CMPE 150 Introduction to Computing If and Switch
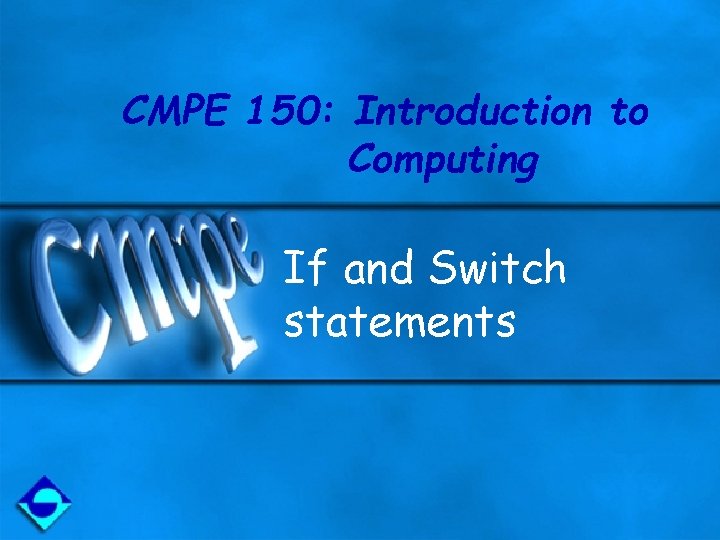
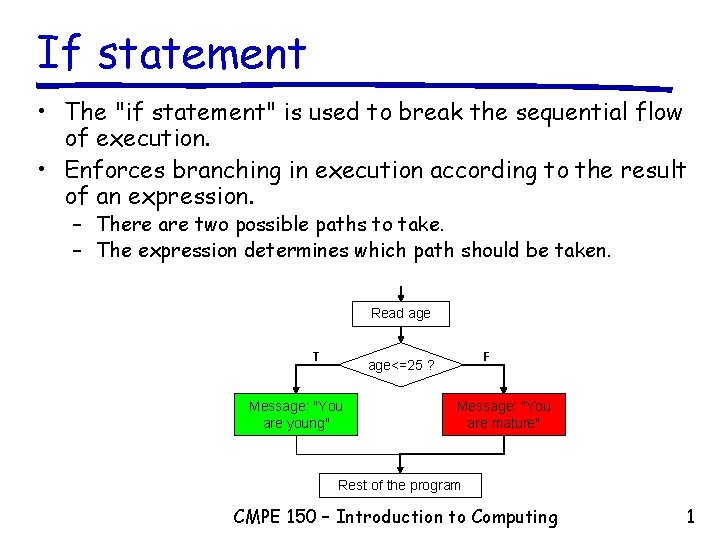
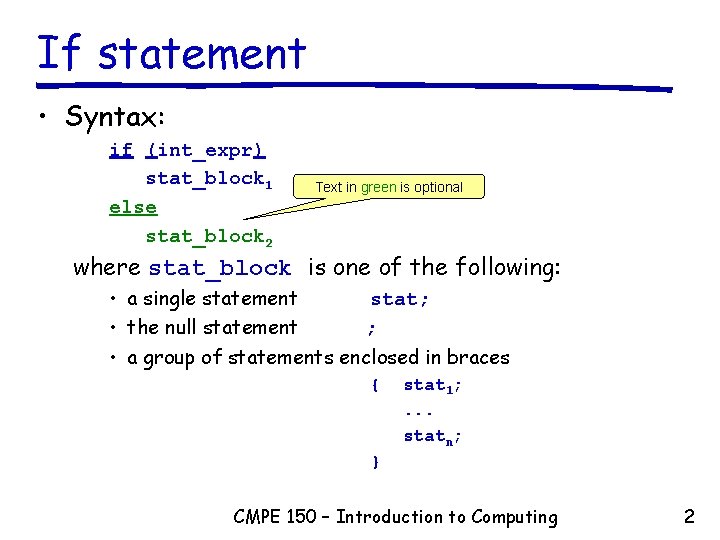
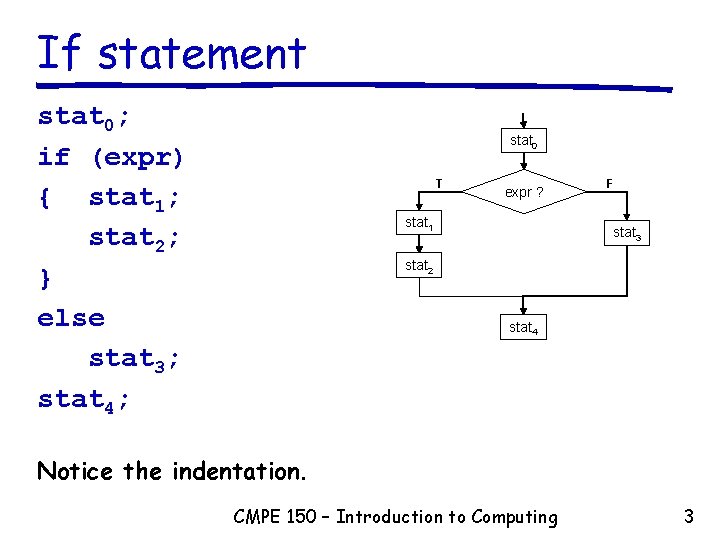
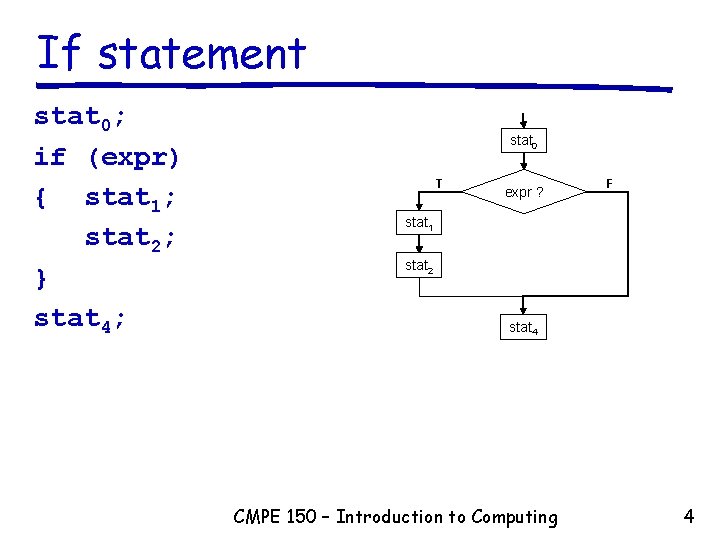
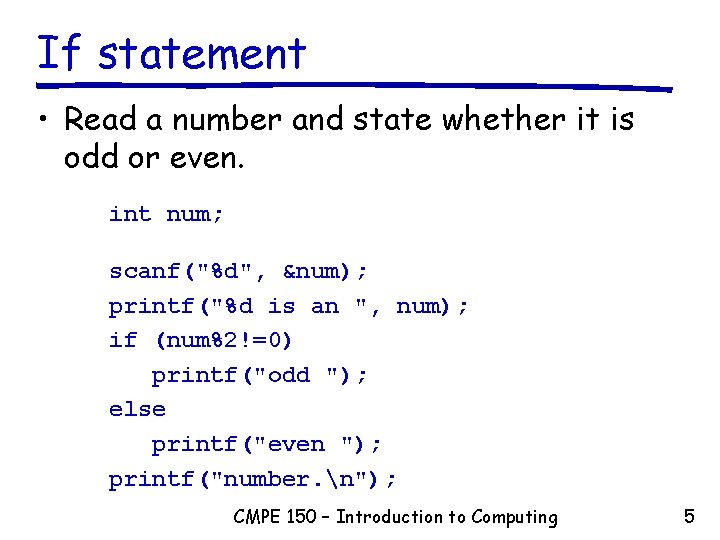
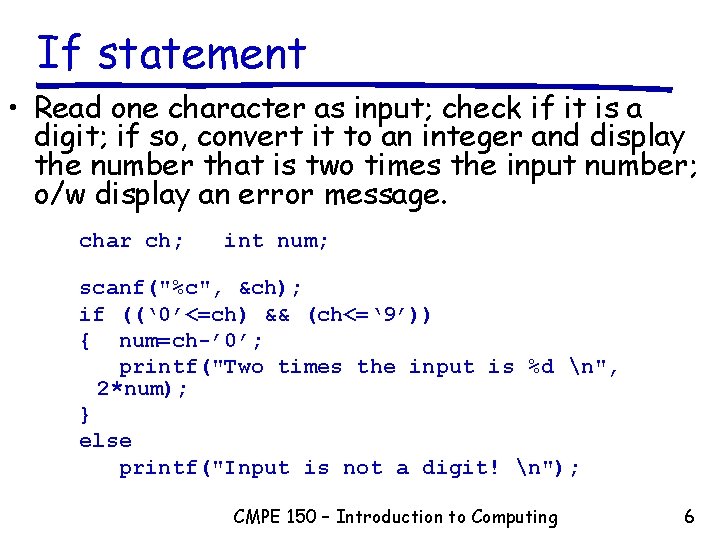
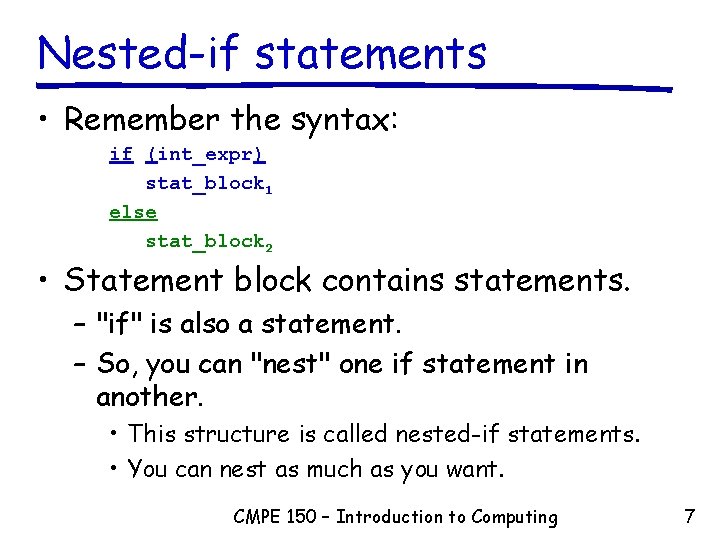
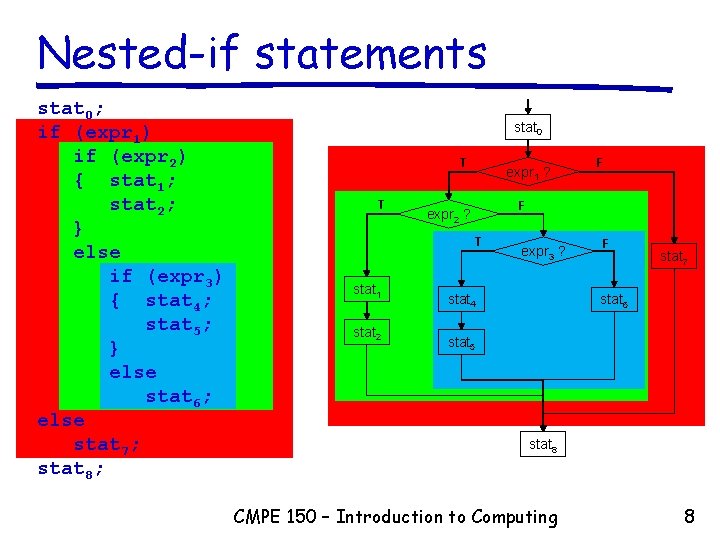
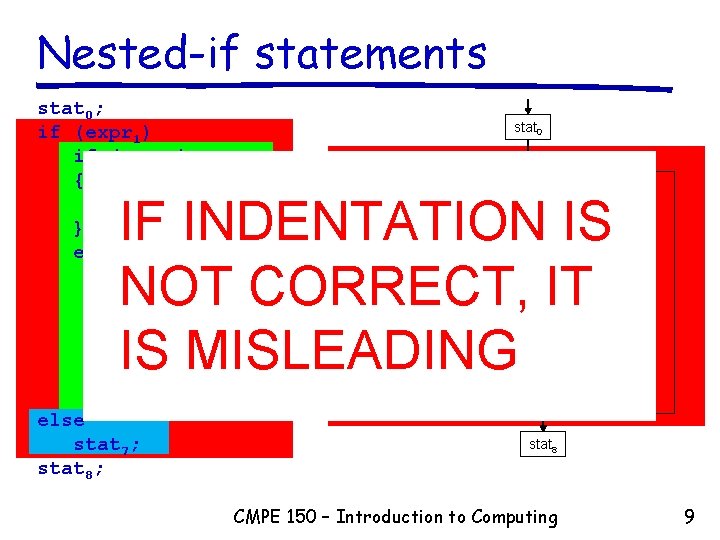
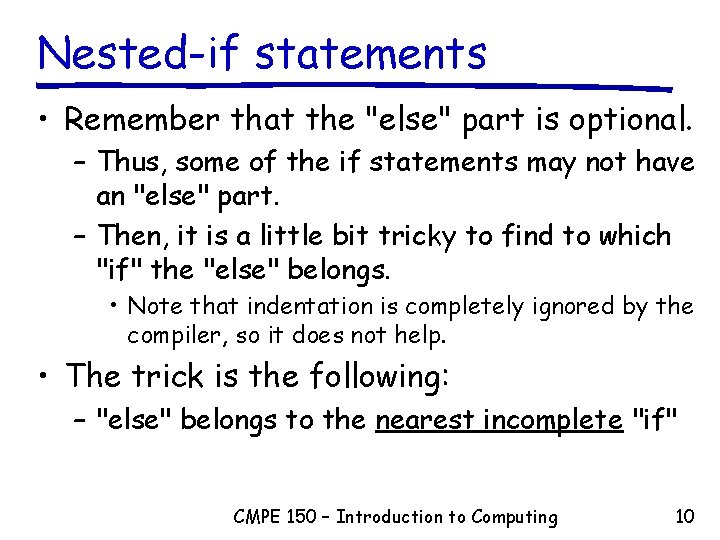
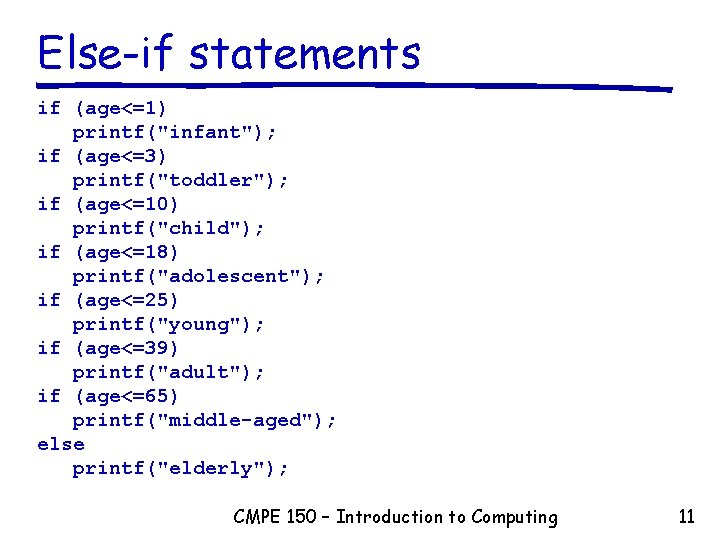
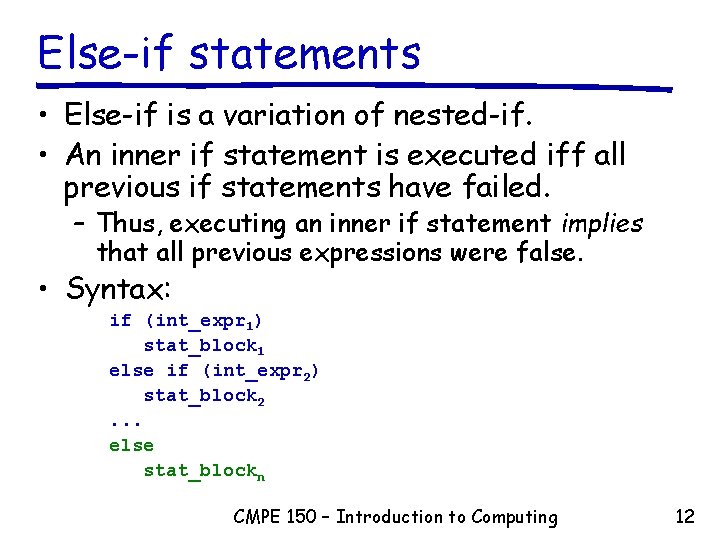
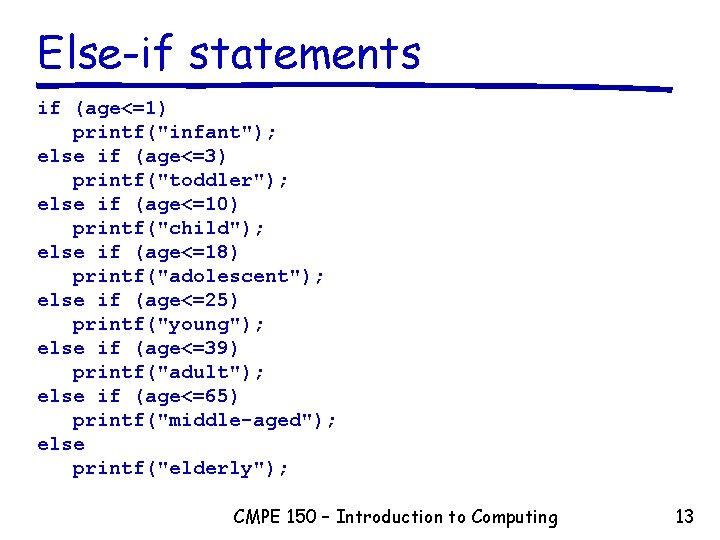
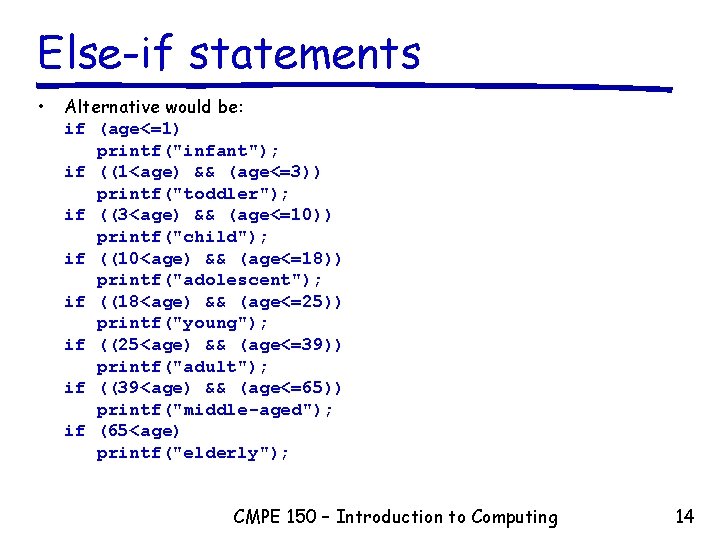
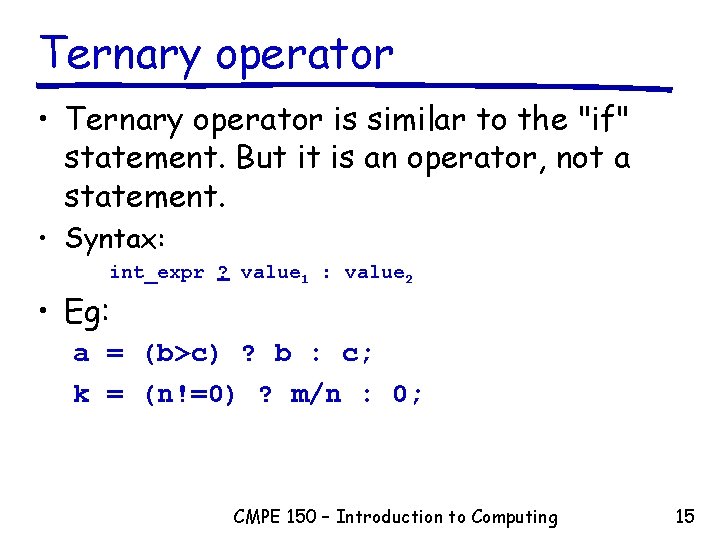
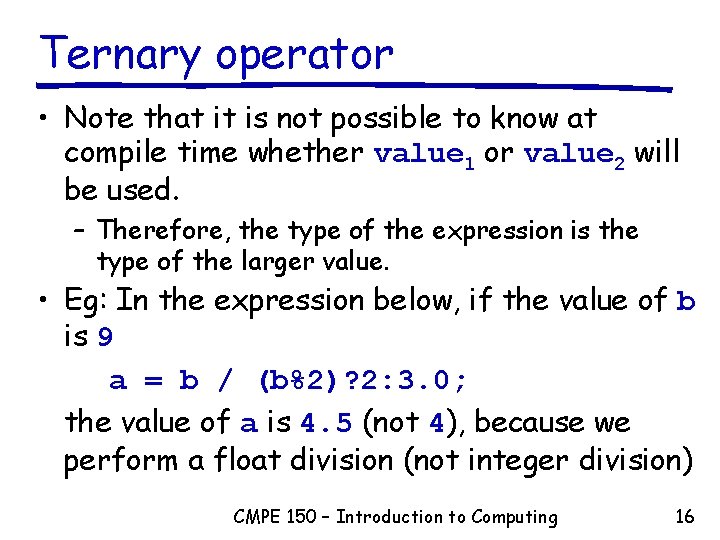
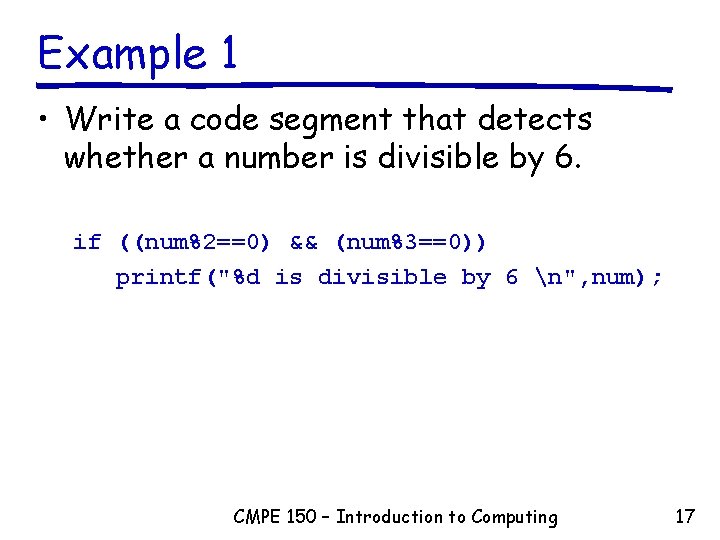
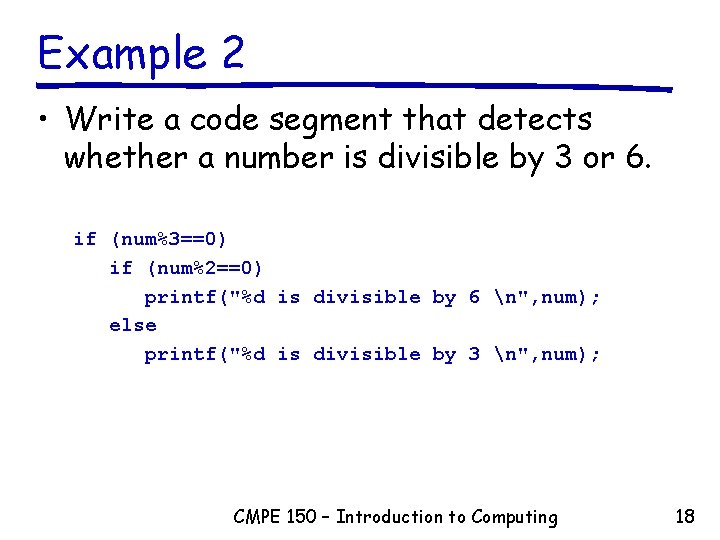
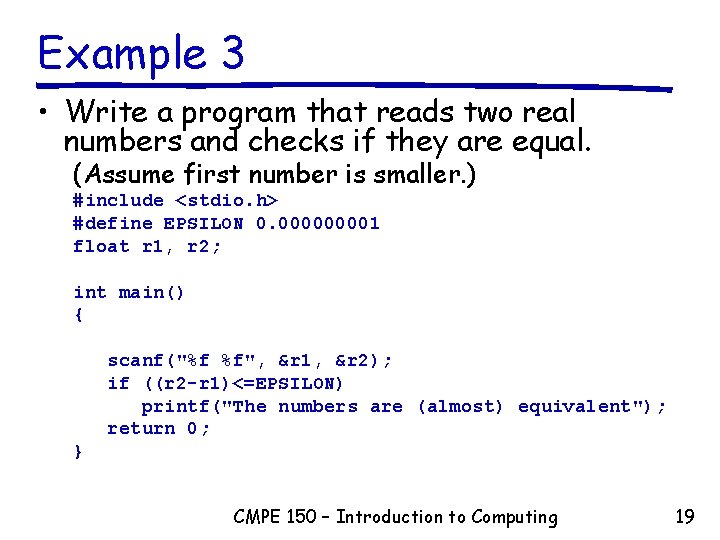
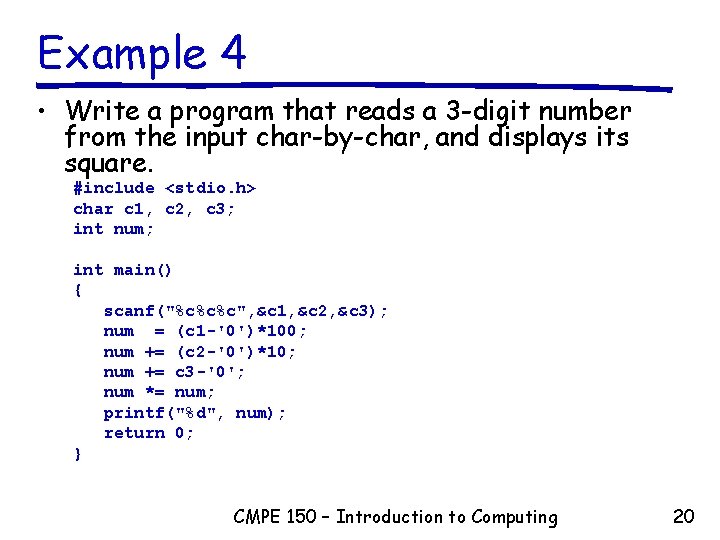
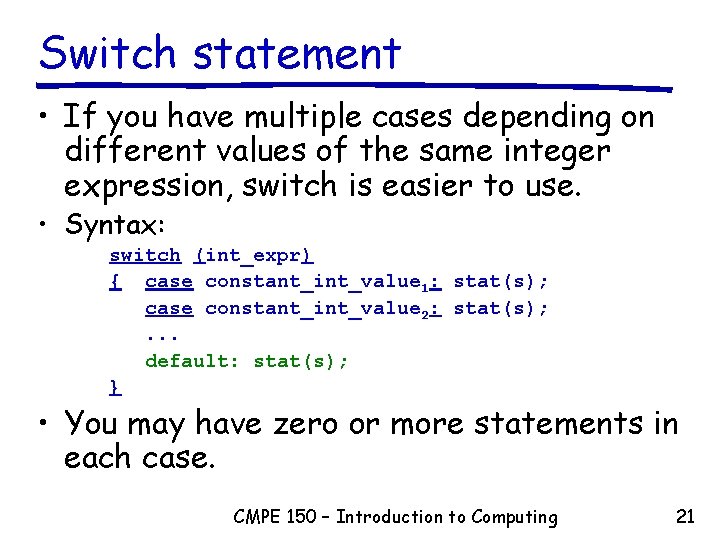
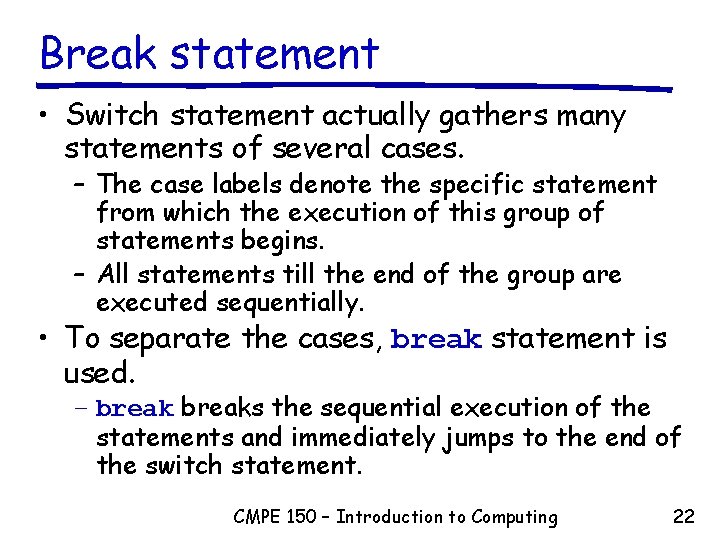
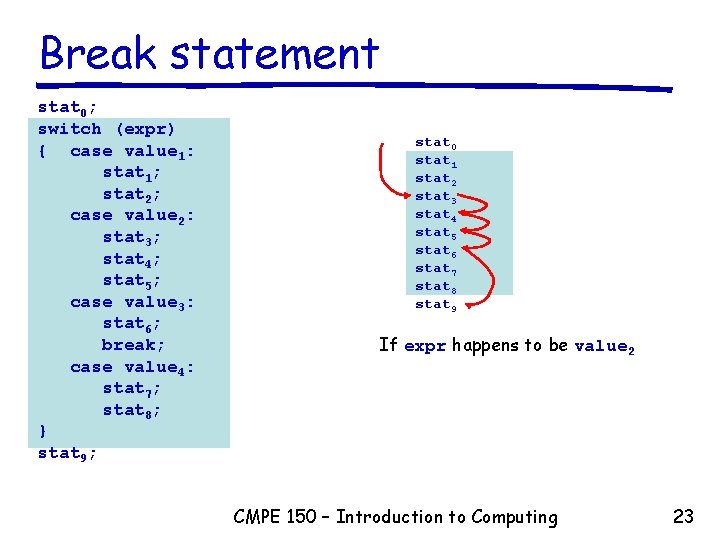
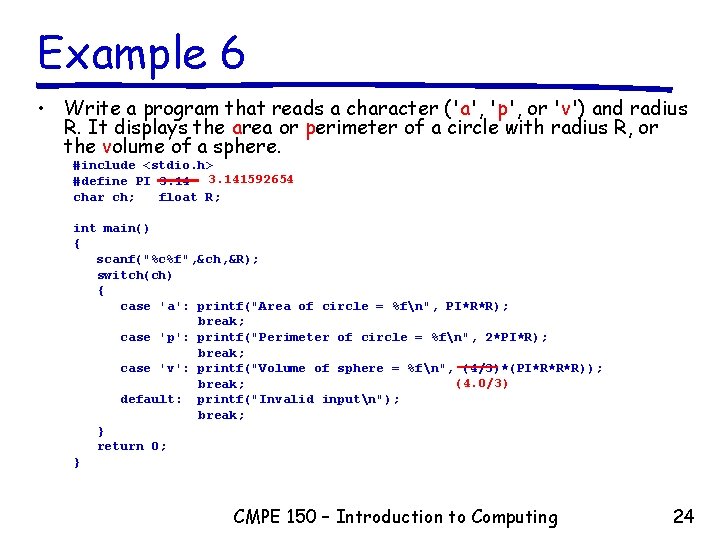
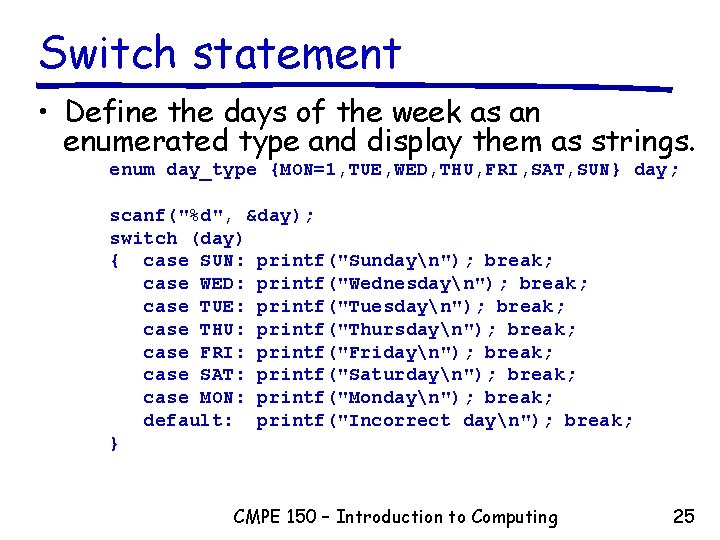
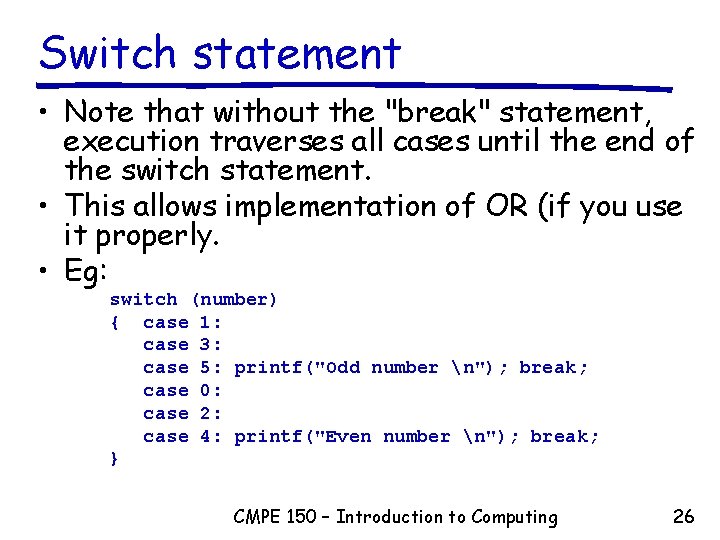
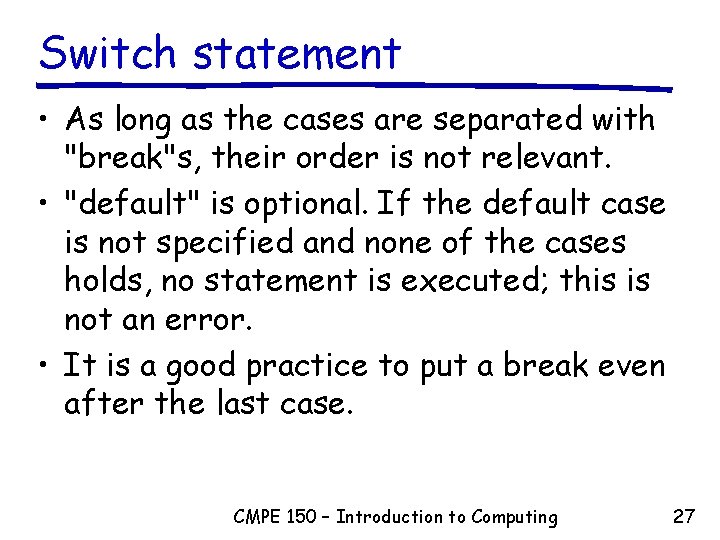
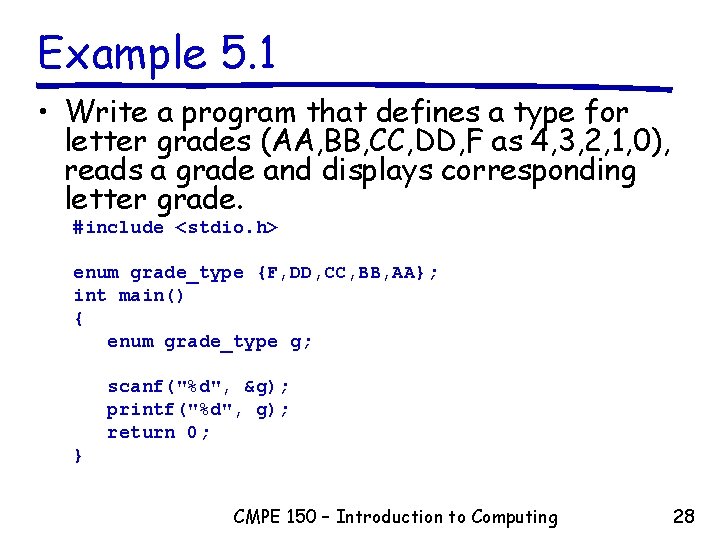
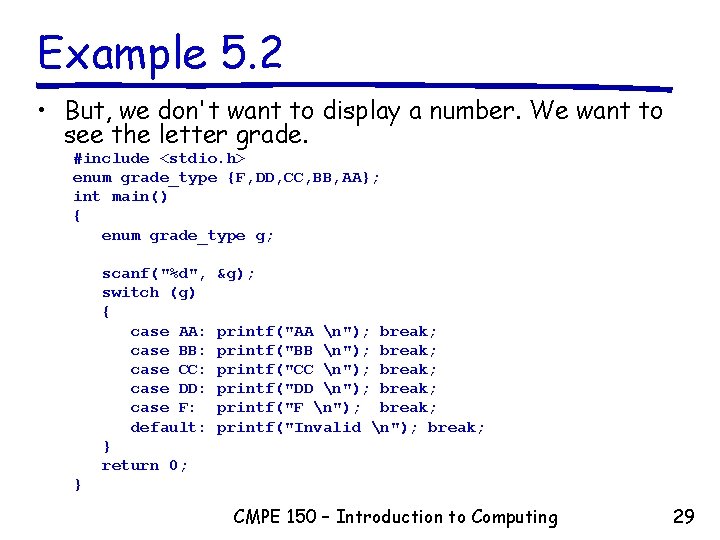
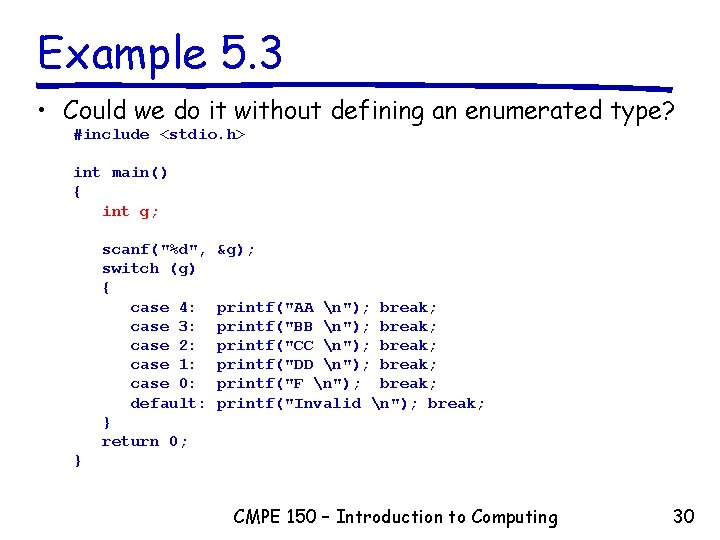
- Slides: 31
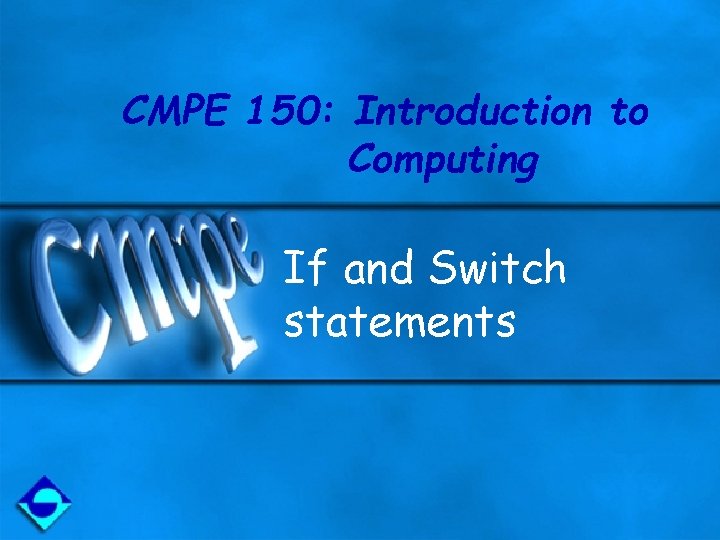
CMPE 150: Introduction to Computing If and Switch statements
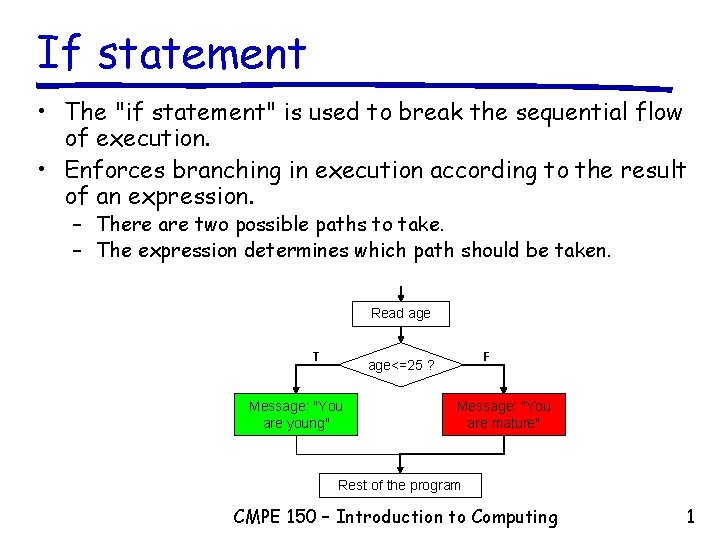
If statement • The "if statement" is used to break the sequential flow of execution. • Enforces branching in execution according to the result of an expression. – There are two possible paths to take. – The expression determines which path should be taken. Read age T F age<=25 ? Message: "You are young" Message: "You are mature" Rest of the program CMPE 150 – Introduction to Computing 1
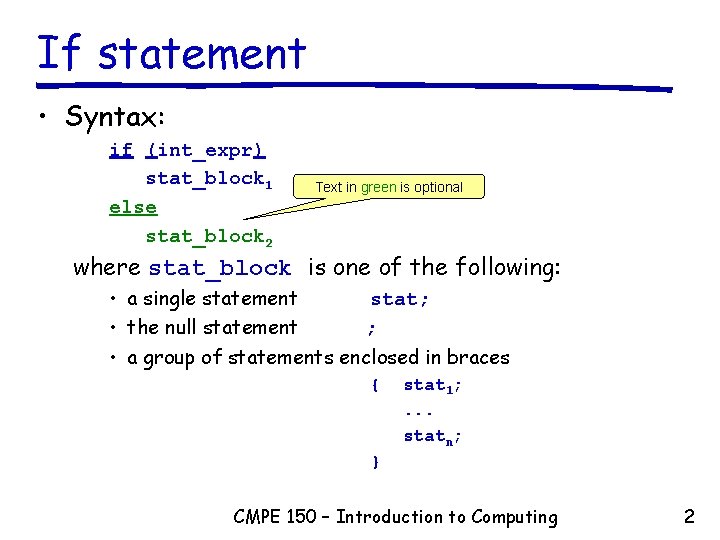
If statement • Syntax: if (int_expr) stat_block 1 else stat_block 2 Text in green is optional where stat_block is one of the following: • a single statement stat; • the null statement ; • a group of statements enclosed in braces { stat 1; . . . statn; } CMPE 150 – Introduction to Computing 2
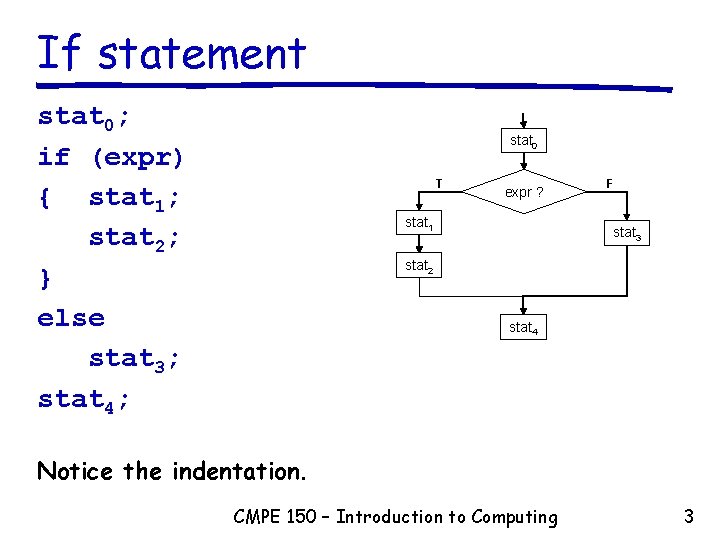
If statement stat 0; if (expr) { stat 1; stat 2; } else stat 3; stat 4; stat 0 T expr ? stat 1 F stat 3 stat 2 stat 4 Notice the indentation. CMPE 150 – Introduction to Computing 3
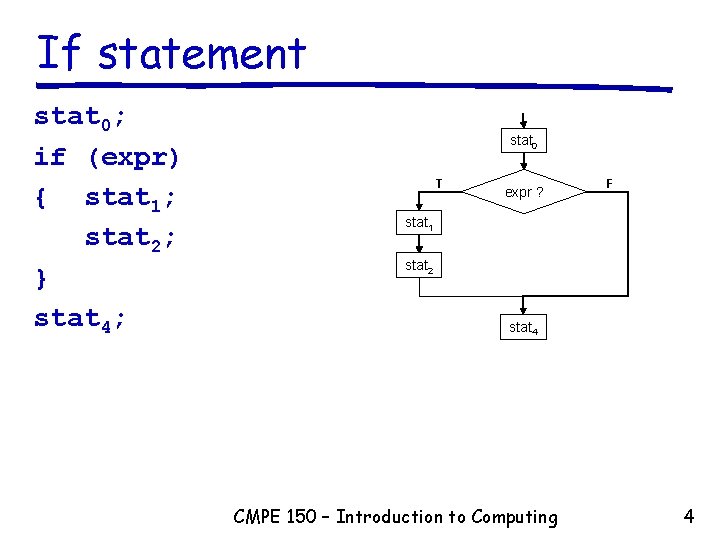
If statement stat 0; if (expr) { stat 1; stat 2; } stat 4; stat 0 T expr ? F stat 1 stat 2 stat 4 CMPE 150 – Introduction to Computing 4
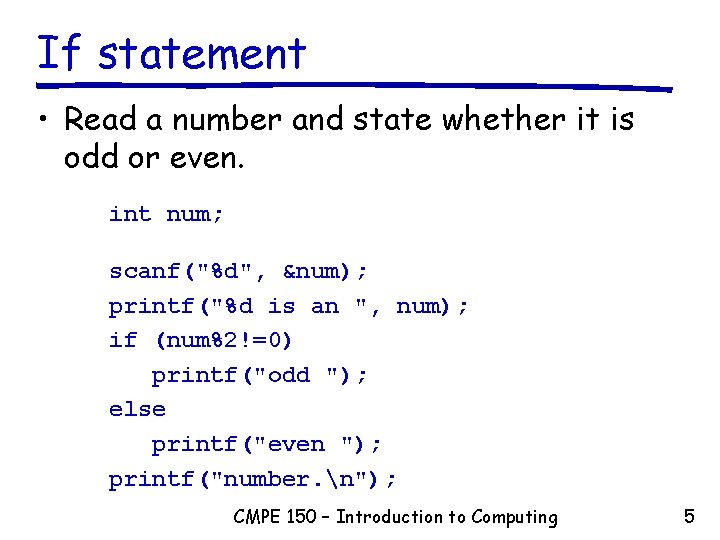
If statement • Read a number and state whether it is odd or even. int num; scanf("%d", &num); printf("%d is an ", num); if (num%2!=0) printf("odd "); else printf("even "); printf("number. n"); CMPE 150 – Introduction to Computing 5
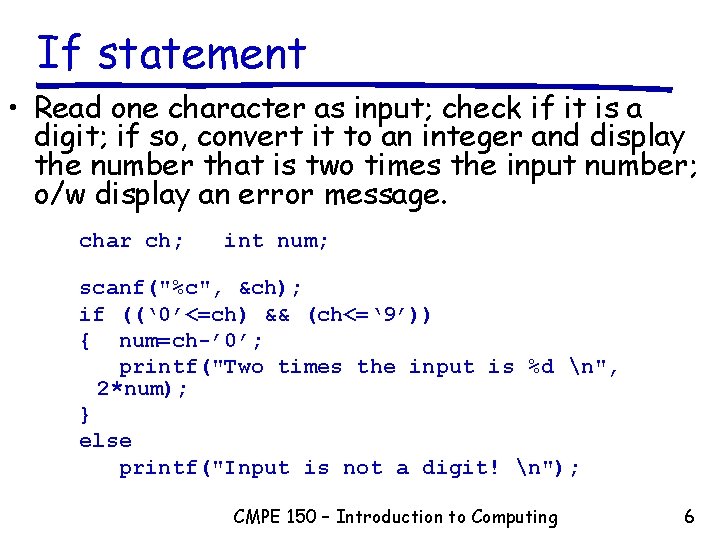
If statement • Read one character as input; check if it is a digit; if so, convert it to an integer and display the number that is two times the input number; o/w display an error message. char ch; int num; scanf("%c", &ch); if ((‘ 0’<=ch) && (ch<=‘ 9’)) { num=ch-’ 0’; printf("Two times the input is %d n", 2*num); } else printf("Input is not a digit! n"); CMPE 150 – Introduction to Computing 6
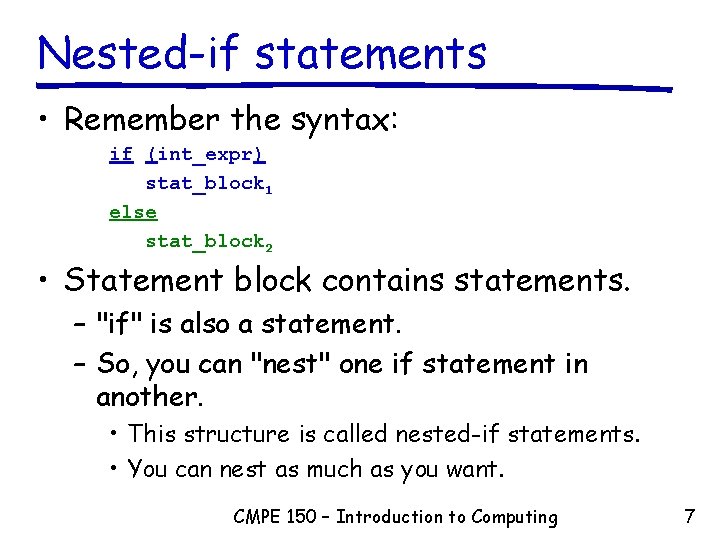
Nested-if statements • Remember the syntax: if (int_expr) stat_block 1 else stat_block 2 • Statement block contains statements. – "if" is also a statement. – So, you can "nest" one if statement in another. • This structure is called nested-if statements. • You can nest as much as you want. CMPE 150 – Introduction to Computing 7
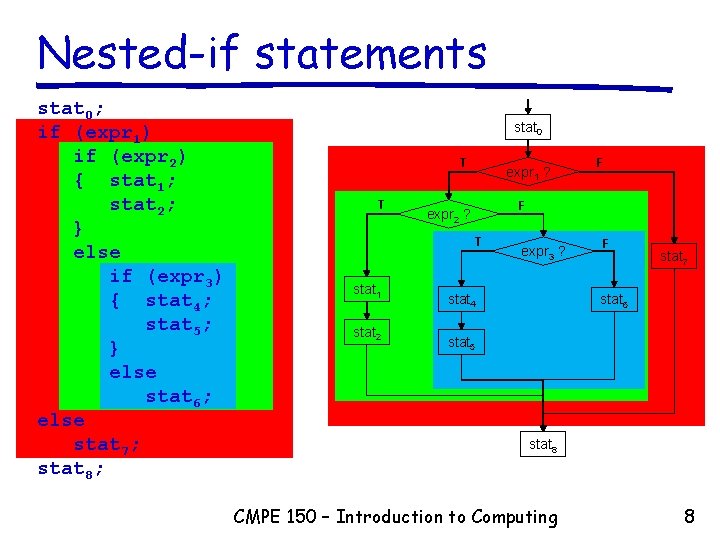
Nested-if statements stat 0; if (expr 1) if (expr 2) { stat 1; stat 2; } else if (expr 3) { stat 4; stat 5; } else stat 6; else stat 7; stat 8; stat 0 T T expr 1 ? F expr 2 ? T stat 1 stat 2 F expr 3 ? stat 4 F stat 7 stat 6 stat 5 stat 8 CMPE 150 – Introduction to Computing 8
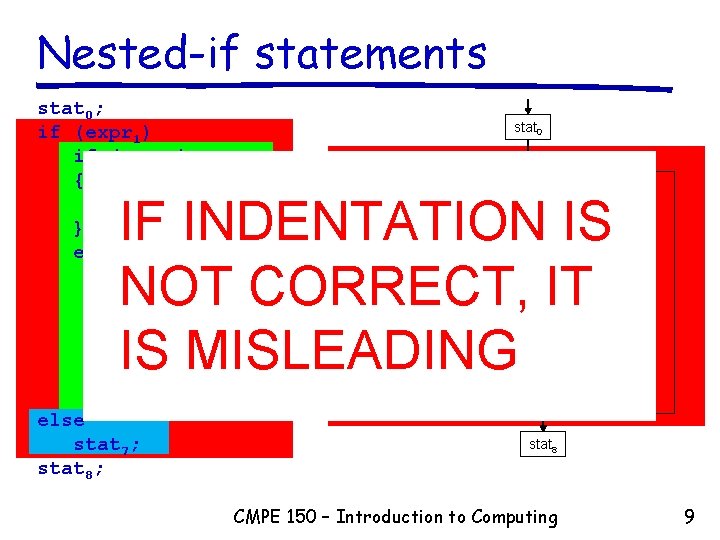
Nested-if statements stat 0; if (expr 1) if (expr 2) { stat 1; stat 2; } else if (expr 3) { stat 4; stat 5; } else stat 6; else stat 7; stat 8; stat 0 T expr 1 ? F IF INDENTATION IS NOT CORRECT, IT IS MISLEADING T F expr 2 ? T stat 1 stat 2 expr 3 ? stat 4 F stat 7 stat 5 stat 8 CMPE 150 – Introduction to Computing 9
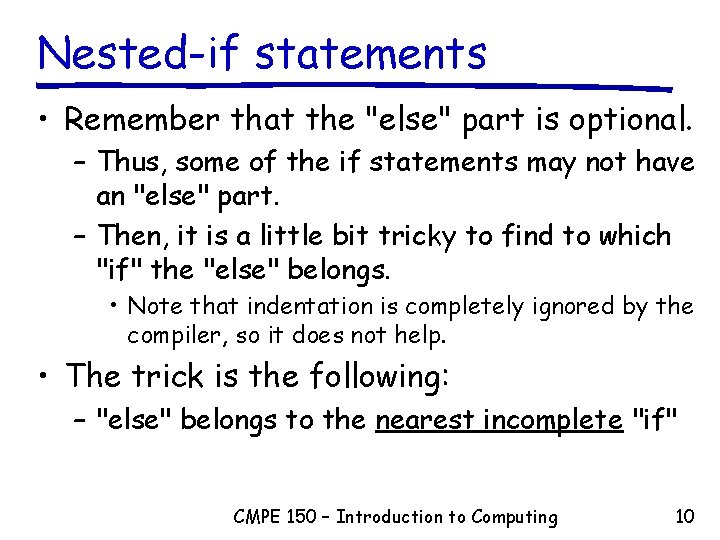
Nested-if statements • Remember that the "else" part is optional. – Thus, some of the if statements may not have an "else" part. – Then, it is a little bit tricky to find to which "if" the "else" belongs. • Note that indentation is completely ignored by the compiler, so it does not help. • The trick is the following: – "else" belongs to the nearest incomplete "if" CMPE 150 – Introduction to Computing 10
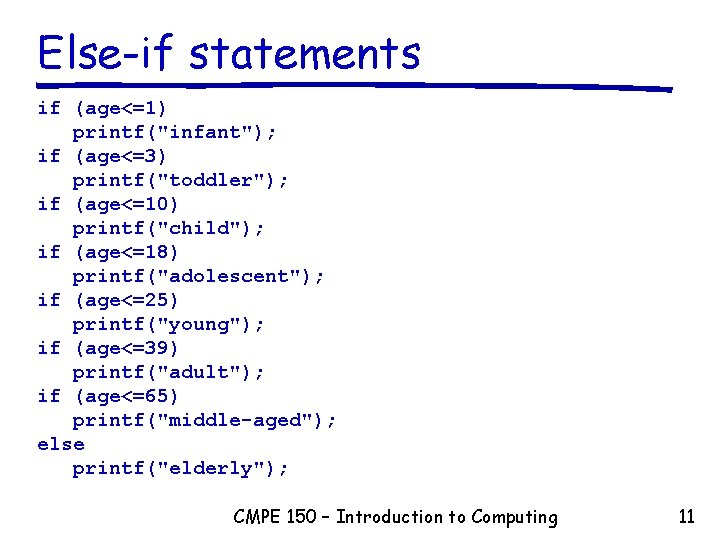
Else-if statements if (age<=1) printf("infant"); if (age<=3) printf("toddler"); if (age<=10) printf("child"); if (age<=18) printf("adolescent"); if (age<=25) printf("young"); if (age<=39) printf("adult"); if (age<=65) printf("middle-aged"); else printf("elderly"); CMPE 150 – Introduction to Computing 11
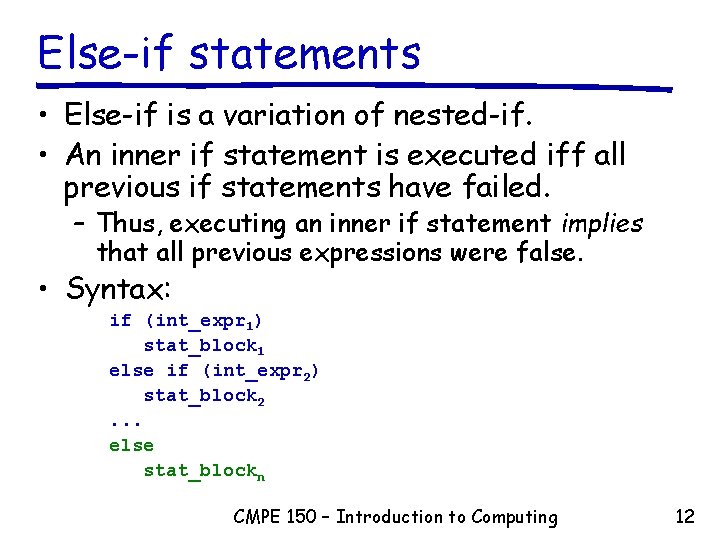
Else-if statements • Else-if is a variation of nested-if. • An inner if statement is executed iff all previous if statements have failed. – Thus, executing an inner if statement implies that all previous expressions were false. • Syntax: if (int_expr 1) stat_block 1 else if (int_expr 2) stat_block 2. . . else stat_blockn CMPE 150 – Introduction to Computing 12
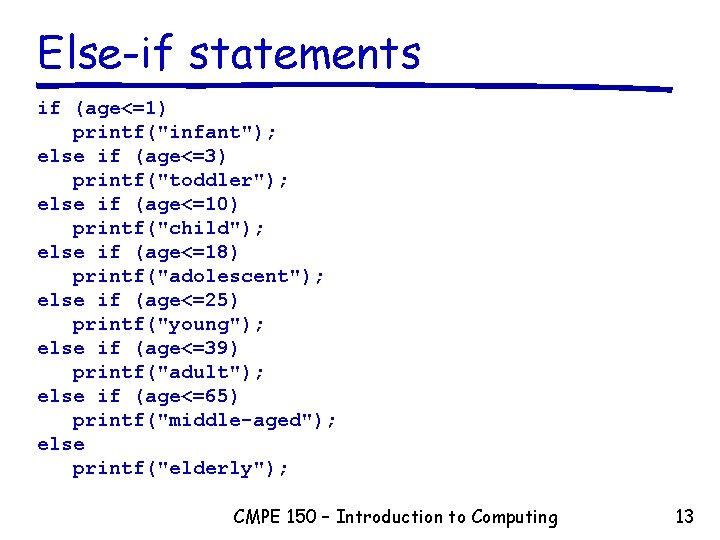
Else-if statements if (age<=1) printf("infant"); else if (age<=3) printf("toddler"); else if (age<=10) printf("child"); else if (age<=18) printf("adolescent"); else if (age<=25) printf("young"); else if (age<=39) printf("adult"); else if (age<=65) printf("middle-aged"); else printf("elderly"); CMPE 150 – Introduction to Computing 13
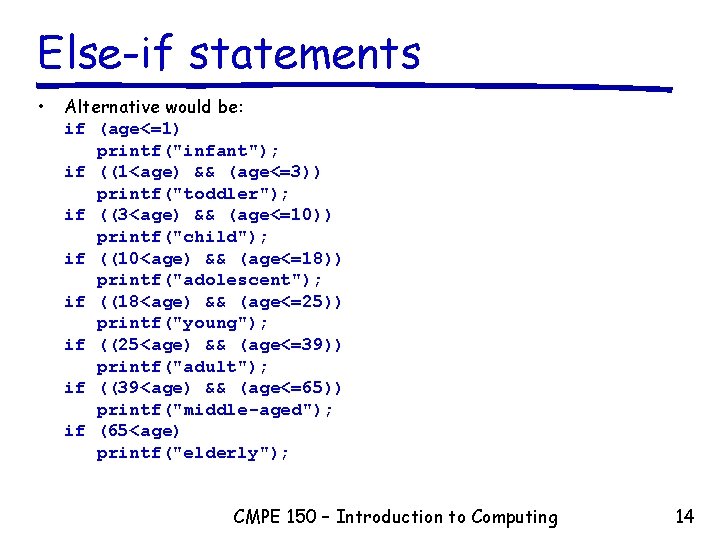
Else-if statements • Alternative would be: if (age<=1) printf("infant"); if ((1<age) && (age<=3)) printf("toddler"); if ((3<age) && (age<=10)) printf("child"); if ((10<age) && (age<=18)) printf("adolescent"); if ((18<age) && (age<=25)) printf("young"); if ((25<age) && (age<=39)) printf("adult"); if ((39<age) && (age<=65)) printf("middle-aged"); if (65<age) printf("elderly"); CMPE 150 – Introduction to Computing 14
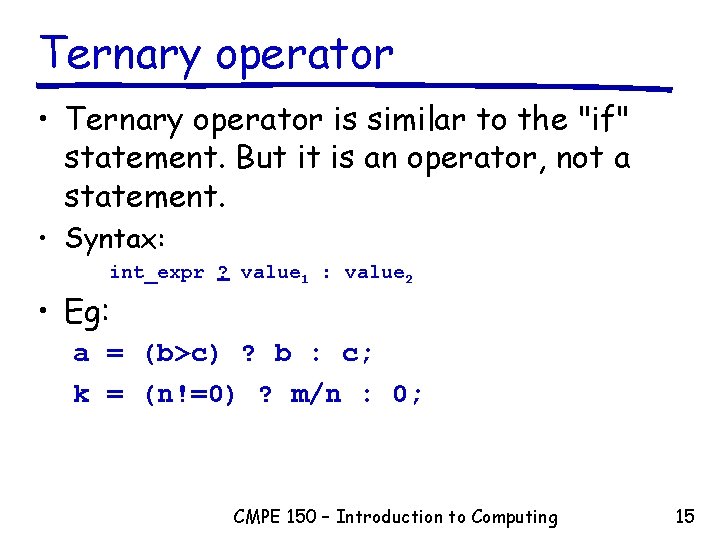
Ternary operator • Ternary operator is similar to the "if" statement. But it is an operator, not a statement. • Syntax: int_expr ? value 1 : value 2 • Eg: a = (b>c) ? b : c; k = (n!=0) ? m/n : 0; CMPE 150 – Introduction to Computing 15
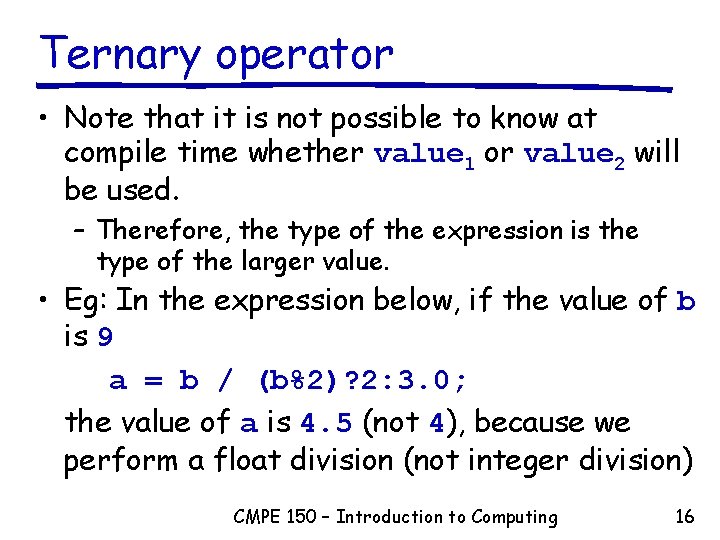
Ternary operator • Note that it is not possible to know at compile time whether value 1 or value 2 will be used. – Therefore, the type of the expression is the type of the larger value. • Eg: In the expression below, if the value of b is 9 a = b / (b%2)? 2: 3. 0; the value of a is 4. 5 (not 4), because we perform a float division (not integer division) CMPE 150 – Introduction to Computing 16
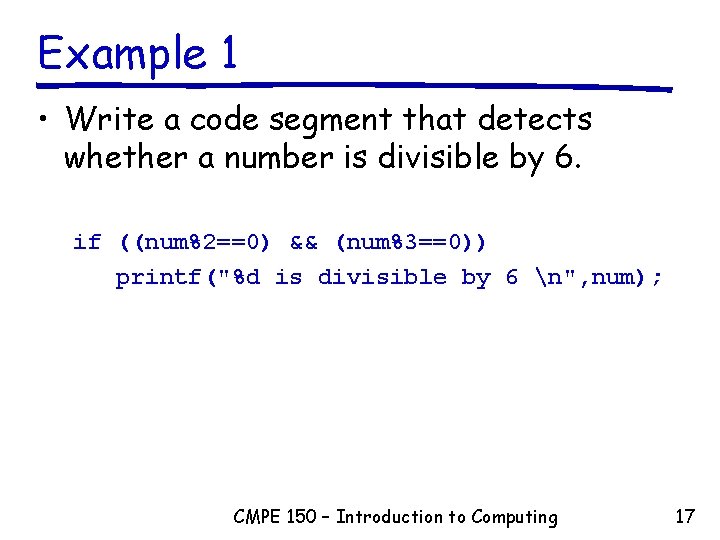
Example 1 • Write a code segment that detects whether a number is divisible by 6. if ((num%2==0) && (num%3==0)) printf("%d is divisible by 6 n", num); CMPE 150 – Introduction to Computing 17
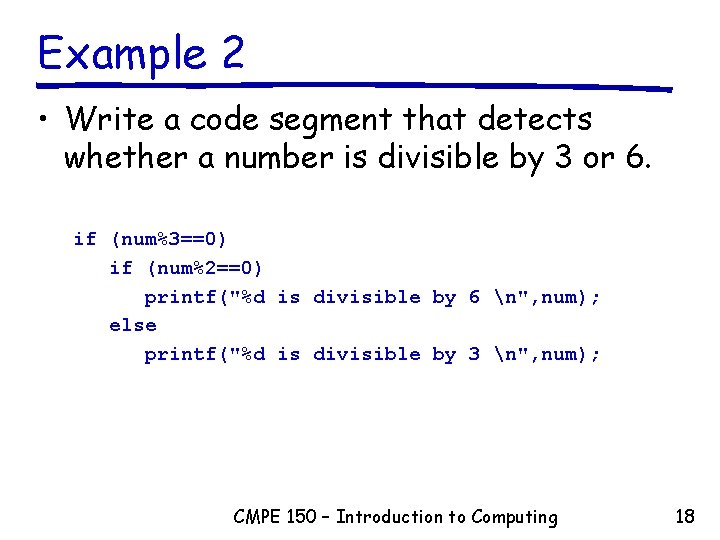
Example 2 • Write a code segment that detects whether a number is divisible by 3 or 6. if (num%3==0) if (num%2==0) printf("%d is divisible by 6 n", num); else printf("%d is divisible by 3 n", num); CMPE 150 – Introduction to Computing 18
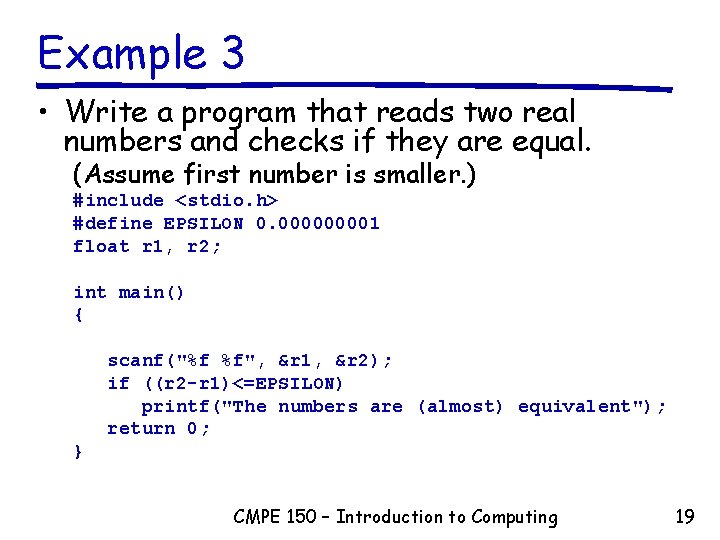
Example 3 • Write a program that reads two real numbers and checks if they are equal. (Assume first number is smaller. ) #include <stdio. h> #define EPSILON 0. 00001 float r 1, r 2; int main() { scanf("%f %f", &r 1, &r 2); if ((r 2 -r 1)<=EPSILON) printf("The numbers are (almost) equivalent"); return 0; } CMPE 150 – Introduction to Computing 19
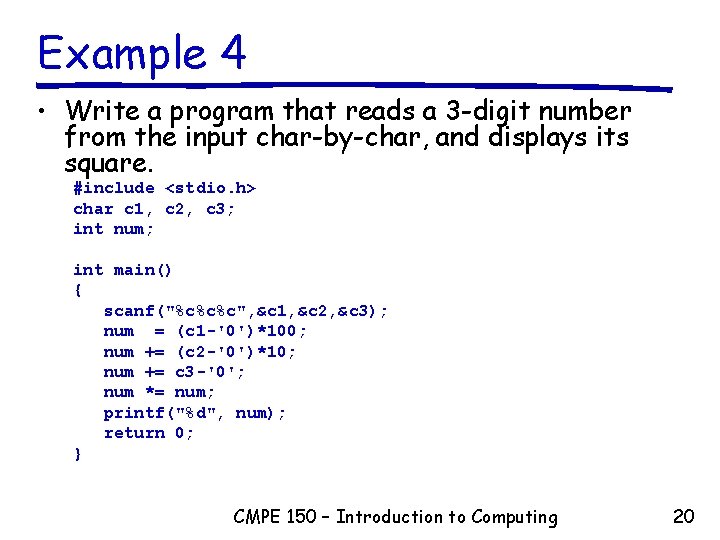
Example 4 • Write a program that reads a 3 -digit number from the input char-by-char, and displays its square. #include <stdio. h> char c 1, c 2, c 3; int num; int main() { scanf("%c%c%c", &c 1, &c 2, &c 3); num = (c 1 -'0')*100; num += (c 2 -'0')*10; num += c 3 -'0'; num *= num; printf("%d", num); return 0; } CMPE 150 – Introduction to Computing 20
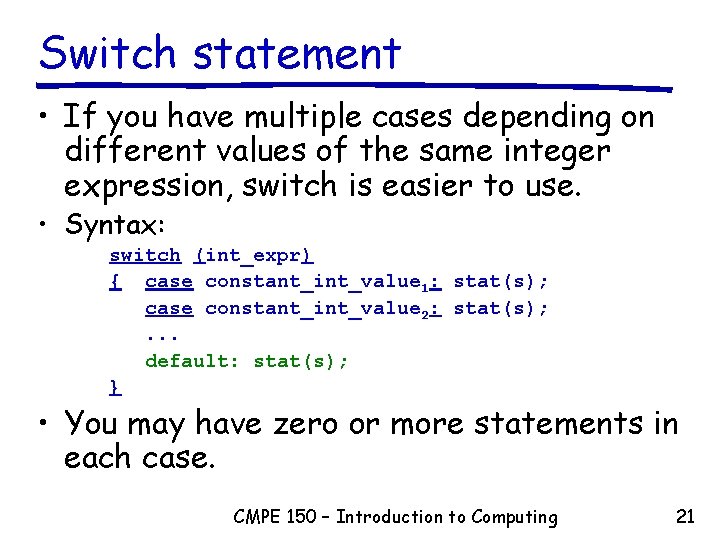
Switch statement • If you have multiple cases depending on different values of the same integer expression, switch is easier to use. • Syntax: switch (int_expr) { case constant_int_value 1: stat(s); case constant_int_value 2: stat(s); . . . default: stat(s); } • You may have zero or more statements in each case. CMPE 150 – Introduction to Computing 21
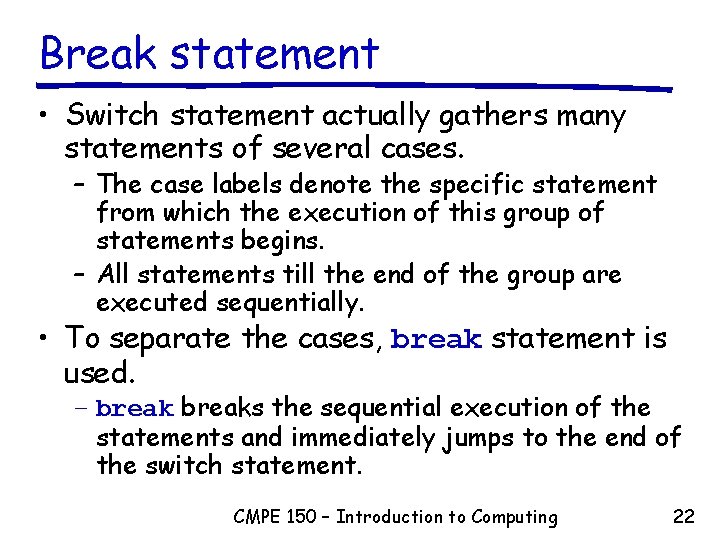
Break statement • Switch statement actually gathers many statements of several cases. – The case labels denote the specific statement from which the execution of this group of statements begins. – All statements till the end of the group are executed sequentially. • To separate the cases, break statement is used. – breaks the sequential execution of the statements and immediately jumps to the end of the switch statement. CMPE 150 – Introduction to Computing 22
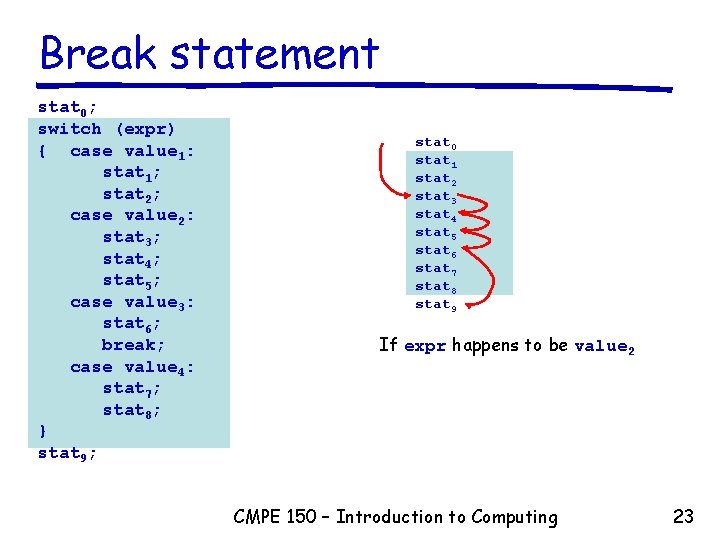
Break statement stat 0; switch (expr) { case value 1: stat 1; stat 2; case value 2: stat 3; stat 4; stat 5; case value 3: stat 6; break; case value 4: stat 7; stat 8; } stat 9; stat 0 stat 1 stat 2 stat 3 stat 4 stat 5 stat 6 stat 7 stat 8 stat 9 If expr happens to be value 2 CMPE 150 – Introduction to Computing 23
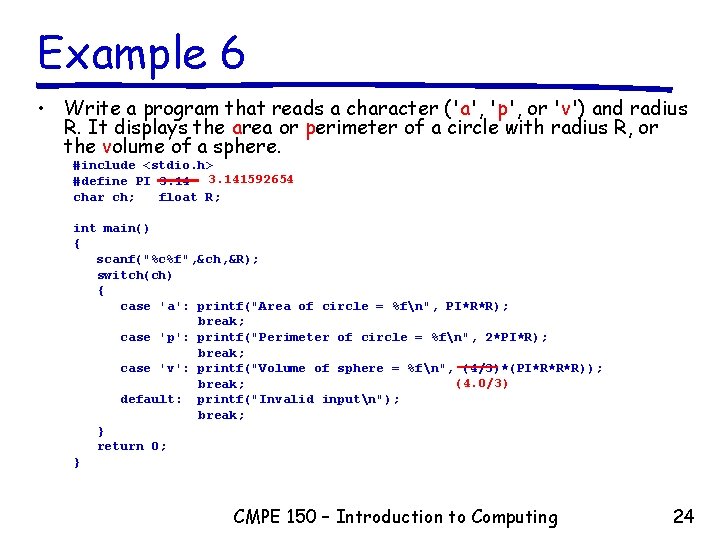
Example 6 • Write a program that reads a character ('a', 'p', or 'v') and radius R. It displays the area or perimeter of a circle with radius R, or the volume of a sphere. #include <stdio. h> #define PI 3. 141592654 char ch; float R; int main() { scanf("%c%f", &ch, &R); switch(ch) { case 'a': printf("Area of circle = %fn", PI*R*R); break; case 'p': printf("Perimeter of circle = %fn", 2*PI*R); break; case 'v': printf("Volume of sphere = %fn", (4/3)*(PI*R*R*R)); (4. 0/3) break; default: printf("Invalid inputn"); break; } return 0; } CMPE 150 – Introduction to Computing 24
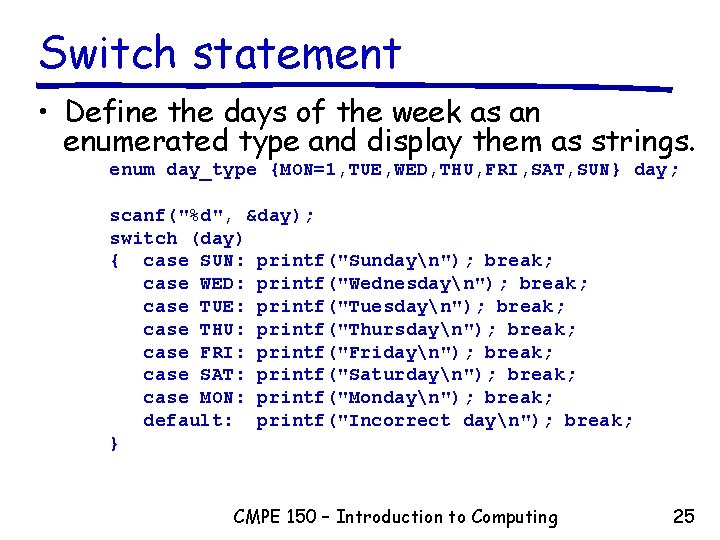
Switch statement • Define the days of the week as an enumerated type and display them as strings. enum day_type {MON=1, TUE, WED, THU, FRI, SAT, SUN} day; scanf("%d", &day); switch (day) { case SUN: printf("Sundayn"); break; case WED: printf("Wednesdayn"); break; case TUE: printf("Tuesdayn"); break; case THU: printf("Thursdayn"); break; case FRI: printf("Fridayn"); break; case SAT: printf("Saturdayn"); break; case MON: printf("Mondayn"); break; default: printf("Incorrect dayn"); break; } CMPE 150 – Introduction to Computing 25
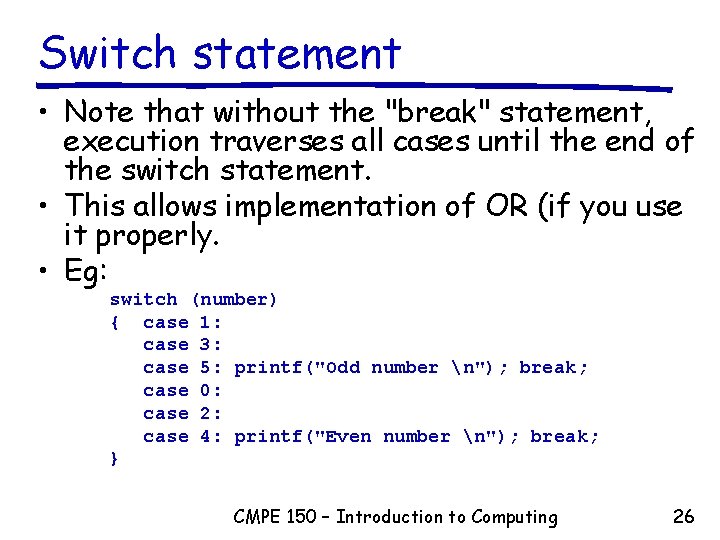
Switch statement • Note that without the "break" statement, execution traverses all cases until the end of the switch statement. • This allows implementation of OR (if you use it properly. • Eg: switch (number) { case 1: case 3: case 5: printf("Odd number n"); break; case 0: case 2: case 4: printf("Even number n"); break; } CMPE 150 – Introduction to Computing 26
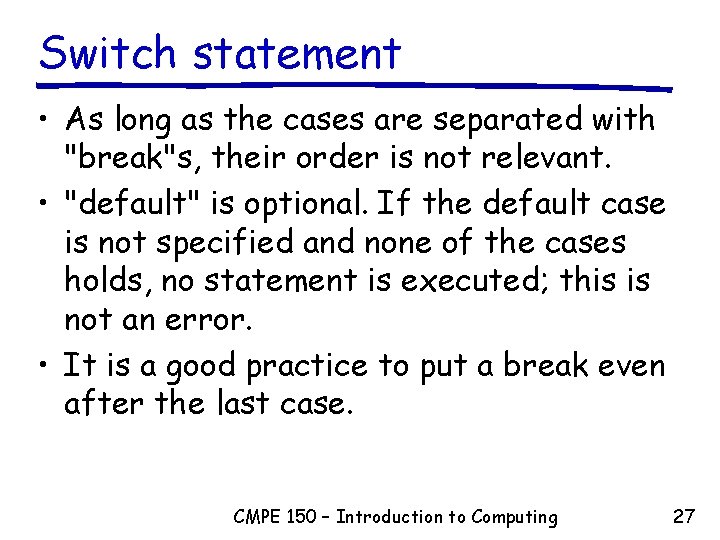
Switch statement • As long as the cases are separated with "break"s, their order is not relevant. • "default" is optional. If the default case is not specified and none of the cases holds, no statement is executed; this is not an error. • It is a good practice to put a break even after the last case. CMPE 150 – Introduction to Computing 27
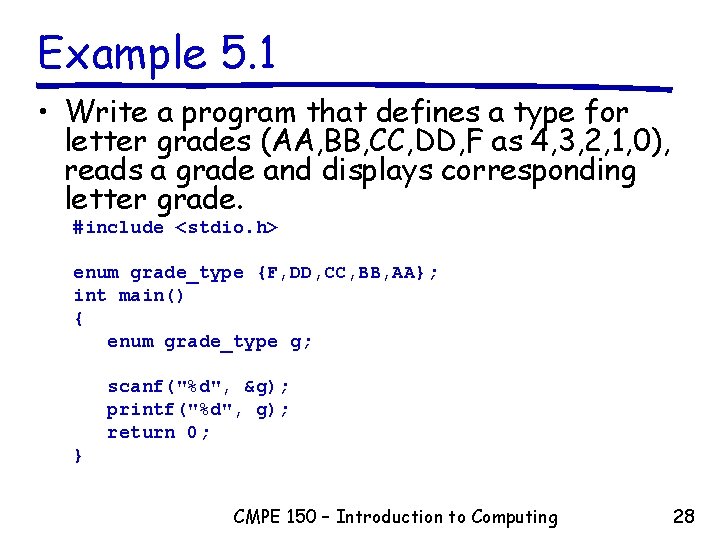
Example 5. 1 • Write a program that defines a type for letter grades (AA, BB, CC, DD, F as 4, 3, 2, 1, 0), reads a grade and displays corresponding letter grade. #include <stdio. h> enum grade_type {F, DD, CC, BB, AA}; int main() { enum grade_type g; scanf("%d", &g); printf("%d", g); return 0; } CMPE 150 – Introduction to Computing 28
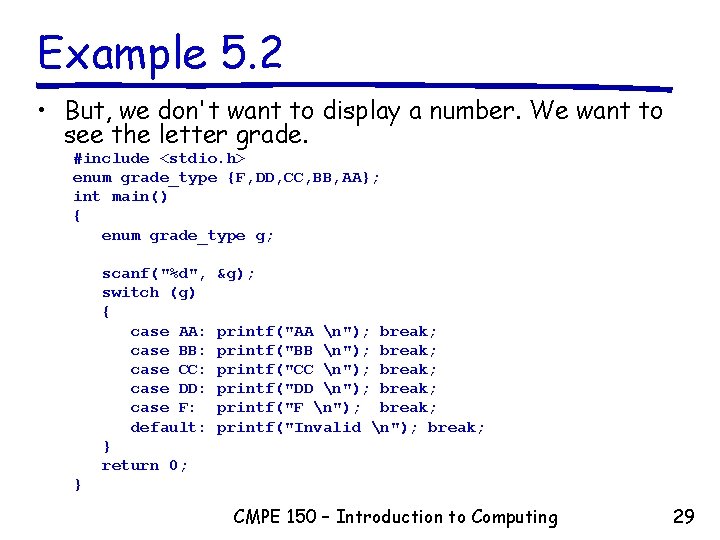
Example 5. 2 • But, we don't want to display a number. We want to see the letter grade. #include <stdio. h> enum grade_type {F, DD, CC, BB, AA}; int main() { enum grade_type g; scanf("%d", switch (g) { case AA: case BB: case CC: case DD: case F: default: } return 0; &g); printf("AA n"); break; printf("BB n"); break; printf("CC n"); break; printf("DD n"); break; printf("F n"); break; printf("Invalid n"); break; } CMPE 150 – Introduction to Computing 29
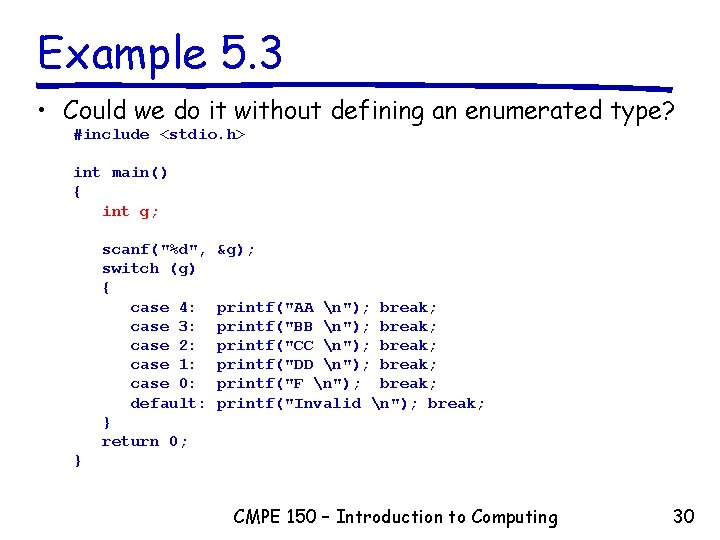
Example 5. 3 • Could we do it without defining an enumerated type? #include <stdio. h> int main() { int g; scanf("%d", switch (g) { case 4: case 3: case 2: case 1: case 0: default: } return 0; &g); printf("AA n"); break; printf("BB n"); break; printf("CC n"); break; printf("DD n"); break; printf("F n"); break; printf("Invalid n"); break; } CMPE 150 – Introduction to Computing 30
Cmpe 150
Cmpe 150
Cmpe150
Cmpe 150
In a banyan switch micro switch
Go switch model 11
Conventional computing and intelligent computing
Cmpe 294 sjsu
Emu computer engineering
Cmpe 280
Cmpe 273
Cmpe 252
Cmpe 252
Computing flowchart
Cantor paradoksu
Ron mak sjsu
Cmpe 280
Cmpe 252
Cmpe 252
Cmpe 280
Cmpe 280
Cmpe 252
Yunan harfleri
Cmpe 212
Cmpe 280
Cmpe226
Cmpe emu
Dr. karabatak
Cmpe 220
Cmpe 273
Duygu çelik ertuğrul
Cmpe 3 ucsc