CISC 181 Introduction to Computer Science Dr Mc
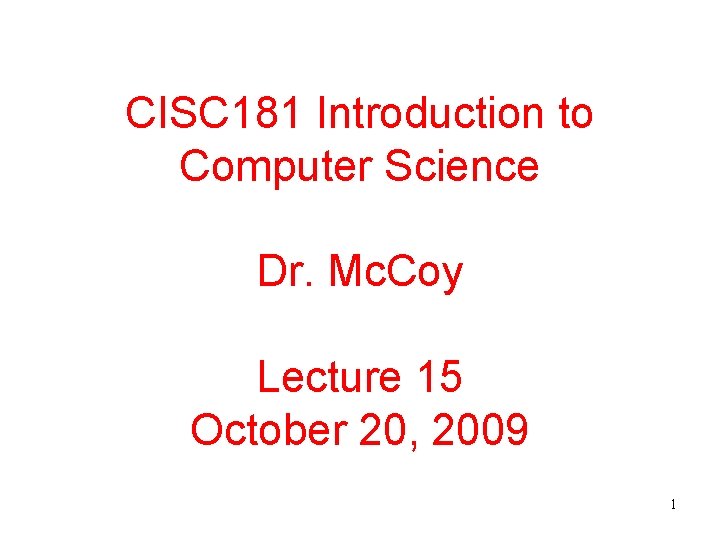
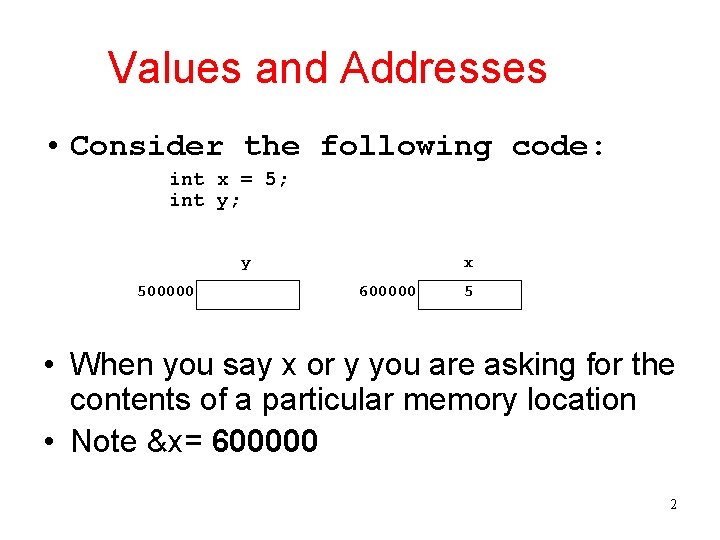
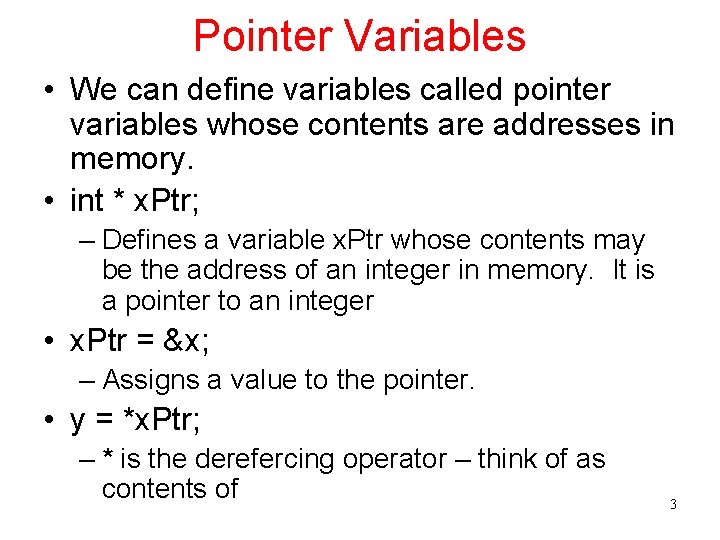
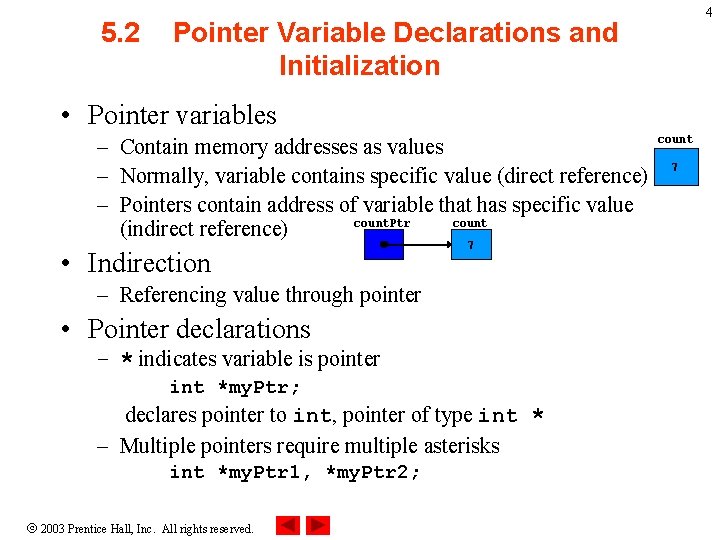
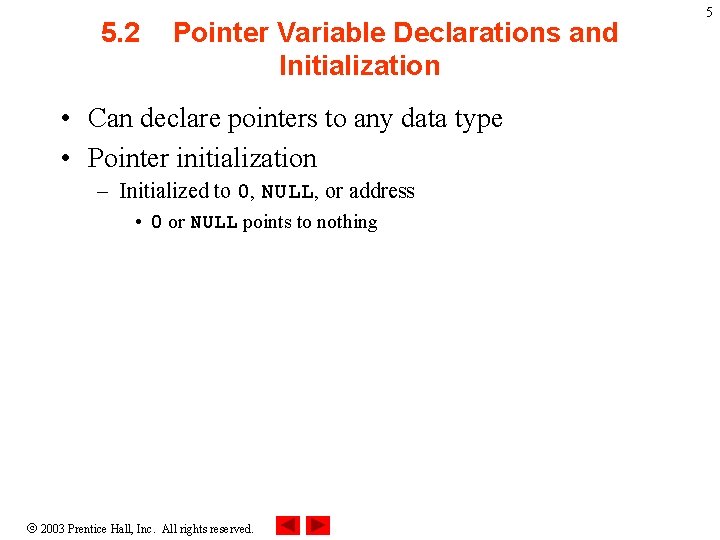
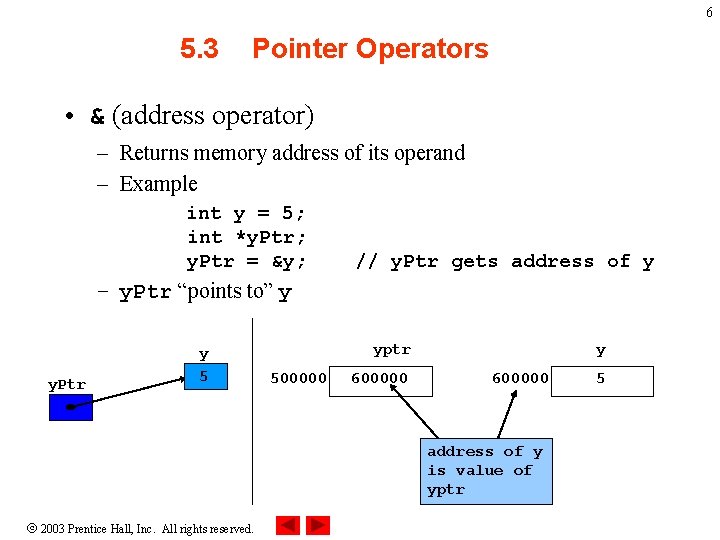
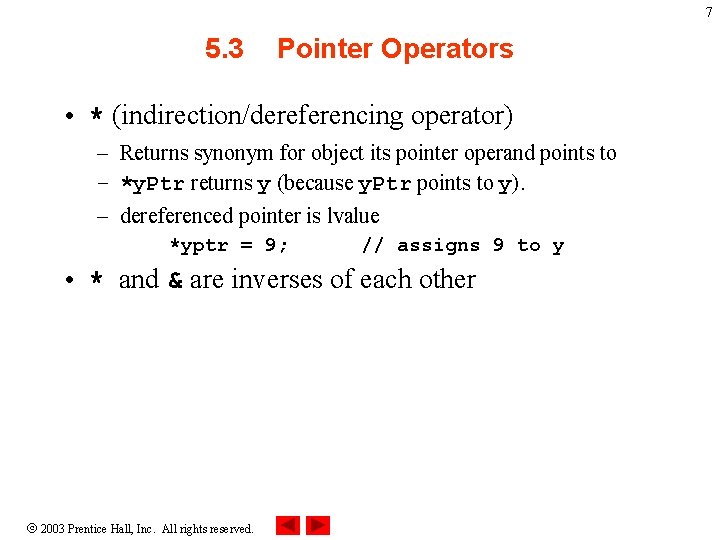
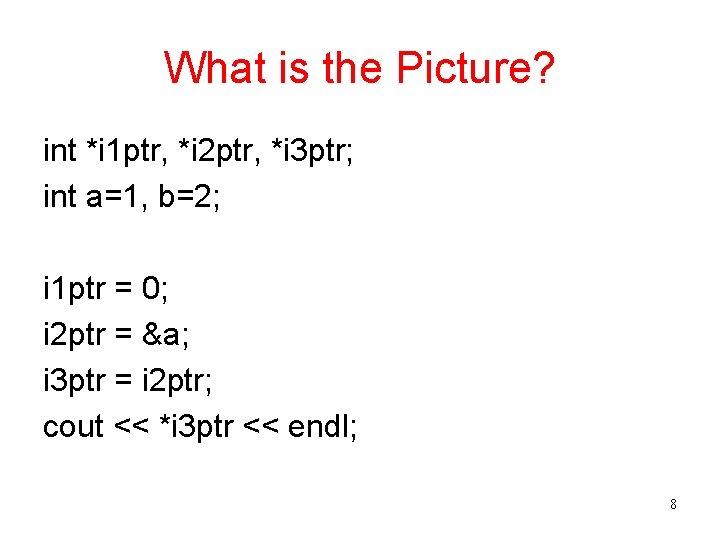
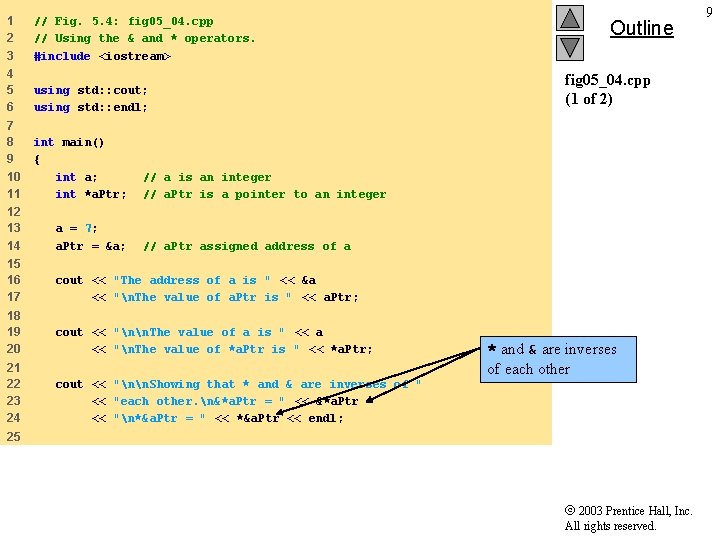
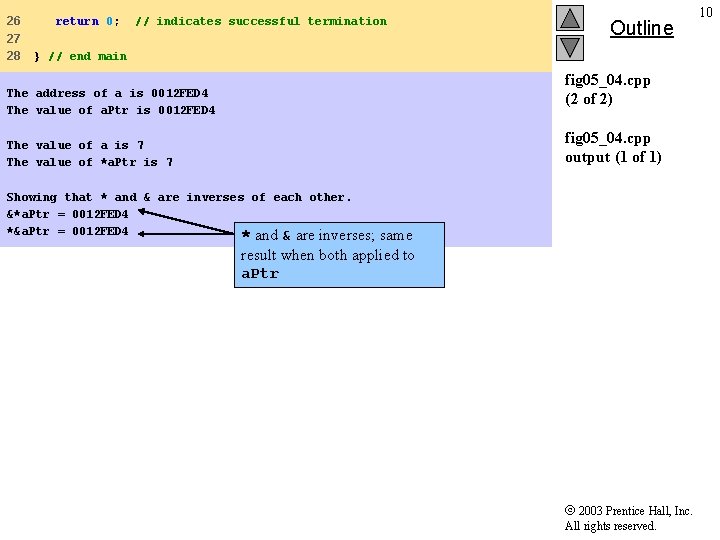
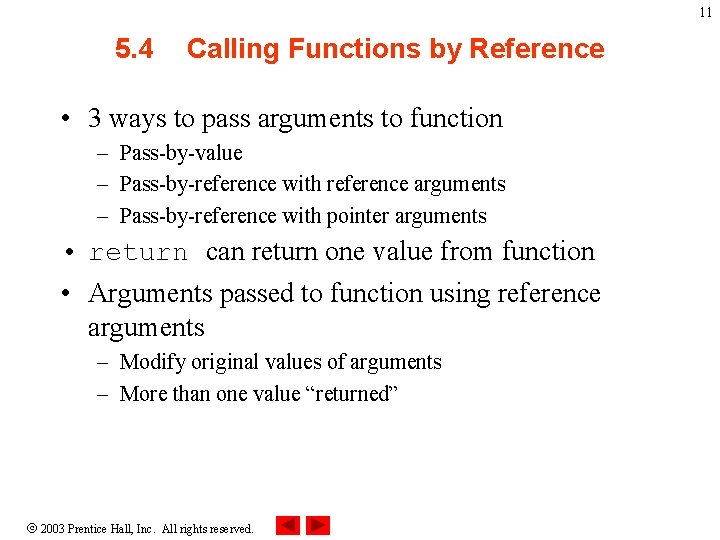
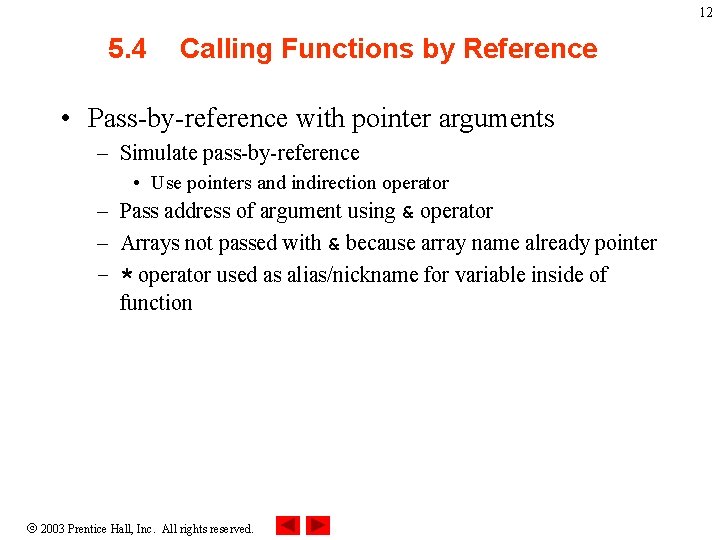
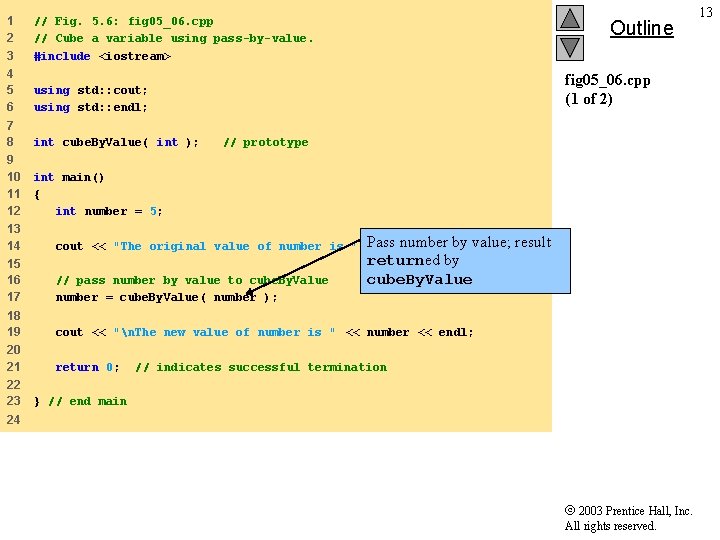
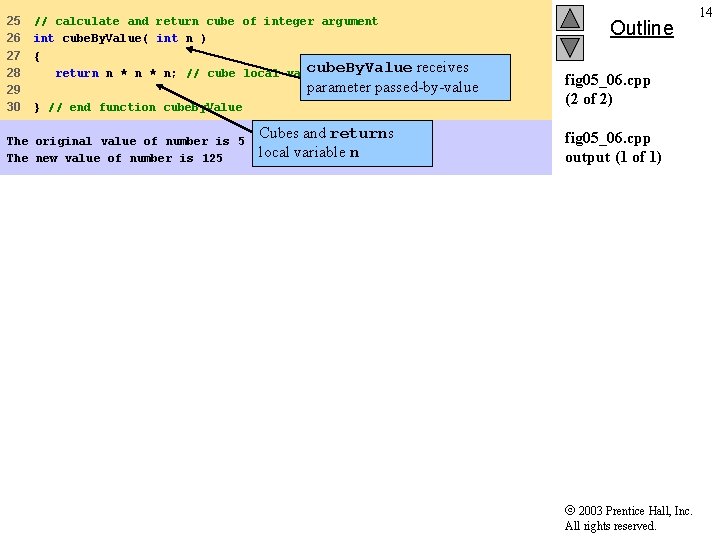
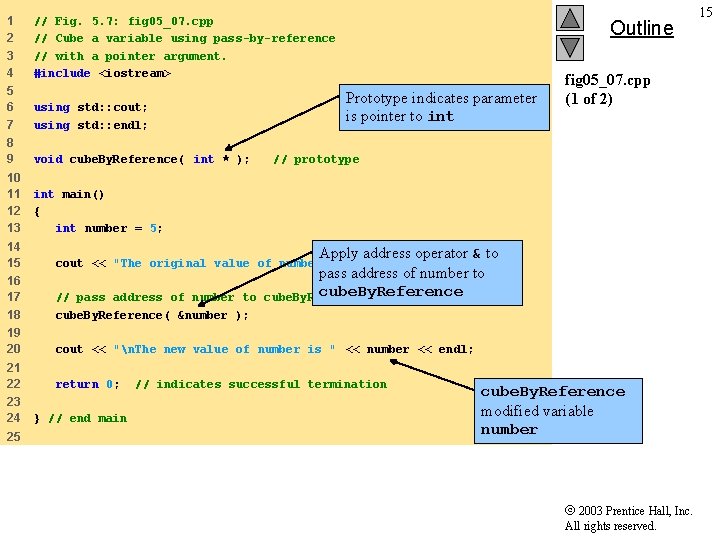
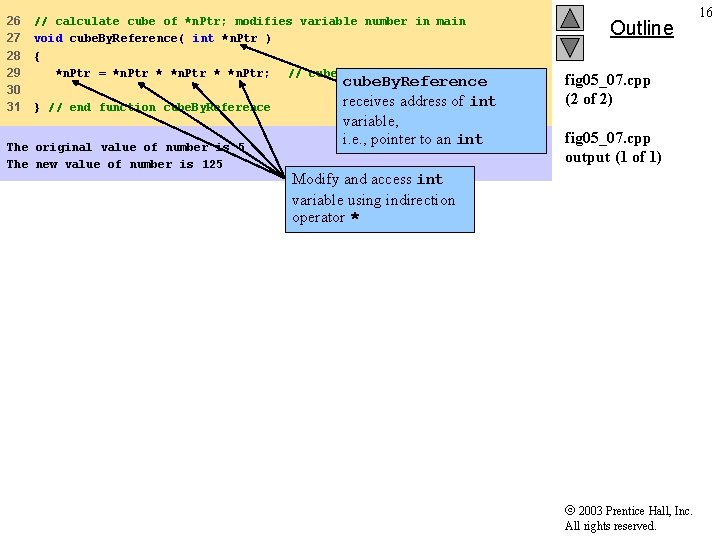
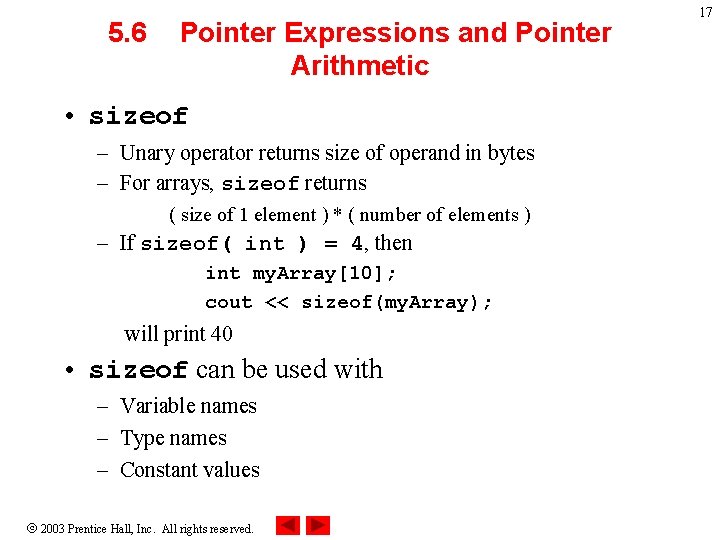
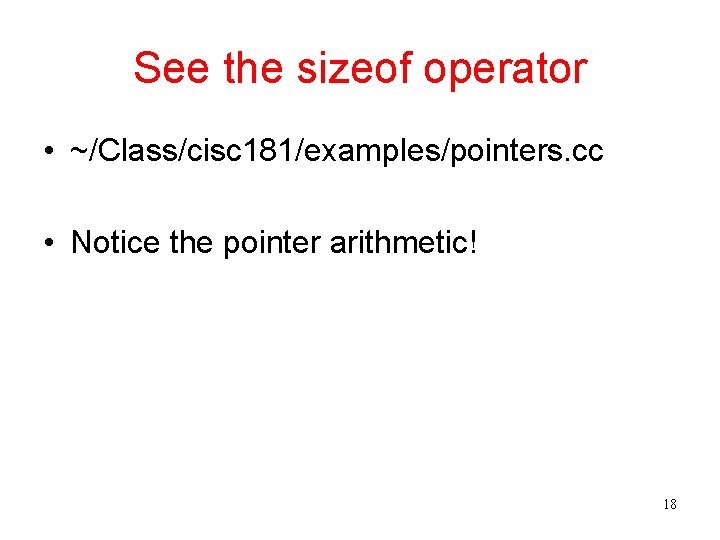
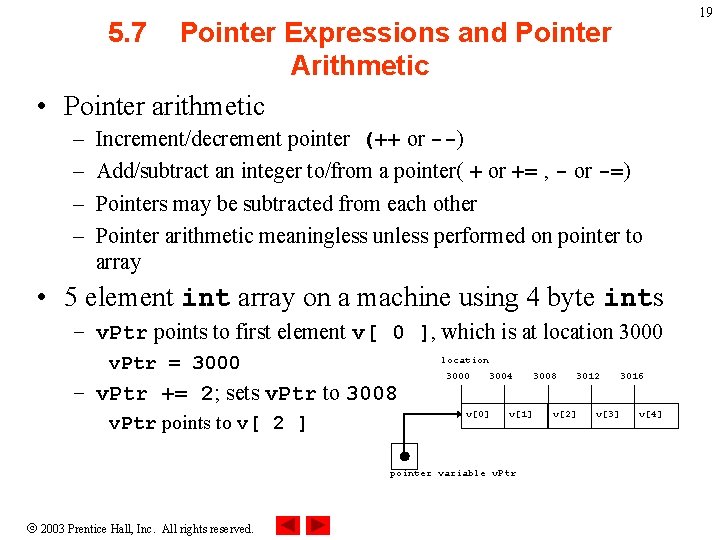
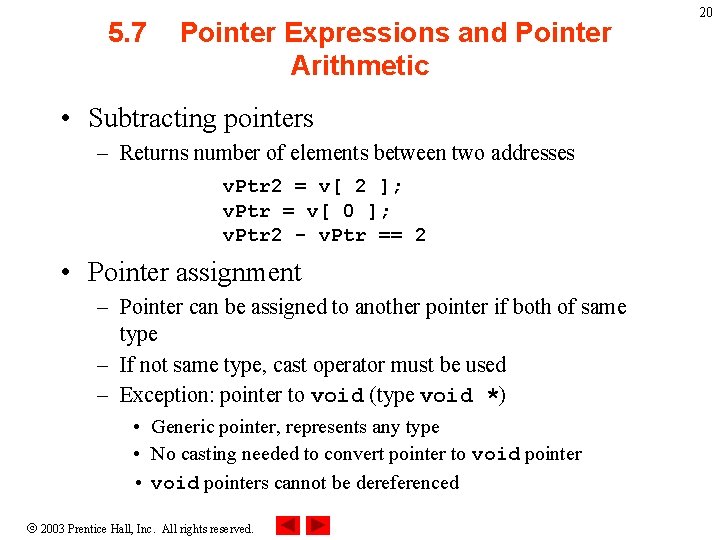
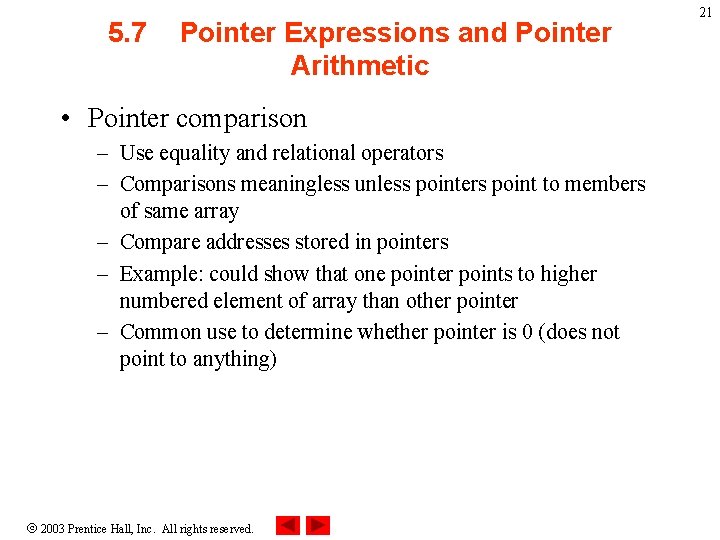
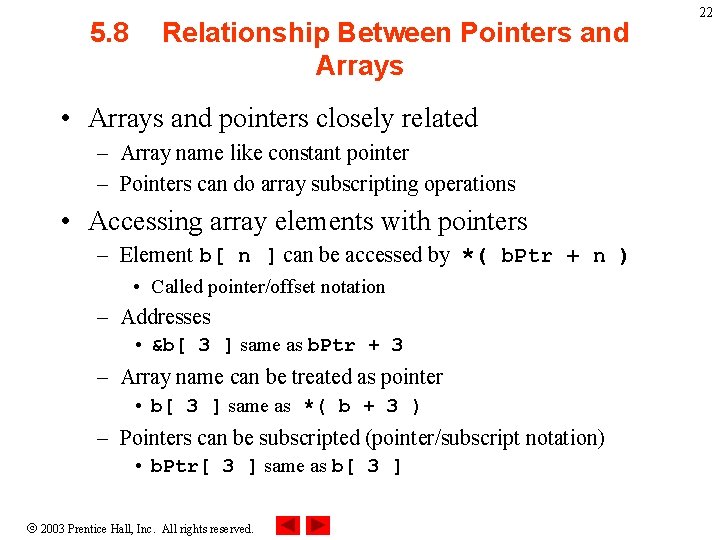
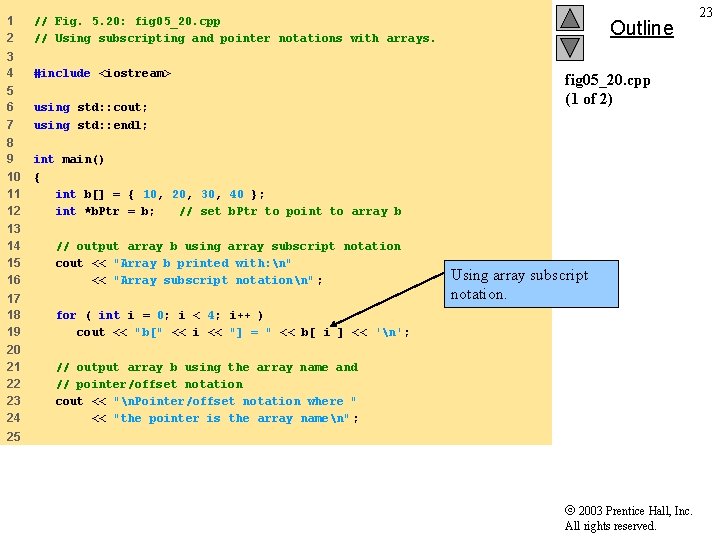
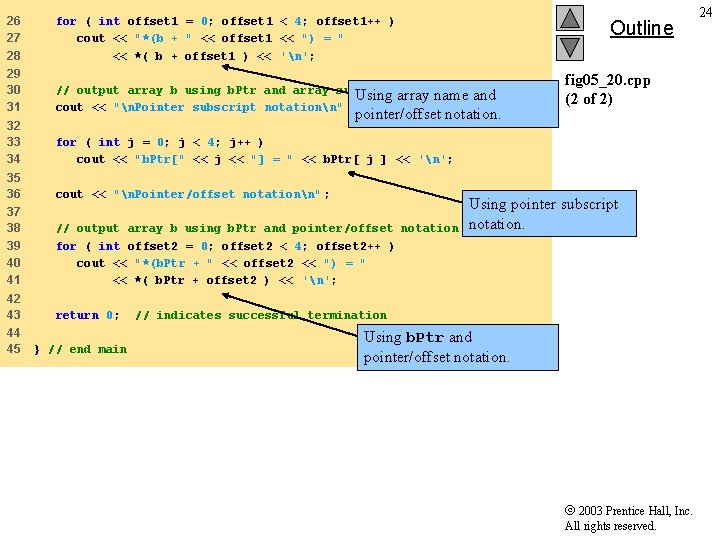
![Array b printed with: Array subscript notation b[0] = 10 b[1] = 20 b[2] Array b printed with: Array subscript notation b[0] = 10 b[1] = 20 b[2]](https://slidetodoc.com/presentation_image_h2/63508d25c6a14ae040b0a1a3f943e14f/image-25.jpg)
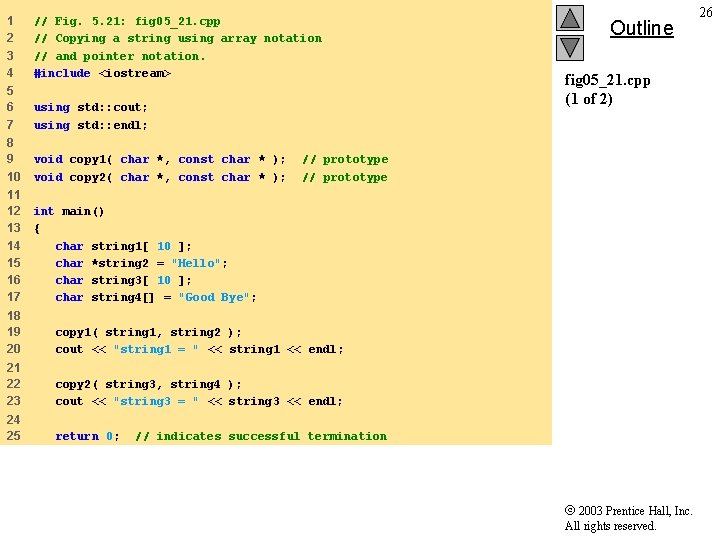
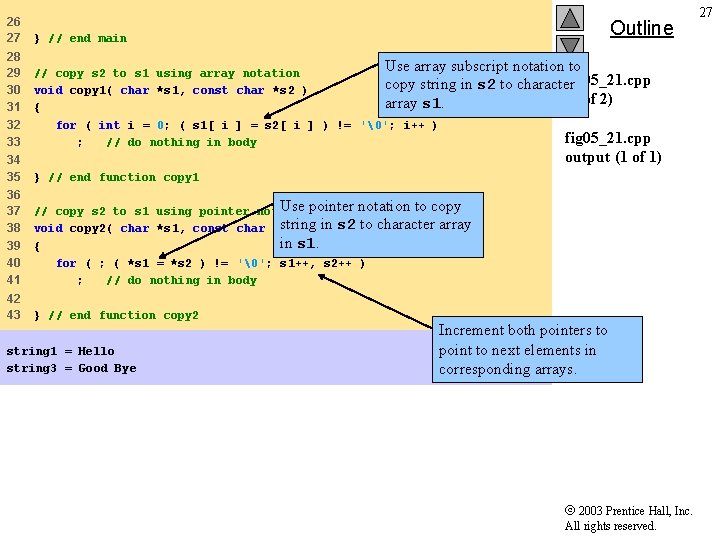
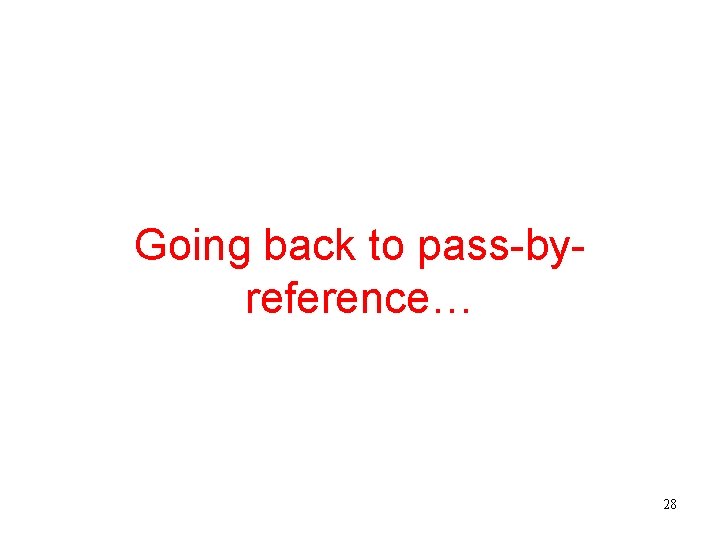
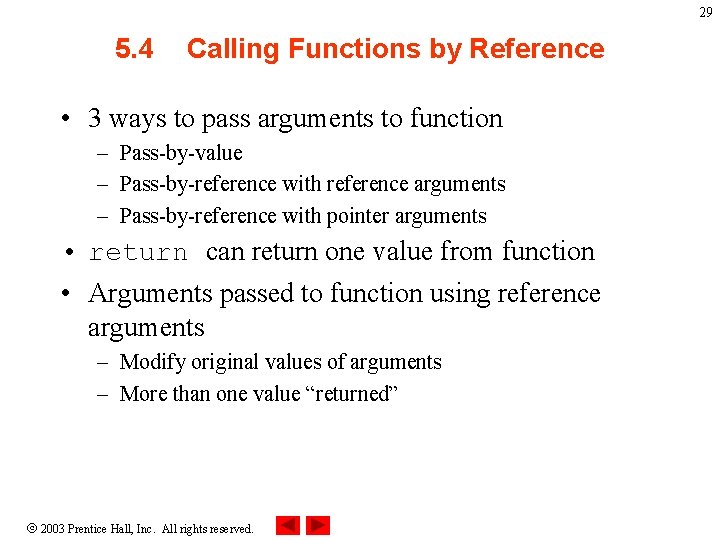
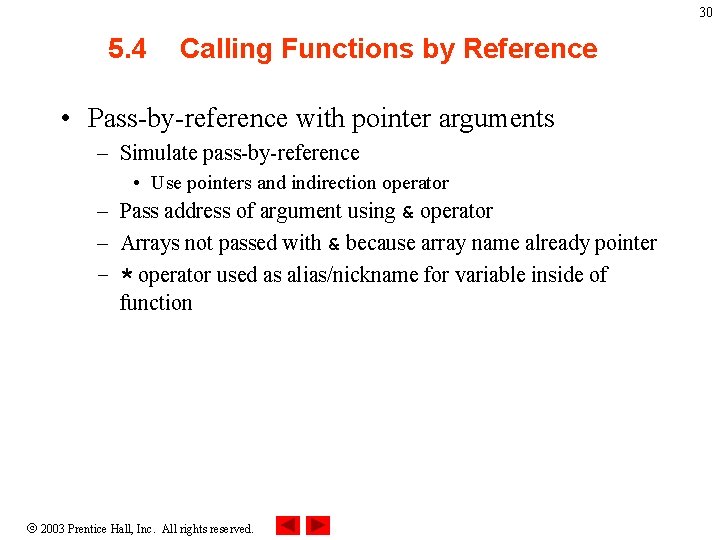
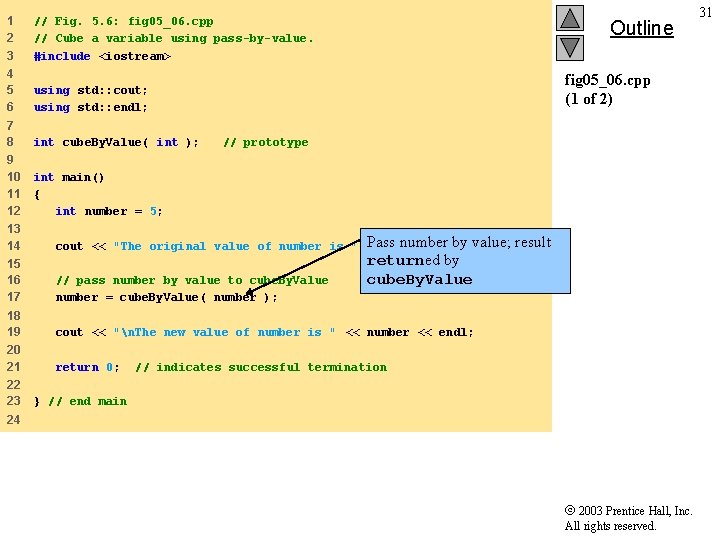
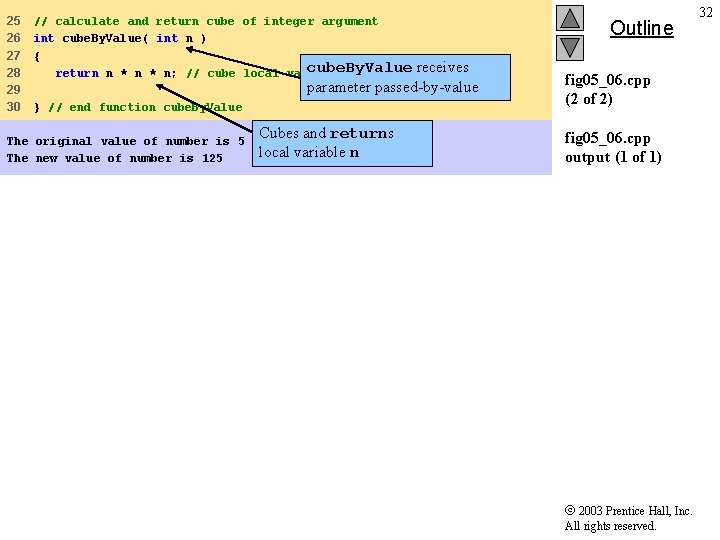
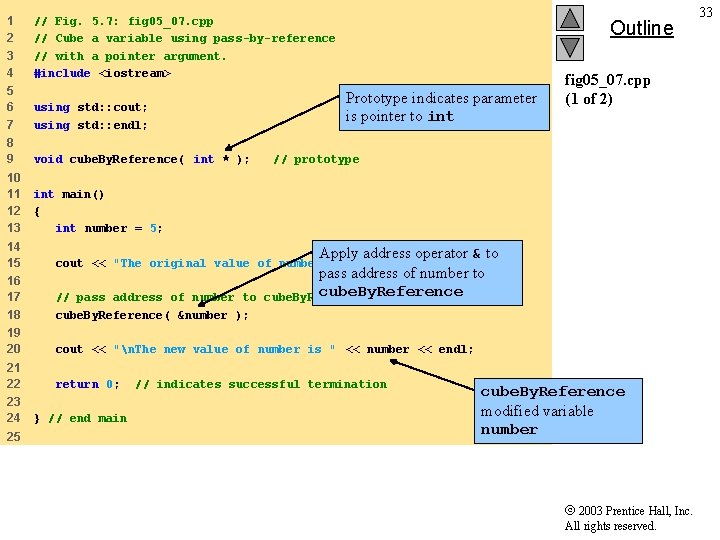
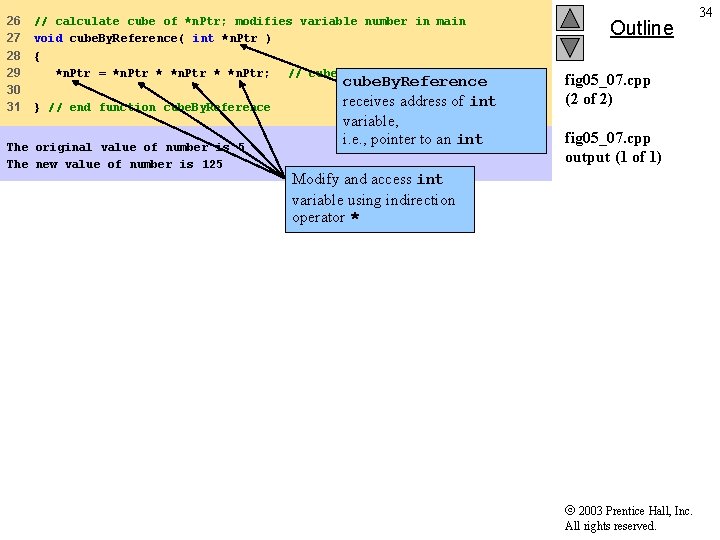
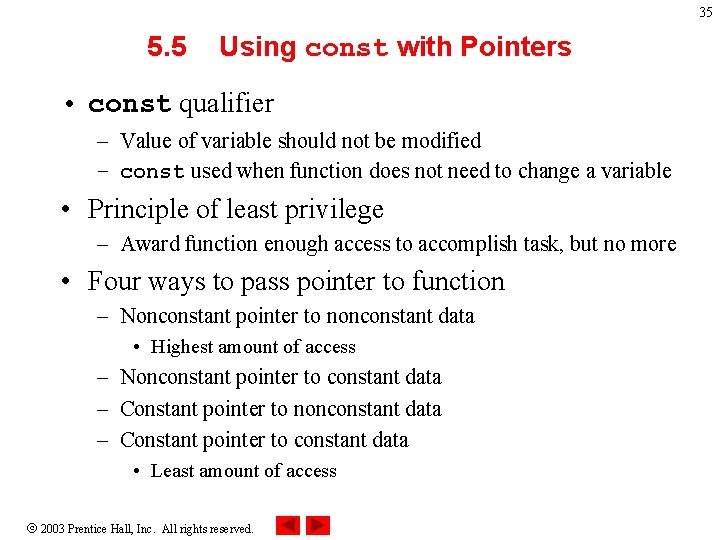
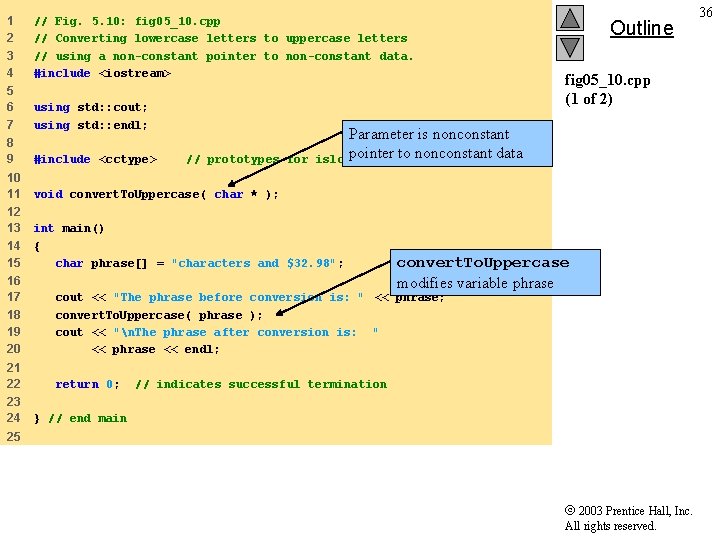
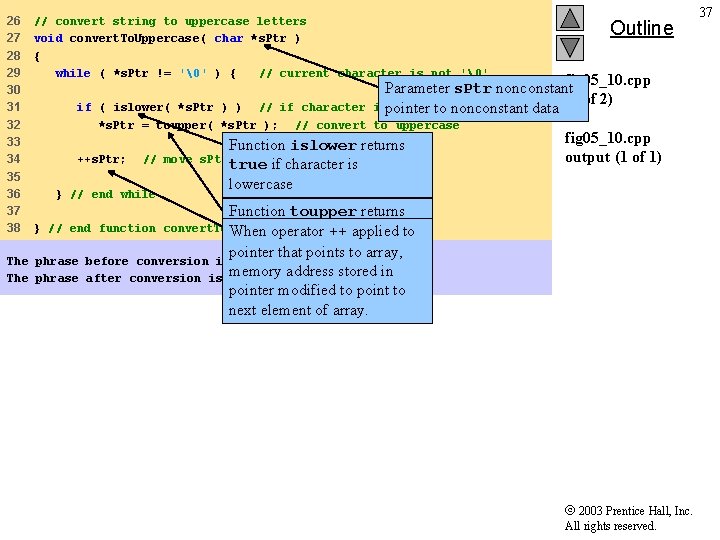
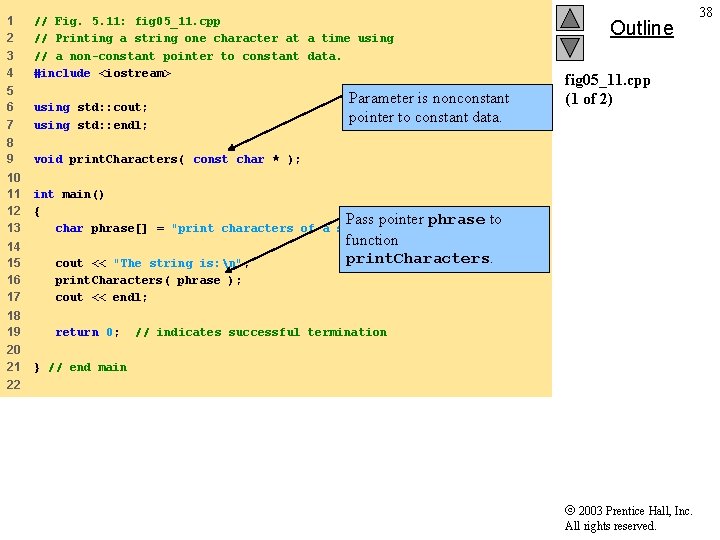
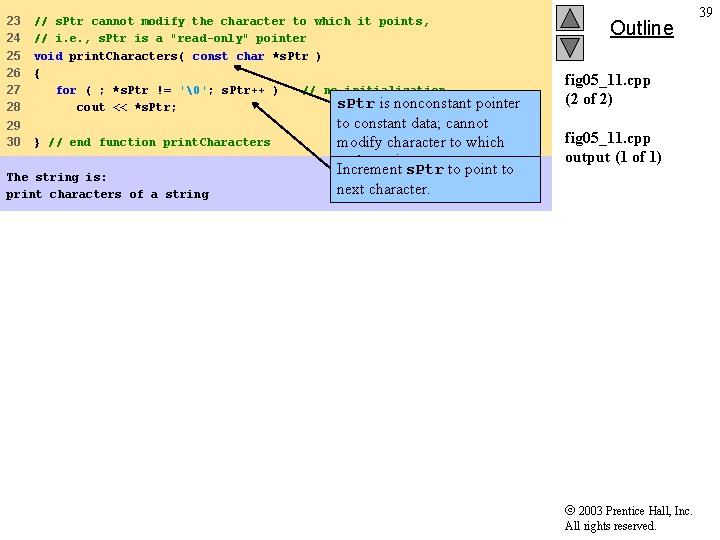
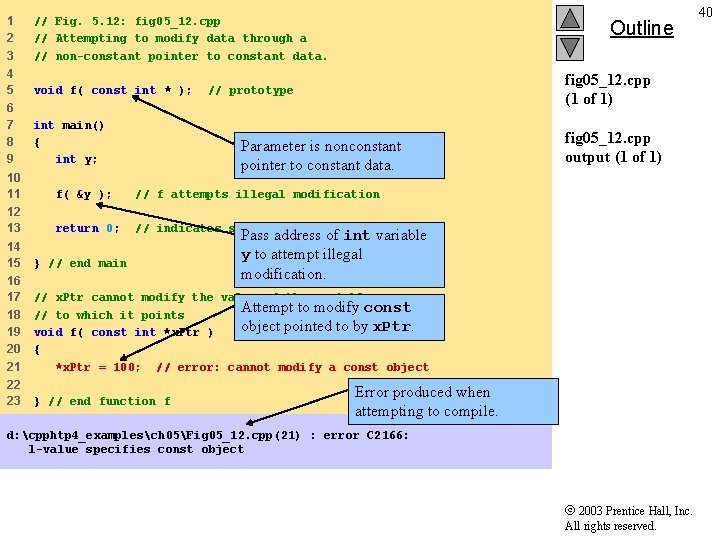
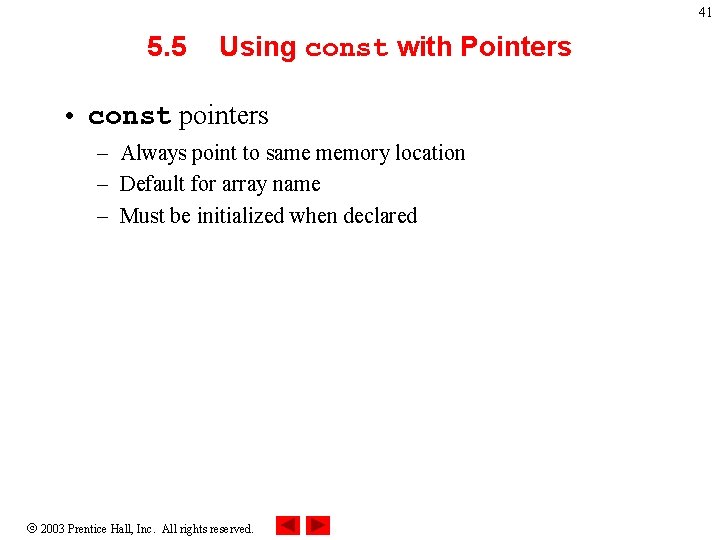
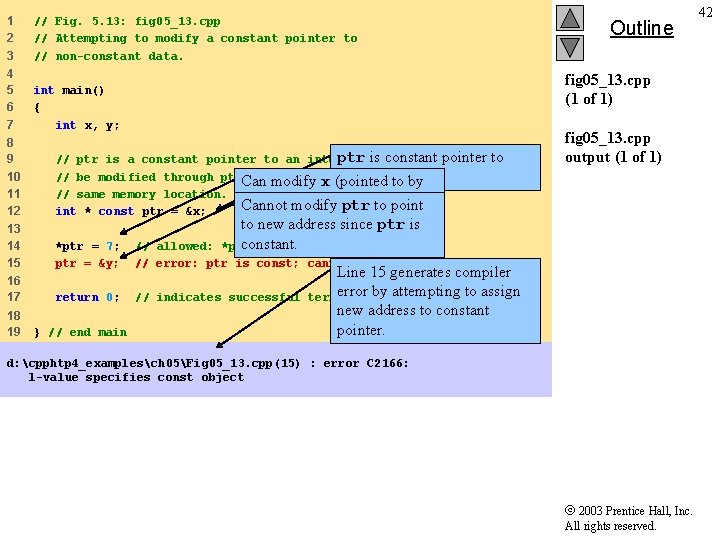
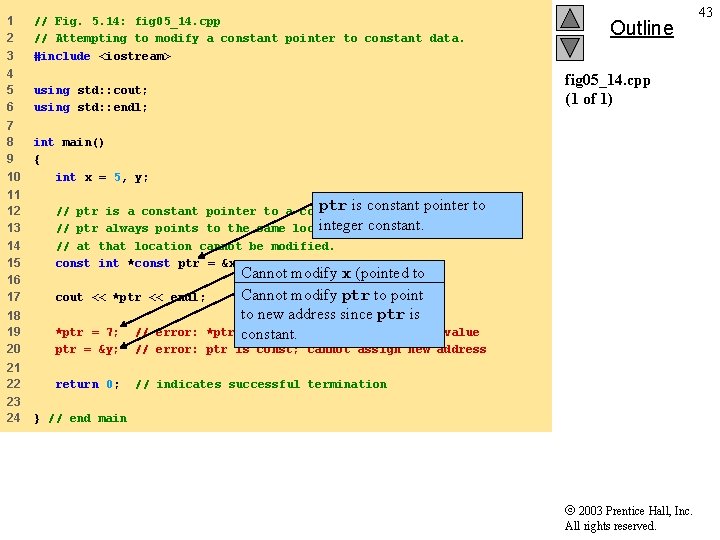
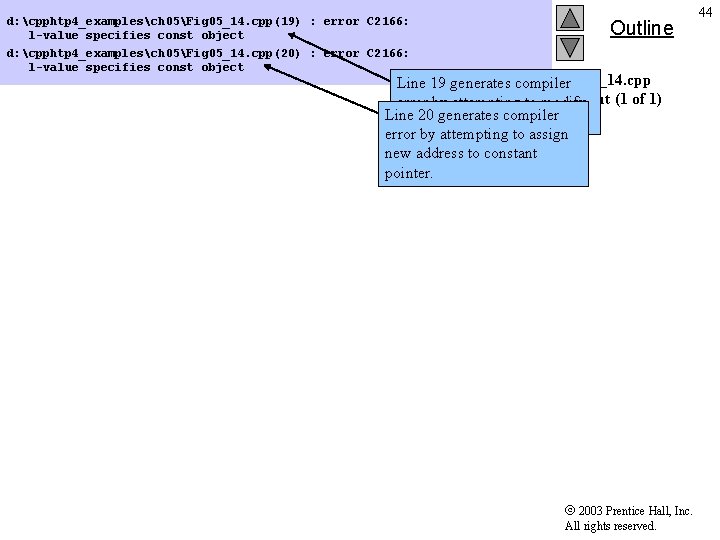
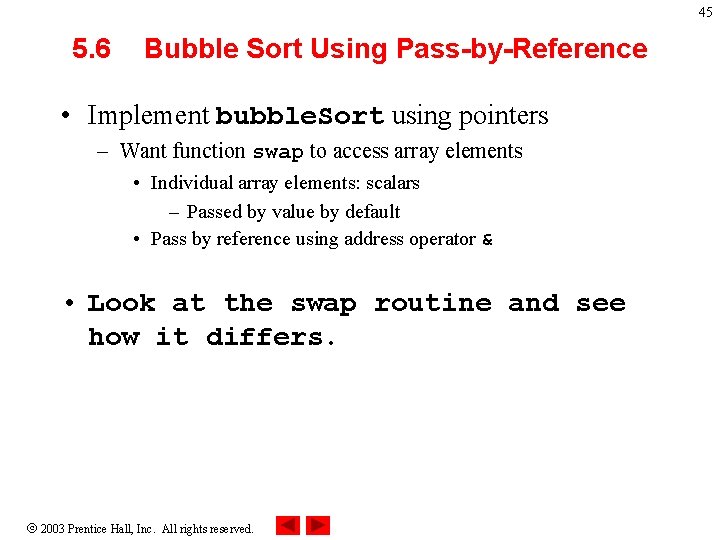
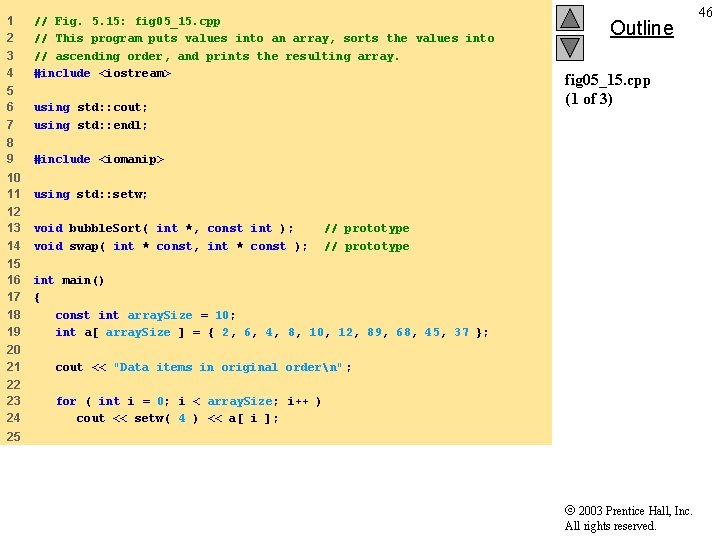
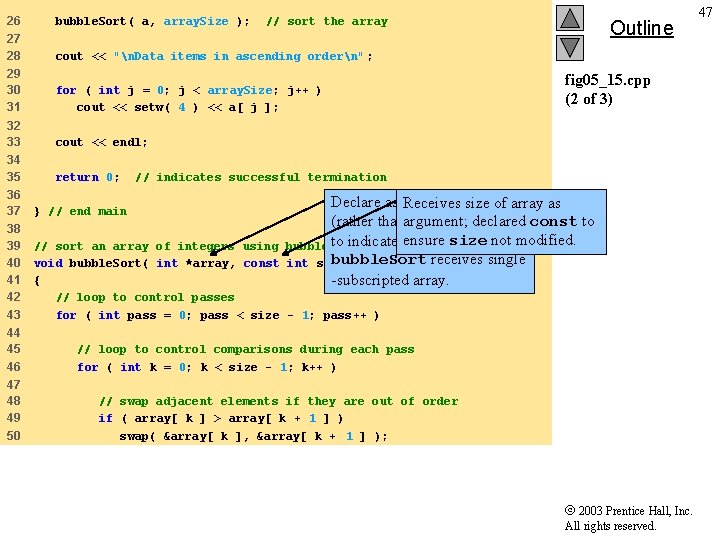
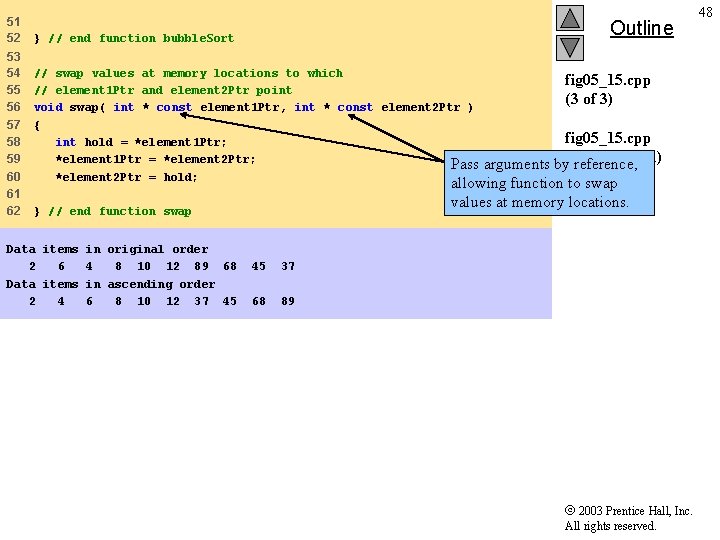
- Slides: 48
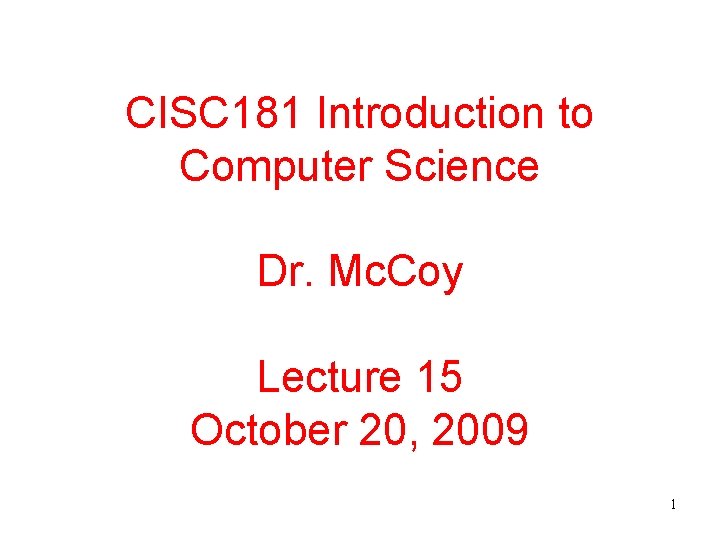
CISC 181 Introduction to Computer Science Dr. Mc. Coy Lecture 15 October 20, 2009 1
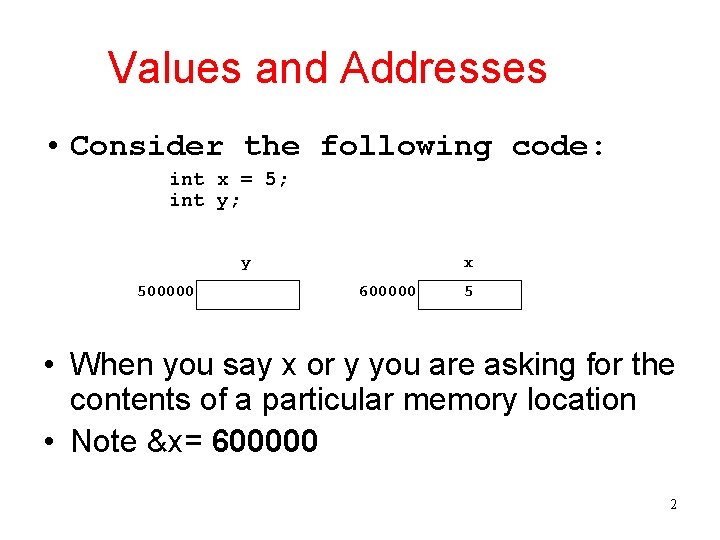
Values and Addresses • Consider the following code: int x = 5; int y; y 500000 x 600000 5 • When you say x or y you are asking for the contents of a particular memory location • Note &x= 600000 2
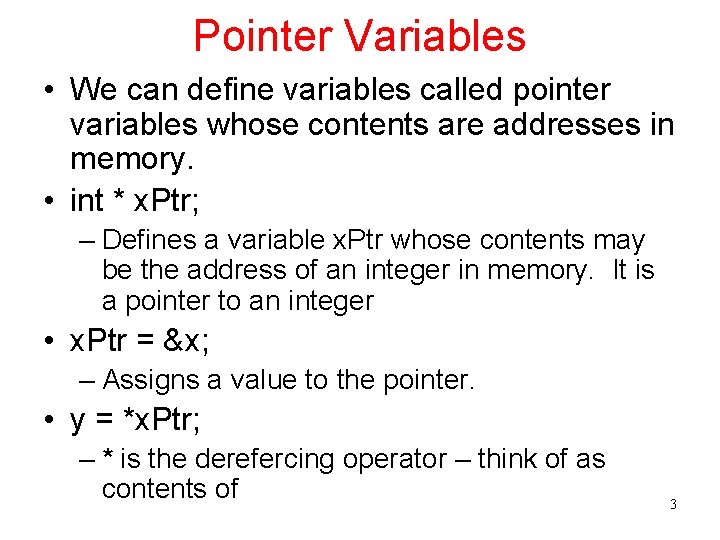
Pointer Variables • We can define variables called pointer variables whose contents are addresses in memory. • int * x. Ptr; – Defines a variable x. Ptr whose contents may be the address of an integer in memory. It is a pointer to an integer • x. Ptr = &x; – Assigns a value to the pointer. • y = *x. Ptr; – * is the derefercing operator – think of as contents of 3
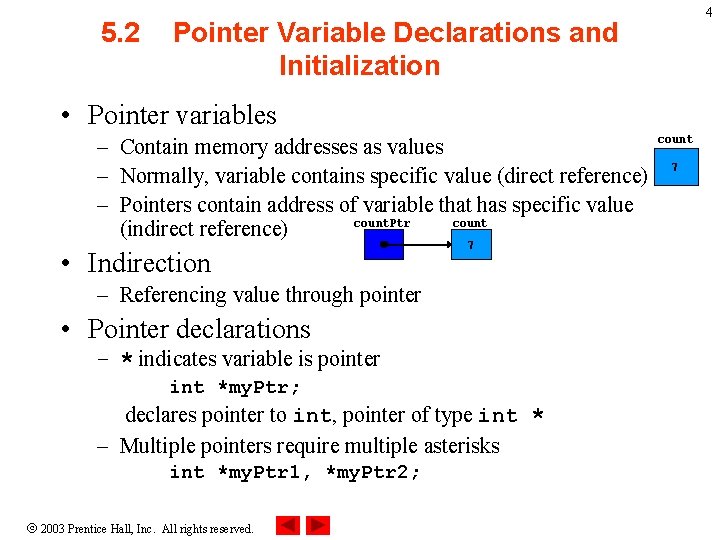
5. 2 4 Pointer Variable Declarations and Initialization • Pointer variables – Contain memory addresses as values – Normally, variable contains specific value (direct reference) – Pointers contain address of variable that has specific value count. Ptr (indirect reference) • Indirection 7 – Referencing value through pointer • Pointer declarations – * indicates variable is pointer int *my. Ptr; declares pointer to int, pointer of type int * – Multiple pointers require multiple asterisks int *my. Ptr 1, *my. Ptr 2; 2003 Prentice Hall, Inc. All rights reserved. count 7
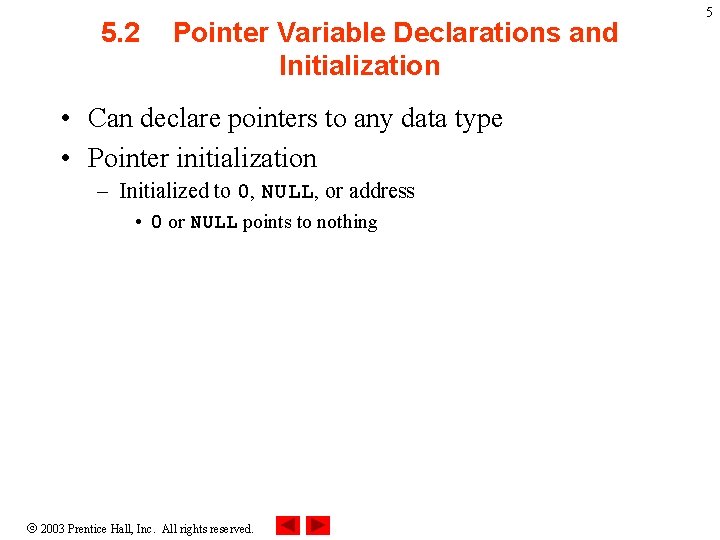
5. 2 Pointer Variable Declarations and Initialization • Can declare pointers to any data type • Pointer initialization – Initialized to 0, NULL, or address • 0 or NULL points to nothing 2003 Prentice Hall, Inc. All rights reserved. 5
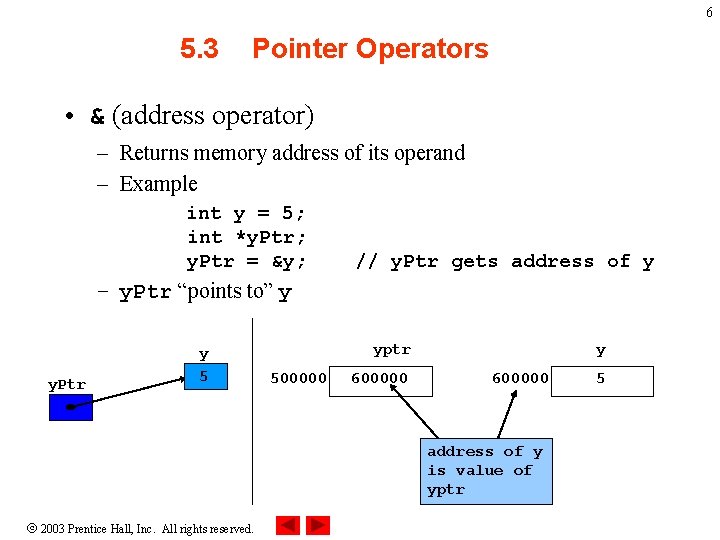
6 5. 3 Pointer Operators • & (address operator) – Returns memory address of its operand – Example int y = 5; int *y. Ptr; y. Ptr = &y; // y. Ptr gets address of y – y. Ptr “points to” y y. Ptr y 5 yptr 500000 600000 y 600000 address of y is value of yptr 2003 Prentice Hall, Inc. All rights reserved. 5
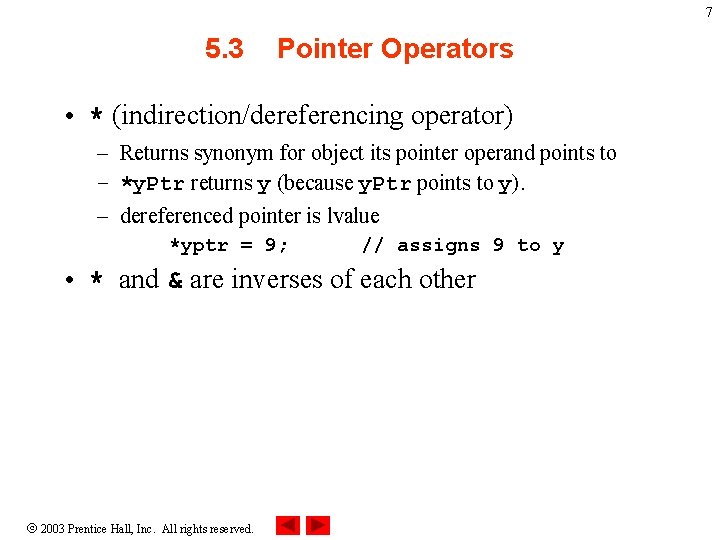
7 5. 3 Pointer Operators • * (indirection/dereferencing operator) – Returns synonym for object its pointer operand points to – *y. Ptr returns y (because y. Ptr points to y). – dereferenced pointer is lvalue *yptr = 9; // assigns 9 to y • * and & are inverses of each other 2003 Prentice Hall, Inc. All rights reserved.
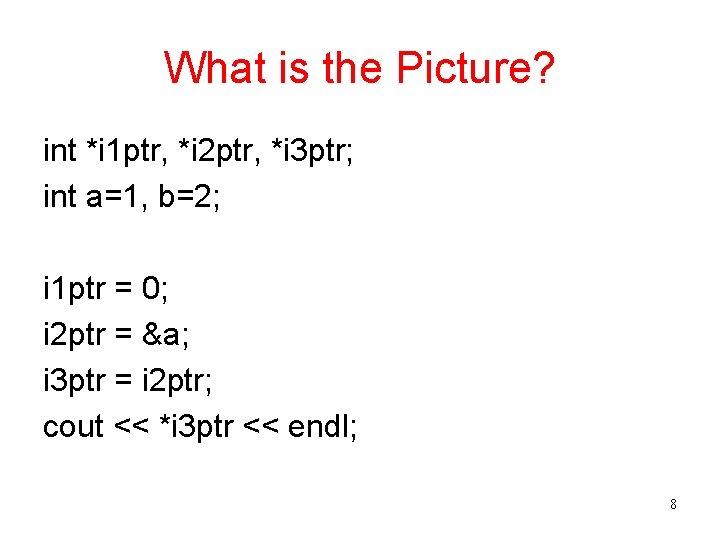
What is the Picture? int *i 1 ptr, *i 2 ptr, *i 3 ptr; int a=1, b=2; i 1 ptr = 0; i 2 ptr = &a; i 3 ptr = i 2 ptr; cout << *i 3 ptr << endl; 8
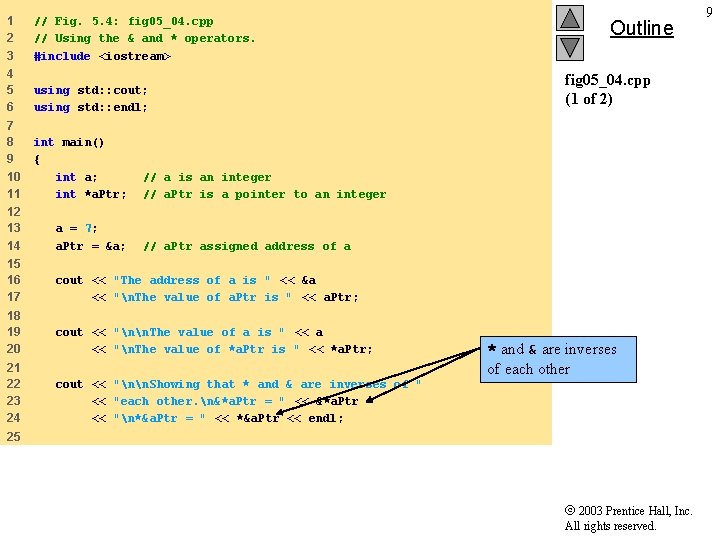
1 2 3 // Fig. 5. 4: fig 05_04. cpp // Using the & and * operators. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 9 10 11 int main() { int a; int *a. Ptr; 12 13 14 a = 7; a. Ptr = &a; 15 16 17 cout << "The address of a is " << &a << "n. The value of a. Ptr is " << a. Ptr; 18 19 20 cout << "nn. The value of a is " << a << "n. The value of *a. Ptr is " << *a. Ptr; 21 22 23 24 cout << "nn. Showing that * and & are inverses of " << "each other. n&*a. Ptr = " << &*a. Ptr << "n*&a. Ptr = " << *&a. Ptr << endl; Outline fig 05_04. cpp (1 of 2) // a is an integer // a. Ptr is a pointer to an integer // a. Ptr assigned address of a * and & are inverses of each other 25 2003 Prentice Hall, Inc. All rights reserved. 9
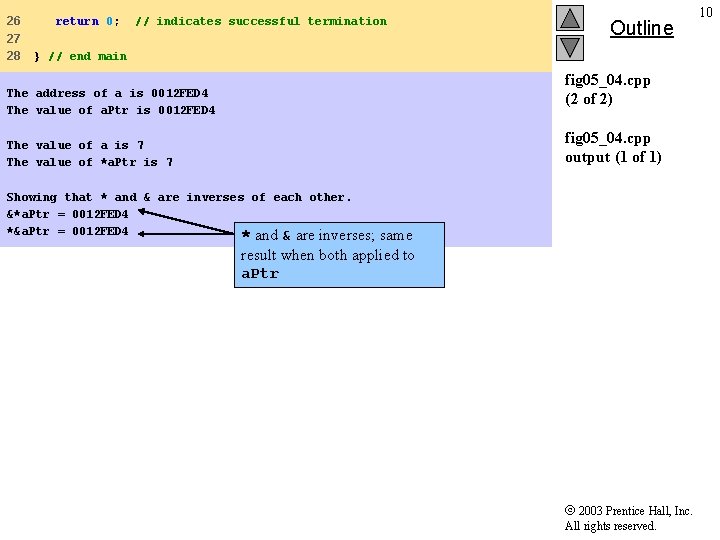
26 27 28 return 0; // indicates successful termination Outline } // end main fig 05_04. cpp (2 of 2) The address of a is 0012 FED 4 The value of a. Ptr is 0012 FED 4 fig 05_04. cpp output (1 of 1) The value of a is 7 The value of *a. Ptr is 7 Showing that * and & are inverses of each other. &*a. Ptr = 0012 FED 4 *&a. Ptr = 0012 FED 4 * and & are inverses; same result when both applied to a. Ptr 2003 Prentice Hall, Inc. All rights reserved. 10
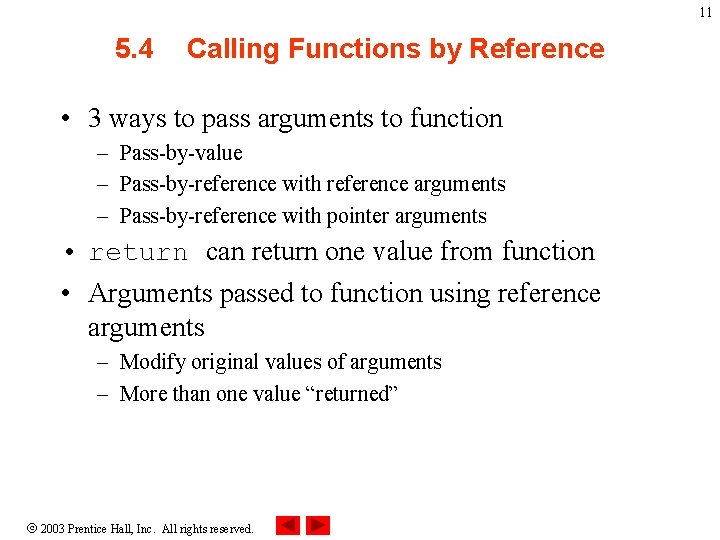
11 5. 4 Calling Functions by Reference • 3 ways to pass arguments to function – Pass-by-value – Pass-by-reference with reference arguments – Pass-by-reference with pointer arguments • return can return one value from function • Arguments passed to function using reference arguments – Modify original values of arguments – More than one value “returned” 2003 Prentice Hall, Inc. All rights reserved.
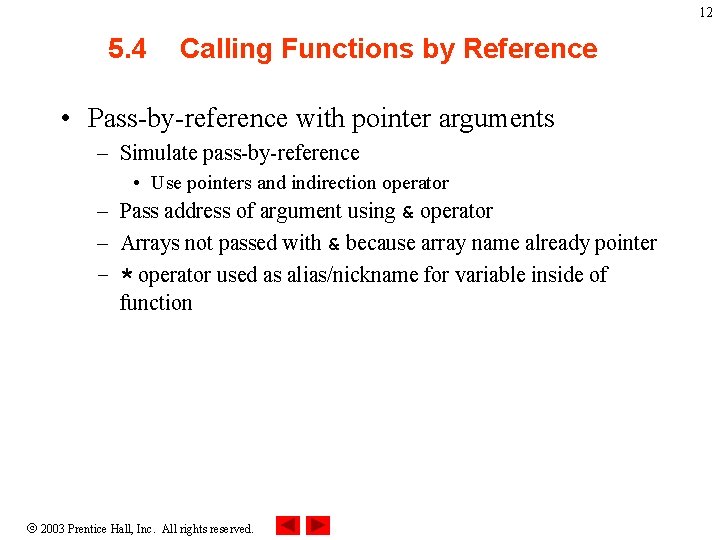
12 5. 4 Calling Functions by Reference • Pass-by-reference with pointer arguments – Simulate pass-by-reference • Use pointers and indirection operator – Pass address of argument using & operator – Arrays not passed with & because array name already pointer – * operator used as alias/nickname for variable inside of function 2003 Prentice Hall, Inc. All rights reserved.
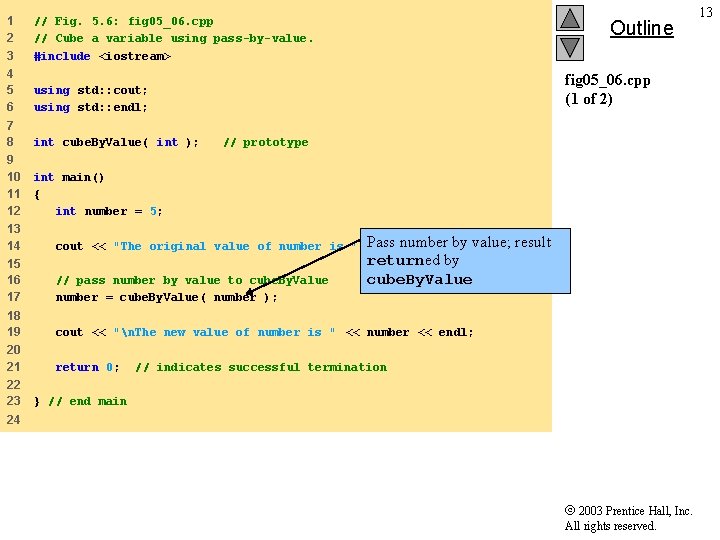
1 2 3 // Fig. 5. 6: fig 05_06. cpp // Cube a variable using pass-by-value. #include <iostream> 4 5 6 using std: : cout; using std: : endl; 7 8 int cube. By. Value( int ); 9 10 11 12 int main() { int number = 5; Outline fig 05_06. cpp (1 of 2) // prototype 13 14 number cout << "The original value of number is " Pass << number; 15 16 17 // pass number by value to cube. By. Value number = cube. By. Value( number ); 18 19 cout << "n. The new value of number is " << number << endl; 20 21 return 0; 22 23 by value; result returned by cube. By. Value // indicates successful termination } // end main 24 2003 Prentice Hall, Inc. All rights reserved. 13
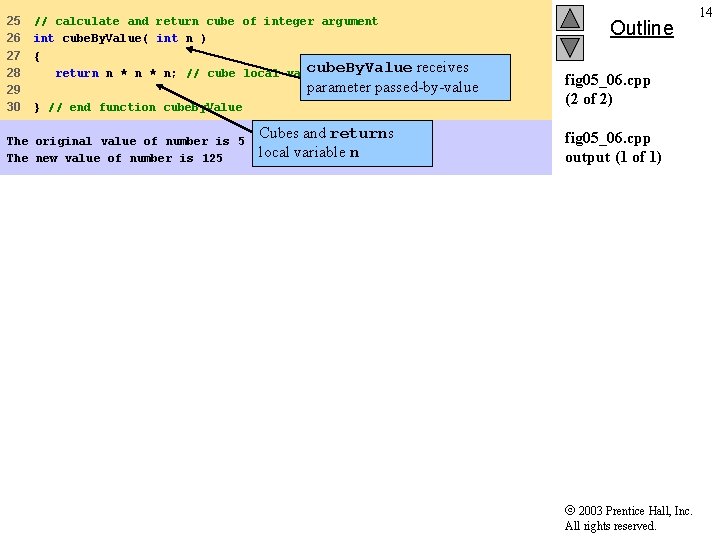
25 26 27 28 29 30 // calculate and return cube of integer argument int cube. By. Value( int n ) { cube. By. Value receives return n * n; // cube local variable n and return result parameter passed-by-value } // end function cube. By. Value The original value of number is 5 The new value of number is 125 Cubes and returns local variable n Outline fig 05_06. cpp (2 of 2) fig 05_06. cpp output (1 of 1) 2003 Prentice Hall, Inc. All rights reserved. 14
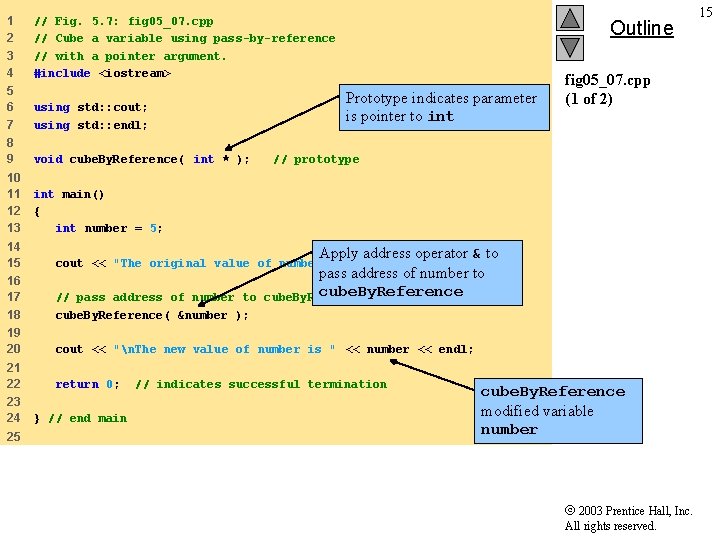
1 2 3 4 // Fig. 5. 7: fig 05_07. cpp // Cube a variable using pass-by-reference // with a pointer argument. #include <iostream> 5 6 7 using std: : cout; using std: : endl; 8 9 void cube. By. Reference( int * ); 10 11 12 13 int main() { int number = 5; Outline Prototype indicates parameter is pointer to int // prototype 14 15 cout << "The original value of number is " << number; 16 17 18 // pass address of number to cube. By. Reference( &number ); 19 20 cout << "n. The new value of number is " << number << endl; 21 22 return 0; 23 24 25 } // end main fig 05_07. cpp (1 of 2) Apply address operator & to pass address of number to cube. By. Reference // indicates successful termination cube. By. Reference modified variable number 2003 Prentice Hall, Inc. All rights reserved. 15
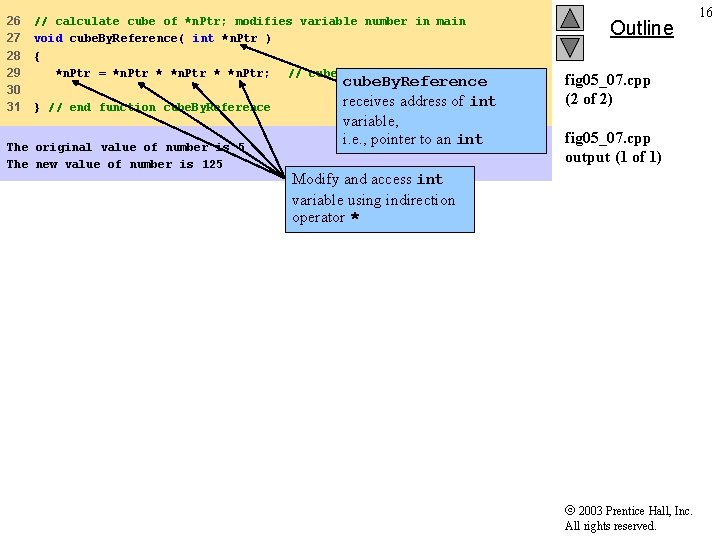
26 27 28 29 30 31 // calculate cube of *n. Ptr; modifies variable number in main void cube. By. Reference( int *n. Ptr ) { *n. Ptr = *n. Ptr * *n. Ptr; // cube *n. Ptr } // end function cube. By. Reference The original value of number is 5 The new value of number is 125 cube. By. Reference receives address of int variable, i. e. , pointer to an int Outline fig 05_07. cpp (2 of 2) fig 05_07. cpp output (1 of 1) Modify and access int variable using indirection operator * 2003 Prentice Hall, Inc. All rights reserved. 16
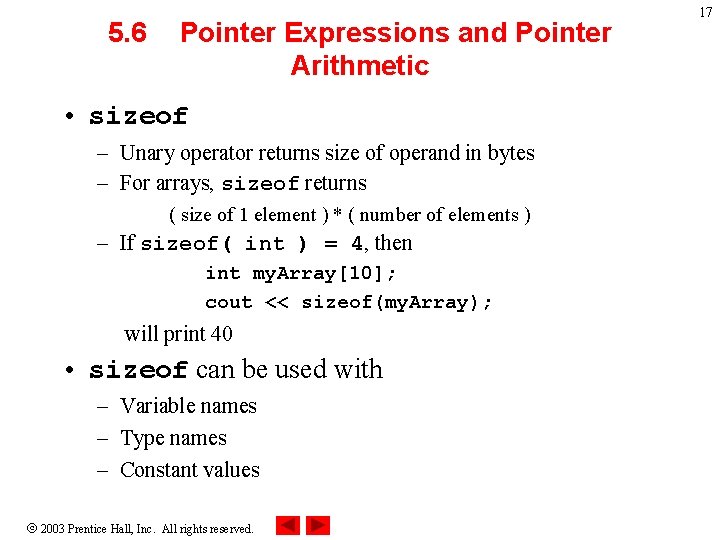
5. 6 Pointer Expressions and Pointer Arithmetic • sizeof – Unary operator returns size of operand in bytes – For arrays, sizeof returns ( size of 1 element ) * ( number of elements ) – If sizeof( int ) = 4, then int my. Array[10]; cout << sizeof(my. Array); will print 40 • sizeof can be used with – Variable names – Type names – Constant values 2003 Prentice Hall, Inc. All rights reserved. 17
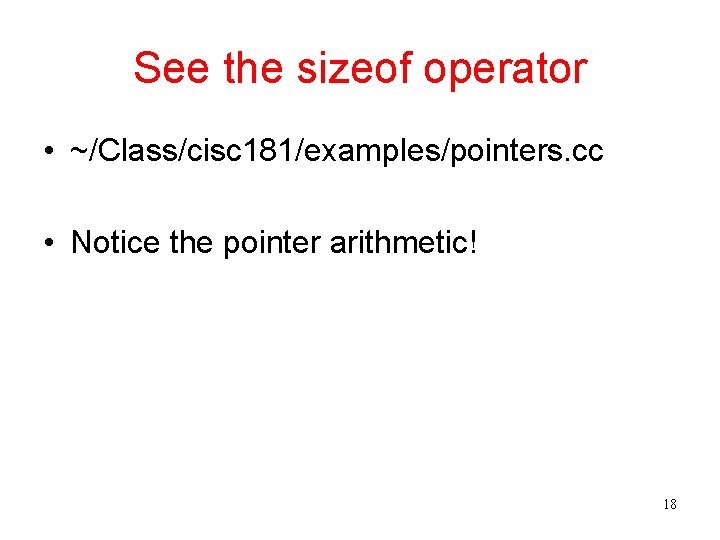
See the sizeof operator • ~/Class/cisc 181/examples/pointers. cc • Notice the pointer arithmetic! 18
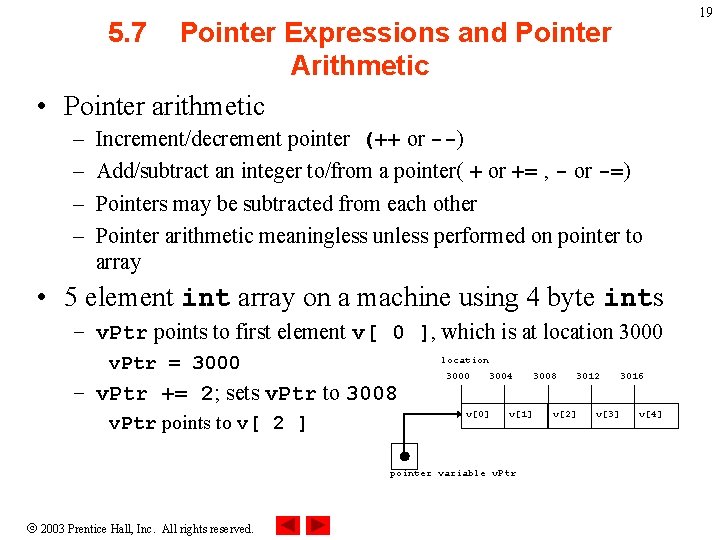
19 5. 7 Pointer Expressions and Pointer Arithmetic • Pointer arithmetic – – Increment/decrement pointer (++ or --) Add/subtract an integer to/from a pointer( + or += , - or -=) Pointers may be subtracted from each other Pointer arithmetic meaningless unless performed on pointer to array • 5 element int array on a machine using 4 byte ints – v. Ptr points to first element v[ 0 ], which is at location 3000 v. Ptr = 3000 location – v. Ptr += 2; sets v. Ptr to 3008 v. Ptr points to v[ 2 ] 3000 3004 v[0] v[1] pointer variable v. Ptr 2003 Prentice Hall, Inc. All rights reserved. 3008 3012 v[2] 3016 v[3] v[4]
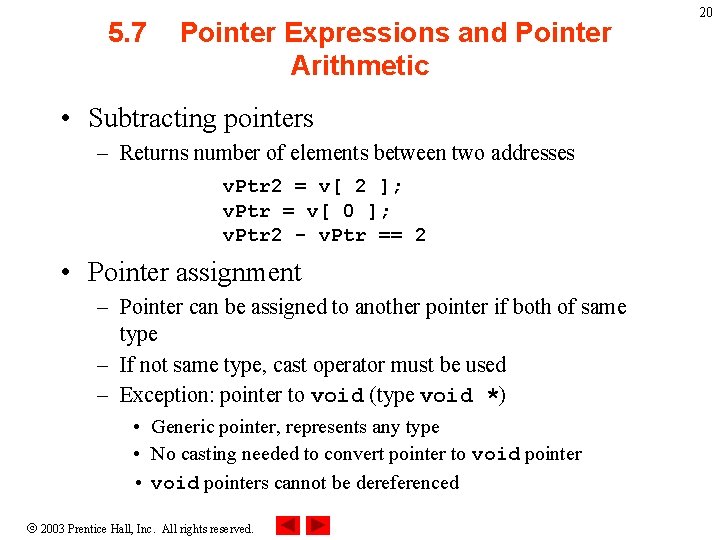
5. 7 Pointer Expressions and Pointer Arithmetic • Subtracting pointers – Returns number of elements between two addresses v. Ptr 2 = v[ 2 ]; v. Ptr = v[ 0 ]; v. Ptr 2 - v. Ptr == 2 • Pointer assignment – Pointer can be assigned to another pointer if both of same type – If not same type, cast operator must be used – Exception: pointer to void (type void *) • Generic pointer, represents any type • No casting needed to convert pointer to void pointer • void pointers cannot be dereferenced 2003 Prentice Hall, Inc. All rights reserved. 20
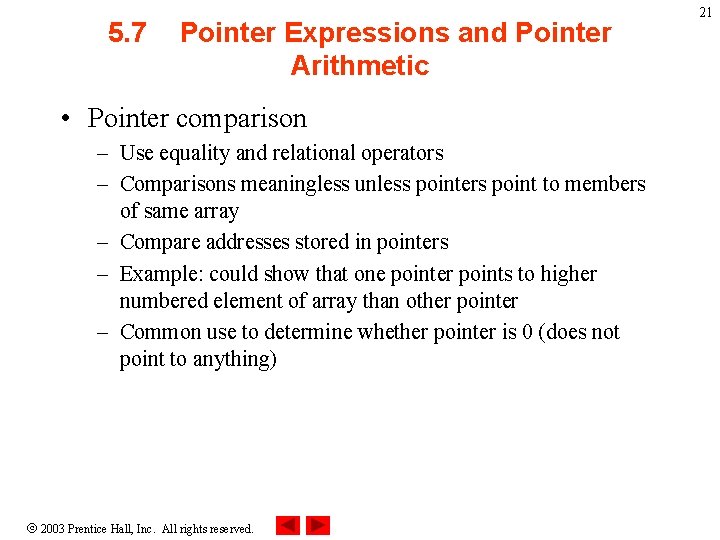
5. 7 Pointer Expressions and Pointer Arithmetic • Pointer comparison – Use equality and relational operators – Comparisons meaningless unless pointers point to members of same array – Compare addresses stored in pointers – Example: could show that one pointer points to higher numbered element of array than other pointer – Common use to determine whether pointer is 0 (does not point to anything) 2003 Prentice Hall, Inc. All rights reserved. 21
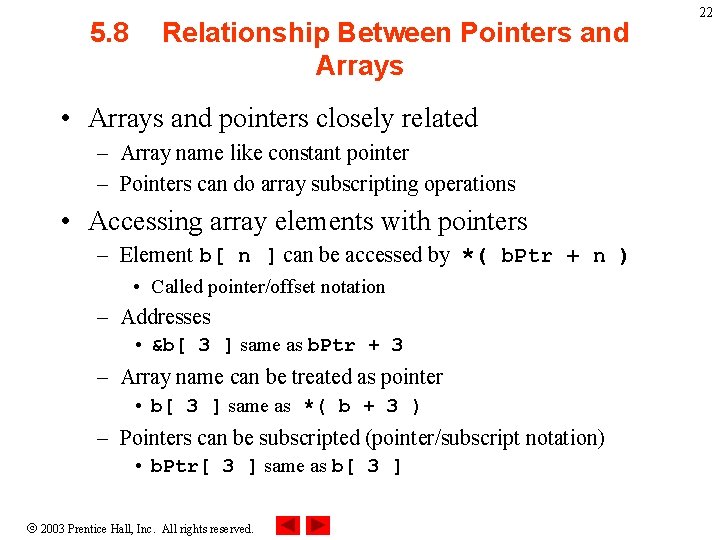
5. 8 Relationship Between Pointers and Arrays • Arrays and pointers closely related – Array name like constant pointer – Pointers can do array subscripting operations • Accessing array elements with pointers – Element b[ n ] can be accessed by *( b. Ptr + n ) • Called pointer/offset notation – Addresses • &b[ 3 ] same as b. Ptr + 3 – Array name can be treated as pointer • b[ 3 ] same as *( b + 3 ) – Pointers can be subscripted (pointer/subscript notation) • b. Ptr[ 3 ] same as b[ 3 ] 2003 Prentice Hall, Inc. All rights reserved. 22
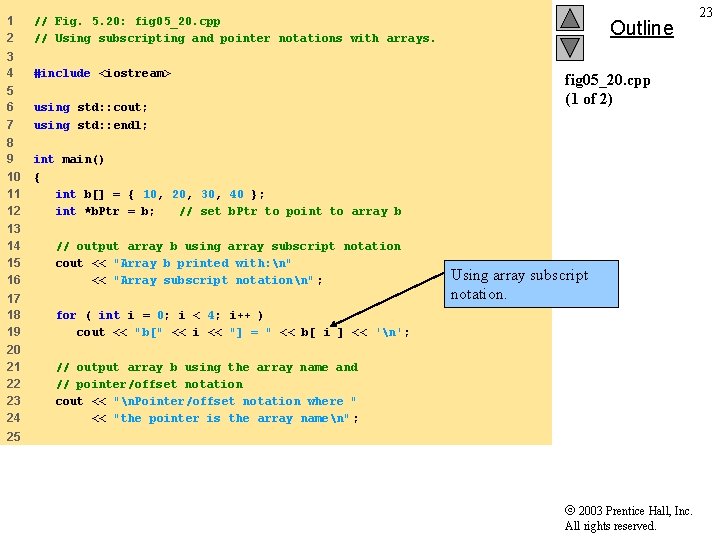
1 2 // Fig. 5. 20: fig 05_20. cpp // Using subscripting and pointer notations with arrays. 3 4 #include <iostream> 5 6 7 using std: : cout; using std: : endl; 8 9 10 11 12 int main() { int b[] = { 10, 20, 30, 40 }; int *b. Ptr = b; // set b. Ptr to point to array b 13 14 15 16 // output array b using array subscript notation cout << "Array b printed with: n" << "Array subscript notationn" ; 17 18 19 for ( int i = 0; i < 4; i++ ) cout << "b[" << i << "] = " << b[ i ] << 'n'; 20 21 22 23 24 // output array b using the array name and // pointer/offset notation cout << "n. Pointer/offset notation where " << "the pointer is the array namen" ; Outline fig 05_20. cpp (1 of 2) Using array subscript notation. 25 2003 Prentice Hall, Inc. All rights reserved. 23
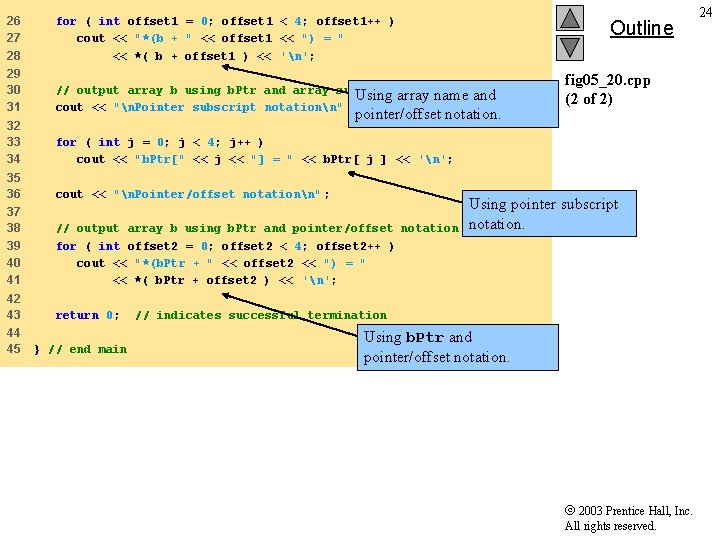
26 27 28 for ( int offset 1 = 0; offset 1 < 4; offset 1++ ) cout << "*(b + " << offset 1 << ") = " << *( b + offset 1 ) << 'n'; 29 30 31 // output array b using b. Ptr and array subscript notation Using array name cout << "n. Pointer subscript notationn" ; 32 33 34 for ( int j = 0; j < 4; j++ ) cout << "b. Ptr[" << j << "] = " << b. Ptr[ j ] << 'n'; 35 36 cout << "n. Pointer/offset notationn" ; 37 38 39 40 41 // output array b using b. Ptr and pointer/offset notation for ( int offset 2 = 0; offset 2 < 4; offset 2++ ) cout << "*(b. Ptr + " << offset 2 << ") = " << *( b. Ptr + offset 2 ) << 'n'; 42 43 return 0; 44 45 Outline and pointer/offset notation. } // end main fig 05_20. cpp (2 of 2) Using pointer subscript notation. // indicates successful termination Using b. Ptr and pointer/offset notation. 2003 Prentice Hall, Inc. All rights reserved. 24
![Array b printed with Array subscript notation b0 10 b1 20 b2 Array b printed with: Array subscript notation b[0] = 10 b[1] = 20 b[2]](https://slidetodoc.com/presentation_image_h2/63508d25c6a14ae040b0a1a3f943e14f/image-25.jpg)
Array b printed with: Array subscript notation b[0] = 10 b[1] = 20 b[2] = 30 b[3] = 40 Outline fig 05_20. cpp output (1 of 1) Pointer/offset notation where the pointer is the array name *(b + 0) = 10 *(b + 1) = 20 *(b + 2) = 30 *(b + 3) = 40 Pointer b. Ptr[0] b. Ptr[1] b. Ptr[2] b. Ptr[3] subscript notation = 10 = 20 = 30 = 40 Pointer/offset notation *(b. Ptr + 0) = 10 *(b. Ptr + 1) = 20 *(b. Ptr + 2) = 30 *(b. Ptr + 3) = 40 2003 Prentice Hall, Inc. All rights reserved. 25
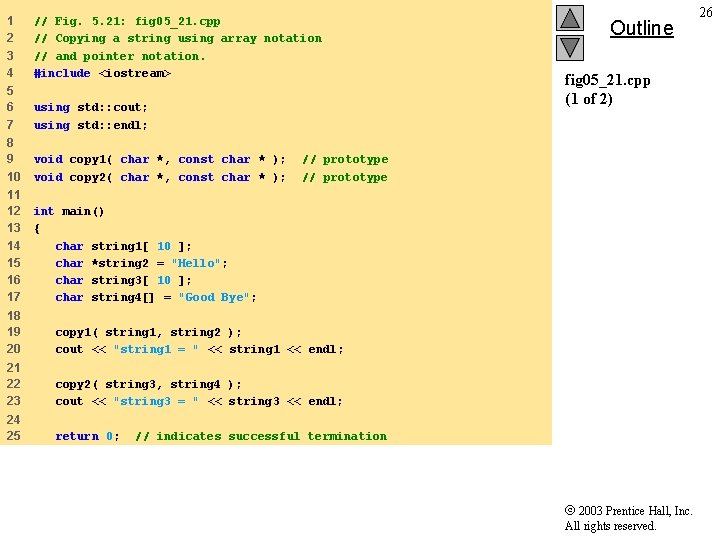
1 2 3 4 // Fig. 5. 21: fig 05_21. cpp // Copying a string using array notation // and pointer notation. #include <iostream> 5 6 7 using std: : cout; using std: : endl; 8 9 10 void copy 1( char *, const char * ); void copy 2( char *, const char * ); 11 12 13 14 15 16 17 int main() { char string 1[ 10 ]; char *string 2 = "Hello"; char string 3[ 10 ]; char string 4[] = "Good Bye"; Outline fig 05_21. cpp (1 of 2) // prototype 18 19 20 copy 1( string 1, string 2 ); cout << "string 1 = " << string 1 << endl; 21 22 23 copy 2( string 3, string 4 ); cout << "string 3 = " << string 3 << endl; 24 25 return 0; // indicates successful termination 2003 Prentice Hall, Inc. All rights reserved. 26
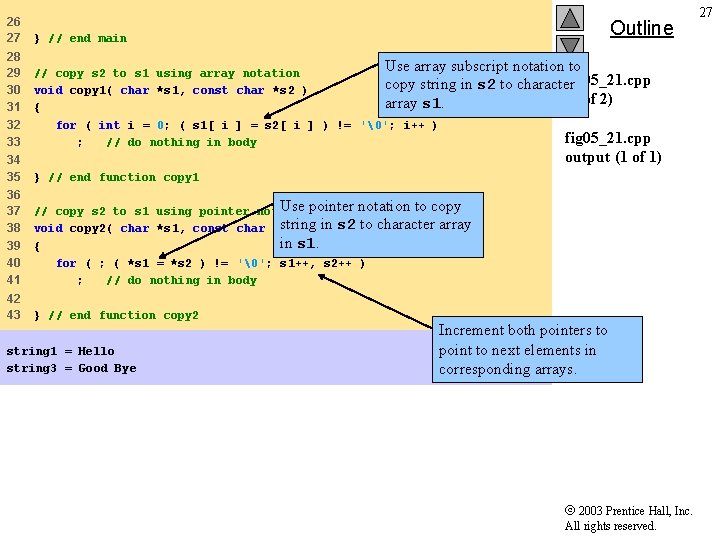
26 27 } // end main 28 29 30 31 32 33 Use array subscript notation to // copy s 2 to s 1 using array notation fig 05_21. cpp copy string in s 2 to character void copy 1( char *s 1, const char *s 2 ) (2 of 2) array s 1. { for ( int i = 0; ( s 1[ i ] = s 2[ i ] ) != '