CHAPTER FIVE Classes Classes a class is like
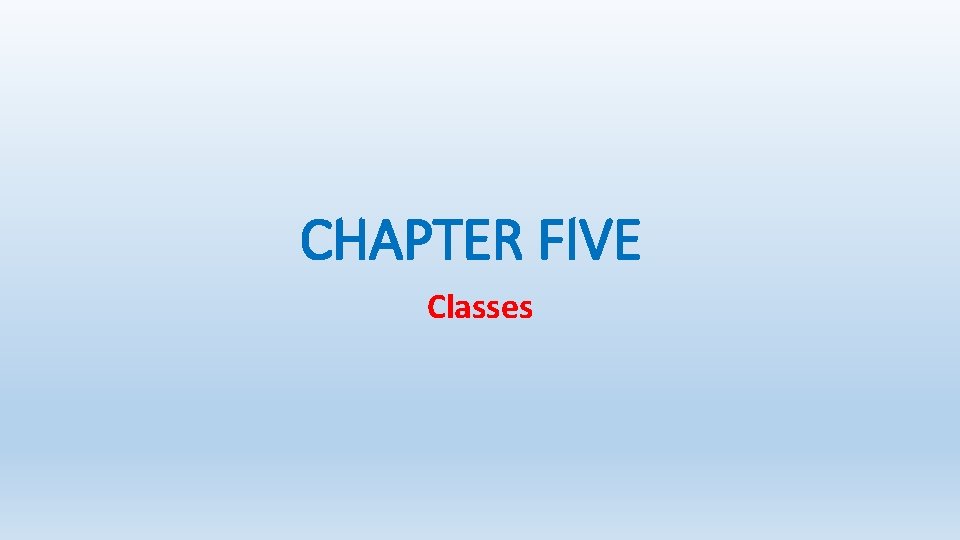
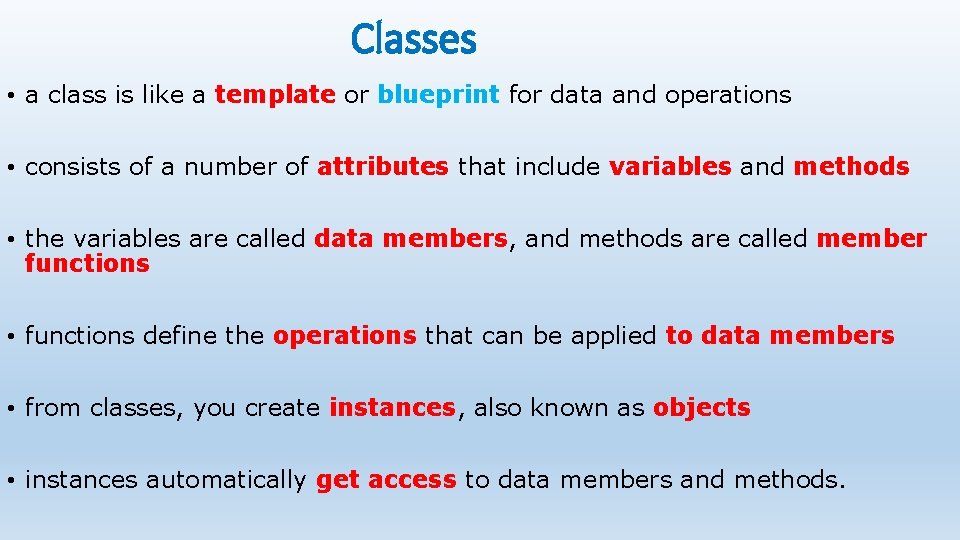
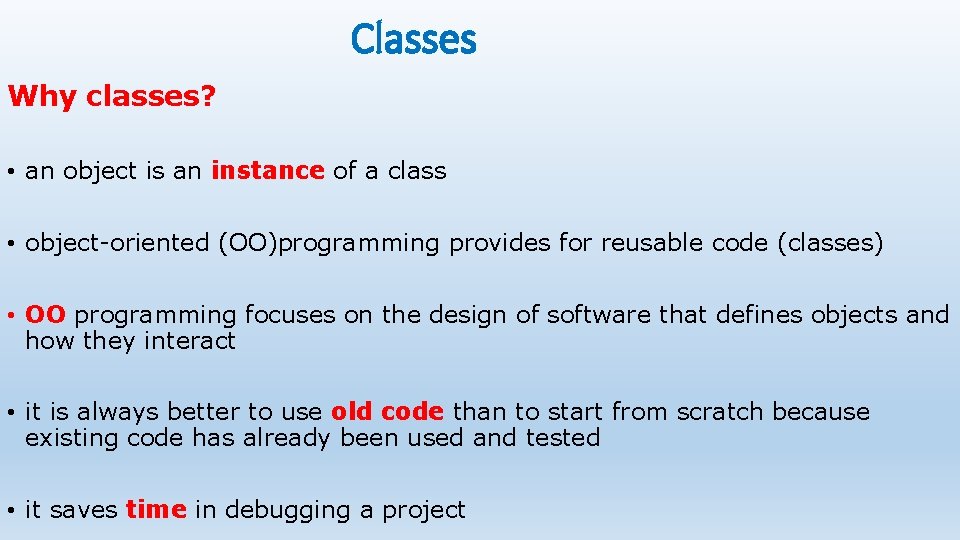
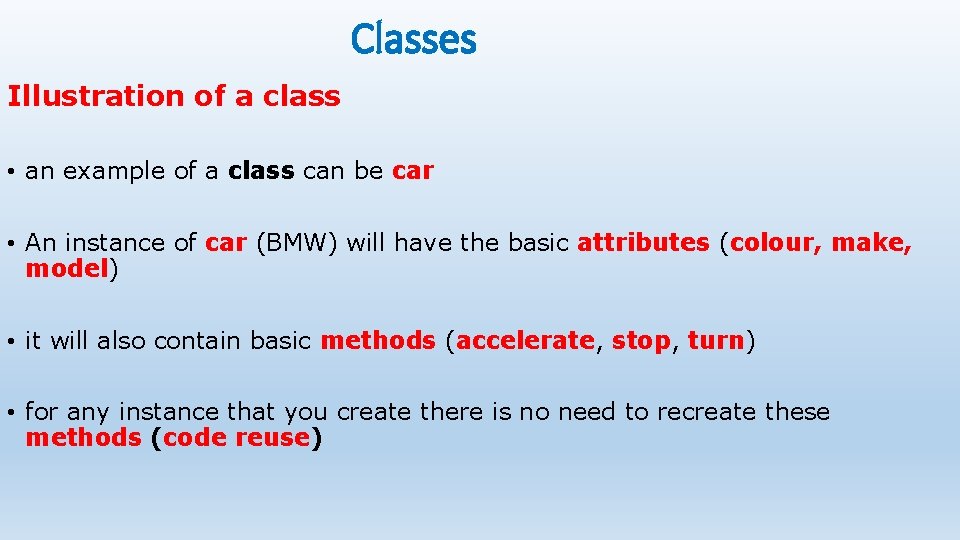
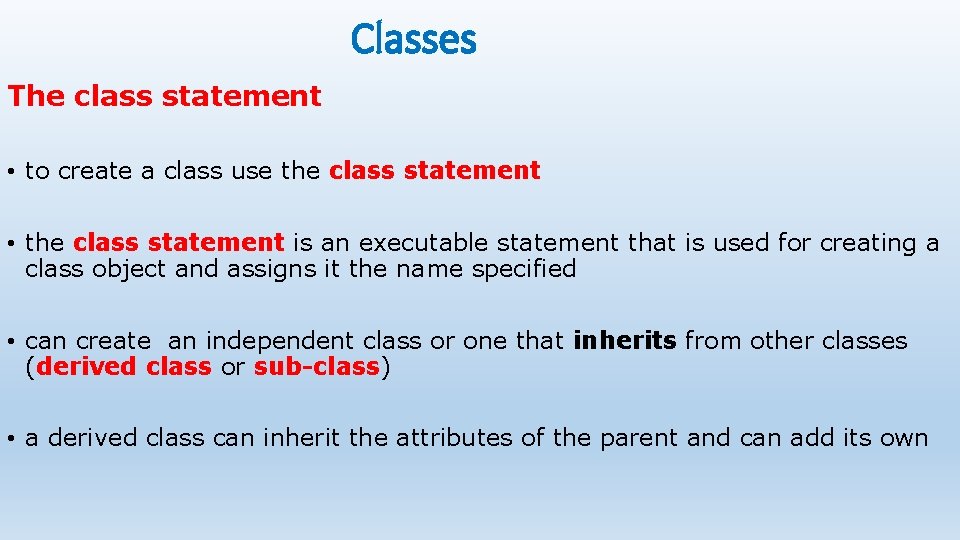
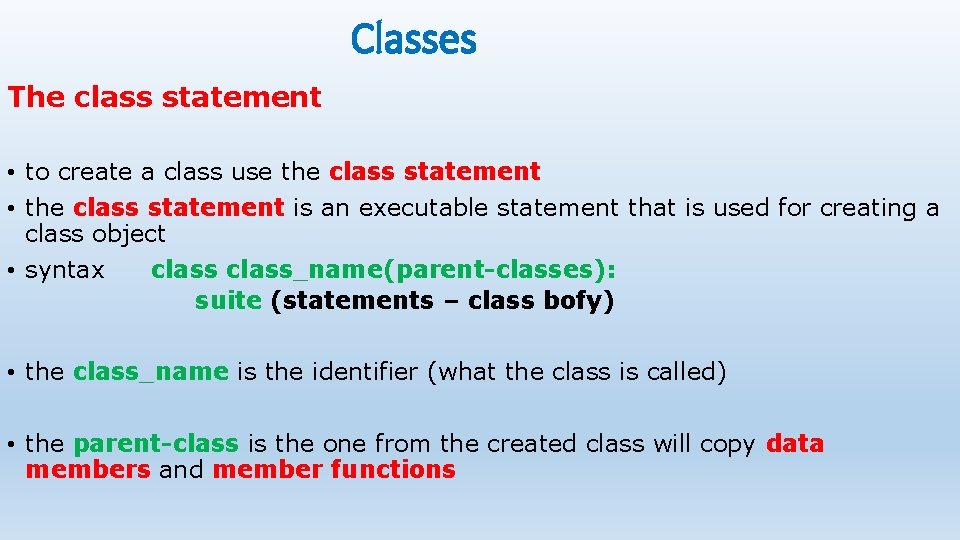
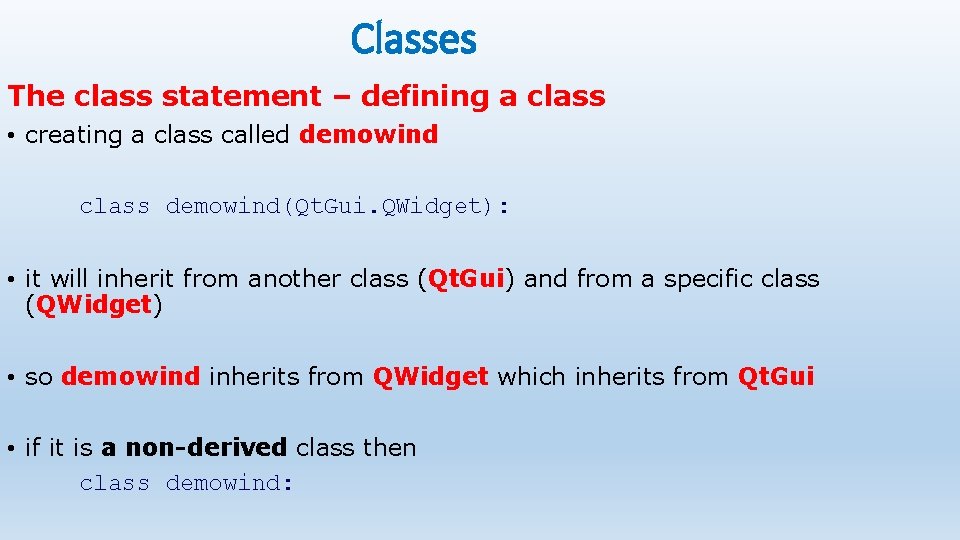
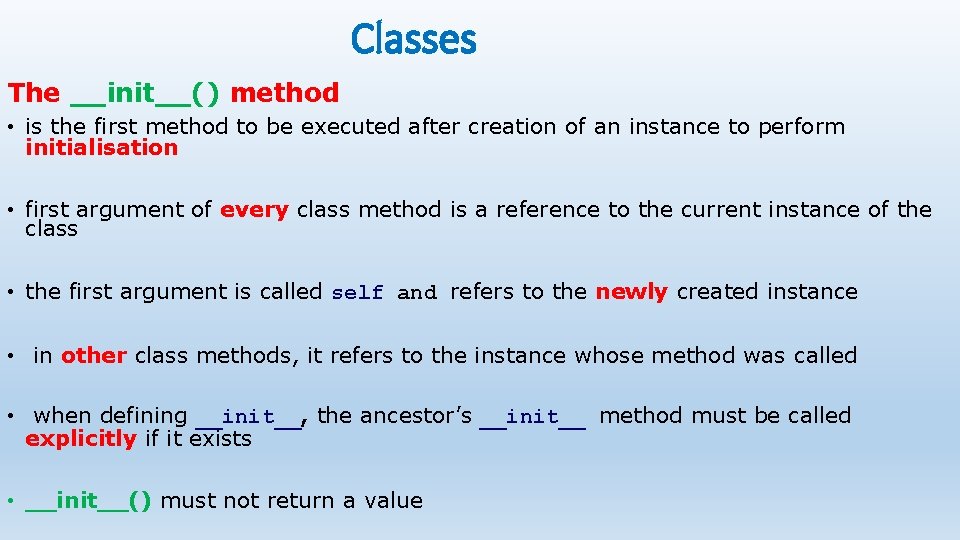
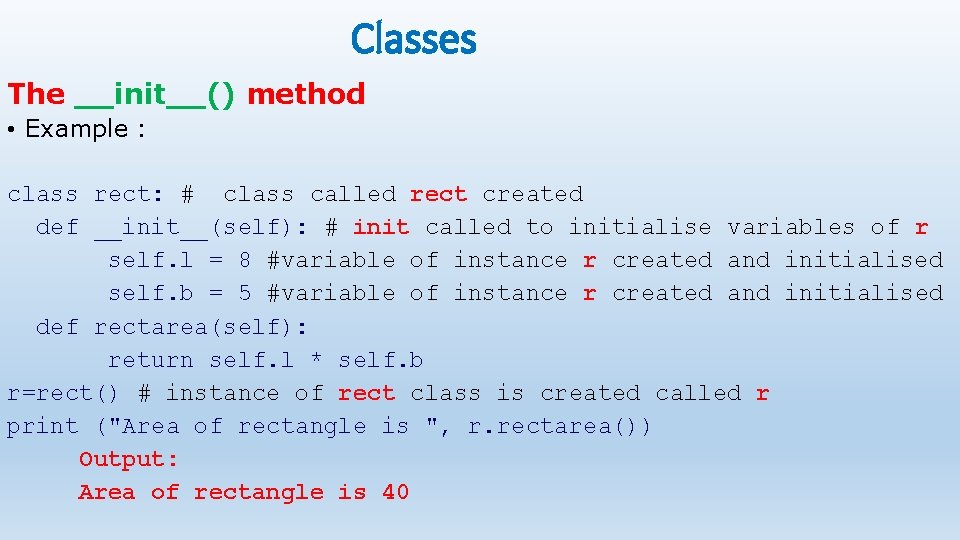
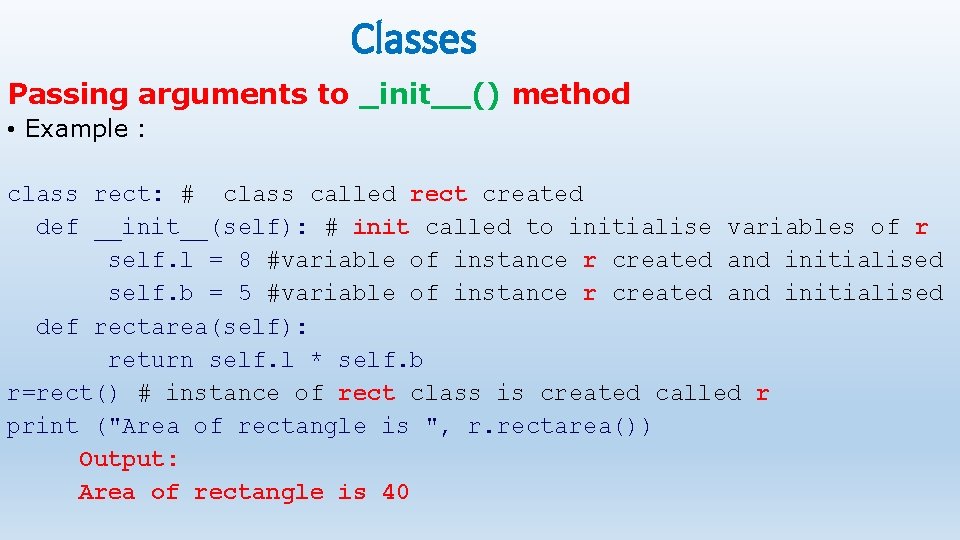
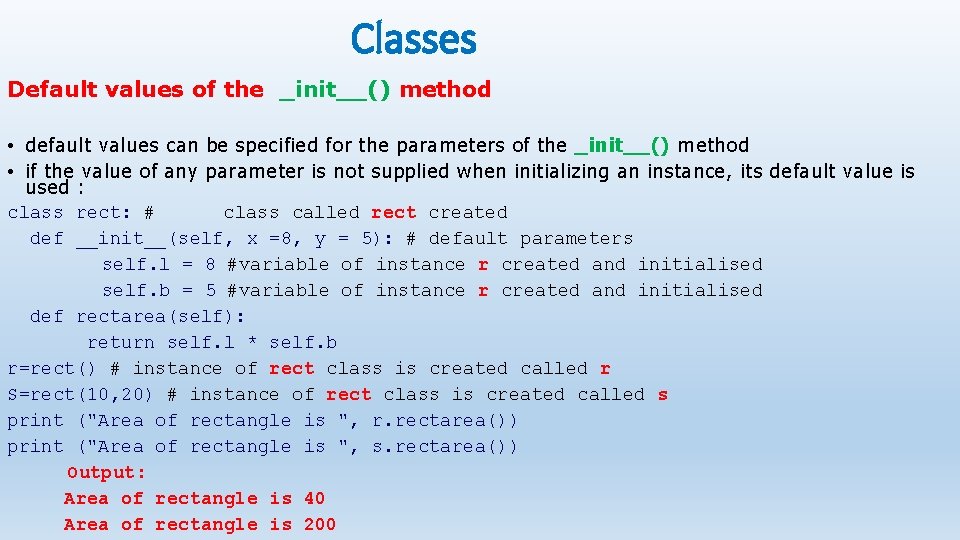
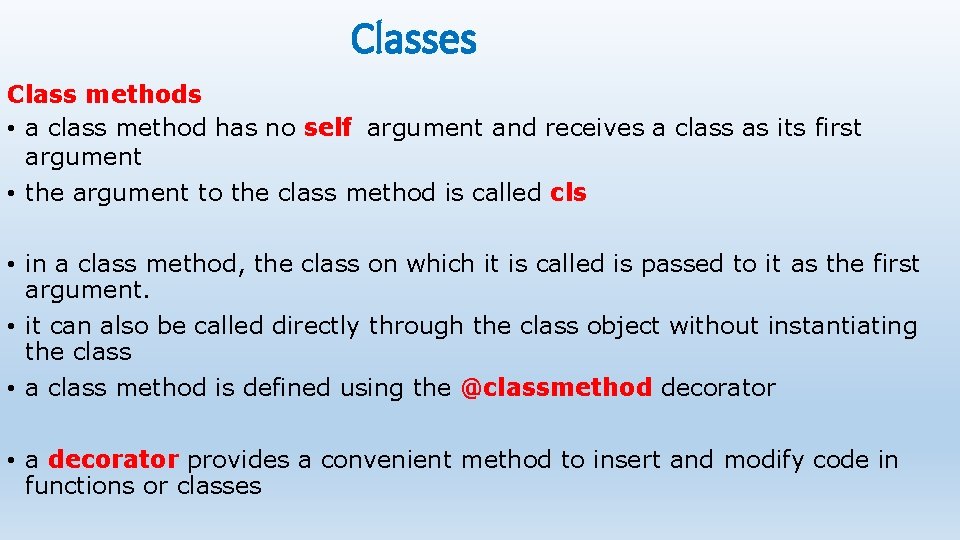
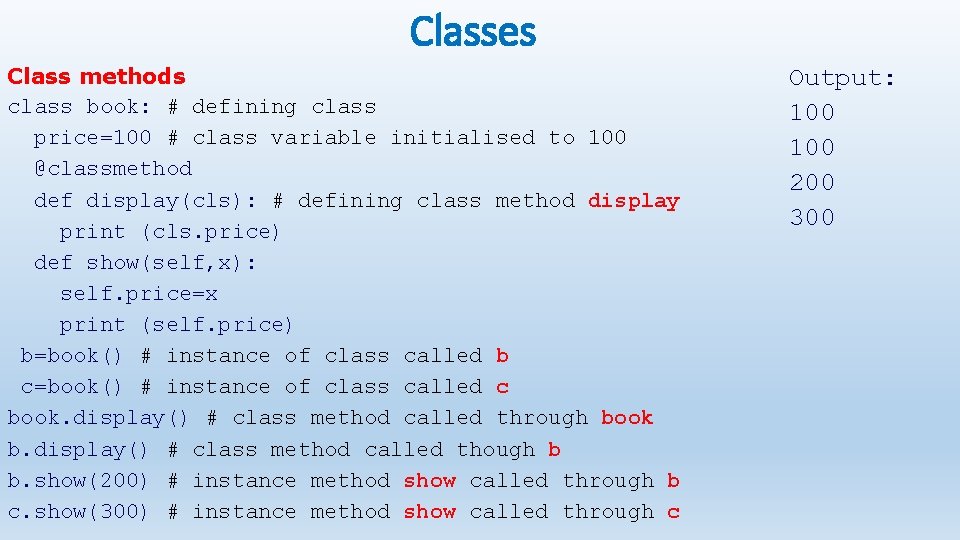
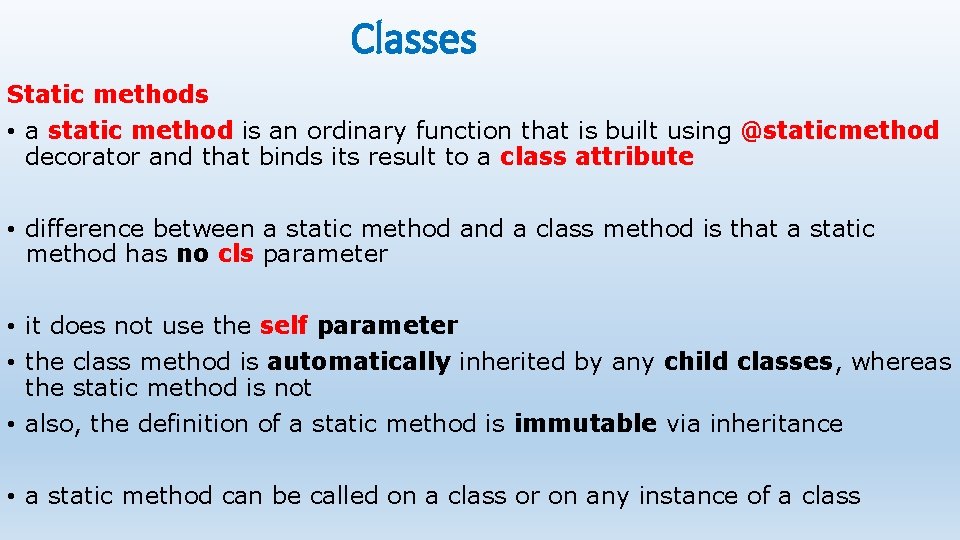
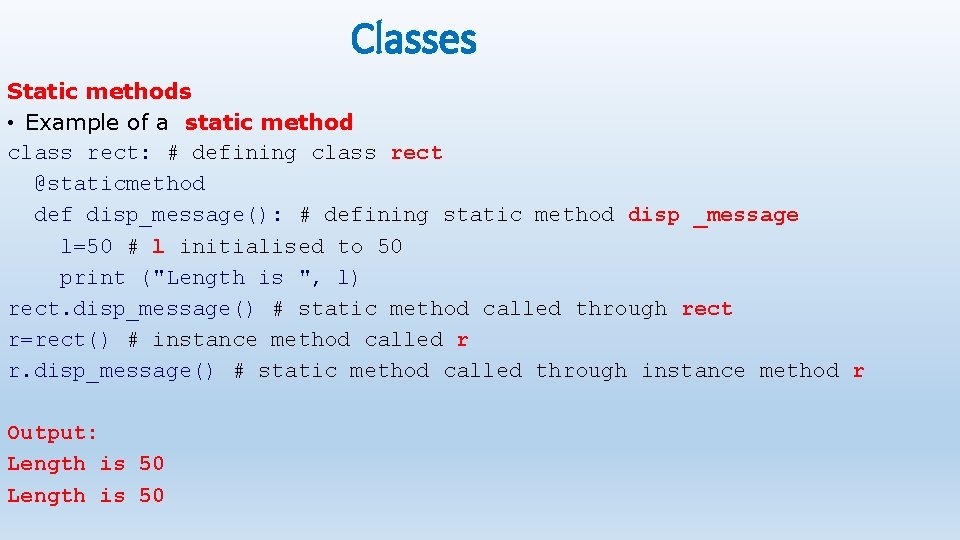
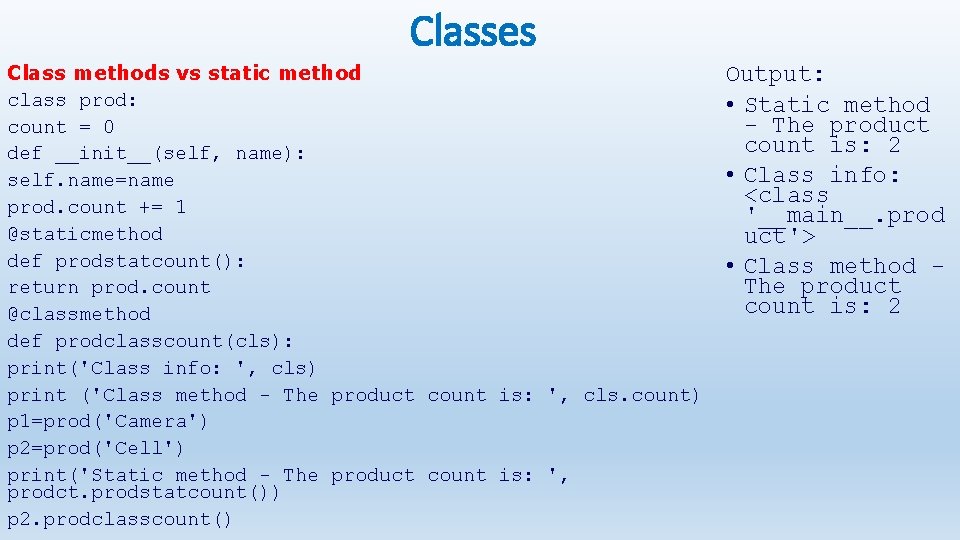
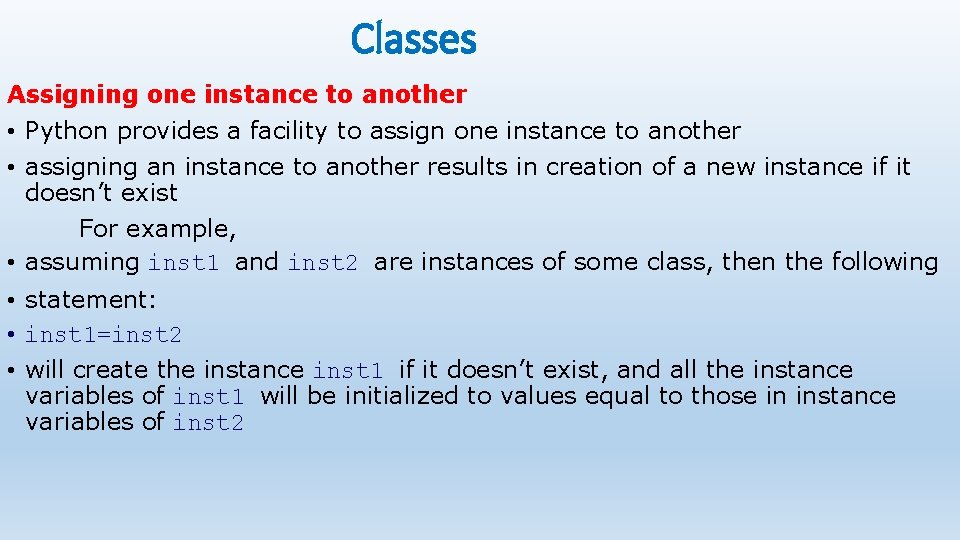
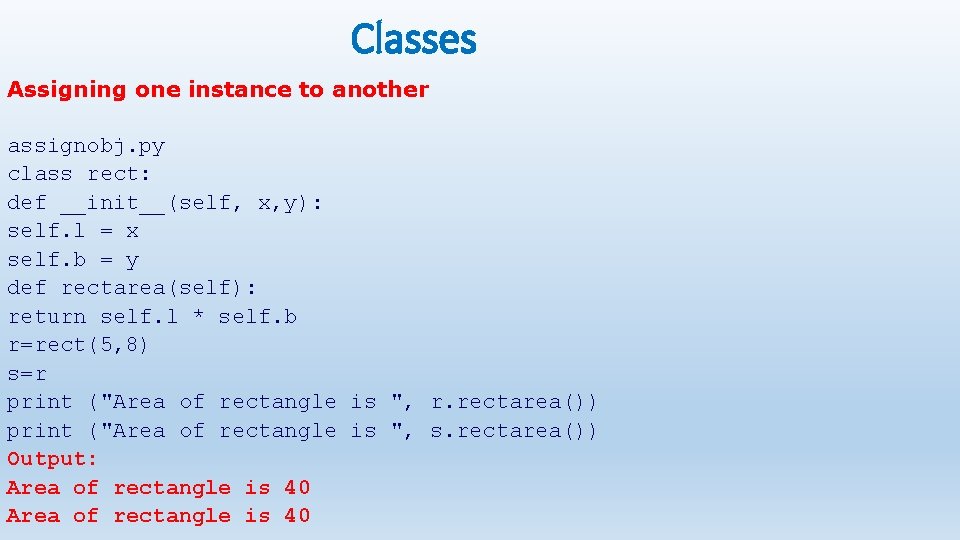
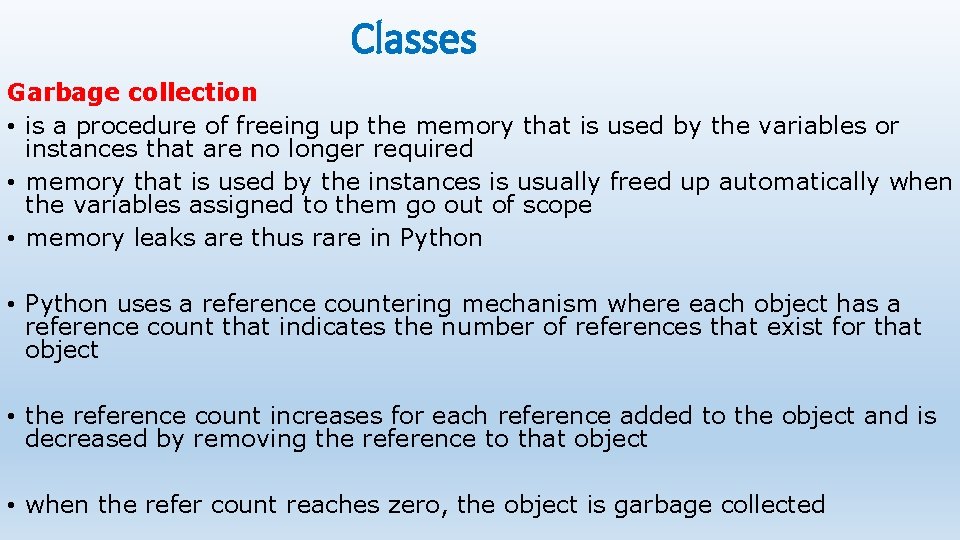
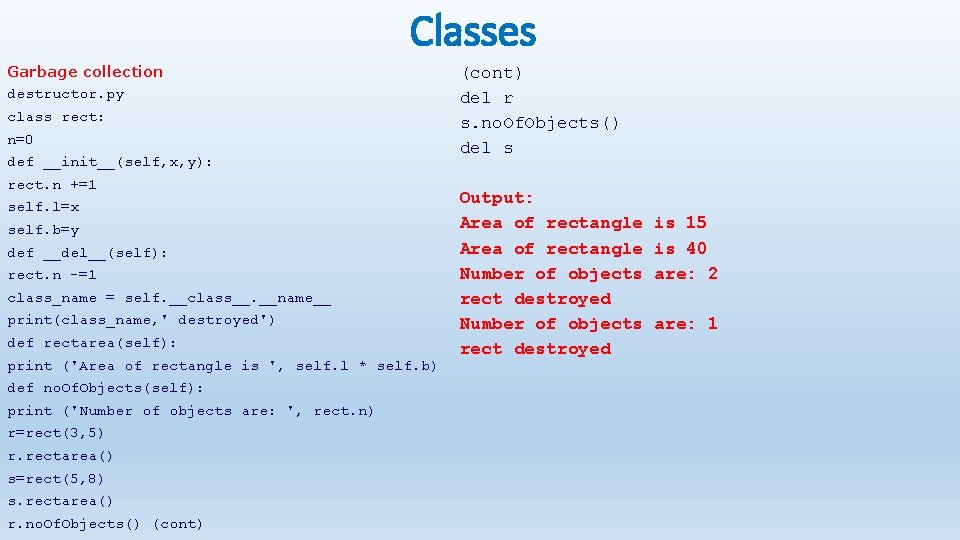
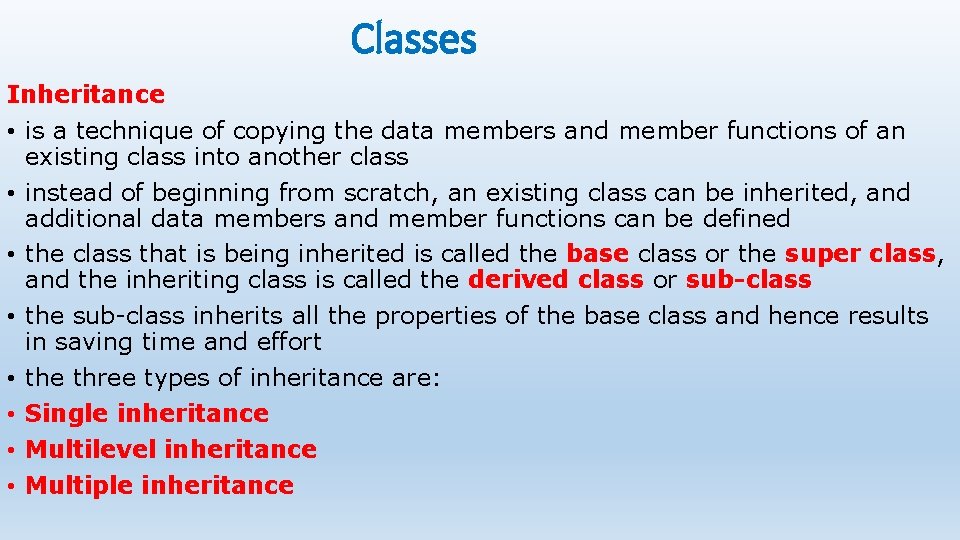
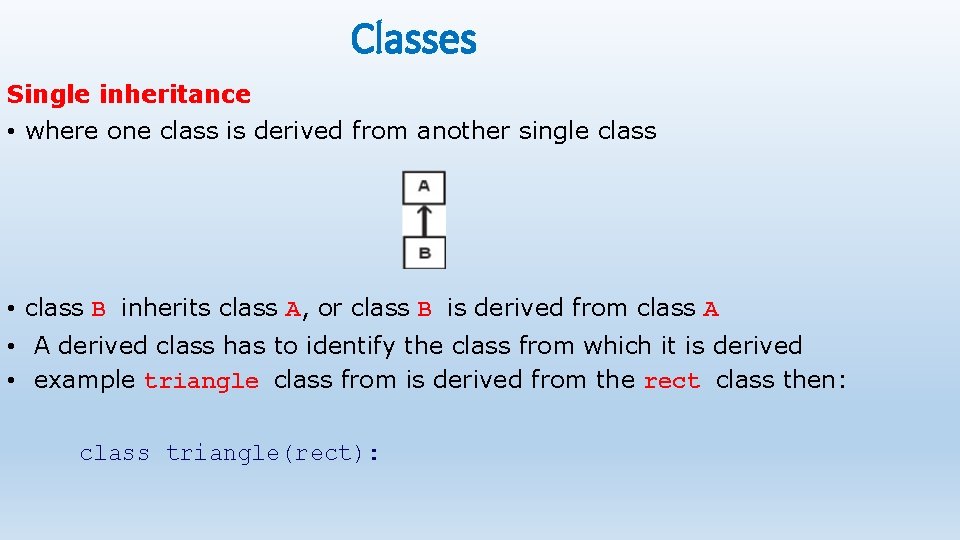
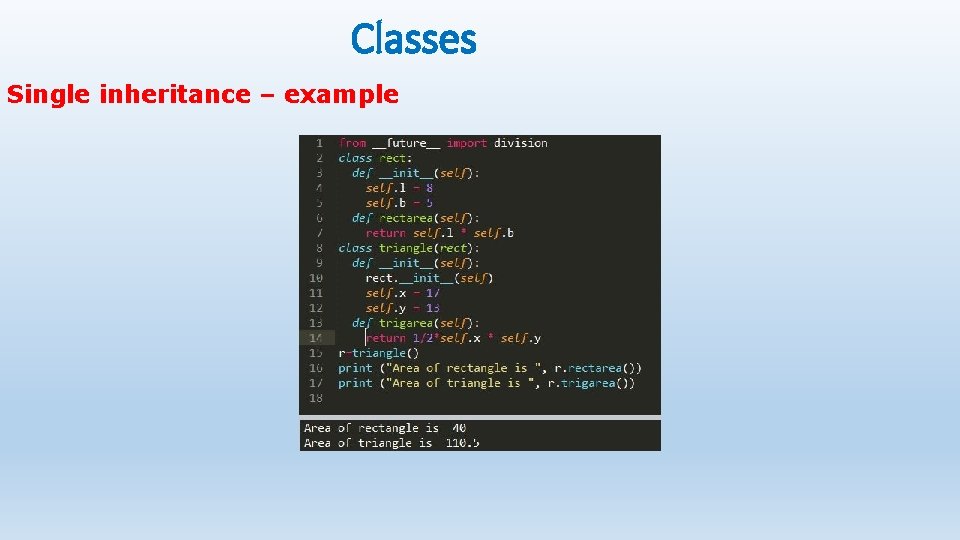
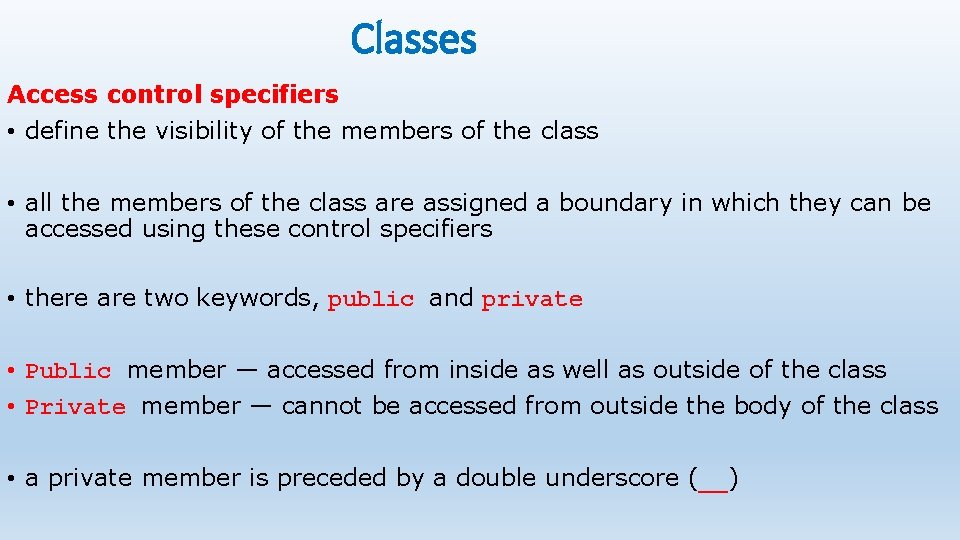
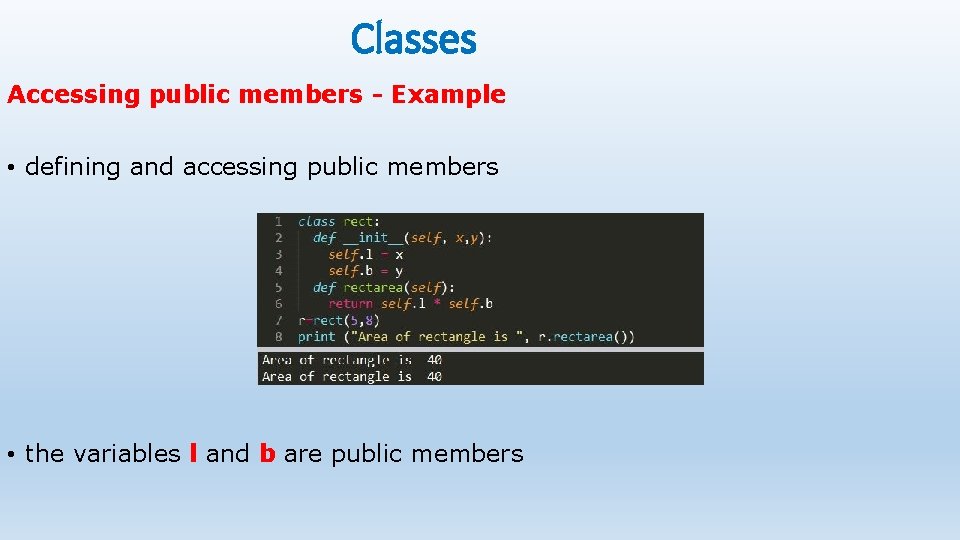
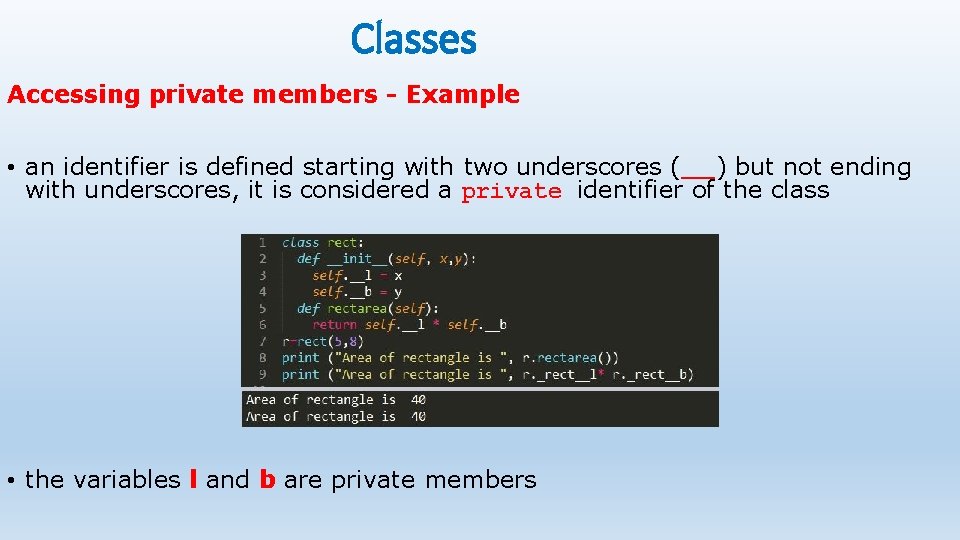
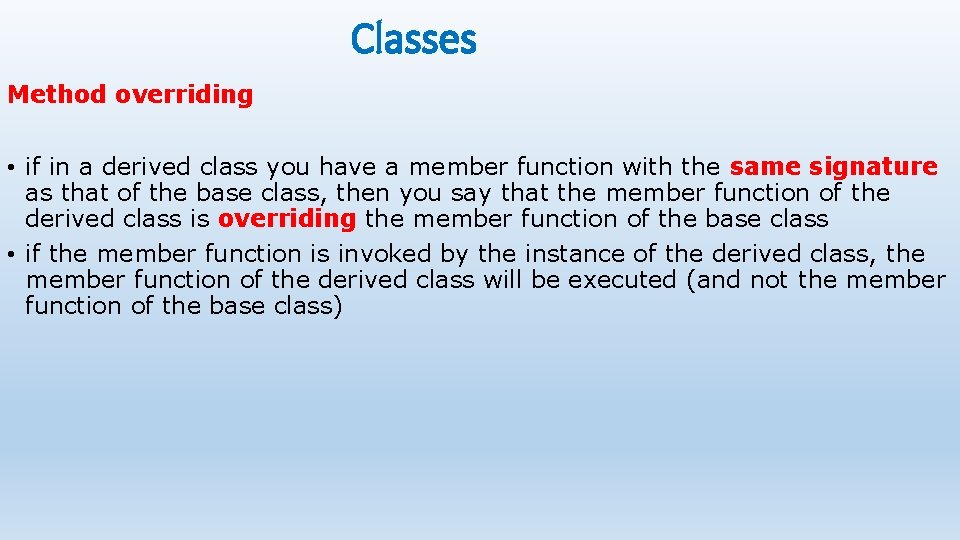
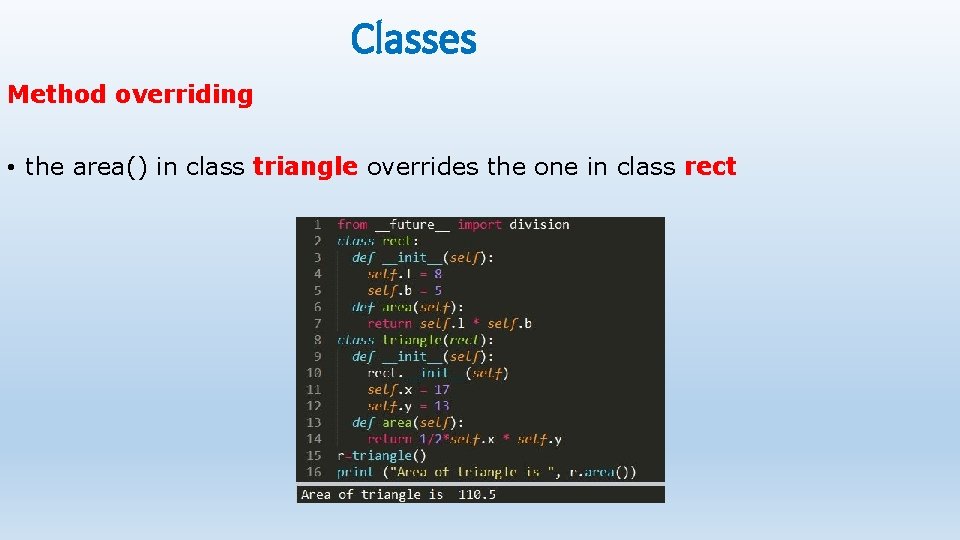
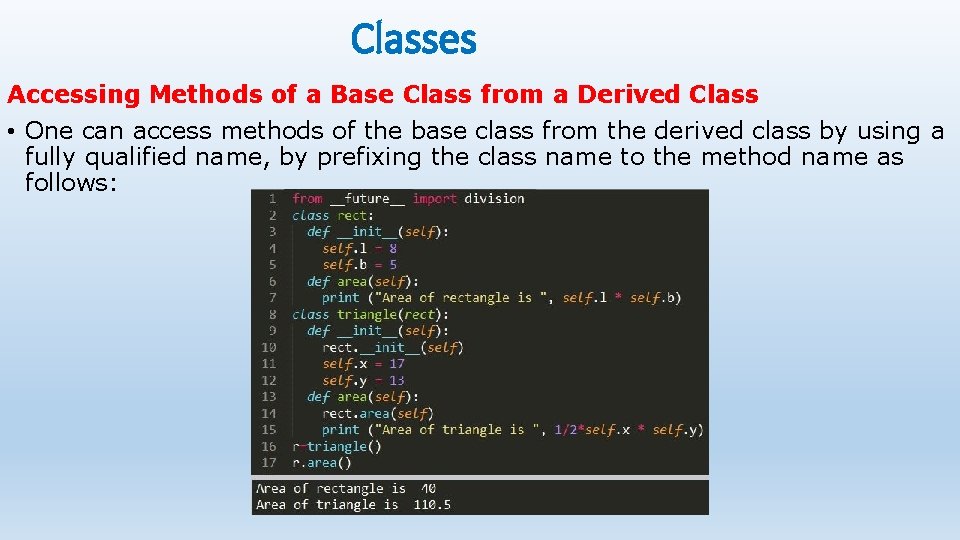
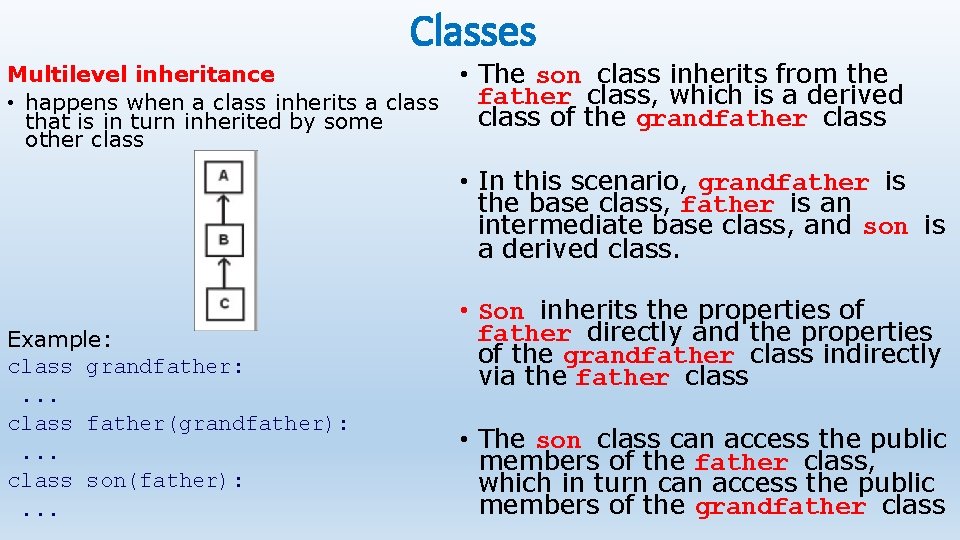
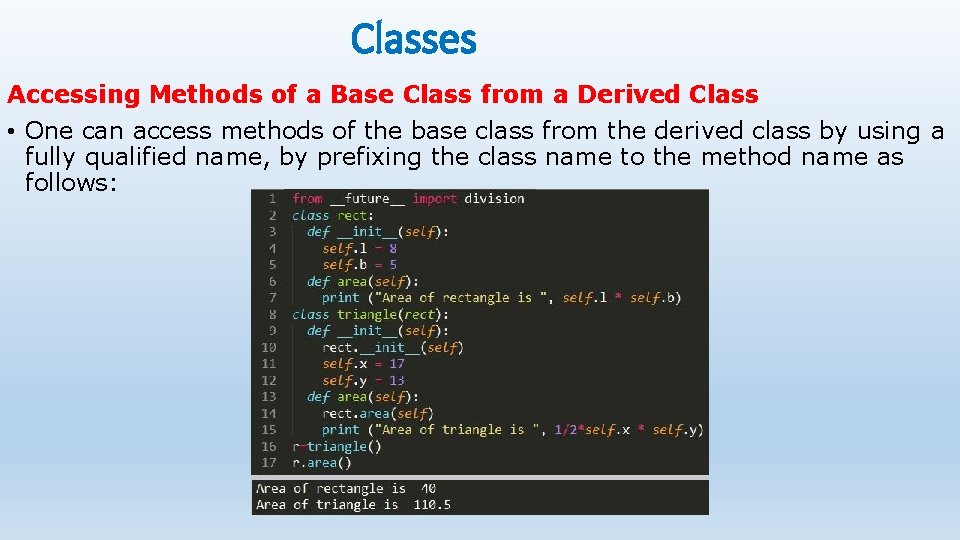
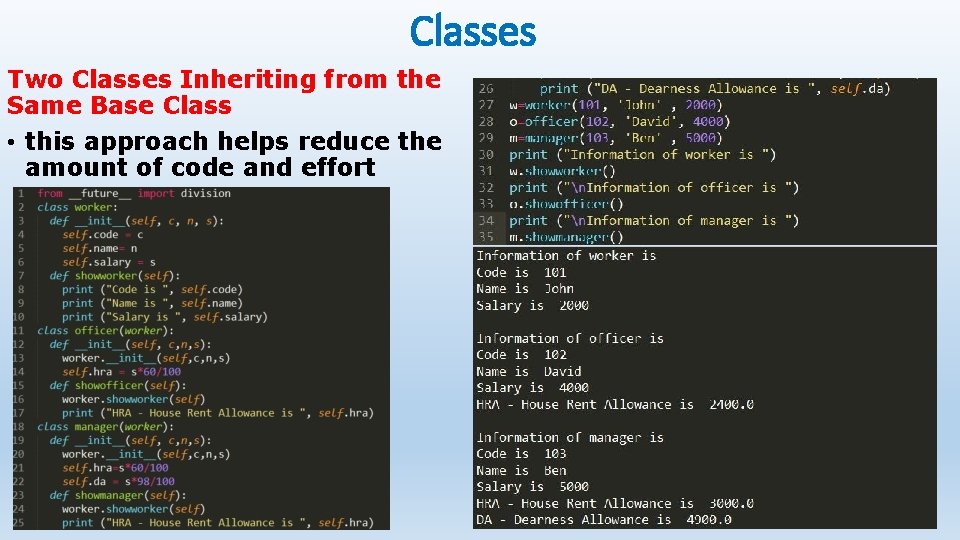
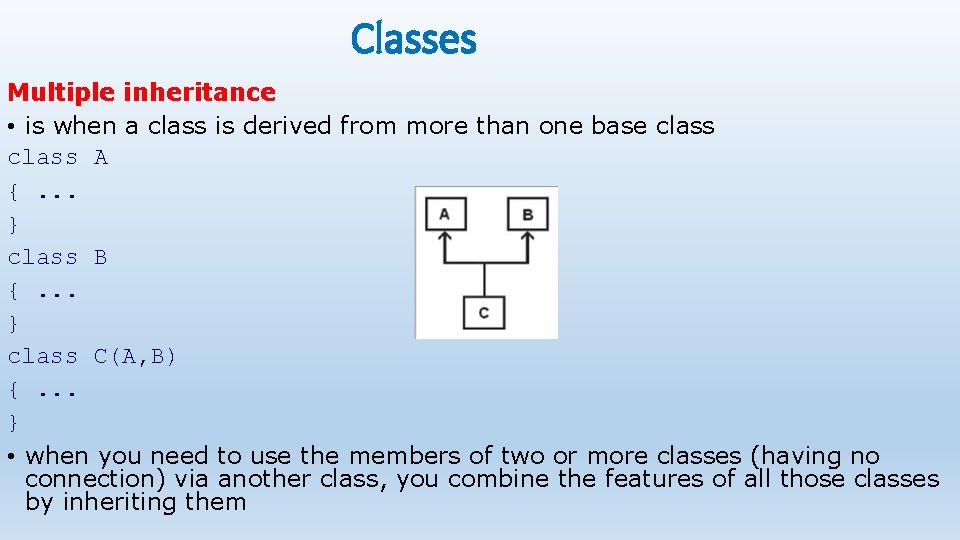
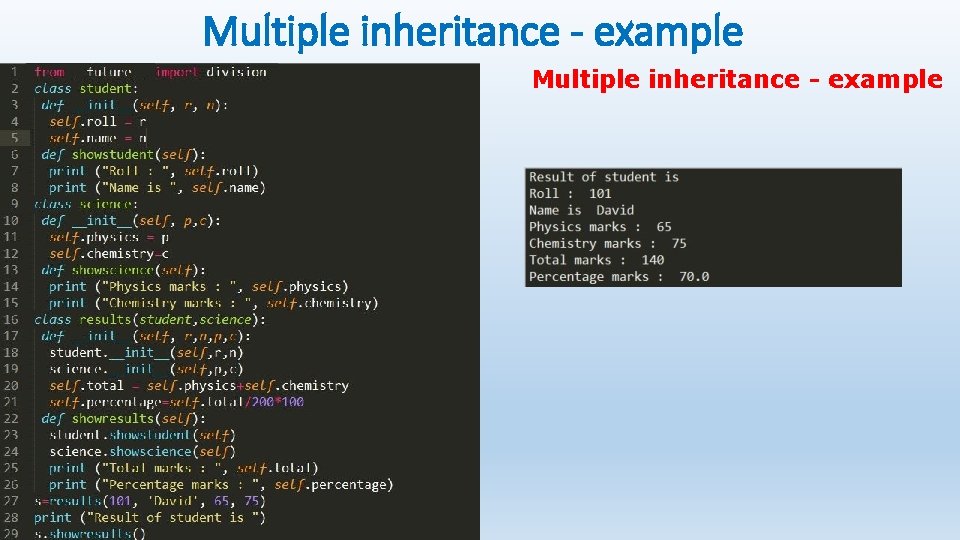
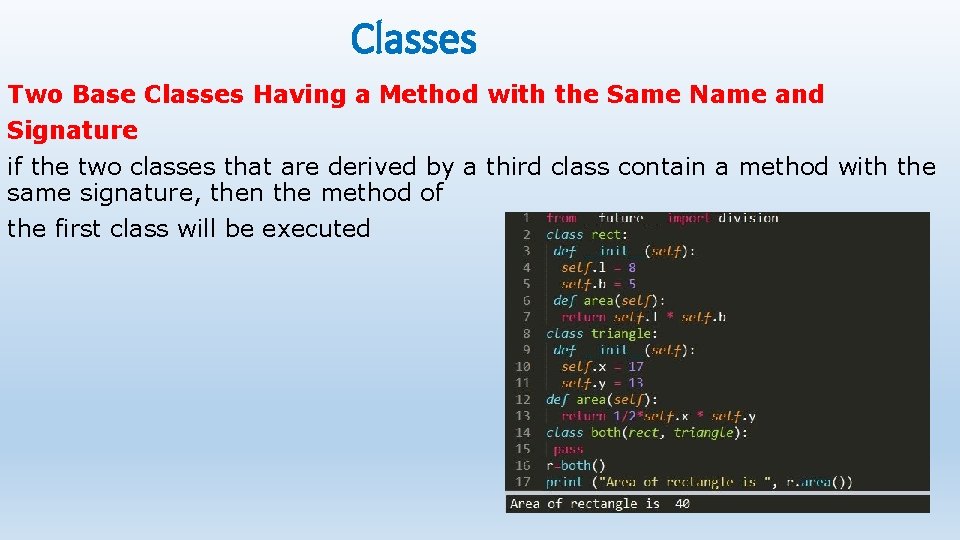
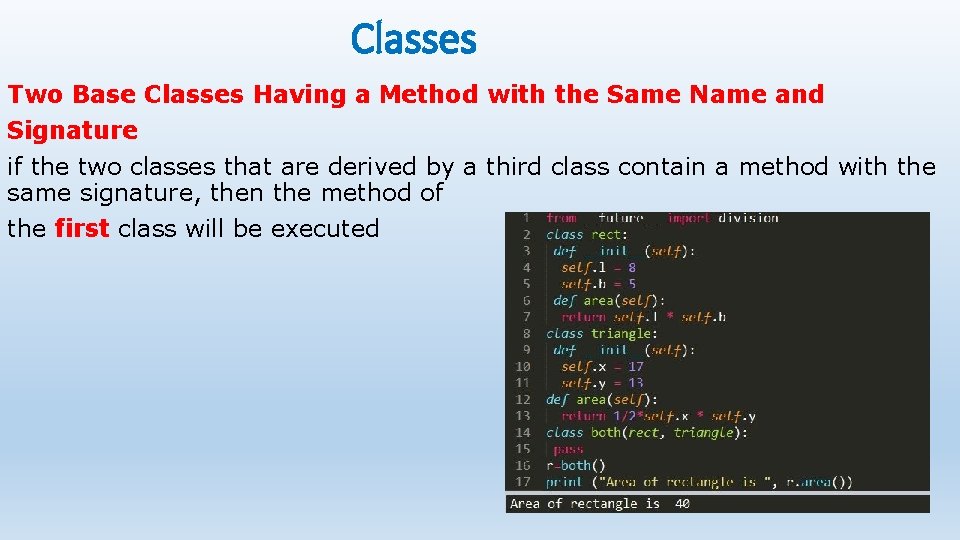
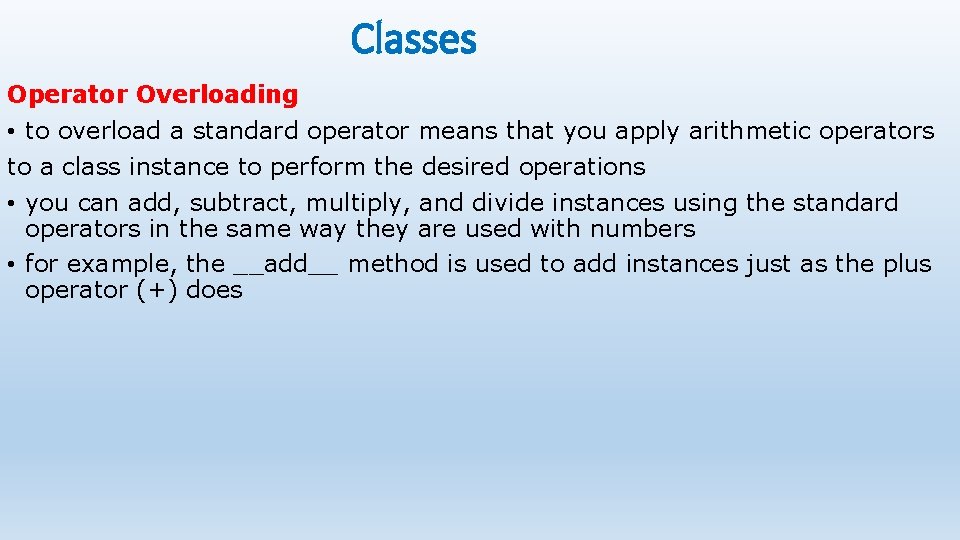
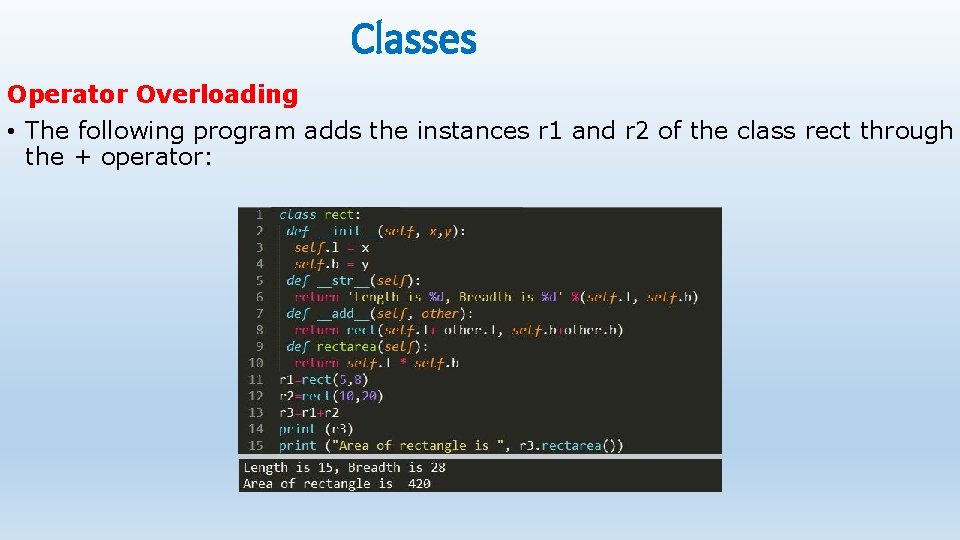
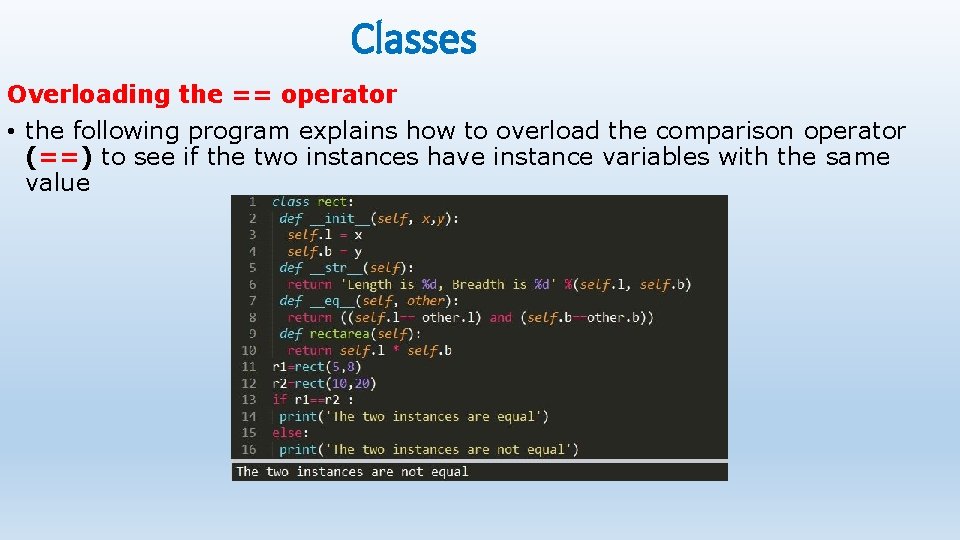
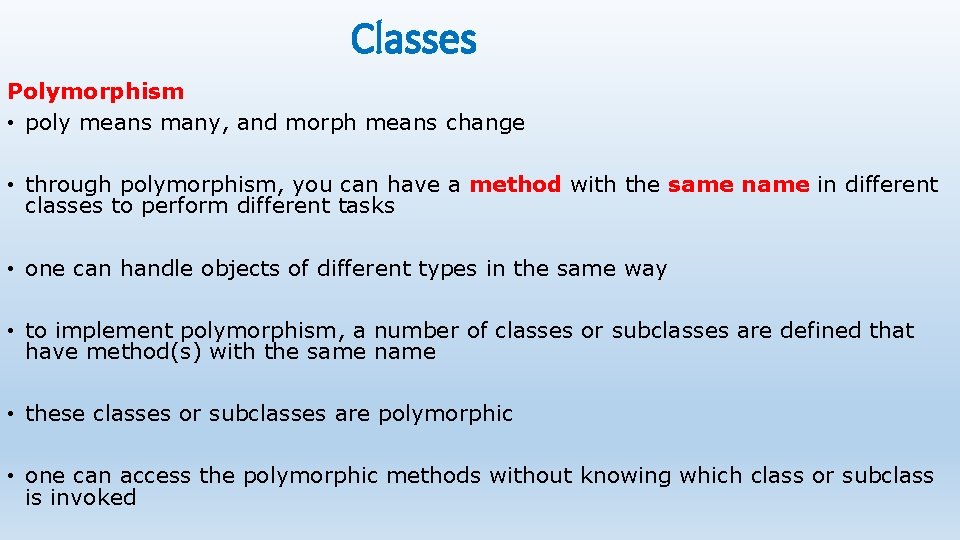
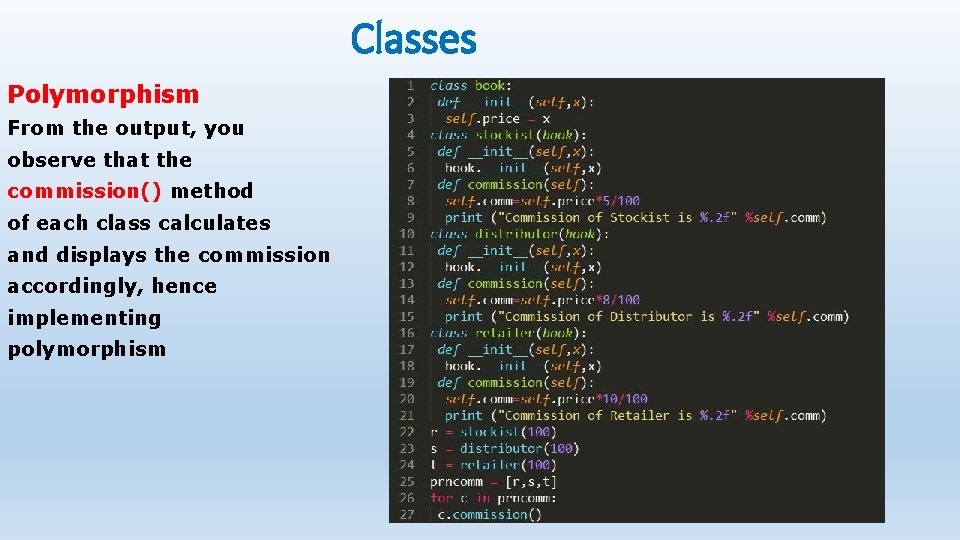
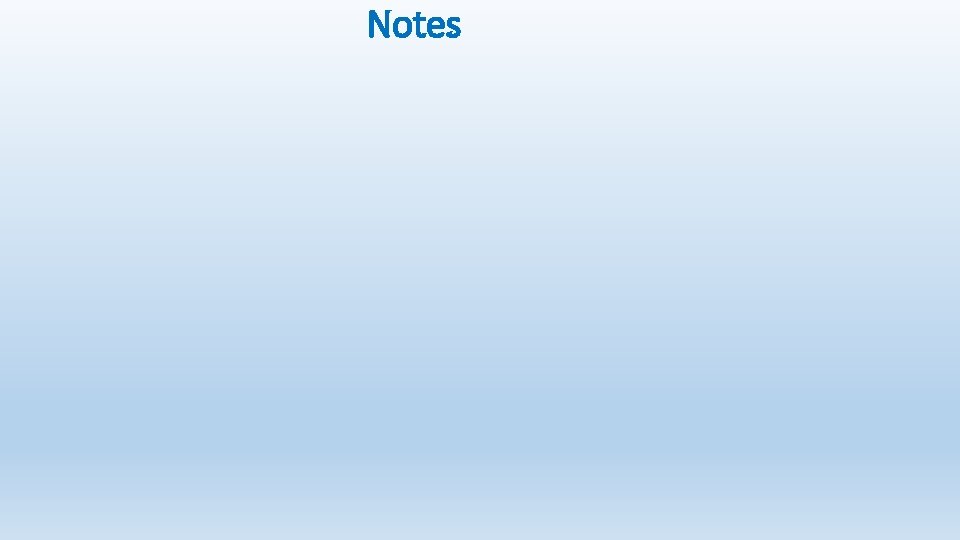
- Slides: 42
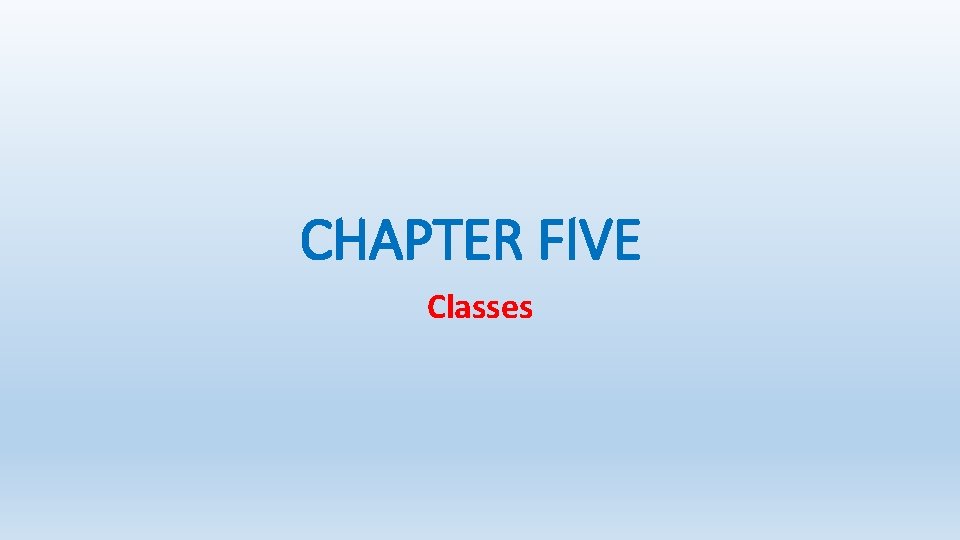
CHAPTER FIVE Classes
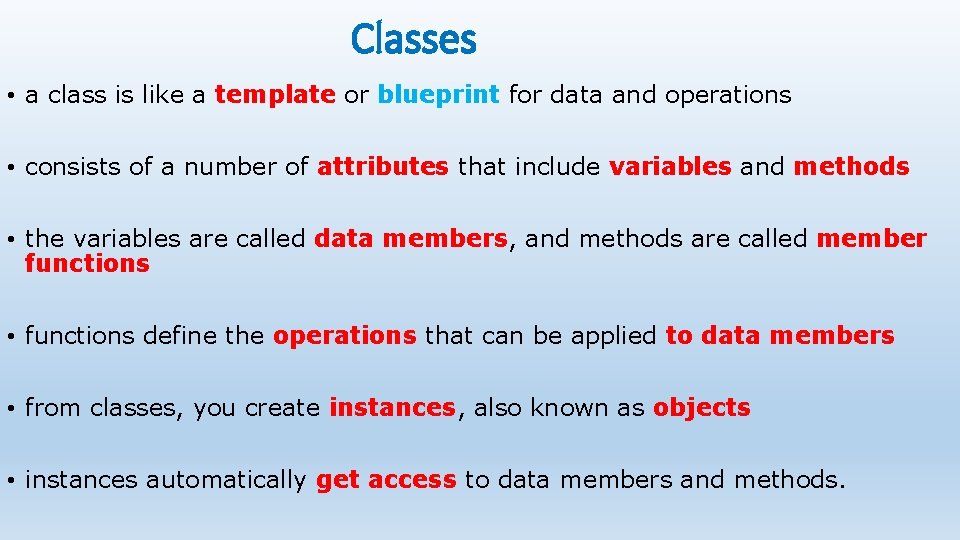
Classes • a class is like a template or blueprint for data and operations • consists of a number of attributes that include variables and methods • the variables are called data members, and methods are called member functions • functions define the operations that can be applied to data members • from classes, you create instances, also known as objects • instances automatically get access to data members and methods.
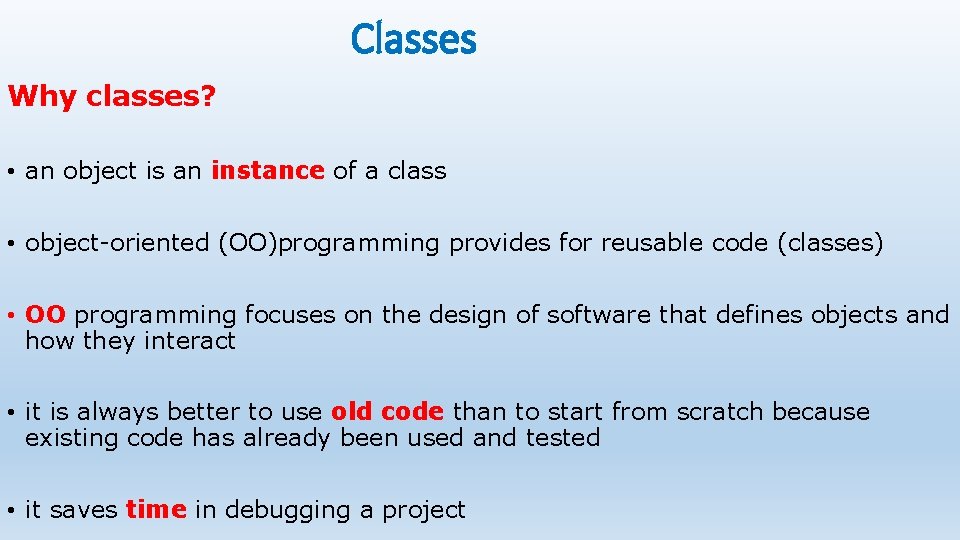
Classes Why classes? • an object is an instance of a class • object-oriented (OO)programming provides for reusable code (classes) • OO programming focuses on the design of software that defines objects and how they interact • it is always better to use old code than to start from scratch because existing code has already been used and tested • it saves time in debugging a project
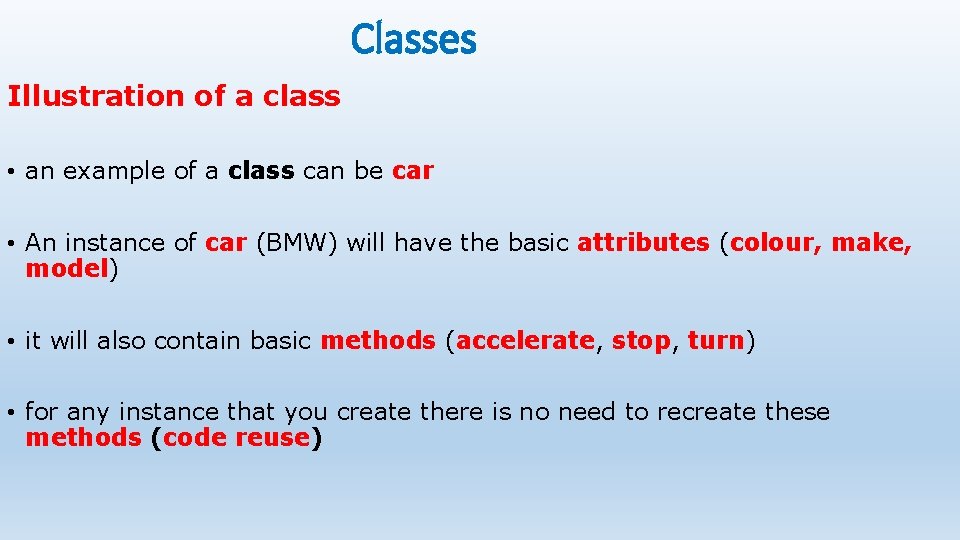
Classes Illustration of a class • an example of a class can be car • An instance of car (BMW) will have the basic attributes (colour, make, model) • it will also contain basic methods (accelerate, stop, turn) • for any instance that you create there is no need to recreate these methods (code reuse)
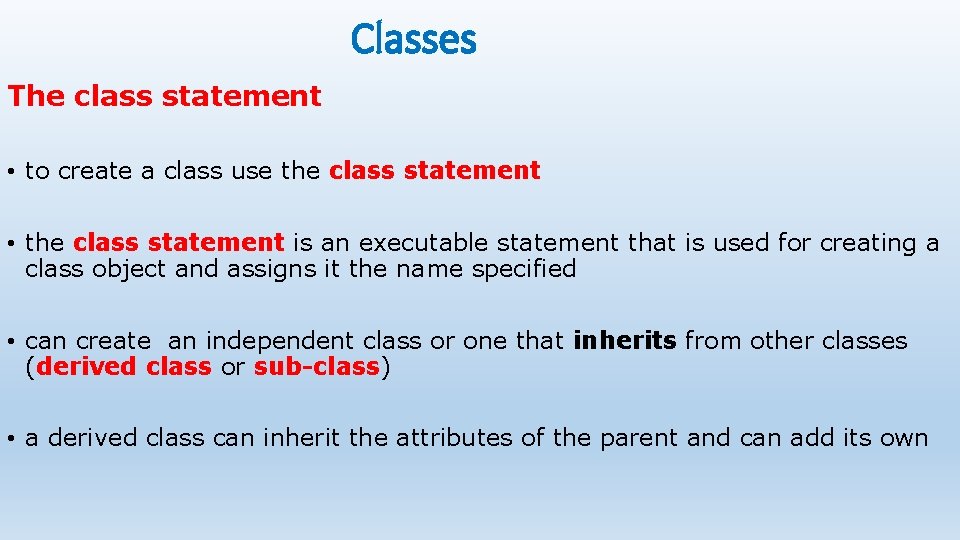
Classes The class statement • to create a class use the class statement • the class statement is an executable statement that is used for creating a class object and assigns it the name specified • can create an independent class or one that inherits from other classes (derived class or sub-class) • a derived class can inherit the attributes of the parent and can add its own
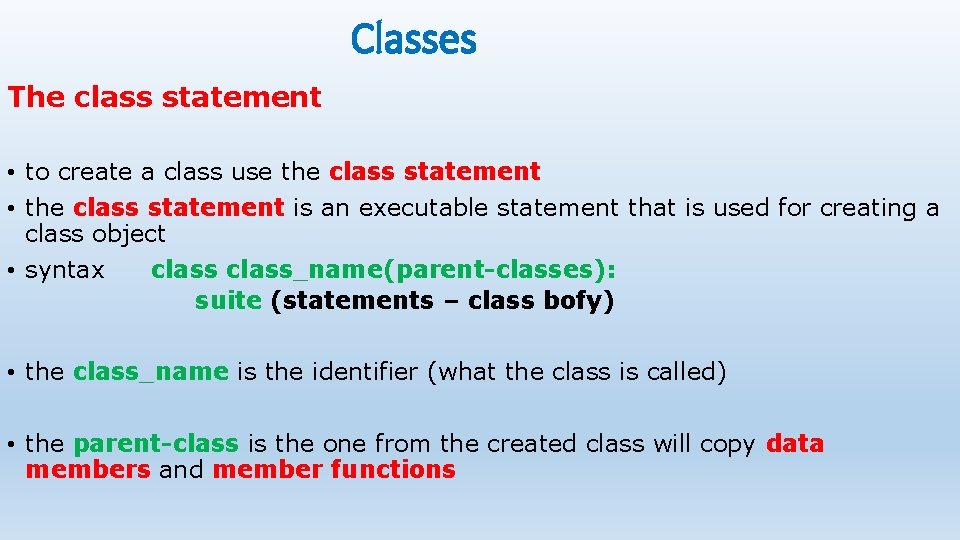
Classes The class statement • to create a class use the class statement • the class statement is an executable statement that is used for creating a class object • syntax class_name(parent-classes): suite (statements – class bofy) • the class_name is the identifier (what the class is called) • the parent-class is the one from the created class will copy data members and member functions
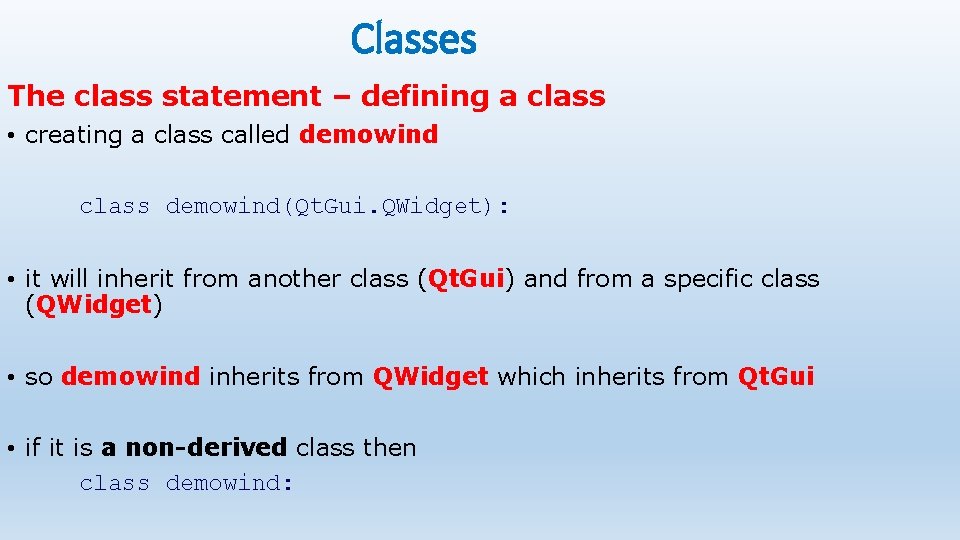
Classes The class statement – defining a class • creating a class called demowind class demowind(Qt. Gui. QWidget): • it will inherit from another class (Qt. Gui) and from a specific class (QWidget) • so demowind inherits from QWidget which inherits from Qt. Gui • if it is a non-derived class then class demowind:
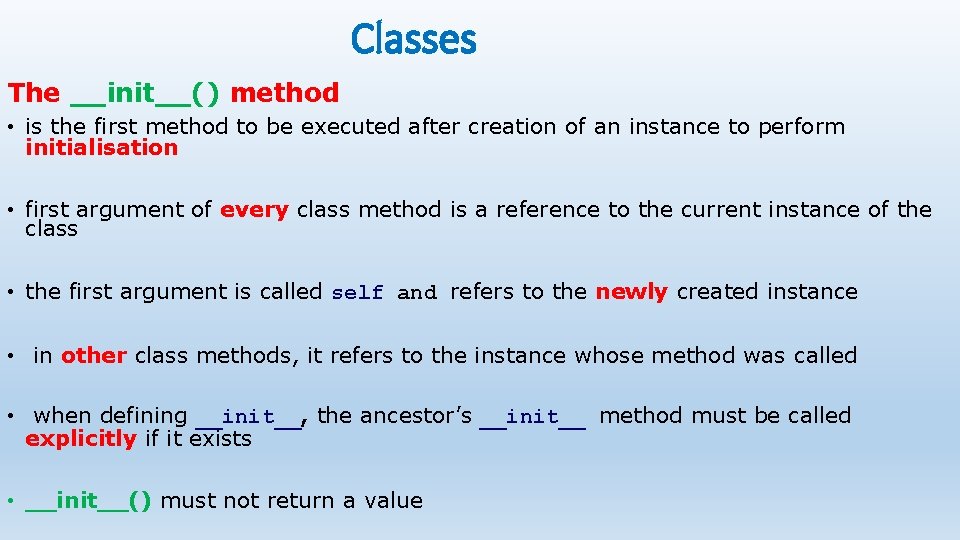
Classes The __init__() method • is the first method to be executed after creation of an instance to perform initialisation • first argument of every class method is a reference to the current instance of the class • the first argument is called self and refers to the newly created instance • in other class methods, it refers to the instance whose method was called • when defining __init__, the ancestor’s __init__ method must be called explicitly if it exists • __init__() must not return a value
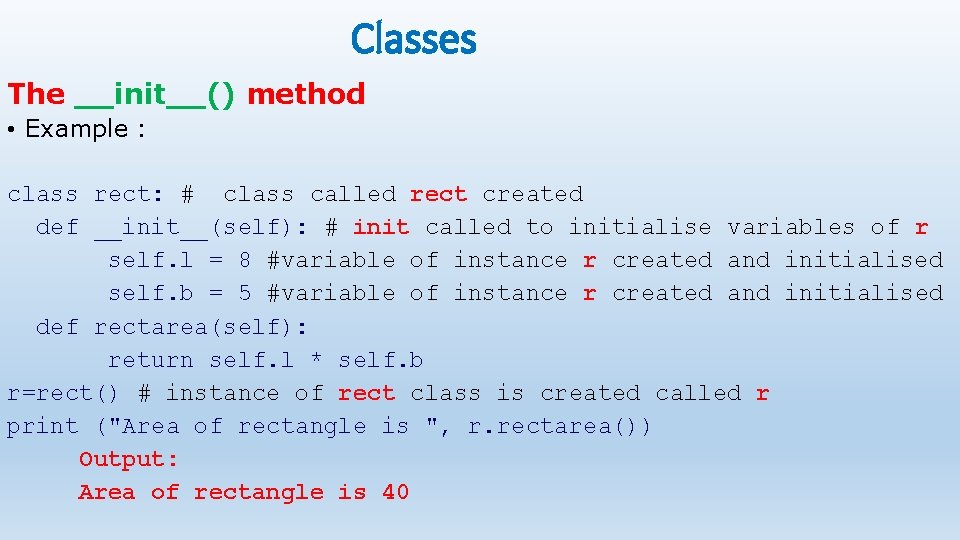
Classes The __init__() method • Example : class rect: # class called rect created def __init__(self): # init called to initialise variables of r self. l = 8 #variable of instance r created and initialised self. b = 5 #variable of instance r created and initialised def rectarea(self): return self. l * self. b r=rect() # instance of rect class is created called r print ("Area of rectangle is ", r. rectarea()) Output: Area of rectangle is 40
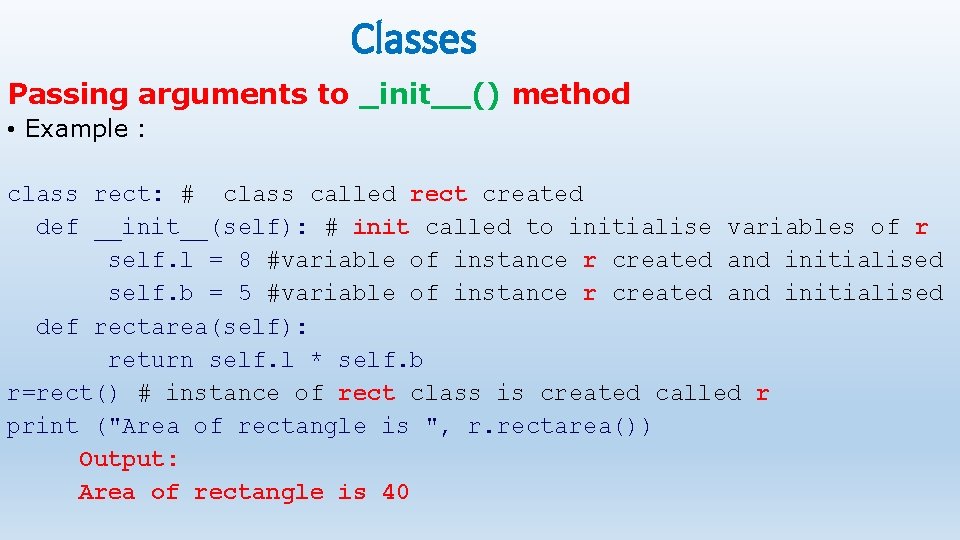
Classes Passing arguments to _init__() method • Example : class rect: # class called rect created def __init__(self): # init called to initialise variables of r self. l = 8 #variable of instance r created and initialised self. b = 5 #variable of instance r created and initialised def rectarea(self): return self. l * self. b r=rect() # instance of rect class is created called r print ("Area of rectangle is ", r. rectarea()) Output: Area of rectangle is 40
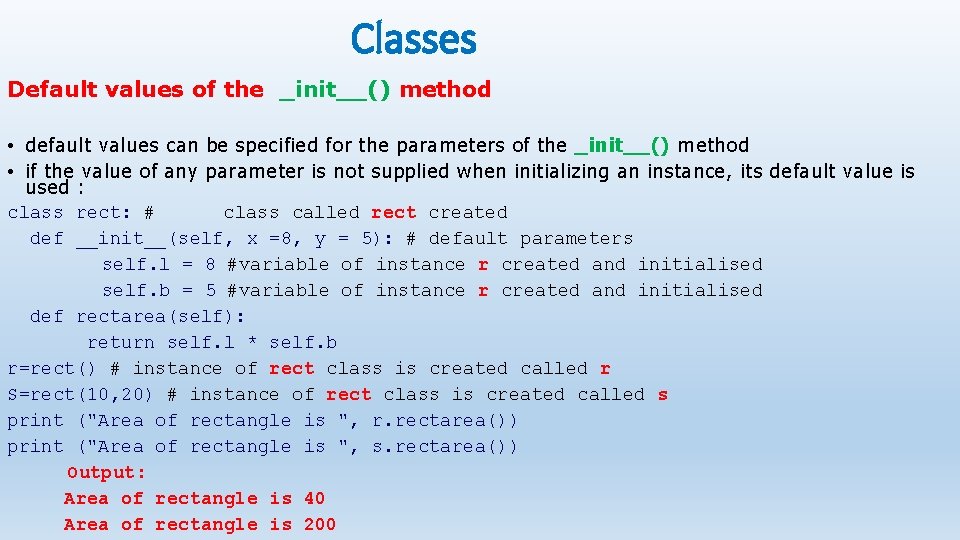
Classes Default values of the _init__() method • default values can be specified for the parameters of the _init__() method • if the value of any parameter is not supplied when initializing an instance, its default value is used : class rect: # class called rect created def __init__(self, x =8, y = 5): # default parameters self. l = 8 #variable of instance r created and initialised self. b = 5 #variable of instance r created and initialised def rectarea(self): return self. l * self. b r=rect() # instance of rect class is created called r S=rect(10, 20) # instance of rect class is created called s print ("Area of rectangle is ", r. rectarea()) print ("Area of rectangle is ", s. rectarea()) Output: Area of rectangle is 40 Area of rectangle is 200
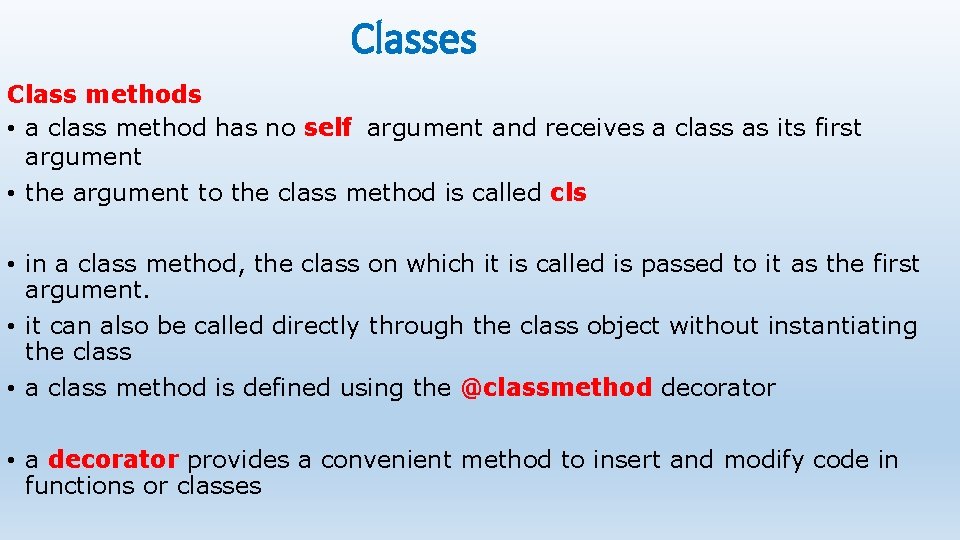
Classes Class methods • a class method has no self argument and receives a class as its first argument • the argument to the class method is called cls • in a class method, the class on which it is called is passed to it as the first argument. • it can also be called directly through the class object without instantiating the class • a class method is defined using the @classmethod decorator • a decorator provides a convenient method to insert and modify code in functions or classes
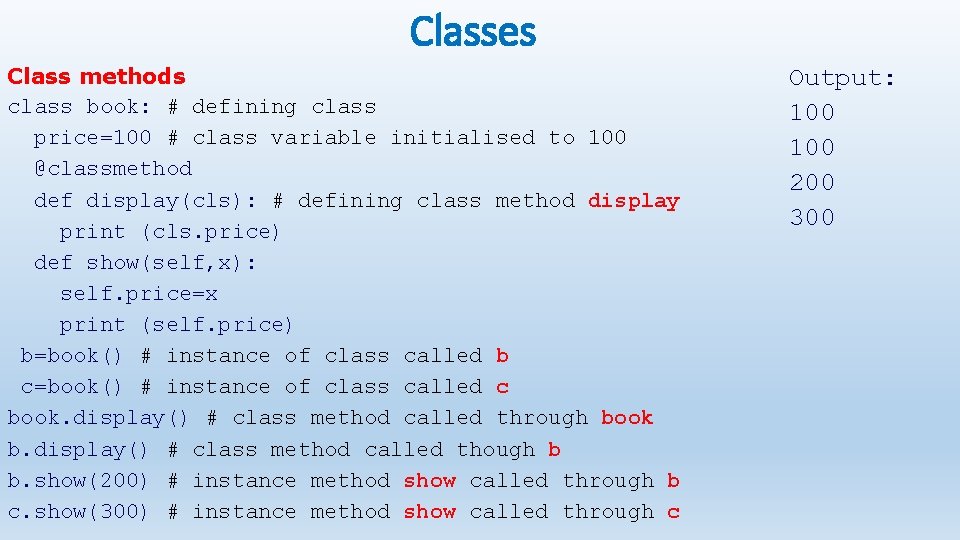
Classes Class methods class book: # defining class price=100 # class variable initialised to 100 @classmethod def display(cls): # defining class method display print (cls. price) def show(self, x): self. price=x print (self. price) b=book() # instance of class called b c=book() # instance of class called c book. display() # class method called through book b. display() # class method called though b b. show(200) # instance method show called through b c. show(300) # instance method show called through c Output: 100 200 300
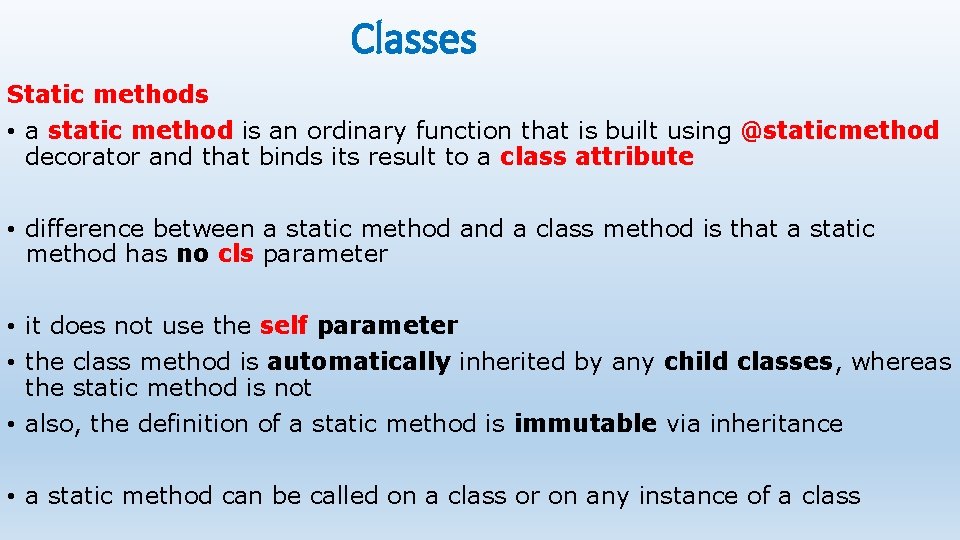
Classes Static methods • a static method is an ordinary function that is built using @staticmethod decorator and that binds its result to a class attribute • difference between a static method and a class method is that a static method has no cls parameter • it does not use the self parameter • the class method is automatically inherited by any child classes, whereas the static method is not • also, the definition of a static method is immutable via inheritance • a static method can be called on a class or on any instance of a class
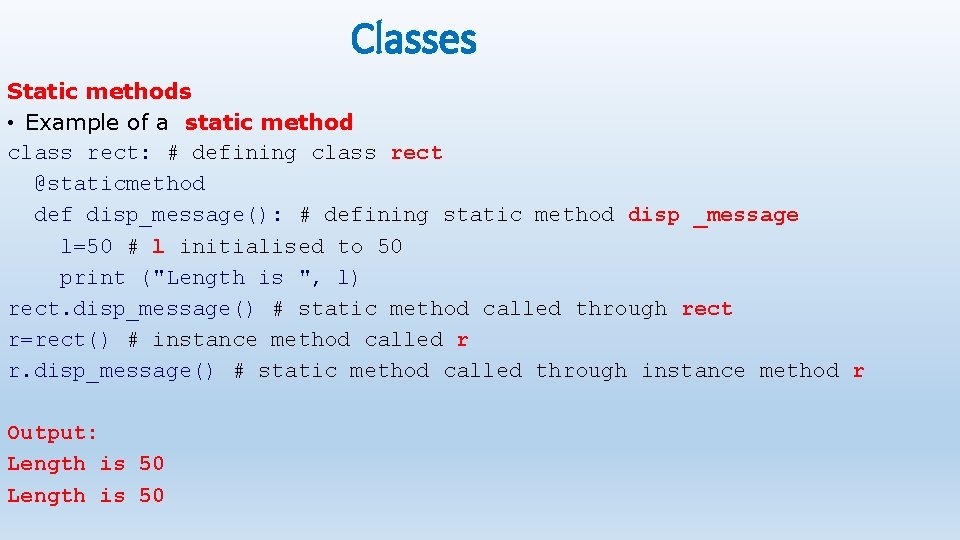
Classes Static methods • Example of a static method class rect: # defining class rect @staticmethod def disp_message(): # defining static method disp _message l=50 # l initialised to 50 print ("Length is ", l) rect. disp_message() # static method called through rect r=rect() # instance method called r r. disp_message() # static method called through instance method r Output: Length is 50
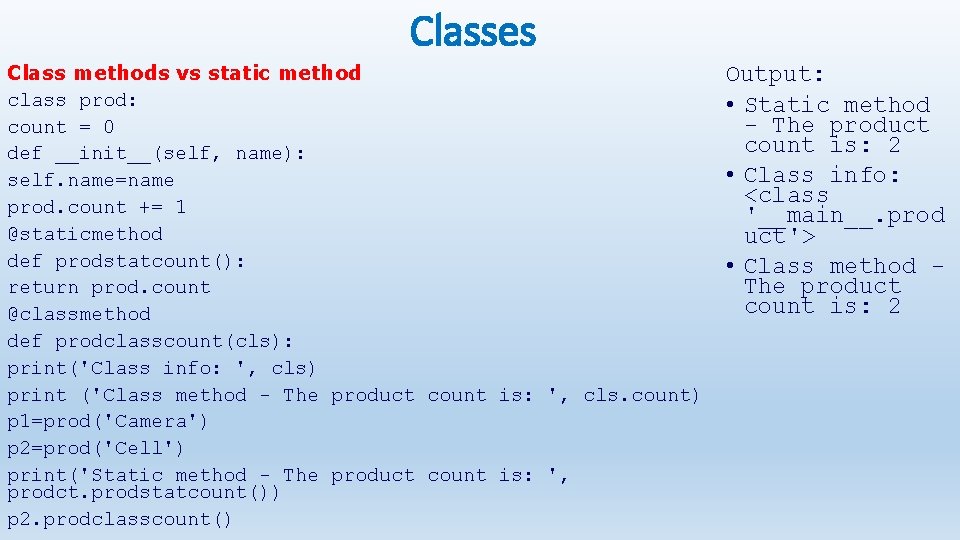
Classes Class methods vs static method class prod: count = 0 def __init__(self, name): self. name=name prod. count += 1 @staticmethod def prodstatcount(): return prod. count @classmethod def prodclasscount(cls): print('Class info: ', cls) print ('Class method - The product count is: ', cls. count) p 1=prod('Camera') p 2=prod('Cell') print('Static method - The product count is: ', prodct. prodstatcount()) p 2. prodclasscount() Output: • Static method - The product count is: 2 • Class info: <class '__main__. prod uct'> • Class method The product count is: 2
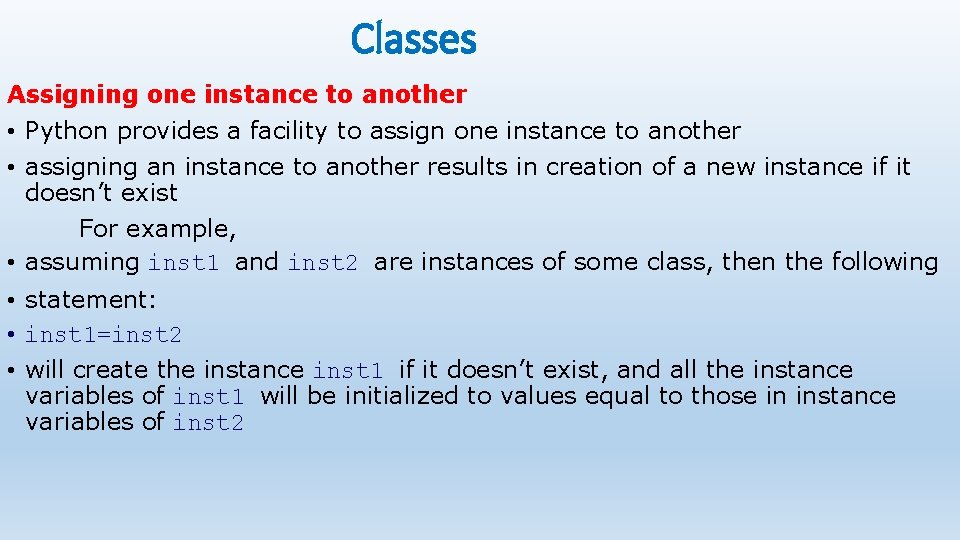
Classes Assigning one instance to another • Python provides a facility to assign one instance to another • assigning an instance to another results in creation of a new instance if it doesn’t exist For example, • assuming inst 1 and inst 2 are instances of some class, then the following • statement: • inst 1=inst 2 • will create the instance inst 1 if it doesn’t exist, and all the instance variables of inst 1 will be initialized to values equal to those in instance variables of inst 2
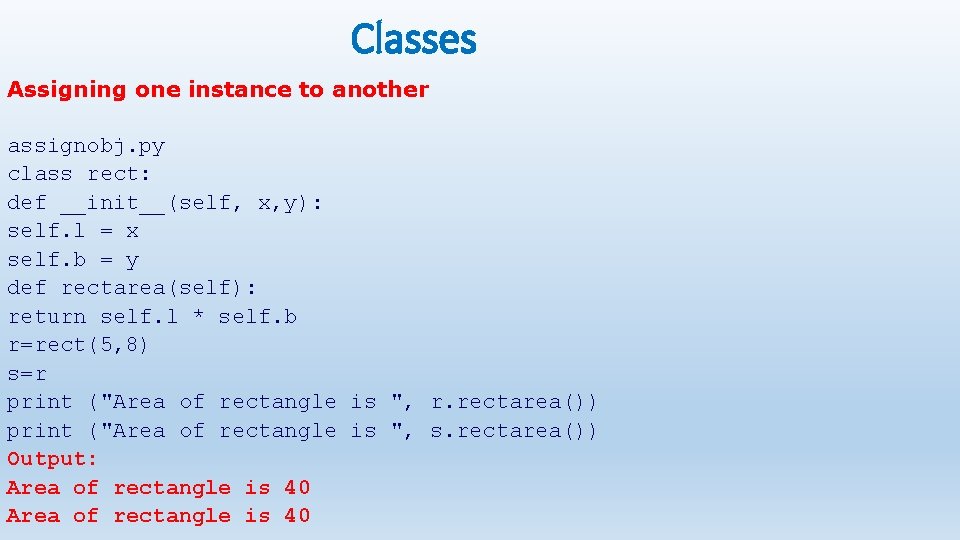
Classes Assigning one instance to another assignobj. py class rect: def __init__(self, x, y): self. l = x self. b = y def rectarea(self): return self. l * self. b r=rect(5, 8) s=r print ("Area of rectangle is ", r. rectarea()) print ("Area of rectangle is ", s. rectarea()) Output: Area of rectangle is 40
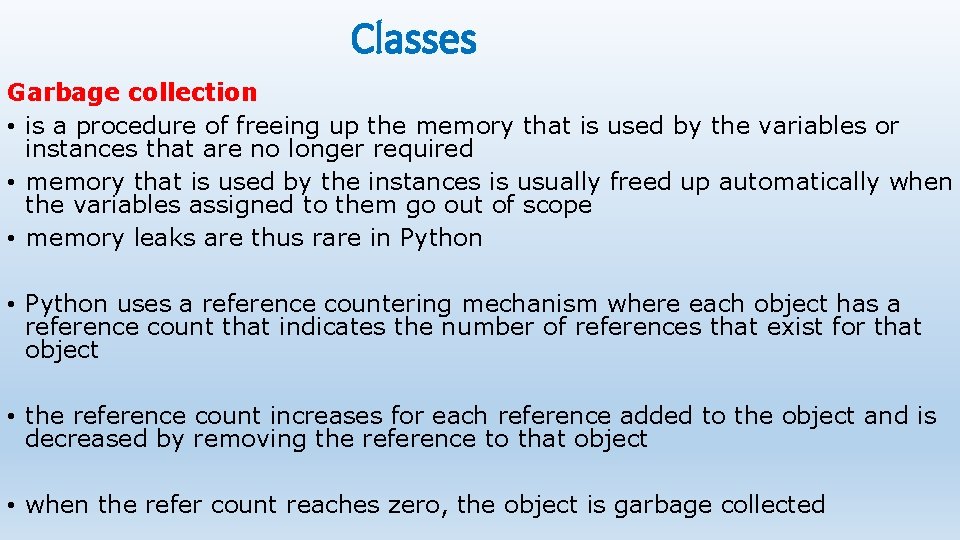
Classes Garbage collection • is a procedure of freeing up the memory that is used by the variables or instances that are no longer required • memory that is used by the instances is usually freed up automatically when the variables assigned to them go out of scope • memory leaks are thus rare in Python • Python uses a reference countering mechanism where each object has a reference count that indicates the number of references that exist for that object • the reference count increases for each reference added to the object and is decreased by removing the reference to that object • when the refer count reaches zero, the object is garbage collected
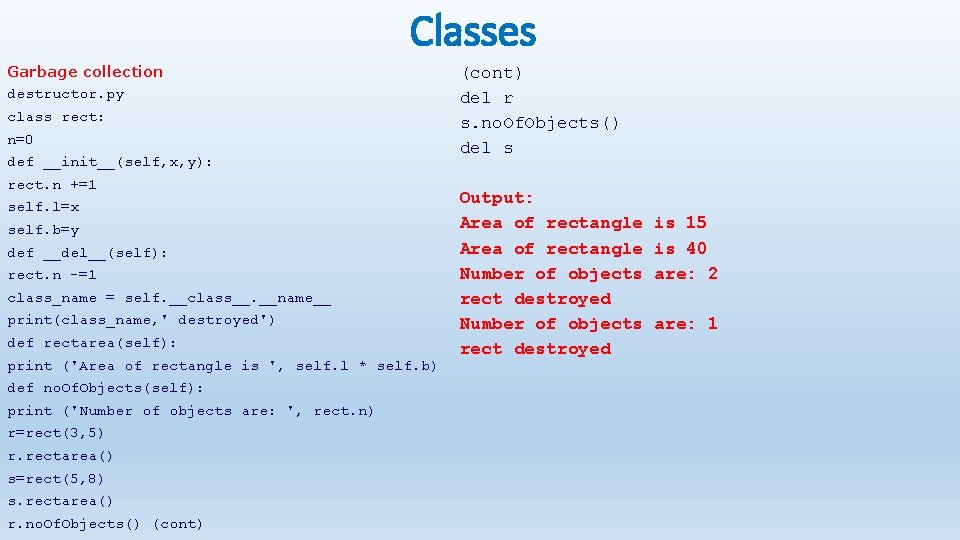
Classes Garbage collection destructor. py class rect: n=0 def __init__(self, x, y): rect. n +=1 self. l=x self. b=y def __del__(self): rect. n -=1 class_name = self. __class__. __name__ print(class_name, ' destroyed') def rectarea(self): print ('Area of rectangle is ', self. l * self. b) def no. Of. Objects(self): print ('Number of objects are: ', rect. n) r=rect(3, 5) r. rectarea() s=rect(5, 8) s. rectarea() r. no. Of. Objects() (cont) del r s. no. Of. Objects() del s Output: Area of rectangle Number of objects rect destroyed is 15 is 40 are: 2 are: 1
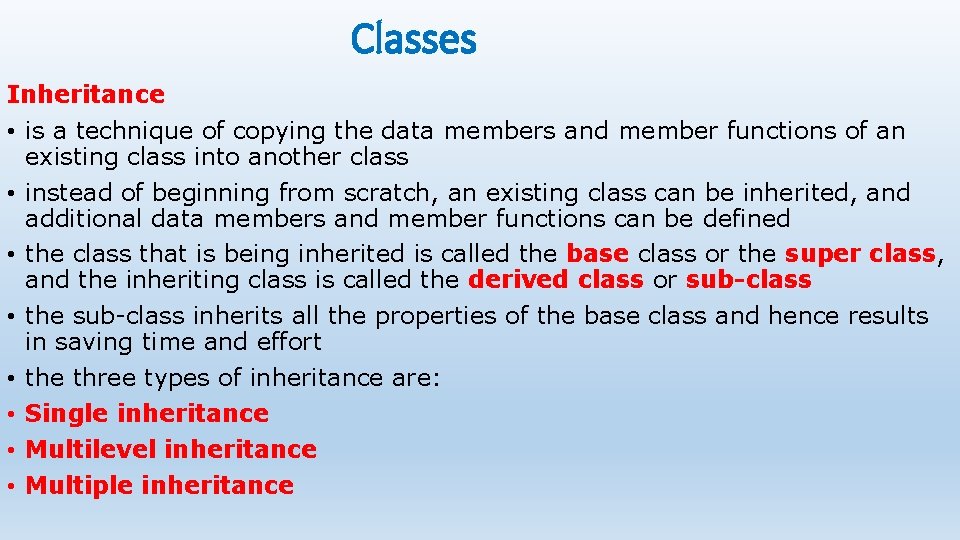
Classes Inheritance • is a technique of copying the data members and member functions of an existing class into another class • instead of beginning from scratch, an existing class can be inherited, and additional data members and member functions can be defined • the class that is being inherited is called the base class or the super class, and the inheriting class is called the derived class or sub-class • the sub-class inherits all the properties of the base class and hence results in saving time and effort • the three types of inheritance are: • Single inheritance • Multilevel inheritance • Multiple inheritance
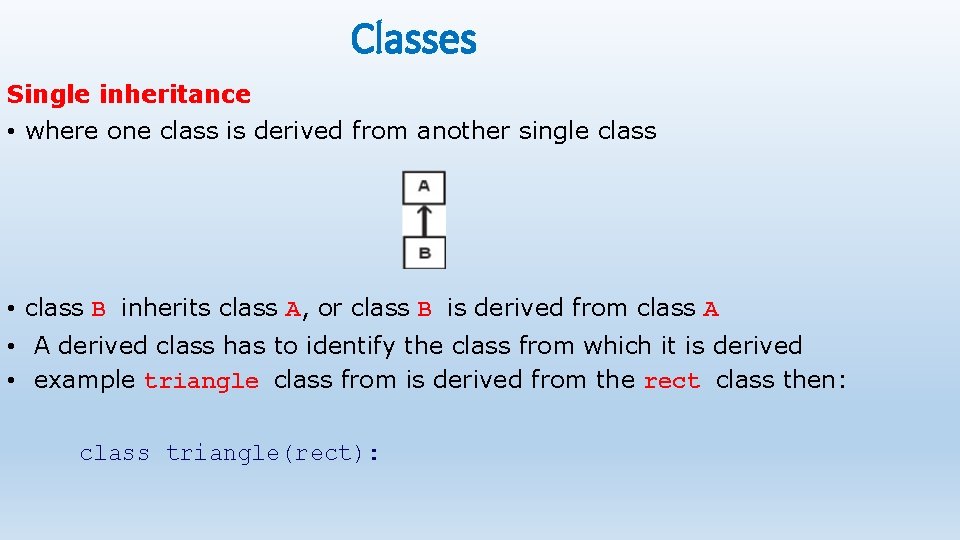
Classes Single inheritance • where one class is derived from another single class • class B inherits class A, or class B is derived from class A • A derived class has to identify the class from which it is derived • example triangle class from is derived from the rect class then: class triangle(rect):
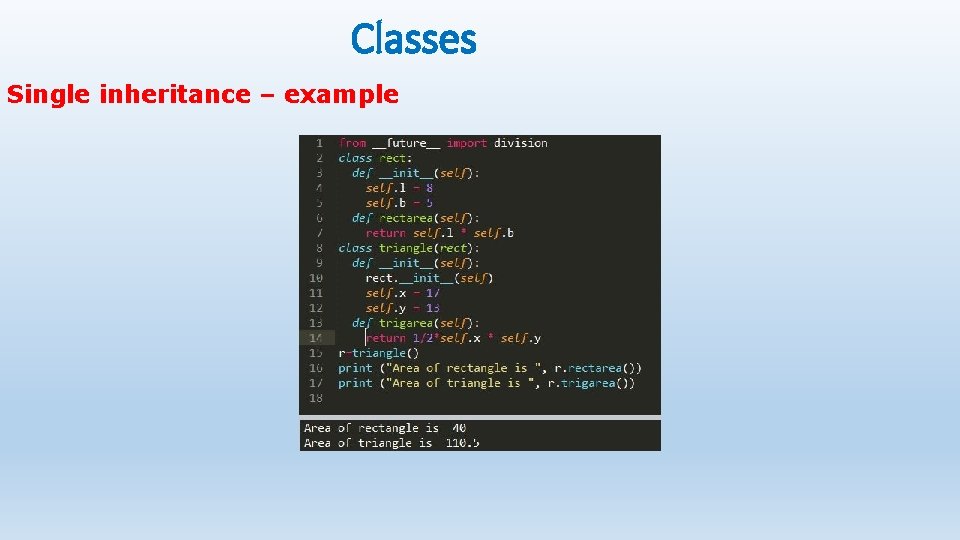
Classes Single inheritance – example
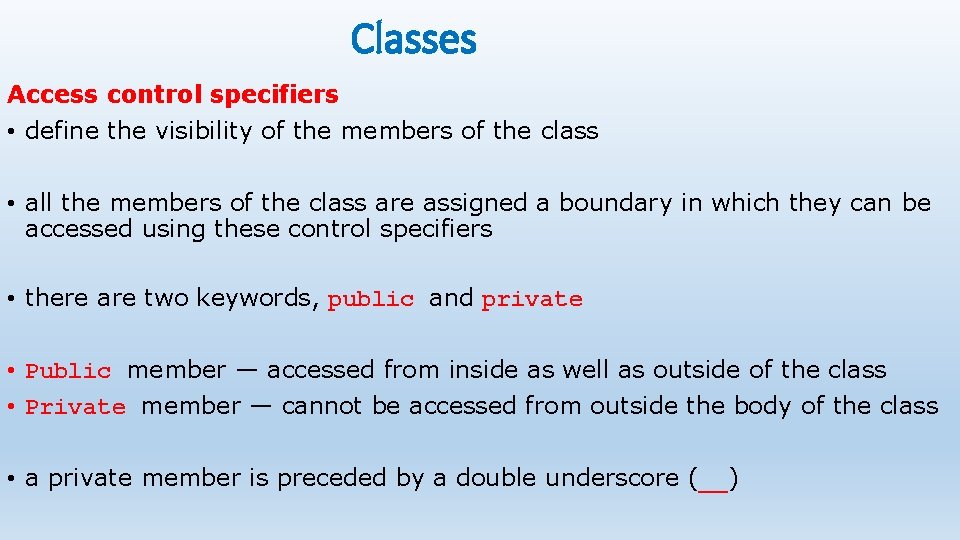
Classes Access control specifiers • define the visibility of the members of the class • all the members of the class are assigned a boundary in which they can be accessed using these control specifiers • there are two keywords, public and private • Public member — accessed from inside as well as outside of the class • Private member — cannot be accessed from outside the body of the class • a private member is preceded by a double underscore (__)
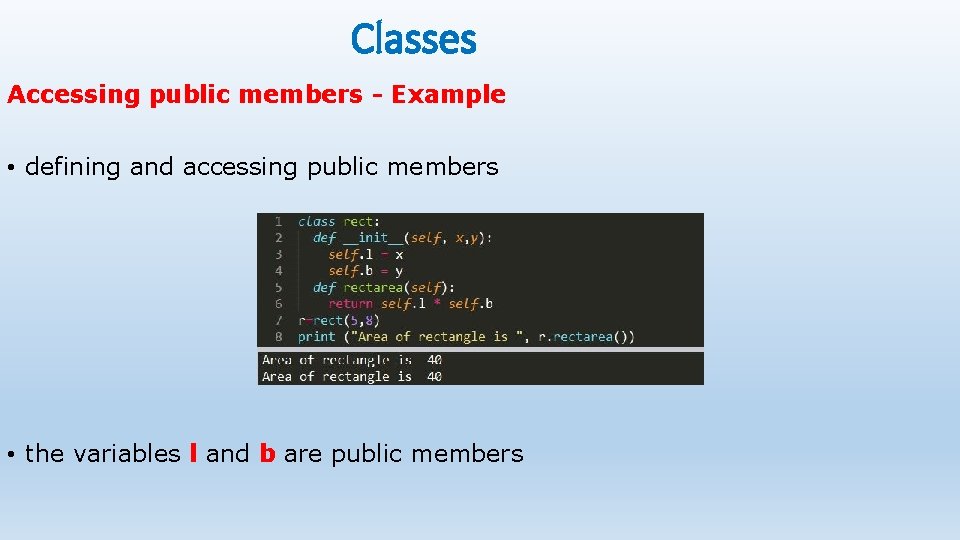
Classes Accessing public members - Example • defining and accessing public members • the variables l and b are public members
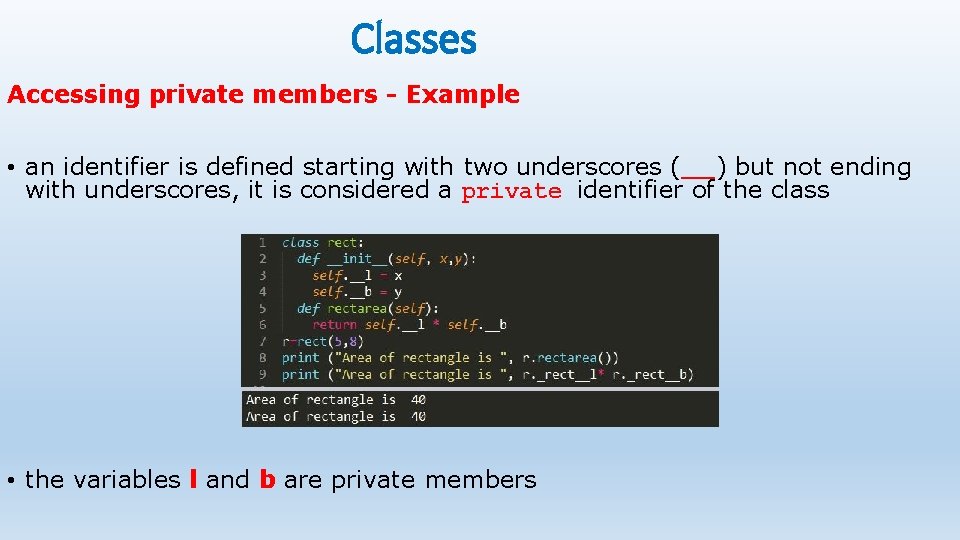
Classes Accessing private members - Example • an identifier is defined starting with two underscores (__) but not ending with underscores, it is considered a private identifier of the class • the variables l and b are private members
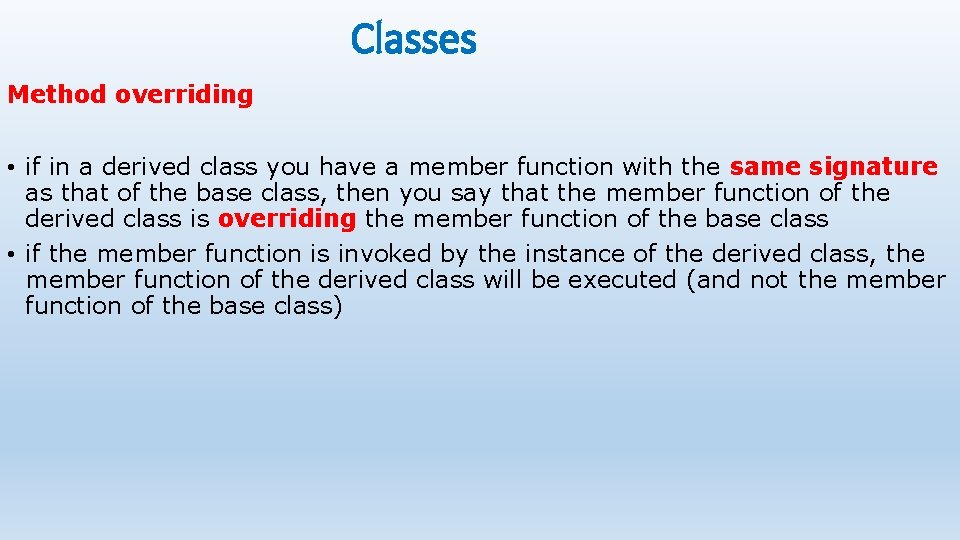
Classes Method overriding • if in a derived class you have a member function with the same signature as that of the base class, then you say that the member function of the derived class is overriding the member function of the base class • if the member function is invoked by the instance of the derived class, the member function of the derived class will be executed (and not the member function of the base class)
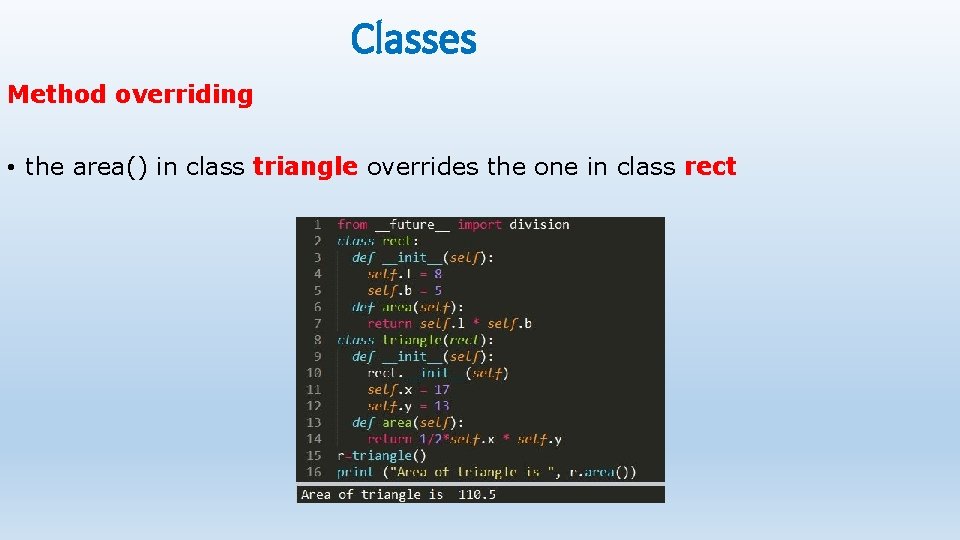
Classes Method overriding • the area() in class triangle overrides the one in class rect
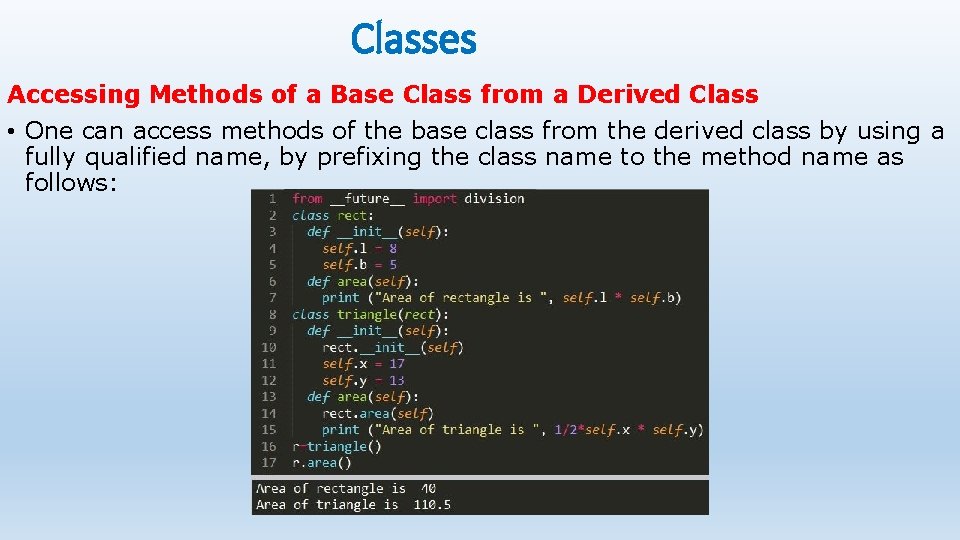
Classes Accessing Methods of a Base Class from a Derived Class • One can access methods of the base class from the derived class by using a fully qualified name, by prefixing the class name to the method name as follows:
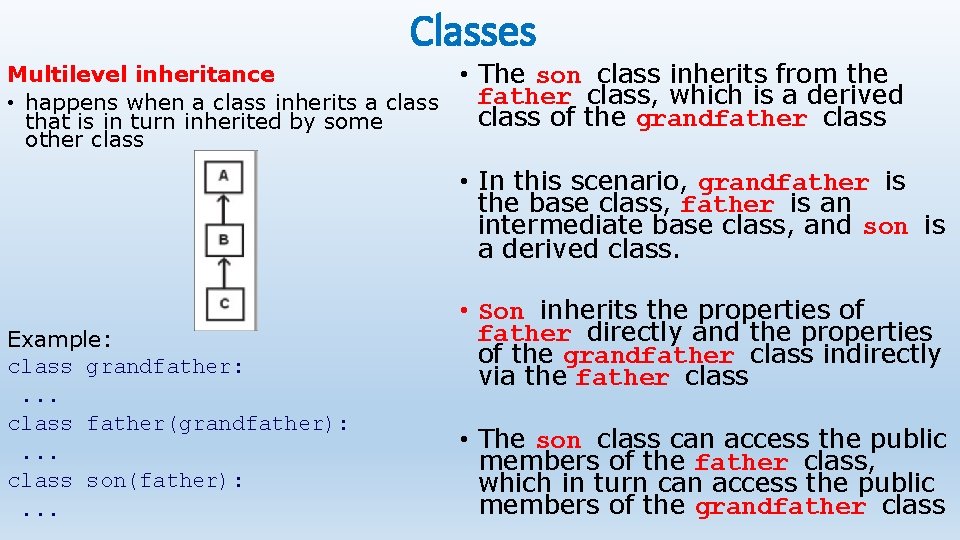
Classes Multilevel inheritance • happens when a class inherits a class that is in turn inherited by some other class • The son class inherits from the father class, which is a derived class of the grandfather class • In this scenario, grandfather is the base class, father is an intermediate base class, and son is a derived class. Example: class grandfather: . . . class father(grandfather): . . . class son(father): . . . • Son inherits the properties of father directly and the properties of the grandfather class indirectly via the father class • The son class can access the public members of the father class, which in turn can access the public members of the grandfather class
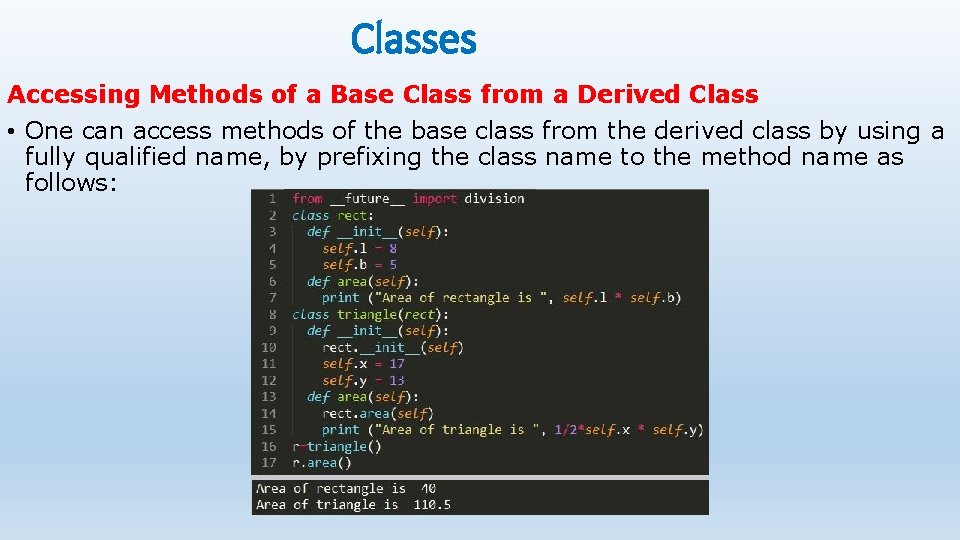
Classes Accessing Methods of a Base Class from a Derived Class • One can access methods of the base class from the derived class by using a fully qualified name, by prefixing the class name to the method name as follows:
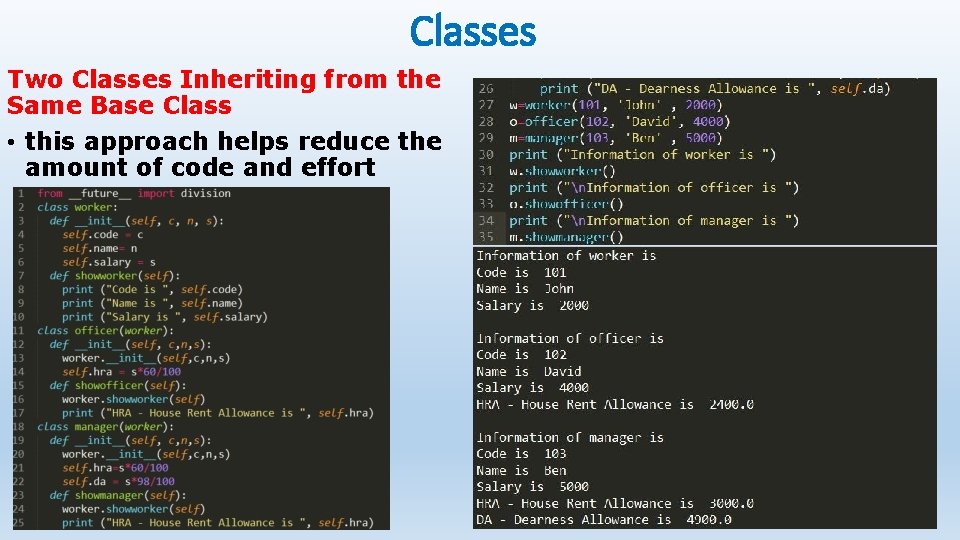
Classes Two Classes Inheriting from the Same Base Class • this approach helps reduce the amount of code and effort
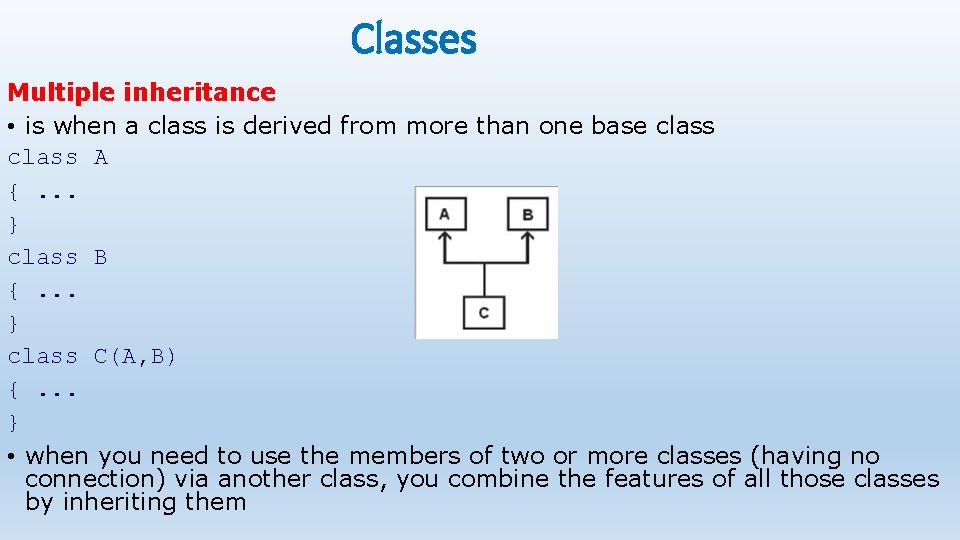
Classes Multiple inheritance • is when a class is derived from more than one base class A {. . . } class B {. . . } class C(A, B) {. . . } • when you need to use the members of two or more classes (having no connection) via another class, you combine the features of all those classes by inheriting them
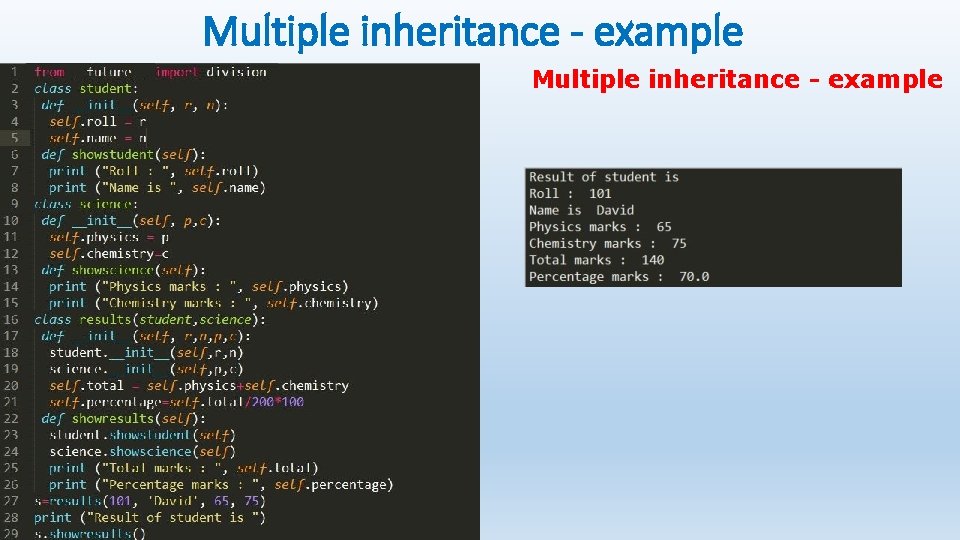
Multiple inheritance - example
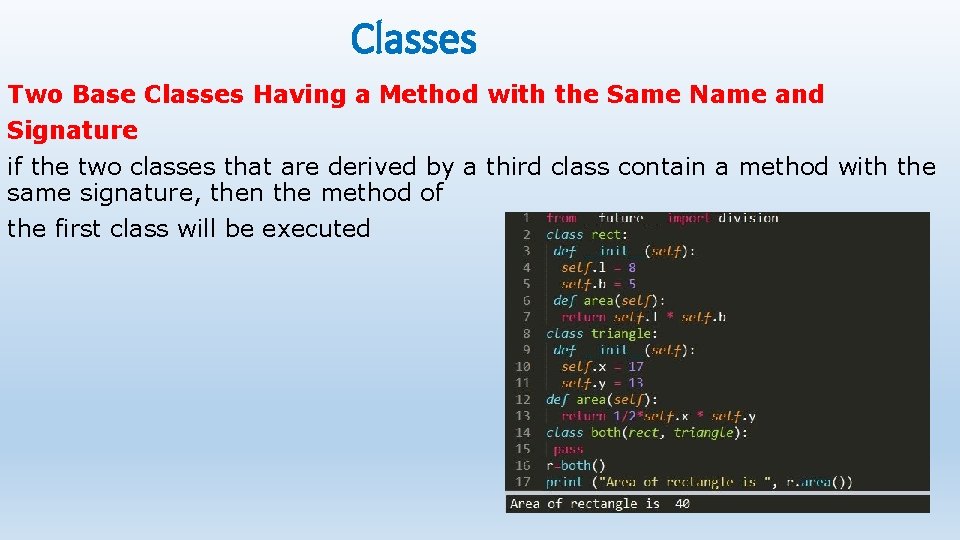
Classes Two Base Classes Having a Method with the Same Name and Signature if the two classes that are derived by a third class contain a method with the same signature, then the method of the first class will be executed
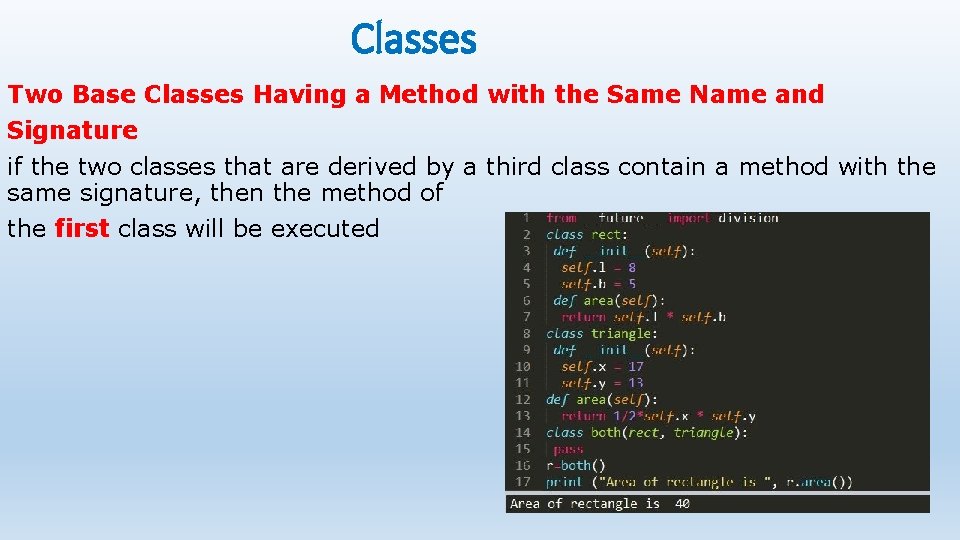
Classes Two Base Classes Having a Method with the Same Name and Signature if the two classes that are derived by a third class contain a method with the same signature, then the method of the first class will be executed
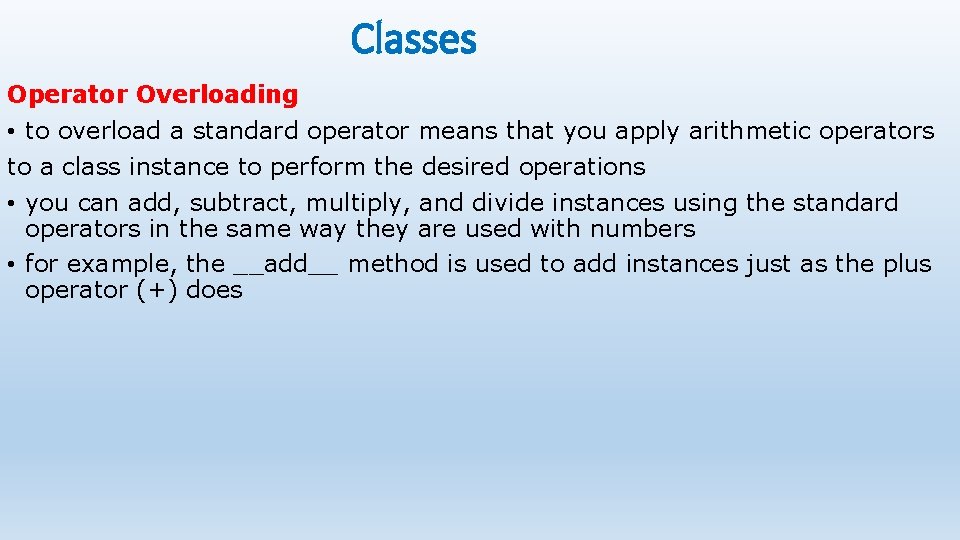
Classes Operator Overloading • to overload a standard operator means that you apply arithmetic operators to a class instance to perform the desired operations • you can add, subtract, multiply, and divide instances using the standard operators in the same way they are used with numbers • for example, the __add__ method is used to add instances just as the plus operator (+) does
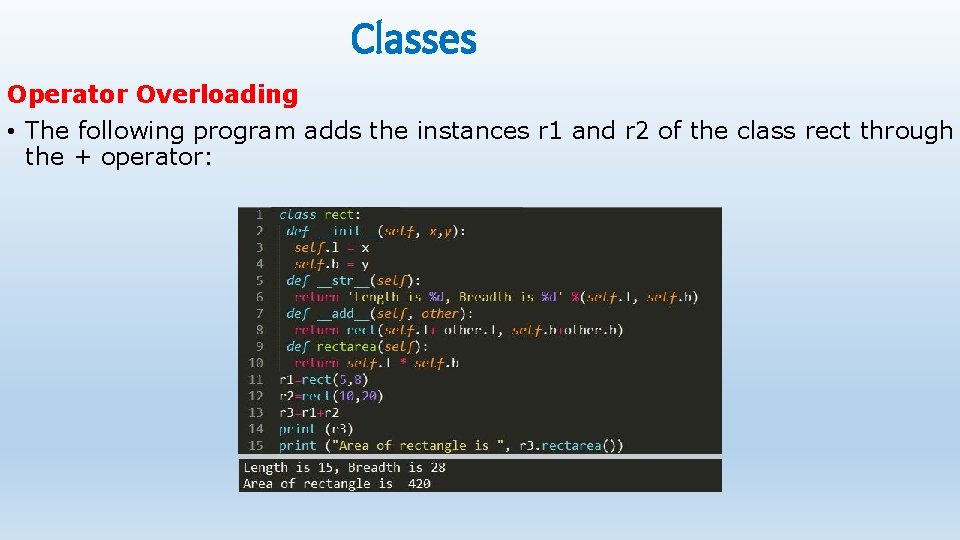
Classes Operator Overloading • The following program adds the instances r 1 and r 2 of the class rect through the + operator:
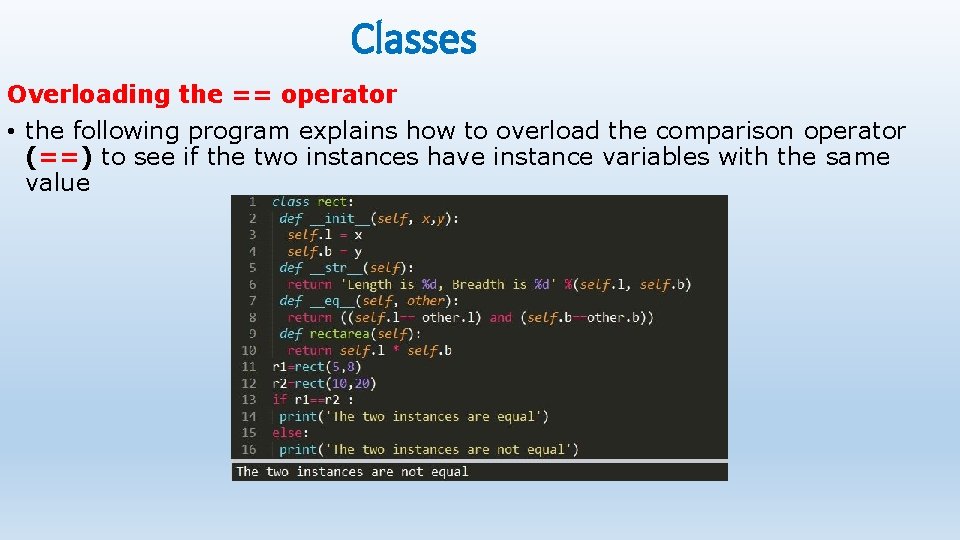
Classes Overloading the == operator • the following program explains how to overload the comparison operator (==) to see if the two instances have instance variables with the same value
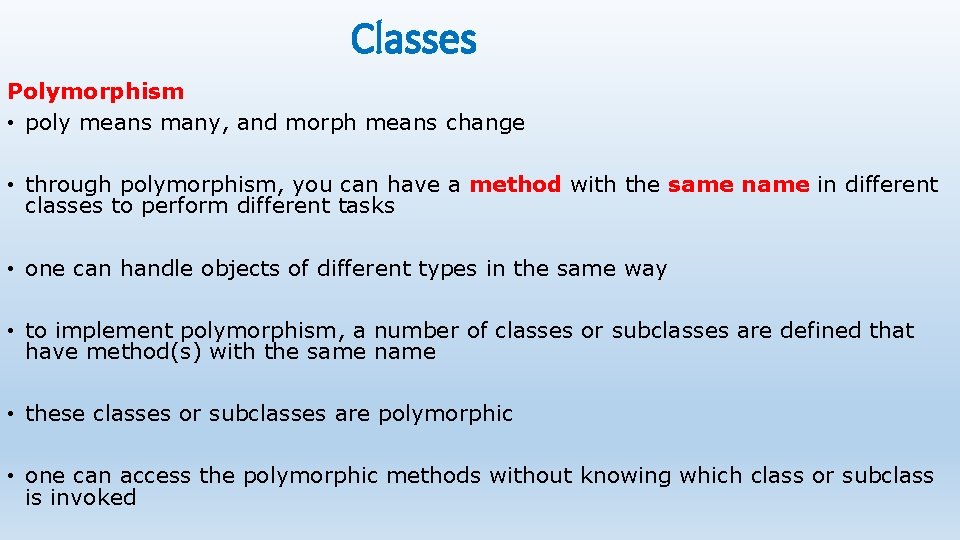
Classes Polymorphism • poly means many, and morph means change • through polymorphism, you can have a method with the same name in different classes to perform different tasks • one can handle objects of different types in the same way • to implement polymorphism, a number of classes or subclasses are defined that have method(s) with the same name • these classes or subclasses are polymorphic • one can access the polymorphic methods without knowing which class or subclass is invoked
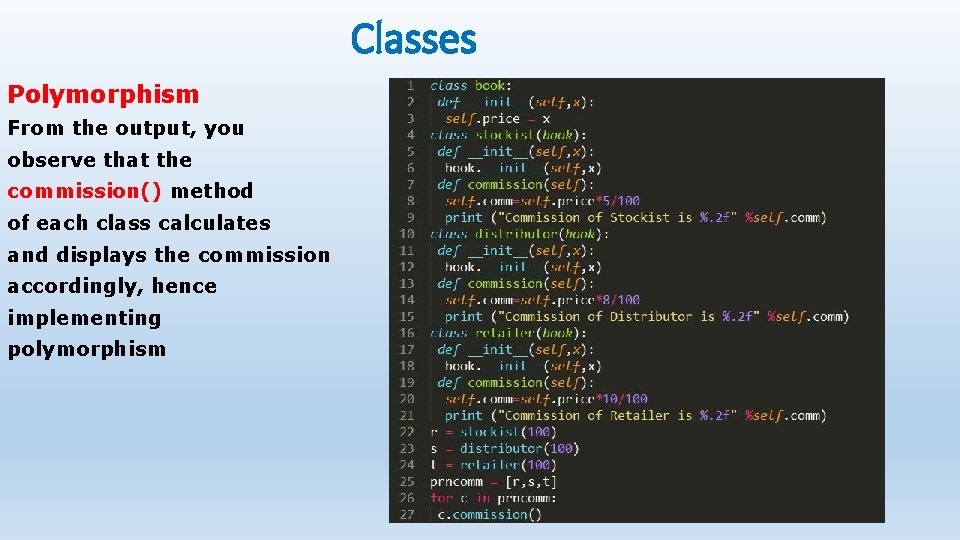
Classes Polymorphism From the output, you observe that the commission() method of each class calculates and displays the commission accordingly, hence implementing polymorphism
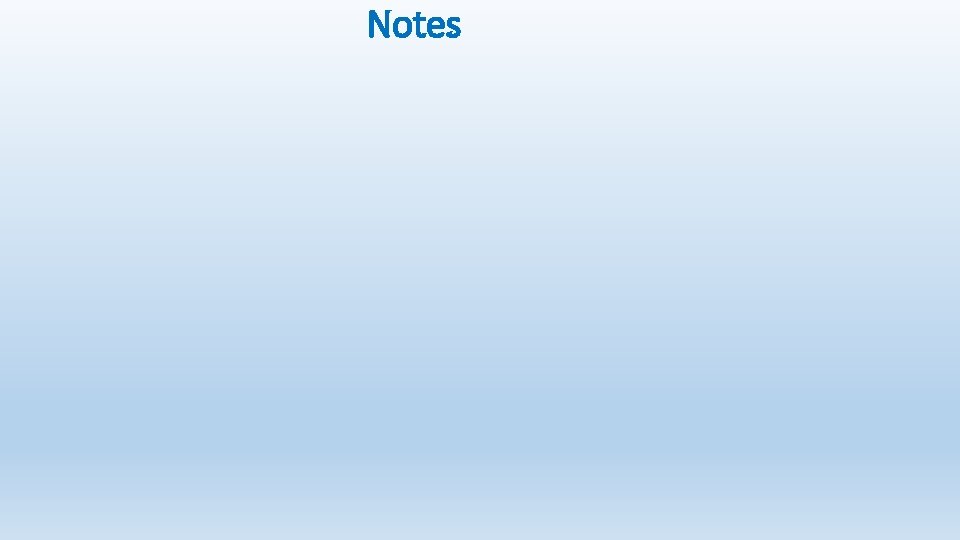
Notes
Este classe e subclasse
Pre ap classes vs regular classes
The five classes of fire
The five classes of fire
And now with gleams of half-extinguished thought
One in five challenge
5 elements and 5 senses
Macbeth act five scene five
Class five english
Why plants and animals depend on each other
Class five science
Verb after would rather
Ualberta remo
Which features of the sun look like huge cloudlike arches
Why does ethanol look like water but behave more like wood?
Like minds like mine
What he looks like or how he looks like
What does he look like
Lied i like the flowers
Time flies like arrow fruit flies like banana
Which is worse, stealing like karl or cheating like bob
Like father like son in italian
Deduce the resultant of two like parallel forces
Todays class
Package mypackage; class first { /* class body */ }
Introduction to ooad
Lower boundary of the modal class
Class i vs class ii mhc
Difference between abstract class and concrete class
How to get class interval
Stimuli vs stimulus
Discrimination training
7 rights of medication administration in order
Class maths student student1 class student string name
What is the class width for the given class (28-33)
In greenfoot, you can cast an actor class to a world class?
Static and dynamic class loading in java
Esd class levels
Uml class diagram static
Class 2 class 3
Extends testcase
Package mypackage class first class body
Class third class