CS 378 Computer Game Technology Game Engine Architecture
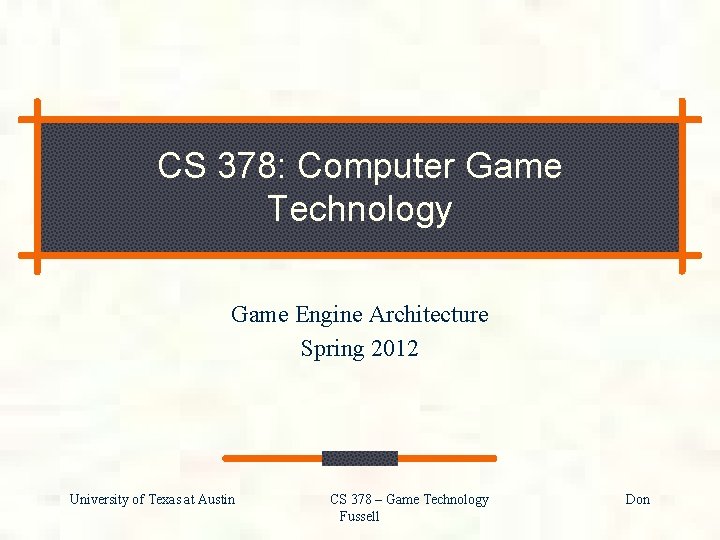
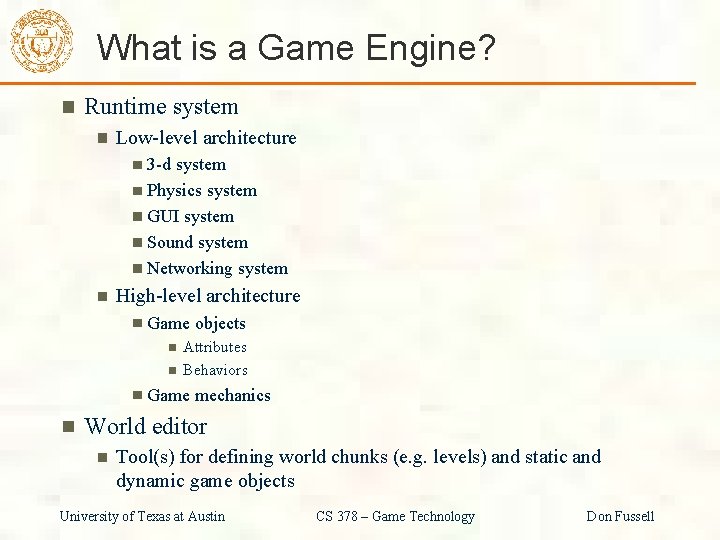
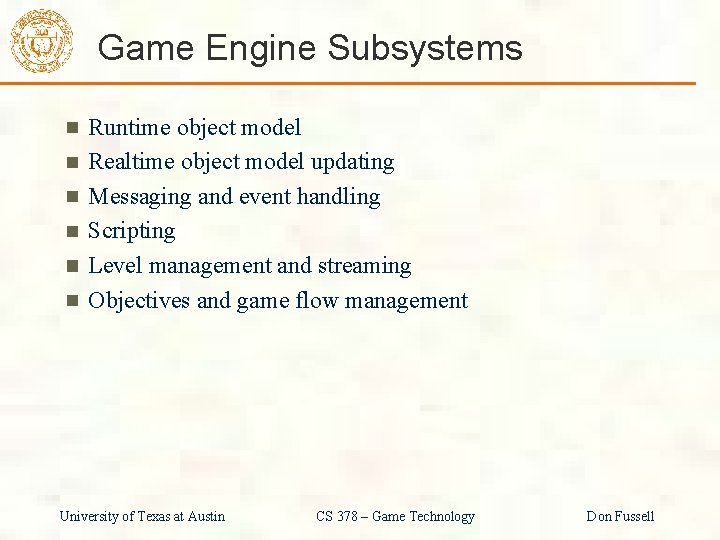
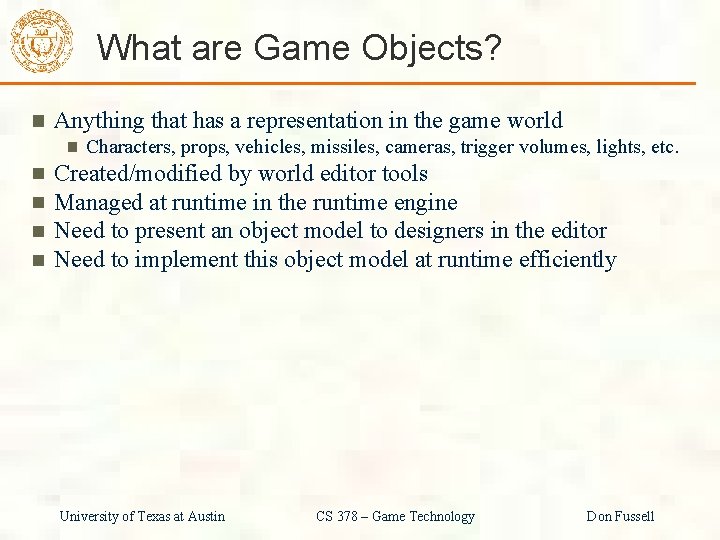
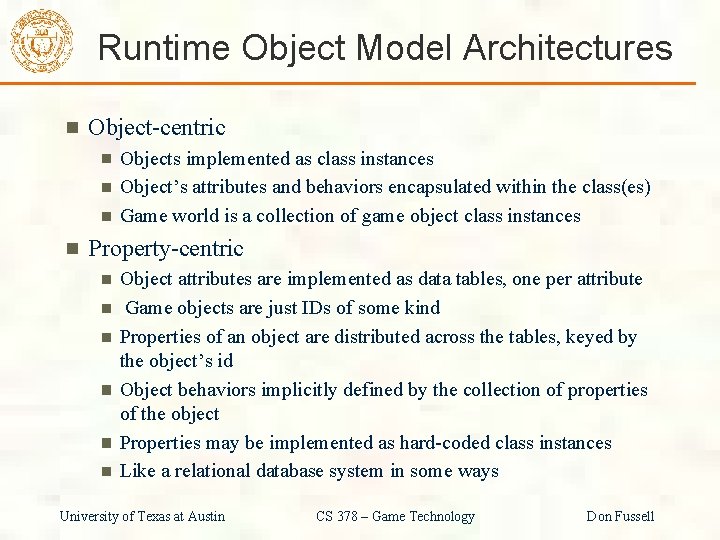
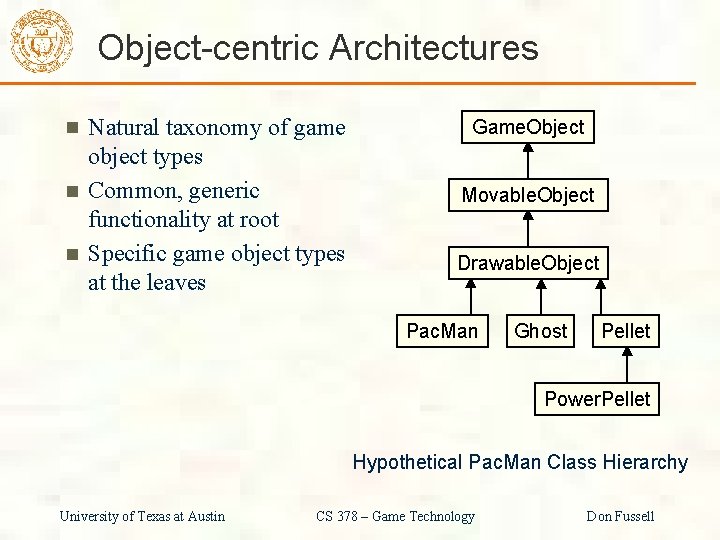
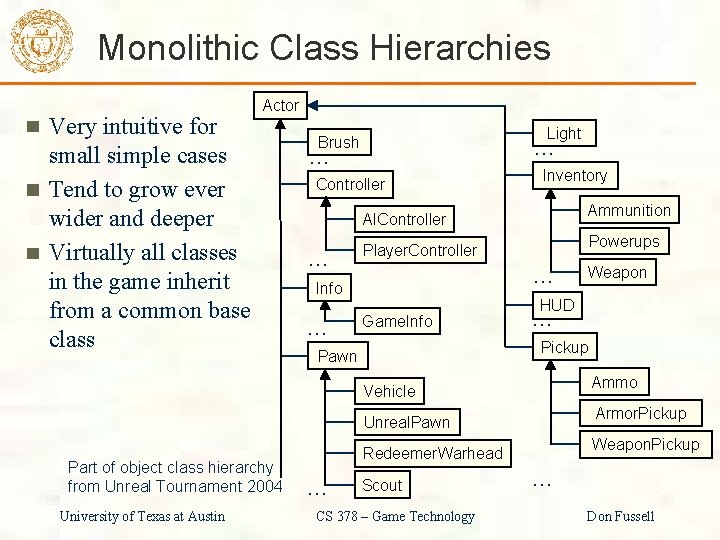
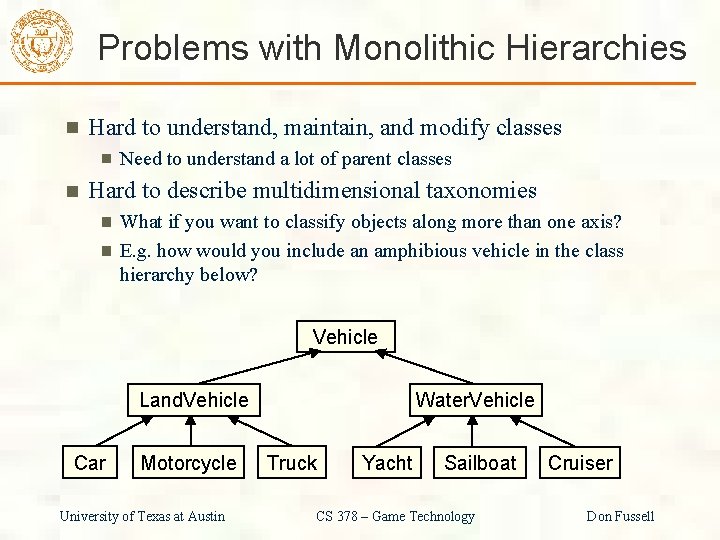
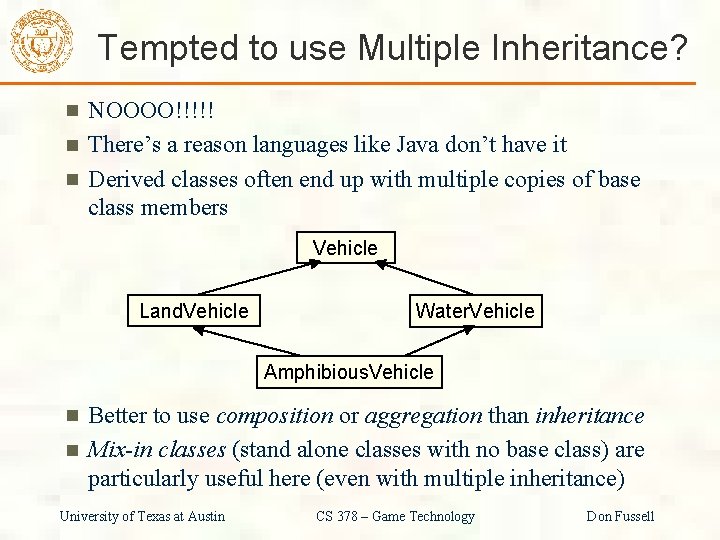
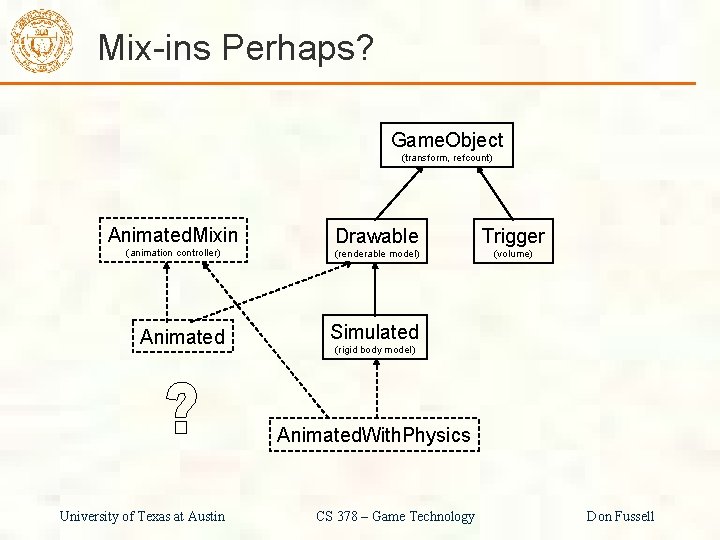
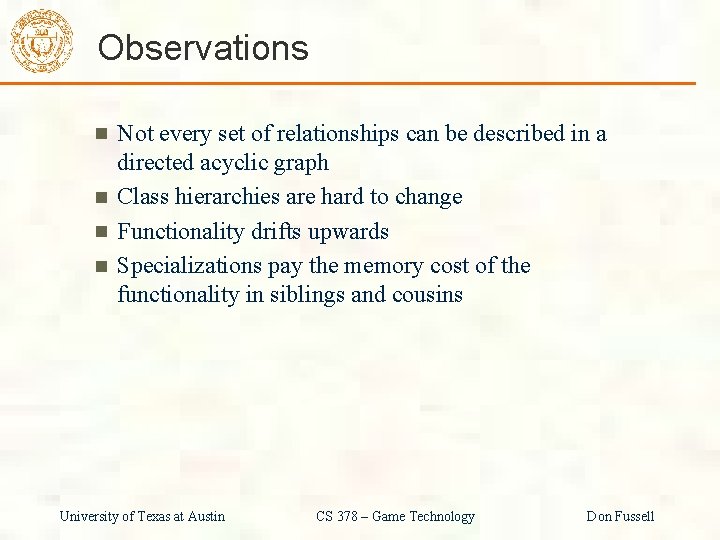
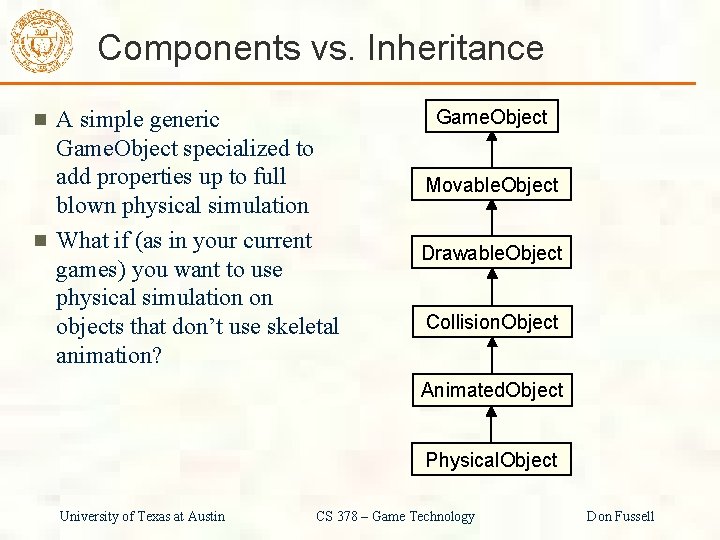
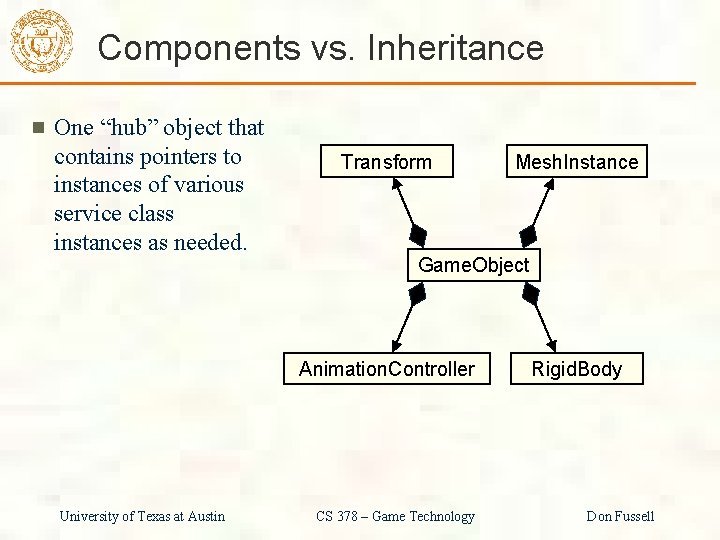
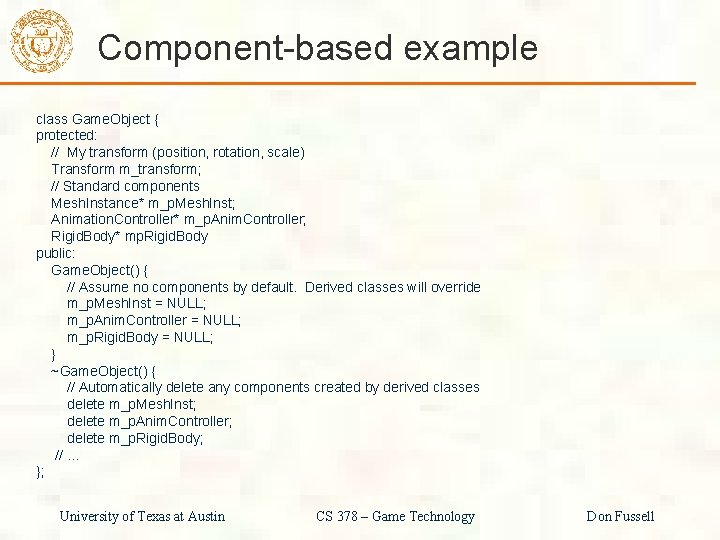
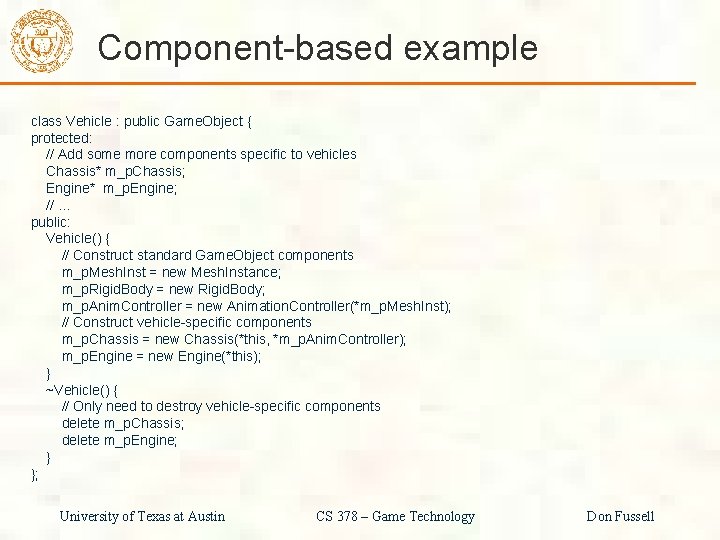
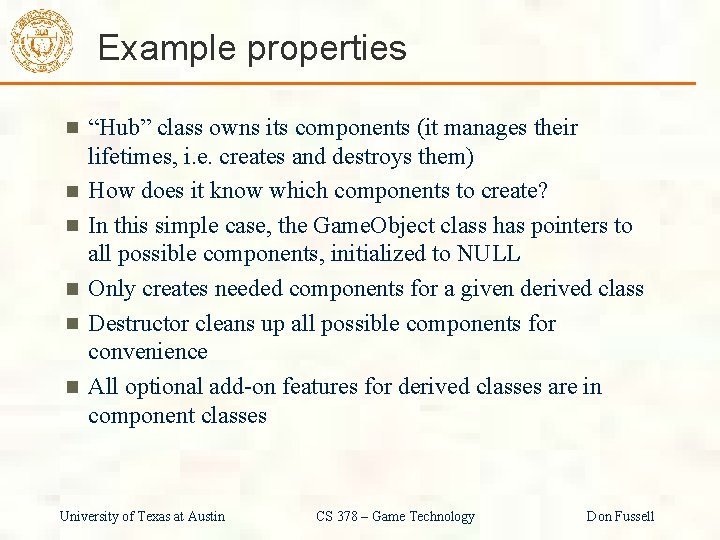
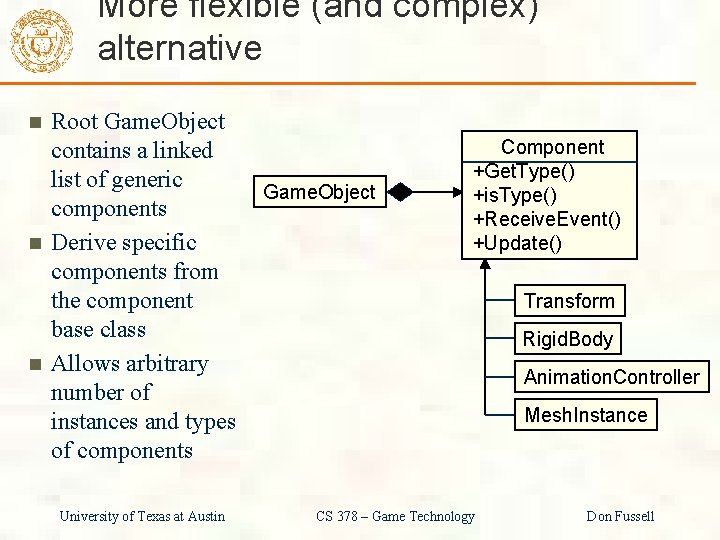
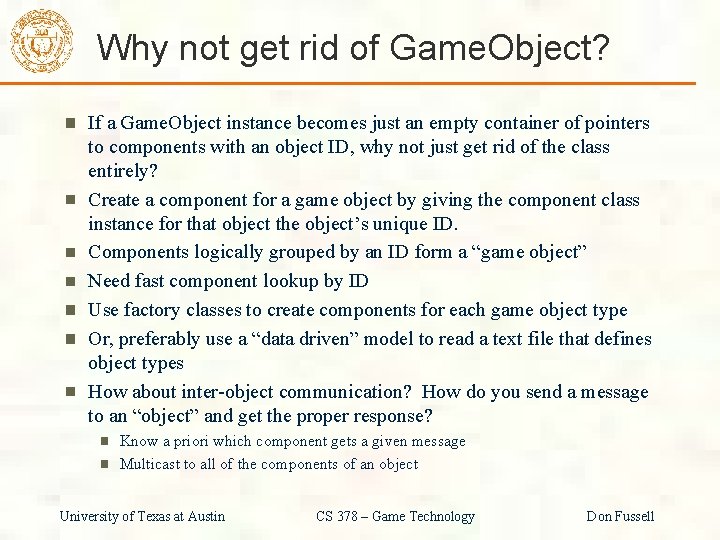
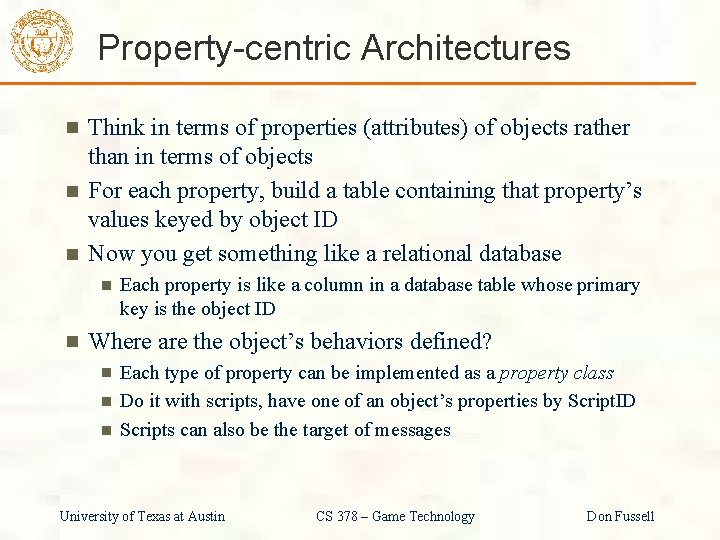
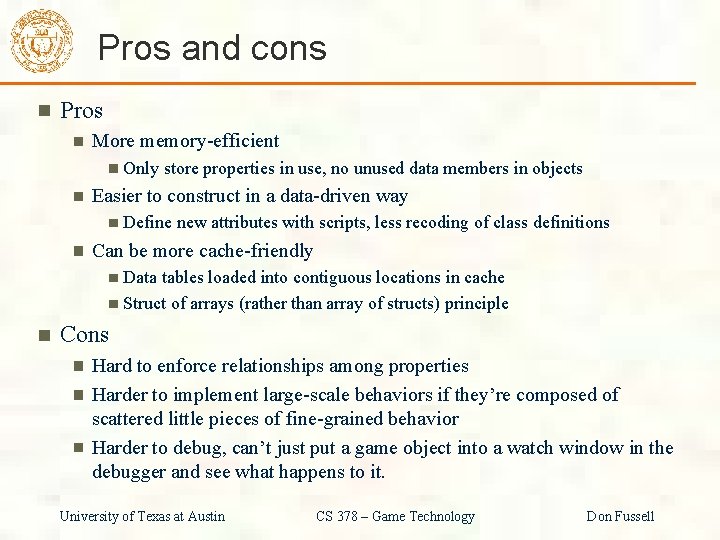
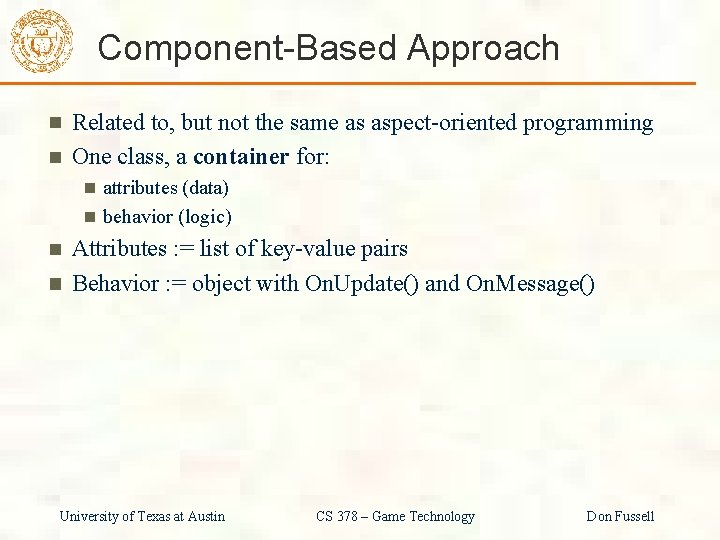
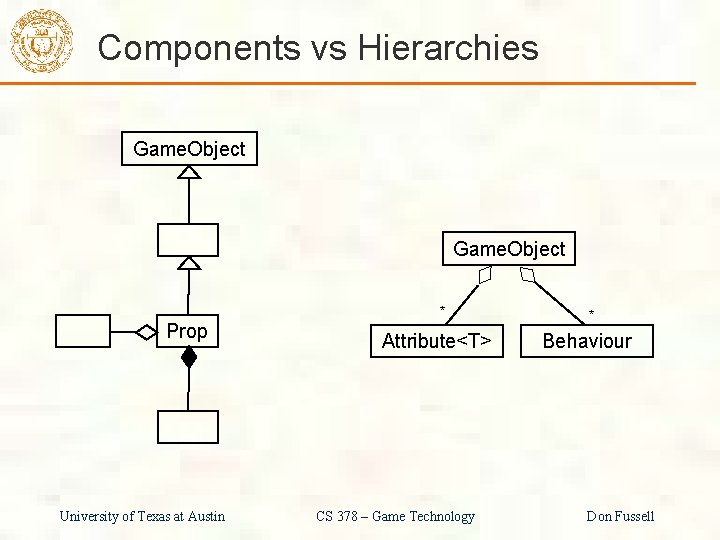
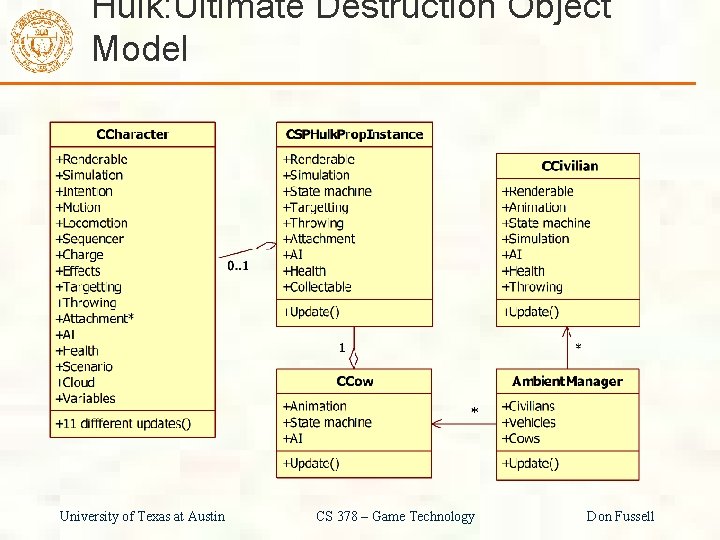
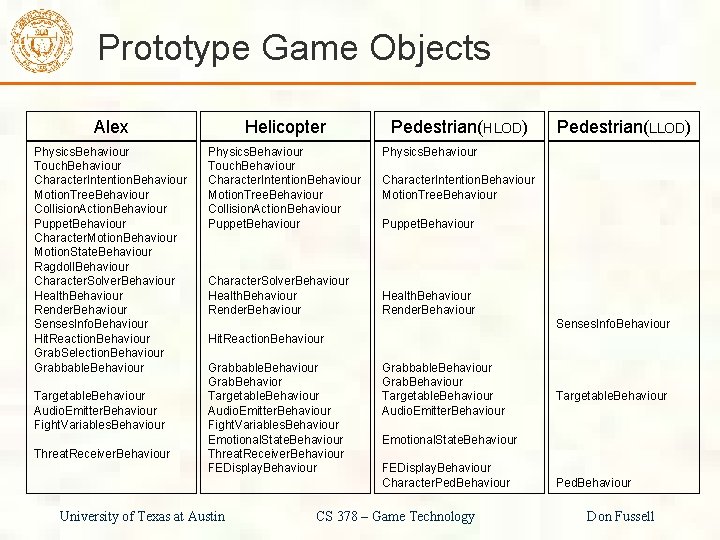
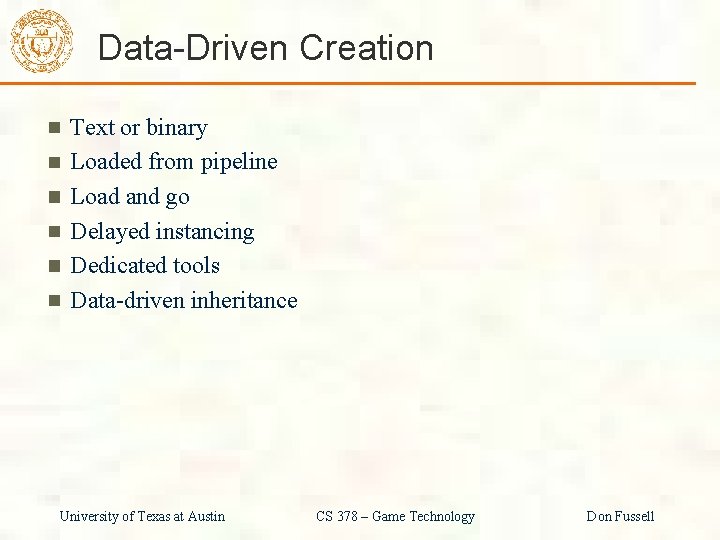
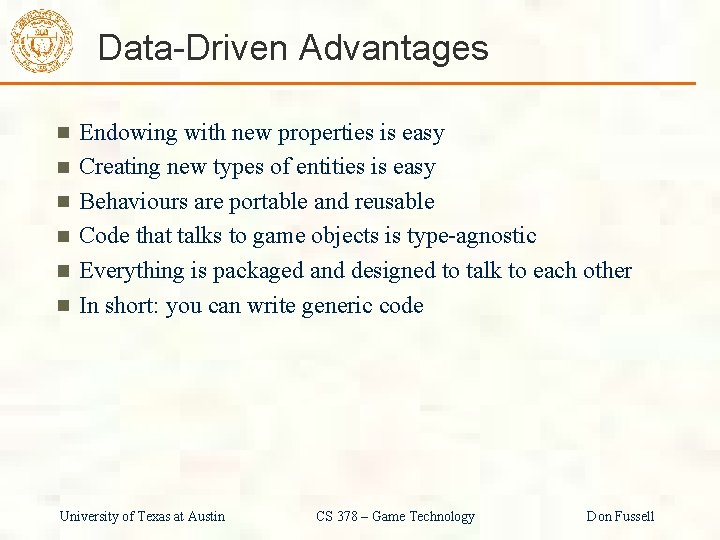
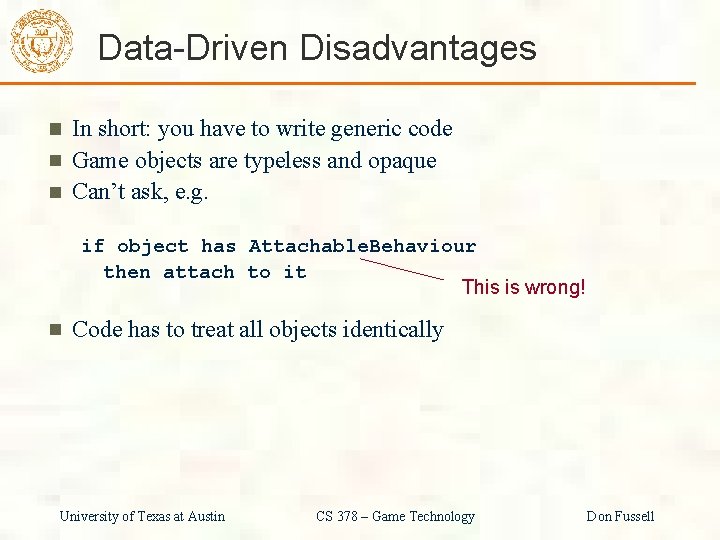
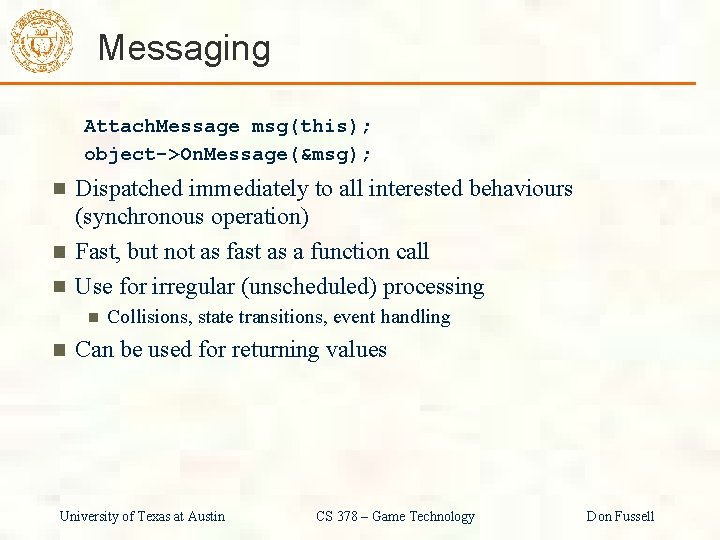
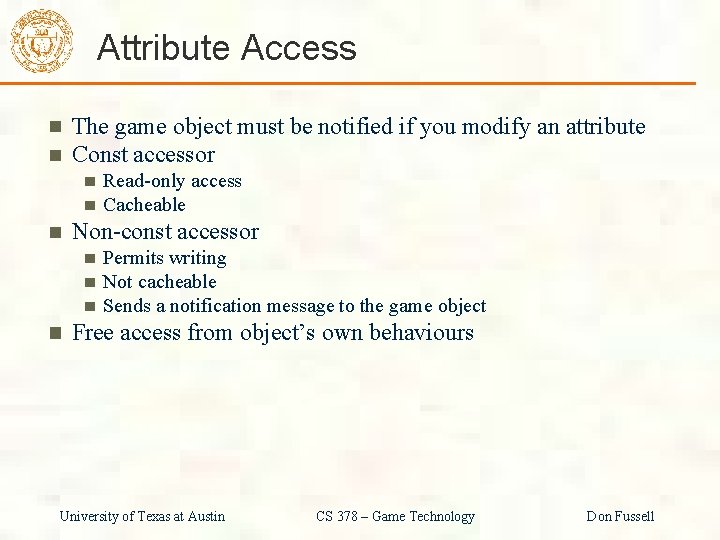
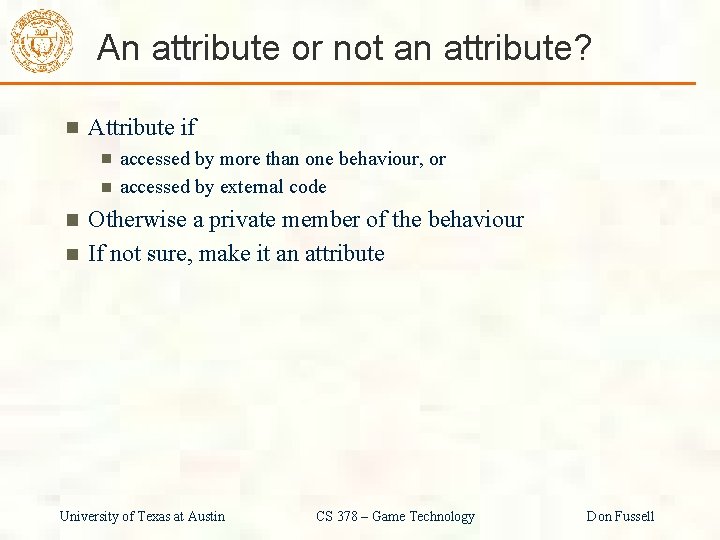
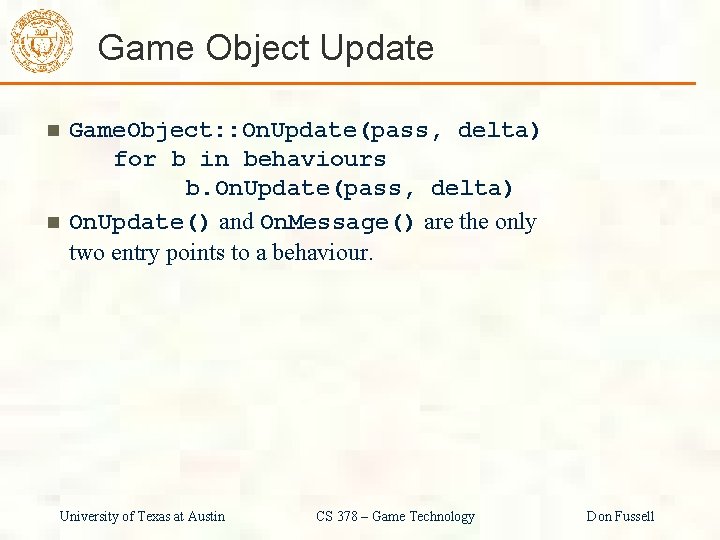
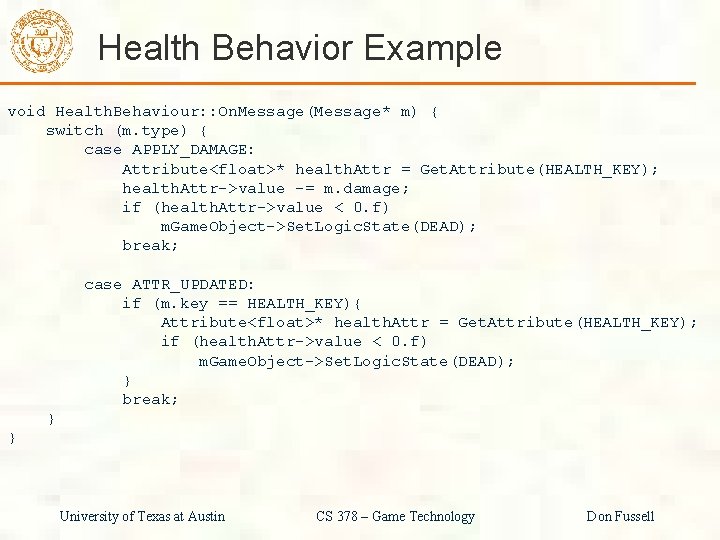
![Components in Practice Behaviours and Attributes in [PROTOTYPE] University of Texas at Austin CS Components in Practice Behaviours and Attributes in [PROTOTYPE] University of Texas at Austin CS](https://slidetodoc.com/presentation_image_h2/76aec75b6a5ee83b584d1512af757216/image-33.jpg)
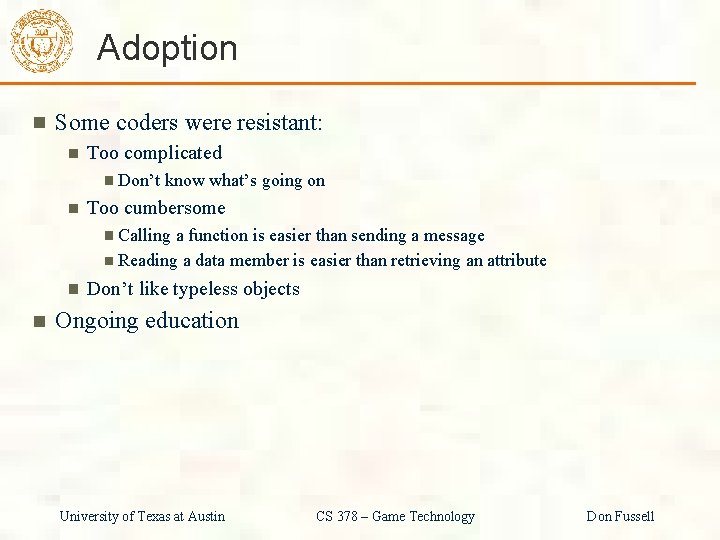
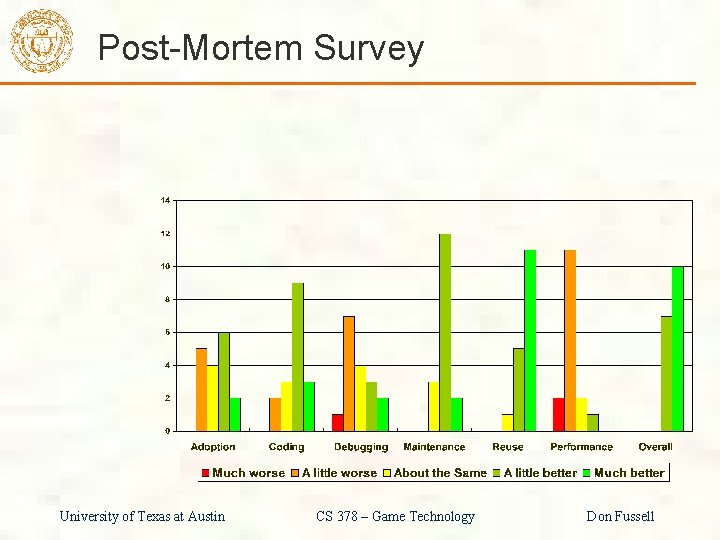
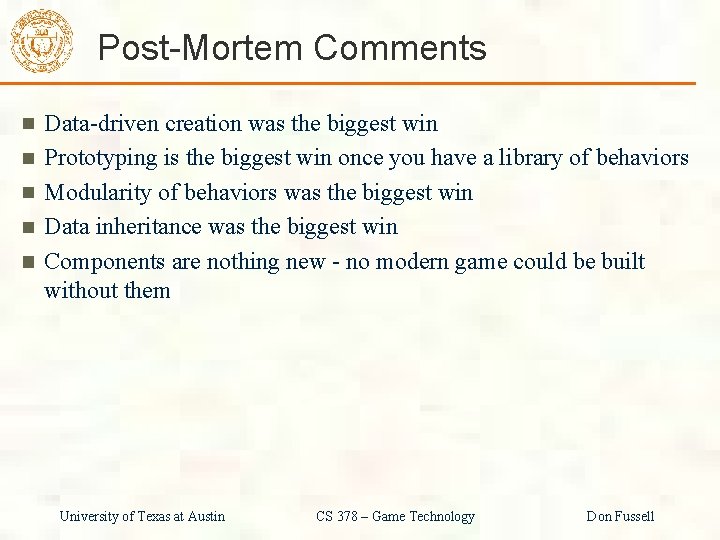
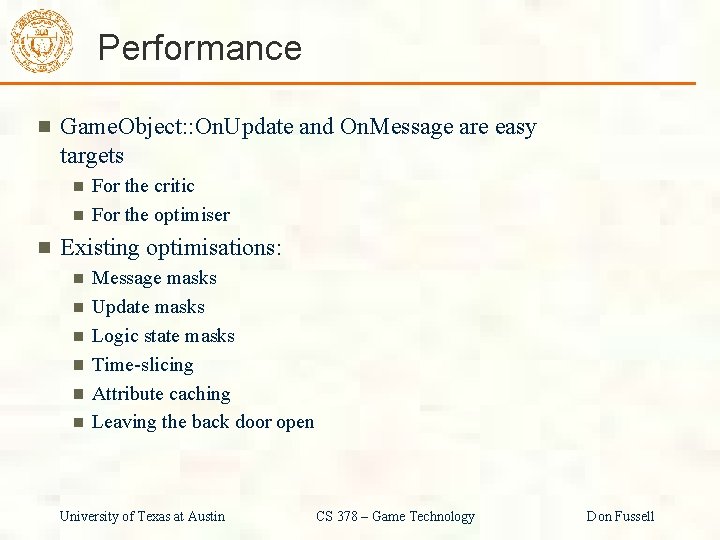
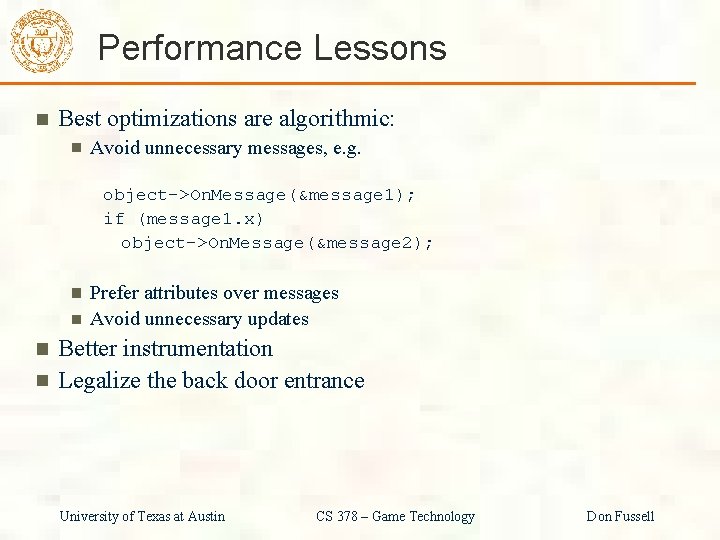
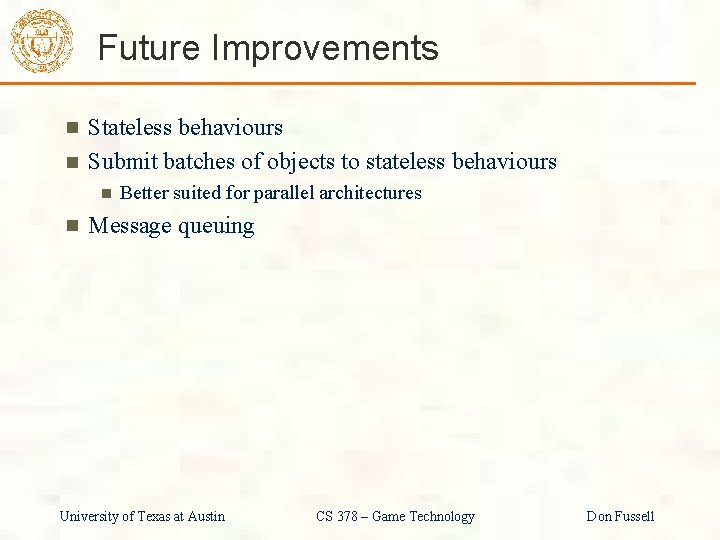
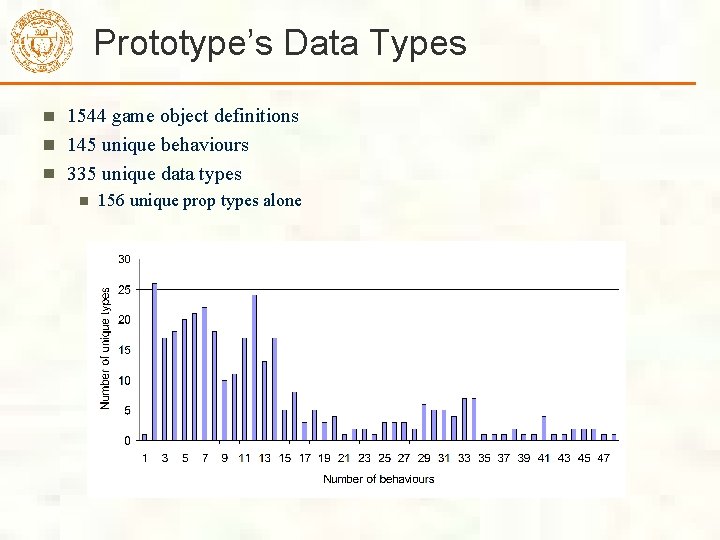
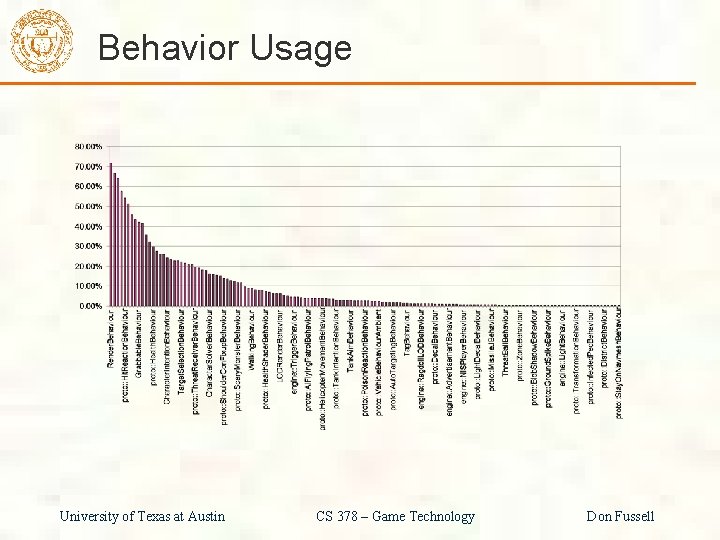
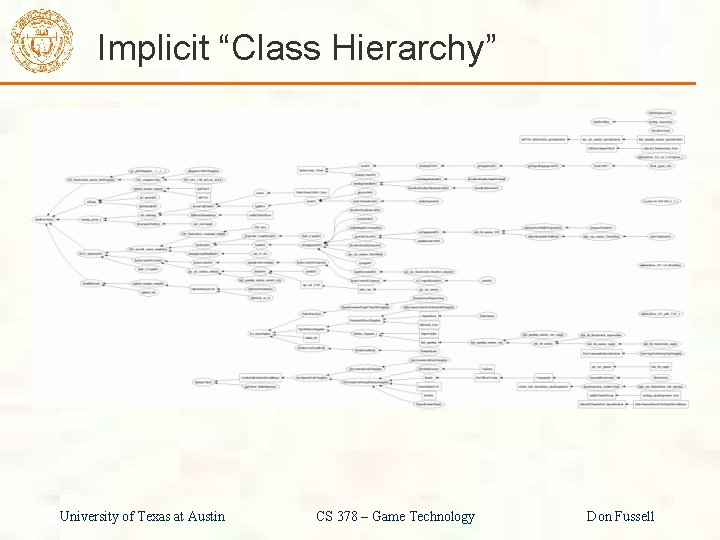
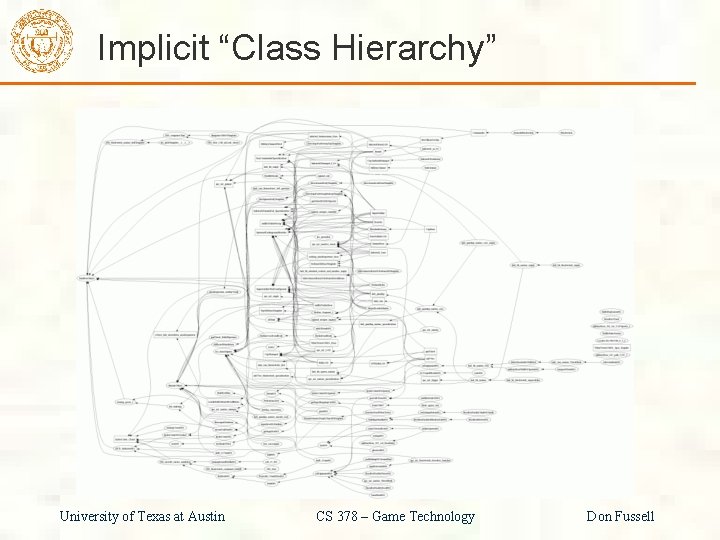
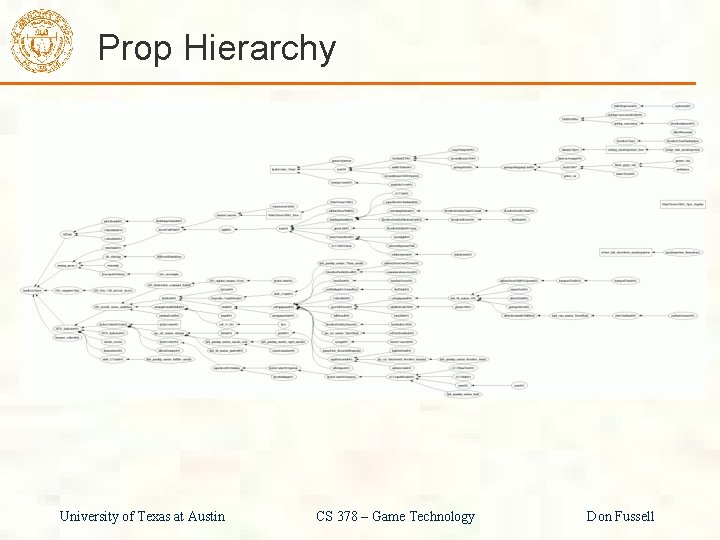
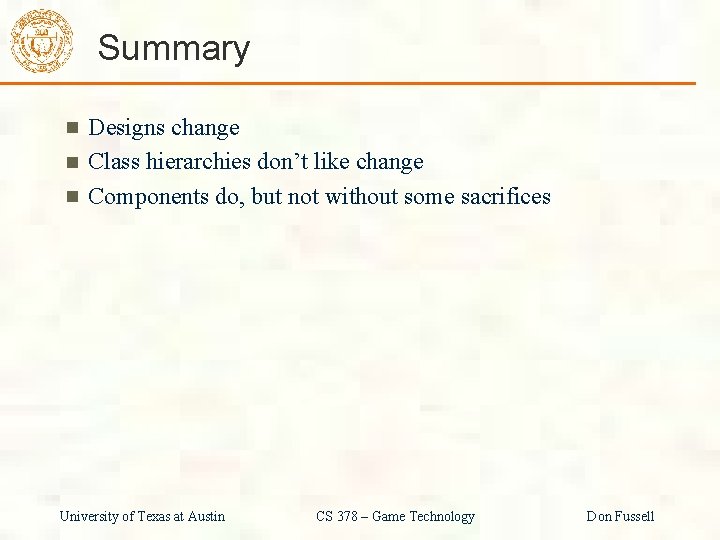
- Slides: 45
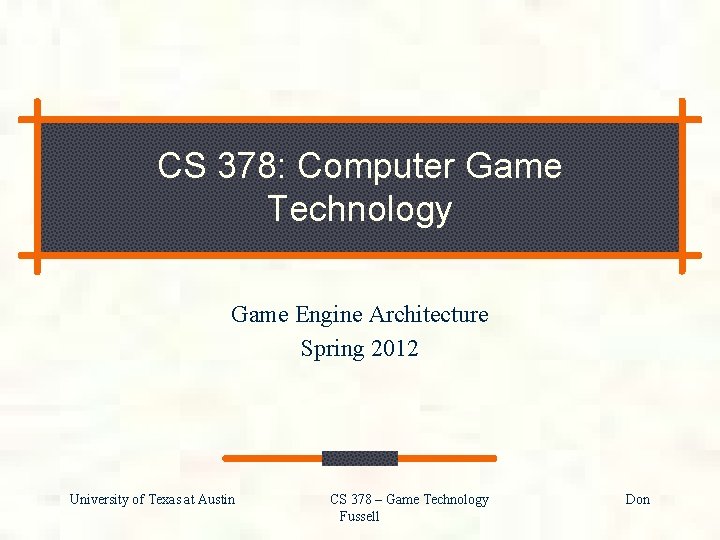
CS 378: Computer Game Technology Game Engine Architecture Spring 2012 University of Texas at Austin CS 378 – Game Technology Fussell Don
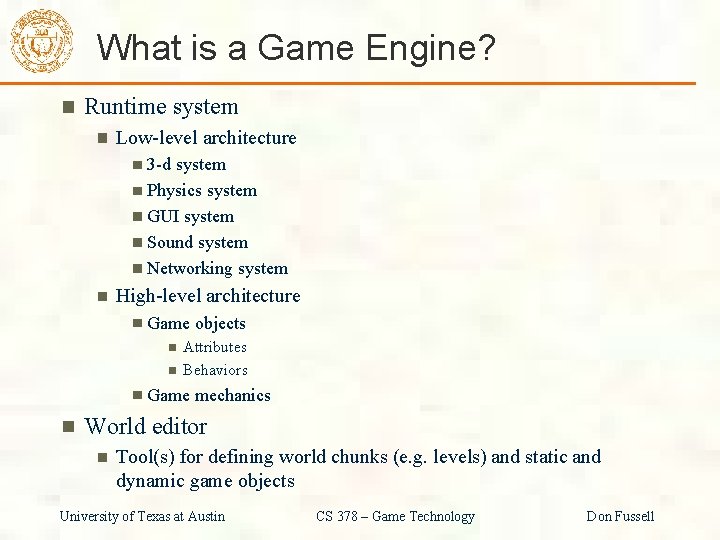
What is a Game Engine? Runtime system Low-level architecture 3 -d system Physics system GUI system Sound system Networking system High-level architecture Game objects Attributes Behaviors Game mechanics World editor Tool(s) for defining world chunks (e. g. levels) and static and dynamic game objects University of Texas at Austin CS 378 – Game Technology Don Fussell
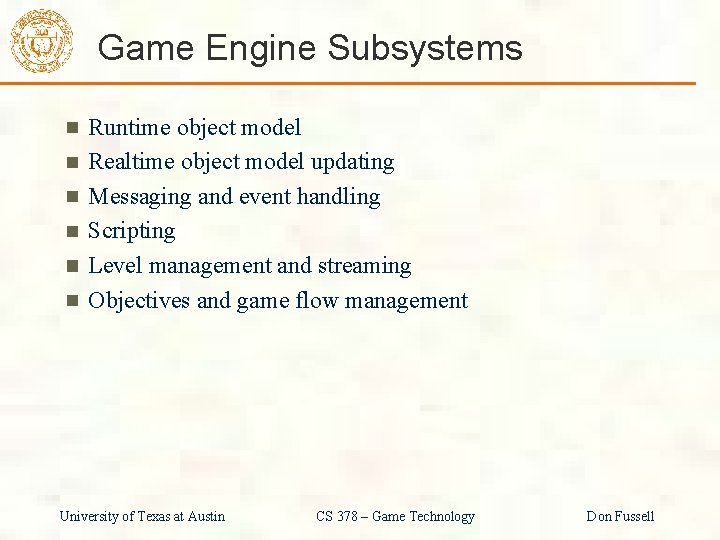
Game Engine Subsystems Runtime object model Realtime object model updating Messaging and event handling Scripting Level management and streaming Objectives and game flow management University of Texas at Austin CS 378 – Game Technology Don Fussell
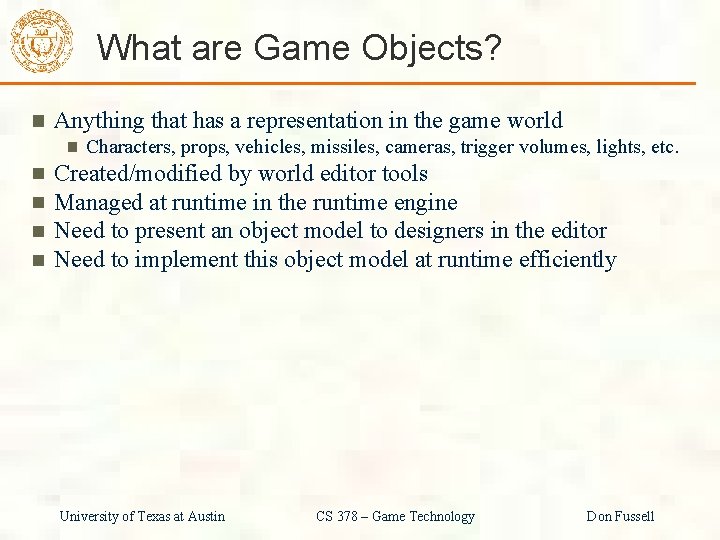
What are Game Objects? Anything that has a representation in the game world Characters, props, vehicles, missiles, cameras, trigger volumes, lights, etc. Created/modified by world editor tools Managed at runtime in the runtime engine Need to present an object model to designers in the editor Need to implement this object model at runtime efficiently University of Texas at Austin CS 378 – Game Technology Don Fussell
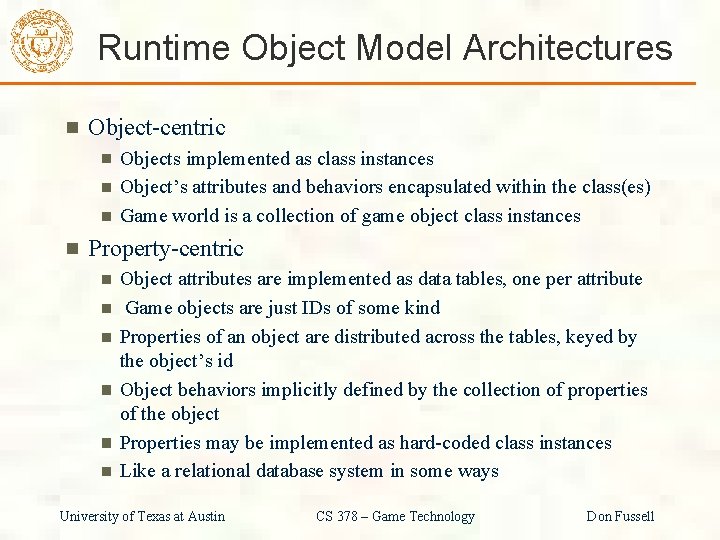
Runtime Object Model Architectures Object-centric Objects implemented as class instances Object’s attributes and behaviors encapsulated within the class(es) Game world is a collection of game object class instances Property-centric Object attributes are implemented as data tables, one per attribute Game objects are just IDs of some kind Properties of an object are distributed across the tables, keyed by the object’s id Object behaviors implicitly defined by the collection of properties of the object Properties may be implemented as hard-coded class instances Like a relational database system in some ways University of Texas at Austin CS 378 – Game Technology Don Fussell
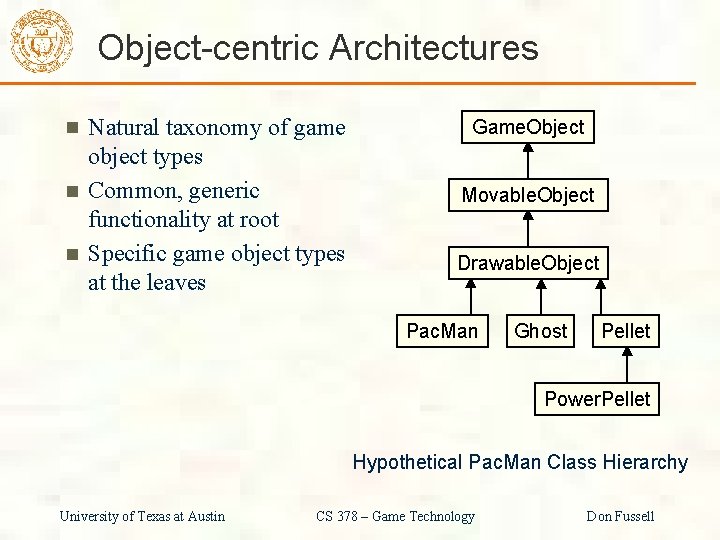
Object-centric Architectures Natural taxonomy of game object types Common, generic functionality at root Specific game object types at the leaves Game. Object Movable. Object Drawable. Object Pac. Man Ghost Pellet Power. Pellet Hypothetical Pac. Man Class Hierarchy University of Texas at Austin CS 378 – Game Technology Don Fussell
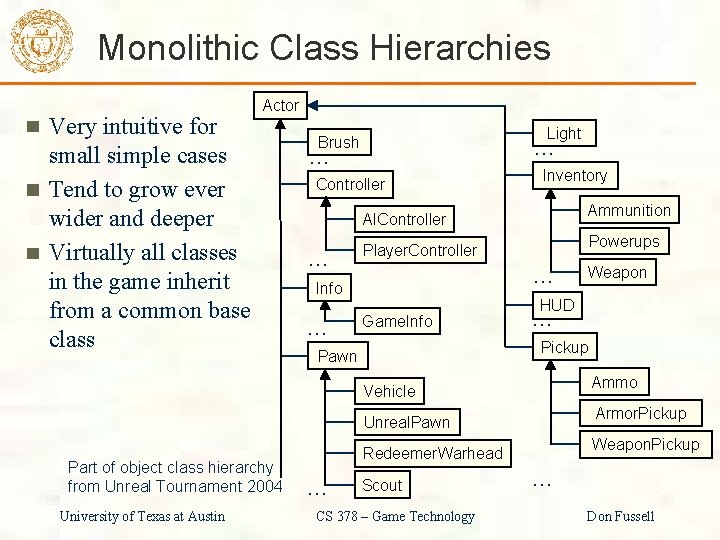
Monolithic Class Hierarchies Very intuitive for small simple cases Tend to grow ever wider and deeper Virtually all classes in the game inherit from a common base class Actor Light … Brush … Controller Inventory Ammunition AIController … … Info … Powerups Player. Controller Game. Info HUD … Pickup Pawn Ammo Vehicle Armor. Pickup Unreal. Pawn Part of object class hierarchy from Unreal Tournament 2004 University of Texas at Austin Weapon. Pickup Redeemer. Warhead … Weapon Scout CS 378 – Game Technology … Don Fussell
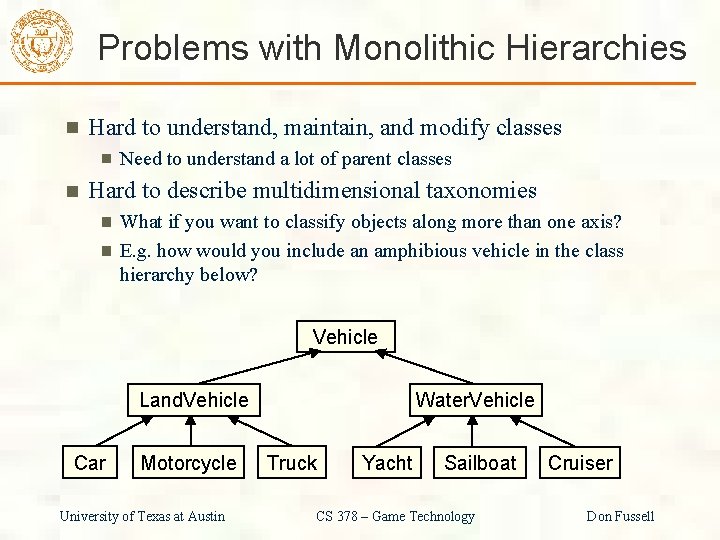
Problems with Monolithic Hierarchies Hard to understand, maintain, and modify classes Need to understand a lot of parent classes Hard to describe multidimensional taxonomies What if you want to classify objects along more than one axis? E. g. how would you include an amphibious vehicle in the class hierarchy below? Vehicle Land. Vehicle Car Motorcycle University of Texas at Austin Water. Vehicle Truck Yacht Sailboat CS 378 – Game Technology Cruiser Don Fussell
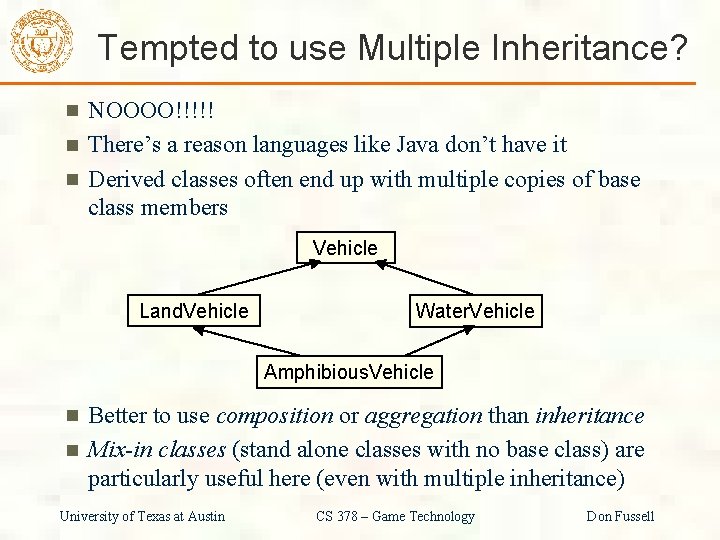
Tempted to use Multiple Inheritance? NOOOO!!!!! There’s a reason languages like Java don’t have it Derived classes often end up with multiple copies of base class members Vehicle Land. Vehicle Water. Vehicle Amphibious. Vehicle Better to use composition or aggregation than inheritance Mix-in classes (stand alone classes with no base class) are particularly useful here (even with multiple inheritance) University of Texas at Austin CS 378 – Game Technology Don Fussell
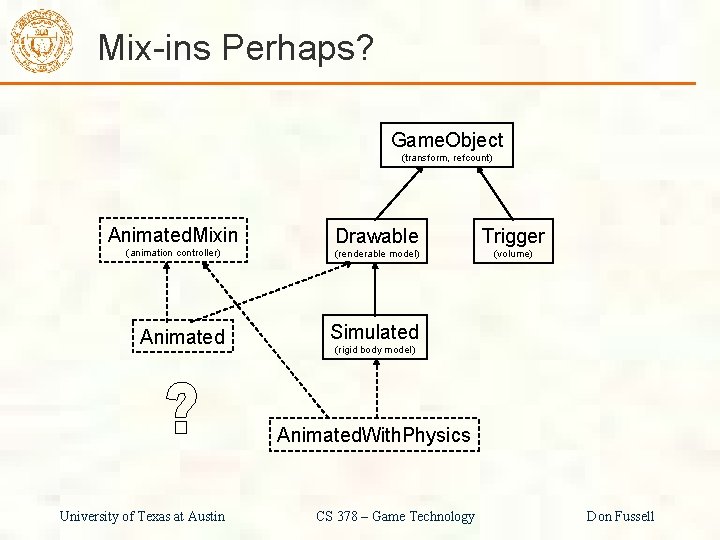
Mix-ins Perhaps? Game. Object (transform, refcount) Animated. Mixin (animation controller) Animated Drawable Trigger (renderable model) (volume) Simulated (rigid body model) Animated. With. Physics University of Texas at Austin CS 378 – Game Technology Don Fussell
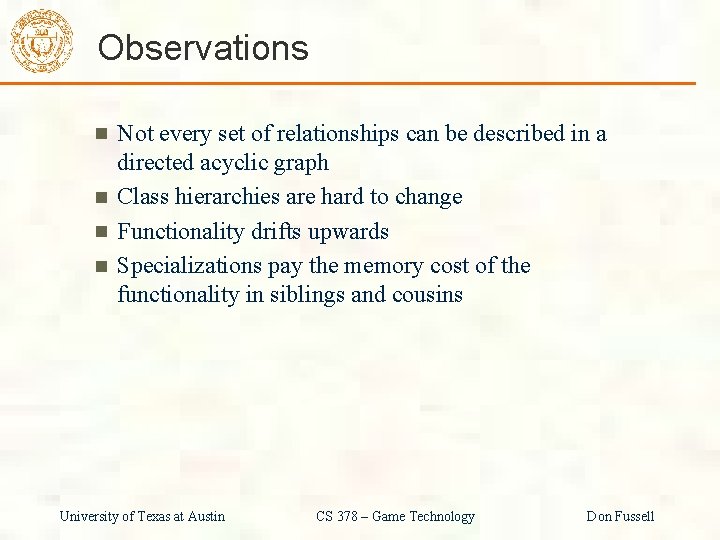
Observations Not every set of relationships can be described in a directed acyclic graph Class hierarchies are hard to change Functionality drifts upwards Specializations pay the memory cost of the functionality in siblings and cousins University of Texas at Austin CS 378 – Game Technology Don Fussell
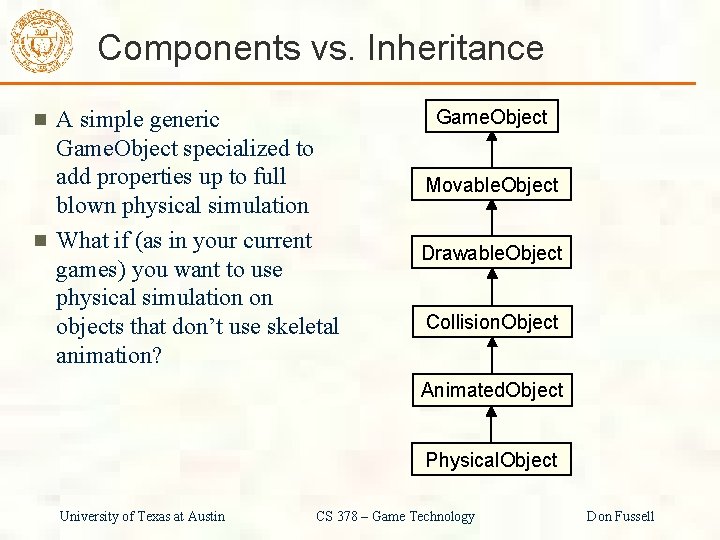
Components vs. Inheritance A simple generic Game. Object specialized to add properties up to full blown physical simulation What if (as in your current games) you want to use physical simulation on objects that don’t use skeletal animation? Game. Object Movable. Object Drawable. Object Collision. Object Animated. Object Physical. Object University of Texas at Austin CS 378 – Game Technology Don Fussell
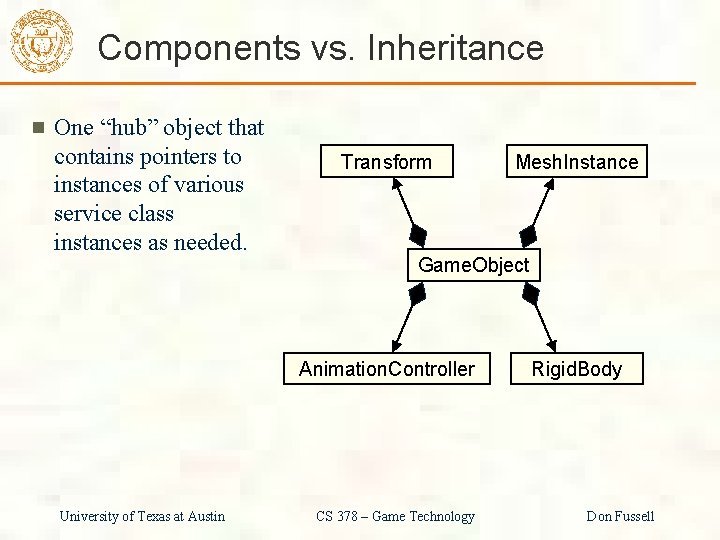
Components vs. Inheritance One “hub” object that contains pointers to instances of various service class instances as needed. Transform Game. Object Animation. Controller University of Texas at Austin Mesh. Instance CS 378 – Game Technology Rigid. Body Don Fussell
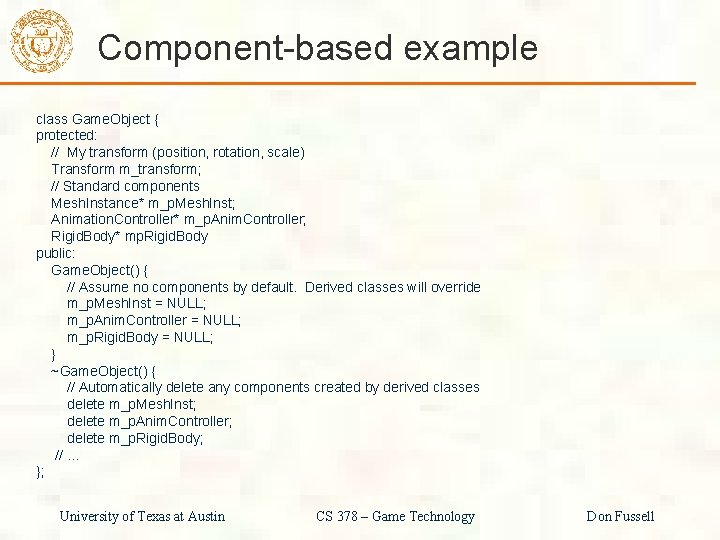
Component-based example class Game. Object { protected: // My transform (position, rotation, scale) Transform m_transform; // Standard components Mesh. Instance* m_p. Mesh. Inst; Animation. Controller* m_p. Anim. Controller; Rigid. Body* mp. Rigid. Body public: Game. Object() { // Assume no components by default. Derived classes will override m_p. Mesh. Inst = NULL; m_p. Anim. Controller = NULL; m_p. Rigid. Body = NULL; } ~Game. Object() { // Automatically delete any components created by derived classes delete m_p. Mesh. Inst; delete m_p. Anim. Controller; delete m_p. Rigid. Body; // … }; University of Texas at Austin CS 378 – Game Technology Don Fussell
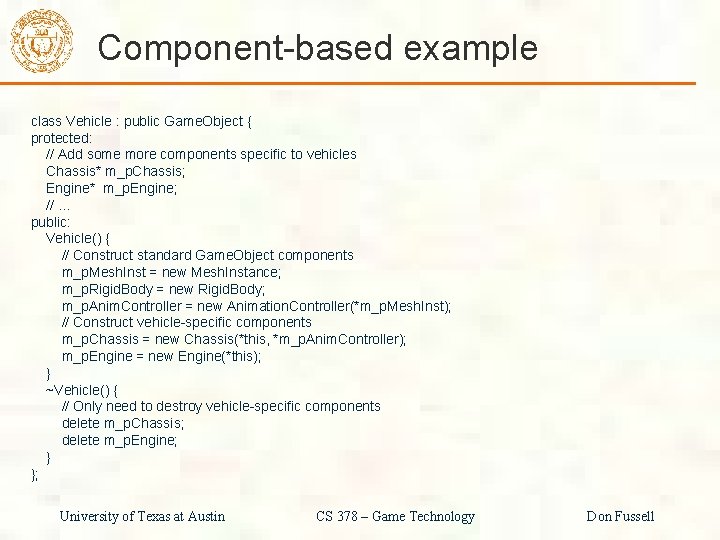
Component-based example class Vehicle : public Game. Object { protected: // Add some more components specific to vehicles Chassis* m_p. Chassis; Engine* m_p. Engine; // … public: Vehicle() { // Construct standard Game. Object components m_p. Mesh. Inst = new Mesh. Instance; m_p. Rigid. Body = new Rigid. Body; m_p. Anim. Controller = new Animation. Controller(*m_p. Mesh. Inst); // Construct vehicle-specific components m_p. Chassis = new Chassis(*this, *m_p. Anim. Controller); m_p. Engine = new Engine(*this); } ~Vehicle() { // Only need to destroy vehicle-specific components delete m_p. Chassis; delete m_p. Engine; } }; University of Texas at Austin CS 378 – Game Technology Don Fussell
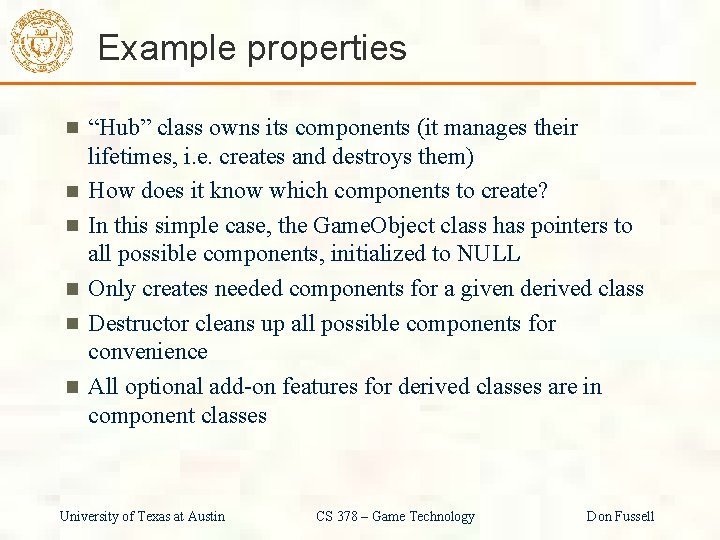
Example properties “Hub” class owns its components (it manages their lifetimes, i. e. creates and destroys them) How does it know which components to create? In this simple case, the Game. Object class has pointers to all possible components, initialized to NULL Only creates needed components for a given derived class Destructor cleans up all possible components for convenience All optional add-on features for derived classes are in component classes University of Texas at Austin CS 378 – Game Technology Don Fussell
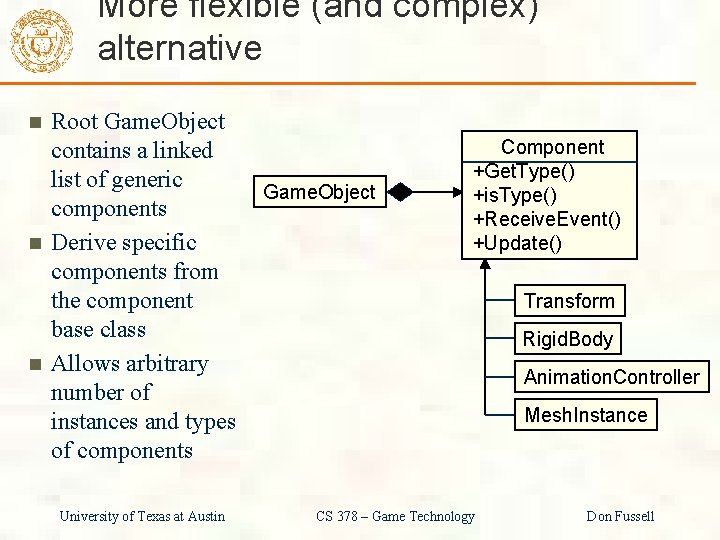
More flexible (and complex) alternative Root Game. Object contains a linked list of generic components Derive specific components from the component base class Allows arbitrary number of instances and types of components University of Texas at Austin Game. Object Component +Get. Type() +is. Type() +Receive. Event() +Update() Transform Rigid. Body Animation. Controller Mesh. Instance CS 378 – Game Technology Don Fussell
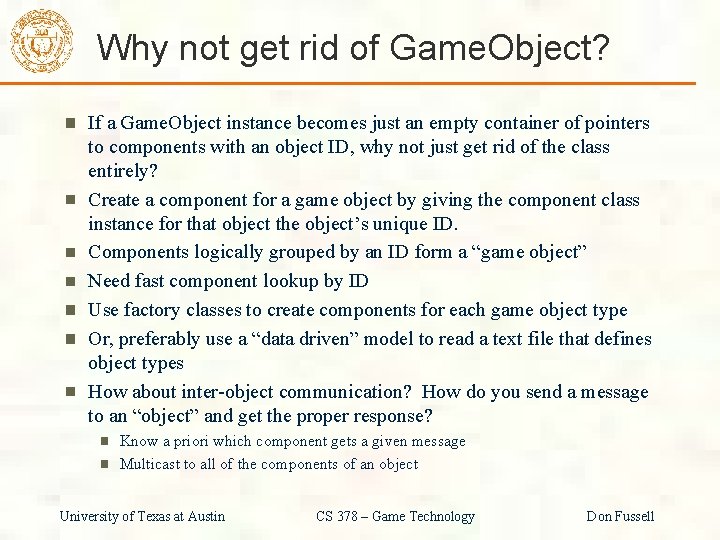
Why not get rid of Game. Object? If a Game. Object instance becomes just an empty container of pointers to components with an object ID, why not just get rid of the class entirely? Create a component for a game object by giving the component class instance for that object the object’s unique ID. Components logically grouped by an ID form a “game object” Need fast component lookup by ID Use factory classes to create components for each game object type Or, preferably use a “data driven” model to read a text file that defines object types How about inter-object communication? How do you send a message to an “object” and get the proper response? Know a priori which component gets a given message Multicast to all of the components of an object University of Texas at Austin CS 378 – Game Technology Don Fussell
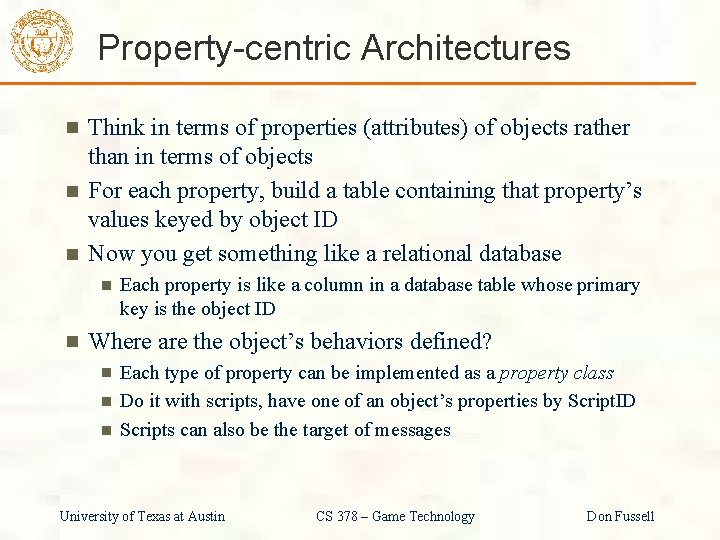
Property-centric Architectures Think in terms of properties (attributes) of objects rather than in terms of objects For each property, build a table containing that property’s values keyed by object ID Now you get something like a relational database Each property is like a column in a database table whose primary key is the object ID Where are the object’s behaviors defined? Each type of property can be implemented as a property class Do it with scripts, have one of an object’s properties by Script. ID Scripts can also be the target of messages University of Texas at Austin CS 378 – Game Technology Don Fussell
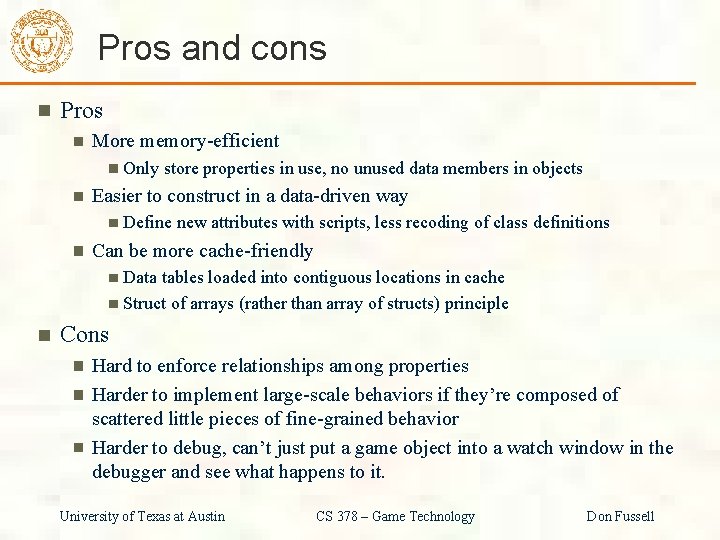
Pros and cons Pros More memory-efficient Only store properties in use, no unused data members in objects Easier to construct in a data-driven way Define new attributes with scripts, less recoding of class definitions Can be more cache-friendly Data tables loaded into contiguous locations in cache Struct of arrays (rather than array of structs) principle Cons Hard to enforce relationships among properties Harder to implement large-scale behaviors if they’re composed of scattered little pieces of fine-grained behavior Harder to debug, can’t just put a game object into a watch window in the debugger and see what happens to it. University of Texas at Austin CS 378 – Game Technology Don Fussell
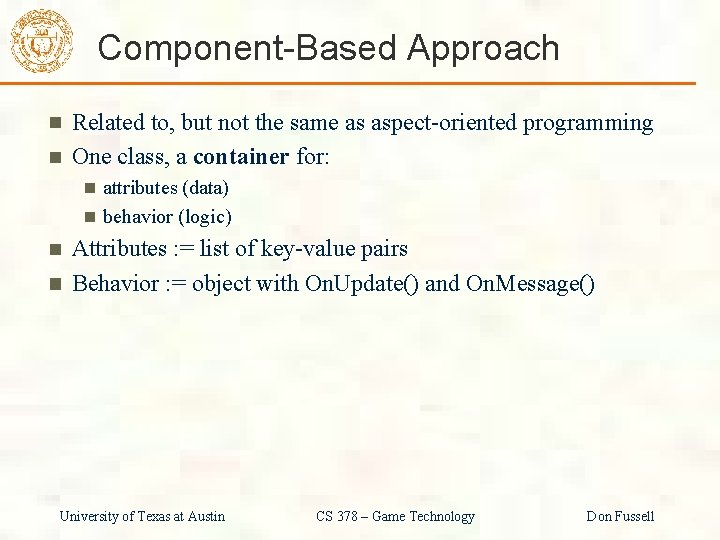
Component-Based Approach Related to, but not the same as aspect-oriented programming One class, a container for: attributes (data) behavior (logic) Attributes : = list of key-value pairs Behavior : = object with On. Update() and On. Message() University of Texas at Austin CS 378 – Game Technology Don Fussell
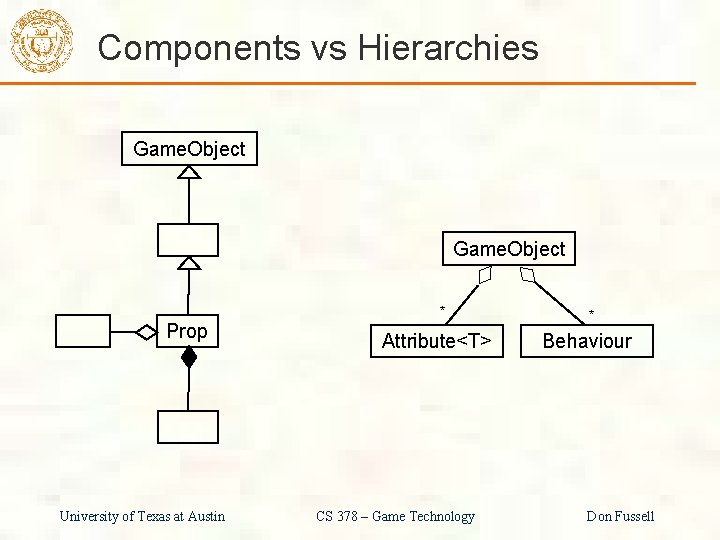
Components vs Hierarchies Game. Object * Prop University of Texas at Austin Attribute<T> CS 378 – Game Technology * Behaviour Don Fussell
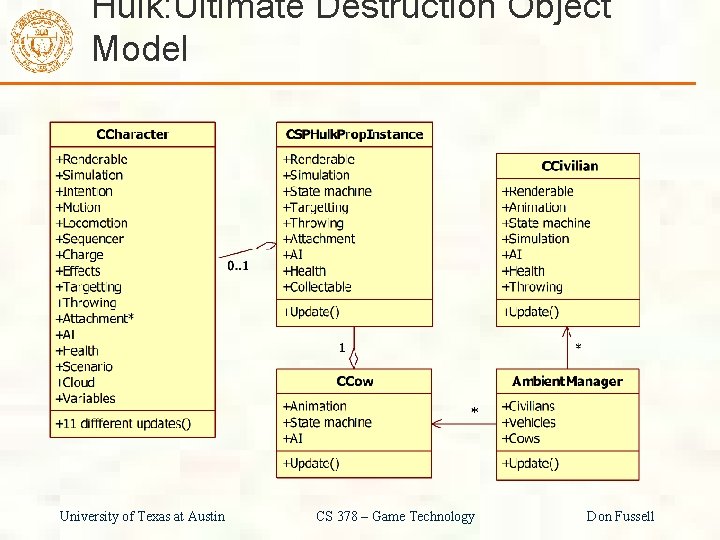
Hulk: Ultimate Destruction Object Model University of Texas at Austin CS 378 – Game Technology Don Fussell
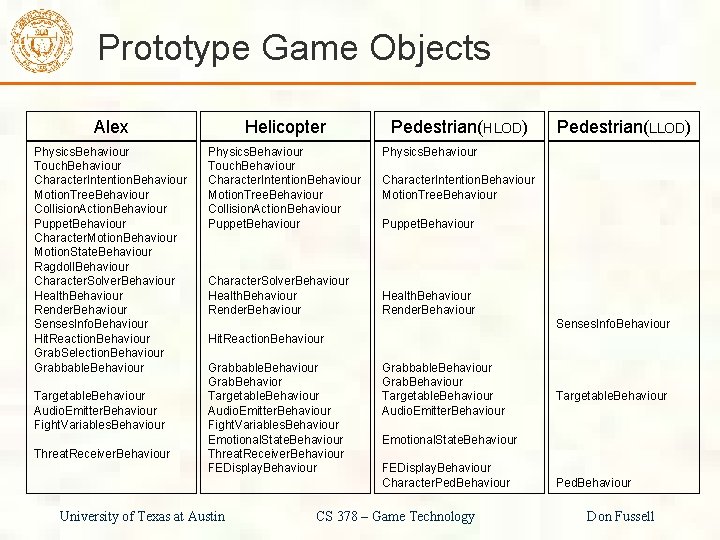
Prototype Game Objects Alex Helicopter Physics. Behaviour Touch. Behaviour Character. Intention. Behaviour Motion. Tree. Behaviour Collision. Action. Behaviour Puppet. Behaviour Character. Motion. Behaviour Motion. State. Behaviour Ragdoll. Behaviour Character. Solver. Behaviour Health. Behaviour Render. Behaviour Senses. Info. Behaviour Hit. Reaction. Behaviour Grab. Selection. Behaviour Grabbable. Behaviour Physics. Behaviour Touch. Behaviour Character. Intention. Behaviour Motion. Tree. Behaviour Collision. Action. Behaviour Puppet. Behaviour Targetable. Behaviour Audio. Emitter. Behaviour Fight. Variables. Behaviour Threat. Receiver. Behaviour Character. Solver. Behaviour Health. Behaviour Render. Behaviour Pedestrian(HLOD) Pedestrian(LLOD) Physics. Behaviour Character. Intention. Behaviour Motion. Tree. Behaviour Puppet. Behaviour Health. Behaviour Render. Behaviour Senses. Info. Behaviour Hit. Reaction. Behaviour Grabbable. Behaviour Grab. Behavior Targetable. Behaviour Audio. Emitter. Behaviour Fight. Variables. Behaviour Emotional. State. Behaviour Threat. Receiver. Behaviour FEDisplay. Behaviour University of Texas at Austin Grabbable. Behaviour Grab. Behaviour Targetable. Behaviour Audio. Emitter. Behaviour Targetable. Behaviour Emotional. State. Behaviour FEDisplay. Behaviour Character. Ped. Behaviour CS 378 – Game Technology Ped. Behaviour Don Fussell
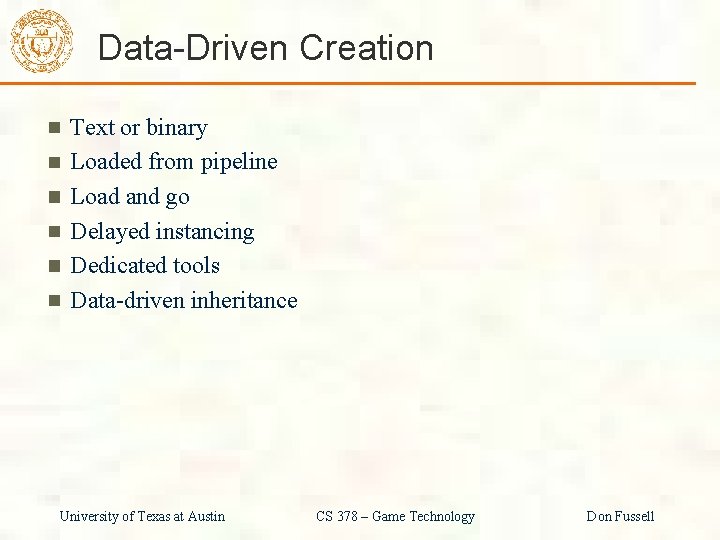
Data-Driven Creation Text or binary Loaded from pipeline Load and go Delayed instancing Dedicated tools Data-driven inheritance University of Texas at Austin CS 378 – Game Technology Don Fussell
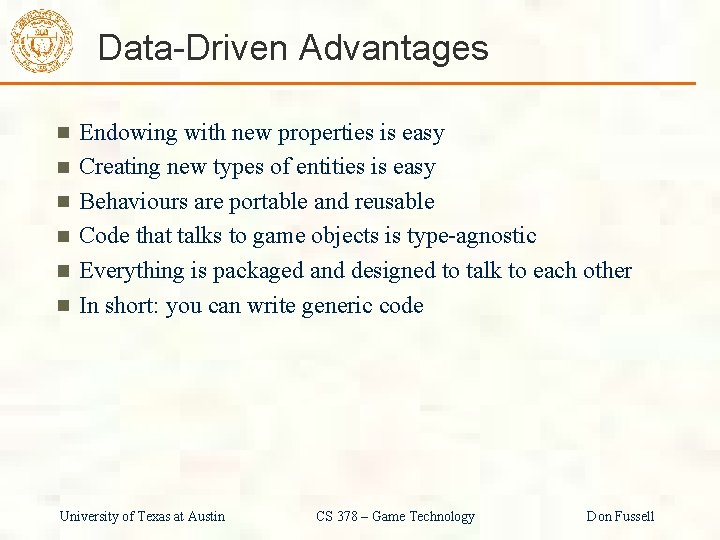
Data-Driven Advantages Endowing with new properties is easy Creating new types of entities is easy Behaviours are portable and reusable Code that talks to game objects is type-agnostic Everything is packaged and designed to talk to each other In short: you can write generic code University of Texas at Austin CS 378 – Game Technology Don Fussell
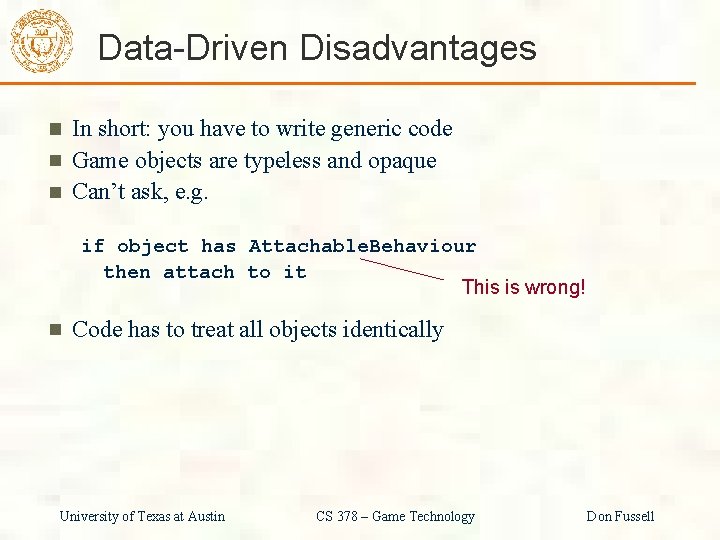
Data-Driven Disadvantages In short: you have to write generic code Game objects are typeless and opaque Can’t ask, e. g. if object has Attachable. Behaviour then attach to it This is wrong! Code has to treat all objects identically University of Texas at Austin CS 378 – Game Technology Don Fussell
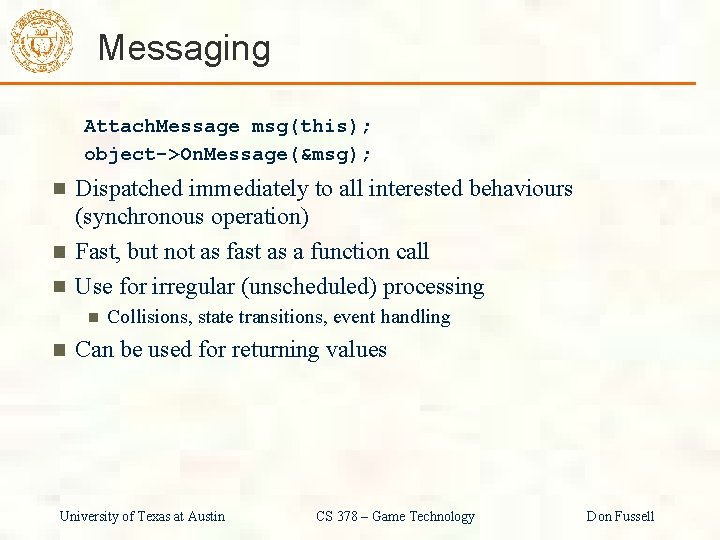
Messaging Attach. Message msg(this); object->On. Message(&msg); Dispatched immediately to all interested behaviours (synchronous operation) Fast, but not as fast as a function call Use for irregular (unscheduled) processing Collisions, state transitions, event handling Can be used for returning values University of Texas at Austin CS 378 – Game Technology Don Fussell
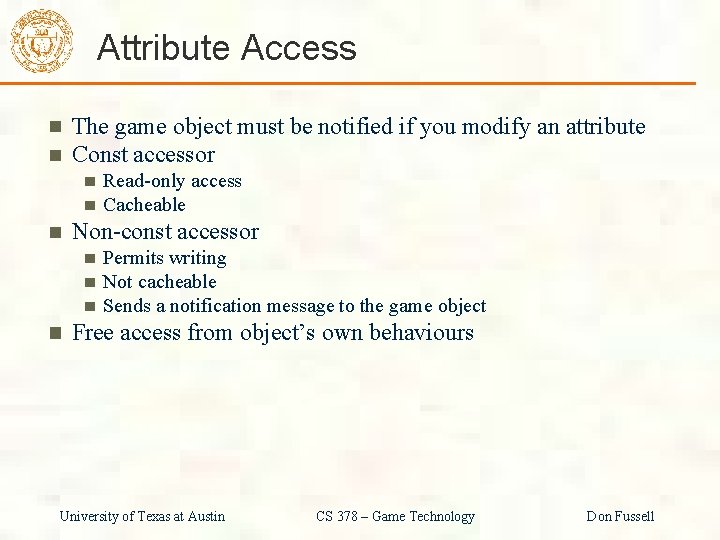
Attribute Access The game object must be notified if you modify an attribute Const accessor Read-only access Cacheable Non-const accessor Permits writing Not cacheable Sends a notification message to the game object Free access from object’s own behaviours University of Texas at Austin CS 378 – Game Technology Don Fussell
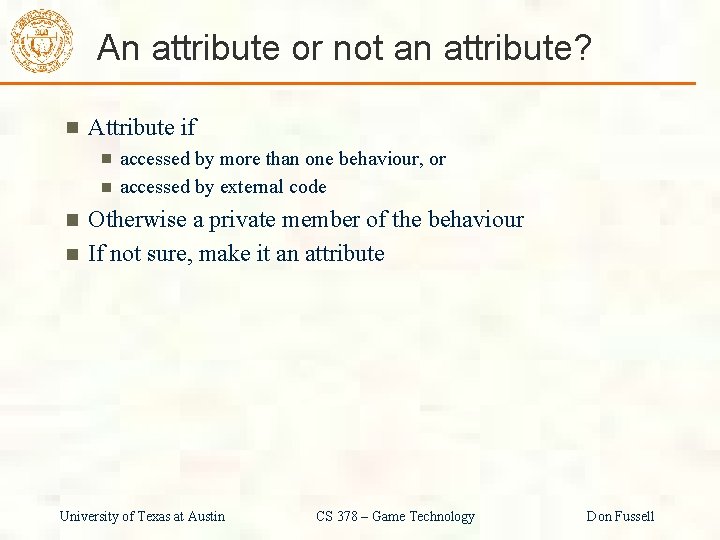
An attribute or not an attribute? Attribute if accessed by more than one behaviour, or accessed by external code Otherwise a private member of the behaviour If not sure, make it an attribute University of Texas at Austin CS 378 – Game Technology Don Fussell
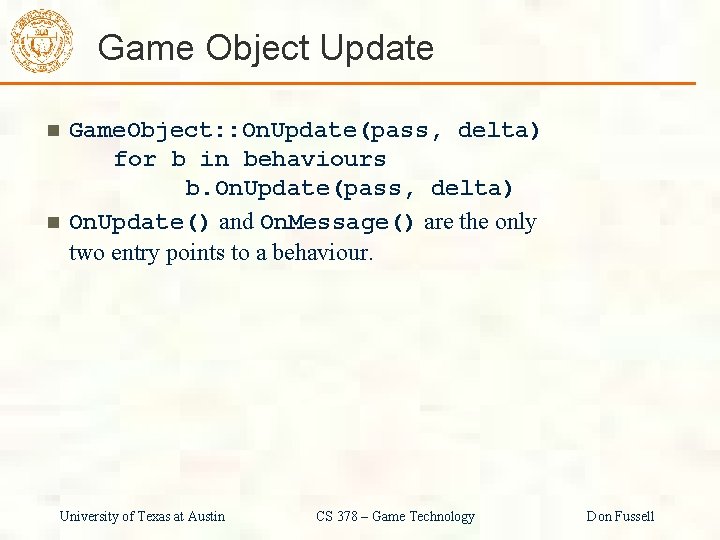
Game Object Update Game. Object: : On. Update(pass, delta) for b in behaviours b. On. Update(pass, delta) On. Update() and On. Message() are the only two entry points to a behaviour. University of Texas at Austin CS 378 – Game Technology Don Fussell
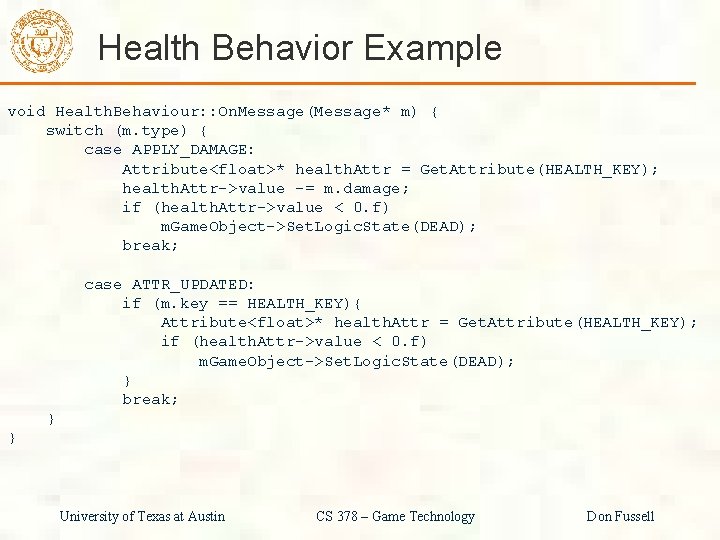
Health Behavior Example void Health. Behaviour: : On. Message(Message* m) { switch (m. type) { case APPLY_DAMAGE: Attribute<float>* health. Attr = Get. Attribute(HEALTH_KEY); health. Attr->value -= m. damage; if (health. Attr->value < 0. f) m. Game. Object->Set. Logic. State(DEAD); break; case ATTR_UPDATED: if (m. key == HEALTH_KEY){ Attribute<float>* health. Attr = Get. Attribute(HEALTH_KEY); if (health. Attr->value < 0. f) m. Game. Object->Set. Logic. State(DEAD); } break; } } University of Texas at Austin CS 378 – Game Technology Don Fussell
![Components in Practice Behaviours and Attributes in PROTOTYPE University of Texas at Austin CS Components in Practice Behaviours and Attributes in [PROTOTYPE] University of Texas at Austin CS](https://slidetodoc.com/presentation_image_h2/76aec75b6a5ee83b584d1512af757216/image-33.jpg)
Components in Practice Behaviours and Attributes in [PROTOTYPE] University of Texas at Austin CS 378 – Game Technology Don Fussell
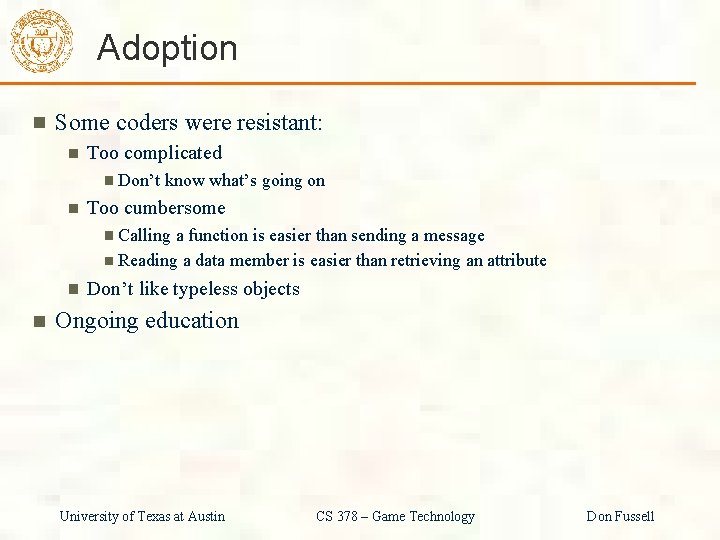
Adoption Some coders were resistant: Too complicated Don’t know what’s going on Too cumbersome Calling a function is easier than sending a message Reading a data member is easier than retrieving an attribute Don’t like typeless objects Ongoing education University of Texas at Austin CS 378 – Game Technology Don Fussell
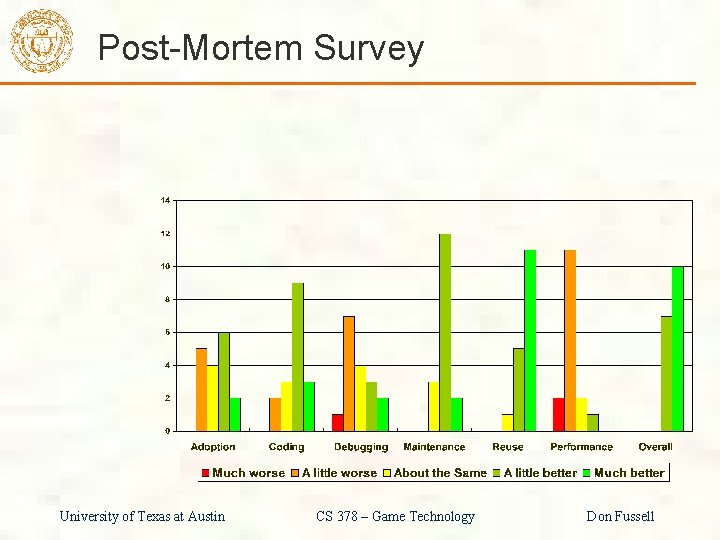
Post-Mortem Survey University of Texas at Austin CS 378 – Game Technology Don Fussell
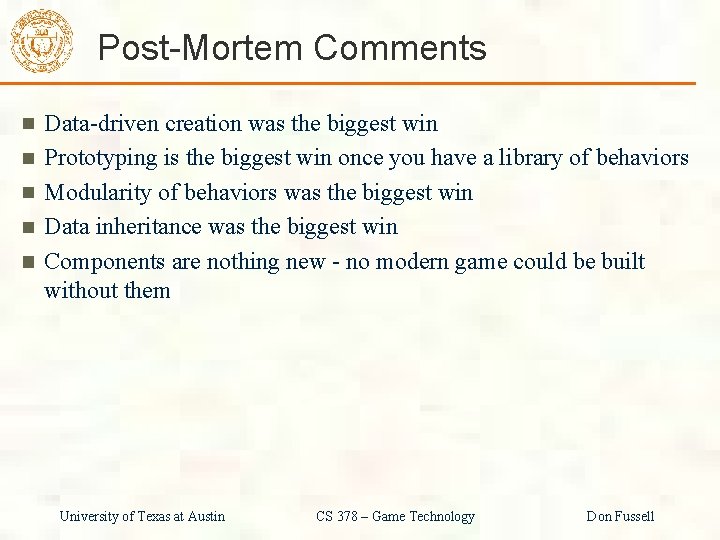
Post-Mortem Comments Data-driven creation was the biggest win Prototyping is the biggest win once you have a library of behaviors Modularity of behaviors was the biggest win Data inheritance was the biggest win Components are nothing new - no modern game could be built without them University of Texas at Austin CS 378 – Game Technology Don Fussell
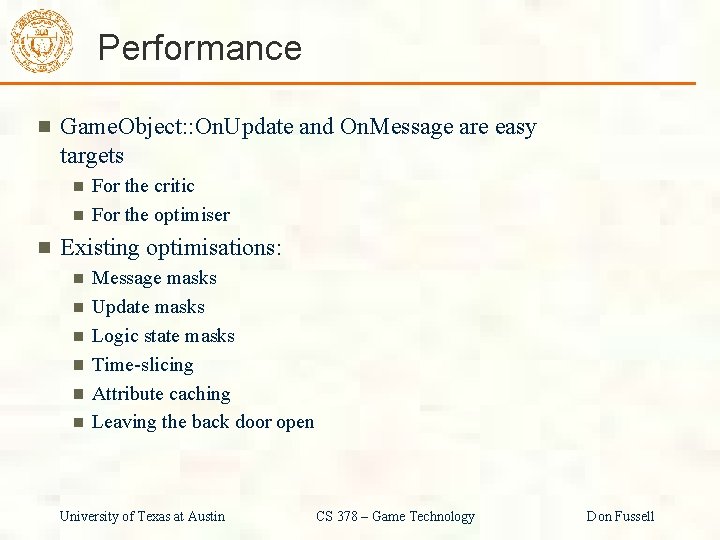
Performance Game. Object: : On. Update and On. Message are easy targets For the critic For the optimiser Existing optimisations: Message masks Update masks Logic state masks Time-slicing Attribute caching Leaving the back door open University of Texas at Austin CS 378 – Game Technology Don Fussell
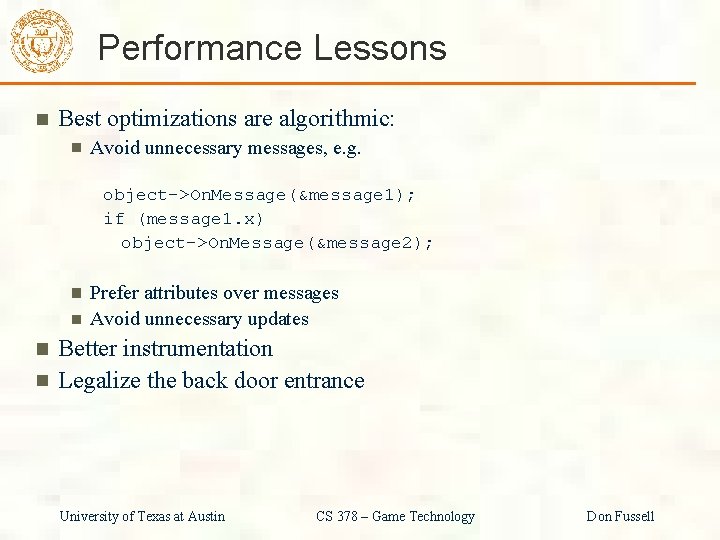
Performance Lessons Best optimizations are algorithmic: Avoid unnecessary messages, e. g. object->On. Message(&message 1); if (message 1. x) object->On. Message(&message 2); Prefer attributes over messages Avoid unnecessary updates Better instrumentation Legalize the back door entrance University of Texas at Austin CS 378 – Game Technology Don Fussell
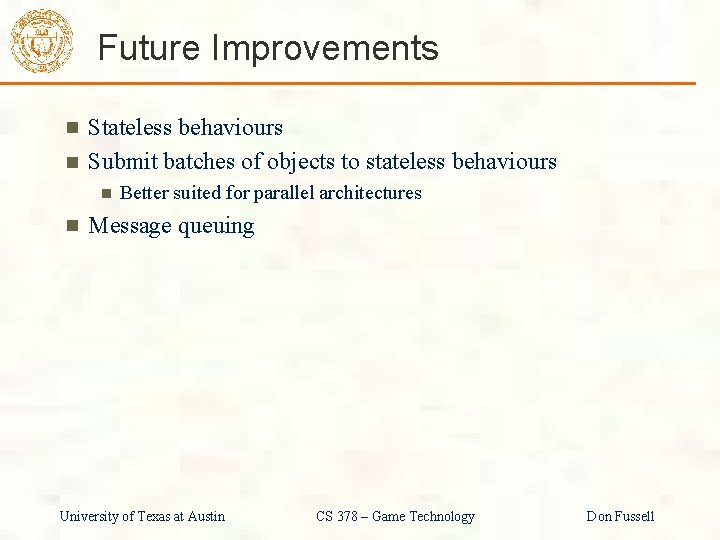
Future Improvements Stateless behaviours Submit batches of objects to stateless behaviours Better suited for parallel architectures Message queuing University of Texas at Austin CS 378 – Game Technology Don Fussell
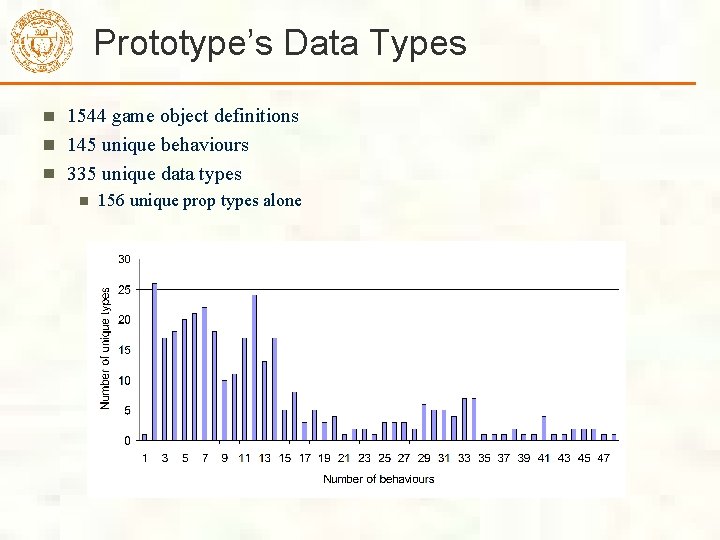
Prototype’s Data Types 1544 game object definitions 145 unique behaviours 335 unique data types 156 unique prop types alone
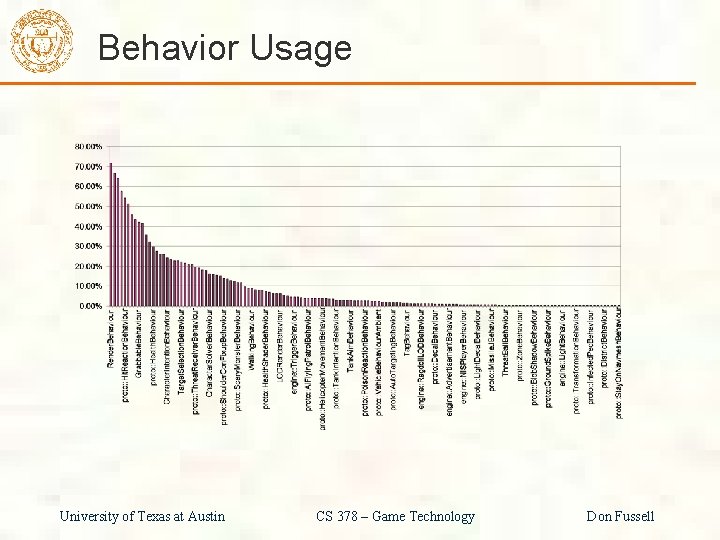
Behavior Usage University of Texas at Austin CS 378 – Game Technology Don Fussell
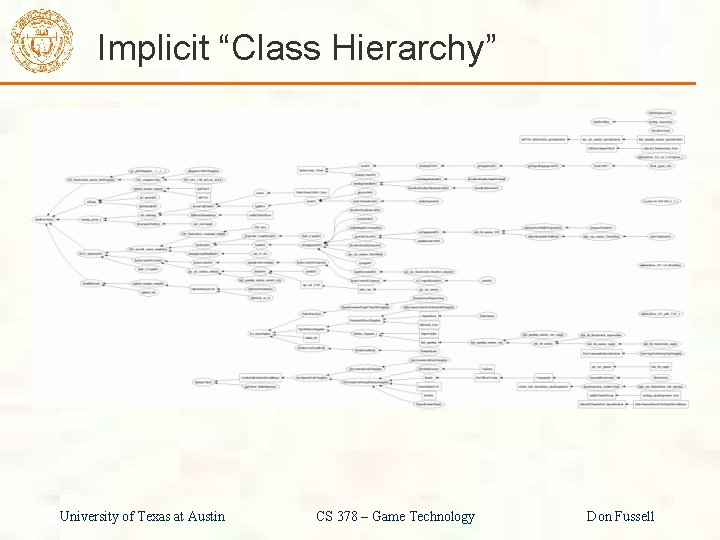
Implicit “Class Hierarchy” University of Texas at Austin CS 378 – Game Technology Don Fussell
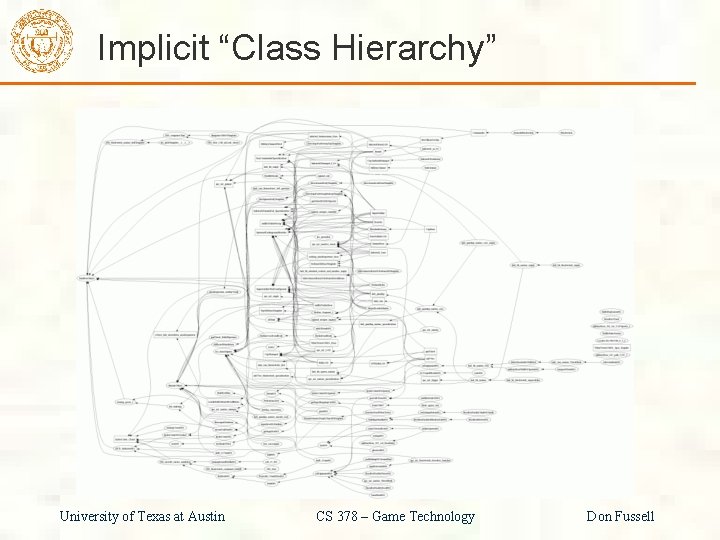
Implicit “Class Hierarchy” University of Texas at Austin CS 378 – Game Technology Don Fussell
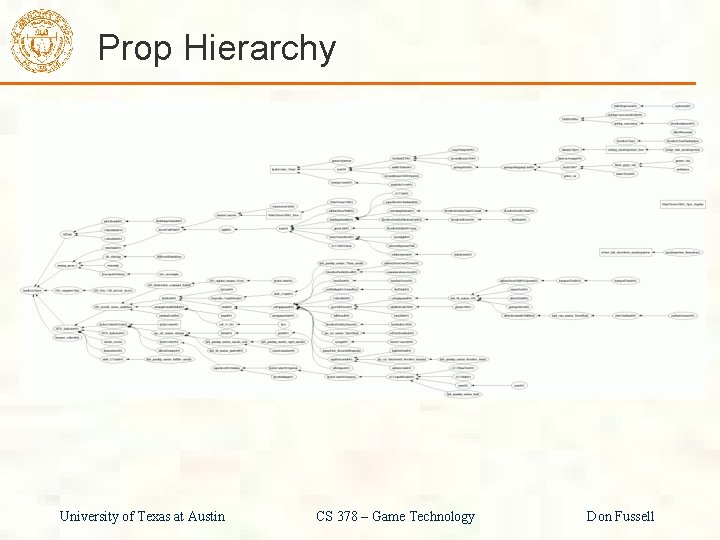
Prop Hierarchy University of Texas at Austin CS 378 – Game Technology Don Fussell
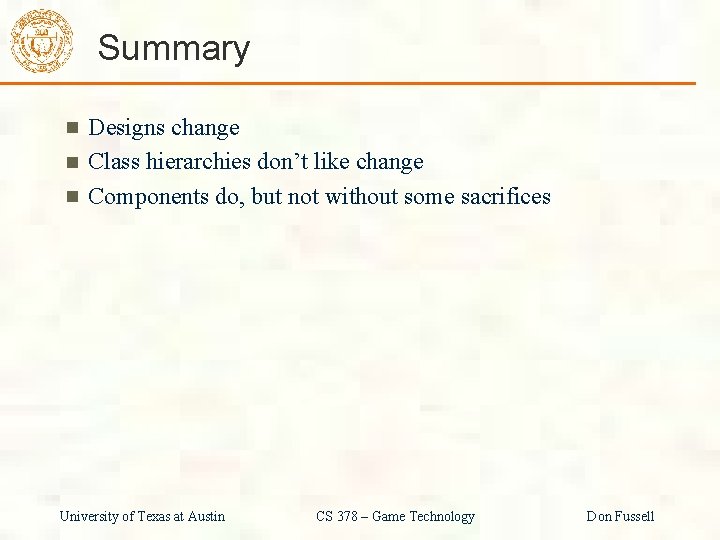
Summary Designs change Class hierarchies don’t like change Components do, but not without some sacrifices University of Texas at Austin CS 378 – Game Technology Don Fussell
Seksyen 4 ajk 1997
Opwekking 497 tekst
Prism vs pyramid
Article 378 of the uae penal code
Game engine architecture
Game engine architecture
Emerging memory technologies
Internal combustion engine vs external combustion engine
Three bus architecture
Computer architecture and computer organization difference
Flow chart for interrupt cycle
Slatten racing engines
Search engine architecture
Matching engine architecture
Architecture search engines
Architecture search engines
Promotion engine architecture
Cell broadband engine architecture
Huazheng wang
Sage game engine
Ogre game engine tutorial
Game engine for rts
Acid game engine
Gii game engine
Unreal engine game loop
Core games engine
Gamebryo cost
Torque script
Flame game engine
Gifi technology
E commerce architecture and technologies in web technology
Abc in software architecture
Call and return architecture in software architecture
Modular vs integral product architecture example
Product architecture
Ender's game desk
Muhammad computer technology
Introduction to computer
Classify computer networks based on transmission technology
Chapter 12 computer technology in health care
Computer abstractions and technology
Types of computer technology
Computer technology review
Computer abstractions and technology
5 pen pc technology
A gift of fire 4th edition