Chapter 7 Queues Introduction Queue applications Implementations Ch
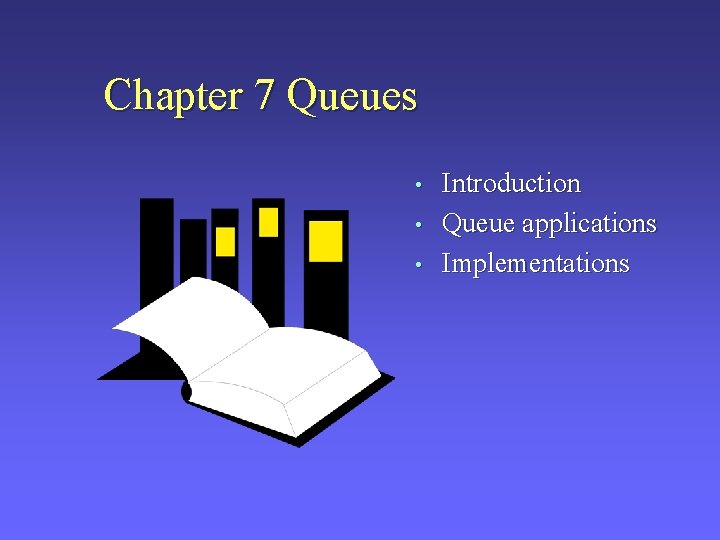
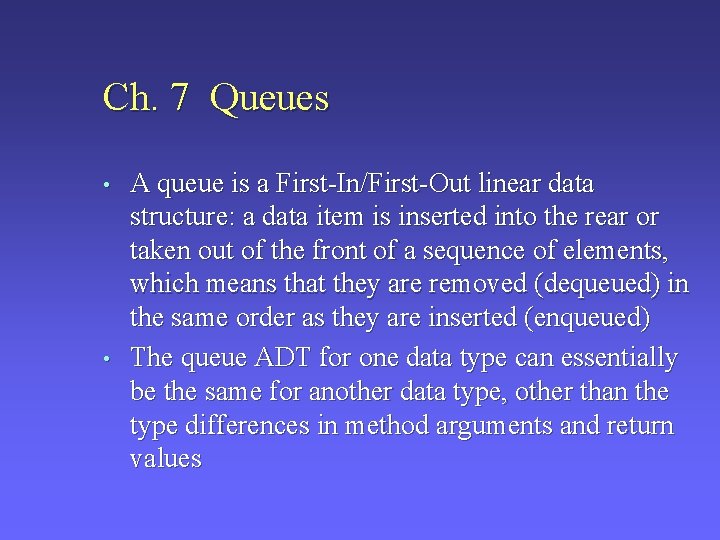
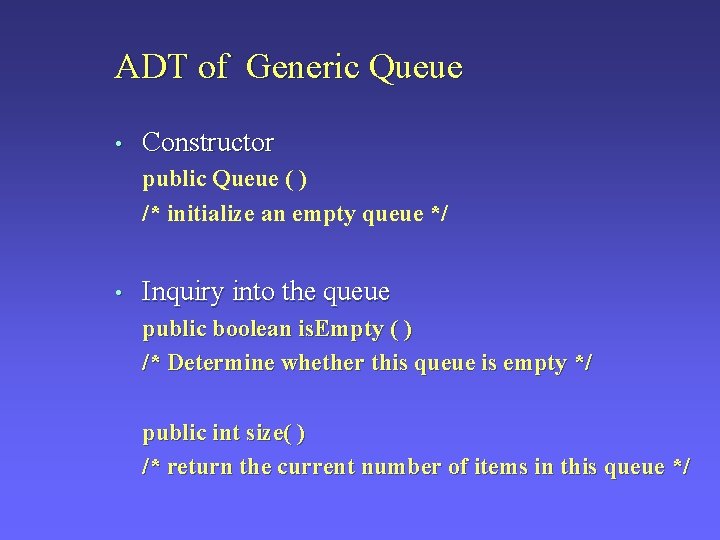
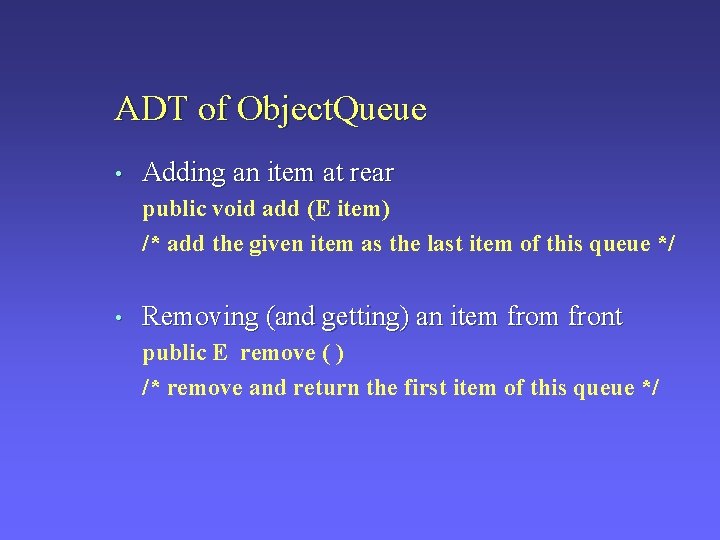
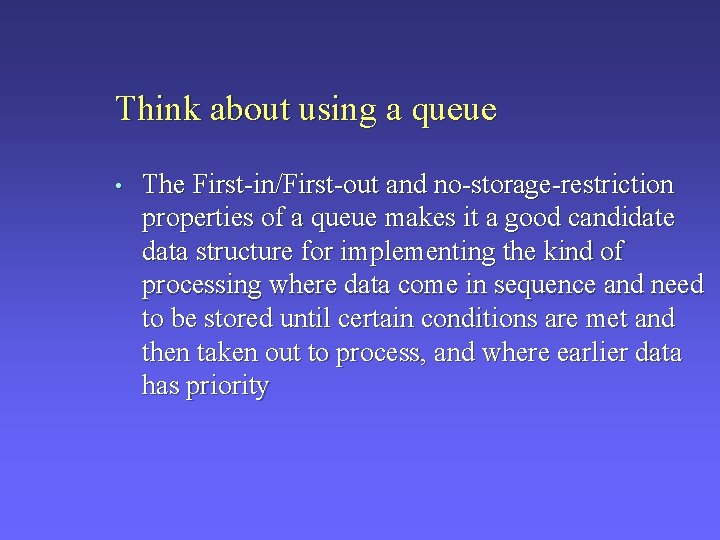
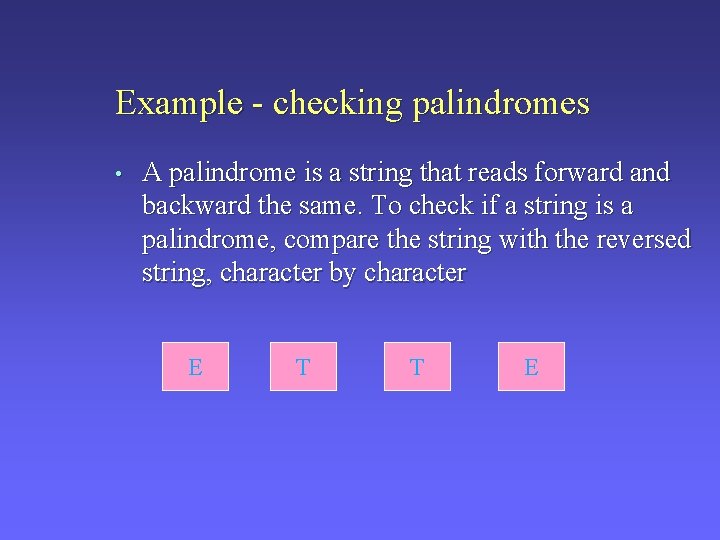
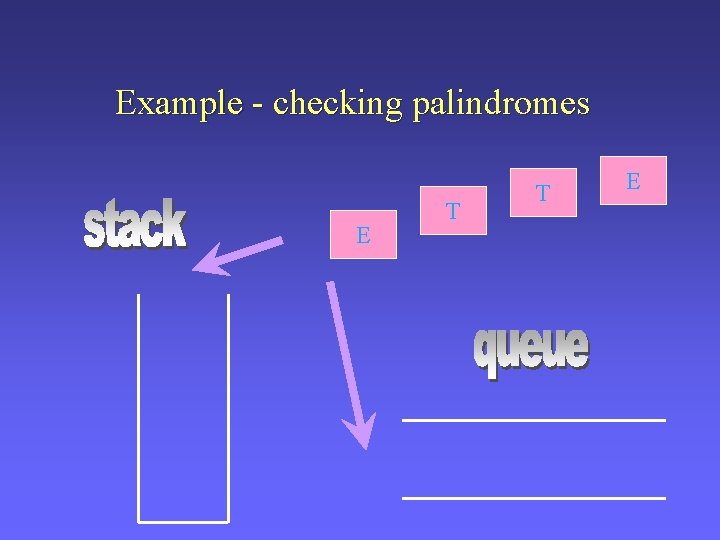
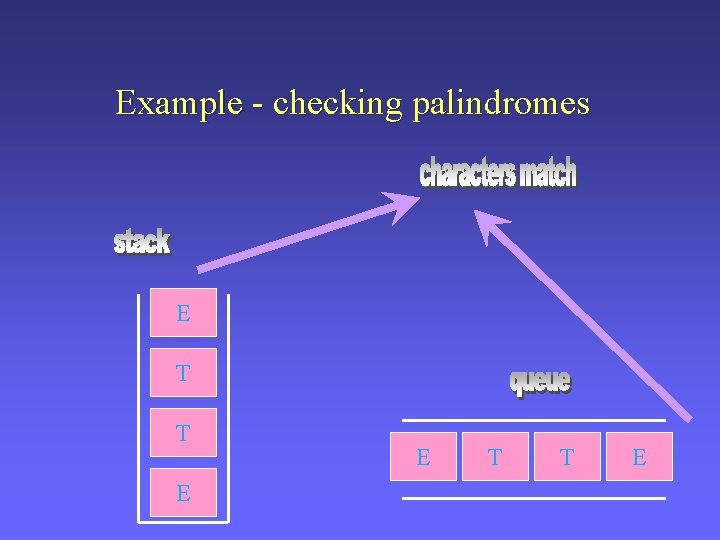
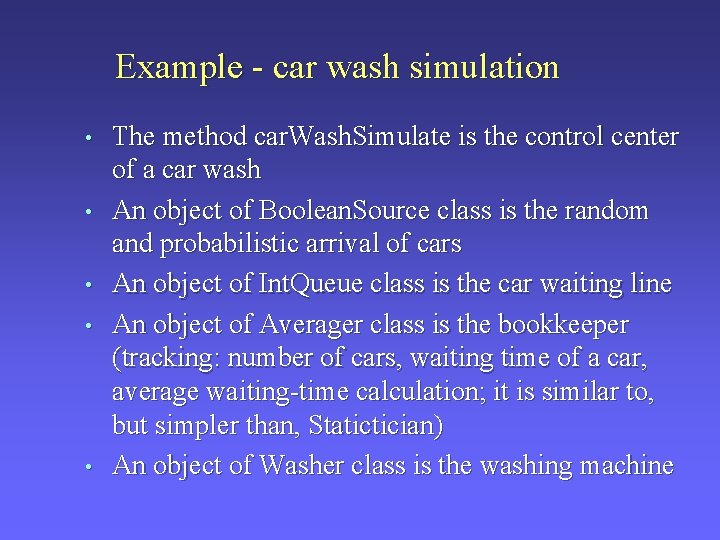
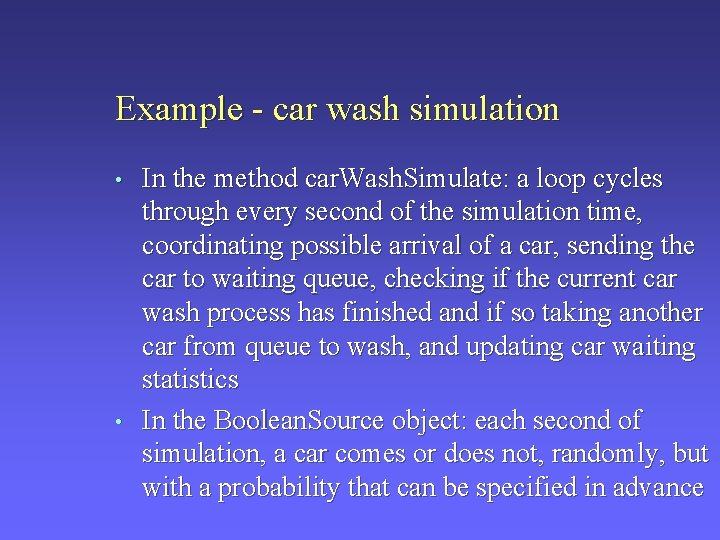
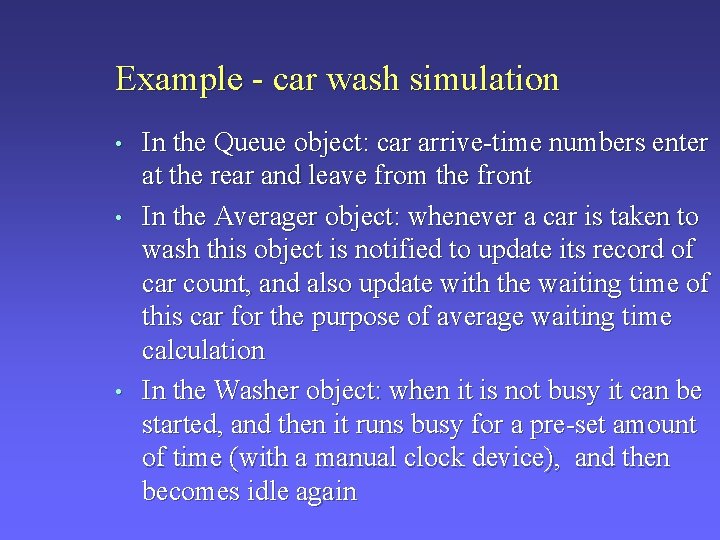
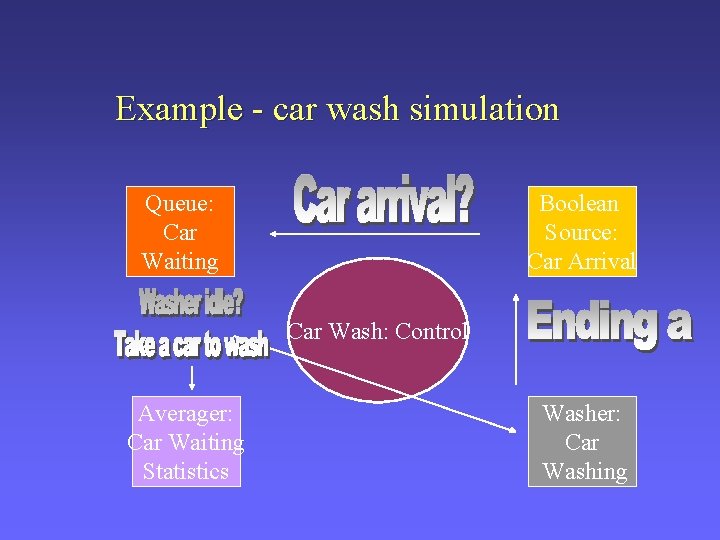
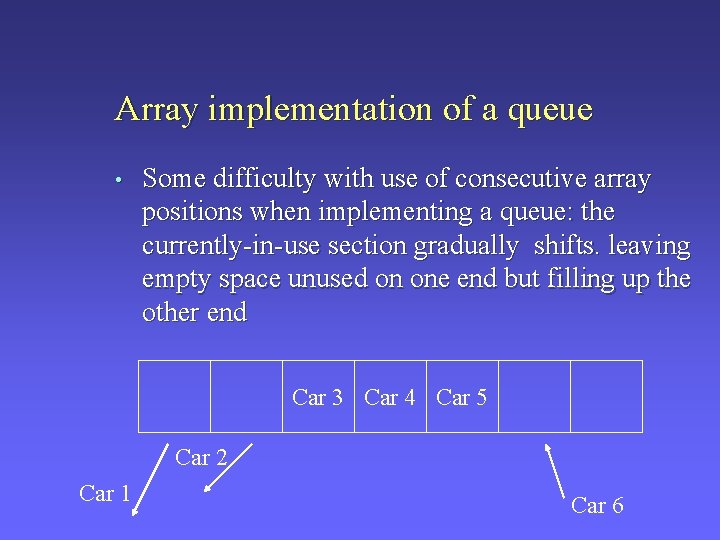
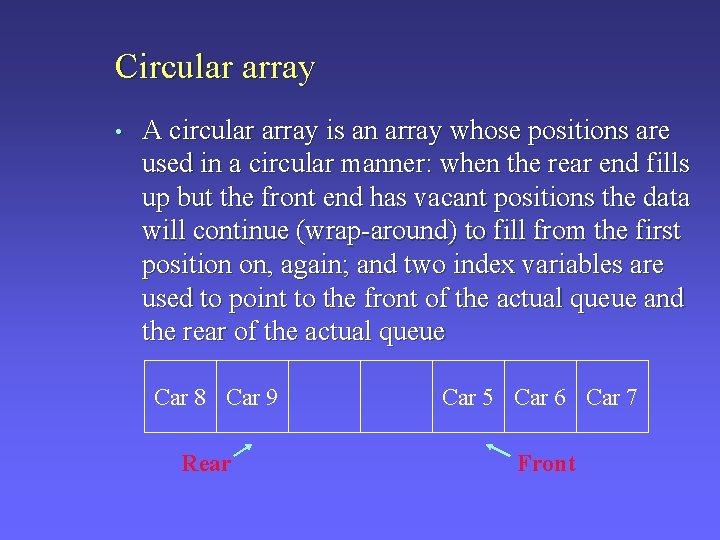
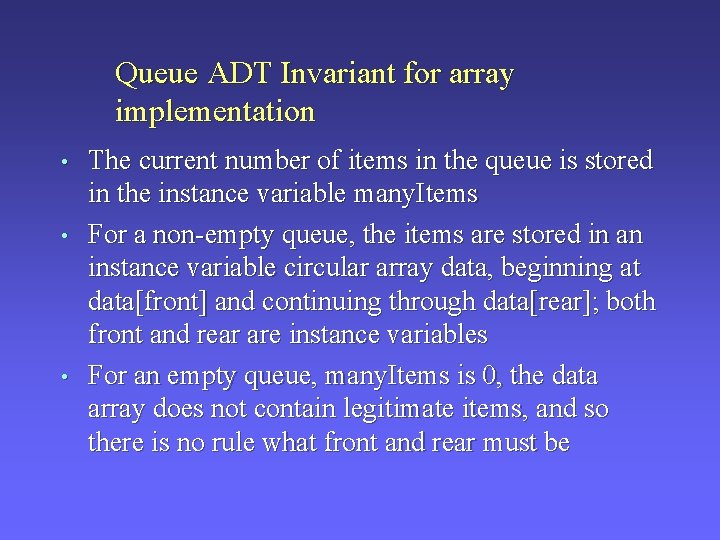
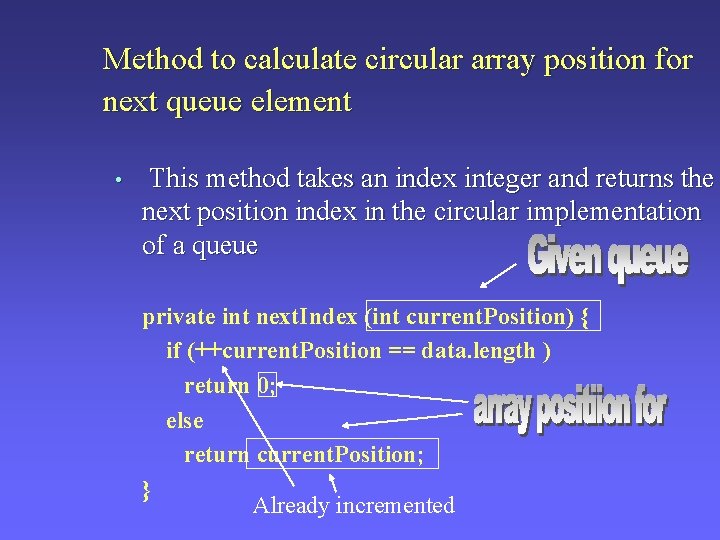
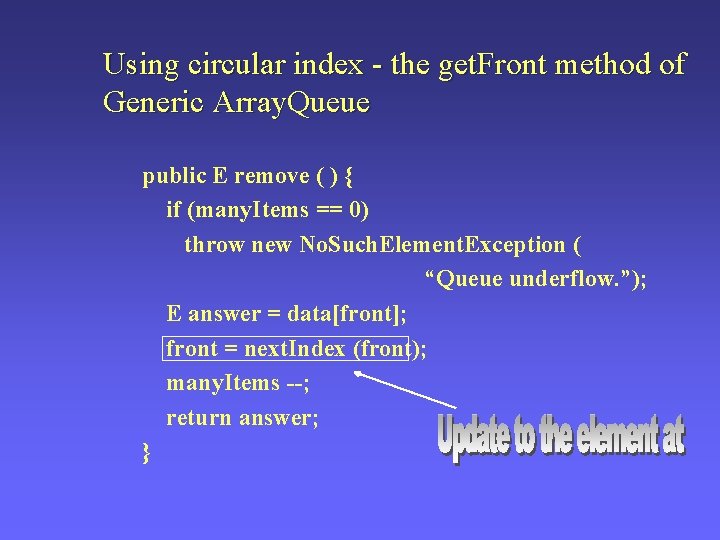
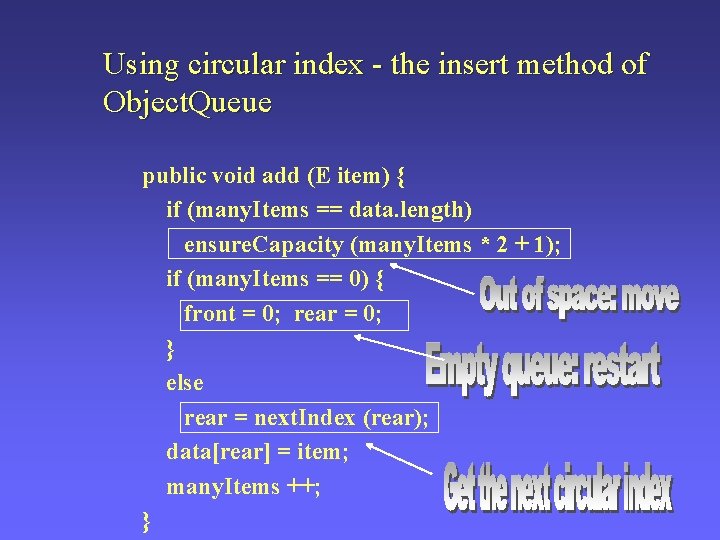
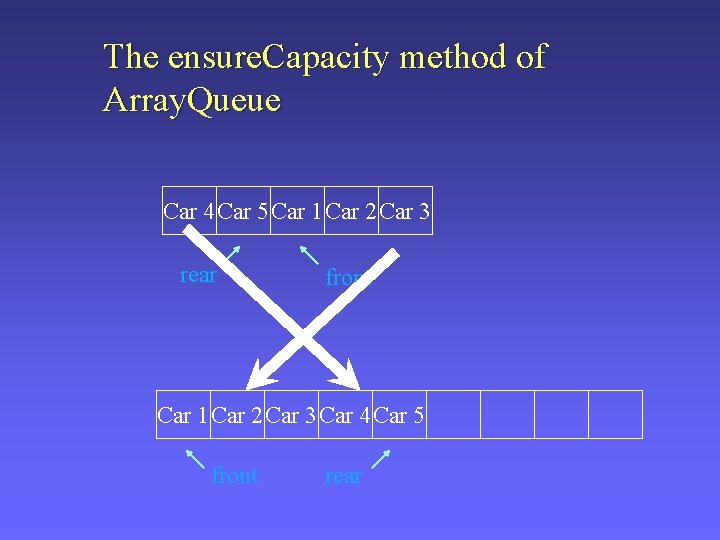
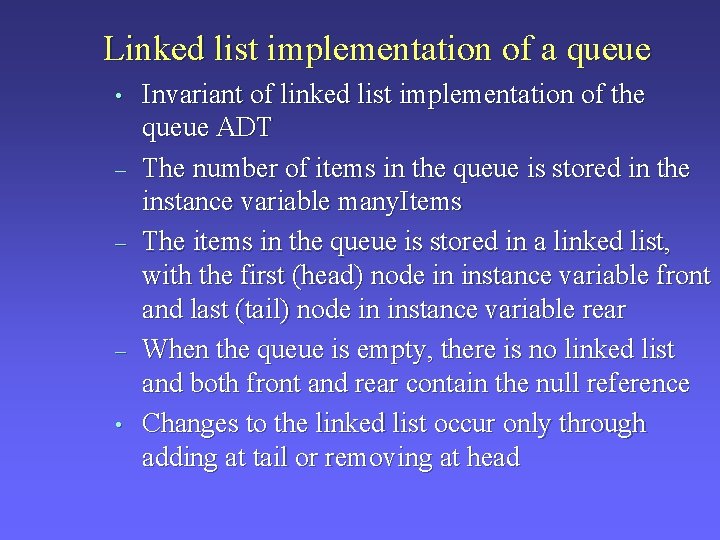
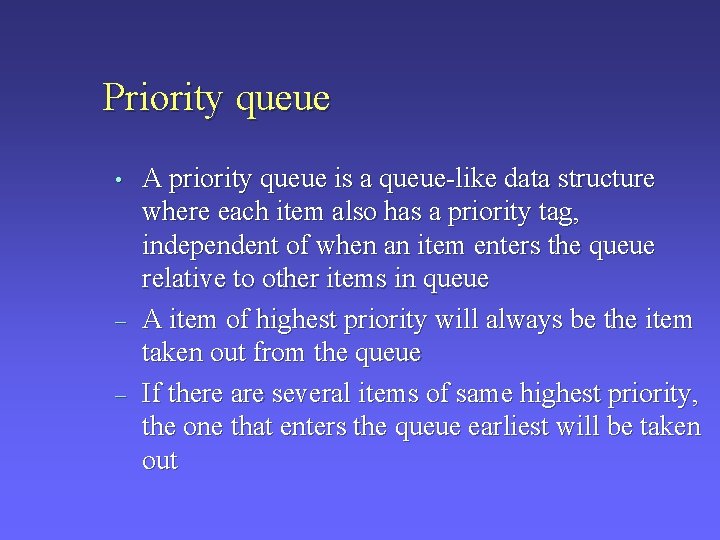
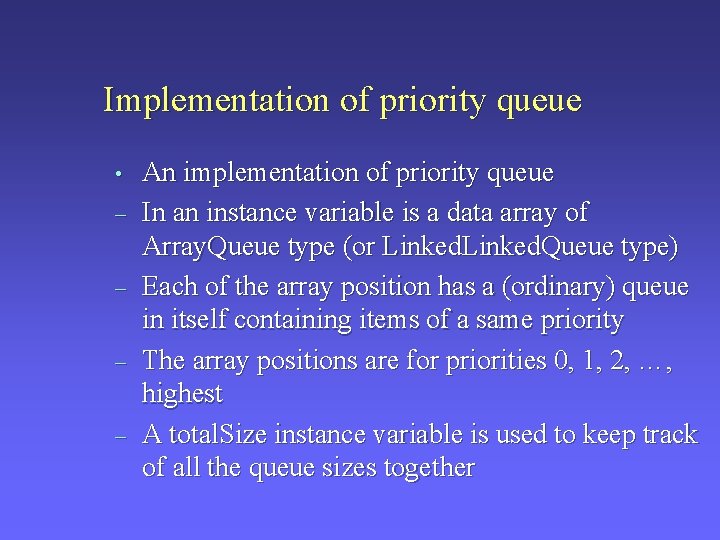
- Slides: 22
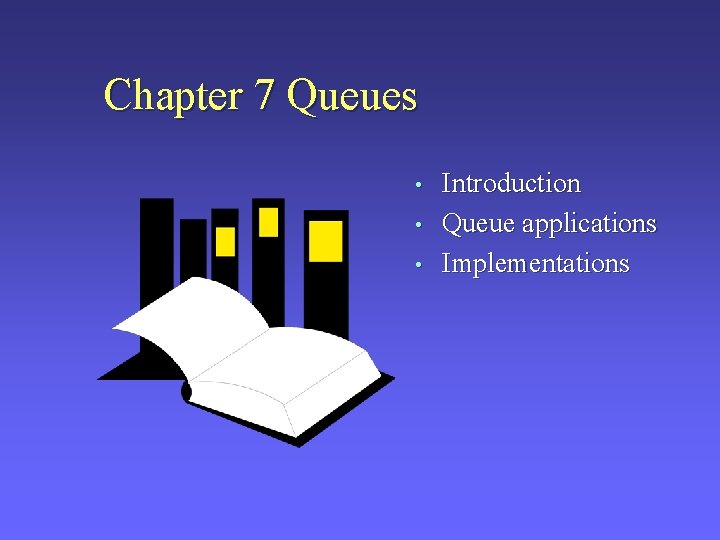
Chapter 7 Queues • • • Introduction Queue applications Implementations
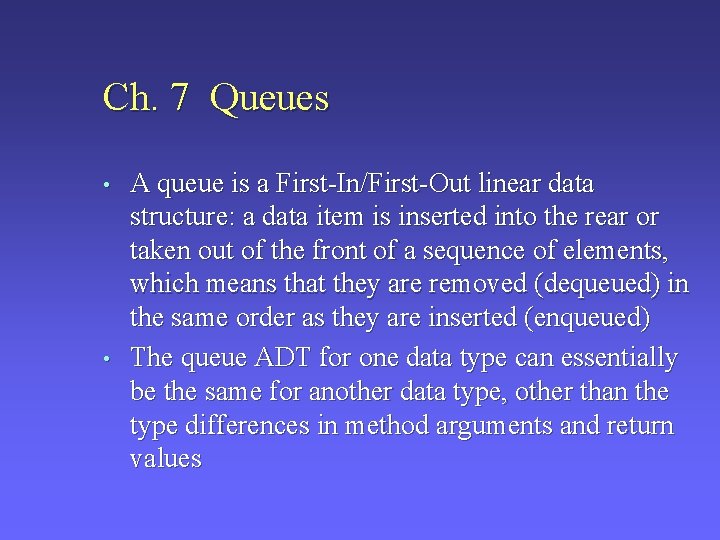
Ch. 7 Queues • • A queue is a First-In/First-Out linear data structure: a data item is inserted into the rear or taken out of the front of a sequence of elements, which means that they are removed (dequeued) in the same order as they are inserted (enqueued) The queue ADT for one data type can essentially be the same for another data type, other than the type differences in method arguments and return values
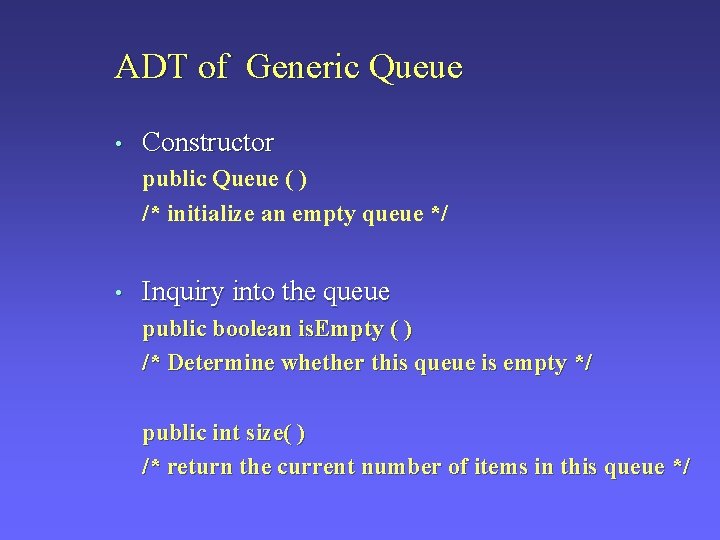
ADT of Generic Queue • Constructor public Queue ( ) /* initialize an empty queue */ • Inquiry into the queue public boolean is. Empty ( ) /* Determine whether this queue is empty */ public int size( ) /* return the current number of items in this queue */
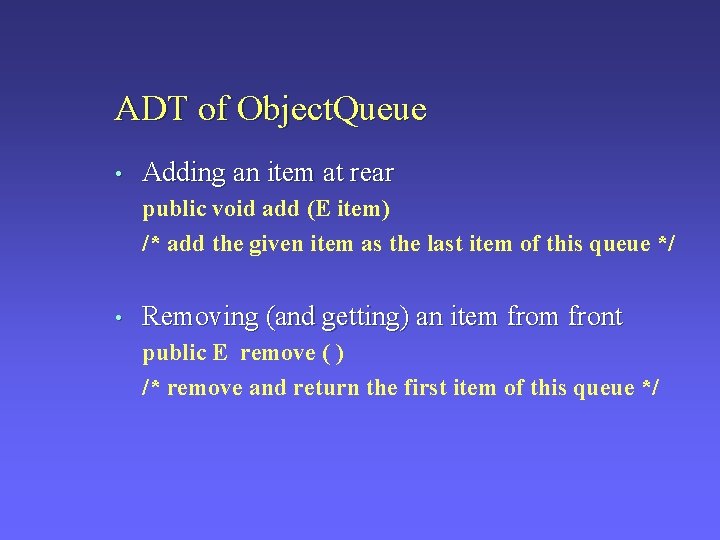
ADT of Object. Queue • Adding an item at rear public void add (E item) /* add the given item as the last item of this queue */ • Removing (and getting) an item front public E remove ( ) /* remove and return the first item of this queue */
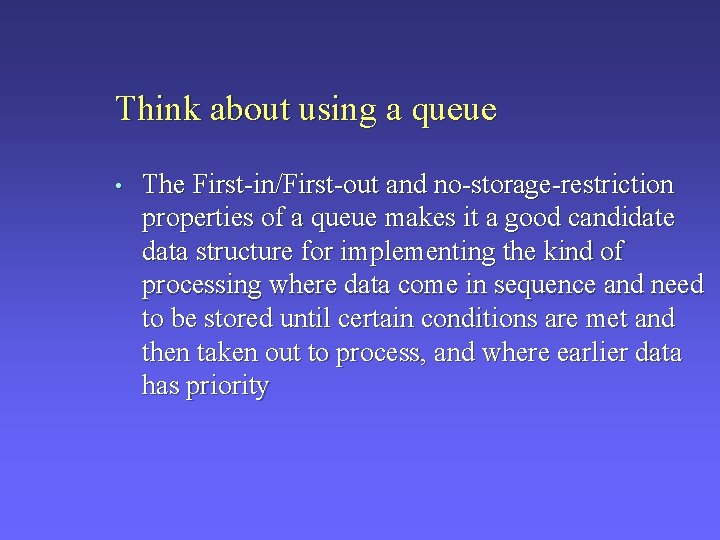
Think about using a queue • The First-in/First-out and no-storage-restriction properties of a queue makes it a good candidate data structure for implementing the kind of processing where data come in sequence and need to be stored until certain conditions are met and then taken out to process, and where earlier data has priority
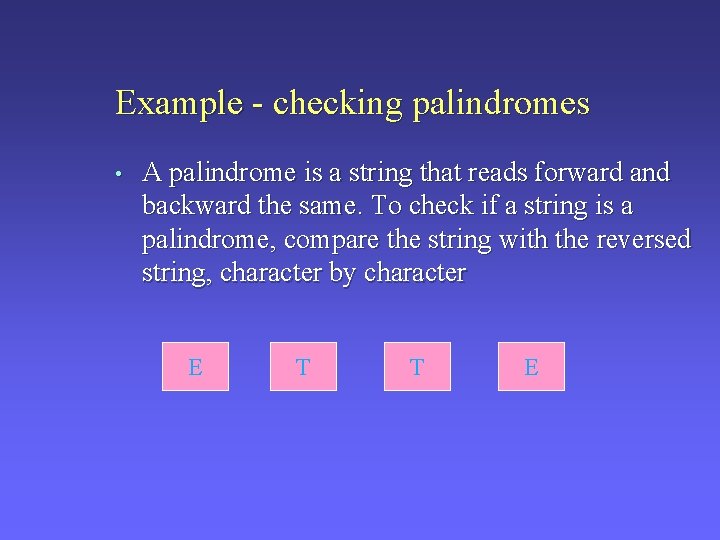
Example - checking palindromes • A palindrome is a string that reads forward and backward the same. To check if a string is a palindrome, compare the string with the reversed string, character by character E T T E
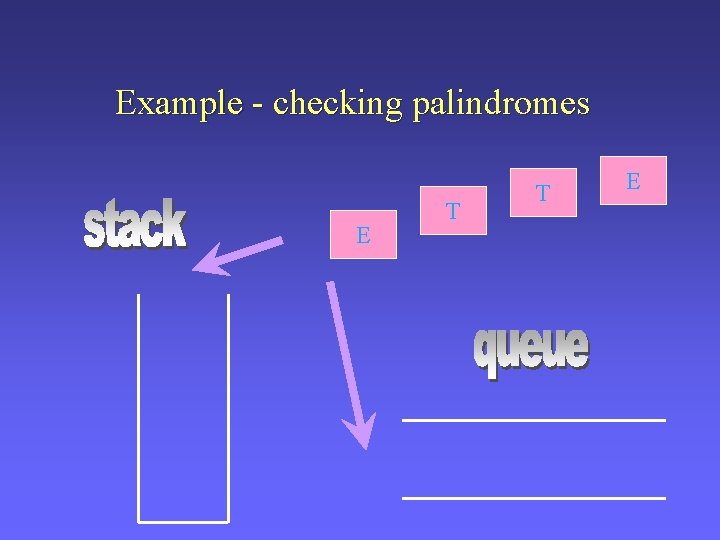
Example - checking palindromes E T T E
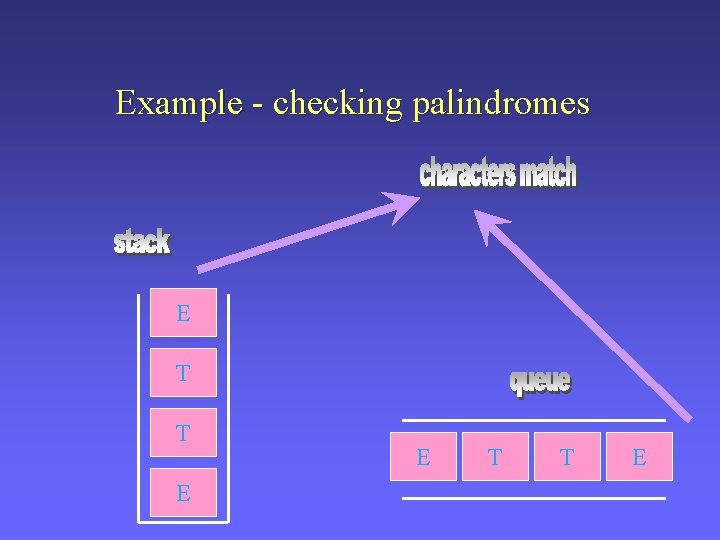
Example - checking palindromes E T T E
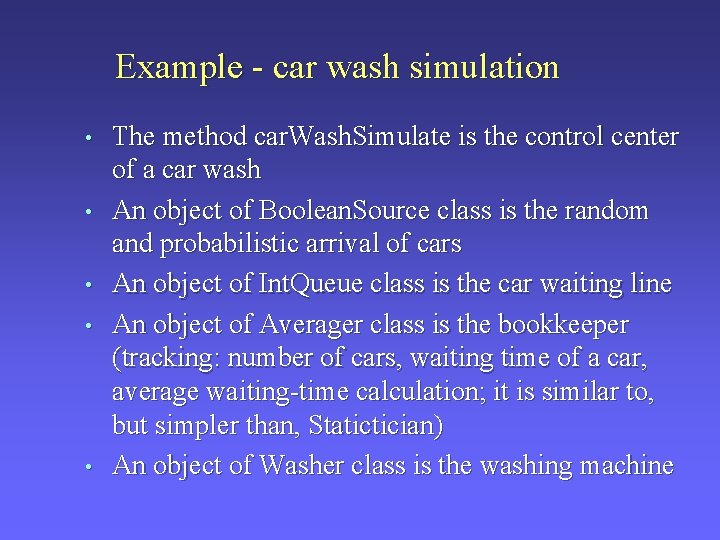
Example - car wash simulation • • • The method car. Wash. Simulate is the control center of a car wash An object of Boolean. Source class is the random and probabilistic arrival of cars An object of Int. Queue class is the car waiting line An object of Averager class is the bookkeeper (tracking: number of cars, waiting time of a car, average waiting-time calculation; it is similar to, but simpler than, Statictician) An object of Washer class is the washing machine
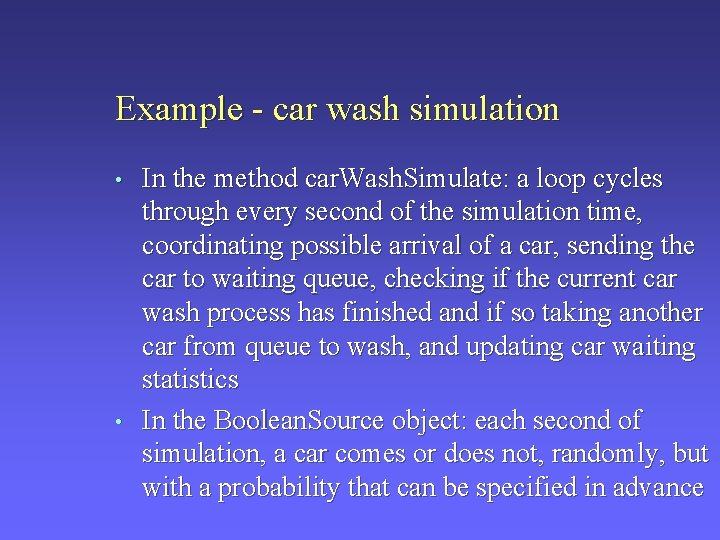
Example - car wash simulation • • In the method car. Wash. Simulate: a loop cycles through every second of the simulation time, coordinating possible arrival of a car, sending the car to waiting queue, checking if the current car wash process has finished and if so taking another car from queue to wash, and updating car waiting statistics In the Boolean. Source object: each second of simulation, a car comes or does not, randomly, but with a probability that can be specified in advance
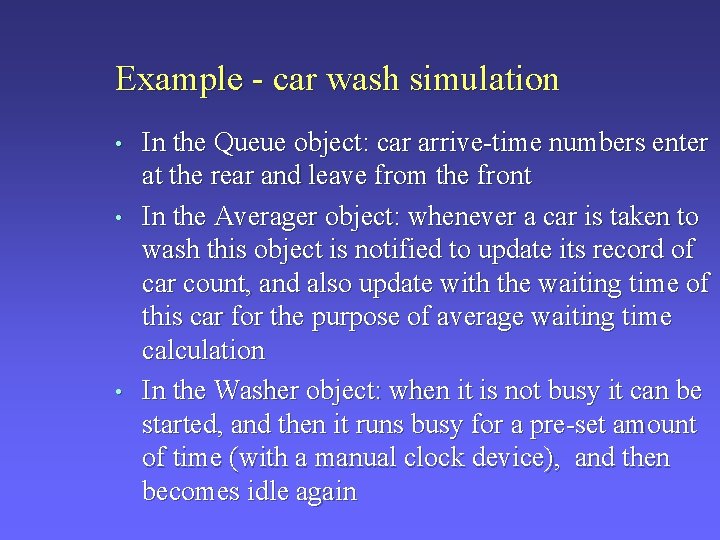
Example - car wash simulation • • • In the Queue object: car arrive-time numbers enter at the rear and leave from the front In the Averager object: whenever a car is taken to wash this object is notified to update its record of car count, and also update with the waiting time of this car for the purpose of average waiting time calculation In the Washer object: when it is not busy it can be started, and then it runs busy for a pre-set amount of time (with a manual clock device), and then becomes idle again
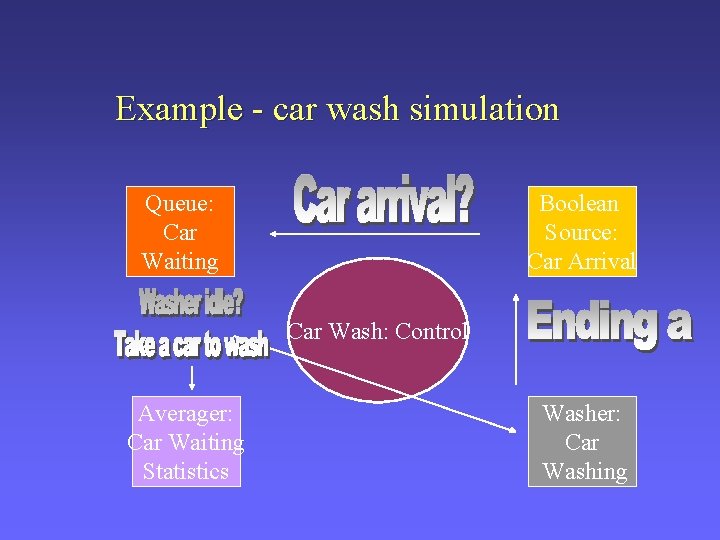
Example - car wash simulation Queue: Car Waiting Boolean Source: Car Arrival Car Wash: Control Averager: Car Waiting Statistics Washer: Car Washing
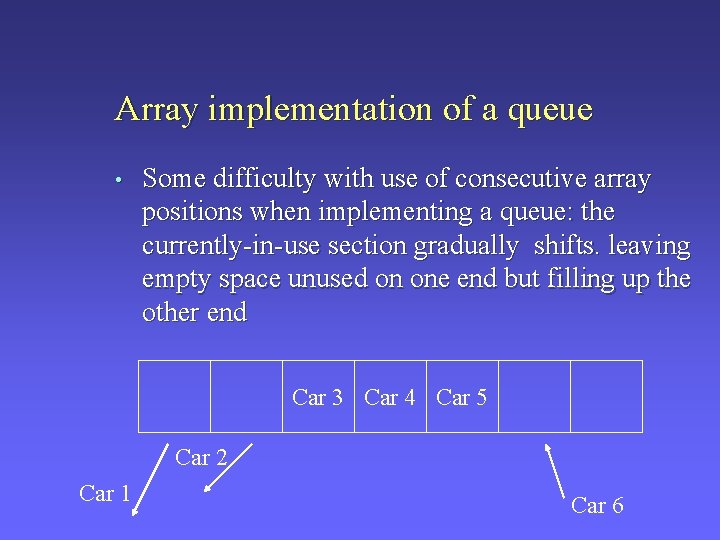
Array implementation of a queue • Some difficulty with use of consecutive array positions when implementing a queue: the currently-in-use section gradually shifts. leaving empty space unused on one end but filling up the other end Car 3 Car 4 Car 5 Car 2 Car 1 Car 6
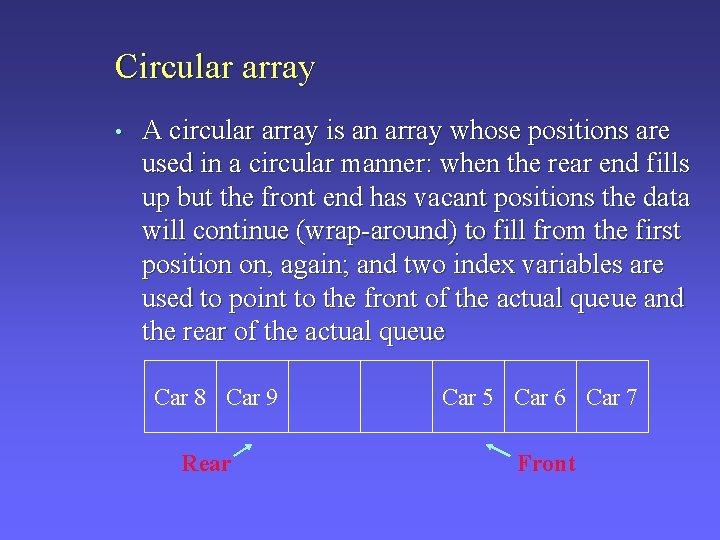
Circular array • A circular array is an array whose positions are used in a circular manner: when the rear end fills up but the front end has vacant positions the data will continue (wrap-around) to fill from the first position on, again; and two index variables are used to point to the front of the actual queue and the rear of the actual queue Car 8 Car 9 Rear Car 5 Car 6 Car 7 Front
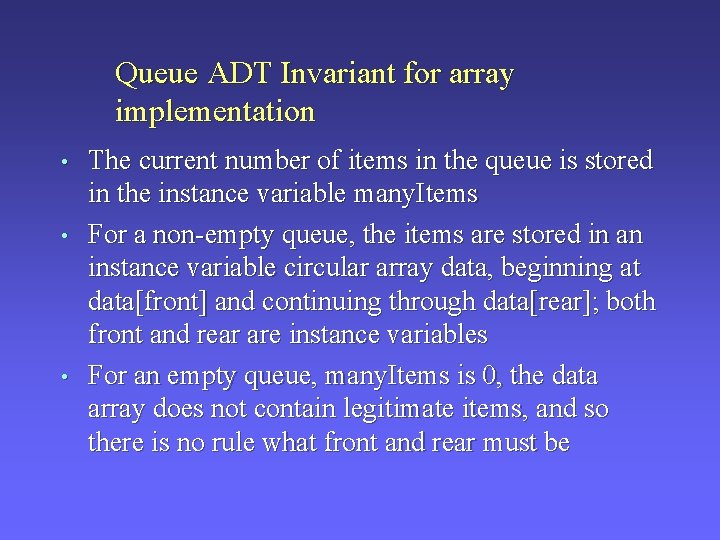
Queue ADT Invariant for array implementation • • • The current number of items in the queue is stored in the instance variable many. Items For a non-empty queue, the items are stored in an instance variable circular array data, beginning at data[front] and continuing through data[rear]; both front and rear are instance variables For an empty queue, many. Items is 0, the data array does not contain legitimate items, and so there is no rule what front and rear must be
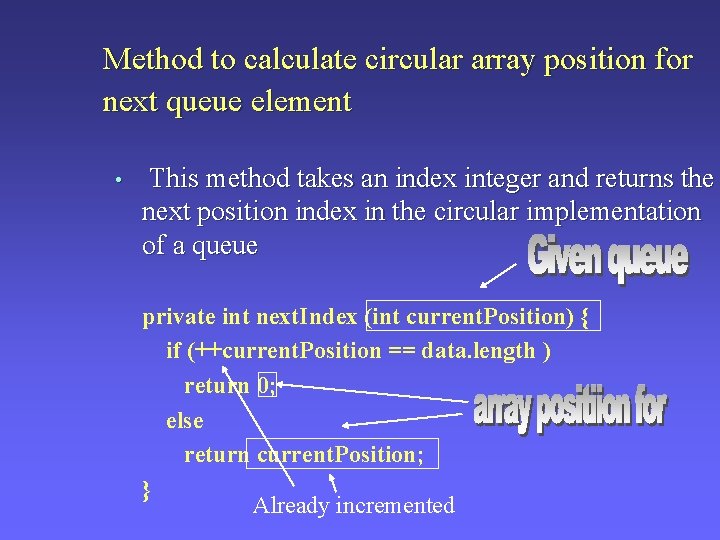
Method to calculate circular array position for next queue element • This method takes an index integer and returns the next position index in the circular implementation of a queue private int next. Index (int current. Position) { if (++current. Position == data. length ) return 0; else return current. Position; } Already incremented
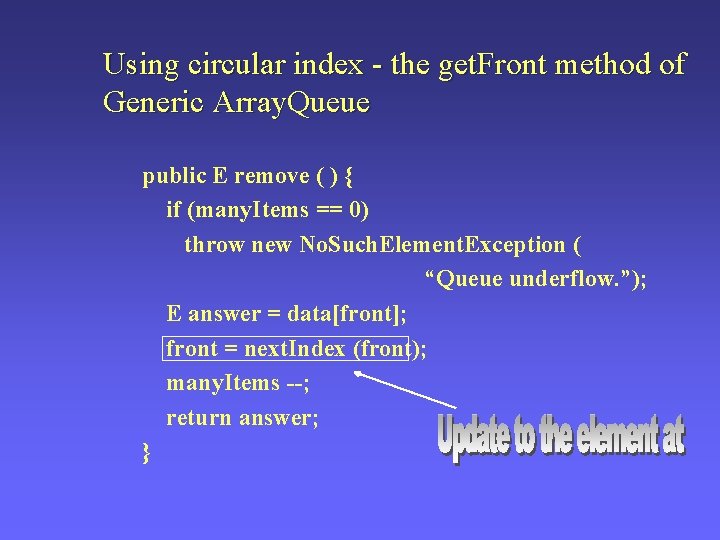
Using circular index - the get. Front method of Generic Array. Queue public E remove ( ) { if (many. Items == 0) throw new No. Such. Element. Exception ( “Queue underflow. ”); E answer = data[front]; front = next. Index (front); many. Items --; return answer; }
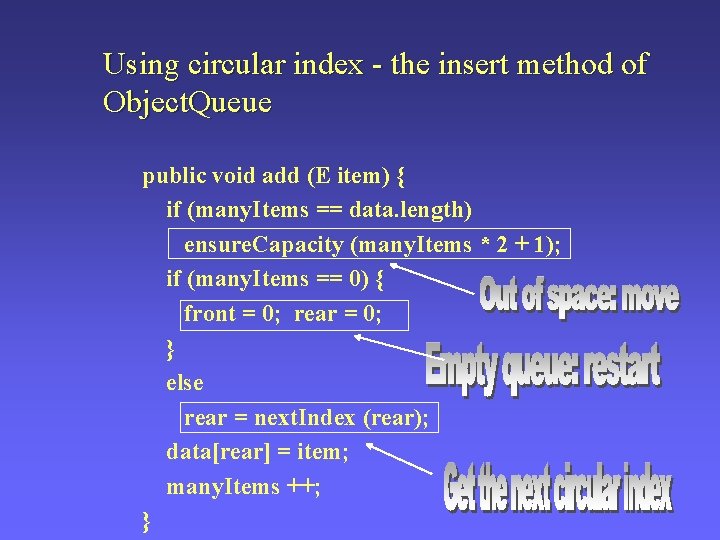
Using circular index - the insert method of Object. Queue public void add (E item) { if (many. Items == data. length) ensure. Capacity (many. Items * 2 + 1); if (many. Items == 0) { front = 0; rear = 0; } else rear = next. Index (rear); data[rear] = item; many. Items ++; }
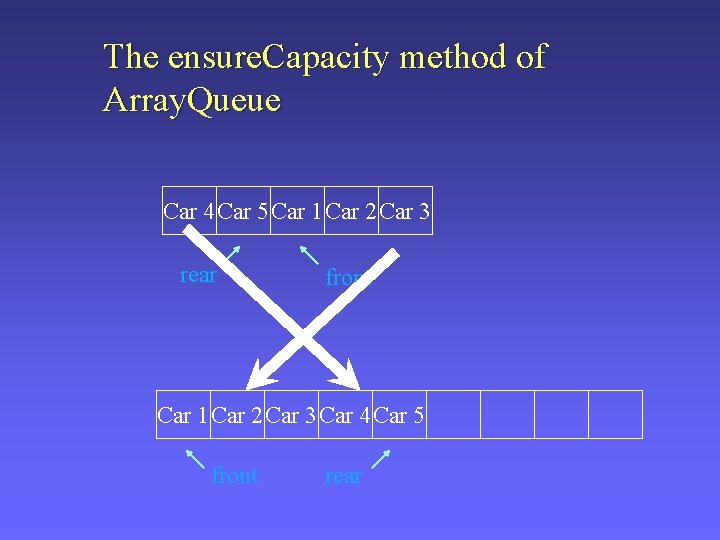
The ensure. Capacity method of Array. Queue Car 4 Car 5 Car 1 Car 2 Car 3 rear front Car 1 Car 2 Car 3 Car 4 Car 5 front rear
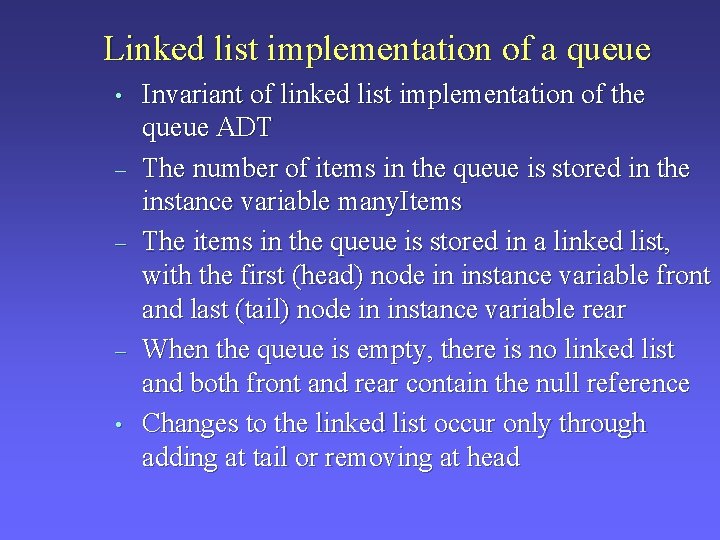
Linked list implementation of a queue • – – – • Invariant of linked list implementation of the queue ADT The number of items in the queue is stored in the instance variable many. Items The items in the queue is stored in a linked list, with the first (head) node in instance variable front and last (tail) node in instance variable rear When the queue is empty, there is no linked list and both front and rear contain the null reference Changes to the linked list occur only through adding at tail or removing at head
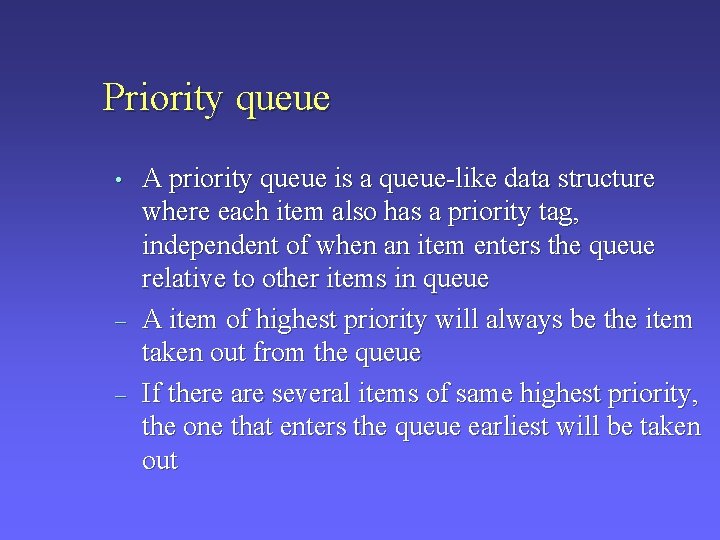
Priority queue • – – A priority queue is a queue-like data structure where each item also has a priority tag, independent of when an item enters the queue relative to other items in queue A item of highest priority will always be the item taken out from the queue If there are several items of same highest priority, the one that enters the queue earliest will be taken out
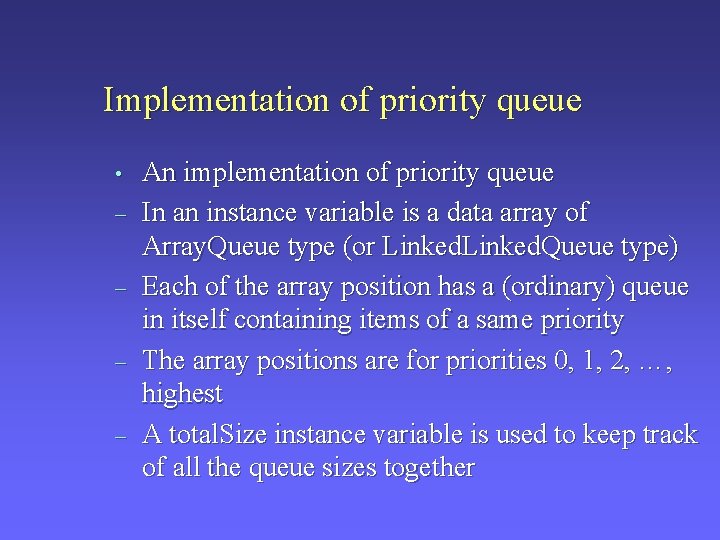
Implementation of priority queue • – – An implementation of priority queue In an instance variable is a data array of Array. Queue type (or Linked. Queue type) Each of the array position has a (ordinary) queue in itself containing items of a same priority The array positions are for priorities 0, 1, 2, …, highest A total. Size instance variable is used to keep track of all the queue sizes together