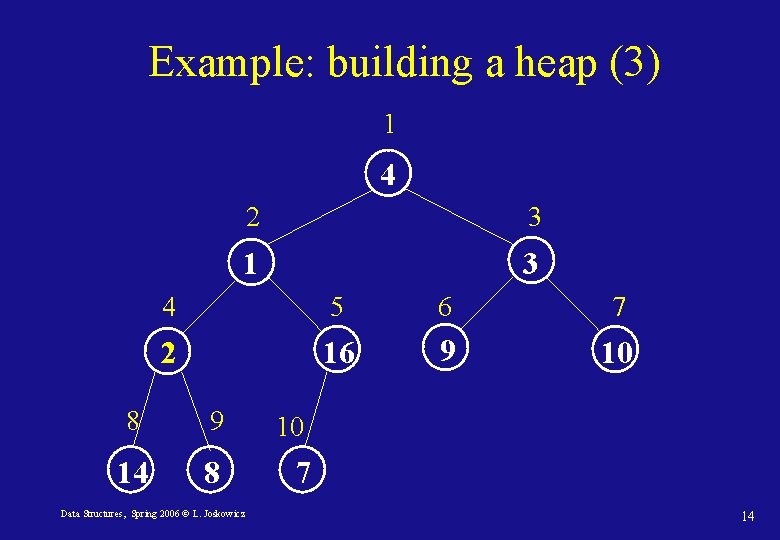
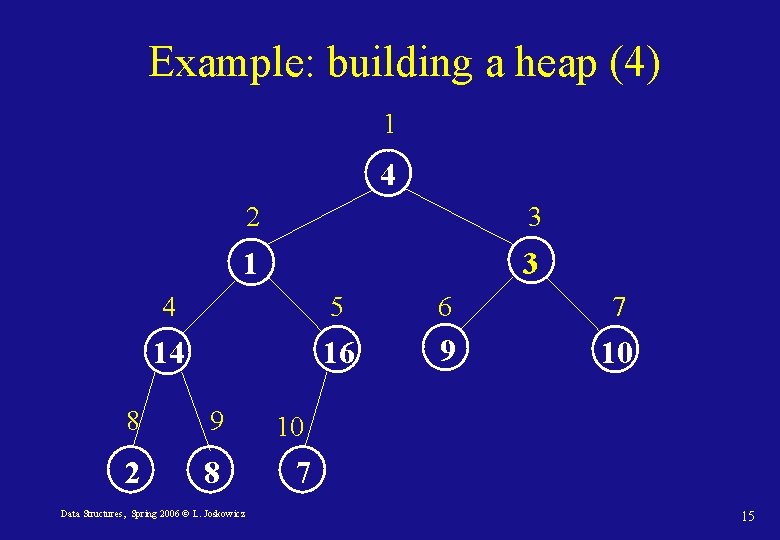
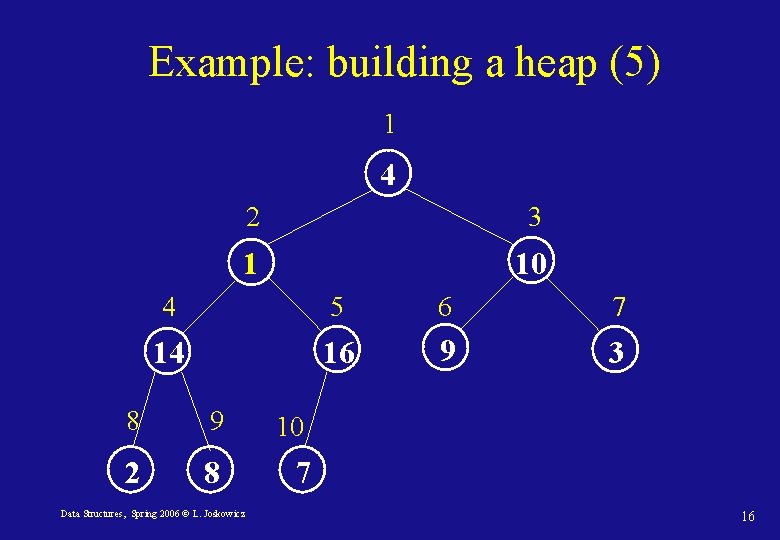
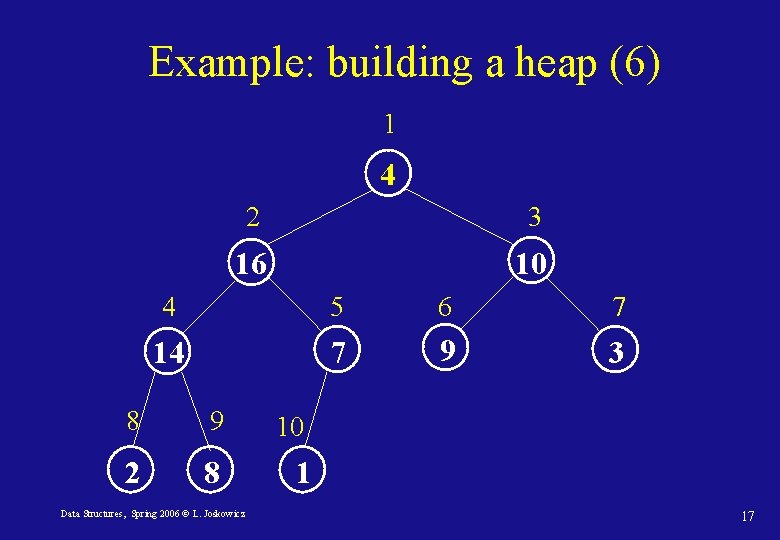
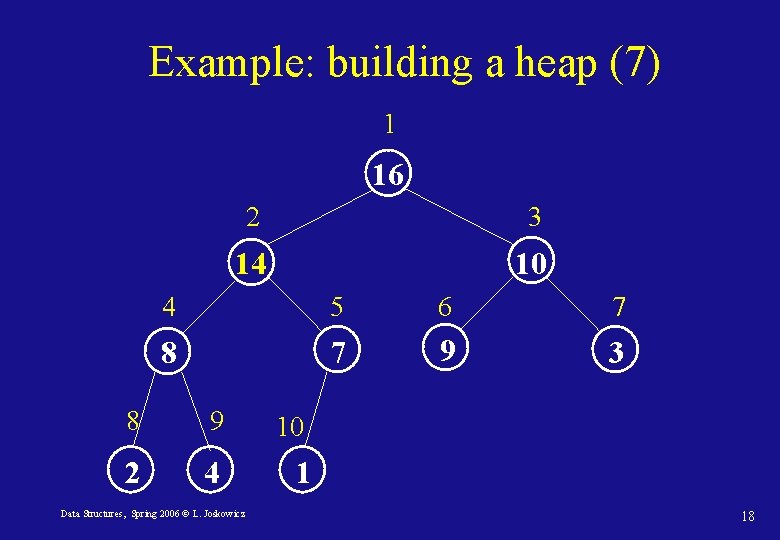
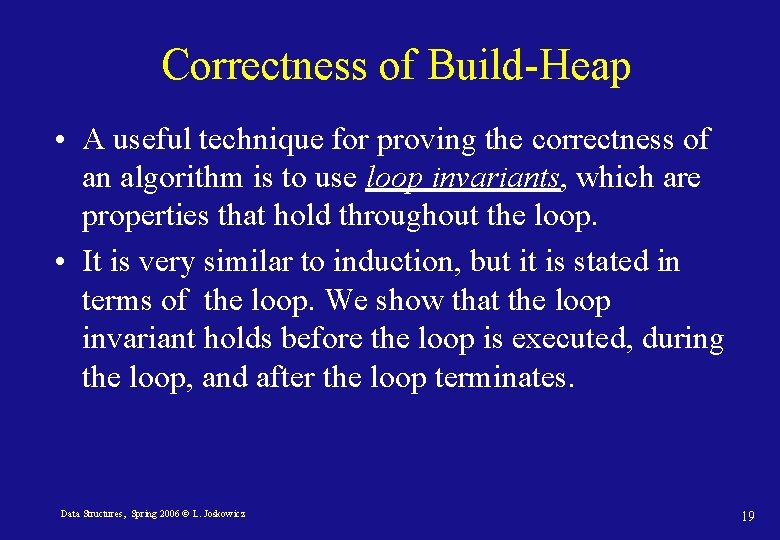
![Invariant of Build-Heap Build-Max-Heap(A) 1. heapsize[A] length(A) 2. for i downto 1 3. do Invariant of Build-Heap Build-Max-Heap(A) 1. heapsize[A] length(A) 2. for i downto 1 3. do](https://slidetodoc.com/presentation_image_h/35b388dd831010ed01b00b482cfb9e6b/image-20.jpg)
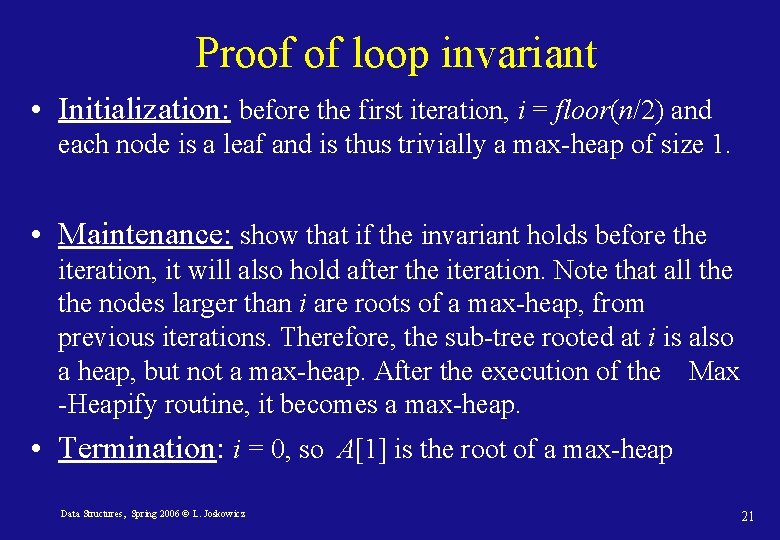
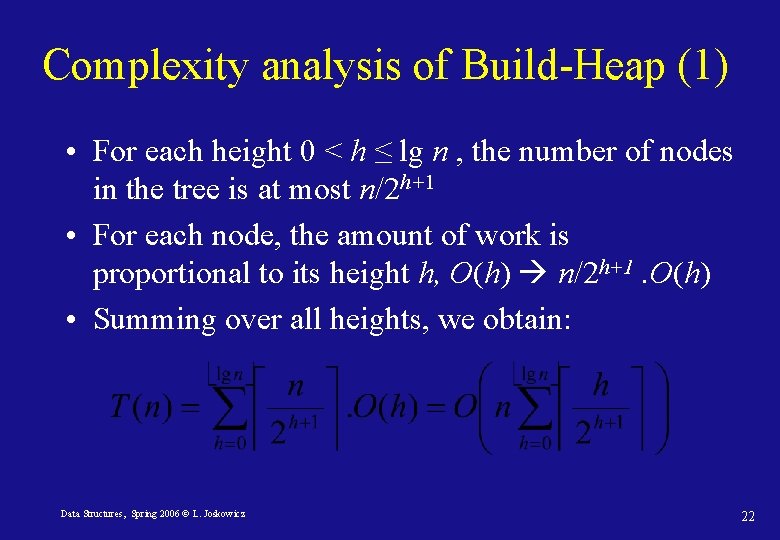
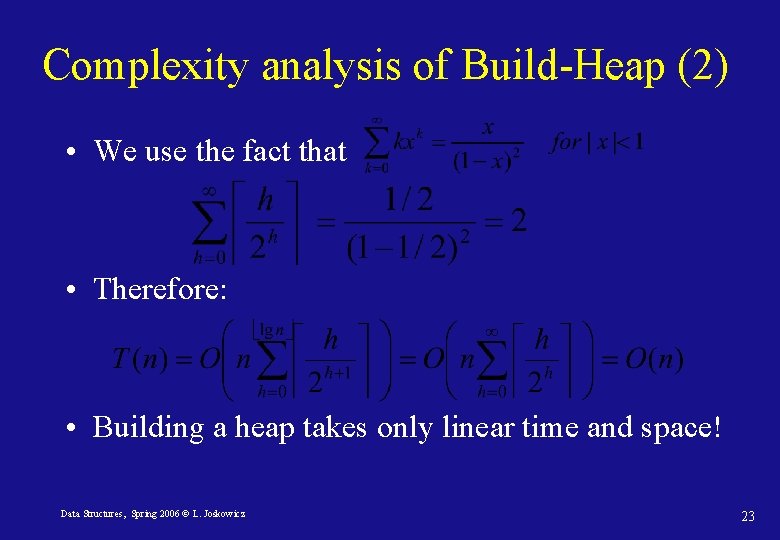
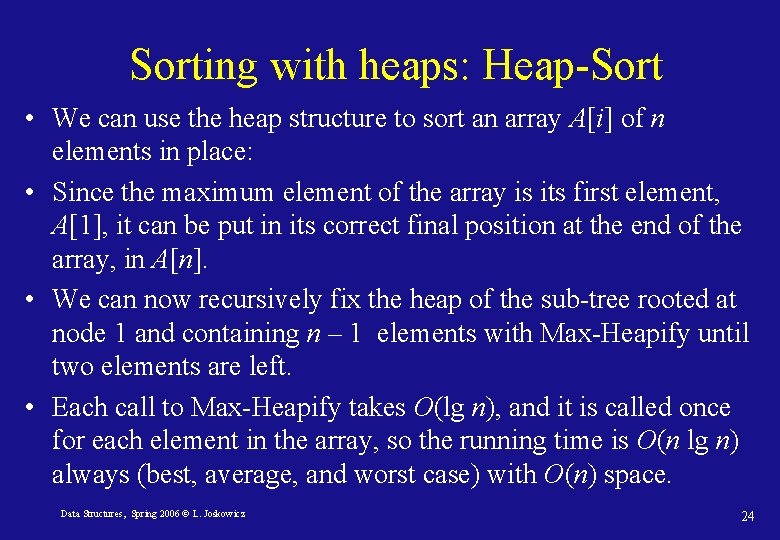
![Heap-Sort(A) 1. Build-Max-Heap(A) 2. heapsize[A] length(A) 3. for i length[A] downto 2 put maximum Heap-Sort(A) 1. Build-Max-Heap(A) 2. heapsize[A] length(A) 3. for i length[A] downto 2 put maximum](https://slidetodoc.com/presentation_image_h/35b388dd831010ed01b00b482cfb9e6b/image-25.jpg)
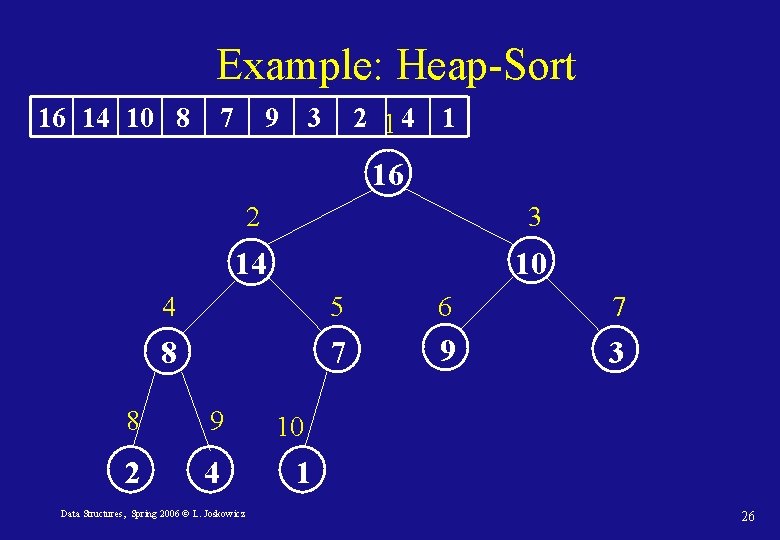
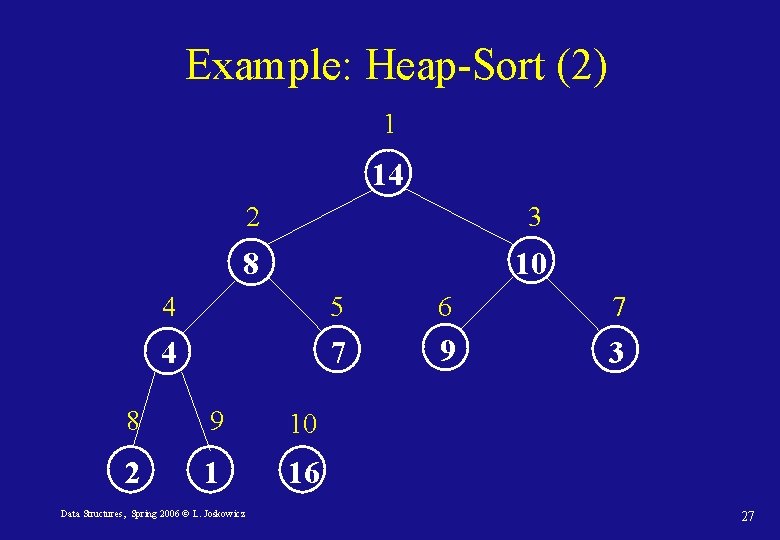
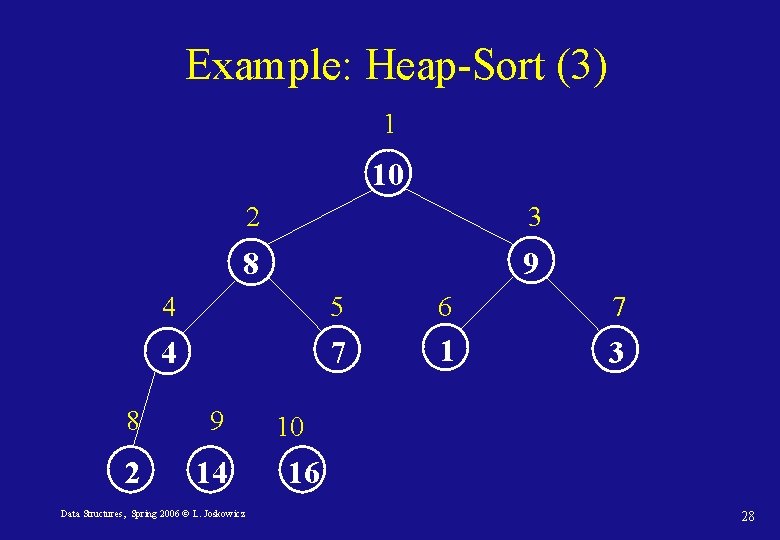
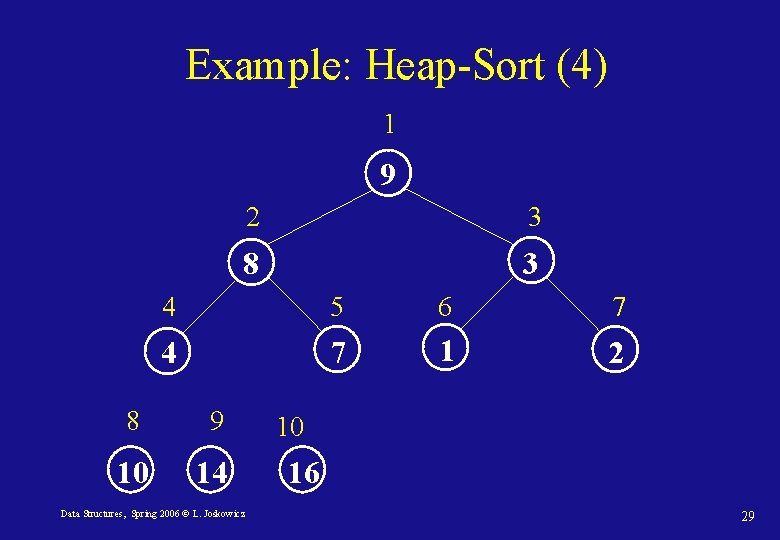
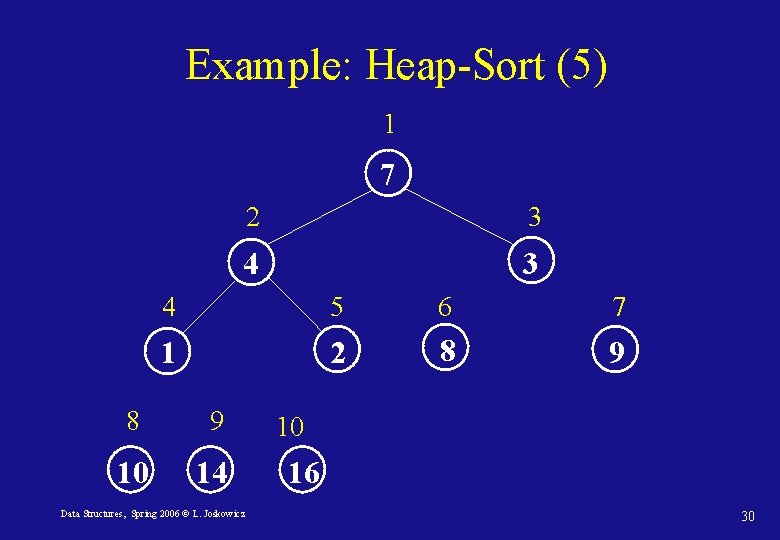
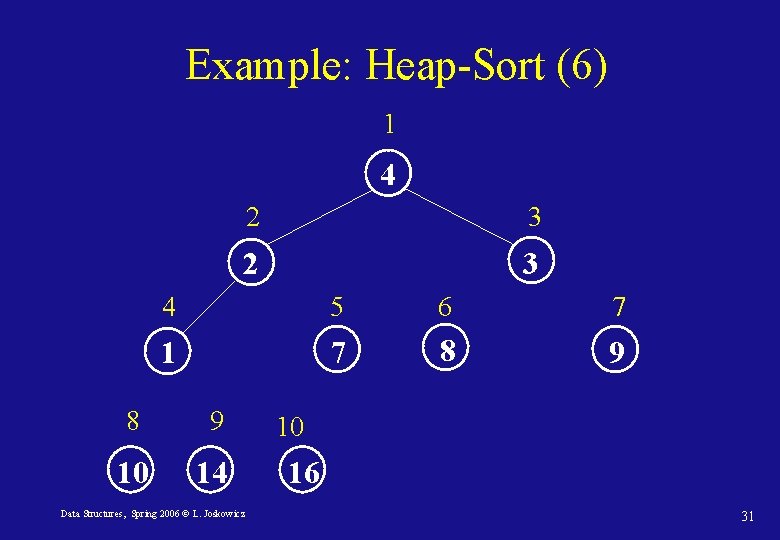
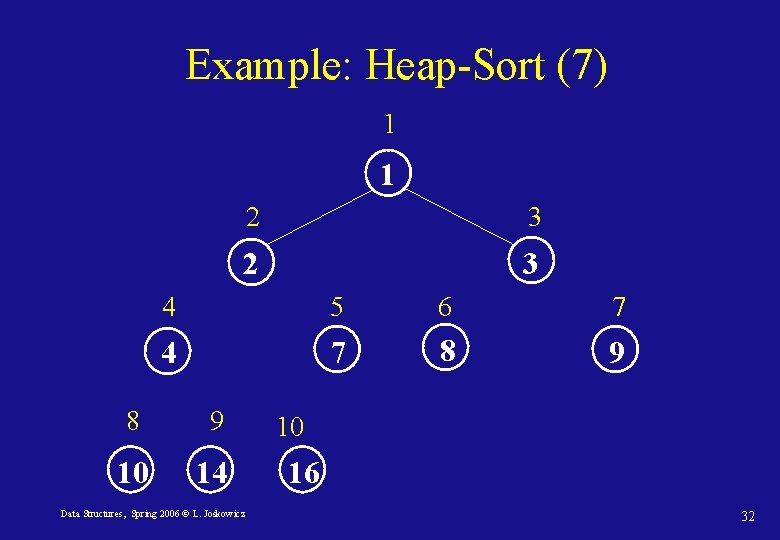
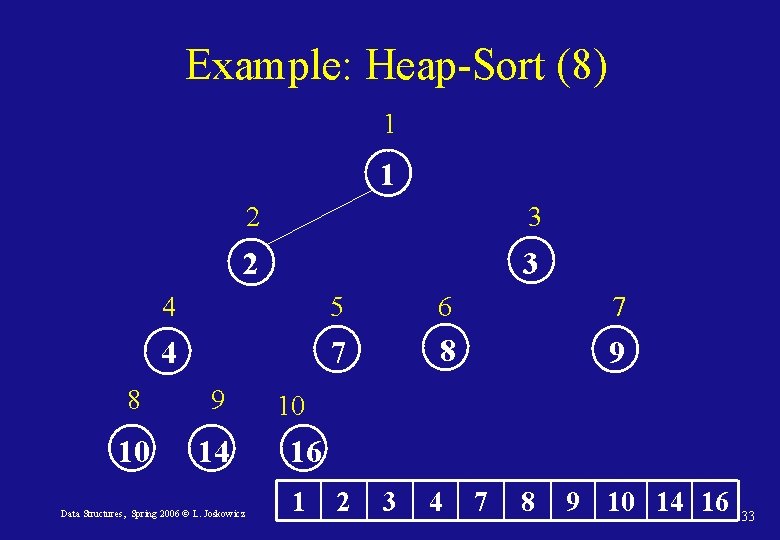
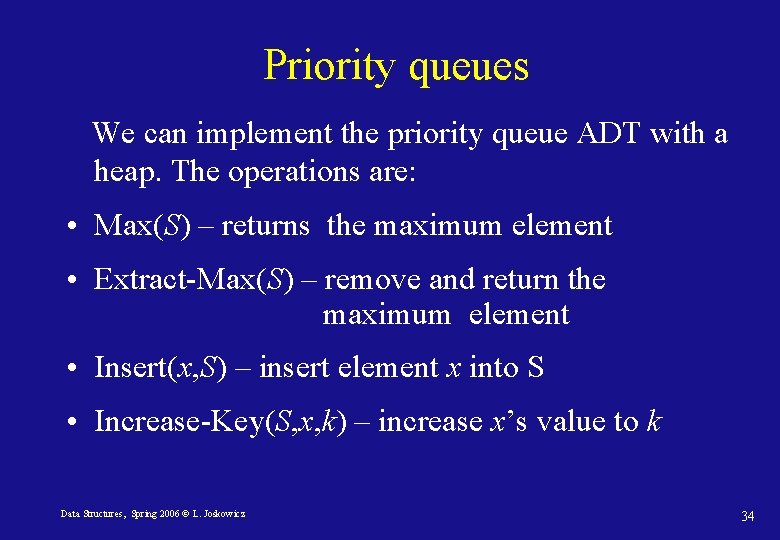
![Priority queues: Extract-Max Heap-Maximum(A) return A[1] Heap-Extract-Max(A) 1. if heapsize[A] < 1 2. then Priority queues: Extract-Max Heap-Maximum(A) return A[1] Heap-Extract-Max(A) 1. if heapsize[A] < 1 2. then](https://slidetodoc.com/presentation_image_h/35b388dd831010ed01b00b482cfb9e6b/image-35.jpg)
![Priority queues: Increase-Key Heap-Increase-key(A, i, key) 1. if key < A[i] 2. then error Priority queues: Increase-Key Heap-Increase-key(A, i, key) 1. if key < A[i] 2. then error](https://slidetodoc.com/presentation_image_h/35b388dd831010ed01b00b482cfb9e6b/image-36.jpg)
![Priority queues: Insert-Max Heap-Insert-Max(A, key) 1. heapsize[A] + 1 2. A[heapsize[A]] – ∞ 3. Priority queues: Insert-Max Heap-Insert-Max(A, key) 1. heapsize[A] + 1 2. A[heapsize[A]] – ∞ 3.](https://slidetodoc.com/presentation_image_h/35b388dd831010ed01b00b482cfb9e6b/image-37.jpg)
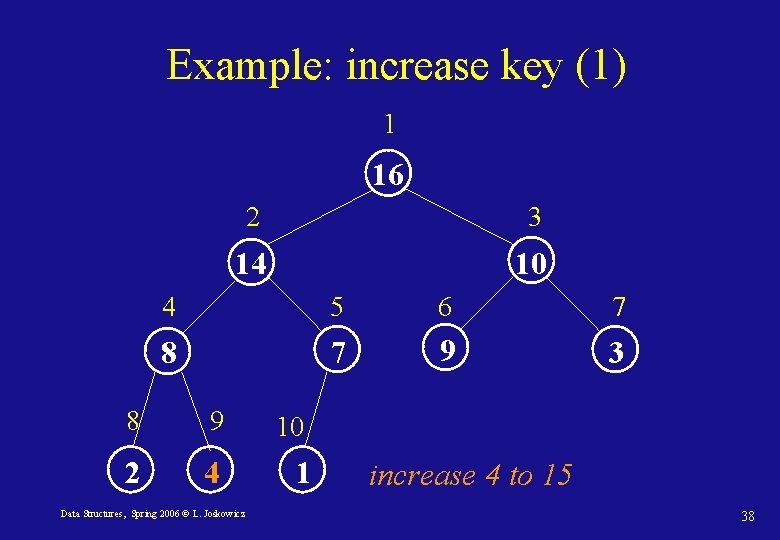
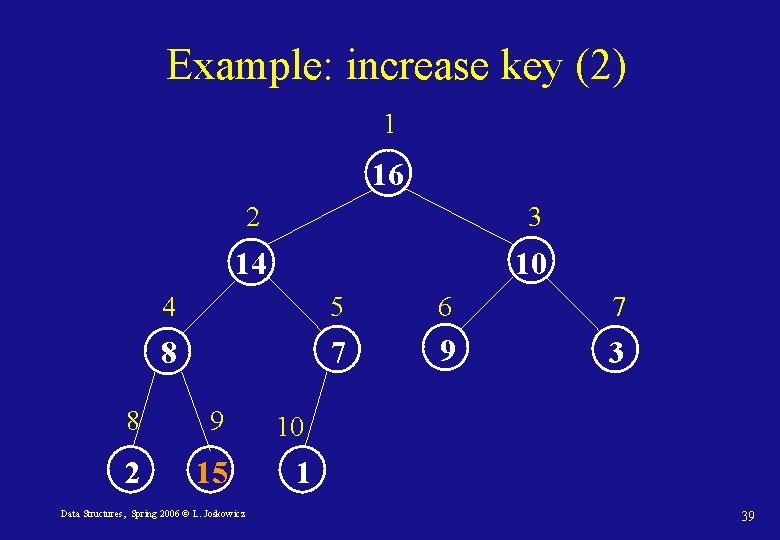
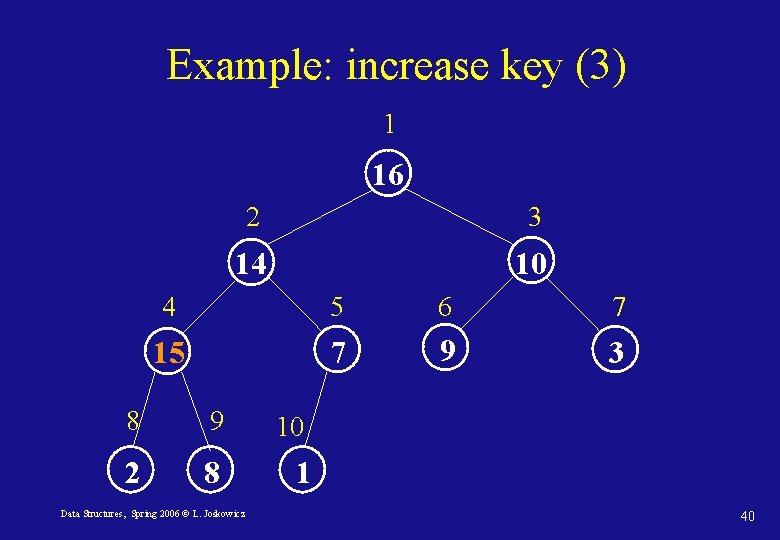
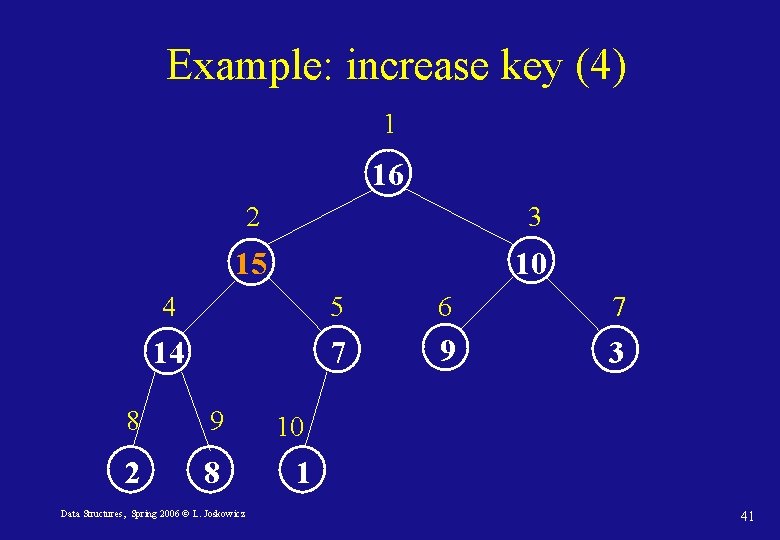
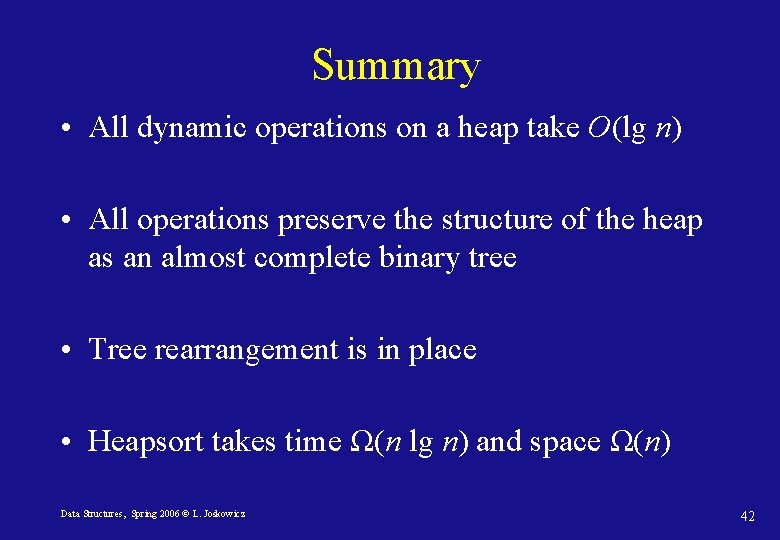
- Slides: 42
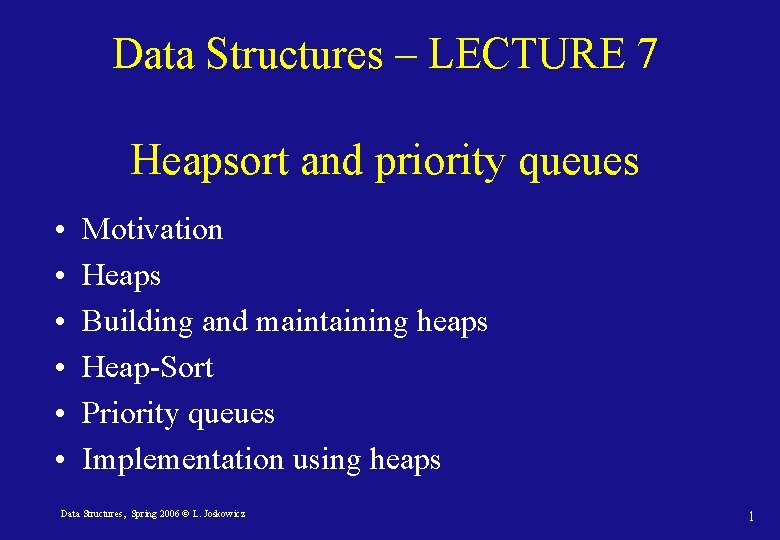
Data Structures – LECTURE 7 Heapsort and priority queues • • • Motivation Heaps Building and maintaining heaps Heap-Sort Priority queues Implementation using heaps Data Structures, Spring 2006 © L. Joskowicz 1
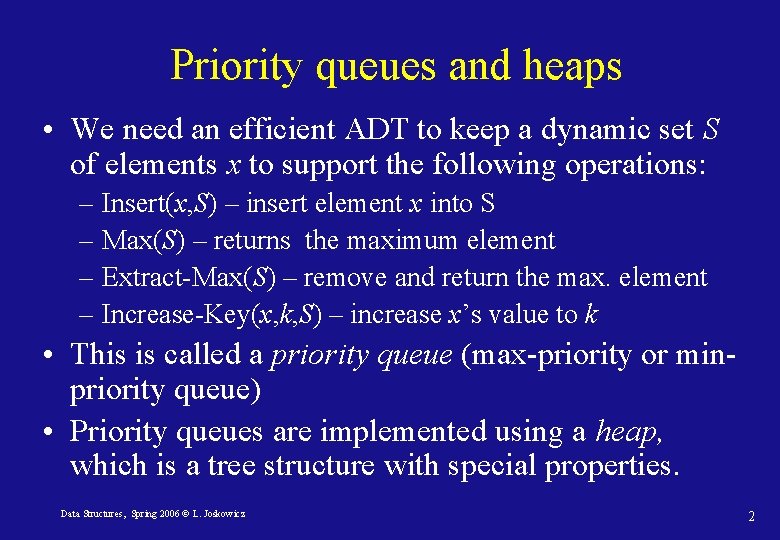
Priority queues and heaps • We need an efficient ADT to keep a dynamic set S of elements x to support the following operations: – Insert(x, S) – insert element x into S – Max(S) – returns the maximum element – Extract-Max(S) – remove and return the max. element – Increase-Key(x, k, S) – increase x’s value to k • This is called a priority queue (max-priority or minpriority queue) • Priority queues are implemented using a heap, which is a tree structure with special properties. Data Structures, Spring 2006 © L. Joskowicz 2
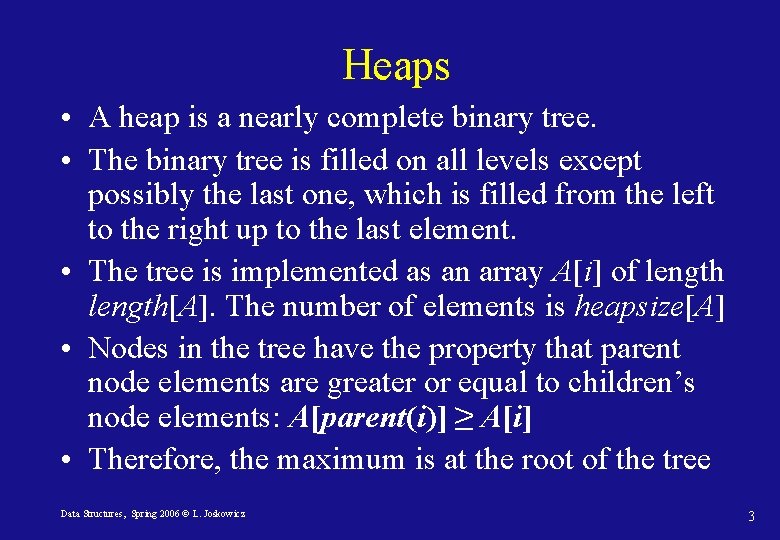
Heaps • A heap is a nearly complete binary tree. • The binary tree is filled on all levels except possibly the last one, which is filled from the left to the right up to the last element. • The tree is implemented as an array A[i] of length[A]. The number of elements is heapsize[A] • Nodes in the tree have the property that parent node elements are greater or equal to children’s node elements: A[parent(i)] ≥ A[i] • Therefore, the maximum is at the root of the tree Data Structures, Spring 2006 © L. Joskowicz 3
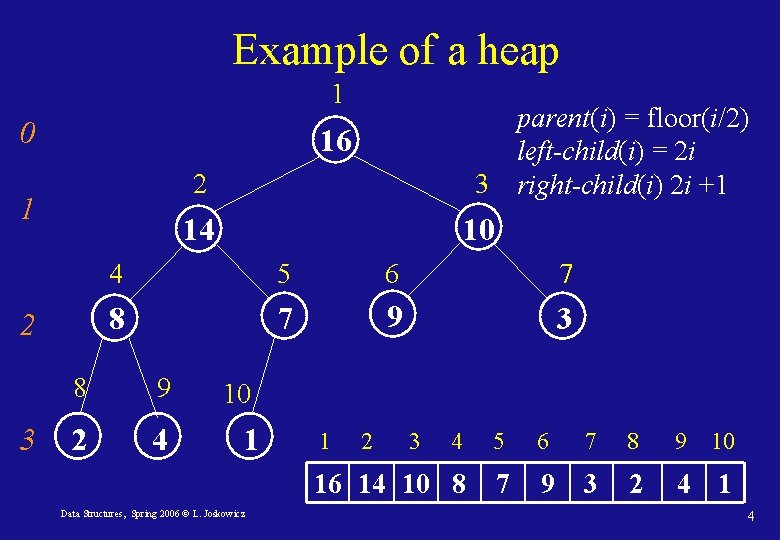
Example of a heap 1 0 16 2 1 14 2 3 parent(i) = floor(i/2) left-child(i) = 2 i 3 right-child(i) 2 i +1 10 4 5 6 7 8 7 9 3 8 9 2 4 10 1 Data Structures, Spring 2006 © L. Joskowicz 1 2 3 4 5 6 7 8 9 10 16 14 10 8 7 9 3 2 4 1 4
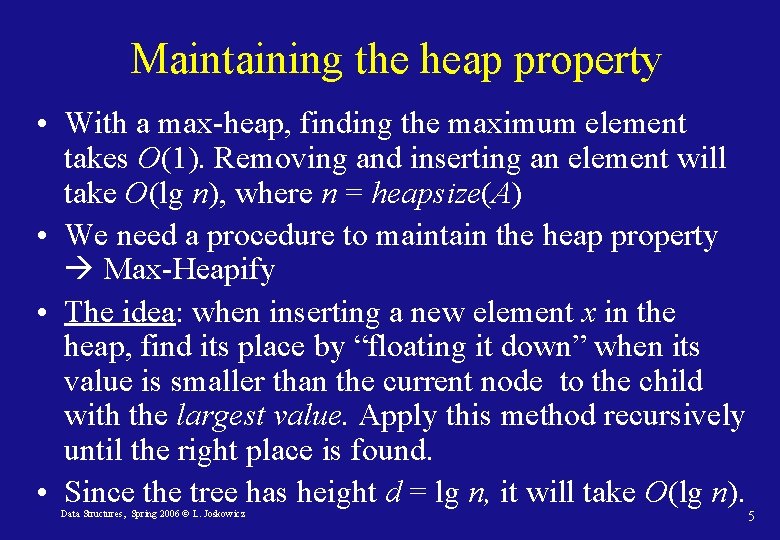
Maintaining the heap property • With a max-heap, finding the maximum element takes O(1). Removing and inserting an element will take O(lg n), where n = heapsize(A) • We need a procedure to maintain the heap property Max-Heapify • The idea: when inserting a new element x in the heap, find its place by “floating it down” when its value is smaller than the current node to the child with the largest value. Apply this method recursively until the right place is found. • Since the tree has height d = lg n, it will take O(lg n). Data Structures, Spring 2006 © L. Joskowicz 5
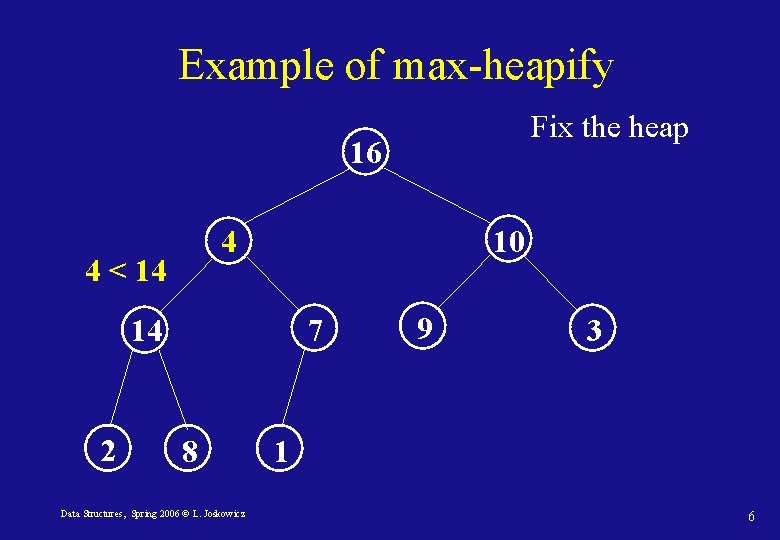
Example of max-heapify Fix the heap 16 4 4 < 14 10 14 2 7 8 Data Structures, Spring 2006 © L. Joskowicz 9 3 1 6
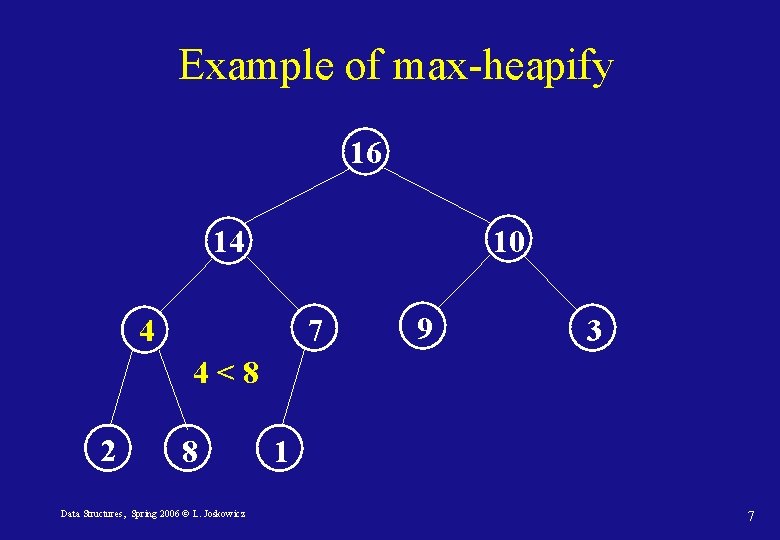
Example of max-heapify 16 14 10 4 7 9 3 4<8 2 8 Data Structures, Spring 2006 © L. Joskowicz 1 7
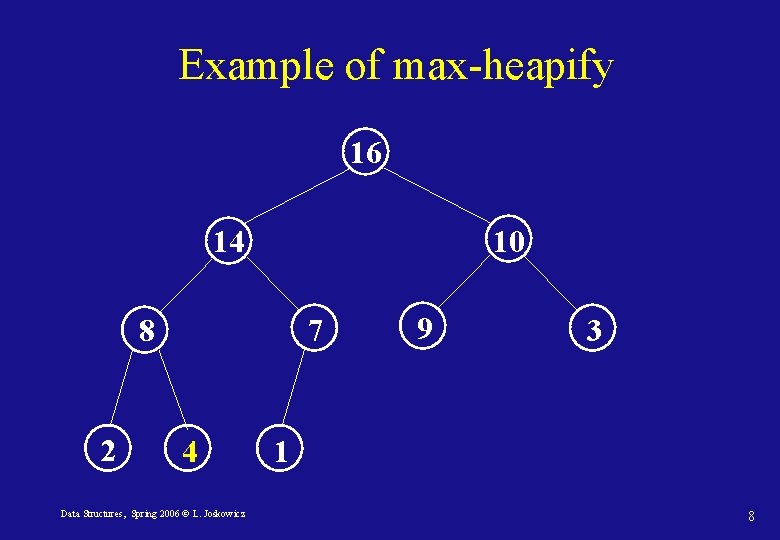
Example of max-heapify 16 14 10 8 2 7 4 Data Structures, Spring 2006 © L. Joskowicz 9 3 1 8
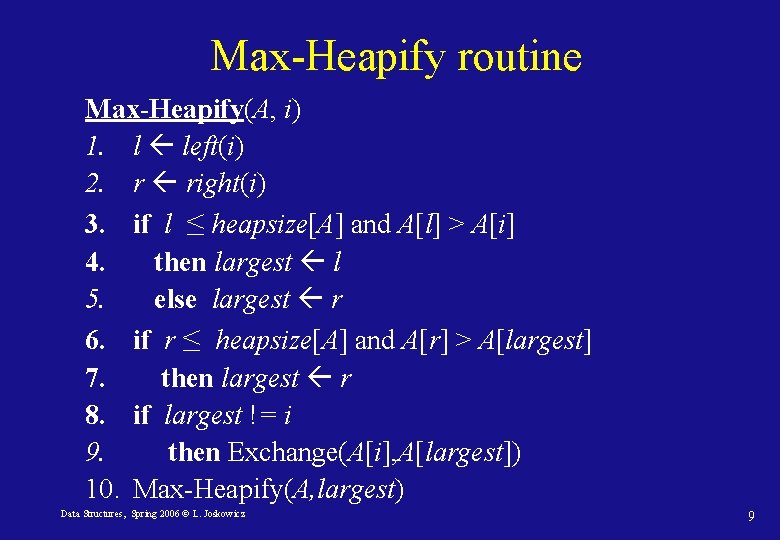
Max-Heapify routine Max-Heapify(A, i) 1. l left(i) 2. r right(i) 3. if l ≤ heapsize[A] and A[l] > A[i] 4. then largest l 5. else largest r 6. if r ≤ heapsize[A] and A[r] > A[largest] 7. then largest r 8. if largest != i 9. then Exchange(A[i], A[largest]) 10. Max-Heapify(A, largest) Data Structures, Spring 2006 © L. Joskowicz 9
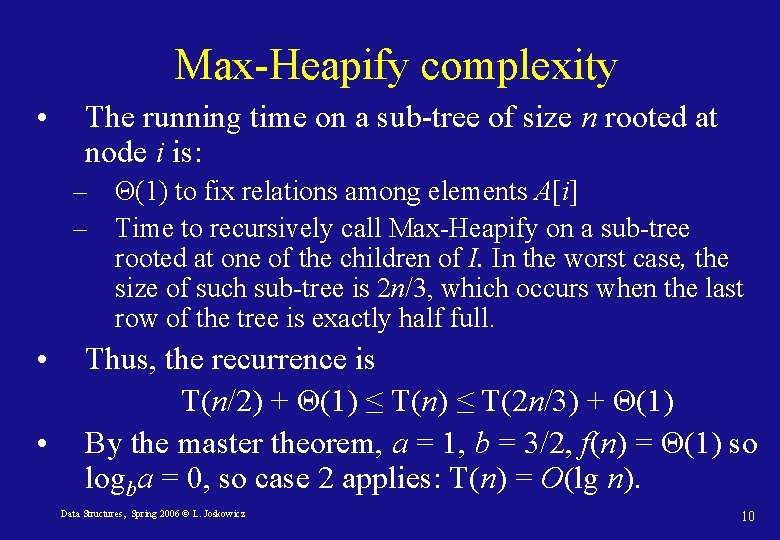
Max-Heapify complexity • The running time on a sub-tree of size n rooted at node i is: – Θ(1) to fix relations among elements A[i] – Time to recursively call Max-Heapify on a sub-tree rooted at one of the children of I. In the worst case, the size of such sub-tree is 2 n/3, which occurs when the last row of the tree is exactly half full. • • Thus, the recurrence is T(n/2) + Θ(1) ≤ T(n) ≤ T(2 n/3) + Θ(1) By the master theorem, a = 1, b = 3/2, f(n) = Θ(1) so logba = 0, so case 2 applies: T(n) = O(lg n). Data Structures, Spring 2006 © L. Joskowicz 10
![Building a heap Use MaxHeapify to recursively convert the array Ai into a Building a heap • Use Max-Heapify to recursively convert the array A[i] into a](https://slidetodoc.com/presentation_image_h/35b388dd831010ed01b00b482cfb9e6b/image-11.jpg)
Building a heap • Use Max-Heapify to recursively convert the array A[i] into a max-heap from bottom to top • The elements in the sub-array are all leaves of the tree, so each is a 1 -element heap to begin with. The Build-Max-Heap procedure has to go through the remaining nodes of the tree and run Max-Heapify on each one Build-Max-Heap(A) 1. heapsize[A] length(A) 2. for i downto 1 3. do Max-Heapify(A, i) Data Structures, Spring 2006 © L. Joskowicz 11
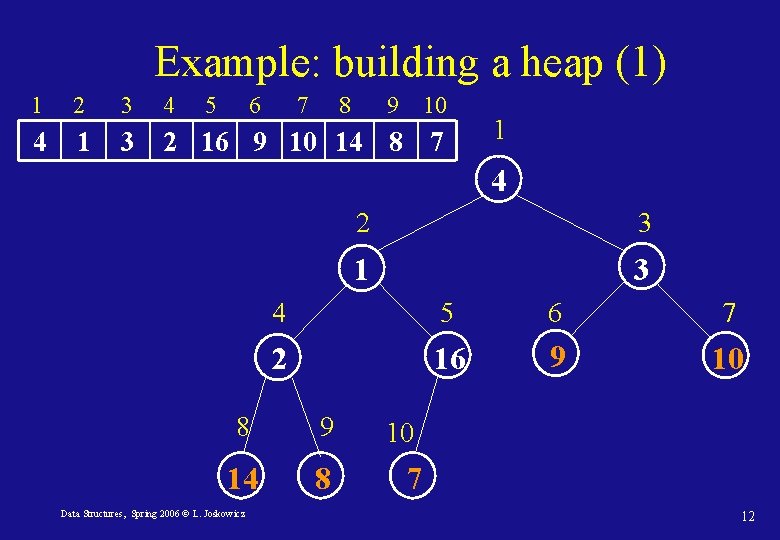
Example: building a heap (1) 1 2 3 4 5 6 7 8 9 10 4 1 3 2 16 9 10 14 8 7 1 4 3 1 3 4 5 6 7 2 16 9 10 8 9 14 8 Data Structures, Spring 2006 © L. Joskowicz 2 10 7 12
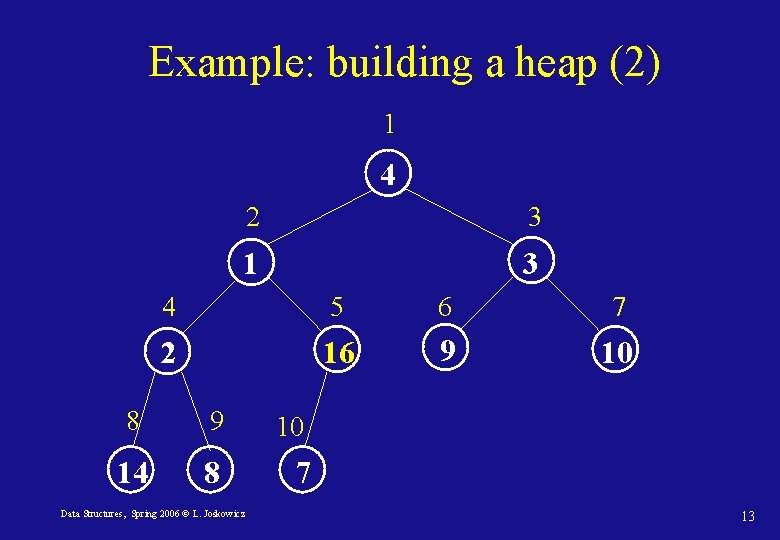
Example: building a heap (2) 1 4 2 3 1 3 4 5 6 7 2 16 9 10 8 9 14 8 Data Structures, Spring 2006 © L. Joskowicz 10 7 13
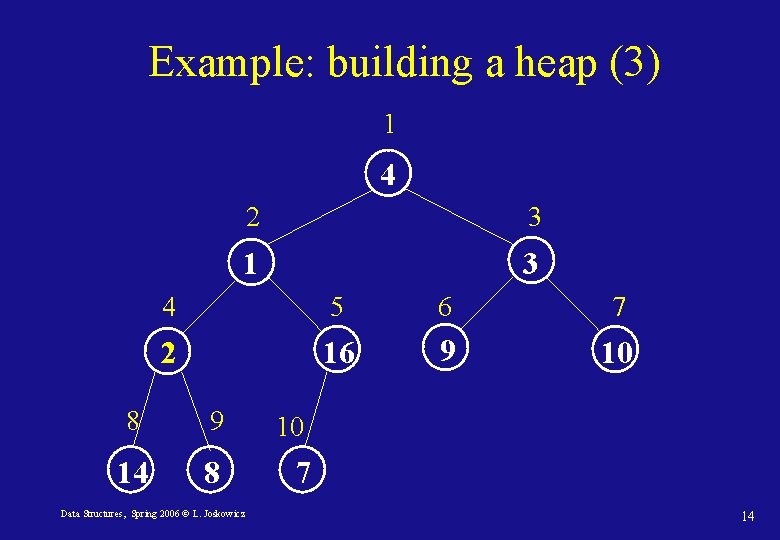
Example: building a heap (3) 1 4 2 3 1 3 4 5 6 7 2 16 9 10 8 9 14 8 Data Structures, Spring 2006 © L. Joskowicz 10 7 14
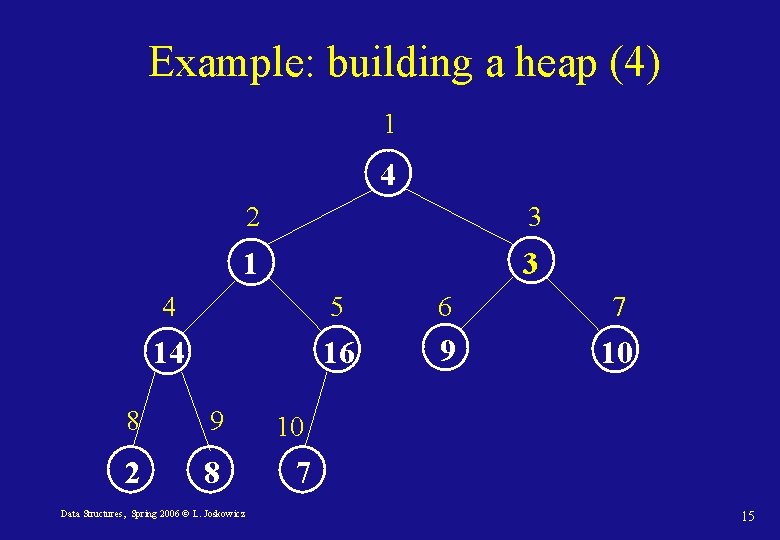
Example: building a heap (4) 1 4 2 3 1 3 4 5 6 7 14 16 9 10 8 9 2 8 Data Structures, Spring 2006 © L. Joskowicz 10 7 15
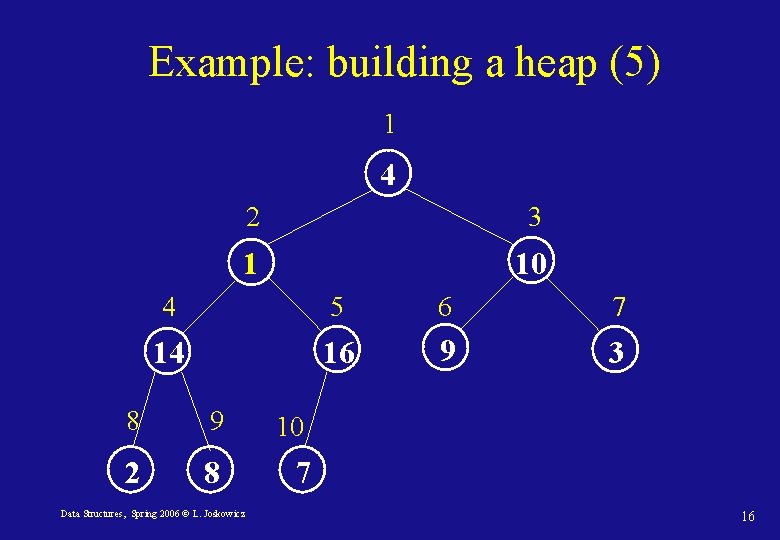
Example: building a heap (5) 1 4 2 3 1 10 4 5 6 7 14 16 9 3 8 9 2 8 Data Structures, Spring 2006 © L. Joskowicz 10 7 16
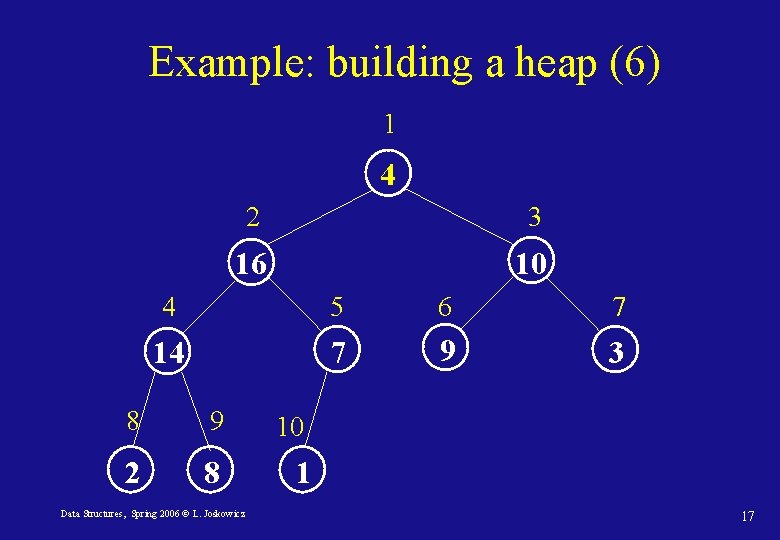
Example: building a heap (6) 1 4 2 3 16 10 4 5 6 7 14 7 9 3 8 9 2 8 Data Structures, Spring 2006 © L. Joskowicz 10 1 17
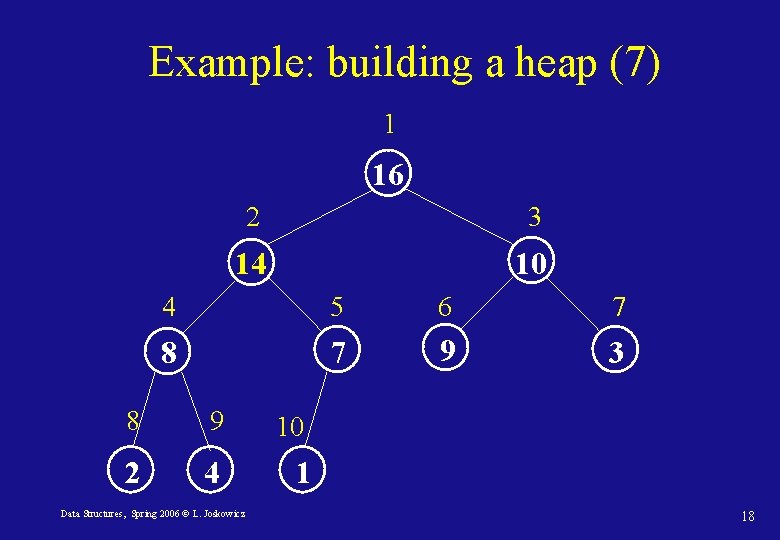
Example: building a heap (7) 1 16 2 3 14 10 4 5 6 7 8 7 9 3 8 9 2 4 Data Structures, Spring 2006 © L. Joskowicz 10 1 18
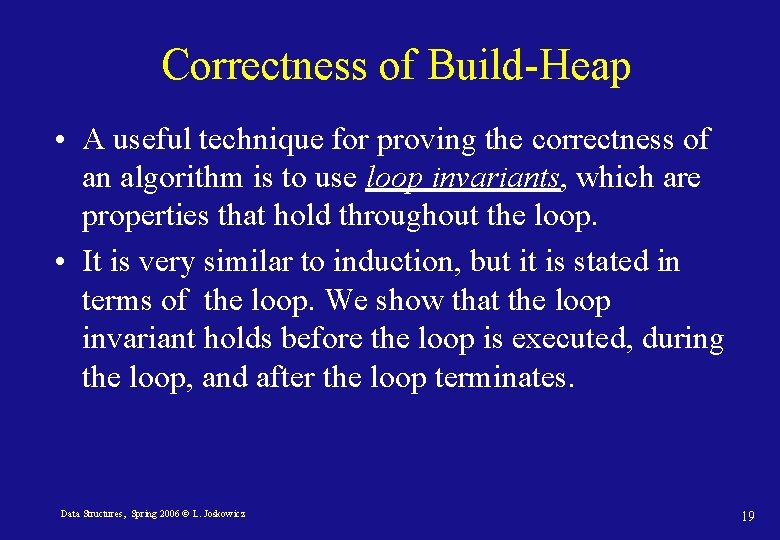
Correctness of Build-Heap • A useful technique for proving the correctness of an algorithm is to use loop invariants, which are properties that hold throughout the loop. • It is very similar to induction, but it is stated in terms of the loop. We show that the loop invariant holds before the loop is executed, during the loop, and after the loop terminates. Data Structures, Spring 2006 © L. Joskowicz 19
![Invariant of BuildHeap BuildMaxHeapA 1 heapsizeA lengthA 2 for i downto 1 3 do Invariant of Build-Heap Build-Max-Heap(A) 1. heapsize[A] length(A) 2. for i downto 1 3. do](https://slidetodoc.com/presentation_image_h/35b388dd831010ed01b00b482cfb9e6b/image-20.jpg)
Invariant of Build-Heap Build-Max-Heap(A) 1. heapsize[A] length(A) 2. for i downto 1 3. do Max-Heapify(A, i) The loop invariant is: Before the execution of each for step each node i + 1, i + 2, … , n is the root of a max-heap Data Structures, Spring 2006 © L. Joskowicz 20
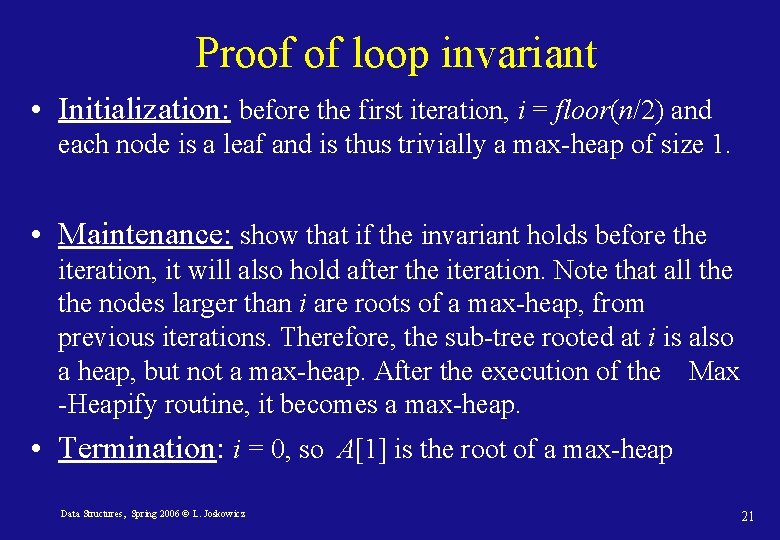
Proof of loop invariant • Initialization: before the first iteration, i = floor(n/2) and each node is a leaf and is thus trivially a max-heap of size 1. • Maintenance: show that if the invariant holds before the iteration, it will also hold after the iteration. Note that all the nodes larger than i are roots of a max-heap, from previous iterations. Therefore, the sub-tree rooted at i is also a heap, but not a max-heap. After the execution of the Max -Heapify routine, it becomes a max-heap. • Termination: i = 0, so A[1] is the root of a max-heap Data Structures, Spring 2006 © L. Joskowicz 21
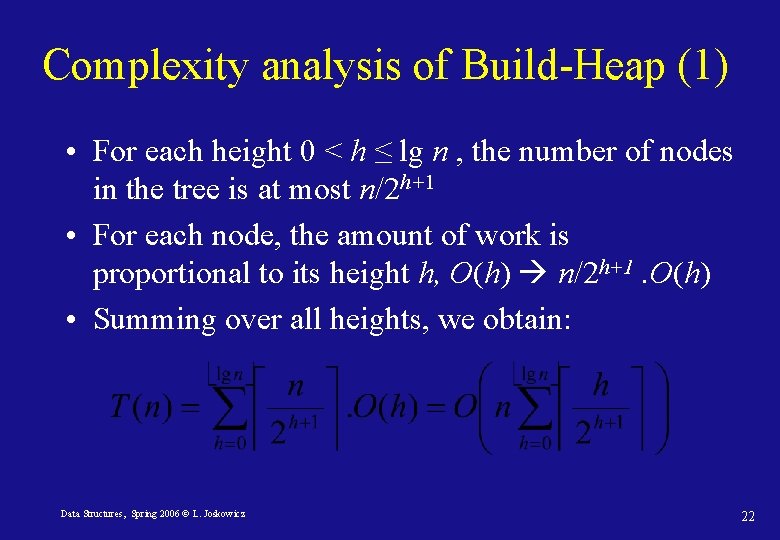
Complexity analysis of Build-Heap (1) • For each height 0 < h ≤ lg n , the number of nodes in the tree is at most n/2 h+1 • For each node, the amount of work is proportional to its height h, O(h) n/2 h+1. O(h) • Summing over all heights, we obtain: Data Structures, Spring 2006 © L. Joskowicz 22
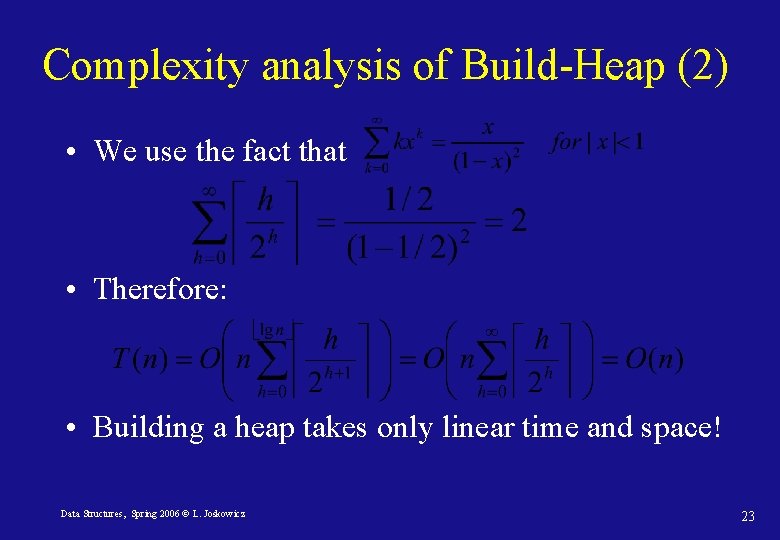
Complexity analysis of Build-Heap (2) • We use the fact that • Therefore: • Building a heap takes only linear time and space! Data Structures, Spring 2006 © L. Joskowicz 23
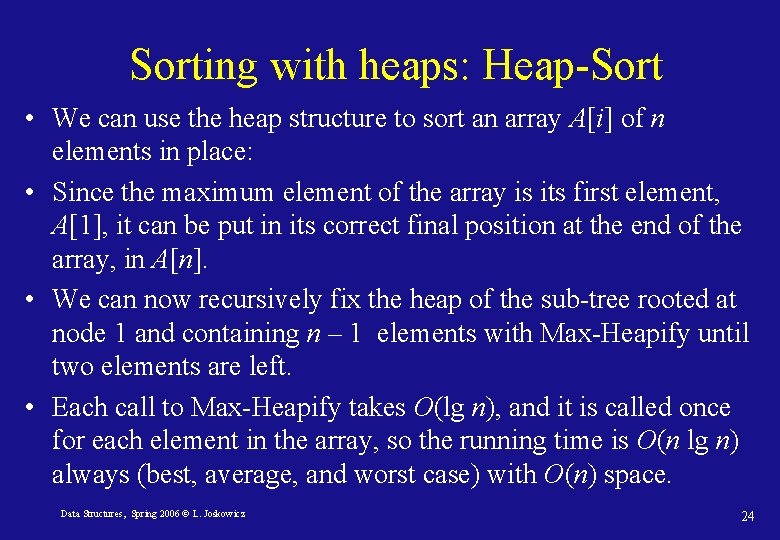
Sorting with heaps: Heap-Sort • We can use the heap structure to sort an array A[i] of n elements in place: • Since the maximum element of the array is its first element, A[1], it can be put in its correct final position at the end of the array, in A[n]. • We can now recursively fix the heap of the sub-tree rooted at node 1 and containing n – 1 elements with Max-Heapify until two elements are left. • Each call to Max-Heapify takes O(lg n), and it is called once for each element in the array, so the running time is O(n lg n) always (best, average, and worst case) with O(n) space. Data Structures, Spring 2006 © L. Joskowicz 24
![HeapSortA 1 BuildMaxHeapA 2 heapsizeA lengthA 3 for i lengthA downto 2 put maximum Heap-Sort(A) 1. Build-Max-Heap(A) 2. heapsize[A] length(A) 3. for i length[A] downto 2 put maximum](https://slidetodoc.com/presentation_image_h/35b388dd831010ed01b00b482cfb9e6b/image-25.jpg)
Heap-Sort(A) 1. Build-Max-Heap(A) 2. heapsize[A] length(A) 3. for i length[A] downto 2 put maximum at the root 4. do Exchange(A[1], A[i]) 5. heapsize[A] length(A) – 1 6. Max-Heapify(A, 1) Data Structures, Spring 2006 © L. Joskowicz 25
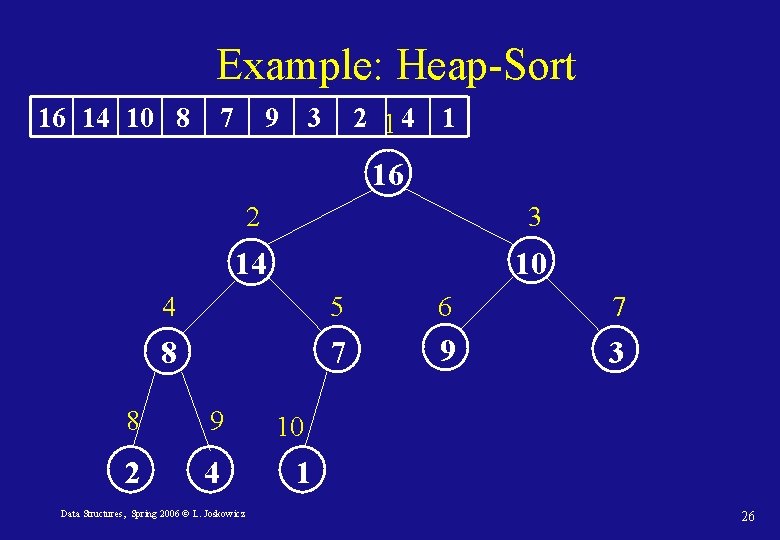
Example: Heap-Sort 16 14 10 8 7 9 3 2 14 1 16 2 3 14 10 4 5 6 7 8 7 9 3 8 9 2 4 Data Structures, Spring 2006 © L. Joskowicz 10 1 26
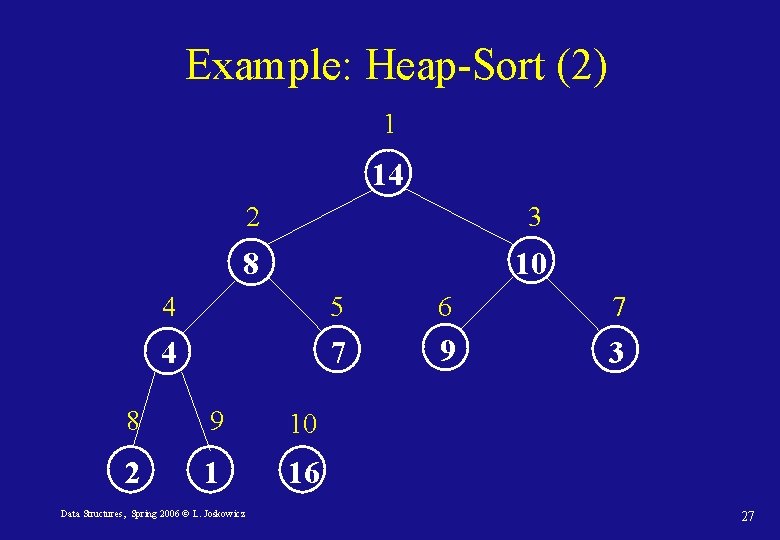
Example: Heap-Sort (2) 1 14 2 3 8 10 4 5 6 7 4 7 9 3 8 9 10 2 1 16 Data Structures, Spring 2006 © L. Joskowicz 27
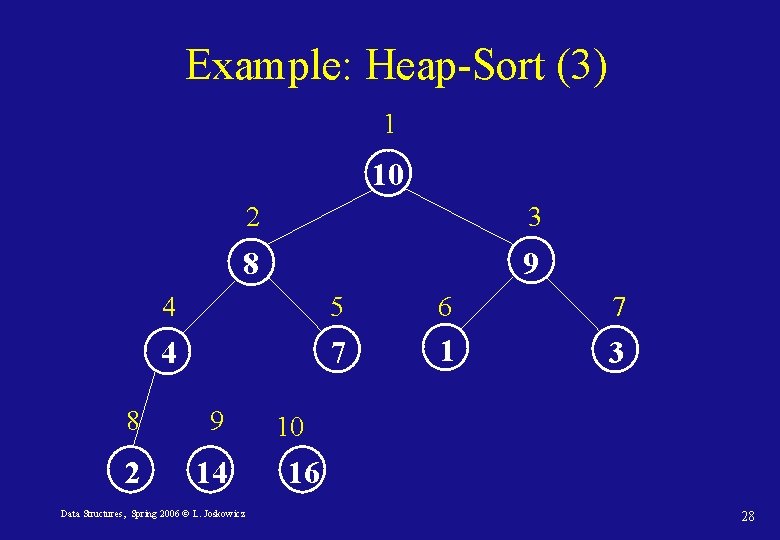
Example: Heap-Sort (3) 1 10 2 3 8 9 4 5 6 7 4 7 1 3 8 9 2 14 Data Structures, Spring 2006 © L. Joskowicz 10 16 28
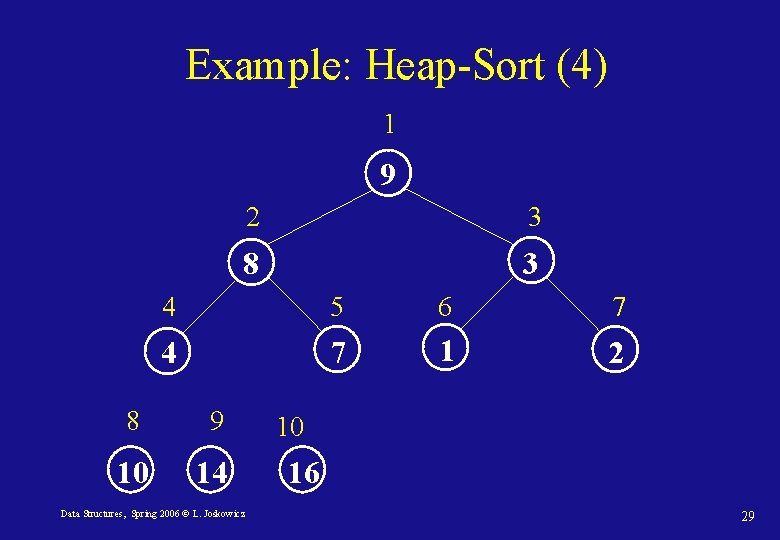
Example: Heap-Sort (4) 1 9 2 3 8 3 4 5 6 7 4 7 1 2 8 9 10 14 Data Structures, Spring 2006 © L. Joskowicz 10 16 29
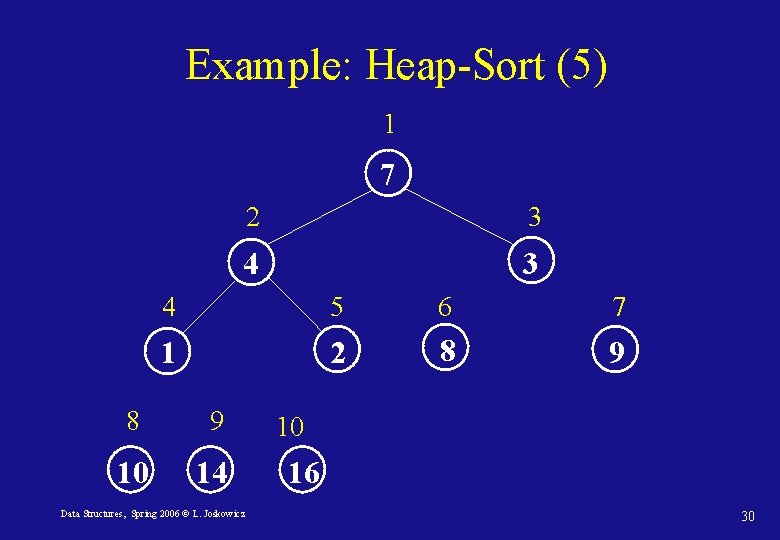
Example: Heap-Sort (5) 1 7 2 3 4 5 6 7 1 2 8 9 10 14 Data Structures, Spring 2006 © L. Joskowicz 10 16 30
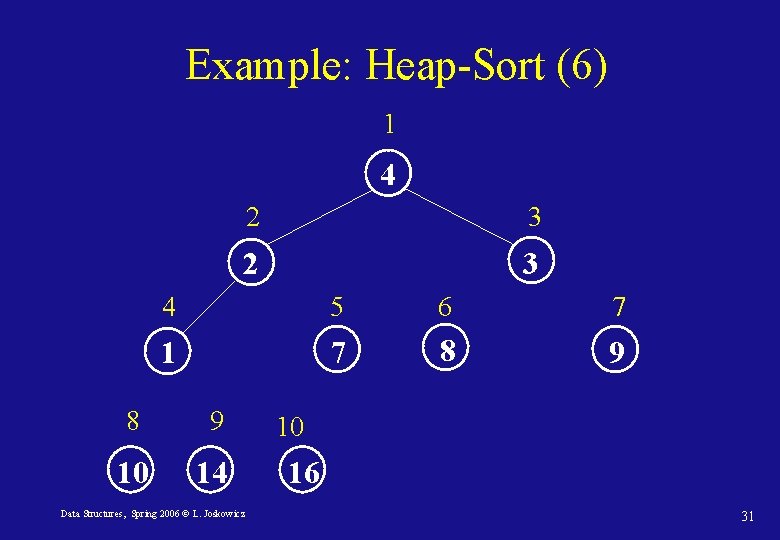
Example: Heap-Sort (6) 1 4 2 3 4 5 6 7 1 7 8 9 10 14 Data Structures, Spring 2006 © L. Joskowicz 10 16 31
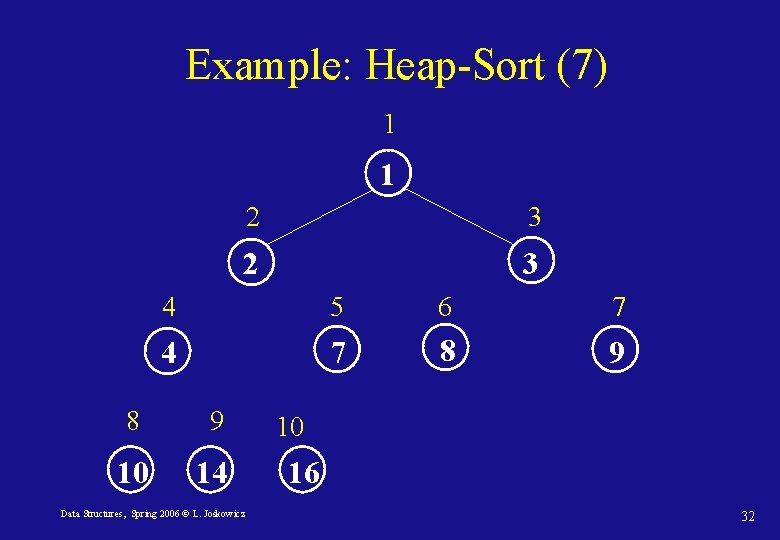
Example: Heap-Sort (7) 1 1 2 3 4 5 6 7 4 7 8 9 10 14 Data Structures, Spring 2006 © L. Joskowicz 10 16 32
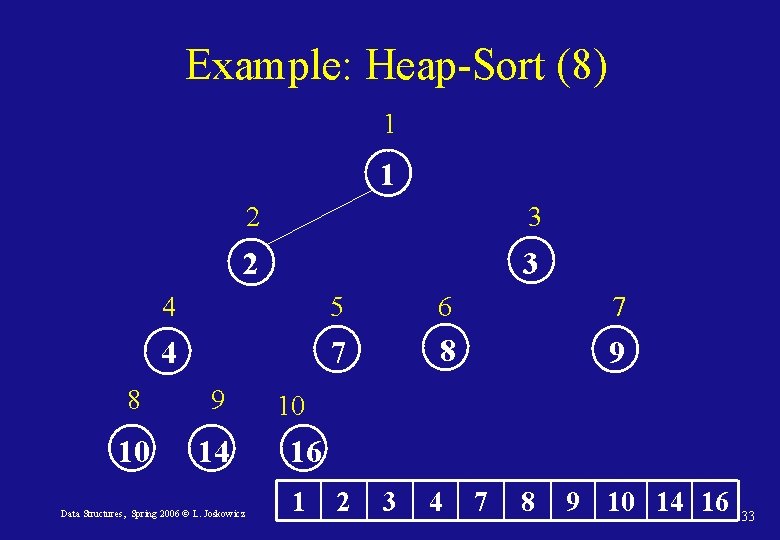
Example: Heap-Sort (8) 1 1 2 3 4 5 6 7 4 7 8 9 10 14 Data Structures, Spring 2006 © L. Joskowicz 10 16 1 2 3 4 7 8 9 10 14 16 33
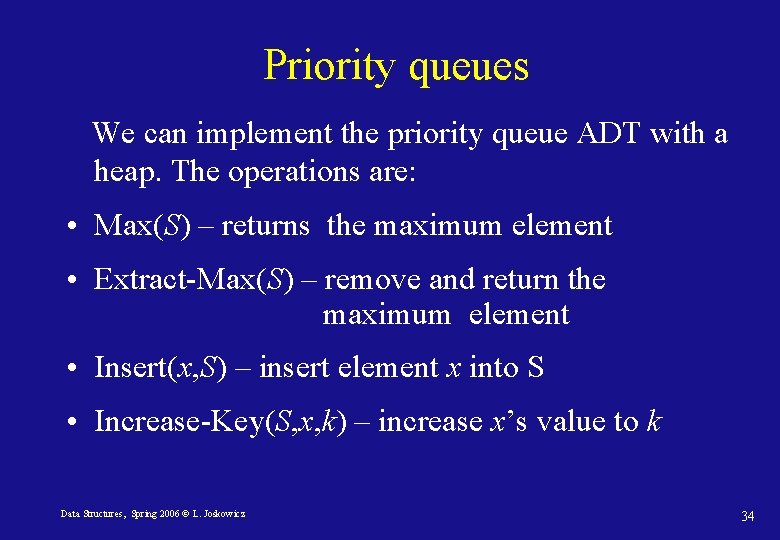
Priority queues We can implement the priority queue ADT with a heap. The operations are: • Max(S) – returns the maximum element • Extract-Max(S) – remove and return the maximum element • Insert(x, S) – insert element x into S • Increase-Key(S, x, k) – increase x’s value to k Data Structures, Spring 2006 © L. Joskowicz 34
![Priority queues ExtractMax HeapMaximumA return A1 HeapExtractMaxA 1 if heapsizeA 1 2 then Priority queues: Extract-Max Heap-Maximum(A) return A[1] Heap-Extract-Max(A) 1. if heapsize[A] < 1 2. then](https://slidetodoc.com/presentation_image_h/35b388dd831010ed01b00b482cfb9e6b/image-35.jpg)
Priority queues: Extract-Max Heap-Maximum(A) return A[1] Heap-Extract-Max(A) 1. if heapsize[A] < 1 2. then error “heap underflow” 3. max A[1] Make last 4. A[1] A[heapsize[A]] element the root 5. heapsize[A] – 1 6. Max-Heapify(A, 1) 7. return max Data Structures, Spring 2006 © L. Joskowicz 35
![Priority queues IncreaseKey HeapIncreasekeyA i key 1 if key Ai 2 then error Priority queues: Increase-Key Heap-Increase-key(A, i, key) 1. if key < A[i] 2. then error](https://slidetodoc.com/presentation_image_h/35b388dd831010ed01b00b482cfb9e6b/image-36.jpg)
Priority queues: Increase-Key Heap-Increase-key(A, i, key) 1. if key < A[i] 2. then error “new key smaller than existing one” 3. A[i] key 4. while i > 1 and A[parent(i)] < A[i] 5. do Exchange(A[i], parent(A[i])) 6. i parent(i) Data Structures, Spring 2006 © L. Joskowicz 36
![Priority queues InsertMax HeapInsertMaxA key 1 heapsizeA 1 2 AheapsizeA 3 Priority queues: Insert-Max Heap-Insert-Max(A, key) 1. heapsize[A] + 1 2. A[heapsize[A]] – ∞ 3.](https://slidetodoc.com/presentation_image_h/35b388dd831010ed01b00b482cfb9e6b/image-37.jpg)
Priority queues: Insert-Max Heap-Insert-Max(A, key) 1. heapsize[A] + 1 2. A[heapsize[A]] – ∞ 3. Heap-Increase-Key(A, heapsize[A], key) Data Structures, Spring 2006 © L. Joskowicz 37
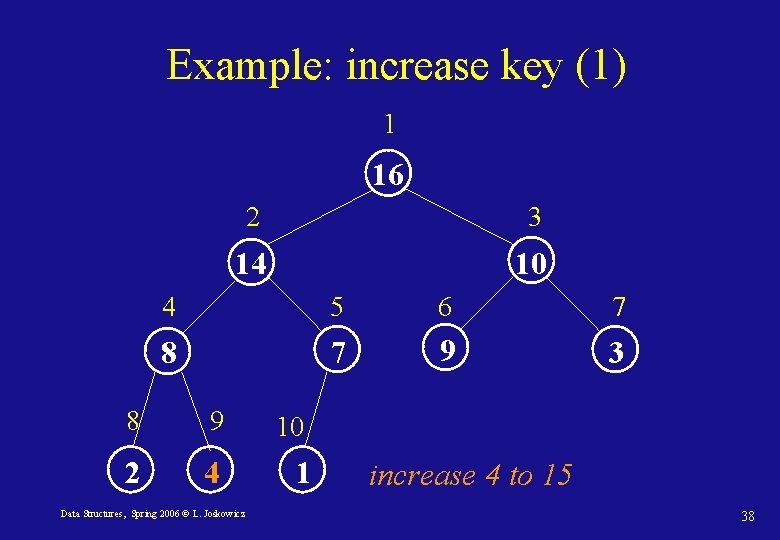
Example: increase key (1) 1 16 2 3 14 10 4 5 6 7 8 7 9 3 8 9 2 4 Data Structures, Spring 2006 © L. Joskowicz 10 1 increase 4 to 15 38
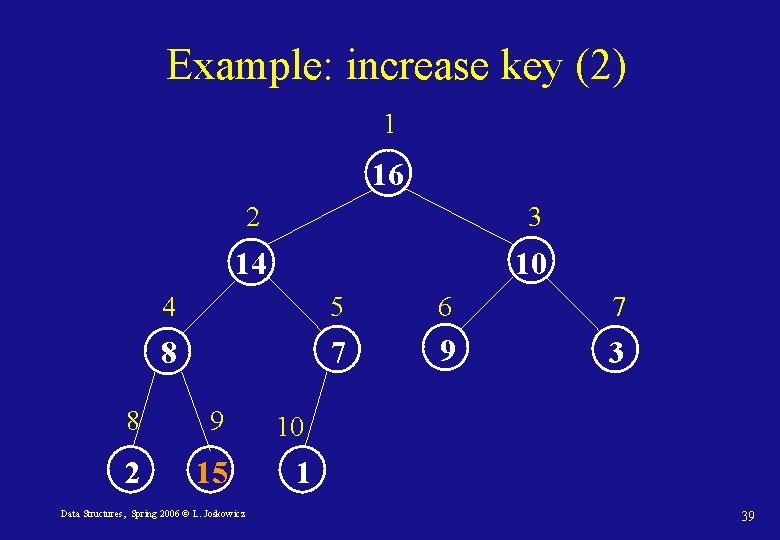
Example: increase key (2) 1 16 2 3 14 10 4 5 6 7 8 7 9 3 8 9 2 15 Data Structures, Spring 2006 © L. Joskowicz 10 1 39
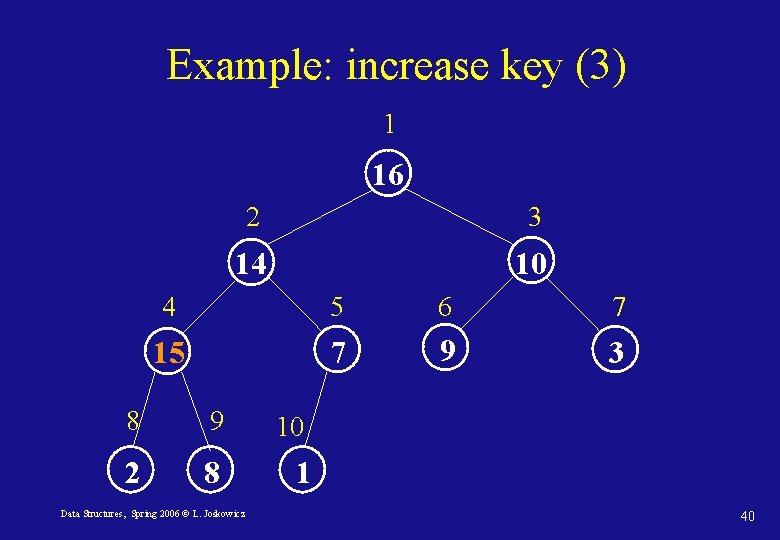
Example: increase key (3) 1 16 2 3 14 10 4 5 6 7 15 7 9 3 8 9 2 8 Data Structures, Spring 2006 © L. Joskowicz 10 1 40
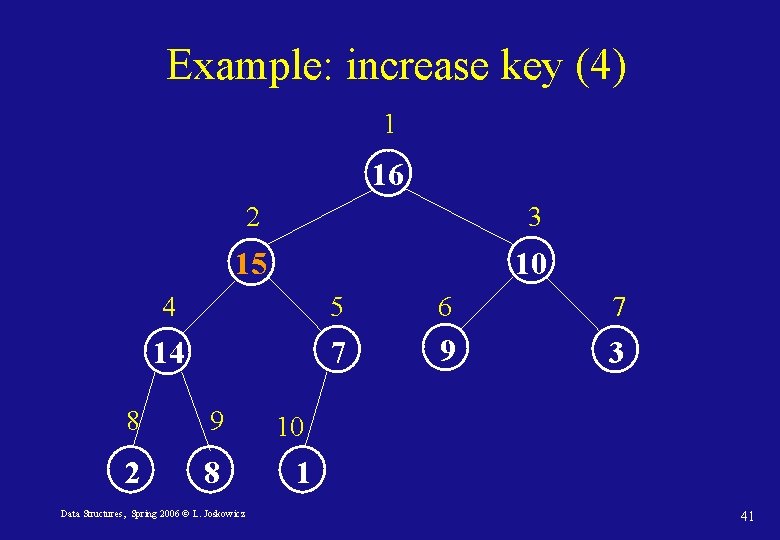
Example: increase key (4) 1 16 2 3 15 10 4 5 6 7 14 7 9 3 8 9 2 8 Data Structures, Spring 2006 © L. Joskowicz 10 1 41
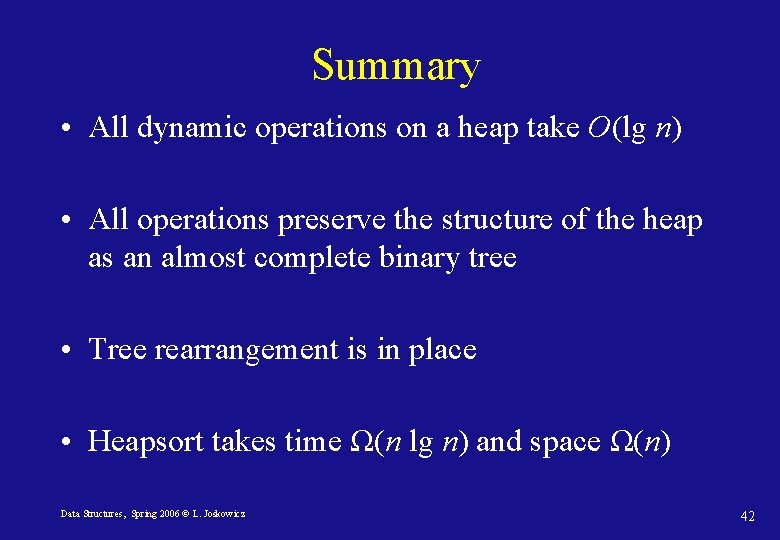
Summary • All dynamic operations on a heap take O(lg n) • All operations preserve the structure of the heap as an almost complete binary tree • Tree rearrangement is in place • Heapsort takes time Ω(n lg n) and space Ω(n) Data Structures, Spring 2006 © L. Joskowicz 42
Queue quiz
Adaptable priority queues
Applications of priority queues
Antrian q
Burman's priority list gives priority to
Priority mail vs priority mail express
Heap sort worst case
Heapsort visualization
Heapsort complexity analysis
Heapsort example
Heapsort inplace
Algoritmo heapsort
Heapsort vs quicksort
Heap sort algoritmo
Heapsort vs quicksort
Cqueue
Java stacks and queues
Java stack exercises
What are stacks
Queue representation
Message queues in unix
Rtos mailbox
Queues definition
Aganj
01:640:244 lecture notes - lecture 15: plat, idah, farad
Homologous
Priority queue abstract data type
Professor ajit diwan
Princeton data structures and algorithms
Data structures and algorithms tutorial
What is conditional macro expansion
Assembler algorithm and data structures
Information retrieval data structures and algorithms
Data structures and abstractions with java
Data structures and algorithms bits pilani
Adts, data structures, and problem solving with c++
Data structures and algorithms iit bombay
Data structures and algorithm
Data structures and algorithms
Data structures and algorithms
Ian munro waterloo
Signature file structure in information retrieval system
Ephemeral data structure